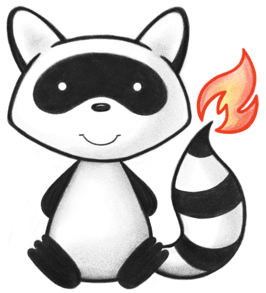
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The details of a healthcare service available at a location. 048 */ 049@ResourceDef(name = "HealthcareService", profile = "http://hl7.org/fhir/StructureDefinition/HealthcareService") 050public class HealthcareService extends DomainResource { 051 052 public enum DaysOfWeek { 053 /** 054 * Monday. 055 */ 056 MON, 057 /** 058 * Tuesday. 059 */ 060 TUE, 061 /** 062 * Wednesday. 063 */ 064 WED, 065 /** 066 * Thursday. 067 */ 068 THU, 069 /** 070 * Friday. 071 */ 072 FRI, 073 /** 074 * Saturday. 075 */ 076 SAT, 077 /** 078 * Sunday. 079 */ 080 SUN, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("mon".equals(codeString)) 090 return MON; 091 if ("tue".equals(codeString)) 092 return TUE; 093 if ("wed".equals(codeString)) 094 return WED; 095 if ("thu".equals(codeString)) 096 return THU; 097 if ("fri".equals(codeString)) 098 return FRI; 099 if ("sat".equals(codeString)) 100 return SAT; 101 if ("sun".equals(codeString)) 102 return SUN; 103 if (Configuration.isAcceptInvalidEnums()) 104 return null; 105 else 106 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case MON: 112 return "mon"; 113 case TUE: 114 return "tue"; 115 case WED: 116 return "wed"; 117 case THU: 118 return "thu"; 119 case FRI: 120 return "fri"; 121 case SAT: 122 return "sat"; 123 case SUN: 124 return "sun"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getSystem() { 133 switch (this) { 134 case MON: 135 return "http://hl7.org/fhir/days-of-week"; 136 case TUE: 137 return "http://hl7.org/fhir/days-of-week"; 138 case WED: 139 return "http://hl7.org/fhir/days-of-week"; 140 case THU: 141 return "http://hl7.org/fhir/days-of-week"; 142 case FRI: 143 return "http://hl7.org/fhir/days-of-week"; 144 case SAT: 145 return "http://hl7.org/fhir/days-of-week"; 146 case SUN: 147 return "http://hl7.org/fhir/days-of-week"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDefinition() { 156 switch (this) { 157 case MON: 158 return "Monday."; 159 case TUE: 160 return "Tuesday."; 161 case WED: 162 return "Wednesday."; 163 case THU: 164 return "Thursday."; 165 case FRI: 166 return "Friday."; 167 case SAT: 168 return "Saturday."; 169 case SUN: 170 return "Sunday."; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDisplay() { 179 switch (this) { 180 case MON: 181 return "Monday"; 182 case TUE: 183 return "Tuesday"; 184 case WED: 185 return "Wednesday"; 186 case THU: 187 return "Thursday"; 188 case FRI: 189 return "Friday"; 190 case SAT: 191 return "Saturday"; 192 case SUN: 193 return "Sunday"; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 } 201 202 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 203 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 204 if (codeString == null || "".equals(codeString)) 205 if (codeString == null || "".equals(codeString)) 206 return null; 207 if ("mon".equals(codeString)) 208 return DaysOfWeek.MON; 209 if ("tue".equals(codeString)) 210 return DaysOfWeek.TUE; 211 if ("wed".equals(codeString)) 212 return DaysOfWeek.WED; 213 if ("thu".equals(codeString)) 214 return DaysOfWeek.THU; 215 if ("fri".equals(codeString)) 216 return DaysOfWeek.FRI; 217 if ("sat".equals(codeString)) 218 return DaysOfWeek.SAT; 219 if ("sun".equals(codeString)) 220 return DaysOfWeek.SUN; 221 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 222 } 223 224 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 225 if (code == null) 226 return null; 227 if (code.isEmpty()) 228 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 229 String codeString = code.asStringValue(); 230 if (codeString == null || "".equals(codeString)) 231 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 232 if ("mon".equals(codeString)) 233 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON, code); 234 if ("tue".equals(codeString)) 235 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE, code); 236 if ("wed".equals(codeString)) 237 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED, code); 238 if ("thu".equals(codeString)) 239 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU, code); 240 if ("fri".equals(codeString)) 241 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI, code); 242 if ("sat".equals(codeString)) 243 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT, code); 244 if ("sun".equals(codeString)) 245 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN, code); 246 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 247 } 248 249 public String toCode(DaysOfWeek code) { 250 if (code == DaysOfWeek.NULL) 251 return null; 252 if (code == DaysOfWeek.MON) 253 return "mon"; 254 if (code == DaysOfWeek.TUE) 255 return "tue"; 256 if (code == DaysOfWeek.WED) 257 return "wed"; 258 if (code == DaysOfWeek.THU) 259 return "thu"; 260 if (code == DaysOfWeek.FRI) 261 return "fri"; 262 if (code == DaysOfWeek.SAT) 263 return "sat"; 264 if (code == DaysOfWeek.SUN) 265 return "sun"; 266 return "?"; 267 } 268 269 public String toSystem(DaysOfWeek code) { 270 return code.getSystem(); 271 } 272 } 273 274 @Block() 275 public static class HealthcareServiceEligibilityComponent extends BackboneElement implements IBaseBackboneElement { 276 /** 277 * Coded value for the eligibility. 278 */ 279 @Child(name = "code", type = { 280 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Coded value for the eligibility", formalDefinition = "Coded value for the eligibility.") 282 protected CodeableConcept code; 283 284 /** 285 * Describes the eligibility conditions for the service. 286 */ 287 @Child(name = "comment", type = { 288 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "Describes the eligibility conditions for the service", formalDefinition = "Describes the eligibility conditions for the service.") 290 protected MarkdownType comment; 291 292 private static final long serialVersionUID = 1078065348L; 293 294 /** 295 * Constructor 296 */ 297 public HealthcareServiceEligibilityComponent() { 298 super(); 299 } 300 301 /** 302 * @return {@link #code} (Coded value for the eligibility.) 303 */ 304 public CodeableConcept getCode() { 305 if (this.code == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create HealthcareServiceEligibilityComponent.code"); 308 else if (Configuration.doAutoCreate()) 309 this.code = new CodeableConcept(); // cc 310 return this.code; 311 } 312 313 public boolean hasCode() { 314 return this.code != null && !this.code.isEmpty(); 315 } 316 317 /** 318 * @param value {@link #code} (Coded value for the eligibility.) 319 */ 320 public HealthcareServiceEligibilityComponent setCode(CodeableConcept value) { 321 this.code = value; 322 return this; 323 } 324 325 /** 326 * @return {@link #comment} (Describes the eligibility conditions for the 327 * service.). This is the underlying object with id, value and 328 * extensions. The accessor "getComment" gives direct access to the 329 * value 330 */ 331 public MarkdownType getCommentElement() { 332 if (this.comment == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create HealthcareServiceEligibilityComponent.comment"); 335 else if (Configuration.doAutoCreate()) 336 this.comment = new MarkdownType(); // bb 337 return this.comment; 338 } 339 340 public boolean hasCommentElement() { 341 return this.comment != null && !this.comment.isEmpty(); 342 } 343 344 public boolean hasComment() { 345 return this.comment != null && !this.comment.isEmpty(); 346 } 347 348 /** 349 * @param value {@link #comment} (Describes the eligibility conditions for the 350 * service.). This is the underlying object with id, value and 351 * extensions. The accessor "getComment" gives direct access to the 352 * value 353 */ 354 public HealthcareServiceEligibilityComponent setCommentElement(MarkdownType value) { 355 this.comment = value; 356 return this; 357 } 358 359 /** 360 * @return Describes the eligibility conditions for the service. 361 */ 362 public String getComment() { 363 return this.comment == null ? null : this.comment.getValue(); 364 } 365 366 /** 367 * @param value Describes the eligibility conditions for the service. 368 */ 369 public HealthcareServiceEligibilityComponent setComment(String value) { 370 if (value == null) 371 this.comment = null; 372 else { 373 if (this.comment == null) 374 this.comment = new MarkdownType(); 375 this.comment.setValue(value); 376 } 377 return this; 378 } 379 380 protected void listChildren(List<Property> children) { 381 super.listChildren(children); 382 children.add(new Property("code", "CodeableConcept", "Coded value for the eligibility.", 0, 1, code)); 383 children.add( 384 new Property("comment", "markdown", "Describes the eligibility conditions for the service.", 0, 1, comment)); 385 } 386 387 @Override 388 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 389 switch (_hash) { 390 case 3059181: 391 /* code */ return new Property("code", "CodeableConcept", "Coded value for the eligibility.", 0, 1, code); 392 case 950398559: 393 /* comment */ return new Property("comment", "markdown", 394 "Describes the eligibility conditions for the service.", 0, 1, comment); 395 default: 396 return super.getNamedProperty(_hash, _name, _checkValid); 397 } 398 399 } 400 401 @Override 402 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 403 switch (hash) { 404 case 3059181: 405 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 406 case 950398559: 407 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // MarkdownType 408 default: 409 return super.getProperty(hash, name, checkValid); 410 } 411 412 } 413 414 @Override 415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 416 switch (hash) { 417 case 3059181: // code 418 this.code = castToCodeableConcept(value); // CodeableConcept 419 return value; 420 case 950398559: // comment 421 this.comment = castToMarkdown(value); // MarkdownType 422 return value; 423 default: 424 return super.setProperty(hash, name, value); 425 } 426 427 } 428 429 @Override 430 public Base setProperty(String name, Base value) throws FHIRException { 431 if (name.equals("code")) { 432 this.code = castToCodeableConcept(value); // CodeableConcept 433 } else if (name.equals("comment")) { 434 this.comment = castToMarkdown(value); // MarkdownType 435 } else 436 return super.setProperty(name, value); 437 return value; 438 } 439 440 @Override 441 public void removeChild(String name, Base value) throws FHIRException { 442 if (name.equals("code")) { 443 this.code = null; 444 } else if (name.equals("comment")) { 445 this.comment = null; 446 } else 447 super.removeChild(name, value); 448 449 } 450 451 @Override 452 public Base makeProperty(int hash, String name) throws FHIRException { 453 switch (hash) { 454 case 3059181: 455 return getCode(); 456 case 950398559: 457 return getCommentElement(); 458 default: 459 return super.makeProperty(hash, name); 460 } 461 462 } 463 464 @Override 465 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 466 switch (hash) { 467 case 3059181: 468 /* code */ return new String[] { "CodeableConcept" }; 469 case 950398559: 470 /* comment */ return new String[] { "markdown" }; 471 default: 472 return super.getTypesForProperty(hash, name); 473 } 474 475 } 476 477 @Override 478 public Base addChild(String name) throws FHIRException { 479 if (name.equals("code")) { 480 this.code = new CodeableConcept(); 481 return this.code; 482 } else if (name.equals("comment")) { 483 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 484 } else 485 return super.addChild(name); 486 } 487 488 public HealthcareServiceEligibilityComponent copy() { 489 HealthcareServiceEligibilityComponent dst = new HealthcareServiceEligibilityComponent(); 490 copyValues(dst); 491 return dst; 492 } 493 494 public void copyValues(HealthcareServiceEligibilityComponent dst) { 495 super.copyValues(dst); 496 dst.code = code == null ? null : code.copy(); 497 dst.comment = comment == null ? null : comment.copy(); 498 } 499 500 @Override 501 public boolean equalsDeep(Base other_) { 502 if (!super.equalsDeep(other_)) 503 return false; 504 if (!(other_ instanceof HealthcareServiceEligibilityComponent)) 505 return false; 506 HealthcareServiceEligibilityComponent o = (HealthcareServiceEligibilityComponent) other_; 507 return compareDeep(code, o.code, true) && compareDeep(comment, o.comment, true); 508 } 509 510 @Override 511 public boolean equalsShallow(Base other_) { 512 if (!super.equalsShallow(other_)) 513 return false; 514 if (!(other_ instanceof HealthcareServiceEligibilityComponent)) 515 return false; 516 HealthcareServiceEligibilityComponent o = (HealthcareServiceEligibilityComponent) other_; 517 return compareValues(comment, o.comment, true); 518 } 519 520 public boolean isEmpty() { 521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, comment); 522 } 523 524 public String fhirType() { 525 return "HealthcareService.eligibility"; 526 527 } 528 529 } 530 531 @Block() 532 public static class HealthcareServiceAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 533 /** 534 * Indicates which days of the week are available between the start and end 535 * Times. 536 */ 537 @Child(name = "daysOfWeek", type = { 538 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 539 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 540 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 541 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 542 543 /** 544 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 545 */ 546 @Child(name = "allDay", type = { 547 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 548 @Description(shortDefinition = "Always available? e.g. 24 hour service", formalDefinition = "Is this always available? (hence times are irrelevant) e.g. 24 hour service.") 549 protected BooleanType allDay; 550 551 /** 552 * The opening time of day. Note: If the AllDay flag is set, then this time is 553 * ignored. 554 */ 555 @Child(name = "availableStartTime", type = { 556 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 557 @Description(shortDefinition = "Opening time of day (ignored if allDay = true)", formalDefinition = "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.") 558 protected TimeType availableStartTime; 559 560 /** 561 * The closing time of day. Note: If the AllDay flag is set, then this time is 562 * ignored. 563 */ 564 @Child(name = "availableEndTime", type = { 565 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 566 @Description(shortDefinition = "Closing time of day (ignored if allDay = true)", formalDefinition = "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.") 567 protected TimeType availableEndTime; 568 569 private static final long serialVersionUID = -2139510127L; 570 571 /** 572 * Constructor 573 */ 574 public HealthcareServiceAvailableTimeComponent() { 575 super(); 576 } 577 578 /** 579 * @return {@link #daysOfWeek} (Indicates which days of the week are available 580 * between the start and end Times.) 581 */ 582 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 583 if (this.daysOfWeek == null) 584 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 585 return this.daysOfWeek; 586 } 587 588 /** 589 * @return Returns a reference to <code>this</code> for easy method chaining 590 */ 591 public HealthcareServiceAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 592 this.daysOfWeek = theDaysOfWeek; 593 return this; 594 } 595 596 public boolean hasDaysOfWeek() { 597 if (this.daysOfWeek == null) 598 return false; 599 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 600 if (!item.isEmpty()) 601 return true; 602 return false; 603 } 604 605 /** 606 * @return {@link #daysOfWeek} (Indicates which days of the week are available 607 * between the start and end Times.) 608 */ 609 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 610 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 611 if (this.daysOfWeek == null) 612 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 613 this.daysOfWeek.add(t); 614 return t; 615 } 616 617 /** 618 * @param value {@link #daysOfWeek} (Indicates which days of the week are 619 * available between the start and end Times.) 620 */ 621 public HealthcareServiceAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { // 1 622 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 623 t.setValue(value); 624 if (this.daysOfWeek == null) 625 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 626 this.daysOfWeek.add(t); 627 return this; 628 } 629 630 /** 631 * @param value {@link #daysOfWeek} (Indicates which days of the week are 632 * available between the start and end Times.) 633 */ 634 public boolean hasDaysOfWeek(DaysOfWeek value) { 635 if (this.daysOfWeek == null) 636 return false; 637 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 638 if (v.getValue().equals(value)) // code 639 return true; 640 return false; 641 } 642 643 /** 644 * @return {@link #allDay} (Is this always available? (hence times are 645 * irrelevant) e.g. 24 hour service.). This is the underlying object 646 * with id, value and extensions. The accessor "getAllDay" gives direct 647 * access to the value 648 */ 649 public BooleanType getAllDayElement() { 650 if (this.allDay == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.allDay"); 653 else if (Configuration.doAutoCreate()) 654 this.allDay = new BooleanType(); // bb 655 return this.allDay; 656 } 657 658 public boolean hasAllDayElement() { 659 return this.allDay != null && !this.allDay.isEmpty(); 660 } 661 662 public boolean hasAllDay() { 663 return this.allDay != null && !this.allDay.