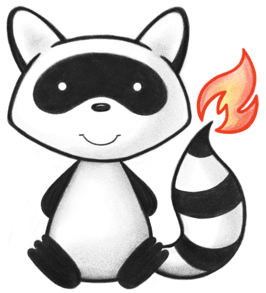
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Representation of the content produced in a DICOM imaging study. A study 049 * comprises a set of series, each of which includes a set of Service-Object 050 * Pair Instances (SOP Instances - images or other data) acquired or produced in 051 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 052 * ultrasound), but a study may have multiple series of different modalities. 053 */ 054@ResourceDef(name = "ImagingStudy", profile = "http://hl7.org/fhir/StructureDefinition/ImagingStudy") 055public class ImagingStudy extends DomainResource { 056 057 public enum ImagingStudyStatus { 058 /** 059 * The existence of the imaging study is registered, but there is nothing yet 060 * available. 061 */ 062 REGISTERED, 063 /** 064 * At least one instance has been associated with this imaging study. 065 */ 066 AVAILABLE, 067 /** 068 * The imaging study is unavailable because the imaging study was not started or 069 * not completed (also sometimes called "aborted"). 070 */ 071 CANCELLED, 072 /** 073 * The imaging study has been withdrawn following a previous final release. This 074 * electronic record should never have existed, though it is possible that 075 * real-world decisions were based on it. (If real-world activity has occurred, 076 * the status should be "cancelled" rather than "entered-in-error".). 077 */ 078 ENTEREDINERROR, 079 /** 080 * The system does not know which of the status values currently applies for 081 * this request. Note: This concept is not to be used for "other" - one of the 082 * listed statuses is presumed to apply, it's just not known which one. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 090 public static ImagingStudyStatus fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("registered".equals(codeString)) 094 return REGISTERED; 095 if ("available".equals(codeString)) 096 return AVAILABLE; 097 if ("cancelled".equals(codeString)) 098 return CANCELLED; 099 if ("entered-in-error".equals(codeString)) 100 return ENTEREDINERROR; 101 if ("unknown".equals(codeString)) 102 return UNKNOWN; 103 if (Configuration.isAcceptInvalidEnums()) 104 return null; 105 else 106 throw new FHIRException("Unknown ImagingStudyStatus code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case REGISTERED: 112 return "registered"; 113 case AVAILABLE: 114 return "available"; 115 case CANCELLED: 116 return "cancelled"; 117 case ENTEREDINERROR: 118 return "entered-in-error"; 119 case UNKNOWN: 120 return "unknown"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getSystem() { 129 switch (this) { 130 case REGISTERED: 131 return "http://hl7.org/fhir/imagingstudy-status"; 132 case AVAILABLE: 133 return "http://hl7.org/fhir/imagingstudy-status"; 134 case CANCELLED: 135 return "http://hl7.org/fhir/imagingstudy-status"; 136 case ENTEREDINERROR: 137 return "http://hl7.org/fhir/imagingstudy-status"; 138 case UNKNOWN: 139 return "http://hl7.org/fhir/imagingstudy-status"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case REGISTERED: 150 return "The existence of the imaging study is registered, but there is nothing yet available."; 151 case AVAILABLE: 152 return "At least one instance has been associated with this imaging study."; 153 case CANCELLED: 154 return "The imaging study is unavailable because the imaging study was not started or not completed (also sometimes called \"aborted\")."; 155 case ENTEREDINERROR: 156 return "The imaging study has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 157 case UNKNOWN: 158 return "The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDisplay() { 167 switch (this) { 168 case REGISTERED: 169 return "Registered"; 170 case AVAILABLE: 171 return "Available"; 172 case CANCELLED: 173 return "Cancelled"; 174 case ENTEREDINERROR: 175 return "Entered in Error"; 176 case UNKNOWN: 177 return "Unknown"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 } 185 186 public static class ImagingStudyStatusEnumFactory implements EnumFactory<ImagingStudyStatus> { 187 public ImagingStudyStatus fromCode(String codeString) throws IllegalArgumentException { 188 if (codeString == null || "".equals(codeString)) 189 if (codeString == null || "".equals(codeString)) 190 return null; 191 if ("registered".equals(codeString)) 192 return ImagingStudyStatus.REGISTERED; 193 if ("available".equals(codeString)) 194 return ImagingStudyStatus.AVAILABLE; 195 if ("cancelled".equals(codeString)) 196 return ImagingStudyStatus.CANCELLED; 197 if ("entered-in-error".equals(codeString)) 198 return ImagingStudyStatus.ENTEREDINERROR; 199 if ("unknown".equals(codeString)) 200 return ImagingStudyStatus.UNKNOWN; 201 throw new IllegalArgumentException("Unknown ImagingStudyStatus code '" + codeString + "'"); 202 } 203 204 public Enumeration<ImagingStudyStatus> fromType(PrimitiveType<?> code) throws FHIRException { 205 if (code == null) 206 return null; 207 if (code.isEmpty()) 208 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.NULL, code); 209 String codeString = code.asStringValue(); 210 if (codeString == null || "".equals(codeString)) 211 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.NULL, code); 212 if ("registered".equals(codeString)) 213 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.REGISTERED, code); 214 if ("available".equals(codeString)) 215 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.AVAILABLE, code); 216 if ("cancelled".equals(codeString)) 217 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.CANCELLED, code); 218 if ("entered-in-error".equals(codeString)) 219 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.ENTEREDINERROR, code); 220 if ("unknown".equals(codeString)) 221 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.UNKNOWN, code); 222 throw new FHIRException("Unknown ImagingStudyStatus code '" + codeString + "'"); 223 } 224 225 public String toCode(ImagingStudyStatus code) { 226 if (code == ImagingStudyStatus.NULL) 227 return null; 228 if (code == ImagingStudyStatus.REGISTERED) 229 return "registered"; 230 if (code == ImagingStudyStatus.AVAILABLE) 231 return "available"; 232 if (code == ImagingStudyStatus.CANCELLED) 233 return "cancelled"; 234 if (code == ImagingStudyStatus.ENTEREDINERROR) 235 return "entered-in-error"; 236 if (code == ImagingStudyStatus.UNKNOWN) 237 return "unknown"; 238 return "?"; 239 } 240 241 public String toSystem(ImagingStudyStatus code) { 242 return code.getSystem(); 243 } 244 } 245 246 @Block() 247 public static class ImagingStudySeriesComponent extends BackboneElement implements IBaseBackboneElement { 248 /** 249 * The DICOM Series Instance UID for the series. 250 */ 251 @Child(name = "uid", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 252 @Description(shortDefinition = "DICOM Series Instance UID for the series", formalDefinition = "The DICOM Series Instance UID for the series.") 253 protected IdType uid; 254 255 /** 256 * The numeric identifier of this series in the study. 257 */ 258 @Child(name = "number", type = { 259 UnsignedIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 260 @Description(shortDefinition = "Numeric identifier of this series", formalDefinition = "The numeric identifier of this series in the study.") 261 protected UnsignedIntType number; 262 263 /** 264 * The modality of this series sequence. 265 */ 266 @Child(name = "modality", type = { Coding.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 267 @Description(shortDefinition = "The modality of the instances in the series", formalDefinition = "The modality of this series sequence.") 268 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_29.html") 269 protected Coding modality; 270 271 /** 272 * A description of the series. 273 */ 274 @Child(name = "description", type = { 275 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 276 @Description(shortDefinition = "A short human readable summary of the series", formalDefinition = "A description of the series.") 277 protected StringType description; 278 279 /** 280 * Number of SOP Instances in the Study. The value given may be larger than the 281 * number of instance elements this resource contains due to resource 282 * availability, security, or other factors. This element should be present if 283 * any instance elements are present. 284 */ 285 @Child(name = "numberOfInstances", type = { 286 UnsignedIntType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 287 @Description(shortDefinition = "Number of Series Related Instances", formalDefinition = "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.") 288 protected UnsignedIntType numberOfInstances; 289 290 /** 291 * The network service providing access (e.g., query, view, or retrieval) for 292 * this series. See implementation notes for information about using DICOM 293 * endpoints. A series-level endpoint, if present, has precedence over a 294 * study-level endpoint with the same Endpoint.connectionType. 295 */ 296 @Child(name = "endpoint", type = { 297 Endpoint.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 298 @Description(shortDefinition = "Series access endpoint", formalDefinition = "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType.") 299 protected List<Reference> endpoint; 300 /** 301 * The actual objects that are the target of the reference (The network service 302 * providing access (e.g., query, view, or retrieval) for this series. See 303 * implementation notes for information about using DICOM endpoints. A 304 * series-level endpoint, if present, has precedence over a study-level endpoint 305 * with the same Endpoint.connectionType.) 306 */ 307 protected List<Endpoint> endpointTarget; 308 309 /** 310 * The anatomic structures examined. See DICOM Part 16 Annex L 311 * (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) 312 * for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of 313 * body part imaged; if so, it shall be consistent with any content of 314 * ImagingStudy.series.laterality. 315 */ 316 @Child(name = "bodySite", type = { Coding.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 317 @Description(shortDefinition = "Body part examined", formalDefinition = "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.") 318 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 319 protected Coding bodySite; 320 321 /** 322 * The laterality of the (possibly paired) anatomic structures examined. E.g., 323 * the left knee, both lungs, or unpaired abdomen. If present, shall be 324 * consistent with any laterality information indicated in 325 * ImagingStudy.series.bodySite. 326 */ 327 @Child(name = "laterality", type = { Coding.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 328 @Description(shortDefinition = "Body part laterality", formalDefinition = "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.") 329 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/bodysite-laterality") 330 protected Coding laterality; 331 332 /** 333 * The specimen imaged, e.g., for whole slide imaging of a biopsy. 334 */ 335 @Child(name = "specimen", type = { 336 Specimen.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 337 @Description(shortDefinition = "Specimen imaged", formalDefinition = "The specimen imaged, e.g., for whole slide imaging of a biopsy.") 338 protected List<Reference> specimen; 339 /** 340 * The actual objects that are the target of the reference (The specimen imaged, 341 * e.g., for whole slide imaging of a biopsy.) 342 */ 343 protected List<Specimen> specimenTarget; 344 345 /** 346 * The date and time the series was started. 347 */ 348 @Child(name = "started", type = { 349 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 350 @Description(shortDefinition = "When the series started", formalDefinition = "The date and time the series was started.") 351 protected DateTimeType started; 352 353 /** 354 * Indicates who or what performed the series and how they were involved. 355 */ 356 @Child(name = "performer", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 357 @Description(shortDefinition = "Who performed the series", formalDefinition = "Indicates who or what performed the series and how they were involved.") 358 protected List<ImagingStudySeriesPerformerComponent> performer; 359 360 /** 361 * A single SOP instance within the series, e.g. an image, or presentation 362 * state. 363 */ 364 @Child(name = "instance", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 365 @Description(shortDefinition = "A single SOP instance from the series", formalDefinition = "A single SOP instance within the series, e.g. an image, or presentation state.") 366 protected List<ImagingStudySeriesInstanceComponent> instance; 367 368 private static final long serialVersionUID = -11423429L; 369 370 /** 371 * Constructor 372 */ 373 public ImagingStudySeriesComponent() { 374 super(); 375 } 376 377 /** 378 * Constructor 379 */ 380 public ImagingStudySeriesComponent(IdType uid, Coding modality) { 381 super(); 382 this.uid = uid; 383 this.modality = modality; 384 } 385 386 /** 387 * @return {@link #uid} (The DICOM Series Instance UID for the series.). This is 388 * the underlying object with id, value and extensions. The accessor 389 * "getUid" gives direct access to the value 390 */ 391 public IdType getUidElement() { 392 if (this.uid == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.uid"); 395 else if (Configuration.doAutoCreate()) 396 this.uid = new IdType(); // bb 397 return this.uid; 398 } 399 400 public boolean hasUidElement() { 401 return this.uid != null && !this.uid.isEmpty(); 402 } 403 404 public boolean hasUid() { 405 return this.uid != null && !this.uid.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #uid} (The DICOM Series Instance UID for the series.). 410 * This is the underlying object with id, value and extensions. The 411 * accessor "getUid" gives direct access to the value 412 */ 413 public ImagingStudySeriesComponent setUidElement(IdType value) { 414 this.uid = value; 415 return this; 416 } 417 418 /** 419 * @return The DICOM Series Instance UID for the series. 420 */ 421 public String getUid() { 422 return this.uid == null ? null : this.uid.getValue(); 423 } 424 425 /** 426 * @param value The DICOM Series Instance UID for the series. 427 */ 428 public ImagingStudySeriesComponent setUid(String value) { 429 if (this.uid == null) 430 this.uid = new IdType(); 431 this.uid.setValue(value); 432 return this; 433 } 434 435 /** 436 * @return {@link #number} (The numeric identifier of this series in the 437 * study.). This is the underlying object with id, value and extensions. 438 * The accessor "getNumber" gives direct access to the value 439 */ 440 public UnsignedIntType getNumberElement() { 441 if (this.number == null) 442 if (Configuration.errorOnAutoCreate()) 443 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.number"); 444 else if (Configuration.doAutoCreate()) 445 this.number = new UnsignedIntType(); // bb 446 return this.number; 447 } 448 449 public boolean hasNumberElement() { 450 return this.number != null && !this.number.isEmpty(); 451 } 452 453 public boolean hasNumber() { 454 return this.number != null && !this.number.isEmpty(); 455 } 456 457 /** 458 * @param value {@link #number} (The numeric identifier of this series in the 459 * study.). This is the underlying object with id, value and 460 * extensions. The accessor "getNumber" gives direct access to the 461 * value 462 */ 463 public ImagingStudySeriesComponent setNumberElement(UnsignedIntType value) { 464 this.number = value; 465 return this; 466 } 467 468 /** 469 * @return The numeric identifier of this series in the study. 470 */ 471 public int getNumber() { 472 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 473 } 474 475 /** 476 * @param value The numeric identifier of this series in the study. 477 */ 478 public ImagingStudySeriesComponent setNumber(int value) { 479 if (this.number == null) 480 this.number = new UnsignedIntType(); 481 this.number.setValue(value); 482 return this; 483 } 484 485 /** 486 * @return {@link #modality} (The modality of this series sequence.) 487 */ 488 public Coding getModality() { 489 if (this.modality == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.modality"); 492 else if (Configuration.doAutoCreate()) 493 this.modality = new Coding(); // cc 494 return this.modality; 495 } 496 497 public boolean hasModality() { 498 return this.modality != null && !this.modality.isEmpty(); 499 } 500 501 /** 502 * @param value {@link #modality} (The modality of this series sequence.) 503 */ 504 public ImagingStudySeriesComponent setModality(Coding value) { 505 this.modality = value; 506 return this; 507 } 508 509 /** 510 * @return {@link #description} (A description of the series.). This is the 511 * underlying object with id, value and extensions. The accessor 512 * "getDescription" gives direct access to the value 513 */ 514 public StringType getDescriptionElement() { 515 if (this.description == null) 516 if (Configuration.errorOnAutoCreate()) 517 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.description"); 518 else if (Configuration.doAutoCreate()) 519 this.description = new StringType(); // bb 520 return this.description; 521 } 522 523 public boolean hasDescriptionElement() { 524 return this.description != null && !this.description.isEmpty(); 525 } 526 527 public boolean hasDescription() { 528 return this.description != null && !this.description.isEmpty(); 529 } 530 531 /** 532 * @param value {@link #description} (A description of the series.). This is the 533 * underlying object with id, value and extensions. The accessor 534 * "getDescription" gives direct access to the value 535 */ 536 public ImagingStudySeriesComponent setDescriptionElement(StringType value) { 537 this.description = value; 538 return this; 539 } 540 541 /** 542 * @return A description of the series. 543 */ 544 public String getDescription() { 545 return this.description == null ? null : this.description.getValue(); 546 } 547 548 /** 549 * @param value A description of the series. 550 */ 551 public ImagingStudySeriesComponent setDescription(String value) { 552 if (Utilities.noString(value)) 553 this.description = null; 554 else { 555 if (this.description == null) 556 this.description = new StringType(); 557 this.description.setValue(value); 558 } 559 return this; 560 } 561 562 /** 563 * @return {@link #numberOfInstances} (Number of SOP Instances in the Study. The 564 * value given may be larger than the number of instance elements this 565 * resource contains due to resource availability, security, or other 566 * factors. This element should be present if any instance elements are 567 * present.). This is the underlying object with id, value and 568 * extensions. The accessor "getNumberOfInstances" gives direct access 569 * to the value 570 */ 571 public UnsignedIntType getNumberOfInstancesElement() { 572 if (this.numberOfInstances == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.numberOfInstances"); 575 else if (Configuration.doAutoCreate()) 576 this.numberOfInstances = new UnsignedIntType(); // bb 577 return this.numberOfInstances; 578 } 579 580 public boolean hasNumberOfInstancesElement() { 581 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 582 } 583 584 public boolean hasNumberOfInstances() { 585 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 586 } 587 588 /** 589 * @param value {@link #numberOfInstances} (Number of SOP Instances in the 590 * Study. The value given may be larger than the number of instance 591 * elements this resource contains due to resource availability, 592 * security, or other factors. This element should be present if 593 * any instance elements are present.). This is the underlying 594 * object with id, value and extensions. The accessor 595 * "getNumberOfInstances" gives direct access to the value 596 */ 597 public ImagingStudySeriesComponent setNumberOfInstancesElement(UnsignedIntType value) { 598 this.numberOfInstances = value; 599 return this; 600 } 601 602 /** 603 * @return Number of SOP Instances in the Study. The value given may be larger 604 * than the number of instance elements this resource contains due to 605 * resource availability, security, or other factors. This element 606 * should be present if any instance elements are present. 607 */ 608 public int getNumberOfInstances() { 609 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 610 } 611 612 /** 613 * @param value Number of SOP Instances in the Study. The value given may be 614 * larger than the number of instance elements this resource 615 * contains due to resource availability, security, or other 616 * factors. This element should be present if any instance elements 617 * are present. 