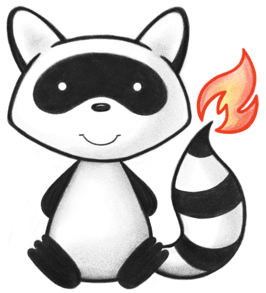
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.instance.model.api.IPrimitiveType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.util.DatatypeUtil; 045 046/** 047 * A human's name with the ability to identify parts and usage. 048 */ 049@DatatypeDef(name = "HumanName") 050public class HumanName extends Type implements ICompositeType { 051 052 public enum NameUse { 053 /** 054 * Known as/conventional/the one you normally use. 055 */ 056 USUAL, 057 /** 058 * The formal name as registered in an official (government) registry, but which 059 * name might not be commonly used. May be called "legal name". 060 */ 061 OFFICIAL, 062 /** 063 * A temporary name. Name.period can provide more detailed information. This may 064 * also be used for temporary names assigned at birth or in emergency 065 * situations. 066 */ 067 TEMP, 068 /** 069 * A name that is used to address the person in an informal manner, but is not 070 * part of their formal or usual name. 071 */ 072 NICKNAME, 073 /** 074 * Anonymous assigned name, alias, or pseudonym (used to protect a person's 075 * identity for privacy reasons). 076 */ 077 ANONYMOUS, 078 /** 079 * This name is no longer in use (or was never correct, but retained for 080 * records). 081 */ 082 OLD, 083 /** 084 * A name used prior to changing name because of marriage. This name use is for 085 * use by applications that collect and store names that were used prior to a 086 * marriage. Marriage naming customs vary greatly around the world, and are 087 * constantly changing. This term is not gender specific. The use of this term 088 * does not imply any particular history for a person's name. 089 */ 090 MAIDEN, 091 /** 092 * added to help the parsers with the generic types 093 */ 094 NULL; 095 096 public static NameUse fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("usual".equals(codeString)) 100 return USUAL; 101 if ("official".equals(codeString)) 102 return OFFICIAL; 103 if ("temp".equals(codeString)) 104 return TEMP; 105 if ("nickname".equals(codeString)) 106 return NICKNAME; 107 if ("anonymous".equals(codeString)) 108 return ANONYMOUS; 109 if ("old".equals(codeString)) 110 return OLD; 111 if ("maiden".equals(codeString)) 112 return MAIDEN; 113 if (Configuration.isAcceptInvalidEnums()) 114 return null; 115 else 116 throw new FHIRException("Unknown NameUse code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case USUAL: 122 return "usual"; 123 case OFFICIAL: 124 return "official"; 125 case TEMP: 126 return "temp"; 127 case NICKNAME: 128 return "nickname"; 129 case ANONYMOUS: 130 return "anonymous"; 131 case OLD: 132 return "old"; 133 case MAIDEN: 134 return "maiden"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getSystem() { 143 switch (this) { 144 case USUAL: 145 return "http://hl7.org/fhir/name-use"; 146 case OFFICIAL: 147 return "http://hl7.org/fhir/name-use"; 148 case TEMP: 149 return "http://hl7.org/fhir/name-use"; 150 case NICKNAME: 151 return "http://hl7.org/fhir/name-use"; 152 case ANONYMOUS: 153 return "http://hl7.org/fhir/name-use"; 154 case OLD: 155 return "http://hl7.org/fhir/name-use"; 156 case MAIDEN: 157 return "http://hl7.org/fhir/name-use"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDefinition() { 166 switch (this) { 167 case USUAL: 168 return "Known as/conventional/the one you normally use."; 169 case OFFICIAL: 170 return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 171 case TEMP: 172 return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 173 case NICKNAME: 174 return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name."; 175 case ANONYMOUS: 176 return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)."; 177 case OLD: 178 return "This name is no longer in use (or was never correct, but retained for records)."; 179 case MAIDEN: 180 return "A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name."; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188 public String getDisplay() { 189 switch (this) { 190 case USUAL: 191 return "Usual"; 192 case OFFICIAL: 193 return "Official"; 194 case TEMP: 195 return "Temp"; 196 case NICKNAME: 197 return "Nickname"; 198 case ANONYMOUS: 199 return "Anonymous"; 200 case OLD: 201 return "Old"; 202 case MAIDEN: 203 