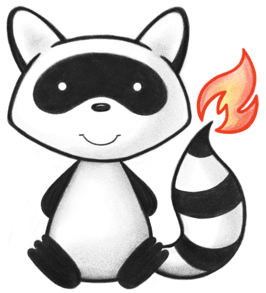
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Represents a defined collection of entities that may be discussed or acted 048 * upon collectively but which are not expected to act collectively, and are not 049 * formally or legally recognized; i.e. a collection of entities that isn't an 050 * Organization. 051 */ 052@ResourceDef(name = "Group", profile = "http://hl7.org/fhir/StructureDefinition/Group") 053public class Group extends DomainResource { 054 055 public enum GroupType { 056 /** 057 * Group contains "person" Patient resources. 058 */ 059 PERSON, 060 /** 061 * Group contains "animal" Patient resources. 062 */ 063 ANIMAL, 064 /** 065 * Group contains healthcare practitioner resources (Practitioner or 066 * PractitionerRole). 067 */ 068 PRACTITIONER, 069 /** 070 * Group contains Device resources. 071 */ 072 DEVICE, 073 /** 074 * Group contains Medication resources. 075 */ 076 MEDICATION, 077 /** 078 * Group contains Substance resources. 079 */ 080 SUBSTANCE, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static GroupType fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("person".equals(codeString)) 090 return PERSON; 091 if ("animal".equals(codeString)) 092 return ANIMAL; 093 if ("practitioner".equals(codeString)) 094 return PRACTITIONER; 095 if ("device".equals(codeString)) 096 return DEVICE; 097 if ("medication".equals(codeString)) 098 return MEDICATION; 099 if ("substance".equals(codeString)) 100 return SUBSTANCE; 101 if (Configuration.isAcceptInvalidEnums()) 102 return null; 103 else 104 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case PERSON: 110 return "person"; 111 case ANIMAL: 112 return "animal"; 113 case PRACTITIONER: 114 return "practitioner"; 115 case DEVICE: 116 return "device"; 117 case MEDICATION: 118 return "medication"; 119 case SUBSTANCE: 120 return "substance"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getSystem() { 129 switch (this) { 130 case PERSON: 131 return "http://hl7.org/fhir/group-type"; 132 case ANIMAL: 133 return "http://hl7.org/fhir/group-type"; 134 case PRACTITIONER: 135 return "http://hl7.org/fhir/group-type"; 136 case DEVICE: 137 return "http://hl7.org/fhir/group-type"; 138 case MEDICATION: 139 return "http://hl7.org/fhir/group-type"; 140 case SUBSTANCE: 141 return "http://hl7.org/fhir/group-type"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case PERSON: 152 return "Group contains \"person\" Patient resources."; 153 case ANIMAL: 154 return "Group contains \"animal\" Patient resources."; 155 case PRACTITIONER: 156 return "Group contains healthcare practitioner resources (Practitioner or PractitionerRole)."; 157 case DEVICE: 158 return "Group contains Device resources."; 159 case MEDICATION: 160 return "Group contains Medication resources."; 161 case SUBSTANCE: 162 return "Group contains Substance resources."; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getDisplay() { 171 switch (this) { 172 case PERSON: 173 return "Person"; 174 case ANIMAL: 175 return "Animal"; 176 case PRACTITIONER: 177 return "Practitioner"; 178 case DEVICE: 179 return "Device"; 180 case MEDICATION: 181 return "Medication"; 182 case SUBSTANCE: 183 return "Substance"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 } 191 192 public static class GroupTypeEnumFactory implements EnumFactory<GroupType> { 193 public GroupType fromCode(String codeString) throws IllegalArgumentException { 194 if (codeString == null || "".equals(codeString)) 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("person".equals(codeString)) 198 return GroupType.PERSON; 199 if ("animal".equals(codeString)) 200 return GroupType.ANIMAL; 201 if ("practitioner".equals(codeString)) 202 return GroupType.PRACTITIONER; 203 if ("device".equals(codeString)) 204 return GroupType.DEVICE; 205 if ("medication".equals(codeString)) 206 return GroupType.MEDICATION; 207 if ("substance".equals(codeString)) 208 return GroupType.SUBSTANCE; 209 throw new IllegalArgumentException("Unknown GroupType code '" + codeString + "'"); 210 } 211 212 public Enumeration<GroupType> fromType(PrimitiveType<?> code) throws FHIRException { 213 if (code == null) 214 return null; 215 if (code.isEmpty()) 216 return new Enumeration<GroupType>(this, GroupType.NULL, code); 217 String codeString = code.asStringValue(); 218 if (codeString == null || "".equals(codeString)) 219 return new Enumeration<GroupType>(this, GroupType.NULL, code); 220 if ("person".equals(codeString)) 221 return new Enumeration<GroupType>(this, GroupType.PERSON, code); 222 if ("animal".equals(codeString)) 223 return new Enumeration<GroupType>(this, GroupType.ANIMAL, code); 224 if ("practitioner".equals(codeString)) 225 return new Enumeration<GroupType>(this, GroupType.PRACTITIONER, code); 226 if ("device".equals(codeString)) 227 return new Enumeration<GroupType>(this, GroupType.DEVICE, code); 228 if ("medication".equals(codeString)) 229 return new Enumeration<GroupType>(this, GroupType.MEDICATION, code); 230 if ("substance".equals(codeString)) 231 return new Enumeration<GroupType>(this, GroupType.SUBSTANCE, code); 232 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 233 } 234 235 public String toCode(GroupType code) { 236 if (code == GroupType.PERSON) 237 return "person"; 238 if (code == GroupType.ANIMAL) 239 return "animal"; 240 if (code == GroupType.PRACTITIONER) 241 return "practitioner"; 242 if (code == GroupType.DEVICE) 243 return "device"; 244 if (code == GroupType.MEDICATION) 245 return "medication"; 246 if (code == GroupType.SUBSTANCE) 247 return "substance"; 248 return "?"; 249 } 250 251 public String toSystem(GroupType code) { 252 return code.getSystem(); 253 } 254 } 255 256 @Block() 257 public static class GroupCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 258 /** 259 * A code that identifies the kind of trait being asserted. 260 */ 261 @Child(name = "code", type = { 262 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 263 @Description(shortDefinition = "Kind of characteristic", formalDefinition = "A code that identifies the kind of trait being asserted.") 264 protected CodeableConcept code; 265 266 /** 267 * The value of the trait that holds (or does not hold - see 'exclude') for 268 * members of the group. 269 */ 270 @Child(name = "value", type = { CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, 271 Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 272 @Description(shortDefinition = "Value held by characteristic", formalDefinition = "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.") 273 protected Type value; 274 275 /** 276 * If true, indicates the characteristic is one that is NOT held by members of 277 * the group. 278 */ 279 @Child(name = "exclude", type = { 280 BooleanType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Group includes or excludes", formalDefinition = "If true, indicates the characteristic is one that is NOT held by members of the group.") 282 protected BooleanType exclude; 283 284 /** 285 * The period over which the characteristic is tested; e.g. the patient had an 286 * operation during the month of June. 287 */ 288 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "Period over which characteristic is tested", formalDefinition = "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.") 290 protected Period period; 291 292 private static final long serialVersionUID = -1000688967L; 293 294 /** 295 * Constructor 296 */ 297 public GroupCharacteristicComponent() { 298 super(); 299 } 300 301 /** 302 * Constructor 303 */ 304 public GroupCharacteristicComponent(CodeableConcept code, Type value, BooleanType exclude) { 305 super(); 306 this.code = code; 307 this.value = value; 308 this.exclude = exclude; 309 } 310 311 /** 312 * @return {@link #code} (A code that identifies the kind of trait being 313 * asserted.) 314 */ 315 public CodeableConcept getCode() { 316 if (this.code == null) 317 if (Configuration.errorOnAutoCreate()) 318 throw new Error("Attempt to auto-create GroupCharacteristicComponent.code"); 319 else if (Configuration.doAutoCreate()) 320 this.code = new CodeableConcept(); // cc 321 return this.code; 322 } 323 324 public boolean hasCode() { 325 return this.code != null && !this.code.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #code} (A code that identifies the kind of trait being 330 * asserted.) 331 */ 332 public GroupCharacteristicComponent setCode(CodeableConcept value) { 333 this.code = value; 334 return this; 335 } 336 337 /** 338 * @return {@link #value} (The value of the trait that holds (or does not hold - 339 * see 'exclude') for members of the group.) 340 */ 341 public Type getValue() { 342 return this.value; 343 } 344 345 /** 346 * @return {@link #value} (The value of the trait that holds (or does not hold - 347 * see 'exclude') for members of the group.) 348 */ 349 public CodeableConcept getValueCodeableConcept() throws FHIRException { 350 if (this.value == null) 351 this.value = new CodeableConcept(); 352 if (!(this.value instanceof CodeableConcept)) 353 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 354 + this.value.getClass().getName() + " was encountered"); 355 return (CodeableConcept) this.value; 356 } 357 358 public boolean hasValueCodeableConcept() { 359 return this != null && this.value instanceof CodeableConcept; 360 } 361 362 /** 363 * @return {@link #value} (The value of the trait that holds (or does not hold - 364 * see 'exclude') for members of the group.) 365 */ 366 public BooleanType getValueBooleanType() throws FHIRException { 367 if (this.value == null) 368 this.value = new BooleanType(); 369 if (!