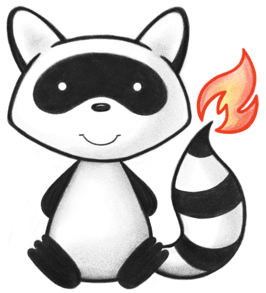
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes the event of a patient being administered a vaccine or a record of 049 * an immunization as reported by a patient, a clinician or another party. 050 */ 051@ResourceDef(name = "Immunization", profile = "http://hl7.org/fhir/StructureDefinition/Immunization") 052public class Immunization extends DomainResource { 053 054 public enum ImmunizationStatus { 055 /** 056 * null 057 */ 058 COMPLETED, 059 /** 060 * null 061 */ 062 ENTEREDINERROR, 063 /** 064 * null 065 */ 066 NOTDONE, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static ImmunizationStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("completed".equals(codeString)) 076 return COMPLETED; 077 if ("entered-in-error".equals(codeString)) 078 return ENTEREDINERROR; 079 if ("not-done".equals(codeString)) 080 return NOTDONE; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown ImmunizationStatus code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case COMPLETED: 090 return "completed"; 091 case ENTEREDINERROR: 092 return "entered-in-error"; 093 case NOTDONE: 094 return "not-done"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 switch (this) { 104 case COMPLETED: 105 return "http://hl7.org/fhir/event-status"; 106 case ENTEREDINERROR: 107 return "http://hl7.org/fhir/event-status"; 108 case NOTDONE: 109 return "http://hl7.org/fhir/event-status"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case COMPLETED: 120 return ""; 121 case ENTEREDINERROR: 122 return ""; 123 case NOTDONE: 124 return ""; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case COMPLETED: 135 return "completed"; 136 case ENTEREDINERROR: 137 return "entered-in-error"; 138 case NOTDONE: 139 return "not-done"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 } 147 148 public static class ImmunizationStatusEnumFactory implements EnumFactory<ImmunizationStatus> { 149 public ImmunizationStatus fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("completed".equals(codeString)) 154 return ImmunizationStatus.COMPLETED; 155 if ("entered-in-error".equals(codeString)) 156 return ImmunizationStatus.ENTEREDINERROR; 157 if ("not-done".equals(codeString)) 158 return ImmunizationStatus.NOTDONE; 159 throw new IllegalArgumentException("Unknown ImmunizationStatus code '" + codeString + "'"); 160 } 161 162 public Enumeration<ImmunizationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.NULL, code); 167 String codeString = code.asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.NULL, code); 170 if ("completed".equals(codeString)) 171 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.COMPLETED, code); 172 if ("entered-in-error".equals(codeString)) 173 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.ENTEREDINERROR, code); 174 if ("not-done".equals(codeString)) 175 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.NOTDONE, code); 176 throw new FHIRException("Unknown ImmunizationStatus code '" + codeString + "'"); 177 } 178 179 public String toCode(ImmunizationStatus code) { 180 if (code == ImmunizationStatus.NULL) 181 return null; 182 if (code == ImmunizationStatus.COMPLETED) 183 return "completed"; 184 if (code == ImmunizationStatus.ENTEREDINERROR) 185 return "entered-in-error"; 186 if (code == ImmunizationStatus.NOTDONE) 187 return "not-done"; 188 return "?"; 189 } 190 191 public String toSystem(ImmunizationStatus code) { 192 return code.getSystem(); 193 } 194 } 195 196 @Block() 197 public static class ImmunizationPerformerComponent extends BackboneElement implements IBaseBackboneElement { 198 /** 199 * Describes the type of performance (e.g. ordering provider, administering 200 * provider, etc.). 201 */ 202 @Child(name = "function", type = { 203 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 204 @Description(shortDefinition = "What type of performance was done", formalDefinition = "Describes the type of performance (e.g. ordering provider, administering provider, etc.).") 205 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-function") 206 protected CodeableConcept function; 207 208 /** 209 * The practitioner or organization who performed the action. 210 */ 211 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, 212 Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 213 @Description(shortDefinition = "Individual or organization who was performing", formalDefinition = "The practitioner or organization who performed the action.") 214 protected Reference actor; 215 216 /** 217 * The actual object that is the target of the reference (The practitioner or 218 * organization who performed the action.) 219 */ 220 protected Resource actorTarget; 221 222 private static final long serialVersionUID = 1424001049L; 223 224 /** 225 * Constructor 226 */ 227 public ImmunizationPerformerComponent() { 228 super(); 229 } 230 231 /** 232 * Constructor 233 */ 234 public ImmunizationPerformerComponent(Reference actor) { 235 super(); 236 this.actor = actor; 237 } 238 239 /** 240 * @return {@link #function} (Describes the type of performance (e.g. ordering 241 * provider, administering provider, etc.).) 242 */ 243 public CodeableConcept getFunction() { 244 if (this.function == null) 245 if (Configuration.errorOnAutoCreate()) 246 throw new Error("Attempt to auto-create ImmunizationPerformerComponent.function"); 247 else if (Configuration.doAutoCreate()) 248 this.function = new CodeableConcept(); // cc 249 return this.function; 250 } 251 252 public boolean hasFunction() { 253 return this.function != null && !this.function.isEmpty(); 254 } 255 256 /** 257 * @param value {@link #function} (Describes the type of performance (e.g. 258 * ordering provider, administering provider, etc.).) 259 */ 260 public ImmunizationPerformerComponent setFunction(CodeableConcept value) { 261 this.function = value; 262 return this; 263 } 264 265 /** 266 * @return {@link #actor} (The practitioner or organization who performed the 267 * action.) 268 */ 269 public Reference getActor() { 270 if (this.actor == null) 271 if (Configuration.errorOnAutoCreate()) 272 throw new Error("Attempt to auto-create ImmunizationPerformerComponent.actor"); 273 else if (Configuration.doAutoCreate()) 274 this.actor = new Reference(); // cc 275 return this.actor; 276 } 277 278 public boolean hasActor() { 279 return this.actor != null && !this.actor.isEmpty(); 280 } 281 282 /** 283 * @param value {@link #actor} (The practitioner or organization who performed 284 * the action.) 285 */ 286 public ImmunizationPerformerComponent setActor(Reference value) { 287 this.actor = value; 288 return this; 289 } 290 291 /** 292 * @return {@link #actor} The actual object that is the target of the reference. 293 * The reference library doesn't populate this, but you can use it to 294 * hold the resource if you resolve it. (The practitioner or 295 * organization who performed the action.) 296 */ 297 public Resource getActorTarget() { 298 return this.actorTarget; 299 } 300 301 /** 302 * @param value {@link #actor} The actual object that is the target of the 303 * reference. The reference library doesn't use these, but you can 304 * use it to hold the resource if you resolve it. (The practitioner 305 * or organization who performed the action.) 306 */ 307 public ImmunizationPerformerComponent setActorTarget(Resource value) { 308 this.actorTarget = value; 309 return this; 310 } 311 312 protected void listChildren(List<Property> children) { 313 super.listChildren(children); 314 children.add(new Property("function", "CodeableConcept", 315 "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, function)); 316 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization)", 317 "The practitioner or organization who performed the action.", 0, 1, actor)); 318 } 319 320 @Override 321 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 322 switch (_hash) { 323 case 1380938712: 324 /* function */ return new Property("function", "CodeableConcept", 325 "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, 326 function); 327 case 92645877: 328 /* actor */ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization)", 329 "The practitioner or organization who performed the action.", 0, 1, actor); 330 default: 331 return super.getNamedProperty(_hash, _name, _checkValid); 332 } 333 334 } 335 336 @Override 337 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 338 switch (hash) { 339 case 1380938712: 340 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 341 case 92645877: 342 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 343 default: 344 return super.getProperty(hash, name, checkValid); 345 } 346 347 } 348 349 @Override 350 public Base setProperty(int hash, String name, Base value) throws FHIRException { 351 switch (hash) { 352 case 1380938712: // function 353 this.function = castToCodeableConcept(value); // CodeableConcept 354 return value; 355 case 92645877: // actor 356 this.actor = castToReference(value); // Reference 357 return value; 358 default: 359 return super.setProperty(hash, name, value); 360 } 361 362 } 363 364 @Override 365 public Base setProperty(String name, Base value) throws FHIRException { 366 if (name.equals("function")) { 367 this.function = castToCodeableConcept(value); // CodeableConcept 368 } else if (name.equals("actor")) { 369 this.actor = castToReference(value); // Reference 370 } else 371 return super.setProperty(name, value); 372 return value; 373 } 374 375 @Override 376 public void removeChild(String name, Base value) throws FHIRException { 377 if (name.equals("function")) { 378 this.function = null; 379 } else if (name.equals("actor")) { 380 this.actor = null; 381 } else 382 super.removeChild(name, value); 383 384 } 385 386 @Override 387 public Base makeProperty(int hash, String name) throws FHIRException { 388 switch (hash) { 389 case 1380938712: 390 return getFunction(); 391 case 92645877: 392 return getActor(); 393 default: 394 return super.makeProperty(hash, name); 395 } 396 397 } 398 399 @Override 400 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 401 switch (hash) { 402 case 1380938712: 403 /* function */ return new String[] { "CodeableConcept" }; 404 case 92645877: 405 /* actor */ return new String[] { "Reference" }; 406 default: 407 return super.getTypesForProperty(hash, name); 408 } 409 410 } 411 412 @Override 413 public Base addChild(String name) throws FHIRException { 414 if (name.equals("function")) { 415 this.function = new CodeableConcept(); 416 return this.function; 417 } else if (name.equals("actor")) { 418 this.actor = new Reference(); 419 return this.actor; 420 } else 421 return super.addChild(name); 422 } 423 424 public ImmunizationPerformerComponent copy() { 425 ImmunizationPerformerComponent dst = new ImmunizationPerformerComponent(); 426 copyValues(dst); 427 return dst; 428 } 429 430 public void copyValues(ImmunizationPerformerComponent dst) { 431 super.copyValues(dst); 432 dst.function = function == null ? null : function.copy(); 433 dst.actor = actor == null ? null : actor.copy(); 434 } 435 436 @Override 437 public boolean equalsDeep(Base other_) { 438 if (!super.equalsDeep(other_)) 439 return false; 440 if (!(other_ instanceof ImmunizationPerformerComponent)) 441 return false; 442 ImmunizationPerformerComponent o = (ImmunizationPerformerComponent) other_; 443 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 444 } 445 446 @Override 447 public boolean equalsShallow(Base other_) { 448 if (!super.equalsShallow(other_)) 449 return false; 450 if (!(other_ instanceof ImmunizationPerformerComponent)) 451 return false; 452 ImmunizationPerformerComponent o = (ImmunizationPerformerComponent) other_; 453 return true; 454 } 455 456 public boolean isEmpty() { 457 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 458 } 459 460 public String fhirType() { 461 return "Immunization.performer"; 462 463 } 464 465 } 466 467 @Block() 468 public static class ImmunizationEducationComponent extends BackboneElement implements IBaseBackboneElement { 469 /** 470 * Identifier of the material presented to the patient. 471 */ 472 @Child(name = "documentType", type = { 473 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 474 @Description(shortDefinition = "Educational material document identifier", formalDefinition = "Identifier of the material presented to the patient.") 475 protected StringType documentType; 476 477 /** 478 * Reference pointer to the educational material given to the patient if the 479 * information was on line. 480 */ 481 @Child(name = "reference", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 482 @Description(shortDefinition = "Educational material reference pointer", formalDefinition = "Reference pointer to the educational material given to the patient if the information was on line.") 483 protected UriType reference; 484 485 /** 486 * Date the educational material was published. 487 */ 488 @Child(name = "publicationDate", type = { 489 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 490 @Description(shortDefinition = "Educational material publication date", formalDefinition = "Date the educational material was published.") 491 protected DateTimeType publicationDate; 492 493 /** 494 * Date the educational material was given to the patient. 495 */ 496 @Child(name = "presentationDate", type = { 497 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 498 @Description(shortDefinition = "Educational material presentation date", formalDefinition = "Date the educational material was given to the patient.") 499 protected DateTimeType presentationDate; 500 501 private static final long serialVersionUID = -1277654827L; 502 503 /** 504 * Constructor 505 */ 506 public ImmunizationEducationComponent() { 507 super(); 508 } 509 510 /** 511 * @return {@link #documentType} (Identifier of the material presented to the 512 * patient.). This is the underlying object with id, value and 513 * extensions. The accessor "getDocumentType" gives direct access to the 514 * value 515 */ 516 public StringType getDocumentTypeElement() { 517 if (this.documentType == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create ImmunizationEducationComponent.documentType"); 520 else if (Configuration.doAutoCreate()) 521 this.documentType = new StringType(); // bb 522 return this.documentType; 523 } 524 525 public boolean hasDocumentTypeElement() { 526 return this.documentType != null && !this.documentType.isEmpty(); 527 } 528 529 public boolean hasDocumentType() { 530 return this.documentType != null && !this.documentType.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #documentType} (Identifier of the material presented to 535 * the patient.). This is the underlying object with id, value and 536 * extensions. The accessor "getDocumentType" gives direct access 537 * to the value 538 */ 539 public ImmunizationEducationComponent setDocumentTypeElement(StringType value) { 540 this.documentType = value; 541 return this; 542 } 543 544 /** 545 * @return Identifier of the material presented to the patient. 546 */ 547 public String getDocumentType() { 548 return this.documentType == null ? null : this.documentType.getValue(); 549 } 550 551 /** 552 * @param value Identifier of the material presented to the patient. 553 */ 554 public ImmunizationEducationComponent setDocumentType(String value) { 555 if (Utilities.noString(value)) 556 this.documentType = null; 557 else { 558 if (this.documentType == null) 559 this.documentType = new StringType(); 560 this.documentType.setValue(value); 561 } 562 return this; 563 } 564 565 /** 566 * @return {@link #reference} (Reference pointer to the educational material 567 * given to the patient if the information was on line.). This is the 568 * underlying object with id, value and extensions. The accessor 569 * "getReference" gives direct access to the value 570 */ 571 public UriType getReferenceElement() { 572 if (this.reference == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create ImmunizationEducationComponent.reference"); 575 else if (Configuration.doAutoCreate()) 576 this.reference = new UriType(); // bb 577 return this.reference; 578 } 579 580 public boolean hasReferenceElement() { 581 return this.reference != null && !this.reference.isEmpty(); 582 } 583 584 public boolean hasReference() { 585 return this.reference != null && !this.reference.isEmpty(); 586 } 587 588 /** 589 * @param value {@link #reference} (Reference pointer to the educational 590 * material given to the patient if the information was on line.). 591 * This is the underlying object with id, value and extensions. The 592 * accessor "getReference" gives direct access to the value 593 */ 594 public ImmunizationEducationComponent setReferenceElement(UriType value) { 595 this.reference = value; 596 return this; 597 } 598 599 /** 600 * @return Reference pointer to the educational material given to the patient if 601 * the information was on line. 602 */ 603 public String getReference() { 604 return this.reference == null ? null : this.reference.getValue(); 605 } 606 607 /** 608 * @param value Reference pointer to the educational material given to the 609 * patient if the information was on line. 610 */ 611 public ImmunizationEducationComponent setReference(String value) { 612 if (Utilities.noString(value)) 613 this.reference = null; 614 else { 615 if (this.reference == null) 616 this.reference = new UriType(); 617 this.reference.setValue(value); 618 } 619 return this; 620 } 621 622 /** 623 * @return {@link #publicationDate} (Date the educational material was 624 * published.). This is the underlying object with id, value and 625 * extensions. The accessor "getPublicationDate" gives direct access to 626 * the value 627 */ 628 public DateTimeType getPublicationDateElement() { 629 if (this.publicationDate == null) 630 if (Configuration.errorOnAutoCreate()) 631 throw new Error("Attempt to auto-create ImmunizationEducationComponent.publicationDate"); 632 else if (Configuration.doAutoCreate()) 633 this.publicationDate = new DateTimeType(); // bb 634 return this.publicationDate; 635 } 636 637 public boolean hasPublicationDateElement() { 638 return this.publicationDate != null && !this.publicationDate.isEmpty(); 639 } 640 641 public boolean hasPublicationDate() { 642 return this.publicationDate != null && !this.publicationDate.isEmpty(); 643 } 644 645 /** 646 * @param value {@link #publicationDate} (Date the educational material was 647 * published.). This is the underlying object with id, value and 648 * extensions. The accessor "getPublicationDate" gives direct 649 * access to the value 650 */ 651 public ImmunizationEducationComponent setPublicationDateElement(DateTimeType value) { 652 this.publicationDate = value; 653 return this; 654 } 655 656 /** 657 * @return Date the educational material was published. 658 */ 659 public Date getPublicationDate() { 660 return this.publicationDate == null ? null : this.publicationDate.getValue(); 661 } 662 663 /** 664 * @param value Date the educational material was published. 665 */ 666 public ImmunizationEducationComponent setPublicationDate(Date value) { 667 if (value == null) 668 this.publicationDate = null; 669 else { 670 if (this.publicationDate == null) 671 this.publicationDate = new DateTimeType(); 672 this.publicationDate.setValue(value); 673 } 674 return this; 675 } 676 677 /** 678 * @return {@link #presentationDate} (Date the educational material was given to 679 * the patient.). This is the underlying object with id, value and 680 * extensions. The accessor "getPresentationDate" gives direct access to 681 * the value 682 */ 683 public DateTimeType getPresentationDateElement() { 684 if (this.presentationDate == null) 685 if (Configuration.errorOnAutoCreate()) 686 throw new Error("Attempt to auto-create ImmunizationEducationComponent.presentationDate"); 687 else if (Configuration.doAutoCreate()) 688 this.presentationDate = new DateTimeType(); // bb 689 return this.presentationDate; 690 } 691 692 public boolean hasPresentationDateElement() { 693 return this.presentationDate != null && !this.presentationDate.isEmpty(); 694 } 695 696 public boolean hasPresentationDate() { 697 return this.presentationDate != null && !this.presentationDate.isEmpty(); 698 } 699 700 /** 701 * @param value {@link #presentationDate} (Date the educational material was 702 * given to the patient.). This is the underlying object with id, 703 * value and extensions. The accessor "getPresentationDate" gives 704 * direct access to the value 705 */ 706 public ImmunizationEducationComponent setPresentationDateElement(DateTimeType value) { 707 this.presentationDate = value; 708 return this; 709 } 710 711 /** 712 * @return Date the educational material was given to the patient. 713 */ 714 public Date getPresentationDate() { 715 return this.presentationDate == null ? null : this.presentationDate.getValue(); 716 } 717 718 /** 719 * @param value Date the educational material was given to the patient. 