return "Name changed for Marriage"; 204 case NULL: 205 return null; 206 default: 207 return "?"; 208 } 209 } 210 } 211 212 public static class NameUseEnumFactory implements EnumFactory<NameUse> { 213 public NameUse fromCode(String codeString) throws IllegalArgumentException { 214 if (codeString == null || "".equals(codeString)) 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("usual".equals(codeString)) 218 return NameUse.USUAL; 219 if ("official".equals(codeString)) 220 return NameUse.OFFICIAL; 221 if ("temp".equals(codeString)) 222 return NameUse.TEMP; 223 if ("nickname".equals(codeString)) 224 return NameUse.NICKNAME; 225 if ("anonymous".equals(codeString)) 226 return NameUse.ANONYMOUS; 227 if ("old".equals(codeString)) 228 return NameUse.OLD; 229 if ("maiden".equals(codeString)) 230 return NameUse.MAIDEN; 231 throw new IllegalArgumentException("Unknown NameUse code '" + codeString + "'"); 232 } 233 234 public Enumeration<NameUse> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<NameUse>(this, NameUse.NULL, code); 239 String codeString = code.asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<NameUse>(this, NameUse.NULL, code); 242 if ("usual".equals(codeString)) 243 return new Enumeration<NameUse>(this, NameUse.USUAL, code); 244 if ("official".equals(codeString)) 245 return new Enumeration<NameUse>(this, NameUse.OFFICIAL, code); 246 if ("temp".equals(codeString)) 247 return new Enumeration<NameUse>(this, NameUse.TEMP, code); 248 if ("nickname".equals(codeString)) 249 return new Enumeration<NameUse>(this, NameUse.NICKNAME, code); 250 if ("anonymous".equals(codeString)) 251 return new Enumeration<NameUse>(this, NameUse.ANONYMOUS, code); 252 if ("old".equals(codeString)) 253 return new Enumeration<NameUse>(this, NameUse.OLD, code); 254 if ("maiden".equals(codeString)) 255 return new Enumeration<NameUse>(this, NameUse.MAIDEN, code); 256 throw new FHIRException("Unknown NameUse code '" + codeString + "'"); 257 } 258 259 public String toCode(NameUse code) { 260 if (code == NameUse.NULL) 261 return null; 262 if (code == NameUse.USUAL) 263 return "usual"; 264 if (code == NameUse.OFFICIAL) 265 return "official"; 266 if (code == NameUse.TEMP) 267 return "temp"; 268 if (code == NameUse.NICKNAME) 269 return "nickname"; 270 if (code == NameUse.ANONYMOUS) 271 return "anonymous"; 272 if (code == NameUse.OLD) 273 return "old"; 274 if (code == NameUse.MAIDEN) 275 return "maiden"; 276 return "?"; 277 } 278 279 public String toSystem(NameUse code) { 280 return code.getSystem(); 281 } 282 } 283 284 /** 285 * Identifies the purpose for this name. 286 */ 287 @Child(name = "use", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = true, summary = true) 288 @Description(shortDefinition = "usual | official | temp | nickname | anonymous | old | maiden", formalDefinition = "Identifies the purpose for this name.") 289 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/name-use") 290 protected Enumeration<NameUse> use; 291 292 /** 293 * Specifies the entire name as it should be displayed e.g. on an application 294 * UI. This may be provided instead of or as well as the specific parts. 295 */ 296 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 297 @Description(shortDefinition = "Text representation of the full name", formalDefinition = "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.") 298 protected StringType text; 299 300 /** 301 * The part of a name that links to the genealogy. In some cultures (e.g. 302 * Eritrea) the family name of a son is the first name of his father. 303 */ 304 @Child(name = "family", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 305 @Description(shortDefinition = "Family name (often called 'Surname')", formalDefinition = "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.") 306 protected StringType family; 307 308 /** 309 * Given name. 310 */ 311 @Child(name = "given", type = { 312 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 313 @Description(shortDefinition = "Given names (not always 'first'). Includes middle names", formalDefinition = "Given name.") 314 protected List<StringType> given; 315 316 /** 317 * Part of the name that is acquired as a title due to academic, legal, 318 * employment or nobility status, etc. and that appears at the start of the 319 * name. 320 */ 321 @Child(name = "prefix", type = { 322 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 323 @Description(shortDefinition = "Parts that come before the name", formalDefinition = "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.") 