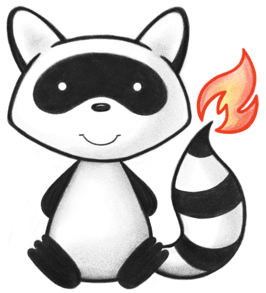
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * Describes a comparison of an immunization event against published 047 * recommendations to determine if the administration is "valid" in relation to 048 * those recommendations. 049 */ 050@ResourceDef(name = "ImmunizationEvaluation", profile = "http://hl7.org/fhir/StructureDefinition/ImmunizationEvaluation") 051public class ImmunizationEvaluation extends DomainResource { 052 053 public enum ImmunizationEvaluationStatus { 054 /** 055 * null 056 */ 057 COMPLETED, 058 /** 059 * null 060 */ 061 ENTEREDINERROR, 062 /** 063 * added to help the parsers with the generic types 064 */ 065 NULL; 066 067 public static ImmunizationEvaluationStatus fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("completed".equals(codeString)) 071 return COMPLETED; 072 if ("entered-in-error".equals(codeString)) 073 return ENTEREDINERROR; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown ImmunizationEvaluationStatus code '" + codeString + "'"); 078 } 079 080 public String toCode() { 081 switch (this) { 082 case COMPLETED: 083 return "completed"; 084 case ENTEREDINERROR: 085 return "entered-in-error"; 086 case NULL: 087 return null; 088 default: 089 return "?"; 090 } 091 } 092 093 public String getSystem() { 094 switch (this) { 095 case COMPLETED: 096 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 097 case ENTEREDINERROR: 098 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case COMPLETED: 109 return ""; 110 case ENTEREDINERROR: 111 return ""; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDisplay() { 120 switch (this) { 121 case COMPLETED: 122 return "completed"; 123 case ENTEREDINERROR: 124 return "entered-in-error"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 } 132 133 public static class ImmunizationEvaluationStatusEnumFactory implements EnumFactory<ImmunizationEvaluationStatus> { 134 public ImmunizationEvaluationStatus fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("completed".equals(codeString)) 139 return ImmunizationEvaluationStatus.COMPLETED; 140 if ("entered-in-error".equals(codeString)) 141 return ImmunizationEvaluationStatus.ENTEREDINERROR; 142 throw new IllegalArgumentException("Unknown ImmunizationEvaluationStatus code '" + codeString + "'"); 143 } 144 145 public Enumeration<ImmunizationEvaluationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<ImmunizationEvaluationStatus>(this, ImmunizationEvaluationStatus.NULL, code); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return new Enumeration<ImmunizationEvaluationStatus>(this, ImmunizationEvaluationStatus.NULL, code); 153 if ("completed".equals(codeString)) 154 return new Enumeration<ImmunizationEvaluationStatus>(this, ImmunizationEvaluationStatus.COMPLETED, code); 155 if ("entered-in-error".equals(codeString)) 156 return new Enumeration<ImmunizationEvaluationStatus>(this, ImmunizationEvaluationStatus.ENTEREDINERROR, code); 157 throw new FHIRException("Unknown ImmunizationEvaluationStatus code '" + codeString + "'"); 158 } 159 160 public String toCode(ImmunizationEvaluationStatus code) { 161 if (code == ImmunizationEvaluationStatus.NULL) 162 return null; 163 if (code == ImmunizationEvaluationStatus.COMPLETED) 164 return "completed"; 165 if (code == ImmunizationEvaluationStatus.ENTEREDINERROR) 166 return "entered-in-error"; 167 return "?"; 168 } 169 170 public String toSystem(ImmunizationEvaluationStatus code) { 171 return code.getSystem(); 172 } 173 } 174 175 /** 176 * A unique identifier assigned to this immunization evaluation record. 177 */ 178 @Child(name = "identifier", type = { 179 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 180 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this immunization evaluation record.") 181 protected List<Identifier> identifier; 182 183 /** 184 * Indicates the current status of the evaluation of the vaccination 185 * administration event. 186 */ 187 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 188 @Description(shortDefinition = "completed | entered-in-error", formalDefinition = "Indicates the current status of the evaluation of the vaccination administration event.") 189 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-evaluation-status") 190 protected Enumeration<ImmunizationEvaluationStatus> status; 191 192 /** 193 * The individual for whom the evaluation is being done. 194 */ 195 @Child(name = "patient", type = { Patient.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 196 @Description(shortDefinition = "Who this evaluation is for", formalDefinition = "The individual for whom the evaluation is being done.") 197 protected Reference patient; 198 199 /** 200 * The actual object that is the target of the reference (The individual for 201 * whom the evaluation is being done.) 202 */ 203 protected Patient patientTarget; 204 205 /** 206 * The date the evaluation of the vaccine administration event was performed. 207 */ 208 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 209 @Description(shortDefinition = "Date evaluation was performed", formalDefinition = "The date the evaluation of the vaccine administration event was performed.") 210 protected DateTimeType date; 211 212 /** 213 * Indicates the authority who published the protocol (e.g. ACIP). 214 */ 215 @Child(name = "authority", type = { 216 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 217 @Description(shortDefinition = "Who is responsible for publishing the recommendations", formalDefinition = "Indicates the authority who published the protocol (e.g. ACIP).") 218 protected Reference authority; 219 220 /** 221 * The actual object that is the target of the reference (Indicates the 222 * authority who published the protocol (e.g. ACIP).) 223 */ 224 protected Organization authorityTarget; 225 226 /** 227 * The vaccine preventable disease the dose is being evaluated against. 228 */ 229 @Child(name = "targetDisease", type = { 230 CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 231 @Description(shortDefinition = "Evaluation target disease", formalDefinition = "The vaccine preventable disease the dose is being evaluated against.") 232 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-evaluation-target-disease") 233 protected CodeableConcept targetDisease; 234 235 /** 236 * The vaccine administration event being evaluated. 237 */ 238 @Child(name = "immunizationEvent", type = { 239 Immunization.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 240 @Description(shortDefinition = "Immunization being evaluated", formalDefinition = "The vaccine administration event being evaluated.") 241 protected Reference immunizationEvent; 242 243 /** 244 * The actual object that is the target of the reference (The vaccine 245 * administration event being evaluated.) 246 */ 247 protected Immunization immunizationEventTarget; 248 249 /** 250 * Indicates if the dose is valid or not valid with respect to the published 251 * recommendations. 252 */ 253 @Child(name = "doseStatus", type = { 254 CodeableConcept.