720 */ 721 public ImmunizationEducationComponent setPresentationDate(Date value) { 722 if (value == null) 723 this.presentationDate = null; 724 else { 725 if (this.presentationDate == null) 726 this.presentationDate = new DateTimeType(); 727 this.presentationDate.setValue(value); 728 } 729 return this; 730 } 731 732 protected void listChildren(List<Property> children) { 733 super.listChildren(children); 734 children.add(new Property("documentType", "string", "Identifier of the material presented to the patient.", 0, 1, 735 documentType)); 736 children.add(new Property("reference", "uri", 737 "Reference pointer to the educational material given to the patient if the information was on line.", 0, 1, 738 reference)); 739 children.add(new Property("publicationDate", "dateTime", "Date the educational material was published.", 0, 1, 740 publicationDate)); 741 children.add(new Property("presentationDate", "dateTime", 742 "Date the educational material was given to the patient.", 0, 1, presentationDate)); 743 } 744 745 @Override 746 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 747 switch (_hash) { 748 case -1473196299: 749 /* documentType */ return new Property("documentType", "string", 750 "Identifier of the material presented to the patient.", 0, 1, documentType); 751 case -925155509: 752 /* reference */ return new Property("reference", "uri", 753 "Reference pointer to the educational material given to the patient if the information was on line.", 0, 1, 754 reference); 755 case 1470566394: 756 /* publicationDate */ return new Property("publicationDate", "dateTime", 757 "Date the educational material was published.", 0, 1, publicationDate); 758 case 1602373096: 759 /* presentationDate */ return new Property("presentationDate", "dateTime", 760 "Date the educational material was given to the patient.", 0, 1, presentationDate); 761 default: 762 return super.getNamedProperty(_hash, _name, _checkValid); 763 } 764 765 } 766 767 @Override 768 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 769 switch (hash) { 770 case -1473196299: 771 /* documentType */ return this.documentType == null ? new Base[0] : new Base[] { this.documentType }; // StringType 772 case -925155509: 773 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // UriType 774 case 1470566394: 775 /* publicationDate */ return this.publicationDate == null ? new Base[0] : new Base[] { this.publicationDate }; // DateTimeType 776 case 1602373096: 777 /* presentationDate */ return this.presentationDate == null ? new Base[0] 778 : new Base[] { this.presentationDate }; // DateTimeType 779 default: 780 return super.getProperty(hash, name, checkValid); 781 } 782 783 } 784 785 @Override 786 public Base setProperty(int hash, String name, Base value) throws FHIRException { 787 switch (hash) { 788 case -1473196299: // documentType 789 this.documentType = castToString(value); // StringType 790 return value; 791 case -925155509: // reference 792 this.reference = castToUri(value); // UriType 793 return value; 794 case 1470566394: // publicationDate 795 this.publicationDate = castToDateTime(value); // DateTimeType 796 return value; 797 case 1602373096: // presentationDate 798 this.presentationDate = castToDateTime(value); // DateTimeType 799 return value; 800 default: 801 return super.setProperty(hash, name, value); 802 } 803 804 } 805 806 @Override 807 public Base setProperty(String name, Base value) throws FHIRException { 808 if (name.equals("documentType")) { 809 this.documentType = castToString(value); // StringType 810 } else if (name.equals("reference")) { 811 this.reference = castToUri(value); // UriType 812 } else if (name.equals("publicationDate")) { 813 this.publicationDate = castToDateTime(value); // DateTimeType 814 } else if (name.equals("presentationDate")) { 815 this.presentationDate = castToDateTime(value); // DateTimeType 816 } else 817 return super.setProperty(name, value); 818 return value; 819 } 820 821 @Override 822 public void removeChild(String name, Base value) throws FHIRException { 823 if (name.equals("documentType")) { 824 this.documentType = null; 825 } else if (name.equals("reference")) { 826 this.reference = null; 827 } else if (name.equals("publicationDate")) { 828 this.publicationDate = null; 829 } else if (name.equals("presentationDate")) { 830 this.presentationDate = null; 831 } else 832 super.removeChild(name, value); 833 834 } 835 836 @Override 837 public Base makeProperty(int hash, String name) throws FHIRException { 838 switch (hash) { 839 case -1473196299: 840 return getDocumentTypeElement(); 841 case -925155509: 842 return getReferenceElement(); 843 case 1470566394: 844 return getPublicationDateElement(); 845 case 1602373096: 846 return getPresentationDateElement(); 847 default: 848 return super.makeProperty(hash, name); 849 } 850 851 } 852 853 @Override 854 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 855 switch (hash) { 856 case -1473196299: 857 /* documentType */ return new String[] { "string" }; 858 case -925155509: 859 /* reference */ return new String[] { "uri" }; 860 case 1470566394: 861 /* publicationDate */ return new String[] { "dateTime" }; 862 case 1602373096: 863 /* presentationDate */ return new String[] { "dateTime" }; 864 default: 865 return super.getTypesForProperty(hash, name); 866 } 867 868 } 869 870 @Override 871 public Base addChild(String name) throws FHIRException { 872 if (name.equals("documentType")) { 873 throw new FHIRException("Cannot call addChild on a singleton property Immunization.documentType"); 874 } else if (name.equals("reference")) { 875 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reference"); 876 } else if (name.equals("publicationDate")) { 877 throw new FHIRException("Cannot call addChild on a singleton property Immunization.publicationDate"); 878 } else if (name.equals("presentationDate")) { 879 throw new FHIRException("Cannot call addChild on a singleton property Immunization.presentationDate"); 880 } else 881 return super.addChild(name); 882 } 883 884 public ImmunizationEducationComponent copy() { 885 ImmunizationEducationComponent dst = new ImmunizationEducationComponent(); 886 copyValues(dst); 887 return dst; 888 } 889 890 public void copyValues(ImmunizationEducationComponent dst) { 891 super.copyValues(dst); 892 dst.documentType = documentType == null ? null : documentType.copy(); 893 dst.reference = reference == null ? null : reference.copy(); 894 dst.publicationDate = publicationDate == null ? null : publicationDate.copy(); 895 dst.presentationDate = presentationDate == null ? null : presentationDate.copy(); 896 } 897 898 @Override 899 public boolean equalsDeep(Base other_) { 900 if (!super.equalsDeep(other_)) 901 return false; 902 if (!(other_ instanceof ImmunizationEducationComponent)) 903 return false; 904 ImmunizationEducationComponent o = (ImmunizationEducationComponent) other_; 905 return compareDeep(documentType, o.documentType, true) && compareDeep(reference, o.reference, true) 906 && compareDeep(publicationDate, o.publicationDate, true) 907 && compareDeep(presentationDate, o.presentationDate, true); 908 } 909 910 @Override 911 public boolean equalsShallow(Base other_) { 912 if (!super.equalsShallow(other_)) 913 return false; 914 if (!(other_ instanceof ImmunizationEducationComponent)) 915 return false; 916 ImmunizationEducationComponent o = (ImmunizationEducationComponent) other_; 917 return compareValues(documentType, o.documentType, true) && compareValues(reference, o.reference, true) 918 && compareValues(publicationDate, o.publicationDate, true) 919 && compareValues(presentationDate, o.presentationDate, true); 920 } 921 922 public boolean isEmpty() { 923 return super.isEmpty() 924 && ca.uhn.fhir.util.ElementUtil.isEmpty(documentType, reference, publicationDate, presentationDate); 925 } 926 927 public String fhirType() { 928 return "Immunization.education"; 929 930 } 931 932 } 933 934 @Block() 935 public static class ImmunizationReactionComponent extends BackboneElement implements IBaseBackboneElement { 936 /** 937 * Date of reaction to the immunization. 938 */ 939 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 940 @Description(shortDefinition = "When reaction started", formalDefinition = "Date of reaction to the immunization.") 941 protected DateTimeType date; 942 943 /** 944 * Details of the reaction. 945 */ 946 @Child(name = "detail", type = { 947 Observation.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 948 @Description(shortDefinition = "Additional information on reaction", formalDefinition = "Details of the reaction.") 949 protected Reference detail; 950 951 /** 952 * The actual object that is the target of the reference (Details of the 953 * reaction.) 954 */ 955 protected Observation detailTarget; 956 957 /** 958 * Self-reported indicator. 959 */ 960 @Child(name = "reported", type = { 961 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 962 @Description(shortDefinition = "Indicates self-reported reaction", formalDefinition = "Self-reported indicator.") 963 protected BooleanType reported; 964 965 private static final long serialVersionUID = -1297668556L; 966 967 /** 968 * Constructor 969 */ 970 public ImmunizationReactionComponent() { 971 super(); 972 } 973 974 /** 975 * @return {@link #date} (Date of reaction to the immunization.). This is the 976 * underlying object with id, value and extensions. The accessor 977 * "getDate" gives direct access to the value 978 */ 979 public DateTimeType getDateElement() { 980 if (this.date == null) 981 if (Configuration.errorOnAutoCreate()) 982 throw new Error("Attempt to auto-create ImmunizationReactionComponent.date"); 983 else if (Configuration.doAutoCreate()) 984 this.date = new DateTimeType(); // bb 985 return this.date; 986 } 987 988 public boolean hasDateElement() { 989 return this.date != null && !this.date.isEmpty(); 990 } 991 992 public boolean hasDate() { 993 return this.date != null && !this.date.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #date} (Date of reaction to the immunization.). This is 998 * the underlying object with id, value and extensions. The 999 * accessor "getDate" gives direct access to the value 1000 */ 1001 public ImmunizationReactionComponent setDateElement(DateTimeType value) { 1002 this.date = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return Date of reaction to the immunization. 1008 */ 1009 public Date getDate() { 1010 return this.date == null ? null : this.date.getValue(); 1011 } 1012 1013 /** 1014 * @param value Date of reaction to the immunization. 1015 */ 1016 public ImmunizationReactionComponent setDate(Date value) { 1017 if (value == null) 1018 this.date = null; 1019 else { 1020 if (this.date == null) 1021 this.date = new DateTimeType(); 1022 this.date.setValue(value); 1023 } 1024 return this; 1025 } 1026 1027 /** 1028 * @return {@link #detail} (Details of the reaction.) 1029 */ 1030 public Reference getDetail() { 1031 if (this.detail == null) 1032 if (Configuration.errorOnAutoCreate()) 1033 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 1034 else if (Configuration.doAutoCreate()) 1035 this.detail = new Reference(); // cc 1036 return this.detail; 1037 } 1038 1039 public boolean hasDetail() { 1040 return this.detail != null && !this.detail.isEmpty(); 1041 } 1042 1043 /** 1044 * @param value {@link #detail} (Details of the reaction.) 1045 */ 1046 public ImmunizationReactionComponent setDetail(Reference value) { 1047 this.detail = value; 1048 return this; 1049 } 1050 1051 /** 1052 * @return {@link #detail} The actual object that is the target of the 1053 * reference. The reference library doesn't populate this, but you can 1054 * use it to hold the resource if you resolve it. (Details of the 1055 * reaction.) 1056 */ 1057 public Observation getDetailTarget() { 1058 if (this.detailTarget == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 1061 else if (Configuration.doAutoCreate()) 1062 this.detailTarget = new Observation(); // aa 1063 return this.detailTarget; 1064 } 1065 1066 /** 1067 * @param value {@link #detail} The actual object that is the target of the 1068 * reference. The reference library doesn't use these, but you can 1069 * use it to hold the resource if you resolve it. (Details of the 1070 * reaction.) 1071 */ 1072 public ImmunizationReactionComponent setDetailTarget(Observation value) { 1073 this.detailTarget = value; 1074 return this; 1075 } 1076 1077 /** 1078 * @return {@link #reported} (Self-reported indicator.). This is the underlying 1079 * object with id, value and extensions. The accessor "getReported" 1080 * gives direct access to the value 1081 */ 1082 public BooleanType getReportedElement() { 1083 if (this.reported == null) 1084 if (Configuration.errorOnAutoCreate()) 1085 throw new Error("Attempt to auto-create ImmunizationReactionComponent.reported"); 1086 else if (Configuration.doAutoCreate()) 1087 this.reported = new BooleanType(); // bb 1088 return this.reported; 1089 } 1090 1091 public boolean hasReportedElement() { 1092 return this.reported != null && !this.reported.isEmpty(); 1093 } 1094 1095 public boolean hasReported() { 1096 return this.reported != null && !this.reported.isEmpty(); 1097 } 1098 1099 /** 1100 * @param value {@link #reported} (Self-reported indicator.). This is the 1101 * underlying object with id, value and extensions. The accessor 1102 * "getReported" gives direct access to the value 1103 */ 1104 public ImmunizationReactionComponent setReportedElement(BooleanType value) { 1105 this.reported = value; 1106 return this; 1107 } 1108 1109 /** 1110 * @return Self-reported indicator. 1111 */ 1112 public boolean getReported() { 1113 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 1114 } 1115 1116 /** 1117 * @param value Self-reported indicator. 1118 */ 1119 public ImmunizationReactionComponent setReported(boolean value) { 1120 if (this.reported == null) 1121 this.reported = new BooleanType(); 1122 this.reported.setValue(value); 1123 return this; 1124 } 1125 1126 protected void listChildren(List<Property> children) { 1127 super.listChildren(children); 1128 children.add(new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date)); 1129 children.add(new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail)); 1130 children.add(new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported)); 1131 } 1132 1133 @Override 1134 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1135 switch (_hash) { 1136 case 3076014: 1137 /* date */ return new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date); 1138 case -1335224239: 1139 /* detail */ return new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail); 1140 case -427039533: 1141 /* reported */ return new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported); 1142 default: 1143 return super.getNamedProperty(_hash, _name, _checkValid); 1144 } 1145 1146 } 1147 1148 @Override 1149 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1150 switch (hash) { 1151 case 3076014: 1152 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1153 case -1335224239: 1154 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // Reference 1155 case -427039533: 1156 /* reported */ return this.reported == null ? new Base[0] : new Base[] { this.reported }; // BooleanType 1157 default: 1158 return super.getProperty(hash, name, checkValid); 1159 } 1160 1161 } 1162 1163 @Override 1164 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1165 switch (hash) { 1166 case 3076014: // date 1167 this.date = castToDateTime(value); // DateTimeType 1168 return value; 1169 case -1335224239: // detail 1170 this.detail = castToReference(value); // Reference 1171 return value; 1172 case -427039533: // reported 1173 this.reported = castToBoolean(value); // BooleanType 1174 return value; 1175 default: 1176 return super.setProperty(hash, name, value); 1177 } 1178 1179 } 1180 1181 @Override 1182 public Base setProperty(String name, Base value) throws FHIRException { 1183 if (name.equals("date")) { 1184 this.date = castToDateTime(value); // DateTimeType 1185 } else if (name.equals("detail")) { 1186 this.detail = castToReference(value); // Reference 1187 } else if (name.equals("reported")) { 1188 this.reported = castToBoolean(value); // BooleanType 1189 } else 1190 return super.setProperty(name, value); 1191 return value; 1192 } 1193 1194 @Override 1195 public void removeChild(String name, Base value) throws FHIRException { 1196 if (name.equals("date")) { 1197 this.date = null; 1198 } else if (name.equals("detail")) { 1199 this.detail = null; 1200 } else if (name.equals("reported")) { 1201 this.reported = null; 1202 } else 1203 super.removeChild(name, value); 1204 1205 } 1206 1207 @Override 1208 public Base makeProperty(int hash, String name) throws FHIRException { 1209 switch (hash) { 1210 case 3076014: 1211 return getDateElement(); 1212 case -1335224239: 1213 return getDetail(); 1214 case -427039533: 1215 return getReportedElement(); 1216 default: 1217 return super.makeProperty(hash, name); 1218 } 1219 1220 } 1221 1222 @Override 1223 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1224 switch (hash) { 1225 case 3076014: 1226 /* date */ return new String[] { "dateTime" }; 1227 case -1335224239: 1228 /* detail */ return new String[] { "Reference" }; 1229 case -427039533: 1230 /* reported */ return new String[] { "boolean" }; 1231 default: 1232 return super.getTypesForProperty(hash, name); 1233 } 1234 1235 } 1236 1237 @Override 1238 public Base addChild(String name) throws FHIRException { 1239 if (name.equals("date")) { 1240 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 1241 } else if (name.equals("detail")) { 1242 this.detail = new Reference(); 1243 return this.detail; 1244 } else if (name.equals("reported")) { 1245 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reported"); 1246 } else 1247 return super.addChild(name); 1248 } 1249 1250 public ImmunizationReactionComponent copy() { 1251 ImmunizationReactionComponent dst = new ImmunizationReactionComponent(); 1252 copyValues(dst); 1253 return dst; 1254 } 1255 1256 public void copyValues(ImmunizationReactionComponent dst) { 1257 super.copyValues(dst); 1258 dst.date = date == null ? null : date.copy(); 1259 dst.detail = detail == null ? null : detail.copy(); 1260 dst.reported = reported == null ? null : reported.copy(); 1261 } 1262 1263 @Override 1264 public boolean equalsDeep(Base other_) { 1265 if (!super.equalsDeep(other_)) 1266 return false; 1267 if (!(other_ instanceof ImmunizationReactionComponent)) 1268 return false; 1269 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 1270 return compareDeep(date, o.date, true) && compareDeep(detail, o.detail, true) 1271 && compareDeep(reported, o.reported, true); 1272 } 1273 1274 @Override 1275 public boolean equalsShallow(Base other_) { 1276 if (!super.equalsShallow(other_)) 1277 return false; 1278 if (!(other_ instanceof ImmunizationReactionComponent)) 1279 return false; 1280 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 1281 return compareValues(date, o.date, true) && compareValues(reported, o.reported, true); 1282 } 1283 1284 public boolean isEmpty() { 1285 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, detail, reported); 1286 } 1287 1288 public String fhirType() { 1289 return "Immunization.reaction"; 1290 1291 } 1292 1293 } 1294 1295 @Block() 1296 public static class ImmunizationProtocolAppliedComponent extends BackboneElement implements IBaseBackboneElement { 1297 /** 1298 * One possible path to achieve presumed immunity against a disease - within the 1299 * context of an authority. 1300 */ 1301 @Child(name = "series", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1302 @Description(shortDefinition = "Name of vaccine series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 1303 protected StringType series; 1304 1305 /** 1306 * Indicates the authority who published the protocol (e.g. ACIP) that is being 1307 * followed. 1308 */ 1309 @Child(name = "authority", type = { 1310 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1311 @Description(shortDefinition = "Who is responsible for publishing the recommendations", formalDefinition = "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.") 1312 protected Reference authority; 1313 1314 /** 1315 * The actual object that is the target of the reference (Indicates the 1316 * authority who published the protocol (e.g. ACIP) that is being followed.) 1317 */ 1318 protected Organization authorityTarget; 1319 1320 /** 1321 * The vaccine preventable disease the dose is being administered against. 1322 */ 1323 @Child(name = "targetDisease", type = { 1324 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1325 @Description(shortDefinition = "Vaccine preventatable disease being targetted", formalDefinition = "The vaccine preventable disease the dose is being administered against.") 1326 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-target-disease") 1327 protected List<CodeableConcept> targetDisease; 1328 1329 /** 1330 * Nominal position in a series. 1331 */ 1332 @Child(name = "doseNumber", type = { PositiveIntType.class, 1333 StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 1334 @Description(shortDefinition = "Dose number within series", formalDefinition = "Nominal position in a series.") 1335 protected Type doseNumber; 1336 1337 /** 1338 * The recommended number of doses to achieve immunity. 1339 */ 1340 @Child(name = "seriesDoses", type = { PositiveIntType.class, 1341 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1342 @Description(shortDefinition = "Recommended number of doses for immunity", formalDefinition = "The recommended number of doses to achieve immunity.") 