324 protected List<StringType> prefix; 325 326 /** 327 * Part of the name that is acquired as a title due to academic, legal, 328 * employment or nobility status, etc. and that appears at the end of the name. 329 */ 330 @Child(name = "suffix", type = { 331 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 332 @Description(shortDefinition = "Parts that come after the name", formalDefinition = "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.") 333 protected List<StringType> suffix; 334 335 /** 336 * Indicates the period of time when this name was valid for the named person. 337 */ 338 @Child(name = "period", type = { Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 339 @Description(shortDefinition = "Time period when name was/is in use", formalDefinition = "Indicates the period of time when this name was valid for the named person.") 340 protected Period period; 341 342 private static final long serialVersionUID = -507469160L; 343 344 /** 345 * Constructor 346 */ 347 public HumanName() { 348 super(); 349 } 350 351 /** 352 * @return {@link #use} (Identifies the purpose for this name.). This is the 353 * underlying object with id, value and extensions. The accessor 354 * "getUse" gives direct access to the value 355 */ 356 public Enumeration<NameUse> getUseElement() { 357 if (this.use == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create HumanName.use"); 360 else if (Configuration.doAutoCreate()) 361 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); // bb 362 return this.use; 363 } 364 365 public boolean hasUseElement() { 366 return this.use != null && !this.use.isEmpty(); 367 } 368 369 public boolean hasUse() { 370 return this.use != null && !this.use.isEmpty(); 371 } 372 373 /** 374 * @param value {@link #use} (Identifies the purpose for this name.). This is 375 * the underlying object with id, value and extensions. The 376 * accessor "getUse" gives direct access to the value 377 */ 378 public HumanName setUseElement(Enumeration<NameUse> value) { 379 this.use = value; 380 return this; 381 } 382 383 /** 384 * @return Identifies the purpose for this name. 385 */ 386 public NameUse getUse() { 387 return this.use == null ? null : this.use.getValue(); 388 } 389 390 /** 391 * @param value Identifies the purpose for this name. 392 */ 393 public HumanName setUse(NameUse value) { 394 if (value == null) 395 this.use = null; 396 else { 397 if (this.use == null) 398 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); 399 this.use.setValue(value); 400 } 401 return this; 402 } 403 404 /** 405 * @return {@link #text} (Specifies the entire name as it should be displayed 406 * e.g. on an application UI. This may be provided instead of or as well 407 * as the specific parts.). This is the underlying object with id, value 408 * and extensions. The accessor "getText" gives direct access to the 409 * value 410 */ 411 public StringType getTextElement() { 412 if (this.text == null) 413 if (Configuration.errorOnAutoCreate()) 414 throw new Error("Attempt to auto-create HumanName.text"); 415 else if (Configuration.doAutoCreate()) 416 this.text = new StringType(); // bb 417 return this.text; 418 } 419 420 public boolean hasTextElement() { 421 return this.text != null && !this.text.isEmpty(); 422 } 423 424 public boolean hasText() { 425 return this.text != null && !this.text.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #text} (Specifies the entire name as it should be 430 * displayed e.g. on an application UI. This may be provided 431 * instead of or as well as the specific parts.). This is the 432 * underlying object with id, value and extensions. The accessor 433 * "getText" gives direct access to the value 434 */ 435 public HumanName setTextElement(StringType value) { 436 this.text = value; 437 return this; 438 } 439 440 /** 441 * @return Specifies the entire name as it should be displayed e.g. on an 442 * application UI. This may be provided instead of or as well as the 443 * specific parts. 