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 255 @Description(shortDefinition = "Status of the dose relative to published recommendations", formalDefinition = "Indicates if the dose is valid or not valid with respect to the published recommendations.") 256 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-evaluation-dose-status") 257 protected CodeableConcept doseStatus; 258 259 /** 260 * Provides an explanation as to why the vaccine administration event is valid 261 * or not relative to the published recommendations. 262 */ 263 @Child(name = "doseStatusReason", type = { 264 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 265 @Description(shortDefinition = "Reason for the dose status", formalDefinition = "Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations.") 266 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-evaluation-dose-status-reason") 267 protected List<CodeableConcept> doseStatusReason; 268 269 /** 270 * Additional information about the evaluation. 271 */ 272 @Child(name = "description", type = { 273 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 274 @Description(shortDefinition = "Evaluation notes", formalDefinition = "Additional information about the evaluation.") 275 protected StringType description; 276 277 /** 278 * One possible path to achieve presumed immunity against a disease - within the 279 * context of an authority. 280 */ 281 @Child(name = "series", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 282 @Description(shortDefinition = "Name of vaccine series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 283 protected StringType series; 284 285 /** 286 * Nominal position in a series. 287 */ 288 @Child(name = "doseNumber", type = { PositiveIntType.class, 289 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 290 @Description(shortDefinition = "Dose number within series", formalDefinition = "Nominal position in a series.") 291 protected Type doseNumber; 292 293 /** 294 * The recommended number of doses to achieve immunity. 295 */ 296 @Child(name = "seriesDoses", type = { PositiveIntType.class, 297 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 298 @Description(shortDefinition = "Recommended number of doses for immunity", formalDefinition = "The recommended number of doses to achieve immunity.") 299 protected Type seriesDoses; 300 301 private static final long serialVersionUID = 1248741226L; 302 303 /** 304 * Constructor 305 */ 306 public ImmunizationEvaluation() { 307 super(); 308 } 309 310 /** 311 * Constructor 312 */ 313 public ImmunizationEvaluation(Enumeration<ImmunizationEvaluationStatus> status, Reference patient, 314 CodeableConcept targetDisease, Reference immunizationEvent, CodeableConcept doseStatus) { 315 super(); 316 this.status = status; 317 this.patient = patient; 318 this.targetDisease = targetDisease; 319 this.immunizationEvent = immunizationEvent; 320 this.doseStatus = doseStatus; 321 } 322 323 /** 324 * @return {@link #identifier} (A unique identifier assigned to this 325 * immunization evaluation record.) 326 */ 327 public List<Identifier> getIdentifier() { 328 if (this.identifier == null) 329 this.identifier = new ArrayList<Identifier>(); 330 return this.identifier; 331 } 332 333 /** 334 * @return Returns a reference to <code>this</code> for easy method chaining 335 */ 336 public ImmunizationEvaluation setIdentifier(List<Identifier> theIdentifier) { 337 this.identifier = theIdentifier; 338 return this; 339 } 340 341 public boolean hasIdentifier() { 342 if (this.identifier == null) 343 return false; 344 for (Identifier item : this.identifier) 345 if (!item.isEmpty()) 346 return true; 347 return false; 348 } 349 350 public Identifier addIdentifier() { // 3 351 Identifier t = new Identifier(); 352 if (this.identifier == null) 353 this.identifier = new ArrayList<Identifier>(); 354 this.identifier.add(t); 355 return t; 356 } 357 358 public ImmunizationEvaluation addIdentifier(Identifier t) { // 3 359 if (t == null) 360 return this; 361 if (this.identifier == null) 362 this.identifier = new ArrayList<Identifier>(); 363 this.identifier.add(t); 364 return this; 365 } 366 367 /** 368 * @return The first repetition of repeating field {@link #identifier}, creating 369 * it if it does not already exist 370 */ 371 public Identifier getIdentifierFirstRep() { 372 if (getIdentifier().isEmpty()) { 373 addIdentifier(); 374 } 375 return getIdentifier().get(0); 376 } 377 378 /** 379 * @return {@link #status} (Indicates the current status of the evaluation of 380 * the vaccination administration event.). This is the underlying object 381 * with id, value and extensions. The accessor "getStatus" gives direct 382 * access to the value 383 */ 384 public Enumeration<ImmunizationEvaluationStatus> getStatusElement() { 385 if (this.status == null) 386 if (Configuration.errorOnAutoCreate()) 387 throw new Error("Attempt to auto-create ImmunizationEvaluation.status"); 388 else if (Configuration.doAutoCreate()) 389 this.status = new Enumeration<ImmunizationEvaluationStatus>(new ImmunizationEvaluationStatusEnumFactory()); // bb 390 return this.status; 391 } 392 393 public boolean hasStatusElement() { 394 return this.status != null && !this.status.isEmpty(); 395 } 396 397 public boolean hasStatus() { 398 return this.status != null && !this.status.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #status} (Indicates the current status of the evaluation 403 * of the vaccination administration event.). This is the 404 * underlying object with id, value and extensions. The accessor 405 * "getStatus" gives direct access to the value 406 */ 407 public ImmunizationEvaluation setStatusElement(Enumeration<ImmunizationEvaluationStatus> value) { 408 this.status = value; 409 return this; 410 } 411 412 /** 413 * @return Indicates the current status of the evaluation of the vaccination 414 * administration event. 415 */ 416 public ImmunizationEvaluationStatus getStatus() { 417 return this.status == null ? null : this.status.getValue(); 418 } 419 420 /** 421 * @param value Indicates the current status of the evaluation of the 422 * vaccination administration event. 423 */ 424 public ImmunizationEvaluation setStatus(ImmunizationEvaluationStatus value) { 425 if (this.status == null) 426 this.status = new Enumeration<ImmunizationEvaluationStatus>(new ImmunizationEvaluationStatusEnumFactory()); 427 this.status.setValue(value); 428 return this; 429 } 430 431 /** 432 * @return {@link #patient} (The individual for whom the evaluation is being 433 * done.) 434 */ 435 public Reference getPatient() { 436 if (this.patient == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create ImmunizationEvaluation.patient"); 439 else if (Configuration.doAutoCreate()) 440 this.patient = new Reference(); // cc 441 return this.patient; 442 } 443 444 public boolean hasPatient() { 445 return this.patient != null && !this.patient.isEmpty(); 446 } 447 448 /** 449 * @param value {@link #patient} (The individual for whom the evaluation is 450 * being done.) 451 */ 452 public ImmunizationEvaluation setPatient(Reference value) { 453 this.patient = value; 454 return this; 455 } 456 457 /** 458 * @return {@link #patient} The actual object that is the target of the 459 * reference. The reference library doesn't populate this, but