(this.value instanceof BooleanType)) 370 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 371 + this.value.getClass().getName() + " was encountered"); 372 return (BooleanType) this.value; 373 } 374 375 public boolean hasValueBooleanType() { 376 return this != null && this.value instanceof BooleanType; 377 } 378 379 /** 380 * @return {@link #value} (The value of the trait that holds (or does not hold - 381 * see 'exclude') for members of the group.) 382 */ 383 public Quantity getValueQuantity() throws FHIRException { 384 if (this.value == null) 385 this.value = new Quantity(); 386 if (!(this.value instanceof Quantity)) 387 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 388 + " was encountered"); 389 return (Quantity) this.value; 390 } 391 392 public boolean hasValueQuantity() { 393 return this != null && this.value instanceof Quantity; 394 } 395 396 /** 397 * @return {@link #value} (The value of the trait that holds (or does not hold - 398 * see 'exclude') for members of the group.) 399 */ 400 public Range getValueRange() throws FHIRException { 401 if (this.value == null) 402 this.value = new Range(); 403 if (!(this.value instanceof Range)) 404 throw new FHIRException( 405 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 406 return (Range) this.value; 407 } 408 409 public boolean hasValueRange() { 410 return this != null && this.value instanceof Range; 411 } 412 413 /** 414 * @return {@link #value} (The value of the trait that holds (or does not hold - 415 * see 'exclude') for members of the group.) 416 */ 417 public Reference getValueReference() throws FHIRException { 418 if (this.value == null) 419 this.value = new Reference(); 420 if (!(this.value instanceof Reference)) 421 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 422 + " was encountered"); 423 return (Reference) this.value; 424 } 425 426 public boolean hasValueReference() { 427 return this != null && this.value instanceof Reference; 428 } 429 430 public boolean hasValue() { 431 return this.value != null && !this.value.isEmpty(); 432 } 433 434 /** 435 * @param value {@link #value} (The value of the trait that holds (or does not 436 * hold - see 'exclude') for members of the group.) 437 */ 438 public GroupCharacteristicComponent setValue(Type value) { 439 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType 440 || value instanceof Quantity || value instanceof Range || value instanceof Reference)) 441 throw new Error("Not the right type for Group.characteristic.value[x]: " + value.fhirType()); 442 this.value = value; 443 return this; 444 } 445 446 /** 447 * @return {@link #exclude} (If true, indicates the characteristic is one that 448 * is NOT held by members of the group.). This is the underlying object 449 * with id, value and extensions. The accessor "getExclude" gives direct 450 * access to the value 451 */ 452 public BooleanType getExcludeElement() { 453 if (this.exclude == null) 454 if (Configuration.errorOnAutoCreate()) 455 throw new Error("Attempt to auto-create GroupCharacteristicComponent.exclude"); 456 else if (Configuration.doAutoCreate()) 457 this.exclude = new BooleanType(); // bb 458 return this.exclude; 459 } 460 461 public boolean hasExcludeElement() { 462 return this.exclude != null && !this.exclude.isEmpty(); 463 } 464 465 public boolean hasExclude() { 466 return this.exclude != null && !this.exclude.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #exclude} (If true, indicates the characteristic is one 471 * that is NOT held by members of the group.). This is the 472 * underlying object with id, value and extensions. The accessor 473 * "getExclude" gives direct access to the value 474 */ 475 public GroupCharacteristicComponent setExcludeElement(BooleanType value) { 476 this.exclude = value; 477 return this; 478 } 479 480 /** 481 * @return If true, indicates the characteristic is one that is NOT held by 482 * members of the group. 483 */ 484 public boolean getExclude() { 485 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 486 } 487 488 /** 489 * @param value If true, indicates the characteristic is one that is NOT held by 490 * members of the group. 491 */ 492 public GroupCharacteristicComponent setExclude(boolean value) { 493 if (this.exclude == null) 494 this.exclude = new BooleanType(); 495 this.exclude.setValue(value); 496 return this; 497 } 498 499 /** 500 * @return {@link #period} (The period over which the characteristic is tested; 501 * e.g. the patient had an operation during the month of June.) 502 */ 503 public Period getPeriod() { 504 if (this.period == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create GroupCharacteristicComponent.period"); 507 else if (Configuration.doAutoCreate()) 508 this.period = new Period(); // cc 509 return this.period; 510 } 511 512 public boolean hasPeriod() { 513 return this.period != null && !this.period.isEmpty(); 514 } 515 516 /** 517 * @param value {@link #period} (The period over which the characteristic is 518 * tested; e.g. the patient had an operation during the month of 519 * June.) 520 */ 521 public GroupCharacteristicComponent setPeriod(Period value) { 522 this.period = value; 523 return this; 524 } 525 526 protected void listChildren(List<Property> children) { 527 super.listChildren(children); 528 children.add(new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 529 0, 1, code)); 530 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 531 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 532 value)); 533 children.add(new Property("exclude", "boolean", 534 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude)); 535 children.add(new Property("period", "Period", 536 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 537 0, 1, period)); 538 } 539 540 @Override 541 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 542 switch (_hash) { 543 case 3059181: 544 /* code */ return new Property("code", "CodeableConcept", 545 "A code that identifies the kind of trait being asserted.", 0, 1, code); 546 case -1410166417: 547 /* value[x] */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 548 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 549 value); 550 case 111972721: 551 /* value */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 552 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 553 value); 554 case 924902896: 555 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 556 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 557 value); 558 case 733421943: 559 /* valueBoolean */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 560 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 561 value); 562 case -2029823716: 563 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 564 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 565 value); 566 case 2030761548: 567 /* valueRange */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 568 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 569 value); 570 case 1755241690: 571 /* valueReference */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 572 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 573 value); 574 case -1321148966: 575 /* exclude */ return new Property("exclude", "boolean", 576 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude); 577 case -991726143: 578 /* period */ return new Property("period", "Period", 579 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 580 0, 1, period); 581 default: 582 return super.getNamedProperty(_hash, _name, _checkValid); 583 } 584 585 } 586 587 @Override 588 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 589 switch (hash) { 590 case 3059181: 591 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 592 case 111972721: 593 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 594 case -1321148966: 595 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 596 case -991726143: 597 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 598 default: 599 return super.getProperty(hash, name, checkValid); 600 } 601 602 } 603 604 @Override 605 public Base setProperty(int hash, String name, Base value) throws FHIRException { 606 switch (hash) { 607 case 3059181: // code 608 this.code = castToCodeableConcept(value); // CodeableConcept 609 return value; 610 case 111972721: // value 611 this.value = castToType(value); // Type 612 return value; 613 case -1321148966: // exclude 614 this.exclude = castToBoolean(value); // BooleanType 615 return value; 616 case -991726143: // period 617 this.period = castToPeriod(value); // Period 618 return value; 619 default: 620 return super.setProperty(hash, name, value); 621 } 622 623 } 624 625 @Override 626 public Base setProperty(String name, Base value) throws FHIRException { 627 if (name.equals("code")) { 628 this.code = castToCodeableConcept(value); // CodeableConcept 629 } else if (name.equals("value[x]")) { 630 this.value = castToType(value); // Type 631 } else if (name.equals("exclude")) { 632 this.exclude = castToBoolean(value); // BooleanType 633 } else if (name.equals("period")) { 634 this.period = castToPeriod(value); // Period 635 } else 636 return super.setProperty(name, value); 637 return value; 638 } 639 640 @Override 641 public void removeChild(String name, Base value) throws FHIRException { 642 if (name.equals("code")) { 643 this.code = null; 644 } else if (name.equals("value[x]")) { 645 this.value = null; 646 } else if (name.equals("exclude")) { 647 this.exclude = null; 648 } else if (name.equals("period")) { 649 this.period = null; 650 } else 651 super.removeChild(name, value); 652 653 } 654 655 @Override 656 public Base makeProperty(int hash, String name) throws FHIRException { 657 switch (hash) { 658 case 3059181: 659 return getCode(); 660 case -1410166417: 661 return getValue(); 662 case 111972721: 663 return getValue(); 664 case -1321148966: 665 return getExcludeElement(); 666 case -991726143: 667 return getPeriod(); 668 default: 669 return super.makeProperty(hash, name); 670 } 671 672 } 673 674 @Override 675 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 676 switch (hash) { 677 case 3059181: 