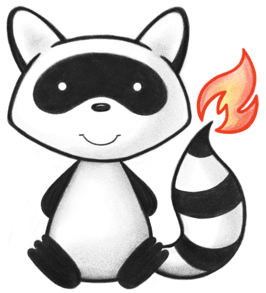
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * An identifier - identifies some entity uniquely and unambiguously. Typically 045 * this is used for business identifiers. 046 */ 047@DatatypeDef(name = "Identifier") 048public class Identifier extends Type implements ICompositeType { 049 050 public enum IdentifierUse { 051 /** 052 * The identifier recommended for display and use in real-world interactions. 053 */ 054 USUAL, 055 /** 056 * The identifier considered to be most trusted for the identification of this 057 * item. Sometimes also known as "primary" and "main". The determination of 058 * "official" is subjective and implementation guides often provide additional 059 * guidelines for use. 060 */ 061 OFFICIAL, 062 /** 063 * A temporary identifier. 064 */ 065 TEMP, 066 /** 067 * An identifier that was assigned in secondary use - it serves to identify the 068 * object in a relative context, but cannot be consistently assigned to the same 069 * object again in a different context. 070 */ 071 SECONDARY, 072 /** 073 * The identifier id no longer considered valid, but may be relevant for search 074 * purposes. E.g. Changes to identifier schemes, account merges, etc. 075 */ 076 OLD, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static IdentifierUse fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("usual".equals(codeString)) 086 return USUAL; 087 if ("official".equals(codeString)) 088 return OFFICIAL; 089 if ("temp".equals(codeString)) 090 return TEMP; 091 if ("secondary".equals(codeString)) 092 return SECONDARY; 093 if ("old".equals(codeString)) 094 return OLD; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown IdentifierUse code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case USUAL: 104 return "usual"; 105 case OFFICIAL: 106 return "official"; 107 case TEMP: 108 return "temp"; 109 case SECONDARY: 110 return "secondary"; 111 case OLD: 112 return "old"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case USUAL: 123 return "http://hl7.org/fhir/identifier-use"; 124 case OFFICIAL: 125 return "http://hl7.org/fhir/identifier-use"; 126 case TEMP: 127 return "http://hl7.org/fhir/identifier-use"; 128 case SECONDARY: 129 return "http://hl7.org/fhir/identifier-use"; 130 case OLD: 131 return "http://hl7.org/fhir/identifier-use"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case USUAL: 142 return "The identifier recommended for display and use in real-world interactions."; 143 case OFFICIAL: 144 return "The identifier considered to be most trusted for the identification of this item. Sometimes also known as \"primary\" and \"main\". The determination of \"official\" is subjective and implementation guides often provide additional guidelines for use."; 145 case TEMP: 146 return "A temporary identifier."; 147 case SECONDARY: 148 return "An identifier that was assigned in secondary use - it serves to identify the object in a relative context, but cannot be consistently assigned to the same object again in a different context."; 149 case OLD: 150 return "The identifier id no longer considered valid, but may be relevant for search purposes. E.g. Changes to identifier schemes, account merges, etc."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case USUAL: 161 return "Usual"; 162 case OFFICIAL: 163 return "Official"; 164 case TEMP: 165 return "Temp"; 166 case SECONDARY: 167 return "Secondary"; 168 case OLD: 169 return "Old"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class IdentifierUseEnumFactory implements EnumFactory<IdentifierUse> { 179 public IdentifierUse fromCode(String codeString) throws IllegalArgumentException { 180 if (codeString == null || "".equals(codeString)) 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("usual".equals(codeString)) 184 return IdentifierUse.USUAL; 185 if ("official".equals(codeString)) 186 return IdentifierUse.OFFICIAL; 187 if ("temp".equals(codeString)) 188 return IdentifierUse.TEMP; 189 if ("secondary".equals(codeString)) 190 return IdentifierUse.SECONDARY; 191 if ("old".equals(codeString)) 192 return IdentifierUse.OLD; 193 throw new IllegalArgumentException("Unknown IdentifierUse code '" + codeString + "'"); 194 } 195 196 public Enumeration<IdentifierUse> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<IdentifierUse>(this, IdentifierUse.NULL, code); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<IdentifierUse>(this, IdentifierUse.NULL, code); 204 if ("usual".equals(codeString)) 205 return new Enumeration<IdentifierUse>(this, IdentifierUse.USUAL, code); 206 if ("official".equals(codeString)) 207 return new Enumeration<IdentifierUse>(this, IdentifierUse.OFFICIAL, code); 208 if ("temp".equals(codeString)) 209 return new Enumeration<IdentifierUse>(this, IdentifierUse.TEMP, code); 210 if ("secondary".equals(codeString)) 211 return new Enumeration<IdentifierUse>(this, IdentifierUse.SECONDARY, code); 212 if ("old".equals(codeString)) 213 return new Enumeration<IdentifierUse>(this, IdentifierUse.OLD, code); 214 throw new FHIRException("Unknown IdentifierUse code '" + codeString + "'"); 215 } 216 217 public String toCode(IdentifierUse code) { 218 if (code == IdentifierUse.USUAL) 219 return "usual"; 220 if (code == IdentifierUse.OFFICIAL) 221 return "official"; 222 if (code == IdentifierUse.TEMP) 223 return "temp"; 224 if (code == IdentifierUse.SECONDARY) 225 return "secondary"; 226 if (code == IdentifierUse.OLD) 227 return "old"; 228 return "?"; 229 } 230 231 public String toSystem(IdentifierUse code) { 232 return code.getSystem(); 233 } 234 } 235 236 /** 237 * The purpose of this identifier. 