1343 protected Type seriesDoses; 1344 1345 private static final long serialVersionUID = -1022717242L; 1346 1347 /** 1348 * Constructor 1349 */ 1350 public ImmunizationProtocolAppliedComponent() { 1351 super(); 1352 } 1353 1354 /** 1355 * Constructor 1356 */ 1357 public ImmunizationProtocolAppliedComponent(Type doseNumber) { 1358 super(); 1359 this.doseNumber = doseNumber; 1360 } 1361 1362 /** 1363 * @return {@link #series} (One possible path to achieve presumed immunity 1364 * against a disease - within the context of an authority.). This is the 1365 * underlying object with id, value and extensions. The accessor 1366 * "getSeries" gives direct access to the value 1367 */ 1368 public StringType getSeriesElement() { 1369 if (this.series == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.series"); 1372 else if (Configuration.doAutoCreate()) 1373 this.series = new StringType(); // bb 1374 return this.series; 1375 } 1376 1377 public boolean hasSeriesElement() { 1378 return this.series != null && !this.series.isEmpty(); 1379 } 1380 1381 public boolean hasSeries() { 1382 return this.series != null && !this.series.isEmpty(); 1383 } 1384 1385 /** 1386 * @param value {@link #series} (One possible path to achieve presumed immunity 1387 * against a disease - within the context of an authority.). This 1388 * is the underlying object with id, value and extensions. The 1389 * accessor "getSeries" gives direct access to the value 1390 */ 1391 public ImmunizationProtocolAppliedComponent setSeriesElement(StringType value) { 1392 this.series = value; 1393 return this; 1394 } 1395 1396 /** 1397 * @return One possible path to achieve presumed immunity against a disease - 1398 * within the context of an authority. 1399 */ 1400 public String getSeries() { 1401 return this.series == null ? null : this.series.getValue(); 1402 } 1403 1404 /** 1405 * @param value One possible path to achieve presumed immunity against a disease 1406 * - within the context of an authority. 1407 */ 1408 public ImmunizationProtocolAppliedComponent setSeries(String value) { 1409 if (Utilities.noString(value)) 1410 this.series = null; 1411 else { 1412 if (this.series == null) 1413 this.series = new StringType(); 1414 this.series.setValue(value); 1415 } 1416 return this; 1417 } 1418 1419 /** 1420 * @return {@link #authority} (Indicates the authority who published the 1421 * protocol (e.g. ACIP) that is being followed.) 1422 */ 1423 public Reference getAuthority() { 1424 if (this.authority == null) 1425 if (Configuration.errorOnAutoCreate()) 1426 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.authority"); 1427 else if (Configuration.doAutoCreate()) 1428 this.authority = new Reference(); // cc 1429 return this.authority; 1430 } 1431 1432 public boolean hasAuthority() { 1433 return this.authority != null && !this.authority.isEmpty(); 1434 } 1435 1436 /** 1437 * @param value {@link #authority} (Indicates the authority who published the 1438 * protocol (e.g. ACIP) that is being followed.) 1439 */ 1440 public ImmunizationProtocolAppliedComponent setAuthority(Reference value) { 1441 this.authority = value; 1442 return this; 1443 } 1444 1445 /** 1446 * @return {@link #authority} The actual object that is the target of the 1447 * reference. The reference library doesn't populate this, but you can 1448 * use it to hold the resource if you resolve it. (Indicates the 1449 * authority who published the protocol (e.g. ACIP) that is being 1450 * followed.) 1451 */ 1452 public Organization getAuthorityTarget() { 1453 if (this.authorityTarget == null) 1454 if (Configuration.errorOnAutoCreate()) 1455 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.authority"); 1456 else if (Configuration.doAutoCreate()) 1457 this.authorityTarget = new Organization(); // aa 1458 return this.authorityTarget; 1459 } 1460 1461 /** 1462 * @param value {@link #authority} The actual object that is the target of the 1463 * reference. The reference library doesn't use these, but you can 1464 * use it to hold the resource if you resolve it. (Indicates the 1465 * authority who published the protocol (e.g. ACIP) that is being 1466 * followed.) 1467 */ 1468 public ImmunizationProtocolAppliedComponent setAuthorityTarget(Organization value) { 1469 this.authorityTarget = value; 1470 return this; 1471 } 1472 1473 /** 1474 * @return {@link #targetDisease} (The vaccine preventable disease the dose is 1475 * being administered against.) 1476 */ 1477 public List<CodeableConcept> getTargetDisease() { 1478 if (this.targetDisease == null) 1479 this.targetDisease = new ArrayList<CodeableConcept>(); 1480 return this.targetDisease; 1481 } 1482 1483 /** 1484 * @return Returns a reference to <code>this</code> for easy method chaining 1485 */ 1486 public ImmunizationProtocolAppliedComponent setTargetDisease(List<CodeableConcept> theTargetDisease) { 1487 this.targetDisease = theTargetDisease; 1488 return this; 1489 } 1490 1491 public boolean hasTargetDisease() { 1492 if (this.targetDisease == null) 1493 return false; 1494 for (CodeableConcept item : this.targetDisease) 1495 if (!item.isEmpty()) 1496 return true; 1497 return false; 1498 } 1499 1500 public CodeableConcept addTargetDisease() { // 3 1501 CodeableConcept t = new CodeableConcept(); 1502 if (this.targetDisease == null) 1503 this.targetDisease = new ArrayList<CodeableConcept>(); 1504 this.targetDisease.add(t); 1505 return t; 1506 } 1507 1508 public ImmunizationProtocolAppliedComponent addTargetDisease(CodeableConcept t) { // 3 1509 if (t == null) 1510 return this; 1511 if (this.targetDisease == null) 1512 this.targetDisease = new ArrayList<CodeableConcept>(); 1513 this.targetDisease.add(t); 1514 return this; 1515 } 1516 1517 /** 1518 * @return The first repetition of repeating field {@link #targetDisease}, 1519 * creating it if it does not already exist 1520 */ 1521 public CodeableConcept getTargetDiseaseFirstRep() { 1522 if (getTargetDisease().isEmpty()) { 1523 addTargetDisease(); 1524 } 1525 return getTargetDisease().get(0); 1526 } 1527 1528 /** 1529 * @return {@link #doseNumber} (Nominal position in a series.) 1530 */ 1531 public Type getDoseNumber() { 1532 return this.doseNumber; 1533 } 1534 1535 /** 1536 * @return {@link #doseNumber} (Nominal position in a series.) 1537 */ 1538 public PositiveIntType getDoseNumberPositiveIntType() throws FHIRException { 1539 if (this.doseNumber == null) 1540 this.doseNumber = new PositiveIntType(); 1541 if (!(this.doseNumber instanceof PositiveIntType)) 1542 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 1543 + this.doseNumber.getClass().getName() + " was encountered"); 1544 return (PositiveIntType) this.doseNumber; 1545 } 1546 1547 public boolean hasDoseNumberPositiveIntType() { 1548 return this != null && this.doseNumber instanceof PositiveIntType; 1549 } 1550 1551 /** 1552 * @return {@link #doseNumber} (Nominal position in a series.) 1553 */ 1554 public StringType getDoseNumberStringType() throws FHIRException { 1555 if (this.doseNumber == null) 1556 this.doseNumber = new StringType(); 1557 if (!(this.doseNumber instanceof StringType)) 1558 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1559 + this.doseNumber.getClass().getName() + " was encountered"); 1560 return (StringType) this.doseNumber; 1561 } 1562 1563 public boolean hasDoseNumberStringType() { 1564 return this != null && this.doseNumber instanceof StringType; 1565 } 1566 1567 public boolean hasDoseNumber() { 1568 return this.doseNumber != null && !this.doseNumber.isEmpty(); 1569 } 1570 1571 /** 1572 * @param value {@link #doseNumber} (Nominal position in a series.) 1573 */ 1574 public ImmunizationProtocolAppliedComponent setDoseNumber(Type value) { 1575 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 1576 throw new Error("Not the right type for Immunization.protocolApplied.doseNumber[x]: " + value.fhirType()); 1577 this.doseNumber = value; 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #seriesDoses} (The recommended number of doses to achieve 1583 * immunity.) 1584 */ 1585 public Type getSeriesDoses() { 1586 return this.seriesDoses; 1587 } 1588 1589 /** 1590 * @return {@link #seriesDoses} (The recommended number of doses to achieve 1591 * immunity.) 1592 */ 1593 public PositiveIntType getSeriesDosesPositiveIntType() throws FHIRException { 1594 if (this.seriesDoses == null) 1595 this.seriesDoses = new PositiveIntType(); 1596 if (!(this.seriesDoses instanceof PositiveIntType)) 1597 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 1598 + this.seriesDoses.getClass().getName() + " was encountered"); 1599 return (PositiveIntType) this.seriesDoses; 1600 } 1601 1602 public boolean hasSeriesDosesPositiveIntType() { 1603 return this != null && this.seriesDoses instanceof PositiveIntType; 1604 } 1605 1606 /** 1607 * @return {@link #seriesDoses} (The recommended number of doses to achieve 1608 * immunity.) 1609 */ 1610 public StringType getSeriesDosesStringType() throws FHIRException { 1611 if (this.seriesDoses == null) 1612 this.seriesDoses = new StringType(); 1613 if (!(this.seriesDoses instanceof StringType)) 1614 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1615 + this.seriesDoses.getClass().getName() + " was encountered"); 1616 return (StringType) this.seriesDoses; 1617 } 1618 1619 public boolean hasSeriesDosesStringType() { 1620 return this != null && this.seriesDoses instanceof StringType; 1621 } 1622 1623 public boolean hasSeriesDoses() { 1624 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1625 } 1626 1627 /** 1628 * @param value {@link #seriesDoses} (The recommended number of doses to achieve 1629 * immunity.) 1630 */ 1631 public ImmunizationProtocolAppliedComponent setSeriesDoses(Type value) { 1632 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 1633 throw new Error("Not the right type for Immunization.protocolApplied.seriesDoses[x]: " + value.fhirType()); 1634 this.seriesDoses = value; 1635 return this; 1636 } 1637 1638 protected void listChildren(List<Property> children) { 1639 super.listChildren(children); 1640 children.add(new Property("series", "string", 1641 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1642 1, series)); 1643 children.add(new Property("authority", "Reference(Organization)", 1644 "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.", 0, 1, authority)); 1645 children.add(new Property("targetDisease", "CodeableConcept", 1646 "The vaccine preventable disease the dose is being administered against.", 0, java.lang.Integer.MAX_VALUE, 1647 targetDisease)); 1648 children 1649 .add(new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1, doseNumber)); 1650 children.add(new Property("seriesDoses[x]", "positiveInt|string", 1651 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 1652 } 1653 1654 @Override 1655 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1656 switch (_hash) { 1657 case -905838985: 1658 /* series */ return new Property("series", "string", 1659 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1660 1, series); 1661 case 1475610435: 1662 /* authority */ return new Property("authority", "Reference(Organization)", 1663 "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.", 0, 1, authority); 1664 case -319593813: 1665 /* targetDisease */ return new Property("targetDisease", "CodeableConcept", 1666 "The vaccine preventable disease the dose is being administered against.", 0, java.lang.Integer.MAX_VALUE, 1667 targetDisease); 1668 case -1632295686: 1669 /* doseNumber[x] */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 1670 0, 1, doseNumber); 1671 case -887709242: 1672 /* doseNumber */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1673 1, doseNumber); 1674 case -1826134640: 1675 /* doseNumberPositiveInt */ return new Property("doseNumber[x]", "positiveInt|string", 1676 "Nominal position in a series.", 0, 1, doseNumber); 1677 case -333053577: 1678 /* doseNumberString */ return new Property("doseNumber[x]", "positiveInt|string", 1679 "Nominal position in a series.", 0, 1, doseNumber); 1680 case 1553560673: 1681 /* seriesDoses[x] */ return new Property("seriesDoses[x]", "positiveInt|string", 1682 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1683 case -1936727105: 1684 /* seriesDoses */ return new Property("seriesDoses[x]", "positiveInt|string", 1685 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1686 case -220897801: 1687 /* seriesDosesPositiveInt */ return new Property("seriesDoses[x]", "positiveInt|string", 1688 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1689 case -673569616: 1690 /* seriesDosesString */ return new Property("seriesDoses[x]", "positiveInt|string", 1691 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1692 default: 1693 return super.getNamedProperty(_hash, _name, _checkValid); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1700 switch (hash) { 1701 case -905838985: 1702 /* series */ return this.series == null ? new Base[0] : new Base[] { this.series }; // StringType 1703 case 1475610435: 1704 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // Reference 1705 case -319593813: 1706 /* targetDisease */ return this.targetDisease == null ? new Base[0] 1707 : this.targetDisease.toArray(new Base[this.targetDisease.size()]); // CodeableConcept 1708 case -887709242: 1709 /* doseNumber */ return this.doseNumber == null ? new Base[0] : new Base[] { this.doseNumber }; // Type 1710 case -1936727105: 1711 /* seriesDoses */ return this.seriesDoses == null ? new Base[0] : new Base[] { this.seriesDoses }; // Type 1712 default: 1713 return super.getProperty(hash, name, checkValid); 1714 } 1715 1716 } 1717 1718 @Override 1719 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1720 switch (hash) { 1721 case -905838985: // series 1722 this.series = castToString(value); // StringType 1723 return value; 1724 case 1475610435: // authority 1725 this.authority = castToReference(value); // Reference 1726 return value; 1727 case -319593813: // targetDisease 1728 this.getTargetDisease().add(castToCodeableConcept(value)); // CodeableConcept 1729 return value; 1730 case -887709242: // doseNumber 1731 this.doseNumber = castToType(value); // Type 1732 return value; 1733 case -1936727105: // seriesDoses 1734 this.seriesDoses = castToType(value); // Type 1735 return value; 1736 default: 1737 return super.setProperty(hash, name, value); 1738 } 1739 1740 } 1741 1742 @Override 1743 public Base setProperty(String name, Base value) throws FHIRException { 1744 if (name.equals("series")) { 1745 this.series = castToString(value); // StringType 1746 } else if (name.equals("authority")) { 1747 this.authority = castToReference(value); // Reference 1748 } else if (name.equals("targetDisease")) { 1749 this.getTargetDisease().add(castToCodeableConcept(value)); 1750 } else if (name.equals("doseNumber[x]")) { 1751 this.doseNumber = castToType(value); // Type 1752 } else if (name.equals("seriesDoses[x]")) { 1753 this.seriesDoses = castToType(value); // Type 1754 } else 1755 return super.setProperty(name, value); 1756 return value; 1757 } 1758 1759 @Override 1760 public void removeChild(String name, Base value) throws FHIRException { 1761 if (name.equals("series")) { 1762 this.series = null; 1763 } else if (name.equals("authority")) { 1764 this.authority = null; 1765 } else if (name.equals("targetDisease")) { 1766 this.getTargetDisease().remove(castToCodeableConcept(value)); 1767 } else if (name.equals("doseNumber[x]")) { 1768 this.doseNumber = null; 1769 } else if (name.equals("seriesDoses[x]")) { 1770 this.seriesDoses = null; 1771 } else 1772 super.removeChild(name, value); 1773 1774 } 1775 1776 @Override 1777 public Base makeProperty(int hash, String name) throws FHIRException { 1778 switch (hash) { 1779 case -905838985: 1780 return getSeriesElement(); 1781 case 1475610435: 1782 return getAuthority(); 1783 case -319593813: 1784 return addTargetDisease(); 1785 case -1632295686: 1786 return getDoseNumber(); 1787 case -887709242: 1788 return getDoseNumber(); 1789 case 1553560673: 1790 return getSeriesDoses(); 1791 case -1936727105: 1792 return getSeriesDoses(); 1793 default: 1794 return super.makeProperty(hash, name); 1795 } 1796 1797 } 1798 1799 @Override 1800 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1801 switch (hash) { 1802 case -905838985: 1803 /* series */ return new String[] { "string" }; 1804 case 1475610435: 1805 /* authority */ return new String[] { "Reference" }; 1806 case -319593813: 1807 /* targetDisease */ return new String[] { "CodeableConcept" }; 1808 case -887709242: 1809 /* doseNumber */ return new String[] { "positiveInt", "string" }; 1810 case -1936727105: 1811 /* seriesDoses */ return new String[] { "positiveInt", "string" }; 1812 default: 1813 return super.getTypesForProperty(hash, name); 1814 } 1815 1816 } 1817 1818 @Override 1819 public Base addChild(String name) throws FHIRException { 1820 if (name.equals("series")) { 1821 throw new FHIRException("Cannot call addChild on a singleton property Immunization.series"); 1822 } else if (name.equals("authority")) { 1823 this.authority = new Reference(); 1824 return this.authority; 1825 } else if (name.equals("targetDisease")) { 1826 return addTargetDisease(); 1827 } else if (name.equals("doseNumberPositiveInt")) { 1828 this.doseNumber = new PositiveIntType(); 1829 return this.doseNumber; 1830 } else if (name.equals("doseNumberString")) { 1831 this.doseNumber = new StringType(); 1832 return this.doseNumber; 1833 } else if (name.equals("seriesDosesPositiveInt")) { 1834 this.seriesDoses = new PositiveIntType(); 1835 return this.seriesDoses; 1836 } else if (name.equals("seriesDosesString")) { 1837 this.seriesDoses = new StringType(); 1838 return this.seriesDoses; 1839 } else 1840 return super.addChild(name); 1841 } 1842 1843 public ImmunizationProtocolAppliedComponent copy() { 1844 ImmunizationProtocolAppliedComponent dst = new ImmunizationProtocolAppliedComponent(); 1845 copyValues(dst); 1846 return dst; 1847 } 1848 1849 public void copyValues(ImmunizationProtocolAppliedComponent dst) { 1850 super.copyValues(dst); 1851 dst.series = series == null ? null : series.copy(); 1852 dst.authority = authority == null ? null : authority.copy(); 1853 if (targetDisease != null) { 1854 dst.targetDisease = new ArrayList<CodeableConcept>(); 1855 for (CodeableConcept i : targetDisease) 1856 dst.targetDisease.add(i.copy()); 1857 } 1858 ; 1859 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1860 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1861 } 1862 1863 @Override 1864 public boolean equalsDeep(Base other_) { 1865 if (!super.equalsDeep(other_)) 1866 return false; 1867 if (!(other_ instanceof ImmunizationProtocolAppliedComponent)) 1868 return false; 1869 ImmunizationProtocolAppliedComponent o = (ImmunizationProtocolAppliedComponent) other_; 1870 return compareDeep(series, o.series, true) && compareDeep(authority, o.authority, true) 1871 && compareDeep(targetDisease, o.targetDisease, true) && compareDeep(doseNumber, o.doseNumber, true) 1872 && compareDeep(seriesDoses, o.seriesDoses, true); 1873 } 1874 1875 @Override 1876 public boolean equalsShallow(Base other_) { 1877 if (!super.equalsShallow(other_)) 1878 return false; 1879 if (!(other_ instanceof ImmunizationProtocolAppliedComponent)) 1880 return false; 1881 ImmunizationProtocolAppliedComponent o = (ImmunizationProtocolAppliedComponent) other_; 1882 return compareValues(series, o.series, true); 1883 } 1884 1885 public boolean isEmpty() { 1886 return super.isEmpty() 1887 && ca.uhn.fhir.util.ElementUtil.isEmpty(series, authority, targetDisease, doseNumber, seriesDoses); 1888 } 1889 1890 public String fhirType() { 1891 return "Immunization.protocolApplied"; 1892 1893 } 1894 1895 } 1896 1897 /** 1898 * A unique identifier assigned to this immunization record. 1899 */ 1900 @Child(name = "identifier", type = { 1901 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1902 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this immunization record.") 1903 protected List<Identifier> identifier; 1904 1905 /** 1906 * Indicates the current status of the immunization event. 1907 */ 1908 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1909 @Description(shortDefinition = "completed | entered-in-error | not-done", formalDefinition = "Indicates the current status of the immunization event.") 1910 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-status") 1911 protected Enumeration<ImmunizationStatus> status; 1912 1913 /** 1914 * Indicates the reason the immunization event was not performed. 1915 */ 1916 @Child(name = "statusReason", type = { 1917 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1918 @Description(shortDefinition = "Reason not done", formalDefinition = "Indicates the reason the immunization event was not performed.") 1919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-status-reason") 1920 protected CodeableConcept statusReason; 1921 1922 /** 1923 * Vaccine that was administered or was to be administered. 1924 */ 1925 @Child(name = "vaccineCode", type = { 1926 CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1927 @Description(shortDefinition = "Vaccine product administered", formalDefinition = "Vaccine that was administered or was to be administered.") 1928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vaccine-code") 1929 protected CodeableConcept vaccineCode; 1930 1931 /** 1932 * The patient who either received or did not receive the immunization. 1933 */ 1934 @Child(name = "patient", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1935 @Description(shortDefinition = "Who was immunized", formalDefinition = "The patient who either received or did not receive the immunization.") 1936 protected Reference patient; 1937 1938 /** 1939 * The actual object that is the target of the reference (The patient who either 1940 * received or did not receive the immunization.) 1941 */ 1942 protected Patient patientTarget; 1943 1944 /** 1945 * The visit or admission or other contact between patient and health care 1946 * provider the immunization was performed as part of. 1947 */ 1948 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1949 @Description(shortDefinition = "Encounter immunization was part of", formalDefinition = "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.") 