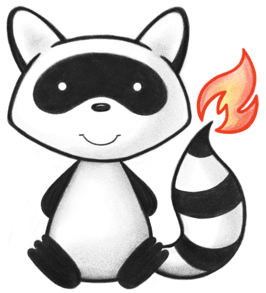
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.FHIRVersion; 040import org.hl7.fhir.r4.model.Enumerations.FHIRVersionEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051 052/** 053 * A set of rules of how a particular interoperability or standards problem is 054 * solved - typically through the use of FHIR resources. This resource is used 055 * to gather all the parts of an implementation guide into a logical whole and 056 * to publish a computable definition of all the parts. 057 */ 058@ResourceDef(name = "ImplementationGuide", profile = "http://hl7.org/fhir/StructureDefinition/ImplementationGuide") 059@ChildOrder(names = { "url", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", 060 "description", "useContext", "jurisdiction", "copyright", "packageId", "license", "fhirVersion", "dependsOn", 061 "global", "definition", "manifest" }) 062public class ImplementationGuide extends MetadataResource { 063 064 public enum SPDXLicense { 065 /** 066 * BSD Zero Clause License 067 */ 068 _0BSD, 069 /** 070 * Attribution Assurance License 071 */ 072 AAL, 073 /** 074 * Abstyles License 075 */ 076 ABSTYLES, 077 /** 078 * AdaCore Doc License 079 */ 080 ADACORE_DOC, 081 /** 082 * Adobe Systems Incorporated Source Code License Agreement 083 */ 084 ADOBE_2006, 085 /** 086 * Adobe Glyph List License 087 */ 088 ADOBE_GLYPH, 089 /** 090 * Amazon Digital Services License 091 */ 092 ADSL, 093 /** 094 * Academic Free License v1.1 095 */ 096 AFL_1_1, 097 /** 098 * Academic Free License v1.2 099 */ 100 AFL_1_2, 101 /** 102 * Academic Free License v2.0 103 */ 104 AFL_2_0, 105 /** 106 * Academic Free License v2.1 107 */ 108 AFL_2_1, 109 /** 110 * Academic Free License v3.0 111 */ 112 AFL_3_0, 113 /** 114 * Afmparse License 115 */ 116 AFMPARSE, 117 /** 118 * Affero General Public License v1.0 119 */ 120 AGPL_1_0, 121 /** 122 * Affero General Public License v1.0 only 123 */ 124 AGPL_1_0_ONLY, 125 /** 126 * Affero General Public License v1.0 or later 127 */ 128 AGPL_1_0_OR_LATER, 129 /** 130 * GNU Affero General Public License v3.0 131 */ 132 AGPL_3_0, 133 /** 134 * GNU Affero General Public License v3.0 only 135 */ 136 AGPL_3_0_ONLY, 137 /** 138 * GNU Affero General Public License v3.0 or later 139 */ 140 AGPL_3_0_OR_LATER, 141 /** 142 * Aladdin Free Public License 143 */ 144 ALADDIN, 145 /** 146 * AMD's plpa_map.c License 147 */ 148 AMDPLPA, 149 /** 150 * Apple MIT License 151 */ 152 AML, 153 /** 154 * Academy of Motion Picture Arts and Sciences BSD 155 */ 156 AMPAS, 157 /** 158 * ANTLR Software Rights Notice 159 */ 160 ANTLR_PD, 161 /** 162 * ANTLR Software Rights Notice with license fallback 163 */ 164 ANTLR_PD_FALLBACK, 165 /** 166 * Apache License 1.0 167 */ 168 APACHE_1_0, 169 /** 170 * Apache License 1.1 171 */ 172 APACHE_1_1, 173 /** 174 * Apache License 2.0 175 */ 176 APACHE_2_0, 177 /** 178 * Adobe Postscript AFM License 179 */ 180 APAFML, 181 /** 182 * Adaptive Public License 1.0 183 */ 184 APL_1_0, 185 /** 186 * App::s2p License 187 */ 188 APP_S2P, 189 /** 190 * Apple Public Source License 1.0 191 */ 192 APSL_1_0, 193 /** 194 * Apple Public Source License 1.1 195 */ 196 APSL_1_1, 197 /** 198 * Apple Public Source License 1.2 199 */ 200 APSL_1_2, 201 /** 202 * Apple Public Source License 2.0 203 */ 204 APSL_2_0, 205 /** 206 * Arphic Public License 207 */ 208 ARPHIC_1999, 209 /** 210 * Artistic License 1.0 211 */ 212 ARTISTIC_1_0, 213 /** 214 * Artistic License 1.0 w/clause 8 215 */ 216 ARTISTIC_1_0_CL8, 217 /** 218 * Artistic License 1.0 (Perl) 219 */ 220 ARTISTIC_1_0_PERL, 221 /** 222 * Artistic License 2.0 223 */ 224 ARTISTIC_2_0, 225 /** 226 * ASWF Digital Assets License version 1.0 227 */ 228 ASWF_DIGITAL_ASSETS_1_0, 229 /** 230 * ASWF Digital Assets License 1.1 231 */ 232 ASWF_DIGITAL_ASSETS_1_1, 233 /** 234 * Baekmuk License 235 */ 236 BAEKMUK, 237 /** 238 * Bahyph License 239 */ 240 BAHYPH, 241 /** 242 * Barr License 243 */ 244 BARR, 245 /** 246 * Beerware License 247 */ 248 BEERWARE, 249 /** 250 * Bitstream Charter Font License 251 */ 252 BITSTREAM_CHARTER, 253 /** 254 * Bitstream Vera Font License 255 */ 256 BITSTREAM_VERA, 257 /** 258 * BitTorrent Open Source License v1.0 259 */ 260 BITTORRENT_1_0, 261 /** 262 * BitTorrent Open Source License v1.1 263 */ 264 BITTORRENT_1_1, 265 /** 266 * SQLite Blessing 267 */ 268 BLESSING, 269 /** 270 * Blue Oak Model License 1.0.0 271 */ 272 BLUEOAK_1_0_0, 273 /** 274 * Boehm-Demers-Weiser GC License 275 */ 276 BOEHM_GC, 277 /** 278 * Borceux license 279 */ 280 BORCEUX, 281 /** 282 * Brian Gladman 3-Clause License 283 */ 284 BRIAN_GLADMAN_3_CLAUSE, 285 /** 286 * BSD 1-Clause License 287 */ 288 BSD_1_CLAUSE, 289 /** 290 * BSD 2-Clause "Simplified" License 291 */ 292 BSD_2_CLAUSE, 293 /** 294 * BSD 2-Clause FreeBSD License 295 */ 296 BSD_2_CLAUSE_FREEBSD, 297 /** 298 * BSD 2-Clause NetBSD License 299 */ 300 BSD_2_CLAUSE_NETBSD, 301 /** 302 * BSD-2-Clause Plus Patent License 303 */ 304 BSD_2_CLAUSE_PATENT, 305 /** 306 * BSD 2-Clause with views sentence 307 */ 308 BSD_2_CLAUSE_VIEWS, 309 /** 310 * BSD 3-Clause "New" or "Revised" License 311 */ 312 BSD_3_CLAUSE, 313 /** 314 * BSD with attribution 315 */ 316 BSD_3_CLAUSE_ATTRIBUTION, 317 /** 318 * BSD 3-Clause Clear License 319 */ 320 BSD_3_CLAUSE_CLEAR, 321 /** 322 * Lawrence Berkeley National Labs BSD variant license 323 */ 324 BSD_3_CLAUSE_LBNL, 325 /** 326 * BSD 3-Clause Modification 327 */ 328 BSD_3_CLAUSE_MODIFICATION, 329 /** 330 * BSD 3-Clause No Military License 331 */ 332 BSD_3_CLAUSE_NO_MILITARY_LICENSE, 333 /** 334 * BSD 3-Clause No Nuclear License 335 */ 336 BSD_3_CLAUSE_NO_NUCLEAR_LICENSE, 337 /** 338 * BSD 3-Clause No Nuclear License 2014 339 */ 340 BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014, 341 /** 342 * BSD 3-Clause No Nuclear Warranty 343 */ 344 BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY, 345 /** 346 * BSD 3-Clause Open MPI variant 347 */ 348 BSD_3_CLAUSE_OPEN_MPI, 349 /** 350 * BSD 4-Clause "Original" or "Old" License 351 */ 352 BSD_4_CLAUSE, 353 /** 354 * BSD 4 Clause Shortened 355 */ 356 BSD_4_CLAUSE_SHORTENED, 357 /** 358 * BSD-4-Clause (University of California-Specific) 359 */ 360 BSD_4_CLAUSE_UC, 361 /** 362 * BSD 4.3 RENO License 363 */ 364 BSD_4_3RENO, 365 /** 366 * BSD 4.3 TAHOE License 367 */ 368 BSD_4_3TAHOE, 369 /** 370 * BSD Advertising Acknowledgement License 371 */ 372 BSD_ADVERTISING_ACKNOWLEDGEMENT, 373 /** 374 * BSD with Attribution and HPND disclaimer 375 */ 376 BSD_ATTRIBUTION_HPND_DISCLAIMER, 377 /** 378 * BSD Protection License 379 */ 380 BSD_PROTECTION, 381 /** 382 * BSD Source Code Attribution 383 */ 384 BSD_SOURCE_CODE, 385 /** 386 * Boost Software License 1.0 387 */ 388 BSL_1_0, 389 /** 390 * Business Source License 1.1 391 */ 392 BUSL_1_1, 393 /** 394 * bzip2 and libbzip2 License v1.0.5 395 */ 396 BZIP2_1_0_5, 397 /** 398 * bzip2 and libbzip2 License v1.0.6 399 */ 400 BZIP2_1_0_6, 401 /** 402 * Computational Use of Data Agreement v1.0 403 */ 404 C_UDA_1_0, 405 /** 406 * Cryptographic Autonomy License 1.0 407 */ 408 CAL_1_0, 409 /** 410 * Cryptographic Autonomy License 1.0 (Combined Work Exception) 411 */ 412 CAL_1_0_COMBINED_WORK_EXCEPTION, 413 /** 414 * Caldera License 415 */ 416 CALDERA, 417 /** 418 * Computer Associates Trusted Open Source License 1.1 419 */ 420 CATOSL_1_1, 421 /** 422 * Creative Commons Attribution 1.0 Generic 423 */ 424 CC_BY_1_0, 425 /** 426 * Creative Commons Attribution 2.0 Generic 427 */ 428 CC_BY_2_0, 429 /** 430 * Creative Commons Attribution 2.5 Generic 431 */ 432 CC_BY_2_5, 433 /** 434 * Creative Commons Attribution 2.5 Australia 435 */ 436 CC_BY_2_5_AU, 437 /** 438 * Creative Commons Attribution 3.0 Unported 439 */ 440 CC_BY_3_0, 441 /** 442 * Creative Commons Attribution 3.0 Austria 443 */ 444 CC_BY_3_0_AT, 445 /** 446 * Creative Commons Attribution 3.0 Germany 447 */ 448 CC_BY_3_0_DE, 449 /** 450 * Creative Commons Attribution 3.0 IGO 451 */ 452 CC_BY_3_0_IGO, 453 /** 454 * Creative Commons Attribution 3.0 Netherlands 455 */ 456 CC_BY_3_0_NL, 457 /** 458 * Creative Commons Attribution 3.0 United States 459 */ 460 CC_BY_3_0_US, 461 /** 462 * Creative Commons Attribution 4.0 International 463 */ 464 CC_BY_4_0, 465 /** 466 * Creative Commons Attribution Non Commercial 1.0 Generic 467 */ 468 CC_BY_NC_1_0, 469 /** 470 * Creative Commons Attribution Non Commercial 2.0 Generic 471 */ 472 CC_BY_NC_2_0, 473 /** 474 * Creative Commons Attribution Non Commercial 2.5 Generic 475 */ 476 CC_BY_NC_2_5, 477 /** 478 * Creative Commons Attribution Non Commercial 3.0 Unported 479 */ 480 CC_BY_NC_3_0, 481 /** 482 * Creative Commons Attribution Non Commercial 3.0 Germany 483 */ 484 CC_BY_NC_3_0_DE, 485 /** 486 * Creative Commons Attribution Non Commercial 4.0 International 487 */ 488 CC_BY_NC_4_0, 489 /** 490 * Creative Commons Attribution Non Commercial No Derivatives 1.0 Generic 491 */ 492 CC_BY_NC_ND_1_0, 493 /** 494 * Creative Commons Attribution Non Commercial No Derivatives 2.0 Generic 495 */ 496 CC_BY_NC_ND_2_0, 497 /** 498 * Creative Commons Attribution Non Commercial No Derivatives 2.5 Generic 499 */ 500 CC_BY_NC_ND_2_5, 501 /** 502 * Creative Commons Attribution Non Commercial No Derivatives 3.0 Unported 503 */ 504 CC_BY_NC_ND_3_0, 505 /** 506 * Creative Commons Attribution Non Commercial No Derivatives 3.0 Germany 507 */ 508 CC_BY_NC_ND_3_0_DE, 509 /** 510 * Creative Commons Attribution Non Commercial No Derivatives 3.0 IGO 511 */ 512 CC_BY_NC_ND_3_0_IGO, 513 /** 514 * Creative Commons Attribution Non Commercial No Derivatives 4.0 International 515 */ 516 CC_BY_NC_ND_4_0, 517 /** 518 * Creative Commons Attribution Non Commercial Share Alike 1.0 Generic 519 */ 520 CC_BY_NC_SA_1_0, 521 /** 522 * Creative Commons Attribution Non Commercial Share Alike 2.0 Generic 523 */ 524 CC_BY_NC_SA_2_0, 525 /** 526 * Creative Commons Attribution Non Commercial Share Alike 2.0 Germany 527 */ 528 CC_BY_NC_SA_2_0_DE, 529 /** 530 * Creative Commons Attribution-NonCommercial-ShareAlike 2.0 France 531 */ 532 CC_BY_NC_SA_2_0_FR, 533 /** 534 * Creative Commons Attribution Non Commercial Share Alike 2.0 England and Wales 535 */ 536 CC_BY_NC_SA_2_0_UK, 537 /** 538 * Creative Commons Attribution Non Commercial Share Alike 2.5 Generic 539 */ 540 CC_BY_NC_SA_2_5, 541 /** 542 * Creative Commons Attribution Non Commercial Share Alike 3.0 Unported 543 */ 544 CC_BY_NC_SA_3_0, 545 /** 546 * Creative Commons Attribution Non Commercial Share Alike 3.0 Germany 547 */ 548 CC_BY_NC_SA_3_0_DE, 549 /** 550 * Creative Commons Attribution Non Commercial Share Alike 3.0 IGO 551 */ 552 CC_BY_NC_SA_3_0_IGO, 553 /** 554 * Creative Commons Attribution Non Commercial Share Alike 4.0 International 555 */ 556 CC_BY_NC_SA_4_0, 557 /** 558 * Creative Commons Attribution No Derivatives 1.0 Generic 559 */ 560 CC_BY_ND_1_0, 561 /** 562 * Creative Commons Attribution No Derivatives 2.0 Generic 563 */ 564 CC_BY_ND_2_0, 565 /** 566 * Creative Commons Attribution No Derivatives 2.5 Generic 567 */ 568 CC_BY_ND_2_5, 569 /** 570 * Creative Commons Attribution No Derivatives 3.0 Unported 571 */ 572 CC_BY_ND_3_0, 573 /** 574 * Creative Commons Attribution No Derivatives 3.0 Germany 575 */ 576 CC_BY_ND_3_0_DE, 577 /** 578 * Creative Commons Attribution No Derivatives 4.0 International 579 */ 580 CC_BY_ND_4_0, 581 /** 582 * Creative Commons Attribution Share Alike 1.0 Generic 583 */ 584 CC_BY_SA_1_0, 585 /** 586 * Creative Commons Attribution Share Alike 2.0 Generic 587 */ 588 CC_BY_SA_2_0, 589 /** 590 * Creative Commons Attribution Share Alike 2.0 England and Wales 591 */ 592 CC_BY_SA_2_0_UK, 593 /** 594 * Creative Commons Attribution Share Alike 2.1 Japan 595 */ 596 CC_BY_SA_2_1_JP, 597 /** 598 * Creative Commons Attribution Share Alike 2.5 Generic 599 */ 600 CC_BY_SA_2_5, 601 /** 602 * Creative Commons Attribution Share Alike 3.0 Unported 603 */ 604 CC_BY_SA_3_0, 605 /** 606 * Creative Commons Attribution Share Alike 3.0 Austria 607 */ 608 CC_BY_SA_3_0_AT, 609 /** 610 * Creative Commons Attribution Share Alike 3.0 Germany 611 */ 612 CC_BY_SA_3_0_DE, 613 /** 614 * Creative Commons Attribution-ShareAlike 3.0 IGO 615 */ 616 CC_BY_SA_3_0_IGO, 617 /** 618 * Creative Commons Attribution Share Alike 4.0 International 619 */ 620 CC_BY_SA_4_0, 621 /** 622 * Creative Commons Public Domain Dedication and Certification 623 */ 624 CC_PDDC, 625 /** 626 * Creative Commons Zero v1.0 Universal 627 */ 628 CC0_1_0, 629 /** 630 * Common Development and Distribution License 1.0 631 */ 632 CDDL_1_0, 633 /** 634 * Common Development and Distribution License 1.1 635 */ 636 CDDL_1_1, 637 /** 638 * Common Documentation License 1.0 639 */ 640 CDL_1_0, 641 /** 642 * Community Data License Agreement Permissive 1.0 643 */ 644 CDLA_PERMISSIVE_1_0, 645 /** 646 * Community Data License Agreement Permissive 2.0 647 */ 648 CDLA_PERMISSIVE_2_0, 649 /** 650 * Community Data License Agreement Sharing 1.0 651 */ 652 CDLA_SHARING_1_0, 653 /** 654 * CeCILL Free Software License Agreement v1.0 655 */ 656 CECILL_1_0, 657 /** 658 * CeCILL Free Software License Agreement v1.1 659 */ 660 CECILL_1_1, 661 /** 662 * CeCILL Free Software License Agreement v2.0 663 */ 664 CECILL_2_0, 665 /** 666 * CeCILL Free Software License Agreement v2.1 667 */ 668 CECILL_2_1, 669 /** 670 * CeCILL-B Free Software License Agreement 671 */ 672 CECILL_B, 673 /** 674 * CeCILL-C Free Software License Agreement 675 */ 676 CECILL_C, 677 /** 678 * CERN Open Hardware Licence v1.1 679 */ 680 CERN_OHL_1_1, 681 /** 682 * CERN Open Hardware Licence v1.2 683 */ 684 CERN_OHL_1_2, 685 /** 686 * CERN Open Hardware Licence Version 2 - Permissive 687 */ 688 CERN_OHL_P_2_0, 689 /** 690 * CERN Open Hardware Licence Version 2 - Strongly Reciprocal 691 */ 692 CERN_OHL_S_2_0, 693 /** 694 * CERN Open Hardware Licence Version 2 - Weakly Reciprocal 695 */ 696 CERN_OHL_W_2_0, 697 /** 698 * CFITSIO License 699 */ 700 CFITSIO, 701 /** 702 * Checkmk License 703 */ 704 CHECKMK, 705 /** 706 * Clarified Artistic License 707 */ 708 CLARTISTIC, 709 /** 710 * Clips License 711 */ 712 CLIPS, 713 /** 714 * CMU Mach License 715 */ 716 CMU_MACH, 717 /** 718 * CNRI Jython License 719 */ 720 CNRI_JYTHON, 721 /** 722 * CNRI Python License 723 */ 724 CNRI_PYTHON, 725 /** 726 * CNRI Python Open Source GPL Compatible License Agreement 727 */ 728 CNRI_PYTHON_GPL_COMPATIBLE, 729 /** 730 * Copyfree Open Innovation License 731 */ 732 COIL_1_0, 733 /** 734 * Community Specification License 1.0 735 */ 736 COMMUNITY_SPEC_1_0, 737 /** 738 * Condor Public License v1.1 739 */ 740 CONDOR_1_1, 741 /** 742 * copyleft-next 0.3.0 743 */ 744 COPYLEFT_NEXT_0_3_0, 745 /** 746 * copyleft-next 0.3.1 747 */ 748 COPYLEFT_NEXT_0_3_1, 749 /** 750 * Cornell Lossless JPEG License 751 */ 752 CORNELL_LOSSLESS_JPEG, 753 /** 754 * Common Public Attribution License 1.0 755 */ 756 CPAL_1_0, 757 /** 758 * Common Public License 1.0 759 */ 760 CPL_1_0, 761 /** 762 * Code Project Open License 1.02 763 */ 764 CPOL_1_02, 765 /** 766 * Crossword License 767 */ 768 CROSSWORD, 769 /** 770 * CrystalStacker License 771 */ 772 CRYSTALSTACKER, 773 /** 774 * CUA Office Public License v1.0 775 */ 776 CUA_OPL_1_0, 777 /** 778 * Cube License 779 */ 780 CUBE, 781 /** 782 * curl License 783 */ 784 CURL, 785 /** 786 * Deutsche Freie Software Lizenz 787 */ 788 D_FSL_1_0, 789 /** 790 * diffmark license 791 */ 792 DIFFMARK, 793 /** 794 * Data licence Germany ? attribution ? version 2.0 795 */ 796 DL_DE_BY_2_0, 797 /** 798 * DOC License 799 */ 800 DOC, 801 /** 802 * Dotseqn License 803 */ 804 DOTSEQN, 805 /** 806 * Detection Rule License 1.0 807 */ 808 DRL_1_0, 809 /** 810 * DSDP License 811 */ 812 DSDP, 813 /** 814 * David M. Gay dtoa License 815 */ 816 DTOA, 817 /** 818 * dvipdfm License 819 */ 820 DVIPDFM, 821 /** 822 * Educational Community License v1.0 823 */ 824 ECL_1_0, 825 /** 826 * Educational Community License v2.0 827 */ 828 ECL_2_0, 829 /** 830 * eCos license version 2.0 831 */ 832 ECOS_2_0, 833 /** 834 * Eiffel Forum License v1.0 835 */ 836 EFL_1_0, 837 /** 838 * Eiffel Forum License v2.0 839 */ 840 EFL_2_0, 841 /** 842 * eGenix.com Public License 1.1.0 843 */ 844 EGENIX, 845 /** 846 * Elastic License 2.0 847 */ 848 ELASTIC_2_0, 849 /** 850 * Entessa Public License v1.0 851 */ 852 ENTESSA, 853 /** 854 * EPICS Open License 855 */ 856 EPICS, 857 /** 858 * Eclipse Public License 1.0 859 */ 860 EPL_1_0, 861 /** 862 * Eclipse Public License 2.0 863 */ 864 EPL_2_0, 865 /** 866 * Erlang Public License v1.1 867 */ 868 ERLPL_1_1, 869 /** 870 * Etalab Open License 2.0 871 */ 872 ETALAB_2_0, 873 /** 874 * EU DataGrid Software License 875 */ 876 EUDATAGRID, 877 /** 878 * European Union Public License 1.0 879 */ 880 EUPL_1_0, 881 /** 882 * European Union Public License 1.1 883 */ 884 EUPL_1_1, 885 /** 886 * European Union Public License 1.2 887 */ 888 EUPL_1_2, 889 /** 890 * Eurosym License 891 */ 892 EUROSYM, 893 /** 894 * Fair License 895 */ 896 FAIR, 897 /** 898 * Fraunhofer FDK AAC Codec Library 899 */ 900 FDK_AAC, 901 /** 902 * Frameworx Open License 1.0 903 */ 904 FRAMEWORX_1_0, 905 /** 906 * FreeBSD Documentation License 907 */ 908 FREEBSD_DOC, 909 /** 910 * FreeImage Public License v1.0 911 */ 912 FREEIMAGE, 913 /** 914 * FSF All Permissive License 915 */ 916 FSFAP, 917 /** 918 * FSF Unlimited License 919 */ 920 FSFUL, 921 /** 922 * FSF Unlimited License (with License Retention) 923 */ 924 FSFULLR, 925 /** 926 * FSF Unlimited License (With License Retention and Warranty Disclaimer) 927 */ 928 FSFULLRWD, 929 /** 930 * Freetype Project License 931 */ 932 FTL, 933 /** 934 * GD License 935 */ 936 GD, 937 /** 938 * GNU Free Documentation License v1.1 939 */ 940 GFDL_1_1, 941 /** 942 * GNU Free Documentation License v1.1 only - invariants 943 */ 944 GFDL_1_1_INVARIANTS_ONLY, 945 /** 946 * GNU Free Documentation License v1.1 or later - invariants 947 */ 948 GFDL_1_1_INVARIANTS_OR_LATER, 949 /** 950 * GNU Free Documentation License v1.1 only - no invariants 951 */ 952 GFDL_1_1_NO_INVARIANTS_ONLY, 953 /** 954 * GNU Free Documentation License v1.1 or later - no invariants 955 */ 956 GFDL_1_1_NO_INVARIANTS_OR_LATER, 957 /** 958 * GNU Free Documentation License v1.1 only 959 */ 960 GFDL_1_1_ONLY, 961 /** 962 * GNU Free Documentation License v1.1 or later 963 */ 964 GFDL_1_1_OR_LATER, 965 /** 966 * GNU Free Documentation License v1.2 967 */ 968 GFDL_1_2, 969 /** 970 * GNU Free Documentation License v1.2 only - invariants 971 */ 972 GFDL_1_2_INVARIANTS_ONLY, 973 /** 974 * GNU Free Documentation License v1.2 or later - invariants 975 */ 976 GFDL_1_2_INVARIANTS_OR_LATER, 977 /** 978 * GNU Free Documentation License v1.2 only - no invariants 979 */ 980 GFDL_1_2_NO_INVARIANTS_ONLY, 981 /** 982 * GNU Free Documentation License v1.2 or later - no invariants 983 */ 984 GFDL_1_2_NO_INVARIANTS_OR_LATER, 985 /** 986 * GNU Free Documentation License v1.2 only 987 */ 988 GFDL_1_2_ONLY, 989 /** 990 * GNU Free Documentation License v1.2 or later 991 */ 992 GFDL_1_2_OR_LATER, 993 /** 994 * GNU Free Documentation License v1.3 995 */ 996 GFDL_1_3, 997 /** 998 * GNU Free Documentation License v1.3 only - invariants 999 */ 1000 GFDL_1_3_INVARIANTS_ONLY, 1001 /** 1002 * GNU Free Documentation License v1.3 or later - invariants 1003 */ 1004 GFDL_1_3_INVARIANTS_OR_LATER, 1005 /** 1006 * GNU Free Documentation License v1.3 only - no invariants 1007 */ 1008 GFDL_1_3_NO_INVARIANTS_ONLY, 1009 /** 1010 * GNU Free Documentation License v1.3 or later - no invariants 1011 */ 1012 GFDL_1_3_NO_INVARIANTS_OR_LATER, 1013 /** 1014 * GNU Free Documentation License v1.3 only 1015 */ 1016 GFDL_1_3_ONLY, 1017 /** 1018 * GNU Free Documentation License v1.3 or later 1019 */ 1020 GFDL_1_3_OR_LATER, 1021 /** 1022 * Giftware License 1023 */ 1024 GIFTWARE, 1025 /** 1026 * GL2PS License 1027 */ 1028 GL2PS, 1029 /** 1030 * 3dfx Glide License 1031 */ 1032 GLIDE, 1033 /** 1034 * Glulxe License 1035 */ 1036 GLULXE, 1037 /** 1038 * Good Luck With That Public License 1039 */ 1040 GLWTPL, 1041 /** 1042 * gnuplot License 1043 */ 1044 GNUPLOT, 1045 /** 1046 * GNU General Public License v1.0 only 1047 */ 1048 GPL_1_0, 1049 /** 1050 * GNU General Public License v1.0 or later 1051 */ 1052 GPL_1_0PLUS, 1053 /** 1054 * GNU General Public License v1.0 only 1055 */ 1056 GPL_1_0_ONLY, 1057 /** 1058 * GNU General Public License v1.0 or later 1059 */ 1060 GPL_1_0_OR_LATER, 1061 /** 1062 * GNU General Public License v2.0 only 1063 */ 1064 GPL_2_0, 1065 /** 1066 * GNU General Public License v2.0 or later 1067 */ 1068 GPL_2_0PLUS, 1069 /** 1070 * GNU General Public License v2.0 only 1071 */ 1072 GPL_2_0_ONLY, 1073 /** 1074 * GNU General Public License v2.0 or later 1075 */ 1076 GPL_2_0_OR_LATER, 1077 /** 1078 * GNU General Public License v2.0 w/Autoconf exception 1079 */ 1080 GPL_2_0_WITH_AUTOCONF_EXCEPTION, 1081 /** 1082 * GNU General Public License v2.0 w/Bison exception 1083 */ 1084 GPL_2_0_WITH_BISON_EXCEPTION, 1085 /** 1086 * GNU General Public License v2.0 w/Classpath exception 1087 */ 1088 GPL_2_0_WITH_CLASSPATH_EXCEPTION, 1089 /** 1090 * GNU General Public License v2.0 w/Font exception 1091 */ 1092 GPL_2_0_WITH_FONT_EXCEPTION, 1093 /** 1094 * GNU General Public License v2.0 w/GCC Runtime Library exception 1095 */ 1096 GPL_2_0_WITH_GCC_EXCEPTION, 1097 /** 1098 * GNU General Public License v3.0 only 1099 */ 1100 GPL_3_0, 1101 /** 1102 * GNU General Public License v3.0 or later 1103 */ 1104 GPL_3_0PLUS, 1105 /** 1106 * GNU General Public License v3.0 only 1107 */ 1108 GPL_3_0_ONLY, 1109 /** 1110 * GNU General Public License v3.0 or later 1111 */ 1112 GPL_3_0_OR_LATER, 1113 /** 1114 * GNU General Public License v3.0 w/Autoconf exception 1115 */ 1116 GPL_3_0_WITH_AUTOCONF_EXCEPTION, 1117 /** 1118 * GNU General Public License v3.0 w/GCC Runtime Library exception 1119 */ 1120 GPL_3_0_WITH_GCC_EXCEPTION, 1121 /** 1122 * Graphics Gems License 1123 */ 1124 GRAPHICS_GEMS, 1125 /** 1126 * gSOAP Public License v1.3b 1127 */ 1128 GSOAP_1_3B, 1129 /** 1130 * Haskell Language Report License 1131 */ 1132 HASKELLREPORT, 1133 /** 1134 * Hippocratic License 2.1 1135 */ 1136 HIPPOCRATIC_2_1, 1137 /** 1138 * Hewlett-Packard 1986 License 1139 */ 1140 HP_1986, 1141 /** 1142 * Historical Permission Notice and Disclaimer 1143 */ 1144 HPND, 1145 /** 1146 * HPND with US Government export control warning 1147 */ 1148 HPND_EXPORT_US, 1149 /** 1150 * Historical Permission Notice and Disclaimer - Markus Kuhn variant 1151 */ 1152 HPND_MARKUS_KUHN, 1153 /** 1154 * Historical Permission Notice and Disclaimer - sell variant 1155 */ 1156 HPND_SELL_VARIANT, 1157 /** 1158 * HPND sell variant with MIT disclaimer 1159 */ 1160 HPND_SELL_VARIANT_MIT_DISCLAIMER, 1161 /** 1162 * HTML Tidy License 1163 */ 1164 HTMLTIDY, 1165 /** 1166 * IBM PowerPC Initialization and Boot Software 1167 */ 1168 IBM_PIBS, 1169 /** 1170 * ICU License 1171 */ 1172 ICU, 1173 /** 1174 * IEC Code Components End-user licence agreement 1175 */ 1176 IEC_CODE_COMPONENTS_EULA, 1177 /** 1178 * Independent JPEG Group License 1179 */ 1180 IJG, 1181 /** 1182 * Independent JPEG Group License - short 1183 */ 1184 IJG_SHORT, 1185 /** 1186 * ImageMagick License 1187 */ 1188 IMAGEMAGICK, 1189 /** 1190 * iMatix Standard Function Library Agreement 1191 */ 1192 IMATIX, 1193 /** 1194 * Imlib2 License 1195 */ 1196 IMLIB2, 1197 /** 1198 * Info-ZIP License 1199 */ 1200 INFO_ZIP, 1201 /** 1202 * Inner Net License v2.0 1203 */ 1204 INNER_NET_2_0, 1205 /** 1206 * Intel Open Source License 1207 */ 1208 INTEL, 1209 /** 1210 * Intel ACPI Software License Agreement 1211 */ 1212 INTEL_ACPI, 1213 /** 1214 * Interbase Public License v1.0 1215 */ 1216 INTERBASE_1_0, 1217 /** 1218 * IPA Font License 1219 */ 1220 IPA, 1221 /** 1222 * IBM Public License v1.0 1223 */ 1224 IPL_1_0, 1225 /** 1226 * ISC License 1227 */ 1228 ISC, 1229 /** 1230 * Jam License 1231 */ 1232 JAM, 1233 /** 1234 * JasPer License 1235 */ 1236 JASPER_2_0, 1237 /** 1238 * JPL Image Use Policy 1239 */ 1240 JPL_IMAGE, 1241 /** 1242 * Japan Network Information Center License 1243 */ 1244 JPNIC, 1245 /** 1246 * JSON License 1247 */ 1248 JSON, 1249 /** 1250 * Kazlib License 1251 */ 1252 KAZLIB, 1253 /** 1254 * Knuth CTAN License 1255 */ 1256 KNUTH_CTAN, 1257 /** 1258 * Licence Art Libre 1.2 1259 */ 1260 LAL_1_2, 1261 /** 1262 * Licence Art Libre 1.3 1263 */ 1264 LAL_1_3, 1265 /** 1266 * Latex2e License 1267 */ 1268 LATEX2E, 1269 /** 1270 * Latex2e with translated notice permission 1271 */ 1272 LATEX2E_TRANSLATED_NOTICE, 1273 /** 1274 * Leptonica License 1275 */ 1276 LEPTONICA, 1277 /** 1278 * GNU Library General Public License v2 only 1279 */ 1280 LGPL_2_0, 1281 /** 1282 * GNU Library General Public License v2 or later 1283 */ 1284 LGPL_2_0PLUS, 1285 /** 1286 * GNU Library General Public License v2 only 1287 */ 1288 LGPL_2_0_ONLY, 1289 /** 1290 * GNU Library General Public License v2 or later 1291 */ 1292 LGPL_2_0_OR_LATER, 1293 /** 1294 * GNU Lesser General Public License v2.1 only 1295 */ 1296 LGPL_2_1, 1297 /** 1298 * GNU Lesser General Public License v2.1 or later 1299 */ 1300 LGPL_2_1PLUS, 1301 /** 1302 * GNU Lesser General Public License v2.1 only 1303 */ 1304 LGPL_2_1_ONLY, 1305 /** 1306 * GNU Lesser General Public License v2.1 or later 1307 */ 1308 LGPL_2_1_OR_LATER, 1309 /** 1310 * GNU Lesser General Public License v3.0 only 1311 */ 1312 LGPL_3_0, 1313 /** 1314 * GNU Lesser General Public License v3.0 or later 1315 */ 1316 LGPL_3_0PLUS, 1317 /** 1318 * GNU Lesser General Public License v3.0 only 1319 */ 1320 LGPL_3_0_ONLY, 1321 /** 1322 * GNU Lesser General Public License v3.0 or later 1323 */ 1324 LGPL_3_0_OR_LATER, 1325 /** 1326 * Lesser General Public License For Linguistic Resources 1327 */ 1328 LGPLLR, 1329 /** 1330 * libpng License 1331 */ 1332 LIBPNG, 1333 /** 1334 * PNG Reference Library version 2 1335 */ 1336 LIBPNG_2_0, 1337 /** 1338 * libselinux public domain notice 1339 */ 1340 LIBSELINUX_1_0, 1341 /** 1342 * libtiff License 1343 */ 1344 LIBTIFF, 1345 /** 1346 * libutil David Nugent License 1347 */ 1348 LIBUTIL_DAVID_NUGENT, 1349 /** 1350 * Licence Libre du Québec ? Permissive version 1.1 1351 */ 1352 LILIQ_P_1_1, 1353 /** 1354 * Licence Libre du Québec ? Réciprocité version 1.1 1355 */ 1356 LILIQ_R_1_1, 1357 /** 1358 * Licence Libre du Québec ? Réciprocité forte version 1.1 1359 */ 1360 LILIQ_RPLUS_1_1, 1361 /** 1362 * Linux man-pages - 1 paragraph 1363 */ 1364 LINUX_MAN_PAGES_1_PARA, 1365 /** 1366 * Linux man-pages Copyleft 1367 */ 1368 LINUX_MAN_PAGES_COPYLEFT, 1369 /** 1370 * Linux man-pages Copyleft - 2 paragraphs 1371 */ 1372 LINUX_MAN_PAGES_COPYLEFT_2_PARA, 1373 /** 1374 * Linux man-pages Copyleft Variant 1375 */ 1376 LINUX_MAN_PAGES_COPYLEFT_VAR, 1377 /** 1378 * Linux Kernel Variant of OpenIB.org license 1379 */ 1380 LINUX_OPENIB, 1381 /** 1382 * Common Lisp LOOP License 1383 */ 1384 LOOP, 1385 /** 1386 * Lucent Public License Version 1.0 1387 */ 1388 LPL_1_0, 1389 /** 1390 * Lucent Public License v1.02 1391 */ 1392 LPL_1_02, 1393 /** 1394 * LaTeX Project Public License v1.0 1395 */ 1396 LPPL_1_0, 1397 /** 1398 * LaTeX Project Public License v1.1 1399 */ 1400 LPPL_1_1, 1401 /** 1402 * LaTeX Project Public License v1.2 1403 */ 1404 LPPL_1_2, 1405 /** 1406 * LaTeX Project Public License v1.3a 1407 */ 1408 LPPL_1_3A, 1409 /** 1410 * LaTeX Project Public License v1.3c 1411 */ 1412 LPPL_1_3C, 1413 /** 1414 * LZMA SDK License (versions 9.11 to 9.20) 1415 */ 1416 LZMA_SDK_9_11_TO_9_20, 1417 /** 1418 * LZMA SDK License (versions 9.22 and beyond) 1419 */ 1420 LZMA_SDK_9_22, 1421 /** 1422 * MakeIndex License 1423 */ 1424 MAKEINDEX, 1425 /** 1426 * Martin Birgmeier License 1427 */ 1428 MARTIN_BIRGMEIER, 1429 /** 1430 * metamail License 1431 */ 1432 METAMAIL, 1433 /** 1434 * Minpack License 1435 */ 1436 MINPACK, 1437 /** 1438 * The MirOS Licence 1439 */ 1440 MIROS, 1441 /** 1442 * MIT License 1443 */ 1444 MIT, 1445 /** 1446 * MIT No Attribution 1447 */ 1448 MIT_0, 1449 /** 1450 * Enlightenment License (e16) 1451 */ 1452 MIT_ADVERTISING, 1453 /** 1454 * CMU License 1455 */ 1456 MIT_CMU, 1457 /** 1458 * enna License 1459 */ 1460 MIT_ENNA, 1461 /** 1462 * feh License 1463 */ 1464 MIT_FEH, 1465 /** 1466 * MIT Festival Variant 1467 */ 1468 MIT_FESTIVAL, 1469 /** 1470 * MIT License Modern Variant 1471 */ 1472 MIT_MODERN_VARIANT, 1473 /** 1474 * MIT Open Group variant 1475 */ 1476 MIT_OPEN_GROUP, 1477 /** 1478 * MIT Tom Wu Variant 1479 */ 1480 MIT_WU, 1481 /** 1482 * MIT +no-false-attribs license 1483 */ 1484 MITNFA, 1485 /** 1486 * Motosoto License 1487 */ 1488 MOTOSOTO, 1489 /** 1490 * mpi Permissive License 1491 */ 1492 MPI_PERMISSIVE, 1493 /** 1494 * mpich2 License 1495 */ 1496 MPICH2, 1497 /** 1498 * Mozilla Public License 1.0 1499 */ 1500 MPL_1_0, 1501 /** 1502 * Mozilla Public License 1.1 1503 */ 1504 MPL_1_1, 1505 /** 1506 * Mozilla Public License 2.0 1507 */ 1508 MPL_2_0, 1509 /** 1510 * Mozilla Public License 2.0 (no copyleft exception) 1511 */ 1512 MPL_2_0_NO_COPYLEFT_EXCEPTION, 1513 /** 1514 * mplus Font License 1515 */ 1516 MPLUS, 1517 /** 1518 * Microsoft Limited Public License 1519 */ 1520 MS_LPL, 1521 /** 1522 * Microsoft Public License 1523 */ 1524 MS_PL, 1525 /** 1526 * Microsoft Reciprocal License 1527 */ 1528 MS_RL, 1529 /** 1530 * Matrix Template Library License 1531 */ 1532 MTLL, 1533 /** 1534 * Mulan Permissive Software License, Version 1 1535 */ 1536 MULANPSL_1_0, 1537 /** 1538 * Mulan Permissive Software License, Version 2 1539 */ 1540 MULANPSL_2_0, 1541 /** 1542 * Multics License 1543 */ 1544 MULTICS, 1545 /** 1546 * Mup License 1547 */ 1548 MUP, 1549 /** 1550 * Nara Institute of Science and Technology License (2003) 1551 */ 1552 NAIST_2003, 1553 /** 1554 * NASA Open Source Agreement 1.3 1555 */ 1556 NASA_1_3, 1557 /** 1558 * Naumen Public License 1559 */ 1560 NAUMEN, 1561 /** 1562 * Net Boolean Public License v1 1563 */ 1564 NBPL_1_0, 1565 /** 1566 * Non-Commercial Government Licence 1567 */ 1568 NCGL_UK_2_0, 1569 /** 1570 * University of Illinois/NCSA Open Source License 1571 */ 1572 NCSA, 1573 /** 1574 * Net-SNMP License 1575 */ 1576 NET_SNMP, 1577 /** 1578 * NetCDF license 1579 */ 1580 NETCDF, 1581 /** 1582 * Newsletr License 1583 */ 1584 NEWSLETR, 1585 /** 1586 * Nethack General Public License 1587 */ 1588 NGPL, 1589 /** 1590 * NICTA Public Software License, Version 1.0 1591 */ 1592 NICTA_1_0, 1593 /** 1594 * NIST Public Domain Notice 1595 */ 1596 NIST_PD, 1597 /** 1598 * NIST Public Domain Notice with license fallback 1599 */ 1600 NIST_PD_FALLBACK, 1601 /** 1602 * NIST Software License 1603 */ 1604 NIST_SOFTWARE, 1605 /** 1606 * Norwegian Licence for Open Government Data (NLOD) 1.0 1607 */ 1608 NLOD_1_0, 1609 /** 1610 * Norwegian Licence for Open Government Data (NLOD) 2.0 1611 */ 1612 NLOD_2_0, 1613 /** 1614 * No Limit Public License 1615 */ 1616 NLPL, 1617 /** 1618 * Nokia Open Source License 1619 */ 1620 NOKIA, 1621 /** 1622 * Netizen Open Source License 1623 */ 1624 NOSL, 1625 /** 1626 * Not an open source license. 