618 */ 619 public ImagingStudySeriesComponent setNumberOfInstances(int value) { 620 if (this.numberOfInstances == null) 621 this.numberOfInstances = new UnsignedIntType(); 622 this.numberOfInstances.setValue(value); 623 return this; 624 } 625 626 /** 627 * @return {@link #endpoint} (The network service providing access (e.g., query, 628 * view, or retrieval) for this series. See implementation notes for 629 * information about using DICOM endpoints. A series-level endpoint, if 630 * present, has precedence over a study-level endpoint with the same 631 * Endpoint.connectionType.) 632 */ 633 public List<Reference> getEndpoint() { 634 if (this.endpoint == null) 635 this.endpoint = new ArrayList<Reference>(); 636 return this.endpoint; 637 } 638 639 /** 640 * @return Returns a reference to <code>this</code> for easy method chaining 641 */ 642 public ImagingStudySeriesComponent setEndpoint(List<Reference> theEndpoint) { 643 this.endpoint = theEndpoint; 644 return this; 645 } 646 647 public boolean hasEndpoint() { 648 if (this.endpoint == null) 649 return false; 650 for (Reference item : this.endpoint) 651 if (!item.isEmpty()) 652 return true; 653 return false; 654 } 655 656 public Reference addEndpoint() { // 3 657 Reference t = new Reference(); 658 if (this.endpoint == null) 659 this.endpoint = new ArrayList<Reference>(); 660 this.endpoint.add(t); 661 return t; 662 } 663 664 public ImagingStudySeriesComponent addEndpoint(Reference t) { // 3 665 if (t == null) 666 return this; 667 if (this.endpoint == null) 668 this.endpoint = new ArrayList<Reference>(); 669 this.endpoint.add(t); 670 return this; 671 } 672 673 /** 674 * @return The first repetition of repeating field {@link #endpoint}, creating 675 * it if it does not already exist 676 */ 677 public Reference getEndpointFirstRep() { 678 if (getEndpoint().isEmpty()) { 679 addEndpoint(); 680 } 681 return getEndpoint().get(0); 682 } 683 684 /** 685 * @deprecated Use Reference#setResource(IBaseResource) instead 686 */ 687 @Deprecated 688 public List<Endpoint> getEndpointTarget() { 689 if (this.endpointTarget == null) 690 this.endpointTarget = new ArrayList<Endpoint>(); 691 return this.endpointTarget; 692 } 693 694 /** 695 * @deprecated Use Reference#setResource(IBaseResource) instead 696 */ 697 @Deprecated 698 public Endpoint addEndpointTarget() { 699 Endpoint r = new Endpoint(); 700 if (this.endpointTarget == null) 701 this.endpointTarget = new ArrayList<Endpoint>(); 702 this.endpointTarget.add(r); 703 return r; 704 } 705 706 /** 707 * @return {@link #bodySite} (The anatomic structures examined. See DICOM Part 708 * 16 Annex L 709 * (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) 710 * for DICOM to SNOMED-CT mappings. The bodySite may indicate the 711 * laterality of body part imaged; if so, it shall be consistent with 712 * any content of ImagingStudy.series.laterality.) 713 */ 714 public Coding getBodySite() { 715 if (this.bodySite == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.bodySite"); 718 else if (Configuration.doAutoCreate()) 719 this.bodySite = new Coding(); // cc 720 return this.bodySite; 721 } 722 723 public boolean hasBodySite() { 724 return this.bodySite != null && !this.bodySite.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #bodySite} (The anatomic structures examined. See DICOM 729 * Part 16 Annex L 730 * (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) 731 * for DICOM to SNOMED-CT mappings. The bodySite may indicate the 732 * laterality of body part imaged; if so, it shall be consistent 733 * with any content of ImagingStudy.series.laterality.) 734 */ 735 public ImagingStudySeriesComponent setBodySite(Coding value) { 736 this.bodySite = value; 737 return this; 738 } 739 740 /** 741 * @return {@link #laterality} (The laterality of the (possibly paired) anatomic 742 * structures examined. E.g., the left knee, both lungs, or unpaired 743 * abdomen. If present, shall be consistent with any laterality 744 * information indicated in ImagingStudy.series.bodySite.) 745 */ 746 public Coding getLaterality() { 747 if (this.laterality == null) 748 if (Configuration.errorOnAutoCreate()) 749 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.laterality"); 750 else if (Configuration.doAutoCreate()) 751 this.laterality = new Coding(); // cc 752 return this.laterality; 753 } 754 755 public boolean hasLaterality() { 756 return this.laterality != null && !this.laterality.isEmpty(); 757 } 758 759 /** 760 * @param value {@link #laterality} (The laterality of the (possibly paired) 761 * anatomic structures examined. E.g., the left knee, both lungs, 762 * or unpaired abdomen. If present, shall be consistent with any 763 * laterality information indicated in 764 * ImagingStudy.series.bodySite.) 765 */ 766 public ImagingStudySeriesComponent setLaterality(Coding value) { 767 this.laterality = value; 768 return this; 769 } 770 771 /** 772 * @return {@link #specimen} (The specimen imaged, e.g., for whole slide imaging 773 * of a biopsy.) 774 */ 775 public List<Reference> getSpecimen() { 776 if (this.specimen == null) 777 this.specimen = new ArrayList<Reference>(); 778 return this.specimen; 779 } 780 781 /** 782 * @return Returns a reference to <code>this</code> for easy method chaining 783 */ 784 public ImagingStudySeriesComponent setSpecimen(List<Reference> theSpecimen) { 785 this.specimen = theSpecimen; 786 return this; 787 } 788 789 public boolean hasSpecimen() { 790 if (this.specimen == null) 791 return false; 792 for (Reference item : this.specimen) 793 if (!item.isEmpty()) 794 return true; 795 return false; 796 } 797 798 public Reference addSpecimen() { // 3 799 Reference t = new Reference(); 800 if (this.specimen == null) 801 this.specimen = new ArrayList<Reference>(); 802 this.specimen.add(t); 803 return t; 804 } 805 806 public ImagingStudySeriesComponent addSpecimen(Reference t) { // 3 807 if (t == null) 808 return this; 809 if (this.specimen == null) 810 this.specimen = new ArrayList<Reference>(); 811 this.specimen.add(t); 812 return this; 813 } 814 815 /** 816 * @return The first repetition of repeating field {@link #specimen}, creating 817 * it if it does not already exist 818 */ 819 public Reference getSpecimenFirstRep() { 820 if (getSpecimen().isEmpty()) { 821 addSpecimen(); 822 } 823 return getSpecimen().get(0); 824 } 825 826 /** 827 * @deprecated Use Reference#setResource(IBaseResource) instead 828 */ 829 @Deprecated 830 public List<Specimen> getSpecimenTarget() { 831 if (this.specimenTarget == null) 832 this.specimenTarget = new ArrayList<Specimen>(); 833 return this.specimenTarget; 834 } 835 836 /** 837 * @deprecated Use Reference#setResource(IBaseResource) instead 838 */ 839 @Deprecated 840 public Specimen addSpecimenTarget() { 841 Specimen r = new Specimen(); 842 if (this.specimenTarget == null) 843 this.specimenTarget = new ArrayList<Specimen>(); 844 this.specimenTarget.add(r); 845 return r; 846 } 847 848 /** 849 * @return {@link #started} (The date and time the series was started.). This is 850 * the underlying object with id, value and extensions. The accessor 851 * "getStarted" gives direct access to the value 852 */ 853 public DateTimeType getStartedElement() { 854 if (this.started == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.started"); 857 else if (Configuration.doAutoCreate()) 858 this.started = new DateTimeType(); // bb 859 return this.started; 860 } 861 862 public boolean hasStartedElement() { 863 return this.started != null && !this.started.isEmpty(); 864 } 865 866 public boolean hasStarted() { 867 return this.started != null && !this.started.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #started} (The date and time the series was started.). 872 * This is the underlying object with id, value and extensions. The 873 * accessor "getStarted" gives direct access to the value 874 */ 875 public ImagingStudySeriesComponent setStartedElement(DateTimeType value) { 876 this.started = value; 877 return this; 878 } 879 880 /** 881 * @return The date and time the series was started. 882 */ 883 public Date getStarted() { 884 return this.started == null ? null : this.started.getValue(); 885 } 886 887 /** 888 * @param value The date and time the series was started. 889 */ 890 public ImagingStudySeriesComponent setStarted(Date value) { 891 if (value == null) 892 this.started = null; 893 else { 894 if (this.started == null) 895 this.started = new DateTimeType(); 896 this.started.setValue(value); 897 } 898 return this; 899 } 900 901 /** 902 * @return {@link #performer} (Indicates who or what performed the series and 903 * how they were involved.) 904 */ 905 public List<ImagingStudySeriesPerformerComponent> getPerformer() { 906 if (this.performer == null) 907 this.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 908 return this.performer; 909 } 910 911 /** 912 * @return Returns a reference to <code>this</code> for easy method chaining 913 */ 914 public ImagingStudySeriesComponent setPerformer(List<ImagingStudySeriesPerformerComponent> thePerformer) { 915 this.performer = thePerformer; 916 return this; 917 } 918 919 public boolean hasPerformer() { 920 if (this.performer == null) 921 return false; 922 for (ImagingStudySeriesPerformerComponent item : this.performer) 923 if (!item.isEmpty()) 924 return true; 925 return false; 926 } 927 928 public ImagingStudySeriesPerformerComponent addPerformer() { // 3 929 ImagingStudySeriesPerformerComponent t = new ImagingStudySeriesPerformerComponent(); 930 if (this.performer == null) 931 this.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 932 this.performer.add(t); 933 return t; 934 } 935 936 public ImagingStudySeriesComponent addPerformer(ImagingStudySeriesPerformerComponent t) { // 3 937 if (t == null) 938 return this; 939 if (this.performer == null) 940 this.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 941 this.performer.add(t); 942 return this; 943 } 944 945 /** 946 * @return The first repetition of repeating field {@link #performer}, creating 947 * it if it does not already exist 948 */ 949 public ImagingStudySeriesPerformerComponent getPerformerFirstRep() { 950 if (getPerformer().isEmpty()) { 951 addPerformer(); 952 } 953 return getPerformer().get(0); 954 } 955 956 /** 957 * @return {@link #instance} (A single SOP instance within the series, e.g. an 958 * image, or presentation state.) 959 */ 960 public List<ImagingStudySeriesInstanceComponent> getInstance() { 961 if (this.instance == null) 962 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 963 return this.instance; 964 } 965 966 /** 967 * @return Returns a reference to <code>this</code> for easy method chaining 968 */ 969 public ImagingStudySeriesComponent setInstance(List<ImagingStudySeriesInstanceComponent> theInstance) { 970 this.instance = theInstance; 971 return this; 972 } 973 974 public boolean hasInstance() { 975 if (this.instance == null) 976 return false; 977 for (ImagingStudySeriesInstanceComponent item : this.instance) 978 if (!item.isEmpty()) 979 return true; 980 return false; 981 } 982 983 public ImagingStudySeriesInstanceComponent addInstance() { // 3 984 ImagingStudySeriesInstanceComponent t = new ImagingStudySeriesInstanceComponent(); 985 if (this.instance == null) 986 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 987 this.instance.add(t); 988 return t; 989 } 990 991 public ImagingStudySeriesComponent addInstance(ImagingStudySeriesInstanceComponent t) { // 3 992 if (t == null) 993 return this; 994 if (this.instance == null) 995 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 996 this.instance.add(t); 997 return this; 998 } 999 1000 /** 1001 * @return The first repetition of repeating field {@link #instance}, creating 1002 * it if it does not already exist 1003 */ 1004 public ImagingStudySeriesInstanceComponent getInstanceFirstRep() { 1005 if (getInstance().isEmpty()) { 1006 addInstance(); 1007 } 1008 return getInstance().get(0); 1009 } 1010 1011 protected void listChildren(List<Property> children) { 1012 super.listChildren(children); 1013 children.add(new Property("uid", "id", "The DICOM Series Instance UID for the series.", 0, 1, uid)); 1014 children.add( 1015 new Property("number", "unsignedInt", "The numeric identifier of this series in the study.", 0, 1, number)); 1016 children.add(new Property("modality", "Coding", "The modality of this series sequence.", 0, 1, modality)); 1017 children.add(new Property("description", "string", "A description of the series.", 0, 1, description)); 1018 children.add(new Property("numberOfInstances", "unsignedInt", 1019 "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 1020 0, 1, numberOfInstances)); 1021 children.add(new Property("endpoint", "Reference(Endpoint)", 1022 "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType.", 1023 0, java.lang.Integer.MAX_VALUE, endpoint)); 1024 children.add(new Property("bodySite", "Coding", 1025 "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.", 1026 0, 1, bodySite)); 1027 children.add(new Property("laterality", "Coding", 1028 "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.", 1029 0, 1, laterality)); 1030 children.add(new Property("specimen", "Reference(Specimen)", 1031 "The specimen imaged, e.g., for whole slide imaging of a biopsy.", 0, java.lang.Integer.MAX_VALUE, specimen)); 1032 children.add(new Property("started", "dateTime", "The date and time the series was started.", 0, 1, started)); 1033 children 1034 .add(new Property("performer", "", "Indicates who or what performed the series and how they were involved.", 1035 0, java.lang.Integer.MAX_VALUE, performer)); 1036 children.add( 1037 new Property("instance", "", "A single SOP instance within the series, e.g. an image, or presentation state.", 1038 0, java.lang.Integer.MAX_VALUE, instance)); 1039 } 1040 1041 @Override 1042 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1043 switch (_hash) { 1044 case 115792: 1045 /* uid */ return new Property("uid", "id", "The DICOM Series Instance UID for the series.", 0, 1, uid); 1046 case -1034364087: 1047 /* number */ return new Property("number", "unsignedInt", "The numeric identifier of this series in the study.", 1048 0, 1, number); 1049 case -622722335: 1050 /* modality */ return new Property("modality", "Coding", "The modality of this series sequence.", 0, 1, 1051 modality); 1052 case -1724546052: 1053 /* description */ return new Property("description", "string", "A description of the series.", 0, 1, 1054 description); 1055 case -1043544226: 1056 /* numberOfInstances */ return new Property("numberOfInstances", "unsignedInt", 1057 "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 1058 0, 1, numberOfInstances); 1059 case 1741102485: 1060 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 1061 "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType.", 1062 0, java.lang.Integer.MAX_VALUE, endpoint); 1063 case 1702620169: 1064 /* bodySite */ return new Property("bodySite", "Coding", 1065 "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.", 1066 0, 1, bodySite); 1067 case -170291817: 1068 /* laterality */ return new Property("laterality", "Coding", 1069 "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.", 1070 0, 1, laterality); 1071 case -2132868344: 1072 /* specimen */ return new Property("specimen", "Reference(Specimen)", 1073 "The specimen imaged, e.g., for whole slide imaging of a biopsy.", 0, java.lang.Integer.MAX_VALUE, 1074 specimen); 1075 case -1897185151: 1076 /* started */ return new Property("started", "dateTime", "The date and time the series was started.", 0, 1, 1077 started); 1078 case 481140686: 1079 /* performer */ return new Property("performer", "", 1080 "Indicates who or what performed the series and how they were involved.", 0, java.lang.Integer.MAX_VALUE, 1081 performer); 1082 case 555127957: 1083 /* instance */ return new Property("instance", "", 1084 "A single SOP instance within the series, e.g. an image, or presentation state.", 0, 1085 java.lang.Integer.MAX_VALUE, instance); 1086 default: 1087 return super.getNamedProperty(_hash, _name, _checkValid); 1088 } 1089 1090 } 1091 1092 @Override 1093 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1094 switch (hash) { 1095 case 115792: 1096 /* uid */ return this.uid == null ? new Base[0] : new Base[] { this.uid }; // IdType 1097 case -1034364087: 1098 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // UnsignedIntType 1099 case -622722335: 1100 /* modality */ return this.modality == null ? new Base[0] : new Base[] { this.modality }; // Coding 1101 case -1724546052: 1102 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1103 case -1043544226: 1104 /* numberOfInstances */ return this.numberOfInstances == null ? new Base[0] 1105 : new Base[] { this.numberOfInstances }; // UnsignedIntType 1106 case 1741102485: 1107 /* endpoint */ return this.endpoint == null ? new Base[0] 1108 : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1109 case 1702620169: 1110 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // Coding 1111 case -170291817: 1112 /* laterality */ return this.laterality == null ? new Base[0] : new Base[] { this.laterality }; // Coding 1113 case -2132868344: 1114 /* specimen */ return this.specimen == null ? new Base[0] 1115 : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 1116 case -1897185151: 1117 /* started */ return this.started == null ? new Base[0] : new Base[] { this.started }; // DateTimeType 1118 case 481140686: 1119 /* performer */ return this.performer == null ? new Base[0] 1120 : this.performer.toArray(new Base[this.performer.size()]); // ImagingStudySeriesPerformerComponent 1121 case 555127957: 1122 /* instance */ return this.instance == null ? new Base[0] 1123 : this.instance.toArray(new Base[this.instance.size()]); // ImagingStudySeriesInstanceComponent 1124 default: 1125 return super.getProperty(hash, name, checkValid); 1126 } 1127 1128 } 1129 1130 @Override 1131 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1132 switch (hash) { 1133 case 115792: // uid 1134 this.uid = castToId(value); // IdType 1135 return value; 1136 case -1034364087: // number 1137 this.number = castToUnsignedInt(value); // UnsignedIntType 1138 return value; 1139 case -622722335: // modality 1140 this.modality = castToCoding(value); // Coding 1141 return value; 1142 case -1724546052: // description 1143 this.description = castToString(value); // StringType 1144 return value; 1145 case -1043544226: // numberOfInstances 1146 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 1147 return value; 1148 case 1741102485: // endpoint 1149 this.getEndpoint().add(castToReference(value)); // Reference 1150 return value; 1151 case 1702620169: // bodySite 1152 this.bodySite = castToCoding(value); // Coding 1153 return value; 1154 case -170291817: // laterality 1155 this.laterality = castToCoding(value); // Coding 1156 return value; 1157 case -2132868344: // specimen 1158 this.getSpecimen().add(castToReference(value)); // Reference 1159 return value; 1160 case -1897185151: // started 1161 this.started = castToDateTime(value); // DateTimeType 1162 return value; 1163 case 481140686: // performer 1164 this.getPerformer().add((ImagingStudySeriesPerformerComponent) value); // ImagingStudySeriesPerformerComponent 1165 return value; 1166 case 555127957: // instance 1167 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); // ImagingStudySeriesInstanceComponent 1168 return value; 1169 default: 1170 return super.setProperty(hash, name, value); 1171 } 1172 1173 } 1174 1175 @Override 1176 public Base setProperty(String name, Base value) throws FHIRException { 1177 if (name.equals("uid")) { 1178 this.uid = castToId(value); // IdType 1179 } else if (name.equals("number")) { 1180 this.number = castToUnsignedInt(value); // UnsignedIntType 1181 } else if (name.equals("modality")) { 1182 this.modality = castToCoding(value); // Coding 1183 } else if (name.equals("description")) { 1184 this.description = castToString(value); // StringType 1185 } else if (name.equals("numberOfInstances")) { 1186 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 1187 } else if (name.equals("endpoint")) { 1188 this.getEndpoint().add(castToReference(value)); 1189 } else if (name.equals("bodySite")) { 1190 this.bodySite = castToCoding(value); // Coding 1191 } else if (name.equals("laterality")) { 