you can 460 * use it to hold the resource if you resolve it. (The individual for 461 * whom the evaluation is being done.) 462 */ 463 public Patient getPatientTarget() { 464 if (this.patientTarget == null) 465 if (Configuration.errorOnAutoCreate()) 466 throw new Error("Attempt to auto-create ImmunizationEvaluation.patient"); 467 else if (Configuration.doAutoCreate()) 468 this.patientTarget = new Patient(); // aa 469 return this.patientTarget; 470 } 471 472 /** 473 * @param value {@link #patient} The actual object that is the target of the 474 * reference. The reference library doesn't use these, but you can 475 * use it to hold the resource if you resolve it. (The individual 476 * for whom the evaluation is being done.) 477 */ 478 public ImmunizationEvaluation setPatientTarget(Patient value) { 479 this.patientTarget = value; 480 return this; 481 } 482 483 /** 484 * @return {@link #date} (The date the evaluation of the vaccine administration 485 * event was performed.). This is the underlying object with id, value 486 * and extensions. The accessor "getDate" gives direct access to the 487 * value 488 */ 489 public DateTimeType getDateElement() { 490 if (this.date == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create ImmunizationEvaluation.date"); 493 else if (Configuration.doAutoCreate()) 494 this.date = new DateTimeType(); // bb 495 return this.date; 496 } 497 498 public boolean hasDateElement() { 499 return this.date != null && !this.date.isEmpty(); 500 } 501 502 public boolean hasDate() { 503 return this.date != null && !this.date.isEmpty(); 504 } 505 506 /** 507 * @param value {@link #date} (The date the evaluation of the vaccine 508 * administration event was performed.). This is the underlying 509 * object with id, value and extensions. The accessor "getDate" 510 * gives direct access to the value 511 */ 512 public ImmunizationEvaluation setDateElement(DateTimeType value) { 513 this.date = value; 514 return this; 515 } 516 517 /** 518 * @return The date the evaluation of the vaccine administration event was 519 * performed. 520 */ 521 public Date getDate() { 522 return this.date == null ? null : this.date.getValue(); 523 } 524 525 /** 526 * @param value The date the evaluation of the vaccine administration event was 527 * performed. 528 */ 529 public ImmunizationEvaluation setDate(Date value) { 530 if (value == null) 531 this.date = null; 532 else { 533 if (this.date == null) 534 this.date = new DateTimeType(); 535 this.date.setValue(value); 536 } 537 return this; 538 } 539 540 /** 541 * @return {@link #authority} (Indicates the authority who published the 542 * protocol (e.g. ACIP).) 543 */ 544 public Reference getAuthority() { 545 if (this.authority == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create ImmunizationEvaluation.authority"); 548 else if (Configuration.doAutoCreate()) 549 this.authority = new Reference(); // cc 550 return this.authority; 551 } 552 553 public boolean hasAuthority() { 554 return this.authority != null && !this.authority.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #authority} (Indicates the authority who published the 559 * protocol (e.g. ACIP).) 560 */ 561 public ImmunizationEvaluation setAuthority(Reference value) { 562 this.authority = value; 563 return this; 564 } 565 566 /** 567 * @return {@link #authority} The actual object that is the target of the 568 * reference. The reference library doesn't populate this, but you can 569 * use it to hold the resource if you resolve it. (Indicates the 570 * authority who published the protocol (e.g. ACIP).) 571 */ 572 public Organization getAuthorityTarget() { 573 if (this.authorityTarget == null) 574 if (Configuration.errorOnAutoCreate()) 575 throw new Error("Attempt to auto-create ImmunizationEvaluation.authority"); 576 else if (Configuration.doAutoCreate()) 577 this.authorityTarget = new Organization(); // aa 578 return this.authorityTarget; 579 } 580 581 /** 582 * @param value {@link #authority} The actual object that is the target of the 583 * reference. The reference library doesn't use these, but you can 584 * use it to hold the resource if you resolve it. (Indicates the 585 * authority who published the protocol (e.g. ACIP).) 586 */ 587 public ImmunizationEvaluation setAuthorityTarget(Organization value) { 588 this.authorityTarget = value; 589 return this; 590 } 591 592 /** 593 * @return {@link #targetDisease} (The vaccine preventable disease the dose is 594 * being evaluated against.) 595 */ 596 public CodeableConcept getTargetDisease() { 597 if (this.targetDisease == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create ImmunizationEvaluation.targetDisease"); 600 else if (Configuration.doAutoCreate()) 601 this.targetDisease = new CodeableConcept(); // cc 602 return this.targetDisease; 603 } 604 605 public boolean hasTargetDisease() { 606 return this.targetDisease != null && !this.targetDisease.isEmpty(); 607 } 608 609 /** 610 * @param value {@link #targetDisease} (The vaccine preventable disease the dose 611 * is being evaluated against.) 612 */ 613 public ImmunizationEvaluation setTargetDisease(CodeableConcept value) { 614 this.targetDisease = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #immunizationEvent} (The vaccine administration event being 620 * evaluated.) 621 */ 622 public Reference getImmunizationEvent() { 623 if (this.immunizationEvent == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create ImmunizationEvaluation.immunizationEvent"); 626 else if (Configuration.doAutoCreate()) 627 this.immunizationEvent = new Reference(); // cc 628 return this.immunizationEvent; 629 } 630 631 public boolean hasImmunizationEvent() { 632 return this.immunizationEvent != null && !this.immunizationEvent.isEmpty(); 633 } 634 635 /** 636 * @param value {@link #immunizationEvent} (The vaccine administration event 637 * being evaluated.) 638 */ 639 public ImmunizationEvaluation setImmunizationEvent(Reference value) { 640 this.immunizationEvent = value; 641 return this; 642 } 643 644 /** 645 * @return {@link #immunizationEvent} The actual object that is the target of 646 * the reference. The reference library doesn't populate this, but you 647 * can use it to hold the resource if you resolve it. (The vaccine 648 * administration event being evaluated.) 649 */ 650 public Immunization getImmunizationEventTarget() { 651 if (this.immunizationEventTarget == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create ImmunizationEvaluation.immunizationEvent"); 654 else if (Configuration.doAutoCreate()) 655 this.immunizationEventTarget = new Immunization(); // aa 656 return this.immunizationEventTarget; 657 } 658 659 /** 660 * @param value {@link #immunizationEvent} The actual object that is the target 661 * of the reference. The reference library doesn't use these, but 662 * you can use it to hold the resource if you resolve it. (The 663 * vaccine administration event being evaluated.) 664 */ 665 public ImmunizationEvaluation setImmunizationEventTarget(Immunization value) { 666 this.immunizationEventTarget = value; 667 return this; 668 } 669 670 /** 671 * @return {@link #doseStatus} (Indicates if the dose is valid or not valid with 672 * respect to the published recommendations.) 