238 */ 239 @Child(name = "use", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = true, summary = true) 240 @Description(shortDefinition = "usual | official | temp | secondary | old (If known)", formalDefinition = "The purpose of this identifier.") 241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/identifier-use") 242 protected Enumeration<IdentifierUse> use; 243 244 /** 245 * A coded type for the identifier that can be used to determine which 246 * identifier to use for a specific purpose. 247 */ 248 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 249 @Description(shortDefinition = "Description of identifier", formalDefinition = "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.") 250 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/identifier-type") 251 protected CodeableConcept type; 252 253 /** 254 * Establishes the namespace for the value - that is, a URL that describes a set 255 * values that are unique. 256 */ 257 @Child(name = "system", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 258 @Description(shortDefinition = "The namespace for the identifier value", formalDefinition = "Establishes the namespace for the value - that is, a URL that describes a set values that are unique.") 259 protected UriType system; 260 261 /** 262 * The portion of the identifier typically relevant to the user and which is 263 * unique within the context of the system. 264 */ 265 @Child(name = "value", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 266 @Description(shortDefinition = "The value that is unique", formalDefinition = "The portion of the identifier typically relevant to the user and which is unique within the context of the system.") 267 protected StringType value; 268 269 /** 270 * Time period during which identifier is/was valid for use. 271 */ 272 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 273 @Description(shortDefinition = "Time period when id is/was valid for use", formalDefinition = "Time period during which identifier is/was valid for use.") 274 protected Period period; 275 276 /** 277 * Organization that issued/manages the identifier. 278 */ 279 @Child(name = "assigner", type = { 280 Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 281 @Description(shortDefinition = "Organization that issued id (may be just text)", formalDefinition = "Organization that issued/manages the identifier.") 282 protected Reference assigner; 283 284 /** 285 * The actual object that is the target of the reference (Organization that 286 * issued/manages the identifier.) 287 */ 288 protected Organization assignerTarget; 289 290 private static final long serialVersionUID = -478840981L; 291 292 /** 293 * Constructor 294 */ 295 public Identifier() { 296 super(); 297 } 298 299 /** 300 * @return {@link #use} (The purpose of this identifier.). This is the 301 * underlying object with id, value and extensions. The accessor 302 * "getUse" gives direct access to the value 303 */ 304 public Enumeration<IdentifierUse> getUseElement() { 305 if (this.use == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create Identifier.use"); 308 else if (Configuration.doAutoCreate()) 309 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); // bb 310 return this.use; 311 } 312 313 public boolean hasUseElement() { 314 return this.use != null && !this.use.isEmpty(); 315 } 316 317 public boolean hasUse() { 318 return this.use != null && !this.use.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #use} (The purpose of this identifier.). This is the 323 * underlying object with id, value and extensions. The accessor 324 * "getUse" gives direct access to the value 325 */ 326 public Identifier setUseElement(Enumeration<IdentifierUse> value) { 327 this.use = value; 328 return this; 329 } 330 331 /** 332 * @return The purpose of this identifier. 333 */ 334 public IdentifierUse getUse() { 335 return this.use == null ? null : this.use.getValue(); 336 } 337 338 /** 339 * @param value The purpose of this identifier. 340 */ 341 public Identifier setUse(IdentifierUse value) { 342 if (value == null) 343 this.use = null; 344 else { 345 if (this.use == null) 346 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); 347 this.use.setValue(value); 348 } 349 return this; 350 } 351 352 /** 353 * @return {@link #type} (A coded type for the identifier that can be used to 354 * determine which identifier to use for a specific purpose.) 