1192 this.laterality = castToCoding(value); // Coding 1193 } else if (name.equals("specimen")) { 1194 this.getSpecimen().add(castToReference(value)); 1195 } else if (name.equals("started")) { 1196 this.started = castToDateTime(value); // DateTimeType 1197 } else if (name.equals("performer")) { 1198 this.getPerformer().add((ImagingStudySeriesPerformerComponent) value); 1199 } else if (name.equals("instance")) { 1200 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); 1201 } else 1202 return super.setProperty(name, value); 1203 return value; 1204 } 1205 1206 @Override 1207 public void removeChild(String name, Base value) throws FHIRException { 1208 if (name.equals("uid")) { 1209 this.uid = null; 1210 } else if (name.equals("number")) { 1211 this.number = null; 1212 } else if (name.equals("modality")) { 1213 this.modality = null; 1214 } else if (name.equals("description")) { 1215 this.description = null; 1216 } else if (name.equals("numberOfInstances")) { 1217 this.numberOfInstances = null; 1218 } else if (name.equals("endpoint")) { 1219 this.getEndpoint().remove(castToReference(value)); 1220 } else if (name.equals("bodySite")) { 1221 this.bodySite = null; 1222 } else if (name.equals("laterality")) { 1223 this.laterality = null; 1224 } else if (name.equals("specimen")) { 1225 this.getSpecimen().remove(castToReference(value)); 1226 } else if (name.equals("started")) { 1227 this.started = null; 1228 } else if (name.equals("performer")) { 1229 this.getPerformer().remove((ImagingStudySeriesPerformerComponent) value); 1230 } else if (name.equals("instance")) { 1231 this.getInstance().remove((ImagingStudySeriesInstanceComponent) value); 1232 } else 1233 super.removeChild(name, value); 1234 1235 } 1236 1237 @Override 1238 public Base makeProperty(int hash, String name) throws FHIRException { 1239 switch (hash) { 1240 case 115792: 1241 return getUidElement(); 1242 case -1034364087: 1243 return getNumberElement(); 1244 case -622722335: 1245 return getModality(); 1246 case -1724546052: 1247 return getDescriptionElement(); 1248 case -1043544226: 1249 return getNumberOfInstancesElement(); 1250 case 1741102485: 1251 return addEndpoint(); 1252 case 1702620169: 1253 return getBodySite(); 1254 case -170291817: 1255 return getLaterality(); 1256 case -2132868344: 1257 return addSpecimen(); 1258 case -1897185151: 1259 return getStartedElement(); 1260 case 481140686: 1261 return addPerformer(); 1262 case 555127957: 1263 return addInstance(); 1264 default: 1265 return super.makeProperty(hash, name); 1266 } 1267 1268 } 1269 1270 @Override 1271 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1272 switch (hash) { 1273 case 115792: 1274 /* uid */ return new String[] { "id" }; 1275 case -1034364087: 1276 /* number */ return new String[] { "unsignedInt" }; 1277 case -622722335: 1278 /* modality */ return new String[] { "Coding" }; 1279 case -1724546052: 1280 /* description */ return new String[] { "string" }; 1281 case -1043544226: 1282 /* numberOfInstances */ return new String[] { "unsignedInt" }; 1283 case 1741102485: 1284 /* endpoint */ return new String[] { "Reference" }; 1285 case 1702620169: 1286 /* bodySite */ return new String[] { "Coding" }; 1287 case -170291817: 1288 /* laterality */ return new String[] { "Coding" }; 1289 case -2132868344: 1290 /* specimen */ return new String[] { "Reference" }; 1291 case -1897185151: 1292 /* started */ return new String[] { "dateTime" }; 1293 case 481140686: 1294 /* performer */ return new String[] {}; 1295 case 555127957: 1296 /* instance */ return new String[] {}; 1297 default: 1298 return super.getTypesForProperty(hash, name); 1299 } 1300 1301 } 1302 1303 @Override 1304 public Base addChild(String name) throws FHIRException { 1305 if (name.equals("uid")) { 1306 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 1307 } else if (name.equals("number")) { 1308 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.number"); 1309 } else if (name.equals("modality")) { 1310 this.modality = new Coding(); 1311 return this.modality; 1312 } else if (name.equals("description")) { 1313 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 1314 } else if (name.equals("numberOfInstances")) { 1315 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 1316 } else if (name.equals("endpoint")) { 1317 return addEndpoint(); 1318 } else if (name.equals("bodySite")) { 1319 this.bodySite = new Coding(); 1320 return this.bodySite; 1321 } else if (name.equals("laterality")) { 1322 this.laterality = new Coding(); 1323 return this.laterality; 1324 } else if (name.equals("specimen")) { 1325 return addSpecimen(); 1326 } else if (name.equals("started")) { 1327 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 1328 } else if (name.equals("performer")) { 1329 return addPerformer(); 1330 } else if (name.equals("instance")) { 1331 return addInstance(); 1332 } else 1333 return super.addChild(name); 1334 } 1335 1336 public ImagingStudySeriesComponent copy() { 1337 ImagingStudySeriesComponent dst = new ImagingStudySeriesComponent(); 1338 copyValues(dst); 1339 return dst; 1340 } 1341 1342 public void copyValues(ImagingStudySeriesComponent dst) { 1343 super.copyValues(dst); 1344 dst.uid = uid == null ? null : uid.copy(); 1345 dst.number = number == null ? null : number.copy(); 1346 dst.modality = modality == null ? null : modality.copy(); 1347 dst.description = description == null ? null : description.copy(); 1348 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 1349 if (endpoint != null) { 1350 dst.endpoint = new ArrayList<Reference>(); 1351 for (Reference i : endpoint) 1352 dst.endpoint.add(i.copy()); 1353 } 1354 ; 1355 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1356 dst.laterality = laterality == null ? null : laterality.copy(); 1357 if (specimen != null) { 1358 dst.specimen = new ArrayList<Reference>(); 1359 for (Reference i : specimen) 1360 dst.specimen.add(i.copy()); 1361 } 1362 ; 1363 dst.started = started == null ? null : started.copy(); 1364 if (performer != null) { 1365 dst.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 1366 for (ImagingStudySeriesPerformerComponent i : performer) 1367 dst.performer.add(i.copy()); 1368 } 1369 ; 1370 if (instance != null) { 1371 dst.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 1372 for (ImagingStudySeriesInstanceComponent i : instance) 1373 dst.instance.add(i.copy()); 1374 } 1375 ; 1376 } 1377 1378 @Override 1379 public boolean equalsDeep(Base other_) { 1380 if (!super.equalsDeep(other_)) 1381 return false; 1382 if (!(other_ instanceof ImagingStudySeriesComponent)) 1383 return false; 1384 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other_; 1385 return compareDeep(uid, o.uid, true) && compareDeep(number, o.number, true) 1386 && compareDeep(modality, o.modality, true) && compareDeep(description, o.description, true) 1387 && compareDeep(numberOfInstances, o.numberOfInstances, true) && compareDeep(endpoint, o.endpoint, true) 1388 && compareDeep(bodySite, o.bodySite, true) && compareDeep(laterality, o.laterality, true) 1389 && compareDeep(specimen, o.specimen, true) && compareDeep(started, o.started, true) 1390 && compareDeep(performer, o.performer, true) && compareDeep(instance, o.instance, true); 1391 } 1392 1393 @Override 1394 public boolean equalsShallow(Base other_) { 1395 if (!super.equalsShallow(other_)) 1396 return false; 1397 if (!(other_ instanceof ImagingStudySeriesComponent)) 1398 return false; 1399 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other_; 1400 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) 1401 && compareValues(description, o.description, true) 1402 && compareValues(numberOfInstances, o.numberOfInstances, true) && compareValues(started, o.started, true); 1403 } 1404 1405 public boolean isEmpty() { 1406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, number, modality, description, 1407 numberOfInstances, endpoint, bodySite, laterality, specimen, started, performer, instance); 1408 } 1409 1410 public String fhirType() { 1411 return "ImagingStudy.series"; 1412 1413 } 1414 1415 } 1416 1417 @Block() 1418 public static class ImagingStudySeriesPerformerComponent extends BackboneElement implements IBaseBackboneElement { 1419 /** 1420 * Distinguishes the type of involvement of the performer in the series. 1421 */ 1422 @Child(name = "function", type = { 1423 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1424 @Description(shortDefinition = "Type of performance", formalDefinition = "Distinguishes the type of involvement of the performer in the series.") 1425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/series-performer-function") 1426 protected CodeableConcept function; 1427 1428 /** 1429 * Indicates who or what performed the series. 1430 */ 1431 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 1432 Patient.class, Device.class, 1433 RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1434 @Description(shortDefinition = "Who performed the series", formalDefinition = "Indicates who or what performed the series.") 1435 protected Reference actor; 1436 1437 /** 1438 * The actual object that is the target of the reference (Indicates who or what 1439 * performed the series.) 1440 */ 1441 protected Resource actorTarget; 1442 1443 private static final long serialVersionUID = 1424001049L; 1444 1445 /** 1446 * Constructor 1447 */ 1448 public ImagingStudySeriesPerformerComponent() { 1449 super(); 1450 } 1451 1452 /** 1453 * Constructor 1454 */ 1455 public ImagingStudySeriesPerformerComponent(Reference actor) { 1456 super(); 1457 this.actor = actor; 1458 } 1459 1460 /** 1461 * @return {@link #function} (Distinguishes the type of involvement of the 1462 * performer in the series.) 1463 */ 1464 public CodeableConcept getFunction() { 1465 if (this.function == null) 1466 if (Configuration.errorOnAutoCreate()) 1467 throw new Error("Attempt to auto-create ImagingStudySeriesPerformerComponent.function"); 1468 else if (Configuration.doAutoCreate()) 1469 this.function = new CodeableConcept(); // cc 1470 return this.function; 1471 } 1472 1473 public boolean hasFunction() { 1474 return this.function != null && !this.function.isEmpty(); 1475 } 1476 1477 /** 1478 * @param value {@link #function} (Distinguishes the type of involvement of the 1479 * performer in the series.) 1480 */ 1481 public ImagingStudySeriesPerformerComponent setFunction(CodeableConcept value) { 1482 this.function = value; 1483 return this; 1484 } 1485 1486 /** 1487 * @return {@link #actor} (Indicates who or what performed the series.) 1488 */ 1489 public Reference getActor() { 1490 if (this.actor == null) 1491 if (Configuration.errorOnAutoCreate()) 1492 throw new Error("Attempt to auto-create ImagingStudySeriesPerformerComponent.actor"); 1493 else if (Configuration.doAutoCreate()) 1494 this.actor = new Reference(); // cc 1495 return this.actor; 1496 } 1497 1498 public boolean hasActor() { 1499 return this.actor != null && !this.actor.isEmpty(); 1500 } 1501 1502 /** 1503 * @param value {@link #actor} (Indicates who or what performed the series.) 1504 */ 1505 public ImagingStudySeriesPerformerComponent setActor(Reference value) { 1506 this.actor = value; 1507 return this; 1508 } 1509 1510 /** 1511 * @return {@link #actor} The actual object that is the target of the reference. 1512 * The reference library doesn't populate this, but you can use it to 1513 * hold the resource if you resolve it. (Indicates who or what performed 1514 * the series.) 1515 */ 1516 public Resource getActorTarget() { 1517 return this.actorTarget; 1518 } 1519 1520 /** 1521 * @param value {@link #actor} The actual object that is the target of the 1522 * reference. The reference library doesn't use these, but you can 1523 * use it to hold the resource if you resolve it. (Indicates who or 1524 * what performed the series.) 1525 */ 1526 public ImagingStudySeriesPerformerComponent setActorTarget(Resource value) { 1527 this.actorTarget = value; 1528 return this; 1529 } 1530 1531 protected void listChildren(List<Property> children) { 1532 super.listChildren(children); 1533 children.add(new Property("function", "CodeableConcept", 1534 "Distinguishes the type of involvement of the performer in the series.", 0, 1, function)); 1535 children.add(new Property("actor", 1536 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 1537 "Indicates who or what performed the series.", 0, 1, actor)); 1538 } 1539 1540 @Override 1541 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1542 switch (_hash) { 1543 case 1380938712: 1544 /* function */ return new Property("function", "CodeableConcept", 1545 "Distinguishes the type of involvement of the performer in the series.", 0, 1, function); 1546 case 92645877: 1547 /* actor */ return new Property("actor", 1548 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 1549 "Indicates who or what performed the series.", 0, 1, actor); 1550 default: 1551 return super.getNamedProperty(_hash, _name, _checkValid); 1552 } 1553 1554 } 1555 1556 @Override 1557 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1558 switch (hash) { 1559 case 1380938712: 1560 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 1561 case 92645877: 1562 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 1563 default: 1564 return super.getProperty(hash, name, checkValid); 1565 } 1566 1567 } 1568 1569 @Override 1570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1571 switch (hash) { 1572 case 1380938712: // function 1573 this.function = castToCodeableConcept(value); // CodeableConcept 1574 return value; 1575 case 92645877: // actor 1576 this.actor = castToReference(value); // Reference 1577 return value; 1578 default: 1579 return super.setProperty(hash, name, value); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base setProperty(String name, Base value) throws FHIRException { 1586 if (name.equals("function")) { 1587 this.function = castToCodeableConcept(value); // CodeableConcept 1588 } else if (name.equals("actor")) { 1589 this.actor = castToReference(value); // Reference 1590 } else 1591 return super.setProperty(name, value); 1592 return value; 1593 } 1594 1595 @Override 1596 public void removeChild(String name, Base value) throws FHIRException { 1597 if (name.equals("function")) { 1598 this.function = null; 1599 } else if (name.equals("actor")) { 1600 this.actor = null; 1601 } else 1602 super.removeChild(name, value); 1603 1604 } 1605 1606 @Override 1607 public Base makeProperty(int hash, String name) throws FHIRException { 1608 switch (hash) { 1609 case 1380938712: 1610 return getFunction(); 1611 case 92645877: 1612 return getActor(); 1613 default: 1614 return super.makeProperty(hash, name); 1615 } 1616 1617 } 1618 1619 @Override 1620 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1621 switch (hash) { 1622 case 1380938712: 1623 /* function */ return new String[] { "CodeableConcept" }; 1624 case 92645877: 1625 /* actor */ return new String[] { "Reference" }; 1626 default: 1627 return super.getTypesForProperty(hash, name); 1628 } 1629 1630 } 1631 1632 @Override 1633 public Base addChild(String name) throws FHIRException { 1634 if (name.equals("function")) { 1635 this.function = new CodeableConcept(); 1636 return this.function; 1637 } else if (name.equals("actor")) { 1638 this.actor = new Reference(); 1639 return this.actor; 1640 } else 1641 return super.addChild(name); 1642 } 1643 1644 public ImagingStudySeriesPerformerComponent copy() { 1645 ImagingStudySeriesPerformerComponent dst = new ImagingStudySeriesPerformerComponent(); 1646 copyValues(dst); 1647 return dst; 1648 } 1649 1650 public void copyValues(ImagingStudySeriesPerformerComponent dst) { 1651 super.copyValues(dst); 1652 dst.function = function == null ? null : function.copy(); 1653 dst.actor = actor == null ? null : actor.copy(); 1654 } 1655 1656 @Override 1657 public boolean equalsDeep(Base other_) { 1658 if (!super.equalsDeep(other_)) 1659 return false; 1660 if (!(other_ instanceof ImagingStudySeriesPerformerComponent)) 1661 return false; 1662 ImagingStudySeriesPerformerComponent o = (ImagingStudySeriesPerformerComponent) other_; 1663 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 1664 } 1665 1666 @Override 1667 public boolean equalsShallow(Base other_) { 1668 if (!super.equalsShallow(other_)) 1669 return false; 1670 if (!(other_ instanceof ImagingStudySeriesPerformerComponent)) 1671 return false; 1672 ImagingStudySeriesPerformerComponent o = (ImagingStudySeriesPerformerComponent) other_; 1673 return true; 1674 } 1675 1676 public boolean isEmpty() { 1677 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 1678 } 1679 1680 public String fhirType() { 1681 return "ImagingStudy.series.performer"; 1682 1683 } 1684 1685 } 1686 1687 @Block() 1688 public static class ImagingStudySeriesInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1689 /** 1690 * The DICOM SOP Instance UID for this image or other DICOM content. 1691 */ 1692 @Child(name = "uid", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1693 @Description(shortDefinition = "DICOM SOP Instance UID", formalDefinition = "The DICOM SOP Instance UID for this image or other DICOM content.") 1694 protected IdType uid; 1695 1696 /** 1697 * DICOM instance type. 1698 */ 1699 @Child(name = "sopClass", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1700 @Description(shortDefinition = "DICOM class type", formalDefinition = "DICOM instance type.") 1701 protected Coding sopClass; 1702 1703 /** 1704 * The number of instance in the series. 1705 */ 1706 @Child(name = "number", type = { 1707 UnsignedIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1708 @Description(shortDefinition = "The number of this instance in the series", formalDefinition = "The number of instance in the series.") 1709 protected UnsignedIntType number; 1710 1711 /** 1712 * The description of the instance. 1713 */ 1714 @Child(name = "title", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1715 @Description(shortDefinition = "Description of instance", formalDefinition = "The description of the instance.") 1716 protected StringType title; 1717 1718 private static final long serialVersionUID = -888152445L; 1719 1720 /** 1721 * Constructor 1722 */ 1723 public ImagingStudySeriesInstanceComponent() { 1724 super(); 1725 } 1726 1727 /** 1728 * Constructor 1729 */ 1730 public ImagingStudySeriesInstanceComponent(IdType uid, Coding sopClass) { 1731 super(); 1732 this.uid = uid; 1733 this.sopClass = sopClass; 1734 } 1735 1736 /** 1737 * @return {@link #uid} (The DICOM SOP Instance UID for this image or other 1738 * DICOM content.). This is the underlying object with id, value and 1739 * extensions. The accessor "getUid" gives direct access to the value 1740 */ 1741 public IdType getUidElement() { 1742 if (this.uid == null) 1743 if (Configuration.errorOnAutoCreate()) 1744 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.uid"); 1745 else if (Configuration.doAutoCreate()) 1746 this.uid = new IdType(); // bb 1747 return this.uid; 1748 } 1749 1750 public boolean hasUidElement() { 1751 return this.uid != null && !this.uid.isEmpty(); 1752 } 1753 1754 public boolean hasUid() { 1755 return this.uid != null && !this.uid.isEmpty(); 1756 } 1757 1758 /** 1759 * @param value {@link #uid} (The DICOM SOP Instance UID for this image or other 1760 * DICOM content.). This is the underlying object with id, value 1761 * and extensions. The accessor "getUid" gives direct access to the 1762 * value 1763 */ 1764 public ImagingStudySeriesInstanceComponent setUidElement(IdType value) { 1765 this.uid = value; 1766 return this; 1767 } 1768 1769 /** 1770 * @return The DICOM SOP Instance UID for this image or other DICOM content. 1771 */ 1772 public String getUid() { 1773 return this.uid == null ? null : this.uid.getValue(); 1774 } 1775 1776 /** 1777 * @param value The DICOM SOP Instance UID for this image or other DICOM 1778 * content. 