1627 */ 1628 NOT_OPEN_SOURCE, 1629 /** 1630 * Noweb License 1631 */ 1632 NOWEB, 1633 /** 1634 * Netscape Public License v1.0 1635 */ 1636 NPL_1_0, 1637 /** 1638 * Netscape Public License v1.1 1639 */ 1640 NPL_1_1, 1641 /** 1642 * Non-Profit Open Software License 3.0 1643 */ 1644 NPOSL_3_0, 1645 /** 1646 * NRL License 1647 */ 1648 NRL, 1649 /** 1650 * NTP License 1651 */ 1652 NTP, 1653 /** 1654 * NTP No Attribution 1655 */ 1656 NTP_0, 1657 /** 1658 * Nunit License 1659 */ 1660 NUNIT, 1661 /** 1662 * Open Use of Data Agreement v1.0 1663 */ 1664 O_UDA_1_0, 1665 /** 1666 * Open CASCADE Technology Public License 1667 */ 1668 OCCT_PL, 1669 /** 1670 * OCLC Research Public License 2.0 1671 */ 1672 OCLC_2_0, 1673 /** 1674 * Open Data Commons Open Database License v1.0 1675 */ 1676 ODBL_1_0, 1677 /** 1678 * Open Data Commons Attribution License v1.0 1679 */ 1680 ODC_BY_1_0, 1681 /** 1682 * OFFIS License 1683 */ 1684 OFFIS, 1685 /** 1686 * SIL Open Font License 1.0 1687 */ 1688 OFL_1_0, 1689 /** 1690 * SIL Open Font License 1.0 with no Reserved Font Name 1691 */ 1692 OFL_1_0_NO_RFN, 1693 /** 1694 * SIL Open Font License 1.0 with Reserved Font Name 1695 */ 1696 OFL_1_0_RFN, 1697 /** 1698 * SIL Open Font License 1.1 1699 */ 1700 OFL_1_1, 1701 /** 1702 * SIL Open Font License 1.1 with no Reserved Font Name 1703 */ 1704 OFL_1_1_NO_RFN, 1705 /** 1706 * SIL Open Font License 1.1 with Reserved Font Name 1707 */ 1708 OFL_1_1_RFN, 1709 /** 1710 * OGC Software License, Version 1.0 1711 */ 1712 OGC_1_0, 1713 /** 1714 * Taiwan Open Government Data License, version 1.0 1715 */ 1716 OGDL_TAIWAN_1_0, 1717 /** 1718 * Open Government Licence - Canada 1719 */ 1720 OGL_CANADA_2_0, 1721 /** 1722 * Open Government Licence v1.0 1723 */ 1724 OGL_UK_1_0, 1725 /** 1726 * Open Government Licence v2.0 1727 */ 1728 OGL_UK_2_0, 1729 /** 1730 * Open Government Licence v3.0 1731 */ 1732 OGL_UK_3_0, 1733 /** 1734 * Open Group Test Suite License 1735 */ 1736 OGTSL, 1737 /** 1738 * Open LDAP Public License v1.1 1739 */ 1740 OLDAP_1_1, 1741 /** 1742 * Open LDAP Public License v1.2 1743 */ 1744 OLDAP_1_2, 1745 /** 1746 * Open LDAP Public License v1.3 1747 */ 1748 OLDAP_1_3, 1749 /** 1750 * Open LDAP Public License v1.4 1751 */ 1752 OLDAP_1_4, 1753 /** 1754 * Open LDAP Public License v2.0 (or possibly 2.0A and 2.0B) 1755 */ 1756 OLDAP_2_0, 1757 /** 1758 * Open LDAP Public License v2.0.1 1759 */ 1760 OLDAP_2_0_1, 1761 /** 1762 * Open LDAP Public License v2.1 1763 */ 1764 OLDAP_2_1, 1765 /** 1766 * Open LDAP Public License v2.2 1767 */ 1768 OLDAP_2_2, 1769 /** 1770 * Open LDAP Public License v2.2.1 1771 */ 1772 OLDAP_2_2_1, 1773 /** 1774 * Open LDAP Public License 2.2.2 1775 */ 1776 OLDAP_2_2_2, 1777 /** 1778 * Open LDAP Public License v2.3 1779 */ 1780 OLDAP_2_3, 1781 /** 1782 * Open LDAP Public License v2.4 1783 */ 1784 OLDAP_2_4, 1785 /** 1786 * Open LDAP Public License v2.5 1787 */ 1788 OLDAP_2_5, 1789 /** 1790 * Open LDAP Public License v2.6 1791 */ 1792 OLDAP_2_6, 1793 /** 1794 * Open LDAP Public License v2.7 1795 */ 1796 OLDAP_2_7, 1797 /** 1798 * Open LDAP Public License v2.8 1799 */ 1800 OLDAP_2_8, 1801 /** 1802 * Open Logistics Foundation License Version 1.3 1803 */ 1804 OLFL_1_3, 1805 /** 1806 * Open Market License 1807 */ 1808 OML, 1809 /** 1810 * OpenPBS v2.3 Software License 1811 */ 1812 OPENPBS_2_3, 1813 /** 1814 * OpenSSL License 1815 */ 1816 OPENSSL, 1817 /** 1818 * Open Public License v1.0 1819 */ 1820 OPL_1_0, 1821 /** 1822 * United Kingdom Open Parliament Licence v3.0 1823 */ 1824 OPL_UK_3_0, 1825 /** 1826 * Open Publication License v1.0 1827 */ 1828 OPUBL_1_0, 1829 /** 1830 * OSET Public License version 2.1 1831 */ 1832 OSET_PL_2_1, 1833 /** 1834 * Open Software License 1.0 1835 */ 1836 OSL_1_0, 1837 /** 1838 * Open Software License 1.1 1839 */ 1840 OSL_1_1, 1841 /** 1842 * Open Software License 2.0 1843 */ 1844 OSL_2_0, 1845 /** 1846 * Open Software License 2.1 1847 */ 1848 OSL_2_1, 1849 /** 1850 * Open Software License 3.0 1851 */ 1852 OSL_3_0, 1853 /** 1854 * The Parity Public License 6.0.0 1855 */ 1856 PARITY_6_0_0, 1857 /** 1858 * The Parity Public License 7.0.0 1859 */ 1860 PARITY_7_0_0, 1861 /** 1862 * Open Data Commons Public Domain Dedication & License 1.0 1863 */ 1864 PDDL_1_0, 1865 /** 1866 * PHP License v3.0 1867 */ 1868 PHP_3_0, 1869 /** 1870 * PHP License v3.01 1871 */ 1872 PHP_3_01, 1873 /** 1874 * Plexus Classworlds License 1875 */ 1876 PLEXUS, 1877 /** 1878 * PolyForm Noncommercial License 1.0.0 1879 */ 1880 POLYFORM_NONCOMMERCIAL_1_0_0, 1881 /** 1882 * PolyForm Small Business License 1.0.0 1883 */ 1884 POLYFORM_SMALL_BUSINESS_1_0_0, 1885 /** 1886 * PostgreSQL License 1887 */ 1888 POSTGRESQL, 1889 /** 1890 * Python Software Foundation License 2.0 1891 */ 1892 PSF_2_0, 1893 /** 1894 * psfrag License 1895 */ 1896 PSFRAG, 1897 /** 1898 * psutils License 1899 */ 1900 PSUTILS, 1901 /** 1902 * Python License 2.0 1903 */ 1904 PYTHON_2_0, 1905 /** 1906 * Python License 2.0.1 1907 */ 1908 PYTHON_2_0_1, 1909 /** 1910 * Qhull License 1911 */ 1912 QHULL, 1913 /** 1914 * Q Public License 1.0 1915 */ 1916 QPL_1_0, 1917 /** 1918 * Q Public License 1.0 - INRIA 2004 variant 1919 */ 1920 QPL_1_0_INRIA_2004, 1921 /** 1922 * Rdisc License 1923 */ 1924 RDISC, 1925 /** 1926 * Red Hat eCos Public License v1.1 1927 */ 1928 RHECOS_1_1, 1929 /** 1930 * Reciprocal Public License 1.1 1931 */ 1932 RPL_1_1, 1933 /** 1934 * Reciprocal Public License 1.5 1935 */ 1936 RPL_1_5, 1937 /** 1938 * RealNetworks Public Source License v1.0 1939 */ 1940 RPSL_1_0, 1941 /** 1942 * RSA Message-Digest License 1943 */ 1944 RSA_MD, 1945 /** 1946 * Ricoh Source Code Public License 1947 */ 1948 RSCPL, 1949 /** 1950 * Ruby License 1951 */ 1952 RUBY, 1953 /** 1954 * Sax Public Domain Notice 1955 */ 1956 SAX_PD, 1957 /** 1958 * Saxpath License 1959 */ 1960 SAXPATH, 1961 /** 1962 * SCEA Shared Source License 1963 */ 1964 SCEA, 1965 /** 1966 * Scheme Language Report License 1967 */ 1968 SCHEMEREPORT, 1969 /** 1970 * Sendmail License 1971 */ 1972 SENDMAIL, 1973 /** 1974 * Sendmail License 8.23 1975 */ 1976 SENDMAIL_8_23, 1977 /** 1978 * SGI Free Software License B v1.0 1979 */ 1980 SGI_B_1_0, 1981 /** 1982 * SGI Free Software License B v1.1 1983 */ 1984 SGI_B_1_1, 1985 /** 1986 * SGI Free Software License B v2.0 1987 */ 1988 SGI_B_2_0, 1989 /** 1990 * SGP4 Permission Notice 1991 */ 1992 SGP4, 1993 /** 1994 * Solderpad Hardware License v0.5 1995 */ 1996 SHL_0_5, 1997 /** 1998 * Solderpad Hardware License, Version 0.51 1999 */ 2000 SHL_0_51, 2001 /** 2002 * Simple Public License 2.0 2003 */ 2004 SIMPL_2_0, 2005 /** 2006 * Sun Industry Standards Source License v1.1 2007 */ 2008 SISSL, 2009 /** 2010 * Sun Industry Standards Source License v1.2 2011 */ 2012 SISSL_1_2, 2013 /** 2014 * Sleepycat License 2015 */ 2016 SLEEPYCAT, 2017 /** 2018 * Standard ML of New Jersey License 2019 */ 2020 SMLNJ, 2021 /** 2022 * Secure Messaging Protocol Public License 2023 */ 2024 SMPPL, 2025 /** 2026 * SNIA Public License 1.1 2027 */ 2028 SNIA, 2029 /** 2030 * snprintf License 2031 */ 2032 SNPRINTF, 2033 /** 2034 * Spencer License 86 2035 */ 2036 SPENCER_86, 2037 /** 2038 * Spencer License 94 2039 */ 2040 SPENCER_94, 2041 /** 2042 * Spencer License 99 2043 */ 2044 SPENCER_99, 2045 /** 2046 * Sun Public License v1.0 2047 */ 2048 SPL_1_0, 2049 /** 2050 * SSH OpenSSH license 2051 */ 2052 SSH_OPENSSH, 2053 /** 2054 * SSH short notice 2055 */ 2056 SSH_SHORT, 2057 /** 2058 * Server Side Public License, v 1 2059 */ 2060 SSPL_1_0, 2061 /** 2062 * Standard ML of New Jersey License 2063 */ 2064 STANDARDML_NJ, 2065 /** 2066 * SugarCRM Public License v1.1.3 2067 */ 2068 SUGARCRM_1_1_3, 2069 /** 2070 * SunPro License 2071 */ 2072 SUNPRO, 2073 /** 2074 * Scheme Widget Library (SWL) Software License Agreement 2075 */ 2076 SWL, 2077 /** 2078 * Symlinks License 2079 */ 2080 SYMLINKS, 2081 /** 2082 * TAPR Open Hardware License v1.0 2083 */ 2084 TAPR_OHL_1_0, 2085 /** 2086 * TCL/TK License 2087 */ 2088 TCL, 2089 /** 2090 * TCP Wrappers License 2091 */ 2092 TCP_WRAPPERS, 2093 /** 2094 * TermReadKey License 2095 */ 2096 TERMREADKEY, 2097 /** 2098 * TMate Open Source License 2099 */ 2100 TMATE, 2101 /** 2102 * TORQUE v2.5+ Software License v1.1 2103 */ 2104 TORQUE_1_1, 2105 /** 2106 * Trusster Open Source License 2107 */ 2108 TOSL, 2109 /** 2110 * Time::ParseDate License 2111 */ 2112 TPDL, 2113 /** 2114 * THOR Public License 1.0 2115 */ 2116 TPL_1_0, 2117 /** 2118 * Text-Tabs+Wrap License 2119 */ 2120 TTWL, 2121 /** 2122 * Technische Universitaet Berlin License 1.0 2123 */ 2124 TU_BERLIN_1_0, 2125 /** 2126 * Technische Universitaet Berlin License 2.0 2127 */ 2128 TU_BERLIN_2_0, 2129 /** 2130 * UCAR License 2131 */ 2132 UCAR, 2133 /** 2134 * Upstream Compatibility License v1.0 2135 */ 2136 UCL_1_0, 2137 /** 2138 * Unicode License Agreement - Data Files and Software (2015) 2139 */ 2140 UNICODE_DFS_2015, 2141 /** 2142 * Unicode License Agreement - Data Files and Software (2016) 2143 */ 2144 UNICODE_DFS_2016, 2145 /** 2146 * Unicode Terms of Use 2147 */ 2148 UNICODE_TOU, 2149 /** 2150 * UnixCrypt License 2151 */ 2152 UNIXCRYPT, 2153 /** 2154 * The Unlicense 2155 */ 2156 UNLICENSE, 2157 /** 2158 * Universal Permissive License v1.0 2159 */ 2160 UPL_1_0, 2161 /** 2162 * Vim License 2163 */ 2164 VIM, 2165 /** 2166 * VOSTROM Public License for Open Source 2167 */ 2168 VOSTROM, 2169 /** 2170 * Vovida Software License v1.0 2171 */ 2172 VSL_1_0, 2173 /** 2174 * W3C Software Notice and License (2002-12-31) 2175 */ 2176 W3C, 2177 /** 2178 * W3C Software Notice and License (1998-07-20) 2179 */ 2180 W3C_19980720, 2181 /** 2182 * W3C Software Notice and Document License (2015-05-13) 2183 */ 2184 W3C_20150513, 2185 /** 2186 * w3m License 2187 */ 2188 W3M, 2189 /** 2190 * Sybase Open Watcom Public License 1.0 2191 */ 2192 WATCOM_1_0, 2193 /** 2194 * Widget Workshop License 2195 */ 2196 WIDGET_WORKSHOP, 2197 /** 2198 * Wsuipa License 2199 */ 2200 WSUIPA, 2201 /** 2202 * Do What The F*ck You Want To Public License 2203 */ 2204 WTFPL, 2205 /** 2206 * wxWindows Library License 2207 */ 2208 WXWINDOWS, 2209 /** 2210 * X11 License 2211 */ 2212 X11, 2213 /** 2214 * X11 License Distribution Modification Variant 2215 */ 2216 X11_DISTRIBUTE_MODIFICATIONS_VARIANT, 2217 /** 2218 * Xdebug License v 1.03 2219 */ 2220 XDEBUG_1_03, 2221 /** 2222 * Xerox License 2223 */ 2224 XEROX, 2225 /** 2226 * Xfig License 2227 */ 2228 XFIG, 2229 /** 2230 * XFree86 License 1.1 2231 */ 2232 XFREE86_1_1, 2233 /** 2234 * xinetd License 2235 */ 2236 XINETD, 2237 /** 2238 * xlock License 2239 */ 2240 XLOCK, 2241 /** 2242 * X.Net License 2243 */ 2244 XNET, 2245 /** 2246 * XPP License 2247 */ 2248 XPP, 2249 /** 2250 * XSkat License 2251 */ 2252 XSKAT, 2253 /** 2254 * Yahoo! Public License v1.0 2255 */ 2256 YPL_1_0, 2257 /** 2258 * Yahoo! Public License v1.1 2259 */ 2260 YPL_1_1, 2261 /** 2262 * Zed License 2263 */ 2264 ZED, 2265 /** 2266 * Zend License v2.0 2267 */ 2268 ZEND_2_0, 2269 /** 2270 * Zimbra Public License v1.3 2271 */ 2272 ZIMBRA_1_3, 2273 /** 2274 * Zimbra Public License v1.4 2275 */ 2276 ZIMBRA_1_4, 2277 /** 2278 * zlib License 2279 */ 2280 ZLIB, 2281 /** 2282 * zlib/libpng License with Acknowledgement 2283 */ 2284 ZLIB_ACKNOWLEDGEMENT, 2285 /** 2286 * Zope Public License 1.1 2287 */ 2288 ZPL_1_1, 2289 /** 2290 * Zope Public License 2.0 2291 */ 2292 ZPL_2_0, 2293 /** 2294 * Zope Public License 2.1 2295 */ 2296 ZPL_2_1, 2297 /** 2298 * added to help the parsers 2299 */ 2300 NULL; 2301 2302 public static SPDXLicense fromCode(String codeString) throws FHIRException { 2303 if (codeString == null || "".equals(codeString)) 2304 return null; 2305 if ("0BSD".equals(codeString)) 2306 return _0BSD; 2307 if ("AAL".equals(codeString)) 2308 return AAL; 2309 if ("Abstyles".equals(codeString)) 2310 return ABSTYLES; 2311 if ("AdaCore-doc".equals(codeString)) 2312 return ADACORE_DOC; 2313 if ("Adobe-2006".equals(codeString)) 2314 return ADOBE_2006; 2315 if ("Adobe-Glyph".equals(codeString)) 2316 return ADOBE_GLYPH; 2317 if ("ADSL".equals(codeString)) 2318 return ADSL; 2319 if ("AFL-1.1".equals(codeString)) 2320 return AFL_1_1; 2321 if ("AFL-1.2".equals(codeString)) 2322 return AFL_1_2; 2323 if ("AFL-2.0".equals(codeString)) 2324 return AFL_2_0; 2325 if ("AFL-2.1".equals(codeString)) 2326 return AFL_2_1; 2327 if ("AFL-3.0".equals(codeString)) 2328 return AFL_3_0; 2329 if ("Afmparse".equals(codeString)) 2330 return AFMPARSE; 2331 if ("AGPL-1.0".equals(codeString)) 2332 return AGPL_1_0; 2333 if ("AGPL-1.0-only".equals(codeString)) 2334 return AGPL_1_0_ONLY; 2335 if ("AGPL-1.0-or-later".equals(codeString)) 2336 return AGPL_1_0_OR_LATER; 2337 if ("AGPL-3.0".equals(codeString)) 2338 return AGPL_3_0; 2339 if ("AGPL-3.0-only".equals(codeString)) 2340 return AGPL_3_0_ONLY; 2341 if ("AGPL-3.0-or-later".equals(codeString)) 2342 return AGPL_3_0_OR_LATER; 2343 if ("Aladdin".equals(codeString)) 2344 return ALADDIN; 2345 if ("AMDPLPA".equals(codeString)) 2346 return AMDPLPA; 2347 if ("AML".equals(codeString)) 2348 return AML; 2349 if ("AMPAS".equals(codeString)) 2350 return AMPAS; 2351 if ("ANTLR-PD".equals(codeString)) 2352 return ANTLR_PD; 2353 if ("ANTLR-PD-fallback".equals(codeString)) 2354 return ANTLR_PD_FALLBACK; 2355 if ("Apache-1.0".equals(codeString)) 2356 return APACHE_1_0; 2357 if ("Apache-1.1".equals(codeString)) 2358 return APACHE_1_1; 2359 if ("Apache-2.0".equals(codeString)) 2360 return APACHE_2_0; 2361 if ("APAFML".equals(codeString)) 2362 return APAFML; 2363 if ("APL-1.0".equals(codeString)) 2364 return APL_1_0; 2365 if ("App-s2p".equals(codeString)) 2366 return APP_S2P; 2367 if ("APSL-1.0".equals(codeString)) 2368 return APSL_1_0; 2369 if ("APSL-1.1".equals(codeString)) 2370 return APSL_1_1; 2371 if ("APSL-1.2".equals(codeString)) 2372 return APSL_1_2; 2373 if ("APSL-2.0".equals(codeString)) 2374 return APSL_2_0; 2375 if ("Arphic-1999".equals(codeString)) 2376 return ARPHIC_1999; 2377 if ("Artistic-1.0".equals(codeString)) 2378 return ARTISTIC_1_0; 2379 if ("Artistic-1.0-cl8".equals(codeString)) 2380 return ARTISTIC_1_0_CL8; 2381 if ("Artistic-1.0-Perl".equals(codeString)) 2382 return ARTISTIC_1_0_PERL; 2383 if ("Artistic-2.0".equals(codeString)) 2384 return ARTISTIC_2_0; 2385 if ("ASWF-Digital-Assets-1.0".equals(codeString)) 2386 return ASWF_DIGITAL_ASSETS_1_0; 2387 if ("ASWF-Digital-Assets-1.1".equals(codeString)) 2388 return ASWF_DIGITAL_ASSETS_1_1; 2389 if ("Baekmuk".equals(codeString)) 2390 return BAEKMUK; 2391 if ("Bahyph".equals(codeString)) 2392 return BAHYPH; 2393 if ("Barr".equals(codeString)) 2394 return BARR; 2395 if ("Beerware".equals(codeString)) 2396 return BEERWARE; 2397 if ("Bitstream-Charter".equals(codeString)) 2398 return BITSTREAM_CHARTER; 2399 if ("Bitstream-Vera".equals(codeString)) 2400 return BITSTREAM_VERA; 2401 if ("BitTorrent-1.0".equals(codeString)) 2402 return BITTORRENT_1_0; 2403 if ("BitTorrent-1.1".equals(codeString)) 2404 return BITTORRENT_1_1; 2405 if ("blessing".equals(codeString)) 2406 return BLESSING; 2407 if ("BlueOak-1.0.0".equals(codeString)) 2408 return BLUEOAK_1_0_0; 2409 if ("Boehm-GC".equals(codeString)) 2410 return BOEHM_GC; 2411 if ("Borceux".equals(codeString)) 2412 return BORCEUX; 2413 if ("Brian-Gladman-3-Clause".equals(codeString)) 2414 return BRIAN_GLADMAN_3_CLAUSE; 2415 if ("BSD-1-Clause".equals(codeString)) 2416 return BSD_1_CLAUSE; 2417 if ("BSD-2-Clause".equals(codeString)) 2418 return BSD_2_CLAUSE; 2419 if ("BSD-2-Clause-FreeBSD".equals(codeString)) 2420 return BSD_2_CLAUSE_FREEBSD; 2421 if ("BSD-2-Clause-NetBSD".equals(codeString)) 2422 return BSD_2_CLAUSE_NETBSD; 2423 if ("BSD-2-Clause-Patent".equals(codeString)) 2424 return BSD_2_CLAUSE_PATENT; 2425 if ("BSD-2-Clause-Views".equals(codeString)) 2426 return BSD_2_CLAUSE_VIEWS; 2427 if ("BSD-3-Clause".equals(codeString)) 2428 return BSD_3_CLAUSE; 2429 if ("BSD-3-Clause-Attribution".equals(codeString)) 2430 return BSD_3_CLAUSE_ATTRIBUTION; 2431 if ("BSD-3-Clause-Clear".equals(codeString)) 2432 return BSD_3_CLAUSE_CLEAR; 2433 if ("BSD-3-Clause-LBNL".equals(codeString)) 2434 return BSD_3_CLAUSE_LBNL; 2435 if ("BSD-3-Clause-Modification".equals(codeString)) 2436 return BSD_3_CLAUSE_MODIFICATION; 2437 if ("BSD-3-Clause-No-Military-License".equals(codeString)) 2438 return BSD_3_CLAUSE_NO_MILITARY_LICENSE; 2439 if ("BSD-3-Clause-No-Nuclear-License".equals(codeString)) 2440 return BSD_3_CLAUSE_NO_NUCLEAR_LICENSE; 2441 if ("BSD-3-Clause-No-Nuclear-License-2014".equals(codeString)) 2442 return BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014; 2443 if ("BSD-3-Clause-No-Nuclear-Warranty".equals(codeString)) 2444 return BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY; 2445 if ("BSD-3-Clause-Open-MPI".equals(codeString)) 2446 return BSD_3_CLAUSE_OPEN_MPI; 2447 if ("BSD-4-Clause".equals(codeString)) 2448 return BSD_4_CLAUSE; 2449 if ("BSD-4-Clause-Shortened".equals(codeString)) 2450 return BSD_4_CLAUSE_SHORTENED; 2451 if ("BSD-4-Clause-UC".equals(codeString)) 2452 return BSD_4_CLAUSE_UC; 2453 if ("BSD-4.3RENO".equals(codeString)) 2454 return BSD_4_3RENO; 2455 if ("BSD-4.3TAHOE".equals(codeString)) 2456 return BSD_4_3TAHOE; 2457 if ("BSD-Advertising-Acknowledgement".equals(codeString)) 2458 return BSD_ADVERTISING_ACKNOWLEDGEMENT; 2459 if ("BSD-Attribution-HPND-disclaimer".equals(codeString)) 2460 return BSD_ATTRIBUTION_HPND_DISCLAIMER; 2461 if ("BSD-Protection".equals(codeString)) 2462 return BSD_PROTECTION; 2463 if ("BSD-Source-Code".equals(codeString)) 2464 return BSD_SOURCE_CODE; 2465 if ("BSL-1.0".equals(codeString)) 2466 return BSL_1_0; 2467 if ("BUSL-1.1".equals(codeString)) 2468 return BUSL_1_1; 2469 if ("bzip2-1.0.5".equals(codeString)) 2470 return BZIP2_1_0_5; 2471 if ("bzip2-1.0.6".equals(codeString)) 2472 return BZIP2_1_0_6; 2473 if ("C-UDA-1.0".equals(codeString)) 2474 return C_UDA_1_0; 2475 if ("CAL-1.0".equals(codeString)) 2476 return CAL_1_0; 2477 if ("CAL-1.0-Combined-Work-Exception".equals(codeString)) 2478 return CAL_1_0_COMBINED_WORK_EXCEPTION; 2479 if ("Caldera".equals(codeString)) 2480 return CALDERA; 2481 if ("CATOSL-1.1".equals(codeString)) 2482 return CATOSL_1_1; 2483 if ("CC-BY-1.0".equals(codeString)) 2484 return CC_BY_1_0; 2485 if ("CC-BY-2.0".equals(codeString)) 2486 return CC_BY_2_0; 2487 if ("CC-BY-2.5".equals(codeString)) 2488 return CC_BY_2_5; 2489 if ("CC-BY-2.5-AU".equals(codeString)) 2490 return CC_BY_2_5_AU; 2491 if ("CC-BY-3.0".equals(codeString)) 2492 return CC_BY_3_0; 2493 if ("CC-BY-3.0-AT".equals(codeString)) 2494 return CC_BY_3_0_AT; 2495 if ("CC-BY-3.0-DE".equals(codeString)) 2496 return CC_BY_3_0_DE; 2497 if ("CC-BY-3.0-IGO".equals(codeString)) 2498 return CC_BY_3_0_IGO; 2499 if ("CC-BY-3.0-NL".equals(codeString)) 2500 return CC_BY_3_0_NL; 2501 if ("CC-BY-3.0-US".equals(codeString)) 2502 return CC_BY_3_0_US; 2503 if ("CC-BY-4.0".equals(codeString)) 2504 return CC_BY_4_0; 2505 if ("CC-BY-NC-1.0".equals(codeString)) 2506 return CC_BY_NC_1_0; 2507 if ("CC-BY-NC-2.0".equals(codeString)) 2508 return CC_BY_NC_2_0; 2509 if ("CC-BY-NC-2.5".equals(codeString)) 2510 return CC_BY_NC_2_5; 2511 if ("CC-BY-NC-3.0".equals(codeString)) 2512 return CC_BY_NC_3_0; 2513 if ("CC-BY-NC-3.0-DE".equals(codeString)) 2514 return CC_BY_NC_3_0_DE; 2515 if ("CC-BY-NC-4.0".equals(codeString)) 2516 return CC_BY_NC_4_0; 2517 if ("CC-BY-NC-ND-1.0".equals(codeString)) 2518 return CC_BY_NC_ND_1_0; 2519 if ("CC-BY-NC-ND-2.0".equals(codeString)) 2520 return CC_BY_NC_ND_2_0; 2521 if ("CC-BY-NC-ND-2.5".equals(codeString)) 2522 return CC_BY_NC_ND_2_5; 2523 if ("CC-BY-NC-ND-3.0".equals(codeString)) 2524 return CC_BY_NC_ND_3_0; 2525 if ("CC-BY-NC-ND-3.0-DE".equals(codeString)) 2526 return CC_BY_NC_ND_3_0_DE; 2527 if ("CC-BY-NC-ND-3.0-IGO".equals(codeString)) 2528 return CC_BY_NC_ND_3_0_IGO; 2529 if ("CC-BY-NC-ND-4.0".equals(codeString)) 2530 return CC_BY_NC_ND_4_0; 2531 if ("CC-BY-NC-SA-1.0".equals(codeString)) 2532 return CC_BY_NC_SA_1_0; 2533 if ("CC-BY-NC-SA-2.0".equals(codeString)) 2534 return CC_BY_NC_SA_2_0; 2535 if ("CC-BY-NC-SA-2.0-DE".equals(codeString)) 2536 return CC_BY_NC_SA_2_0_DE; 2537 if ("CC-BY-NC-SA-2.0-FR".equals(codeString)) 2538 return CC_BY_NC_SA_2_0_FR; 2539 if ("CC-BY-NC-SA-2.0-UK".equals(codeString)) 2540 return CC_BY_NC_SA_2_0_UK; 2541 if ("CC-BY-NC-SA-2.5".equals(codeString)) 2542 return CC_BY_NC_SA_2_5; 2543 if ("CC-BY-NC-SA-3.0".equals(codeString)) 2544 return CC_BY_NC_SA_3_0; 2545 if ("CC-BY-NC-SA-3.0-DE".equals(codeString)) 2546 return CC_BY_NC_SA_3_0_DE; 2547 if ("CC-BY-NC-SA-3.0-IGO".equals(codeString)) 2548 return CC_BY_NC_SA_3_0_IGO; 2549 if ("CC-BY-NC-SA-4.0".equals(codeString)) 2550 return CC_BY_NC_SA_4_0; 2551 if ("CC-BY-ND-1.0".equals(codeString)) 2552 return CC_BY_ND_1_0; 2553 if ("CC-BY-ND-2.0".equals(codeString)) 2554 return CC_BY_ND_2_0; 2555 if ("CC-BY-ND-2.5".equals(codeString)) 2556 return CC_BY_ND_2_5; 2557 if ("CC-BY-ND-3.0".equals(codeString)) 2558 return CC_BY_ND_3_0; 2559 if ("CC-BY-ND-3.0-DE".equals(codeString)) 2560 return CC_BY_ND_3_0_DE; 2561 if ("CC-BY-ND-4.0".equals(codeString)) 2562 return CC_BY_ND_4_0; 2563 if ("CC-BY-SA-1.0".equals(codeString)) 2564 return CC_BY_SA_1_0; 2565 if ("CC-BY-SA-2.0".equals(codeString)) 2566 return CC_BY_SA_2_0; 2567 if ("CC-BY-SA-2.0-UK".equals(codeString)) 2568 return CC_BY_SA_2_0_UK; 2569 if ("CC-BY-SA-2.1-JP".equals(codeString)) 2570 return CC_BY_SA_2_1_JP; 2571 if ("CC-BY-SA-2.5".equals(codeString)) 2572 return CC_BY_SA_2_5; 2573 if ("CC-BY-SA-3.0".equals(codeString)) 2574 return CC_BY_SA_3_0; 2575 if ("CC-BY-SA-3.0-AT".equals(codeString)) 2576 return CC_BY_SA_3_0_AT; 2577 if ("CC-BY-SA-3.0-DE".equals(codeString)) 2578 return CC_BY_SA_3_0_DE; 2579 if ("CC-BY-SA-3.0-IGO".equals(codeString)) 2580 return CC_BY_SA_3_0_IGO; 2581 if ("CC-BY-SA-4.0".equals(codeString)) 2582 return CC_BY_SA_4_0; 2583 if ("CC-PDDC".equals(codeString)) 2584 return CC_PDDC; 2585 if ("CC0-1.0".equals(codeString)) 2586 return CC0_1_0; 2587 if ("CDDL-1.0".equals(codeString)) 2588 return CDDL_1_0; 2589 if ("CDDL-1.1".equals(codeString)) 2590 return CDDL_1_1; 2591 if ("CDL-1.0".equals(codeString)) 2592 return CDL_1_0; 2593 if ("CDLA-Permissive-1.0".equals(codeString)) 2594 return CDLA_PERMISSIVE_1_0; 2595 if ("CDLA-Permissive-2.0".equals(codeString)) 2596 return CDLA_PERMISSIVE_2_0; 2597 if ("CDLA-Sharing-1.0".equals(codeString)) 2598 return CDLA_SHARING_1_0; 2599 if ("CECILL-1.0".equals(codeString)) 2600 return CECILL_1_0; 2601 if ("CECILL-1.1".equals(codeString)) 2602 return CECILL_1_1; 2603 if ("CECILL-2.0".equals(codeString)) 2604 return CECILL_2_0; 2605 if ("CECILL-2.1".equals(codeString)) 2606 return CECILL_2_1; 2607 if ("CECILL-B".equals(codeString)) 2608 return CECILL_B; 2609 if ("CECILL-C".equals(codeString)) 2610 return CECILL_C; 2611 if ("CERN-OHL-1.1".equals(codeString)) 2612 return CERN_OHL_1_1; 2613 if ("CERN-OHL-1.2".equals(codeString)) 2614 return CERN_OHL_1_2; 2615 if ("CERN-OHL-P-2.0".equals(codeString)) 2616 return CERN_OHL_P_2_0; 2617 if ("CERN-OHL-S-2.0".equals(codeString)) 2618 return CERN_OHL_S_2_0; 2619 if ("CERN-OHL-W-2.0".equals(codeString)) 2620 return CERN_OHL_W_2_0; 2621 if ("CFITSIO".equals(codeString)) 2622 return CFITSIO; 2623 if ("checkmk".equals(codeString)) 2624 return CHECKMK; 2625 if ("ClArtistic".equals(codeString)) 2626 return CLARTISTIC; 2627 if ("Clips".equals(codeString)) 2628 return CLIPS; 2629 if ("CMU-Mach".equals(codeString)) 2630 return CMU_MACH; 2631 if ("CNRI-Jython".equals(codeString)) 2632 return CNRI_JYTHON; 2633 if ("CNRI-Python".equals(codeString)) 2634 return CNRI_PYTHON; 2635 if ("CNRI-Python-GPL-Compatible".equals(codeString)) 2636 return CNRI_PYTHON_GPL_COMPATIBLE; 2637 if ("COIL-1.0".equals(codeString)) 2638 return COIL_1_0; 2639 if ("Community-Spec-1.0".equals(codeString)) 2640 return COMMUNITY_SPEC_1_0; 2641 if ("Condor-1.1".equals(codeString)) 2642 return CONDOR_1_1; 2643 if ("copyleft-next-0.3.0".equals(codeString)) 2644 return COPYLEFT_NEXT_0_3_0; 2645 if ("copyleft-next-0.3.1".equals(codeString)) 2646 return