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #allDay} (Is this always available? (hence times are 668 * irrelevant) e.g. 24 hour service.). This is the underlying 669 * object with id, value and extensions. The accessor "getAllDay" 670 * gives direct access to the value 671 */ 672 public HealthcareServiceAvailableTimeComponent setAllDayElement(BooleanType value) { 673 this.allDay = value; 674 return this; 675 } 676 677 /** 678 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour 679 * service. 680 */ 681 public boolean getAllDay() { 682 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 683 } 684 685 /** 686 * @param value Is this always available? (hence times are irrelevant) e.g. 24 687 * hour service. 688 */ 689 public HealthcareServiceAvailableTimeComponent setAllDay(boolean value) { 690 if (this.allDay == null) 691 this.allDay = new BooleanType(); 692 this.allDay.setValue(value); 693 return this; 694 } 695 696 /** 697 * @return {@link #availableStartTime} (The opening time of day. Note: If the 698 * AllDay flag is set, then this time is ignored.). This is the 699 * underlying object with id, value and extensions. The accessor 700 * "getAvailableStartTime" gives direct access to the value 701 */ 702 public TimeType getAvailableStartTimeElement() { 703 if (this.availableStartTime == null) 704 if (Configuration.errorOnAutoCreate()) 705 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableStartTime"); 706 else if (Configuration.doAutoCreate()) 707 this.availableStartTime = new TimeType(); // bb 708 return this.availableStartTime; 709 } 710 711 public boolean hasAvailableStartTimeElement() { 712 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 713 } 714 715 public boolean hasAvailableStartTime() { 716 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 717 } 718 719 /** 720 * @param value {@link #availableStartTime} (The opening time of day. Note: If 721 * the AllDay flag is set, then this time is ignored.). This is the 722 * underlying object with id, value and extensions. The accessor 723 * "getAvailableStartTime" gives direct access to the value 724 */ 725 public HealthcareServiceAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 726 this.availableStartTime = value; 727 return this; 728 } 729 730 /** 731 * @return The opening time of day. Note: If the AllDay flag is set, then this 732 * time is ignored. 733 */ 734 public String getAvailableStartTime() { 735 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 736 } 737 738 /** 739 * @param value The opening time of day. Note: If the AllDay flag is set, then 740 * this time is ignored. 741 */ 742 public HealthcareServiceAvailableTimeComponent setAvailableStartTime(String value) { 743 if (value == null) 744 this.availableStartTime = null; 745 else { 746 if (this.availableStartTime == null) 747 this.availableStartTime = new TimeType(); 748 this.availableStartTime.setValue(value); 749 } 750 return this; 751 } 752 753 /** 754 * @return {@link #availableEndTime} (The closing time of day. Note: If the 755 * AllDay flag is set, then this time is ignored.). This is the 756 * underlying object with id, value and extensions. The accessor 757 * "getAvailableEndTime" gives direct access to the value 758 */ 759 public TimeType getAvailableEndTimeElement() { 760 if (this.availableEndTime == null) 761 if (Configuration.errorOnAutoCreate()) 762 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableEndTime"); 763 else if (Configuration.doAutoCreate()) 764 this.availableEndTime = new TimeType(); // bb 765 return this.availableEndTime; 766 } 767 768 public boolean hasAvailableEndTimeElement() { 769 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 770 } 771 772 public boolean hasAvailableEndTime() { 773 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 774 } 775 776 /** 777 * @param value {@link #availableEndTime} (The closing time of day. Note: If the 778 * AllDay flag is set, then this time is ignored.). This is the 779 * underlying object with id, value and extensions. The accessor 780 * "getAvailableEndTime" gives direct access to the value 781 */ 782 public HealthcareServiceAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 783 this.availableEndTime = value; 784 return this; 785 } 786 787 /** 788 * @return The closing time of day. Note: If the AllDay flag is set, then this 789 * time is ignored. 790 */ 791 public String getAvailableEndTime() { 792 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 793 } 794 795 /** 796 * @param value The closing time of day. Note: If the AllDay flag is set, then 797 * this time is ignored. 798 */ 799 public HealthcareServiceAvailableTimeComponent setAvailableEndTime(String value) { 800 if (value == null) 801 this.availableEndTime = null; 802 else { 803 if (this.availableEndTime == null) 804 this.availableEndTime = new TimeType(); 805 this.availableEndTime.setValue(value); 806 } 807 return this; 808 } 809 810 protected void listChildren(List<Property> children) { 811 super.listChildren(children); 812 children.add(new Property("daysOfWeek", "code", 813 "Indicates which days of the week are available between the start and end Times.", 0, 814 java.lang.Integer.MAX_VALUE, daysOfWeek)); 815 children.add(new Property("allDay", "boolean", 816 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 817 children.add(new Property("availableStartTime", "time", 818 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 819 availableStartTime)); 820 children.add(new Property("availableEndTime", "time", 821 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 822 availableEndTime)); 823 } 824 825 @Override 826 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 827 switch (_hash) { 828 case 68050338: 829 /* daysOfWeek */ return new Property("daysOfWeek", "code", 830 "Indicates which days of the week are available between the start and end Times.", 0, 831 java.lang.Integer.MAX_VALUE, daysOfWeek); 832 case -1414913477: 833 /* allDay */ return new Property("allDay", "boolean", 834 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 835 case -1039453818: 836 /* availableStartTime */ return new Property("availableStartTime", "time", 837 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 838 availableStartTime); 839 case 101151551: 840 /* availableEndTime */ return new Property("availableEndTime", "time", 841 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 842 availableEndTime); 843 default: 844 return super.getNamedProperty(_hash, _name, _checkValid); 845 } 846 847 } 848 849 @Override 850 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 851 switch (hash) { 852 case 68050338: 853 /* daysOfWeek */ return this.daysOfWeek == null ? new Base[0] 854 : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 855 case -1414913477: 856 /* allDay */ return this.allDay == null ? new Base[0] : new Base[] { this.allDay }; // BooleanType 857 case -1039453818: 858 /* availableStartTime */ return this.availableStartTime == null ? new Base[0] 859 : new Base[] { this.availableStartTime }; // TimeType 860 case 101151551: 861 /* availableEndTime */ return this.availableEndTime == null ? new Base[0] 862 : new Base[] { this.availableEndTime }; // TimeType 863 default: 864 return super.getProperty(hash, name, checkValid); 865 } 866 867 } 868 869 @Override 870 public Base setProperty(int hash, String name, Base value) throws FHIRException { 871 switch (hash) { 872 case 68050338: // daysOfWeek 873 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 874 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 875 return value; 876 case -1414913477: // allDay 877 this.allDay = castToBoolean(value); // BooleanType 878 return value; 879 case -1039453818: // availableStartTime 880 this.availableStartTime = castToTime(value); // TimeType 881 return value; 882 case 101151551: // availableEndTime 883 this.availableEndTime = castToTime(value); // TimeType 884 return value; 885 default: 886 return super.setProperty(hash, name, value); 887 } 888 889 } 890 891 @Override 892 public Base setProperty(String name, Base value) throws FHIRException { 893 if (name.equals("daysOfWeek")) { 894 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 895 this.getDaysOfWeek().add((Enumeration) value); 896 } else if (name.equals("allDay")) { 897 this.allDay = castToBoolean(value); // BooleanType 898 } else if (name.equals("availableStartTime")) { 899 this.availableStartTime = castToTime(value); // TimeType 900 } else if (name.equals("availableEndTime")) { 901 this.availableEndTime = castToTime(value); // TimeType 902 } else 903 return super.setProperty(name, value); 904 return value; 905 } 906 907 @Override 908 public void removeChild(String name, Base value) throws FHIRException { 909 if (name.equals("daysOfWeek")) { 910 this.getDaysOfWeek().remove((Enumeration) value); 911 } else if (name.equals("allDay")) { 912 this.allDay = null; 913 } else if (name.equals("availableStartTime")) { 914 this.availableStartTime = null; 915 } else if (name.equals("availableEndTime")) { 916 this.availableEndTime = null; 917 } else 918 super.removeChild(name, value); 919 920 } 921 922 @Override 923 public Base makeProperty(int hash, String name) throws FHIRException { 924 switch (hash) { 925 case 68050338: 926 return addDaysOfWeekElement(); 927 case -1414913477: 928 return getAllDayElement(); 929 case -1039453818: 930 return getAvailableStartTimeElement(); 931 case 101151551: 932 return getAvailableEndTimeElement(); 933 default: 934 return super.makeProperty(hash, name); 935 } 936 937 } 938 939 @Override 940 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 941 switch (hash) { 942 case 68050338: 943 /* daysOfWeek */ return new String[] { "code" }; 944 case -1414913477: 945 /* allDay */ return new String[] { "boolean" }; 946 case -1039453818: 947 /* availableStartTime */ return new String[] { "time" }; 948 case 101151551: 949 /* availableEndTime */ return new String[] { "time" }; 950 default: 951 return super.getTypesForProperty(hash, name); 952 } 953 954 } 955 956 @Override 957 public Base addChild(String name) throws FHIRException { 958 if (name.equals("daysOfWeek")) { 959 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.daysOfWeek"); 960 } else if (name.equals("allDay")) { 961 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.allDay"); 962 } else if (name.equals("availableStartTime")) { 963 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableStartTime"); 964 } else if (name.equals("availableEndTime")) { 965 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableEndTime"); 966 } else 967 return super.addChild(name); 968 } 969 970 public HealthcareServiceAvailableTimeComponent copy() { 971 HealthcareServiceAvailableTimeComponent dst = new HealthcareServiceAvailableTimeComponent(); 972 copyValues(dst); 973 return dst; 974 } 975 976 public void copyValues(HealthcareServiceAvailableTimeComponent dst) { 977 super.copyValues(dst); 978 if (daysOfWeek != null) { 979 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 980 for (Enumeration<DaysOfWeek> i : daysOfWeek) 981 dst.daysOfWeek.add(i.copy()); 982 } 983 ; 984 dst.allDay = allDay == null ? null : allDay.copy(); 985 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 986 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 987 } 988 989 @Override 990 public boolean equalsDeep(Base other_) { 991 if (!super.equalsDeep(other_)) 992 return false; 993 if (!(other_ instanceof HealthcareServiceAvailableTimeComponent)) 994 return false; 995 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other_; 996 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 997 && compareDeep(availableStartTime, o.availableStartTime, true) 998 && compareDeep(availableEndTime, o.availableEndTime, true); 999 } 1000 1001 @Override 1002 public boolean equalsShallow(Base other_) { 1003 if (!super.equalsShallow(other_)) 1004 return false; 1005 if (!(other_ instanceof HealthcareServiceAvailableTimeComponent)) 1006 return false; 1007 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other_; 1008 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 1009 && compareValues(availableStartTime, o.availableStartTime, true) 1010 && compareValues(availableEndTime, o.availableEndTime, true); 1011 } 1012 1013 public boolean isEmpty() { 1014 return super.isEmpty() 1015 && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime, availableEndTime); 1016 } 1017 1018 public String fhirType() { 1019 return "HealthcareService.availableTime"; 1020 1021 } 1022 1023 } 1024 1025 @Block() 1026 public static class HealthcareServiceNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 1027 /** 1028 * The reason that can be presented to the user as to why this time is not 1029 * available. 1030 */ 1031 @Child(name = "description", type = { 1032 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1033 @Description(shortDefinition = "Reason presented to the user explaining why time not available", formalDefinition = "The reason that can be presented to the user as to why this time is not available.") 1034 protected StringType description; 1035 1036 /** 1037 * Service is not available (seasonally or for a public holiday) from this date. 1038 */ 1039 @Child(name = "during", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1040 @Description(shortDefinition = "Service not available from this date", formalDefinition = "Service is not available (seasonally or for a public holiday) from this date.") 1041 protected Period during; 1042 1043 private static final long serialVersionUID = 310849929L; 1044 1045 /** 1046 * Constructor 1047 */ 1048 public HealthcareServiceNotAvailableComponent() { 1049 super(); 1050 } 1051 1052 /** 1053 * Constructor 1054 */ 1055 public HealthcareServiceNotAvailableComponent(StringType description) { 1056 super(); 1057 this.description = description; 1058 } 1059 1060 /** 1061 * @return {@link #description} (The reason that can be presented to the user as 1062 * to why this time is not available.). This is the underlying object 1063 * with id, value and extensions. The accessor "getDescription" gives 1064 * direct access to the value 1065 */ 1066 public StringType getDescriptionElement() { 1067 if (this.description == null) 1068 if (Configuration.errorOnAutoCreate()) 1069 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.description"); 1070 else if (Configuration.doAutoCreate()) 1071 this.description = new StringType(); // bb 1072 return this.description; 1073 } 1074 1075 public boolean hasDescriptionElement() { 1076 return this.description != null && !this.description.isEmpty(); 1077 } 1078 1079 public boolean hasDescription() { 1080 return this.description != null && !this.description.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #description} (The reason that can be presented to the 1085 * user as to why this time is not available.). This is the 1086 * underlying object with id, value and extensions. The accessor 1087 * "getDescription" gives direct access to the value 1088 */ 1089 public HealthcareServiceNotAvailableComponent setDescriptionElement(StringType value) { 1090 this.description = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return The reason that can be presented to the user as to why this time is 1096 * not available. 1097 */ 1098 public String getDescription() { 1099 return this.description == null ? null : this.description.getValue(); 1100 } 1101 1102 /** 1103 * @param value The reason that can be presented to the user as to why this time 1104 * is not available. 1105 */ 1106 public HealthcareServiceNotAvailableComponent setDescription(String value) { 1107 if (this.description == null) 1108 this.description = new StringType(); 1109 this.description.setValue(value); 1110 return this; 1111 } 1112 1113 /** 1114 * @return {@link #during} (Service is not available (seasonally or for a public 1115 * holiday) from this date.) 1116 */ 1117 public Period getDuring() { 1118 if (this.during == null) 1119 if (Configuration.errorOnAutoCreate()) 1120 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.during"); 1121 else if (Configuration.doAutoCreate()) 1122 this.during = new Period(); // cc 1123 return this.during; 1124 } 1125 1126 public boolean hasDuring() { 1127 return this.during != null && !this.during.