1779 */ 1780 public ImagingStudySeriesInstanceComponent setUid(String value) { 1781 if (this.uid == null) 1782 this.uid = new IdType(); 1783 this.uid.setValue(value); 1784 return this; 1785 } 1786 1787 /** 1788 * @return {@link #sopClass} (DICOM instance type.) 1789 */ 1790 public Coding getSopClass() { 1791 if (this.sopClass == null) 1792 if (Configuration.errorOnAutoCreate()) 1793 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.sopClass"); 1794 else if (Configuration.doAutoCreate()) 1795 this.sopClass = new Coding(); // cc 1796 return this.sopClass; 1797 } 1798 1799 public boolean hasSopClass() { 1800 return this.sopClass != null && !this.sopClass.isEmpty(); 1801 } 1802 1803 /** 1804 * @param value {@link #sopClass} (DICOM instance type.) 1805 */ 1806 public ImagingStudySeriesInstanceComponent setSopClass(Coding value) { 1807 this.sopClass = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return {@link #number} (The number of instance in the series.). This is the 1813 * underlying object with id, value and extensions. The accessor 1814 * "getNumber" gives direct access to the value 1815 */ 1816 public UnsignedIntType getNumberElement() { 1817 if (this.number == null) 1818 if (Configuration.errorOnAutoCreate()) 1819 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.number"); 1820 else if (Configuration.doAutoCreate()) 1821 this.number = new UnsignedIntType(); // bb 1822 return this.number; 1823 } 1824 1825 public boolean hasNumberElement() { 1826 return this.number != null && !this.number.isEmpty(); 1827 } 1828 1829 public boolean hasNumber() { 1830 return this.number != null && !this.number.isEmpty(); 1831 } 1832 1833 /** 1834 * @param value {@link #number} (The number of instance in the series.). This is 1835 * the underlying object with id, value and extensions. The 1836 * accessor "getNumber" gives direct access to the value 1837 */ 1838 public ImagingStudySeriesInstanceComponent setNumberElement(UnsignedIntType value) { 1839 this.number = value; 1840 return this; 1841 } 1842 1843 /** 1844 * @return The number of instance in the series. 1845 */ 1846 public int getNumber() { 1847 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 1848 } 1849 1850 /** 1851 * @param value The number of instance in the series. 1852 */ 1853 public ImagingStudySeriesInstanceComponent setNumber(int value) { 1854 if (this.number == null) 1855 this.number = new UnsignedIntType(); 1856 this.number.setValue(value); 1857 return this; 1858 } 1859 1860 /** 1861 * @return {@link #title} (The description of the instance.). This is the 1862 * underlying object with id, value and extensions. The accessor 1863 * "getTitle" gives direct access to the value 1864 */ 1865 public StringType getTitleElement() { 1866 if (this.title == null) 1867 if (Configuration.errorOnAutoCreate()) 1868 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.title"); 1869 else if (Configuration.doAutoCreate()) 1870 this.title = new StringType(); // bb 1871 return this.title; 1872 } 1873 1874 public boolean hasTitleElement() { 1875 return this.title != null && !this.title.isEmpty(); 1876 } 1877 1878 public boolean hasTitle() { 1879 return this.title != null && !this.title.isEmpty(); 1880 } 1881 1882 /** 1883 * @param value {@link #title} (The description of the instance.). This is the 1884 * underlying object with id, value and extensions. The accessor 1885 * "getTitle" gives direct access to the value 1886 */ 1887 public ImagingStudySeriesInstanceComponent setTitleElement(StringType value) { 1888 this.title = value; 1889 return this; 1890 } 1891 1892 /** 1893 * @return The description of the instance. 1894 */ 1895 public String getTitle() { 1896 return this.title == null ? null : this.title.getValue(); 1897 } 1898 1899 /** 1900 * @param value The description of the instance. 1901 */ 1902 public ImagingStudySeriesInstanceComponent setTitle(String value) { 1903 if (Utilities.noString(value)) 1904 this.title = null; 1905 else { 1906 if (this.title == null) 1907 this.title = new StringType(); 1908 this.title.setValue(value); 1909 } 1910 return this; 1911 } 1912 1913 protected void listChildren(List<Property> children) { 1914 super.listChildren(children); 1915 children.add( 1916 new Property("uid", "id", "The DICOM SOP Instance UID for this image or other DICOM content.", 0, 1, uid)); 1917 children.add(new Property("sopClass", "Coding", "DICOM instance type.", 0, 1, sopClass)); 1918 children.add(new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1, number)); 1919 children.add(new Property("title", "string", "The description of the instance.", 0, 1, title)); 1920 } 1921 1922 @Override 1923 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1924 switch (_hash) { 1925 case 115792: 1926 /* uid */ return new Property("uid", "id", "The DICOM SOP Instance UID for this image or other DICOM content.", 1927 0, 1, uid); 1928 case 1560041540: 1929 /* sopClass */ return new Property("sopClass", "Coding", "DICOM instance type.", 0, 1, sopClass); 1930 case -1034364087: 1931 /* number */ return new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1, 1932 number); 1933 case 110371416: 1934 /* title */ return new Property("title", "string", "The description of the instance.", 0, 1, title); 1935 default: 1936 return super.getNamedProperty(_hash, _name, _checkValid); 1937 } 1938 1939 } 1940 1941 @Override 1942 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1943 switch (hash) { 1944 case 115792: 1945 /* uid */ return this.uid == null ? new Base[0] : new Base[] { this.uid }; // IdType 1946 case 1560041540: 1947 /* sopClass */ return this.sopClass == null ? new Base[0] : new Base[] { this.sopClass }; // Coding 1948 case -1034364087: 1949 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // UnsignedIntType 1950 case 110371416: 1951 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 1952 default: 1953 return super.getProperty(hash, name, checkValid); 1954 } 1955 1956 } 1957 1958 @Override 1959 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1960 switch (hash) { 1961 case 115792: // uid 1962 this.uid = castToId(value); // IdType 1963 return value; 1964 case 1560041540: // sopClass 1965 this.sopClass = castToCoding(value); // Coding 1966 return value; 1967 case -1034364087: // number 1968 this.number = castToUnsignedInt(value); // UnsignedIntType 1969 return value; 1970 case 110371416: // title 1971 this.title = castToString(value); // StringType 1972 return value; 1973 default: 1974 return super.setProperty(hash, name, value); 1975 } 1976 1977 } 1978 1979 @Override 1980 public Base setProperty(String name, Base value) throws FHIRException { 1981 if (name.equals("uid")) { 1982 this.uid = castToId(value); // IdType 1983 } else if (name.equals("sopClass")) { 1984 this.sopClass = castToCoding(value); // Coding 1985 } else if (name.equals("number")) { 1986 this.number = castToUnsignedInt(value); // UnsignedIntType 1987 } else if (name.equals("title")) { 1988 this.title = castToString(value); // StringType 1989 } else 1990 return super.setProperty(name, value); 1991 return value; 1992 } 1993 1994 @Override 1995 public void removeChild(String name, Base value) throws FHIRException { 1996 if (name.equals("uid")) { 1997 this.uid = null; 1998 } else if (name.equals("sopClass")) { 1999 this.sopClass = null; 2000 } else if (name.equals("number")) { 2001 this.number = null; 2002 } else if (name.equals("title")) { 2003 this.title = null; 2004 } else 2005 super.removeChild(name, value); 2006 2007 } 2008 2009 @Override 2010 public Base makeProperty(int hash, String name) throws FHIRException { 2011 switch (hash) { 2012 case 115792: 2013 return getUidElement(); 2014 case 1560041540: 2015 return getSopClass(); 2016 case -1034364087: 2017 return getNumberElement(); 2018 case 110371416: 2019 return getTitleElement(); 2020 default: 2021 return super.makeProperty(hash, name); 2022 } 2023 2024 } 2025 2026 @Override 2027 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2028 switch (hash) { 2029 case 115792: 2030 /* uid */ return new String[] { "id" }; 2031 case 1560041540: 2032 /* sopClass */ return new String[] { "Coding" }; 2033 case -1034364087: 2034 /* number */ return new String[] { "unsignedInt" }; 2035 case 110371416: 2036 /* title */ return new String[] { "string" }; 2037 default: 2038 return super.getTypesForProperty(hash, name); 2039 } 2040 2041 } 2042 2043 @Override 2044 public Base addChild(String name) throws FHIRException { 2045 if (name.equals("uid")) { 2046 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 2047 } else if (name.equals("sopClass")) { 2048 this.sopClass = new Coding(); 2049 return this.sopClass; 2050 } else if (name.equals("number")) { 2051 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.number"); 2052 } else if (name.equals("title")) { 2053 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.title"); 2054 } else 2055 return super.addChild(name); 2056 } 2057 2058 public ImagingStudySeriesInstanceComponent copy() { 2059 ImagingStudySeriesInstanceComponent dst = new ImagingStudySeriesInstanceComponent(); 2060 copyValues(dst); 2061 return dst; 2062 } 2063 2064 public void copyValues(ImagingStudySeriesInstanceComponent dst) { 2065 super.copyValues(dst); 2066 dst.uid = uid == null ? null : uid.copy(); 2067 dst.sopClass = sopClass == null ? null : sopClass.copy(); 2068 dst.number = number == null ? null : number.copy(); 2069 dst.title = title == null ? null : title.copy(); 2070 } 2071 2072 @Override 2073 public boolean equalsDeep(Base other_) { 2074 if (!super.equalsDeep(other_)) 2075 return false; 2076 if (!(other_ instanceof ImagingStudySeriesInstanceComponent)) 2077 return false; 2078 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other_; 2079 return compareDeep(uid, o.uid, true) && compareDeep(sopClass, o.sopClass, true) 2080 && compareDeep(number, o.number, true) && compareDeep(title, o.title, true); 2081 } 2082 2083 @Override 2084 public boolean equalsShallow(Base other_) { 2085 if (!super.equalsShallow(other_)) 2086 return false; 2087 if (!(other_ instanceof ImagingStudySeriesInstanceComponent)) 2088 return false; 2089 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other_; 2090 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) 2091 && compareValues(title, o.title, true); 2092 } 2093 2094 public boolean isEmpty() { 2095 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, sopClass, number, title); 2096 } 2097 2098 public String fhirType() { 2099 return "ImagingStudy.series.instance"; 2100 2101 } 2102 2103 } 2104 2105 /** 2106 * Identifiers for the ImagingStudy such as DICOM Study Instance UID, and 2107 * Accession Number. 2108 */ 2109 @Child(name = "identifier", type = { 2110 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2111 @Description(shortDefinition = "Identifiers for the whole study", formalDefinition = "Identifiers for the ImagingStudy such as DICOM Study Instance UID, and Accession Number.") 2112 protected List<Identifier> identifier; 2113 2114 /** 2115 * The current state of the ImagingStudy. 2116 */ 2117 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2118 @Description(shortDefinition = "registered | available | cancelled | entered-in-error | unknown", formalDefinition = "The current state of the ImagingStudy.") 2119 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/imagingstudy-status") 2120 protected Enumeration<ImagingStudyStatus> status; 2121 2122 /** 2123 * A list of all the series.modality values that are actual acquisition 2124 * modalities, i.e. those in the DICOM Context Group 29 (value set OID 2125 * 1.2.840.10008.6.1.19). 2126 */ 2127 @Child(name = "modality", type = { 2128 Coding.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2129 @Description(shortDefinition = "All series modality if actual acquisition modalities", formalDefinition = "A list of all the series.modality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).") 2130 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_29.html") 2131 protected List<Coding> modality; 2132 2133 /** 2134 * The subject, typically a patient, of the imaging study. 2135 */ 2136 @Child(name = "subject", type = { Patient.class, Device.class, 2137 Group.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2138 @Description(shortDefinition = "Who or what is the subject of the study", formalDefinition = "The subject, typically a patient, of the imaging study.") 2139 protected Reference subject; 2140 2141 /** 2142 * The actual object that is the target of the reference (The subject, typically 2143 * a patient, of the imaging study.) 2144 */ 2145 protected Resource subjectTarget; 2146 2147 /** 2148 * The healthcare event (e.g. a patient and healthcare provider interaction) 2149 * during which this ImagingStudy is made. 2150 */ 2151 @Child(name = "encounter", type = { Encounter.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2152 @Description(shortDefinition = "Encounter with which this imaging study is associated", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.") 2153 protected Reference encounter; 2154 2155 /** 2156 * The actual object that is the target of the reference (The healthcare event 2157 * (e.g. a patient and healthcare provider interaction) during which this 2158 * ImagingStudy is made.) 2159 */ 2160 protected Encounter encounterTarget; 2161 2162 /** 2163 * Date and time the study started. 2164 */ 2165 @Child(name = "started", type = { DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2166 @Description(shortDefinition = "When the study was started", formalDefinition = "Date and time the study started.") 2167 protected DateTimeType started; 2168 2169 /** 2170 * A list of the diagnostic requests that resulted in this imaging study being 2171 * performed. 2172 */ 2173 @Child(name = "basedOn", type = { CarePlan.class, ServiceRequest.class, Appointment.class, AppointmentResponse.class, 2174 Task.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2175 @Description(shortDefinition = "Request fulfilled", formalDefinition = "A list of the diagnostic requests that resulted in this imaging study being performed.") 2176 protected List<Reference> basedOn; 2177 /** 2178 * The actual objects that are the target of the reference (A list of the 2179 * diagnostic requests that resulted in this imaging study being performed.) 2180 */ 2181 protected List<Resource> basedOnTarget; 2182 2183 /** 2184 * The requesting/referring physician. 2185 */ 2186 @Child(name = "referrer", type = { Practitioner.class, 2187 PractitionerRole.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2188 @Description(shortDefinition = "Referring physician", formalDefinition = "The requesting/referring physician.") 2189 protected Reference referrer; 2190 2191 /** 2192 * The actual object that is the target of the reference (The 2193 * requesting/referring physician.) 2194 */ 2195 protected Resource referrerTarget; 2196 2197 /** 2198 * Who read the study and interpreted the images or other content. 2199 */ 2200 @Child(name = "interpreter", type = { Practitioner.class, 2201 PractitionerRole.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2202 @Description(shortDefinition = "Who interpreted images", formalDefinition = "Who read the study and interpreted the images or other content.") 2203 protected List<Reference> interpreter; 2204 /** 2205 * The actual objects that are the target of the reference (Who read the study 2206 * and interpreted the images or other content.) 2207 */ 2208 protected List<Resource> interpreterTarget; 2209 2210 /** 2211 * The network service providing access (e.g., query, view, or retrieval) for 2212 * the study. See implementation notes for information about using DICOM 2213 * endpoints. A study-level endpoint applies to each series in the study, unless 2214 * overridden by a series-level endpoint with the same Endpoint.connectionType. 2215 */ 2216 @Child(name = "endpoint", type = { 2217 Endpoint.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2218 @Description(shortDefinition = "Study access endpoint", formalDefinition = "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType.") 2219 protected List<Reference> endpoint; 2220 /** 2221 * The actual objects that are the target of the reference (The network service 2222 * providing access (e.g., query, view, or retrieval) for the study. See 2223 * implementation notes for information about using DICOM endpoints. A 2224 * study-level endpoint applies to each series in the study, unless overridden 2225 * by a series-level endpoint with the same Endpoint.connectionType.) 2226 */ 2227 protected List<Endpoint> endpointTarget; 2228 2229 /** 2230 * Number of Series in the Study. This value given may be larger than the number 2231 * of series elements this Resource contains due to resource availability, 2232 * security, or other factors. This element should be present if any series 2233 * elements are present. 2234 */ 2235 @Child(name = "numberOfSeries", type = { 2236 UnsignedIntType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2237 @Description(shortDefinition = "Number of Study Related Series", formalDefinition = "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.") 2238 protected UnsignedIntType numberOfSeries; 2239 2240 /** 2241 * Number of SOP Instances in Study. This value given may be larger than the 2242 * number of instance elements this resource contains due to resource 2243 * availability, security, or other factors. This element should be present if 2244 * any instance elements are present. 2245 */ 2246 @Child(name = "numberOfInstances", type = { 2247 UnsignedIntType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2248 @Description(shortDefinition = "Number of Study Related Instances", formalDefinition = "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.") 2249 protected UnsignedIntType numberOfInstances; 2250 2251 /** 2252 * The procedure which this ImagingStudy was part of. 2253 */ 2254 @Child(name = "procedureReference", type = { 2255 Procedure.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2256 @Description(shortDefinition = "The performed Procedure reference", formalDefinition = "The procedure which this ImagingStudy was part of.") 2257 protected Reference procedureReference; 2258 2259 /** 2260 * The actual object that is the target of the reference (The procedure which 2261 * this ImagingStudy was part of.) 2262 */ 2263 protected Procedure procedureReferenceTarget; 2264 2265 /** 2266 * The code for the performed procedure type. 2267 */ 2268 @Child(name = "procedureCode", type = { 2269 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2270 @Description(shortDefinition = "The performed procedure code", formalDefinition = "The code for the performed procedure type.") 2271 protected List<CodeableConcept> procedureCode; 2272 2273 /** 2274 * The principal physical location where the ImagingStudy was performed. 2275 */ 2276 @Child(name = "location", type = { Location.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 2277 @Description(shortDefinition = "Where ImagingStudy occurred", formalDefinition = "The principal physical location where the ImagingStudy was performed.") 2278 protected Reference location; 2279 2280 /** 2281 * The actual object that is the target of the reference (The principal physical 2282 * location where the ImagingStudy was performed.) 2283 */ 2284 protected Location locationTarget; 2285 2286 /** 2287 * Description of clinical condition indicating why the ImagingStudy was 2288 * requested. 2289 */ 2290 @Child(name = "reasonCode", type = { 2291 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2292 @Description(shortDefinition = "Why the study was requested", formalDefinition = "Description of clinical condition indicating why the ImagingStudy was requested.") 2293 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-reason") 2294 protected List<CodeableConcept> reasonCode; 2295 2296 /** 2297 * Indicates another resource whose existence justifies this Study. 2298 */ 2299 @Child(name = "reasonReference", type = { Condition.class, Observation.class, Media.class, DiagnosticReport.class, 2300 DocumentReference.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2301 @Description(shortDefinition = "Why was study performed", formalDefinition = "Indicates another resource whose existence justifies this Study.") 2302 protected List<Reference> reasonReference; 2303 /** 2304 * The actual objects that are the target of the reference (Indicates another 2305 * resource whose existence justifies this Study.) 2306 */ 2307 protected List<Resource> reasonReferenceTarget; 2308 2309 /** 2310 * Per the recommended DICOM mapping, this element is derived from the Study 2311 * Description attribute (0008,1030). Observations or findings about the imaging 2312 * study should be recorded in another resource, e.g. Observation, and not in 2313 * this element. 2314 */ 2315 @Child(name = "note", type = { 2316 Annotation.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2317 @Description(shortDefinition = "User-defined comments", formalDefinition = "Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element.") 