678 /* code */ return new String[] { "CodeableConcept" }; 679 case 111972721: 680 /* value */ return new String[] { "CodeableConcept", "boolean", "Quantity", "Range", "Reference" }; 681 case -1321148966: 682 /* exclude */ return new String[] { "boolean" }; 683 case -991726143: 684 /* period */ return new String[] { "Period" }; 685 default: 686 return super.getTypesForProperty(hash, name); 687 } 688 689 } 690 691 @Override 692 public Base addChild(String name) throws FHIRException { 693 if (name.equals("code")) { 694 this.code = new CodeableConcept(); 695 return this.code; 696 } else if (name.equals("valueCodeableConcept")) { 697 this.value = new CodeableConcept(); 698 return this.value; 699 } else if (name.equals("valueBoolean")) { 700 this.value = new BooleanType(); 701 return this.value; 702 } else if (name.equals("valueQuantity")) { 703 this.value = new Quantity(); 704 return this.value; 705 } else if (name.equals("valueRange")) { 706 this.value = new Range(); 707 return this.value; 708 } else if (name.equals("valueReference")) { 709 this.value = new Reference(); 710 return this.value; 711 } else if (name.equals("exclude")) { 712 throw new FHIRException("Cannot call addChild on a singleton property Group.exclude"); 713 } else if (name.equals("period")) { 714 this.period = new Period(); 715 return this.period; 716 } else 717 return super.addChild(name); 718 } 719 720 public GroupCharacteristicComponent copy() { 721 GroupCharacteristicComponent dst = new GroupCharacteristicComponent(); 722 copyValues(dst); 723 return dst; 724 } 725 726 public void copyValues(GroupCharacteristicComponent dst) { 727 super.copyValues(dst); 728 dst.code = code == null ? null : code.copy(); 729 dst.value = value == null ? null : value.copy(); 730 dst.exclude = exclude == null ? null : exclude.copy(); 731 dst.period = period == null ? null : period.copy(); 732 } 733 734 @Override 735 public boolean equalsDeep(Base other_) { 736 if (!super.equalsDeep(other_)) 737 return false; 738 if (!(other_ instanceof GroupCharacteristicComponent)) 739 return false; 740 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 741 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 742 && compareDeep(exclude, o.exclude, true) && compareDeep(period, o.period, true); 743 } 744 745 @Override 746 public boolean equalsShallow(Base other_) { 747 if (!super.equalsShallow(other_)) 748 return false; 749 if (!(other_ instanceof GroupCharacteristicComponent)) 750 return false; 751 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 752 return compareValues(exclude, o.exclude, true); 753 } 754 755 public boolean isEmpty() { 756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period); 757 } 758 759 public String fhirType() { 760 return "Group.characteristic"; 761 762 } 763 764 } 765 766 @Block() 767 public static class GroupMemberComponent extends BackboneElement implements IBaseBackboneElement { 768 /** 769 * A reference to the entity that is a member of the group. Must be consistent 770 * with Group.type. If the entity is another group, then the type must be the 771 * same. 772 */ 773 @Child(name = "entity", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 774 Medication.class, Substance.class, 775 Group.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 776 @Description(shortDefinition = "Reference to the group member", formalDefinition = "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.") 777 protected Reference entity; 778 779 /** 780 * The actual object that is the target of the reference (A reference to the 781 * entity that is a member of the group. Must be consistent with Group.type. If 782 * the entity is another group, then the type must be the same.) 783 */ 784 protected Resource entityTarget; 785 786 /** 787 * The period that the member was in the group, if known. 788 */ 789 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 790 @Description(shortDefinition = "Period member belonged to the group", formalDefinition = "The period that the member was in the group, if known.") 791 protected Period period; 792 793 /** 794 * A flag to indicate that the member is no longer in the group, but previously 795 * may have been a member. 796 */ 797 @Child(name = "inactive", type = { 798 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 799 @Description(shortDefinition = "If member is no longer in group", formalDefinition = "A flag to indicate that the member is no longer in the group, but previously may have been a member.") 800 protected BooleanType inactive; 801 802 private static final long serialVersionUID = -333869055L; 803 804 /** 805 * Constructor 806 */ 807 public GroupMemberComponent() { 808 super(); 809 } 810 811 /** 812 * Constructor 813 */ 814 public GroupMemberComponent(Reference entity) { 815 super(); 816 this.entity = entity; 817 } 818 819 /** 820 * @return {@link #entity} (A reference to the entity that is a member of the 821 * group. Must be consistent with Group.type. If the entity is another 822 * group, then the type must be the same.) 823 */ 824 public Reference getEntity() { 825 if (this.entity == null) 826 if (Configuration.errorOnAutoCreate()) 827 throw new Error("Attempt to auto-create GroupMemberComponent.entity"); 828 else if (Configuration.doAutoCreate()) 829 this.entity = new Reference(); // cc 830 return this.entity; 831 } 832 833 public boolean hasEntity() { 834 return this.entity != null && !this.entity.isEmpty(); 835 } 836 837 /** 838 * @param value {@link #entity} (A reference to the entity that is a member of 839 * the group. Must be consistent with Group.type. If the entity is 840 * another group, then the type must be the same.) 841 */ 842 public GroupMemberComponent setEntity(Reference value) { 843 this.entity = value; 844 return this; 845 } 846 847 /** 848 * @return {@link #entity} The actual object that is the target of the 849 * reference. The reference library doesn't populate this, but you can 850 * use it to hold the resource if you resolve it. (A reference to the 851 * entity that is a member of the group. Must be consistent with 852 * Group.type. If the entity is another group, then the type must be the 853 * same.) 854 */ 855 public Resource getEntityTarget() { 856 return this.entityTarget; 857 } 858 859 /** 860 * @param value {@link #entity} The actual object that is the target of the 861 * reference. The reference library doesn't use these, but you can 862 * use it to hold the resource if you resolve it. (A reference to 863 * the entity that is a member of the group. Must be consistent 864 * with Group.type. If the entity is another group, then the type 865 * must be the same.) 866 */ 867 public GroupMemberComponent setEntityTarget(Resource value) { 868 this.entityTarget = value; 869 return this; 870 } 871 872 /** 873 * @return {@link #period} (The period that the member was in the group, if 874 * known.) 875 */ 876 public Period getPeriod() { 877 if (this.period == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create GroupMemberComponent.period"); 880 else if (Configuration.doAutoCreate()) 881 this.period = new Period(); // cc 882 return this.period; 883 } 884 885 public boolean hasPeriod() { 886 return this.period != null && !this.period.isEmpty(); 887 } 888 889 /** 890 * @param value {@link #period} (The period that the member was in the group, if 891 * known.) 892 */ 893 public GroupMemberComponent setPeriod(Period value) { 894 this.period = value; 895 return this; 896 } 897 898 /** 899 * @return {@link #inactive} (A flag to indicate that the member is no longer in 900 * the group, but previously may have been a member.). This is the 901 * underlying object with id, value and extensions. The accessor 902 * "getInactive" gives direct access to the value 903 */ 904 public BooleanType getInactiveElement() { 905 if (this.inactive == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create GroupMemberComponent.inactive"); 908 else if (Configuration.doAutoCreate()) 909 this.inactive = new BooleanType(); // bb 910 return this.inactive; 911 } 912 913 public boolean hasInactiveElement() { 914 return this.inactive != null && !this.inactive.isEmpty(); 915 } 916 917 public boolean hasInactive() { 918 return this.inactive != null && !this.inactive.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #inactive} (A flag to indicate that the member is no 923 * longer in the group, but previously may have been a member.). 924 * This is the underlying object with id, value and extensions. The 925 * accessor "getInactive" gives direct access to the value 926 */ 927 public GroupMemberComponent setInactiveElement(BooleanType value) { 928 this.inactive = value; 929 return this; 930 } 931 932 /** 933 * @return A flag to indicate that the member is no longer in the group, but 934 * previously may have been a member. 935 */ 936 public boolean getInactive() { 937 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 938 } 939 940 /** 941 * @param value A flag to indicate that the member is no longer in the group, 942 * but previously may have been a member. 