673 */ 674 public CodeableConcept getDoseStatus() { 675 if (this.doseStatus == null) 676 if (Configuration.errorOnAutoCreate()) 677 throw new Error("Attempt to auto-create ImmunizationEvaluation.doseStatus"); 678 else if (Configuration.doAutoCreate()) 679 this.doseStatus = new CodeableConcept(); // cc 680 return this.doseStatus; 681 } 682 683 public boolean hasDoseStatus() { 684 return this.doseStatus != null && !this.doseStatus.isEmpty(); 685 } 686 687 /** 688 * @param value {@link #doseStatus} (Indicates if the dose is valid or not valid 689 * with respect to the published recommendations.) 690 */ 691 public ImmunizationEvaluation setDoseStatus(CodeableConcept value) { 692 this.doseStatus = value; 693 return this; 694 } 695 696 /** 697 * @return {@link #doseStatusReason} (Provides an explanation as to why the 698 * vaccine administration event is valid or not relative to the 699 * published recommendations.) 700 */ 701 public List<CodeableConcept> getDoseStatusReason() { 702 if (this.doseStatusReason == null) 703 this.doseStatusReason = new ArrayList<CodeableConcept>(); 704 return this.doseStatusReason; 705 } 706 707 /** 708 * @return Returns a reference to <code>this</code> for easy method chaining 709 */ 710 public ImmunizationEvaluation setDoseStatusReason(List<CodeableConcept> theDoseStatusReason) { 711 this.doseStatusReason = theDoseStatusReason; 712 return this; 713 } 714 715 public boolean hasDoseStatusReason() { 716 if (this.doseStatusReason == null) 717 return false; 718 for (CodeableConcept item : this.doseStatusReason) 719 if (!item.isEmpty()) 720 return true; 721 return false; 722 } 723 724 public CodeableConcept addDoseStatusReason() { // 3 725 CodeableConcept t = new CodeableConcept(); 726 if (this.doseStatusReason == null) 727 this.doseStatusReason = new ArrayList<CodeableConcept>(); 728 this.doseStatusReason.add(t); 729 return t; 730 } 731 732 public ImmunizationEvaluation addDoseStatusReason(CodeableConcept t) { // 3 733 if (t == null) 734 return this; 735 if (this.doseStatusReason == null) 736 this.doseStatusReason = new ArrayList<CodeableConcept>(); 737 this.doseStatusReason.add(t); 738 return this; 739 } 740 741 /** 742 * @return The first repetition of repeating field {@link #doseStatusReason}, 743 * creating it if it does not already exist 744 */ 745 public CodeableConcept getDoseStatusReasonFirstRep() { 746 if (getDoseStatusReason().isEmpty()) { 747 addDoseStatusReason(); 748 } 749 return getDoseStatusReason().get(0); 750 } 751 752 /** 753 * @return {@link #description} (Additional information about the evaluation.). 754 * This is the underlying object with id, value and extensions. The 755 * accessor "getDescription" gives direct access to the value 756 */ 757 public StringType getDescriptionElement() { 758 if (this.description == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create ImmunizationEvaluation.description"); 761 else if (Configuration.doAutoCreate()) 762 this.description = new StringType(); // bb 763 return this.description; 764 } 765 766 public boolean hasDescriptionElement() { 767 return this.description != null && !this.description.isEmpty(); 768 } 769 770 public boolean hasDescription() { 771 return this.description != null && !this.description.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #description} (Additional information about the 776 * evaluation.). This is the underlying object with id, value and 777 * extensions. The accessor "getDescription" gives direct access to 778 * the value 779 */ 780 public ImmunizationEvaluation setDescriptionElement(StringType value) { 781 this.description = value; 782 return this; 783 } 784 785 /** 786 * @return Additional information about the evaluation. 787 */ 788 public String getDescription() { 789 return this.description == null ? null : this.description.getValue(); 790 } 791 792 /** 793 * @param value Additional information about the evaluation. 794 */ 795 public ImmunizationEvaluation setDescription(String value) { 796 if (Utilities.noString(value)) 797 this.description = null; 798 else { 799 if (this.description == null) 800 this.description = new StringType(); 801 this.description.setValue(value); 802 } 803 return this; 804 } 805 806 /** 807 * @return {@link #series} (One possible path to achieve presumed immunity 808 * against a disease - within the context of an authority.). This is the 809 * underlying object with id, value and extensions. The accessor 810 * "getSeries" gives direct access to the value 811 */ 812 public StringType getSeriesElement() { 813 if (this.series == null) 814 if (Configuration.errorOnAutoCreate()) 815 throw new Error("Attempt to auto-create ImmunizationEvaluation.series"); 816 else if (Configuration.doAutoCreate()) 817 this.series = new StringType(); // bb 818 return this.series; 819 } 820 821 public boolean hasSeriesElement() { 822 return this.series != null && !this.series.isEmpty(); 823 } 824 825 public boolean hasSeries() { 826 return this.series != null && !this.series.isEmpty(); 827 } 828 829 /** 830 * @param value {@link #series} (One possible path to achieve presumed immunity 831 * against a disease - within the context of an authority.). This 832 * is the underlying object with id, value and extensions. The 833 * accessor "getSeries" gives direct access to the value 834 */ 835 public ImmunizationEvaluation setSeriesElement(StringType value) { 836 this.series = value; 837 return this; 838 } 839 840 /** 841 * @return One possible path to achieve presumed immunity against a disease - 842 * within the context of an authority. 843 */ 844 public String getSeries() { 845 return this.series == null ? null : this.series.getValue(); 846 } 847 848 /** 849 * @param value One possible path to achieve presumed immunity against a disease 850 * - within the context of an authority. 851 */ 852 public ImmunizationEvaluation setSeries(String value) { 853 if (Utilities.noString(value)) 854 this.series = null; 855 else { 856 if (this.series == null) 857 this.series = new StringType(); 858 this.series.setValue(value); 859 } 860 return this; 861 } 862 863 /** 864 * @return {@link #doseNumber} (Nominal position in a series.) 865 */ 866 public Type getDoseNumber() { 867 return this.doseNumber; 868 } 869 870 /** 871 * @return {@link #doseNumber} (Nominal position in a series.) 872 */ 873 public PositiveIntType getDoseNumberPositiveIntType() throws FHIRException { 874 if (this.doseNumber == null) 875 this.doseNumber = new PositiveIntType(); 876 if (!(this.doseNumber instanceof PositiveIntType)) 877 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 878 + this.doseNumber.getClass().getName() + " was encountered"); 879 return (PositiveIntType) this.doseNumber; 880 } 881 882 public boolean hasDoseNumberPositiveIntType() { 883 return this.doseNumber instanceof PositiveIntType; 884 } 885 886 /** 887 * @return {@link #doseNumber} (Nominal position in a series.) 888 */ 889 public StringType getDoseNumberStringType() throws FHIRException { 890 if (this.doseNumber == null) 891 this.doseNumber = new StringType(); 892 if (!