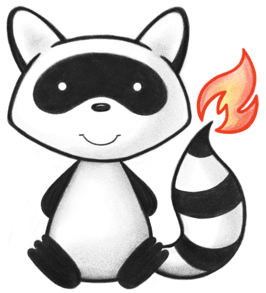
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A patient's point-in-time set of recommendations (i.e. forecasting) according 049 * to a published schedule with optional supporting justification. 050 */ 051@ResourceDef(name = "ImmunizationRecommendation", profile = "http://hl7.org/fhir/StructureDefinition/ImmunizationRecommendation") 052public class ImmunizationRecommendation extends DomainResource { 053 054 @Block() 055 public static class ImmunizationRecommendationRecommendationComponent extends BackboneElement 056 implements IBaseBackboneElement { 057 /** 058 * Vaccine(s) or vaccine group that pertain to the recommendation. 059 */ 060 @Child(name = "vaccineCode", type = { 061 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 062 @Description(shortDefinition = "Vaccine or vaccine group recommendation applies to", formalDefinition = "Vaccine(s) or vaccine group that pertain to the recommendation.") 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vaccine-code") 064 protected List<CodeableConcept> vaccineCode; 065 066 /** 067 * The targeted disease for the recommendation. 068 */ 069 @Child(name = "targetDisease", type = { 070 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 071 @Description(shortDefinition = "Disease to be immunized against", formalDefinition = "The targeted disease for the recommendation.") 072 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-target-disease") 073 protected CodeableConcept targetDisease; 074 075 /** 076 * Vaccine(s) which should not be used to fulfill the recommendation. 077 */ 078 @Child(name = "contraindicatedVaccineCode", type = { 079 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 080 @Description(shortDefinition = "Vaccine which is contraindicated to fulfill the recommendation", formalDefinition = "Vaccine(s) which should not be used to fulfill the recommendation.") 081 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vaccine-code") 082 protected List<CodeableConcept> contraindicatedVaccineCode; 083 084 /** 085 * Indicates the patient status with respect to the path to immunity for the 086 * target disease. 087 */ 088 @Child(name = "forecastStatus", type = { 089 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 090 @Description(shortDefinition = "Vaccine recommendation status", formalDefinition = "Indicates the patient status with respect to the path to immunity for the target disease.") 091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-status") 092 protected CodeableConcept forecastStatus; 093 094 /** 095 * The reason for the assigned forecast status. 096 */ 097 @Child(name = "forecastReason", type = { 098 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 099 @Description(shortDefinition = "Vaccine administration status reason", formalDefinition = "The reason for the assigned forecast status.") 100 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-reason") 101 protected List<CodeableConcept> forecastReason; 102 103 /** 104 * Vaccine date recommendations. For example, earliest date to administer, 105 * latest date to administer, etc. 106 */ 107 @Child(name = "dateCriterion", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 108 @Description(shortDefinition = "Dates governing proposed immunization", formalDefinition = "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.") 109 protected List<ImmunizationRecommendationRecommendationDateCriterionComponent> dateCriterion; 110 111 /** 112 * Contains the description about the protocol under which the vaccine was 113 * administered. 114 */ 115 @Child(name = "description", type = { 116 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 117 @Description(shortDefinition = "Protocol details", formalDefinition = "Contains the description about the protocol under which the vaccine was administered.") 118 protected StringType description; 119 120 /** 121 * One possible path to achieve presumed immunity against a disease - within the 122 * context of an authority. 123 */ 124 @Child(name = "series", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 125 @Description(shortDefinition = "Name of vaccination series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 126 protected StringType series; 127 128 /** 129 * Nominal position of the recommended dose in a series (e.g. dose 2 is the next 130 * recommended dose). 131 */ 132 @Child(name = "doseNumber", type = { PositiveIntType.class, 133 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 134 @Description(shortDefinition = "Recommended dose number within series", formalDefinition = "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).") 135 protected Type doseNumber; 136 137 /** 138 * The recommended number of doses to achieve immunity. 139 */ 140 @Child(name = "seriesDoses", type = { PositiveIntType.class, 141 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 142 @Description(shortDefinition = "Recommended number of doses for immunity", formalDefinition = "The recommended number of doses to achieve immunity.") 143 protected Type seriesDoses; 144 145 /** 146 * Immunization event history and/or evaluation that supports the status and 147 * recommendation. 148 */ 149 @Child(name = "supportingImmunization", type = { Immunization.class, 150 ImmunizationEvaluation.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 151 @Description(shortDefinition = "Past immunizations supporting recommendation", formalDefinition = "Immunization event history and/or evaluation that supports the status and recommendation.") 152 protected List<Reference> supportingImmunization; 153 /** 154 * The actual objects that are the target of the reference (Immunization event 155 * history and/or evaluation that supports the status and recommendation.) 156 */ 157 protected List<Resource> supportingImmunizationTarget; 158 159 /** 160 * Patient Information that supports the status and recommendation. This 161 * includes patient observations, adverse reactions and allergy/intolerance 162 * information. 163 */ 164 @Child(name = "supportingPatientInformation", type = { 165 Reference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 166 @Description(shortDefinition = "Patient observations supporting recommendation", formalDefinition = "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.") 167 protected List<Reference> supportingPatientInformation; 168 /** 169 * The actual objects that are the target of the reference (Patient Information 170 * that supports the status and recommendation. This includes patient 171 * observations, adverse reactions and allergy/intolerance information.) 172 */ 173 protected List<Resource> supportingPatientInformationTarget; 174 175 private static final long serialVersionUID = -667399405L; 176 177 /** 178 * Constructor 179 */ 180 public ImmunizationRecommendationRecommendationComponent() { 181 super(); 182 } 183 184 /** 185 * Constructor 186 */ 187 public ImmunizationRecommendationRecommendationComponent(CodeableConcept forecastStatus) { 188 super(); 189 this.forecastStatus = forecastStatus; 190 } 191 192 /** 193 * @return {@link #vaccineCode} (Vaccine(s) or vaccine group that pertain to the 194 * recommendation.) 195 */ 196 public List<CodeableConcept> getVaccineCode() { 197 if (this.vaccineCode == null) 198 this.vaccineCode = new ArrayList<CodeableConcept>(); 199 return this.vaccineCode; 200 } 201 202 /** 203 * @return Returns a reference to <code>this</code> for easy method chaining 204 */ 205 public ImmunizationRecommendationRecommendationComponent setVaccineCode(List<CodeableConcept> theVaccineCode) { 206 this.vaccineCode = theVaccineCode; 207 return this; 208 } 209 210 public boolean hasVaccineCode() { 211 if (this.vaccineCode == null) 212 return false; 213 for (CodeableConcept item : this.vaccineCode) 214 if (!item.isEmpty()) 215 return true; 216 return false; 217 } 218 219 public CodeableConcept addVaccineCode() { // 3 220 CodeableConcept t = new CodeableConcept(); 221 if (this.vaccineCode == null) 222 this.vaccineCode = new ArrayList<CodeableConcept>(); 223 this.vaccineCode.add(t); 224 return t; 225 } 226 227 public ImmunizationRecommendationRecommendationComponent addVaccineCode(CodeableConcept t) { // 3 228 if (t == null) 229 return this; 230 if (this.vaccineCode == null) 231 this.vaccineCode = new ArrayList<CodeableConcept>(); 232 this.vaccineCode.add(t); 233 return this; 234 } 235 236 /** 237 * @return The first repetition of repeating field {@link #vaccineCode}, 238 * creating it if it does not already exist 239 */ 240 public CodeableConcept getVaccineCodeFirstRep() { 241 if (getVaccineCode().isEmpty()) { 242 addVaccineCode(); 243 } 244 return getVaccineCode().get(0); 245 } 246 247 /** 248 * @return {@link #targetDisease} (The targeted disease for the recommendation.) 249 */ 250 public CodeableConcept getTargetDisease() { 251 if (this.targetDisease == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.targetDisease"); 254 else if (Configuration.doAutoCreate()) 255 this.targetDisease = new CodeableConcept(); // cc 256 return this.targetDisease; 257 } 258 259 public boolean hasTargetDisease() { 260 return this.targetDisease != null && !this.targetDisease.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #targetDisease} (The targeted disease for the 265 * recommendation.) 266 */ 267 public ImmunizationRecommendationRecommendationComponent setTargetDisease(CodeableConcept value) { 268 this.targetDisease = value; 269 return this; 270 } 271 272 /** 273 * @return {@link #contraindicatedVaccineCode} (Vaccine(s) which should not be 274 * used to fulfill the recommendation.) 275 */ 276 public List<CodeableConcept> getContraindicatedVaccineCode() { 277 if (this.contraindicatedVaccineCode == null) 278 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 279 return this.contraindicatedVaccineCode; 280 } 281 282 /** 283 * @return Returns a reference to <code>this</code> for easy method chaining 284 */ 285 public ImmunizationRecommendationRecommendationComponent setContraindicatedVaccineCode( 286 List<CodeableConcept> theContraindicatedVaccineCode) { 287 this.contraindicatedVaccineCode = theContraindicatedVaccineCode; 288 return this; 289 } 290 291 public boolean hasContraindicatedVaccineCode() { 292 if (this.contraindicatedVaccineCode == null) 293 return false; 294 for (CodeableConcept item : this.contraindicatedVaccineCode) 295 if (!item.isEmpty()) 296 return true; 297 return false; 298 } 299 300 public CodeableConcept addContraindicatedVaccineCode() { // 3 301 CodeableConcept t = new CodeableConcept(); 302 if (this.contraindicatedVaccineCode == null) 303 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 304 this.contraindicatedVaccineCode.add(t); 305 return t; 306 } 307 308 public ImmunizationRecommendationRecommendationComponent addContraindicatedVaccineCode(CodeableConcept t) { // 3 309 if (t == null) 310 return this; 311 if (this.contraindicatedVaccineCode == null) 312 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 313 this.contraindicatedVaccineCode.add(t); 314 return this; 315 } 316 317 /** 318 * @return The first repetition of repeating field 319 * {@link #contraindicatedVaccineCode}, creating it if it does not 320 * already exist 321 */ 322 public CodeableConcept getContraindicatedVaccineCodeFirstRep() { 323 if (getContraindicatedVaccineCode().isEmpty()) { 324 addContraindicatedVaccineCode(); 325 } 326 return getContraindicatedVaccineCode().get(0); 327 } 328 329 /** 330 * @return {@link #forecastStatus} (Indicates the patient status with respect to 331 * the path to immunity for the target disease.) 