943 */ 944 public GroupMemberComponent setInactive(boolean value) { 945 if (this.inactive == null) 946 this.inactive = new BooleanType(); 947 this.inactive.setValue(value); 948 return this; 949 } 950 951 protected void listChildren(List<Property> children) { 952 super.listChildren(children); 953 children.add(new Property("entity", 954 "Reference(Patient|Practitioner|PractitionerRole|Device|Medication|Substance|Group)", 955 "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 956 0, 1, entity)); 957 children.add( 958 new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period)); 959 children.add(new Property("inactive", "boolean", 960 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, 961 inactive)); 962 } 963 964 @Override 965 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 966 switch (_hash) { 967 case -1298275357: 968 /* entity */ return new Property("entity", 969 "Reference(Patient|Practitioner|PractitionerRole|Device|Medication|Substance|Group)", 970 "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 971 0, 1, entity); 972 case -991726143: 973 /* period */ return new Property("period", "Period", "The period that the member was in the group, if known.", 974 0, 1, period); 975 case 24665195: 976 /* inactive */ return new Property("inactive", "boolean", 977 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 978 1, inactive); 979 default: 980 return super.getNamedProperty(_hash, _name, _checkValid); 981 } 982 983 } 984 985 @Override 986 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 987 switch (hash) { 988 case -1298275357: 989 /* entity */ return this.entity == null ? new Base[0] : new Base[] { this.entity }; // Reference 990 case -991726143: 991 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 992 case 24665195: 993 /* inactive */ return this.inactive == null ? new Base[0] : new Base[] { this.inactive }; // BooleanType 994 default: 995 return super.getProperty(hash, name, checkValid); 996 } 997 998 } 999 1000 @Override 1001 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1002 switch (hash) { 1003 case -1298275357: // entity 1004 this.entity = castToReference(value); // Reference 1005 return value; 1006 case -991726143: // period 1007 this.period = castToPeriod(value); // Period 1008 return value; 1009 case 24665195: // inactive 1010 this.inactive = castToBoolean(value); // BooleanType 1011 return value; 1012 default: 1013 return super.setProperty(hash, name, value); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(String name, Base value) throws FHIRException { 1020 if (name.equals("entity")) { 1021 this.entity = castToReference(value); // Reference 1022 } else if (name.equals("period")) { 1023 this.period = castToPeriod(value); // Period 1024 } else if (name.equals("inactive")) { 1025 this.inactive = castToBoolean(value); // BooleanType 1026 } else 1027 return super.setProperty(name, value); 1028 return value; 1029 } 1030 1031 @Override 1032 public void removeChild(String name, Base value) throws FHIRException { 1033 if (name.equals("entity")) { 1034 this.entity = null; 1035 } else if (name.equals("period")) { 1036 this.period = null; 1037 } else if (name.equals("inactive")) { 1038 this.inactive = null; 1039 } else 1040 super.removeChild(name, value); 1041 1042 } 1043 1044 @Override 1045 public Base makeProperty(int hash, String name) throws FHIRException { 1046 switch (hash) { 1047 case -1298275357: 1048 return getEntity(); 1049 case -991726143: 1050 return getPeriod(); 1051 case 24665195: 1052 return getInactiveElement(); 1053 default: 1054 return super.makeProperty(hash, name); 1055 } 1056 1057 } 1058 1059 @Override 1060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1061 switch (hash) { 1062 case -1298275357: 1063 /* entity */ return new String[] { "Reference" }; 1064 case -991726143: 1065 /* period */ return new String[] { "Period" }; 1066 case 24665195: 1067 /* inactive */ return new String[] { "boolean" }; 1068 default: 1069 return super.getTypesForProperty(hash, name); 1070 } 1071 1072 } 1073 1074 @Override 1075 public Base addChild(String name) throws FHIRException { 1076 if (name.equals("entity")) { 1077 this.entity = new Reference(); 1078 return this.entity; 1079 } else if (name.equals("period")) { 1080 this.period = new Period(); 1081 return this.period; 1082 } else if (name.equals("inactive")) { 1083 throw new FHIRException("Cannot call addChild on a singleton property Group.inactive"); 1084 } else 1085 return super.addChild(name); 1086 } 1087 1088 public GroupMemberComponent copy() { 1089 GroupMemberComponent dst = new GroupMemberComponent(); 1090 copyValues(dst); 1091 return dst; 1092 } 1093 1094 public void copyValues(GroupMemberComponent dst) { 1095 super.copyValues(dst); 1096 dst.entity = entity == null ? null : entity.copy(); 1097 dst.period = period == null ? null : period.copy(); 1098 dst.inactive = inactive == null ? null : inactive.copy(); 1099 } 1100 1101 @Override 1102 public boolean equalsDeep(Base other_) { 1103 if (!super.equalsDeep(other_)) 1104 return false; 1105 if (!(other_ instanceof GroupMemberComponent)) 1106 return false; 1107 GroupMemberComponent o = (GroupMemberComponent) other_; 1108 return compareDeep(entity, o.entity, true) && compareDeep(period, o.period, true) 1109 && compareDeep(inactive, o.inactive, true); 1110 } 1111 1112 @Override 1113 public boolean equalsShallow(Base other_) { 1114 if (!super.equalsShallow(other_)) 1115 return false; 1116 if (!(other_ instanceof GroupMemberComponent)) 1117 return false; 1118 GroupMemberComponent o = (GroupMemberComponent) other_; 1119 return compareValues(inactive, o.inactive, true); 1120 } 1121 1122 public boolean isEmpty() { 1123 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, period, inactive); 1124 } 1125 1126 public String fhirType() { 1127 return "Group.member"; 1128 1129 } 1130 1131 } 1132 1133 /** 1134 * A unique business identifier for this group. 1135 */ 1136 @Child(name = "identifier", type = { 1137 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1138 @Description(shortDefinition = "Unique id", formalDefinition = "A unique business identifier for this group.") 1139 protected List<Identifier> identifier; 1140 1141 /** 1142 * Indicates whether the record for the group is available for use or is merely 1143 * being retained for historical purposes. 1144 */ 1145 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1146 @Description(shortDefinition = "Whether this group's record is in active use", formalDefinition = "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.") 1147 protected BooleanType active; 1148 1149 /** 1150 * Identifies the broad classification of the kind of resources the group 1151 * includes. 1152 */ 1153 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1154 @Description(shortDefinition = "person | animal | practitioner | device | medication | substance", formalDefinition = "Identifies the broad classification of the kind of resources the group includes.") 1155 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-type") 1156 protected Enumeration<GroupType> type; 1157 1158 /** 1159 * If true, indicates that the resource refers to a specific group of real 1160 * individuals. If false, the group defines a set of intended individuals. 1161 */ 1162 @Child(name = "actual", type = { BooleanType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1163 @Description(shortDefinition = "Descriptive or actual", formalDefinition = "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.") 1164 protected BooleanType actual; 1165 1166 /** 1167 * Provides a specific type of resource the group includes; e.g. "cow", 1168 * "syringe", etc. 1169 */ 1170 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1171 @Description(shortDefinition = "Kind of Group members", formalDefinition = "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.") 1172 protected CodeableConcept code; 1173 1174 /** 1175 * A label assigned to the group for human identification and communication. 1176 */ 1177 @Child(name = "name", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1178 @Description(shortDefinition = "Label for Group", formalDefinition = "A label assigned to the group for human identification and communication.") 1179 protected StringType name; 1180 1181 /** 1182 * A count of the number of resource instances that are part of the group. 1183 */ 1184 @Child(name = "quantity", type = { 1185 UnsignedIntType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1186 @Description(shortDefinition = "Number of members", formalDefinition = "A count of the number of resource instances that are part of the group.") 1187 protected UnsignedIntType quantity; 1188 1189 /** 1190 * Entity responsible for defining and maintaining Group characteristics and/or 1191 * registered members. 1192 */ 1193 @Child(name = "managingEntity", type = { Organization.class, RelatedPerson.class, Practitioner.class, 1194 PractitionerRole.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1195 @Description(shortDefinition = "Entity that is the custodian of the Group's definition", formalDefinition = "Entity responsible for defining and maintaining Group characteristics and/or registered members.") 1196 protected Reference managingEntity; 1197 1198 /** 1199 * The actual object that is the target of the reference (Entity responsible for 1200 * defining and maintaining Group characteristics and/or registered members.) 1201 */ 1202 protected Resource managingEntityTarget; 1203 1204 /** 1205 * Identifies traits whose presence r absence is shared by members of the group. 1206 */ 1207 @Child(name = "characteristic", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1208 @Description(shortDefinition = "Include / Exclude group members by Trait", formalDefinition = "Identifies traits whose presence r absence is shared by members of the group.") 1209 protected List<GroupCharacteristicComponent> characteristic; 1210 1211 /** 1212 * Identifies the resource instances that are members of the group. 1213 */ 1214 @Child(name = "member", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1215 @Description(shortDefinition = "Who or what is in group", formalDefinition = "Identifies the resource instances that are members of the group.") 1216 protected List<GroupMemberComponent> member; 1217 1218 private static final long serialVersionUID = -550945963L; 1219 1220 /** 1221 * Constructor 1222 */ 1223 public Group() { 1224 super(); 1225 } 1226 1227 /** 1228 * Constructor 1229 */ 1230 public Group(Enumeration<GroupType> type, BooleanType actual) { 1231 super(); 1232 this.type = type; 1233 this.actual = actual; 1234 } 1235 1236 /** 1237 * @return {@link #identifier} (A unique business identifier for this group.) 