2318 protected List<Annotation> note; 2319 2320 /** 2321 * The Imaging Manager description of the study. Institution-generated 2322 * description or classification of the Study (component) performed. 2323 */ 2324 @Child(name = "description", type = { 2325 StringType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 2326 @Description(shortDefinition = "Institution-generated description", formalDefinition = "The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.") 2327 protected StringType description; 2328 2329 /** 2330 * Each study has one or more series of images or other content. 2331 */ 2332 @Child(name = "series", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2333 @Description(shortDefinition = "Each study has one or more series of instances", formalDefinition = "Each study has one or more series of images or other content.") 2334 protected List<ImagingStudySeriesComponent> series; 2335 2336 private static final long serialVersionUID = -647973361L; 2337 2338 /** 2339 * Constructor 2340 */ 2341 public ImagingStudy() { 2342 super(); 2343 } 2344 2345 /** 2346 * Constructor 2347 */ 2348 public ImagingStudy(Enumeration<ImagingStudyStatus> status, Reference subject) { 2349 super(); 2350 this.status = status; 2351 this.subject = subject; 2352 } 2353 2354 /** 2355 * @return {@link #identifier} (Identifiers for the ImagingStudy such as DICOM 2356 * Study Instance UID, and Accession Number.) 2357 */ 2358 public List<Identifier> getIdentifier() { 2359 if (this.identifier == null) 2360 this.identifier = new ArrayList<Identifier>(); 2361 return this.identifier; 2362 } 2363 2364 /** 2365 * @return Returns a reference to <code>this</code> for easy method chaining 2366 */ 2367 public ImagingStudy setIdentifier(List<Identifier> theIdentifier) { 2368 this.identifier = theIdentifier; 2369 return this; 2370 } 2371 2372 public boolean hasIdentifier() { 2373 if (this.identifier == null) 2374 return false; 2375 for (Identifier item : this.identifier) 2376 if (!item.isEmpty()) 2377 return true; 2378 return false; 2379 } 2380 2381 public Identifier addIdentifier() { // 3 2382 Identifier t = new Identifier(); 2383 if (this.identifier == null) 2384 this.identifier = new ArrayList<Identifier>(); 2385 this.identifier.add(t); 2386 return t; 2387 } 2388 2389 public ImagingStudy addIdentifier(Identifier t) { // 3 2390 if (t == null) 2391 return this; 2392 if (this.identifier == null) 2393 this.identifier = new ArrayList<Identifier>(); 2394 this.identifier.add(t); 2395 return this; 2396 } 2397 2398 /** 2399 * @return The first repetition of repeating field {@link #identifier}, creating 2400 * it if it does not already exist 2401 */ 2402 public Identifier getIdentifierFirstRep() { 2403 if (getIdentifier().isEmpty()) { 2404 addIdentifier(); 2405 } 2406 return getIdentifier().get(0); 2407 } 2408 2409 /** 2410 * @return {@link #status} (The current state of the ImagingStudy.). This is the 2411 * underlying object with id, value and extensions. The accessor 2412 * "getStatus" gives direct access to the value 2413 */ 2414 public Enumeration<ImagingStudyStatus> getStatusElement() { 2415 if (this.status == null) 2416 if (Configuration.errorOnAutoCreate()) 2417 throw new Error("Attempt to auto-create ImagingStudy.status"); 2418 else if (Configuration.doAutoCreate()) 2419 this.status = new Enumeration<ImagingStudyStatus>(new ImagingStudyStatusEnumFactory()); // bb 2420 return this.status; 2421 } 2422 2423 public boolean hasStatusElement() { 2424 return this.status != null && !this.status.isEmpty(); 2425 } 2426 2427 public boolean hasStatus() { 2428 return this.status != null && !this.status.isEmpty(); 2429 } 2430 2431 /** 2432 * @param value {@link #status} (The current state of the ImagingStudy.). This 2433 * is the underlying object with id, value and extensions. The 2434 * accessor "getStatus" gives direct access to the value 2435 */ 2436 public ImagingStudy setStatusElement(Enumeration<ImagingStudyStatus> value) { 2437 this.status = value; 2438 return this; 2439 } 2440 2441 /** 2442 * @return The current state of the ImagingStudy. 2443 */ 2444 public ImagingStudyStatus getStatus() { 2445 return this.status == null ? null : this.status.getValue(); 2446 } 2447 2448 /** 2449 * @param value The current state of the ImagingStudy. 2450 */ 2451 public ImagingStudy setStatus(ImagingStudyStatus value) { 2452 if (this.status == null) 2453 this.status = new Enumeration<ImagingStudyStatus>(new ImagingStudyStatusEnumFactory()); 2454 this.status.setValue(value); 2455 return this; 2456 } 2457 2458 /** 2459 * @return {@link #modality} (A list of all the series.modality values that are 2460 * actual acquisition modalities, i.e. those in the DICOM Context Group 2461 * 29 (value set OID 1.2.840.10008.6.1.19).) 2462 */ 2463 public List<Coding> getModality() { 2464 if (this.modality == null) 2465 this.modality = new ArrayList<Coding>(); 2466 return this.modality; 2467 } 2468 2469 /** 2470 * @return Returns a reference to <code>this</code> for easy method chaining 2471 */ 2472 public ImagingStudy setModality(List<Coding> theModality) { 2473 this.modality = theModality; 2474 return this; 2475 } 2476 2477 public boolean hasModality() { 2478 if (this.modality == null) 2479 return false; 2480 for (Coding item : this.modality) 2481 if (!item.isEmpty()) 2482 return true; 2483 return false; 2484 } 2485 2486 public Coding addModality() { // 3 2487 Coding t = new Coding(); 2488 if (this.modality == null) 2489 this.modality = new ArrayList<Coding>(); 2490 this.modality.add(t); 2491 return t; 2492 } 2493 2494 public ImagingStudy addModality(Coding t) { // 3 2495 if (t == null) 2496 return this; 2497 if (this.modality == null) 2498 this.modality = new ArrayList<Coding>(); 2499 this.modality.add(t); 2500 return this; 2501 } 2502 2503 /** 2504 * @return The first repetition of repeating field {@link #modality}, creating 2505 * it if it does not already exist 2506 */ 2507 public Coding getModalityFirstRep() { 2508 if (getModality().isEmpty()) { 2509 addModality(); 2510 } 2511 return getModality().get(0); 2512 } 2513 2514 /** 2515 * @return {@link #subject} (The subject, typically a patient, of the imaging 2516 * study.) 2517 */ 2518 public Reference getSubject() { 2519 if (this.subject == null) 2520 if (Configuration.errorOnAutoCreate()) 2521 throw new Error("Attempt to auto-create ImagingStudy.subject"); 2522 else if (Configuration.doAutoCreate()) 2523 this.subject = new Reference(); // cc 2524 return this.subject; 2525 } 2526 2527 public boolean hasSubject() { 2528 return this.subject != null && !this.subject.isEmpty(); 2529 } 2530 2531 /** 2532 * @param value {@link #subject} (The subject, typically a patient, of the 2533 * imaging study.) 2534 */ 2535 public ImagingStudy setSubject(Reference value) { 2536 this.subject = value; 2537 return this; 2538 } 2539 2540 /** 2541 * @return {@link #subject} The actual object that is the target of the 2542 * reference. The reference library doesn't populate this, but you can 2543 * use it to hold the resource if you resolve it. (The subject, 2544 * typically a patient, of the imaging study.) 2545 */ 2546 public Resource getSubjectTarget() { 2547 return this.subjectTarget; 2548 } 2549 2550 /** 2551 * @param value {@link #subject} The actual object that is the target of the 2552 * reference. The reference library doesn't use these, but you can 2553 * use it to hold the resource if you resolve it. (The subject, 2554 * typically a patient, of the imaging study.) 2555 */ 2556 public ImagingStudy setSubjectTarget(Resource value) { 2557 this.subjectTarget = value; 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #encounter} (The healthcare event (e.g. a patient and 2563 * healthcare provider interaction) during which this ImagingStudy is 2564 * made.) 2565 */ 2566 public Reference getEncounter() { 2567 if (this.encounter == null) 2568 if (Configuration.errorOnAutoCreate()) 2569 throw new Error("Attempt to auto-create ImagingStudy.encounter"); 2570 else if (Configuration.doAutoCreate()) 2571 this.encounter = new Reference(); // cc 2572 return this.encounter; 2573 } 2574 2575 public boolean hasEncounter() { 2576 return this.encounter != null && !this.encounter.isEmpty(); 2577 } 2578 2579 /** 2580 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 2581 * healthcare provider interaction) during which this ImagingStudy 2582 * is made.) 2583 */ 2584 public ImagingStudy setEncounter(Reference value) { 2585 this.encounter = value; 2586 return this; 2587 } 2588 2589 /** 2590 * @return {@link #encounter} The actual object that is the target of the 2591 * reference. The reference library doesn't populate this, but you can 2592 * use it to hold the resource if you resolve it. (The healthcare event 2593 * (e.g. a patient and healthcare provider interaction) during which 2594 * this ImagingStudy is made.) 2595 */ 2596 public Encounter getEncounterTarget() { 2597 if (this.encounterTarget == null) 2598 if (Configuration.errorOnAutoCreate()) 2599 throw new Error("Attempt to auto-create ImagingStudy.encounter"); 2600 else if (Configuration.doAutoCreate()) 2601 this.encounterTarget = new Encounter(); // aa 2602 return this.encounterTarget; 2603 } 2604 2605 /** 2606 * @param value {@link #encounter} The actual object that is the target of the 2607 * reference. The reference library doesn't use these, but you can 2608 * use it to hold the resource if you resolve it. (The healthcare 2609 * event (e.g. a patient and healthcare provider interaction) 2610 * during which this ImagingStudy is made.) 2611 */ 2612 public ImagingStudy setEncounterTarget(Encounter value) { 2613 this.encounterTarget = value; 2614 return this; 2615 } 2616 2617 /** 2618 * @return {@link #started} (Date and time the study started.). This is the 2619 * underlying object with id, value and extensions. The accessor 2620 * "getStarted" gives direct access to the value 2621 */ 2622 public DateTimeType getStartedElement() { 2623 if (this.started == null) 2624 if (Configuration.errorOnAutoCreate()) 2625 throw new Error("Attempt to auto-create ImagingStudy.started"); 2626 else if (Configuration.doAutoCreate()) 2627 this.started = new DateTimeType(); // bb 2628 return this.started; 2629 } 2630 2631 public boolean hasStartedElement() { 2632 return this.started != null && !this.started.isEmpty(); 2633 } 2634 2635 public boolean hasStarted() { 2636 return this.started != null && !this.started.isEmpty(); 2637 } 2638 2639 /** 2640 * @param value {@link #started} (Date and time the study started.). This is the 2641 * underlying object with id, value and extensions. The accessor 2642 * "getStarted" gives direct access to the value 2643 */ 2644 public ImagingStudy setStartedElement(DateTimeType value) { 2645 this.started = value; 2646 return this; 2647 } 2648 2649 /** 2650 * @return Date and time the study started. 2651 */ 2652 public Date getStarted() { 2653 return this.started == null ? null : this.started.getValue(); 2654 } 2655 2656 /** 2657 * @param value Date and time the study started. 2658 */ 2659 public ImagingStudy setStarted(Date value) { 2660 if (value == null) 2661 this.started = null; 2662 else { 2663 if (this.started == null) 2664 this.started = new DateTimeType(); 2665 this.started.setValue(value); 2666 } 2667 return this; 2668 } 2669 2670 /** 2671 * @return {@link #basedOn} (A list of the diagnostic requests that resulted in 2672 * this imaging study being performed.) 2673 */ 2674 public List<Reference> getBasedOn() { 2675 if (this.basedOn == null) 2676 this.basedOn = new ArrayList<Reference>(); 2677 return this.basedOn; 2678 } 2679 2680 /** 2681 * @return Returns a reference to <code>this</code> for easy method chaining 2682 */ 2683 public ImagingStudy setBasedOn(List<Reference> theBasedOn) { 2684 this.basedOn = theBasedOn; 2685 return this; 2686 } 2687 2688 public boolean hasBasedOn() { 2689 if (this.basedOn == null) 2690 return false; 2691 for (Reference item : this.basedOn) 2692 if (!item.isEmpty()) 2693 return true; 2694 return false; 2695 } 2696 2697 public Reference addBasedOn() { // 3 2698 Reference t = new Reference(); 2699 if (this.basedOn == null) 2700 this.basedOn = new ArrayList<Reference>(); 2701 this.basedOn.add(t); 2702 return t; 2703 } 2704 2705 public ImagingStudy addBasedOn(Reference t) { // 3 2706 if (t == null) 2707 return this; 2708 if (this.basedOn == null) 2709 this.basedOn = new ArrayList<Reference>(); 2710 this.basedOn.add(t); 2711 return this; 2712 } 2713 2714 /** 2715 * @return The first repetition of repeating field {@link #basedOn}, creating it 2716 * if it does not already exist 2717 */ 2718 public Reference getBasedOnFirstRep() { 2719 if (getBasedOn().isEmpty()) { 2720 addBasedOn(); 2721 } 2722 return getBasedOn().get(0); 2723 } 2724 2725 /** 2726 * @deprecated Use Reference#setResource(IBaseResource) instead 2727 */ 2728 @Deprecated 2729 public List<Resource> getBasedOnTarget() { 2730 if (this.basedOnTarget == null) 2731 this.basedOnTarget = new ArrayList<Resource>(); 2732 return this.basedOnTarget; 2733 } 2734 2735 /** 2736 * @return {@link #referrer} (The requesting/referring physician.) 2737 */ 2738 public Reference getReferrer() { 2739 if (this.referrer == null) 2740 if (Configuration.errorOnAutoCreate()) 2741 throw new Error("Attempt to auto-create ImagingStudy.referrer"); 2742 else if (Configuration.doAutoCreate()) 2743 this.referrer = new Reference(); // cc 2744 return this.referrer; 2745 } 2746 2747 public boolean hasReferrer() { 2748 return this.referrer != null && !this.referrer.isEmpty(); 2749 } 2750 2751 /** 2752 * @param value {@link #referrer} (The requesting/referring physician.) 2753 */ 2754 public ImagingStudy setReferrer(Reference value) { 2755 this.referrer = value; 2756 return this; 2757 } 2758 2759 /** 2760 * @return {@link #referrer} The actual object that is the target of the 2761 * reference. The reference library doesn't populate this, but you can 2762 * use it to hold the resource if you resolve it. (The 2763 * requesting/referring physician.) 2764 */ 2765 public Resource getReferrerTarget() { 2766 return this.referrerTarget; 2767 } 2768 2769 /** 2770 * @param value {@link #referrer} The actual object that is the target of the 2771 * reference. The reference library doesn't use these, but you can 2772 * use it to hold the resource if you resolve it. (The 2773 * requesting/referring physician.) 2774 */ 2775 public ImagingStudy setReferrerTarget(Resource value) { 2776 this.referrerTarget = value; 2777 return this; 2778 } 2779 2780 /** 2781 * @return {@link #interpreter} (Who read the study and interpreted the images 2782 * or other content.) 2783 */ 2784 public List<Reference> getInterpreter() { 2785 if (this.interpreter == null) 2786 this.interpreter = new ArrayList<Reference>(); 2787 return this.interpreter; 2788 } 2789 2790 /** 2791 * @return Returns a reference to <code>this</code> for easy method chaining 2792 */ 2793 public ImagingStudy setInterpreter(List<Reference> theInterpreter) { 2794 this.interpreter = theInterpreter; 2795 return this; 2796 } 2797 2798 public boolean hasInterpreter() { 2799 if (this.interpreter == null) 2800 return false; 2801 for (Reference item : this.interpreter) 2802 if (!item.isEmpty()) 2803 return true; 2804 return false; 2805 } 2806 2807 public Reference addInterpreter() { // 3 2808 Reference t = new Reference(); 2809 if (this.interpreter == null) 2810 this.interpreter = new ArrayList<Reference>(); 2811 this.interpreter.add(t); 2812 return t; 2813 } 2814 2815 public ImagingStudy addInterpreter(Reference t) { // 3 2816 if (t == null) 2817 return this; 2818 if (this.interpreter == null) 2819 this.interpreter = new ArrayList<Reference>(); 2820 this.interpreter.add(t); 2821 return this; 2822 } 2823 2824 /** 2825 * @return The first repetition of repeating field {@link #interpreter}, 2826 * creating it if it does not already exist 2827 */ 2828 public Reference getInterpreterFirstRep() { 2829 if (getInterpreter().isEmpty()) { 2830 addInterpreter(); 2831 } 2832 return getInterpreter().get(0); 2833 } 2834 2835 /** 2836 * @deprecated Use Reference#setResource(IBaseResource) instead 2837 */ 2838 @Deprecated 2839 public List<Resource> getInterpreterTarget() { 2840 if (this.interpreterTarget == null) 2841 this.interpreterTarget = new ArrayList<Resource>(); 2842 return this.interpreterTarget; 2843 } 2844 2845 /** 2846 * @return {@link #endpoint} (The network service providing access (e.g., query, 2847 * view, or retrieval) for the study. See implementation notes for 2848 * information about using DICOM endpoints. A study-level endpoint 2849 * applies to each series in the study, unless overridden by a 2850 * series-level endpoint with the same Endpoint.connectionType.) 2851 */ 2852 public List<Reference> getEndpoint() { 2853 if (this.endpoint == null) 2854 this.endpoint = new ArrayList<Reference>(); 2855 return this.endpoint; 2856 } 2857 2858 /** 2859 * @return Returns a reference to <code>this</code> for easy method chaining 2860 */ 2861 public ImagingStudy setEndpoint(List<Reference> theEndpoint) { 2862 this.endpoint = theEndpoint; 2863 return this; 2864 } 2865 2866 public boolean hasEndpoint() { 2867 if (this.endpoint == null) 2868 return false; 2869 for (Reference item : this.endpoint) 2870 if (!item.isEmpty()) 2871 return true; 2872 return false; 2873 } 2874 2875 public Reference addEndpoint() { // 3 2876 Reference t = new Reference(); 2877 if (this.endpoint == null) 2878 this.endpoint = new ArrayList<Reference>(); 2879 this.endpoint.add(t); 2880 return t; 2881 } 2882 2883 public ImagingStudy addEndpoint(Reference t) { // 3 2884 if (t == null) 2885 return this; 2886 if (this.endpoint == null) 2887 this.endpoint = new ArrayList<Reference>(); 2888 this.endpoint.add(t); 2889 return this; 2890 } 2891 2892 /** 2893 * @return The first repetition of repeating field {@link #endpoint}, creating 2894 * it if it does not already exist 2895 */ 2896 public Reference getEndpointFirstRep() { 2897 if (getEndpoint().isEmpty()) { 2898 addEndpoint(); 2899 } 2900 return getEndpoint().get(0); 2901 } 2902 2903 /** 2904 * @deprecated Use Reference#setResource(IBaseResource) instead 2905 */ 2906 @Deprecated 2907 public List<Endpoint> getEndpointTarget() { 2908 if (this.endpointTarget == null) 2909 this.endpointTarget = new ArrayList<Endpoint>(); 2910 return this.endpointTarget; 2911 } 2912 2913 /** 2914 * @deprecated Use Reference#setResource(IBaseResource) instead 2915 */ 2916 @Deprecated 2917 public Endpoint addEndpointTarget() { 2918 Endpoint r = new Endpoint(); 2919 if (this.endpointTarget == null) 2920 this.endpointTarget = new ArrayList<Endpoint>(); 2921 this.endpointTarget.add(r); 2922 return r; 2923 } 2924 2925 /** 2926 * @return {@link #numberOfSeries} (Number of Series in the Study. This value 2927 * given may be larger than the number of series elements this Resource 2928 * contains due to resource availability, security, or other factors. 2929 * This element should be present if any series elements are present.). 2930 * This is the underlying object with id, value and extensions. The 2931 * accessor "getNumberOfSeries" gives direct access to the value 2932 */ 2933 public UnsignedIntType getNumberOfSeriesElement() { 2934 if (this.numberOfSeries == null) 2935 if (Configuration.errorOnAutoCreate()) 2936 throw new Error("Attempt to auto-create ImagingStudy.numberOfSeries"); 2937 else if (Configuration.doAutoCreate()) 2938 this.numberOfSeries = new UnsignedIntType(); // bb 2939 return this.numberOfSeries; 2940 } 2941 2942 public boolean hasNumberOfSeriesElement() { 2943 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2944 } 2945 2946 public boolean hasNumberOfSeries() { 2947 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2948 } 2949 2950 /** 2951 * @param value {@link #numberOfSeries} (Number of Series in the Study. This 2952 * value given may be larger than the number of series elements 2953 * this Resource contains due to resource availability, security, 2954 * or other factors. This element should be present if any series 2955 * elements are present.). This is the underlying object with id, 2956 * value and extensions. The accessor "getNumberOfSeries" gives 2957 * direct access to the value 2958 */ 2959 public ImagingStudy setNumberOfSeriesElement(UnsignedIntType value) { 2960 this.numberOfSeries = value; 2961 return this; 2962 } 2963 2964 /** 2965 * @return Number of Series in the Study. This value given may be larger than 2966 * the number of series elements this Resource contains due to resource 2967 * availability, security, or other factors. This element should be 2968 * present if any series elements are present. 