COPYLEFT_NEXT_0_3_1; 2647 if ("Cornell-Lossless-JPEG".equals(codeString)) 2648 return CORNELL_LOSSLESS_JPEG; 2649 if ("CPAL-1.0".equals(codeString)) 2650 return CPAL_1_0; 2651 if ("CPL-1.0".equals(codeString)) 2652 return CPL_1_0; 2653 if ("CPOL-1.02".equals(codeString)) 2654 return CPOL_1_02; 2655 if ("Crossword".equals(codeString)) 2656 return CROSSWORD; 2657 if ("CrystalStacker".equals(codeString)) 2658 return CRYSTALSTACKER; 2659 if ("CUA-OPL-1.0".equals(codeString)) 2660 return CUA_OPL_1_0; 2661 if ("Cube".equals(codeString)) 2662 return CUBE; 2663 if ("curl".equals(codeString)) 2664 return CURL; 2665 if ("D-FSL-1.0".equals(codeString)) 2666 return D_FSL_1_0; 2667 if ("diffmark".equals(codeString)) 2668 return DIFFMARK; 2669 if ("DL-DE-BY-2.0".equals(codeString)) 2670 return DL_DE_BY_2_0; 2671 if ("DOC".equals(codeString)) 2672 return DOC; 2673 if ("Dotseqn".equals(codeString)) 2674 return DOTSEQN; 2675 if ("DRL-1.0".equals(codeString)) 2676 return DRL_1_0; 2677 if ("DSDP".equals(codeString)) 2678 return DSDP; 2679 if ("dtoa".equals(codeString)) 2680 return DTOA; 2681 if ("dvipdfm".equals(codeString)) 2682 return DVIPDFM; 2683 if ("ECL-1.0".equals(codeString)) 2684 return ECL_1_0; 2685 if ("ECL-2.0".equals(codeString)) 2686 return ECL_2_0; 2687 if ("eCos-2.0".equals(codeString)) 2688 return ECOS_2_0; 2689 if ("EFL-1.0".equals(codeString)) 2690 return EFL_1_0; 2691 if ("EFL-2.0".equals(codeString)) 2692 return EFL_2_0; 2693 if ("eGenix".equals(codeString)) 2694 return EGENIX; 2695 if ("Elastic-2.0".equals(codeString)) 2696 return ELASTIC_2_0; 2697 if ("Entessa".equals(codeString)) 2698 return ENTESSA; 2699 if ("EPICS".equals(codeString)) 2700 return EPICS; 2701 if ("EPL-1.0".equals(codeString)) 2702 return EPL_1_0; 2703 if ("EPL-2.0".equals(codeString)) 2704 return EPL_2_0; 2705 if ("ErlPL-1.1".equals(codeString)) 2706 return ERLPL_1_1; 2707 if ("etalab-2.0".equals(codeString)) 2708 return ETALAB_2_0; 2709 if ("EUDatagrid".equals(codeString)) 2710 return EUDATAGRID; 2711 if ("EUPL-1.0".equals(codeString)) 2712 return EUPL_1_0; 2713 if ("EUPL-1.1".equals(codeString)) 2714 return EUPL_1_1; 2715 if ("EUPL-1.2".equals(codeString)) 2716 return EUPL_1_2; 2717 if ("Eurosym".equals(codeString)) 2718 return EUROSYM; 2719 if ("Fair".equals(codeString)) 2720 return FAIR; 2721 if ("FDK-AAC".equals(codeString)) 2722 return FDK_AAC; 2723 if ("Frameworx-1.0".equals(codeString)) 2724 return FRAMEWORX_1_0; 2725 if ("FreeBSD-DOC".equals(codeString)) 2726 return FREEBSD_DOC; 2727 if ("FreeImage".equals(codeString)) 2728 return FREEIMAGE; 2729 if ("FSFAP".equals(codeString)) 2730 return FSFAP; 2731 if ("FSFUL".equals(codeString)) 2732 return FSFUL; 2733 if ("FSFULLR".equals(codeString)) 2734 return FSFULLR; 2735 if ("FSFULLRWD".equals(codeString)) 2736 return FSFULLRWD; 2737 if ("FTL".equals(codeString)) 2738 return FTL; 2739 if ("GD".equals(codeString)) 2740 return GD; 2741 if ("GFDL-1.1".equals(codeString)) 2742 return GFDL_1_1; 2743 if ("GFDL-1.1-invariants-only".equals(codeString)) 2744 return GFDL_1_1_INVARIANTS_ONLY; 2745 if ("GFDL-1.1-invariants-or-later".equals(codeString)) 2746 return GFDL_1_1_INVARIANTS_OR_LATER; 2747 if ("GFDL-1.1-no-invariants-only".equals(codeString)) 2748 return GFDL_1_1_NO_INVARIANTS_ONLY; 2749 if ("GFDL-1.1-no-invariants-or-later".equals(codeString)) 2750 return GFDL_1_1_NO_INVARIANTS_OR_LATER; 2751 if ("GFDL-1.1-only".equals(codeString)) 2752 return GFDL_1_1_ONLY; 2753 if ("GFDL-1.1-or-later".equals(codeString)) 2754 return GFDL_1_1_OR_LATER; 2755 if ("GFDL-1.2".equals(codeString)) 2756 return GFDL_1_2; 2757 if ("GFDL-1.2-invariants-only".equals(codeString)) 2758 return GFDL_1_2_INVARIANTS_ONLY; 2759 if ("GFDL-1.2-invariants-or-later".equals(codeString)) 2760 return GFDL_1_2_INVARIANTS_OR_LATER; 2761 if ("GFDL-1.2-no-invariants-only".equals(codeString)) 2762 return GFDL_1_2_NO_INVARIANTS_ONLY; 2763 if ("GFDL-1.2-no-invariants-or-later".equals(codeString)) 2764 return GFDL_1_2_NO_INVARIANTS_OR_LATER; 2765 if ("GFDL-1.2-only".equals(codeString)) 2766 return GFDL_1_2_ONLY; 2767 if ("GFDL-1.2-or-later".equals(codeString)) 2768 return GFDL_1_2_OR_LATER; 2769 if ("GFDL-1.3".equals(codeString)) 2770 return GFDL_1_3; 2771 if ("GFDL-1.3-invariants-only".equals(codeString)) 2772 return GFDL_1_3_INVARIANTS_ONLY; 2773 if ("GFDL-1.3-invariants-or-later".equals(codeString)) 2774 return GFDL_1_3_INVARIANTS_OR_LATER; 2775 if ("GFDL-1.3-no-invariants-only".equals(codeString)) 2776 return GFDL_1_3_NO_INVARIANTS_ONLY; 2777 if ("GFDL-1.3-no-invariants-or-later".equals(codeString)) 2778 return GFDL_1_3_NO_INVARIANTS_OR_LATER; 2779 if ("GFDL-1.3-only".equals(codeString)) 2780 return GFDL_1_3_ONLY; 2781 if ("GFDL-1.3-or-later".equals(codeString)) 2782 return GFDL_1_3_OR_LATER; 2783 if ("Giftware".equals(codeString)) 2784 return GIFTWARE; 2785 if ("GL2PS".equals(codeString)) 2786 return GL2PS; 2787 if ("Glide".equals(codeString)) 2788 return GLIDE; 2789 if ("Glulxe".equals(codeString)) 2790 return GLULXE; 2791 if ("GLWTPL".equals(codeString)) 2792 return GLWTPL; 2793 if ("gnuplot".equals(codeString)) 2794 return GNUPLOT; 2795 if ("GPL-1.0".equals(codeString)) 2796 return GPL_1_0; 2797 if ("GPL-1.0+".equals(codeString)) 2798 return GPL_1_0PLUS; 2799 if ("GPL-1.0-only".equals(codeString)) 2800 return GPL_1_0_ONLY; 2801 if ("GPL-1.0-or-later".equals(codeString)) 2802 return GPL_1_0_OR_LATER; 2803 if ("GPL-2.0".equals(codeString)) 2804 return GPL_2_0; 2805 if ("GPL-2.0+".equals(codeString)) 2806 return GPL_2_0PLUS; 2807 if ("GPL-2.0-only".equals(codeString)) 2808 return GPL_2_0_ONLY; 2809 if ("GPL-2.0-or-later".equals(codeString)) 2810 return GPL_2_0_OR_LATER; 2811 if ("GPL-2.0-with-autoconf-exception".equals(codeString)) 2812 return GPL_2_0_WITH_AUTOCONF_EXCEPTION; 2813 if ("GPL-2.0-with-bison-exception".equals(codeString)) 2814 return GPL_2_0_WITH_BISON_EXCEPTION; 2815 if ("GPL-2.0-with-classpath-exception".equals(codeString)) 2816 return GPL_2_0_WITH_CLASSPATH_EXCEPTION; 2817 if ("GPL-2.0-with-font-exception".equals(codeString)) 2818 return GPL_2_0_WITH_FONT_EXCEPTION; 2819 if ("GPL-2.0-with-GCC-exception".equals(codeString)) 2820 return GPL_2_0_WITH_GCC_EXCEPTION; 2821 if ("GPL-3.0".equals(codeString)) 2822 return GPL_3_0; 2823 if ("GPL-3.0+".equals(codeString)) 2824 return GPL_3_0PLUS; 2825 if ("GPL-3.0-only".equals(codeString)) 2826 return GPL_3_0_ONLY; 2827 if ("GPL-3.0-or-later".equals(codeString)) 2828 return GPL_3_0_OR_LATER; 2829 if ("GPL-3.0-with-autoconf-exception".equals(codeString)) 2830 return GPL_3_0_WITH_AUTOCONF_EXCEPTION; 2831 if ("GPL-3.0-with-GCC-exception".equals(codeString)) 2832 return GPL_3_0_WITH_GCC_EXCEPTION; 2833 if ("Graphics-Gems".equals(codeString)) 2834 return GRAPHICS_GEMS; 2835 if ("gSOAP-1.3b".equals(codeString)) 2836 return GSOAP_1_3B; 2837 if ("HaskellReport".equals(codeString)) 2838 return HASKELLREPORT; 2839 if ("Hippocratic-2.1".equals(codeString)) 2840 return HIPPOCRATIC_2_1; 2841 if ("HP-1986".equals(codeString)) 2842 return HP_1986; 2843 if ("HPND".equals(codeString)) 2844 return HPND; 2845 if ("HPND-export-US".equals(codeString)) 2846 return HPND_EXPORT_US; 2847 if ("HPND-Markus-Kuhn".equals(codeString)) 2848 return HPND_MARKUS_KUHN; 2849 if ("HPND-sell-variant".equals(codeString)) 2850 return HPND_SELL_VARIANT; 2851 if ("HPND-sell-variant-MIT-disclaimer".equals(codeString)) 2852 return HPND_SELL_VARIANT_MIT_DISCLAIMER; 2853 if ("HTMLTIDY".equals(codeString)) 2854 return HTMLTIDY; 2855 if ("IBM-pibs".equals(codeString)) 2856 return IBM_PIBS; 2857 if ("ICU".equals(codeString)) 2858 return ICU; 2859 if ("IEC-Code-Components-EULA".equals(codeString)) 2860 return IEC_CODE_COMPONENTS_EULA; 2861 if ("IJG".equals(codeString)) 2862 return IJG; 2863 if ("IJG-short".equals(codeString)) 2864 return IJG_SHORT; 2865 if ("ImageMagick".equals(codeString)) 2866 return IMAGEMAGICK; 2867 if ("iMatix".equals(codeString)) 2868 return IMATIX; 2869 if ("Imlib2".equals(codeString)) 2870 return IMLIB2; 2871 if ("Info-ZIP".equals(codeString)) 2872 return INFO_ZIP; 2873 if ("Inner-Net-2.0".equals(codeString)) 2874 return INNER_NET_2_0; 2875 if ("Intel".equals(codeString)) 2876 return INTEL; 2877 if ("Intel-ACPI".equals(codeString)) 2878 return INTEL_ACPI; 2879 if ("Interbase-1.0".equals(codeString)) 2880 return INTERBASE_1_0; 2881 if ("IPA".equals(codeString)) 2882 return IPA; 2883 if ("IPL-1.0".equals(codeString)) 2884 return IPL_1_0; 2885 if ("ISC".equals(codeString)) 2886 return ISC; 2887 if ("Jam".equals(codeString)) 2888 return JAM; 2889 if ("JasPer-2.0".equals(codeString)) 2890 return JASPER_2_0; 2891 if ("JPL-image".equals(codeString)) 2892 return JPL_IMAGE; 2893 if ("JPNIC".equals(codeString)) 2894 return JPNIC; 2895 if ("JSON".equals(codeString)) 2896 return JSON; 2897 if ("Kazlib".equals(codeString)) 2898 return KAZLIB; 2899 if ("Knuth-CTAN".equals(codeString)) 2900 return KNUTH_CTAN; 2901 if ("LAL-1.2".equals(codeString)) 2902 return LAL_1_2; 2903 if ("LAL-1.3".equals(codeString)) 2904 return LAL_1_3; 2905 if ("Latex2e".equals(codeString)) 2906 return LATEX2E; 2907 if ("Latex2e-translated-notice".equals(codeString)) 2908 return LATEX2E_TRANSLATED_NOTICE; 2909 if ("Leptonica".equals(codeString)) 2910 return LEPTONICA; 2911 if ("LGPL-2.0".equals(codeString)) 2912 return LGPL_2_0; 2913 if ("LGPL-2.0+".equals(codeString)) 2914 return LGPL_2_0PLUS; 2915 if ("LGPL-2.0-only".equals(codeString)) 2916 return LGPL_2_0_ONLY; 2917 if ("LGPL-2.0-or-later".equals(codeString)) 2918 return LGPL_2_0_OR_LATER; 2919 if ("LGPL-2.1".equals(codeString)) 2920 return LGPL_2_1; 2921 if ("LGPL-2.1+".equals(codeString)) 2922 return LGPL_2_1PLUS; 2923 if ("LGPL-2.1-only".equals(codeString)) 2924 return LGPL_2_1_ONLY; 2925 if ("LGPL-2.1-or-later".equals(codeString)) 2926 return LGPL_2_1_OR_LATER; 2927 if ("LGPL-3.0".equals(codeString)) 2928 return LGPL_3_0; 2929 if ("LGPL-3.0+".equals(codeString)) 2930 return LGPL_3_0PLUS; 2931 if ("LGPL-3.0-only".equals(codeString)) 2932 return LGPL_3_0_ONLY; 2933 if ("LGPL-3.0-or-later".equals(codeString)) 2934 return LGPL_3_0_OR_LATER; 2935 if ("LGPLLR".equals(codeString)) 2936 return LGPLLR; 2937 if ("Libpng".equals(codeString)) 2938 return LIBPNG; 2939 if ("libpng-2.0".equals(codeString)) 2940 return LIBPNG_2_0; 2941 if ("libselinux-1.0".equals(codeString)) 2942 return LIBSELINUX_1_0; 2943 if ("libtiff".equals(codeString)) 2944 return LIBTIFF; 2945 if ("libutil-David-Nugent".equals(codeString)) 2946 return LIBUTIL_DAVID_NUGENT; 2947 if ("LiLiQ-P-1.1".equals(codeString)) 2948 return LILIQ_P_1_1; 2949 if ("LiLiQ-R-1.1".equals(codeString)) 2950 return LILIQ_R_1_1; 2951 if ("LiLiQ-Rplus-1.1".equals(codeString)) 2952 return LILIQ_RPLUS_1_1; 2953 if ("Linux-man-pages-1-para".equals(codeString)) 2954 return LINUX_MAN_PAGES_1_PARA; 2955 if ("Linux-man-pages-copyleft".equals(codeString)) 2956 return LINUX_MAN_PAGES_COPYLEFT; 2957 if ("Linux-man-pages-copyleft-2-para".equals(codeString)) 2958 return LINUX_MAN_PAGES_COPYLEFT_2_PARA; 2959 if ("Linux-man-pages-copyleft-var".equals(codeString)) 2960 return LINUX_MAN_PAGES_COPYLEFT_VAR; 2961 if ("Linux-OpenIB".equals(codeString)) 2962 return LINUX_OPENIB; 2963 if ("LOOP".equals(codeString)) 2964 return LOOP; 2965 if ("LPL-1.0".equals(codeString)) 2966 return LPL_1_0; 2967 if ("LPL-1.02".equals(codeString)) 2968 return LPL_1_02; 2969 if ("LPPL-1.0".equals(codeString)) 2970 return LPPL_1_0; 2971 if ("LPPL-1.1".equals(codeString)) 2972 return LPPL_1_1; 2973 if ("LPPL-1.2".equals(codeString)) 2974 return LPPL_1_2; 2975 if ("LPPL-1.3a".equals(codeString)) 2976 return LPPL_1_3A; 2977 if ("LPPL-1.3c".equals(codeString)) 2978 return LPPL_1_3C; 2979 if ("LZMA-SDK-9.11-to-9.20".equals(codeString)) 2980 return LZMA_SDK_9_11_TO_9_20; 2981 if ("LZMA-SDK-9.22".equals(codeString)) 2982 return LZMA_SDK_9_22; 2983 if ("MakeIndex".equals(codeString)) 2984 return MAKEINDEX; 2985 if ("Martin-Birgmeier".equals(codeString)) 2986 return MARTIN_BIRGMEIER; 2987 if ("metamail".equals(codeString)) 2988 return METAMAIL; 2989 if ("Minpack".equals(codeString)) 2990 return MINPACK; 2991 if ("MirOS".equals(codeString)) 2992 return MIROS; 2993 if ("MIT".equals(codeString)) 2994 return MIT; 2995 if ("MIT-0".equals(codeString)) 2996 return MIT_0; 2997 if ("MIT-advertising".equals(codeString)) 2998 return MIT_ADVERTISING; 2999 if ("MIT-CMU".equals(codeString)) 3000 return MIT_CMU; 3001 if ("MIT-enna".equals(codeString)) 3002 return MIT_ENNA; 3003 if ("MIT-feh".equals(codeString)) 3004 return MIT_FEH; 3005 if ("MIT-Festival".equals(codeString)) 3006 return MIT_FESTIVAL; 3007 if ("MIT-Modern-Variant".equals(codeString)) 3008 return MIT_MODERN_VARIANT; 3009 if ("MIT-open-group".equals(codeString)) 3010 return MIT_OPEN_GROUP; 3011 if ("MIT-Wu".equals(codeString)) 3012 return MIT_WU; 3013 if ("MITNFA".equals(codeString)) 3014 return MITNFA; 3015 if ("Motosoto".equals(codeString)) 3016 return MOTOSOTO; 3017 if ("mpi-permissive".equals(codeString)) 3018 return MPI_PERMISSIVE; 3019 if ("mpich2".equals(codeString)) 3020 return MPICH2; 3021 if ("MPL-1.0".equals(codeString)) 3022 return MPL_1_0; 3023 if ("MPL-1.1".equals(codeString)) 3024 return MPL_1_1; 3025 if ("MPL-2.0".equals(codeString)) 3026 return MPL_2_0; 3027 if ("MPL-2.0-no-copyleft-exception".equals(codeString)) 3028 return MPL_2_0_NO_COPYLEFT_EXCEPTION; 3029 if ("mplus".equals(codeString)) 3030 return MPLUS; 3031 if ("MS-LPL".equals(codeString)) 3032 return MS_LPL; 3033 if ("MS-PL".equals(codeString)) 3034 return MS_PL; 3035 if ("MS-RL".equals(codeString)) 3036 return MS_RL; 3037 if ("MTLL".equals(codeString)) 3038 return MTLL; 3039 if ("MulanPSL-1.0".equals(codeString)) 3040 return MULANPSL_1_0; 3041 if ("MulanPSL-2.0".equals(codeString)) 3042 return MULANPSL_2_0; 3043 if ("Multics".equals(codeString)) 3044 return MULTICS; 3045 if ("Mup".equals(codeString)) 3046 return MUP; 3047 if ("NAIST-2003".equals(codeString)) 3048 return NAIST_2003; 3049 if ("NASA-1.3".equals(codeString)) 3050 return NASA_1_3; 3051 if ("Naumen".equals(codeString)) 3052 return NAUMEN; 3053 if ("NBPL-1.0".equals(codeString)) 3054 return NBPL_1_0; 3055 if ("NCGL-UK-2.0".equals(codeString)) 3056 return NCGL_UK_2_0; 3057 if ("NCSA".equals(codeString)) 3058 return NCSA; 3059 if ("Net-SNMP".equals(codeString)) 3060 return NET_SNMP; 3061 if ("NetCDF".equals(codeString)) 3062 return NETCDF; 3063 if ("Newsletr".equals(codeString)) 3064 return NEWSLETR; 3065 if ("NGPL".equals(codeString)) 3066 return NGPL; 3067 if ("NICTA-1.0".equals(codeString)) 3068 return NICTA_1_0; 3069 if ("NIST-PD".equals(codeString)) 3070 return NIST_PD; 3071 if ("NIST-PD-fallback".equals(codeString)) 3072 return NIST_PD_FALLBACK; 3073 if ("NIST-Software".equals(codeString)) 3074 return NIST_SOFTWARE; 3075 if ("NLOD-1.0".equals(codeString)) 3076 return NLOD_1_0; 3077 if ("NLOD-2.0".equals(codeString)) 3078 return NLOD_2_0; 3079 if ("NLPL".equals(codeString)) 3080 return NLPL; 3081 if ("Nokia".equals(codeString)) 3082 return NOKIA; 3083 if ("NOSL".equals(codeString)) 3084 return NOSL; 3085 if ("not-open-source".equals(codeString)) 3086 return NOT_OPEN_SOURCE; 3087 if ("Noweb".equals(codeString)) 3088 return NOWEB; 3089 if ("NPL-1.0".equals(codeString)) 3090 return NPL_1_0; 3091 if ("NPL-1.1".equals(codeString)) 3092 return NPL_1_1; 3093 if ("NPOSL-3.0".equals(codeString)) 3094 return NPOSL_3_0; 3095 if ("NRL".equals(codeString)) 3096 return NRL; 3097 if ("NTP".equals(codeString)) 3098 return NTP; 3099 if ("NTP-0".equals(codeString)) 3100 return NTP_0; 3101 if ("Nunit".equals(codeString)) 3102 return NUNIT; 3103 if ("O-UDA-1.0".equals(codeString)) 3104 return O_UDA_1_0; 3105 if ("OCCT-PL".equals(codeString)) 3106 return OCCT_PL; 3107 if ("OCLC-2.0".equals(codeString)) 3108 return OCLC_2_0; 3109 if ("ODbL-1.0".equals(codeString)) 3110 return ODBL_1_0; 3111 if ("ODC-By-1.0".equals(codeString)) 3112 return ODC_BY_1_0; 3113 if ("OFFIS".equals(codeString)) 3114 return OFFIS; 3115 if ("OFL-1.0".equals(codeString)) 3116 return OFL_1_0; 3117 if ("OFL-1.0-no-RFN".equals(codeString)) 3118 return OFL_1_0_NO_RFN; 3119 if ("OFL-1.0-RFN".equals(codeString)) 3120 return OFL_1_0_RFN; 3121 if ("OFL-1.1".equals(codeString)) 3122 return OFL_1_1; 3123 if ("OFL-1.1-no-RFN".equals(codeString)) 3124 return OFL_1_1_NO_RFN; 3125 if ("OFL-1.1-RFN".equals(codeString)) 3126 return OFL_1_1_RFN; 3127 if ("OGC-1.0".equals(codeString)) 3128 return OGC_1_0; 3129 if ("OGDL-Taiwan-1.0".equals(codeString)) 3130 return OGDL_TAIWAN_1_0; 3131 if ("OGL-Canada-2.0".equals(codeString)) 3132 return OGL_CANADA_2_0; 3133 if ("OGL-UK-1.0".equals(codeString)) 3134 return OGL_UK_1_0; 3135 if ("OGL-UK-2.0".equals(codeString)) 3136 return OGL_UK_2_0; 3137 if ("OGL-UK-3.0".equals(codeString)) 3138 return OGL_UK_3_0; 3139 if ("OGTSL".equals(codeString)) 3140 return OGTSL; 3141 if ("OLDAP-1.1".equals(codeString)) 3142 return OLDAP_1_1; 3143 if ("OLDAP-1.2".equals(codeString)) 3144 return OLDAP_1_2; 3145 if ("OLDAP-1.3".equals(codeString)) 3146 return OLDAP_1_3; 3147 if ("OLDAP-1.4".equals(codeString)) 3148 return OLDAP_1_4; 3149 if ("OLDAP-2.0".equals(codeString)) 3150 return OLDAP_2_0; 3151 if ("OLDAP-2.0.1".equals(codeString)) 3152 return OLDAP_2_0_1; 3153 if ("OLDAP-2.1".equals(codeString)) 3154 return OLDAP_2_1; 3155 if ("OLDAP-2.2".equals(codeString)) 3156 return OLDAP_2_2; 3157 if ("OLDAP-2.2.1".equals(codeString)) 3158 return OLDAP_2_2_1; 3159 if ("OLDAP-2.2.2".equals(codeString)) 3160 return OLDAP_2_2_2; 3161 if ("OLDAP-2.3".equals(codeString)) 3162 return OLDAP_2_3; 3163 if ("OLDAP-2.4".equals(codeString)) 3164 return OLDAP_2_4; 3165 if ("OLDAP-2.5".equals(codeString)) 3166 return OLDAP_2_5; 3167 if ("OLDAP-2.6".equals(codeString)) 3168 return OLDAP_2_6; 3169 if ("OLDAP-2.7".equals(codeString)) 3170 return OLDAP_2_7; 3171 if ("OLDAP-2.8".equals(codeString)) 3172 return OLDAP_2_8; 3173 if ("OLFL-1.3".equals(codeString)) 3174 return OLFL_1_3; 3175 if ("OML".equals(codeString)) 3176 return OML; 3177 if ("OpenPBS-2.3".equals(codeString)) 3178 return OPENPBS_2_3; 3179 if ("OpenSSL".equals(codeString)) 3180 return OPENSSL; 3181 if ("OPL-1.0".equals(codeString)) 3182 return OPL_1_0; 3183 if ("OPL-UK-3.0".equals(codeString)) 3184 return OPL_UK_3_0; 3185 if ("OPUBL-1.0".equals(codeString)) 3186 return OPUBL_1_0; 3187 if ("OSET-PL-2.1".equals(codeString)) 3188 return OSET_PL_2_1; 3189 if ("OSL-1.0".equals(codeString)) 3190 return OSL_1_0; 3191 if ("OSL-1.1".equals(codeString)) 3192 return OSL_1_1; 3193 if ("OSL-2.0".equals(codeString)) 3194 return OSL_2_0; 3195 if ("OSL-2.1".equals(codeString)) 3196 return OSL_2_1; 3197 if ("OSL-3.0".equals(codeString)) 3198 return OSL_3_0; 3199 if ("Parity-6.0.0".equals(codeString)) 3200 return PARITY_6_0_0; 3201 if ("Parity-7.0.0".equals(codeString)) 3202 return PARITY_7_0_0; 3203 if ("PDDL-1.0".equals(codeString)) 3204 return PDDL_1_0; 3205 if ("PHP-3.0".equals(codeString)) 3206 return PHP_3_0; 3207 if ("PHP-3.01".equals(codeString)) 3208 return PHP_3_01; 3209 if ("Plexus".equals(codeString)) 3210 return PLEXUS; 3211 if ("PolyForm-Noncommercial-1.0.0".equals(codeString)) 3212 return POLYFORM_NONCOMMERCIAL_1_0_0; 3213 if ("PolyForm-Small-Business-1.0.0".equals(codeString)) 3214 return POLYFORM_SMALL_BUSINESS_1_0_0; 3215 if ("PostgreSQL".equals(codeString)) 3216 return POSTGRESQL; 3217 if ("PSF-2.0".equals(codeString)) 3218 return PSF_2_0; 3219 if ("psfrag".equals(codeString)) 3220 return PSFRAG; 3221 if ("psutils".equals(codeString)) 3222 return PSUTILS; 3223 if ("Python-2.0".equals(codeString)) 3224 return PYTHON_2_0; 3225 if ("Python-2.0.1".equals(codeString)) 3226 return PYTHON_2_0_1; 3227 if ("Qhull".equals(codeString)) 3228 return QHULL; 3229 if ("QPL-1.0".equals(codeString)) 3230 return QPL_1_0; 3231 if ("QPL-1.0-INRIA-2004".equals(codeString)) 3232 return QPL_1_0_INRIA_2004; 3233 if ("Rdisc".equals(codeString)) 3234 return RDISC; 3235 if ("RHeCos-1.1".equals(codeString)) 3236 return RHECOS_1_1; 3237 if ("RPL-1.1".equals(codeString)) 3238 return RPL_1_1; 3239 if ("RPL-1.5".equals(codeString)) 3240 return RPL_1_5; 3241 if ("RPSL-1.0".equals(codeString)) 3242 return RPSL_1_0; 3243 if ("RSA-MD".equals(codeString)) 3244 return RSA_MD; 3245 if ("RSCPL".equals(codeString)) 3246 return RSCPL; 3247 if ("Ruby".equals(codeString)) 3248 return RUBY; 3249 if ("SAX-PD".equals(codeString)) 3250 return SAX_PD; 3251 if ("Saxpath".equals(codeString)) 3252 return SAXPATH; 3253 if ("SCEA".equals(codeString)) 3254 return SCEA; 3255 if ("SchemeReport".equals(codeString)) 3256 return SCHEMEREPORT; 3257 if ("Sendmail".equals(codeString)) 3258 return SENDMAIL; 3259 if ("Sendmail-8.23".equals(codeString)) 3260 return SENDMAIL_8_23; 3261 if ("SGI-B-1.0".equals(codeString)) 3262 return SGI_B_1_0; 3263 if ("SGI-B-1.1".equals(codeString)) 3264 return SGI_B_1_1; 3265 if ("SGI-B-2.0".equals(codeString)) 3266 return SGI_B_2_0; 3267 if ("SGP4".equals(codeString)) 3268 return SGP4; 3269 if ("SHL-0.5".equals(codeString)) 3270 return SHL_0_5; 3271 if ("SHL-0.51".equals(codeString)) 3272 return SHL_0_51; 3273 if ("SimPL-2.0".equals(codeString)) 3274 return SIMPL_2_0; 3275 if ("SISSL".equals(codeString)) 3276 return SISSL; 3277 if ("SISSL-1.2".equals(codeString)) 3278 return SISSL_1_2; 3279 if ("Sleepycat".equals(codeString)) 3280 return SLEEPYCAT; 3281 if ("SMLNJ".equals(codeString)) 3282 return SMLNJ; 3283 if ("SMPPL".equals(codeString)) 3284 return SMPPL; 3285 if ("SNIA".equals(codeString)) 3286 return SNIA; 3287 if ("snprintf".equals(codeString)) 3288 return SNPRINTF; 3289 if ("Spencer-86".equals(codeString)) 3290 return SPENCER_86; 3291 if ("Spencer-94".equals(codeString)) 3292 return SPENCER_94; 3293 if ("Spencer-99".equals(codeString)) 3294 return SPENCER_99; 3295 if ("SPL-1.0".equals(codeString)) 3296 return SPL_1_0; 3297 if ("SSH-OpenSSH".equals(codeString)) 3298 return SSH_OPENSSH; 3299 if ("SSH-short".equals(codeString)) 3300 return SSH_SHORT; 3301 if ("SSPL-1.0".equals(codeString)) 3302 return SSPL_1_0; 3303 if ("StandardML-NJ".equals(codeString)) 3304 return STANDARDML_NJ; 3305 if ("SugarCRM-1.1.3".equals(codeString)) 3306 return SUGARCRM_1_1_3; 3307 if ("SunPro".equals(codeString)) 3308 return SUNPRO; 3309 if ("SWL".equals(codeString)) 3310 return SWL; 3311 if ("Symlinks".equals(codeString)) 3312 return SYMLINKS; 3313 if ("TAPR-OHL-1.0".equals(codeString)) 3314 return TAPR_OHL_1_0; 3315 if ("TCL".equals(codeString)) 3316 return TCL; 3317 if ("TCP-wrappers".equals(codeString)) 3318 return TCP_WRAPPERS; 3319 if ("TermReadKey".equals(codeString)) 3320 return TERMREADKEY; 3321 if ("TMate".equals(codeString)) 3322 return TMATE; 3323 if ("TORQUE-1.1".equals(codeString)) 3324 return TORQUE_1_1; 3325 if ("TOSL".equals(codeString)) 3326 return TOSL; 3327 if ("TPDL".equals(codeString)) 3328 return TPDL; 3329 if ("TPL-1.0".equals(codeString)) 3330 return TPL_1_0; 3331 if ("TTWL".equals(codeString)) 3332 return TTWL; 3333 if ("TU-Berlin-1.0".equals(codeString)) 3334 return TU_BERLIN_1_0; 3335 if ("TU-Berlin-2.0".equals(codeString)) 3336 return TU_BERLIN_2_0; 3337 if ("UCAR".equals(codeString)) 3338 return UCAR; 3339 if ("UCL-1.0".equals(codeString)) 3340 return UCL_1_0; 3341 if ("Unicode-DFS-2015".equals(codeString)) 3342 return UNICODE_DFS_2015; 3343 if ("Unicode-DFS-2016".equals(codeString)) 3344 return UNICODE_DFS_2016; 3345 if ("Unicode-TOU".equals(codeString)) 3346 return UNICODE_TOU; 3347 if ("UnixCrypt".equals(codeString)) 3348 return UNIXCRYPT; 3349 if ("Unlicense".equals(codeString)) 3350 return UNLICENSE; 3351 if ("UPL-1.0".equals(codeString)) 3352 return UPL_1_0; 3353 if ("Vim".equals(codeString)) 3354 return VIM; 3355 if ("VOSTROM".equals(codeString)) 3356 return VOSTROM; 3357 if ("VSL-1.0".equals(codeString)) 3358 return VSL_1_0; 3359 if ("W3C".equals(codeString)) 3360 return W3C; 3361 if ("W3C-19980720".equals(codeString)) 3362 return W3C_19980720; 3363 if ("W3C-20150513".equals(codeString)) 3364 return W3C_20150513; 3365 if ("w3m".equals(codeString)) 3366 return W3M; 3367 if ("Watcom-1.0".equals(codeString)) 3368 return WATCOM_1_0; 3369 if ("Widget-Workshop".equals(codeString)) 3370 return WIDGET_WORKSHOP; 3371 if ("Wsuipa".equals(codeString)) 3372 return WSUIPA; 3373 if ("WTFPL".equals(codeString)) 3374 return WTFPL; 3375 if ("wxWindows".equals(codeString)) 3376 return WXWINDOWS; 3377 if ("X11".equals(codeString)) 3378 return X11; 3379 if ("X11-distribute-modifications-variant".equals(codeString)) 3380 return X11_DISTRIBUTE_MODIFICATIONS_VARIANT; 3381 if ("Xdebug-1.03".equals(codeString)) 3382 return XDEBUG_1_03; 3383 if ("Xerox".equals(codeString)) 3384 return XEROX; 3385 if ("Xfig".equals(codeString)) 3386 return XFIG; 3387 if ("XFree86-1.1".equals(codeString)) 3388 return XFREE86_1_1; 3389 if ("xinetd".equals(codeString)) 3390 return XINETD; 3391 if ("xlock".equals(codeString)) 3392 return XLOCK; 3393 if ("Xnet".equals(codeString)) 3394 return XNET; 3395 if ("xpp".equals(codeString)) 3396 return XPP; 3397 if ("XSkat".equals(codeString)) 3398 return XSKAT; 3399 if ("YPL-1.0".equals(codeString)) 3400 return YPL_1_0; 3401 if ("YPL-1.1".equals(codeString)) 3402 return YPL_1_1; 3403 if ("Zed".equals(codeString)) 3404 return ZED; 3405 if ("Zend-2.0".equals(codeString)) 3406 return ZEND_2_0; 3407 if ("Zimbra-1.3".equals(codeString)) 3408 return ZIMBRA_1_3; 3409 if ("Zimbra-1.4".equals(codeString)) 3410 return ZIMBRA_1_4; 3411 if ("Zlib".equals(codeString)) 3412 return ZLIB; 3413 if ("zlib-acknowledgement".equals(codeString)) 3414 return ZLIB_ACKNOWLEDGEMENT; 3415 if ("ZPL-1.1".equals(codeString)) 3416 return ZPL_1_1; 3417 if ("ZPL-2.0".equals(codeString)) 3418 return ZPL_2_0; 3419 if ("ZPL-2.1".equals(codeString)) 3420 return ZPL_2_1; 3421 throw new FHIRException("Unknown SPDXLicense code '" + codeString + "'"); 3422 } 3423 3424 public static boolean isValidCode(String codeString) { 3425 if (codeString == null || "".equals(codeString)) 3426 return false; 3427 return Utilities.existsInList(codeString, "0BSD", "AAL", "Abstyles", "AdaCore-doc", "Adobe-2006", "Adobe-Glyph", 3428 "ADSL", "AFL-1.1", "AFL-1.2", "AFL-2.0", "AFL-2.1", "AFL-3.0", "Afmparse", "AGPL-1.0", "AGPL-1.0-only", 3429 "AGPL-1.0-or-later", "AGPL-3.0", "AGPL-3.0-only", "AGPL-3.0-or-later", "Aladdin", "AMDPLPA", "AML", "AMPAS", 3430 "ANTLR-PD", "ANTLR-PD-fallback", "Apache-1.0", "Apache-1.1", "Apache-2.0", "APAFML", "APL-1.0", "App-s2p", 3431 "APSL-1.0", "APSL-1.1", "APSL-1.2", "APSL-2.0", "Arphic-1999", "Artistic-1.0", "Artistic-1.0-cl8", 3432 "Artistic-1.0-Perl", "Artistic-2.0", "ASWF-Digital-Assets-1.0", "ASWF-Digital-Assets-1.1", "Baekmuk", 3433 "Bahyph", "Barr", "Beerware", "Bitstream-Charter", "Bitstream-Vera", "BitTorrent-1.0", "BitTorrent-1.1", 3434 "blessing", "BlueOak-1.0.0", "Boehm-GC", "Borceux", "Brian-Gladman-3-Clause", "BSD-1-Clause", "BSD-2-Clause", 3435 "BSD-2-Clause-FreeBSD", "BSD-2-Clause-NetBSD", "BSD-2-Clause-Patent", "BSD-2-Clause-Views", "BSD-3-Clause", 3436 "BSD-3-Clause-Attribution", "BSD-3-Clause-Clear", "BSD-3-Clause-LBNL", "BSD-3-Clause-Modification", 3437 "BSD-3-Clause-No-Military-License", "BSD-3-Clause-No-Nuclear-License", "BSD-3-Clause-No-Nuclear-License-2014", 3438 "BSD-3-Clause-No-Nuclear-Warranty", "BSD-3-Clause-Open-MPI", "BSD-4-Clause", "BSD-4-Clause-Shortened", 3439 "BSD-4-Clause-UC", "BSD-4.3RENO", "BSD-4.3TAHOE", "BSD-Advertising-Acknowledgement", 3440 "BSD-Attribution-HPND-disclaimer", "BSD-Protection", "BSD-Source-Code", "BSL-1.0", "BUSL-1.1", "bzip2-1.0.5", 3441 "bzip2-1.0.6", "C-UDA-1.0", "CAL-1.0", "CAL-1.0-Combined-Work-Exception", "Caldera", "CATOSL-1.1", 3442 "CC-BY-1.0", "CC-BY-2.0", "CC-BY-2.5", "CC-BY-2.5-AU", "CC-BY-3.0", "CC-BY-3.0-AT", "CC-BY-3.0-DE", 3443 "CC-BY-3.0-IGO", "CC-BY-3.0-NL", "CC-BY-3.0-US", "CC-BY-4.0", "CC-BY-NC-1.0", "CC-BY-NC-2.0", "CC-BY-NC-2.5", 3444 "CC-BY-NC-3.0", "CC-BY-NC-3.0-DE", "CC-BY-NC-4.0", "CC-BY-NC-ND-1.0", "CC-BY-NC-ND-2.0", "CC-BY-NC-ND-2.5", 3445 "CC-BY-NC-ND-3.0", "CC-BY-NC-ND-3.0-DE", "CC-BY-NC-ND-3.0-IGO", "CC-BY-NC-ND-4.0", "CC-BY-NC-SA-1.0", 3446 "CC-BY-NC-SA-2.0", "CC-BY-NC-SA-2.0-DE", "CC-BY-NC-SA-2.0-FR", "CC-BY-NC-SA-2.0-UK", "CC-BY-NC-SA-2.5", 3447 "CC-BY-NC-SA-3.0", "CC-BY-NC-SA-3.0-DE", "CC-BY-NC-SA-3.0-IGO", "CC-BY-NC-SA-4.0", "CC-BY-ND-1.0", 3448 "CC-BY-ND-2.0", "CC-BY-ND-2.5", "CC-BY-ND-3.0", "CC-BY-ND-3.0-DE", "CC-BY-ND-4.0", "CC-BY-SA-1.0", 3449 "CC-BY-SA-2.0", "CC-BY-SA-2.0-UK", "CC-BY-SA-2.1-JP", "CC-BY-SA-2.5", "CC-BY-SA-3.0", "CC-BY-SA-3.0-AT", 3450 "CC-BY-SA-3.0-DE", "CC-BY-SA-3.0-IGO", "CC-BY-SA-4.0", "CC-PDDC", "CC0-1.0", "CDDL-1.0", "CDDL-1.1", 3451 "CDL-1.0", "CDLA-Permissive-1.0", "CDLA-Permissive-2.0", "CDLA-Sharing-1.0", "CECILL-1.0", "CECILL-1.1", 3452 "CECILL-2.0", "CECILL-2.1", "CECILL-B", "CECILL-C", "CERN-OHL-1.1", "CERN-OHL-1.2", "CERN-OHL-P-2.0", 3453 "CERN-OHL-S-2.0", "CERN-OHL-W-2.0", "CFITSIO", "checkmk", "ClArtistic", "Clips", "CMU-Mach", "CNRI-Jython", 3454 "CNRI-Python", "CNRI-Python-GPL-Compatible", "COIL-1.0", "Community-Spec-1.0", "Condor-1.1", 3455 "copyleft-next-0.3.0", "copyleft-next-0.3.1", "Cornell-Lossless-JPEG", "CPAL-1.0", "CPL-1.0", "CPOL-1.02", 3456 "Crossword", "CrystalStacker", "CUA-OPL-1.0", "Cube", "curl", "D-FSL-1.0", "diffmark", "DL-DE-BY-2.0", "DOC", 3457 "Dotseqn", "DRL-1.0", "DSDP", "dtoa", "dvipdfm", "ECL-1.0", "ECL-2.0", "eCos-2.0", "EFL-1.0", "EFL-2.0", 3458 "eGenix", "Elastic-2.0", "Entessa", "EPICS", "EPL-1.0", "EPL-2.0", "ErlPL-1.1", "etalab-2.0", "EUDatagrid", 3459 "EUPL-1.0", "EUPL-1.1", "EUPL-1.2", "Eurosym", "Fair", "FDK-AAC", "Frameworx-1.0", "FreeBSD-DOC", "FreeImage", 3460 "FSFAP", "FSFUL", "FSFULLR", "FSFULLRWD", "FTL", "GD", "GFDL-1.1", "GFDL-1.1-invariants-only", 3461 "GFDL-1.1-invariants-or-later", "GFDL-1.1-no-invariants-only", "GFDL-1.1-no-invariants-or-later", 3462 "GFDL-1.1-only", "GFDL-1.1-or-later", "GFDL-1.2", "GFDL-1.2-invariants-only", "GFDL-1.2-invariants-or-later", 3463 "GFDL-1.2-no-invariants-only", "GFDL-1.2-no-invariants-or-later", "GFDL-1.2-only", "GFDL-1.2-or-later", 3464 "GFDL-1.3", "GFDL-1.3-invariants-only", "GFDL-1.3-invariants-or-later", "GFDL-1.3-no-invariants-only", 3465 "GFDL-1.3-no-invariants-or-later", "GFDL-1.3-only", "GFDL-1.3-or-later", "Giftware", "GL2PS", "Glide", 3466 "Glulxe", "GLWTPL", "gnuplot", "GPL-1.0", "GPL-1.0+", "GPL-1.0-only", "GPL-1.0-or-later", "GPL-2.0", 3467 "GPL-2.0+", "GPL-2.0-only", "GPL-2.0-or-later", "GPL-2.0-with-autoconf-exception", 3468 "GPL-2.0-with-bison-exception", "GPL-2.0-with-classpath-exception", "GPL-2.0-with-font-exception", 3469 "GPL-2.0-with-GCC-exception", "GPL-3.0", "GPL-3.0+", "GPL-3.0-only", "GPL-3.0-or-later", 3470 "GPL-3.0-with-autoconf-exception", "GPL-3.0-with-GCC-exception", "Graphics-Gems", "gSOAP-1.3b", 3471 "HaskellReport", "Hippocratic-2.1", "HP-1986", "HPND", "HPND-export-US", "HPND-Markus-Kuhn", 3472 "HPND-sell-variant", "HPND-sell-variant-MIT-disclaimer", "HTMLTIDY", "IBM-pibs", "ICU", 3473 "IEC-Code-Components-EULA", "IJG", "IJG-short", "ImageMagick", "iMatix", "Imlib2", "Info-ZIP", 3474 "Inner-Net-2.0", "Intel", "Intel-ACPI", "Interbase-1.0", "IPA", "IPL-1.0", "ISC", "Jam", "JasPer-2.0", 3475 "JPL-image", "JPNIC", "JSON", "Kazlib", "Knuth-CTAN", "LAL-1.2", "LAL-1.3", "Latex2e", 3476 "Latex2e-translated-notice", "Leptonica", "LGPL-2.0", "LGPL-2.0+", "LGPL-2.0-only", "LGPL-2.0-or-later", 3477 "LGPL-2.1", "LGPL-2.1+", "LGPL-2.1-only", "LGPL-2.1-or-later", "LGPL-3.0", "LGPL-3.0+", "LGPL-3.0-only", 3478 "LGPL-3.0-or-later", "LGPLLR", "Libpng", "libpng-2.0", "libselinux-1.0", "libtiff", "libutil-David-Nugent", 3479 "LiLiQ-P-1.1", "LiLiQ-R-1.1", "LiLiQ-Rplus-1.1", "Linux-man-pages-1-para", "Linux-man-pages-copyleft", 3480 "Linux-man-pages-copyleft-2-para", "Linux-man-pages-copyleft-var", "Linux-OpenIB", "LOOP", "LPL-1.0", 3481 "LPL-1.02", "LPPL-1.0", "LPPL-1.1", "LPPL-1.2", "LPPL-1.3a", "LPPL-1.3c", "LZMA-SDK-9.11-to-9.20", 3482 "LZMA-SDK-9.22", "MakeIndex", "Martin-Birgmeier", "metamail", "Minpack", "MirOS", "MIT", "MIT-0", 3483 "MIT-advertising", "MIT-CMU", "MIT-enna", "MIT-feh", "MIT-Festival", "MIT-Modern-Variant", "MIT-open-group", 3484 "MIT-Wu", "MITNFA", "Motosoto", "mpi-permissive", "mpich2", "MPL-1.0", "MPL-1.1", "MPL-2.0", 3485 "MPL-2.0-no-copyleft-exception", "mplus", "MS-LPL", "MS-PL", "MS-RL", "MTLL", "MulanPSL-1.0", "MulanPSL-2.0", 3486 "Multics", "Mup", "NAIST-2003", "NASA-1.3", "Naumen", "NBPL-1.0", "NCGL-UK-2.0", "NCSA", "Net-SNMP", "NetCDF", 3487 "Newsletr", "NGPL", "NICTA-1.0", "NIST-PD", "NIST-PD-fallback", "NIST-Software", "NLOD-1.0", "NLOD-2.0", 3488 "NLPL", "Nokia", "NOSL", "not-open-source", "Noweb", "NPL-1.0", "NPL-1.1", "NPOSL-3.0", "NRL", "NTP", "NTP-0", 3489 "Nunit", "O-UDA-1.0", "OCCT-PL", "OCLC-2.0", "ODbL-1.0", "ODC-By-1.0", "OFFIS", "OFL-1.0", "OFL-1.0-no-RFN", 3490 "OFL-1.0-RFN", "OFL-1.1", "OFL-1.1-no-RFN", "OFL-1.1-RFN", "OGC-1.0", "OGDL-Taiwan-1.0", "OGL-Canada-2.0", 3491 "OGL-UK-1.0", "OGL-UK-2.0", "OGL-UK-3.0", "OGTSL", "OLDAP-1.1", "OLDAP-1.2", "OLDAP-1.3", "OLDAP-1.4", 3492 "OLDAP-2.0", "OLDAP-2.0.1", "OLDAP-2.1", "OLDAP-2.2", "OLDAP-2.2.1", "OLDAP-2.2.2", "OLDAP-2.3", "OLDAP-2.4", 3493 "OLDAP-2.5", "OLDAP-2.6", "OLDAP-2.7", "OLDAP-2.8", "OLFL-1.3", "OML", "OpenPBS-2.3", "OpenSSL", "OPL-1.0", 3494 "OPL-UK-3.0", "OPUBL-1.0", "OSET-PL-2.1", "OSL-1.0", "OSL-1.1", "OSL-2.0", "OSL-2.1", "OSL-3.0", 3495 "Parity-6.0.0", "Parity-7.0.0", "PDDL-1.0", "PHP-3.0", "PHP-3.01", "Plexus", "PolyForm-Noncommercial-1.0.0", 3496 "PolyForm-Small-Business-1.0.0", "PostgreSQL", "PSF-2.0", "psfrag", "psutils", "Python-2.0", "Python-2.0.1", 3497 "Qhull", "QPL-1.0", "QPL-1.0-INRIA-2004", "Rdisc", "RHeCos-1.1", "RPL-1.1", "RPL-1.5", "RPSL-1.0", "RSA-MD", 3498 "RSCPL", "Ruby", "SAX-PD", "Saxpath", "SCEA", "SchemeReport", "Sendmail", "Sendmail-8.23", "SGI-B-1.0", 3499 "SGI-B-1.1", "SGI-B-2.0", "SGP4", "SHL-0.5", "SHL-0.51", "SimPL-2.0", "SISSL", "SISSL-1.2", "Sleepycat", 3500 "SMLNJ", "SMPPL", "SNIA", "snprintf", "Spencer-86", "Spencer-94", "Spencer-99", "SPL-1.0", "SSH-OpenSSH", 3501 "SSH-short", "SSPL-1.0", "StandardML-NJ", "SugarCRM-1.1.3", "SunPro", "SWL", "Symlinks", "TAPR-OHL-1.0", 3502 "TCL", "TCP-wrappers", "TermReadKey", "TMate", "TORQUE-1.1", "TOSL", "TPDL", "TPL-1.0", "TTWL", 3503 "TU-Berlin-1.0", "TU-Berlin-2.0", "UCAR", "UCL-1.0", "Unicode-DFS-2015", "Unicode-DFS-2016", "Unicode-TOU", 3504 "UnixCrypt", "Unlicense", "UPL-1.0", "Vim", "VOSTROM", "VSL-1.0", "W3C", "W3C-19980720", "W3C-20150513", 3505 "w3m", "Watcom-1.0", "Widget-Workshop", "Wsuipa", "WTFPL", "wxWindows", "X11", 3506 "X11-distribute-modifications-variant", "Xdebug-1.03", "Xerox", "Xfig", "XFree86-1.1", "xinetd", "xlock", 3507 "Xnet", "xpp", "XSkat", "YPL-1.0", "YPL-1.1", "Zed", "Zend-2.0", "Zimbra-1.3", "Zimbra-1.4", "Zlib", 3508 "zlib-acknowledgement", "ZPL-1.1", "ZPL-2.0", "ZPL-2.1"); 3509 } 3510 3511 public String toCode() { 3512 switch (this) { 3513 case _0BSD: 3514 return "0BSD"; 3515 case AAL: 3516 return "AAL"; 3517 case ABSTYLES: 3518 return "Abstyles"; 3519 case ADACORE_DOC: 3520 return "AdaCore-doc"; 3521 case ADOBE_2006: 3522 return "Adobe-2006"; 3523 case ADOBE_GLYPH: 3524 return "Adobe-Glyph"; 3525 case ADSL: 3526 return "ADSL"; 3527 case AFL_1_1: 3528 return "AFL-1.1"; 3529 case AFL_1_2: 3530 return "AFL-1.2"; 3531 case AFL_2_0: 3532 return "AFL-2.0"; 3533 case AFL_2_1: 3534 return "AFL-2.1"; 3535 case AFL_3_0: 3536 return "AFL-3.0"; 3537 case AFMPARSE: 3538 return "Afmparse"; 3539 case AGPL_1_0: 3540 return "AGPL-1.0"; 3541 case AGPL_1_0_ONLY: 3542 return "AGPL-1.0-only"; 3543 case AGPL_1_0_OR_LATER: 3544 return "AGPL-1.0-or-later"; 3545 case AGPL_3_0: 3546 return "AGPL-3.0"; 3547 case AGPL_3_0_ONLY: 3548 return "AGPL-3.0-only"; 3549 case AGPL_3_0_OR_LATER: 3550 return "AGPL-3.0-or-later"; 3551 case ALADDIN: 3552 return "Aladdin"; 3553 case AMDPLPA: 3554 return "AMDPLPA"; 3555 case AML: 3556 return "AML"; 3557 case AMPAS: 3558 return "AMPAS"; 3559 case ANTLR_PD: 3560 return "ANTLR-PD"; 3561 case ANTLR_PD_FALLBACK: 3562 return "ANTLR-PD-fallback"; 3563 case APACHE_1_0: 3564 return "Apache-1.0"; 3565 case APACHE_1_1: 3566 return "Apache-1.1"; 3567 case APACHE_2_0: 3568 return "Apache-2.0"; 3569 case APAFML: 3570 return "APAFML"; 3571 case APL_1_0: 3572 return "APL-1.0"; 3573 case APP_S2P: 3574 return "App-s2p"; 3575 case APSL_1_0: 3576 return "APSL-1.0"; 3577 case APSL_1_1: 3578 return "APSL-1.1"; 3579 case APSL_1_2: 3580 return "APSL-1.2"; 3581 case APSL_2_0: 3582 return "APSL-2.0"; 3583 case ARPHIC_1999: 3584 return "Arphic-1999"; 3585 case ARTISTIC_1_0: 3586 return "Artistic-1.0"; 3587 case ARTISTIC_1_0_CL8: 3588 return "Artistic-1.0-cl8"; 3589 case ARTISTIC_1_0_PERL: 3590 return "Artistic-1.0-Perl"; 3591 case ARTISTIC_2_0: 3592 return "Artistic-2.0"; 3593 case ASWF_DIGITAL_ASSETS_1_0: 3594 return "ASWF-Digital-Assets-1.0"; 3595 case ASWF_DIGITAL_ASSETS_1_1: 3596 return "ASWF-Digital-Assets-1.1"; 3597 case BAEKMUK: 3598 return "Baekmuk"; 3599 case BAHYPH: 3600 return "Bahyph"; 3601 case BARR: 3602 return "Barr"; 3603 case BEERWARE: 3604 return "Beerware"; 3605 case BITSTREAM_CHARTER: 3606 return "Bitstream-Charter"; 3607 case BITSTREAM_VERA: 3608 return "Bitstream-Vera"; 3609 case BITTORRENT_1_0: 3610 return "BitTorrent-1.0"; 3611 case BITTORRENT_1_1: 3612 return "BitTorrent-1.1"; 3613 case BLESSING: 3614 return "blessing"; 3615 case BLUEOAK_1_0_0: 3616 return "BlueOak-1.0.0"; 3617 case BOEHM_GC: 3618 return "Boehm-GC"; 3619 case BORCEUX: 3620 return "Borceux"; 3621 case BRIAN_GLADMAN_3_CLAUSE: 3622 return "Brian-Gladman-3-Clause"; 3623 case BSD_1_CLAUSE: 3624 return "BSD-1-Clause"; 3625 case BSD_2_CLAUSE: 3626 return "BSD-2-Clause"; 3627 case BSD_2_CLAUSE_FREEBSD: 3628 return "BSD-2-Clause-FreeBSD"; 3629 case BSD_2_CLAUSE_NETBSD: 3630 return "BSD-2-Clause-NetBSD"; 3631 case BSD_2_CLAUSE_PATENT: 3632 return "BSD-2-Clause-Patent"; 3633 case BSD_2_CLAUSE_VIEWS: 3634 return "BSD-2-Clause-Views"; 3635 case BSD_3_CLAUSE: 3636 return "BSD-3-Clause"; 3637 case BSD_3_CLAUSE_ATTRIBUTION: 3638 return "BSD-3-Clause-Attribution"; 3639 case BSD_3_CLAUSE_CLEAR: 3640 return "BSD-3-Clause-Clear"; 3641 case BSD_3_CLAUSE_LBNL: 3642 return "BSD-3-Clause-LBNL"; 3643 case BSD_3_CLAUSE_MODIFICATION: 3644 return "BSD-3-Clause-Modification"; 3645 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: 3646 return "BSD-3-Clause-No-Military-License"; 3647 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: 3648 return "BSD-3-Clause-No-Nuclear-License"; 3649 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: 3650 return "BSD-3-Clause-No-Nuclear-License-2014"; 3651 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: 3652 return "BSD-3-Clause-No-Nuclear-Warranty"; 3653 case BSD_3_CLAUSE_OPEN_MPI: 3654 return "BSD-3-Clause-Open-MPI"; 3655 case BSD_4_CLAUSE: 3656 return "BSD-4-Clause"; 3657 case BSD_4_CLAUSE_SHORTENED: 3658 return "BSD-4-Clause-Shortened"; 3659 case BSD_4_CLAUSE_UC: 3660 return "BSD-4-Clause-UC"; 3661 case BSD_4_3RENO: 3662 return "BSD-4.3RENO"; 3663 case BSD_4_3TAHOE: 3664 return "BSD-4.3TAHOE"; 3665 case BSD_ADVERTISING_ACKNOWLEDGEMENT: 3666 return "BSD-Advertising-Acknowledgement"; 3667 case BSD_ATTRIBUTION_HPND_DISCLAIMER: 3668 return "BSD-Attribution-HPND-disclaimer"; 3669 case BSD_PROTECTION: 3670 return "BSD-Protection"; 3671 case BSD_SOURCE_CODE: 3672 return "BSD-Source-Code"; 3673 case BSL_1_0: 3674 return "BSL-1.0"; 3675 case BUSL_1_1: 3676 return "BUSL-1.1"; 3677 case BZIP2_1_0_5: 3678 return "bzip2-1.0.5"; 3679 case BZIP2_1_0_6: 3680 return "bzip2-1.0.6"; 3681 case C_UDA_1_0: 3682 return "C-UDA-1.0"; 3683 case CAL_1_0: 3684 return "CAL-1.0"; 3685 case CAL_1_0_COMBINED_WORK_EXCEPTION: 3686 return "CAL-1.0-Combined-Work-Exception"; 3687 case CALDERA: 3688 return "Caldera"; 3689 case CATOSL_1_1: 3690 return "CATOSL-1.1"; 3691 case CC_BY_1_0: 3692 return "CC-BY-1.0"; 3693 case CC_BY_2_0: 3694 return "CC-BY-2.0"; 3695 case CC_BY_2_5: 3696 return "CC-BY-2.5"; 3697 case CC_BY_2_5_AU: 3698 return "CC-BY-2.5-AU"; 3699 case CC_BY_3_0: 3700 return "CC-BY-3.0"; 3701 case CC_BY_3_0_AT: 3702 return "CC-BY-3.0-AT"; 3703 case CC_BY_3_0_DE: 3704 return "CC-BY-3.0-DE"; 3705 case CC_BY_3_0_IGO: 3706 return "CC-BY-3.0-IGO"; 3707 case CC_BY_3_0_NL: 3708 return "CC-BY-3.0-NL"; 3709 case CC_BY_3_0_US: 3710 return "CC-BY-3.0-US"; 3711 case CC_BY_4_0: 3712 return "CC-BY-4.0"; 3713 case CC_BY_NC_1_0: 3714 return "CC-BY-NC-1.0"; 3715 case CC_BY_NC_2_0: 3716 return "CC-BY-NC-2.0"; 3717 case CC_BY_NC_2_5: 3718 return "CC-BY-NC-2.5"; 3719 case CC_BY_NC_3_0: 3720 return "CC-BY-NC-3.0"; 3721 case CC_BY_NC_3_0_DE: 3722 return "CC-BY-NC-3.0-DE"; 3723 case CC_BY_NC_4_0: 3724 return "CC-BY-NC-4.0"; 3725 case CC_BY_NC_ND_1_0: 3726 return "CC-BY-NC-ND-1.0"; 3727 case CC_BY_NC_ND_2_0: 3728 return "CC-BY-NC-ND-2.0"; 3729 case CC_BY_NC_ND_2_5: 3730 return "CC-BY-NC-ND-2.5"; 3731 case CC_BY_NC_ND_3_0: 3732 return "CC-BY-NC-ND-3.0"; 3733 case CC_BY_NC_ND_3_0_DE: 3734 return "CC-BY-NC-ND-3.0-DE"; 3735 case CC_BY_NC_ND_3_0_IGO: 3736 return "CC-BY-NC-ND-3.0-IGO"; 3737 case CC_BY_NC_ND_4_0: 3738 return "CC-BY-NC-ND-4.0"; 3739 case CC_BY_NC_SA_1_0: 3740 return "CC-BY-NC-SA-1.0"; 3741 case CC_BY_NC_SA_2_0: 3742 return "CC-BY-NC-SA-2.0"; 3743 case CC_BY_NC_SA_2_0_DE: 3744 return "CC-BY-NC-SA-2.0-DE"; 3745 case CC_BY_NC_SA_2_0_FR: 3746 return "CC-BY-NC-SA-2.0-FR"; 3747 case CC_BY_NC_SA_2_0_UK: 3748 return "CC-BY-NC-SA-2.0-UK"; 3749 case CC_BY_NC_SA_2_5: 3750 return "CC-BY-NC-SA-2.5"; 3751 case CC_BY_NC_SA_3_0: 3752 return "CC-BY-NC-SA-3.0"; 3753 case CC_BY_NC_SA_3_0_DE: 3754 return "CC-BY-NC-SA-3.0-DE"; 3755 case CC_BY_NC_SA_3_0_IGO: 3756 return "CC-BY-NC-SA-3.0-IGO"; 3757 case CC_BY_NC_SA_4_0: 3758 return "CC-BY-NC-SA-4.0"; 3759 case CC_BY_ND_1_0: 3760 return "CC-BY-ND-1.0"; 3761 case CC_BY_ND_2_0: 3762 return "CC-BY-ND-2.0"; 3763 case CC_BY_ND_2_5: 3764 return "CC-BY-ND-2.5"; 3765 case CC_BY_ND_3_0: 3766 return "CC-BY-ND-3.0"; 3767 case CC_BY_ND_3_0_DE: 3768 return "CC-BY-ND-3.0-DE"; 3769 case CC_BY_ND_4_0: 3770 return "CC-BY-ND-4.0"; 3771 case CC_BY_SA_1_0: 3772 return "CC-BY-SA-1.0"; 3773 case CC_BY_SA_2_0: 3774 return "CC-BY-SA-2.0"; 3775 case CC_BY_SA_2_0_UK: 3776 return "CC-BY-SA-2.0-UK"; 3777 case CC_BY_SA_2_1_JP: 3778 return "CC-BY-SA-2.1-JP"; 3779 case CC_BY_SA_2_5: 3780 return "CC-BY-SA-2.5"; 3781 case CC_BY_SA_3_0: 3782 return "CC-BY-SA-3.0"; 3783 case CC_BY_SA_3_0_AT: 3784 return "CC-BY-SA-3.0-AT"; 3785 case CC_BY_SA_3_0_DE: 3786 return "CC-BY-SA-3.0-DE"; 3787 case CC_BY_SA_3_0_IGO: 3788 return "CC-BY-SA-3.0-IGO"; 3789 case CC_BY_SA_4_0: 3790 return "CC-BY-SA-4.0"; 3791 case CC_PDDC: 3792 return "CC-PDDC"; 3793 case CC0_1_0: 3794 return "CC0-1.0"; 3795 case CDDL_1_0: 3796 return "CDDL-1.0"; 3797 case CDDL_1_1: 3798 return "CDDL-1.1"; 3799 case CDL_1_0: 3800 return "CDL-1.0"; 3801 case CDLA_PERMISSIVE_1_0: 3802 return "CDLA-Permissive-1.0"; 3803 case CDLA_PERMISSIVE_2_0: 3804 return "CDLA-Permissive-2.0"; 3805 case CDLA_SHARING_1_0: 3806 return "CDLA-Sharing-1.0"; 3807 case CECILL_1_0: 3808 return "CECILL-1.0"; 3809 case CECILL_1_1: 3810 return "CECILL-1.1"; 3811 case CECILL_2_0: 3812 return "CECILL-2.0"; 3813 case CECILL_2_1: 3814 return "CECILL-2.1"; 3815 case CECILL_B: 3816 return "CECILL-B"; 3817 case CECILL_C: 3818 return "CECILL-C"; 3819 case CERN_OHL_1_1: 3820 return "CERN-OHL-1.1"; 3821 case CERN_OHL_1_2: 3822 return "CERN-OHL-1.2"; 3823 case CERN_OHL_P_2_0: 3824 return "CERN-OHL-P-2.0"; 3825 case CERN_OHL_S_2_0: 3826 return "CERN-OHL-S-2.0"; 3827 case CERN_OHL_W_2_0: 3828 return "CERN-OHL-W-2.0"; 3829 case CFITSIO: 3830 return "CFITSIO"; 3831 case CHECKMK: 3832 return "checkmk"; 3833 case CLARTISTIC: 3834 return "ClArtistic"; 3835 case CLIPS: 3836 return "Clips"; 3837 case CMU_MACH: 3838 return "CMU-Mach"; 3839 case CNRI_JYTHON: 3840 return "CNRI-Jython"; 3841 case CNRI_PYTHON: 3842 return "CNRI-Python"; 3843 case CNRI_PYTHON_GPL_COMPATIBLE: 3844 return "CNRI-Python-GPL-Compatible"; 3845 case COIL_1_0: 3846 return "COIL-1.0"; 3847 case COMMUNITY_SPEC_1_0: 3848 return "Community-Spec-1.0"; 3849 case CONDOR_1_1: 3850 return "Condor-1.1"; 3851 case COPYLEFT_NEXT_0_3_0: 3852 return "copyleft-next-0.3.0"; 3853 case COPYLEFT_NEXT_0_3_1: 3854 return "copyleft-next-0.3.1"; 3855 case CORNELL_LOSSLESS_JPEG: 3856 return "Cornell-Lossless-JPEG"; 3857 case CPAL_1_0: 3858 return "CPAL-1.0"; 3859 case CPL_1_0: 3860 return "CPL-1.0"; 3861 case CPOL_1_02: 3862 return "CPOL-1.02"; 3863 case CROSSWORD: 3864 return "Crossword"; 3865 case CRYSTALSTACKER: 3866 return "CrystalStacker"; 3867 case CUA_OPL_1_0: 3868 return "CUA-OPL-1.0"; 3869 case CUBE: 3870 return "Cube"; 3871 case CURL: 3872 return "curl"; 3873 case D_FSL_1_0: 3874 return "D-FSL-1.0"; 3875 case DIFFMARK: 3876 return "diffmark"; 3877 case DL_DE_BY_2_0: 3878 return "DL-DE-BY-2.0"; 3879 case DOC: 3880 return "DOC"; 3881 case DOTSEQN: 3882 return "Dotseqn"; 3883 case DRL_1_0: 3884 return "DRL-1.0"; 3885 case DSDP: 3886 return "DSDP"; 3887 case DTOA: 3888 return "dtoa"; 3889 case DVIPDFM: 3890 return "dvipdfm"; 3891 case ECL_1_0: 3892 return "ECL-1.0"; 3893 case ECL_2_0: 3894 return "ECL-2.0"; 3895 case ECOS_2_0: 3896 return "eCos-2.0"; 3897 case EFL_1_0: 3898 return "EFL-1.0"; 3899 case EFL_2_0: 3900 return "EFL-2.0"; 3901 case EGENIX: 3902 return "eGenix"; 3903 case ELASTIC_2_0: 3904 return "Elastic-2.0"; 3905 case ENTESSA: 3906 return "Entessa"; 3907 case EPICS: 3908 return "EPICS"; 3909 case EPL_1_0: 3910 return "EPL-1.0"; 3911 case EPL_2_0: 3912 return "EPL-2.0"; 3913 case ERLPL_1_1: 3914 return "ErlPL-1.1"; 3915 case ETALAB_2_0: 3916 return "etalab-2.0"; 3917 case EUDATAGRID: 3918 return "EUDatagrid"; 3919 case EUPL_1_0: 3920 return "EUPL-1.0"; 3921 case EUPL_1_1: 3922 return "EUPL-1.1"; 3923 case EUPL_1_2: 3924 return "EUPL-1.2"; 3925 case EUROSYM: 3926 return "Eurosym"; 3927 case FAIR: 3928 return "Fair"; 3929 case FDK_AAC: 3930 return "FDK-AAC"; 3931 case FRAMEWORX_1_0: 3932 return "Frameworx-1.0"; 3933 case FREEBSD_DOC: 3934 return "FreeBSD-DOC"; 3935 case FREEIMAGE: 3936 return "FreeImage"; 3937 case FSFAP: 3938 return "FSFAP"; 3939 case FSFUL: 3940 return "FSFUL"; 3941 case FSFULLR: 3942 return "FSFULLR"; 3943 case FSFULLRWD: 3944 return "FSFULLRWD"; 3945 case FTL: 3946 return "FTL"; 3947 case GD: 3948 return "GD"; 3949 case GFDL_1_1: 3950 return "GFDL-1.1"; 3951 case GFDL_1_1_INVARIANTS_ONLY: 3952 return "GFDL-1.1-invariants-only"; 3953 case GFDL_1_1_INVARIANTS_OR_LATER: 3954 return "GFDL-1.1-invariants-or-later"; 3955 case GFDL_1_1_NO_INVARIANTS_ONLY: 3956 return "GFDL-1.1-no-invariants-only"; 3957 case GFDL_1_1_NO_INVARIANTS_OR_LATER: 3958 return "GFDL-1.1-no-invariants-or-later"; 3959 case GFDL_1_1_ONLY: 3960 return "GFDL-1.1-only"; 3961 case GFDL_1_1_OR_LATER: 3962 return "GFDL-1.1-or-later"; 3963 case GFDL_1_2: 3964 return "GFDL-1.2"; 3965 case GFDL_1_2_INVARIANTS_ONLY: 3966 return "GFDL-1.2-invariants-only"; 3967 case GFDL_1_2_INVARIANTS_OR_LATER: 3968 return "GFDL-1.2-invariants-or-later"; 3969 case GFDL_1_2_NO_INVARIANTS_ONLY: 3970 return "GFDL-1.2-no-invariants-only"; 3971 case GFDL_1_2_NO_INVARIANTS_OR_LATER: 3972 return "GFDL-1.2-no-invariants-or-later"; 3973 case GFDL_1_2_ONLY: 3974 return "GFDL-1.2-only"; 3975 case GFDL_1_2_OR_LATER: 3976 return "GFDL-1.2-or-later"; 3977 case GFDL_1_3: 3978 return "GFDL-1.3"; 3979 case GFDL_1_3_INVARIANTS_ONLY: 3980 return "GFDL-1.3-invariants-only"; 3981 case GFDL_1_3_INVARIANTS_OR_LATER: 3982 return "GFDL-1.3-invariants-or-later"; 3983 case GFDL_1_3_NO_INVARIANTS_ONLY: 3984 return "GFDL-1.3-no-invariants-only"; 3985 case GFDL_1_3_NO_INVARIANTS_OR_LATER: 3986 return "GFDL-1.3-no-invariants-or-later"; 3987 case GFDL_1_3_ONLY: 3988 return "GFDL-1.3-only"; 3989 case GFDL_1_3_OR_LATER: 3990 return "GFDL-1.3-or-later"; 3991 case GIFTWARE: 3992 return "Giftware"; 3993 case GL2PS: 3994 return "GL2PS"; 3995 case GLIDE: 3996 return "Glide"; 3997 case GLULXE: 3998 return "Glulxe"; 3999 case GLWTPL: 4000 return "GLWTPL"; 4001 case GNUPLOT: 4002 return "gnuplot"; 4003 case GPL_1_0: 4004 return "GPL-1.0"; 4005 case GPL_1_0PLUS: 4006 return "GPL-1.0+"; 4007 case GPL_1_0_ONLY: 4008 return "GPL-1.0-only"; 4009 case GPL_1_0_OR_LATER: 4010 return "GPL-1.0-or-later"; 4011 case GPL_2_0: 4012 return "GPL-2.0"; 4013 case GPL_2_0PLUS: 4014 return "GPL-2.0+"; 4015 case GPL_2_0_ONLY: 4016 return "GPL-2.0-only"; 4017 case GPL_2_0_OR_LATER: 4018 return "GPL-2.0-or-later"; 4019 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: 4020 return "GPL-2.0-with-autoconf-exception"; 4021 case GPL_2_0_WITH_BISON_EXCEPTION: 4022 return "GPL-2.0-with-bison-exception"; 4023 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: 4024 return "GPL-2.0-with-classpath-exception"; 4025 case GPL_2_0_WITH_FONT_EXCEPTION: 4026 return "GPL-2.0-with-font-exception"; 4027 case GPL_2_0_WITH_GCC_EXCEPTION: 4028 return "GPL-2.0-with-GCC-exception"; 4029 case GPL_3_0: 4030 return "GPL-3.0"; 4031 case GPL_3_0PLUS: 4032 return "GPL-3.0+"; 4033 case GPL_3_0_ONLY: 4034 return "GPL-3.0-only"; 4035 case GPL_3_0_OR_LATER: 4036 return "GPL-3.0-or-later"; 4037 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: 4038 return "GPL-3.0-with-autoconf-exception"; 4039 case GPL_3_0_WITH_GCC_EXCEPTION: 4040 return "GPL-3.0-with-GCC-exception"; 4041 case GRAPHICS_GEMS: 4042 return "Graphics-Gems"; 4043 case GSOAP_1_3B: 4044 return "gSOAP-1.3b"; 4045 case HASKELLREPORT: 4046 return "HaskellReport"; 4047 case HIPPOCRATIC_2_1: 4048 return "Hippocratic-2.1"; 4049 case HP_1986: 4050 return "HP-1986"; 4051 case HPND: 4052 return "HPND"; 4053 case HPND_EXPORT_US: 4054 return "HPND-export-US"; 4055 case HPND_MARKUS_KUHN: 4056 return "HPND-Markus-Kuhn"; 4057 case HPND_SELL_VARIANT: 4058 return "HPND-sell-variant"; 4059 case HPND_SELL_VARIANT_MIT_DISCLAIMER: 4060 return "HPND-sell-variant-MIT-disclaimer"; 4061 case HTMLTIDY: 4062 return "HTMLTIDY"; 4063 case IBM_PIBS: 4064 return "IBM-pibs"; 4065 case ICU: 4066 return "ICU"; 4067 case IEC_CODE_COMPONENTS_EULA: 4068 return "IEC-Code-Components-EULA"; 4069 case IJG: 4070 return "IJG"; 4071 case IJG_SHORT: 4072 return "IJG-short"; 4073 case IMAGEMAGICK: 4074 return "ImageMagick"; 4075 case IMATIX: 4076 return "iMatix"; 4077 case IMLIB2: 4078 return "Imlib2"; 4079 case INFO_ZIP: 4080 return "Info-ZIP"; 4081 case INNER_NET_2_0: 4082 return "Inner-Net-2.0"; 4083 case INTEL: 4084 return "Intel"; 4085 case INTEL_ACPI: 4086 return "Intel-ACPI"; 4087 case INTERBASE_1_0: 4088 return "Interbase-1.0"; 4089 case IPA: 4090 return "IPA"; 4091 case IPL_1_0: 4092 return "IPL-1.0"; 4093 case ISC: 4094 return "ISC"; 4095 case JAM: 4096 return "Jam"; 4097 case JASPER_2_0: 4098 return "JasPer-2.0"; 4099 case JPL_IMAGE: 4100 return "JPL-image"; 4101 case JPNIC: 4102 return "JPNIC"; 4103 case JSON: 4104 return "JSON"; 4105 case KAZLIB: 4106 return "Kazlib"; 4107 case KNUTH_CTAN: 4108 return "Knuth-CTAN"; 4109 case LAL_1_2: 4110 return "LAL-1.2"; 4111 case LAL_1_3: 4112 return "LAL-1.3"; 4113 case LATEX2E: 4114 return "Latex2e"; 4115 case LATEX2E_TRANSLATED_NOTICE: 4116 return "Latex2e-translated-notice"; 4117 case LEPTONICA: 4118 return "Leptonica"; 4119 case LGPL_2_0: 4120 return "LGPL-2.0"; 4121 case LGPL_2_0PLUS: 4122 return "LGPL-2.0+"; 4123 case LGPL_2_0_ONLY: 4124 return "LGPL-2.0-only"; 4125 case LGPL_2_0_OR_LATER: 4126 return "LGPL-2.0-or-later"; 4127 case LGPL_2_1: 4128 return "LGPL-2.1"; 4129 case LGPL_2_1PLUS: 4130 return "LGPL-2.1+"; 4131 case LGPL_2_1_ONLY: 4132 return "LGPL-2.1-only"; 4133 case LGPL_2_1_OR_LATER: 4134 return "LGPL-2.1-or-later"; 4135 case LGPL_3_0: 4136 return "LGPL-3.0"; 4137 case LGPL_3_0PLUS: 4138 return "LGPL-3.0+"; 4139 case LGPL_3_0_ONLY: 4140 return "LGPL-3.0-only"; 4141 case LGPL_3_0_OR_LATER: 4142 return "LGPL-3.0-or-later"; 4143 case LGPLLR: 4144 return "LGPLLR"; 4145 case LIBPNG: 4146 return "Libpng"; 4147 case LIBPNG_2_0: 4148 return "libpng-2.0"; 4149 case LIBSELINUX_1_0: 4150 return "libselinux-1.0"; 4151 case LIBTIFF: 4152 return "libtiff"; 4153 case LIBUTIL_DAVID_NUGENT: 4154 return "libutil-David-Nugent"; 4155 case LILIQ_P_1_1: 4156 return "LiLiQ-P-1.1"; 4157 case LILIQ_R_1_1: 4158 return "LiLiQ-R-1.1"; 4159 case LILIQ_RPLUS_1_1: 4160 return "LiLiQ-Rplus-1.1"; 4161 case LINUX_MAN_PAGES_1_PARA: 4162 return "Linux-man-pages-1-para"; 4163 case LINUX_MAN_PAGES_COPYLEFT: 4164 return "Linux-man-pages-copyleft"; 4165 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: 4166 return "Linux-man-pages-copyleft-2-para"; 4167 case LINUX_MAN_PAGES_COPYLEFT_VAR: 4168 return "Linux-man-pages-copyleft-var"; 4169 case LINUX_OPENIB: 4170 return "Linux-OpenIB"; 4171 case LOOP: 4172 return "LOOP"; 4173 case LPL_1_0: 4174 return "LPL-1.0"; 4175 case LPL_1_02: 4176 return "LPL-1.02"; 4177 case LPPL_1_0: 4178 return "LPPL-1.0"; 4179 case LPPL_1_1: 4180 return "LPPL-1.1"; 4181 case LPPL_1_2: 4182 return "LPPL-1.2"; 4183 case LPPL_1_3A: 4184 return "LPPL-1.3a"; 4185 case LPPL_1_3C: 4186 return "LPPL-1.3c"; 4187 case LZMA_SDK_9_11_TO_9_20: 4188 return "LZMA-SDK-9.11-to-9.20"; 4189 case LZMA_SDK_9_22: 4190 return "LZMA-SDK-9.22"; 4191 case MAKEINDEX: 4192 return "MakeIndex"; 4193 case MARTIN_BIRGMEIER: 4194 return "Martin-Birgmeier"; 4195 case METAMAIL: 4196 return "metamail"; 4197 case MINPACK: 4198 return "Minpack"; 4199 case MIROS: 4200 return "MirOS"; 4201 case MIT: 4202 return "MIT"; 4203 case MIT_0: 4204 return "MIT-0"; 4205 case MIT_ADVERTISING: 4206 return "MIT-advertising"; 4207 case MIT_CMU: 4208 return "MIT-CMU"; 4209 case MIT_ENNA: 4210 return "MIT-enna"; 4211 case MIT_FEH: 4212 return "MIT-feh"; 4213 case MIT_FESTIVAL: 4214 return "MIT-Festival"; 4215 case MIT_MODERN_VARIANT: 4216 return "MIT-Modern-Variant"; 4217 case MIT_OPEN_GROUP: 4218 return "MIT-open-group"; 4219 case MIT_WU: 4220 return "MIT-Wu"; 4221 case MITNFA: 4222 return "MITNFA"; 4223 case MOTOSOTO: 4224 return "Motosoto"; 4225 case MPI_PERMISSIVE: 4226 return "mpi-permissive"; 4227 case MPICH2: 4228 return "mpich2"; 4229 case MPL_1_0: 4230 return "MPL-1.0"; 4231 case MPL_1_1: 4232 return "MPL-1.1"; 4233 case MPL_2_0: 4234 return "MPL-2.0"; 4235 case MPL_2_0_NO_COPYLEFT_EXCEPTION: 4236 return "MPL-2.0-no-copyleft-exception"; 4237 case MPLUS: 4238 return "mplus"; 4239 case MS_LPL: 4240 return "MS-LPL"; 4241 case MS_PL: 4242 return "MS-PL"; 4243 case MS_RL: 4244 return "MS-RL"; 4245 case MTLL: 4246 return "MTLL"; 4247 case MULANPSL_1_0: 4248 return "MulanPSL-1.0"; 4249 case MULANPSL_2_0: 4250 return "MulanPSL-2.0"; 4251 case MULTICS: 4252 return "Multics"; 4253 case MUP: 4254 return "Mup"; 4255 case NAIST_2003: 4256 return "NAIST-2003"; 4257 case NASA_1_3: 4258 return "NASA-1.3"; 4259 case NAUMEN: 4260 return "Naumen"; 4261 case NBPL_1_0: 4262 return "NBPL-1.0"; 4263 case NCGL_UK_2_0: 4264 return "NCGL-UK-2.0"; 4265 case NCSA: 4266 return "NCSA"; 4267 case NET_SNMP: 4268 return "Net-SNMP"; 4269 case NETCDF: 4270 return "NetCDF"; 4271 case NEWSLETR: 4272 return "Newsletr"; 4273 case NGPL: 4274 return "NGPL"; 4275 case NICTA_1_0: 4276 return "NICTA-1.0"; 4277 case NIST_PD: 4278 return "NIST-PD"; 4279 case NIST_PD_FALLBACK: 4280 