332 */ 333 public CodeableConcept getForecastStatus() { 334 if (this.forecastStatus == null) 335 if (Configuration.errorOnAutoCreate()) 336 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.forecastStatus"); 337 else if (Configuration.doAutoCreate()) 338 this.forecastStatus = new CodeableConcept(); // cc 339 return this.forecastStatus; 340 } 341 342 public boolean hasForecastStatus() { 343 return this.forecastStatus != null && !this.forecastStatus.isEmpty(); 344 } 345 346 /** 347 * @param value {@link #forecastStatus} (Indicates the patient status with 348 * respect to the path to immunity for the target disease.) 349 */ 350 public ImmunizationRecommendationRecommendationComponent setForecastStatus(CodeableConcept value) { 351 this.forecastStatus = value; 352 return this; 353 } 354 355 /** 356 * @return {@link #forecastReason} (The reason for the assigned forecast 357 * status.) 358 */ 359 public List<CodeableConcept> getForecastReason() { 360 if (this.forecastReason == null) 361 this.forecastReason = new ArrayList<CodeableConcept>(); 362 return this.forecastReason; 363 } 364 365 /** 366 * @return Returns a reference to <code>this</code> for easy method chaining 367 */ 368 public ImmunizationRecommendationRecommendationComponent setForecastReason( 369 List<CodeableConcept> theForecastReason) { 370 this.forecastReason = theForecastReason; 371 return this; 372 } 373 374 public boolean hasForecastReason() { 375 if (this.forecastReason == null) 376 return false; 377 for (CodeableConcept item : this.forecastReason) 378 if (!item.isEmpty()) 379 return true; 380 return false; 381 } 382 383 public CodeableConcept addForecastReason() { // 3 384 CodeableConcept t = new CodeableConcept(); 385 if (this.forecastReason == null) 386 this.forecastReason = new ArrayList<CodeableConcept>(); 387 this.forecastReason.add(t); 388 return t; 389 } 390 391 public ImmunizationRecommendationRecommendationComponent addForecastReason(CodeableConcept t) { // 3 392 if (t == null) 393 return this; 394 if (this.forecastReason == null) 395 this.forecastReason = new ArrayList<CodeableConcept>(); 396 this.forecastReason.add(t); 397 return this; 398 } 399 400 /** 401 * @return The first repetition of repeating field {@link #forecastReason}, 402 * creating it if it does not already exist 403 */ 404 public CodeableConcept getForecastReasonFirstRep() { 405 if (getForecastReason().isEmpty()) { 406 addForecastReason(); 407 } 408 return getForecastReason().get(0); 409 } 410 411 /** 412 * @return {@link #dateCriterion} (Vaccine date recommendations. For example, 413 * earliest date to administer, latest date to administer, etc.) 414 */ 415 public List<ImmunizationRecommendationRecommendationDateCriterionComponent> getDateCriterion() { 416 if (this.dateCriterion == null) 417 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 418 return this.dateCriterion; 419 } 420 421 /** 422 * @return Returns a reference to <code>this</code> for easy method chaining 423 */ 424 public ImmunizationRecommendationRecommendationComponent setDateCriterion( 425 List<ImmunizationRecommendationRecommendationDateCriterionComponent> theDateCriterion) { 426 this.dateCriterion = theDateCriterion; 427 return this; 428 } 429 430 public boolean hasDateCriterion() { 431 if (this.dateCriterion == null) 432 return false; 433 for (ImmunizationRecommendationRecommendationDateCriterionComponent item : this.dateCriterion) 434 if (!item.isEmpty()) 435 return true; 436 return false; 437 } 438 439 public ImmunizationRecommendationRecommendationDateCriterionComponent addDateCriterion() { // 3 440 ImmunizationRecommendationRecommendationDateCriterionComponent t = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 441 if (this.dateCriterion == null) 442 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 443 this.dateCriterion.add(t); 444 return t; 445 } 446 447 public ImmunizationRecommendationRecommendationComponent addDateCriterion( 448 ImmunizationRecommendationRecommendationDateCriterionComponent t) { // 3 449 if (t == null) 450 return this; 451 if (this.dateCriterion == null) 452 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 453 this.dateCriterion.add(t); 454 return this; 455 } 456 457 /** 458 * @return The first repetition of repeating field {@link #dateCriterion}, 459 * creating it if it does not already exist 460 */ 461 public ImmunizationRecommendationRecommendationDateCriterionComponent getDateCriterionFirstRep() { 462 if (getDateCriterion().isEmpty()) { 463 addDateCriterion(); 464 } 465 return getDateCriterion().get(0); 466 } 467 468 /** 469 * @return {@link #description} (Contains the description about the protocol 470 * under which the vaccine was administered.). This is the underlying 471 * object with id, value and extensions. The accessor "getDescription" 472 * gives direct access to the value 473 */ 474 public StringType getDescriptionElement() { 475 if (this.description == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.description"); 478 else if (Configuration.doAutoCreate()) 479 this.description = new StringType(); // bb 480 return this.description; 481 } 482 483 public boolean hasDescriptionElement() { 484 return this.description != null && !this.description.isEmpty(); 485 } 486 487 public boolean hasDescription() { 488 return this.description != null && !this.description.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #description} (Contains the description about the 493 * protocol under which the vaccine was administered.). This is the 494 * underlying object with id, value and extensions. The accessor 495 * "getDescription" gives direct access to the value 496 */ 497 public ImmunizationRecommendationRecommendationComponent setDescriptionElement(StringType value) { 498 this.description = value; 499 return this; 500 } 501 502 /** 503 * @return Contains the description about the protocol under which the vaccine 504 * was administered. 505 */ 506 public String getDescription() { 507 return this.description == null ? null : this.description.getValue(); 508 } 509 510 /** 511 * @param value Contains the description about the protocol under which the 512 * vaccine was administered. 513 */ 514 public ImmunizationRecommendationRecommendationComponent setDescription(String value) { 515 if (Utilities.noString(value)) 516 this.description = null; 517 else { 518 if (this.description == null) 519 this.description = new StringType(); 520 this.description.setValue(value); 521 } 522 return this; 523 } 524 525 /** 526 * @return {@link #series} (One possible path to achieve presumed immunity 527 * against a disease - within the context of an authority.). This is the 528 * underlying object with id, value and extensions. The accessor 529 * "getSeries" gives direct access to the value 530 */ 531 public StringType getSeriesElement() { 532 if (this.series == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.series"); 535 else if (Configuration.doAutoCreate()) 536 this.series = new StringType(); // bb 537 return this.series; 538 } 539 540 public boolean hasSeriesElement() { 541 return this.series != null && !this.series.isEmpty(); 542 } 543 544 public boolean hasSeries() { 545 return this.series != null && !this.series.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #series} (One possible path to achieve presumed immunity 550 * against a disease - within the context of an authority.). This 551 * is the underlying object with id, value and extensions. The 552 * accessor "getSeries" gives direct access to the value 553 */ 554 public ImmunizationRecommendationRecommendationComponent setSeriesElement(StringType value) { 555 this.series = value; 556 return this; 557 } 558 559 /** 560 * @return One possible path to achieve presumed immunity against a disease - 561 * within the context of an authority. 562 */ 563 public String getSeries() { 564 return this.series == null ? null : this.series.getValue(); 565 } 566 567 /** 568 * @param value One possible path to achieve presumed immunity against a disease 569 * - within the context of an authority. 570 */ 571 public ImmunizationRecommendationRecommendationComponent setSeries(String value) { 572 if (Utilities.noString(value)) 573 this.series = null; 574 else { 575 if (this.series == null) 576 this.series = new StringType(); 577 this.series.setValue(value); 578 } 579 return this; 580 } 581 582 /** 583 * @return {@link #doseNumber} (Nominal position of the recommended dose in a 584 * series (e.g. dose 2 is the next recommended dose).) 585 */ 586 public Type getDoseNumber() { 587 return this.doseNumber; 588 } 589 590 /** 591 * @return {@link #doseNumber} (Nominal position of the recommended dose in a 592 * series (e.g. dose 2 is the next recommended dose).) 593 */ 594 public PositiveIntType getDoseNumberPositiveIntType() throws FHIRException { 595 if (this.doseNumber == null) 596 this.doseNumber = new PositiveIntType(); 597 if (!(this.doseNumber instanceof PositiveIntType)) 598 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 599 + this.doseNumber.getClass().getName() + " was encountered"); 600 return (PositiveIntType) this.doseNumber; 601 } 602 603 public boolean hasDoseNumberPositiveIntType() { 604 return this != null && this.doseNumber instanceof PositiveIntType; 605 } 606 607 /** 608 * @return {@link #doseNumber} (Nominal position of the recommended dose in a 609 * series (e.g. dose 2 is the next recommended dose).) 610 */ 611 public StringType getDoseNumberStringType() throws FHIRException { 612 if (this.doseNumber == null) 613 this.doseNumber = new StringType(); 614 if (!