1238 */ 1239 public List<Identifier> getIdentifier() { 1240 if (this.identifier == null) 1241 this.identifier = new ArrayList<Identifier>(); 1242 return this.identifier; 1243 } 1244 1245 /** 1246 * @return Returns a reference to <code>this</code> for easy method chaining 1247 */ 1248 public Group setIdentifier(List<Identifier> theIdentifier) { 1249 this.identifier = theIdentifier; 1250 return this; 1251 } 1252 1253 public boolean hasIdentifier() { 1254 if (this.identifier == null) 1255 return false; 1256 for (Identifier item : this.identifier) 1257 if (!item.isEmpty()) 1258 return true; 1259 return false; 1260 } 1261 1262 public Identifier addIdentifier() { // 3 1263 Identifier t = new Identifier(); 1264 if (this.identifier == null) 1265 this.identifier = new ArrayList<Identifier>(); 1266 this.identifier.add(t); 1267 return t; 1268 } 1269 1270 public Group addIdentifier(Identifier t) { // 3 1271 if (t == null) 1272 return this; 1273 if (this.identifier == null) 1274 this.identifier = new ArrayList<Identifier>(); 1275 this.identifier.add(t); 1276 return this; 1277 } 1278 1279 /** 1280 * @return The first repetition of repeating field {@link #identifier}, creating 1281 * it if it does not already exist 1282 */ 1283 public Identifier getIdentifierFirstRep() { 1284 if (getIdentifier().isEmpty()) { 1285 addIdentifier(); 1286 } 1287 return getIdentifier().get(0); 1288 } 1289 1290 /** 1291 * @return {@link #active} (Indicates whether the record for the group is 1292 * available for use or is merely being retained for historical 1293 * purposes.). This is the underlying object with id, value and 1294 * extensions. The accessor "getActive" gives direct access to the value 1295 */ 1296 public BooleanType getActiveElement() { 1297 if (this.active == null) 1298 if (Configuration.errorOnAutoCreate()) 1299 throw new Error("Attempt to auto-create Group.active"); 1300 else if (Configuration.doAutoCreate()) 1301 this.active = new BooleanType(); // bb 1302 return this.active; 1303 } 1304 1305 public boolean hasActiveElement() { 1306 return this.active != null && !this.active.isEmpty(); 1307 } 1308 1309 public boolean hasActive() { 1310 return this.active != null && !this.active.isEmpty(); 1311 } 1312 1313 /** 1314 * @param value {@link #active} (Indicates whether the record for the group is 1315 * available for use or is merely being retained for historical 1316 * purposes.). This is the underlying object with id, value and 1317 * extensions. The accessor "getActive" gives direct access to the 1318 * value 1319 */ 1320 public Group setActiveElement(BooleanType value) { 1321 this.active = value; 1322 return this; 1323 } 1324 1325 /** 1326 * @return Indicates whether the record for the group is available for use or is 1327 * merely being retained for historical purposes. 1328 */ 1329 public boolean getActive() { 1330 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1331 } 1332 1333 /** 1334 * @param value Indicates whether the record for the group is available for use 1335 * or is merely being retained for historical purposes. 1336 */ 1337 public Group setActive(boolean value) { 1338 if (this.active == null) 1339 this.active = new BooleanType(); 1340 this.active.setValue(value); 1341 return this; 1342 } 1343 1344 /** 1345 * @return {@link #type} (Identifies the broad classification of the kind of 1346 * resources the group includes.). This is the underlying object with 1347 * id, value and extensions. The accessor "getType" gives direct access 1348 * to the value 1349 */ 1350 public Enumeration<GroupType> getTypeElement() { 1351 if (this.type == null) 1352 if (Configuration.errorOnAutoCreate()) 1353 throw new Error("Attempt to auto-create Group.type"); 1354 else if (Configuration.doAutoCreate()) 1355 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); // bb 1356 return this.type; 1357 } 1358 1359 public boolean hasTypeElement() { 1360 return this.type != null && !this.type.isEmpty(); 1361 } 1362 1363 public boolean hasType() { 1364 return this.type != null && !this.type.isEmpty(); 1365 } 1366 1367 /** 1368 * @param value {@link #type} (Identifies the broad classification of the kind 1369 * of resources the group includes.). This is the underlying object 1370 * with id, value and extensions. The accessor "getType" gives 1371 * direct access to the value 1372 */ 1373 public Group setTypeElement(Enumeration<GroupType> value) { 1374 this.type = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return Identifies the broad classification of the kind of resources the 1380 * group includes. 1381 */ 1382 public GroupType getType() { 1383 return this.type == null ? null : this.type.getValue(); 1384 } 1385 1386 /** 1387 * @param value Identifies the broad classification of the kind of resources the 1388 * group includes. 1389 */ 1390 public Group setType(GroupType value) { 1391 if (this.type == null) 1392 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); 1393 this.type.setValue(value); 1394 return this; 1395 } 1396 1397 /** 1398 * @return {@link #actual} (If true, indicates that the resource refers to a 1399 * specific group of real individuals. If false, the group defines a set 1400 * of intended individuals.). This is the underlying object with id, 1401 * value and extensions. The accessor "getActual" gives direct access to 1402 * the value 1403 */ 1404 public BooleanType getActualElement() { 1405 if (this.actual == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create Group.actual"); 1408 else if (Configuration.doAutoCreate()) 1409 this.actual = new BooleanType(); // bb 1410 return this.actual; 1411 } 1412 1413 public boolean hasActualElement() { 1414 return this.actual != null && !this.actual.isEmpty(); 1415 } 1416 1417 public boolean hasActual() { 1418 return this.actual != null && !this.actual.isEmpty(); 1419 } 1420 1421 /** 1422 * @param value {@link #actual} (If true, indicates that the resource refers to 1423 * a specific group of real individuals. If false, the group 1424 * defines a set of intended individuals.). This is the underlying 1425 * object with id, value and extensions. The accessor "getActual" 1426 * gives direct access to the value 1427 */ 1428 public Group setActualElement(BooleanType value) { 1429 this.actual = value; 1430 return this; 1431 } 1432 1433 /** 1434 * @return If true, indicates that the resource refers to a specific group of 1435 * real individuals. If false, the group defines a set of intended 1436 * individuals. 1437 */ 1438 public boolean getActual() { 1439 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 1440 } 1441 1442 /** 1443 * @param value If true, indicates that the resource refers to a specific group 1444 * of real individuals. If false, the group defines a set of 1445 * intended individuals. 1446 */ 1447 public Group setActual(boolean value) { 1448 if (this.actual == null) 1449 this.actual = new BooleanType(); 1450 this.actual.setValue(value); 1451 return this; 1452 } 1453 1454 /** 1455 * @return {@link #code} (Provides a specific type of resource the group 1456 * includes; e.g. "cow", "syringe", etc.) 1457 */ 1458 public CodeableConcept getCode() { 1459 if (this.code == null) 1460 if (Configuration.errorOnAutoCreate()) 1461 throw new Error("Attempt to auto-create Group.code"); 1462 else if (Configuration.doAutoCreate()) 1463 this.code = new CodeableConcept(); // cc 1464 return this.code; 1465 } 1466 1467 public boolean hasCode() { 1468 return this.code != null && !this.code.isEmpty(); 1469 } 1470 1471 /** 1472 * @param value {@link #code} (Provides a specific type of resource the group 1473 * includes; e.g. "cow", "syringe", etc.) 1474 */ 1475 public Group setCode(CodeableConcept value) { 1476 this.code = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return {@link #name} (A label assigned to the group for human identification 1482 * and communication.). This is the underlying object with id, value and 1483 * extensions. The accessor "getName" gives direct access to the value 1484 */ 1485 public StringType getNameElement() { 1486 if (this.name == null) 1487 if (Configuration.errorOnAutoCreate()) 1488 throw new Error("Attempt to auto-create Group.name"); 1489 else if (Configuration.doAutoCreate()) 1490 this.name = new StringType(); // bb 1491 return this.name; 1492 } 1493 1494 public boolean hasNameElement() { 1495 return this.name != null && !this.name.isEmpty(); 1496 } 1497 1498 public boolean hasName() { 1499 return this.name != null && !this.name.isEmpty(); 1500 } 1501 1502 /** 1503 * @param value {@link #name} (A label assigned to the group for human 1504 * identification and communication.). This is the underlying 1505 * object with id, value and extensions. The accessor "getName" 1506 * gives direct access to the value 1507 */ 1508 public Group setNameElement(StringType value) { 1509 this.name = value; 1510 return this; 1511 } 1512 1513 /** 1514 * @return A label assigned to the group for human identification and 1515 * communication. 1516 */ 1517 public String getName() { 1518 return this.name == null ? null : this.name.getValue(); 1519 } 1520 1521 /** 1522 * @param value A label assigned to the group for human identification and 1523 * communication. 