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #during} (Service is not available (seasonally or for a 1132 * public holiday) from this date.) 1133 */ 1134 public HealthcareServiceNotAvailableComponent setDuring(Period value) { 1135 this.during = value; 1136 return this; 1137 } 1138 1139 protected void listChildren(List<Property> children) { 1140 super.listChildren(children); 1141 children.add(new Property("description", "string", 1142 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 1143 children.add(new Property("during", "Period", 1144 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 1145 } 1146 1147 @Override 1148 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1149 switch (_hash) { 1150 case -1724546052: 1151 /* description */ return new Property("description", "string", 1152 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 1153 case -1320499647: 1154 /* during */ return new Property("during", "Period", 1155 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 1156 default: 1157 return super.getNamedProperty(_hash, _name, _checkValid); 1158 } 1159 1160 } 1161 1162 @Override 1163 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1164 switch (hash) { 1165 case -1724546052: 1166 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1167 case -1320499647: 1168 /* during */ return this.during == null ? new Base[0] : new Base[] { this.during }; // Period 1169 default: 1170 return super.getProperty(hash, name, checkValid); 1171 } 1172 1173 } 1174 1175 @Override 1176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1177 switch (hash) { 1178 case -1724546052: // description 1179 this.description = castToString(value); // StringType 1180 return value; 1181 case -1320499647: // during 1182 this.during = castToPeriod(value); // Period 1183 return value; 1184 default: 1185 return super.setProperty(hash, name, value); 1186 } 1187 1188 } 1189 1190 @Override 1191 public Base setProperty(String name, Base value) throws FHIRException { 1192 if (name.equals("description")) { 1193 this.description = castToString(value); // StringType 1194 } else if (name.equals("during")) { 1195 this.during = castToPeriod(value); // Period 1196 } else 1197 return super.setProperty(name, value); 1198 return value; 1199 } 1200 1201 @Override 1202 public void removeChild(String name, Base value) throws FHIRException { 1203 if (name.equals("description")) { 1204 this.description = null; 1205 } else if (name.equals("during")) { 1206 this.during = null; 1207 } else 1208 super.removeChild(name, value); 1209 1210 } 1211 1212 @Override 1213 public Base makeProperty(int hash, String name) throws FHIRException { 1214 switch (hash) { 1215 case -1724546052: 1216 return getDescriptionElement(); 1217 case -1320499647: 1218 return getDuring(); 1219 default: 1220 return super.makeProperty(hash, name); 1221 } 1222 1223 } 1224 1225 @Override 1226 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1227 switch (hash) { 1228 case -1724546052: 1229 /* description */ return new String[] { "string" }; 1230 case -1320499647: 1231 /* during */ return new String[] { "Period" }; 1232 default: 1233 return super.getTypesForProperty(hash, name); 1234 } 1235 1236 } 1237 1238 @Override 1239 public Base addChild(String name) throws FHIRException { 1240 if (name.equals("description")) { 1241 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.description"); 1242 } else if (name.equals("during")) { 1243 this.during = new Period(); 1244 return this.during; 1245 } else 1246 return super.addChild(name); 1247 } 1248 1249 public HealthcareServiceNotAvailableComponent copy() { 1250 HealthcareServiceNotAvailableComponent dst = new HealthcareServiceNotAvailableComponent(); 1251 copyValues(dst); 1252 return dst; 1253 } 1254 1255 public void copyValues(HealthcareServiceNotAvailableComponent dst) { 1256 super.copyValues(dst); 1257 dst.description = description == null ? null : description.copy(); 1258 dst.during = during == null ? null : during.copy(); 1259 } 1260 1261 @Override 1262 public boolean equalsDeep(Base other_) { 1263 if (!super.equalsDeep(other_)) 1264 return false; 1265 if (!(other_ instanceof HealthcareServiceNotAvailableComponent)) 1266 return false; 1267 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other_; 1268 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 1269 } 1270 1271 @Override 1272 public boolean equalsShallow(Base other_) { 1273 if (!super.equalsShallow(other_)) 1274 return false; 1275 if (!(other_ instanceof HealthcareServiceNotAvailableComponent)) 1276 return false; 1277 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other_; 1278 return compareValues(description, o.description, true); 1279 } 1280 1281 public boolean isEmpty() { 1282 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 1283 } 1284 1285 public String fhirType() { 1286 return "HealthcareService.notAvailable"; 1287 1288 } 1289 1290 } 1291 1292 /** 1293 * External identifiers for this item. 1294 */ 1295 @Child(name = "identifier", type = { 1296 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1297 @Description(shortDefinition = "External identifiers for this item", formalDefinition = "External identifiers for this item.") 1298 protected List<Identifier> identifier; 1299 1300 /** 1301 * This flag is used to mark the record to not be used. This is not used when a 1302 * center is closed for maintenance, or for holidays, the notAvailable period is 1303 * to be used for this. 1304 */ 1305 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1306 @Description(shortDefinition = "Whether this HealthcareService record is in active use", formalDefinition = "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.") 1307 protected BooleanType active; 1308 1309 /** 1310 * The organization that provides this healthcare service. 1311 */ 1312 @Child(name = "providedBy", type = { 1313 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1314 @Description(shortDefinition = "Organization that provides this service", formalDefinition = "The organization that provides this healthcare service.") 1315 protected Reference providedBy; 1316 1317 /** 1318 * The actual object that is the target of the reference (The organization that 1319 * provides this healthcare service.) 1320 */ 1321 protected Organization providedByTarget; 1322 1323 /** 1324 * Identifies the broad category of service being performed or delivered. 1325 */ 1326 @Child(name = "category", type = { 1327 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1328 @Description(shortDefinition = "Broad category of service being performed or delivered", formalDefinition = "Identifies the broad category of service being performed or delivered.") 1329 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 1330 protected List<CodeableConcept> category; 1331 1332 /** 1333 * The specific type of service that may be delivered or performed. 1334 */ 1335 @Child(name = "type", type = { 1336 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1337 @Description(shortDefinition = "Type of service that may be delivered or performed", formalDefinition = "The specific type of service that may be delivered or performed.") 1338 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 1339 protected List<CodeableConcept> type; 1340 1341 /** 1342 * Collection of specialties handled by the service site. This is more of a 1343 * medical term. 1344 */ 1345 @Child(name = "specialty", type = { 1346 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1347 @Description(shortDefinition = "Specialties handled by the HealthcareService", formalDefinition = "Collection of specialties handled by the service site. This is more of a medical term.") 1348 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 1349 protected List<CodeableConcept> specialty; 1350 1351 /** 1352 * The location(s) where this healthcare service may be provided. 1353 */ 1354 @Child(name = "location", type = { 1355 Location.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1356 @Description(shortDefinition = "Location(s) where service may be provided", formalDefinition = "The location(s) where this healthcare service may be provided.") 1357 protected List<Reference> location; 1358 /** 1359 * The actual objects that are the target of the reference (The location(s) 1360 * where this healthcare service may be provided.) 1361 */ 1362 protected List<Location> locationTarget; 1363 1364 /** 1365 * Further description of the service as it would be presented to a consumer 1366 * while searching. 1367 */ 1368 @Child(name = "name", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1369 @Description(shortDefinition = "Description of service as presented to a consumer while searching", formalDefinition = "Further description of the service as it would be presented to a consumer while searching.") 1370 protected StringType name; 1371 1372 /** 1373 * Any additional description of the service and/or any specific issues not 1374 * covered by the other attributes, which can be displayed as further detail 1375 * under the serviceName. 1376 */ 1377 @Child(name = "comment", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1378 @Description(shortDefinition = "Additional description and/or any specific issues not covered elsewhere", formalDefinition = "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.") 1379 protected StringType comment; 1380 1381 /** 1382 * Extra details about the service that can't be placed in the other fields. 1383 */ 1384 @Child(name = "extraDetails", type = { 1385 MarkdownType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1386 @Description(shortDefinition = "Extra details about the service that can't be placed in the other fields", formalDefinition = "Extra details about the service that can't be placed in the other fields.") 1387 protected MarkdownType extraDetails; 1388 1389 /** 1390 * If there is a photo/symbol associated with this HealthcareService, it may be 1391 * included here to facilitate quick identification of the service in a list. 1392 */ 1393 @Child(name = "photo", type = { Attachment.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1394 @Description(shortDefinition = "Facilitates quick identification of the service", formalDefinition = "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.") 1395 protected Attachment photo; 1396 1397 /** 1398 * List of contacts related to this specific healthcare service. 1399 */ 1400 @Child(name = "telecom", type = { 1401 ContactPoint.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1402 @Description(shortDefinition = "Contacts related to the healthcare service", formalDefinition = "List of contacts related to this specific healthcare service.") 1403 protected List<ContactPoint> telecom; 1404 1405 /** 1406 * The location(s) that this service is available to (not where the service is 1407 * provided). 1408 */ 1409 @Child(name = "coverageArea", type = { 1410 Location.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1411 @Description(shortDefinition = "Location(s) service is intended for/available to", formalDefinition = "The location(s) that this service is available to (not where the service is provided).") 1412 protected List<Reference> coverageArea; 1413 /** 1414 * The actual objects that are the target of the reference (The location(s) that 1415 * this service is available to (not where the service is provided).) 1416 */ 1417 protected List<Location> coverageAreaTarget; 1418 1419 /** 1420 * The code(s) that detail the conditions under which the healthcare service is 1421 * available/offered. 1422 */ 1423 @Child(name = "serviceProvisionCode", type = { 1424 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1425 @Description(shortDefinition = "Conditions under which service is available/offered", formalDefinition = "The code(s) that detail the conditions under which the healthcare service is available/offered.") 1426 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-provision-conditions") 1427 protected List<CodeableConcept> serviceProvisionCode; 1428 1429 /** 1430 * Does this service have specific eligibility requirements that need to be met 1431 * in order to use the service? 1432 */ 1433 @Child(name = "eligibility", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1434 @Description(shortDefinition = "Specific eligibility requirements required to use the service", formalDefinition = "Does this service have specific eligibility requirements that need to be met in order to use the service?") 1435 protected List<HealthcareServiceEligibilityComponent> eligibility; 1436 1437 /** 1438 * Programs that this service is applicable to. 1439 */ 1440 @Child(name = "program", type = { 1441 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1442 @Description(shortDefinition = "Programs that this service is applicable to", formalDefinition = "Programs that this service is applicable to.") 1443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/program") 1444 protected List<CodeableConcept> program; 1445 1446 /** 1447 * Collection of characteristics (attributes). 1448 */ 1449 @Child(name = "characteristic", type = { 1450 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1451 @Description(shortDefinition = "Collection of characteristics (attributes)", formalDefinition = "Collection of characteristics (attributes).") 1452 protected List<CodeableConcept> characteristic; 1453 1454 /** 1455 * Some services are specifically made available in multiple languages, this 1456 * property permits a directory to declare the languages this is offered in. 1457 * Typically this is only provided where a service operates in communities with 1458 * mixed languages used. 1459 */ 1460 @Child(name = "communication", type = { 1461 CodeableConcept.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1462 @Description(shortDefinition = "The language that this service is offered in", formalDefinition = "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.") 1463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 1464 protected List<CodeableConcept> communication; 1465 1466 /** 1467 * Ways that the service accepts referrals, if this is not provided then it is 1468 * implied that no referral is required. 1469 */ 1470 @Child(name = "referralMethod", type = { 1471 CodeableConcept.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1472 @Description(shortDefinition = "Ways that the service accepts referrals", formalDefinition = "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.") 1473 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-referral-method") 1474 protected List<CodeableConcept> referralMethod; 1475 1476 /** 1477 * Indicates whether or not a prospective consumer will require an appointment 1478 * for a particular service at a site to be provided by the Organization. 1479 * Indicates if an appointment is required for access to this service. 1480 */ 1481 @Child(name = "appointmentRequired", type = { 1482 BooleanType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 1483 @Description(shortDefinition = "If an appointment is required for access to this service", formalDefinition = "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.") 1484 protected BooleanType appointmentRequired; 1485 1486 /** 1487 * A collection of times that the Service Site is available. 1488 */ 1489 @Child(name = "availableTime", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1490 @Description(shortDefinition = "Times the Service Site is available", formalDefinition = "A collection of times that the Service Site is available.") 1491 protected List<HealthcareServiceAvailableTimeComponent> availableTime; 1492 1493 /** 1494 * The HealthcareService is not available during this period of time due to the 1495 * provided reason. 1496 */ 1497 @Child(name = "notAvailable", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1498 @Description(shortDefinition = "Not available during this time due to provided reason", formalDefinition = "The HealthcareService is not available during this period of time due to the provided reason.") 1499 protected List<HealthcareServiceNotAvailableComponent> notAvailable; 1500 1501 /** 1502 * A description of site availability exceptions, e.g. public holiday 1503 * availability. Succinctly describing all possible exceptions to normal site 1504 * availability as details in the available Times and not available Times. 1505 */ 1506 @Child(name = "availabilityExceptions", type = { 1507 StringType.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 1508 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.") 1509 protected StringType availabilityExceptions; 1510 1511 /** 1512 * Technical endpoints providing access to services operated for the specific 1513 * healthcare services defined at this resource. 1514 */ 1515 @Child(name = "endpoint", type = { 1516 Endpoint.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1517 @Description(shortDefinition = "Technical endpoints providing access to electronic services operated for the healthcare service", formalDefinition = "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.") 1518 protected List<Reference> endpoint; 1519 /** 1520 * The actual objects that are the target of the reference (Technical endpoints 1521 * providing access to services operated for the specific healthcare services 1522 * defined at this resource.) 