355 */ 356 public CodeableConcept getType() { 357 if (this.type == null) 358 if (Configuration.errorOnAutoCreate()) 359 throw new Error("Attempt to auto-create Identifier.type"); 360 else if (Configuration.doAutoCreate()) 361 this.type = new CodeableConcept(); // cc 362 return this.type; 363 } 364 365 public boolean hasType() { 366 return this.type != null && !this.type.isEmpty(); 367 } 368 369 /** 370 * @param value {@link #type} (A coded type for the identifier that can be used 371 * to determine which identifier to use for a specific purpose.) 372 */ 373 public Identifier setType(CodeableConcept value) { 374 this.type = value; 375 return this; 376 } 377 378 /** 379 * @return {@link #system} (Establishes the namespace for the value - that is, a 380 * URL that describes a set values that are unique.). This is the 381 * underlying object with id, value and extensions. The accessor 382 * "getSystem" gives direct access to the value 383 */ 384 public UriType getSystemElement() { 385 if (this.system == null) 386 if (Configuration.errorOnAutoCreate()) 387 throw new Error("Attempt to auto-create Identifier.system"); 388 else if (Configuration.doAutoCreate()) 389 this.system = new UriType(); // bb 390 return this.system; 391 } 392 393 public boolean hasSystemElement() { 394 return this.system != null && !this.system.isEmpty(); 395 } 396 397 public boolean hasSystem() { 398 return this.system != null && !this.system.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #system} (Establishes the namespace for the value - that 403 * is, a URL that describes a set values that are unique.). This is 404 * the underlying object with id, value and extensions. The 405 * accessor "getSystem" gives direct access to the value 406 */ 407 public Identifier setSystemElement(UriType value) { 408 this.system = value; 409 return this; 410 } 411 412 /** 413 * @return Establishes the namespace for the value - that is, a URL that 414 * describes a set values that are unique. 415 */ 416 public String getSystem() { 417 return this.system == null ? null : this.system.getValue(); 418 } 419 420 /** 421 * @param value Establishes the namespace for the value - that is, a URL that 422 * describes a set values that are unique. 423 */ 424 public Identifier setSystem(String value) { 425 if (Utilities.noString(value)) 426 this.system = null; 427 else { 428 if (this.system == null) 429 this.system = new UriType(); 430 this.system.setValue(value); 431 } 432 return this; 433 } 434 435 /** 436 * @return {@link #value} (The portion of the identifier typically relevant to 437 * the user and which is unique within the context of the system.). This 438 * is the underlying object with id, value and extensions. The accessor 439 * "getValue" gives direct access to the value 440 */ 441 public StringType getValueElement() { 442 if (this.value == null) 443 if (Configuration.errorOnAutoCreate()) 444 throw new Error("Attempt to auto-create Identifier.value"); 445 else if (Configuration.doAutoCreate()) 446 this.value = new StringType(); // bb 447 return this.value; 448 } 449 450 public boolean hasValueElement() { 451 return this.value != null && !this.value.isEmpty(); 452 } 453 454 public boolean hasValue() { 455 return this.value != null && !this.value.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #value} (The portion of the identifier typically relevant 460 * to the user and which is unique within the context of the 461 * system.). This is the underlying object with id, value and 462 * extensions. The accessor "getValue" gives direct access to the 463 * value 464 */ 465 public Identifier setValueElement(StringType value) { 466 this.value = value; 467 return this; 468 } 469 470 /** 471 * @return The portion of the identifier typically relevant to the user and 472 * which is unique within the context of the system. 473 */ 474 public String getValue() { 475 return this.value == null ? null : this.value.getValue(); 476 } 477 478 /** 479 * @param value The portion of the identifier typically relevant to the user and 480 * which is unique within the context of the system. 481 */ 482 public Identifier setValue(String value) { 483 if (Utilities.noString(value)) 484 this.value = null; 485 else { 486 if (this.value == null) 487 this.value = new StringType(); 488 this.value.setValue(value); 489 } 490 return this; 491 } 492 493 /** 494 * @return {@link #period} (Time period during which identifier is/was valid for 495 * use.) 496 */ 497 public Period getPeriod() { 498 if (this.period == null) 499 if (Configuration.errorOnAutoCreate()) 500 throw new Error("Attempt to auto-create Identifier.period"); 501 else if (Configuration.doAutoCreate()) 502 this.period = new Period(); // cc 503 return this.period; 504 } 505 506 public boolean hasPeriod() { 507 return this.period != null && !this.period.