1524 */ 1525 public Group setName(String value) { 1526 if (Utilities.noString(value)) 1527 this.name = null; 1528 else { 1529 if (this.name == null) 1530 this.name = new StringType(); 1531 this.name.setValue(value); 1532 } 1533 return this; 1534 } 1535 1536 /** 1537 * @return {@link #quantity} (A count of the number of resource instances that 1538 * are part of the group.). This is the underlying object with id, value 1539 * and extensions. The accessor "getQuantity" gives direct access to the 1540 * value 1541 */ 1542 public UnsignedIntType getQuantityElement() { 1543 if (this.quantity == null) 1544 if (Configuration.errorOnAutoCreate()) 1545 throw new Error("Attempt to auto-create Group.quantity"); 1546 else if (Configuration.doAutoCreate()) 1547 this.quantity = new UnsignedIntType(); // bb 1548 return this.quantity; 1549 } 1550 1551 public boolean hasQuantityElement() { 1552 return this.quantity != null && !this.quantity.isEmpty(); 1553 } 1554 1555 public boolean hasQuantity() { 1556 return this.quantity != null && !this.quantity.isEmpty(); 1557 } 1558 1559 /** 1560 * @param value {@link #quantity} (A count of the number of resource instances 1561 * that are part of the group.). This is the underlying object with 1562 * id, value and extensions. The accessor "getQuantity" gives 1563 * direct access to the value 1564 */ 1565 public Group setQuantityElement(UnsignedIntType value) { 1566 this.quantity = value; 1567 return this; 1568 } 1569 1570 /** 1571 * @return A count of the number of resource instances that are part of the 1572 * group. 1573 */ 1574 public int getQuantity() { 1575 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1576 } 1577 1578 /** 1579 * @param value A count of the number of resource instances that are part of the 1580 * group. 1581 */ 1582 public Group setQuantity(int value) { 1583 if (this.quantity == null) 1584 this.quantity = new UnsignedIntType(); 1585 this.quantity.setValue(value); 1586 return this; 1587 } 1588 1589 /** 1590 * @return {@link #managingEntity} (Entity responsible for defining and 1591 * maintaining Group characteristics and/or registered members.) 1592 */ 1593 public Reference getManagingEntity() { 1594 if (this.managingEntity == null) 1595 if (Configuration.errorOnAutoCreate()) 1596 throw new Error("Attempt to auto-create Group.managingEntity"); 1597 else if (Configuration.doAutoCreate()) 1598 this.managingEntity = new Reference(); // cc 1599 return this.managingEntity; 1600 } 1601 1602 public boolean hasManagingEntity() { 1603 return this.managingEntity != null && !this.managingEntity.isEmpty(); 1604 } 1605 1606 /** 1607 * @param value {@link #managingEntity} (Entity responsible for defining and 1608 * maintaining Group characteristics and/or registered members.) 1609 */ 1610 public Group setManagingEntity(Reference value) { 1611 this.managingEntity = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #managingEntity} The actual object that is the target of the 1617 * reference. The reference library doesn't populate this, but you can 1618 * use it to hold the resource if you resolve it. (Entity responsible 1619 * for defining and maintaining Group characteristics and/or registered 1620 * members.) 1621 */ 1622 public Resource getManagingEntityTarget() { 1623 return this.managingEntityTarget; 1624 } 1625 1626 /** 1627 * @param value {@link #managingEntity} The actual object that is the target of 1628 * the reference. The reference library doesn't use these, but you 1629 * can use it to hold the resource if you resolve it. (Entity 1630 * responsible for defining and maintaining Group characteristics 1631 * and/or registered members.) 1632 */ 1633 public Group setManagingEntityTarget(Resource value) { 1634 this.managingEntityTarget = value; 1635 return this; 1636 } 1637 1638 /** 1639 * @return {@link #characteristic} (Identifies traits whose presence r absence 1640 * is shared by members of the group.) 1641 */ 1642 public List<GroupCharacteristicComponent> getCharacteristic() { 1643 if (this.characteristic == null) 1644 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1645 return this.characteristic; 1646 } 1647 1648 /** 1649 * @return Returns a reference to <code>this</code> for easy method chaining 1650 */ 1651 public Group setCharacteristic(List<GroupCharacteristicComponent> theCharacteristic) { 1652 this.characteristic = theCharacteristic; 1653 return this; 1654 } 1655 1656 public boolean hasCharacteristic() { 1657 if (this.characteristic == null) 1658 return false; 1659 for (GroupCharacteristicComponent item : this.characteristic) 1660 if (!item.isEmpty()) 1661 return true; 1662 return false; 1663 } 1664 1665 public GroupCharacteristicComponent addCharacteristic() { // 3 1666 GroupCharacteristicComponent t = new GroupCharacteristicComponent(); 1667 if (this.characteristic == null) 1668 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1669 this.characteristic.add(t); 1670 return t; 1671 } 1672 1673 public Group addCharacteristic(GroupCharacteristicComponent t) { // 3 1674 if (t == null) 1675 return this; 1676 if (this.characteristic == null) 1677 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1678 this.characteristic.add(t); 1679 return this; 1680 } 1681 1682 /** 1683 * @return The first repetition of repeating field {@link #characteristic}, 1684 * creating it if it does not already exist 1685 */ 1686 public GroupCharacteristicComponent getCharacteristicFirstRep() { 1687 if (getCharacteristic().isEmpty()) { 1688 addCharacteristic(); 1689 } 1690 return getCharacteristic().get(0); 1691 } 1692 1693 /** 1694 * @return {@link #member} (Identifies the resource instances that are members 1695 * of the group.) 1696 */ 1697 public List<GroupMemberComponent> getMember() { 1698 if (this.member == null) 1699 this.member = new ArrayList<GroupMemberComponent>(); 1700 return this.member; 1701 } 1702 1703 /** 1704 * @return Returns a reference to <code>this</code> for easy method chaining 1705 */ 1706 public Group setMember(List<GroupMemberComponent> theMember) { 1707 this.member = theMember; 1708 return this; 1709 } 1710 1711 public boolean hasMember() { 1712 if (this.member == null) 1713 return false; 1714 for (GroupMemberComponent item : this.member) 1715 if (!item.isEmpty()) 1716 return true; 1717 return false; 1718 } 1719 1720 public GroupMemberComponent addMember() { // 3 1721 GroupMemberComponent t = new GroupMemberComponent(); 1722 if (this.member == null) 1723 this.member = new ArrayList<GroupMemberComponent>(); 1724 this.member.add(t); 1725 return t; 1726 } 1727 1728 public Group addMember(GroupMemberComponent t) { // 3 1729 if (t == null) 1730 return this; 1731 if (this.member == null) 1732 this.member = new ArrayList<GroupMemberComponent>(); 1733 this.member.add(t); 1734 return this; 1735 } 1736 1737 /** 1738 * @return The first repetition of repeating field {@link #member}, creating it 1739 * if it does not already exist 1740 */ 1741 public GroupMemberComponent getMemberFirstRep() { 1742 if (getMember().isEmpty()) { 1743 addMember(); 1744 } 1745 return getMember().get(0); 1746 } 1747 1748 protected void listChildren(List<Property> children) { 1749 super.listChildren(children); 1750 children.add(new Property("identifier", "Identifier", "A unique business identifier for this group.", 0, 1751 java.lang.Integer.MAX_VALUE, identifier)); 1752 children.add(new Property("active", "boolean", 1753 "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 1754 0, 1, active)); 1755 children.add(new Property("type", "code", 1756 "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type)); 1757 children.add(new Property("actual", "boolean", 1758 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1759 0, 1, actual)); 1760 children.add(new Property("code", "CodeableConcept", 1761 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code)); 1762 children.add(new Property("name", "string", 1763 "A label assigned to the group for human identification and communication.", 0, 1, name)); 1764 children.add(new Property("quantity", "unsignedInt", 1765 "A count of the number of resource instances that are part of the group.", 0, 1, quantity)); 1766 children.add(new Property("managingEntity", "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", 1767 "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, 1768 managingEntity)); 1769 children.add(new Property("characteristic", "", 1770 "Identifies traits whose presence r absence is shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, 1771 characteristic)); 1772 children.add(new Property("member", "", "Identifies the resource instances that are members of the group.", 0, 1773 java.lang.Integer.MAX_VALUE, member)); 1774 } 1775 1776 @Override 1777 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1778 switch (_hash) { 1779 case -1618432855: 1780 /* identifier */ return new Property("identifier", "Identifier", "A unique business identifier for this group.", 1781 0, java.lang.Integer.MAX_VALUE, identifier); 1782 case -1422950650: 1783 /* active */ return new Property("active", "boolean", 1784 "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 1785 0, 1, active); 1786 case 3575610: 1787 /* type */ return new Property("type", "code", 1788 "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type); 1789 case -1422939762: 1790 /* actual */ return new Property("actual", "boolean", 1791 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1792 0, 1, actual); 1793 case 3059181: 1794 /* code */ return new Property("code", "CodeableConcept", 1795 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code); 1796 case 3373707: 1797 /* name */ return new Property("name", "string", 1798 "A label assigned to the group for human identification and communication.", 0, 1, name); 1799 case -1285004149: 1800 /* quantity */ return new Property("quantity", "unsignedInt", 1801 "A count of the number of resource instances that are part of the group.", 0, 1, quantity); 1802 case -988474523: 1803 /* managingEntity */ return new Property("managingEntity", 1804 "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", 1805 "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, 1806 managingEntity); 1807 case 366313883: 1808 /* characteristic */ return new Property("characteristic", "", 1809 "Identifies traits whose presence r absence is shared by members of the group.", 0, 1810 java.lang.Integer.MAX_VALUE, characteristic); 1811 case -1077769574: 