2969 */ 2970 public int getNumberOfSeries() { 2971 return this.numberOfSeries == null || this.numberOfSeries.isEmpty() ? 0 : this.numberOfSeries.getValue(); 2972 } 2973 2974 /** 2975 * @param value Number of Series in the Study. This value given may be larger 2976 * than the number of series elements this Resource contains due to 2977 * resource availability, security, or other factors. This element 2978 * should be present if any series elements are present. 2979 */ 2980 public ImagingStudy setNumberOfSeries(int value) { 2981 if (this.numberOfSeries == null) 2982 this.numberOfSeries = new UnsignedIntType(); 2983 this.numberOfSeries.setValue(value); 2984 return this; 2985 } 2986 2987 /** 2988 * @return {@link #numberOfInstances} (Number of SOP Instances in Study. This 2989 * value given may be larger than the number of instance elements this 2990 * resource contains due to resource availability, security, or other 2991 * factors. This element should be present if any instance elements are 2992 * present.). This is the underlying object with id, value and 2993 * extensions. The accessor "getNumberOfInstances" gives direct access 2994 * to the value 2995 */ 2996 public UnsignedIntType getNumberOfInstancesElement() { 2997 if (this.numberOfInstances == null) 2998 if (Configuration.errorOnAutoCreate()) 2999 throw new Error("Attempt to auto-create ImagingStudy.numberOfInstances"); 3000 else if (Configuration.doAutoCreate()) 3001 this.numberOfInstances = new UnsignedIntType(); // bb 3002 return this.numberOfInstances; 3003 } 3004 3005 public boolean hasNumberOfInstancesElement() { 3006 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 3007 } 3008 3009 public boolean hasNumberOfInstances() { 3010 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 3011 } 3012 3013 /** 3014 * @param value {@link #numberOfInstances} (Number of SOP Instances in Study. 3015 * This value given may be larger than the number of instance 3016 * elements this resource contains due to resource availability, 3017 * security, or other factors. This element should be present if 3018 * any instance elements are present.). This is the underlying 3019 * object with id, value and extensions. The accessor 3020 * "getNumberOfInstances" gives direct access to the value 3021 */ 3022 public ImagingStudy setNumberOfInstancesElement(UnsignedIntType value) { 3023 this.numberOfInstances = value; 3024 return this; 3025 } 3026 3027 /** 3028 * @return Number of SOP Instances in Study. This value given may be larger than 3029 * the number of instance elements this resource contains due to 3030 * resource availability, security, or other factors. This element 3031 * should be present if any instance elements are present. 3032 */ 3033 public int getNumberOfInstances() { 3034 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 3035 } 3036 3037 /** 3038 * @param value Number of SOP Instances in Study. This value given may be larger 3039 * than the number of instance elements this resource contains due 3040 * to resource availability, security, or other factors. This 3041 * element should be present if any instance elements are present. 3042 */ 3043 public ImagingStudy setNumberOfInstances(int value) { 3044 if (this.numberOfInstances == null) 3045 this.numberOfInstances = new UnsignedIntType(); 3046 this.numberOfInstances.setValue(value); 3047 return this; 3048 } 3049 3050 /** 3051 * @return {@link #procedureReference} (The procedure which this ImagingStudy 3052 * was part of.) 3053 */ 3054 public Reference getProcedureReference() { 3055 if (this.procedureReference == null) 3056 if (Configuration.errorOnAutoCreate()) 3057 throw new Error("Attempt to auto-create ImagingStudy.procedureReference"); 3058 else if (Configuration.doAutoCreate()) 3059 this.procedureReference = new Reference(); // cc 3060 return this.procedureReference; 3061 } 3062 3063 public boolean hasProcedureReference() { 3064 return this.procedureReference != null && !this.procedureReference.isEmpty(); 3065 } 3066 3067 /** 3068 * @param value {@link #procedureReference} (The procedure which this 3069 * ImagingStudy was part of.) 3070 */ 3071 public ImagingStudy setProcedureReference(Reference value) { 3072 this.procedureReference = value; 3073 return this; 3074 } 3075 3076 /** 3077 * @return {@link #procedureReference} The actual object that is the target of 3078 * the reference. The reference library doesn't populate this, but you 3079 * can use it to hold the resource if you resolve it. (The procedure 3080 * which this ImagingStudy was part of.) 3081 */ 3082 public Procedure getProcedureReferenceTarget() { 3083 if (this.procedureReferenceTarget == null) 3084 if (Configuration.errorOnAutoCreate()) 3085 throw new Error("Attempt to auto-create ImagingStudy.procedureReference"); 3086 else if (Configuration.doAutoCreate()) 3087 this.procedureReferenceTarget = new Procedure(); // aa 3088 return this.procedureReferenceTarget; 3089 } 3090 3091 /** 3092 * @param value {@link #procedureReference} The actual object that is the target 3093 * of the reference. The reference library doesn't use these, but 3094 * you can use it to hold the resource if you resolve it. (The 3095 * procedure which this ImagingStudy was part of.) 3096 */ 3097 public ImagingStudy setProcedureReferenceTarget(Procedure value) { 3098 this.procedureReferenceTarget = value; 3099 return this; 3100 } 3101 3102 /** 3103 * @return {@link #procedureCode} (The code for the performed procedure type.) 3104 */ 3105 public List<CodeableConcept> getProcedureCode() { 3106 if (this.procedureCode == null) 3107 this.procedureCode = new ArrayList<CodeableConcept>(); 3108 return this.procedureCode; 3109 } 3110 3111 /** 3112 * @return Returns a reference to <code>this</code> for easy method chaining 3113 */ 3114 public ImagingStudy setProcedureCode(List<CodeableConcept> theProcedureCode) { 3115 this.procedureCode = theProcedureCode; 3116 return this; 3117 } 3118 3119 public boolean hasProcedureCode() { 3120 if (this.procedureCode == null) 3121 return false; 3122 for (CodeableConcept item : this.procedureCode) 3123 if (!item.isEmpty()) 3124 return true; 3125 return false; 3126 } 3127 3128 public CodeableConcept addProcedureCode() { // 3 3129 CodeableConcept t = new CodeableConcept(); 3130 if (this.procedureCode == null) 3131 this.procedureCode = new ArrayList<CodeableConcept>(); 3132 this.procedureCode.add(t); 3133 return t; 3134 } 3135 3136 public ImagingStudy addProcedureCode(CodeableConcept t) { // 3 3137 if (t == null) 3138 return this; 3139 if (this.procedureCode == null) 3140 this.procedureCode = new ArrayList<CodeableConcept>(); 3141 this.procedureCode.add(t); 3142 return this; 3143 } 3144 3145 /** 3146 * @return The first repetition of repeating field {@link #procedureCode}, 3147 * creating it if it does not already exist 3148 */ 3149 public CodeableConcept getProcedureCodeFirstRep() { 3150 if (getProcedureCode().isEmpty()) { 3151 addProcedureCode(); 3152 } 3153 return getProcedureCode().get(0); 3154 } 3155 3156 /** 3157 * @return {@link #location} (The principal physical location where the 3158 * ImagingStudy was performed.) 3159 */ 3160 public Reference getLocation() { 3161 if (this.location == null) 3162 if (Configuration.errorOnAutoCreate()) 3163 throw new Error("Attempt to auto-create ImagingStudy.location"); 3164 else if (Configuration.doAutoCreate()) 3165 this.location = new Reference(); // cc 3166 return this.location; 3167 } 3168 3169 public boolean hasLocation() { 3170 return this.location != null && !this.location.isEmpty(); 3171 } 3172 3173 /** 3174 * @param value {@link #location} (The principal physical location where the 3175 * ImagingStudy was performed.) 3176 */ 3177 public ImagingStudy setLocation(Reference value) { 3178 this.location = value; 3179 return this; 3180 } 3181 3182 /** 3183 * @return {@link #location} The actual object that is the target of the 3184 * reference. The reference library doesn't populate this, but you can 3185 * use it to hold the resource if you resolve it. (The principal 3186 * physical location where the ImagingStudy was performed.) 3187 */ 3188 public Location getLocationTarget() { 3189 if (this.locationTarget == null) 3190 if (Configuration.errorOnAutoCreate()) 3191 throw new Error("Attempt to auto-create ImagingStudy.location"); 3192 else if (Configuration.doAutoCreate()) 3193 this.locationTarget = new Location(); // aa 3194 return this.locationTarget; 3195 } 3196 3197 /** 3198 * @param value {@link #location} The actual object that is the target of the 3199 * reference. The reference library doesn't use these, but you can 3200 * use it to hold the resource if you resolve it. (The principal 3201 * physical location where the ImagingStudy was performed.) 3202 */ 3203 public ImagingStudy setLocationTarget(Location value) { 3204 this.locationTarget = value; 3205 return this; 3206 } 3207 3208 /** 3209 * @return {@link #reasonCode} (Description of clinical condition indicating why 3210 * the ImagingStudy was requested.) 3211 */ 3212 public List<CodeableConcept> getReasonCode() { 3213 if (this.reasonCode == null) 3214 this.reasonCode = new ArrayList<CodeableConcept>(); 3215 return this.reasonCode; 3216 } 3217 3218 /** 3219 * @return Returns a reference to <code>this</code> for easy method chaining 3220 */ 3221 public ImagingStudy setReasonCode(List<CodeableConcept> theReasonCode) { 3222 this.reasonCode = theReasonCode; 3223 return this; 3224 } 3225 3226 public boolean hasReasonCode() { 3227 if (this.reasonCode == null) 3228 return false; 3229 for (CodeableConcept item : this.reasonCode) 3230 if (!item.isEmpty()) 3231 return true; 3232 return false; 3233 } 3234 3235 public CodeableConcept addReasonCode() { // 3 3236 CodeableConcept t = new CodeableConcept(); 3237 if (this.reasonCode == null) 3238 this.reasonCode = new ArrayList<CodeableConcept>(); 3239 this.reasonCode.add(t); 3240 return t; 3241 } 3242 3243 public ImagingStudy addReasonCode(CodeableConcept t) { // 3 3244 if (t == null) 3245 return this; 3246 if (this.reasonCode == null) 3247 this.reasonCode = new ArrayList<CodeableConcept>(); 3248 this.reasonCode.add(t); 3249 return this; 3250 } 3251 3252 /** 3253 * @return The first repetition of repeating field {@link #reasonCode}, creating 3254 * it if it does not already exist 3255 */ 3256 public CodeableConcept getReasonCodeFirstRep() { 3257 if (getReasonCode().isEmpty()) { 3258 addReasonCode(); 3259 } 3260 return getReasonCode().get(0); 3261 } 3262 3263 /** 3264 * @return {@link #reasonReference} (Indicates another resource whose existence 3265 * justifies this Study.) 3266 */ 3267 public List<Reference> getReasonReference() { 3268 if (this.reasonReference == null) 3269 this.reasonReference = new ArrayList<Reference>(); 3270 return this.reasonReference; 3271 } 3272 3273 /** 3274 * @return Returns a reference to <code>this</code> for easy method chaining 3275 */ 3276 public ImagingStudy setReasonReference(List<Reference> theReasonReference) { 3277 this.reasonReference = theReasonReference; 3278 return this; 3279 } 3280 3281 public boolean hasReasonReference() { 3282 if (this.reasonReference == null) 3283 return false; 3284 for (Reference item : this.reasonReference) 3285 if (!item.isEmpty()) 3286 return true; 3287 return false; 3288 } 3289 3290 public Reference addReasonReference() { // 3 3291 Reference t = new Reference(); 3292 if (this.reasonReference == null) 3293 this.reasonReference = new ArrayList<Reference>(); 3294 this.reasonReference.add(t); 3295 return t; 3296 } 3297 3298 public ImagingStudy addReasonReference(Reference t) { // 3 3299 if (t == null) 3300 return this; 3301 if (this.reasonReference == null) 3302 this.reasonReference = new ArrayList<Reference>(); 3303 this.reasonReference.add(t); 3304 return this; 3305 } 3306 3307 /** 3308 * @return The first repetition of repeating field {@link #reasonReference}, 3309 * creating it if it does not already exist 3310 */ 3311 public Reference getReasonReferenceFirstRep() { 3312 if (getReasonReference().isEmpty()) { 3313 addReasonReference(); 3314 } 3315 return getReasonReference().get(0); 3316 } 3317 3318 /** 3319 * @deprecated Use Reference#setResource(IBaseResource) instead 3320 */ 3321 @Deprecated 3322 public List<Resource> getReasonReferenceTarget() { 3323 if (this.reasonReferenceTarget == null) 3324 this.reasonReferenceTarget = new ArrayList<Resource>(); 3325 return this.reasonReferenceTarget; 3326 } 3327 3328 /** 3329 * @return {@link #note} (Per the recommended DICOM mapping, this element is 3330 * derived from the Study Description attribute (0008,1030). 3331 * Observations or findings about the imaging study should be recorded 3332 * in another resource, e.g. Observation, and not in this element.) 3333 */ 3334 public List<Annotation> getNote() { 3335 if (this.note == null) 3336 this.note = new ArrayList<Annotation>(); 3337 return this.note; 3338 } 3339 3340 /** 3341 * @return Returns a reference to <code>this</code> for easy method chaining 3342 */ 3343 public ImagingStudy setNote(List<Annotation> theNote) { 3344 this.note = theNote; 3345 return this; 3346 } 3347 3348 public boolean hasNote() { 3349 if (this.note == null) 3350 return false; 3351 for (Annotation item : this.note) 3352 if (!item.isEmpty()) 3353 return true; 3354 return false; 3355 } 3356 3357 public Annotation addNote() { // 3 3358 Annotation t = new Annotation(); 3359 if (this.note == null) 3360 this.note = new ArrayList<Annotation>(); 3361 this.note.add(t); 3362 return t; 3363 } 3364 3365 public ImagingStudy addNote(Annotation t) { // 3 3366 if (t == null) 3367 return this; 3368 if (this.note == null) 3369 this.note = new ArrayList<Annotation>(); 3370 this.note.add(t); 3371 return this; 3372 } 3373 3374 /** 3375 * @return The first repetition of repeating field {@link #note}, creating it if 3376 * it does not already exist 3377 */ 3378 public Annotation getNoteFirstRep() { 3379 if (getNote().isEmpty()) { 3380 addNote(); 3381 } 3382 return getNote().get(0); 3383 } 3384 3385 /** 3386 * @return {@link #description} (The Imaging Manager description of the study. 3387 * Institution-generated description or classification of the Study 3388 * (component) performed.). This is the underlying object with id, value 3389 * and extensions. The accessor "getDescription" gives direct access to 3390 * the value 3391 */ 3392 public StringType getDescriptionElement() { 3393 if (this.description == null) 3394 if (Configuration.errorOnAutoCreate()) 3395 throw new Error("Attempt to auto-create ImagingStudy.description"); 3396 else if (Configuration.doAutoCreate()) 3397 this.description = new StringType(); // bb 3398 return this.description; 3399 } 3400 3401 public boolean hasDescriptionElement() { 3402 return this.description != null && !this.description.isEmpty(); 3403 } 3404 3405 public boolean hasDescription() { 3406 return this.description != null && !this.description.isEmpty(); 3407 } 3408 3409 /** 3410 * @param value {@link #description} (The Imaging Manager description of the 3411 * study. Institution-generated description or classification of 3412 * the Study (component) performed.). This is the underlying object 3413 * with id, value and extensions. The accessor "getDescription" 3414 * gives direct access to the value 3415 */ 3416 public ImagingStudy setDescriptionElement(StringType value) { 3417 this.description = value; 3418 return this; 3419 } 3420 3421 /** 3422 * @return The Imaging Manager description of the study. Institution-generated 3423 * description or classification of the Study (component) performed. 3424 */ 3425 public String getDescription() { 3426 return this.description == null ? null : this.description.getValue(); 3427 } 3428 3429 /** 3430 * @param value The Imaging Manager description of the study. 3431 * Institution-generated description or classification of the Study 3432 * (component) performed. 3433 */ 3434 public ImagingStudy setDescription(String value) { 3435 if (Utilities.noString(value)) 3436 this.description = null; 3437 else { 3438 if (this.description == null) 3439 this.description = new StringType(); 3440 this.description.setValue(value); 3441 } 3442 return this; 3443 } 3444 3445 /** 3446 * @return {@link #series} (Each study has one or more series of images or other 3447 * content.) 3448 */ 3449 public List<ImagingStudySeriesComponent> getSeries() { 3450 if (this.series == null) 3451 this.series = new ArrayList<ImagingStudySeriesComponent>(); 3452 return this.series; 3453 } 3454 3455 /** 3456 * @return Returns a reference to <code>this</code> for easy method chaining 3457 */ 3458 public ImagingStudy setSeries(List<ImagingStudySeriesComponent> theSeries) { 3459 this.series = theSeries; 3460 return this; 3461 } 3462 3463 public boolean hasSeries() { 3464 if (this.series == null) 3465 return false; 3466 for (ImagingStudySeriesComponent item : this.series) 3467 if (!item.isEmpty()) 3468 return true; 3469 return false; 3470 } 3471 3472 public ImagingStudySeriesComponent addSeries() { // 3 3473 ImagingStudySeriesComponent t = new ImagingStudySeriesComponent(); 3474 if (this.series == null) 3475 this.series = new ArrayList<ImagingStudySeriesComponent>(); 3476 this.series.add(t); 3477 return t; 3478 } 3479 3480 public ImagingStudy addSeries(ImagingStudySeriesComponent t) { // 3 3481 if (t == null) 3482 return this; 3483 if (this.series == null) 3484 this.series = new ArrayList<ImagingStudySeriesComponent>(); 3485 this.series.add(t); 3486 return this; 3487 } 3488 3489 /** 3490 * @return The first repetition of repeating field {@link #series}, creating it 3491 * if it does not already exist 3492 */ 3493 public ImagingStudySeriesComponent getSeriesFirstRep() { 3494 if (getSeries().isEmpty()) { 3495 addSeries(); 3496 } 3497 return getSeries().get(0); 3498 } 3499 3500 protected void listChildren(List<Property> children) { 3501 super.listChildren(children); 3502 children.add(new Property("identifier", "Identifier", 3503 "Identifiers for the ImagingStudy such as DICOM Study Instance UID, and Accession Number.", 0, 3504 java.lang.Integer.MAX_VALUE, identifier)); 3505 children.add(new Property("status", "code", "The current state of the ImagingStudy.", 0, 1, status)); 3506 children.add(new Property("modality", "Coding", 3507 "A list of all the series.modality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).", 3508 0, java.lang.Integer.MAX_VALUE, modality)); 3509 children.add(new Property("subject", "Reference(Patient|Device|Group)", 3510 "The subject, typically a patient, of the imaging study.", 0, 1, subject)); 3511 children.add(new Property("encounter", "Reference(Encounter)", 3512 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.", 3513 0, 1, encounter)); 3514 children.add(new Property("started", "dateTime", "Date and time the study started.", 0, 1, started)); 3515 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", 3516 "A list of the diagnostic requests that resulted in this imaging study being performed.", 0, 3517 java.lang.Integer.MAX_VALUE, basedOn)); 3518 children.add(new Property("referrer", "Reference(Practitioner|PractitionerRole)", 3519 "The requesting/referring physician.", 0, 1, referrer)); 3520 children.add(new Property("interpreter", "Reference(Practitioner|PractitionerRole)", 3521 "Who read the study and interpreted the images or other content.", 0, java.lang.Integer.MAX_VALUE, 3522 interpreter)); 3523 children.add(new Property("endpoint", "Reference(Endpoint)", 3524 "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType.", 3525 0, java.lang.Integer.MAX_VALUE, endpoint)); 3526 children.add(new Property("numberOfSeries", "unsignedInt", 3527 "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.", 3528 0, 1, numberOfSeries)); 3529 children.add(new Property("numberOfInstances", "unsignedInt", 3530 "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 3531 0, 1, numberOfInstances)); 3532 children.add(new Property("procedureReference", "Reference(Procedure)", 3533 "The procedure which this ImagingStudy was part of.", 0, 1, procedureReference)); 3534 children.add(new Property("procedureCode", "CodeableConcept", "The code for the performed procedure type.", 0, 3535 java.lang.Integer.MAX_VALUE, procedureCode)); 3536 children.add(new Property("location", "Reference(Location)", 3537 "The principal physical location where the ImagingStudy was performed.", 0, 1, location)); 3538 children.add(new Property("reasonCode", "CodeableConcept", 3539 "Description of clinical condition indicating why the ImagingStudy was requested.", 0, 3540 java.lang.Integer.MAX_VALUE, reasonCode)); 3541 children.add( 3542 new Property("reasonReference", "Reference(Condition|Observation|Media|DiagnosticReport|DocumentReference)", 3543 "Indicates another resource whose existence justifies this Study.", 0, java.lang.Integer.MAX_VALUE, 3544 reasonReference)); 3545 children.add(new Property("note", "Annotation", 3546 "Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element.", 3547 0, java.lang.Integer.MAX_VALUE, note)); 3548 children.add(new Property("description", "string", 3549 "The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.", 3550 0, 1, description)); 3551 children.add(new Property("series", "", "Each study has one or more series of images or other content.", 0, 3552 java.lang.Integer.MAX_VALUE, series)); 3553 } 3554 3555 @Override 3556 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3557 switch (_hash) { 3558 case -1618432855: 3559 /* identifier */ return new Property("identifier", "Identifier", 3560 "Identifiers for the ImagingStudy such as DICOM Study Instance UID, and Accession Number.", 0, 3561 java.lang.Integer.MAX_VALUE, identifier); 3562 case -892481550: 3563 /* status */ return new Property("status", "code", "The current state of the ImagingStudy.", 0, 1, status); 3564 case -622722335: 3565 /* modality */ return new Property("modality", "Coding", 3566 "A list of all the series.modality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).", 3567 0, java.lang.Integer.MAX_VALUE, modality); 3568 case -1867885268: 3569 /* subject */ return new Property("subject", "Reference(Patient|Device|Group)", 3570 "The subject, typically a patient, of the imaging study.", 0, 1, subject); 3571 case 1524132147: 3572 /* encounter */ return new Property("encounter", "Reference(Encounter)", 3573 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.", 3574 0, 1, encounter); 3575 case -1897185151: 3576 /* started */ return new Property("started", "dateTime", "Date and time the study started.", 0, 1, started); 3577 case -332612366: 3578 /* basedOn */ return new Property("basedOn", 3579 "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", 3580 "A list of the diagnostic requests that resulted in this imaging study being performed.", 0, 3581 java.lang.Integer.MAX_VALUE, basedOn); 3582 case -722568161: 3583 /* referrer */ return new Property("referrer", "Reference(Practitioner|PractitionerRole)", 3584 "The requesting/referring physician.", 0, 1, referrer); 3585 case -2008009094: 3586 /* interpreter */ return new Property("interpreter", "Reference(Practitioner|PractitionerRole)", 3587 "Who read the study and interpreted the images or other content.", 0, java.lang.Integer.MAX_VALUE, 3588 interpreter); 3589 case 1741102485: 3590 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 3591 "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType.", 3592 0, java.lang.Integer.MAX_VALUE, endpoint); 3593 case 1920000407: 3594 /* numberOfSeries */ return new Property("numberOfSeries", "unsignedInt", 3595 "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.", 3596 0, 1, numberOfSeries); 3597 case -1043544226: 3598 /* numberOfInstances */ return new Property("numberOfInstances", "unsignedInt", 3599 "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 3600 0, 1, numberOfInstances); 3601 case 881809848: 3602 /* procedureReference */ return new Property("procedureReference", "Reference(Procedure)", 3603 "The procedure which this ImagingStudy was part of.", 0, 1, procedureReference); 3604 case -698023072: 3605 /* procedureCode */ return new Property("procedureCode", "CodeableConcept", 3606 "The code for the performed procedure type.", 0, java.lang.Integer.MAX_VALUE, procedureCode); 3607 case 1901043637: 3608 /* location */ return new Property("location", "Reference(Location)", 3609 "The principal physical location where the ImagingStudy was performed.", 0, 1, location); 3610 case 722137681: 3611 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 3612 "Description of clinical condition indicating why the ImagingStudy was requested.", 0, 3613 java.lang.Integer.MAX_VALUE, reasonCode); 3614 case -1146218137: 3615 /* reasonReference */ return new Property("reasonReference", 3616 "Reference(Condition|Observation|Media|DiagnosticReport|DocumentReference)", 3617 "Indicates another resource whose existence justifies this Study.", 0, java.lang.Integer.MAX_VALUE, 3618 reasonReference); 3619 case 3387378: 3620 /* note */ return new Property("note", "Annotation", 3621 "Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element.", 3622 0, java.lang.Integer.MAX_VALUE, note); 3623 case -1724546052: 3624 /* description */ return new Property("description", "string", 3625 "The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.", 3626 0, 1, description); 3627 case -905838985: 3628 /* series */ return new Property("series", "", "Each study has one or more series of images or other content.", 0, 3629 java.lang.Integer.MAX_VALUE, series); 3630 default: 3631 return super.getNamedProperty(_hash, _name, _checkValid); 3632 } 3633 3634 } 3635 3636 @Override 3637 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3638 switch (hash) { 3639 case -1618432855: 3640 /* identifier */ return this.identifier == null ? new Base[0] 3641 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3642 case -892481550: 3643 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ImagingStudyStatus> 3644 case -622722335: 3645 /* modality */ return this.modality == null ? new Base[0] : this.modality.toArray(new Base[this.modality.size()]); // Coding 3646 case -1867885268: 3647 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 3648 case 1524132147: 3649 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3650 case -1897185151: 3651 /* started */ return this.started == null ? new Base[0] : new Base[] { this.started }; // DateTimeType 3652 case -332612366: 3653 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3654 case -722568161: 3655 /* referrer */ return this.referrer == null ? new Base[0] : new Base[] { this.referrer }; // Reference 3656 case -2008009094: 3657 /* interpreter */ return this.interpreter == null ? new Base[0] 3658 : this.interpreter.toArray(new Base[this.interpreter.size()]); // Reference 3659 case 1741102485: 3660 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 3661 case 1920000407: 3662 /* numberOfSeries */ return this.numberOfSeries == null ? new Base[0] : new Base[] { this.numberOfSeries }; // UnsignedIntType 3663 case -1043544226: 3664 /* numberOfInstances */ return this.numberOfInstances == null ? new Base[0] 3665 : new Base[] { this.numberOfInstances }; // UnsignedIntType 3666 case 881809848: 3667 /* procedureReference */ return this.procedureReference == null ? new Base[0] 3668 : new Base[] { this.procedureReference }; // Reference 3669 case -698023072: 3670 /* procedureCode */ return this.procedureCode == null ? new Base[0] 3671 : this.procedureCode.toArray(new Base[this.procedureCode.size()]); // CodeableConcept 3672 case 1901043637: 3673 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 3674 case 722137681: 3675 /* reasonCode */ return this.reasonCode == null ? new Base[0] 3676 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 3677 case -1146218137: 3678 /* reasonReference */ return this.reasonReference == null ? new Base[0] 3679 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 3680 case 3387378: 3681 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3682 case -1724546052: 3683 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 3684 case -905838985: 3685 /* series */ return this.series == null ? new Base[0] : this.series.toArray(new Base[this.series.size()]); // ImagingStudySeriesComponent 3686 default: 3687 return super.getProperty(hash, name, checkValid); 3688 } 3689 3690 } 3691 3692 @Override 3693 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3694 switch (hash) { 3695 case -1618432855: // identifier 3696 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3697 return value; 3698 case -892481550: // status 3699 value = new ImagingStudyStatusEnumFactory().fromType(castToCode(value)); 3700 this.status = (Enumeration) value; // Enumeration<ImagingStudyStatus> 3701 return value; 3702 case -622722335: // modality 3703 this.getModality().add(castToCoding(value)); // Coding 3704 return value; 3705 case -1867885268: // subject 3706 this.subject = castToReference(value); // Reference 3707 return value; 3708 case 1524132147: // encounter 3709 this.encounter = castToReference(value); // Reference 3710 return value; 3711 case -1897185151: // started 3712 this.started = castToDateTime(value); // DateTimeType 3713 return value; 3714 case -332612366: // basedOn 3715 this.getBasedOn().add(castToReference(value)); // Reference 3716 return value; 3717 case -722568161: // referrer 3718 this.referrer = castToReference(value); // Reference 3719 return value; 3720 case -2008009094: // interpreter 3721 this.getInterpreter().add(castToReference(value)); // Reference 3722 return value; 3723 case 1741102485: // endpoint 3724 this.getEndpoint().add(castToReference(value)); // Reference 3725 return value; 3726 case 1920000407: // numberOfSeries 3727 this.numberOfSeries = castToUnsignedInt(value); // UnsignedIntType 3728 return value; 3729 case -1043544226: // numberOfInstances 3730 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 3731 return value; 3732 case 881809848: // procedureReference 3733 this.procedureReference = castToReference(value); // Reference 3734 return value; 3735 case -698023072: // procedureCode 3736 this.getProcedureCode().add(castToCodeableConcept(value)); // CodeableConcept 3737 return value; 3738 case 1901043637: // location 3739 this.location = castToReference(value); // Reference 3740 return value; 3741 case 722137681: // reasonCode 3742 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 3743 return value; 3744 case -1146218137: // reasonReference 3745 this.getReasonReference().add(castToReference(value)); // Reference 3746 return value; 3747 case 3387378: // note 3748 this.getNote().add(castToAnnotation(value)); // Annotation 3749 return value; 3750 case -1724546052: // description 3751 this.description = castToString(value); // StringType 3752 return value; 3753 case -905838985: // series 3754 this.getSeries().add((ImagingStudySeriesComponent) value); // ImagingStudySeriesComponent 3755 return value; 3756 default: 3757 return super.setProperty(hash, name, value); 3758 } 3759 3760 } 3761 3762 @Override 3763 public Base setProperty(String name, Base value) throws FHIRException { 3764 if (name.equals("identifier")) { 3765 this.getIdentifier().add(castToIdentifier(value)); 3766 } else if (name.equals("status")) { 3767 value = new ImagingStudyStatusEnumFactory().fromType(castToCode(value)); 3768 this.status = (Enumeration) value; // Enumeration<ImagingStudyStatus> 3769 } else if (name.equals("modality")) { 3770 this.getModality().add(castToCoding(value)); 3771 } else if (name.equals("subject")) { 3772 this.subject = castToReference(value); // Reference 3773 } else if (name.equals("encounter")) { 3774 this.encounter = castToReference(value); // Reference 3775 } else if (name.equals("started")) { 3776 this.started = castToDateTime(value); // DateTimeType 3777 } else if (name.equals("basedOn")) { 3778 this.getBasedOn().add(castToReference(value)); 3779 } else if (name.equals("referrer")) { 3780 this.referrer = castToReference(value); // Reference 3781 } else if (name.equals("interpreter")) { 3782 this.getInterpreter().add(castToReference(value)); 3783 } else if (name.equals("endpoint")) { 3784 this.getEndpoint().add(castToReference(value)); 3785 } else if (name.equals("numberOfSeries")) { 3786 this.numberOfSeries = castToUnsignedInt(value); // UnsignedIntType 3787 } else if (name.equals("numberOfInstances")) { 3788 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 3789 } else if (name.equals("procedureReference")) { 3790 this.procedureReference = castToReference(value); // Reference 3791 } else if (name.equals("procedureCode")) { 3792 this.getProcedureCode().add(castToCodeableConcept(value)); 3793 } else if (name.equals("location")) { 3794 this.location = castToReference(value); // Reference 3795 } else if (name.equals("reasonCode")) { 3796 this.getReasonCode().add(castToCodeableConcept(value)); 3797 } else if (name.equals("reasonReference")) { 3798 this.getReasonReference().add(castToReference(value)); 3799 } else if (name.equals("note")) { 3800 this.getNote().add(castToAnnotation(value)); 3801 } else if (name.equals("description")) { 3802 this.description = castToString(value); // StringType 3803 } else if (name.equals("series")) { 3804 this.getSeries().add((ImagingStudySeriesComponent) value); 3805 } else 3806 return super.setProperty(name, value); 3807 return value; 3808 } 3809 3810 @Override 3811 public void removeChild(String name, Base value) throws FHIRException { 3812 if (name.equals("identifier")) { 3813 this.getIdentifier().remove(castToIdentifier(value)); 3814 } else if (name.equals("status")) { 3815 this.status = null; 3816 } else if (name.equals("modality")) { 3817 this.getModality().remove(castToCoding(value)); 3818 } else if (name.equals("subject")) { 3819 this.subject = null; 3820 } else if (name.equals("encounter")) { 3821 this.encounter = null; 3822 } else if (name.equals("started")) { 3823 this.started = null; 3824 } else if (name.equals("basedOn")) { 3825 this.getBasedOn().remove(castToReference(value)); 3826 } else if (name.equals("referrer")) { 3827 this.referrer = null; 3828 } else if (name.equals("interpreter")) { 3829 this.getInterpreter().remove(castToReference(value)); 3830 } else if (name.equals("endpoint")) { 3831 this.getEndpoint().remove(castToReference(value)); 3832 } else if (name.equals("numberOfSeries")) { 3833 this.numberOfSeries = null; 3834 } else if (name.equals("numberOfInstances")) { 3835 this.numberOfInstances = null; 3836 } else if (name.equals("procedureReference")) { 3837 this.procedureReference = null; 3838 } else if (name.equals("procedureCode")) { 3839 this.getProcedureCode().remove(castToCodeableConcept(value)); 3840 } else if (name.equals("location")) { 3841 this.location = null; 3842 } else if (name.equals("reasonCode")) { 3843 this.getReasonCode().remove(castToCodeableConcept(value)); 3844 } else if (name.equals("reasonReference")) { 3845 this.getReasonReference().remove(castToReference(value)); 3846 } else if (name.equals("note")) { 3847 this.getNote().remove(castToAnnotation(value)); 3848 } else if (name.equals("description")) { 3849 this.description = null; 3850 } else if (name.equals("series")) { 3851 this.getSeries().remove((ImagingStudySeriesComponent) value); 3852 } else 3853 super.removeChild(name, value); 3854 3855 } 3856 3857 @Override 3858 public Base makeProperty(int hash, String name) throws FHIRException { 3859 switch (hash) { 3860 case -1618432855: 3861 return addIdentifier(); 3862 case -892481550: 3863 return getStatusElement(); 3864 case -622722335: 3865 return addModality(); 3866 case -1867885268: 3867 return getSubject(); 3868 case 1524132147: 3869 return getEncounter(); 3870 case -1897185151: 3871 return getStartedElement(); 3872 case -332612366: 3873 return addBasedOn(); 3874 case -722568161: 3875 return getReferrer(); 3876 case -2008009094: 3877 return addInterpreter(); 3878 case 1741102485: 3879 return addEndpoint(); 3880 case 1920000407: 3881 return getNumberOfSeriesElement(); 3882 case -1043544226: 3883 return getNumberOfInstancesElement(); 3884 case 881809848: 3885 return getProcedureReference(); 3886 case -698023072: 3887 return addProcedureCode(); 3888 case 1901043637: 3889 return getLocation(); 3890 case 722137681: 3891 return addReasonCode(); 3892 case -1146218137: 3893 return addReasonReference(); 3894 case 3387378: 3895 return addNote(); 3896 case -1724546052: 3897 return getDescriptionElement(); 3898 case -905838985: 3899 return addSeries(); 3900 default: 3901 return super.makeProperty(hash, name); 3902 } 3903 3904 } 3905 3906 @Override 3907 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3908 switch (hash) { 3909 case -1618432855: 3910 /* identifier */ return new String[] { "Identifier" }; 3911 case -892481550: 3912 /* status */ return new String[] { "code" }; 3913 case -622722335: 3914 /* modality */ return new String[] { "Coding" }; 3915 case -1867885268: 3916 /* subject */ return new String[] { "Reference" }; 3917 case 1524132147: 3918 /* encounter */ return new String[] { "Reference" }; 3919 case -1897185151: 3920 /* started */ return new String[] { "dateTime" }; 3921 case -332612366: 3922 /* basedOn */ return new String[] { "Reference" }; 3923 case -722568161: 3924 /* referrer */ return new String[] { "Reference" }; 3925 case -2008009094: 3926 /* interpreter */ return new String[] { "Reference" }; 3927 case 1741102485: 3928 /* endpoint */ return new String[] { "Reference" }; 3929 case 1920000407: 3930 /* numberOfSeries */ return new String[] { "unsignedInt" }; 3931 case -1043544226: 3932 /* numberOfInstances */ return new String[] { "unsignedInt" }; 3933 case 881809848: 3934 /* procedureReference */ return new String[] { "Reference" }; 3935 case -698023072: 3936 /* procedureCode */ return new String[] { "CodeableConcept" }; 3937 case 1901043637: 3938 /* location */ return new String[] { "Reference" }; 3939 case 722137681: 3940 /* reasonCode */ return new String[] { "CodeableConcept" }; 3941 case -1146218137: 3942 /* reasonReference */ return new String[] { "Reference" }; 3943 case 3387378: 3944 /* note */ return new String[] { "Annotation" }; 3945 case -1724546052: 3946 /* description */ return new String[] { "string" }; 3947 case -905838985: 3948 /* series */ return new String[] {}; 3949 default: 3950 return super.getTypesForProperty(hash, name); 3951 } 3952 3953 } 3954 3955 @Override 3956 public Base addChild(String name) throws FHIRException { 3957 if (name.equals("identifier")) { 3958 return addIdentifier(); 3959 } else if (name.equals("status")) { 3960 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.status"); 3961 } else if (name.equals("modality")) { 3962 return addModality(); 3963 } else if (name.equals("subject")) { 3964 this.subject = new Reference(); 3965 return this.subject; 3966 } else if (name.equals("encounter")) { 3967 this.encounter = new Reference(); 3968 return this.encounter; 3969 } else if (name.equals("started")) { 3970 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 3971 } else if (name.equals("basedOn")) { 3972 return addBasedOn(); 3973 } else if (name.equals("referrer")) { 3974 this.referrer = new Reference(); 3975 return this.referrer; 3976 } else if (name.equals("interpreter")) { 3977 return addInterpreter(); 3978 } else if (name.equals("endpoint")) { 3979 return addEndpoint(); 3980 } else if (name.equals("numberOfSeries")) { 3981 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfSeries"); 3982 } else if (name.equals("numberOfInstances")) { 3983 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 3984 } else if (name.equals("procedureReference")) { 3985 this.procedureReference = new Reference(); 3986 return this.procedureReference; 3987 } else if (name.equals("procedureCode")) { 3988 return addProcedureCode(); 3989 } else if (name.equals("location")) { 3990 this.location = new Reference(); 3991 return this.location; 3992 } else if (name.equals("reasonCode")) { 3993 return addReasonCode(); 3994 } else if (name.equals("reasonReference")) { 3995 return addReasonReference(); 3996 } else if (name.equals("note")) { 3997 return addNote(); 3998 } else if (name.equals("description")) { 3999 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 4000 } else if (name.equals("series")) { 4001 return addSeries(); 4002 } else 4003 return super.addChild(name); 4004 } 4005 4006 public String fhirType() { 4007 return "ImagingStudy"; 4008 4009 } 4010 4011 public ImagingStudy copy() { 4012 ImagingStudy dst = new ImagingStudy(); 4013 copyValues(dst); 4014 return dst; 4015 } 4016 4017 public void copyValues(ImagingStudy dst) { 4018 super.copyValues(dst); 4019 if (identifier != null) { 4020 dst.identifier = new ArrayList<Identifier>(); 4021 for (Identifier i : identifier) 4022 dst.identifier.add(i.copy()); 4023 } 4024 ; 4025 dst.status = status == null ? null : status.copy(); 4026 if (modality != null) { 4027 dst.modality = new ArrayList<Coding>(); 4028 for (Coding i : modality) 4029 dst.modality.add(i.copy()); 4030 } 4031 ; 4032 dst.subject = subject == null ? null : subject.copy(); 4033 dst.encounter = encounter == null ? null : encounter.copy(); 4034 dst.started = started == null ? null : started.copy(); 4035 if (basedOn != null) { 4036 dst.basedOn = new ArrayList<Reference>(); 4037 for (Reference i : basedOn) 4038 dst.basedOn.add(i.copy()); 4039 } 4040 ; 4041 dst.referrer = referrer == null ? null : referrer.copy(); 4042 if (interpreter != null) { 4043 dst.interpreter = new ArrayList<Reference>(); 4044 for (Reference i : interpreter) 4045 dst.interpreter.add(i.copy()); 4046 } 4047 ; 4048 if (endpoint != null) { 4049 dst.endpoint = new ArrayList<Reference>(); 4050 for (Reference i : endpoint) 4051 dst.endpoint.add(i.copy()); 4052 } 4053 ; 4054 dst.numberOfSeries = numberOfSeries == null ? null : numberOfSeries.copy(); 4055 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 4056 dst.procedureReference = procedureReference == null ? null : procedureReference.copy(); 4057 if (procedureCode != null) { 4058 dst.procedureCode = new ArrayList<CodeableConcept>(); 4059 for (CodeableConcept i : procedureCode) 4060 dst.procedureCode.add(i.copy()); 4061 } 4062 ; 4063 dst.location = location == null ? null : location.copy(); 4064 if (reasonCode != null) { 4065 dst.reasonCode = new ArrayList<CodeableConcept>(); 4066 for (CodeableConcept i : reasonCode) 4067 dst.reasonCode.add(i.copy()); 4068 } 4069 ; 4070 if (reasonReference != null) { 4071 dst.reasonReference = new ArrayList<Reference>(); 4072 for (Reference i : reasonReference) 4073 dst.reasonReference.add(i.copy()); 4074 } 4075 ; 4076 if (note != null) { 4077 dst.note = new ArrayList<Annotation>(); 4078 for (Annotation i : note) 4079 dst.note.add(i.copy()); 4080 } 4081 ; 4082 dst.description = description == null ? null : description.copy(); 4083 if (series != null) { 4084 dst.series = new ArrayList<ImagingStudySeriesComponent>(); 4085 for (ImagingStudySeriesComponent i : series) 4086 dst.series.add(i.copy()); 4087 } 4088 ; 4089 } 4090 4091 protected ImagingStudy typedCopy() { 4092 return copy(); 4093 } 4094 4095 @Override 4096 public boolean equalsDeep(Base other_) { 4097 if (!super.equalsDeep(other_)) 4098 return false; 4099 if (!