1523 */ 1524 protected List<Endpoint> endpointTarget; 1525 1526 private static final long serialVersionUID = -2002412666L; 1527 1528 /** 1529 * Constructor 1530 */ 1531 public HealthcareService() { 1532 super(); 1533 } 1534 1535 /** 1536 * @return {@link #identifier} (External identifiers for this item.) 1537 */ 1538 public List<Identifier> getIdentifier() { 1539 if (this.identifier == null) 1540 this.identifier = new ArrayList<Identifier>(); 1541 return this.identifier; 1542 } 1543 1544 /** 1545 * @return Returns a reference to <code>this</code> for easy method chaining 1546 */ 1547 public HealthcareService setIdentifier(List<Identifier> theIdentifier) { 1548 this.identifier = theIdentifier; 1549 return this; 1550 } 1551 1552 public boolean hasIdentifier() { 1553 if (this.identifier == null) 1554 return false; 1555 for (Identifier item : this.identifier) 1556 if (!item.isEmpty()) 1557 return true; 1558 return false; 1559 } 1560 1561 public Identifier addIdentifier() { // 3 1562 Identifier t = new Identifier(); 1563 if (this.identifier == null) 1564 this.identifier = new ArrayList<Identifier>(); 1565 this.identifier.add(t); 1566 return t; 1567 } 1568 1569 public HealthcareService addIdentifier(Identifier t) { // 3 1570 if (t == null) 1571 return this; 1572 if (this.identifier == null) 1573 this.identifier = new ArrayList<Identifier>(); 1574 this.identifier.add(t); 1575 return this; 1576 } 1577 1578 /** 1579 * @return The first repetition of repeating field {@link #identifier}, creating 1580 * it if it does not already exist 1581 */ 1582 public Identifier getIdentifierFirstRep() { 1583 if (getIdentifier().isEmpty()) { 1584 addIdentifier(); 1585 } 1586 return getIdentifier().get(0); 1587 } 1588 1589 /** 1590 * @return {@link #active} (This flag is used to mark the record to not be used. 1591 * This is not used when a center is closed for maintenance, or for 1592 * holidays, the notAvailable period is to be used for this.). This is 1593 * the underlying object with id, value and extensions. The accessor 1594 * "getActive" gives direct access to the value 1595 */ 1596 public BooleanType getActiveElement() { 1597 if (this.active == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create HealthcareService.active"); 1600 else if (Configuration.doAutoCreate()) 1601 this.active = new BooleanType(); // bb 1602 return this.active; 1603 } 1604 1605 public boolean hasActiveElement() { 1606 return this.active != null && !this.active.isEmpty(); 1607 } 1608 1609 public boolean hasActive() { 1610 return this.active != null && !this.active.isEmpty(); 1611 } 1612 1613 /** 1614 * @param value {@link #active} (This flag is used to mark the record to not be 1615 * used. This is not used when a center is closed for maintenance, 1616 * or for holidays, the notAvailable period is to be used for 1617 * this.). This is the underlying object with id, value and 1618 * extensions. The accessor "getActive" gives direct access to the 1619 * value 1620 */ 1621 public HealthcareService setActiveElement(BooleanType value) { 1622 this.active = value; 1623 return this; 1624 } 1625 1626 /** 1627 * @return This flag is used to mark the record to not be used. This is not used 1628 * when a center is closed for maintenance, or for holidays, the 1629 * notAvailable period is to be used for this. 1630 */ 1631 public boolean getActive() { 1632 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1633 } 1634 1635 /** 1636 * @param value This flag is used to mark the record to not be used. This is not 1637 * used when a center is closed for maintenance, or for holidays, 1638 * the notAvailable period is to be used for this. 1639 */ 1640 public HealthcareService setActive(boolean value) { 1641 if (this.active == null) 1642 this.active = new BooleanType(); 1643 this.active.setValue(value); 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #providedBy} (The organization that provides this healthcare 1649 * service.) 1650 */ 1651 public Reference getProvidedBy() { 1652 if (this.providedBy == null) 1653 if (Configuration.errorOnAutoCreate()) 1654 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1655 else if (Configuration.doAutoCreate()) 1656 this.providedBy = new Reference(); // cc 1657 return this.providedBy; 1658 } 1659 1660 public boolean hasProvidedBy() { 1661 return this.providedBy != null && !this.providedBy.isEmpty(); 1662 } 1663 1664 /** 1665 * @param value {@link #providedBy} (The organization that provides this 1666 * healthcare service.) 1667 */ 1668 public HealthcareService setProvidedBy(Reference value) { 1669 this.providedBy = value; 1670 return this; 1671 } 1672 1673 /** 1674 * @return {@link #providedBy} The actual object that is the target of the 1675 * reference. The reference library doesn't populate this, but you can 1676 * use it to hold the resource if you resolve it. (The organization that 1677 * provides this healthcare service.) 1678 */ 1679 public Organization getProvidedByTarget() { 1680 if (this.providedByTarget == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1683 else if (Configuration.doAutoCreate()) 1684 this.providedByTarget = new Organization(); // aa 1685 return this.providedByTarget; 1686 } 1687 1688 /** 1689 * @param value {@link #providedBy} The actual object that is the target of the 1690 * reference. The reference library doesn't use these, but you can 1691 * use it to hold the resource if you resolve it. (The organization 1692 * that provides this healthcare service.) 1693 */ 1694 public HealthcareService setProvidedByTarget(Organization value) { 1695 this.providedByTarget = value; 1696 return this; 1697 } 1698 1699 /** 1700 * @return {@link #category} (Identifies the broad category of service being 1701 * performed or delivered.) 1702 */ 1703 public List<CodeableConcept> getCategory() { 1704 if (this.category == null) 1705 this.category = new ArrayList<CodeableConcept>(); 1706 return this.category; 1707 } 1708 1709 /** 1710 * @return Returns a reference to <code>this</code> for easy method chaining 1711 */ 1712 public HealthcareService setCategory(List<CodeableConcept> theCategory) { 1713 this.category = theCategory; 1714 return this; 1715 } 1716 1717 public boolean hasCategory() { 1718 if (this.category == null) 1719 return false; 1720 for (CodeableConcept item : this.category) 1721 if (!item.isEmpty()) 1722 return true; 1723 return false; 1724 } 1725 1726 public CodeableConcept addCategory() { // 3 1727 CodeableConcept t = new CodeableConcept(); 1728 if (this.category == null) 1729 this.category = new ArrayList<CodeableConcept>(); 1730 this.category.add(t); 1731 return t; 1732 } 1733 1734 public HealthcareService addCategory(CodeableConcept t) { // 3 1735 if (t == null) 1736 return this; 1737 if (this.category == null) 1738 this.category = new ArrayList<CodeableConcept>(); 1739 this.category.add(t); 1740 return this; 1741 } 1742 1743 /** 1744 * @return The first repetition of repeating field {@link #category}, creating 1745 * it if it does not already exist 1746 */ 1747 public CodeableConcept getCategoryFirstRep() { 1748 if (getCategory().isEmpty()) { 1749 addCategory(); 1750 } 1751 return getCategory().get(0); 1752 } 1753 1754 /** 1755 * @return {@link #type} (The specific type of service that may be delivered or 1756 * performed.) 1757 */ 1758 public List<CodeableConcept> getType() { 1759 if (this.type == null) 1760 this.type = new ArrayList<CodeableConcept>(); 1761 return this.type; 1762 } 1763 1764 /** 1765 * @return Returns a reference to <code>this</code> for easy method chaining 1766 */ 1767 public HealthcareService setType(List<CodeableConcept> theType) { 1768 this.type = theType; 1769 return this; 1770 } 1771 1772 public boolean hasType() { 1773 if (this.type == null) 1774 return false; 1775 for (CodeableConcept item : this.type) 1776 if (!item.isEmpty()) 1777 return true; 1778 return false; 1779 } 1780 1781 public CodeableConcept addType() { // 3 1782 CodeableConcept t = new CodeableConcept(); 1783 if (this.type == null) 1784 this.type = new ArrayList<CodeableConcept>(); 1785 this.type.add(t); 1786 return t; 1787 } 1788 1789 public HealthcareService addType(CodeableConcept t) { // 3 1790 if (t == null) 1791 return this; 1792 if (this.type == null) 1793 this.type = new ArrayList<CodeableConcept>(); 1794 this.type.add(t); 1795 return this; 1796 } 1797 1798 /** 1799 * @return The first repetition of repeating field {@link #type}, creating it if 1800 * it does not already exist 1801 */ 1802 public CodeableConcept getTypeFirstRep() { 1803 if (getType().isEmpty()) { 1804 addType(); 1805 } 1806 return getType().get(0); 1807 } 1808 1809 /** 1810 * @return {@link #specialty} (Collection of specialties handled by the service 1811 * site. This is more of a medical term.) 1812 */ 1813 public List<CodeableConcept> getSpecialty() { 1814 if (this.specialty == null) 1815 this.specialty = new ArrayList<CodeableConcept>(); 1816 return this.specialty; 1817 } 1818 1819 /** 1820 * @return Returns a reference to <code>this</code> for easy method chaining 1821 */ 1822 public HealthcareService setSpecialty(List<CodeableConcept> theSpecialty) { 1823 this.specialty = theSpecialty; 1824 return this; 1825 } 1826 1827 public boolean hasSpecialty() { 1828 if (this.specialty == null) 1829 return false; 1830 for (CodeableConcept item : this.specialty) 1831 if (!item.isEmpty()) 1832 return true; 1833 return false; 1834 } 1835 1836 public CodeableConcept addSpecialty() { // 3 1837 CodeableConcept t = new CodeableConcept(); 1838 if (this.specialty == null) 1839 this.specialty = new ArrayList<CodeableConcept>(); 1840 this.specialty.add(t); 1841 return t; 1842 } 1843 1844 public HealthcareService addSpecialty(CodeableConcept t) { // 3 1845 if (t == null) 1846 return this; 1847 if (this.specialty == null) 1848 this.specialty = new ArrayList<CodeableConcept>(); 1849 this.specialty.add(t); 1850 return this; 1851 } 1852 1853 /** 1854 * @return The first repetition of repeating field {@link #specialty}, creating 1855 * it if it does not already exist 1856 */ 1857 public CodeableConcept getSpecialtyFirstRep() { 1858 if (getSpecialty().isEmpty()) { 1859 addSpecialty(); 1860 } 1861 return getSpecialty().get(0); 1862 } 1863 1864 /** 1865 * @return {@link #location} (The location(s) where this healthcare service may 1866 * be provided.) 1867 */ 1868 public List<Reference> getLocation() { 1869 if (this.location == null) 1870 this.location = new ArrayList<Reference>(); 1871 return this.location; 1872 } 1873 1874 /** 1875 * @return Returns a reference to <code>this</code> for easy method chaining 1876 */ 1877 public HealthcareService setLocation(List<Reference> theLocation) { 1878 this.location = theLocation; 1879 return this; 1880 } 1881 1882 public boolean hasLocation() { 1883 if (this.location == null) 1884 return false; 1885 for (Reference item : this.location) 1886 if (!item.isEmpty()) 1887 return true; 1888 return false; 1889 } 1890 1891 public Reference addLocation() { // 3 1892 Reference t = new Reference(); 1893 if (this.location == null) 1894 this.location = new ArrayList<Reference>(); 1895 this.location.add(t); 1896 return t; 1897 } 1898 1899 public HealthcareService addLocation(Reference t) { // 3 1900 if (t == null) 1901 return this; 1902 if (this.location == null) 1903 this.location = new ArrayList<Reference>(); 1904 this.location.add(t); 1905 return this; 1906 } 1907 1908 /** 1909 * @return The first repetition of repeating field {@link #location}, creating 1910 * it if it does not already exist 1911 */ 1912 public Reference getLocationFirstRep() { 1913 if (getLocation().isEmpty()) { 1914 addLocation(); 1915 } 1916 return getLocation().get(0); 1917 } 1918 1919 /** 1920 * @deprecated Use Reference#setResource(IBaseResource) instead 1921 */ 1922 @Deprecated 1923 public List<Location> getLocationTarget() { 1924 if (this.locationTarget == null) 1925 this.locationTarget = new ArrayList<Location>(); 1926 return this.locationTarget; 1927 } 1928 1929 /** 1930 * @deprecated Use Reference#setResource(IBaseResource) instead 1931 */ 1932 @Deprecated 1933 public Location addLocationTarget() { 1934 Location r = new Location(); 1935 if (this.locationTarget == null) 1936 this.locationTarget = new ArrayList<Location>(); 1937 this.locationTarget.add(r); 1938 return r; 1939 } 1940 1941 /** 1942 * @return {@link #name} (Further description of the service as it would be 1943 * presented to a consumer while searching.). This is the underlying 1944 * object with id, value and extensions. The accessor "getName" gives 1945 * direct access to the value 1946 */ 1947 public StringType getNameElement() { 1948 if (this.name == null) 1949 if (Configuration.errorOnAutoCreate()) 1950 throw new Error("Attempt to auto-create HealthcareService.name"); 1951 else if (Configuration.doAutoCreate()) 1952 this.name = new StringType(); // bb 1953 return this.name; 1954 } 1955 1956 public boolean hasNameElement() { 1957 return this.name != null && !this.name.isEmpty(); 1958 } 1959 1960 public boolean hasName() { 1961 return this.name != null && !this.name.isEmpty(); 1962 } 1963 1964 /** 1965 * @param value {@link #name} (Further description of the service as it would be 1966 * presented to a consumer while searching.). This is the 1967 * underlying object with id, value and extensions. The accessor 1968 * "getName" gives direct access to the value 1969 */ 1970 public HealthcareService setNameElement(StringType value) { 1971 this.name = value; 1972 return this; 1973 } 1974 1975 /** 1976 * @return Further description of the service as it would be presented to a 1977 * consumer while searching. 1978 */ 1979 public String getName() { 1980 return this.name == null ? null : this.name.getValue(); 1981 } 1982 1983 /** 1984 * @param value Further description of the service as it would be presented to a 1985 * consumer while searching. 1986 */ 1987 public HealthcareService setName(String value) { 1988 if (Utilities.noString(value)) 1989 this.name = null; 1990 else { 1991 if (this.name == null) 1992 this.name = new StringType(); 1993 this.name.setValue(value); 1994 } 1995 return this; 1996 } 1997 1998 /** 1999 * @return {@link #comment} (Any additional description of the service and/or 2000 * any specific issues not covered by the other attributes, which can be 2001 * displayed as further detail under the serviceName.). This is the 2002 * underlying object with id, value and extensions. The accessor 2003 * "getComment" gives direct access to the value 2004 */ 2005 public StringType getCommentElement() { 2006 if (this.comment == null) 2007 if (Configuration.errorOnAutoCreate()) 2008 throw new Error("Attempt to auto-create HealthcareService.comment"); 2009 else if (Configuration.doAutoCreate()) 2010 this.comment = new StringType(); // bb 2011 return this.comment; 2012 } 2013 2014 public boolean hasCommentElement() { 2015 return this.comment != null && !this.comment.isEmpty(); 2016 } 2017 2018 public boolean hasComment() { 2019 return this.comment != null && !this.comment.isEmpty(); 2020 } 2021 2022 /** 2023 * @param value {@link #comment} (Any additional description of the service 2024 * and/or any specific issues not covered by the other attributes, 2025 * which can be displayed as further detail under the 2026 * serviceName.). This is the underlying object with id, value and 2027 * extensions. The accessor "getComment" gives direct access to the 2028 * value 2029 */ 2030 public HealthcareService setCommentElement(StringType value) { 2031 this.comment = value; 2032 return this; 2033 } 2034 2035 /** 2036 * @return Any additional description of the service and/or any specific issues 2037 * not covered by the other attributes, which can be displayed as 2038 * further detail under the serviceName. 2039 */ 2040 public String getComment() { 2041 return this.comment == null ? null : this.comment.getValue(); 2042 } 2043 2044 /** 2045 * @param value Any additional description of the service and/or any specific 2046 * issues not covered by the other attributes, which can be 2047 * displayed as further detail under the serviceName. 2048 */ 2049 public HealthcareService setComment(String value) { 2050 if (Utilities.noString(value)) 2051 this.comment = null; 2052 else { 2053 if (this.comment == null) 2054 this.comment = new StringType(); 2055 this.comment.setValue(value); 2056 } 2057 return this; 2058 } 2059 2060 /** 2061 * @return {@link #extraDetails} (Extra details about the service that can't be 2062 * placed in the other fields.). This is the underlying object with id, 2063 * value and extensions. The accessor "getExtraDetails" gives direct 2064 * access to the value 2065 */ 2066 public MarkdownType getExtraDetailsElement() { 2067 if (this.extraDetails == null) 2068 if (Configuration.errorOnAutoCreate()) 2069 throw new Error("Attempt to auto-create HealthcareService.extraDetails"); 2070 else if (Configuration.doAutoCreate()) 2071 this.extraDetails = new MarkdownType(); // bb 2072 return this.extraDetails; 2073 } 2074 2075 public boolean hasExtraDetailsElement() { 2076 return this.extraDetails != null && !this.extraDetails.isEmpty(); 2077 } 2078 2079 public boolean hasExtraDetails() { 2080 return this.extraDetails != null && !this.extraDetails.isEmpty(); 2081 } 2082 2083 /** 2084 * @param value {@link #extraDetails} (Extra details about the service that 2085 * can't be placed in the other fields.). This is the underlying 2086 * object with id, value and extensions. The accessor 2087 * "getExtraDetails" gives direct access to the value 2088 */ 2089 public HealthcareService setExtraDetailsElement(MarkdownType value) { 2090 this.extraDetails = value; 2091 return this; 2092 } 2093 2094 /** 2095 * @return Extra details about the service that can't be placed in the other 2096 * fields. 2097 */ 2098 public String getExtraDetails() { 2099 return this.extraDetails == null ? null : this.extraDetails.getValue(); 2100 } 2101 2102 /** 2103 * @param value Extra details about the service that can't be placed in the 2104 * other fields. 2105 */ 2106 public HealthcareService setExtraDetails(String value) { 2107 if (value == null) 2108 this.extraDetails = null; 2109 else { 2110 if (this.extraDetails == null) 2111 this.extraDetails = new MarkdownType(); 2112 this.extraDetails.setValue(value); 2113 } 2114 return this; 2115 } 2116 2117 /** 2118 * @return {@link #photo} (If there is a photo/symbol associated with this 2119 * HealthcareService, it may be included here to facilitate quick 2120 * identification of the service in a list.) 2121 */ 2122 public Attachment getPhoto() { 2123 if (this.photo == null) 2124 if (Configuration.errorOnAutoCreate()) 2125 throw new Error("Attempt to auto-create HealthcareService.photo"); 2126 else if (Configuration.doAutoCreate()) 2127 this.photo = new Attachment(); // cc 2128 return this.photo; 2129 } 2130 2131 public boolean hasPhoto() { 2132 return this.photo != null && !this.photo.isEmpty(); 2133 } 2134 2135 /** 2136 * @param value {@link #photo} (If there is a photo/symbol associated with this 2137 * HealthcareService, it may be included here to facilitate quick 2138 * identification of the service in a list.) 2139 */ 2140 public HealthcareService setPhoto(Attachment value) { 2141 this.photo = value; 2142 return this; 2143 } 2144 2145 /** 2146 * @return {@link #telecom} (List of contacts related to this specific 2147 * healthcare service.) 