(this.doseNumber instanceof StringType)) 615 throw new FHIRException("Type mismatch: the type StringType was expected, but " 616 + this.doseNumber.getClass().getName() + " was encountered"); 617 return (StringType) this.doseNumber; 618 } 619 620 public boolean hasDoseNumberStringType() { 621 return this != null && this.doseNumber instanceof StringType; 622 } 623 624 public boolean hasDoseNumber() { 625 return this.doseNumber != null && !this.doseNumber.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #doseNumber} (Nominal position of the recommended dose in 630 * a series (e.g. dose 2 is the next recommended dose).) 631 */ 632 public ImmunizationRecommendationRecommendationComponent setDoseNumber(Type value) { 633 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 634 throw new Error( 635 "Not the right type for ImmunizationRecommendation.recommendation.doseNumber[x]: " + value.fhirType()); 636 this.doseNumber = value; 637 return this; 638 } 639 640 /** 641 * @return {@link #seriesDoses} (The recommended number of doses to achieve 642 * immunity.) 643 */ 644 public Type getSeriesDoses() { 645 return this.seriesDoses; 646 } 647 648 /** 649 * @return {@link #seriesDoses} (The recommended number of doses to achieve 650 * immunity.) 651 */ 652 public PositiveIntType getSeriesDosesPositiveIntType() throws FHIRException { 653 if (this.seriesDoses == null) 654 this.seriesDoses = new PositiveIntType(); 655 if (!(this.seriesDoses instanceof PositiveIntType)) 656 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 657 + this.seriesDoses.getClass().getName() + " was encountered"); 658 return (PositiveIntType) this.seriesDoses; 659 } 660 661 public boolean hasSeriesDosesPositiveIntType() { 662 return this != null && this.seriesDoses instanceof PositiveIntType; 663 } 664 665 /** 666 * @return {@link #seriesDoses} (The recommended number of doses to achieve 667 * immunity.) 668 */ 669 public StringType getSeriesDosesStringType() throws FHIRException { 670 if (this.seriesDoses == null) 671 this.seriesDoses = new StringType(); 672 if (!(this.seriesDoses instanceof StringType)) 673 throw new FHIRException("Type mismatch: the type StringType was expected, but " 674 + this.seriesDoses.getClass().getName() + " was encountered"); 675 return (StringType) this.seriesDoses; 676 } 677 678 public boolean hasSeriesDosesStringType() { 679 return this != null && this.seriesDoses instanceof StringType; 680 } 681 682 public boolean hasSeriesDoses() { 683 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #seriesDoses} (The recommended number of doses to achieve 688 * immunity.) 689 */ 690 public ImmunizationRecommendationRecommendationComponent setSeriesDoses(Type value) { 691 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 692 throw new Error( 693 "Not the right type for ImmunizationRecommendation.recommendation.seriesDoses[x]: " + value.fhirType()); 694 this.seriesDoses = value; 695 return this; 696 } 697 698 /** 699 * @return {@link #supportingImmunization} (Immunization event history and/or 700 * evaluation that supports the status and recommendation.) 701 */ 702 public List<Reference> getSupportingImmunization() { 703 if (this.supportingImmunization == null) 704 this.supportingImmunization = new ArrayList<Reference>(); 705 return this.supportingImmunization; 706 } 707 708 /** 709 * @return Returns a reference to <code>this</code> for easy method chaining 710 */ 711 public ImmunizationRecommendationRecommendationComponent setSupportingImmunization( 712 List<Reference> theSupportingImmunization) { 713 this.supportingImmunization = theSupportingImmunization; 714 return this; 715 } 716 717 public boolean hasSupportingImmunization() { 718 if (this.supportingImmunization == null) 719 return false; 720 for (Reference item : this.supportingImmunization) 721 if (!item.isEmpty()) 722 return true; 723 return false; 724 } 725 726 public Reference addSupportingImmunization() { // 3 727 Reference t = new Reference(); 728 if (this.supportingImmunization == null) 729 this.supportingImmunization = new ArrayList<Reference>(); 730 this.supportingImmunization.add(t); 731 return t; 732 } 733 734 public ImmunizationRecommendationRecommendationComponent addSupportingImmunization(Reference t) { // 3 735 if (t == null) 736 return this; 737 if (this.supportingImmunization == null) 738 this.supportingImmunization = new ArrayList<Reference>(); 739 this.supportingImmunization.add(t); 740 return this; 741 } 742 743 /** 744 * @return The first repetition of repeating field 745 * {@link #supportingImmunization}, creating it if it does not already 746 * exist 747 */ 748 public Reference getSupportingImmunizationFirstRep() { 749 if (getSupportingImmunization().isEmpty()) { 750 addSupportingImmunization(); 751 } 752 return getSupportingImmunization().get(0); 753 } 754 755 /** 756 * @deprecated Use Reference#setResource(IBaseResource) instead 757 */ 758 @Deprecated 759 public List<Resource> getSupportingImmunizationTarget() { 760 if (this.supportingImmunizationTarget == null) 761 this.supportingImmunizationTarget = new ArrayList<Resource>(); 762 return this.supportingImmunizationTarget; 763 } 764 765 /** 766 * @return {@link #supportingPatientInformation} (Patient Information that 767 * supports the status and recommendation. This includes patient 768 * observations, adverse reactions and allergy/intolerance information.) 769 */ 770 public List<Reference> getSupportingPatientInformation() { 771 if (this.supportingPatientInformation == null) 772 this.supportingPatientInformation = new ArrayList<Reference>(); 773 return this.supportingPatientInformation; 774 } 775 776 /** 777 * @return Returns a reference to <code>this</code> for easy method chaining 778 */ 779 public ImmunizationRecommendationRecommendationComponent setSupportingPatientInformation( 780 List<Reference> theSupportingPatientInformation) { 781 this.supportingPatientInformation = theSupportingPatientInformation; 782 return this; 783 } 784 785 public boolean hasSupportingPatientInformation() { 786 if (this.supportingPatientInformation == null) 787 return false; 788 for (Reference item : this.supportingPatientInformation) 789 if (!item.isEmpty()) 790 return true; 791 return false; 792 } 793 794 public Reference addSupportingPatientInformation() { // 3 795 Reference t = new Reference(); 796 if (this.supportingPatientInformation == null) 797 this.supportingPatientInformation = new ArrayList<Reference>(); 798 this.supportingPatientInformation.add(t); 799 return t; 800 } 801 802 public ImmunizationRecommendationRecommendationComponent addSupportingPatientInformation(Reference t) { // 3 803 if (t == null) 804 return this; 805 if (this.supportingPatientInformation == null) 806 this.supportingPatientInformation = new ArrayList<Reference>(); 807 this.supportingPatientInformation.add(t); 808 return this; 809 } 810 811 /** 812 * @return The first repetition of repeating field 813 * {@link #supportingPatientInformation}, creating it if it does not 814 * already exist 815 */ 816 public Reference getSupportingPatientInformationFirstRep() { 817 if (getSupportingPatientInformation().isEmpty()) { 818 addSupportingPatientInformation(); 819 } 820 return getSupportingPatientInformation().get(0); 821 } 822 823 /** 824 * @deprecated Use Reference#setResource(IBaseResource) instead 825 */ 826 @Deprecated 827 public List<Resource> getSupportingPatientInformationTarget() { 828 if (this.supportingPatientInformationTarget == null) 829 this.supportingPatientInformationTarget = new ArrayList<Resource>(); 830 return this.supportingPatientInformationTarget; 831 } 832 833 protected void listChildren(List<Property> children) { 834 super.listChildren(children); 835 children.add(new Property("vaccineCode", "CodeableConcept", 836 "Vaccine(s) or vaccine group that pertain to the recommendation.", 0, java.lang.Integer.MAX_VALUE, 837 vaccineCode)); 838 children.add(new Property("targetDisease", "CodeableConcept", "The targeted disease for the recommendation.", 0, 839 1, targetDisease)); 840 children.add(new Property("contraindicatedVaccineCode", "CodeableConcept", 841 "Vaccine(s) which should not be used to fulfill the recommendation.", 0, java.lang.Integer.MAX_VALUE, 842 contraindicatedVaccineCode)); 843 children.add(new Property("forecastStatus", "CodeableConcept", 844 "Indicates the patient status with respect to the path to immunity for the target disease.", 0, 1, 845 forecastStatus)); 846 children.add(new Property("forecastReason", "CodeableConcept", "The reason for the assigned forecast status.", 0, 847 java.lang.Integer.MAX_VALUE, forecastReason)); 848 children.add(new Property("dateCriterion", "", 849 "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, 850 java.lang.Integer.MAX_VALUE, dateCriterion)); 851 children.add(new Property("description", "string", 852 "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description)); 853 children.add(new Property("series", "string", 854 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 855 1, series)); 856 children.add(new Property("doseNumber[x]", "positiveInt|string", 857 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 858 doseNumber)); 859 children.add(new Property("seriesDoses[x]", "positiveInt|string", 860 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 861 children.add(new Property("supportingImmunization", "Reference(Immunization|ImmunizationEvaluation)", 862 "Immunization event history and/or evaluation that supports the status and recommendation.", 0, 863 java.lang.Integer.MAX_VALUE, supportingImmunization)); 864 children.add(new Property("supportingPatientInformation", "Reference(Any)", 865 "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 866 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation)); 867 } 868 869 @Override 870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 871 switch (_hash) { 872 case 664556354: 873 /* vaccineCode */ return new Property("vaccineCode", "CodeableConcept", 874 "Vaccine(s) or vaccine group that pertain to the recommendation.", 0, java.lang.Integer.MAX_VALUE, 875 vaccineCode); 876 case -319593813: 877 /* targetDisease */ return new Property("targetDisease", "CodeableConcept", 878 "The targeted disease for the recommendation.", 0, 1, targetDisease); 879 case 571105240: 880 /* contraindicatedVaccineCode */ return new Property("contraindicatedVaccineCode", "CodeableConcept", 881 "Vaccine(s) which should not be used to fulfill the recommendation.", 0, java.lang.Integer.MAX_VALUE, 882 contraindicatedVaccineCode); 883 case 1904598477: 884 /* forecastStatus */ return new Property("forecastStatus", "CodeableConcept", 885 "Indicates the patient status with respect to the path to immunity for the target disease.", 0, 1, 886 forecastStatus); 887 case 1862115359: 888 /* forecastReason */ return new Property("forecastReason", "CodeableConcept", 889 "The reason for