1812 /* member */ return new Property("member", "", "Identifies the resource instances that are members of the group.", 1813 0, java.lang.Integer.MAX_VALUE, member); 1814 default: 1815 return super.getNamedProperty(_hash, _name, _checkValid); 1816 } 1817 1818 } 1819 1820 @Override 1821 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1822 switch (hash) { 1823 case -1618432855: 1824 /* identifier */ return this.identifier == null ? new Base[0] 1825 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1826 case -1422950650: 1827 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1828 case 3575610: 1829 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<GroupType> 1830 case -1422939762: 1831 /* actual */ return this.actual == null ? new Base[0] : new Base[] { this.actual }; // BooleanType 1832 case 3059181: 1833 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1834 case 3373707: 1835 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1836 case -1285004149: 1837 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // UnsignedIntType 1838 case -988474523: 1839 /* managingEntity */ return this.managingEntity == null ? new Base[0] : new Base[] { this.managingEntity }; // Reference 1840 case 366313883: 1841 /* characteristic */ return this.characteristic == null ? new Base[0] 1842 : this.characteristic.toArray(new Base[this.characteristic.size()]); // GroupCharacteristicComponent 1843 case -1077769574: 1844 /* member */ return this.member == null ? new Base[0] : this.member.toArray(new Base[this.member.size()]); // GroupMemberComponent 1845 default: 1846 return super.getProperty(hash, name, checkValid); 1847 } 1848 1849 } 1850 1851 @Override 1852 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1853 switch (hash) { 1854 case -1618432855: // identifier 1855 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1856 return value; 1857 case -1422950650: // active 1858 this.active = castToBoolean(value); // BooleanType 1859 return value; 1860 case 3575610: // type 1861 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1862 this.type = (Enumeration) value; // Enumeration<GroupType> 1863 return value; 1864 case -1422939762: // actual 1865 this.actual = castToBoolean(value); // BooleanType 1866 return value; 1867 case 3059181: // code 1868 this.code = castToCodeableConcept(value); // CodeableConcept 1869 return value; 1870 case 3373707: // name 1871 this.name = castToString(value); // StringType 1872 return value; 1873 case -1285004149: // quantity 1874 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1875 return value; 1876 case -988474523: // managingEntity 1877 this.managingEntity = castToReference(value); // Reference 1878 return value; 1879 case 366313883: // characteristic 1880 this.getCharacteristic().add((GroupCharacteristicComponent) value); // GroupCharacteristicComponent 1881 return value; 1882 case -1077769574: // member 1883 this.getMember().add((GroupMemberComponent) value); // GroupMemberComponent 1884 return value; 1885 default: 1886 return super.setProperty(hash, name, value); 1887 } 1888 1889 } 1890 1891 @Override 1892 public Base setProperty(String name, Base value) throws FHIRException { 1893 if (name.equals("identifier")) { 1894 this.getIdentifier().add(castToIdentifier(value)); 1895 } else if (name.equals("active")) { 1896 this.active = castToBoolean(value); // BooleanType 1897 } else if (name.equals("type")) { 1898 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1899 this.type = (Enumeration) value; // Enumeration<GroupType> 1900 } else if (name.equals("actual")) { 1901 this.actual = castToBoolean(value); // BooleanType 1902 } else if (name.equals("code")) { 1903 this.code = castToCodeableConcept(value); // CodeableConcept 1904 } else if (name.equals("name")) { 1905 this.name = castToString(value); // StringType 1906 } else if (name.equals("quantity")) { 1907 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1908 } else if (name.equals("managingEntity")) { 1909 this.managingEntity = castToReference(value); // Reference 1910 } else if (name.equals("characteristic")) { 1911 this.getCharacteristic().add((GroupCharacteristicComponent) value); 1912 } else if (name.equals("member")) { 1913 this.getMember().add((GroupMemberComponent) value); 1914 } else 1915 return super.setProperty(name, value); 1916 return value; 1917 } 1918 1919 @Override 1920 public void removeChild(String name, Base value) throws FHIRException { 1921 if (name.equals("identifier")) { 1922 this.getIdentifier().remove(castToIdentifier(value)); 1923 } else if (name.equals("active")) { 1924 this.active = null; 1925 } else if (name.equals("type")) { 1926 this.type = null; 1927 } else if (name.equals("actual")) { 1928 this.actual = null; 1929 } else if (name.equals("code")) { 1930 this.code = null; 1931 } else if (name.equals("name")) { 1932 this.name = null; 1933 } else if (name.equals("quantity")) { 1934 this.quantity = null; 1935 } else if (name.equals("managingEntity")) { 1936 this.managingEntity = null; 1937 } else if (name.equals("characteristic")) { 1938 this.getCharacteristic().remove((GroupCharacteristicComponent) value); 1939 } else if (name.equals("member")) { 1940 this.getMember().remove((GroupMemberComponent) value); 1941 } else 1942 super.removeChild(name, value); 1943 1944 } 1945 1946 @Override 1947 public Base makeProperty(int hash, String name) throws FHIRException { 1948 switch (hash) { 1949 case -1618432855: 1950 return addIdentifier(); 1951 case -1422950650: 1952 return getActiveElement(); 1953 case 3575610: 1954 return getTypeElement(); 1955 case -1422939762: 1956 return getActualElement(); 1957 case 3059181: 1958 return getCode(); 1959 case 3373707: 1960 return getNameElement(); 1961 case -1285004149: 1962 return getQuantityElement(); 1963 case -988474523: 1964 return getManagingEntity(); 1965 case 366313883: 1966 return addCharacteristic(); 1967 case -1077769574: 1968 return addMember(); 1969 default: 1970 return super.makeProperty(hash, name); 1971 } 1972 1973 } 1974 1975 @Override 1976 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1977 switch (hash) { 1978 case -1618432855: 1979 /* identifier */ return new String[] { "Identifier" }; 1980 case -1422950650: 1981 /* active */ return new String[] { "boolean" }; 1982 case 3575610: 1983 /* type */ return new String[] { "code" }; 1984 case -1422939762: 1985 /* actual */ return new String[] { "boolean" }; 1986 case 3059181: 1987 /* code */ return new String[] { "CodeableConcept" }; 1988 case 3373707: 1989 /* name */ return new String[] { "string" }; 1990 case -1285004149: 1991 /* quantity */ return new String[] { "unsignedInt" }; 1992 case -988474523: 1993 /* managingEntity */ return new String[] { "Reference" }; 1994 case 366313883: 1995 /* characteristic */ return new String[] {}; 1996 case -1077769574: 1997 /* member */ return new String[] {}; 1998 default: 1999 return super.getTypesForProperty(hash, name); 2000 } 2001 2002 } 2003 2004 @Override 2005 public Base addChild(String name) throws FHIRException { 2006 if (name.equals("identifier")) { 2007 return addIdentifier(); 2008 } else if (name.equals("active")) { 2009 throw new FHIRException("Cannot call addChild on a singleton property Group.active"); 2010 } else if (name.equals("type")) { 2011 throw new FHIRException("Cannot call addChild on a singleton property Group.type"); 2012 } else if (name.equals("actual")) { 2013 throw new FHIRException("Cannot call addChild on a singleton property Group.actual"); 2014 } else if (name.equals("code")) { 2015 this.code = new CodeableConcept(); 2016 return this.code; 2017 } else if (name.equals("name")) { 2018 throw new FHIRException("Cannot call addChild on a singleton property Group.name"); 2019 } else if (name.equals("quantity")) { 2020 throw new FHIRException("Cannot call addChild on a singleton property Group.quantity"); 2021 } else if (name.equals("managingEntity")) { 2022 this.managingEntity = new Reference(); 2023 return this.managingEntity; 2024 } else if (name.equals("characteristic")) { 2025 return addCharacteristic(); 2026 } else if (name.equals("member")) { 2027 return addMember(); 2028 } else 2029 return super.addChild(name); 2030 } 2031 2032 public String fhirType() { 2033 return "Group"; 2034 2035 } 2036 2037 public Group copy() { 2038 Group dst = new Group(); 2039 copyValues(dst); 2040 return dst; 2041 } 2042 2043 public void copyValues(Group dst) { 2044 super.copyValues(dst); 2045 if (identifier != null) { 2046 dst.identifier = new ArrayList<Identifier>(); 2047 for (Identifier i : identifier) 2048 dst.identifier.add(i.copy()); 2049 } 2050 ; 2051 dst.active = active == null ? null : active.copy(); 2052 dst.type = type == null ? null : type.copy(); 2053 dst.actual = actual == null ? null : actual.copy(); 2054 dst.code = code == null ? null : code.copy(); 2055 dst.name = name == null ? null : name.copy(); 2056 dst.quantity = quantity == null ? null : quantity.copy(); 2057 dst.managingEntity = managingEntity == null ? null : managingEntity.copy(); 2058 if (characteristic != null) { 2059 dst.characteristic = new ArrayList<GroupCharacteristicComponent>(); 2060 for (GroupCharacteristicComponent i : characteristic) 2061 dst.characteristic.add(i.copy()); 2062 } 2063 ; 2064 if (member != null) { 2065 dst.member = new ArrayList<GroupMemberComponent>(); 2066 for (GroupMemberComponent i : member) 2067 dst.member.add(i.copy()); 2068 } 2069 ; 2070 } 2071 2072 protected Group typedCopy() { 2073 return copy(); 2074 } 2075 2076 @Override 2077 public boolean equalsDeep(Base other_) { 2078 if (!super.equalsDeep(other_)) 2079 return false; 2080 if (!