2148 */ 2149 public List<ContactPoint> getTelecom() { 2150 if (this.telecom == null) 2151 this.telecom = new ArrayList<ContactPoint>(); 2152 return this.telecom; 2153 } 2154 2155 /** 2156 * @return Returns a reference to <code>this</code> for easy method chaining 2157 */ 2158 public HealthcareService setTelecom(List<ContactPoint> theTelecom) { 2159 this.telecom = theTelecom; 2160 return this; 2161 } 2162 2163 public boolean hasTelecom() { 2164 if (this.telecom == null) 2165 return false; 2166 for (ContactPoint item : this.telecom) 2167 if (!item.isEmpty()) 2168 return true; 2169 return false; 2170 } 2171 2172 public ContactPoint addTelecom() { // 3 2173 ContactPoint t = new ContactPoint(); 2174 if (this.telecom == null) 2175 this.telecom = new ArrayList<ContactPoint>(); 2176 this.telecom.add(t); 2177 return t; 2178 } 2179 2180 public HealthcareService addTelecom(ContactPoint t) { // 3 2181 if (t == null) 2182 return this; 2183 if (this.telecom == null) 2184 this.telecom = new ArrayList<ContactPoint>(); 2185 this.telecom.add(t); 2186 return this; 2187 } 2188 2189 /** 2190 * @return The first repetition of repeating field {@link #telecom}, creating it 2191 * if it does not already exist 2192 */ 2193 public ContactPoint getTelecomFirstRep() { 2194 if (getTelecom().isEmpty()) { 2195 addTelecom(); 2196 } 2197 return getTelecom().get(0); 2198 } 2199 2200 /** 2201 * @return {@link #coverageArea} (The location(s) that this service is available 2202 * to (not where the service is provided).) 2203 */ 2204 public List<Reference> getCoverageArea() { 2205 if (this.coverageArea == null) 2206 this.coverageArea = new ArrayList<Reference>(); 2207 return this.coverageArea; 2208 } 2209 2210 /** 2211 * @return Returns a reference to <code>this</code> for easy method chaining 2212 */ 2213 public HealthcareService setCoverageArea(List<Reference> theCoverageArea) { 2214 this.coverageArea = theCoverageArea; 2215 return this; 2216 } 2217 2218 public boolean hasCoverageArea() { 2219 if (this.coverageArea == null) 2220 return false; 2221 for (Reference item : this.coverageArea) 2222 if (!item.isEmpty()) 2223 return true; 2224 return false; 2225 } 2226 2227 public Reference addCoverageArea() { // 3 2228 Reference t = new Reference(); 2229 if (this.coverageArea == null) 2230 this.coverageArea = new ArrayList<Reference>(); 2231 this.coverageArea.add(t); 2232 return t; 2233 } 2234 2235 public HealthcareService addCoverageArea(Reference t) { // 3 2236 if (t == null) 2237 return this; 2238 if (this.coverageArea == null) 2239 this.coverageArea = new ArrayList<Reference>(); 2240 this.coverageArea.add(t); 2241 return this; 2242 } 2243 2244 /** 2245 * @return The first repetition of repeating field {@link #coverageArea}, 2246 * creating it if it does not already exist 2247 */ 2248 public Reference getCoverageAreaFirstRep() { 2249 if (getCoverageArea().isEmpty()) { 2250 addCoverageArea(); 2251 } 2252 return getCoverageArea().get(0); 2253 } 2254 2255 /** 2256 * @deprecated Use Reference#setResource(IBaseResource) instead 2257 */ 2258 @Deprecated 2259 public List<Location> getCoverageAreaTarget() { 2260 if (this.coverageAreaTarget == null) 2261 this.coverageAreaTarget = new ArrayList<Location>(); 2262 return this.coverageAreaTarget; 2263 } 2264 2265 /** 2266 * @deprecated Use Reference#setResource(IBaseResource) instead 2267 */ 2268 @Deprecated 2269 public Location addCoverageAreaTarget() { 2270 Location r = new Location(); 2271 if (this.coverageAreaTarget == null) 2272 this.coverageAreaTarget = new ArrayList<Location>(); 2273 this.coverageAreaTarget.add(r); 2274 return r; 2275 } 2276 2277 /** 2278 * @return {@link #serviceProvisionCode} (The code(s) that detail the conditions 2279 * under which the healthcare service is available/offered.) 2280 */ 2281 public List<CodeableConcept> getServiceProvisionCode() { 2282 if (this.serviceProvisionCode == null) 2283 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2284 return this.serviceProvisionCode; 2285 } 2286 2287 /** 2288 * @return Returns a reference to <code>this</code> for easy method chaining 2289 */ 2290 public HealthcareService setServiceProvisionCode(List<CodeableConcept> theServiceProvisionCode) { 2291 this.serviceProvisionCode = theServiceProvisionCode; 2292 return this; 2293 } 2294 2295 public boolean hasServiceProvisionCode() { 2296 if (this.serviceProvisionCode == null) 2297 return false; 2298 for (CodeableConcept item : this.serviceProvisionCode) 2299 if (!item.isEmpty()) 2300 return true; 2301 return false; 2302 } 2303 2304 public CodeableConcept addServiceProvisionCode() { // 3 2305 CodeableConcept t = new CodeableConcept(); 2306 if (this.serviceProvisionCode == null) 2307 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2308 this.serviceProvisionCode.add(t); 2309 return t; 2310 } 2311 2312 public HealthcareService addServiceProvisionCode(CodeableConcept t) { // 3 2313 if (t == null) 2314 return this; 2315 if (this.serviceProvisionCode == null) 2316 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2317 this.serviceProvisionCode.add(t); 2318 return this; 2319 } 2320 2321 /** 2322 * @return The first repetition of repeating field 2323 * {@link #serviceProvisionCode}, creating it if it does not already 2324 * exist 2325 */ 2326 public CodeableConcept getServiceProvisionCodeFirstRep() { 2327 if (getServiceProvisionCode().isEmpty()) { 2328 addServiceProvisionCode(); 2329 } 2330 return getServiceProvisionCode().get(0); 2331 } 2332 2333 /** 2334 * @return {@link #eligibility} (Does this service have specific eligibility 2335 * requirements that need to be met in order to use the service?) 2336 */ 2337 public List<HealthcareServiceEligibilityComponent> getEligibility() { 2338 if (this.eligibility == null) 2339 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2340 return this.eligibility; 2341 } 2342 2343 /** 2344 * @return Returns a reference to <code>this</code> for easy method chaining 2345 */ 2346 public HealthcareService setEligibility(List<HealthcareServiceEligibilityComponent> theEligibility) { 2347 this.eligibility = theEligibility; 2348 return this; 2349 } 2350 2351 public boolean hasEligibility() { 2352 if (this.eligibility == null) 2353 return false; 2354 for (HealthcareServiceEligibilityComponent item : this.eligibility) 2355 if (!item.isEmpty()) 2356 return true; 2357 return false; 2358 } 2359 2360 public HealthcareServiceEligibilityComponent addEligibility() { // 3 2361 HealthcareServiceEligibilityComponent t = new HealthcareServiceEligibilityComponent(); 2362 if (this.eligibility == null) 2363 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2364 this.eligibility.add(t); 2365 return t; 2366 } 2367 2368 public HealthcareService addEligibility(HealthcareServiceEligibilityComponent t) { // 3 2369 if (t == null) 2370 return this; 2371 if (this.eligibility == null) 2372 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2373 this.eligibility.add(t); 2374 return this; 2375 } 2376 2377 /** 2378 * @return The first repetition of repeating field {@link #eligibility}, 2379 * creating it if it does not already exist 2380 */ 2381 public HealthcareServiceEligibilityComponent getEligibilityFirstRep() { 2382 if (getEligibility().isEmpty()) { 2383 addEligibility(); 2384 } 2385 return getEligibility().get(0); 2386 } 2387 2388 /** 2389 * @return {@link #program} (Programs that this service is applicable to.) 2390 */ 2391 public List<CodeableConcept> getProgram() { 2392 if (this.program == null) 2393 this.program = new ArrayList<CodeableConcept>(); 2394 return this.program; 2395 } 2396 2397 /** 2398 * @return Returns a reference to <code>this</code> for easy method chaining 2399 */ 2400 public HealthcareService setProgram(List<CodeableConcept> theProgram) { 2401 this.program = theProgram; 2402 return this; 2403 } 2404 2405 public boolean hasProgram() { 2406 if (this.program == null) 2407 return false; 2408 for (CodeableConcept item : this.program) 2409 if (!item.isEmpty()) 2410 return true; 2411 return false; 2412 } 2413 2414 public CodeableConcept addProgram() { // 3 2415 CodeableConcept t = new CodeableConcept(); 2416 if (this.program == null) 2417 this.program = new ArrayList<CodeableConcept>(); 2418 this.program.add(t); 2419 return t; 2420 } 2421 2422 public HealthcareService addProgram(CodeableConcept t) { // 3 2423 if (t == null) 2424 return this; 2425 if (this.program == null) 2426 this.program = new ArrayList<CodeableConcept>(); 2427 this.program.add(t); 2428 return this; 2429 } 2430 2431 /** 2432 * @return The first repetition of repeating field {@link #program}, creating it 2433 * if it does not already exist 2434 */ 2435 public CodeableConcept getProgramFirstRep() { 2436 if (getProgram().isEmpty()) { 2437 addProgram(); 2438 } 2439 return getProgram().get(0); 2440 } 2441 2442 /** 2443 * @return {@link #characteristic} (Collection of characteristics (attributes).) 2444 */ 2445 public List<CodeableConcept> getCharacteristic() { 2446 if (this.characteristic == null) 2447 this.characteristic = new ArrayList<CodeableConcept>(); 2448 return this.characteristic; 2449 } 2450 2451 /** 2452 * @return Returns a reference to <code>this</code> for easy method chaining 2453 */ 2454 public HealthcareService setCharacteristic(List<CodeableConcept> theCharacteristic) { 2455 this.characteristic = theCharacteristic; 2456 return this; 2457 } 2458 2459 public boolean hasCharacteristic() { 2460 if (this.characteristic == null) 2461 return false; 2462 for (CodeableConcept item : this.characteristic) 2463 if (!item.isEmpty()) 2464 return true; 2465 return false; 2466 } 2467 2468 public CodeableConcept addCharacteristic() { // 3 2469 CodeableConcept t = new CodeableConcept(); 2470 if (this.characteristic == null) 2471 this.characteristic = new ArrayList<CodeableConcept>(); 2472 this.characteristic.add(t); 2473 return t; 2474 } 2475 2476 public HealthcareService addCharacteristic(CodeableConcept t) { // 3 2477 if (t == null) 2478 return this; 2479 if (this.characteristic == null) 2480 this.characteristic = new ArrayList<CodeableConcept>(); 2481 this.characteristic.add(t); 2482 return this; 2483 } 2484 2485 /** 2486 * @return The first repetition of repeating field {@link #characteristic}, 2487 * creating it if it does not already exist 2488 */ 2489 public CodeableConcept getCharacteristicFirstRep() { 2490 if (getCharacteristic().isEmpty()) { 2491 addCharacteristic(); 2492 } 2493 return getCharacteristic().get(0); 2494 } 2495 2496 /** 2497 * @return {@link #communication} (Some services are specifically made available 2498 * in multiple languages, this property permits a directory to declare 2499 * the languages this is offered in. Typically this is only provided 2500 * where a service operates in communities with mixed languages used.) 2501 */ 2502 public List<CodeableConcept> getCommunication() { 2503 if (this.communication == null) 2504 this.communication = new ArrayList<CodeableConcept>(); 2505 return this.communication; 2506 } 2507 2508 /** 2509 * @return Returns a reference to <code>this</code> for easy method chaining 2510 */ 2511 public HealthcareService setCommunication(List<CodeableConcept> theCommunication) { 2512 this.communication = theCommunication; 2513 return this; 2514 } 2515 2516 public boolean hasCommunication() { 2517 if (this.communication == null) 2518 return false; 2519 for (CodeableConcept item : this.communication) 2520 if (!item.isEmpty()) 2521 return true; 2522 return false; 2523 } 2524 2525 public CodeableConcept addCommunication() { // 3 2526 CodeableConcept t = new CodeableConcept(); 2527 if (this.communication == null) 2528 this.communication = new ArrayList<CodeableConcept>(); 2529 this.communication.add(t); 2530 return t; 2531 } 2532 2533 public HealthcareService addCommunication(CodeableConcept t) { // 3 2534 if (t == null) 2535 return this; 2536 if (this.communication == null) 2537 this.communication = new ArrayList<CodeableConcept>(); 2538 this.communication.add(t); 2539 return this; 2540 } 2541 2542 /** 2543 * @return The first repetition of repeating field {@link #communication}, 2544 * creating it if it does not already exist 2545 */ 2546 public CodeableConcept getCommunicationFirstRep() { 2547 if (getCommunication().isEmpty()) { 2548 addCommunication(); 2549 } 2550 return getCommunication().get(0); 2551 } 2552 2553 /** 2554 * @return {@link #referralMethod} (Ways that the service accepts referrals, if 2555 * this is not provided then it is implied that no referral is 2556 * required.) 2557 */ 2558 public List<CodeableConcept> getReferralMethod() { 2559 if (this.referralMethod == null) 2560 this.referralMethod = new ArrayList<CodeableConcept>(); 2561 return this.referralMethod; 2562 } 2563 2564 /** 2565 * @return Returns a reference to <code>this</code> for easy method chaining 2566 */ 2567 public HealthcareService setReferralMethod(List<CodeableConcept> theReferralMethod) { 2568 this.referralMethod = theReferralMethod; 2569 return this; 2570 } 2571 2572 public boolean hasReferralMethod() { 2573 if (this.referralMethod == null) 2574 return false; 2575 for (CodeableConcept item : this.referralMethod) 2576 if (!item.isEmpty()) 2577 return true; 2578 return false; 2579 } 2580 2581 public CodeableConcept addReferralMethod() { // 3 2582 CodeableConcept t = new CodeableConcept(); 2583 if (this.referralMethod == null) 2584 this.referralMethod = new ArrayList<CodeableConcept>(); 2585 this.referralMethod.add(t); 2586 return t; 2587 } 2588 2589 public HealthcareService addReferralMethod(CodeableConcept t) { // 3 2590 if (t == null) 2591 return this; 2592 if (this.referralMethod == null) 2593 this.referralMethod = new ArrayList<CodeableConcept>(); 2594 this.referralMethod.add(t); 2595 return this; 2596 } 2597 2598 /** 2599 * @return The first repetition of repeating field {@link #referralMethod}, 2600 * creating it if it does not already exist 2601 */ 2602 public CodeableConcept getReferralMethodFirstRep() { 2603 if (getReferralMethod().isEmpty()) { 2604 addReferralMethod(); 2605 } 2606 return getReferralMethod().get(0); 2607 } 2608 2609 /** 2610 * @return {@link #appointmentRequired} (Indicates whether or not a prospective 2611 * consumer will require an appointment for a particular service at a 2612 * site to be provided by the Organization. Indicates if an appointment 2613 * is required for access to this service.). This is the underlying 2614 * object with id, value and extensions. The accessor 2615 * "getAppointmentRequired" gives direct access to the value 2616 */ 2617 public BooleanType getAppointmentRequiredElement() { 2618 if (this.appointmentRequired == null) 2619 if (Configuration.errorOnAutoCreate()) 2620 throw new Error("Attempt to auto-create HealthcareService.appointmentRequired"); 2621 else if (Configuration.doAutoCreate()) 2622 this.appointmentRequired = new BooleanType(); // bb 2623 return this.appointmentRequired; 2624 } 2625 2626 public boolean hasAppointmentRequiredElement() { 2627 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2628 } 2629 2630 public boolean hasAppointmentRequired() { 2631 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2632 } 2633 2634 /** 2635 * @param value {@link #appointmentRequired} (Indicates whether or not a 2636 * prospective consumer will require an appointment for a 2637 * particular service at a site to be provided by the Organization. 2638 * Indicates if an appointment is required for access to this 2639 * service.). This is the underlying object with id, value and 2640 * extensions. The accessor "getAppointmentRequired" gives direct 2641 * access to the value 2642 */ 2643 public HealthcareService setAppointmentRequiredElement(BooleanType value) { 2644 this.appointmentRequired = value; 2645 return this; 2646 } 2647 2648 /** 2649 * @return Indicates whether or not a prospective consumer will require an 2650 * appointment for a particular service at a site to be provided by the 2651 * Organization. Indicates if an appointment is required for access to 2652 * this service. 2653 */ 2654 public boolean getAppointmentRequired() { 2655 return this.appointmentRequired == null || this.appointmentRequired.isEmpty() ? false 2656 : this.appointmentRequired.getValue(); 2657 } 2658 2659 /** 2660 * @param value Indicates whether or not a prospective consumer will require an 2661 * appointment for a particular service at a site to be provided by 2662 * the Organization. Indicates if an appointment is required for 2663 * access to this service. 2664 */ 2665 public HealthcareService setAppointmentRequired(boolean value) { 2666 if (this.appointmentRequired == null) 2667 this.appointmentRequired = new BooleanType(); 2668 this.appointmentRequired.setValue(value); 2669 return this; 2670 } 2671 2672 /** 2673 * @return {@link #availableTime} (A collection of times that the Service Site 2674 * is available.) 