the assigned forecast status.", 0, java.lang.Integer.MAX_VALUE, forecastReason); 890 case 2087518867: 891 /* dateCriterion */ return new Property("dateCriterion", "", 892 "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 893 0, java.lang.Integer.MAX_VALUE, dateCriterion); 894 case -1724546052: 895 /* description */ return new Property("description", "string", 896 "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description); 897 case -905838985: 898 /* series */ return new Property("series", "string", 899 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 900 1, series); 901 case -1632295686: 902 /* doseNumber[x] */ return new Property("doseNumber[x]", "positiveInt|string", 903 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 904 doseNumber); 905 case -887709242: 906 /* doseNumber */ return new Property("doseNumber[x]", "positiveInt|string", 907 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 908 doseNumber); 909 case -1826134640: 910 /* doseNumberPositiveInt */ return new Property("doseNumber[x]", "positiveInt|string", 911 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 912 doseNumber); 913 case -333053577: 914 /* doseNumberString */ return new Property("doseNumber[x]", "positiveInt|string", 915 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 916 doseNumber); 917 case 1553560673: 918 /* seriesDoses[x] */ return new Property("seriesDoses[x]", "positiveInt|string", 919 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 920 case -1936727105: 921 /* seriesDoses */ return new Property("seriesDoses[x]", "positiveInt|string", 922 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 923 case -220897801: 924 /* seriesDosesPositiveInt */ return new Property("seriesDoses[x]", "positiveInt|string", 925 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 926 case -673569616: 927 /* seriesDosesString */ return new Property("seriesDoses[x]", "positiveInt|string", 928 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 929 case 1171592021: 930 /* supportingImmunization */ return new Property("supportingImmunization", 931 "Reference(Immunization|ImmunizationEvaluation)", 932 "Immunization event history and/or evaluation that supports the status and recommendation.", 0, 933 java.lang.Integer.MAX_VALUE, supportingImmunization); 934 case -1234160646: 935 /* supportingPatientInformation */ return new Property("supportingPatientInformation", "Reference(Any)", 936 "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 937 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation); 938 default: 939 return super.getNamedProperty(_hash, _name, _checkValid); 940 } 941 942 } 943 944 @Override 945 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 946 switch (hash) { 947 case 664556354: 948 /* vaccineCode */ return this.vaccineCode == null ? new Base[0] 949 : this.vaccineCode.toArray(new Base[this.vaccineCode.size()]); // CodeableConcept 950 case -319593813: 951 /* targetDisease */ return this.targetDisease == null ? new Base[0] : new Base[] { this.targetDisease }; // CodeableConcept 952 case 571105240: 953 /* contraindicatedVaccineCode */ return this.contraindicatedVaccineCode == null ? new Base[0] 954 : this.contraindicatedVaccineCode.toArray(new Base[this.contraindicatedVaccineCode.size()]); // CodeableConcept 955 case 1904598477: 956 /* forecastStatus */ return this.forecastStatus == null ? new Base[0] : new Base[] { this.forecastStatus }; // CodeableConcept 957 case 1862115359: 958 /* forecastReason */ return this.forecastReason == null ? new Base[0] 959 : this.forecastReason.toArray(new Base[this.forecastReason.size()]); // CodeableConcept 960 case 2087518867: 961 /* dateCriterion */ return this.dateCriterion == null ? new Base[0] 962 : this.dateCriterion.toArray(new Base[this.dateCriterion.size()]); // ImmunizationRecommendationRecommendationDateCriterionComponent 963 case -1724546052: 964 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 965 case -905838985: 966 /* series */ return this.series == null ? new Base[0] : new Base[] { this.series }; // StringType 967 case -887709242: 968 /* doseNumber */ return this.doseNumber == null ? new Base[0] : new Base[] { this.doseNumber }; // Type 969 case -1936727105: 970 /* seriesDoses */ return this.seriesDoses == null ? new Base[0] : new Base[] { this.seriesDoses }; // Type 971 case 1171592021: 972 /* supportingImmunization */ return this.supportingImmunization == null ? new Base[0] 973 : this.supportingImmunization.toArray(new Base[this.supportingImmunization.size()]); // Reference 974 case -1234160646: 975 /* supportingPatientInformation */ return this.supportingPatientInformation == null ? new Base[0] 976 : this.supportingPatientInformation.toArray(new Base[this.supportingPatientInformation.size()]); // Reference 977 default: 978 return super.getProperty(hash, name, checkValid); 979 } 980 981 } 982 983 @Override 984 public Base setProperty(int hash, String name, Base value) throws FHIRException { 985 switch (hash) { 986 case 664556354: // vaccineCode 987 this.getVaccineCode().add(castToCodeableConcept(value)); // CodeableConcept 988 return value; 989 case -319593813: // targetDisease 990 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 991 return value; 992 case 571105240: // contraindicatedVaccineCode 993 this.getContraindicatedVaccineCode().add(castToCodeableConcept(value)); // CodeableConcept 994 return value; 995 case 1904598477: // forecastStatus 996 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 997 return value; 998 case 1862115359: // forecastReason 999 this.getForecastReason().add(castToCodeableConcept(value)); // CodeableConcept 1000 return value; 1001 case 2087518867: // dateCriterion 1002 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); // ImmunizationRecommendationRecommendationDateCriterionComponent 1003 return value; 1004 case -1724546052: // description 1005 this.description = castToString(value); // StringType 1006 return value; 1007 case -905838985: // series 1008 this.series = castToString(value); // StringType 1009 return value; 1010 case -887709242: // doseNumber 1011 this.doseNumber = castToType(value); // Type 1012 return value; 1013 case -1936727105: // seriesDoses 1014 this.seriesDoses = castToType(value); // Type 1015 return value; 1016 case 1171592021: // supportingImmunization 1017 this.getSupportingImmunization().add(castToReference(value)); // Reference 1018 return value; 1019 case -1234160646: // supportingPatientInformation 1020 this.getSupportingPatientInformation().add(castToReference(value)); // Reference 1021 return value; 1022 default: 1023 return super.setProperty(hash, name, value); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(String name, Base value) throws FHIRException { 1030 if (name.equals("vaccineCode")) { 1031 this.getVaccineCode().add(castToCodeableConcept(value)); 1032 } else if (name.equals("targetDisease")) { 1033 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 1034 } else if (name.equals("contraindicatedVaccineCode")) { 1035 this.getContraindicatedVaccineCode().add(castToCodeableConcept(value)); 1036 } else if (name.equals("forecastStatus")) { 1037 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 1038 } else if (name.equals("forecastReason")) { 1039 this.getForecastReason().add(castToCodeableConcept(value)); 1040 } else if (name.equals("dateCriterion")) { 1041 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 1042 } else if (name.equals("description")) { 1043 this.description = castToString(value); // StringType 1044 } else if (name.equals("series")) { 1045 this.series = castToString(value); // StringType 1046 } else if (name.equals("doseNumber[x]")) { 1047 this.doseNumber = castToType(value); // Type 1048 } else if (name.equals("seriesDoses[x]")) { 1049 this.seriesDoses = castToType(value); // Type 1050 } else if (name.equals("supportingImmunization")) { 1051 this.getSupportingImmunization().add(castToReference(value)); 1052 } else if (name.equals("supportingPatientInformation")) { 1053 this.getSupportingPatientInformation().add(castToReference(value)); 1054 } else 1055 return super.setProperty(name, value); 1056 return value; 1057 } 1058 1059 @Override 1060 public void removeChild(String name, Base value) throws FHIRException { 1061 if (name.equals("vaccineCode")) { 1062 this.getVaccineCode().remove(castToCodeableConcept(value)); 1063 } else if (name.equals("targetDisease")) { 1064 this.targetDisease = null; 1065 } else if (name.equals("contraindicatedVaccineCode")) { 1066 this.getContraindicatedVaccineCode().remove(castToCodeableConcept(value)); 1067 } else if (name.equals("forecastStatus")) { 1068 this.forecastStatus = null; 1069 } else if (name.equals("forecastReason")) { 1070 this.getForecastReason().remove(castToCodeableConcept(value)); 1071 } else if (name.equals("dateCriterion")) { 1072 this.getDateCriterion().remove((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 1073 } else if (name.equals("description")) { 1074 this.description = null; 1075 } else if (name.equals("series")) { 1076 this.series = null; 1077 } else if (name.equals("doseNumber[x]")) { 1078 this.doseNumber = null; 1079 } else if (name.equals("seriesDoses[x]")) { 1080 this.seriesDoses = null; 1081 } else if (name.equals("supportingImmunization")) { 1082 this.getSupportingImmunization().remove(castToReference(value)); 1083 } else if (name.equals("supportingPatientInformation")) { 1084 this.getSupportingPatientInformation().remove(castToReference(value)); 1085 } else 1086 super.removeChild(name, value); 1087 1088 } 1089 1090 @Override 1091 public Base makeProperty(int hash, String name) throws FHIRException { 1092 switch (hash) { 1093 case 664556354: 1094 return addVaccineCode(); 1095 case -319593813: 1096 return getTargetDisease(); 1097 case 571105240: 1098 return addContraindicatedVaccineCode(); 1099 case 1904598477: 1100 return getForecastStatus(); 1101 case 1862115359: 1102 return addForecastReason(); 1103 case 2087518867: 1104 return addDateCriterion(); 1105 case -1724546052: 1106 return getDescriptionElement(); 1107 case -905838985: 1108 return getSeriesElement(); 1109 case -1632295686: 1110 return getDoseNumber(); 1111 case -887709242: 1112 return getDoseNumber(); 1113 case 1553560673: 1114 return getSeriesDoses(); 1115 case -1936727105: 1116 return getSeriesDoses(); 1117 case 1171592021: 1118 return addSupportingImmunization(); 1119 case -1234160646: 1120 return addSupportingPatientInformation(); 1121 default: 1122 return super.makeProperty(hash, name); 1123 } 1124 1125 } 1126 1127 @Override 1128 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1129 switch (hash) { 1130 case 664556354: 1131 /* vaccineCode */ return new String[] { "CodeableConcept" }; 1132 case -319593813: 1133 /* targetDisease */ return new String[] { "CodeableConcept" }; 1134 case 571105240: 1135 /* contraindicatedVaccineCode */ return new String[] { "CodeableConcept" }; 1136 case 1904598477: 1137 /* forecastStatus */ return new String[] { "CodeableConcept" }; 1138 case 1862115359: 1139 /* forecastReason */ return new String[] { "CodeableConcept" }; 1140 case 2087518867: 1141 /* dateCriterion */ return new String[] {}; 1142 case -1724546052: 1143 /* description */ return new String[] { "string" }; 1144 case -905838985: 1145 /* series */ return new String[] { "string" }; 1146 case -887709242: 1147 /* doseNumber */ return new String[] { "positiveInt", "string" }; 1148 case -1936727105: 1149 /* seriesDoses */ return new String[] { "positiveInt", "string" }; 1150 case 1171592021: 1151 /* supportingImmunization */ return new String[] { "Reference" }; 1152 case -1234160646: 1153 /* supportingPatientInformation */ return new String[] { "Reference" }; 1154 default: 1155 return super.getTypesForProperty(hash, name); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base addChild(String name) throws FHIRException { 1162 if (name.equals("vaccineCode")) { 1163 return addVaccineCode(); 1164 } else if (name.equals("targetDisease")) { 1165 this.targetDisease = new CodeableConcept(); 1166 return this.targetDisease; 1167 } else if (name.equals("contraindicatedVaccineCode")) { 1168 return addContraindicatedVaccineCode(); 1169 } else if (name.equals("forecastStatus")) { 1170 this.forecastStatus = new CodeableConcept(); 1171 return this.forecastStatus; 1172 } else if (name.equals("forecastReason")) { 1173 return addForecastReason(); 1174 } else if (name.equals("dateCriterion")) { 1175 return addDateCriterion(); 1176 } else if (name.equals("description")) { 1177 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.description"); 1178 } else if (name.equals("series")) { 1179 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.series"); 1180 } else if (name.equals("doseNumberPositiveInt")) { 1181 this.doseNumber = new PositiveIntType(); 1182 return this.doseNumber; 1183 } else if (name.equals("doseNumberString")) { 1184 this.doseNumber = new StringType(); 1185 return this.doseNumber; 1186 } else if (name.equals("seriesDosesPositiveInt")) { 1187 this.seriesDoses = new PositiveIntType(); 1188 return this.seriesDoses; 1189 } else if (name.equals("seriesDosesString")) { 1190 this.seriesDoses = new StringType(); 1191 return this.seriesDoses; 1192 } else if (name.equals("supportingImmunization")) { 1193 return addSupportingImmunization(); 1194 } else if (name.equals("supportingPatientInformation")) { 1195 return addSupportingPatientInformation(); 1196 } else 1197 return super.addChild(name); 1198 } 1199 1200 public ImmunizationRecommendationRecommendationComponent copy() { 1201 ImmunizationRecommendationRecommendationComponent dst = new ImmunizationRecommendationRecommendationComponent(); 1202 copyValues(dst); 1203 return dst; 1204 } 1205 1206 public void copyValues(ImmunizationRecommendationRecommendationComponent dst) { 1207 super.copyValues(dst); 1208 if (vaccineCode != null) { 1209 dst.vaccineCode = new ArrayList<CodeableConcept>(); 1210 for (CodeableConcept i : vaccineCode) 1211 dst.vaccineCode.add(i.copy()); 1212 } 1213 ; 1214 dst.targetDisease = targetDisease == null ? null : targetDisease.copy(); 1215 if (contraindicatedVaccineCode != null) { 1216 dst.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 1217 for (CodeableConcept i : contraindicatedVaccineCode) 1218 dst.contraindicatedVaccineCode.add(i.copy()); 1219 } 1220 ; 1221 dst.forecastStatus = forecastStatus == null ? null : forecastStatus.copy(); 1222 if (forecastReason != null) { 1223 dst.forecastReason = new ArrayList<CodeableConcept>(); 1224 for (CodeableConcept i : forecastReason) 1225 dst.forecastReason.add(i.copy()); 1226 } 1227 ; 1228 if (dateCriterion != null) { 1229 dst.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 1230 for (ImmunizationRecommendationRecommendationDateCriterionComponent i : dateCriterion) 1231 dst.dateCriterion.add(i.copy()); 1232 } 1233 ; 1234 dst.description = description == null ? null : description.copy(); 1235 dst.series = series == null ? null : series.copy(); 1236 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1237 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1238 if (supportingImmunization != null) { 1239 dst.supportingImmunization = new ArrayList<Reference>(); 1240 for (Reference i : supportingImmunization) 1241 dst.supportingImmunization.add(i.copy()); 1242 } 1243 ; 1244 if (supportingPatientInformation != null) { 1245 dst.supportingPatientInformation = new ArrayList<Reference>(); 1246 for (Reference i : supportingPatientInformation) 1247 dst.supportingPatientInformation.add(i.copy()); 1248 } 1249 ; 1250 } 1251 1252 @Override 1253 public boolean equalsDeep(Base other_) { 1254 if (!super.equalsDeep(other_)) 1255 return false; 1256 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 1257 return false; 1258 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 1259 return compareDeep(vaccineCode, o.vaccineCode, true) && compareDeep(targetDisease, o.targetDisease, true) 1260 && compareDeep(contraindicatedVaccineCode, o.contraindicatedVaccineCode, true) 1261 && compareDeep(forecastStatus, o.forecastStatus, true) && compareDeep(forecastReason, o.forecastReason, true) 1262 && compareDeep(dateCriterion, o.dateCriterion, true) && compareDeep(description, o.description, true) 1263 && compareDeep(series, o.series, true) && compareDeep(doseNumber, o.doseNumber, true) 1264 && compareDeep(seriesDoses, o.seriesDoses, true) 1265 && compareDeep(supportingImmunization, o.supportingImmunization, true) 1266 && compareDeep(supportingPatientInformation, o.supportingPatientInformation, true); 1267 } 1268 1269 @Override 1270 public boolean equalsShallow(Base other_) { 1271 if (!super.equalsShallow(other_)) 1272 return false; 1273 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 1274 return false; 1275 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 1276 return compareValues(description, o.description, true) && compareValues(series, o.series, true); 1277 } 1278 1279 public boolean isEmpty() { 1280 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(vaccineCode, targetDisease, 1281 contraindicatedVaccineCode, forecastStatus, forecastReason, dateCriterion, description, series, doseNumber, 1282 seriesDoses, supportingImmunization, supportingPatientInformation); 1283 } 1284 1285 public String fhirType() { 1286 return "ImmunizationRecommendation.recommendation"; 1287 1288 } 1289 1290 } 1291 1292 @Block() 1293 public static class ImmunizationRecommendationRecommendationDateCriterionComponent extends BackboneElement 1294 implements IBaseBackboneElement { 1295 /** 1296 * Date classification of recommendation. For example, earliest date to give, 1297 * latest date to give, etc. 1298 */ 1299 @Child(name = "code", type = { 1300 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1301 @Description(shortDefinition = "Type of date", formalDefinition = "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.") 1302 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-date-criterion") 1303 protected CodeableConcept code; 1304 1305 /** 1306 * The date whose meaning is specified by dateCriterion.code. 1307 */ 1308 @Child(name = "value", type = { 1309 DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1310 @Description(shortDefinition = "Recommended date", formalDefinition = "The date whose meaning is specified by dateCriterion.code.") 1311 protected DateTimeType value; 1312 1313 private static final long serialVersionUID = 1036994566L; 1314 1315 /** 1316 * Constructor 1317 */ 1318 public ImmunizationRecommendationRecommendationDateCriterionComponent() { 1319 super(); 1320 } 1321 1322 /** 1323 * Constructor 1324 */ 1325 public ImmunizationRecommendationRecommendationDateCriterionComponent(CodeableConcept code, DateTimeType value) { 1326 super(); 1327 this.code = code; 1328 this.value = value; 1329 } 1330 1331 /** 1332 * @return {@link #code} (Date classification of recommendation. For example, 1333 * earliest date to give, latest date to give, etc.) 1334 */ 1335 public CodeableConcept getCode() { 1336 if (this.code == null) 1337 if (Configuration.errorOnAutoCreate()) 1338 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.code"); 1339 else if (Configuration.doAutoCreate()) 1340 this.code = new CodeableConcept(); // cc 1341 return this.code; 1342 } 1343 1344 public boolean hasCode() { 1345 return this.code != null && !this.code.isEmpty(); 1346 } 1347 1348 /** 1349 * @param value {@link #code} (Date classification of recommendation. For 1350 * example, earliest date to give, latest date to give, etc.) 1351 */ 1352 public ImmunizationRecommendationRecommendationDateCriterionComponent setCode(CodeableConcept value) { 1353 this.code = value; 1354 return this; 1355 } 1356 1357 /** 1358 * @return {@link #value} (The date whose meaning is specified by 1359 * dateCriterion.code.). This is the underlying object with id, value 1360 * and extensions. The accessor "getValue" gives direct access to the 1361 * value 1362 */ 1363 public DateTimeType getValueElement() { 1364 if (this.value == null) 1365 if (Configuration.errorOnAutoCreate()) 1366 throw new Error( 1367 "Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.value"); 1368 else if (Configuration.doAutoCreate()) 1369 this.value = new DateTimeType(); // bb 1370 return this.value; 1371 } 1372 1373 public boolean hasValueElement() { 1374 return this.value != null && !this.value.isEmpty(); 1375 } 1376 1377 public boolean hasValue() { 1378 return this.value != null && !this.value.isEmpty(); 1379 } 1380 1381 /** 1382 * @param value {@link #value} (The date whose meaning is specified by 1383 * dateCriterion.code.). This is the underlying object with id, 1384 * value and extensions. The accessor "getValue" gives direct 1385 * access to the value 1386 */ 1387 public ImmunizationRecommendationRecommendationDateCriterionComponent setValueElement(DateTimeType value) { 1388 this.value = value; 1389 return this; 1390 } 1391 1392 /** 1393 * @return The date whose meaning is specified by dateCriterion.code. 1394 */ 1395 public Date getValue() { 1396 return this.value == null ? null : this.value.getValue(); 1397 } 1398 1399 /** 1400 * @param value The date whose meaning is specified by dateCriterion.code. 1401 */ 1402 public ImmunizationRecommendationRecommendationDateCriterionComponent setValue(Date value) { 1403 if (this.value == null) 1404 this.value = new DateTimeType(); 1405 