(other_ instanceof Group)) 2081 return false; 2082 Group o = (Group) other_; 2083 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2084 && compareDeep(type, o.type, true) && compareDeep(actual, o.actual, true) && compareDeep(code, o.code, true) 2085 && compareDeep(name, o.name, true) && compareDeep(quantity, o.quantity, true) 2086 && compareDeep(managingEntity, o.managingEntity, true) && compareDeep(characteristic, o.characteristic, true) 2087 && compareDeep(member, o.member, true); 2088 } 2089 2090 @Override 2091 public boolean equalsShallow(Base other_) { 2092 if (!super.equalsShallow(other_)) 2093 return false; 2094 if (!(other_ instanceof Group)) 2095 return false; 2096 Group o = (Group) other_; 2097 return compareValues(active, o.active, true) && compareValues(type, o.type, true) 2098 && compareValues(actual, o.actual, true) && compareValues(name, o.name, true) 2099 && compareValues(quantity, o.quantity, true); 2100 } 2101 2102 public boolean isEmpty() { 2103 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type, actual, code, name, 2104 quantity, managingEntity, characteristic, member); 2105 } 2106 2107 @Override 2108 public ResourceType getResourceType() { 2109 return ResourceType.Group; 2110 } 2111 2112 /** 2113 * Search parameter: <b>actual</b> 2114 * <p> 2115 * Description: <b>Descriptive or actual</b><br> 2116 * Type: <b>token</b><br> 2117 * Path: <b>Group.actual</b><br> 2118 * </p> 2119 */ 2120 @SearchParamDefinition(name = "actual", path = "Group.actual", description = "Descriptive or actual", type = "token") 2121 public static final String SP_ACTUAL = "actual"; 2122 /** 2123 * <b>Fluent Client</b> search parameter constant for <b>actual</b> 2124 * <p> 2125 * Description: <b>Descriptive or actual</b><br> 2126 * Type: <b>token</b><br> 2127 * Path: <b>Group.actual</b><br> 2128 * </p> 2129 */ 2130 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUAL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2131 SP_ACTUAL); 2132 2133 /** 2134 * Search parameter: <b>identifier</b> 2135 * <p> 2136 * Description: <b>Unique id</b><br> 2137 * Type: <b>token</b><br> 2138 * Path: <b>Group.identifier</b><br> 2139 * </p> 2140 */ 2141 @SearchParamDefinition(name = "identifier", path = "Group.identifier", description = "Unique id", type = "token") 2142 public static final String SP_IDENTIFIER = "identifier"; 2143 /** 2144 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2145 * <p> 2146 * Description: <b>Unique id</b><br> 2147 * Type: <b>token</b><br> 2148 * Path: <b>Group.identifier</b><br> 2149 * </p> 2150 */ 2151 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2152 SP_IDENTIFIER); 2153 2154 /** 2155 * Search parameter: <b>characteristic-value</b> 2156 * <p> 2157 * Description: <b>A composite of both characteristic and value</b><br> 2158 * Type: <b>composite</b><br> 2159 * Path: <b></b><br> 2160 * </p> 2161 */ 2162 @SearchParamDefinition(name = "characteristic-value", path = "Group.characteristic", description = "A composite of both characteristic and value", type = "composite", compositeOf = { 2163 "characteristic", "value" }) 2164 public static final String SP_CHARACTERISTIC_VALUE = "characteristic-value"; 2165 /** 2166 * <b>Fluent Client</b> search parameter constant for 2167 * <b>characteristic-value</b> 2168 * <p> 2169 * Description: <b>A composite of both characteristic and value</b><br> 2170 * Type: <b>composite</b><br> 2171 * Path: <b></b><br> 2172 * </p> 2173 */ 2174 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CHARACTERISTIC_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2175 SP_CHARACTERISTIC_VALUE); 2176 2177 /** 2178 * Search parameter: <b>managing-entity</b> 2179 * <p> 2180 * Description: <b>Entity that is the custodian of the Group's 2181 * definition</b><br> 2182 * Type: <b>reference</b><br> 2183 * Path: <b>Group.managingEntity</b><br> 2184 * </p> 2185 */ 2186 @SearchParamDefinition(name = "managing-entity", path = "Group.managingEntity", description = "Entity that is the custodian of the Group's definition", type = "reference", target = { 2187 Organization.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2188 public static final String SP_MANAGING_ENTITY = "managing-entity"; 2189 /** 2190 * <b>Fluent Client</b> search parameter constant for <b>managing-entity</b> 2191 * <p> 2192 * Description: <b>Entity that is the custodian of the Group's 2193 * definition</b><br> 2194 * Type: <b>reference</b><br> 2195 * Path: <b>Group.managingEntity</b><br> 2196 * </p> 2197 */ 2198 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANAGING_ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2199 SP_MANAGING_ENTITY); 2200 2201 /** 2202 * Constant for fluent queries to be used to add include statements. Specifies 2203 * the path value of "<b>Group:managing-entity</b>". 2204 */ 2205 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANAGING_ENTITY = new ca.uhn.fhir.model.api.Include( 2206 "Group:managing-entity").toLocked(); 2207 2208 /** 2209 * Search parameter: <b>code</b> 2210 * <p> 2211 * Description: <b>The kind of resources contained</b><br> 2212 * Type: <b>token</b><br> 2213 * Path: <b>Group.code</b><br> 2214 * </p> 2215 */ 2216 @SearchParamDefinition(name = "code", path = "Group.code", description = "The kind of resources contained", type = "token") 2217 public static final String SP_CODE = "code"; 2218 /** 2219 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2220 * <p> 2221 * Description: <b>The kind of resources contained</b><br> 2222 * Type: <b>token</b><br> 2223 * Path: <b>Group.code</b><br> 2224 * </p> 2225 */ 2226 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2227 SP_CODE); 2228 2229 /** 2230 * Search parameter: <b>member</b> 2231 * <p> 2232 * Description: <b>Reference to the group member</b><br> 2233 * Type: <b>reference</b><br> 2234 * Path: <b>Group.member.entity</b><br> 2235 * </p> 2236 */ 2237 @SearchParamDefinition(name = "member", path = "Group.member.entity", description = "Reference to the group member", type = "reference", providesMembershipIn = { 2238 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2239 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2240 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 2241 Medication.class, Patient.class, Practitioner.class, PractitionerRole.class, Substance.class }) 2242 public static final String SP_MEMBER = "member"; 2243 /** 2244 * <b>Fluent Client</b> search parameter constant for <b>member</b> 2245 * <p> 2246 * Description: <b>Reference to the group member</b><br> 2247 * Type: <b>reference</b><br> 2248 * Path: <b>Group.member.entity</b><br> 2249 * </p> 2250 */ 2251 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2252 SP_MEMBER); 2253 2254 /** 2255 * Constant for fluent queries to be used to add include statements. Specifies 2256 * the path value of "<b>Group:member</b>". 2257 */ 2258 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEMBER = new ca.uhn.fhir.model.api.Include("Group:member") 2259 .toLocked(); 2260 2261 /** 2262 * Search parameter: <b>exclude</b> 2263 * <p> 2264 * Description: <b>Group includes or excludes</b><br> 2265 * Type: <b>token</b><br> 2266 * Path: <b>Group.characteristic.exclude</b><br> 2267 * </p> 2268 */ 2269 @SearchParamDefinition(name = "exclude", path = "Group.characteristic.exclude", description = "Group includes or excludes", type = "token") 2270 public static final String SP_EXCLUDE = "exclude"; 2271 /** 2272 * <b>Fluent Client</b> search parameter constant for <b>exclude</b> 2273 * <p> 2274 * Description: <b>Group includes or excludes</b><br> 2275 * Type: <b>token</b><br> 2276 * Path: <b>Group.characteristic.exclude</b><br> 2277 * </p> 2278 */ 2279 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXCLUDE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2280 SP_EXCLUDE); 2281 2282 /** 2283 * Search parameter: <b>type</b> 2284 * <p> 2285 * Description: <b>The type of resources the group contains</b><br> 2286 * Type: <b>token</b><br> 2287 * Path: <b>Group.type</b><br> 2288 * </p> 2289 */ 2290 @SearchParamDefinition(name = "type", path = "Group.type", description = "The type of resources the group contains", type = "token") 2291 public static final String SP_TYPE = "type"; 2292 /** 2293 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2294 * <p> 2295 * Description: <b>The type of resources the group contains</b><br> 2296 * Type: <b>token</b><br> 2297 * Path: <b>Group.type</b><br> 2298 * </p> 2299 */ 2300 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2301 SP_TYPE); 2302 2303 /** 2304 * Search parameter: <b>value</b> 2305 * <p> 2306 * Description: <b>Value held by characteristic</b><br> 2307 * Type: <b>token</b><br> 2308 * Path: <b>Group.characteristic.value[x]</b><br> 2309 * </p> 2310 */ 2311 @SearchParamDefinition(name = "value", path = "(Group.characteristic.value as CodeableConcept) | (Group.characteristic.value as boolean)", description = "Value held by characteristic", type = "token") 2312 public static final String SP_VALUE = "value"; 2313 /** 2314 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2315 * <p> 2316 * Description: <b>Value held by characteristic</b><br> 2317 * Type: <b>token</b><br> 2318 * Path: <b>Group.characteristic.value[x]</b><br> 2319 * </p> 2320 */ 2321 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2322 SP_VALUE); 2323 2324 /** 2325 * Search parameter: <b>characteristic</b> 2326 * <p> 2327 * Description: <b>Kind of characteristic</b><br> 2328 * Type: <b>token</b><br> 2329 * Path: <b>Group.characteristic.code</b><br> 2330 * </p> 2331 */ 2332 @SearchParamDefinition(name = "characteristic", path = "Group.characteristic.code", description = "Kind of characteristic", type = "token") 2333 public static final String SP_CHARACTERISTIC = "characteristic"; 2334 /** 2335 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2336 * <p> 2337 * Description: <b>Kind of characteristic</b><br> 2338 * Type: <b>token</b><br> 2339 * Path: <b>Group.characteristic.code</b><br> 2340 * </p> 2341 */ 2342 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2343 SP_CHARACTERISTIC); 2344 2345}