1950 protected Reference encounter; 1951 1952 /** 1953 * The actual object that is the target of the reference (The visit or admission 1954 * or other contact between patient and health care provider the immunization 1955 * was performed as part of.) 1956 */ 1957 protected Encounter encounterTarget; 1958 1959 /** 1960 * Date vaccine administered or was to be administered. 1961 */ 1962 @Child(name = "occurrence", type = { DateTimeType.class, 1963 StringType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1964 @Description(shortDefinition = "Vaccine administration date", formalDefinition = "Date vaccine administered or was to be administered.") 1965 protected Type occurrence; 1966 1967 /** 1968 * The date the occurrence of the immunization was first captured in the record 1969 * - potentially significantly after the occurrence of the event. 1970 */ 1971 @Child(name = "recorded", type = { 1972 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1973 @Description(shortDefinition = "When the immunization was first captured in the subject's record", formalDefinition = "The date the occurrence of the immunization was first captured in the record - potentially significantly after the occurrence of the event.") 1974 protected DateTimeType recorded; 1975 1976 /** 1977 * An indication that the content of the record is based on information from the 1978 * person who administered the vaccine. This reflects the context under which 1979 * the data was originally recorded. 1980 */ 1981 @Child(name = "primarySource", type = { 1982 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1983 @Description(shortDefinition = "Indicates context the data was recorded in", formalDefinition = "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.") 1984 protected BooleanType primarySource; 1985 1986 /** 1987 * The source of the data when the report of the immunization event is not based 1988 * on information from the person who administered the vaccine. 1989 */ 1990 @Child(name = "reportOrigin", type = { 1991 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1992 @Description(shortDefinition = "Indicates the source of a secondarily reported record", formalDefinition = "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.") 1993 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-origin") 1994 protected CodeableConcept reportOrigin; 1995 1996 /** 1997 * The service delivery location where the vaccine administration occurred. 1998 */ 1999 @Child(name = "location", type = { Location.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2000 @Description(shortDefinition = "Where immunization occurred", formalDefinition = "The service delivery location where the vaccine administration occurred.") 2001 protected Reference location; 2002 2003 /** 2004 * The actual object that is the target of the reference (The service delivery 2005 * location where the vaccine administration occurred.) 2006 */ 2007 protected Location locationTarget; 2008 2009 /** 2010 * Name of vaccine manufacturer. 2011 */ 2012 @Child(name = "manufacturer", type = { 2013 Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 2014 @Description(shortDefinition = "Vaccine manufacturer", formalDefinition = "Name of vaccine manufacturer.") 2015 protected Reference manufacturer; 2016 2017 /** 2018 * The actual object that is the target of the reference (Name of vaccine 2019 * manufacturer.) 2020 */ 2021 protected Organization manufacturerTarget; 2022 2023 /** 2024 * Lot number of the vaccine product. 2025 */ 2026 @Child(name = "lotNumber", type = { 2027 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2028 @Description(shortDefinition = "Vaccine lot number", formalDefinition = "Lot number of the vaccine product.") 2029 protected StringType lotNumber; 2030 2031 /** 2032 * Date vaccine batch expires. 2033 */ 2034 @Child(name = "expirationDate", type = { 2035 DateType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2036 @Description(shortDefinition = "Vaccine expiration date", formalDefinition = "Date vaccine batch expires.") 2037 protected DateType expirationDate; 2038 2039 /** 2040 * Body site where vaccine was administered. 2041 */ 2042 @Child(name = "site", type = { 2043 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2044 @Description(shortDefinition = "Body site vaccine was administered", formalDefinition = "Body site where vaccine was administered.") 2045 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-site") 2046 protected CodeableConcept site; 2047 2048 /** 2049 * The path by which the vaccine product is taken into the body. 2050 */ 2051 @Child(name = "route", type = { 2052 CodeableConcept.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 2053 @Description(shortDefinition = "How vaccine entered body", formalDefinition = "The path by which the vaccine product is taken into the body.") 2054 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-route") 2055 protected CodeableConcept route; 2056 2057 /** 2058 * The quantity of vaccine product that was administered. 2059 */ 2060 @Child(name = "doseQuantity", type = { 2061 Quantity.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2062 @Description(shortDefinition = "Amount of vaccine administered", formalDefinition = "The quantity of vaccine product that was administered.") 2063 protected Quantity doseQuantity; 2064 2065 /** 2066 * Indicates who performed the immunization event. 2067 */ 2068 @Child(name = "performer", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2069 @Description(shortDefinition = "Who performed event", formalDefinition = "Indicates who performed the immunization event.") 2070 protected List<ImmunizationPerformerComponent> performer; 2071 2072 /** 2073 * Extra information about the immunization that is not conveyed by the other 2074 * attributes. 2075 */ 2076 @Child(name = "note", type = { 2077 Annotation.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2078 @Description(shortDefinition = "Additional immunization notes", formalDefinition = "Extra information about the immunization that is not conveyed by the other attributes.") 2079 protected List<Annotation> note; 2080 2081 /** 2082 * Reasons why the vaccine was administered. 2083 */ 2084 @Child(name = "reasonCode", type = { 2085 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2086 @Description(shortDefinition = "Why immunization occurred", formalDefinition = "Reasons why the vaccine was administered.") 2087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-reason") 2088 protected List<CodeableConcept> reasonCode; 2089 2090 /** 2091 * Condition, Observation or DiagnosticReport that supports why the immunization 2092 * was administered. 2093 */ 2094 @Child(name = "reasonReference", type = { Condition.class, Observation.class, 2095 DiagnosticReport.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2096 @Description(shortDefinition = "Why immunization occurred", formalDefinition = "Condition, Observation or DiagnosticReport that supports why the immunization was administered.") 2097 protected List<Reference> reasonReference; 2098 /** 2099 * The actual objects that are the target of the reference (Condition, 2100 * Observation or DiagnosticReport that supports why the immunization was 2101 * administered.) 2102 */ 2103 protected List<Resource> reasonReferenceTarget; 2104 2105 /** 2106 * Indication if a dose is considered to be subpotent. By default, a dose should 2107 * be considered to be potent. 2108 */ 2109 @Child(name = "isSubpotent", type = { 2110 BooleanType.class }, order = 21, min = 0, max = 1, modifier = true, summary = true) 2111 @Description(shortDefinition = "Dose potency", formalDefinition = "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.") 2112 protected BooleanType isSubpotent; 2113 2114 /** 2115 * Reason why a dose is considered to be subpotent. 2116 */ 2117 @Child(name = "subpotentReason", type = { 2118 CodeableConcept.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2119 @Description(shortDefinition = "Reason for being subpotent", formalDefinition = "Reason why a dose is considered to be subpotent.") 2120 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-subpotent-reason") 2121 protected List<CodeableConcept> subpotentReason; 2122 2123 /** 2124 * Educational material presented to the patient (or guardian) at the time of 2125 * vaccine administration. 2126 */ 2127 @Child(name = "education", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2128 @Description(shortDefinition = "Educational material presented to patient", formalDefinition = "Educational material presented to the patient (or guardian) at the time of vaccine administration.") 2129 protected List<ImmunizationEducationComponent> education; 2130 2131 /** 2132 * Indicates a patient's eligibility for a funding program. 2133 */ 2134 @Child(name = "programEligibility", type = { 2135 CodeableConcept.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2136 @Description(shortDefinition = "Patient eligibility for a vaccination program", formalDefinition = "Indicates a patient's eligibility for a funding program.") 2137 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-program-eligibility") 2138 protected List<CodeableConcept> programEligibility; 2139 2140 /** 2141 * Indicates the source of the vaccine actually administered. This may be 2142 * different than the patient eligibility (e.g. the patient may be eligible for 2143 * a publically purchased vaccine but due to inventory issues, vaccine purchased 2144 * with private funds was actually administered). 2145 */ 2146 @Child(name = "fundingSource", type = { 2147 CodeableConcept.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 2148 @Description(shortDefinition = "Funding source for the vaccine", formalDefinition = "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).") 2149 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-funding-source") 2150 protected CodeableConcept fundingSource; 2151 2152 /** 2153 * Categorical data indicating that an adverse event is associated in time to an 2154 * immunization. 2155 */ 2156 @Child(name = "reaction", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2157 @Description(shortDefinition = "Details of a reaction that follows immunization", formalDefinition = "Categorical data indicating that an adverse event is associated in time to an immunization.") 2158 protected List<ImmunizationReactionComponent> reaction; 2159 2160 /** 2161 * The protocol (set of recommendations) being followed by the provider who 2162 * administered the dose. 2163 */ 2164 @Child(name = "protocolApplied", type = {}, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2165 @Description(shortDefinition = "Protocol followed by the provider", formalDefinition = "The protocol (set of recommendations) being followed by the provider who administered the dose.") 2166 protected List<ImmunizationProtocolAppliedComponent> protocolApplied; 2167 2168 private static final long serialVersionUID = 1946730839L; 2169 2170 /** 2171 * Constructor 2172 */ 2173 public Immunization() { 2174 super(); 2175 } 2176 2177 /** 2178 * Constructor 2179 */ 2180 public Immunization(Enumeration<ImmunizationStatus> status, CodeableConcept vaccineCode, Reference patient, 2181 Type occurrence) { 2182 super(); 2183 this.status = status; 2184 this.vaccineCode = vaccineCode; 2185 this.patient = patient; 2186 this.occurrence = occurrence; 2187 } 2188 2189 /** 2190 * @return {@link #identifier} (A unique identifier assigned to this 2191 * immunization record.) 2192 */ 2193 public List<Identifier> getIdentifier() { 2194 if (this.identifier == null) 2195 this.identifier = new ArrayList<Identifier>(); 2196 return this.identifier; 2197 } 2198 2199 /** 2200 * @return Returns a reference to <code>this</code> for easy method chaining 2201 */ 2202 public Immunization setIdentifier(List<Identifier> theIdentifier) { 2203 this.identifier = theIdentifier; 2204 return this; 2205 } 2206 2207 public boolean hasIdentifier() { 2208 if (this.identifier == null) 2209 return false; 2210 for (Identifier item : this.identifier) 2211 if (!item.isEmpty()) 2212 return true; 2213 return false; 2214 } 2215 2216 public Identifier addIdentifier() { // 3 2217 Identifier t = new Identifier(); 2218 if (this.identifier == null) 2219 this.identifier = new ArrayList<Identifier>(); 2220 this.identifier.add(t); 2221 return t; 2222 } 2223 2224 public Immunization addIdentifier(Identifier t) { // 3 2225 if (t == null) 2226 return this; 2227 if (this.identifier == null) 2228 this.identifier = new ArrayList<Identifier>(); 2229 this.identifier.add(t); 2230 return this; 2231 } 2232 2233 /** 2234 * @return The first repetition of repeating field {@link #identifier}, creating 2235 * it if it does not already exist 2236 */ 2237 public Identifier getIdentifierFirstRep() { 2238 if (getIdentifier().isEmpty()) { 2239 addIdentifier(); 2240 } 2241 return getIdentifier().get(0); 2242 } 2243 2244 /** 2245 * @return {@link #status} (Indicates the current status of the immunization 2246 * event.). This is the underlying object with id, value and extensions. 2247 * The accessor "getStatus" gives direct access to the value 2248 */ 2249 public Enumeration<ImmunizationStatus> getStatusElement() { 2250 if (this.status == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create Immunization.status"); 2253 else if (Configuration.doAutoCreate()) 2254 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); // bb 2255 return this.status; 2256 } 2257 2258 public boolean hasStatusElement() { 2259 return this.status != null && !this.status.isEmpty(); 2260 } 2261 2262 public boolean hasStatus() { 2263 return this.status != null && !this.status.isEmpty(); 2264 } 2265 2266 /** 2267 * @param value {@link #status} (Indicates the current status of the 2268 * immunization event.). This is the underlying object with id, 2269 * value and extensions. The accessor "getStatus" gives direct 2270 * access to the value 2271 */ 2272 public Immunization setStatusElement(Enumeration<ImmunizationStatus> value) { 2273 this.status = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return Indicates the current status of the immunization event. 2279 */ 2280 public ImmunizationStatus getStatus() { 2281 return this.status == null ? null : this.status.getValue(); 2282 } 2283 2284 /** 2285 * @param value Indicates the current status of the immunization event. 2286 */ 2287 public Immunization setStatus(ImmunizationStatus value) { 2288 if (this.status == null) 2289 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); 2290 this.status.setValue(value); 2291 return this; 2292 } 2293 2294 /** 2295 * @return {@link #statusReason} (Indicates the reason the immunization event 2296 * was not performed.) 2297 */ 2298 public CodeableConcept getStatusReason() { 2299 if (this.statusReason == null) 2300 if (Configuration.errorOnAutoCreate()) 2301 throw new Error("Attempt to auto-create Immunization.statusReason"); 2302 else if (Configuration.doAutoCreate()) 2303 this.statusReason = new CodeableConcept(); // cc 2304 return this.statusReason; 2305 } 2306 2307 public boolean hasStatusReason() { 2308 return this.statusReason != null && !this.statusReason.isEmpty(); 2309 } 2310 2311 /** 2312 * @param value {@link #statusReason} (Indicates the reason the immunization 2313 * event was not performed.) 2314 */ 2315 public Immunization setStatusReason(CodeableConcept value) { 2316 this.statusReason = value; 2317 return this; 2318 } 2319 2320 /** 2321 * @return {@link #vaccineCode} (Vaccine that was administered or was to be 2322 * administered.) 2323 */ 2324 public CodeableConcept getVaccineCode() { 2325 if (this.vaccineCode == null) 2326 if (Configuration.errorOnAutoCreate()) 2327 throw new Error("Attempt to auto-create Immunization.vaccineCode"); 2328 else if (Configuration.doAutoCreate()) 2329 this.vaccineCode = new CodeableConcept(); // cc 2330 return this.vaccineCode; 2331 } 2332 2333 public boolean hasVaccineCode() { 2334 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 2335 } 2336 2337 /** 2338 * @param value {@link #vaccineCode} (Vaccine that was administered or was to be 2339 * administered.) 2340 */ 2341 public Immunization setVaccineCode(CodeableConcept value) { 2342 this.vaccineCode = value; 2343 return this; 2344 } 2345 2346 /** 2347 * @return {@link #patient} (The patient who either received or did not receive 2348 * the immunization.) 2349 */ 2350 public Reference getPatient() { 2351 if (this.patient == null) 2352 if (Configuration.errorOnAutoCreate()) 2353 throw new Error("Attempt to auto-create Immunization.patient"); 2354 else if (Configuration.doAutoCreate()) 2355 this.patient = new Reference(); // cc 2356 return this.patient; 2357 } 2358 2359 public boolean hasPatient() { 2360 return this.patient != null && !this.patient.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #patient} (The patient who either received or did not 2365 * receive the immunization.) 2366 */ 2367 public Immunization setPatient(Reference value) { 2368 this.patient = value; 2369 return this; 2370 } 2371 2372 /** 2373 * @return {@link #patient} The actual object that is the target of the 2374 * reference. The reference library doesn't populate this, but you can 2375 * use it to hold the resource if you resolve it. (The patient who 2376 * either received or did not receive the immunization.) 2377 */ 2378 public Patient getPatientTarget() { 2379 if (this.patientTarget == null) 2380 if (Configuration.errorOnAutoCreate()) 2381 throw new Error("Attempt to auto-create Immunization.patient"); 2382 else if (Configuration.doAutoCreate()) 2383 this.patientTarget = new Patient(); // aa 2384 return this.patientTarget; 2385 } 2386 2387 /** 2388 * @param value {@link #patient} The actual object that is the target of the 2389 * reference. The reference library doesn't use these, but you can 2390 * use it to hold the resource if you resolve it. (The patient who 2391 * either received or did not receive the immunization.) 2392 */ 2393 public Immunization setPatientTarget(Patient value) { 2394 this.patientTarget = value; 2395 return this; 2396 } 2397 2398 /** 2399 * @return {@link #encounter} (The visit or admission or other contact between 2400 * patient and health care provider the immunization was performed as 2401 * part of.) 2402 */ 2403 public Reference getEncounter() { 2404 if (this.encounter == null) 2405 if (Configuration.errorOnAutoCreate()) 2406 throw new Error("Attempt to auto-create Immunization.encounter"); 2407 else if (Configuration.doAutoCreate()) 2408 this.encounter = new Reference(); // cc 2409 return this.encounter; 2410 } 2411 2412 public boolean hasEncounter() { 2413 return this.encounter != null && !this.encounter.isEmpty(); 2414 } 2415 2416 /** 2417 * @param value {@link #encounter} (The visit or admission or other contact 2418 * between patient and health care provider the immunization was 2419 * performed as part of.) 2420 */ 2421 public Immunization setEncounter(Reference value) { 2422 this.encounter = value; 2423 return this; 2424 } 2425 2426 /** 2427 * @return {@link #encounter} The actual object that is the target of the 2428 * reference. The reference library doesn't populate this, but you can 2429 * use it to hold the resource if you resolve it. (The visit or 2430 * admission or other contact between patient and health care provider 2431 * the immunization was performed as part of.) 2432 */ 2433 public Encounter getEncounterTarget() { 2434 if (this.encounterTarget == null) 2435 if (Configuration.errorOnAutoCreate()) 2436 throw new Error("Attempt to auto-create Immunization.encounter"); 2437 else if (Configuration.doAutoCreate()) 2438 this.encounterTarget = new Encounter(); // aa 2439 return this.encounterTarget; 2440 } 2441 2442 /** 2443 * @param value {@link #encounter} The actual object that is the target of the 2444 * reference. The reference library doesn't use these, but you can 2445 * use it to hold the resource if you resolve it. (The visit or 2446 * admission or other contact between patient and health care 2447 * provider the immunization was performed as part of.) 2448 */ 2449 public Immunization setEncounterTarget(Encounter value) { 2450 this.encounterTarget = value; 2451 return this; 2452 } 2453 2454 /** 2455 * @return {@link #occurrence} (Date vaccine administered or was to be 2456 * administered.) 2457 */ 2458 public Type getOccurrence() { 2459 return this.occurrence; 2460 } 2461 2462 /** 2463 * @return {@link #occurrence} (Date vaccine administered or was to be 2464 * administered.) 2465 */ 2466 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2467 if (this.occurrence == null) 2468 this.occurrence = new DateTimeType(); 2469 if (!(this.occurrence instanceof DateTimeType)) 2470 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2471 + this.occurrence.getClass().getName() + " was encountered"); 2472 return (DateTimeType) this.occurrence; 2473 } 2474 2475 public boolean hasOccurrenceDateTimeType() { 2476 return this != null && this.occurrence instanceof DateTimeType; 2477 } 2478 2479 /** 2480 * @return {@link #occurrence} (Date vaccine administered or was to be 2481 * administered.) 2482 */ 2483 public StringType getOccurrenceStringType() throws FHIRException { 2484 if (this.occurrence == null) 2485 this.occurrence = new StringType(); 2486 if (!