this.value.setValue(value); 1406 return this; 1407 } 1408 1409 protected void listChildren(List<Property> children) { 1410 super.listChildren(children); 1411 children.add(new Property("code", "CodeableConcept", 1412 "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1, 1413 code)); 1414 children.add( 1415 new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 0, 1, value)); 1416 } 1417 1418 @Override 1419 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1420 switch (_hash) { 1421 case 3059181: 1422 /* code */ return new Property("code", "CodeableConcept", 1423 "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1424 1, code); 1425 case 111972721: 1426 /* value */ return new Property("value", "dateTime", 1427 "The date whose meaning is specified by dateCriterion.code.", 0, 1, value); 1428 default: 1429 return super.getNamedProperty(_hash, _name, _checkValid); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1436 switch (hash) { 1437 case 3059181: 1438 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1439 case 111972721: 1440 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DateTimeType 1441 default: 1442 return super.getProperty(hash, name, checkValid); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1449 switch (hash) { 1450 case 3059181: // code 1451 this.code = castToCodeableConcept(value); // CodeableConcept 1452 return value; 1453 case 111972721: // value 1454 this.value = castToDateTime(value); // DateTimeType 1455 return value; 1456 default: 1457 return super.setProperty(hash, name, value); 1458 } 1459 1460 } 1461 1462 @Override 1463 public Base setProperty(String name, Base value) throws FHIRException { 1464 if (name.equals("code")) { 1465 this.code = castToCodeableConcept(value); // CodeableConcept 1466 } else if (name.equals("value")) { 1467 this.value = castToDateTime(value); // DateTimeType 1468 } else 1469 return super.setProperty(name, value); 1470 return value; 1471 } 1472 1473 @Override 1474 public void removeChild(String name, Base value) throws FHIRException { 1475 if (name.equals("code")) { 1476 this.code = null; 1477 } else if (name.equals("value")) { 1478 this.value = null; 1479 } else 1480 super.removeChild(name, value); 1481 1482 } 1483 1484 @Override 1485 public Base makeProperty(int hash, String name) throws FHIRException { 1486 switch (hash) { 1487 case 3059181: 1488 return getCode(); 1489 case 111972721: 1490 return getValueElement(); 1491 default: 1492 return super.makeProperty(hash, name); 1493 } 1494 1495 } 1496 1497 @Override 1498 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1499 switch (hash) { 1500 case 3059181: 1501 /* code */ return new String[] { "CodeableConcept" }; 1502 case 111972721: 1503 /* value */ return new String[] { "dateTime" }; 1504 default: 1505 return super.getTypesForProperty(hash, name); 1506 } 1507 1508 } 1509 1510 @Override 1511 public Base addChild(String name) throws FHIRException { 1512 if (name.equals("code")) { 1513 this.code = new CodeableConcept(); 1514 return this.code; 1515 } else if (name.equals("value")) { 1516 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.value"); 1517 } else 1518 return super.addChild(name); 1519 } 1520 1521 public ImmunizationRecommendationRecommendationDateCriterionComponent copy() { 1522 ImmunizationRecommendationRecommendationDateCriterionComponent dst = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 1523 copyValues(dst); 1524 return dst; 1525 } 1526 1527 public void copyValues(ImmunizationRecommendationRecommendationDateCriterionComponent dst) { 1528 super.copyValues(dst); 1529 dst.code = code == null ? null : code.copy(); 1530 dst.value = value == null ? null : value.copy(); 1531 } 1532 1533 @Override 1534 public boolean equalsDeep(Base other_) { 1535 if (!super.equalsDeep(other_)) 1536 return false; 1537 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 1538 return false; 1539 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 1540 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 1541 } 1542 1543 @Override 1544 public boolean equalsShallow(Base other_) { 1545 if (!super.equalsShallow(other_)) 1546 return false; 1547 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 1548 return false; 1549 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 1550 return compareValues(value, o.value, true); 1551 } 1552 1553 public boolean isEmpty() { 1554 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 1555 } 1556 1557 public String fhirType() { 1558 return "ImmunizationRecommendation.recommendation.dateCriterion"; 1559 1560 } 1561 1562 } 1563 1564 /** 1565 * A unique identifier assigned to this particular recommendation record. 1566 */ 1567 @Child(name = "identifier", type = { 1568 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1569 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this particular recommendation record.") 1570 protected List<Identifier> identifier; 1571 1572 /** 1573 * The patient the recommendation(s) are for. 1574 */ 1575 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1576 @Description(shortDefinition = "Who this profile is for", formalDefinition = "The patient the recommendation(s) are for.") 1577 protected Reference patient; 1578 1579 /** 1580 * The actual object that is the target of the reference (The patient the 1581 * recommendation(s) are for.) 1582 */ 1583 protected Patient patientTarget; 1584 1585 /** 1586 * The date the immunization recommendation(s) were created. 1587 */ 1588 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1589 @Description(shortDefinition = "Date recommendation(s) created", formalDefinition = "The date the immunization recommendation(s) were created.") 1590 protected DateTimeType date; 1591 1592 /** 1593 * Indicates the authority who published the protocol (e.g. ACIP). 1594 */ 1595 @Child(name = "authority", type = { 1596 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1597 @Description(shortDefinition = "Who is responsible for protocol", formalDefinition = "Indicates the authority who published the protocol (e.g. ACIP).") 1598 protected Reference authority; 1599 1600 /** 1601 * The actual object that is the target of the reference (Indicates the 1602 * authority who published the protocol (e.g. ACIP).) 1603 */ 1604 protected Organization authorityTarget; 1605 1606 /** 1607 * Vaccine administration recommendations. 1608 */ 1609 @Child(name = "recommendation", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1610 @Description(shortDefinition = "Vaccine administration recommendations", formalDefinition = "Vaccine administration recommendations.") 1611 protected List<ImmunizationRecommendationRecommendationComponent> recommendation; 1612 1613 private static final long serialVersionUID = -2031711761L; 1614 1615 /** 1616 * Constructor 1617 */ 1618 public ImmunizationRecommendation() { 1619 super(); 1620 } 1621 1622 /** 1623 * Constructor 1624 */ 1625 public ImmunizationRecommendation(Reference patient, DateTimeType date) { 1626 super(); 1627 this.patient = patient; 1628 this.date = date; 1629 } 1630 1631 /** 1632 * @return {@link #identifier} (A unique identifier assigned to this particular 1633 * recommendation record.) 1634 */ 1635 public List<Identifier> getIdentifier() { 1636 if (this.identifier == null) 1637 this.identifier = new ArrayList<Identifier>(); 1638 return this.identifier; 1639 } 1640 1641 /** 1642 * @return Returns a reference to <code>this</code> for easy method chaining 1643 */ 1644 public ImmunizationRecommendation setIdentifier(List<Identifier> theIdentifier) { 1645 this.identifier = theIdentifier; 1646 return this; 1647 } 1648 1649 public boolean hasIdentifier() { 1650 if (this.identifier == null) 1651 return false; 1652 for (Identifier item : this.identifier) 1653 if (!item.isEmpty()) 1654 return true; 1655 return false; 1656 } 1657 1658 public Identifier addIdentifier() { // 3 1659 Identifier t = new Identifier(); 1660 if (this.identifier == null) 1661 this.identifier = new ArrayList<Identifier>(); 1662 this.identifier.add(t); 1663 return t; 1664 } 1665 1666 public ImmunizationRecommendation addIdentifier(Identifier t) { // 3 1667 if (t == null) 1668 return this; 1669 if (this.identifier == null) 1670 this.identifier = new ArrayList<Identifier>(); 1671 this.identifier.add(t); 1672 return this; 1673 } 1674 1675 /** 1676 * @return The first repetition of repeating field {@link #identifier}, creating 1677 * it if it does not already exist 1678 */ 1679 public Identifier getIdentifierFirstRep() { 1680 if (getIdentifier().isEmpty()) { 1681 addIdentifier(); 1682 } 1683 return getIdentifier().get(0); 1684 } 1685 1686 /** 1687 * @return {@link #patient} (The patient the recommendation(s) are for.) 1688 */ 1689 public Reference getPatient() { 1690 if (this.patient == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1693 else if (Configuration.doAutoCreate()) 1694 this.patient = new Reference(); // cc 1695 return this.patient; 1696 } 1697 1698 public boolean hasPatient() { 1699 return this.patient != null && !this.patient.isEmpty(); 1700 } 1701 1702 /** 1703 * @param value {@link #patient} (The patient the recommendation(s) are for.) 1704 */ 1705 public ImmunizationRecommendation setPatient(Reference value) { 1706 this.patient = value; 1707 return this; 1708 } 1709 1710 /** 1711 * @return {@link #patient} The actual object that is the target of the 1712 * reference. The reference library doesn't populate this, but you can 1713 * use it to hold the resource if you resolve it. (The patient the 1714 * recommendation(s) are for.) 1715 */ 1716 public Patient getPatientTarget() { 1717 if (this.patientTarget == null) 1718 if (Configuration.errorOnAutoCreate()) 1719 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1720 else if (Configuration.doAutoCreate()) 1721 this.patientTarget = new Patient(); // aa 1722 return this.patientTarget; 1723 } 1724 1725 /** 1726 * @param value {@link #patient} The actual object that is the target of the 1727 * reference. The reference library doesn't use these, but you can 1728 * use it to hold the resource if you resolve it. (The patient the 1729 * recommendation(s) are for.) 1730 */ 1731 public ImmunizationRecommendation setPatientTarget(Patient value) { 1732 this.patientTarget = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #date} (The date the immunization recommendation(s) were 1738 * created.). This is the underlying object with id, value and 1739 * extensions. The accessor "getDate" gives direct access to the value 1740 */ 1741 public DateTimeType getDateElement() { 1742 if (this.date == null) 1743 if (Configuration.errorOnAutoCreate()) 1744 throw new Error("Attempt to auto-create ImmunizationRecommendation.date"); 1745 else if (Configuration.doAutoCreate()) 1746 this.date = new DateTimeType(); // bb 1747 return this.date; 1748 } 1749 1750 public boolean hasDateElement() { 1751 return this.date != null && !this.date.isEmpty(); 1752 } 1753 1754 public boolean hasDate() { 1755 return this.date != null && !this.date.isEmpty(); 1756 } 1757 1758 /** 1759 * @param value {@link #date} (The date the immunization recommendation(s) were 1760 * created.). This is the underlying object with id, value and 1761 * extensions. The accessor "getDate" gives direct access to the 1762 * value 1763 */ 1764 public ImmunizationRecommendation setDateElement(DateTimeType value) { 1765 this.date = value; 1766 return this; 1767 } 1768 1769 /** 1770 * @return The date the immunization recommendation(s) were created. 