(other_ instanceof ImagingStudy)) 4100 return false; 4101 ImagingStudy o = (ImagingStudy) other_; 4102 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4103 && compareDeep(modality, o.modality, true) && compareDeep(subject, o.subject, true) 4104 && compareDeep(encounter, o.encounter, true) && compareDeep(started, o.started, true) 4105 && compareDeep(basedOn, o.basedOn, true) && compareDeep(referrer, o.referrer, true) 4106 && compareDeep(interpreter, o.interpreter, true) && compareDeep(endpoint, o.endpoint, true) 4107 && compareDeep(numberOfSeries, o.numberOfSeries, true) 4108 && compareDeep(numberOfInstances, o.numberOfInstances, true) 4109 && compareDeep(procedureReference, o.procedureReference, true) 4110 && compareDeep(procedureCode, o.procedureCode, true) && compareDeep(location, o.location, true) 4111 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 4112 && compareDeep(note, o.note, true) && compareDeep(description, o.description, true) 4113 && compareDeep(series, o.series, true); 4114 } 4115 4116 @Override 4117 public boolean equalsShallow(Base other_) { 4118 if (!super.equalsShallow(other_)) 4119 return false; 4120 if (!(other_ instanceof ImagingStudy)) 4121 return false; 4122 ImagingStudy o = (ImagingStudy) other_; 4123 return compareValues(status, o.status, true) && compareValues(started, o.started, true) 4124 && compareValues(numberOfSeries, o.numberOfSeries, true) 4125 && compareValues(numberOfInstances, o.numberOfInstances, true) 4126 && compareValues(description, o.description, true); 4127 } 4128 4129 public boolean isEmpty() { 4130 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, modality, subject, encounter, 4131 started, basedOn, referrer, interpreter, endpoint, numberOfSeries, numberOfInstances, procedureReference, 4132 procedureCode, location, reasonCode, reasonReference, note, description, series); 4133 } 4134 4135 @Override 4136 public ResourceType getResourceType() { 4137 return ResourceType.ImagingStudy; 4138 } 4139 4140 /** 4141 * Search parameter: <b>identifier</b> 4142 * <p> 4143 * Description: <b>Identifiers for the Study, such as DICOM Study Instance UID 4144 * and Accession number</b><br> 4145 * Type: <b>token</b><br> 4146 * Path: <b>ImagingStudy.identifier</b><br> 4147 * </p> 4148 */ 4149 @SearchParamDefinition(name = "identifier", path = "ImagingStudy.identifier", description = "Identifiers for the Study, such as DICOM Study Instance UID and Accession number", type = "token") 4150 public static final String SP_IDENTIFIER = "identifier"; 4151 /** 4152 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4153 * <p> 4154 * Description: <b>Identifiers for the Study, such as DICOM Study Instance UID 4155 * and Accession number</b><br> 4156 * Type: <b>token</b><br> 4157 * Path: <b>ImagingStudy.identifier</b><br> 4158 * </p> 4159 */ 4160 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4161 SP_IDENTIFIER); 4162 4163 /** 4164 * Search parameter: <b>reason</b> 4165 * <p> 4166 * Description: <b>The reason for the study</b><br> 4167 * Type: <b>token</b><br> 4168 * Path: <b>ImagingStudy.reasonCode</b><br> 4169 * </p> 4170 */ 4171 @SearchParamDefinition(name = "reason", path = "ImagingStudy.reasonCode", description = "The reason for the study", type = "token") 4172 public static final String SP_REASON = "reason"; 4173 /** 4174 * <b>Fluent Client</b> search parameter constant for <b>reason</b> 4175 * <p> 4176 * Description: <b>The reason for the study</b><br> 4177 * Type: <b>token</b><br> 4178 * Path: <b>ImagingStudy.reasonCode</b><br> 4179 * </p> 4180 */ 4181 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4182 SP_REASON); 4183 4184 /** 4185 * Search parameter: <b>dicom-class</b> 4186 * <p> 4187 * Description: <b>The type of the instance</b><br> 4188 * Type: <b>token</b><br> 4189 * Path: <b>ImagingStudy.series.instance.sopClass</b><br> 4190 * </p> 4191 */ 4192 @SearchParamDefinition(name = "dicom-class", path = "ImagingStudy.series.instance.sopClass", description = "The type of the instance", type = "token") 4193 public static final String SP_DICOM_CLASS = "dicom-class"; 4194 /** 4195 * <b>Fluent Client</b> search parameter constant for <b>dicom-class</b> 4196 * <p> 4197 * Description: <b>The type of the instance</b><br> 4198 * Type: <b>token</b><br> 4199 * Path: <b>ImagingStudy.series.instance.sopClass</b><br> 4200 * </p> 4201 */ 4202 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DICOM_CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4203 SP_DICOM_CLASS); 4204 4205 /** 4206 * Search parameter: <b>modality</b> 4207 * <p> 4208 * Description: <b>The modality of the series</b><br> 4209 * Type: <b>token</b><br> 4210 * Path: <b>ImagingStudy.series.modality</b><br> 4211 * </p> 4212 */ 4213 @SearchParamDefinition(name = "modality", path = "ImagingStudy.series.modality", description = "The modality of the series", type = "token") 4214 public static final String SP_MODALITY = "modality"; 4215 /** 4216 * <b>Fluent Client</b> search parameter constant for <b>modality</b> 4217 * <p> 4218 * Description: <b>The modality of the series</b><br> 4219 * Type: <b>token</b><br> 4220 * Path: <b>ImagingStudy.series.modality</b><br> 4221 * </p> 4222 */ 4223 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4224 SP_MODALITY); 4225 4226 /** 4227 * Search parameter: <b>bodysite</b> 4228 * <p> 4229 * Description: <b>The body site studied</b><br> 4230 * Type: <b>token</b><br> 4231 * Path: <b>ImagingStudy.series.bodySite</b><br> 4232 * </p> 4233 */ 4234 @SearchParamDefinition(name = "bodysite", path = "ImagingStudy.series.bodySite", description = "The body site studied", type = "token") 4235 public static final String SP_BODYSITE = "bodysite"; 4236 /** 4237 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 4238 * <p> 4239 * Description: <b>The body site studied</b><br> 4240 * Type: <b>token</b><br> 4241 * Path: <b>ImagingStudy.series.bodySite</b><br> 4242 * </p> 4243 */ 4244 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4245 SP_BODYSITE); 4246 4247 /** 4248 * Search parameter: <b>instance</b> 4249 * <p> 4250 * Description: <b>SOP Instance UID for an instance</b><br> 4251 * Type: <b>token</b><br> 4252 * Path: <b>ImagingStudy.series.instance.uid</b><br> 4253 * </p> 4254 */ 4255 @SearchParamDefinition(name = "instance", path = "ImagingStudy.series.instance.uid", description = "SOP Instance UID for an instance", type = "token") 4256 public static final String SP_INSTANCE = "instance"; 4257 /** 4258 * <b>Fluent Client</b> search parameter constant for <b>instance</b> 4259 * <p> 4260 * Description: <b>SOP Instance UID for an instance</b><br> 4261 * Type: <b>token</b><br> 4262 * Path: <b>ImagingStudy.series.instance.uid</b><br> 4263 * </p> 4264 */ 4265 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INSTANCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4266 SP_INSTANCE); 4267 4268 /** 4269 * Search parameter: <b>performer</b> 4270 * <p> 4271 * Description: <b>The person who performed the study</b><br> 4272 * Type: <b>reference</b><br> 4273 * Path: <b>ImagingStudy.series.performer.actor</b><br> 4274 * </p> 4275 */ 4276 @SearchParamDefinition(name = "performer", path = "ImagingStudy.series.performer.actor", description = "The person who performed the study", type = "reference", target = { 4277 CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 4278 RelatedPerson.class }) 4279 public static final String SP_PERFORMER = "performer"; 4280 /** 4281 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4282 * <p> 4283 * Description: <b>The person who performed the study</b><br> 4284 * Type: <b>reference</b><br> 4285 * Path: <b>ImagingStudy.series.performer.actor</b><br> 4286 * </p> 4287 */ 4288 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4289 SP_PERFORMER); 4290 4291 /** 4292 * Constant for fluent queries to be used to add include statements. Specifies 4293 * the path value of "<b>ImagingStudy:performer</b>". 4294 */ 4295 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 4296 "ImagingStudy:performer").toLocked(); 4297 4298 /** 4299 * Search parameter: <b>subject</b> 4300 * <p> 4301 * Description: <b>Who the study is about</b><br> 4302 * Type: <b>reference</b><br> 4303 * Path: <b>ImagingStudy.subject</b><br> 4304 * </p> 4305 */ 4306 @SearchParamDefinition(name = "subject", path = "ImagingStudy.subject", description = "Who the study is about", type = "reference", target = { 4307 Device.class, Group.class, Patient.class }) 4308 public static final String SP_SUBJECT = "subject"; 4309 /** 4310 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4311 * <p> 4312 * Description: <b>Who the study is about</b><br> 4313 * Type: <b>reference</b><br> 4314 * Path: <b>ImagingStudy.subject</b><br> 4315 * </p> 4316 */ 4317 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4318 SP_SUBJECT); 4319 4320 /** 4321 * Constant for fluent queries to be used to add include statements. Specifies 4322 * the path value of "<b>ImagingStudy:subject</b>". 4323 */ 4324 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 4325 "ImagingStudy:subject").toLocked(); 4326 4327 /** 4328 * Search parameter: <b>started</b> 4329 * <p> 4330 * Description: <b>When the study was started</b><br> 4331 * Type: <b>date</b><br> 4332 * Path: <b>ImagingStudy.started</b><br> 4333 * </p> 4334 */ 4335 @SearchParamDefinition(name = "started", path = "ImagingStudy.started", description = "When the study was started", type = "date") 4336 public static final String SP_STARTED = "started"; 4337 /** 4338 * <b>Fluent Client</b> search parameter constant for <b>started</b> 4339 * <p> 4340 * Description: <b>When the study was started</b><br> 4341 * Type: <b>date</b><br> 4342 * Path: <b>ImagingStudy.started</b><br> 4343 * </p> 4344 */ 4345 public static final ca.uhn.fhir.rest.gclient.DateClientParam STARTED = new ca.uhn.fhir.rest.gclient.DateClientParam( 4346 SP_STARTED); 4347 4348 /** 4349 * Search parameter: <b>interpreter</b> 4350 * <p> 4351 * Description: <b>Who interpreted the images</b><br> 4352 * Type: <b>reference</b><br> 4353 * Path: <b>ImagingStudy.interpreter</b><br> 4354 * </p> 4355 */ 4356 @SearchParamDefinition(name = "interpreter", path = "ImagingStudy.interpreter", description = "Who interpreted the images", type = "reference", target = { 4357 Practitioner.class, PractitionerRole.class }) 4358 public static final String SP_INTERPRETER = "interpreter"; 4359 /** 4360 * <b>Fluent Client</b> search parameter constant for <b>interpreter</b> 4361 * <p> 4362 * Description: <b>Who interpreted the images</b><br> 4363 * Type: <b>reference</b><br> 4364 * Path: <b>ImagingStudy.interpreter</b><br> 4365 * </p> 4366 */ 4367 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTERPRETER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4368 SP_INTERPRETER); 4369 4370 /** 4371 * Constant for fluent queries to be used to add include statements. Specifies 4372 * the path value of "<b>ImagingStudy:interpreter</b>". 4373 */ 4374 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTERPRETER = new ca.uhn.fhir.model.api.Include( 4375 "ImagingStudy:interpreter").toLocked(); 4376 4377 /** 4378 * Search parameter: <b>encounter</b> 4379 * <p> 4380 * Description: <b>The context of the study</b><br> 4381 * Type: <b>reference</b><br> 4382 * Path: <b>ImagingStudy.encounter</b><br> 4383 * </p> 4384 */ 4385 @SearchParamDefinition(name = "encounter", path = "ImagingStudy.encounter", description = "The context of the study", type = "reference", target = { 4386 Encounter.class }) 4387 public static final String SP_ENCOUNTER = "encounter"; 4388 /** 4389 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 4390 * <p> 4391 * Description: <b>The context of the study</b><br> 4392 * Type: <b>reference</b><br> 4393 * Path: <b>ImagingStudy.encounter</b><br> 4394 * </p> 4395 */ 4396 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4397 SP_ENCOUNTER); 4398 4399 /** 4400 * Constant for fluent queries to be used to add include statements. Specifies 4401 * the path value of "<b>ImagingStudy:encounter</b>". 4402 */ 4403 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 4404 "ImagingStudy:encounter").toLocked(); 4405 4406 /** 4407 * Search parameter: <b>referrer</b> 4408 * <p> 4409 * Description: <b>The referring physician</b><br> 4410 * Type: <b>reference</b><br> 4411 * Path: <b>ImagingStudy.referrer</b><br> 4412 * </p> 4413 */ 4414 @SearchParamDefinition(name = "referrer", path = "ImagingStudy.referrer", description = "The referring physician", type = "reference", target = { 4415 Practitioner.class, PractitionerRole.class }) 4416 public static final String SP_REFERRER = "referrer"; 4417 /** 4418 * <b>Fluent Client</b> search parameter constant for <b>referrer</b> 4419 * <p> 4420 * Description: <b>The referring physician</b><br> 4421 * Type: <b>reference</b><br> 4422 * Path: <b>ImagingStudy.referrer</b><br> 4423 * </p> 4424 */ 4425 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REFERRER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4426 SP_REFERRER); 4427 4428 /** 4429 * Constant for fluent queries to be used to add include statements. Specifies 4430 * the path value of "<b>ImagingStudy:referrer</b>". 4431 */ 4432 public static final ca.uhn.fhir.model.api.Include INCLUDE_REFERRER = new ca.uhn.fhir.model.api.Include( 4433 "ImagingStudy:referrer").toLocked(); 4434 4435 /** 4436 * Search parameter: <b>endpoint</b> 4437 * <p> 4438 * Description: <b>The endpoint for the study or series</b><br> 4439 * Type: <b>reference</b><br> 4440 * Path: <b>ImagingStudy.endpoint, ImagingStudy.series.endpoint</b><br> 4441 * </p> 4442 */ 4443 @SearchParamDefinition(name = "endpoint", path = "ImagingStudy.endpoint | ImagingStudy.series.endpoint", description = "The endpoint for the study or series", type = "reference", target = { 4444 Endpoint.class }) 4445 public static final String SP_ENDPOINT = "endpoint"; 4446 /** 4447 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 4448 * <p> 4449 * Description: <b>The endpoint for the study or series</b><br> 4450 * Type: <b>reference</b><br> 4451 * Path: <b>ImagingStudy.endpoint, ImagingStudy.series.endpoint</b><br> 4452 * </p> 4453 */ 4454 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4455 SP_ENDPOINT); 4456 4457 /** 4458 * Constant for fluent queries to be used to add include statements. Specifies 4459 * the path value of "<b>ImagingStudy:endpoint</b>". 4460 */ 4461 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 4462 "ImagingStudy:endpoint").toLocked(); 4463 4464 /** 4465 * Search parameter: <b>patient</b> 4466 * <p> 4467 * Description: <b>Who the study is about</b><br> 4468 * Type: <b>reference</b><br> 4469 * Path: <b>ImagingStudy.subject</b><br> 4470 * </p> 4471 */ 4472 @SearchParamDefinition(name = "patient", path = "ImagingStudy.subject.where(resolve() is Patient)", description = "Who the study is about", type = "reference", providesMembershipIn = { 4473 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4474 public static final String SP_PATIENT = "patient"; 4475 /** 4476 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4477 * <p> 4478 * Description: <b>Who the study is about</b><br> 4479 * Type: <b>reference</b><br> 4480 * Path: <b>ImagingStudy.subject</b><br> 4481 * </p> 4482 */ 4483 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4484 SP_PATIENT); 4485 4486 /** 4487 * Constant for fluent queries to be used to add include statements. Specifies 4488 * the path value of "<b>ImagingStudy:patient</b>". 4489 */ 4490 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4491 "ImagingStudy:patient").toLocked(); 4492 4493 /** 4494 * Search parameter: <b>series</b> 4495 * <p> 4496 * Description: <b>DICOM Series Instance UID for a series</b><br> 4497 * Type: <b>token</b><br> 4498 * Path: <b>ImagingStudy.series.uid</b><br> 4499 * </p> 4500 */ 4501 @SearchParamDefinition(name = "series", path = "ImagingStudy.series.uid", description = "DICOM Series Instance UID for a series", type = "token") 4502 public static final String SP_SERIES = "series"; 4503 /** 4504 * <b>Fluent Client</b> search parameter constant for <b>series</b> 4505 * <p> 4506 * Description: <b>DICOM Series Instance UID for a series</b><br> 4507 * Type: <b>token</b><br> 4508 * Path: <b>ImagingStudy.series.uid</b><br> 4509 * </p> 4510 */ 4511 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIES = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4512 SP_SERIES); 4513 4514 /** 4515 * Search parameter: <b>basedon</b> 4516 * <p> 4517 * Description: <b>The order for the image</b><br> 4518 * Type: <b>reference</b><br> 4519 * Path: <b>ImagingStudy.basedOn</b><br> 4520 * </p> 4521 */ 4522 @SearchParamDefinition(name = "basedon", path = "ImagingStudy.basedOn", description = "The order for the image", type = "reference", target = { 4523 Appointment.class, AppointmentResponse.class, CarePlan.class, ServiceRequest.class, Task.class }) 4524 public static final String SP_BASEDON = "basedon"; 4525 /** 4526 * <b>Fluent Client</b> search parameter constant for <b>basedon</b> 4527 * <p> 4528 * Description: <b>The order for the image</b><br> 4529 * Type: <b>reference</b><br> 4530 * Path: <b>ImagingStudy.basedOn</b><br> 4531 * </p> 4532 */ 4533 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASEDON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4534 SP_BASEDON); 4535 4536 /** 4537 * Constant for fluent queries to be used to add include statements. Specifies 4538 * the path value of "<b>ImagingStudy:basedon</b>". 4539 */ 4540 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASEDON = new ca.uhn.fhir.model.api.Include( 4541 "ImagingStudy:basedon").toLocked(); 4542 4543 /** 4544 * Search parameter: <b>status</b> 4545 * <p> 4546 * Description: <b>The status of the study</b><br> 4547 * Type: <b>token</b><br> 4548 * Path: <b>ImagingStudy.status</b><br> 4549 * </p> 4550 */ 4551 @SearchParamDefinition(name = "status", path = "ImagingStudy.status", description = "The status of the study", type = "token") 4552 public static final String SP_STATUS = "status"; 4553 /** 4554 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4555 * <p> 4556 * Description: <b>The status of the study</b><br> 4557 * Type: <b>token</b><br> 4558 * Path: <b>ImagingStudy.status</b><br> 4559 * </p> 4560 */ 4561 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4562 SP_STATUS); 4563 4564}