(this.occurrence instanceof StringType)) 2487 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2488 + this.occurrence.getClass().getName() + " was encountered"); 2489 return (StringType) this.occurrence; 2490 } 2491 2492 public boolean hasOccurrenceStringType() { 2493 return this != null && this.occurrence instanceof StringType; 2494 } 2495 2496 public boolean hasOccurrence() { 2497 return this.occurrence != null && !this.occurrence.isEmpty(); 2498 } 2499 2500 /** 2501 * @param value {@link #occurrence} (Date vaccine administered or was to be 2502 * administered.) 2503 */ 2504 public Immunization setOccurrence(Type value) { 2505 if (value != null && !(value instanceof DateTimeType || value instanceof StringType)) 2506 throw new Error("Not the right type for Immunization.occurrence[x]: " + value.fhirType()); 2507 this.occurrence = value; 2508 return this; 2509 } 2510 2511 /** 2512 * @return {@link #recorded} (The date the occurrence of the immunization was 2513 * first captured in the record - potentially significantly after the 2514 * occurrence of the event.). This is the underlying object with id, 2515 * value and extensions. The accessor "getRecorded" gives direct access 2516 * to the value 2517 */ 2518 public DateTimeType getRecordedElement() { 2519 if (this.recorded == null) 2520 if (Configuration.errorOnAutoCreate()) 2521 throw new Error("Attempt to auto-create Immunization.recorded"); 2522 else if (Configuration.doAutoCreate()) 2523 this.recorded = new DateTimeType(); // bb 2524 return this.recorded; 2525 } 2526 2527 public boolean hasRecordedElement() { 2528 return this.recorded != null && !this.recorded.isEmpty(); 2529 } 2530 2531 public boolean hasRecorded() { 2532 return this.recorded != null && !this.recorded.isEmpty(); 2533 } 2534 2535 /** 2536 * @param value {@link #recorded} (The date the occurrence of the immunization 2537 * was first captured in the record - potentially significantly 2538 * after the occurrence of the event.). This is the underlying 2539 * object with id, value and extensions. The accessor "getRecorded" 2540 * gives direct access to the value 2541 */ 2542 public Immunization setRecordedElement(DateTimeType value) { 2543 this.recorded = value; 2544 return this; 2545 } 2546 2547 /** 2548 * @return The date the occurrence of the immunization was first captured in the 2549 * record - potentially significantly after the occurrence of the event. 2550 */ 2551 public Date getRecorded() { 2552 return this.recorded == null ? null : this.recorded.getValue(); 2553 } 2554 2555 /** 2556 * @param value The date the occurrence of the immunization was first captured 2557 * in the record - potentially significantly after the occurrence 2558 * of the event. 2559 */ 2560 public Immunization setRecorded(Date value) { 2561 if (value == null) 2562 this.recorded = null; 2563 else { 2564 if (this.recorded == null) 2565 this.recorded = new DateTimeType(); 2566 this.recorded.setValue(value); 2567 } 2568 return this; 2569 } 2570 2571 /** 2572 * @return {@link #primarySource} (An indication that the content of the record 2573 * is based on information from the person who administered the vaccine. 2574 * This reflects the context under which the data was originally 2575 * recorded.). This is the underlying object with id, value and 2576 * extensions. The accessor "getPrimarySource" gives direct access to 2577 * the value 2578 */ 2579 public BooleanType getPrimarySourceElement() { 2580 if (this.primarySource == null) 2581 if (Configuration.errorOnAutoCreate()) 2582 throw new Error("Attempt to auto-create Immunization.primarySource"); 2583 else if (Configuration.doAutoCreate()) 2584 this.primarySource = new BooleanType(); // bb 2585 return this.primarySource; 2586 } 2587 2588 public boolean hasPrimarySourceElement() { 2589 return this.primarySource != null && !this.primarySource.isEmpty(); 2590 } 2591 2592 public boolean hasPrimarySource() { 2593 return this.primarySource != null && !this.primarySource.isEmpty(); 2594 } 2595 2596 /** 2597 * @param value {@link #primarySource} (An indication that the content of the 2598 * record is based on information from the person who administered 2599 * the vaccine. This reflects the context under which the data was 2600 * originally recorded.). This is the underlying object with id, 2601 * value and extensions. The accessor "getPrimarySource" gives 2602 * direct access to the value 2603 */ 2604 public Immunization setPrimarySourceElement(BooleanType value) { 2605 this.primarySource = value; 2606 return this; 2607 } 2608 2609 /** 2610 * @return An indication that the content of the record is based on information 2611 * from the person who administered the vaccine. This reflects the 2612 * context under which the data was originally recorded. 2613 */ 2614 public boolean getPrimarySource() { 2615 return this.primarySource == null || this.primarySource.isEmpty() ? false : this.primarySource.getValue(); 2616 } 2617 2618 /** 2619 * @param value An indication that the content of the record is based on 2620 * information from the person who administered the vaccine. This 2621 * reflects the context under which the data was originally 2622 * recorded. 2623 */ 2624 public Immunization setPrimarySource(boolean value) { 2625 if (this.primarySource == null) 2626 this.primarySource = new BooleanType(); 2627 this.primarySource.setValue(value); 2628 return this; 2629 } 2630 2631 /** 2632 * @return {@link #reportOrigin} (The source of the data when the report of the 2633 * immunization event is not based on information from the person who 2634 * administered the vaccine.) 2635 */ 2636 public CodeableConcept getReportOrigin() { 2637 if (this.reportOrigin == null) 2638 if (Configuration.errorOnAutoCreate()) 2639 throw new Error("Attempt to auto-create Immunization.reportOrigin"); 2640 else if (Configuration.doAutoCreate()) 2641 this.reportOrigin = new CodeableConcept(); // cc 2642 return this.reportOrigin; 2643 } 2644 2645 public boolean hasReportOrigin() { 2646 return this.reportOrigin != null && !this.reportOrigin.isEmpty(); 2647 } 2648 2649 /** 2650 * @param value {@link #reportOrigin} (The source of the data when the report of 2651 * the immunization event is not based on information from the 2652 * person who administered the vaccine.) 2653 */ 2654 public Immunization setReportOrigin(CodeableConcept value) { 2655 this.reportOrigin = value; 2656 return this; 2657 } 2658 2659 /** 2660 * @return {@link #location} (The service delivery location where the vaccine 2661 * administration occurred.) 2662 */ 2663 public Reference getLocation() { 2664 if (this.location == null) 2665 if (Configuration.errorOnAutoCreate()) 2666 throw new Error("Attempt to auto-create Immunization.location"); 2667 else if (Configuration.doAutoCreate()) 2668 this.location = new Reference(); // cc 2669 return this.location; 2670 } 2671 2672 public boolean hasLocation() { 2673 return this.location != null && !this.location.isEmpty(); 2674 } 2675 2676 /** 2677 * @param value {@link #location} (The service delivery location where the 2678 * vaccine administration occurred.) 2679 */ 2680 public Immunization setLocation(Reference value) { 2681 this.location = value; 2682 return this; 2683 } 2684 2685 /** 2686 * @return {@link #location} The actual object that is the target of the 2687 * reference. The reference library doesn't populate this, but you can 2688 * use it to hold the resource if you resolve it. (The service delivery 2689 * location where the vaccine administration occurred.) 2690 */ 2691 public Location getLocationTarget() { 2692 if (this.locationTarget == null) 2693 if (Configuration.errorOnAutoCreate()) 2694 throw new Error("Attempt to auto-create Immunization.location"); 2695 else if (Configuration.doAutoCreate()) 2696 this.locationTarget = new Location(); // aa 2697 return this.locationTarget; 2698 } 2699 2700 /** 2701 * @param value {@link #location} The actual object that is the target of the 2702 * reference. The reference library doesn't use these, but you can 2703 * use it to hold the resource if you resolve it. (The service 2704 * delivery location where the vaccine administration occurred.) 2705 */ 2706 public Immunization setLocationTarget(Location value) { 2707 this.locationTarget = value; 2708 return this; 2709 } 2710 2711 /** 2712 * @return {@link #manufacturer} (Name of vaccine manufacturer.) 2713 */ 2714 public Reference getManufacturer() { 2715 if (this.manufacturer == null) 2716 if (Configuration.errorOnAutoCreate()) 2717 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2718 else if (Configuration.doAutoCreate()) 2719 this.manufacturer = new Reference(); // cc 2720 return this.manufacturer; 2721 } 2722 2723 public boolean hasManufacturer() { 2724 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2725 } 2726 2727 /** 2728 * @param value {@link #manufacturer} (Name of vaccine manufacturer.) 2729 */ 2730 public Immunization setManufacturer(Reference value) { 2731 this.manufacturer = value; 2732 return this; 2733 } 2734 2735 /** 2736 * @return {@link #manufacturer} The actual object that is the target of the 2737 * reference. The reference library doesn't populate this, but you can 2738 * use it to hold the resource if you resolve it. (Name of vaccine 2739 * manufacturer.) 2740 */ 2741 public Organization getManufacturerTarget() { 2742 if (this.manufacturerTarget == null) 2743 if (Configuration.errorOnAutoCreate()) 2744 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2745 else if (Configuration.doAutoCreate()) 2746 this.manufacturerTarget = new Organization(); // aa 2747 return this.manufacturerTarget; 2748 } 2749 2750 /** 2751 * @param value {@link #manufacturer} The actual object that is the target of 2752 * the reference. The reference library doesn't use these, but you 2753 * can use it to hold the resource if you resolve it. (Name of 2754 * vaccine manufacturer.) 2755 */ 2756 public Immunization setManufacturerTarget(Organization value) { 2757 this.manufacturerTarget = value; 2758 return this; 2759 } 2760 2761 /** 2762 * @return {@link #lotNumber} (Lot number of the vaccine product.). This is the 2763 * underlying object with id, value and extensions. The accessor 2764 * "getLotNumber" gives direct access to the value 2765 */ 2766 public StringType getLotNumberElement() { 2767 if (this.lotNumber == null) 2768 if (Configuration.errorOnAutoCreate()) 2769 throw new Error("Attempt to auto-create Immunization.lotNumber"); 2770 else if (Configuration.doAutoCreate()) 2771 this.lotNumber = new StringType(); // bb 2772 return this.lotNumber; 2773 } 2774 2775 public boolean hasLotNumberElement() { 2776 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2777 } 2778 2779 public boolean hasLotNumber() { 2780 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2781 } 2782 2783 /** 2784 * @param value {@link #lotNumber} (Lot number of the vaccine product.). This is 2785 * the underlying object with id, value and extensions. The 2786 * accessor "getLotNumber" gives direct access to the value 2787 */ 2788 public Immunization setLotNumberElement(StringType value) { 2789 this.lotNumber = value; 2790 return this; 2791 } 2792 2793 /** 2794 * @return Lot number of the vaccine product. 2795 */ 2796 public String getLotNumber() { 2797 return this.lotNumber == null ? null : this.lotNumber.getValue(); 2798 } 2799 2800 /** 2801 * @param value Lot number of the vaccine product. 2802 */ 2803 public Immunization setLotNumber(String value) { 2804 if (Utilities.noString(value)) 2805 this.lotNumber = null; 2806 else { 2807 if (this.lotNumber == null) 2808 this.lotNumber = new StringType(); 2809 this.lotNumber.setValue(value); 2810 } 2811 return this; 2812 } 2813 2814 /** 2815 * @return {@link #expirationDate} (Date vaccine batch expires.). This is the 2816 * underlying object with id, value and extensions. The accessor 2817 * "getExpirationDate" gives direct access to the value 2818 */ 2819 public DateType getExpirationDateElement() { 2820 if (this.expirationDate == null) 2821 if (Configuration.errorOnAutoCreate()) 2822 throw new Error("Attempt to auto-create Immunization.expirationDate"); 2823 else if (Configuration.doAutoCreate()) 2824 this.expirationDate = new DateType(); // bb 2825 return this.expirationDate; 2826 } 2827 2828 public boolean hasExpirationDateElement() { 2829 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2830 } 2831 2832 public boolean hasExpirationDate() { 2833 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2834 } 2835 2836 /** 2837 * @param value {@link #expirationDate} (Date vaccine batch expires.). This is 2838 * the underlying object with id, value and extensions. The 2839 * accessor "getExpirationDate" gives direct access to the value 2840 */ 2841 public Immunization setExpirationDateElement(DateType value) { 2842 this.expirationDate = value; 2843 return this; 2844 } 2845 2846 /** 2847 * @return Date vaccine batch expires. 2848 */ 2849 public Date getExpirationDate() { 2850 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2851 } 2852 2853 /** 2854 * @param value Date vaccine batch expires. 2855 */ 2856 public Immunization setExpirationDate(Date value) { 2857 if (value == null) 2858 this.expirationDate = null; 2859 else { 2860 if (this.expirationDate == null) 2861 this.expirationDate = new DateType(); 2862 this.expirationDate.setValue(value); 2863 } 2864 return this; 2865 } 2866 2867 /** 2868 * @return {@link #site} (Body site where vaccine was administered.) 2869 */ 2870 public CodeableConcept getSite() { 2871 if (this.site == null) 2872 if (Configuration.errorOnAutoCreate()) 2873 throw new Error("Attempt to auto-create Immunization.site"); 2874 else if (Configuration.doAutoCreate()) 2875 this.site = new CodeableConcept(); // cc 2876 return this.site; 2877 } 2878 2879 public boolean hasSite() { 2880 return this.site != null && !this.site.isEmpty(); 2881 } 2882 2883 /** 2884 * @param value {@link #site} (Body site where vaccine was administered.) 2885 */ 2886 public Immunization setSite(CodeableConcept value) { 2887 this.site = value; 2888 return this; 2889 } 2890 2891 /** 2892 * @return {@link #route} (The path by which the vaccine product is taken into 2893 * the body.) 2894 */ 2895 public CodeableConcept getRoute() { 2896 if (this.route == null) 2897 if (Configuration.errorOnAutoCreate()) 2898 throw new Error("Attempt to auto-create Immunization.route"); 2899 else if (Configuration.doAutoCreate()) 2900 this.route = new CodeableConcept(); // cc 2901 return this.route; 2902 } 2903 2904 public boolean hasRoute() { 2905 return this.route != null && !this.route.isEmpty(); 2906 } 2907 2908 /** 2909 * @param value {@link #route} (The path by which the vaccine product is taken 2910 * into the body.) 2911 */ 2912 public Immunization setRoute(CodeableConcept value) { 2913 this.route = value; 2914 return this; 2915 } 2916 2917 /** 2918 * @return {@link #doseQuantity} (The quantity of vaccine product that was 2919 * administered.) 2920 */ 2921 public Quantity getDoseQuantity() { 2922 if (this.doseQuantity == null) 2923 if (Configuration.errorOnAutoCreate()) 2924 throw new Error("Attempt to auto-create Immunization.doseQuantity"); 2925 else if (Configuration.doAutoCreate()) 2926 this.doseQuantity = new Quantity(); // cc 2927 return this.doseQuantity; 2928 } 2929 2930 public boolean hasDoseQuantity() { 2931 return this.doseQuantity != null && !this.doseQuantity.isEmpty(); 2932 } 2933 2934 /** 2935 * @param value {@link #doseQuantity} (The quantity of vaccine product that was 2936 * administered.) 2937 */ 2938 public Immunization setDoseQuantity(Quantity value) { 2939 this.doseQuantity = value; 2940 return this; 2941 } 2942 2943 /** 2944 * @return {@link #performer} (Indicates who performed the immunization event.) 2945 */ 2946 public List<ImmunizationPerformerComponent> getPerformer() { 2947 if (this.performer == null) 2948 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2949 return this.performer; 2950 } 2951 2952 /** 2953 * @return Returns a reference to <code>this</code> for easy method chaining 2954 */ 2955 public Immunization setPerformer(List<ImmunizationPerformerComponent> thePerformer) { 2956 this.performer = thePerformer; 2957 return this; 2958 } 2959 2960 public boolean hasPerformer() { 2961 if (this.performer == null) 2962 return false; 2963 for (ImmunizationPerformerComponent item : this.performer) 2964 if (!item.isEmpty()) 2965 return true; 2966 return false; 2967 } 2968 2969 public ImmunizationPerformerComponent addPerformer() { // 3 2970 ImmunizationPerformerComponent t = new ImmunizationPerformerComponent(); 2971 if (this.performer == null) 2972 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2973 this.performer.add(t); 2974 return t; 2975 } 2976 2977 public Immunization addPerformer(ImmunizationPerformerComponent t) { // 3 2978 if (t == null) 2979 return this; 2980 if (this.performer == null) 2981 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2982 this.performer.add(t); 2983 return this; 2984 } 2985 2986 /** 2987 * @return The first repetition of repeating field {@link #performer}, creating 2988 * it if it does not already exist 2989 */ 2990 public ImmunizationPerformerComponent getPerformerFirstRep() { 2991 if (getPerformer().isEmpty()) { 2992 addPerformer(); 2993 } 2994 return getPerformer().get(0); 2995 } 2996 2997 /** 2998 * @return {@link #note} (Extra information about the immunization that is not 2999 * conveyed by the other attributes.) 3000 */ 3001 public List<Annotation> getNote() { 3002 if (this.note == null) 3003 this.note = new ArrayList<Annotation>(); 3004 return this.note; 3005 } 3006 3007 /** 3008 * @return Returns a reference to <code>this</code> for easy method chaining 3009 */ 3010 public Immunization setNote(List<Annotation> theNote) { 3011 this.note = theNote; 3012 return this; 3013 } 3014 3015 public boolean hasNote() { 3016 if (this.note == null) 3017 return false; 3018 for (Annotation item : this.note) 3019 if (!item.isEmpty()) 3020 return true; 3021 return false; 3022 } 3023 3024 public Annotation addNote() { // 3 3025 Annotation t = new Annotation(); 3026 if (this.note == null) 3027 this.note = new ArrayList<Annotation>(); 3028 this.note.add(t); 3029 return t; 3030 } 3031 3032 public Immunization addNote(Annotation t) { // 3 3033 if (t == null) 3034 return this; 3035 if (this.note == null) 3036 this.note = new ArrayList<Annotation>(); 3037 this.note.add(t); 3038 return this; 3039 } 3040 3041 /** 3042 * @return The first repetition of repeating field {@link #note}, creating it if 3043 * it does not already exist 3044 */ 3045 public Annotation getNoteFirstRep() { 3046 if (getNote().isEmpty()) { 3047 addNote(); 3048 } 3049 return getNote().get(0); 3050 } 3051 3052 /** 3053 * @return {@link #reasonCode} (Reasons why the vaccine was administered.) 3054 */ 3055 public List<CodeableConcept> getReasonCode() { 3056 if (this.reasonCode == null) 3057 this.reasonCode = new ArrayList<CodeableConcept>(); 3058 return this.reasonCode; 3059 } 3060 3061 /** 3062 * @return Returns a reference to <code>this</code> for easy method chaining 3063 */ 3064 public Immunization setReasonCode(List<CodeableConcept> theReasonCode) { 3065 this.reasonCode = theReasonCode; 3066 return this; 3067 } 3068 3069 public boolean hasReasonCode() { 3070 if (this.reasonCode == null) 3071 return false; 3072 for (CodeableConcept item : this.reasonCode) 3073 if (!item.isEmpty()) 3074 return true; 3075 return false; 3076 } 3077 3078 public CodeableConcept addReasonCode() { // 3 3079 CodeableConcept t = new CodeableConcept(); 3080 if (this.reasonCode == null) 3081 this.reasonCode = new ArrayList<CodeableConcept>(); 3082 this.reasonCode.add(t); 3083 return t; 3084 } 3085 3086 public Immunization addReasonCode(CodeableConcept t) { // 3 3087 if (t == null) 3088 return this; 3089 if (this.reasonCode == null) 3090 this.reasonCode = new ArrayList<CodeableConcept>(); 3091 this.reasonCode.add(t); 3092 return this; 3093 } 3094 3095 /** 3096 * @return The first repetition of repeating field {@link #reasonCode}, creating 3097 * it if it does not already exist 3098 */ 3099 public CodeableConcept getReasonCodeFirstRep() { 3100 if (getReasonCode().isEmpty()) { 3101 addReasonCode(); 3102 } 3103 return getReasonCode().get(0); 3104 } 3105 3106 /** 3107 * @return {@link #reasonReference} (Condition, Observation or DiagnosticReport 3108 * that supports why the immunization was administered.) 3109 */ 3110 public List<Reference> getReasonReference() { 3111 if (this.reasonReference == null) 3112 this.reasonReference = new ArrayList<Reference>(); 3113 return this.reasonReference; 3114 } 3115 3116 /** 3117 * @return Returns a reference to <code>this</code> for easy method chaining 3118 */ 3119 public Immunization setReasonReference(List<Reference> theReasonReference) { 3120 this.reasonReference = theReasonReference; 3121 return this; 3122 } 3123 3124 public boolean hasReasonReference() { 3125 if (this.reasonReference == null) 3126 return false; 3127 for (Reference item : this.reasonReference) 3128 if (!item.isEmpty()) 3129 return true; 3130 return false; 3131 } 3132 3133 public Reference addReasonReference() { // 3 3134 Reference t = new Reference(); 3135 if (this.reasonReference == null) 3136 this.reasonReference = new ArrayList<Reference>(); 3137 this.reasonReference.add(t); 3138 return t; 3139 } 3140 3141 public Immunization addReasonReference(Reference t) { // 3 3142 if (t == null) 3143 return this; 3144 if (this.reasonReference == null) 3145 this.reasonReference = new ArrayList<Reference>(); 3146 this.reasonReference.add(t); 3147 return this; 3148 } 3149 3150 /** 3151 * @return The first repetition of repeating field {@link #reasonReference}, 3152 * creating it if it does not already exist 3153 */ 3154 public Reference getReasonReferenceFirstRep() { 3155 if (getReasonReference().isEmpty()) { 3156 addReasonReference(); 3157 } 3158 return getReasonReference().get(0); 3159 } 3160 3161 /** 3162 * @deprecated Use Reference#setResource(IBaseResource) instead 3163 */ 3164 @Deprecated 3165 public List<Resource> getReasonReferenceTarget() { 3166 if (this.reasonReferenceTarget == null) 3167 this.reasonReferenceTarget = new ArrayList<Resource>(); 3168 return this.reasonReferenceTarget; 3169 } 3170 3171 /** 3172 * @return {@link #isSubpotent} (Indication if a dose is considered to be 3173 * subpotent. By default, a dose should be considered to be potent.). 