1771 */ 1772 public Date getDate() { 1773 return this.date == null ? null : this.date.getValue(); 1774 } 1775 1776 /** 1777 * @param value The date the immunization recommendation(s) were created. 1778 */ 1779 public ImmunizationRecommendation setDate(Date value) { 1780 if (this.date == null) 1781 this.date = new DateTimeType(); 1782 this.date.setValue(value); 1783 return this; 1784 } 1785 1786 /** 1787 * @return {@link #authority} (Indicates the authority who published the 1788 * protocol (e.g. ACIP).) 1789 */ 1790 public Reference getAuthority() { 1791 if (this.authority == null) 1792 if (Configuration.errorOnAutoCreate()) 1793 throw new Error("Attempt to auto-create ImmunizationRecommendation.authority"); 1794 else if (Configuration.doAutoCreate()) 1795 this.authority = new Reference(); // cc 1796 return this.authority; 1797 } 1798 1799 public boolean hasAuthority() { 1800 return this.authority != null && !this.authority.isEmpty(); 1801 } 1802 1803 /** 1804 * @param value {@link #authority} (Indicates the authority who published the 1805 * protocol (e.g. ACIP).) 1806 */ 1807 public ImmunizationRecommendation setAuthority(Reference value) { 1808 this.authority = value; 1809 return this; 1810 } 1811 1812 /** 1813 * @return {@link #authority} The actual object that is the target of the 1814 * reference. The reference library doesn't populate this, but you can 1815 * use it to hold the resource if you resolve it. (Indicates the 1816 * authority who published the protocol (e.g. ACIP).) 1817 */ 1818 public Organization getAuthorityTarget() { 1819 if (this.authorityTarget == null) 1820 if (Configuration.errorOnAutoCreate()) 1821 throw new Error("Attempt to auto-create ImmunizationRecommendation.authority"); 1822 else if (Configuration.doAutoCreate()) 1823 this.authorityTarget = new Organization(); // aa 1824 return this.authorityTarget; 1825 } 1826 1827 /** 1828 * @param value {@link #authority} The actual object that is the target of the 1829 * reference. The reference library doesn't use these, but you can 1830 * use it to hold the resource if you resolve it. (Indicates the 1831 * authority who published the protocol (e.g. ACIP).) 1832 */ 1833 public ImmunizationRecommendation setAuthorityTarget(Organization value) { 1834 this.authorityTarget = value; 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #recommendation} (Vaccine administration recommendations.) 1840 */ 1841 public List<ImmunizationRecommendationRecommendationComponent> getRecommendation() { 1842 if (this.recommendation == null) 1843 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1844 return this.recommendation; 1845 } 1846 1847 /** 1848 * @return Returns a reference to <code>this</code> for easy method chaining 1849 */ 1850 public ImmunizationRecommendation setRecommendation( 1851 List<ImmunizationRecommendationRecommendationComponent> theRecommendation) { 1852 this.recommendation = theRecommendation; 1853 return this; 1854 } 1855 1856 public boolean hasRecommendation() { 1857 if (this.recommendation == null) 1858 return false; 1859 for (ImmunizationRecommendationRecommendationComponent item : this.recommendation) 1860 if (!item.isEmpty()) 1861 return true; 1862 return false; 1863 } 1864 1865 public ImmunizationRecommendationRecommendationComponent addRecommendation() { // 3 1866 ImmunizationRecommendationRecommendationComponent t = new ImmunizationRecommendationRecommendationComponent(); 1867 if (this.recommendation == null) 1868 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1869 this.recommendation.add(t); 1870 return t; 1871 } 1872 1873 public ImmunizationRecommendation addRecommendation(ImmunizationRecommendationRecommendationComponent t) { // 3 1874 if (t == null) 1875 return this; 1876 if (this.recommendation == null) 1877 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1878 this.recommendation.add(t); 1879 return this; 1880 } 1881 1882 /** 1883 * @return The first repetition of repeating field {@link #recommendation}, 1884 * creating it if it does not already exist 1885 */ 1886 public ImmunizationRecommendationRecommendationComponent getRecommendationFirstRep() { 1887 if (getRecommendation().isEmpty()) { 1888 addRecommendation(); 1889 } 1890 return getRecommendation().get(0); 1891 } 1892 1893 protected void listChildren(List<Property> children) { 1894 super.listChildren(children); 1895 children.add(new Property("identifier", "Identifier", 1896 "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, 1897 identifier)); 1898 children.add( 1899 new Property("patient", "Reference(Patient)", "The patient the recommendation(s) are for.", 0, 1, patient)); 1900 children 1901 .add(new Property("date", "dateTime", "The date the immunization recommendation(s) were created.", 0, 1, date)); 1902 children.add(new Property("authority", "Reference(Organization)", 1903 "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority)); 1904 children.add(new Property("recommendation", "", "Vaccine administration recommendations.", 0, 1905 java.lang.Integer.MAX_VALUE, recommendation)); 1906 } 1907 1908 @Override 1909 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1910 switch (_hash) { 1911 case -1618432855: 1912 /* identifier */ return new Property("identifier", "Identifier", 1913 "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, 1914 identifier); 1915 case -791418107: 1916 /* patient */ return new Property("patient", "Reference(Patient)", "The patient the recommendation(s) are for.", 1917 0, 1, patient); 1918 case 3076014: 1919 /* date */ return new Property("date", "dateTime", "The date the immunization recommendation(s) were created.", 0, 1920 1, date); 1921 case 1475610435: 1922 /* authority */ return new Property("authority", "Reference(Organization)", 1923 "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority); 1924 case -1028636743: 1925 /* recommendation */ return new Property("recommendation", "", "Vaccine administration recommendations.", 0, 1926 java.lang.Integer.MAX_VALUE, recommendation); 1927 default: 1928 return super.getNamedProperty(_hash, _name, _checkValid); 1929 } 1930 1931 } 1932 1933 @Override 1934 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1935 switch (hash) { 1936 case -1618432855: 1937 /* identifier */ return this.identifier == null ? new Base[0] 1938 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1939 case -791418107: 1940 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1941 case 3076014: 1942 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1943 case 1475610435: 1944 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // Reference 1945 case -1028636743: 1946 /* recommendation */ return this.recommendation == null ? new Base[0] 1947 : this.recommendation.toArray(new Base[this.recommendation.size()]); // ImmunizationRecommendationRecommendationComponent 1948 default: 1949 return super.getProperty(hash, name, checkValid); 1950 } 1951 1952 } 1953 1954 @Override 1955 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1956 switch (hash) { 1957 case -1618432855: // identifier 1958 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1959 return value; 1960 case -791418107: // patient 1961 this.patient = castToReference(value); // Reference 1962 return value; 1963 case 3076014: // date 1964 this.date = castToDateTime(value); // DateTimeType 1965 return value; 1966 case 1475610435: // authority 1967 this.authority = castToReference(value); // Reference 1968 return value; 1969 case -1028636743: // recommendation 1970 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); // ImmunizationRecommendationRecommendationComponent 1971 return value; 1972 default: 1973 return super.setProperty(hash, name, value); 1974 } 1975 1976 } 1977 1978 @Override 1979 public Base setProperty(String name, Base value) throws FHIRException { 1980 if (name.equals("identifier")) { 1981 this.getIdentifier().add(castToIdentifier(value)); 1982 } else if (name.equals("patient")) { 1983 this.patient = castToReference(value); // Reference 1984 } else if (name.equals("date")) { 1985 this.date = castToDateTime(value); // DateTimeType 1986 } else if (name.equals("authority")) { 1987 this.authority = castToReference(value); // Reference 1988 } else if (name.equals("recommendation")) { 1989 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); 1990 } else 1991 return super.setProperty(name, value); 1992 return value; 1993 } 1994 1995 @Override 1996 public void removeChild(String name, Base value) throws FHIRException { 1997 if (name.equals("identifier")) { 1998 this.getIdentifier().remove(castToIdentifier(value)); 1999 } else if (name.equals("patient")) { 2000 this.patient = null; 2001 } else if (name.equals("date")) { 2002 this.date = null; 2003 } else if (name.equals("authority")) { 2004 this.authority = null; 2005 } else if (name.equals("recommendation")) { 2006 this.getRecommendation().remove((ImmunizationRecommendationRecommendationComponent) value); 2007 } else 2008 super.removeChild(name, value); 2009 2010 } 2011 2012 @Override 2013 public Base makeProperty(int hash, String name) throws FHIRException { 2014 switch (hash) { 2015 case -1618432855: 2016 return addIdentifier(); 2017 case -791418107: 2018 return getPatient(); 2019 case 3076014: 2020 return getDateElement(); 2021 case 1475610435: 2022 return getAuthority(); 2023 case -1028636743: 2024 return addRecommendation(); 2025 default: 2026 return super.makeProperty(hash, name); 2027 } 2028 2029 } 2030 2031 @Override 2032 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2033 switch (hash) { 2034 case -1618432855: 2035 /* identifier */ return new String[] { "Identifier" }; 2036 case -791418107: 2037 /* patient */ return new String[] { "Reference" }; 2038 case 3076014: 2039 /* date */ return new String[] { "dateTime" }; 2040 case 1475610435: 2041 /* authority */ return new String[] { "Reference" }; 2042 case -1028636743: 2043 /* recommendation */ return new String[] {}; 2044 default: 2045 return super.getTypesForProperty(hash, name); 2046 } 2047 2048 } 2049 2050 @Override 2051 public Base addChild(String name) throws FHIRException { 2052 if (name.equals("identifier")) { 2053 return addIdentifier(); 2054 } else if (name.equals("patient")) { 2055 this.patient = new Reference(); 2056 return this.patient; 2057 } else if (name.equals("date")) { 2058 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.date"); 2059 } else if (name.equals("authority")) { 2060 this.authority = new Reference(); 2061 return this.authority; 2062 } else if (name.equals("recommendation")) { 2063 return addRecommendation(); 2064 } else 2065 return super.addChild(name); 2066 } 2067 2068 public String fhirType() { 2069 return "ImmunizationRecommendation"; 2070 2071 } 2072 2073 public ImmunizationRecommendation copy() { 2074 ImmunizationRecommendation dst = new ImmunizationRecommendation(); 2075 copyValues(dst); 2076 return dst; 2077 } 2078 2079 public void copyValues(ImmunizationRecommendation dst) { 2080 super.copyValues(dst); 2081 if (identifier != null) { 2082 dst.identifier = new ArrayList<Identifier>(); 2083 for (Identifier i : identifier) 2084 dst.identifier.add(i.copy()); 2085 } 2086 ; 2087 dst.patient = patient == null ? null : patient.copy(); 2088 dst.date = date == null ? null : date.copy(); 2089 dst.authority = authority == null ? null : authority.copy(); 2090 if (recommendation != null) { 2091 dst.