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #period} (Time period during which identifier is/was 512 * valid for use.) 513 */ 514 public Identifier setPeriod(Period value) { 515 this.period = value; 516 return this; 517 } 518 519 /** 520 * @return {@link #assigner} (Organization that issued/manages the identifier.) 521 */ 522 public Reference getAssigner() { 523 if (this.assigner == null) 524 if (Configuration.errorOnAutoCreate()) 525 throw new Error("Attempt to auto-create Identifier.assigner"); 526 else if (Configuration.doAutoCreate()) 527 this.assigner = new Reference(); // cc 528 return this.assigner; 529 } 530 531 public boolean hasAssigner() { 532 return this.assigner != null && !this.assigner.isEmpty(); 533 } 534 535 /** 536 * @param value {@link #assigner} (Organization that issued/manages the 537 * identifier.) 538 */ 539 public Identifier setAssigner(Reference value) { 540 this.assigner = value; 541 return this; 542 } 543 544 /** 545 * @return {@link #assigner} The actual object that is the target of the 546 * reference. The reference library doesn't populate this, but you can 547 * use it to hold the resource if you resolve it. (Organization that 548 * issued/manages the identifier.) 549 */ 550 public Organization getAssignerTarget() { 551 if (this.assignerTarget == null) 552 if (Configuration.errorOnAutoCreate()) 553 throw new Error("Attempt to auto-create Identifier.assigner"); 554 else if (Configuration.doAutoCreate()) 555 this.assignerTarget = new Organization(); // aa 556 return this.assignerTarget; 557 } 558 559 /** 560 * @param value {@link #assigner} The actual object that is the target of the 561 * reference. The reference library doesn't use these, but you can 562 * use it to hold the resource if you resolve it. (Organization 563 * that issued/manages the identifier.) 564 */ 565 public Identifier setAssignerTarget(Organization value) { 566 this.assignerTarget = value; 567 return this; 568 } 569 570 protected void listChildren(List<Property> children) { 571 super.listChildren(children); 572 children.add(new Property("use", "code", "The purpose of this identifier.", 0, 1, use)); 573 children.add(new Property("type", "CodeableConcept", 574 "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 575 0, 1, type)); 576 children.add(new Property("system", "uri", 577 "Establishes the namespace for the value - that is, a URL that describes a set values that are unique.", 0, 1, 578 system)); 579 children.add(new Property("value", "string", 580 "The portion of the identifier typically relevant to the user and which is unique within the context of the system.", 581 0, 1, value)); 582 children.add( 583 new Property("period", "Period", "Time period during which identifier is/was valid for use.", 0, 1, period)); 584 children.add(new Property("assigner", "Reference(Organization)", "Organization that issued/manages the identifier.", 585 0, 1, assigner)); 586 } 587 588 @Override 589 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 590 switch (_hash) { 591 case 116103: 592 /* use */ return new Property("use", "code", "The purpose of this identifier.", 0, 1, use); 593 case 3575610: 594 /* type */ return new Property("type", "CodeableConcept", 595 "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 596 0, 1, type); 597 case -887328209: 598 /* system */ return new Property("system", "uri", 599 "Establishes the namespace for the value - that is, a URL that describes a set values that are unique.", 0, 1, 600 system); 601 case 111972721: 602 /* value */ return new Property("value", "string", 603 "The portion of the identifier typically relevant to the user and which is unique within the context of the system.", 604 0, 1, value); 605 case -991726143: 606 /* period */ return new Property("period", "Period", "Time period during which identifier is/was valid for use.", 607 0, 1, period); 608 case -369881636: 609 /* assigner */ return new Property("assigner", "Reference(Organization)", 610 "Organization that issued/manages the identifier.", 0, 1, assigner); 611 default: 612 return super.getNamedProperty(_hash, _name, _checkValid); 613 } 614 615 } 616 617 @Override 618 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 619 switch (hash) { 620 case 116103: 621 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<IdentifierUse> 622 case 3575610: 623 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 624 case -887328209: 625 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // UriType 626 case 111972721: 627 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 628 case -991726143: 629 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 630 case -369881636: 631 /* assigner */ return this.assigner == null ? new Base[0] : new Base[] { this.assigner }; // Reference 632 default: 633 return super.getProperty(hash, name, checkValid); 634 } 635 636 } 637 638 @Override 639 public Base setProperty(int hash, String name, Base value) throws FHIRException { 640 switch (hash) { 641 case 116103: // use 642 value = new IdentifierUseEnumFactory().fromType(castToCode(value)); 