444 */ 445 public String getText() { 446 return this.text == null ? null : this.text.getValue(); 447 } 448 449 /** 450 * @param value Specifies the entire name as it should be displayed e.g. on an 451 * application UI. This may be provided instead of or as well as 452 * the specific parts. 453 */ 454 public HumanName setText(String value) { 455 if (Utilities.noString(value)) 456 this.text = null; 457 else { 458 if (this.text == null) 459 this.text = new StringType(); 460 this.text.setValue(value); 461 } 462 return this; 463 } 464 465 /** 466 * @return {@link #family} (The part of a name that links to the genealogy. In 467 * some cultures (e.g. Eritrea) the family name of a son is the first 468 * name of his father.). This is the underlying object with id, value 469 * and extensions. The accessor "getFamily" gives direct access to the 470 * value 471 */ 472 public StringType getFamilyElement() { 473 if (this.family == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create HumanName.family"); 476 else if (Configuration.doAutoCreate()) 477 this.family = new StringType(); // bb 478 return this.family; 479 } 480 481 public boolean hasFamilyElement() { 482 return this.family != null && !this.family.isEmpty(); 483 } 484 485 public boolean hasFamily() { 486 return this.family != null && !this.family.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #family} (The part of a name that links to the genealogy. 491 * In some cultures (e.g. Eritrea) the family name of a son is the 492 * first name of his father.). This is the underlying object with 493 * id, value and extensions. The accessor "getFamily" gives direct 494 * access to the value 495 */ 496 public HumanName setFamilyElement(StringType value) { 497 this.family = value; 498 return this; 499 } 500 501 /** 502 * @return The part of a name that links to the genealogy. In some cultures 503 * (e.g. Eritrea) the family name of a son is the first name of his 504 * father. 505 */ 506 public String getFamily() { 507 return this.family == null ? null : this.family.getValue(); 508 } 509 510 /** 511 * @param value The part of a name that links to the genealogy. In some cultures 512 * (e.g. Eritrea) the family name of a son is the first name of his 513 * father. 514 */ 515 public HumanName setFamily(String value) { 516 if (Utilities.noString(value)) 517 this.family = null; 518 else { 519 if (this.family == null) 520 this.family = new StringType(); 521 this.family.setValue(value); 522 } 523 return this; 524 } 525 526 /** 527 * @return {@link #given} (Given name.) 528 */ 529 public List<StringType> getGiven() { 530 if (this.given == null) 531 this.given = new ArrayList<StringType>(); 532 return this.given; 533 } 534 535 /** 536 * @return Returns a reference to <code>this</code> for easy method chaining 537 */ 538 public HumanName setGiven(List<StringType> theGiven) { 539 this.given = theGiven; 540 return this; 541 } 542 543 public boolean hasGiven() { 544 if (this.given == null) 545 return false; 546 for (StringType item : this.given) 547 if (!item.isEmpty()) 548 return true; 549 return false; 550 } 551 552 /** 553 * @return {@link #given} (Given name.) 554 */ 555 public StringType addGivenElement() {// 2 556 StringType t = new StringType(); 557 if (this.given == null) 558 this.given = new ArrayList<StringType>(); 559 this.given.add(t); 560 return t; 561 } 562 563 /** 564 * @param value {@link #given} (Given name.) 565 */ 566 public HumanName addGiven(String value) { // 1 567 StringType t = new StringType(); 568 t.setValue(value); 569 if (this.given == null) 570 this.given = new ArrayList<StringType>(); 571 this.given.add(t); 572 return this; 573 } 574 575 /** 576 * @param value {@link #given} (Given name.) 577 */ 578 public boolean hasGiven(String value) { 579 if (this.given == null) 580 return false; 581 for (StringType v : this.given) 582 if (v.getValue().equals(value)) // string 583 return true; 584 return false; 585 } 586 587 /** 588 * @return {@link #prefix} (Part of the name that is acquired as a title due to 589 * academic, legal, employment or nobility status, etc. and that appears 590 * at the start of the name.) 591 */ 592 public List<StringType> getPrefix() { 593 if (this.prefix == null) 594 this.prefix = new ArrayList<StringType>(); 595 return