2675 */ 2676 public List<HealthcareServiceAvailableTimeComponent> getAvailableTime() { 2677 if (this.availableTime == null) 2678 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2679 return this.availableTime; 2680 } 2681 2682 /** 2683 * @return Returns a reference to <code>this</code> for easy method chaining 2684 */ 2685 public HealthcareService setAvailableTime(List<HealthcareServiceAvailableTimeComponent> theAvailableTime) { 2686 this.availableTime = theAvailableTime; 2687 return this; 2688 } 2689 2690 public boolean hasAvailableTime() { 2691 if (this.availableTime == null) 2692 return false; 2693 for (HealthcareServiceAvailableTimeComponent item : this.availableTime) 2694 if (!item.isEmpty()) 2695 return true; 2696 return false; 2697 } 2698 2699 public HealthcareServiceAvailableTimeComponent addAvailableTime() { // 3 2700 HealthcareServiceAvailableTimeComponent t = new HealthcareServiceAvailableTimeComponent(); 2701 if (this.availableTime == null) 2702 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2703 this.availableTime.add(t); 2704 return t; 2705 } 2706 2707 public HealthcareService addAvailableTime(HealthcareServiceAvailableTimeComponent t) { // 3 2708 if (t == null) 2709 return this; 2710 if (this.availableTime == null) 2711 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2712 this.availableTime.add(t); 2713 return this; 2714 } 2715 2716 /** 2717 * @return The first repetition of repeating field {@link #availableTime}, 2718 * creating it if it does not already exist 2719 */ 2720 public HealthcareServiceAvailableTimeComponent getAvailableTimeFirstRep() { 2721 if (getAvailableTime().isEmpty()) { 2722 addAvailableTime(); 2723 } 2724 return getAvailableTime().get(0); 2725 } 2726 2727 /** 2728 * @return {@link #notAvailable} (The HealthcareService is not available during 2729 * this period of time due to the provided reason.) 2730 */ 2731 public List<HealthcareServiceNotAvailableComponent> getNotAvailable() { 2732 if (this.notAvailable == null) 2733 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2734 return this.notAvailable; 2735 } 2736 2737 /** 2738 * @return Returns a reference to <code>this</code> for easy method chaining 2739 */ 2740 public HealthcareService setNotAvailable(List<HealthcareServiceNotAvailableComponent> theNotAvailable) { 2741 this.notAvailable = theNotAvailable; 2742 return this; 2743 } 2744 2745 public boolean hasNotAvailable() { 2746 if (this.notAvailable == null) 2747 return false; 2748 for (HealthcareServiceNotAvailableComponent item : this.notAvailable) 2749 if (!item.isEmpty()) 2750 return true; 2751 return false; 2752 } 2753 2754 public HealthcareServiceNotAvailableComponent addNotAvailable() { // 3 2755 HealthcareServiceNotAvailableComponent t = new HealthcareServiceNotAvailableComponent(); 2756 if (this.notAvailable == null) 2757 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2758 this.notAvailable.add(t); 2759 return t; 2760 } 2761 2762 public HealthcareService addNotAvailable(HealthcareServiceNotAvailableComponent t) { // 3 2763 if (t == null) 2764 return this; 2765 if (this.notAvailable == null) 2766 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2767 this.notAvailable.add(t); 2768 return this; 2769 } 2770 2771 /** 2772 * @return The first repetition of repeating field {@link #notAvailable}, 2773 * creating it if it does not already exist 2774 */ 2775 public HealthcareServiceNotAvailableComponent getNotAvailableFirstRep() { 2776 if (getNotAvailable().isEmpty()) { 2777 addNotAvailable(); 2778 } 2779 return getNotAvailable().get(0); 2780 } 2781 2782 /** 2783 * @return {@link #availabilityExceptions} (A description of site availability 2784 * exceptions, e.g. public holiday availability. Succinctly describing 2785 * all possible exceptions to normal site availability as details in the 2786 * available Times and not available Times.). This is the underlying 2787 * object with id, value and extensions. The accessor 2788 * "getAvailabilityExceptions" gives direct access to the value 2789 */ 2790 public StringType getAvailabilityExceptionsElement() { 2791 if (this.availabilityExceptions == null) 2792 if (Configuration.errorOnAutoCreate()) 2793 throw new Error("Attempt to auto-create HealthcareService.availabilityExceptions"); 2794 else if (Configuration.doAutoCreate()) 2795 this.availabilityExceptions = new StringType(); // bb 2796 return this.availabilityExceptions; 2797 } 2798 2799 public boolean hasAvailabilityExceptionsElement() { 2800 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2801 } 2802 2803 public boolean hasAvailabilityExceptions() { 2804 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2805 } 2806 2807 /** 2808 * @param value {@link #availabilityExceptions} (A description of site 2809 * availability exceptions, e.g. public holiday availability. 2810 * Succinctly describing all possible exceptions to normal site 2811 * availability as details in the available Times and not available 2812 * Times.). This is the underlying object with id, value and 2813 * extensions. The accessor "getAvailabilityExceptions" gives 2814 * direct access to the value 2815 */ 2816 public HealthcareService setAvailabilityExceptionsElement(StringType value) { 2817 this.availabilityExceptions = value; 2818 return this; 2819 } 2820 2821 /** 2822 * @return A description of site availability exceptions, e.g. public holiday 2823 * availability. Succinctly describing all possible exceptions to normal 2824 * site availability as details in the available Times and not available 2825 * Times. 2826 */ 2827 public String getAvailabilityExceptions() { 2828 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 2829 } 2830 2831 /** 2832 * @param value A description of site availability exceptions, e.g. public 2833 * holiday availability. Succinctly describing all possible 2834 * exceptions to normal site availability as details in the 2835 * available Times and not available Times. 2836 */ 2837 public HealthcareService setAvailabilityExceptions(String value) { 2838 if (Utilities.noString(value)) 2839 this.availabilityExceptions = null; 2840 else { 2841 if (this.availabilityExceptions == null) 2842 this.availabilityExceptions = new StringType(); 2843 this.availabilityExceptions.setValue(value); 2844 } 2845 return this; 2846 } 2847 2848 /** 2849 * @return {@link #endpoint} (Technical endpoints providing access to services 2850 * operated for the specific healthcare services defined at this 2851 * resource.) 2852 */ 2853 public List<Reference> getEndpoint() { 2854 if (this.endpoint == null) 2855 this.endpoint = new ArrayList<Reference>(); 2856 return this.endpoint; 2857 } 2858 2859 /** 2860 * @return Returns a reference to <code>this</code> for easy method chaining 2861 */ 2862 public HealthcareService setEndpoint(List<Reference> theEndpoint) { 2863 this.endpoint = theEndpoint; 2864 return this; 2865 } 2866 2867 public boolean hasEndpoint() { 2868 if (this.endpoint == null) 2869 return false; 2870 for (Reference item : this.endpoint) 2871 if (!item.isEmpty()) 2872 return true; 2873 return false; 2874 } 2875 2876 public Reference addEndpoint() { // 3 2877 Reference t = new Reference(); 2878 if (this.endpoint == null) 2879 this.endpoint = new ArrayList<Reference>(); 2880 this.endpoint.add(t); 2881 return t; 2882 } 2883 2884 public HealthcareService addEndpoint(Reference t) { // 3 2885 if (t == null) 2886 return this; 2887 if (this.endpoint == null) 2888 this.endpoint = new ArrayList<Reference>(); 2889 this.endpoint.add(t); 2890 return this; 2891 } 2892 2893 /** 2894 * @return The first repetition of repeating field {@link #endpoint}, creating 2895 * it if it does not already exist 2896 */ 2897 public Reference getEndpointFirstRep() { 2898 if (getEndpoint().isEmpty()) { 2899 addEndpoint(); 2900 } 2901 return getEndpoint().get(0); 2902 } 2903 2904 /** 2905 * @deprecated Use Reference#setResource(IBaseResource) instead 2906 */ 2907 @Deprecated 2908 public List<Endpoint> getEndpointTarget() { 2909 if (this.endpointTarget == null) 2910 this.endpointTarget = new ArrayList<Endpoint>(); 2911 return this.endpointTarget; 2912 } 2913 2914 /** 2915 * @deprecated Use Reference#setResource(IBaseResource) instead 2916 */ 2917 @Deprecated 2918 public Endpoint addEndpointTarget() { 2919 Endpoint r = new Endpoint(); 2920 if (this.endpointTarget == null) 2921 this.endpointTarget = new ArrayList<Endpoint>(); 2922 this.endpointTarget.add(r); 2923 return r; 2924 } 2925 2926 protected void listChildren(List<Property> children) { 2927 super.listChildren(children); 2928 children.add(new Property("identifier", "Identifier", "External identifiers for this item.", 0, 2929 java.lang.Integer.MAX_VALUE, identifier)); 2930 children.add(new Property("active", "boolean", 2931 "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.", 2932 0, 1, active)); 2933 children.add(new Property("providedBy", "Reference(Organization)", 2934 "The organization that provides this healthcare service.", 0, 1, providedBy)); 2935 children.add(new Property("category", "CodeableConcept", 2936 "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, 2937 category)); 2938 children.add(new Property("type", "CodeableConcept", 2939 "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type)); 2940 children.add(new Property("specialty", "CodeableConcept", 2941 "Collection of specialties handled by the service site. This is more of a medical term.", 0, 2942 java.lang.Integer.MAX_VALUE, specialty)); 2943 children.add(new Property("location", "Reference(Location)", 2944 "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location)); 2945 children.add(new Property("name", "string", 2946 "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name)); 2947 children.add(new Property("comment", "string", 2948 "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 2949 0, 1, comment)); 2950 children.add(new Property("extraDetails", "markdown", 2951 "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails)); 2952 children.add(new Property("photo", "Attachment", 2953 "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 2954 0, 1, photo)); 2955 children.add(new Property("telecom", "ContactPoint", 2956 "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2957 children.add(new Property("coverageArea", "Reference(Location)", 2958 "The location(s) that this service is available to (not where the service is provided).", 0, 2959 java.lang.Integer.MAX_VALUE, coverageArea)); 2960 children.add(new Property("serviceProvisionCode", "CodeableConcept", 2961 "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, 2962 java.lang.Integer.MAX_VALUE, serviceProvisionCode)); 2963 children.add(new Property("eligibility", "", 2964 "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, 2965 java.lang.Integer.MAX_VALUE, eligibility)); 2966 children.add(new Property("program", "CodeableConcept", "Programs that this service is applicable to.", 0, 2967 java.lang.Integer.MAX_VALUE, program)); 2968 children.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, 2969 java.lang.Integer.MAX_VALUE, characteristic)); 2970 children.add(new Property("communication", "CodeableConcept", 2971 "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.", 2972 0, java.lang.Integer.MAX_VALUE, communication)); 2973 children.add(new Property("referralMethod", "CodeableConcept", 2974 "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 2975 0, java.lang.Integer.MAX_VALUE, referralMethod)); 2976 children.add(new Property("appointmentRequired", "boolean", 2977 "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 2978 0, 1, appointmentRequired)); 2979 children.add(new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, 2980 java.lang.Integer.MAX_VALUE, availableTime)); 2981 children.add(new Property("notAvailable", "", 2982 "The HealthcareService is not available during this period of time due to the provided reason.", 0, 2983 java.lang.Integer.MAX_VALUE, notAvailable)); 2984 children.add(new Property("availabilityExceptions", "string", 2985 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2986 0, 1, availabilityExceptions)); 2987 children.add(new Property("endpoint", "Reference(Endpoint)", 2988 "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 2989 0, java.lang.Integer.MAX_VALUE, endpoint)); 2990 } 2991 2992 @Override 2993 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2994 switch (_hash) { 2995 case -1618432855: 2996 /* identifier */ return new Property("identifier", "Identifier", "External identifiers for this item.", 0, 2997 java.lang.Integer.MAX_VALUE, identifier); 2998 case -1422950650: 2999 /* active */ return new Property("active", "boolean", 3000 "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.", 3001 0, 1, active); 3002 case 205136282: 3003 /* providedBy */ return new Property("providedBy", "Reference(Organization)", 3004 "The organization that provides this healthcare service.", 0, 1, providedBy); 3005 case 50511102: 3006 /* category */ return new Property("category", "CodeableConcept", 3007 "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, 3008 category); 3009 case 3575610: 3010 /* type */ return new Property("type", "CodeableConcept", 3011 "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type); 3012 case -1694759682: 3013 /* specialty */ return new Property("specialty", "CodeableConcept", 3014 "Collection of specialties handled by the service site. This is more of a medical term.", 0, 3015 java.lang.Integer.MAX_VALUE, specialty); 3016 case 1901043637: 3017 /* location */ return new Property("location", "Reference(Location)", 3018 "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location); 3019 case 3373707: 3020 /* name */ return new Property("name", "string", 3021 "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name); 3022 case 950398559: 3023 /* comment */ return new Property("comment", "string", 3024 "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 3025 0, 1, comment); 3026 case -1469168622: 3027 /* extraDetails */ return new Property("extraDetails", "markdown", 3028 "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails); 3029 case 106642994: 3030 /* photo */ return new Property("photo", "Attachment", 3031 "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 3032 0, 1, photo); 3033 case -1429363305: 3034 /* telecom */ return new Property("telecom", "ContactPoint", 3035 "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom); 3036 case -1532328299: 3037 /* coverageArea */ return new Property("coverageArea", "Reference(Location)", 3038 "The location(s) that this service is available to (not where the service is provided).", 0, 3039 java.lang.Integer.MAX_VALUE, coverageArea); 3040 case 1504575405: 3041 /* serviceProvisionCode */ return new Property("serviceProvisionCode", "CodeableConcept", 3042 "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, 3043 java.lang.Integer.MAX_VALUE, serviceProvisionCode); 3044 case -930847859: 3045 /* eligibility */ return new Property("eligibility", "", 3046 "Does this service have specific eligibility requirements that need to be met in order to use the service?", 3047 0, java.lang.Integer.MAX_VALUE, eligibility); 3048 case -309387644: 3049 /* program */ return new Property("program", "CodeableConcept", "Programs that this service is applicable to.", 0, 3050 java.lang.Integer.MAX_VALUE, program); 3051 case 366313883: 3052 /* characteristic */ return new Property("characteristic", "CodeableConcept", 3053 "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic); 3054 case -1035284522: 3055 /* communication */ return new Property("communication", "CodeableConcept", 3056 "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.", 3057 0, java.lang.Integer.MAX_VALUE, communication); 3058 case -2092740898: 3059 /* referralMethod */ return new Property("referralMethod", "CodeableConcept", 3060 "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 3061 0, java.lang.Integer.MAX_VALUE, referralMethod); 3062 case 427220062: 3063 /* appointmentRequired */ return new Property("appointmentRequired", "boolean", 3064 "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 3065 0, 1, appointmentRequired); 3066 case 1873069366: 3067 /* availableTime */ return new Property("availableTime", "", 3068 "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 3069 case -629572298: 3070 /* notAvailable */ return new Property("notAvailable", "", 3071 "The HealthcareService is not available during this period of time due to the provided reason.", 0, 3072 java.lang.Integer.MAX_VALUE, notAvailable); 3073 case -1149143617: 3074 /* availabilityExceptions */ return new Property("availabilityExceptions", "string", 3075 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 3076 0, 1, availabilityExceptions); 3077 case 1741102485: 3078 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 3079 "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 3080 0, java.lang.Integer.MAX_VALUE, endpoint); 3081 default: 3082 return super.getNamedProperty(_hash, _name, _checkValid); 3083 } 3084 3085 } 3086 3087 @Override 3088 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3089 switch (hash) { 3090 case -1618432855: 3091 /* identifier */ return this.identifier == null ? new Base[0] 3092 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3093 case -1422950650: 3094 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 3095 case 205136282: 3096 /* providedBy */ return this.providedBy == null ? new Base[0] : new Base[] { this.providedBy }; // Reference 3097 case 50511102: 3098 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3099 case 3575610: 3100 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3101 case -1694759682: 3102 /* specialty */ return this.specialty == null ? new Base[0] 3103 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 3104 case 1901043637: 3105 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 