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 2092 for (ImmunizationRecommendationRecommendationComponent i : recommendation) 2093 dst.recommendation.add(i.copy()); 2094 } 2095 ; 2096 } 2097 2098 protected ImmunizationRecommendation typedCopy() { 2099 return copy(); 2100 } 2101 2102 @Override 2103 public boolean equalsDeep(Base other_) { 2104 if (!super.equalsDeep(other_)) 2105 return false; 2106 if (!(other_ instanceof ImmunizationRecommendation)) 2107 return false; 2108 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 2109 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 2110 && compareDeep(date, o.date, true) && compareDeep(authority, o.authority, true) 2111 && compareDeep(recommendation, o.recommendation, true); 2112 } 2113 2114 @Override 2115 public boolean equalsShallow(Base other_) { 2116 if (!super.equalsShallow(other_)) 2117 return false; 2118 if (!(other_ instanceof ImmunizationRecommendation)) 2119 return false; 2120 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 2121 return compareValues(date, o.date, true); 2122 } 2123 2124 public boolean isEmpty() { 2125 return super.isEmpty() 2126 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, patient, date, authority, recommendation); 2127 } 2128 2129 @Override 2130 public ResourceType getResourceType() { 2131 return ResourceType.ImmunizationRecommendation; 2132 } 2133 2134 /** 2135 * Search parameter: <b>date</b> 2136 * <p> 2137 * Description: <b>Date recommendation(s) created</b><br> 2138 * Type: <b>date</b><br> 2139 * Path: <b>ImmunizationRecommendation.date</b><br> 2140 * </p> 2141 */ 2142 @SearchParamDefinition(name = "date", path = "ImmunizationRecommendation.date", description = "Date recommendation(s) created", type = "date") 2143 public static final String SP_DATE = "date"; 2144 /** 2145 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2146 * <p> 2147 * Description: <b>Date recommendation(s) created</b><br> 2148 * Type: <b>date</b><br> 2149 * Path: <b>ImmunizationRecommendation.date</b><br> 2150 * </p> 2151 */ 2152 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2153 SP_DATE); 2154 2155 /** 2156 * Search parameter: <b>identifier</b> 2157 * <p> 2158 * Description: <b>Business identifier</b><br> 2159 * Type: <b>token</b><br> 2160 * Path: <b>ImmunizationRecommendation.identifier</b><br> 2161 * </p> 2162 */ 2163 @SearchParamDefinition(name = "identifier", path = "ImmunizationRecommendation.identifier", description = "Business identifier", type = "token") 2164 public static final String SP_IDENTIFIER = "identifier"; 2165 /** 2166 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2167 * <p> 2168 * Description: <b>Business identifier</b><br> 2169 * Type: <b>token</b><br> 2170 * Path: <b>ImmunizationRecommendation.identifier</b><br> 2171 * </p> 2172 */ 2173 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2174 SP_IDENTIFIER); 2175 2176 /** 2177 * Search parameter: <b>target-disease</b> 2178 * <p> 2179 * Description: <b>Disease to be immunized against</b><br> 2180 * Type: <b>token</b><br> 2181 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 2182 * </p> 2183 */ 2184 @SearchParamDefinition(name = "target-disease", path = "ImmunizationRecommendation.recommendation.targetDisease", description = "Disease to be immunized against", type = "token") 2185 public static final String SP_TARGET_DISEASE = "target-disease"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 2188 * <p> 2189 * Description: <b>Disease to be immunized against</b><br> 2190 * Type: <b>token</b><br> 2191 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 2192 * </p> 2193 */ 2194 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2195 SP_TARGET_DISEASE); 2196 2197 /** 2198 * Search parameter: <b>patient</b> 2199 * <p> 2200 * Description: <b>Who this profile is for</b><br> 2201 * Type: <b>reference</b><br> 2202 * Path: <b>ImmunizationRecommendation.patient</b><br> 2203 * </p> 2204 */ 2205 @SearchParamDefinition(name = "patient", path = "ImmunizationRecommendation.patient", description = "Who this profile is for", type = "reference", providesMembershipIn = { 2206 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2207 public static final String SP_PATIENT = "patient"; 2208 /** 2209 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2210 * <p> 2211 * Description: <b>Who this profile is for</b><br> 2212 * Type: <b>reference</b><br> 2213 * Path: <b>ImmunizationRecommendation.patient</b><br> 2214 * </p> 2215 */ 2216 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2217 SP_PATIENT); 2218 2219 /** 2220 * Constant for fluent queries to be used to add include statements. Specifies 2221 * the path value of "<b>ImmunizationRecommendation:patient</b>". 2222 */ 2223 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2224 "ImmunizationRecommendation:patient").toLocked(); 2225 2226 /** 2227 * Search parameter: <b>vaccine-type</b> 2228 * <p> 2229 * Description: <b>Vaccine or vaccine group recommendation applies to</b><br> 2230 * Type: <b>token</b><br> 2231 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 2232 * </p> 2233 */ 2234 @SearchParamDefinition(name = "vaccine-type", path = "ImmunizationRecommendation.recommendation.vaccineCode", description = "Vaccine or vaccine group recommendation applies to", type = "token") 2235 public static final String SP_VACCINE_TYPE = "vaccine-type"; 2236 /** 2237 * <b>Fluent Client</b> search parameter constant for <b>vaccine-type</b> 2238 * <p> 2239 * Description: <b>Vaccine or vaccine group recommendation applies to</b><br> 2240 * Type: <b>token</b><br> 2241 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 2242 * </p> 2243 */ 2244 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2245 SP_VACCINE_TYPE); 2246 2247 /** 2248 * Search parameter: <b>information</b> 2249 * <p> 2250 * Description: <b>Patient observations supporting recommendation</b><br> 2251 * Type: <b>reference</b><br> 2252 * Path: 2253 * <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 2254 * </p> 2255 */ 2256 @SearchParamDefinition(name = "information", path = "ImmunizationRecommendation.recommendation.supportingPatientInformation", description = "Patient observations supporting recommendation", type = "reference") 2257 public static final String SP_INFORMATION = "information"; 2258 /** 2259 * <b>Fluent Client</b> search parameter constant for <b>information</b> 2260 * <p> 2261 * Description: <b>Patient observations supporting recommendation</b><br> 2262 * Type: <b>reference</b><br> 2263 * Path: 2264 * <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 2265 * </p> 2266 */ 2267 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INFORMATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2268 SP_INFORMATION); 2269 2270 /** 2271 * Constant for fluent queries to be used to add include statements. Specifies 2272 * the path value of "<b>ImmunizationRecommendation:information</b>". 2273 */ 2274 public static final ca.uhn.fhir.model.api.Include INCLUDE_INFORMATION = new ca.uhn.fhir.model.api.Include( 2275 "ImmunizationRecommendation:information").toLocked(); 2276 2277 /** 2278 * Search parameter: <b>support</b> 2279 * <p> 2280 * Description: <b>Past immunizations supporting recommendation</b><br> 2281 * Type: <b>reference</b><br> 2282 * Path: 2283 * <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 2284 * </p> 2285 */ 2286 @SearchParamDefinition(name = "support", path = "ImmunizationRecommendation.recommendation.supportingImmunization", description = "Past immunizations supporting recommendation", type = "reference", target = { 2287 Immunization.class, ImmunizationEvaluation.class }) 2288 public static final String SP_SUPPORT = "support"; 2289 /** 2290 * <b>Fluent Client</b> search parameter constant for <b>support</b> 2291 * <p> 2292 * Description: <b>Past immunizations supporting recommendation</b><br> 2293 * Type: <b>reference</b><br> 2294 * Path: 2295 * <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 2296 * </p> 2297 */ 2298 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2299 SP_SUPPORT); 2300 2301 /** 2302 * Constant for fluent queries to be used to add include statements. Specifies 2303 * the path value of "<b>ImmunizationRecommendation:support</b>". 2304 */ 2305 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORT = new ca.uhn.fhir.model.api.Include( 2306 "ImmunizationRecommendation:support").toLocked(); 2307 2308 /** 2309 * Search parameter: <b>status</b> 2310 * <p> 2311 * Description: <b>Vaccine recommendation status</b><br> 2312 * Type: <b>token</b><br> 2313 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 2314 * </p> 2315 */ 2316 @SearchParamDefinition(name = "status", path = "ImmunizationRecommendation.recommendation.forecastStatus", description = "Vaccine recommendation status", type = "token") 2317 public static final String SP_STATUS = "status"; 2318 /** 2319 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2320 * <p> 2321 * Description: <b>Vaccine recommendation status</b><br> 2322 * Type: <b>token</b><br> 2323 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 2324 * </p> 2325 */ 2326 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2327 SP_STATUS); 2328 2329}