(this.doseNumber instanceof StringType)) 893 throw new FHIRException("Type mismatch: the type StringType was expected, but " 894 + this.doseNumber.getClass().getName() + " was encountered"); 895 return (StringType) this.doseNumber; 896 } 897 898 public boolean hasDoseNumberStringType() { 899 return this.doseNumber instanceof StringType; 900 } 901 902 public boolean hasDoseNumber() { 903 return this.doseNumber != null && !this.doseNumber.isEmpty(); 904 } 905 906 /** 907 * @param value {@link #doseNumber} (Nominal position in a series.) 908 */ 909 public ImmunizationEvaluation setDoseNumber(Type value) { 910 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 911 throw new Error("Not the right type for ImmunizationEvaluation.doseNumber[x]: " + value.fhirType()); 912 this.doseNumber = value; 913 return this; 914 } 915 916 /** 917 * @return {@link #seriesDoses} (The recommended number of doses to achieve 918 * immunity.) 919 */ 920 public Type getSeriesDoses() { 921 return this.seriesDoses; 922 } 923 924 /** 925 * @return {@link #seriesDoses} (The recommended number of doses to achieve 926 * immunity.) 927 */ 928 public PositiveIntType getSeriesDosesPositiveIntType() throws FHIRException { 929 if (this.seriesDoses == null) 930 this.seriesDoses = new PositiveIntType(); 931 if (!(this.seriesDoses instanceof PositiveIntType)) 932 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 933 + this.seriesDoses.getClass().getName() + " was encountered"); 934 return (PositiveIntType) this.seriesDoses; 935 } 936 937 public boolean hasSeriesDosesPositiveIntType() { 938 return this.seriesDoses instanceof PositiveIntType; 939 } 940 941 /** 942 * @return {@link #seriesDoses} (The recommended number of doses to achieve 943 * immunity.) 944 */ 945 public StringType getSeriesDosesStringType() throws FHIRException { 946 if (this.seriesDoses == null) 947 this.seriesDoses = new StringType(); 948 if (!(this.seriesDoses instanceof StringType)) 949 throw new FHIRException("Type mismatch: the type StringType was expected, but " 950 + this.seriesDoses.getClass().getName() + " was encountered"); 951 return (StringType) this.seriesDoses; 952 } 953 954 public boolean hasSeriesDosesStringType() { 955 return this.seriesDoses instanceof StringType; 956 } 957 958 public boolean hasSeriesDoses() { 959 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #seriesDoses} (The recommended number of doses to achieve 964 * immunity.) 965 */ 966 public ImmunizationEvaluation setSeriesDoses(Type value) { 967 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 968 throw new Error("Not the right type for ImmunizationEvaluation.seriesDoses[x]: " + value.fhirType()); 969 this.seriesDoses = value; 970 return this; 971 } 972 973 protected void listChildren(List<Property> children) { 974 super.listChildren(children); 975 children.add( 976 new Property("identifier", "Identifier", "A unique identifier assigned to this immunization evaluation record.", 977 0, java.lang.Integer.MAX_VALUE, identifier)); 978 children.add(new Property("status", "code", 979 "Indicates the current status of the evaluation of the vaccination administration event.", 0, 1, status)); 980 children.add(new Property("patient", "Reference(Patient)", "The individual for whom the evaluation is being done.", 981 0, 1, patient)); 982 children.add(new Property("date", "dateTime", 983 "The date the evaluation of the vaccine administration event was performed.", 0, 1, date)); 984 children.add(new Property("authority", "Reference(Organization)", 985 "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority)); 986 children.add(new Property("targetDisease", "CodeableConcept", 987 "The vaccine preventable disease the dose is being evaluated against.", 0, 1, targetDisease)); 988 children.add(new Property("immunizationEvent", "Reference(Immunization)", 989 "The vaccine administration event being evaluated.", 0, 1, immunizationEvent)); 990 children.add(new Property("doseStatus", "CodeableConcept", 991 "Indicates if the dose is valid or not valid with respect to the published recommendations.", 0, 1, 992 doseStatus)); 993 children.add(new Property("doseStatusReason", "CodeableConcept", 994 "Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations.", 995 0, java.lang.Integer.MAX_VALUE, doseStatusReason)); 996 children 997 .add(new Property("description", "string", "Additional information about the evaluation.", 0, 1, description)); 998 children.add(new Property("series", "string", 999 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, 1000 series)); 1001 children 1002 .add(new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1, doseNumber)); 1003 children.add(new Property("seriesDoses[x]", "positiveInt|string", 1004 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 1005 } 1006 1007 @Override 1008 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1009 switch (_hash) { 1010 case -1618432855: 1011 /* identifier */ return new Property("identifier", "Identifier", 1012 "A unique identifier assigned to this immunization evaluation record.", 0, java.lang.Integer.MAX_VALUE, 1013 identifier); 1014 case -892481550: 1015 /* status */ return new Property("status", "code", 1016 "Indicates the current status of the evaluation of the vaccination administration event.", 0, 1, status); 1017 case -791418107: 1018 /* patient */ return new Property("patient", "Reference(Patient)", 1019 "The individual for whom the evaluation is being done.", 0, 1, patient); 1020 case 3076014: 1021 /* date */ return new Property("date", "dateTime", 1022 "The date the evaluation of the vaccine administration event was performed.", 0, 1, date); 1023 case 1475610435: 1024 /* authority */ return new Property("authority", "Reference(Organization)", 1025 "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority); 1026 case -319593813: 1027 /* targetDisease */ return new Property("targetDisease", "CodeableConcept", 1028 "The vaccine preventable disease the dose is being evaluated against.", 0, 1, targetDisease); 1029 case 1081446840: 1030 /* immunizationEvent */ return new Property("immunizationEvent", "Reference(Immunization)", 1031 "The vaccine administration event being evaluated.", 0, 1, immunizationEvent); 1032 case -745826705: 1033 /* doseStatus */ return new Property("doseStatus", "CodeableConcept", 1034 "Indicates if the dose is valid or not valid with respect to the published recommendations.", 0, 1, 1035 doseStatus); 1036 case 662783379: 1037 /* doseStatusReason */ return new Property("doseStatusReason", "CodeableConcept", 1038 "Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations.", 1039 0, java.lang.Integer.MAX_VALUE, doseStatusReason); 1040 case -1724546052: 1041 /* description */ return new Property("description", "string", "Additional information about the evaluation.", 0, 1042 1, description); 1043 case -905838985: 1044 /* series */ return new Property("series", "string", 1045 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1046 1, series); 1047 case -1632295686: 1048 /* doseNumber[x] */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1049 1, doseNumber); 1050 case -887709242: 1051 /* doseNumber */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1, 