3106 case 3373707: 3107 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3108 case 950398559: 3109 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 3110 case -1469168622: 3111 /* extraDetails */ return this.extraDetails == null ? new Base[0] : new Base[] { this.extraDetails }; // MarkdownType 3112 case 106642994: 3113 /* photo */ return this.photo == null ? new Base[0] : new Base[] { this.photo }; // Attachment 3114 case -1429363305: 3115 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 3116 case -1532328299: 3117 /* coverageArea */ return this.coverageArea == null ? new Base[0] 3118 : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 3119 case 1504575405: 3120 /* serviceProvisionCode */ return this.serviceProvisionCode == null ? new Base[0] 3121 : this.serviceProvisionCode.toArray(new Base[this.serviceProvisionCode.size()]); // CodeableConcept 3122 case -930847859: 3123 /* eligibility */ return this.eligibility == null ? new Base[0] 3124 : this.eligibility.toArray(new Base[this.eligibility.size()]); // HealthcareServiceEligibilityComponent 3125 case -309387644: 3126 /* program */ return this.program == null ? new Base[0] : this.program.toArray(new Base[this.program.size()]); // CodeableConcept 3127 case 366313883: 3128 /* characteristic */ return this.characteristic == null ? new Base[0] 3129 : this.characteristic.toArray(new Base[this.characteristic.size()]); // CodeableConcept 3130 case -1035284522: 3131 /* communication */ return this.communication == null ? new Base[0] 3132 : this.communication.toArray(new Base[this.communication.size()]); // CodeableConcept 3133 case -2092740898: 3134 /* referralMethod */ return this.referralMethod == null ? new Base[0] 3135 : this.referralMethod.toArray(new Base[this.referralMethod.size()]); // CodeableConcept 3136 case 427220062: 3137 /* appointmentRequired */ return this.appointmentRequired == null ? new Base[0] 3138 : new Base[] { this.appointmentRequired }; // BooleanType 3139 case 1873069366: 3140 /* availableTime */ return this.availableTime == null ? new Base[0] 3141 : this.availableTime.toArray(new Base[this.availableTime.size()]); // HealthcareServiceAvailableTimeComponent 3142 case -629572298: 3143 /* notAvailable */ return this.notAvailable == null ? new Base[0] 3144 : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // HealthcareServiceNotAvailableComponent 3145 case -1149143617: 3146 /* availabilityExceptions */ return this.availabilityExceptions == null ? new Base[0] 3147 : new Base[] { this.availabilityExceptions }; // StringType 3148 case 1741102485: 3149 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 3150 default: 3151 return super.getProperty(hash, name, checkValid); 3152 } 3153 3154 } 3155 3156 @Override 3157 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3158 switch (hash) { 3159 case -1618432855: // identifier 3160 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3161 return value; 3162 case -1422950650: // active 3163 this.active = castToBoolean(value); // BooleanType 3164 return value; 3165 case 205136282: // providedBy 3166 this.providedBy = castToReference(value); // Reference 3167 return value; 3168 case 50511102: // category 3169 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3170 return value; 3171 case 3575610: // type 3172 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3173 return value; 3174 case -1694759682: // specialty 3175 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 3176 return value; 3177 case 1901043637: // location 3178 this.getLocation().add(castToReference(value)); // Reference 3179 return value; 3180 case 3373707: // name 3181 this.name = castToString(value); // StringType 3182 return value; 3183 case 950398559: // comment 3184 this.comment = castToString(value); // StringType 3185 return value; 3186 case -1469168622: // extraDetails 3187 this.extraDetails = castToMarkdown(value); // MarkdownType 3188 return value; 3189 case 106642994: // photo 3190 this.photo = castToAttachment(value); // Attachment 3191 return value; 3192 case -1429363305: // telecom 3193 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 3194 return value; 3195 case -1532328299: // coverageArea 3196 this.getCoverageArea().add(castToReference(value)); // Reference 3197 return value; 3198 case 1504575405: // serviceProvisionCode 3199 this.getServiceProvisionCode().add(castToCodeableConcept(value)); // CodeableConcept 3200 return value; 3201 case -930847859: // eligibility 3202 this.getEligibility().add((HealthcareServiceEligibilityComponent) value); // HealthcareServiceEligibilityComponent 3203 return value; 3204 case -309387644: // program 3205 this.getProgram().add(castToCodeableConcept(value)); // CodeableConcept 3206 return value; 3207 case 366313883: // characteristic 3208 this.getCharacteristic().add(castToCodeableConcept(value)); // CodeableConcept 3209 return value; 3210 case -1035284522: // communication 3211 this.getCommunication().add(castToCodeableConcept(value)); // CodeableConcept 3212 return value; 3213 case -2092740898: // referralMethod 3214 this.getReferralMethod().add(castToCodeableConcept(value)); // CodeableConcept 3215 return value; 3216 case 427220062: // appointmentRequired 3217 this.appointmentRequired = castToBoolean(value); // BooleanType 3218 return value; 3219 case 1873069366: // availableTime 3220 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); // HealthcareServiceAvailableTimeComponent 3221 return value; 3222 case -629572298: // notAvailable 3223 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); // HealthcareServiceNotAvailableComponent 3224 return value; 3225 case -1149143617: // availabilityExceptions 3226 this.availabilityExceptions = castToString(value); // StringType 3227 return value; 3228 case 1741102485: // endpoint 3229 this.getEndpoint().add(castToReference(value)); // Reference 3230 return value; 3231 default: 3232 return super.setProperty(hash, name, value); 3233 } 3234 3235 } 3236 3237 @Override 3238 public Base setProperty(String name, Base value) throws FHIRException { 3239 if (name.equals("identifier")) { 3240 this.getIdentifier().add(castToIdentifier(value)); 3241 } else if (name.equals("active")) { 3242 this.active = castToBoolean(value); // BooleanType 3243 } else if (name.equals("providedBy")) { 3244 this.providedBy = castToReference(value); // Reference 3245 } else if (name.equals("category")) { 3246 this.getCategory().add(castToCodeableConcept(value)); 3247 } else if (name.equals("type")) { 3248 this.getType().add(castToCodeableConcept(value)); 3249 } else if (name.equals("specialty")) { 3250 this.getSpecialty().add(castToCodeableConcept(value)); 3251 } else if (name.equals("location")) { 3252 this.getLocation().add(castToReference(value)); 3253 } else if (name.equals("name")) { 3254 this.name = castToString(value); // StringType 3255 } else if (name.equals("comment")) { 3256 this.comment = castToString(value); // StringType 3257 } else if (name.equals("extraDetails")) { 3258 this.extraDetails = castToMarkdown(value); // MarkdownType 3259 } else if (name.equals("photo")) { 3260 this.photo = castToAttachment(value); // Attachment 3261 } else if (name.equals("telecom")) { 3262 this.getTelecom().add(castToContactPoint(value)); 3263 } else if (name.equals("coverageArea")) { 3264 this.getCoverageArea().add(castToReference(value)); 3265 } else if (name.equals("serviceProvisionCode")) { 3266 this.getServiceProvisionCode().add(castToCodeableConcept(value)); 3267 } else if (name.equals("eligibility")) { 3268 this.getEligibility().add((HealthcareServiceEligibilityComponent) value); 3269 } else if (name.equals("program")) { 3270 this.getProgram().add(castToCodeableConcept(value)); 3271 } else if (name.equals("characteristic")) { 3272 this.getCharacteristic().add(castToCodeableConcept(value)); 3273 } else if (name.equals("communication")) { 3274 this.getCommunication().add(castToCodeableConcept(value)); 3275 } else if (name.equals("referralMethod")) { 3276 this.getReferralMethod().add(castToCodeableConcept(value)); 3277 } else if (name.equals("appointmentRequired")) { 3278 this.appointmentRequired = castToBoolean(value); // BooleanType 3279 } else if (name.equals("availableTime")) { 3280 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); 3281 } else if (name.equals("notAvailable")) { 3282 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); 3283 } else if (name.equals("availabilityExceptions")) { 3284 this.availabilityExceptions = castToString(value); // StringType 3285 } else if (name.equals("endpoint")) { 3286 this.getEndpoint().add(castToReference(value)); 3287 } else 3288 return super.setProperty(name, value); 3289 return value; 3290 } 3291 3292 @Override 3293 public void removeChild(String name, Base value) throws FHIRException { 3294 if (name.equals("identifier")) { 3295 this.getIdentifier().remove(castToIdentifier(value)); 3296 } else if (name.equals("active")) { 3297 this.active = null; 3298 } else if (name.equals("providedBy")) { 3299 this.providedBy = null; 3300 } else if (name.equals("category")) { 3301 this.getCategory().remove(castToCodeableConcept(value)); 3302 } else if (name.equals("type")) { 3303 this.getType().remove(castToCodeableConcept(value)); 3304 } else if (name.equals("specialty")) { 3305 this.getSpecialty().remove(castToCodeableConcept(value)); 3306 } else if (name.equals("location")) { 3307 this.getLocation().remove(castToReference(value)); 3308 } else if (name.equals("name")) { 3309 this.name = null; 3310 } else if (name.equals("comment")) { 3311 this.comment = null; 3312 } else if (name.equals("extraDetails")) { 3313 this.extraDetails = null; 3314 } else if (name.equals("photo")) { 3315 this.photo = null; 3316 } else if (name.equals("telecom")) { 3317 this.getTelecom().remove(castToContactPoint(value)); 3318 } else if (name.equals("coverageArea")) { 3319 this.getCoverageArea().remove(castToReference(value)); 3320 } else if (name.equals("serviceProvisionCode")) { 3321 this.getServiceProvisionCode().remove(castToCodeableConcept(value)); 3322 } else if (name.equals("eligibility")) { 3323 this.getEligibility().remove((HealthcareServiceEligibilityComponent) value); 3324 } else if (name.equals("program")) { 3325 this.getProgram().remove(castToCodeableConcept(value)); 3326 } else if (name.equals("characteristic")) { 3327 this.getCharacteristic().remove(castToCodeableConcept(value)); 3328 } else if (name.equals("communication")) { 3329 this.getCommunication().remove(castToCodeableConcept(value)); 3330 } else if (name.equals("referralMethod")) { 3331 this.getReferralMethod().remove(castToCodeableConcept(value)); 3332 } else if (name.equals("appointmentRequired")) { 3333 this.appointmentRequired = null; 3334 } else if (name.equals("availableTime")) { 3335 this.getAvailableTime().remove((HealthcareServiceAvailableTimeComponent) value); 3336 } else if (name.equals("notAvailable")) { 3337 this.getNotAvailable().remove((HealthcareServiceNotAvailableComponent) value); 3338 } else if (name.equals("availabilityExceptions")) { 3339 this.availabilityExceptions = null; 3340 } else if (name.equals("endpoint")) { 3341 this.getEndpoint().remove(castToReference(value)); 3342 } else 3343 super.removeChild(name, value); 3344 3345 } 3346 3347 @Override 3348 public Base makeProperty(int hash, String name) throws FHIRException { 3349 switch (hash) { 3350 case -1618432855: 3351 return addIdentifier(); 3352 case -1422950650: 3353 return getActiveElement(); 3354 case 205136282: 3355 return getProvidedBy(); 3356 case 50511102: 3357 return addCategory(); 3358 case 3575610: 3359 return addType(); 3360 case -1694759682: 3361 return addSpecialty(); 3362 case 1901043637: 3363 return addLocation(); 3364 case 3373707: 3365 return getNameElement(); 3366 case 950398559: 3367 return getCommentElement(); 3368 case -1469168622: 3369 return getExtraDetailsElement(); 3370 case 106642994: 3371 return getPhoto(); 3372 case -1429363305: 3373 return addTelecom(); 3374 case -1532328299: 3375 return addCoverageArea(); 3376 case 1504575405: 3377 return addServiceProvisionCode(); 3378 case -930847859: 3379 return addEligibility(); 3380 case -309387644: 3381 return addProgram(); 3382 case 366313883: 3383 return addCharacteristic(); 3384 case -1035284522: 3385 return addCommunication(); 3386 case -2092740898: 3387 return addReferralMethod(); 3388 case 427220062: 3389 return getAppointmentRequiredElement(); 3390 case 1873069366: 3391 return addAvailableTime(); 3392 case -629572298: 3393 return addNotAvailable(); 3394 case -1149143617: 3395 return getAvailabilityExceptionsElement(); 3396 case 1741102485: 3397 return addEndpoint(); 3398 default: 3399 return super.makeProperty(hash, name); 3400 } 3401 3402 } 3403 3404 @Override 3405 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3406 switch (hash) { 3407 case -1618432855: 3408 /* identifier */ return new String[] { "Identifier" }; 3409 case -1422950650: 3410 /* active */ return new String[] { "boolean" }; 3411 case 205136282: 3412 /* providedBy */ return new String[] { "Reference" }; 3413 case 50511102: 3414 /* category */ return new String[] { "CodeableConcept" }; 3415 case 3575610: 3416 /* type */ return new String[] { "CodeableConcept" }; 3417 case -1694759682: 3418 /* specialty */ return new String[] { "CodeableConcept" }; 3419 case 1901043637: 3420 /* location */ return new String[] { "Reference" }; 3421 case 3373707: 3422 /* name */ return new String[] { "string" }; 3423 case 950398559: 3424 /* comment */ return new String[] { "string" }; 3425 case -1469168622: 3426 /* extraDetails */ return new String[] { "markdown" }; 3427 case 106642994: 3428 /* photo */ return new String[] { "Attachment" }; 3429 case -1429363305: 3430 /* telecom */ return new String[] { "ContactPoint" }; 3431 case -1532328299: 3432 /* coverageArea */ return new String[] { "Reference" }; 3433 case 1504575405: 3434 /* serviceProvisionCode */ return new String[] { "CodeableConcept" }; 3435 case -930847859: 3436 /* eligibility */ return new String[] {}; 3437 case -309387644: 3438 /* program */ return new String[] { "CodeableConcept" }; 3439 case 366313883: 3440 /* characteristic */ return new String[] { "CodeableConcept" }; 3441 case -1035284522: 3442 /* communication */ return new String[] { "CodeableConcept" }; 3443 case -2092740898: 3444 /* referralMethod */ return new String[] { "CodeableConcept" }; 3445 case 427220062: 3446 /* appointmentRequired */ return new String[] { "boolean" }; 3447 case 1873069366: 3448 /* availableTime */ return new String[] {}; 3449 case -629572298: 3450 /* notAvailable */ return new String[] {}; 3451 case -1149143617: 3452 /* availabilityExceptions */ return new String[] { "string" }; 3453 case 1741102485: 3454 /* endpoint */ return new String[] { "Reference" }; 3455 default: 3456 return super.getTypesForProperty(hash, name); 3457 } 3458 3459 } 3460 3461 @Override 3462 public Base addChild(String name) throws FHIRException { 3463 if (name.equals("identifier")) { 3464 return addIdentifier(); 3465 } else if (name.equals("active")) { 3466 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.active"); 3467 } else if (name.equals("providedBy")) { 3468 this.providedBy = new Reference(); 3469 return this.providedBy; 3470 } else if (name.equals("category")) { 3471 return addCategory(); 3472 } else if (name.equals("type")) { 3473 return addType(); 3474 } else if (name.equals("specialty")) { 3475 return addSpecialty(); 3476 } else if (name.equals("location")) { 3477 return addLocation(); 3478 } else if (name.equals("name")) { 3479 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.name"); 3480 } else if (name.equals("comment")) { 3481 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 3482 } else if (name.equals("extraDetails")) { 3483 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.extraDetails"); 3484 } else if (name.equals("photo")) { 3485 this.photo = new Attachment(); 3486 return this.photo; 3487 } else if (name.equals("telecom")) { 3488 return addTelecom(); 3489 } else if (name.equals("coverageArea")) { 3490 return addCoverageArea(); 3491 } else if (name.equals("serviceProvisionCode")) { 3492 return addServiceProvisionCode(); 3493 } else if (name.equals("eligibility")) { 3494 return addEligibility(); 3495 } else if (name.equals("program")) { 3496 return addProgram(); 3497 } else if (name.equals("characteristic")) { 3498 return addCharacteristic(); 3499 } else if (name.equals("communication")) { 3500 return addCommunication(); 3501 } else if (name.equals("referralMethod")) { 3502 return addReferralMethod(); 3503 } else if (name.equals("appointmentRequired")) { 3504 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.appointmentRequired"); 3505 } else if (name.equals("availableTime")) { 3506 return addAvailableTime(); 3507 } else if (name.equals("notAvailable")) { 3508 return addNotAvailable(); 3509 } else if (name.equals("availabilityExceptions")) { 3510 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availabilityExceptions"); 3511 } else if (name.equals("endpoint")) { 3512 return addEndpoint(); 3513 } else 3514 return super.addChild(name); 3515 } 3516 3517 public String fhirType() { 3518 return "HealthcareService"; 3519 3520 } 3521 3522 public HealthcareService copy() { 3523 HealthcareService dst = new HealthcareService(); 3524 copyValues(dst); 3525 return dst; 3526 } 3527 3528 public void copyValues(HealthcareService dst) { 3529 super.copyValues(dst); 3530 if (identifier != null) { 3531 dst.identifier = new ArrayList<Identifier>(); 3532 for (Identifier i : identifier) 3533 dst.identifier.add(i.copy()); 3534 } 3535 ; 3536 dst.active = active == null ? null : active.copy(); 3537 dst.providedBy = providedBy == null ? null : providedBy.copy(); 3538 if (category != null) { 3539 dst.category = new ArrayList<CodeableConcept>(); 3540 for (CodeableConcept i : category) 3541 dst.category.add(i.copy()); 3542 } 3543 ; 3544 if (type != null) { 3545 dst.type = new ArrayList<CodeableConcept>(); 3546 for (CodeableConcept i : type) 3547 dst.type.add(i.copy()); 3548 } 3549 ; 3550 if (specialty != null) { 3551 dst.specialty = new ArrayList<CodeableConcept>(); 3552 for (CodeableConcept i : specialty) 3553 dst.specialty.add(i.copy()); 3554 } 3555 ; 3556 if (location != null) { 3557 dst.location = new ArrayList<Reference>(); 3558 for (Reference i : location) 3559 dst.location.add(i.copy()); 3560 } 3561 ; 3562 dst.name = name == null ? null : name.copy(); 3563 dst.comment = comment == null ? null : comment.copy(); 3564 dst.extraDetails = extraDetails == null ? null : extraDetails.copy(); 3565 dst.photo = photo == null ? null : photo.copy(); 3566 if (telecom != null) { 3567 dst.telecom = new ArrayList<ContactPoint>(); 3568 for (ContactPoint i : telecom) 3569 dst.telecom.add(i.copy()); 3570 } 3571 ; 3572 if (coverageArea != null) { 3573 dst.coverageArea = new ArrayList<Reference>(); 3574 for (Reference i : coverageArea) 3575 dst.coverageArea.add(i.copy()); 3576 } 3577 ; 3578 if (serviceProvisionCode != null) { 3579 dst.serviceProvisionCode = new ArrayList<CodeableConcept>(); 3580 for (CodeableConcept i : serviceProvisionCode) 3581 dst.serviceProvisionCode.add(i.copy()); 3582 } 3583 ; 3584 if (eligibility != null) { 3585 dst.