3174 * This is the underlying object with id, value and extensions. The 3175 * accessor "getIsSubpotent" gives direct access to the value 3176 */ 3177 public BooleanType getIsSubpotentElement() { 3178 if (this.isSubpotent == null) 3179 if (Configuration.errorOnAutoCreate()) 3180 throw new Error("Attempt to auto-create Immunization.isSubpotent"); 3181 else if (Configuration.doAutoCreate()) 3182 this.isSubpotent = new BooleanType(); // bb 3183 return this.isSubpotent; 3184 } 3185 3186 public boolean hasIsSubpotentElement() { 3187 return this.isSubpotent != null && !this.isSubpotent.isEmpty(); 3188 } 3189 3190 public boolean hasIsSubpotent() { 3191 return this.isSubpotent != null && !this.isSubpotent.isEmpty(); 3192 } 3193 3194 /** 3195 * @param value {@link #isSubpotent} (Indication if a dose is considered to be 3196 * subpotent. By default, a dose should be considered to be 3197 * potent.). This is the underlying object with id, value and 3198 * extensions. The accessor "getIsSubpotent" gives direct access to 3199 * the value 3200 */ 3201 public Immunization setIsSubpotentElement(BooleanType value) { 3202 this.isSubpotent = value; 3203 return this; 3204 } 3205 3206 /** 3207 * @return Indication if a dose is considered to be subpotent. By default, a 3208 * dose should be considered to be potent. 3209 */ 3210 public boolean getIsSubpotent() { 3211 return this.isSubpotent == null || this.isSubpotent.isEmpty() ? false : this.isSubpotent.getValue(); 3212 } 3213 3214 /** 3215 * @param value Indication if a dose is considered to be subpotent. By default, 3216 * a dose should be considered to be potent. 3217 */ 3218 public Immunization setIsSubpotent(boolean value) { 3219 if (this.isSubpotent == null) 3220 this.isSubpotent = new BooleanType(); 3221 this.isSubpotent.setValue(value); 3222 return this; 3223 } 3224 3225 /** 3226 * @return {@link #subpotentReason} (Reason why a dose is considered to be 3227 * subpotent.) 3228 */ 3229 public List<CodeableConcept> getSubpotentReason() { 3230 if (this.subpotentReason == null) 3231 this.subpotentReason = new ArrayList<CodeableConcept>(); 3232 return this.subpotentReason; 3233 } 3234 3235 /** 3236 * @return Returns a reference to <code>this</code> for easy method chaining 3237 */ 3238 public Immunization setSubpotentReason(List<CodeableConcept> theSubpotentReason) { 3239 this.subpotentReason = theSubpotentReason; 3240 return this; 3241 } 3242 3243 public boolean hasSubpotentReason() { 3244 if (this.subpotentReason == null) 3245 return false; 3246 for (CodeableConcept item : this.subpotentReason) 3247 if (!item.isEmpty()) 3248 return true; 3249 return false; 3250 } 3251 3252 public CodeableConcept addSubpotentReason() { // 3 3253 CodeableConcept t = new CodeableConcept(); 3254 if (this.subpotentReason == null) 3255 this.subpotentReason = new ArrayList<CodeableConcept>(); 3256 this.subpotentReason.add(t); 3257 return t; 3258 } 3259 3260 public Immunization addSubpotentReason(CodeableConcept t) { // 3 3261 if (t == null) 3262 return this; 3263 if (this.subpotentReason == null) 3264 this.subpotentReason = new ArrayList<CodeableConcept>(); 3265 this.subpotentReason.add(t); 3266 return this; 3267 } 3268 3269 /** 3270 * @return The first repetition of repeating field {@link #subpotentReason}, 3271 * creating it if it does not already exist 3272 */ 3273 public CodeableConcept getSubpotentReasonFirstRep() { 3274 if (getSubpotentReason().isEmpty()) { 3275 addSubpotentReason(); 3276 } 3277 return getSubpotentReason().get(0); 3278 } 3279 3280 /** 3281 * @return {@link #education} (Educational material presented to the patient (or 3282 * guardian) at the time of vaccine administration.) 3283 */ 3284 public List<ImmunizationEducationComponent> getEducation() { 3285 if (this.education == null) 3286 this.education = new ArrayList<ImmunizationEducationComponent>(); 3287 return this.education; 3288 } 3289 3290 /** 3291 * @return Returns a reference to <code>this</code> for easy method chaining 3292 */ 3293 public Immunization setEducation(List<ImmunizationEducationComponent> theEducation) { 3294 this.education = theEducation; 3295 return this; 3296 } 3297 3298 public boolean hasEducation() { 3299 if (this.education == null) 3300 return false; 3301 for (ImmunizationEducationComponent item : this.education) 3302 if (!item.isEmpty()) 3303 return true; 3304 return false; 3305 } 3306 3307 public ImmunizationEducationComponent addEducation() { // 3 3308 ImmunizationEducationComponent t = new ImmunizationEducationComponent(); 3309 if (this.education == null) 3310 this.education = new ArrayList<ImmunizationEducationComponent>(); 3311 this.education.add(t); 3312 return t; 3313 } 3314 3315 public Immunization addEducation(ImmunizationEducationComponent t) { // 3 3316 if (t == null) 3317 return this; 3318 if (this.education == null) 3319 this.education = new ArrayList<ImmunizationEducationComponent>(); 3320 this.education.add(t); 3321 return this; 3322 } 3323 3324 /** 3325 * @return The first repetition of repeating field {@link #education}, creating 3326 * it if it does not already exist 3327 */ 3328 public ImmunizationEducationComponent getEducationFirstRep() { 3329 if (getEducation().isEmpty()) { 3330 addEducation(); 3331 } 3332 return getEducation().get(0); 3333 } 3334 3335 /** 3336 * @return {@link #programEligibility} (Indicates a patient's eligibility for a 3337 * funding program.) 3338 */ 3339 public List<CodeableConcept> getProgramEligibility() { 3340 if (this.programEligibility == null) 3341 this.programEligibility = new ArrayList<CodeableConcept>(); 3342 return this.programEligibility; 3343 } 3344 3345 /** 3346 * @return Returns a reference to <code>this</code> for easy method chaining 3347 */ 3348 public Immunization setProgramEligibility(List<CodeableConcept> theProgramEligibility) { 3349 this.programEligibility = theProgramEligibility; 3350 return this; 3351 } 3352 3353 public boolean hasProgramEligibility() { 3354 if (this.programEligibility == null) 3355 return false; 3356 for (CodeableConcept item : this.programEligibility) 3357 if (!item.isEmpty()) 3358 return true; 3359 return false; 3360 } 3361 3362 public CodeableConcept addProgramEligibility() { // 3 3363 CodeableConcept t = new CodeableConcept(); 3364 if (this.programEligibility == null) 3365 this.programEligibility = new ArrayList<CodeableConcept>(); 3366 this.programEligibility.add(t); 3367 return t; 3368 } 3369 3370 public Immunization addProgramEligibility(CodeableConcept t) { // 3 3371 if (t == null) 3372 return this; 3373 if (this.programEligibility == null) 3374 this.programEligibility = new ArrayList<CodeableConcept>(); 3375 this.programEligibility.add(t); 3376 return this; 3377 } 3378 3379 /** 3380 * @return The first repetition of repeating field {@link #programEligibility}, 3381 * creating it if it does not already exist 3382 */ 3383 public CodeableConcept getProgramEligibilityFirstRep() { 3384 if (getProgramEligibility().isEmpty()) { 3385 addProgramEligibility(); 3386 } 3387 return getProgramEligibility().get(0); 3388 } 3389 3390 /** 3391 * @return {@link #fundingSource} (Indicates the source of the vaccine actually 3392 * administered. This may be different than the patient eligibility 3393 * (e.g. the patient may be eligible for a publically purchased vaccine 3394 * but due to inventory issues, vaccine purchased with private funds was 3395 * actually administered).) 3396 */ 3397 public CodeableConcept getFundingSource() { 3398 if (this.fundingSource == null) 3399 if (Configuration.errorOnAutoCreate()) 3400 throw new Error("Attempt to auto-create Immunization.fundingSource"); 3401 else if (Configuration.doAutoCreate()) 3402 this.fundingSource = new CodeableConcept(); // cc 3403 return this.fundingSource; 3404 } 3405 3406 public boolean hasFundingSource() { 3407 return this.fundingSource != null && !this.fundingSource.isEmpty(); 3408 } 3409 3410 /** 3411 * @param value {@link #fundingSource} (Indicates the source of the vaccine 3412 * actually administered. This may be different than the patient 3413 * eligibility (e.g. the patient may be eligible for a publically 3414 * purchased vaccine but due to inventory issues, vaccine purchased 3415 * with private funds was actually administered).) 3416 */ 3417 public Immunization setFundingSource(CodeableConcept value) { 3418 this.fundingSource = value; 3419 return this; 3420 } 3421 3422 /** 3423 * @return {@link #reaction} (Categorical data indicating that an adverse event 3424 * is associated in time to an immunization.) 3425 */ 3426 public List<ImmunizationReactionComponent> getReaction() { 3427 if (this.reaction == null) 3428 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 3429 return this.reaction; 3430 } 3431 3432 /** 3433 * @return Returns a reference to <code>this</code> for easy method chaining 3434 */ 3435 public Immunization setReaction(List<ImmunizationReactionComponent> theReaction) { 3436 this.reaction = theReaction; 3437 return this; 3438 } 3439 3440 public boolean hasReaction() { 3441 if (this.reaction == null) 3442 return false; 3443 for (ImmunizationReactionComponent item : this.reaction) 3444 if (!item.isEmpty()) 3445 return true; 3446 return false; 3447 } 3448 3449 public ImmunizationReactionComponent addReaction() { // 3 3450 ImmunizationReactionComponent t = new ImmunizationReactionComponent(); 3451 if (this.reaction == null) 3452 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 3453 this.reaction.add(t); 3454 return t; 3455 } 3456 3457 public Immunization addReaction(ImmunizationReactionComponent t) { // 3 3458 if (t == null) 3459 return this; 3460 if (this.reaction == null) 3461 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 3462 this.reaction.add(t); 3463 return this; 3464 } 3465 3466 /** 3467 * @return The first repetition of repeating field {@link #reaction}, creating 3468 * it if it does not already exist 3469 */ 3470 public ImmunizationReactionComponent getReactionFirstRep() { 3471 if (getReaction().isEmpty()) { 3472 addReaction(); 3473 } 3474 return getReaction().get(0); 3475 } 3476 3477 /** 3478 * @return {@link #protocolApplied} (The protocol (set of recommendations) being 3479 * followed by the provider who administered the dose.) 3480 */ 3481 public List<ImmunizationProtocolAppliedComponent> getProtocolApplied() { 3482 if (this.protocolApplied == null) 3483 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3484 return this.protocolApplied; 3485 } 3486 3487 /** 3488 * @return Returns a reference to <code>this</code> for easy method chaining 3489 */ 3490 public Immunization setProtocolApplied(List<ImmunizationProtocolAppliedComponent> theProtocolApplied) { 3491 this.protocolApplied = theProtocolApplied; 3492 return this; 3493 } 3494 3495 public boolean hasProtocolApplied() { 3496 if (this.protocolApplied == null) 3497 return false; 3498 for (ImmunizationProtocolAppliedComponent item : this.protocolApplied) 3499 if (!item.isEmpty()) 3500 return true; 3501 return false; 3502 } 3503 3504 public ImmunizationProtocolAppliedComponent addProtocolApplied() { // 3 3505 ImmunizationProtocolAppliedComponent t = new ImmunizationProtocolAppliedComponent(); 3506 if (this.protocolApplied == null) 3507 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3508 this.protocolApplied.add(t); 3509 return t; 3510 } 3511 3512 public Immunization addProtocolApplied(ImmunizationProtocolAppliedComponent t) { // 3 3513 if (t == null) 3514 return this; 3515 if (this.protocolApplied == null) 3516 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3517 this.protocolApplied.add(t); 3518 return this; 3519 } 3520 3521 /** 3522 * @return The first repetition of repeating field {@link #protocolApplied}, 3523 * creating it if it does not already exist 3524 */ 3525 public ImmunizationProtocolAppliedComponent getProtocolAppliedFirstRep() { 3526 if (getProtocolApplied().isEmpty()) { 3527 addProtocolApplied(); 3528 } 3529 return getProtocolApplied().get(0); 3530 } 3531 3532 protected void listChildren(List<Property> children) { 3533 super.listChildren(children); 3534 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 3535 0, java.lang.Integer.MAX_VALUE, identifier)); 3536 children 3537 .add(new Property("status", "code", "Indicates the current status of the immunization event.", 0, 1, status)); 3538 children.add(new Property("statusReason", "CodeableConcept", 3539 "Indicates the reason the immunization event was not performed.", 0, 1, statusReason)); 3540 children.add(new Property("vaccineCode", "CodeableConcept", 3541 "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode)); 3542 children.add(new Property("patient", "Reference(Patient)", 3543 "The patient who either received or did not receive the immunization.", 0, 1, patient)); 3544 children.add(new Property("encounter", "Reference(Encounter)", 3545 "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 3546 0, 1, encounter)); 3547 children.add(new Property("occurrence[x]", "dateTime|string", 3548 "Date vaccine administered or was to be administered.", 0, 1, occurrence)); 3549 children.add(new Property("recorded", "dateTime", 3550 "The date the occurrence of the immunization was first captured in the record - potentially significantly after the occurrence of the event.", 3551 0, 1, recorded)); 3552 children.add(new Property("primarySource", "boolean", 3553 "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 3554 0, 1, primarySource)); 3555 children.add(new Property("reportOrigin", "CodeableConcept", 3556 "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 3557 0, 1, reportOrigin)); 3558 children.add(new Property("location", "Reference(Location)", 3559 "The service delivery location where the vaccine administration occurred.", 0, 1, location)); 3560 children.add( 3561 new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer)); 3562 children.add(new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber)); 3563 children.add(new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate)); 3564 children.add(new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site)); 3565 children.add(new Property("route", "CodeableConcept", 3566 "The path by which the vaccine product is taken into the body.", 0, 1, route)); 3567 children.add(new Property("doseQuantity", "SimpleQuantity", 3568 "The quantity of vaccine product that was administered.", 0, 1, doseQuantity)); 3569 children.add(new Property("performer", "", "Indicates who performed the immunization event.", 0, 3570 java.lang.Integer.MAX_VALUE, performer)); 3571 children.add(new Property("note", "Annotation", 3572 "Extra information about the immunization that is not conveyed by the other attributes.", 0, 3573 java.lang.Integer.MAX_VALUE, note)); 3574 children.add(new Property("reasonCode", "CodeableConcept", "Reasons why the vaccine was administered.", 0, 3575 java.lang.Integer.MAX_VALUE, reasonCode)); 3576 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 3577 "Condition, Observation or DiagnosticReport that supports why the immunization was administered.", 0, 3578 java.lang.Integer.MAX_VALUE, reasonReference)); 3579 children.add(new Property("isSubpotent", "boolean", 3580 "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.", 0, 3581 1, isSubpotent)); 3582 children.add(new Property("subpotentReason", "CodeableConcept", "Reason why a dose is considered to be subpotent.", 3583 0, java.lang.Integer.MAX_VALUE, subpotentReason)); 3584 children.add(new Property("education", "", 3585 "Educational material presented to the patient (or guardian) at the time of vaccine administration.", 0, 3586 java.lang.Integer.MAX_VALUE, education)); 3587 children.add(new Property("programEligibility", "CodeableConcept", 3588 "Indicates a patient's eligibility for a funding program.", 0, java.lang.Integer.MAX_VALUE, 3589 programEligibility)); 3590 children.add(new Property("fundingSource", "CodeableConcept", 3591 "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).", 3592 0, 1, fundingSource)); 3593 children.add(new Property("reaction", "", 3594 "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, 3595 java.lang.Integer.MAX_VALUE, reaction)); 3596 children.add(new Property("protocolApplied", "", 3597 "The protocol (set of recommendations) being followed by the provider who administered the dose.", 0, 3598 java.lang.Integer.MAX_VALUE, protocolApplied)); 3599 } 3600 3601 @Override 3602 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3603 switch (_hash) { 3604 case -1618432855: 3605 /* identifier */ return new Property("identifier", "Identifier", 3606 "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier); 3607 case -892481550: 3608 /* status */ return new Property("status", "code", "Indicates the current status of the immunization event.", 0, 3609 1, status); 3610 case 2051346646: 3611 /* statusReason */ return new Property("statusReason", "CodeableConcept", 3612 "Indicates the reason the immunization event was not performed.", 0, 1, statusReason); 3613 case 664556354: 3614 /* vaccineCode */ return new Property("vaccineCode", "CodeableConcept", 3615 "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode); 3616 case -791418107: 3617 /* patient */ return new Property("patient", "Reference(Patient)", 3618 "The patient who either received or did not receive the immunization.", 0, 1, patient); 3619 case 1524132147: 3620 /* encounter */ return new Property("encounter", "Reference(Encounter)", 3621 "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 3622 0, 1, encounter); 3623 case -2022646513: 3624 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|string", 3625 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3626 case 1687874001: 3627 /* occurrence */ return new Property("occurrence[x]", "dateTime|string", 3628 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3629 case -298443636: 3630 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|string", 3631 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3632 case 1496896834: 3633 /* occurrenceString */ return new Property("occurrence[x]", "dateTime|string", 3634 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3635 case -799233872: 3636 /* recorded */ return new Property("recorded", "dateTime", 3637 "The date the occurrence of the immunization was first captured in the record - potentially significantly after the occurrence of the event.", 3638 0, 1, recorded); 3639 case -528721731: 3640 /* primarySource */ return new Property("primarySource", "boolean", 3641 "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 3642 0, 1, primarySource); 3643 case 486750586: 3644 /* reportOrigin */ return new Property("reportOrigin", "CodeableConcept", 3645 "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 3646 0, 1, reportOrigin); 3647 case 1901043637: 3648 /* location */ return new Property("location", "Reference(Location)", 3649 "The service delivery location where the vaccine administration occurred.", 0, 1, location); 3650 case -1969347631: 3651 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 3652 0, 1, manufacturer); 3653 case 462547450: 3654 /* lotNumber */ return new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, 3655 lotNumber); 3656 case -668811523: 3657 /* expirationDate */ return new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, 3658 expirationDate); 3659 case 3530567: 3660 /* site */ return new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, 3661 site); 3662 case 108704329: 3663 /* route */ return new Property("route", "CodeableConcept", 3664 "The path by which the vaccine product is taken into the body.", 0, 1, route); 3665 case -2083618872: 3666 /* doseQuantity */ return new Property("doseQuantity", "SimpleQuantity", 3667 "The quantity of vaccine product that was administered.", 0, 1, doseQuantity); 3668 case 481140686: 3669 /* performer */ return new Property("performer", "", "Indicates who performed the immunization event.", 0, 3670 java.lang.Integer.MAX_VALUE, performer); 3671 case 3387378: 3672 /* note */ return new Property("note", "Annotation", 3673 "Extra information about the immunization that is not conveyed by the other attributes.", 0, 3674 java.lang.Integer.MAX_VALUE, note); 3675 case 722137681: 3676 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", "Reasons why the vaccine was administered.", 3677 0, java.lang.Integer.MAX_VALUE, reasonCode); 3678 case -1146218137: 3679 /* reasonReference */ return new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 3680 "Condition, Observation or DiagnosticReport that supports why the immunization was administered.", 0, 3681 java.lang.Integer.MAX_VALUE, reasonReference); 3682 case 1618512556: 3683 /* isSubpotent */ return new Property("isSubpotent", "boolean", 3684 "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.", 3685 0, 1, isSubpotent); 3686 case 805168794: 3687 /* subpotentReason */ return new Property("subpotentReason", "CodeableConcept", 3688 "Reason why a dose is considered to be subpotent.", 0, java.lang.Integer.MAX_VALUE, subpotentReason); 3689 case -290756696: 3690 /* education */ return new Property("education", "", 