1052 doseNumber); 1053 case -1826134640: 1054 /* doseNumberPositiveInt */ return new Property("doseNumber[x]", "positiveInt|string", 1055 "Nominal position in a series.", 0, 1, doseNumber); 1056 case -333053577: 1057 /* doseNumberString */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 1058 0, 1, doseNumber); 1059 case 1553560673: 1060 /* seriesDoses[x] */ return new Property("seriesDoses[x]", "positiveInt|string", 1061 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1062 case -1936727105: 1063 /* seriesDoses */ return new Property("seriesDoses[x]", "positiveInt|string", 1064 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1065 case -220897801: 1066 /* seriesDosesPositiveInt */ return new Property("seriesDoses[x]", "positiveInt|string", 1067 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1068 case -673569616: 1069 /* seriesDosesString */ return new Property("seriesDoses[x]", "positiveInt|string", 1070 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1071 default: 1072 return super.getNamedProperty(_hash, _name, _checkValid); 1073 } 1074 1075 } 1076 1077 @Override 1078 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1079 switch (hash) { 1080 case -1618432855: 1081 /* identifier */ return this.identifier == null ? new Base[0] 1082 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1083 case -892481550: 1084 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ImmunizationEvaluationStatus> 1085 case -791418107: 1086 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1087 case 3076014: 1088 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1089 case 1475610435: 1090 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // Reference 1091 case -319593813: 1092 /* targetDisease */ return this.targetDisease == null ? new Base[0] : new Base[] { this.targetDisease }; // CodeableConcept 1093 case 1081446840: 1094 /* immunizationEvent */ return this.immunizationEvent == null ? new Base[0] 1095 : new Base[] { this.immunizationEvent }; // Reference 1096 case -745826705: 1097 /* doseStatus */ return this.doseStatus == null ? new Base[0] : new Base[] { this.doseStatus }; // CodeableConcept 1098 case 662783379: 1099 /* doseStatusReason */ return this.doseStatusReason == null ? new Base[0] 1100 : this.doseStatusReason.toArray(new Base[this.doseStatusReason.size()]); // CodeableConcept 1101 case -1724546052: 1102 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1103 case -905838985: 1104 /* series */ return this.series == null ? new Base[0] : new Base[] { this.series }; // StringType 1105 case -887709242: 1106 /* doseNumber */ return this.doseNumber == null ? new Base[0] : new Base[] { this.doseNumber }; // Type 1107 case -1936727105: 1108 /* seriesDoses */ return this.seriesDoses == null ? new Base[0] : new Base[] { this.seriesDoses }; // Type 1109 default: 1110 return super.getProperty(hash, name, checkValid); 1111 } 1112 1113 } 1114 1115 @Override 1116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1117 switch (hash) { 1118 case -1618432855: // identifier 1119 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1120 return value; 1121 case -892481550: // status 1122 value = new ImmunizationEvaluationStatusEnumFactory().fromType(castToCode(value)); 1123 this.status = (Enumeration) value; // Enumeration<ImmunizationEvaluationStatus> 1124 return value; 1125 case -791418107: // patient 1126 this.patient = castToReference(value); // Reference 1127 return value; 1128 case 3076014: // date 1129 this.date = castToDateTime(value); // DateTimeType 1130 return value; 1131 case 1475610435: // authority 1132 this.authority = castToReference(value); // Reference 1133 return value; 1134 case -319593813: // targetDisease 1135 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 1136 return value; 1137 case 1081446840: // immunizationEvent 1138 this.immunizationEvent = castToReference(value); // Reference 1139 return value; 1140 case -745826705: // doseStatus 1141 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1142 return value; 1143 case 662783379: // doseStatusReason 1144 this.getDoseStatusReason().add(castToCodeableConcept(value)); // CodeableConcept 1145 return value; 1146 case -1724546052: // description 1147 this.description = castToString(value); // StringType 1148 return value; 1149 case -905838985: // series 1150 this.series = castToString(value); // StringType 1151 return value; 1152 case -887709242: // doseNumber 1153 this.doseNumber = castToType(value); // Type 1154 return value; 1155 case -1936727105: // seriesDoses 1156 this.seriesDoses = castToType(value); // Type 1157 return value; 1158 default: 1159 return super.setProperty(hash, name, value); 1160 } 1161 1162 } 1163 1164 @Override 1165 public Base setProperty(String name, Base value) throws FHIRException { 1166 if (name.equals("identifier")) { 1167 this.getIdentifier().add(castToIdentifier(value)); 1168 } else if (name.equals("status")) { 1169 value = new ImmunizationEvaluationStatusEnumFactory().fromType(castToCode(value)); 1170 this.status = (Enumeration) value; // Enumeration<ImmunizationEvaluationStatus> 1171 } else if (name.equals("patient")) { 1172 this.patient = castToReference(value); // Reference 1173 } else if (name.equals("date")) { 1174 this.date = castToDateTime(value); // DateTimeType 1175 } else if (name.equals("authority")) { 1176 this.authority = castToReference(value); // Reference 1177 } else if (name.equals("targetDisease")) { 1178 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 1179 } else if (name.equals("immunizationEvent")) { 1180 this.immunizationEvent = castToReference(value); // Reference 1181 } else if (name.equals("doseStatus")) { 1182 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1183 } else if (name.equals("doseStatusReason")) { 1184 this.getDoseStatusReason().add(castToCodeableConcept(value)); 1185 } else if (name.equals("description")) { 1186 this.description = castToString(value); // StringType 1187 } else if (name.equals("series")) { 1188 this.series = castToString(value); // StringType 1189 } else if (name.equals("doseNumber[x]")) { 1190 this.doseNumber = castToType(value); // Type 1191 } else if (name.equals("seriesDoses[x]")) { 1192 this.seriesDoses = castToType(value); // Type 1193 } else 1194 return super.setProperty(name, value); 1195 return value; 1196 } 1197 1198 @Override 1199 public void removeChild(String name, Base value) throws FHIRException { 1200 if (name.equals("identifier")) { 1201 this.getIdentifier().remove(castToIdentifier(value)); 1202 } else if (name.equals("status")) { 1203 this.status = null; 1204 } else if (name.equals("patient")) { 1205 this.patient = null; 1206 } else if (name.equals("date")) { 1207 this.date = null; 1208 } else if (name.equals("authority")) { 1209 this.authority = null; 1210 } else if (name.equals("targetDisease")) { 1211 this.targetDisease = null; 1212 } else if (name.equals("immunizationEvent")) { 1213 this.immunizationEvent = null; 1214 } else if (name.equals("doseStatus")) { 1215 this.doseStatus = null; 1216 } else if (name.equals("doseStatusReason")) { 1217 this.getDoseStatusReason().remove(castToCodeableConcept(value)); 1218 } else