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 3586 for (HealthcareServiceEligibilityComponent i : eligibility) 3587 dst.eligibility.add(i.copy()); 3588 } 3589 ; 3590 if (program != null) { 3591 dst.program = new ArrayList<CodeableConcept>(); 3592 for (CodeableConcept i : program) 3593 dst.program.add(i.copy()); 3594 } 3595 ; 3596 if (characteristic != null) { 3597 dst.characteristic = new ArrayList<CodeableConcept>(); 3598 for (CodeableConcept i : characteristic) 3599 dst.characteristic.add(i.copy()); 3600 } 3601 ; 3602 if (communication != null) { 3603 dst.communication = new ArrayList<CodeableConcept>(); 3604 for (CodeableConcept i : communication) 3605 dst.communication.add(i.copy()); 3606 } 3607 ; 3608 if (referralMethod != null) { 3609 dst.referralMethod = new ArrayList<CodeableConcept>(); 3610 for (CodeableConcept i : referralMethod) 3611 dst.referralMethod.add(i.copy()); 3612 } 3613 ; 3614 dst.appointmentRequired = appointmentRequired == null ? null : appointmentRequired.copy(); 3615 if (availableTime != null) { 3616 dst.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 3617 for (HealthcareServiceAvailableTimeComponent i : availableTime) 3618 dst.availableTime.add(i.copy()); 3619 } 3620 ; 3621 if (notAvailable != null) { 3622 dst.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 3623 for (HealthcareServiceNotAvailableComponent i : notAvailable) 3624 dst.notAvailable.add(i.copy()); 3625 } 3626 ; 3627 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 3628 if (endpoint != null) { 3629 dst.endpoint = new ArrayList<Reference>(); 3630 for (Reference i : endpoint) 3631 dst.endpoint.add(i.copy()); 3632 } 3633 ; 3634 } 3635 3636 protected HealthcareService typedCopy() { 3637 return copy(); 3638 } 3639 3640 @Override 3641 public boolean equalsDeep(Base other_) { 3642 if (!super.equalsDeep(other_)) 3643 return false; 3644 if (!(other_ instanceof HealthcareService)) 3645 return false; 3646 HealthcareService o = (HealthcareService) other_; 3647 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 3648 && compareDeep(providedBy, o.providedBy, true) && compareDeep(category, o.category, true) 3649 && compareDeep(type, o.type, true) && compareDeep(specialty, o.specialty, true) 3650 && compareDeep(location, o.location, true) && compareDeep(name, o.name, true) 3651 && compareDeep(comment, o.comment, true) && compareDeep(extraDetails, o.extraDetails, true) 3652 && compareDeep(photo, o.photo, true) && compareDeep(telecom, o.telecom, true) 3653 && compareDeep(coverageArea, o.coverageArea, true) 3654 && compareDeep(serviceProvisionCode, o.serviceProvisionCode, true) 3655 && compareDeep(eligibility, o.eligibility, true) && compareDeep(program, o.program, true) 3656 && compareDeep(characteristic, o.characteristic, true) && compareDeep(communication, o.communication, true) 3657 && compareDeep(referralMethod, o.referralMethod, true) 3658 && compareDeep(appointmentRequired, o.appointmentRequired, true) 3659 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 3660 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) 3661 && compareDeep(endpoint, o.endpoint, true); 3662 } 3663 3664 @Override 3665 public boolean equalsShallow(Base other_) { 3666 if (!super.equalsShallow(other_)) 3667 return false; 3668 if (!(other_ instanceof HealthcareService)) 3669 return false; 3670 HealthcareService o = (HealthcareService) other_; 3671 return compareValues(active, o.active, true) && compareValues(name, o.name, true) 3672 && compareValues(comment, o.comment, true) && compareValues(extraDetails, o.extraDetails, true) 3673 && compareValues(appointmentRequired, o.appointmentRequired, true) 3674 && compareValues(availabilityExceptions, o.availabilityExceptions, true); 3675 } 3676 3677 public boolean isEmpty() { 3678 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, providedBy, category, type, 3679 specialty, location, name, comment, extraDetails, photo, telecom, coverageArea, serviceProvisionCode, 3680 eligibility, program, characteristic, communication, referralMethod, appointmentRequired, availableTime, 3681 notAvailable, availabilityExceptions, endpoint); 3682 } 3683 3684 @Override 3685 public ResourceType getResourceType() { 3686 return ResourceType.HealthcareService; 3687 } 3688 3689 /** 3690 * Search parameter: <b>identifier</b> 3691 * <p> 3692 * Description: <b>External identifiers for this item</b><br> 3693 * Type: <b>token</b><br> 3694 * Path: <b>HealthcareService.identifier</b><br> 3695 * </p> 3696 */ 3697 @SearchParamDefinition(name = "identifier", path = "HealthcareService.identifier", description = "External identifiers for this item", type = "token") 3698 public static final String SP_IDENTIFIER = "identifier"; 3699 /** 3700 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3701 * <p> 3702 * Description: <b>External identifiers for this item</b><br> 3703 * Type: <b>token</b><br> 3704 * Path: <b>HealthcareService.identifier</b><br> 3705 * </p> 3706 */ 3707 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3708 SP_IDENTIFIER); 3709 3710 /** 3711 * Search parameter: <b>specialty</b> 3712 * <p> 3713 * Description: <b>The specialty of the service provided by this healthcare 3714 * service</b><br> 3715 * Type: <b>token</b><br> 3716 * Path: <b>HealthcareService.specialty</b><br> 3717 * </p> 3718 */ 3719 @SearchParamDefinition(name = "specialty", path = "HealthcareService.specialty", description = "The specialty of the service provided by this healthcare service", type = "token") 3720 public static final String SP_SPECIALTY = "specialty"; 3721 /** 3722 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 3723 * <p> 3724 * Description: <b>The specialty of the service provided by this healthcare 3725 * service</b><br> 3726 * Type: <b>token</b><br> 3727 * Path: <b>HealthcareService.specialty</b><br> 3728 * </p> 3729 */ 3730 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3731 SP_SPECIALTY); 3732 3733 /** 3734 * Search parameter: <b>endpoint</b> 3735 * <p> 3736 * Description: <b>Technical endpoints providing access to electronic services 3737 * operated for the healthcare service</b><br> 3738 * Type: <b>reference</b><br> 3739 * Path: <b>HealthcareService.endpoint</b><br> 3740 * </p> 3741 */ 3742 @SearchParamDefinition(name = "endpoint", path = "HealthcareService.endpoint", description = "Technical endpoints providing access to electronic services operated for the healthcare service", type = "reference", target = { 3743 Endpoint.class }) 3744 public static final String SP_ENDPOINT = "endpoint"; 3745 /** 3746 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 3747 * <p> 3748 * Description: <b>Technical endpoints providing access to electronic services 3749 * operated for the healthcare service</b><br> 3750 * Type: <b>reference</b><br> 3751 * Path: <b>HealthcareService.endpoint</b><br> 3752 * </p> 3753 */ 3754 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3755 SP_ENDPOINT); 3756 3757 /** 3758 * Constant for fluent queries to be used to add include statements. Specifies 3759 * the path value of "<b>HealthcareService:endpoint</b>". 3760 */ 3761 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 3762 "HealthcareService:endpoint").toLocked(); 3763 3764 /** 3765 * Search parameter: <b>service-category</b> 3766 * <p> 3767 * Description: <b>Service Category of the Healthcare Service</b><br> 3768 * Type: <b>token</b><br> 3769 * Path: <b>HealthcareService.category</b><br> 3770 * </p> 3771 */ 3772 @SearchParamDefinition(name = "service-category", path = "HealthcareService.category", description = "Service Category of the Healthcare Service", type = "token") 3773 public static final String SP_SERVICE_CATEGORY = "service-category"; 3774 /** 3775 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 3776 * <p> 3777 * Description: <b>Service Category of the Healthcare Service</b><br> 3778 * Type: <b>token</b><br> 3779 * Path: <b>HealthcareService.category</b><br> 3780 * </p> 3781 */ 3782 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3783 SP_SERVICE_CATEGORY); 3784 3785 /** 3786 * Search parameter: <b>coverage-area</b> 3787 * <p> 3788 * Description: <b>Location(s) service is intended for/available to</b><br> 3789 * Type: <b>reference</b><br> 3790 * Path: <b>HealthcareService.coverageArea</b><br> 3791 * </p> 3792 */ 3793 @SearchParamDefinition(name = "coverage-area", path = "HealthcareService.coverageArea", description = "Location(s) service is intended for/available to", type = "reference", target = { 3794 Location.class }) 3795 public static final String SP_COVERAGE_AREA = "coverage-area"; 3796 /** 3797 * <b>Fluent Client</b> search parameter constant for <b>coverage-area</b> 3798 * <p> 3799 * Description: <b>Location(s) service is intended for/available to</b><br> 3800 * Type: <b>reference</b><br> 3801 * Path: <b>HealthcareService.coverageArea</b><br> 3802 * </p> 3803 */ 3804 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE_AREA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3805 SP_COVERAGE_AREA); 3806 3807 /** 3808 * Constant for fluent queries to be used to add include statements. Specifies 3809 * the path value of "<b>HealthcareService:coverage-area</b>". 3810 */ 3811 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE_AREA = new ca.uhn.fhir.model.api.Include( 3812 "HealthcareService:coverage-area").toLocked(); 3813 3814 /** 3815 * Search parameter: <b>service-type</b> 3816 * <p> 3817 * Description: <b>The type of service provided by this healthcare 3818 * service</b><br> 3819 * Type: <b>token</b><br> 3820 * Path: <b>HealthcareService.type</b><br> 3821 * </p> 3822 */ 3823 @SearchParamDefinition(name = "service-type", path = "HealthcareService.type", description = "The type of service provided by this healthcare service", type = "token") 3824 public static final String SP_SERVICE_TYPE = "service-type"; 3825 /** 3826 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 3827 * <p> 3828 * Description: <b>The type of service provided by this healthcare 3829 * service</b><br> 3830 * Type: <b>token</b><br> 3831 * Path: <b>HealthcareService.type</b><br> 3832 * </p> 3833 */ 3834 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3835 SP_SERVICE_TYPE); 3836 3837 /** 3838 * Search parameter: <b>organization</b> 3839 * <p> 3840 * Description: <b>The organization that provides this Healthcare 3841 * Service</b><br> 3842 * Type: <b>reference</b><br> 3843 * Path: <b>HealthcareService.providedBy</b><br> 3844 * </p> 3845 */ 3846 @SearchParamDefinition(name = "organization", path = "HealthcareService.providedBy", description = "The organization that provides this Healthcare Service", type = "reference", target = { 3847 Organization.class }) 3848 public static final String SP_ORGANIZATION = "organization"; 3849 /** 3850 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3851 * <p> 3852 * Description: <b>The organization that provides this Healthcare 3853 * Service</b><br> 3854 * Type: <b>reference</b><br> 3855 * Path: <b>HealthcareService.providedBy</b><br> 3856 * </p> 3857 */ 3858 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3859 SP_ORGANIZATION); 3860 3861 /** 3862 * Constant for fluent queries to be used to add include statements. Specifies 3863 * the path value of "<b>HealthcareService:organization</b>". 3864 */ 3865 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3866 "HealthcareService:organization").toLocked(); 3867 3868 /** 3869 * Search parameter: <b>name</b> 3870 * <p> 3871 * Description: <b>A portion of the Healthcare service name</b><br> 3872 * Type: <b>string</b><br> 3873 * Path: <b>HealthcareService.name</b><br> 3874 * </p> 3875 */ 3876 @SearchParamDefinition(name = "name", path = "HealthcareService.name", description = "A portion of the Healthcare service name", type = "string") 3877 public static final String SP_NAME = "name"; 3878 /** 3879 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3880 * <p> 3881 * Description: <b>A portion of the Healthcare service name</b><br> 3882 * Type: <b>string</b><br> 3883 * Path: <b>HealthcareService.name</b><br> 3884 * </p> 3885 */ 3886 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3887 SP_NAME); 3888 3889 /** 3890 * Search parameter: <b>active</b> 3891 * <p> 3892 * Description: <b>The Healthcare Service is currently marked as active</b><br> 3893 * Type: <b>token</b><br> 3894 * Path: <b>HealthcareService.active</b><br> 3895 * </p> 3896 */ 3897 @SearchParamDefinition(name = "active", path = "HealthcareService.active", description = "The Healthcare Service is currently marked as active", type = "token") 3898 public static final String SP_ACTIVE = "active"; 3899 /** 3900 * <b>Fluent Client</b> search parameter constant for <b>active</b> 3901 * <p> 3902 * Description: <b>The Healthcare Service is currently marked as active</b><br> 3903 * Type: <b>token</b><br> 3904 * Path: <b>HealthcareService.active</b><br> 3905 * </p> 3906 */ 3907 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3908 SP_ACTIVE); 3909 3910 /** 3911 * Search parameter: <b>location</b> 3912 * <p> 3913 * Description: <b>The location of the Healthcare Service</b><br> 3914 * Type: <b>reference</b><br> 3915 * Path: <b>HealthcareService.location</b><br> 3916 * </p> 3917 */ 3918 @SearchParamDefinition(name = "location", path = "HealthcareService.location", description = "The location of the Healthcare Service", type = "reference", target = { 3919 Location.class }) 3920 public static final String SP_LOCATION = "location"; 3921 /** 3922 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3923 * <p> 3924 * Description: <b>The location of the Healthcare Service</b><br> 3925 * Type: <b>reference</b><br> 3926 * Path: <b>HealthcareService.location</b><br> 3927 * </p> 3928 */ 3929 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3930 SP_LOCATION); 3931 3932 /** 3933 * Constant for fluent queries to be used to add include statements. Specifies 3934 * the path value of "<b>HealthcareService:location</b>". 3935 */ 3936 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 3937 "HealthcareService:location").toLocked(); 3938 3939 /** 3940 * Search parameter: <b>program</b> 3941 * <p> 3942 * Description: <b>One of the Programs supported by this 3943 * HealthcareService</b><br> 3944 * Type: <b>token</b><br> 3945 * Path: <b>HealthcareService.program</b><br> 3946 * </p> 3947 */ 3948 @SearchParamDefinition(name = "program", path = "HealthcareService.program", description = "One of the Programs supported by this HealthcareService", type = "token") 3949 public static final String SP_PROGRAM = "program"; 3950 /** 3951 * <b>Fluent Client</b> search parameter constant for <b>program</b> 3952 * <p> 3953 * Description: <b>One of the Programs supported by this 3954 * HealthcareService</b><br> 3955 * Type: <b>token</b><br> 3956 * Path: <b>HealthcareService.program</b><br> 3957 * </p> 3958 */ 3959 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PROGRAM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3960 SP_PROGRAM); 3961 3962 /** 3963 * Search parameter: <b>characteristic</b> 3964 * <p> 3965 * Description: <b>One of the HealthcareService's characteristics</b><br> 3966 * Type: <b>token</b><br> 3967 * Path: <b>HealthcareService.characteristic</b><br> 3968 * </p> 3969 */ 3970 @SearchParamDefinition(name = "characteristic", path = "HealthcareService.characteristic", description = "One of the HealthcareService's characteristics", type = "token") 3971 public static final String SP_CHARACTERISTIC = "characteristic"; 3972 /** 3973 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 3974 * <p> 3975 * Description: <b>One of the HealthcareService's characteristics</b><br> 3976 * Type: <b>token</b><br> 3977 * Path: <b>HealthcareService.characteristic</b><br> 3978 * </p> 3979 */ 3980 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3981 SP_CHARACTERISTIC); 3982 3983}