this.prefix; 596 } 597 598 /** 599 * @return Returns a reference to <code>this</code> for easy method chaining 600 */ 601 public HumanName setPrefix(List<StringType> thePrefix) { 602 this.prefix = thePrefix; 603 return this; 604 } 605 606 public boolean hasPrefix() { 607 if (this.prefix == null) 608 return false; 609 for (StringType item : this.prefix) 610 if (!item.isEmpty()) 611 return true; 612 return false; 613 } 614 615 /** 616 * @return {@link #prefix} (Part of the name that is acquired as a title due to 617 * academic, legal, employment or nobility status, etc. and that appears 618 * at the start of the name.) 619 */ 620 public StringType addPrefixElement() {// 2 621 StringType t = new StringType(); 622 if (this.prefix == null) 623 this.prefix = new ArrayList<StringType>(); 624 this.prefix.add(t); 625 return t; 626 } 627 628 /** 629 * @param value {@link #prefix} (Part of the name that is acquired as a title 630 * due to academic, legal, employment or nobility status, etc. and 631 * that appears at the start of the name.) 632 */ 633 public HumanName addPrefix(String value) { // 1 634 StringType t = new StringType(); 635 t.setValue(value); 636 if (this.prefix == null) 637 this.prefix = new ArrayList<StringType>(); 638 this.prefix.add(t); 639 return this; 640 } 641 642 /** 643 * @param value {@link #prefix} (Part of the name that is acquired as a title 644 * due to academic, legal, employment or nobility status, etc. and 645 * that appears at the start of the name.) 646 */ 647 public boolean hasPrefix(String value) { 648 if (this.prefix == null) 649 return false; 650 for (StringType v : this.prefix) 651 if (v.getValue().equals(value)) // string 652 return true; 653 return false; 654 } 655 656 /** 657 * @return {@link #suffix} (Part of the name that is acquired as a title due to 658 * academic, legal, employment or nobility status, etc. and that appears 659 * at the end of the name.) 660 */ 661 public List<StringType> getSuffix() { 662 if (this.suffix == null) 663 this.suffix = new ArrayList<StringType>(); 664 return this.suffix; 665 } 666 667 /** 668 * @return Returns a reference to <code>this</code> for easy method chaining 669 */ 670 public HumanName setSuffix(List<StringType> theSuffix) { 671 this.suffix = theSuffix; 672 return this; 673 } 674 675 public boolean hasSuffix() { 676 if (this.suffix == null) 677 return false; 678 for (StringType item : this.suffix) 679 if (!item.isEmpty()) 680 return true; 681 return false; 682 } 683 684 /** 685 * @return {@link #suffix} (Part of the name that is acquired as a title due to 686 * academic, legal, employment or nobility status, etc. and that appears 687 * at the end of the name.) 688 */ 689 public StringType addSuffixElement() {// 2 690 StringType t = new StringType(); 691 if (this.suffix == null) 692 this.suffix = new ArrayList<StringType>(); 693 this.suffix.add(t); 694 return t; 695 } 696 697 /** 698 * @param value {@link #suffix} (Part of the name that is acquired as a title 699 * due to academic, legal, employment or nobility status, etc. and 700 * that appears at the end of the name.) 701 */ 702 public HumanName addSuffix(String value) { // 1 703 StringType t = new StringType(); 704 t.setValue(value); 705 if (this.suffix == null) 706 this.suffix = new ArrayList<StringType>(); 707 this.suffix.add(t); 708 return this; 709 } 710 711 /** 712 * @param value {@link #suffix} (Part of the name that is acquired as a title 713 * due to academic, legal, employment or nobility status, etc. and 714 * that appears at the end of the name.) 715 */ 716 public boolean hasSuffix(String value) { 717 if (this.suffix == null) 718 return false; 719 for (StringType v : this.suffix) 720 if (v.getValue().equals(value)) // string 721 return true; 722 return false; 723 } 724 725 /** 726 * @return {@link #period} (Indicates the period of time when this name was 727 * valid for the named person.) 728 */ 729 public Period getPeriod() { 730 if (this.period == null) 731 if (Configuration.errorOnAutoCreate()) 732 throw new Error("Attempt to auto-create HumanName.period"); 733 else if (Configuration.doAutoCreate()) 734 this.period = new Period(); // cc 735 return this.period; 736 } 737 738 public boolean hasPeriod() { 739 return this.period != null && !this.period.isEmpty(); 740 } 741 742 /** 743 * @param value {@link #period} (Indicates the period of time when this name was 744 * valid for the named person.) 