if (name.equals("description")) { 1219 this.description = null; 1220 } else if (name.equals("series")) { 1221 this.series = null; 1222 } else if (name.equals("doseNumber[x]")) { 1223 this.doseNumber = null; 1224 } else if (name.equals("seriesDoses[x]")) { 1225 this.seriesDoses = null; 1226 } else 1227 super.removeChild(name, value); 1228 1229 } 1230 1231 @Override 1232 public Base makeProperty(int hash, String name) throws FHIRException { 1233 switch (hash) { 1234 case -1618432855: 1235 return addIdentifier(); 1236 case -892481550: 1237 return getStatusElement(); 1238 case -791418107: 1239 return getPatient(); 1240 case 3076014: 1241 return getDateElement(); 1242 case 1475610435: 1243 return getAuthority(); 1244 case -319593813: 1245 return getTargetDisease(); 1246 case 1081446840: 1247 return getImmunizationEvent(); 1248 case -745826705: 1249 return getDoseStatus(); 1250 case 662783379: 1251 return addDoseStatusReason(); 1252 case -1724546052: 1253 return getDescriptionElement(); 1254 case -905838985: 1255 return getSeriesElement(); 1256 case -1632295686: 1257 return getDoseNumber(); 1258 case -887709242: 1259 return getDoseNumber(); 1260 case 1553560673: 1261 return getSeriesDoses(); 1262 case -1936727105: 1263 return getSeriesDoses(); 1264 default: 1265 return super.makeProperty(hash, name); 1266 } 1267 1268 } 1269 1270 @Override 1271 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1272 switch (hash) { 1273 case -1618432855: 1274 /* identifier */ return new String[] { "Identifier" }; 1275 case -892481550: 1276 /* status */ return new String[] { "code" }; 1277 case -791418107: 1278 /* patient */ return new String[] { "Reference" }; 1279 case 3076014: 1280 /* date */ return new String[] { "dateTime" }; 1281 case 1475610435: 1282 /* authority */ return new String[] { "Reference" }; 1283 case -319593813: 1284 /* targetDisease */ return new String[] { "CodeableConcept" }; 1285 case 1081446840: 1286 /* immunizationEvent */ return new String[] { "Reference" }; 1287 case -745826705: 1288 /* doseStatus */ return new String[] { "CodeableConcept" }; 1289 case 662783379: 1290 /* doseStatusReason */ return new String[] { "CodeableConcept" }; 1291 case -1724546052: 1292 /* description */ return new String[] { "string" }; 1293 case -905838985: 1294 /* series */ return new String[] { "string" }; 1295 case -887709242: 1296 /* doseNumber */ return new String[] { "positiveInt", "string" }; 1297 case -1936727105: 1298 /* seriesDoses */ return new String[] { "positiveInt", "string" }; 1299 default: 1300 return super.getTypesForProperty(hash, name); 1301 } 1302 1303 } 1304 1305 @Override 1306 public Base addChild(String name) throws FHIRException { 1307 if (name.equals("identifier")) { 1308 return addIdentifier(); 1309 } else if (name.equals("status")) { 1310 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.status"); 1311 } else if (name.equals("patient")) { 1312 this.patient = new Reference(); 1313 return this.patient; 1314 } else if (name.equals("date")) { 1315 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.date"); 1316 } else if (name.equals("authority")) { 1317 this.authority = new Reference(); 1318 return this.authority; 1319 } else if (name.equals("targetDisease")) { 1320 this.targetDisease = new CodeableConcept(); 1321 return this.targetDisease; 1322 } else if (name.equals("immunizationEvent")) { 1323 this.immunizationEvent = new Reference(); 1324 return this.immunizationEvent; 1325 } else if (name.equals("doseStatus")) { 1326 this.doseStatus = new CodeableConcept(); 1327 return this.doseStatus; 1328 } else if (name.equals("doseStatusReason")) { 1329 return addDoseStatusReason(); 1330 } else if (name.equals("description")) { 1331 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.description"); 1332 } else if (name.equals("series")) { 1333 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.series"); 1334 } else if (name.equals("doseNumberPositiveInt")) { 1335 this.doseNumber = new PositiveIntType(); 1336 return this.doseNumber; 1337 } else if (name.equals("doseNumberString")) { 1338 this.doseNumber = new StringType(); 1339 return this.doseNumber; 1340 } else if (name.equals("seriesDosesPositiveInt")) { 1341 this.seriesDoses = new PositiveIntType(); 1342 return this.seriesDoses; 1343 } else if (name.equals("seriesDosesString")) { 1344 this.seriesDoses = new StringType(); 1345 return this.seriesDoses; 1346 } else 1347 return super.addChild(name); 1348 } 1349 1350 public String fhirType() { 1351 return "ImmunizationEvaluation"; 1352 1353 } 1354 1355 public ImmunizationEvaluation copy() { 1356 ImmunizationEvaluation dst = new ImmunizationEvaluation(); 1357 copyValues(dst); 1358 return dst; 1359 } 1360 1361 public void copyValues(ImmunizationEvaluation dst) { 1362 super.copyValues(dst); 1363 if (identifier != null) { 1364 dst.identifier = new ArrayList<Identifier>(); 1365 for (Identifier i : identifier) 1366 dst.identifier.add(i.copy()); 1367 } 1368 ; 1369 dst.status = status == null ? null : status.copy(); 1370 dst.patient = patient == null ? null : patient.copy(); 1371 dst.date = date == null ? null : date.copy(); 1372 dst.authority = authority == null ? null : authority.copy(); 1373 dst.targetDisease = targetDisease == null ? null : targetDisease.copy(); 1374 dst.immunizationEvent = immunizationEvent == null ? null : immunizationEvent.copy(); 1375 dst.doseStatus = doseStatus == null ? null : doseStatus.copy(); 1376 if (doseStatusReason != null) { 1377 dst.doseStatusReason = new ArrayList<CodeableConcept>(); 1378 for (CodeableConcept i : doseStatusReason) 1379 dst.doseStatusReason.add(i.copy()); 1380 } 1381 ; 1382 dst.description = description == null ? null : description.copy(); 1383 dst.series = series == null ? null : series.copy(); 1384 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1385 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1386 } 1387 1388 protected ImmunizationEvaluation typedCopy() { 1389 return copy(); 1390 } 1391 1392 @Override 1393 public boolean equalsDeep(Base other_) { 1394 if (!super.equalsDeep(other_)) 1395 return false; 1396 if (!(other_ instanceof ImmunizationEvaluation)) 1397 return false; 1398 ImmunizationEvaluation o = (ImmunizationEvaluation) other_; 1399 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1400 && compareDeep(patient, o.patient, true) && compareDeep(date, o.date, true) 1401 && compareDeep(authority, o.authority, true) && compareDeep(targetDisease, o.targetDisease, true) 1402 && compareDeep(immunizationEvent, o.immunizationEvent, true) && compareDeep(doseStatus, o.doseStatus, true) 1403 && compareDeep(doseStatusReason, o.doseStatusReason, true) && compareDeep(description, o.description, true) 1404 && compareDeep(series, o.series, true) && compareDeep(doseNumber, o.doseNumber, true) 1405 && compareDeep(seriesDoses, o.seriesDoses, true); 1406 } 1407 1408 @Override 1409 public boolean equalsShallow(Base other_) { 1410 if (!super.equalsShallow(other_)) 1411 return false; 1412 if (!