return "NIST-PD-fallback"; 4281 case NIST_SOFTWARE: 4282 return "NIST-Software"; 4283 case NLOD_1_0: 4284 return "NLOD-1.0"; 4285 case NLOD_2_0: 4286 return "NLOD-2.0"; 4287 case NLPL: 4288 return "NLPL"; 4289 case NOKIA: 4290 return "Nokia"; 4291 case NOSL: 4292 return "NOSL"; 4293 case NOT_OPEN_SOURCE: 4294 return "not-open-source"; 4295 case NOWEB: 4296 return "Noweb"; 4297 case NPL_1_0: 4298 return "NPL-1.0"; 4299 case NPL_1_1: 4300 return "NPL-1.1"; 4301 case NPOSL_3_0: 4302 return "NPOSL-3.0"; 4303 case NRL: 4304 return "NRL"; 4305 case NTP: 4306 return "NTP"; 4307 case NTP_0: 4308 return "NTP-0"; 4309 case NUNIT: 4310 return "Nunit"; 4311 case O_UDA_1_0: 4312 return "O-UDA-1.0"; 4313 case OCCT_PL: 4314 return "OCCT-PL"; 4315 case OCLC_2_0: 4316 return "OCLC-2.0"; 4317 case ODBL_1_0: 4318 return "ODbL-1.0"; 4319 case ODC_BY_1_0: 4320 return "ODC-By-1.0"; 4321 case OFFIS: 4322 return "OFFIS"; 4323 case OFL_1_0: 4324 return "OFL-1.0"; 4325 case OFL_1_0_NO_RFN: 4326 return "OFL-1.0-no-RFN"; 4327 case OFL_1_0_RFN: 4328 return "OFL-1.0-RFN"; 4329 case OFL_1_1: 4330 return "OFL-1.1"; 4331 case OFL_1_1_NO_RFN: 4332 return "OFL-1.1-no-RFN"; 4333 case OFL_1_1_RFN: 4334 return "OFL-1.1-RFN"; 4335 case OGC_1_0: 4336 return "OGC-1.0"; 4337 case OGDL_TAIWAN_1_0: 4338 return "OGDL-Taiwan-1.0"; 4339 case OGL_CANADA_2_0: 4340 return "OGL-Canada-2.0"; 4341 case OGL_UK_1_0: 4342 return "OGL-UK-1.0"; 4343 case OGL_UK_2_0: 4344 return "OGL-UK-2.0"; 4345 case OGL_UK_3_0: 4346 return "OGL-UK-3.0"; 4347 case OGTSL: 4348 return "OGTSL"; 4349 case OLDAP_1_1: 4350 return "OLDAP-1.1"; 4351 case OLDAP_1_2: 4352 return "OLDAP-1.2"; 4353 case OLDAP_1_3: 4354 return "OLDAP-1.3"; 4355 case OLDAP_1_4: 4356 return "OLDAP-1.4"; 4357 case OLDAP_2_0: 4358 return "OLDAP-2.0"; 4359 case OLDAP_2_0_1: 4360 return "OLDAP-2.0.1"; 4361 case OLDAP_2_1: 4362 return "OLDAP-2.1"; 4363 case OLDAP_2_2: 4364 return "OLDAP-2.2"; 4365 case OLDAP_2_2_1: 4366 return "OLDAP-2.2.1"; 4367 case OLDAP_2_2_2: 4368 return "OLDAP-2.2.2"; 4369 case OLDAP_2_3: 4370 return "OLDAP-2.3"; 4371 case OLDAP_2_4: 4372 return "OLDAP-2.4"; 4373 case OLDAP_2_5: 4374 return "OLDAP-2.5"; 4375 case OLDAP_2_6: 4376 return "OLDAP-2.6"; 4377 case OLDAP_2_7: 4378 return "OLDAP-2.7"; 4379 case OLDAP_2_8: 4380 return "OLDAP-2.8"; 4381 case OLFL_1_3: 4382 return "OLFL-1.3"; 4383 case OML: 4384 return "OML"; 4385 case OPENPBS_2_3: 4386 return "OpenPBS-2.3"; 4387 case OPENSSL: 4388 return "OpenSSL"; 4389 case OPL_1_0: 4390 return "OPL-1.0"; 4391 case OPL_UK_3_0: 4392 return "OPL-UK-3.0"; 4393 case OPUBL_1_0: 4394 return "OPUBL-1.0"; 4395 case OSET_PL_2_1: 4396 return "OSET-PL-2.1"; 4397 case OSL_1_0: 4398 return "OSL-1.0"; 4399 case OSL_1_1: 4400 return "OSL-1.1"; 4401 case OSL_2_0: 4402 return "OSL-2.0"; 4403 case OSL_2_1: 4404 return "OSL-2.1"; 4405 case OSL_3_0: 4406 return "OSL-3.0"; 4407 case PARITY_6_0_0: 4408 return "Parity-6.0.0"; 4409 case PARITY_7_0_0: 4410 return "Parity-7.0.0"; 4411 case PDDL_1_0: 4412 return "PDDL-1.0"; 4413 case PHP_3_0: 4414 return "PHP-3.0"; 4415 case PHP_3_01: 4416 return "PHP-3.01"; 4417 case PLEXUS: 4418 return "Plexus"; 4419 case POLYFORM_NONCOMMERCIAL_1_0_0: 4420 return "PolyForm-Noncommercial-1.0.0"; 4421 case POLYFORM_SMALL_BUSINESS_1_0_0: 4422 return "PolyForm-Small-Business-1.0.0"; 4423 case POSTGRESQL: 4424 return "PostgreSQL"; 4425 case PSF_2_0: 4426 return "PSF-2.0"; 4427 case PSFRAG: 4428 return "psfrag"; 4429 case PSUTILS: 4430 return "psutils"; 4431 case PYTHON_2_0: 4432 return "Python-2.0"; 4433 case PYTHON_2_0_1: 4434 return "Python-2.0.1"; 4435 case QHULL: 4436 return "Qhull"; 4437 case QPL_1_0: 4438 return "QPL-1.0"; 4439 case QPL_1_0_INRIA_2004: 4440 return "QPL-1.0-INRIA-2004"; 4441 case RDISC: 4442 return "Rdisc"; 4443 case RHECOS_1_1: 4444 return "RHeCos-1.1"; 4445 case RPL_1_1: 4446 return "RPL-1.1"; 4447 case RPL_1_5: 4448 return "RPL-1.5"; 4449 case RPSL_1_0: 4450 return "RPSL-1.0"; 4451 case RSA_MD: 4452 return "RSA-MD"; 4453 case RSCPL: 4454 return "RSCPL"; 4455 case RUBY: 4456 return "Ruby"; 4457 case SAX_PD: 4458 return "SAX-PD"; 4459 case SAXPATH: 4460 return "Saxpath"; 4461 case SCEA: 4462 return "SCEA"; 4463 case SCHEMEREPORT: 4464 return "SchemeReport"; 4465 case SENDMAIL: 4466 return "Sendmail"; 4467 case SENDMAIL_8_23: 4468 return "Sendmail-8.23"; 4469 case SGI_B_1_0: 4470 return "SGI-B-1.0"; 4471 case SGI_B_1_1: 4472 return "SGI-B-1.1"; 4473 case SGI_B_2_0: 4474 return "SGI-B-2.0"; 4475 case SGP4: 4476 return "SGP4"; 4477 case SHL_0_5: 4478 return "SHL-0.5"; 4479 case SHL_0_51: 4480 return "SHL-0.51"; 4481 case SIMPL_2_0: 4482 return "SimPL-2.0"; 4483 case SISSL: 4484 return "SISSL"; 4485 case SISSL_1_2: 4486 return "SISSL-1.2"; 4487 case SLEEPYCAT: 4488 return "Sleepycat"; 4489 case SMLNJ: 4490 return "SMLNJ"; 4491 case SMPPL: 4492 return "SMPPL"; 4493 case SNIA: 4494 return "SNIA"; 4495 case SNPRINTF: 4496 return "snprintf"; 4497 case SPENCER_86: 4498 return "Spencer-86"; 4499 case SPENCER_94: 4500 return "Spencer-94"; 4501 case SPENCER_99: 4502 return "Spencer-99"; 4503 case SPL_1_0: 4504 return "SPL-1.0"; 4505 case SSH_OPENSSH: 4506 return "SSH-OpenSSH"; 4507 case SSH_SHORT: 4508 return "SSH-short"; 4509 case SSPL_1_0: 4510 return "SSPL-1.0"; 4511 case STANDARDML_NJ: 4512 return "StandardML-NJ"; 4513 case SUGARCRM_1_1_3: 4514 return "SugarCRM-1.1.3"; 4515 case SUNPRO: 4516 return "SunPro"; 4517 case SWL: 4518 return "SWL"; 4519 case SYMLINKS: 4520 return "Symlinks"; 4521 case TAPR_OHL_1_0: 4522 return "TAPR-OHL-1.0"; 4523 case TCL: 4524 return "TCL"; 4525 case TCP_WRAPPERS: 4526 return "TCP-wrappers"; 4527 case TERMREADKEY: 4528 return "TermReadKey"; 4529 case TMATE: 4530 return "TMate"; 4531 case TORQUE_1_1: 4532 return "TORQUE-1.1"; 4533 case TOSL: 4534 return "TOSL"; 4535 case TPDL: 4536 return "TPDL"; 4537 case TPL_1_0: 4538 return "TPL-1.0"; 4539 case TTWL: 4540 return "TTWL"; 4541 case TU_BERLIN_1_0: 4542 return "TU-Berlin-1.0"; 4543 case TU_BERLIN_2_0: 4544 return "TU-Berlin-2.0"; 4545 case UCAR: 4546 return "UCAR"; 4547 case UCL_1_0: 4548 return "UCL-1.0"; 4549 case UNICODE_DFS_2015: 4550 return "Unicode-DFS-2015"; 4551 case UNICODE_DFS_2016: 4552 return "Unicode-DFS-2016"; 4553 case UNICODE_TOU: 4554 return "Unicode-TOU"; 4555 case UNIXCRYPT: 4556 return "UnixCrypt"; 4557 case UNLICENSE: 4558 return "Unlicense"; 4559 case UPL_1_0: 4560 return "UPL-1.0"; 4561 case VIM: 4562 return "Vim"; 4563 case VOSTROM: 4564 return "VOSTROM"; 4565 case VSL_1_0: 4566 return "VSL-1.0"; 4567 case W3C: 4568 return "W3C"; 4569 case W3C_19980720: 4570 return "W3C-19980720"; 4571 case W3C_20150513: 4572 return "W3C-20150513"; 4573 case W3M: 4574 return "w3m"; 4575 case WATCOM_1_0: 4576 return "Watcom-1.0"; 4577 case WIDGET_WORKSHOP: 4578 return "Widget-Workshop"; 4579 case WSUIPA: 4580 return "Wsuipa"; 4581 case WTFPL: 4582 return "WTFPL"; 4583 case WXWINDOWS: 4584 return "wxWindows"; 4585 case X11: 4586 return "X11"; 4587 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: 4588 return "X11-distribute-modifications-variant"; 4589 case XDEBUG_1_03: 4590 return "Xdebug-1.03"; 4591 case XEROX: 4592 return "Xerox"; 4593 case XFIG: 4594 return "Xfig"; 4595 case XFREE86_1_1: 4596 return "XFree86-1.1"; 4597 case XINETD: 4598 return "xinetd"; 4599 case XLOCK: 4600 return "xlock"; 4601 case XNET: 4602 return "Xnet"; 4603 case XPP: 4604 return "xpp"; 4605 case XSKAT: 4606 return "XSkat"; 4607 case YPL_1_0: 4608 return "YPL-1.0"; 4609 case YPL_1_1: 4610 return "YPL-1.1"; 4611 case ZED: 4612 return "Zed"; 4613 case ZEND_2_0: 4614 return "Zend-2.0"; 4615 case ZIMBRA_1_3: 4616 return "Zimbra-1.3"; 4617 case ZIMBRA_1_4: 4618 return "Zimbra-1.4"; 4619 case ZLIB: 4620 return "Zlib"; 4621 case ZLIB_ACKNOWLEDGEMENT: 4622 return "zlib-acknowledgement"; 4623 case ZPL_1_1: 4624 return "ZPL-1.1"; 4625 case ZPL_2_0: 4626 return "ZPL-2.0"; 4627 case ZPL_2_1: 4628 return "ZPL-2.1"; 4629 case NULL: 4630 return null; 4631 default: 4632 return "?"; 4633 } 4634 } 4635 4636 public String getSystem() { 4637 switch (this) { 4638 case _0BSD: 4639 return "http://hl7.org/fhir/spdx-license"; 4640 case AAL: 4641 return "http://hl7.org/fhir/spdx-license"; 4642 case ABSTYLES: 4643 return "http://hl7.org/fhir/spdx-license"; 4644 case ADACORE_DOC: 4645 return "http://hl7.org/fhir/spdx-license"; 4646 case ADOBE_2006: 4647 return "http://hl7.org/fhir/spdx-license"; 4648 case ADOBE_GLYPH: 4649 return "http://hl7.org/fhir/spdx-license"; 4650 case ADSL: 4651 return "http://hl7.org/fhir/spdx-license"; 4652 case AFL_1_1: 4653 return "http://hl7.org/fhir/spdx-license"; 4654 case AFL_1_2: 4655 return "http://hl7.org/fhir/spdx-license"; 4656 case AFL_2_0: 4657 return "http://hl7.org/fhir/spdx-license"; 4658 case AFL_2_1: 4659 return "http://hl7.org/fhir/spdx-license"; 4660 case AFL_3_0: 4661 return "http://hl7.org/fhir/spdx-license"; 4662 case AFMPARSE: 4663 return "http://hl7.org/fhir/spdx-license"; 4664 case AGPL_1_0: 4665 return "http://hl7.org/fhir/spdx-license"; 4666 case AGPL_1_0_ONLY: 4667 return "http://hl7.org/fhir/spdx-license"; 4668 case AGPL_1_0_OR_LATER: 4669 return "http://hl7.org/fhir/spdx-license"; 4670 case AGPL_3_0: 4671 return "http://hl7.org/fhir/spdx-license"; 4672 case AGPL_3_0_ONLY: 4673 return "http://hl7.org/fhir/spdx-license"; 4674 case AGPL_3_0_OR_LATER: 4675 return "http://hl7.org/fhir/spdx-license"; 4676 case ALADDIN: 4677 return "http://hl7.org/fhir/spdx-license"; 4678 case AMDPLPA: 4679 return "http://hl7.org/fhir/spdx-license"; 4680 case AML: 4681 return "http://hl7.org/fhir/spdx-license"; 4682 case AMPAS: 4683 return "http://hl7.org/fhir/spdx-license"; 4684 case ANTLR_PD: 4685 return "http://hl7.org/fhir/spdx-license"; 4686 case ANTLR_PD_FALLBACK: 4687 return "http://hl7.org/fhir/spdx-license"; 4688 case APACHE_1_0: 4689 return "http://hl7.org/fhir/spdx-license"; 4690 case APACHE_1_1: 4691 return "http://hl7.org/fhir/spdx-license"; 4692 case APACHE_2_0: 4693 return "http://hl7.org/fhir/spdx-license"; 4694 case APAFML: 4695 return "http://hl7.org/fhir/spdx-license"; 4696 case APL_1_0: 4697 return "http://hl7.org/fhir/spdx-license"; 4698 case APP_S2P: 4699 return "http://hl7.org/fhir/spdx-license"; 4700 case APSL_1_0: 4701 return "http://hl7.org/fhir/spdx-license"; 4702 case APSL_1_1: 4703 return "http://hl7.org/fhir/spdx-license"; 4704 case APSL_1_2: 4705 return "http://hl7.org/fhir/spdx-license"; 4706 case APSL_2_0: 4707 return "http://hl7.org/fhir/spdx-license"; 4708 case ARPHIC_1999: 4709 return "http://hl7.org/fhir/spdx-license"; 4710 case ARTISTIC_1_0: 4711 return "http://hl7.org/fhir/spdx-license"; 4712 case ARTISTIC_1_0_CL8: 4713 return "http://hl7.org/fhir/spdx-license"; 4714 case ARTISTIC_1_0_PERL: 4715 return "http://hl7.org/fhir/spdx-license"; 4716 case ARTISTIC_2_0: 4717 return "http://hl7.org/fhir/spdx-license"; 4718 case ASWF_DIGITAL_ASSETS_1_0: 4719 return "http://hl7.org/fhir/spdx-license"; 4720 case ASWF_DIGITAL_ASSETS_1_1: 4721 return "http://hl7.org/fhir/spdx-license"; 4722 case BAEKMUK: 4723 return "http://hl7.org/fhir/spdx-license"; 4724 case BAHYPH: 4725 return "http://hl7.org/fhir/spdx-license"; 4726 case BARR: 4727 return "http://hl7.org/fhir/spdx-license"; 4728 case BEERWARE: 4729 return "http://hl7.org/fhir/spdx-license"; 4730 case BITSTREAM_CHARTER: 4731 return "http://hl7.org/fhir/spdx-license"; 4732 case BITSTREAM_VERA: 4733 return "http://hl7.org/fhir/spdx-license"; 4734 case BITTORRENT_1_0: 4735 return "http://hl7.org/fhir/spdx-license"; 4736 case BITTORRENT_1_1: 4737 return "http://hl7.org/fhir/spdx-license"; 4738 case BLESSING: 4739 return "http://hl7.org/fhir/spdx-license"; 4740 case BLUEOAK_1_0_0: 4741 return "http://hl7.org/fhir/spdx-license"; 4742 case BOEHM_GC: 4743 return "http://hl7.org/fhir/spdx-license"; 4744 case BORCEUX: 4745 return "http://hl7.org/fhir/spdx-license"; 4746 case BRIAN_GLADMAN_3_CLAUSE: 4747 return "http://hl7.org/fhir/spdx-license"; 4748 case BSD_1_CLAUSE: 4749 return "http://hl7.org/fhir/spdx-license"; 4750 case BSD_2_CLAUSE: 4751 return "http://hl7.org/fhir/spdx-license"; 4752 case BSD_2_CLAUSE_FREEBSD: 4753 return "http://hl7.org/fhir/spdx-license"; 4754 case BSD_2_CLAUSE_NETBSD: 4755 return "http://hl7.org/fhir/spdx-license"; 4756 case BSD_2_CLAUSE_PATENT: 4757 return "http://hl7.org/fhir/spdx-license"; 4758 case BSD_2_CLAUSE_VIEWS: 4759 return "http://hl7.org/fhir/spdx-license"; 4760 case BSD_3_CLAUSE: 4761 return "http://hl7.org/fhir/spdx-license"; 4762 case BSD_3_CLAUSE_ATTRIBUTION: 4763 return "http://hl7.org/fhir/spdx-license"; 4764 case BSD_3_CLAUSE_CLEAR: 4765 return "http://hl7.org/fhir/spdx-license"; 4766 case BSD_3_CLAUSE_LBNL: 4767 return "http://hl7.org/fhir/spdx-license"; 4768 case BSD_3_CLAUSE_MODIFICATION: 4769 return "http://hl7.org/fhir/spdx-license"; 4770 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: 4771 return "http://hl7.org/fhir/spdx-license"; 4772 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: 4773 return "http://hl7.org/fhir/spdx-license"; 4774 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: 4775 return "http://hl7.org/fhir/spdx-license"; 4776 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: 4777 return "http://hl7.org/fhir/spdx-license"; 4778 case BSD_3_CLAUSE_OPEN_MPI: 4779 return "http://hl7.org/fhir/spdx-license"; 4780 case BSD_4_CLAUSE: 4781 return "http://hl7.org/fhir/spdx-license"; 4782 case BSD_4_CLAUSE_SHORTENED: 4783 return "http://hl7.org/fhir/spdx-license"; 4784 case BSD_4_CLAUSE_UC: 4785 return "http://hl7.org/fhir/spdx-license"; 4786 case BSD_4_3RENO: 4787 return "http://hl7.org/fhir/spdx-license"; 4788 case BSD_4_3TAHOE: 4789 return "http://hl7.org/fhir/spdx-license"; 4790 case BSD_ADVERTISING_ACKNOWLEDGEMENT: 4791 return "http://hl7.org/fhir/spdx-license"; 4792 case BSD_ATTRIBUTION_HPND_DISCLAIMER: 4793 return "http://hl7.org/fhir/spdx-license"; 4794 case BSD_PROTECTION: 4795 return "http://hl7.org/fhir/spdx-license"; 4796 case BSD_SOURCE_CODE: 4797 return "http://hl7.org/fhir/spdx-license"; 4798 case BSL_1_0: 4799 return "http://hl7.org/fhir/spdx-license"; 4800 case BUSL_1_1: 4801 return "http://hl7.org/fhir/spdx-license"; 4802 case BZIP2_1_0_5: 4803 return "http://hl7.org/fhir/spdx-license"; 4804 case BZIP2_1_0_6: 4805 return "http://hl7.org/fhir/spdx-license"; 4806 case C_UDA_1_0: 4807 return "http://hl7.org/fhir/spdx-license"; 4808 case CAL_1_0: 4809 return "http://hl7.org/fhir/spdx-license"; 4810 case CAL_1_0_COMBINED_WORK_EXCEPTION: 4811 return "http://hl7.org/fhir/spdx-license"; 4812 case CALDERA: 4813 return "http://hl7.org/fhir/spdx-license"; 4814 case CATOSL_1_1: 4815 return "http://hl7.org/fhir/spdx-license"; 4816 case CC_BY_1_0: 4817 return "http://hl7.org/fhir/spdx-license"; 4818 case CC_BY_2_0: 4819 return "http://hl7.org/fhir/spdx-license"; 4820 case CC_BY_2_5: 4821 return "http://hl7.org/fhir/spdx-license"; 4822 case CC_BY_2_5_AU: 4823 return "http://hl7.org/fhir/spdx-license"; 4824 case CC_BY_3_0: 4825 return "http://hl7.org/fhir/spdx-license"; 4826 case CC_BY_3_0_AT: 4827 return "http://hl7.org/fhir/spdx-license"; 4828 case CC_BY_3_0_DE: 4829 return "http://hl7.org/fhir/spdx-license"; 4830 case CC_BY_3_0_IGO: 4831 return "http://hl7.org/fhir/spdx-license"; 4832 case CC_BY_3_0_NL: 4833 return "http://hl7.org/fhir/spdx-license"; 4834 case CC_BY_3_0_US: 4835 return "http://hl7.org/fhir/spdx-license"; 4836 case CC_BY_4_0: 4837 return "http://hl7.org/fhir/spdx-license"; 4838 case CC_BY_NC_1_0: 4839 return "http://hl7.org/fhir/spdx-license"; 4840 case CC_BY_NC_2_0: 4841 return "http://hl7.org/fhir/spdx-license"; 4842 case CC_BY_NC_2_5: 4843 return "http://hl7.org/fhir/spdx-license"; 4844 case CC_BY_NC_3_0: 4845 return "http://hl7.org/fhir/spdx-license"; 4846 case CC_BY_NC_3_0_DE: 4847 return "http://hl7.org/fhir/spdx-license"; 4848 case CC_BY_NC_4_0: 4849 return "http://hl7.org/fhir/spdx-license"; 4850 case CC_BY_NC_ND_1_0: 4851 return "http://hl7.org/fhir/spdx-license"; 4852 case CC_BY_NC_ND_2_0: 4853 return "http://hl7.org/fhir/spdx-license"; 4854 case CC_BY_NC_ND_2_5: 4855 return "http://hl7.org/fhir/spdx-license"; 4856 case CC_BY_NC_ND_3_0: 4857 return "http://hl7.org/fhir/spdx-license"; 4858 case CC_BY_NC_ND_3_0_DE: 4859 return "http://hl7.org/fhir/spdx-license"; 4860 case CC_BY_NC_ND_3_0_IGO: 4861 return "http://hl7.org/fhir/spdx-license"; 4862 case CC_BY_NC_ND_4_0: 4863 return "http://hl7.org/fhir/spdx-license"; 4864 case CC_BY_NC_SA_1_0: 4865 return "http://hl7.org/fhir/spdx-license"; 4866 case CC_BY_NC_SA_2_0: 4867 return "http://hl7.org/fhir/spdx-license"; 4868 case CC_BY_NC_SA_2_0_DE: 4869 return "http://hl7.org/fhir/spdx-license"; 4870 case CC_BY_NC_SA_2_0_FR: 4871 return "http://hl7.org/fhir/spdx-license"; 4872 case CC_BY_NC_SA_2_0_UK: 4873 return "http://hl7.org/fhir/spdx-license"; 4874 case CC_BY_NC_SA_2_5: 4875 return "http://hl7.org/fhir/spdx-license"; 4876 case CC_BY_NC_SA_3_0: 4877 return "http://hl7.org/fhir/spdx-license"; 4878 case CC_BY_NC_SA_3_0_DE: 4879 return "http://hl7.org/fhir/spdx-license"; 4880 case CC_BY_NC_SA_3_0_IGO: 4881 return "http://hl7.org/fhir/spdx-license"; 4882 case CC_BY_NC_SA_4_0: 4883 return "http://hl7.org/fhir/spdx-license"; 4884 case CC_BY_ND_1_0: 4885 return "http://hl7.org/fhir/spdx-license"; 4886 case CC_BY_ND_2_0: 4887 return "http://hl7.org/fhir/spdx-license"; 4888 case CC_BY_ND_2_5: 4889 return "http://hl7.org/fhir/spdx-license"; 4890 case CC_BY_ND_3_0: 4891 return "http://hl7.org/fhir/spdx-license"; 4892 case CC_BY_ND_3_0_DE: 4893 return "http://hl7.org/fhir/spdx-license"; 4894 case CC_BY_ND_4_0: 4895 return "http://hl7.org/fhir/spdx-license"; 4896 case CC_BY_SA_1_0: 4897 return "http://hl7.org/fhir/spdx-license"; 4898 case CC_BY_SA_2_0: 4899 return "http://hl7.org/fhir/spdx-license"; 4900 case CC_BY_SA_2_0_UK: 4901 return "http://hl7.org/fhir/spdx-license"; 4902 case CC_BY_SA_2_1_JP: 4903 return "http://hl7.org/fhir/spdx-license"; 4904 case CC_BY_SA_2_5: 4905 return "http://hl7.org/fhir/spdx-license"; 4906 case CC_BY_SA_3_0: 4907 return "http://hl7.org/fhir/spdx-license"; 4908 case CC_BY_SA_3_0_AT: 4909 return "http://hl7.org/fhir/spdx-license"; 4910 case CC_BY_SA_3_0_DE: 4911 return "http://hl7.org/fhir/spdx-license"; 4912 case CC_BY_SA_3_0_IGO: 4913 return "http://hl7.org/fhir/spdx-license"; 4914 case CC_BY_SA_4_0: 4915 return "http://hl7.org/fhir/spdx-license"; 4916 case CC_PDDC: 4917 return "http://hl7.org/fhir/spdx-license"; 4918 case CC0_1_0: 4919 return "http://hl7.org/fhir/spdx-license"; 4920 case CDDL_1_0: 4921 return "http://hl7.org/fhir/spdx-license"; 4922 case CDDL_1_1: 4923 return "http://hl7.org/fhir/spdx-license"; 4924 case CDL_1_0: 4925 return "http://hl7.org/fhir/spdx-license"; 4926 case CDLA_PERMISSIVE_1_0: 4927 return "http://hl7.org/fhir/spdx-license"; 4928 case CDLA_PERMISSIVE_2_0: 4929 return "http://hl7.org/fhir/spdx-license"; 4930 case CDLA_SHARING_1_0: 4931 return "http://hl7.org/fhir/spdx-license"; 4932 case CECILL_1_0: 4933 return "http://hl7.org/fhir/spdx-license"; 4934 case CECILL_1_1: 4935 return "http://hl7.org/fhir/spdx-license"; 4936 case CECILL_2_0: 4937 return "http://hl7.org/fhir/spdx-license"; 4938 case CECILL_2_1: 4939 return "http://hl7.org/fhir/spdx-license"; 4940 case CECILL_B: 4941 return "http://hl7.org/fhir/spdx-license"; 4942 case CECILL_C: 4943 return "http://hl7.org/fhir/spdx-license"; 4944 case CERN_OHL_1_1: 4945 return "http://hl7.org/fhir/spdx-license"; 4946 case CERN_OHL_1_2: 4947 return "http://hl7.org/fhir/spdx-license"; 4948 case CERN_OHL_P_2_0: 4949 return "http://hl7.org/fhir/spdx-license"; 4950 case CERN_OHL_S_2_0: 4951 return "http://hl7.org/fhir/spdx-license"; 4952 case CERN_OHL_W_2_0: 4953 return "http://hl7.org/fhir/spdx-license"; 4954 case CFITSIO: 4955 return "http://hl7.org/fhir/spdx-license"; 4956 case CHECKMK: 4957 return "http://hl7.org/fhir/spdx-license"; 4958 case CLARTISTIC: 4959 return "http://hl7.org/fhir/spdx-license"; 4960 case CLIPS: 4961 return "http://hl7.org/fhir/spdx-license"; 4962 case CMU_MACH: 4963 return "http://hl7.org/fhir/spdx-license"; 4964 case CNRI_JYTHON: 4965 return "http://hl7.org/fhir/spdx-license"; 4966 case CNRI_PYTHON: 4967 return "http://hl7.org/fhir/spdx-license"; 4968 case CNRI_PYTHON_GPL_COMPATIBLE: 4969 return "http://hl7.org/fhir/spdx-license"; 4970 case COIL_1_0: 4971 return "http://hl7.org/fhir/spdx-license"; 4972 case COMMUNITY_SPEC_1_0: 4973 return "http://hl7.org/fhir/spdx-license"; 4974 case CONDOR_1_1: 4975 return "http://hl7.org/fhir/spdx-license"; 4976 case COPYLEFT_NEXT_0_3_0: 4977 return "http://hl7.org/fhir/spdx-license"; 4978 case COPYLEFT_NEXT_0_3_1: 4979 return "http://hl7.org/fhir/spdx-license"; 4980 case CORNELL_LOSSLESS_JPEG: 4981 return "http://hl7.org/fhir/spdx-license"; 4982 case CPAL_1_0: 4983 return "http://hl7.org/fhir/spdx-license"; 4984 case CPL_1_0: 4985 return "http://hl7.org/fhir/spdx-license"; 4986 case CPOL_1_02: 4987 return "http://hl7.org/fhir/spdx-license"; 4988 case CROSSWORD: 4989 return "http://hl7.org/fhir/spdx-license"; 4990 case CRYSTALSTACKER: 4991 return "http://hl7.org/fhir/spdx-license"; 4992 case CUA_OPL_1_0: 4993 return "http://hl7.org/fhir/spdx-license"; 4994 case CUBE: 4995 return "http://hl7.org/fhir/spdx-license"; 4996 case CURL: 4997 return "http://hl7.org/fhir/spdx-license"; 4998 case D_FSL_1_0: 4999 return "http://hl7.org/fhir/spdx-license"; 5000 case DIFFMARK: 5001 return "http://hl7.org/fhir/spdx-license"; 5002 case DL_DE_BY_2_0: 5003 return "http://hl7.org/fhir/spdx-license"; 5004 case DOC: 5005 return "http://hl7.org/fhir/spdx-license"; 5006 case DOTSEQN: 5007 return "http://hl7.org/fhir/spdx-license"; 5008 case DRL_1_0: 5009 return "http://hl7.org/fhir/spdx-license"; 5010 case DSDP: 5011 return "http://hl7.org/fhir/spdx-license"; 5012 case DTOA: 5013 return "http://hl7.org/fhir/spdx-license"; 5014 case DVIPDFM: 5015 return "http://hl7.org/fhir/spdx-license"; 5016 case ECL_1_0: 5017 return "http://hl7.org/fhir/spdx-license"; 5018 case ECL_2_0: 5019 return "http://hl7.org/fhir/spdx-license"; 5020 case ECOS_2_0: 5021 return "http://hl7.org/fhir/spdx-license"; 5022 case EFL_1_0: 5023 return "http://hl7.org/fhir/spdx-license"; 5024 case EFL_2_0: 5025 return "http://hl7.org/fhir/spdx-license"; 5026 case EGENIX: 5027 return "http://hl7.org/fhir/spdx-license"; 5028 case ELASTIC_2_0: 5029 return "http://hl7.org/fhir/spdx-license"; 5030 case ENTESSA: 5031 return "http://hl7.org/fhir/spdx-license"; 5032 case EPICS: 5033 return "http://hl7.org/fhir/spdx-license"; 5034 case EPL_1_0: 5035 return "http://hl7.org/fhir/spdx-license"; 5036 case EPL_2_0: 5037 return "http://hl7.org/fhir/spdx-license"; 5038 case ERLPL_1_1: 5039 return "http://hl7.org/fhir/spdx-license"; 5040 case ETALAB_2_0: 5041 return "http://hl7.org/fhir/spdx-license"; 5042 case EUDATAGRID: 5043 return "http://hl7.org/fhir/spdx-license"; 5044 case EUPL_1_0: 5045 return "http://hl7.org/fhir/spdx-license"; 5046 case EUPL_1_1: 5047 return "http://hl7.org/fhir/spdx-license"; 5048 case EUPL_1_2: 5049 return "http://hl7.org/fhir/spdx-license"; 5050 case EUROSYM: 5051 return "http://hl7.org/fhir/spdx-license"; 5052 case FAIR: 5053 return "http://hl7.org/fhir/spdx-license"; 5054 case FDK_AAC: 5055 return "http://hl7.org/fhir/spdx-license"; 5056 case FRAMEWORX_1_0: 5057 return "http://hl7.org/fhir/spdx-license"; 5058 case FREEBSD_DOC: 5059 return "http://hl7.org/fhir/spdx-license"; 5060 case FREEIMAGE: 5061 return "http://hl7.org/fhir/spdx-license"; 5062 case FSFAP: 5063 return "http://hl7.org/fhir/spdx-license"; 5064 case FSFUL: 5065 return "http://hl7.org/fhir/spdx-license"; 5066 case FSFULLR: 5067 return "http://hl7.org/fhir/spdx-license"; 5068 case FSFULLRWD: 5069 return "http://hl7.org/fhir/spdx-license"; 5070 case FTL: 5071 return "http://hl7.org/fhir/spdx-license"; 5072 case GD: 5073 return "http://hl7.org/fhir/spdx-license"; 5074 case GFDL_1_1: 5075 return "http://hl7.org/fhir/spdx-license"; 5076 case GFDL_1_1_INVARIANTS_ONLY: 5077 return "http://hl7.org/fhir/spdx-license"; 5078 case GFDL_1_1_INVARIANTS_OR_LATER: 5079 return "http://hl7.org/fhir/spdx-license"; 5080 case GFDL_1_1_NO_INVARIANTS_ONLY: 5081 return "http://hl7.org/fhir/spdx-license"; 5082 case GFDL_1_1_NO_INVARIANTS_OR_LATER: 5083 return "http://hl7.org/fhir/spdx-license"; 5084 case GFDL_1_1_ONLY: 5085 return "http://hl7.org/fhir/spdx-license"; 5086 case GFDL_1_1_OR_LATER: 5087 return "http://hl7.org/fhir/spdx-license"; 5088 case GFDL_1_2: 5089 return "http://hl7.org/fhir/spdx-license"; 5090 case GFDL_1_2_INVARIANTS_ONLY: 5091 return "http://hl7.org/fhir/spdx-license"; 5092 case GFDL_1_2_INVARIANTS_OR_LATER: 5093 return "http://hl7.org/fhir/spdx-license"; 5094 case GFDL_1_2_NO_INVARIANTS_ONLY: 5095 return "http://hl7.org/fhir/spdx-license"; 5096 case GFDL_1_2_NO_INVARIANTS_OR_LATER: 5097 return "http://hl7.org/fhir/spdx-license"; 5098 case GFDL_1_2_ONLY: 5099 return "http://hl7.org/fhir/spdx-license"; 5100 case GFDL_1_2_OR_LATER: 5101 return "http://hl7.org/fhir/spdx-license"; 5102 case GFDL_1_3: 5103 return "http://hl7.org/fhir/spdx-license"; 5104 case GFDL_1_3_INVARIANTS_ONLY: 5105 return "http://hl7.org/fhir/spdx-license"; 5106 case GFDL_1_3_INVARIANTS_OR_LATER: 5107 return "http://hl7.org/fhir/spdx-license"; 5108 case GFDL_1_3_NO_INVARIANTS_ONLY: 5109 return "http://hl7.org/fhir/spdx-license"; 5110 case GFDL_1_3_NO_INVARIANTS_OR_LATER: 5111 return "http://hl7.org/fhir/spdx-license"; 5112 case GFDL_1_3_ONLY: 5113 return "http://hl7.org/fhir/spdx-license"; 5114 case GFDL_1_3_OR_LATER: 5115 return "http://hl7.org/fhir/spdx-license"; 5116 case GIFTWARE: 5117 return "http://hl7.org/fhir/spdx-license"; 5118 case GL2PS: 5119 return "http://hl7.org/fhir/spdx-license"; 5120 case GLIDE: 5121 return "http://hl7.org/fhir/spdx-license"; 5122 case GLULXE: 5123 return "http://hl7.org/fhir/spdx-license"; 5124 case GLWTPL: 5125 return "http://hl7.org/fhir/spdx-license"; 5126 case GNUPLOT: 5127 return "http://hl7.org/fhir/spdx-license"; 5128 case GPL_1_0: 5129 return "http://hl7.org/fhir/spdx-license"; 5130 case GPL_1_0PLUS: 5131 return "http://hl7.org/fhir/spdx-license"; 5132 case GPL_1_0_ONLY: 5133 return "http://hl7.org/fhir/spdx-license"; 5134 case GPL_1_0_OR_LATER: 5135 return "http://hl7.org/fhir/spdx-license"; 5136 case GPL_2_0: 5137 return "http://hl7.org/fhir/spdx-license"; 5138 case GPL_2_0PLUS: 5139 return "http://hl7.org/fhir/spdx-license"; 5140 case GPL_2_0_ONLY: 5141 return "http://hl7.org/fhir/spdx-license"; 5142 case GPL_2_0_OR_LATER: 5143 return "http://hl7.org/fhir/spdx-license"; 5144 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: 5145 return "http://hl7.org/fhir/spdx-license"; 5146 case GPL_2_0_WITH_BISON_EXCEPTION: 5147 return "http://hl7.org/fhir/spdx-license"; 5148 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: 5149 return "http://hl7.org/fhir/spdx-license"; 5150 case GPL_2_0_WITH_FONT_EXCEPTION: 5151 return "http://hl7.org/fhir/spdx-license"; 5152 case GPL_2_0_WITH_GCC_EXCEPTION: 5153 return "http://hl7.org/fhir/spdx-license"; 5154 case GPL_3_0: 5155 return "http://hl7.org/fhir/spdx-license"; 5156 case GPL_3_0PLUS: 5157 return "http://hl7.org/fhir/spdx-license"; 5158 case GPL_3_0_ONLY: 5159 return "http://hl7.org/fhir/spdx-license"; 5160 case GPL_3_0_OR_LATER: 5161 return "http://hl7.org/fhir/spdx-license"; 5162 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: 5163 return "http://hl7.org/fhir/spdx-license"; 5164 case GPL_3_0_WITH_GCC_EXCEPTION: 5165 return "http://hl7.org/fhir/spdx-license"; 5166 case