745 */ 746 public HumanName setPeriod(Period value) { 747 this.period = value; 748 return this; 749 } 750 751 /** 752 * /** Returns all repetitions of {@link #getGiven() given name} as a space 753 * separated string 754 * 755 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 756 */ 757 public String getGivenAsSingleString() { 758 return joinStringsSpaceSeparated(getGiven()); 759 } 760 761 /** 762 * Returns all repetitions of {@link #getPrefix() prefix name} as a space 763 * separated string 764 * 765 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 766 */ 767 public String getPrefixAsSingleString() { 768 return joinStringsSpaceSeparated(getPrefix()); 769 } 770 771 /** 772 * Returns all repetitions of {@link #getSuffix() suffix} as a space separated 773 * string 774 * 775 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 776 */ 777 public String getSuffixAsSingleString() { 778 return joinStringsSpaceSeparated(getSuffix()); 779 } 780 781 /** 782 * <p> 783 * Returns the {@link #getTextElement() text} element value if it is not null. 784 * </p> 785 * 786 * <p> 787 * If the {@link #getTextElement() text} element value is null, returns all the 788 * components of the name (prefix, given, family, suffix) as a single string 789 * with a single spaced string separating each part. 790 * </p> 791 * 792 * @return the human name as a single string 793 */ 794 public String getNameAsSingleString() { 795 if (hasText()) { 796 return getText().toString(); 797 } 798 799 List<StringType> nameParts = new ArrayList<StringType>(); 800 nameParts.addAll(getPrefix()); 801 nameParts.addAll(getGiven()); 802 if (hasFamilyElement()) { 803 nameParts.add(getFamilyElement()); 804 } 805 nameParts.addAll(getSuffix()); 806 if (nameParts.size() > 0) { 807 return joinStringsSpaceSeparated(nameParts); 808 } else { 809 return getTextElement().getValue(); 810 } 811 } 812 813 /** 814 * Joins a list of strings with a single space (' ') between each string 815 * 816 * TODO: replace with call to 817 * ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated when HAPI upgrades to 818 * 1.4 819 */ 820 private static String joinStringsSpaceSeparated(List<? extends IPrimitiveType<String>> theStrings) { 821 StringBuilder stringBuilder = new StringBuilder(); 822 for (IPrimitiveType<String> string : theStrings) { 823 if (string.isEmpty()) { 824 continue; 825 } 826 if (stringBuilder.length() > 0) { 827 stringBuilder.append(' '); 828 } 829 stringBuilder.append(string.getValue()); 830 } 831 return stringBuilder.toString(); 832 } 833 834 protected void listChildren(List<Property> children) { 835 super.listChildren(children); 836 children.add(new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use)); 837 children.add(new Property("text", "string", 838 "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.", 839 0, 1, text)); 840 children.add(new Property("family", "string", 841 "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 842 0, 1, family)); 843 children.add(new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given)); 844 children.add(new Property("prefix", "string", 845 "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 846 0, java.lang.Integer.MAX_VALUE, prefix)); 847 children.add(new Property("suffix", "string", 848 "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 849 0, java.lang.Integer.MAX_VALUE, suffix)); 850 children.add(new Property("period", "Period", 851 "Indicates the period of time when this name was valid for the named person.", 0, 1, period)); 852 } 853 854 @Override 855 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 856 switch (_hash) { 857 case 116103: 858 /* use */ return new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use); 859 case 3556653: 860 /* text */ return new Property("text", "string", 861 "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.", 862 0, 1, text); 863 case -1281860764: 864 /* family */ return new Property("family", "string", 865 "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 866 0, 1, family); 867 case 98367357: 868 /* given */ return new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given); 