(other_ instanceof ImmunizationEvaluation)) 1413 return false; 1414 ImmunizationEvaluation o = (ImmunizationEvaluation) other_; 1415 return compareValues(status, o.status, true) && compareValues(date, o.date, true) 1416 && compareValues(description, o.description, true) && compareValues(series, o.series, true); 1417 } 1418 1419 public boolean isEmpty() { 1420 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, patient, date, authority, 1421 targetDisease, immunizationEvent, doseStatus, doseStatusReason, description, series, doseNumber, seriesDoses); 1422 } 1423 1424 @Override 1425 public ResourceType getResourceType() { 1426 return ResourceType.ImmunizationEvaluation; 1427 } 1428 1429 /** 1430 * Search parameter: <b>date</b> 1431 * <p> 1432 * Description: <b>Date the evaluation was generated</b><br> 1433 * Type: <b>date</b><br> 1434 * Path: <b>ImmunizationEvaluation.date</b><br> 1435 * </p> 1436 */ 1437 @SearchParamDefinition(name = "date", path = "ImmunizationEvaluation.date", description = "Date the evaluation was generated", type = "date") 1438 public static final String SP_DATE = "date"; 1439 /** 1440 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1441 * <p> 1442 * Description: <b>Date the evaluation was generated</b><br> 1443 * Type: <b>date</b><br> 1444 * Path: <b>ImmunizationEvaluation.date</b><br> 1445 * </p> 1446 */ 1447 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1448 SP_DATE); 1449 1450 /** 1451 * Search parameter: <b>identifier</b> 1452 * <p> 1453 * Description: <b>ID of the evaluation</b><br> 1454 * Type: <b>token</b><br> 1455 * Path: <b>ImmunizationEvaluation.identifier</b><br> 1456 * </p> 1457 */ 1458 @SearchParamDefinition(name = "identifier", path = "ImmunizationEvaluation.identifier", description = "ID of the evaluation", type = "token") 1459 public static final String SP_IDENTIFIER = "identifier"; 1460 /** 1461 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1462 * <p> 1463 * Description: <b>ID of the evaluation</b><br> 1464 * Type: <b>token</b><br> 1465 * Path: <b>ImmunizationEvaluation.identifier</b><br> 1466 * </p> 1467 */ 1468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1469 SP_IDENTIFIER); 1470 1471 /** 1472 * Search parameter: <b>target-disease</b> 1473 * <p> 1474 * Description: <b>The vaccine preventable disease being evaluated 1475 * against</b><br> 1476 * Type: <b>token</b><br> 1477 * Path: <b>ImmunizationEvaluation.targetDisease</b><br> 1478 * </p> 1479 */ 1480 @SearchParamDefinition(name = "target-disease", path = "ImmunizationEvaluation.targetDisease", description = "The vaccine preventable disease being evaluated against", type = "token") 1481 public static final String SP_TARGET_DISEASE = "target-disease"; 1482 /** 1483 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 1484 * <p> 1485 * Description: <b>The vaccine preventable disease being evaluated 1486 * against</b><br> 1487 * Type: <b>token</b><br> 1488 * Path: <b>ImmunizationEvaluation.targetDisease</b><br> 1489 * </p> 1490 */ 1491 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1492 SP_TARGET_DISEASE); 1493 1494 /** 1495 * Search parameter: <b>patient</b> 1496 * <p> 1497 * Description: <b>The patient being evaluated</b><br> 1498 * Type: <b>reference</b><br> 1499 * Path: <b>ImmunizationEvaluation.patient</b><br> 1500 * </p> 1501 */ 1502 @SearchParamDefinition(name = "patient", path = "ImmunizationEvaluation.patient", description = "The patient being evaluated", type = "reference", providesMembershipIn = { 1503 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1504 public static final String SP_PATIENT = "patient"; 1505 /** 1506 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1507 * <p> 1508 * Description: <b>The patient being evaluated</b><br> 1509 * Type: <b>reference</b><br> 1510 * Path: <b>ImmunizationEvaluation.patient</b><br> 1511 * </p> 1512 */ 1513 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1514 SP_PATIENT); 1515 1516 /** 1517 * Constant for fluent queries to be used to add include statements. Specifies 1518 * the path value of "<b>ImmunizationEvaluation:patient</b>". 1519 */ 1520 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1521 "ImmunizationEvaluation:patient").toLocked(); 1522 1523 /** 1524 * Search parameter: <b>dose-status</b> 1525 * <p> 1526 * Description: <b>The status of the dose relative to published 1527 * recommendations</b><br> 1528 * Type: <b>token</b><br> 1529 * Path: <b>ImmunizationEvaluation.doseStatus</b><br> 1530 * </p> 1531 */ 1532 @SearchParamDefinition(name = "dose-status", path = "ImmunizationEvaluation.doseStatus", description = "The status of the dose relative to published recommendations", type = "token") 1533 public static final String SP_DOSE_STATUS = "dose-status"; 1534 /** 1535 * <b>Fluent Client</b> search parameter constant for <b>dose-status</b> 1536 * <p> 1537 * Description: <b>The status of the dose relative to published 1538 * recommendations</b><br> 1539 * Type: <b>token</b><br> 1540 * Path: <b>ImmunizationEvaluation.doseStatus</b><br> 1541 * </p> 1542 */ 1543 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSE_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1544 SP_DOSE_STATUS); 1545 1546 /** 1547 * Search parameter: <b>immunization-event</b> 1548 * <p> 1549 * Description: <b>The vaccine administration event being evaluated</b><br> 1550 * Type: <b>reference</b><br> 1551 * Path: <b>ImmunizationEvaluation.immunizationEvent</b><br> 1552 * </p> 1553 */ 1554 @SearchParamDefinition(name = "immunization-event", path = "ImmunizationEvaluation.immunizationEvent", description = "The vaccine administration event being evaluated", type = "reference", target = { 1555 Immunization.class }) 1556 public static final String SP_IMMUNIZATION_EVENT = "immunization-event"; 1557 /** 1558 * <b>Fluent Client</b> search parameter constant for <b>immunization-event</b> 1559 * <p> 1560 * Description: <b>The vaccine administration event being evaluated</b><br> 1561 * Type: <b>reference</b><br> 1562 * Path: <b>ImmunizationEvaluation.immunizationEvent</b><br> 1563 * </p> 1564 */ 1565 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMMUNIZATION_EVENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1566 SP_IMMUNIZATION_EVENT); 1567 1568 /** 1569 * Constant for fluent queries to be used to add include statements. Specifies 1570 * the path value of "<b>ImmunizationEvaluation:immunization-event</b>". 1571 */ 1572 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMMUNIZATION_EVENT = new ca.uhn.fhir.model.api.Include( 1573 "ImmunizationEvaluation:immunization-event").toLocked(); 1574 1575 /** 1576 * Search parameter: <b>status</b> 1577 * <p> 1578 * Description: <b>Immunization evaluation status</b><br> 1579 * Type: <b>token</b><br> 1580 * Path: <b>ImmunizationEvaluation.status</b><br> 1581 * </p> 1582 */ 1583 @SearchParamDefinition(name = "status", path = "ImmunizationEvaluation.status", description = "Immunization evaluation status", type = "token") 1584 public static final String SP_STATUS = "status"; 1585 /** 1586 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1587 * <p> 1588 * Description: <b>Immunization evaluation status</b><br> 1589 * Type: <b>token</b><br> 1590 * Path: <b>ImmunizationEvaluation.status</b><br> 1591 * </p> 1592 */ 1593 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1594 SP_STATUS); 1595 1596}