3691 "Educational material presented to the patient (or guardian) at the time of vaccine administration.", 0, 3692 java.lang.Integer.MAX_VALUE, education); 3693 case 1207530089: 3694 /* programEligibility */ return new Property("programEligibility", "CodeableConcept", 3695 "Indicates a patient's eligibility for a funding program.", 0, java.lang.Integer.MAX_VALUE, 3696 programEligibility); 3697 case 1120150904: 3698 /* fundingSource */ return new Property("fundingSource", "CodeableConcept", 3699 "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).", 3700 0, 1, fundingSource); 3701 case -867509719: 3702 /* reaction */ return new Property("reaction", "", 3703 "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, 3704 java.lang.Integer.MAX_VALUE, reaction); 3705 case 607985349: 3706 /* protocolApplied */ return new Property("protocolApplied", "", 3707 "The protocol (set of recommendations) being followed by the provider who administered the dose.", 0, 3708 java.lang.Integer.MAX_VALUE, protocolApplied); 3709 default: 3710 return super.getNamedProperty(_hash, _name, _checkValid); 3711 } 3712 3713 } 3714 3715 @Override 3716 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3717 switch (hash) { 3718 case -1618432855: 3719 /* identifier */ return this.identifier == null ? new Base[0] 3720 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3721 case -892481550: 3722 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ImmunizationStatus> 3723 case 2051346646: 3724 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 3725 case 664556354: 3726 /* vaccineCode */ return this.vaccineCode == null ? new Base[0] : new Base[] { this.vaccineCode }; // CodeableConcept 3727 case -791418107: 3728 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 3729 case 1524132147: 3730 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3731 case 1687874001: 3732 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 3733 case -799233872: 3734 /* recorded */ return this.recorded == null ? new Base[0] : new Base[] { this.recorded }; // DateTimeType 3735 case -528721731: 3736 /* primarySource */ return this.primarySource == null ? new Base[0] : new Base[] { this.primarySource }; // BooleanType 3737 case 486750586: 3738 /* reportOrigin */ return this.reportOrigin == null ? new Base[0] : new Base[] { this.reportOrigin }; // CodeableConcept 3739 case 1901043637: 3740 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 3741 case -1969347631: 3742 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // Reference 3743 case 462547450: 3744 /* lotNumber */ return this.lotNumber == null ? new Base[0] : new Base[] { this.lotNumber }; // StringType 3745 case -668811523: 3746 /* expirationDate */ return this.expirationDate == null ? new Base[0] : new Base[] { this.expirationDate }; // DateType 3747 case 3530567: 3748 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // CodeableConcept 3749 case 108704329: 3750 /* route */ return this.route == null ? new Base[0] : new Base[] { this.route }; // CodeableConcept 3751 case -2083618872: 3752 /* doseQuantity */ return this.doseQuantity == null ? new Base[0] : new Base[] { this.doseQuantity }; // Quantity 3753 case 481140686: 3754 /* performer */ return this.performer == null ? new Base[0] 3755 : this.performer.toArray(new Base[this.performer.size()]); // ImmunizationPerformerComponent 3756 case 3387378: 3757 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3758 case 722137681: 3759 /* reasonCode */ return this.reasonCode == null ? new Base[0] 3760 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 3761 case -1146218137: 3762 /* reasonReference */ return this.reasonReference == null ? new Base[0] 3763 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 3764 case 1618512556: 3765 /* isSubpotent */ return this.isSubpotent == null ? new Base[0] : new Base[] { this.isSubpotent }; // BooleanType 3766 case 805168794: 3767 /* subpotentReason */ return this.subpotentReason == null ? new Base[0] 3768 : this.subpotentReason.toArray(new Base[this.subpotentReason.size()]); // CodeableConcept 3769 case -290756696: 3770 /* education */ return this.education == null ? new Base[0] 3771 : this.education.toArray(new Base[this.education.size()]); // ImmunizationEducationComponent 3772 case 1207530089: 3773 /* programEligibility */ return this.programEligibility == null ? new Base[0] 3774 : this.programEligibility.toArray(new Base[this.programEligibility.size()]); // CodeableConcept 3775 case 1120150904: 3776 /* fundingSource */ return this.fundingSource == null ? new Base[0] : new Base[] { this.fundingSource }; // CodeableConcept 3777 case -867509719: 3778 /* reaction */ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // ImmunizationReactionComponent 3779 case 607985349: 3780 /* protocolApplied */ return this.protocolApplied == null ? new Base[0] 3781 : this.protocolApplied.toArray(new Base[this.protocolApplied.size()]); // ImmunizationProtocolAppliedComponent 3782 default: 3783 return super.getProperty(hash, name, checkValid); 3784 } 3785 3786 } 3787 3788 @Override 3789 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3790 switch (hash) { 3791 case -1618432855: // identifier 3792 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3793 return value; 3794 case -892481550: // status 3795 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 3796 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 3797 return value; 3798 case 2051346646: // statusReason 3799 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3800 return value; 3801 case 664556354: // vaccineCode 3802 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 3803 return value; 3804 case -791418107: // patient 3805 this.patient = castToReference(value); // Reference 3806 return value; 3807 case 1524132147: // encounter 3808 this.encounter = castToReference(value); // Reference 3809 return value; 3810 case 1687874001: // occurrence 3811 this.occurrence = castToType(value); // Type 3812 return value; 3813 case -799233872: // recorded 3814 this.recorded = castToDateTime(value); // DateTimeType 3815 return value; 3816 case -528721731: // primarySource 3817 this.primarySource = castToBoolean(value); // BooleanType 3818 return value; 3819 case 486750586: // reportOrigin 3820 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 3821 return value; 3822 case 1901043637: // location 3823 this.location = castToReference(value); // Reference 3824 return value; 3825 case -1969347631: // manufacturer 3826 this.manufacturer = castToReference(value); // Reference 3827 return value; 3828 case 462547450: // lotNumber 3829 this.lotNumber = castToString(value); // StringType 3830 return value; 3831 case -668811523: // expirationDate 3832 this.expirationDate = castToDate(value); // DateType 3833 return value; 3834 case 3530567: // site 3835 this.site = castToCodeableConcept(value); // CodeableConcept 3836 return value; 3837 case 108704329: // route 3838 this.route = castToCodeableConcept(value); // CodeableConcept 3839 return value; 3840 case -2083618872: // doseQuantity 3841 this.doseQuantity = castToQuantity(value); // Quantity 3842 return value; 3843 case 481140686: // performer 3844 this.getPerformer().add((ImmunizationPerformerComponent) value); // ImmunizationPerformerComponent 3845 return value; 3846 case 3387378: // note 3847 this.getNote().add(castToAnnotation(value)); // Annotation 3848 return value; 3849 case 722137681: // reasonCode 3850 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 3851 return value; 3852 case -1146218137: // reasonReference 3853 this.getReasonReference().add(castToReference(value)); // Reference 3854 return value; 3855 case 1618512556: // isSubpotent 3856 this.isSubpotent = castToBoolean(value); // BooleanType 3857 return value; 3858 case 805168794: // subpotentReason 3859 this.getSubpotentReason().add(castToCodeableConcept(value)); // CodeableConcept 3860 return value; 3861 case -290756696: // education 3862 this.getEducation().add((ImmunizationEducationComponent) value); // ImmunizationEducationComponent 3863 return value; 3864 case 1207530089: // programEligibility 3865 this.getProgramEligibility().add(castToCodeableConcept(value)); // CodeableConcept 3866 return value; 3867 case 1120150904: // fundingSource 3868 this.fundingSource = castToCodeableConcept(value); // CodeableConcept 3869 return value; 3870 case -867509719: // reaction 3871 this.getReaction().add((ImmunizationReactionComponent) value); // ImmunizationReactionComponent 3872 return value; 3873 case 607985349: // protocolApplied 3874 this.getProtocolApplied().add((ImmunizationProtocolAppliedComponent) value); // ImmunizationProtocolAppliedComponent 3875 return value; 3876 default: 3877 return super.setProperty(hash, name, value); 3878 } 3879 3880 } 3881 3882 @Override 3883 public Base setProperty(String name, Base value) throws FHIRException { 3884 if (name.equals("identifier")) { 3885 this.getIdentifier().add(castToIdentifier(value)); 3886 } else if (name.equals("status")) { 3887 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 3888 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 3889 } else if (name.equals("statusReason")) { 3890 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3891 } else if (name.equals("vaccineCode")) { 3892 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 3893 } else if (name.equals("patient")) { 3894 this.patient = castToReference(value); // Reference 3895 } else if (name.equals("encounter")) { 3896 this.encounter = castToReference(value); // Reference 3897 } else if (name.equals("occurrence[x]")) { 3898 this.occurrence = castToType(value); // Type 3899 } else if (name.equals("recorded")) { 3900 this.recorded = castToDateTime(value); // DateTimeType 3901 } else if (name.equals("primarySource")) { 3902 this.primarySource = castToBoolean(value); // BooleanType 3903 } else if (name.equals("reportOrigin")) { 3904 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 3905 } else if (name.equals("location")) { 3906 this.location = castToReference(value); // Reference 3907 } else if (name.equals("manufacturer")) { 3908 this.manufacturer = castToReference(value); // Reference 3909 } else if (name.equals("lotNumber")) { 3910 this.lotNumber = castToString(value); // StringType 3911 } else if (name.equals("expirationDate")) { 3912 this.expirationDate = castToDate(value); // DateType 3913 } else if (name.equals("site")) { 3914 this.site = castToCodeableConcept(value); // CodeableConcept 3915 } else if (name.equals("route")) { 3916 this.route = castToCodeableConcept(value); // CodeableConcept 3917 } else if (name.equals("doseQuantity")) { 3918 this.doseQuantity = castToQuantity(value); // Quantity 3919 } else if (name.equals("performer")) { 3920 this.getPerformer().add((ImmunizationPerformerComponent) value); 3921 } else if (name.equals("note")) { 3922 this.getNote().add(castToAnnotation(value)); 3923 } else if (name.equals("reasonCode")) { 3924 this.getReasonCode().add(castToCodeableConcept(value)); 3925 } else if (name.equals("reasonReference")) { 3926 this.getReasonReference().add(castToReference(value)); 3927 } else if (name.equals("isSubpotent")) { 3928 this.isSubpotent = castToBoolean(value); // BooleanType 3929 } else if (name.equals("subpotentReason")) { 3930 this.getSubpotentReason().add(castToCodeableConcept(value)); 3931 } else if (name.equals("education")) { 3932 this.getEducation().add((ImmunizationEducationComponent) value); 3933 } else if (name.equals("programEligibility")) { 3934 this.getProgramEligibility().add(castToCodeableConcept(value)); 3935 } else if (name.equals("fundingSource")) { 3936 this.fundingSource = castToCodeableConcept(value); // CodeableConcept 3937 } else if (name.equals("reaction")) { 3938 this.getReaction().add((ImmunizationReactionComponent) value); 3939 } else if (name.equals("protocolApplied")) { 3940 this.getProtocolApplied().add((ImmunizationProtocolAppliedComponent) value); 3941 } else 3942 return super.setProperty(name, value); 3943 return value; 3944 } 3945 3946 @Override 3947 public void removeChild(String name, Base value) throws FHIRException { 3948 if (name.equals("identifier")) { 3949 this.getIdentifier().remove(castToIdentifier(value)); 3950 } else if (name.equals("status")) { 3951 this.status = null; 3952 } else if (name.equals("statusReason")) { 3953 this.statusReason = null; 3954 } else if (name.equals("vaccineCode")) { 3955 this.vaccineCode = null; 3956 } else if (name.equals("patient")) { 3957 this.patient = null; 3958 } else if (name.equals("encounter")) { 3959 this.encounter = null; 3960 } else if (name.equals("occurrence[x]")) { 3961 this.occurrence = null; 3962 } else if (name.equals("recorded")) { 3963 this.recorded = null; 3964 } else if (name.equals("primarySource")) { 3965 this.primarySource = null; 3966 } else if (name.equals("reportOrigin")) { 3967 this.reportOrigin = null; 3968 } else if (name.equals("location")) { 3969 this.location = null; 3970 } else if (name.equals("manufacturer")) { 3971 this.manufacturer = null; 3972 } else if (name.equals("lotNumber")) { 3973 this.lotNumber = null; 3974 } else if (name.equals("expirationDate")) { 3975 this.expirationDate = null; 3976 } else if (name.equals("site")) { 3977 this.site = null; 3978 } else if (name.equals("route")) { 3979 this.route = null; 3980 } else if (name.equals("doseQuantity")) { 3981 this.doseQuantity = null; 3982 } else if (name.equals("performer")) { 3983 this.getPerformer().remove((ImmunizationPerformerComponent) value); 3984 } else if (name.equals("note")) { 3985 this.getNote().remove(castToAnnotation(value)); 3986 } else if (name.equals("reasonCode")) { 3987 this.getReasonCode().remove(castToCodeableConcept(value)); 3988 } else if (name.equals("reasonReference")) { 3989 this.getReasonReference().remove(castToReference(value)); 3990 } else if (name.equals("isSubpotent")) { 3991 this.isSubpotent = null; 3992 } else if (name.equals("subpotentReason")) { 3993 this.getSubpotentReason().remove(castToCodeableConcept(value)); 3994 } else if (name.equals("education")) { 3995 this.getEducation().remove((ImmunizationEducationComponent) value); 3996 } else if (name.equals("programEligibility")) { 3997 this.getProgramEligibility().remove(castToCodeableConcept(value)); 3998 } else if (name.equals("fundingSource")) { 3999 this.fundingSource = null; 4000 } else if (name.equals("reaction")) { 4001 this.getReaction().remove((ImmunizationReactionComponent) value); 4002 } else if (name.equals("protocolApplied")) { 4003 this.getProtocolApplied().remove((ImmunizationProtocolAppliedComponent) value); 4004 } else 4005 super.removeChild(name, value); 4006 4007 } 4008 4009 @Override 4010 public Base makeProperty(int hash, String name) throws FHIRException { 4011 switch (hash) { 4012 case -1618432855: 4013 return addIdentifier(); 4014 case -892481550: 4015 return getStatusElement(); 4016 case 2051346646: 4017 return getStatusReason(); 4018 case 664556354: 4019 return getVaccineCode(); 4020 case -791418107: 4021 return getPatient(); 4022 case 1524132147: 4023 return getEncounter(); 4024 case -2022646513: 4025 return getOccurrence(); 4026 case 1687874001: 4027 return getOccurrence(); 4028 case -799233872: 4029 return getRecordedElement(); 4030 case -528721731: 4031 return getPrimarySourceElement(); 4032 case 486750586: 4033 return getReportOrigin(); 4034 case 1901043637: 4035 return getLocation(); 4036 case -1969347631: 4037 return getManufacturer(); 4038 case 462547450: 4039 return getLotNumberElement(); 4040 case -668811523: 4041 return getExpirationDateElement(); 4042 case 3530567: 4043 return getSite(); 4044 case 108704329: 4045 return getRoute(); 4046 case -2083618872: 4047 return getDoseQuantity(); 4048 case 481140686: 4049 return addPerformer(); 4050 case 3387378: 4051 return addNote(); 4052 case 722137681: 4053 return addReasonCode(); 4054 case -1146218137: 4055 return addReasonReference(); 4056 case 1618512556: 4057 return getIsSubpotentElement(); 4058 case 805168794: 4059 return addSubpotentReason(); 4060 case -290756696: 4061 return addEducation(); 4062 case 1207530089: 4063 return addProgramEligibility(); 4064 case 1120150904: 4065 return getFundingSource(); 4066 case -867509719: 4067 return addReaction(); 4068 case 607985349: 4069 return addProtocolApplied(); 4070 default: 4071 return super.makeProperty(hash, name); 4072 } 4073 4074 } 4075 4076 @Override 4077 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4078 switch (hash) { 4079 case -1618432855: 4080 /* identifier */ return new String[] { "Identifier" }; 4081 case -892481550: 4082 /* status */ return new String[] { "code" }; 4083 case 2051346646: 4084 /* statusReason */ return new String[] { "CodeableConcept" }; 4085 case 664556354: 4086 /* vaccineCode */ return new String[] { "CodeableConcept" }; 4087 case -791418107: 4088 /* patient */ return new String[] { "Reference" }; 4089 case 1524132147: 4090 /* encounter */ return new String[] { "Reference" }; 4091 case 1687874001: 4092 /* occurrence */ return new String[] { "dateTime", "string" }; 4093 case -799233872: 4094 /* recorded */ return new String[] { "dateTime" }; 4095 case -528721731: 4096 /* primarySource */ return new String[] { "boolean" }; 4097 case 486750586: 4098 /* reportOrigin */ return new String[] { "CodeableConcept" }; 4099 case 1901043637: 4100 /* location */ return new String[] { "Reference" }; 4101 case -1969347631: 4102 /* manufacturer */ return new String[] { "Reference" }; 4103 case 462547450: 4104 /* lotNumber */ return new String[] { "string" }; 4105 case -668811523: 4106 /* expirationDate */ return new String[] { "date" }; 4107 case 3530567: 4108 /* site */ return new String[] { "CodeableConcept" }; 4109 case 108704329: 4110 /* route */ return new String[] { "CodeableConcept" }; 4111 case -2083618872: 4112 /* doseQuantity */ return new String[] { "SimpleQuantity" }; 4113 case 481140686: 4114 /* performer */ return new String[] {}; 4115 case 3387378: 4116 /* note */ return new String[] { "Annotation" }; 4117 case 722137681: 4118 /* reasonCode */ return new String[] { "CodeableConcept" }; 4119 case -1146218137: 4120 /* reasonReference */ return new String[] { "Reference" }; 4121 case 1618512556: 4122 /* isSubpotent */ return new String[] { "boolean" }; 4123 case 805168794: 4124 /* subpotentReason */ return new String[] { "CodeableConcept" }; 4125 case -290756696: 4126 /* education */ return new String[] {}; 4127 case 1207530089: 4128 /* programEligibility */ return new String[] { "CodeableConcept" }; 4129 case 1120150904: 4130 /* fundingSource */ return new String[] { "CodeableConcept" }; 4131 case -867509719: 4132 /* reaction */ return new String[] {}; 4133 case 607985349: 4134 /* protocolApplied */ return new String[] {}; 4135 default: 4136 return super.getTypesForProperty(hash, name); 4137 } 4138 4139 } 4140 4141 @Override 4142 public Base addChild(String name) throws FHIRException { 4143 if (name.equals("identifier")) { 4144 return addIdentifier(); 4145 } else if (name.equals("status")) { 4146 throw new FHIRException("Cannot call addChild on a singleton property Immunization.status"); 4147 } else if (name.equals("statusReason")) { 4148 this.statusReason = new CodeableConcept(); 4149 return this.statusReason; 4150 } else if (name.equals("vaccineCode")) { 4151 this.vaccineCode = new CodeableConcept(); 4152 return this.vaccineCode; 4153 } else if (name.equals("patient")) { 4154 this.patient = new Reference(); 4155 return this.patient; 4156 } else if (name.equals("encounter")) { 4157 this.encounter = new Reference(); 4158 return this.encounter; 4159 } else if (name.equals("occurrenceDateTime")) { 4160 this.occurrence = new DateTimeType(); 4161 return this.occurrence; 4162 } else if (name.equals("occurrenceString")) { 4163 this.occurrence = new StringType(); 4164 return this.occurrence; 4165 } else if (name.equals("recorded")) { 4166 throw new FHIRException("Cannot call addChild on a singleton property Immunization.recorded"); 4167 } else if (name.equals("primarySource")) { 4168 throw new FHIRException("Cannot call addChild on a singleton property Immunization.primarySource"); 4169 } else if (name.equals("reportOrigin")) { 4170 this.reportOrigin = new CodeableConcept(); 4171 return this.reportOrigin; 4172 } else if (name.equals("location")) { 4173 this.location = new Reference(); 4174 return this.location; 4175 } else if (name.equals("manufacturer")) { 4176 this.manufacturer = new Reference(); 4177 return this.manufacturer; 4178 } else if (name.equals("lotNumber")) { 4179 throw new FHIRException("Cannot call addChild on a singleton property Immunization.lotNumber"); 4180 } else if (name.equals("expirationDate")) { 4181 throw new FHIRException("Cannot call addChild on a singleton property Immunization.expirationDate"); 4182 } else if (name.equals("site")) { 4183 this.site = new CodeableConcept(); 4184 return this.site; 4185 } else if (name.equals("route")) { 4186 this.route = new CodeableConcept(); 4187 return this.route; 4188 } else if (name.equals("doseQuantity")) { 4189 this.doseQuantity = new Quantity(); 4190 return this.doseQuantity; 4191 } else if (name.equals("performer")) { 4192 return addPerformer(); 4193 } else if (name.equals("note")) { 4194 return addNote(); 4195 } else if (name.equals("reasonCode")) { 4196 return addReasonCode(); 4197 } else if (name.equals("reasonReference")) { 4198 return addReasonReference(); 4199 } else if (name.equals("isSubpotent")) { 4200 throw new FHIRException("Cannot call addChild on a singleton property Immunization.isSubpotent"); 4201 } else if (name.equals("subpotentReason")) { 4202 