869 case -980110702: 870 /* prefix */ return new Property("prefix", "string", 871 "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 872 0, java.lang.Integer.MAX_VALUE, prefix); 873 case -891422895: 874 /* suffix */ return new Property("suffix", "string", 875 "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 876 0, java.lang.Integer.MAX_VALUE, suffix); 877 case -991726143: 878 /* period */ return new Property("period", "Period", 879 "Indicates the period of time when this name was valid for the named person.", 0, 1, period); 880 default: 881 return super.getNamedProperty(_hash, _name, _checkValid); 882 } 883 884 } 885 886 @Override 887 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 888 switch (hash) { 889 case 116103: 890 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<NameUse> 891 case 3556653: 892 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 893 case -1281860764: 894 /* family */ return this.family == null ? new Base[0] : new Base[] { this.family }; // StringType 895 case 98367357: 896 /* given */ return this.given == null ? new Base[0] : this.given.toArray(new Base[this.given.size()]); // StringType 897 case -980110702: 898 /* prefix */ return this.prefix == null ? new Base[0] : this.prefix.toArray(new Base[this.prefix.size()]); // StringType 899 case -891422895: 900 /* suffix */ return this.suffix == null ? new Base[0] : this.suffix.toArray(new Base[this.suffix.size()]); // StringType 901 case -991726143: 902 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 903 default: 904 return super.getProperty(hash, name, checkValid); 905 } 906 907 } 908 909 @Override 910 public Base setProperty(int hash, String name, Base value) throws FHIRException { 911 switch (hash) { 912 case 116103: // use 913 value = new NameUseEnumFactory().fromType(castToCode(value)); 914 this.use = (Enumeration) value; // Enumeration<NameUse> 915 return value; 916 case 3556653: // text 917 this.text = castToString(value); // StringType 918 return value; 919 case -1281860764: // family 920 this.family = castToString(value); // StringType 921 return value; 922 case 98367357: // given 923 this.getGiven().add(castToString(value)); // StringType 924 return value; 925 case -980110702: // prefix 926 this.getPrefix().add(castToString(value)); // StringType 927 return value; 928 case -891422895: // suffix 929 this.getSuffix().add(castToString(value)); // StringType 930 return value; 931 case -991726143: // period 932 this.period = castToPeriod(value); // Period 933 return value; 934 default: 935 return super.setProperty(hash, name, value); 936 } 937 938 } 939 940 @Override 941 public Base setProperty(String name, Base value) throws FHIRException { 942 if (name.equals("use")) { 943 value = new NameUseEnumFactory().fromType(castToCode(value)); 944 this.use = (Enumeration) value; // Enumeration<NameUse> 945 } else if (name.equals("text")) { 946 this.text = castToString(value); // StringType 947 } else if (name.equals("family")) { 948 this.family = castToString(value); // StringType 949 } else if (name.equals("given")) { 950 this.getGiven().add(castToString(value)); 951 } else if (name.equals("prefix")) { 952 this.getPrefix().add(castToString(value)); 953 } else if (name.equals("suffix")) { 954 this.getSuffix().add(castToString(value)); 955 } else if (name.equals("period")) { 956 this.period = castToPeriod(value); // Period 957 } else 958 return super.setProperty(name, value); 959 return value; 960 } 961 962 @Override 963 public void removeChild(String name, Base value) throws FHIRException { 964 if (name.equals("use")) { 965 this.use = null; 966 } else if (name.equals("text")) { 967 this.text = null; 968 } else if (name.equals("family")) { 969 this.family = null; 970 } else if (name.equals("given")) { 971 this.getGiven().remove(castToString(value)); 972 } else if (name.equals("prefix")) { 973 this.getPrefix().remove(castToString(value)); 974 } else if (name.equals("suffix")) { 975 this.getSuffix().remove(castToString(value)); 976 } else if (name.equals("period")) { 977 this.period = null; 978 } else 979 super.removeChild(name, value); 980 981 } 982 983 @Override 984 public Base makeProperty(int hash, String name) throws FHIRException { 985 switch (hash) { 986 case 116103: 987 return getUseElement(); 