GRAPHICS_GEMS: 5167 return "http://hl7.org/fhir/spdx-license"; 5168 case GSOAP_1_3B: 5169 return "http://hl7.org/fhir/spdx-license"; 5170 case HASKELLREPORT: 5171 return "http://hl7.org/fhir/spdx-license"; 5172 case HIPPOCRATIC_2_1: 5173 return "http://hl7.org/fhir/spdx-license"; 5174 case HP_1986: 5175 return "http://hl7.org/fhir/spdx-license"; 5176 case HPND: 5177 return "http://hl7.org/fhir/spdx-license"; 5178 case HPND_EXPORT_US: 5179 return "http://hl7.org/fhir/spdx-license"; 5180 case HPND_MARKUS_KUHN: 5181 return "http://hl7.org/fhir/spdx-license"; 5182 case HPND_SELL_VARIANT: 5183 return "http://hl7.org/fhir/spdx-license"; 5184 case HPND_SELL_VARIANT_MIT_DISCLAIMER: 5185 return "http://hl7.org/fhir/spdx-license"; 5186 case HTMLTIDY: 5187 return "http://hl7.org/fhir/spdx-license"; 5188 case IBM_PIBS: 5189 return "http://hl7.org/fhir/spdx-license"; 5190 case ICU: 5191 return "http://hl7.org/fhir/spdx-license"; 5192 case IEC_CODE_COMPONENTS_EULA: 5193 return "http://hl7.org/fhir/spdx-license"; 5194 case IJG: 5195 return "http://hl7.org/fhir/spdx-license"; 5196 case IJG_SHORT: 5197 return "http://hl7.org/fhir/spdx-license"; 5198 case IMAGEMAGICK: 5199 return "http://hl7.org/fhir/spdx-license"; 5200 case IMATIX: 5201 return "http://hl7.org/fhir/spdx-license"; 5202 case IMLIB2: 5203 return "http://hl7.org/fhir/spdx-license"; 5204 case INFO_ZIP: 5205 return "http://hl7.org/fhir/spdx-license"; 5206 case INNER_NET_2_0: 5207 return "http://hl7.org/fhir/spdx-license"; 5208 case INTEL: 5209 return "http://hl7.org/fhir/spdx-license"; 5210 case INTEL_ACPI: 5211 return "http://hl7.org/fhir/spdx-license"; 5212 case INTERBASE_1_0: 5213 return "http://hl7.org/fhir/spdx-license"; 5214 case IPA: 5215 return "http://hl7.org/fhir/spdx-license"; 5216 case IPL_1_0: 5217 return "http://hl7.org/fhir/spdx-license"; 5218 case ISC: 5219 return "http://hl7.org/fhir/spdx-license"; 5220 case JAM: 5221 return "http://hl7.org/fhir/spdx-license"; 5222 case JASPER_2_0: 5223 return "http://hl7.org/fhir/spdx-license"; 5224 case JPL_IMAGE: 5225 return "http://hl7.org/fhir/spdx-license"; 5226 case JPNIC: 5227 return "http://hl7.org/fhir/spdx-license"; 5228 case JSON: 5229 return "http://hl7.org/fhir/spdx-license"; 5230 case KAZLIB: 5231 return "http://hl7.org/fhir/spdx-license"; 5232 case KNUTH_CTAN: 5233 return "http://hl7.org/fhir/spdx-license"; 5234 case LAL_1_2: 5235 return "http://hl7.org/fhir/spdx-license"; 5236 case LAL_1_3: 5237 return "http://hl7.org/fhir/spdx-license"; 5238 case LATEX2E: 5239 return "http://hl7.org/fhir/spdx-license"; 5240 case LATEX2E_TRANSLATED_NOTICE: 5241 return "http://hl7.org/fhir/spdx-license"; 5242 case LEPTONICA: 5243 return "http://hl7.org/fhir/spdx-license"; 5244 case LGPL_2_0: 5245 return "http://hl7.org/fhir/spdx-license"; 5246 case LGPL_2_0PLUS: 5247 return "http://hl7.org/fhir/spdx-license"; 5248 case LGPL_2_0_ONLY: 5249 return "http://hl7.org/fhir/spdx-license"; 5250 case LGPL_2_0_OR_LATER: 5251 return "http://hl7.org/fhir/spdx-license"; 5252 case LGPL_2_1: 5253 return "http://hl7.org/fhir/spdx-license"; 5254 case LGPL_2_1PLUS: 5255 return "http://hl7.org/fhir/spdx-license"; 5256 case LGPL_2_1_ONLY: 5257 return "http://hl7.org/fhir/spdx-license"; 5258 case LGPL_2_1_OR_LATER: 5259 return "http://hl7.org/fhir/spdx-license"; 5260 case LGPL_3_0: 5261 return "http://hl7.org/fhir/spdx-license"; 5262 case LGPL_3_0PLUS: 5263 return "http://hl7.org/fhir/spdx-license"; 5264 case LGPL_3_0_ONLY: 5265 return "http://hl7.org/fhir/spdx-license"; 5266 case LGPL_3_0_OR_LATER: 5267 return "http://hl7.org/fhir/spdx-license"; 5268 case LGPLLR: 5269 return "http://hl7.org/fhir/spdx-license"; 5270 case LIBPNG: 5271 return "http://hl7.org/fhir/spdx-license"; 5272 case LIBPNG_2_0: 5273 return "http://hl7.org/fhir/spdx-license"; 5274 case LIBSELINUX_1_0: 5275 return "http://hl7.org/fhir/spdx-license"; 5276 case LIBTIFF: 5277 return "http://hl7.org/fhir/spdx-license"; 5278 case LIBUTIL_DAVID_NUGENT: 5279 return "http://hl7.org/fhir/spdx-license"; 5280 case LILIQ_P_1_1: 5281 return "http://hl7.org/fhir/spdx-license"; 5282 case LILIQ_R_1_1: 5283 return "http://hl7.org/fhir/spdx-license"; 5284 case LILIQ_RPLUS_1_1: 5285 return "http://hl7.org/fhir/spdx-license"; 5286 case LINUX_MAN_PAGES_1_PARA: 5287 return "http://hl7.org/fhir/spdx-license"; 5288 case LINUX_MAN_PAGES_COPYLEFT: 5289 return "http://hl7.org/fhir/spdx-license"; 5290 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: 5291 return "http://hl7.org/fhir/spdx-license"; 5292 case LINUX_MAN_PAGES_COPYLEFT_VAR: 5293 return "http://hl7.org/fhir/spdx-license"; 5294 case LINUX_OPENIB: 5295 return "http://hl7.org/fhir/spdx-license"; 5296 case LOOP: 5297 return "http://hl7.org/fhir/spdx-license"; 5298 case LPL_1_0: 5299 return "http://hl7.org/fhir/spdx-license"; 5300 case LPL_1_02: 5301 return "http://hl7.org/fhir/spdx-license"; 5302 case LPPL_1_0: 5303 return "http://hl7.org/fhir/spdx-license"; 5304 case LPPL_1_1: 5305 return "http://hl7.org/fhir/spdx-license"; 5306 case LPPL_1_2: 5307 return "http://hl7.org/fhir/spdx-license"; 5308 case LPPL_1_3A: 5309 return "http://hl7.org/fhir/spdx-license"; 5310 case LPPL_1_3C: 5311 return "http://hl7.org/fhir/spdx-license"; 5312 case LZMA_SDK_9_11_TO_9_20: 5313 return "http://hl7.org/fhir/spdx-license"; 5314 case LZMA_SDK_9_22: 5315 return "http://hl7.org/fhir/spdx-license"; 5316 case MAKEINDEX: 5317 return "http://hl7.org/fhir/spdx-license"; 5318 case MARTIN_BIRGMEIER: 5319 return "http://hl7.org/fhir/spdx-license"; 5320 case METAMAIL: 5321 return "http://hl7.org/fhir/spdx-license"; 5322 case MINPACK: 5323 return "http://hl7.org/fhir/spdx-license"; 5324 case MIROS: 5325 return "http://hl7.org/fhir/spdx-license"; 5326 case MIT: 5327 return "http://hl7.org/fhir/spdx-license"; 5328 case MIT_0: 5329 return "http://hl7.org/fhir/spdx-license"; 5330 case MIT_ADVERTISING: 5331 return "http://hl7.org/fhir/spdx-license"; 5332 case MIT_CMU: 5333 return "http://hl7.org/fhir/spdx-license"; 5334 case MIT_ENNA: 5335 return "http://hl7.org/fhir/spdx-license"; 5336 case MIT_FEH: 5337 return "http://hl7.org/fhir/spdx-license"; 5338 case MIT_FESTIVAL: 5339 return "http://hl7.org/fhir/spdx-license"; 5340 case MIT_MODERN_VARIANT: 5341 return "http://hl7.org/fhir/spdx-license"; 5342 case MIT_OPEN_GROUP: 5343 return "http://hl7.org/fhir/spdx-license"; 5344 case MIT_WU: 5345 return "http://hl7.org/fhir/spdx-license"; 5346 case MITNFA: 5347 return "http://hl7.org/fhir/spdx-license"; 5348 case MOTOSOTO: 5349 return "http://hl7.org/fhir/spdx-license"; 5350 case MPI_PERMISSIVE: 5351 return "http://hl7.org/fhir/spdx-license"; 5352 case MPICH2: 5353 return "http://hl7.org/fhir/spdx-license"; 5354 case MPL_1_0: 5355 return "http://hl7.org/fhir/spdx-license"; 5356 case MPL_1_1: 5357 return "http://hl7.org/fhir/spdx-license"; 5358 case MPL_2_0: 5359 return "http://hl7.org/fhir/spdx-license"; 5360 case MPL_2_0_NO_COPYLEFT_EXCEPTION: 5361 return "http://hl7.org/fhir/spdx-license"; 5362 case MPLUS: 5363 return "http://hl7.org/fhir/spdx-license"; 5364 case MS_LPL: 5365 return "http://hl7.org/fhir/spdx-license"; 5366 case MS_PL: 5367 return "http://hl7.org/fhir/spdx-license"; 5368 case MS_RL: 5369 return "http://hl7.org/fhir/spdx-license"; 5370 case MTLL: 5371 return "http://hl7.org/fhir/spdx-license"; 5372 case MULANPSL_1_0: 5373 return "http://hl7.org/fhir/spdx-license"; 5374 case MULANPSL_2_0: 5375 return "http://hl7.org/fhir/spdx-license"; 5376 case MULTICS: 5377 return "http://hl7.org/fhir/spdx-license"; 5378 case MUP: 5379 return "http://hl7.org/fhir/spdx-license"; 5380 case NAIST_2003: 5381 return "http://hl7.org/fhir/spdx-license"; 5382 case NASA_1_3: 5383 return "http://hl7.org/fhir/spdx-license"; 5384 case NAUMEN: 5385 return "http://hl7.org/fhir/spdx-license"; 5386 case NBPL_1_0: 5387 return "http://hl7.org/fhir/spdx-license"; 5388 case NCGL_UK_2_0: 5389 return "http://hl7.org/fhir/spdx-license"; 5390 case NCSA: 5391 return "http://hl7.org/fhir/spdx-license"; 5392 case NET_SNMP: 5393 return "http://hl7.org/fhir/spdx-license"; 5394 case NETCDF: 5395 return "http://hl7.org/fhir/spdx-license"; 5396 case NEWSLETR: 5397 return "http://hl7.org/fhir/spdx-license"; 5398 case NGPL: 5399 return "http://hl7.org/fhir/spdx-license"; 5400 case NICTA_1_0: 5401 return "http://hl7.org/fhir/spdx-license"; 5402 case NIST_PD: 5403 return "http://hl7.org/fhir/spdx-license"; 5404 case NIST_PD_FALLBACK: 5405 return "http://hl7.org/fhir/spdx-license"; 5406 case NIST_SOFTWARE: 5407 return "http://hl7.org/fhir/spdx-license"; 5408 case NLOD_1_0: 5409 return "http://hl7.org/fhir/spdx-license"; 5410 case NLOD_2_0: 5411 return "http://hl7.org/fhir/spdx-license"; 5412 case NLPL: 5413 return "http://hl7.org/fhir/spdx-license"; 5414 case NOKIA: 5415 return "http://hl7.org/fhir/spdx-license"; 5416 case NOSL: 5417 return "http://hl7.org/fhir/spdx-license"; 5418 case NOT_OPEN_SOURCE: 5419 return "http://hl7.org/fhir/spdx-license"; 5420 case NOWEB: 5421 return "http://hl7.org/fhir/spdx-license"; 5422 case NPL_1_0: 5423 return "http://hl7.org/fhir/spdx-license"; 5424 case NPL_1_1: 5425 return "http://hl7.org/fhir/spdx-license"; 5426 case NPOSL_3_0: 5427 return "http://hl7.org/fhir/spdx-license"; 5428 case NRL: 5429 return "http://hl7.org/fhir/spdx-license"; 5430 case NTP: 5431 return "http://hl7.org/fhir/spdx-license"; 5432 case NTP_0: 5433 return "http://hl7.org/fhir/spdx-license"; 5434 case NUNIT: 5435 return "http://hl7.org/fhir/spdx-license"; 5436 case O_UDA_1_0: 5437 return "http://hl7.org/fhir/spdx-license"; 5438 case OCCT_PL: 5439 return "http://hl7.org/fhir/spdx-license"; 5440 case OCLC_2_0: 5441 return "http://hl7.org/fhir/spdx-license"; 5442 case ODBL_1_0: 5443 return "http://hl7.org/fhir/spdx-license"; 5444 case ODC_BY_1_0: 5445 return "http://hl7.org/fhir/spdx-license"; 5446 case OFFIS: 5447 return "http://hl7.org/fhir/spdx-license"; 5448 case OFL_1_0: 5449 return "http://hl7.org/fhir/spdx-license"; 5450 case OFL_1_0_NO_RFN: 5451 return "http://hl7.org/fhir/spdx-license"; 5452 case OFL_1_0_RFN: 5453 return "http://hl7.org/fhir/spdx-license"; 5454 case OFL_1_1: 5455 return "http://hl7.org/fhir/spdx-license"; 5456 case OFL_1_1_NO_RFN: 5457 return "http://hl7.org/fhir/spdx-license"; 5458 case OFL_1_1_RFN: 5459 return "http://hl7.org/fhir/spdx-license"; 5460 case OGC_1_0: 5461 return "http://hl7.org/fhir/spdx-license"; 5462 case OGDL_TAIWAN_1_0: 5463 return "http://hl7.org/fhir/spdx-license"; 5464 case OGL_CANADA_2_0: 5465 return "http://hl7.org/fhir/spdx-license"; 5466 case OGL_UK_1_0: 5467 return "http://hl7.org/fhir/spdx-license"; 5468 case OGL_UK_2_0: 5469 return "http://hl7.org/fhir/spdx-license"; 5470 case OGL_UK_3_0: 5471 return "http://hl7.org/fhir/spdx-license"; 5472 case OGTSL: 5473 return "http://hl7.org/fhir/spdx-license"; 5474 case OLDAP_1_1: 5475 return "http://hl7.org/fhir/spdx-license"; 5476 case OLDAP_1_2: 5477 return "http://hl7.org/fhir/spdx-license"; 5478 case OLDAP_1_3: 5479 return "http://hl7.org/fhir/spdx-license"; 5480 case OLDAP_1_4: 5481 return "http://hl7.org/fhir/spdx-license"; 5482 case OLDAP_2_0: 5483 return "http://hl7.org/fhir/spdx-license"; 5484 case OLDAP_2_0_1: 5485 return "http://hl7.org/fhir/spdx-license"; 5486 case OLDAP_2_1: 5487 return "http://hl7.org/fhir/spdx-license"; 5488 case OLDAP_2_2: 5489 return "http://hl7.org/fhir/spdx-license"; 5490 case OLDAP_2_2_1: 5491 return "http://hl7.org/fhir/spdx-license"; 5492 case OLDAP_2_2_2: 5493 return "http://hl7.org/fhir/spdx-license"; 5494 case OLDAP_2_3: 5495 return "http://hl7.org/fhir/spdx-license"; 5496 case OLDAP_2_4: 5497 return "http://hl7.org/fhir/spdx-license"; 5498 case OLDAP_2_5: 5499 return "http://hl7.org/fhir/spdx-license"; 5500 case OLDAP_2_6: 5501 return "http://hl7.org/fhir/spdx-license"; 5502 case OLDAP_2_7: 5503 return "http://hl7.org/fhir/spdx-license"; 5504 case OLDAP_2_8: 5505 return "http://hl7.org/fhir/spdx-license"; 5506 case OLFL_1_3: 5507 return "http://hl7.org/fhir/spdx-license"; 5508 case OML: 5509 return "http://hl7.org/fhir/spdx-license"; 5510 case OPENPBS_2_3: 5511 return "http://hl7.org/fhir/spdx-license"; 5512 case OPENSSL: 5513 return "http://hl7.org/fhir/spdx-license"; 5514 case OPL_1_0: 5515 return "http://hl7.org/fhir/spdx-license"; 5516 case OPL_UK_3_0: 5517 return "http://hl7.org/fhir/spdx-license"; 5518 case OPUBL_1_0: 5519 return "http://hl7.org/fhir/spdx-license"; 5520 case OSET_PL_2_1: 5521 return "http://hl7.org/fhir/spdx-license"; 5522 case OSL_1_0: 5523 return "http://hl7.org/fhir/spdx-license"; 5524 case OSL_1_1: 5525 return "http://hl7.org/fhir/spdx-license"; 5526 case OSL_2_0: 5527 return "http://hl7.org/fhir/spdx-license"; 5528 case OSL_2_1: 5529 return "http://hl7.org/fhir/spdx-license"; 5530 case OSL_3_0: 5531 return "http://hl7.org/fhir/spdx-license"; 5532 case PARITY_6_0_0: 5533 return "http://hl7.org/fhir/spdx-license"; 5534 case PARITY_7_0_0: 5535 return "http://hl7.org/fhir/spdx-license"; 5536 case PDDL_1_0: 5537 return "http://hl7.org/fhir/spdx-license"; 5538 case PHP_3_0: 5539 return "http://hl7.org/fhir/spdx-license"; 5540 case PHP_3_01: 5541 return "http://hl7.org/fhir/spdx-license"; 5542 case PLEXUS: 5543 return "http://hl7.org/fhir/spdx-license"; 5544 case POLYFORM_NONCOMMERCIAL_1_0_0: 5545 return "http://hl7.org/fhir/spdx-license"; 5546 case POLYFORM_SMALL_BUSINESS_1_0_0: 5547 return "http://hl7.org/fhir/spdx-license"; 5548 case POSTGRESQL: 5549 return "http://hl7.org/fhir/spdx-license"; 5550 case PSF_2_0: 5551 return "http://hl7.org/fhir/spdx-license"; 5552 case PSFRAG: 5553 return "http://hl7.org/fhir/spdx-license"; 5554 case PSUTILS: 5555 return "http://hl7.org/fhir/spdx-license"; 5556 case PYTHON_2_0: 5557 return "http://hl7.org/fhir/spdx-license"; 5558 case PYTHON_2_0_1: 5559 return "http://hl7.org/fhir/spdx-license"; 5560 case QHULL: 5561 return "http://hl7.org/fhir/spdx-license"; 5562 case QPL_1_0: 5563 return "http://hl7.org/fhir/spdx-license"; 5564 case QPL_1_0_INRIA_2004: 5565 return "http://hl7.org/fhir/spdx-license"; 5566 case RDISC: 5567 return "http://hl7.org/fhir/spdx-license"; 5568 case RHECOS_1_1: 5569 return "http://hl7.org/fhir/spdx-license"; 5570 case RPL_1_1: 5571 return "http://hl7.org/fhir/spdx-license"; 5572 case RPL_1_5: 5573 return "http://hl7.org/fhir/spdx-license"; 5574 case RPSL_1_0: 5575 return "http://hl7.org/fhir/spdx-license"; 5576 case RSA_MD: 5577 return "http://hl7.org/fhir/spdx-license"; 5578 case RSCPL: 5579 return "http://hl7.org/fhir/spdx-license"; 5580 case RUBY: 5581 return "http://hl7.org/fhir/spdx-license"; 5582 case SAX_PD: 5583 return "http://hl7.org/fhir/spdx-license"; 5584 case SAXPATH: 5585 return "http://hl7.org/fhir/spdx-license"; 5586 case SCEA: 5587 return "http://hl7.org/fhir/spdx-license"; 5588 case SCHEMEREPORT: 5589 return "http://hl7.org/fhir/spdx-license"; 5590 case SENDMAIL: 5591 return "http://hl7.org/fhir/spdx-license"; 5592 case SENDMAIL_8_23: 5593 return "http://hl7.org/fhir/spdx-license"; 5594 case SGI_B_1_0: 5595 return "http://hl7.org/fhir/spdx-license"; 5596 case SGI_B_1_1: 5597 return "http://hl7.org/fhir/spdx-license"; 5598 case SGI_B_2_0: 5599 return "http://hl7.org/fhir/spdx-license"; 5600 case SGP4: 5601 return "http://hl7.org/fhir/spdx-license"; 5602 case SHL_0_5: 5603 return "http://hl7.org/fhir/spdx-license"; 5604 case SHL_0_51: 5605 return "http://hl7.org/fhir/spdx-license"; 5606 case SIMPL_2_0: 5607 return "http://hl7.org/fhir/spdx-license"; 5608 case SISSL: 5609 return "http://hl7.org/fhir/spdx-license"; 5610 case SISSL_1_2: 5611 return "http://hl7.org/fhir/spdx-license"; 5612 case SLEEPYCAT: 5613 return "http://hl7.org/fhir/spdx-license"; 5614 case SMLNJ: 5615 return "http://hl7.org/fhir/spdx-license"; 5616 case SMPPL: 5617 return "http://hl7.org/fhir/spdx-license"; 5618 case SNIA: 5619 return "http://hl7.org/fhir/spdx-license"; 5620 case SNPRINTF: 5621 return "http://hl7.org/fhir/spdx-license"; 5622 case SPENCER_86: 5623 return "http://hl7.org/fhir/spdx-license"; 5624 case SPENCER_94: 5625 return "http://hl7.org/fhir/spdx-license"; 5626 case SPENCER_99: 5627 return "http://hl7.org/fhir/spdx-license"; 5628 case SPL_1_0: 5629 return "http://hl7.org/fhir/spdx-license"; 5630 case SSH_OPENSSH: 5631 return "http://hl7.org/fhir/spdx-license"; 5632 case SSH_SHORT: 5633 return "http://hl7.org/fhir/spdx-license"; 5634 case SSPL_1_0: 5635 return "http://hl7.org/fhir/spdx-license"; 5636 case STANDARDML_NJ: 5637 return "http://hl7.org/fhir/spdx-license"; 5638 case SUGARCRM_1_1_3: 5639 return "http://hl7.org/fhir/spdx-license"; 5640 case SUNPRO: 5641 return "http://hl7.org/fhir/spdx-license"; 5642 case SWL: 5643 return "http://hl7.org/fhir/spdx-license"; 5644 case SYMLINKS: 5645 return "http://hl7.org/fhir/spdx-license"; 5646 case TAPR_OHL_1_0: 5647 return "http://hl7.org/fhir/spdx-license"; 5648 case TCL: 5649 return "http://hl7.org/fhir/spdx-license"; 5650 case TCP_WRAPPERS: 5651 return "http://hl7.org/fhir/spdx-license"; 5652 case TERMREADKEY: 5653 return "http://hl7.org/fhir/spdx-license"; 5654 case TMATE: 5655 return "http://hl7.org/fhir/spdx-license"; 5656 case TORQUE_1_1: 5657 return "http://hl7.org/fhir/spdx-license"; 5658 case TOSL: 5659 return "http://hl7.org/fhir/spdx-license"; 5660 case TPDL: 5661 return "http://hl7.org/fhir/spdx-license"; 5662 case TPL_1_0: 5663 return "http://hl7.org/fhir/spdx-license"; 5664 case TTWL: 5665 return "http://hl7.org/fhir/spdx-license"; 5666 case TU_BERLIN_1_0: 5667 return "http://hl7.org/fhir/spdx-license"; 5668 case TU_BERLIN_2_0: 5669 return "http://hl7.org/fhir/spdx-license"; 5670 case UCAR: 5671 return "http://hl7.org/fhir/spdx-license"; 5672 case UCL_1_0: 5673 return "http://hl7.org/fhir/spdx-license"; 5674 case UNICODE_DFS_2015: 5675 return "http://hl7.org/fhir/spdx-license"; 5676 case UNICODE_DFS_2016: 5677 return "http://hl7.org/fhir/spdx-license"; 5678 case UNICODE_TOU: 5679 return "http://hl7.org/fhir/spdx-license"; 5680 case UNIXCRYPT: 5681 return "http://hl7.org/fhir/spdx-license"; 5682 case UNLICENSE: 5683 return "http://hl7.org/fhir/spdx-license"; 5684 case UPL_1_0: 5685 return "http://hl7.org/fhir/spdx-license"; 5686 case VIM: 5687 return "http://hl7.org/fhir/spdx-license"; 5688 case VOSTROM: 5689 return "http://hl7.org/fhir/spdx-license"; 5690 case VSL_1_0: 5691 return "http://hl7.org/fhir/spdx-license"; 5692 case W3C: 5693 return "http://hl7.org/fhir/spdx-license"; 5694 case W3C_19980720: 5695 return "http://hl7.org/fhir/spdx-license"; 5696 case W3C_20150513: 5697 return "http://hl7.org/fhir/spdx-license"; 5698 case W3M: 5699 return "http://hl7.org/fhir/spdx-license"; 5700 case WATCOM_1_0: 5701 return "http://hl7.org/fhir/spdx-license"; 5702 case WIDGET_WORKSHOP: 5703 return "http://hl7.org/fhir/spdx-license"; 5704 case WSUIPA: 5705 return "http://hl7.org/fhir/spdx-license"; 5706 case WTFPL: 5707 return "http://hl7.org/fhir/spdx-license"; 5708 case WXWINDOWS: 5709 return "http://hl7.org/fhir/spdx-license"; 5710 case X11: 5711 return "http://hl7.org/fhir/spdx-license"; 5712 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: 5713 return "http://hl7.org/fhir/spdx-license"; 5714 case XDEBUG_1_03: 5715 return "http://hl7.org/fhir/spdx-license"; 5716 case XEROX: 5717 return "http://hl7.org/fhir/spdx-license"; 5718 case XFIG: 5719 return "http://hl7.org/fhir/spdx-license"; 5720 case XFREE86_1_1: 5721 return "http://hl7.org/fhir/spdx-license"; 5722 case XINETD: 5723 return "http://hl7.org/fhir/spdx-license"; 5724 case XLOCK: 5725 return "http://hl7.org/fhir/spdx-license"; 5726 case XNET: 5727 return "http://hl7.org/fhir/spdx-license"; 5728 case XPP: 5729 return "http://hl7.org/fhir/spdx-license"; 5730 case XSKAT: 5731 return "http://hl7.org/fhir/spdx-license"; 5732 case YPL_1_0: 5733 return "http://hl7.org/fhir/spdx-license"; 5734 case YPL_1_1: 5735 return "http://hl7.org/fhir/spdx-license"; 5736 case ZED: 5737 return "http://hl7.org/fhir/spdx-license"; 5738 case ZEND_2_0: 5739 return "http://hl7.org/fhir/spdx-license"; 5740 case ZIMBRA_1_3: 5741 return "http://hl7.org/fhir/spdx-license"; 5742 case ZIMBRA_1_4: 5743 return "http://hl7.org/fhir/spdx-license"; 5744 case ZLIB: 5745 return "http://hl7.org/fhir/spdx-license"; 5746 case ZLIB_ACKNOWLEDGEMENT: 5747 return "http://hl7.org/fhir/spdx-license"; 5748 case ZPL_1_1: 5749 return "http://hl7.org/fhir/spdx-license"; 5750 case ZPL_2_0: 5751 return "http://hl7.org/fhir/spdx-license"; 5752 case ZPL_2_1: 5753 return "http://hl7.org/fhir/spdx-license"; 5754 case NULL: 5755 return null; 5756 default: 5757 return "?"; 5758 } 5759 } 5760 5761 public String getDefinition() { 5762 switch (this) { 5763 case _0BSD: 5764 return "BSD Zero Clause License"; 5765 case AAL: 5766 return "Attribution Assurance License"; 5767 case ABSTYLES: 5768 return "Abstyles License"; 5769 case ADACORE_DOC: 5770 return "AdaCore Doc License"; 5771 case ADOBE_2006: 5772 return "Adobe Systems Incorporated Source Code License Agreement"; 5773 case ADOBE_GLYPH: 5774 return "Adobe Glyph List License"; 5775 case ADSL: 5776 return "Amazon Digital Services License"; 5777 case AFL_1_1: 5778 return "Academic Free License v1.1"; 5779 case AFL_1_2: 5780 return "Academic Free License v1.2"; 5781 case AFL_2_0: 5782 return "Academic Free License v2.0"; 5783 case AFL_2_1: 5784 return "Academic Free License v2.1"; 5785 case AFL_3_0: 5786 return "Academic Free License v3.0"; 5787 case AFMPARSE: 5788 return "Afmparse License"; 5789 case AGPL_1_0: 5790 return "Affero General Public License v1.0"; 5791 case AGPL_1_0_ONLY: 5792 return "Affero General Public License v1.0 only"; 5793 case AGPL_1_0_OR_LATER: 5794 return "Affero General Public License v1.0 or later"; 5795 case AGPL_3_0: 5796 return "GNU Affero General Public License v3.0"; 5797 case AGPL_3_0_ONLY: 5798 return "GNU Affero General Public License v3.0 only"; 5799 case AGPL_3_0_OR_LATER: 5800 return "GNU Affero General Public License v3.0 or later"; 5801 case ALADDIN: 5802 return "Aladdin Free Public License"; 5803 case AMDPLPA: 5804 return "AMD's plpa_map.c License"; 5805 case AML: 5806 return "Apple MIT License"; 5807 case AMPAS: 5808 return "Academy of Motion Picture Arts and Sciences BSD"; 5809 case ANTLR_PD: 5810 return "ANTLR Software Rights Notice"; 5811 case ANTLR_PD_FALLBACK: 5812 return "ANTLR Software Rights Notice with license fallback"; 5813 case APACHE_1_0: 5814 return "Apache License 1.0"; 5815 case APACHE_1_1: 5816 return "Apache License 1.1"; 5817 case APACHE_2_0: 5818 return "Apache License 2.0"; 5819 case APAFML: 5820 return "Adobe Postscript AFM License"; 5821 case APL_1_0: 5822 return "Adaptive Public License 1.0"; 5823 case APP_S2P: 5824 return "App::s2p License"; 5825 case APSL_1_0: 5826 return "Apple Public Source License 1.0"; 5827 case APSL_1_1: 5828 return "Apple Public Source License 1.1"; 5829 case APSL_1_2: 5830 return "Apple Public Source License 1.2"; 5831 case APSL_2_0: 5832 return "Apple Public Source License 2.0"; 5833 case ARPHIC_1999: 5834 return "Arphic Public License"; 5835 case ARTISTIC_1_0: 5836 return "Artistic License 1.0"; 5837 case ARTISTIC_1_0_CL8: 5838 return "Artistic License 1.0 w/clause 8"; 5839 case ARTISTIC_1_0_PERL: 5840 return "Artistic License 1.0 (Perl)"; 5841 case ARTISTIC_2_0: 5842 return "Artistic License 2.0"; 5843 case ASWF_DIGITAL_ASSETS_1_0: 5844 return "ASWF Digital Assets License version 1.0"; 5845 case ASWF_DIGITAL_ASSETS_1_1: 5846 return "ASWF Digital Assets License 1.1"; 5847 case BAEKMUK: 5848 return "Baekmuk License"; 5849 case BAHYPH: 5850 return "Bahyph License"; 5851 case BARR: 5852 return "Barr License"; 5853 case BEERWARE: 5854 return "Beerware License"; 5855 case BITSTREAM_CHARTER: 5856 return "Bitstream Charter Font License"; 5857 case BITSTREAM_VERA: 5858 return "Bitstream Vera Font License"; 5859 case BITTORRENT_1_0: 5860 return "BitTorrent Open Source License v1.0"; 5861 case BITTORRENT_1_1: 5862 return "BitTorrent Open Source License v1.1"; 5863 case BLESSING: 5864 return "SQLite Blessing"; 5865 case BLUEOAK_1_0_0: 5866 return "Blue Oak Model License 1.0.0"; 5867 case BOEHM_GC: 5868 return "Boehm-Demers-Weiser GC License"; 5869 case BORCEUX: 5870 return "Borceux license"; 5871 case BRIAN_GLADMAN_3_CLAUSE: 5872 return "Brian Gladman 3-Clause License"; 5873 case BSD_1_CLAUSE: 5874 return "BSD 1-Clause License"; 5875 case BSD_2_CLAUSE: 5876 return "BSD 2-Clause \"Simplified\" License"; 5877 case BSD_2_CLAUSE_FREEBSD: 5878 return "BSD 2-Clause FreeBSD License"; 5879 case BSD_2_CLAUSE_NETBSD: 5880 return "BSD 2-Clause NetBSD License"; 5881 case BSD_2_CLAUSE_PATENT: 5882 return "BSD-2-Clause Plus Patent License"; 5883 case BSD_2_CLAUSE_VIEWS: 5884 return "BSD 2-Clause with views sentence"; 5885 case BSD_3_CLAUSE: 5886 return "BSD 3-Clause \"New\" or \"Revised\" License"; 5887 case BSD_3_CLAUSE_ATTRIBUTION: 5888 return "BSD with attribution"; 5889 case BSD_3_CLAUSE_CLEAR: 5890 return "BSD 3-Clause Clear License"; 5891 case BSD_3_CLAUSE_LBNL: 5892 return "Lawrence Berkeley National Labs BSD variant license"; 5893 case BSD_3_CLAUSE_MODIFICATION: 5894 return "BSD 3-Clause Modification"; 5895 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: 5896 return "BSD 3-Clause No Military License"; 5897 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: 5898 return "BSD 3-Clause No Nuclear License"; 5899 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: 5900 return "BSD 3-Clause No Nuclear License 2014"; 5901 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: 5902 return "BSD 3-Clause No Nuclear Warranty"; 5903 case BSD_3_CLAUSE_OPEN_MPI: 5904 return "BSD 3-Clause Open MPI variant"; 5905 case BSD_4_CLAUSE: 5906 return "BSD 4-Clause \"Original\" or \"Old\" License"; 5907 case BSD_4_CLAUSE_SHORTENED: 5908 return "BSD 4 Clause Shortened"; 5909 case BSD_4_CLAUSE_UC: 5910 return "BSD-4-Clause (University of California-Specific)"; 5911 case BSD_4_3RENO: 5912 return "BSD 4.3 RENO License"; 5913 case BSD_4_3TAHOE: 5914 return "BSD 4.3 TAHOE License"; 5915 case BSD_ADVERTISING_ACKNOWLEDGEMENT: 5916 return "BSD Advertising Acknowledgement License"; 5917 case BSD_ATTRIBUTION_HPND_DISCLAIMER: 5918 return "BSD with Attribution and HPND disclaimer"; 5919 case BSD_PROTECTION: 5920 return "BSD Protection License"; 5921 case BSD_SOURCE_CODE: 5922 return "BSD Source Code Attribution"; 5923 case BSL_1_0: 5924 return "Boost Software License 1.0"; 5925 case BUSL_1_1: 5926 return "Business Source License 1.1"; 5927 case BZIP2_1_0_5: 5928 return "bzip2 and libbzip2 License v1.0.5"; 5929 case BZIP2_1_0_6: 5930 return "bzip2 and libbzip2 License v1.0.6"; 5931 case C_UDA_1_0: 5932 return "Computational Use of Data Agreement v1.0"; 5933 case CAL_1_0: 5934 return "Cryptographic Autonomy License 1.0"; 5935 case CAL_1_0_COMBINED_WORK_EXCEPTION: 5936 return "Cryptographic Autonomy License 1.0 (Combined Work Exception)"; 5937 case CALDERA: 5938 return "Caldera License"; 5939 case CATOSL_1_1: 5940 return "Computer Associates Trusted Open Source License 1.1"; 5941 case CC_BY_1_0: 5942 return "Creative Commons Attribution 1.0 Generic"; 5943 case CC_BY_2_0: 5944 return "Creative Commons Attribution 2.0 Generic"; 5945 case CC_BY_2_5: 5946 return "Creative Commons Attribution 2.5 Generic"; 5947 case CC_BY_2_5_AU: 5948 return "Creative Commons Attribution 2.5 Australia"; 5949 case CC_BY_3_0: 5950 return "Creative Commons Attribution 3.0 Unported"; 5951 case CC_BY_3_0_AT: 5952 return "Creative Commons Attribution 3.0 Austria"; 5953 case CC_BY_3_0_DE: 5954 return "Creative Commons Attribution 