return addSubpotentReason(); 4203 } else if (name.equals("education")) { 4204 return addEducation(); 4205 } else if (name.equals("programEligibility")) { 4206 return addProgramEligibility(); 4207 } else if (name.equals("fundingSource")) { 4208 this.fundingSource = new CodeableConcept(); 4209 return this.fundingSource; 4210 } else if (name.equals("reaction")) { 4211 return addReaction(); 4212 } else if (name.equals("protocolApplied")) { 4213 return addProtocolApplied(); 4214 } else 4215 return super.addChild(name); 4216 } 4217 4218 public String fhirType() { 4219 return "Immunization"; 4220 4221 } 4222 4223 public Immunization copy() { 4224 Immunization dst = new Immunization(); 4225 copyValues(dst); 4226 return dst; 4227 } 4228 4229 public void copyValues(Immunization dst) { 4230 super.copyValues(dst); 4231 if (identifier != null) { 4232 dst.identifier = new ArrayList<Identifier>(); 4233 for (Identifier i : identifier) 4234 dst.identifier.add(i.copy()); 4235 } 4236 ; 4237 dst.status = status == null ? null : status.copy(); 4238 dst.statusReason = statusReason == null ? null : statusReason.copy(); 4239 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 4240 dst.patient = patient == null ? null : patient.copy(); 4241 dst.encounter = encounter == null ? null : encounter.copy(); 4242 dst.occurrence = occurrence == null ? null : occurrence.copy(); 4243 dst.recorded = recorded == null ? null : recorded.copy(); 4244 dst.primarySource = primarySource == null ? null : primarySource.copy(); 4245 dst.reportOrigin = reportOrigin == null ? null : reportOrigin.copy(); 4246 dst.location = location == null ? null : location.copy(); 4247 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 4248 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 4249 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 4250 dst.site = site == null ? null : site.copy(); 4251 dst.route = route == null ? null : route.copy(); 4252 dst.doseQuantity = doseQuantity == null ? null : doseQuantity.copy(); 4253 if (performer != null) { 4254 dst.performer = new ArrayList<ImmunizationPerformerComponent>(); 4255 for (ImmunizationPerformerComponent i : performer) 4256 dst.performer.add(i.copy()); 4257 } 4258 ; 4259 if (note != null) { 4260 dst.note = new ArrayList<Annotation>(); 4261 for (Annotation i : note) 4262 dst.note.add(i.copy()); 4263 } 4264 ; 4265 if (reasonCode != null) { 4266 dst.reasonCode = new ArrayList<CodeableConcept>(); 4267 for (CodeableConcept i : reasonCode) 4268 dst.reasonCode.add(i.copy()); 4269 } 4270 ; 4271 if (reasonReference != null) { 4272 dst.reasonReference = new ArrayList<Reference>(); 4273 for (Reference i : reasonReference) 4274 dst.reasonReference.add(i.copy()); 4275 } 4276 ; 4277 dst.isSubpotent = isSubpotent == null ? null : isSubpotent.copy(); 4278 if (subpotentReason != null) { 4279 dst.subpotentReason = new ArrayList<CodeableConcept>(); 4280 for (CodeableConcept i : subpotentReason) 4281 dst.subpotentReason.add(i.copy()); 4282 } 4283 ; 4284 if (education != null) { 4285 dst.education = new ArrayList<ImmunizationEducationComponent>(); 4286 for (ImmunizationEducationComponent i : education) 4287 dst.education.add(i.copy()); 4288 } 4289 ; 4290 if (programEligibility != null) { 4291 dst.programEligibility = new ArrayList<CodeableConcept>(); 4292 for (CodeableConcept i : programEligibility) 4293 dst.programEligibility.add(i.copy()); 4294 } 4295 ; 4296 dst.fundingSource = fundingSource == null ? null : fundingSource.copy(); 4297 if (reaction != null) { 4298 dst.reaction = new ArrayList<ImmunizationReactionComponent>(); 4299 for (ImmunizationReactionComponent i : reaction) 4300 dst.reaction.add(i.copy()); 4301 } 4302 ; 4303 if (protocolApplied != null) { 4304 dst.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 4305 for (ImmunizationProtocolAppliedComponent i : protocolApplied) 4306 dst.protocolApplied.add(i.copy()); 4307 } 4308 ; 4309 } 4310 4311 protected Immunization typedCopy() { 4312 return copy(); 4313 } 4314 4315 @Override 4316 public boolean equalsDeep(Base other_) { 4317 if (!super.equalsDeep(other_)) 4318 return false; 4319 if (!(other_ instanceof Immunization)) 4320 return false; 4321 Immunization o = (Immunization) other_; 4322 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4323 && compareDeep(statusReason, o.statusReason, true) && compareDeep(vaccineCode, o.vaccineCode, true) 4324 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) 4325 && compareDeep(occurrence, o.occurrence, true) && compareDeep(recorded, o.recorded, true) 4326 && compareDeep(primarySource, o.primarySource, true) && compareDeep(reportOrigin, o.reportOrigin, true) 4327 && compareDeep(location, o.location, true) && compareDeep(manufacturer, o.manufacturer, true) 4328 && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true) 4329 && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 4330 && compareDeep(doseQuantity, o.doseQuantity, true) && compareDeep(performer, o.performer, true) 4331 && compareDeep(note, o.note, true) && compareDeep(reasonCode, o.reasonCode, true) 4332 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(isSubpotent, o.isSubpotent, true) 4333 && compareDeep(subpotentReason, o.subpotentReason, true) && compareDeep(education, o.education, true) 4334 && compareDeep(programEligibility, o.programEligibility, true) 4335 && compareDeep(fundingSource, o.fundingSource, true) && compareDeep(reaction, o.reaction, true) 4336 && compareDeep(protocolApplied, o.protocolApplied, true); 4337 } 4338 4339 @Override 4340 public boolean equalsShallow(Base other_) { 4341 if (!super.equalsShallow(other_)) 4342 return false; 4343 if (!(other_ instanceof Immunization)) 4344 return false; 4345 Immunization o = (Immunization) other_; 4346 return compareValues(status, o.status, true) && compareValues(recorded, o.recorded, true) 4347 && compareValues(primarySource, o.primarySource, true) && compareValues(lotNumber, o.lotNumber, true) 4348 && compareValues(expirationDate, o.expirationDate, true) && compareValues(isSubpotent, o.isSubpotent, true); 4349 } 4350 4351 public boolean isEmpty() { 4352 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusReason, vaccineCode, 4353 patient, encounter, occurrence, recorded, primarySource, reportOrigin, location, manufacturer, lotNumber, 4354 expirationDate, site, route, doseQuantity, performer, note, reasonCode, reasonReference, isSubpotent, 4355 subpotentReason, education, programEligibility, fundingSource, reaction, protocolApplied); 4356 } 4357 4358 @Override 4359 public ResourceType getResourceType() { 4360 return ResourceType.Immunization; 4361 } 4362 4363 /** 4364 * Search parameter: <b>date</b> 4365 * <p> 4366 * Description: <b>Vaccination (non)-Administration Date</b><br> 4367 * Type: <b>date</b><br> 4368 * Path: <b>Immunization.occurrence[x]</b><br> 4369 * </p> 4370 */ 4371 @SearchParamDefinition(name = "date", path = "Immunization.occurrence", description = "Vaccination (non)-Administration Date", type = "date") 4372 public static final String SP_DATE = "date"; 4373 /** 4374 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4375 * <p> 4376 * Description: <b>Vaccination (non)-Administration Date</b><br> 4377 * Type: <b>date</b><br> 4378 * Path: <b>Immunization.occurrence[x]</b><br> 4379 * </p> 4380 */ 4381 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4382 SP_DATE); 4383 4384 /** 4385 * Search parameter: <b>identifier</b> 4386 * <p> 4387 * Description: <b>Business identifier</b><br> 4388 * Type: <b>token</b><br> 4389 * Path: <b>Immunization.identifier</b><br> 4390 * </p> 4391 */ 4392 @SearchParamDefinition(name = "identifier", path = "Immunization.identifier", description = "Business identifier", type = "token") 4393 public static final String SP_IDENTIFIER = "identifier"; 4394 /** 4395 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4396 * <p> 4397 * Description: <b>Business identifier</b><br> 4398 * Type: <b>token</b><br> 4399 * Path: <b>Immunization.identifier</b><br> 4400 * </p> 4401 */ 4402 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4403 SP_IDENTIFIER); 4404 4405 /** 4406 * Search parameter: <b>performer</b> 4407 * <p> 4408 * Description: <b>The practitioner or organization who played a role in the 4409 * vaccination</b><br> 4410 * Type: <b>reference</b><br> 4411 * Path: <b>Immunization.performer.actor</b><br> 4412 * </p> 4413 */ 4414 @SearchParamDefinition(name = "performer", path = "Immunization.performer.actor", description = "The practitioner or organization who played a role in the vaccination", type = "reference", providesMembershipIn = { 4415 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 4416 Practitioner.class, PractitionerRole.class }) 4417 public static final String SP_PERFORMER = "performer"; 4418 /** 4419 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4420 * <p> 4421 * Description: <b>The practitioner or organization who played a role in the 4422 * vaccination</b><br> 4423 * Type: <b>reference</b><br> 4424 * Path: <b>Immunization.performer.actor</b><br> 4425 * </p> 4426 */ 4427 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4428 SP_PERFORMER); 4429 4430 /** 4431 * Constant for fluent queries to be used to add include statements. Specifies 4432 * the path value of "<b>Immunization:performer</b>". 4433 */ 4434 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 4435 "Immunization:performer").toLocked(); 4436 4437 /** 4438 * Search parameter: <b>reaction</b> 4439 * <p> 4440 * Description: <b>Additional information on reaction</b><br> 4441 * Type: <b>reference</b><br> 4442 * Path: <b>Immunization.reaction.detail</b><br> 4443 * </p> 4444 */ 4445 @SearchParamDefinition(name = "reaction", path = "Immunization.reaction.detail", description = "Additional information on reaction", type = "reference", target = { 4446 Observation.class }) 4447 public static final String SP_REACTION = "reaction"; 4448 /** 4449 * <b>Fluent Client</b> search parameter constant for <b>reaction</b> 4450 * <p> 4451 * Description: <b>Additional information on reaction</b><br> 4452 * Type: <b>reference</b><br> 4453 * Path: <b>Immunization.reaction.detail</b><br> 4454 * </p> 4455 */ 4456 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4457 SP_REACTION); 4458 4459 /** 4460 * Constant for fluent queries to be used to add include statements. Specifies 4461 * the path value of "<b>Immunization:reaction</b>". 4462 */ 4463 public static final ca.uhn.fhir.model.api.Include INCLUDE_REACTION = new ca.uhn.fhir.model.api.Include( 4464 "Immunization:reaction").toLocked(); 4465 4466 /** 4467 * Search parameter: <b>lot-number</b> 4468 * <p> 4469 * Description: <b>Vaccine Lot Number</b><br> 4470 * Type: <b>string</b><br> 4471 * Path: <b>Immunization.lotNumber</b><br> 4472 * </p> 4473 */ 4474 @SearchParamDefinition(name = "lot-number", path = "Immunization.lotNumber", description = "Vaccine Lot Number", type = "string") 4475 public static final String SP_LOT_NUMBER = "lot-number"; 4476 /** 4477 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 4478 * <p> 4479 * Description: <b>Vaccine Lot Number</b><br> 4480 * Type: <b>string</b><br> 4481 * Path: <b>Immunization.lotNumber</b><br> 4482 * </p> 4483 */ 4484 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4485 SP_LOT_NUMBER); 4486 4487 /** 4488 * Search parameter: <b>status-reason</b> 4489 * <p> 4490 * Description: <b>Reason why the vaccine was not administered</b><br> 4491 * Type: <b>token</b><br> 4492 * Path: <b>Immunization.statusReason</b><br> 4493 * </p> 4494 */ 4495 @SearchParamDefinition(name = "status-reason", path = "Immunization.statusReason", description = "Reason why the vaccine was not administered", type = "token") 4496 public static final String SP_STATUS_REASON = "status-reason"; 4497 /** 4498 * <b>Fluent Client</b> search parameter constant for <b>status-reason</b> 4499 * <p> 4500 * Description: <b>Reason why the vaccine was not administered</b><br> 4501 * Type: <b>token</b><br> 4502 * Path: <b>Immunization.statusReason</b><br> 4503 * </p> 4504 */ 4505 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4506 SP_STATUS_REASON); 4507 4508 /** 4509 * Search parameter: <b>reason-code</b> 4510 * <p> 4511 * Description: <b>Reason why the vaccine was administered</b><br> 4512 * Type: <b>token</b><br> 4513 * Path: <b>Immunization.reasonCode</b><br> 4514 * </p> 4515 */ 4516 @SearchParamDefinition(name = "reason-code", path = "Immunization.reasonCode", description = "Reason why the vaccine was administered", type = "token") 4517 public static final String SP_REASON_CODE = "reason-code"; 4518 /** 4519 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 4520 * <p> 4521 * Description: <b>Reason why the vaccine was administered</b><br> 4522 * Type: <b>token</b><br> 4523 * Path: <b>Immunization.reasonCode</b><br> 4524 * </p> 4525 */ 4526 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4527 SP_REASON_CODE); 4528 4529 /** 4530 * Search parameter: <b>manufacturer</b> 4531 * <p> 4532 * Description: <b>Vaccine Manufacturer</b><br> 4533 * Type: <b>reference</b><br> 4534 * Path: <b>Immunization.manufacturer</b><br> 4535 * </p> 4536 */ 4537 @SearchParamDefinition(name = "manufacturer", path = "Immunization.manufacturer", description = "Vaccine Manufacturer", type = "reference", target = { 4538 Organization.class }) 4539 public static final String SP_MANUFACTURER = "manufacturer"; 4540 /** 4541 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 4542 * <p> 4543 * Description: <b>Vaccine Manufacturer</b><br> 4544 * Type: <b>reference</b><br> 4545 * Path: <b>Immunization.manufacturer</b><br> 4546 * </p> 4547 */ 4548 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4549 SP_MANUFACTURER); 4550 4551 /** 4552 * Constant for fluent queries to be used to add include statements. Specifies 4553 * the path value of "<b>Immunization:manufacturer</b>". 4554 */ 4555 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include( 4556 "Immunization:manufacturer").toLocked(); 4557 4558 /** 4559 * Search parameter: <b>target-disease</b> 4560 * <p> 4561 * Description: <b>The target disease the dose is being administered 4562 * against</b><br> 4563 * Type: <b>token</b><br> 4564 * Path: <b>Immunization.protocolApplied.targetDisease</b><br> 4565 * </p> 4566 */ 4567 @SearchParamDefinition(name = "target-disease", path = "Immunization.protocolApplied.targetDisease", description = "The target disease the dose is being administered against", type = "token") 4568 public static final String SP_TARGET_DISEASE = "target-disease"; 4569 /** 4570 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 4571 * <p> 4572 * Description: <b>The target disease the dose is being administered 4573 * against</b><br> 4574 * Type: <b>token</b><br> 4575 * Path: <b>Immunization.protocolApplied.targetDisease</b><br> 4576 * </p> 4577 */ 4578 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4579 SP_TARGET_DISEASE); 4580 4581 /** 4582 * Search parameter: <b>patient</b> 4583 * <p> 4584 * Description: <b>The patient for the vaccination record</b><br> 4585 * Type: <b>reference</b><br> 4586 * Path: <b>Immunization.patient</b><br> 4587 * </p> 4588 */ 4589 @SearchParamDefinition(name = "patient", path = "Immunization.patient", description = "The patient for the vaccination record", type = "reference", providesMembershipIn = { 4590 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4591 public static final String SP_PATIENT = "patient"; 4592 /** 4593 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4594 * <p> 4595 * Description: <b>The patient for the vaccination record</b><br> 4596 * Type: <b>reference</b><br> 4597 * Path: <b>Immunization.patient</b><br> 4598 * </p> 4599 */ 4600 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4601 SP_PATIENT); 4602 4603 /** 4604 * Constant for fluent queries to be used to add include statements. Specifies 4605 * the path value of "<b>Immunization:patient</b>". 4606 */ 4607 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4608 "Immunization:patient").toLocked(); 4609 4610 /** 4611 * Search parameter: <b>series</b> 4612 * <p> 4613 * Description: <b>The series being followed by the provider</b><br> 4614 * Type: <b>string</b><br> 4615 * Path: <b>Immunization.protocolApplied.series</b><br> 4616 * </p> 4617 */ 4618 @SearchParamDefinition(name = "series", path = "Immunization.protocolApplied.series", description = "The series being followed by the provider", type = "string") 4619 public static final String SP_SERIES = "series"; 4620 /** 4621 * <b>Fluent Client</b> search parameter constant for <b>series</b> 4622 * <p> 4623 * Description: <b>The series being followed by the provider</b><br> 4624 * Type: <b>string</b><br> 4625 * Path: <b>Immunization.protocolApplied.series</b><br> 4626 * </p> 4627 */ 4628 public static final ca.uhn.fhir.rest.gclient.StringClientParam SERIES = new ca.uhn.fhir.rest.gclient.StringClientParam( 4629 SP_SERIES); 4630 4631 /** 4632 * Search parameter: <b>vaccine-code</b> 4633 * <p> 4634 * Description: <b>Vaccine Product Administered</b><br> 4635 * Type: <b>token</b><br> 4636 * Path: <b>Immunization.vaccineCode</b><br> 4637 * </p> 4638 */ 4639 @SearchParamDefinition(name = "vaccine-code", path = "Immunization.vaccineCode", description = "Vaccine Product Administered", type = "token") 4640 public static final String SP_VACCINE_CODE = "vaccine-code"; 4641 /** 4642 * <b>Fluent Client</b> search parameter constant for <b>vaccine-code</b> 4643 * <p> 4644 * Description: <b>Vaccine Product Administered</b><br> 4645 * Type: <b>token</b><br> 4646 * Path: <b>Immunization.vaccineCode</b><br> 4647 * </p> 4648 */ 4649 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4650 SP_VACCINE_CODE); 4651 4652 /** 4653 * Search parameter: <b>reason-reference</b> 4654 * <p> 4655 * Description: <b>Why immunization occurred</b><br> 4656 * Type: <b>reference</b><br> 4657 * Path: <b>Immunization.reasonReference</b><br> 4658 * </p> 4659 */ 4660 @SearchParamDefinition(name = "reason-reference", path = "Immunization.reasonReference", description = "Why immunization occurred", type = "reference", target = { 4661 Condition.class, DiagnosticReport.class, Observation.class }) 4662 public static final String SP_REASON_REFERENCE = "reason-reference"; 4663 /** 4664 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 4665 * <p> 4666 * Description: <b>Why immunization occurred</b><br> 4667 * Type: <b>reference</b><br> 4668 * Path: <b>Immunization.reasonReference</b><br> 4669 * </p> 4670 */ 4671 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4672 SP_REASON_REFERENCE); 4673 4674 /** 4675 * Constant for fluent queries to be used to add include statements. Specifies 4676 * the path value of "<b>Immunization:reason-reference</b>". 4677 */ 4678 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 4679 "Immunization:reason-reference").toLocked(); 4680 4681 /** 4682 * Search parameter: <b>location</b> 4683 * <p> 4684 * Description: <b>The service delivery location or facility in which the 4685 * vaccine was / was to be administered</b><br> 4686 * Type: <b>reference</b><br> 4687 * Path: <b>Immunization.location</b><br> 4688 * </p> 4689 */ 4690 @SearchParamDefinition(name = "location", path = "Immunization.location", description = "The service delivery location or facility in which the vaccine was / was to be administered", type = "reference", target = { 4691 Location.class }) 4692 public static final String SP_LOCATION = "location"; 4693 /** 4694 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4695 * <p> 4696 * Description: <b>The service delivery location or facility in which the 4697 * vaccine was / was to be administered</b><br> 4698 * Type: <b>reference</b><br> 4699 * Path: <b>Immunization.location</b><br> 4700 * </p> 4701 */ 4702 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4703 SP_LOCATION); 4704 4705 /** 4706 * Constant for fluent queries to be used to add include statements. Specifies 4707 * the path value of "<b>Immunization:location</b>". 4708 */ 4709 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 4710 "Immunization:location").toLocked(); 4711 4712 /** 4713 * Search parameter: <b>status</b> 4714 * <p> 4715 * Description: <b>Immunization event status</b><br> 4716 * Type: <b>token</b><br> 4717 * Path: <b>Immunization.status</b><br> 4718 * </p> 4719 */ 4720 @SearchParamDefinition(name = "status", path = "Immunization.status", description = "Immunization event status", type = "token") 4721 public static final String SP_STATUS = "status"; 4722 /** 4723 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4724 * <p> 4725 * Description: <b>Immunization event status</b><br> 4726 * Type: <b>token</b><br> 4727 * Path: <b>Immunization.status</b><br> 4728 * </p> 4729 */ 4730 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4731 SP_STATUS); 4732 4733 /** 4734 * Search parameter: <b>reaction-date</b> 4735 * <p> 4736 * Description: <b>When reaction started</b><br> 4737 * Type: <b>date</b><br> 4738 * Path: <b>Immunization.reaction.date</b><br> 4739 * </p> 4740 */ 4741 @SearchParamDefinition(name = "reaction-date", path = "Immunization.reaction.date", description = "When reaction started", type = "date") 4742 public static final String SP_REACTION_DATE = "reaction-date"; 4743 /** 4744 * <b>Fluent Client</b> search parameter constant for <b>reaction-date</b> 4745 * <p> 4746 * Description: <b>When reaction started</b><br> 4747 * Type: <b>date</b><br> 4748 * Path: <b>Immunization.reaction.date</b><br> 4749 * </p> 4750 */ 4751 public static final ca.uhn.fhir.rest.gclient.DateClientParam REACTION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4752 SP_REACTION_DATE); 4753 4754}