988 case 3556653: 989 return getTextElement(); 990 case -1281860764: 991 return getFamilyElement(); 992 case 98367357: 993 return addGivenElement(); 994 case -980110702: 995 return addPrefixElement(); 996 case -891422895: 997 return addSuffixElement(); 998 case -991726143: 999 return getPeriod(); 1000 default: 1001 return super.makeProperty(hash, name); 1002 } 1003 1004 } 1005 1006 @Override 1007 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1008 switch (hash) { 1009 case 116103: 1010 /* use */ return new String[] { "code" }; 1011 case 3556653: 1012 /* text */ return new String[] { "string" }; 1013 case -1281860764: 1014 /* family */ return new String[] { "string" }; 1015 case 98367357: 1016 /* given */ return new String[] { "string" }; 1017 case -980110702: 1018 /* prefix */ return new String[] { "string" }; 1019 case -891422895: 1020 /* suffix */ return new String[] { "string" }; 1021 case -991726143: 1022 /* period */ return new String[] { "Period" }; 1023 default: 1024 return super.getTypesForProperty(hash, name); 1025 } 1026 1027 } 1028 1029 @Override 1030 public Base addChild(String name) throws FHIRException { 1031 if (name.equals("use")) { 1032 throw new FHIRException("Cannot call addChild on a singleton property HumanName.use"); 1033 } else if (name.equals("text")) { 1034 throw new FHIRException("Cannot call addChild on a singleton property HumanName.text"); 1035 } else if (name.equals("family")) { 1036 throw new FHIRException("Cannot call addChild on a singleton property HumanName.family"); 1037 } else if (name.equals("given")) { 1038 throw new FHIRException("Cannot call addChild on a singleton property HumanName.given"); 1039 } else if (name.equals("prefix")) { 1040 throw new FHIRException("Cannot call addChild on a singleton property HumanName.prefix"); 1041 } else if (name.equals("suffix")) { 1042 throw new FHIRException("Cannot call addChild on a singleton property HumanName.suffix"); 1043 } else if (name.equals("period")) { 1044 this.period = new Period(); 1045 return this.period; 1046 } else 1047 return super.addChild(name); 1048 } 1049 1050 public String fhirType() { 1051 return "HumanName"; 1052 1053 } 1054 1055 public HumanName copy() { 1056 HumanName dst = new HumanName(); 1057 copyValues(dst); 1058 return dst; 1059 } 1060 1061 public void copyValues(HumanName dst) { 1062 super.copyValues(dst); 1063 dst.use = use == null ? null : use.copy(); 1064 dst.text = text == null ? null : text.copy(); 1065 dst.family = family == null ? null : family.copy(); 1066 if (given != null) { 1067 dst.given = new ArrayList<StringType>(); 1068 for (StringType i : given) 1069 dst.given.add(i.copy()); 1070 } 1071 ; 1072 if (prefix != null) { 1073 dst.prefix = new ArrayList<StringType>(); 1074 for (StringType i : prefix) 1075 dst.prefix.add(i.copy()); 1076 } 1077 ; 1078 if (suffix != null) { 1079 dst.suffix = new ArrayList<StringType>(); 1080 for (StringType i : suffix) 1081 dst.suffix.add(i.copy()); 1082 } 1083 ; 1084 dst.period = period == null ? null : period.copy(); 1085 } 1086 1087 protected HumanName typedCopy() { 1088 return copy(); 1089 } 1090 1091 @Override 1092 public boolean equalsDeep(Base other_) { 1093 if (!super.equalsDeep(other_)) 1094 return false; 1095 if (!(other_ instanceof HumanName)) 1096 return false; 1097 HumanName o = (HumanName) other_; 1098 return compareDeep(use, o.use, true) && compareDeep(text, o.text, true) && compareDeep(family, o.family, true) 1099 && compareDeep(given, o.given, true) && compareDeep(prefix, o.prefix, true) 1100 && compareDeep(suffix, o.suffix, true) && compareDeep(period, o.period, true); 1101 } 1102 1103 @Override 1104 public boolean equalsShallow(Base other_) { 1105 if (!super.equalsShallow(other_)) 1106 return false; 1107 if (!(other_ instanceof HumanName)) 1108 return false; 1109 HumanName o = (HumanName) other_; 1110 return compareValues(use, o.use, true) && compareValues(text, o.text, true) && compareValues(family, o.family, true) 1111 && compareValues(given, o.given, true) && compareValues(prefix, o.prefix, true) 1112 && compareValues(suffix, o.suffix, true); 1113 } 1114 1115 public boolean isEmpty() { 1116 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, text, family, given, prefix, suffix, period); 1117 } 1118 1119}