3.0 Germany"; 5955 case CC_BY_3_0_IGO: 5956 return "Creative Commons Attribution 3.0 IGO"; 5957 case CC_BY_3_0_NL: 5958 return "Creative Commons Attribution 3.0 Netherlands"; 5959 case CC_BY_3_0_US: 5960 return "Creative Commons Attribution 3.0 United States"; 5961 case CC_BY_4_0: 5962 return "Creative Commons Attribution 4.0 International"; 5963 case CC_BY_NC_1_0: 5964 return "Creative Commons Attribution Non Commercial 1.0 Generic"; 5965 case CC_BY_NC_2_0: 5966 return "Creative Commons Attribution Non Commercial 2.0 Generic"; 5967 case CC_BY_NC_2_5: 5968 return "Creative Commons Attribution Non Commercial 2.5 Generic"; 5969 case CC_BY_NC_3_0: 5970 return "Creative Commons Attribution Non Commercial 3.0 Unported"; 5971 case CC_BY_NC_3_0_DE: 5972 return "Creative Commons Attribution Non Commercial 3.0 Germany"; 5973 case CC_BY_NC_4_0: 5974 return "Creative Commons Attribution Non Commercial 4.0 International"; 5975 case CC_BY_NC_ND_1_0: 5976 return "Creative Commons Attribution Non Commercial No Derivatives 1.0 Generic"; 5977 case CC_BY_NC_ND_2_0: 5978 return "Creative Commons Attribution Non Commercial No Derivatives 2.0 Generic"; 5979 case CC_BY_NC_ND_2_5: 5980 return "Creative Commons Attribution Non Commercial No Derivatives 2.5 Generic"; 5981 case CC_BY_NC_ND_3_0: 5982 return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Unported"; 5983 case CC_BY_NC_ND_3_0_DE: 5984 return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Germany"; 5985 case CC_BY_NC_ND_3_0_IGO: 5986 return "Creative Commons Attribution Non Commercial No Derivatives 3.0 IGO"; 5987 case CC_BY_NC_ND_4_0: 5988 return "Creative Commons Attribution Non Commercial No Derivatives 4.0 International"; 5989 case CC_BY_NC_SA_1_0: 5990 return "Creative Commons Attribution Non Commercial Share Alike 1.0 Generic"; 5991 case CC_BY_NC_SA_2_0: 5992 return "Creative Commons Attribution Non Commercial Share Alike 2.0 Generic"; 5993 case CC_BY_NC_SA_2_0_DE: 5994 return "Creative Commons Attribution Non Commercial Share Alike 2.0 Germany"; 5995 case CC_BY_NC_SA_2_0_FR: 5996 return "Creative Commons Attribution-NonCommercial-ShareAlike 2.0 France"; 5997 case CC_BY_NC_SA_2_0_UK: 5998 return "Creative Commons Attribution Non Commercial Share Alike 2.0 England and Wales"; 5999 case CC_BY_NC_SA_2_5: 6000 return "Creative Commons Attribution Non Commercial Share Alike 2.5 Generic"; 6001 case CC_BY_NC_SA_3_0: 6002 return "Creative Commons Attribution Non Commercial Share Alike 3.0 Unported"; 6003 case CC_BY_NC_SA_3_0_DE: 6004 return "Creative Commons Attribution Non Commercial Share Alike 3.0 Germany"; 6005 case CC_BY_NC_SA_3_0_IGO: 6006 return "Creative Commons Attribution Non Commercial Share Alike 3.0 IGO"; 6007 case CC_BY_NC_SA_4_0: 6008 return "Creative Commons Attribution Non Commercial Share Alike 4.0 International"; 6009 case CC_BY_ND_1_0: 6010 return "Creative Commons Attribution No Derivatives 1.0 Generic"; 6011 case CC_BY_ND_2_0: 6012 return "Creative Commons Attribution No Derivatives 2.0 Generic"; 6013 case CC_BY_ND_2_5: 6014 return "Creative Commons Attribution No Derivatives 2.5 Generic"; 6015 case CC_BY_ND_3_0: 6016 return "Creative Commons Attribution No Derivatives 3.0 Unported"; 6017 case CC_BY_ND_3_0_DE: 6018 return "Creative Commons Attribution No Derivatives 3.0 Germany"; 6019 case CC_BY_ND_4_0: 6020 return "Creative Commons Attribution No Derivatives 4.0 International"; 6021 case CC_BY_SA_1_0: 6022 return "Creative Commons Attribution Share Alike 1.0 Generic"; 6023 case CC_BY_SA_2_0: 6024 return "Creative Commons Attribution Share Alike 2.0 Generic"; 6025 case CC_BY_SA_2_0_UK: 6026 return "Creative Commons Attribution Share Alike 2.0 England and Wales"; 6027 case CC_BY_SA_2_1_JP: 6028 return "Creative Commons Attribution Share Alike 2.1 Japan"; 6029 case CC_BY_SA_2_5: 6030 return "Creative Commons Attribution Share Alike 2.5 Generic"; 6031 case CC_BY_SA_3_0: 6032 return "Creative Commons Attribution Share Alike 3.0 Unported"; 6033 case CC_BY_SA_3_0_AT: 6034 return "Creative Commons Attribution Share Alike 3.0 Austria"; 6035 case CC_BY_SA_3_0_DE: 6036 return "Creative Commons Attribution Share Alike 3.0 Germany"; 6037 case CC_BY_SA_3_0_IGO: 6038 return "Creative Commons Attribution-ShareAlike 3.0 IGO"; 6039 case CC_BY_SA_4_0: 6040 return "Creative Commons Attribution Share Alike 4.0 International"; 6041 case CC_PDDC: 6042 return "Creative Commons Public Domain Dedication and Certification"; 6043 case CC0_1_0: 6044 return "Creative Commons Zero v1.0 Universal"; 6045 case CDDL_1_0: 6046 return "Common Development and Distribution License 1.0"; 6047 case CDDL_1_1: 6048 return "Common Development and Distribution License 1.1"; 6049 case CDL_1_0: 6050 return "Common Documentation License 1.0"; 6051 case CDLA_PERMISSIVE_1_0: 6052 return "Community Data License Agreement Permissive 1.0"; 6053 case CDLA_PERMISSIVE_2_0: 6054 return "Community Data License Agreement Permissive 2.0"; 6055 case CDLA_SHARING_1_0: 6056 return "Community Data License Agreement Sharing 1.0"; 6057 case CECILL_1_0: 6058 return "CeCILL Free Software License Agreement v1.0"; 6059 case CECILL_1_1: 6060 return "CeCILL Free Software License Agreement v1.1"; 6061 case CECILL_2_0: 6062 return "CeCILL Free Software License Agreement v2.0"; 6063 case CECILL_2_1: 6064 return "CeCILL Free Software License Agreement v2.1"; 6065 case CECILL_B: 6066 return "CeCILL-B Free Software License Agreement"; 6067 case CECILL_C: 6068 return "CeCILL-C Free Software License Agreement"; 6069 case CERN_OHL_1_1: 6070 return "CERN Open Hardware Licence v1.1"; 6071 case CERN_OHL_1_2: 6072 return "CERN Open Hardware Licence v1.2"; 6073 case CERN_OHL_P_2_0: 6074 return "CERN Open Hardware Licence Version 2 - Permissive"; 6075 case CERN_OHL_S_2_0: 6076 return "CERN Open Hardware Licence Version 2 - Strongly Reciprocal"; 6077 case CERN_OHL_W_2_0: 6078 return "CERN Open Hardware Licence Version 2 - Weakly Reciprocal"; 6079 case CFITSIO: 6080 return "CFITSIO License"; 6081 case CHECKMK: 6082 return "Checkmk License"; 6083 case CLARTISTIC: 6084 return "Clarified Artistic License"; 6085 case CLIPS: 6086 return "Clips License"; 6087 case CMU_MACH: 6088 return "CMU Mach License"; 6089 case CNRI_JYTHON: 6090 return "CNRI Jython License"; 6091 case CNRI_PYTHON: 6092 return "CNRI Python License"; 6093 case CNRI_PYTHON_GPL_COMPATIBLE: 6094 return "CNRI Python Open Source GPL Compatible License Agreement"; 6095 case COIL_1_0: 6096 return "Copyfree Open Innovation License"; 6097 case COMMUNITY_SPEC_1_0: 6098 return "Community Specification License 1.0"; 6099 case CONDOR_1_1: 6100 return "Condor Public License v1.1"; 6101 case COPYLEFT_NEXT_0_3_0: 6102 return "copyleft-next 0.3.0"; 6103 case COPYLEFT_NEXT_0_3_1: 6104 return "copyleft-next 0.3.1"; 6105 case CORNELL_LOSSLESS_JPEG: 6106 return "Cornell Lossless JPEG License"; 6107 case CPAL_1_0: 6108 return "Common Public Attribution License 1.0"; 6109 case CPL_1_0: 6110 return "Common Public License 1.0"; 6111 case CPOL_1_02: 6112 return "Code Project Open License 1.02"; 6113 case CROSSWORD: 6114 return "Crossword License"; 6115 case CRYSTALSTACKER: 6116 return "CrystalStacker License"; 6117 case CUA_OPL_1_0: 6118 return "CUA Office Public License v1.0"; 6119 case CUBE: 6120 return "Cube License"; 6121 case CURL: 6122 return "curl License"; 6123 case D_FSL_1_0: 6124 return "Deutsche Freie Software Lizenz"; 6125 case DIFFMARK: 6126 return "diffmark license"; 6127 case DL_DE_BY_2_0: 6128 return "Data licence Germany ? attribution ? version 2.0"; 6129 case DOC: 6130 return "DOC License"; 6131 case DOTSEQN: 6132 return "Dotseqn License"; 6133 case DRL_1_0: 6134 return "Detection Rule License 1.0"; 6135 case DSDP: 6136 return "DSDP License"; 6137 case DTOA: 6138 return "David M. Gay dtoa License"; 6139 case DVIPDFM: 6140 return "dvipdfm License"; 6141 case ECL_1_0: 6142 return "Educational Community License v1.0"; 6143 case ECL_2_0: 6144 return "Educational Community License v2.0"; 6145 case ECOS_2_0: 6146 return "eCos license version 2.0"; 6147 case EFL_1_0: 6148 return "Eiffel Forum License v1.0"; 6149 case EFL_2_0: 6150 return "Eiffel Forum License v2.0"; 6151 case EGENIX: 6152 return "eGenix.com Public License 1.1.0"; 6153 case ELASTIC_2_0: 6154 return "Elastic License 2.0"; 6155 case ENTESSA: 6156 return "Entessa Public License v1.0"; 6157 case EPICS: 6158 return "EPICS Open License"; 6159 case EPL_1_0: 6160 return "Eclipse Public License 1.0"; 6161 case EPL_2_0: 6162 return "Eclipse Public License 2.0"; 6163 case ERLPL_1_1: 6164 return "Erlang Public License v1.1"; 6165 case ETALAB_2_0: 6166 return "Etalab Open License 2.0"; 6167 case EUDATAGRID: 6168 return "EU DataGrid Software License"; 6169 case EUPL_1_0: 6170 return "European Union Public License 1.0"; 6171 case EUPL_1_1: 6172 return "European Union Public License 1.1"; 6173 case EUPL_1_2: 6174 return "European Union Public License 1.2"; 6175 case EUROSYM: 6176 return "Eurosym License"; 6177 case FAIR: 6178 return "Fair License"; 6179 case FDK_AAC: 6180 return "Fraunhofer FDK AAC Codec Library"; 6181 case FRAMEWORX_1_0: 6182 return "Frameworx Open License 1.0"; 6183 case FREEBSD_DOC: 6184 return "FreeBSD Documentation License"; 6185 case FREEIMAGE: 6186 return "FreeImage Public License v1.0"; 6187 case FSFAP: 6188 return "FSF All Permissive License"; 6189 case FSFUL: 6190 return "FSF Unlimited License"; 6191 case FSFULLR: 6192 return "FSF Unlimited License (with License Retention)"; 6193 case FSFULLRWD: 6194 return "FSF Unlimited License (With License Retention and Warranty Disclaimer)"; 6195 case FTL: 6196 return "Freetype Project License"; 6197 case GD: 6198 return "GD License"; 6199 case GFDL_1_1: 6200 return "GNU Free Documentation License v1.1"; 6201 case GFDL_1_1_INVARIANTS_ONLY: 6202 return "GNU Free Documentation License v1.1 only - invariants"; 6203 case GFDL_1_1_INVARIANTS_OR_LATER: 6204 return "GNU Free Documentation License v1.1 or later - invariants"; 6205 case GFDL_1_1_NO_INVARIANTS_ONLY: 6206 return "GNU Free Documentation License v1.1 only - no invariants"; 6207 case GFDL_1_1_NO_INVARIANTS_OR_LATER: 6208 return "GNU Free Documentation License v1.1 or later - no invariants"; 6209 case GFDL_1_1_ONLY: 6210 return "GNU Free Documentation License v1.1 only"; 6211 case GFDL_1_1_OR_LATER: 6212 return "GNU Free Documentation License v1.1 or later"; 6213 case GFDL_1_2: 6214 return "GNU Free Documentation License v1.2"; 6215 case GFDL_1_2_INVARIANTS_ONLY: 6216 return "GNU Free Documentation License v1.2 only - invariants"; 6217 case GFDL_1_2_INVARIANTS_OR_LATER: 6218 return "GNU Free Documentation License v1.2 or later - invariants"; 6219 case GFDL_1_2_NO_INVARIANTS_ONLY: 6220 return "GNU Free Documentation License v1.2 only - no invariants"; 6221 case GFDL_1_2_NO_INVARIANTS_OR_LATER: 6222 return "GNU Free Documentation License v1.2 or later - no invariants"; 6223 case GFDL_1_2_ONLY: 6224 return "GNU Free Documentation License v1.2 only"; 6225 case GFDL_1_2_OR_LATER: 6226 return "GNU Free Documentation License v1.2 or later"; 6227 case GFDL_1_3: 6228 return "GNU Free Documentation License v1.3"; 6229 case GFDL_1_3_INVARIANTS_ONLY: 6230 return "GNU Free Documentation License v1.3 only - invariants"; 6231 case GFDL_1_3_INVARIANTS_OR_LATER: 6232 return "GNU Free Documentation License v1.3 or later - invariants"; 6233 case GFDL_1_3_NO_INVARIANTS_ONLY: 6234 return "GNU Free Documentation License v1.3 only - no invariants"; 6235 case GFDL_1_3_NO_INVARIANTS_OR_LATER: 6236 return "GNU Free Documentation License v1.3 or later - no invariants"; 6237 case GFDL_1_3_ONLY: 6238 return "GNU Free Documentation License v1.3 only"; 6239 case GFDL_1_3_OR_LATER: 6240 return "GNU Free Documentation License v1.3 or later"; 6241 case GIFTWARE: 6242 return "Giftware License"; 6243 case GL2PS: 6244 return "GL2PS License"; 6245 case GLIDE: 6246 return "3dfx Glide License"; 6247 case GLULXE: 6248 return "Glulxe License"; 6249 case GLWTPL: 6250 return "Good Luck With That Public License"; 6251 case GNUPLOT: 6252 return "gnuplot License"; 6253 case GPL_1_0: 6254 return "GNU General Public License v1.0 only"; 6255 case GPL_1_0PLUS: 6256 return "GNU General Public License v1.0 or later"; 6257 case GPL_1_0_ONLY: 6258 return "GNU General Public License v1.0 only"; 6259 case GPL_1_0_OR_LATER: 6260 return "GNU General Public License v1.0 or later"; 6261 case GPL_2_0: 6262 return "GNU General Public License v2.0 only"; 6263 case GPL_2_0PLUS: 6264 return "GNU General Public License v2.0 or later"; 6265 case GPL_2_0_ONLY: 6266 return "GNU General Public License v2.0 only"; 6267 case GPL_2_0_OR_LATER: 6268 return "GNU General Public License v2.0 or later"; 6269 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: 6270 return "GNU General Public License v2.0 w/Autoconf exception"; 6271 case GPL_2_0_WITH_BISON_EXCEPTION: 6272 return "GNU General Public License v2.0 w/Bison exception"; 6273 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: 6274 return "GNU General Public License v2.0 w/Classpath exception"; 6275 case GPL_2_0_WITH_FONT_EXCEPTION: 6276 return "GNU General Public License v2.0 w/Font exception"; 6277 case GPL_2_0_WITH_GCC_EXCEPTION: 6278 return "GNU General Public License v2.0 w/GCC Runtime Library exception"; 6279 case GPL_3_0: 6280 return "GNU General Public License v3.0 only"; 6281 case GPL_3_0PLUS: 6282 return "GNU General Public License v3.0 or later"; 6283 case GPL_3_0_ONLY: 6284 return "GNU General Public License v3.0 only"; 6285 case GPL_3_0_OR_LATER: 6286 return "GNU General Public License v3.0 or later"; 6287 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: 6288 return "GNU General Public License v3.0 w/Autoconf exception"; 6289 case GPL_3_0_WITH_GCC_EXCEPTION: 6290 return "GNU General Public License v3.0 w/GCC Runtime Library exception"; 6291 case GRAPHICS_GEMS: 6292 return "Graphics Gems License"; 6293 case GSOAP_1_3B: 6294 return "gSOAP Public License v1.3b"; 6295 case HASKELLREPORT: 6296 return "Haskell Language Report License"; 6297 case HIPPOCRATIC_2_1: 6298 return "Hippocratic License 2.1"; 6299 case HP_1986: 6300 return "Hewlett-Packard 1986 License"; 6301 case HPND: 6302 return "Historical Permission Notice and Disclaimer"; 6303 case HPND_EXPORT_US: 6304 return "HPND with US Government export control warning"; 6305 case HPND_MARKUS_KUHN: 6306 return "Historical Permission Notice and Disclaimer - Markus Kuhn variant"; 6307 case HPND_SELL_VARIANT: 6308 return "Historical Permission Notice and Disclaimer - sell variant"; 6309 case HPND_SELL_VARIANT_MIT_DISCLAIMER: 6310 return "HPND sell variant with MIT disclaimer"; 6311 case HTMLTIDY: 6312 return "HTML Tidy License"; 6313 case IBM_PIBS: 6314 return "IBM PowerPC Initialization and Boot Software"; 6315 case ICU: 6316 return "ICU License"; 6317 case IEC_CODE_COMPONENTS_EULA: 6318 return "IEC Code Components End-user licence agreement"; 6319 case IJG: 6320 return "Independent JPEG Group License"; 6321 case IJG_SHORT: 6322 return "Independent JPEG Group License - short"; 6323 case IMAGEMAGICK: 6324 return "ImageMagick License"; 6325 case IMATIX: 6326 return "iMatix Standard Function Library Agreement"; 6327 case IMLIB2: 6328 return "Imlib2 License"; 6329 case INFO_ZIP: 6330 return "Info-ZIP License"; 6331 case INNER_NET_2_0: 6332 return "Inner Net License v2.0"; 6333 case INTEL: 6334 return "Intel Open Source License"; 6335 case INTEL_ACPI: 6336 return "Intel ACPI Software License Agreement"; 6337 case INTERBASE_1_0: 6338 return "Interbase Public License v1.0"; 6339 case IPA: 6340 return "IPA Font License"; 6341 case IPL_1_0: 6342 return "IBM Public License v1.0"; 6343 case ISC: 6344 return "ISC License"; 6345 case JAM: 6346 return "Jam License"; 6347 case JASPER_2_0: 6348 return "JasPer License"; 6349 case JPL_IMAGE: 6350 return "JPL Image Use Policy"; 6351 case JPNIC: 6352 return "Japan Network Information Center License"; 6353 case JSON: 6354 return "JSON License"; 6355 case KAZLIB: 6356 return "Kazlib License"; 6357 case KNUTH_CTAN: 6358 return "Knuth CTAN License"; 6359 case LAL_1_2: 6360 return "Licence Art Libre 1.2"; 6361 case LAL_1_3: 6362 return "Licence Art Libre 1.3"; 6363 case LATEX2E: 6364 return "Latex2e License"; 6365 case LATEX2E_TRANSLATED_NOTICE: 6366 return "Latex2e with translated notice permission"; 6367 case LEPTONICA: 6368 return "Leptonica License"; 6369 case LGPL_2_0: 6370 return "GNU Library General Public License v2 only"; 6371 case LGPL_2_0PLUS: 6372 return "GNU Library General Public License v2 or later"; 6373 case LGPL_2_0_ONLY: 6374 return "GNU Library General Public License v2 only"; 6375 case LGPL_2_0_OR_LATER: 6376 return "GNU Library General Public License v2 or later"; 6377 case LGPL_2_1: 6378 return "GNU Lesser General Public License v2.1 only"; 6379 case LGPL_2_1PLUS: 6380 return "GNU Lesser General Public License v2.1 or later"; 6381 case LGPL_2_1_ONLY: 6382 return "GNU Lesser General Public License v2.1 only"; 6383 case LGPL_2_1_OR_LATER: 6384 return "GNU Lesser General Public License v2.1 or later"; 6385 case LGPL_3_0: 6386 return "GNU Lesser General Public License v3.0 only"; 6387 case LGPL_3_0PLUS: 6388 return "GNU Lesser General Public License v3.0 or later"; 6389 case LGPL_3_0_ONLY: 6390 return "GNU Lesser General Public License v3.0 only"; 6391 case LGPL_3_0_OR_LATER: 6392 return "GNU Lesser General Public License v3.0 or later"; 6393 case LGPLLR: 6394 return "Lesser General Public License For Linguistic Resources"; 6395 case LIBPNG: 6396 return "libpng License"; 6397 case LIBPNG_2_0: 6398 return "PNG Reference Library version 2"; 6399 case LIBSELINUX_1_0: 6400 return "libselinux public domain notice"; 6401 case LIBTIFF: 6402 return "libtiff License"; 6403 case LIBUTIL_DAVID_NUGENT: 6404 return "libutil David Nugent License"; 6405 case LILIQ_P_1_1: 6406 return "Licence Libre du Québec ? Permissive version 1.1"; 6407 case LILIQ_R_1_1: 6408 return "Licence Libre du Québec ? Réciprocité version 1.1"; 6409 case LILIQ_RPLUS_1_1: 6410 return "Licence Libre du Québec ? Réciprocité forte version 1.1"; 6411 case LINUX_MAN_PAGES_1_PARA: 6412 return "Linux man-pages - 1 paragraph"; 6413 case LINUX_MAN_PAGES_COPYLEFT: 6414 return "Linux man-pages Copyleft"; 6415 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: 6416 return "Linux man-pages Copyleft - 2 paragraphs"; 6417 case LINUX_MAN_PAGES_COPYLEFT_VAR: 6418 return "Linux man-pages Copyleft Variant"; 6419 case LINUX_OPENIB: 6420 return "Linux Kernel Variant of OpenIB.org license"; 6421 case LOOP: 6422 return "Common Lisp LOOP License"; 6423 case LPL_1_0: 6424 return "Lucent Public License Version 1.0"; 6425 case LPL_1_02: 6426 return "Lucent Public License v1.02"; 6427 case LPPL_1_0: 6428 return "LaTeX Project Public License v1.0"; 6429 case LPPL_1_1: 6430 return "LaTeX Project Public License v1.1"; 6431 case LPPL_1_2: 6432 return "LaTeX Project Public License v1.2"; 6433 case LPPL_1_3A: 6434 return "LaTeX Project Public License v1.3a"; 6435 case LPPL_1_3C: 6436 return "LaTeX Project Public License v1.3c"; 6437 case LZMA_SDK_9_11_TO_9_20: 6438 return "LZMA SDK License (versions 9.11 to 9.20)"; 6439 case LZMA_SDK_9_22: 6440 return "LZMA SDK License (versions 9.22 and beyond)"; 6441 case MAKEINDEX: 6442 return "MakeIndex License"; 6443 case MARTIN_BIRGMEIER: 6444 return "Martin Birgmeier License"; 6445 case METAMAIL: 6446 return "metamail License"; 6447 case MINPACK: 6448 return "Minpack License"; 6449 case MIROS: 6450 return "The MirOS Licence"; 6451 case MIT: 6452 return "MIT License"; 6453 case MIT_0: 6454 return "MIT No Attribution"; 6455 case MIT_ADVERTISING: 6456 return "Enlightenment License (e16)"; 6457 case MIT_CMU: 6458 return "CMU License"; 6459 case MIT_ENNA: 6460 return "enna License"; 6461 case MIT_FEH: 6462 return "feh License"; 6463 case MIT_FESTIVAL: 6464 return "MIT Festival Variant"; 6465 case MIT_MODERN_VARIANT: 6466 return "MIT License Modern Variant"; 6467 case MIT_OPEN_GROUP: 6468 return "MIT Open Group variant"; 6469 case MIT_WU: 6470 return "MIT Tom Wu Variant"; 6471 case MITNFA: 6472 return "MIT +no-false-attribs license"; 6473 case MOTOSOTO: 6474 return "Motosoto License"; 6475 case MPI_PERMISSIVE: 6476 return "mpi Permissive License"; 6477 case MPICH2: 6478 return "mpich2 License"; 6479 case MPL_1_0: 6480 return "Mozilla Public License 1.0"; 6481 case MPL_1_1: 6482 return "Mozilla Public License 1.1"; 6483 case MPL_2_0: 6484 return "Mozilla Public License 2.0"; 6485 case MPL_2_0_NO_COPYLEFT_EXCEPTION: 6486 return "Mozilla Public License 2.0 (no copyleft exception)"; 6487 case MPLUS: 6488 return "mplus Font License"; 6489 case MS_LPL: 6490 return "Microsoft Limited Public License"; 6491 case MS_PL: 6492 return "Microsoft Public License"; 6493 case MS_RL: 6494 return "Microsoft Reciprocal License"; 6495 case MTLL: 6496 return "Matrix Template Library License"; 6497 case MULANPSL_1_0: 6498 return "Mulan Permissive Software License, Version 1"; 6499 case MULANPSL_2_0: 6500 return "Mulan Permissive Software License, Version 2"; 6501 case MULTICS: 6502 return "Multics License"; 6503 case MUP: 6504 return "Mup License"; 6505 case NAIST_2003: 6506 return "Nara Institute of Science and Technology License (2003)"; 6507 case NASA_1_3: 6508 return "NASA Open Source Agreement 1.3"; 6509 case NAUMEN: 6510 return "Naumen Public License"; 6511 case NBPL_1_0: 6512 return "Net Boolean Public License v1"; 6513 case NCGL_UK_2_0: 6514 return "Non-Commercial Government Licence"; 6515 case NCSA: 6516 return "University of Illinois/NCSA Open Source License"; 6517 case NET_SNMP: 6518 return "Net-SNMP License"; 6519 case NETCDF: 6520 return "NetCDF license"; 6521 case NEWSLETR: 6522 return "Newsletr License"; 6523 case NGPL: 6524 return "Nethack General Public License"; 6525 case NICTA_1_0: 6526 return "NICTA Public Software License, Version 1.0"; 6527 case NIST_PD: 6528 return "NIST Public Domain Notice"; 6529 case NIST_PD_FALLBACK: 6530 return "NIST Public Domain Notice with license fallback"; 6531 case NIST_SOFTWARE: 6532 return "NIST Software License"; 6533 case NLOD_1_0: 6534 return "Norwegian Licence for Open Government Data (NLOD) 1.0"; 6535 case NLOD_2_0: 6536 return "Norwegian Licence for Open Government Data (NLOD) 2.0"; 6537 case NLPL: 6538 return "No Limit Public License"; 6539 case NOKIA: 6540 return "Nokia Open Source License"; 6541 case NOSL: 6542 return "Netizen Open Source License"; 6543 case NOT_OPEN_SOURCE: 6544 return "Not an open source license."; 6545 case NOWEB: 6546 return "Noweb License"; 6547 case NPL_1_0: 6548 return "Netscape Public License v1.0"; 6549 case NPL_1_1: 6550 return "Netscape Public License v1.1"; 6551 case NPOSL_3_0: 6552 return "Non-Profit Open Software License 3.0"; 6553 case NRL: 6554 return "NRL License"; 6555 case NTP: 6556 return "NTP License"; 6557 case NTP_0: 6558 return "NTP No Attribution"; 6559 case NUNIT: 6560 return "Nunit License"; 6561 case O_UDA_1_0: 6562 return "Open Use of Data Agreement v1.0"; 6563 case OCCT_PL: 6564 return "Open CASCADE Technology Public License"; 6565 case OCLC_2_0: 6566 return "OCLC Research Public License 2.0"; 6567 case ODBL_1_0: 6568 return "Open Data Commons Open Database License v1.0"; 6569 case ODC_BY_1_0: 6570 return "Open Data Commons Attribution License v1.0"; 6571 case OFFIS: 6572 return "OFFIS License"; 6573 case OFL_1_0: 6574 return "SIL Open Font License 1.0"; 6575 case OFL_1_0_NO_RFN: 6576 return "SIL Open Font License 1.0 with no Reserved Font Name"; 6577 case OFL_1_0_RFN: 6578 return "SIL Open Font License 1.0 with Reserved Font Name"; 6579 case OFL_1_1: 6580 return "SIL Open Font License 1.1"; 6581 case OFL_1_1_NO_RFN: 6582 return "SIL Open Font License 1.1 with no Reserved Font Name"; 6583 case OFL_1_1_RFN: 6584 return "SIL Open Font License 1.1 with Reserved Font Name"; 6585 case OGC_1_0: 6586 return "OGC Software License, Version 1.0"; 6587 case OGDL_TAIWAN_1_0: 6588 return "Taiwan Open Government Data License, version 1.0"; 6589 case OGL_CANADA_2_0: 6590 return "Open Government Licence - Canada"; 6591 case OGL_UK_1_0: 6592 return "Open Government Licence v1.0"; 6593 case OGL_UK_2_0: 6594 return "Open Government Licence v2.0"; 6595 case OGL_UK_3_0: 6596 return "Open Government Licence v3.0"; 6597 case OGTSL: 6598 return "Open Group Test Suite License"; 6599 case OLDAP_1_1: 6600 return "Open LDAP Public License v1.1"; 6601 case OLDAP_1_2: 6602 return "Open LDAP Public License v1.2"; 6603 case OLDAP_1_3: 6604 return "Open LDAP Public License v1.3"; 6605 case OLDAP_1_4: 6606 return "Open LDAP Public License v1.4"; 6607 case OLDAP_2_0: 6608 return "Open LDAP Public License v2.0 (or possibly 2.0A and 2.0B)"; 6609 case OLDAP_2_0_1: 6610 return "Open LDAP Public License v2.0.1"; 6611 case OLDAP_2_1: 6612 return "Open LDAP Public License v2.1"; 6613 case OLDAP_2_2: 6614 return "Open LDAP Public License v2.2"; 6615 case OLDAP_2_2_1: 6616 return "Open LDAP Public License v2.2.1"; 6617 case OLDAP_2_2_2: 6618 return "Open LDAP Public License 2.2.2"; 6619 case OLDAP_2_3: 6620 return "Open LDAP Public License v2.3"; 6621 case OLDAP_2_4: 6622 return "Open LDAP Public License v2.4"; 6623 case OLDAP_2_5: 6624 return "Open LDAP Public License v2.5"; 6625 case OLDAP_2_6: 6626 return "Open LDAP Public License v2.6"; 6627 case OLDAP_2_7: 6628 return "Open LDAP Public License v2.7"; 6629 case OLDAP_2_8: 6630 return "Open LDAP Public License v2.8"; 6631 case OLFL_1_3: 6632 return "Open Logistics Foundation License Version 1.3"; 6633 case OML: 6634 return "Open Market License"; 6635 case OPENPBS_2_3: 6636 return "OpenPBS v2.3 Software License"; 6637 case OPENSSL: 6638 return "OpenSSL License"; 6639 case OPL_1_0: 6640 return "Open Public License v1.0"; 6641 case OPL_UK_3_0: 6642 return "United Kingdom Open Parliament Licence v3.0"; 6643 case OPUBL_1_0: 6644 return "Open Publication License v1.0"; 6645 case OSET_PL_2_1: 6646 return "OSET Public License version 2.1"; 6647 case OSL_1_0: 6648 return "Open Software License 1.0"; 6649 case OSL_1_1: 6650 return "Open Software License 1.1"; 6651 case OSL_2_0: 6652 return "Open Software License 2.0"; 6653 case OSL_2_1: 6654 return "Open Software License 2.1"; 6655 case OSL_3_0: 6656 return "Open Software License 3.0"; 6657 case PARITY_6_0_0: 6658 return "The Parity Public License 6.0.0"; 6659 case PARITY_7_0_0: 6660 return "The Parity Public License 7.0.0"; 6661 case PDDL_1_0: 6662 return "Open Data Commons Public Domain Dedication & License 1.0"; 6663 case PHP_3_0: 6664 return "PHP License v3.0"; 6665 case PHP_3_01: 6666 return "PHP License v3.01"; 6667 case PLEXUS: 6668 return "Plexus Classworlds License"; 6669 case POLYFORM_NONCOMMERCIAL_1_0_0: 6670 return "PolyForm Noncommercial License 1.0.0"; 6671 case POLYFORM_SMALL_BUSINESS_1_0_0: 6672 return "PolyForm Small Business License 1.0.0"; 6673 case POSTGRESQL: 6674 return "PostgreSQL License"; 6675 case PSF_2_0: 6676 return "Python Software Foundation License 2.0"; 6677 case PSFRAG: 6678 return "psfrag License"; 6679 case PSUTILS: 6680 return "psutils License"; 6681 case PYTHON_2_0: 6682 return "Python License 2.0"; 6683 case PYTHON_2_0_1: 6684 return "Python License 2.0.1"; 6685 case QHULL: 6686 return "Qhull License"; 6687 case QPL_1_0: 6688 return "Q Public License 1.0"; 6689 case QPL_1_0_INRIA_2004: 6690 return "Q Public License 1.0 - INRIA 2004 variant"; 6691 case RDISC: 6692 return "Rdisc License"; 6693 case RHECOS_1_1: 6694 return "Red Hat eCos Public