643 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 644 return value; 645 case 3575610: // type 646 this.type = castToCodeableConcept(value); // CodeableConcept 647 return value; 648 case -887328209: // system 649 this.system = castToUri(value); // UriType 650 return value; 651 case 111972721: // value 652 this.value = castToString(value); // StringType 653 return value; 654 case -991726143: // period 655 this.period = castToPeriod(value); // Period 656 return value; 657 case -369881636: // assigner 658 this.assigner = castToReference(value); // Reference 659 return value; 660 default: 661 return super.setProperty(hash, name, value); 662 } 663 664 } 665 666 @Override 667 public Base setProperty(String name, Base value) throws FHIRException { 668 if (name.equals("use")) { 669 value = new IdentifierUseEnumFactory().fromType(castToCode(value)); 670 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 671 } else if (name.equals("type")) { 672 this.type = castToCodeableConcept(value); // CodeableConcept 673 } else if (name.equals("system")) { 674 this.system = castToUri(value); // UriType 675 } else if (name.equals("value")) { 676 this.value = castToString(value); // StringType 677 } else if (name.equals("period")) { 678 this.period = castToPeriod(value); // Period 679 } else if (name.equals("assigner")) { 680 this.assigner = castToReference(value); // Reference 681 } else 682 return super.setProperty(name, value); 683 return value; 684 } 685 686 @Override 687 public Base makeProperty(int hash, String name) throws FHIRException { 688 switch (hash) { 689 case 116103: 690 return getUseElement(); 691 case 3575610: 692 return getType(); 693 case -887328209: 694 return getSystemElement(); 695 case 111972721: 696 return getValueElement(); 697 case -991726143: 698 return getPeriod(); 699 case -369881636: 700 return getAssigner(); 701 default: 702 return super.makeProperty(hash, name); 703 } 704 705 } 706 707 @Override 708 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 709 switch (hash) { 710 case 116103: 711 /* use */ return new String[] { "code" }; 712 case 3575610: 713 /* type */ return new String[] { "CodeableConcept" }; 714 case -887328209: 715 /* system */ return new String[] { "uri" }; 716 case 111972721: 717 /* value */ return new String[] { "string" }; 718 case -991726143: 719 /* period */ return new String[] { "Period" }; 720 case -369881636: 721 /* assigner */ return new String[] { "Reference" }; 722 default: 723 return super.getTypesForProperty(hash, name); 724 } 725 726 } 727 728 @Override 729 public Base addChild(String name) throws FHIRException { 730 if (name.equals("use")) { 731 throw new FHIRException("Cannot call addChild on a singleton property Identifier.use"); 732 } else if (name.equals("type")) { 733 this.type = new CodeableConcept(); 734 return this.type; 735 } else if (name.equals("system")) { 736 throw new FHIRException("Cannot call addChild on a singleton property Identifier.system"); 737 } else if (name.equals("value")) { 738 throw new FHIRException("Cannot call addChild on a singleton property Identifier.value"); 739 } else if (name.equals("period")) { 740 this.period = new Period(); 741 return this.period; 742 } else if (name.equals("assigner")) { 743 this.assigner = new Reference(); 744 return this.assigner; 745 } else 746 return super.addChild(name); 747 } 748 749 public String fhirType() { 750 return "Identifier"; 751 752 } 753 754 public Identifier copy() { 755 Identifier dst = new Identifier(); 756 copyValues(dst); 757 return dst; 758 } 759 760 public void copyValues(Identifier dst) { 761 super.copyValues(dst); 762 dst.use = use == null ? null : use.copy(); 763 dst.type = type == null ? null : type.copy(); 764 dst.system = system == null ? null : system.copy(); 765 dst.value = value == null ? null : value.copy(); 766 dst.period = period == null ? null : period.copy(); 767 dst.assigner = assigner == null ? null : assigner.copy(); 768 } 769 770 protected Identifier typedCopy() { 771 return copy(); 772 } 773 774 @Override 775 public boolean equalsDeep(Base other_) { 776 if (!super.equalsDeep(other_)) 777 return false; 778 if (!(other_ instanceof Identifier)) 779 return false; 780 Identifier o = (Identifier) other_; 781 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(system, o.system, true) 782 && compareDeep(value, o.value, true) && compareDeep(period, o.period, true) 783 && compareDeep(assigner, o.assigner, true); 784 } 785 786 @Override 787 public boolean equalsShallow(Base other_) { 788 if (!super.equalsShallow(other_)) 789 return false; 790 if (!(other_ instanceof Identifier)) 791 return false; 792 Identifier o = (Identifier) other_; 793 return compareValues(use, o.use, true) && compareValues(system, o.system, true) 794 && compareValues(value, o.value, true); 795 } 796 797 public boolean isEmpty() { 798 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, type, system, value, period, assigner); 799 } 800 801}