License v1.1"; 6695 case RPL_1_1: 6696 return "Reciprocal Public License 1.1"; 6697 case RPL_1_5: 6698 return "Reciprocal Public License 1.5"; 6699 case RPSL_1_0: 6700 return "RealNetworks Public Source License v1.0"; 6701 case RSA_MD: 6702 return "RSA Message-Digest License"; 6703 case RSCPL: 6704 return "Ricoh Source Code Public License"; 6705 case RUBY: 6706 return "Ruby License"; 6707 case SAX_PD: 6708 return "Sax Public Domain Notice"; 6709 case SAXPATH: 6710 return "Saxpath License"; 6711 case SCEA: 6712 return "SCEA Shared Source License"; 6713 case SCHEMEREPORT: 6714 return "Scheme Language Report License"; 6715 case SENDMAIL: 6716 return "Sendmail License"; 6717 case SENDMAIL_8_23: 6718 return "Sendmail License 8.23"; 6719 case SGI_B_1_0: 6720 return "SGI Free Software License B v1.0"; 6721 case SGI_B_1_1: 6722 return "SGI Free Software License B v1.1"; 6723 case SGI_B_2_0: 6724 return "SGI Free Software License B v2.0"; 6725 case SGP4: 6726 return "SGP4 Permission Notice"; 6727 case SHL_0_5: 6728 return "Solderpad Hardware License v0.5"; 6729 case SHL_0_51: 6730 return "Solderpad Hardware License, Version 0.51"; 6731 case SIMPL_2_0: 6732 return "Simple Public License 2.0"; 6733 case SISSL: 6734 return "Sun Industry Standards Source License v1.1"; 6735 case SISSL_1_2: 6736 return "Sun Industry Standards Source License v1.2"; 6737 case SLEEPYCAT: 6738 return "Sleepycat License"; 6739 case SMLNJ: 6740 return "Standard ML of New Jersey License"; 6741 case SMPPL: 6742 return "Secure Messaging Protocol Public License"; 6743 case SNIA: 6744 return "SNIA Public License 1.1"; 6745 case SNPRINTF: 6746 return "snprintf License"; 6747 case SPENCER_86: 6748 return "Spencer License 86"; 6749 case SPENCER_94: 6750 return "Spencer License 94"; 6751 case SPENCER_99: 6752 return "Spencer License 99"; 6753 case SPL_1_0: 6754 return "Sun Public License v1.0"; 6755 case SSH_OPENSSH: 6756 return "SSH OpenSSH license"; 6757 case SSH_SHORT: 6758 return "SSH short notice"; 6759 case SSPL_1_0: 6760 return "Server Side Public License, v 1"; 6761 case STANDARDML_NJ: 6762 return "Standard ML of New Jersey License"; 6763 case SUGARCRM_1_1_3: 6764 return "SugarCRM Public License v1.1.3"; 6765 case SUNPRO: 6766 return "SunPro License"; 6767 case SWL: 6768 return "Scheme Widget Library (SWL) Software License Agreement"; 6769 case SYMLINKS: 6770 return "Symlinks License"; 6771 case TAPR_OHL_1_0: 6772 return "TAPR Open Hardware License v1.0"; 6773 case TCL: 6774 return "TCL/TK License"; 6775 case TCP_WRAPPERS: 6776 return "TCP Wrappers License"; 6777 case TERMREADKEY: 6778 return "TermReadKey License"; 6779 case TMATE: 6780 return "TMate Open Source License"; 6781 case TORQUE_1_1: 6782 return "TORQUE v2.5+ Software License v1.1"; 6783 case TOSL: 6784 return "Trusster Open Source License"; 6785 case TPDL: 6786 return "Time::ParseDate License"; 6787 case TPL_1_0: 6788 return "THOR Public License 1.0"; 6789 case TTWL: 6790 return "Text-Tabs+Wrap License"; 6791 case TU_BERLIN_1_0: 6792 return "Technische Universitaet Berlin License 1.0"; 6793 case TU_BERLIN_2_0: 6794 return "Technische Universitaet Berlin License 2.0"; 6795 case UCAR: 6796 return "UCAR License"; 6797 case UCL_1_0: 6798 return "Upstream Compatibility License v1.0"; 6799 case UNICODE_DFS_2015: 6800 return "Unicode License Agreement - Data Files and Software (2015)"; 6801 case UNICODE_DFS_2016: 6802 return "Unicode License Agreement - Data Files and Software (2016)"; 6803 case UNICODE_TOU: 6804 return "Unicode Terms of Use"; 6805 case UNIXCRYPT: 6806 return "UnixCrypt License"; 6807 case UNLICENSE: 6808 return "The Unlicense"; 6809 case UPL_1_0: 6810 return "Universal Permissive License v1.0"; 6811 case VIM: 6812 return "Vim License"; 6813 case VOSTROM: 6814 return "VOSTROM Public License for Open Source"; 6815 case VSL_1_0: 6816 return "Vovida Software License v1.0"; 6817 case W3C: 6818 return "W3C Software Notice and License (2002-12-31)"; 6819 case W3C_19980720: 6820 return "W3C Software Notice and License (1998-07-20)"; 6821 case W3C_20150513: 6822 return "W3C Software Notice and Document License (2015-05-13)"; 6823 case W3M: 6824 return "w3m License"; 6825 case WATCOM_1_0: 6826 return "Sybase Open Watcom Public License 1.0"; 6827 case WIDGET_WORKSHOP: 6828 return "Widget Workshop License"; 6829 case WSUIPA: 6830 return "Wsuipa License"; 6831 case WTFPL: 6832 return "Do What The F*ck You Want To Public License"; 6833 case WXWINDOWS: 6834 return "wxWindows Library License"; 6835 case X11: 6836 return "X11 License"; 6837 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: 6838 return "X11 License Distribution Modification Variant"; 6839 case XDEBUG_1_03: 6840 return "Xdebug License v 1.03"; 6841 case XEROX: 6842 return "Xerox License"; 6843 case XFIG: 6844 return "Xfig License"; 6845 case XFREE86_1_1: 6846 return "XFree86 License 1.1"; 6847 case XINETD: 6848 return "xinetd License"; 6849 case XLOCK: 6850 return "xlock License"; 6851 case XNET: 6852 return "X.Net License"; 6853 case XPP: 6854 return "XPP License"; 6855 case XSKAT: 6856 return "XSkat License"; 6857 case YPL_1_0: 6858 return "Yahoo! Public License v1.0"; 6859 case YPL_1_1: 6860 return "Yahoo! Public License v1.1"; 6861 case ZED: 6862 return "Zed License"; 6863 case ZEND_2_0: 6864 return "Zend License v2.0"; 6865 case ZIMBRA_1_3: 6866 return "Zimbra Public License v1.3"; 6867 case ZIMBRA_1_4: 6868 return "Zimbra Public License v1.4"; 6869 case ZLIB: 6870 return "zlib License"; 6871 case ZLIB_ACKNOWLEDGEMENT: 6872 return "zlib/libpng License with Acknowledgement"; 6873 case ZPL_1_1: 6874 return "Zope Public License 1.1"; 6875 case ZPL_2_0: 6876 return "Zope Public License 2.0"; 6877 case ZPL_2_1: 6878 return "Zope Public License 2.1"; 6879 case NULL: 6880 return null; 6881 default: 6882 return "?"; 6883 } 6884 } 6885 6886 public String getDisplay() { 6887 switch (this) { 6888 case _0BSD: 6889 return "BSD Zero Clause License"; 6890 case AAL: 6891 return "Attribution Assurance License"; 6892 case ABSTYLES: 6893 return "Abstyles License"; 6894 case ADACORE_DOC: 6895 return "AdaCore Doc License"; 6896 case ADOBE_2006: 6897 return "Adobe Systems Incorporated Source Code License Agreement"; 6898 case ADOBE_GLYPH: 6899 return "Adobe Glyph List License"; 6900 case ADSL: 6901 return "Amazon Digital Services License"; 6902 case AFL_1_1: 6903 return "Academic Free License v1.1"; 6904 case AFL_1_2: 6905 return "Academic Free License v1.2"; 6906 case AFL_2_0: 6907 return "Academic Free License v2.0"; 6908 case AFL_2_1: 6909 return "Academic Free License v2.1"; 6910 case AFL_3_0: 6911 return "Academic Free License v3.0"; 6912 case AFMPARSE: 6913 return "Afmparse License"; 6914 case AGPL_1_0: 6915 return "Affero General Public License v1.0"; 6916 case AGPL_1_0_ONLY: 6917 return "Affero General Public License v1.0 only"; 6918 case AGPL_1_0_OR_LATER: 6919 return "Affero General Public License v1.0 or later"; 6920 case AGPL_3_0: 6921 return "GNU Affero General Public License v3.0"; 6922 case AGPL_3_0_ONLY: 6923 return "GNU Affero General Public License v3.0 only"; 6924 case AGPL_3_0_OR_LATER: 6925 return "GNU Affero General Public License v3.0 or later"; 6926 case ALADDIN: 6927 return "Aladdin Free Public License"; 6928 case AMDPLPA: 6929 return "AMD's plpa_map.c License"; 6930 case AML: 6931 return "Apple MIT License"; 6932 case AMPAS: 6933 return "Academy of Motion Picture Arts and Sciences BSD"; 6934 case ANTLR_PD: 6935 return "ANTLR Software Rights Notice"; 6936 case ANTLR_PD_FALLBACK: 6937 return "ANTLR Software Rights Notice with license fallback"; 6938 case APACHE_1_0: 6939 return "Apache License 1.0"; 6940 case APACHE_1_1: 6941 return "Apache License 1.1"; 6942 case APACHE_2_0: 6943 return "Apache License 2.0"; 6944 case APAFML: 6945 return "Adobe Postscript AFM License"; 6946 case APL_1_0: 6947 return "Adaptive Public License 1.0"; 6948 case APP_S2P: 6949 return "App::s2p License"; 6950 case APSL_1_0: 6951 return "Apple Public Source License 1.0"; 6952 case APSL_1_1: 6953 return "Apple Public Source License 1.1"; 6954 case APSL_1_2: 6955 return "Apple Public Source License 1.2"; 6956 case APSL_2_0: 6957 return "Apple Public Source License 2.0"; 6958 case ARPHIC_1999: 6959 return "Arphic Public License"; 6960 case ARTISTIC_1_0: 6961 return "Artistic License 1.0"; 6962 case ARTISTIC_1_0_CL8: 6963 return "Artistic License 1.0 w/clause 8"; 6964 case ARTISTIC_1_0_PERL: 6965 return "Artistic License 1.0 (Perl)"; 6966 case ARTISTIC_2_0: 6967 return "Artistic License 2.0"; 6968 case ASWF_DIGITAL_ASSETS_1_0: 6969 return "ASWF Digital Assets License version 1.0"; 6970 case ASWF_DIGITAL_ASSETS_1_1: 6971 return "ASWF Digital Assets License 1.1"; 6972 case BAEKMUK: 6973 return "Baekmuk License"; 6974 case BAHYPH: 6975 return "Bahyph License"; 6976 case BARR: 6977 return "Barr License"; 6978 case BEERWARE: 6979 return "Beerware License"; 6980 case BITSTREAM_CHARTER: 6981 return "Bitstream Charter Font License"; 6982 case BITSTREAM_VERA: 6983 return "Bitstream Vera Font License"; 6984 case BITTORRENT_1_0: 6985 return "BitTorrent Open Source License v1.0"; 6986 case BITTORRENT_1_1: 6987 return "BitTorrent Open Source License v1.1"; 6988 case BLESSING: 6989 return "SQLite Blessing"; 6990 case BLUEOAK_1_0_0: 6991 return "Blue Oak Model License 1.0.0"; 6992 case BOEHM_GC: 6993 return "Boehm-Demers-Weiser GC License"; 6994 case BORCEUX: 6995 return "Borceux license"; 6996 case BRIAN_GLADMAN_3_CLAUSE: 6997 return "Brian Gladman 3-Clause License"; 6998 case BSD_1_CLAUSE: 6999 return "BSD 1-Clause License"; 7000 case BSD_2_CLAUSE: 7001 return "BSD 2-Clause \"Simplified\" License"; 7002 case BSD_2_CLAUSE_FREEBSD: 7003 return "BSD 2-Clause FreeBSD License"; 7004 case BSD_2_CLAUSE_NETBSD: 7005 return "BSD 2-Clause NetBSD License"; 7006 case BSD_2_CLAUSE_PATENT: 7007 return "BSD-2-Clause Plus Patent License"; 7008 case BSD_2_CLAUSE_VIEWS: 7009 return "BSD 2-Clause with views sentence"; 7010 case BSD_3_CLAUSE: 7011 return "BSD 3-Clause \"New\" or \"Revised\" License"; 7012 case BSD_3_CLAUSE_ATTRIBUTION: 7013 return "BSD with attribution"; 7014 case BSD_3_CLAUSE_CLEAR: 7015 return "BSD 3-Clause Clear License"; 7016 case BSD_3_CLAUSE_LBNL: 7017 return "Lawrence Berkeley National Labs BSD variant license"; 7018 case BSD_3_CLAUSE_MODIFICATION: 7019 return "BSD 3-Clause Modification"; 7020 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: 7021 return "BSD 3-Clause No Military License"; 7022 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: 7023 return "BSD 3-Clause No Nuclear License"; 7024 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: 7025 return "BSD 3-Clause No Nuclear License 2014"; 7026 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: 7027 return "BSD 3-Clause No Nuclear Warranty"; 7028 case BSD_3_CLAUSE_OPEN_MPI: 7029 return "BSD 3-Clause Open MPI variant"; 7030 case BSD_4_CLAUSE: 7031 return "BSD 4-Clause \"Original\" or \"Old\" License"; 7032 case BSD_4_CLAUSE_SHORTENED: 7033 return "BSD 4 Clause Shortened"; 7034 case BSD_4_CLAUSE_UC: 7035 return "BSD-4-Clause (University of California-Specific)"; 7036 case BSD_4_3RENO: 7037 return "BSD 4.3 RENO License"; 7038 case BSD_4_3TAHOE: 7039 return "BSD 4.3 TAHOE License"; 7040 case BSD_ADVERTISING_ACKNOWLEDGEMENT: 7041 return "BSD Advertising Acknowledgement License"; 7042 case BSD_ATTRIBUTION_HPND_DISCLAIMER: 7043 return "BSD with Attribution and HPND disclaimer"; 7044 case BSD_PROTECTION: 7045 return "BSD Protection License"; 7046 case BSD_SOURCE_CODE: 7047 return "BSD Source Code Attribution"; 7048 case BSL_1_0: 7049 return "Boost Software License 1.0"; 7050 case BUSL_1_1: 7051 return "Business Source License 1.1"; 7052 case BZIP2_1_0_5: 7053 return "bzip2 and libbzip2 License v1.0.5"; 7054 case BZIP2_1_0_6: 7055 return "bzip2 and libbzip2 License v1.0.6"; 7056 case C_UDA_1_0: 7057 return "Computational Use of Data Agreement v1.0"; 7058 case CAL_1_0: 7059 return "Cryptographic Autonomy License 1.0"; 7060 case CAL_1_0_COMBINED_WORK_EXCEPTION: 7061 return "Cryptographic Autonomy License 1.0 (Combined Work Exception)"; 7062 case CALDERA: 7063 return "Caldera License"; 7064 case CATOSL_1_1: 7065 return "Computer Associates Trusted Open Source License 1.1"; 7066 case CC_BY_1_0: 7067 return "Creative Commons Attribution 1.0 Generic"; 7068 case CC_BY_2_0: 7069 return "Creative Commons Attribution 2.0 Generic"; 7070 case CC_BY_2_5: 7071 return "Creative Commons Attribution 2.5 Generic"; 7072 case CC_BY_2_5_AU: 7073 return "Creative Commons Attribution 2.5 Australia"; 7074 case CC_BY_3_0: 7075 return "Creative Commons Attribution 3.0 Unported"; 7076 case CC_BY_3_0_AT: 7077 return "Creative Commons Attribution 3.0 Austria"; 7078 case CC_BY_3_0_DE: 7079 return "Creative Commons Attribution 3.0 Germany"; 7080 case CC_BY_3_0_IGO: 7081 return "Creative Commons Attribution 3.0 IGO"; 7082 case CC_BY_3_0_NL: 7083 return "Creative Commons Attribution 3.0 Netherlands"; 7084 case CC_BY_3_0_US: 7085 return "Creative Commons Attribution 3.0 United States"; 7086 case CC_BY_4_0: 7087 return "Creative Commons Attribution 4.0 International"; 7088 case CC_BY_NC_1_0: 7089 return "Creative Commons Attribution Non Commercial 1.0 Generic"; 7090 case CC_BY_NC_2_0: 7091 return "Creative Commons Attribution Non Commercial 2.0 Generic"; 7092 case CC_BY_NC_2_5: 7093 return "Creative Commons Attribution Non Commercial 2.5 Generic"; 7094 case CC_BY_NC_3_0: 7095 return "Creative Commons Attribution Non Commercial 3.0 Unported"; 7096 case CC_BY_NC_3_0_DE: 7097 return "Creative Commons Attribution Non Commercial 3.0 Germany"; 7098 case CC_BY_NC_4_0: 7099 return "Creative Commons Attribution Non Commercial 4.0 International"; 7100 case CC_BY_NC_ND_1_0: 7101 return "Creative Commons Attribution Non Commercial No Derivatives 1.0 Generic"; 7102 case CC_BY_NC_ND_2_0: 7103 return "Creative Commons Attribution Non Commercial No Derivatives 2.0 Generic"; 7104 case CC_BY_NC_ND_2_5: 7105 return "Creative Commons Attribution Non Commercial No Derivatives 2.5 Generic"; 7106 case CC_BY_NC_ND_3_0: 7107 return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Unported"; 7108 case CC_BY_NC_ND_3_0_DE: 7109 return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Germany"; 7110 case CC_BY_NC_ND_3_0_IGO: 7111 return "Creative Commons Attribution Non Commercial No Derivatives 3.0 IGO"; 7112 case CC_BY_NC_ND_4_0: 7113 return "Creative Commons Attribution Non Commercial No Derivatives 4.0 International"; 7114 case CC_BY_NC_SA_1_0: 7115 return "Creative Commons Attribution Non Commercial Share Alike 1.0 Generic"; 7116 case CC_BY_NC_SA_2_0: 7117 return "Creative Commons Attribution Non Commercial Share Alike 2.0 Generic"; 7118 case CC_BY_NC_SA_2_0_DE: 7119 return "Creative Commons Attribution Non Commercial Share Alike 2.0 Germany"; 7120 case CC_BY_NC_SA_2_0_FR: 7121 return "Creative Commons Attribution-NonCommercial-ShareAlike 2.0 France"; 7122 case CC_BY_NC_SA_2_0_UK: 7123 return "Creative Commons Attribution Non Commercial Share Alike 2.0 England and Wales"; 7124 case CC_BY_NC_SA_2_5: 7125 return "Creative Commons Attribution Non Commercial Share Alike 2.5 Generic"; 7126 case CC_BY_NC_SA_3_0: 7127 return "Creative Commons Attribution Non Commercial Share Alike 3.0 Unported"; 7128 case CC_BY_NC_SA_3_0_DE: 7129 return "Creative Commons Attribution Non Commercial Share Alike 3.0 Germany"; 7130 case CC_BY_NC_SA_3_0_IGO: 7131 return "Creative Commons Attribution Non Commercial Share Alike 3.0 IGO"; 7132 case CC_BY_NC_SA_4_0: 7133 return "Creative Commons Attribution Non Commercial Share Alike 4.0 International"; 7134 case CC_BY_ND_1_0: 7135 return "Creative Commons Attribution No Derivatives 1.0 Generic"; 7136 case CC_BY_ND_2_0: 7137 return "Creative Commons Attribution No Derivatives 2.0 Generic"; 7138 case CC_BY_ND_2_5: 7139 return "Creative Commons Attribution No Derivatives 2.5 Generic"; 7140 case CC_BY_ND_3_0: 7141 return "Creative Commons Attribution No Derivatives 3.0 Unported"; 7142 case CC_BY_ND_3_0_DE: 7143 return "Creative Commons Attribution No Derivatives 3.0 Germany"; 7144 case CC_BY_ND_4_0: 7145 return "Creative Commons Attribution No Derivatives 4.0 International"; 7146 case CC_BY_SA_1_0: 7147 return "Creative Commons Attribution Share Alike 1.0 Generic"; 7148 case CC_BY_SA_2_0: 7149 return "Creative Commons Attribution Share Alike 2.0 Generic"; 7150 case CC_BY_SA_2_0_UK: 7151 return "Creative Commons Attribution Share Alike 2.0 England and Wales"; 7152 case CC_BY_SA_2_1_JP: 7153 return "Creative Commons Attribution Share Alike 2.1 Japan"; 7154 case CC_BY_SA_2_5: 7155 return "Creative Commons Attribution Share Alike 2.5 Generic"; 7156 case CC_BY_SA_3_0: 7157 return "Creative Commons Attribution Share Alike 3.0 Unported"; 7158 case CC_BY_SA_3_0_AT: 7159 return "Creative Commons Attribution Share Alike 3.0 Austria"; 7160 case CC_BY_SA_3_0_DE: 7161 return "Creative Commons Attribution Share Alike 3.0 Germany"; 7162 case CC_BY_SA_3_0_IGO: 7163 return "Creative Commons Attribution-ShareAlike 3.0 IGO"; 7164 case CC_BY_SA_4_0: 7165 return "Creative Commons Attribution Share Alike 4.0 International"; 7166 case CC_PDDC: 7167 return "Creative Commons Public Domain Dedication and Certification"; 7168 case CC0_1_0: 7169 return "Creative Commons Zero v1.0 Universal"; 7170 case CDDL_1_0: 7171 return "Common Development and Distribution License 1.0"; 7172 case CDDL_1_1: 7173 return "Common Development and Distribution License 1.1"; 7174 case CDL_1_0: 7175 return "Common Documentation License 1.0"; 7176 case CDLA_PERMISSIVE_1_0: 7177 return "Community Data License Agreement Permissive 1.0"; 7178 case CDLA_PERMISSIVE_2_0: 7179 return "Community Data License Agreement Permissive 2.0"; 7180 case CDLA_SHARING_1_0: 7181 return "Community Data License Agreement Sharing 1.0"; 7182 case CECILL_1_0: 7183 return "CeCILL Free Software License Agreement v1.0"; 7184 case CECILL_1_1: 7185 return "CeCILL Free Software License Agreement v1.1"; 7186 case CECILL_2_0: 7187 return "CeCILL Free Software License Agreement v2.0"; 7188 case CECILL_2_1: 7189 return "CeCILL Free Software License Agreement v2.1"; 7190 case CECILL_B: 7191 return "CeCILL-B Free Software License Agreement"; 7192 case CECILL_C: 7193 return "CeCILL-C Free Software License Agreement"; 7194 case CERN_OHL_1_1: 7195 return "CERN Open Hardware Licence v1.1"; 7196 case CERN_OHL_1_2: 7197 return "CERN Open Hardware Licence v1.2"; 7198 case CERN_OHL_P_2_0: 7199 return "CERN Open Hardware Licence Version 2 - Permissive"; 7200 case CERN_OHL_S_2_0: 7201 return "CERN Open Hardware Licence Version 2 - Strongly Reciprocal"; 7202 case CERN_OHL_W_2_0: 7203 return "CERN Open Hardware Licence Version 2 - Weakly Reciprocal"; 7204 case CFITSIO: 7205 return "CFITSIO License"; 7206 case CHECKMK: 7207 return "Checkmk License"; 7208 case CLARTISTIC: 7209 return "Clarified Artistic License"; 7210 case CLIPS: 7211 return "Clips License"; 7212 case CMU_MACH: 7213 return "CMU Mach License"; 7214 case CNRI_JYTHON: 7215 return "CNRI Jython License"; 7216 case CNRI_PYTHON: 7217 return "CNRI Python License"; 7218 case CNRI_PYTHON_GPL_COMPATIBLE: 7219 return "CNRI Python Open Source GPL Compatible License Agreement"; 7220 case COIL_1_0: 7221 return "Copyfree Open Innovation License"; 7222 case COMMUNITY_SPEC_1_0: 7223 return "Community Specification License 1.0"; 7224 case CONDOR_1_1: 7225 return "Condor Public License v1.1"; 7226 case COPYLEFT_NEXT_0_3_0: 7227 return "copyleft-next 0.3.0"; 7228 case COPYLEFT_NEXT_0_3_1: 7229 return "copyleft-next 0.3.1"; 7230 case CORNELL_LOSSLESS_JPEG: 7231 return "Cornell Lossless JPEG License"; 7232 case CPAL_1_0: 7233 return "Common Public Attribution License 1.0"; 7234 case CPL_1_0: 7235 return "Common Public License 1.0"; 7236 case CPOL_1_02: 7237 return "Code Project Open License 1.02"; 7238 case CROSSWORD: 7239 return "Crossword License"; 7240 case CRYSTALSTACKER: 7241 return "CrystalStacker License"; 7242 case CUA_OPL_1_0: 7243 return "CUA Office Public License v1.0"; 7244 case CUBE: 7245 return "Cube License"; 7246 case CURL: 7247 return "curl License"; 7248 case D_FSL_1_0: 7249 return "Deutsche Freie Software Lizenz"; 7250 case DIFFMARK: 7251 return "diffmark license"; 7252 case DL_DE_BY_2_0: 7253 return "Data licence Germany ? attribution ? version 2.0"; 7254 case DOC: 7255 return "DOC License"; 7256 case DOTSEQN: 7257 return "Dotseqn License"; 7258 case DRL_1_0: 7259 return "Detection Rule License 1.0"; 7260 case DSDP: 7261 return "DSDP License"; 7262 case DTOA: 7263 return "David M. Gay dtoa License"; 7264 case DVIPDFM: 7265 return "dvipdfm License"; 7266 case ECL_1_0: 7267 return "Educational Community License v1.0"; 7268 case ECL_2_0: 7269 return "Educational Community License v2.0"; 7270 case ECOS_2_0: 7271 return "eCos license version 2.0"; 7272 case EFL_1_0: 7273 return "Eiffel Forum License v1.0"; 7274 case EFL_2_0: 7275 return "Eiffel Forum License v2.0"; 7276 case EGENIX: 7277 return "eGenix.com Public License 1.1.0"; 7278 case ELASTIC_2_0: 7279 return "Elastic License 2.0"; 7280 case ENTESSA: 7281 return "Entessa Public License v1.0"; 7282 case EPICS: 7283 return "EPICS Open License"; 7284 case EPL_1_0: 7285 return "Eclipse Public License 1.0"; 7286 case EPL_2_0: 7287 return "Eclipse Public License 2.0"; 7288 case ERLPL_1_1: 7289 return "Erlang Public License v1.1"; 7290 case ETALAB_2_0: 7291 return "Etalab Open License 2.0"; 7292 case EUDATAGRID: 7293 return "EU DataGrid Software License"; 7294 case EUPL_1_0: 7295 return "European Union Public License 1.0"; 7296 case EUPL_1_1: 7297 return "European Union Public License 1.1"; 7298 case EUPL_1_2: 7299 return "European Union Public License 1.2"; 7300 case EUROSYM: 7301 return "Eurosym License"; 7302 case FAIR: 7303 return "Fair License"; 7304 case FDK_AAC: 7305 return "Fraunhofer FDK AAC Codec Library"; 7306 case FRAMEWORX_1_0: 7307 return "Frameworx Open License 1.0"; 7308 case FREEBSD_DOC: 7309 return "FreeBSD Documentation License"; 7310 case FREEIMAGE: 7311 return "FreeImage Public License v1.0"; 7312 case FSFAP: 7313 return "FSF All Permissive License"; 7314 case FSFUL: 7315 return "FSF Unlimited License"; 7316 case FSFULLR: 7317 return "FSF Unlimited License (with License Retention)"; 7318 case FSFULLRWD: 7319 return "FSF Unlimited License (With License Retention and Warranty Disclaimer)"; 7320 case FTL: 7321 return "Freetype Project License"; 7322 case GD: 7323 return "GD License"; 7324 case GFDL_1_1: 7325 return "GNU Free Documentation License v1.1"; 7326 case GFDL_1_1_INVARIANTS_ONLY: 7327 return "GNU Free Documentation License v1.1 only - invariants"; 7328 case GFDL_1_1_INVARIANTS_OR_LATER: 7329 return "GNU Free Documentation License v1.1 or later - invariants"; 7330 case GFDL_1_1_NO_INVARIANTS_ONLY: 7331 return "GNU Free Documentation License v1.1 only - no invariants"; 7332 case GFDL_1_1_NO_INVARIANTS_OR_LATER: 7333 return "GNU Free Documentation License v1.1 or later - no invariants"; 7334 case GFDL_1_1_ONLY: 7335 return "GNU Free Documentation License v1.1 only"; 7336 case GFDL_1_1_OR_LATER: 7337 return "GNU Free Documentation License v1.1 or later"; 7338 case GFDL_1_2: 7339 return "GNU Free Documentation License v1.2"; 7340 case GFDL_1_2_INVARIANTS_ONLY: 7341 return "GNU Free Documentation License v1.2 only - invariants"; 7342 case GFDL_1_2_INVARIANTS_OR_LATER: 7343 return "GNU Free Documentation License v1.2 or later - invariants"; 7344 case GFDL_1_2_NO_INVARIANTS_ONLY: 7345 return "GNU Free Documentation License v1.2 only - no invariants"; 7346 case GFDL_1_2_NO_INVARIANTS_OR_LATER: 7347 return "GNU Free Documentation License v1.2 or later - no invariants"; 7348 case GFDL_1_2_ONLY: 7349 return "GNU Free Documentation License v1.2 only"; 7350 case GFDL_1_2_OR_LATER: 7351 return "GNU Free Documentation License v1.2 or later"; 7352 case GFDL_1_3: 7353 return "GNU Free Documentation License v1.3"; 7354 case GFDL_1_3_INVARIANTS_ONLY: 7355 return "GNU Free Documentation License v1.3 only - invariants"; 7356 case GFDL_1_3_INVARIANTS_OR_LATER: 7357 return "GNU Free Documentation License v1.3 or later - invariants"; 7358 case GFDL_1_3_NO_INVARIANTS_ONLY: 7359 return "GNU Free Documentation License v1.3 only - no invariants"; 7360 case GFDL_1_3_NO_INVARIANTS_OR_LATER: 7361 return "GNU Free Documentation License v1.3 or later - no invariants"; 7362 case GFDL_1_3_ONLY: 7363 return "GNU Free Documentation License v1.3 only"; 7364 case GFDL_1_3_OR_LATER: 7365 return "GNU Free Documentation License v1.3 or later"; 7366 case GIFTWARE: 7367 return "Giftware License"; 7368 case GL2PS: 7369 return "GL2PS License"; 7370 case GLIDE: 7371 return "3dfx Glide License"; 7372 case GLULXE: 7373 return "Glulxe License"; 7374 case GLWTPL: 7375 return "Good Luck With That Public License"; 7376 case GNUPLOT: 7377 return "gnuplot License"; 7378 case GPL_1_0: 7379 return "GNU General Public License v1.0 only"; 7380 case GPL_1_0PLUS: 7381 return "GNU General Public License v1.0 or later"; 7382 case GPL_1_0_ONLY: 7383 return "GNU General Public License v1.0 only"; 7384 case GPL_1_0_OR_LATER: 7385 return "GNU General Public License v1.0 or later"; 7386 case GPL_2_0: 7387 return "GNU General Public License v2.0 only"; 7388 case GPL_2_0PLUS: 7389 return "GNU General Public License v2.0 or later"; 7390 case GPL_2_0_ONLY: 7391 return "GNU General Public License v2.0 only"; 7392 case GPL_2_0_OR_LATER: 7393 return "GNU General Public License v2.0 or later"; 7394 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: 7395 return "GNU General Public License v2.0 w/Autoconf exception"; 7396 case GPL_2_0_WITH_BISON_EXCEPTION: 7397 return "GNU General Public License v2.0 w/Bison exception"; 7398 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: 7399 return "GNU General Public License v2.0 w/Classpath exception"; 7400 case GPL_2_0_WITH_FONT_EXCEPTION: 7401 return "GNU General Public License v2.0 w/Font exception"; 7402 case GPL_2_0_WITH_GCC_EXCEPTION: 7403 return "GNU General Public License v2.0 w/GCC Runtime Library exception"; 7404 case GPL_3_0: 7405 return "GNU General Public License v3.0 only"; 7406 case GPL_3_0PLUS: 7407 return "GNU General Public License v3.0 or later"; 7408 case GPL_3_0_ONLY: 7409 return "GNU General Public License v3.0 only"; 7410 case GPL_3_0_OR_LATER: 7411 return "GNU General Public License v3.0 or later"; 7412 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: 7413 return "GNU General Public License v3.0 w/Autoconf exception"; 7414 case GPL_3_0_WITH_GCC_EXCEPTION: 7415 return "GNU General Public License v3.0 w/GCC Runtime Library exception"; 7416 case GRAPHICS_GEMS: 7417 return "Graphics Gems License"; 7418 case GSOAP_1_3B: 7419 return "gSOAP Public License v1.3b"; 7420 case HASKELLREPORT: 7421 return "Haskell Language Report License"; 7422 case HIPPOCRATIC_2_1: 7423 return "Hippocratic License 2.1"; 7424 case HP_1986: 7425 return "Hewlett-Packard 1986 License"; 7426 case HPND: 7427 return "Historical Permission Notice and Disclaimer"; 7428 case HPND_EXPORT_US: 7429 return "HPND with US Government export control warning"; 7430 case HPND_MARKUS_KUHN: 7431 return "Historical Permission Notice and Disclaimer - Markus Kuhn variant"; 7432 case HPND_SELL_VARIANT: 7433 return "Historical Permission Notice and Disclaimer - sell variant"; 7434 case HPND_SELL_VARIANT_MIT_DISCLAIMER: 7435 return "HPND sell variant with MIT disclaimer"; 7436 case HTMLTIDY: 7437 return "HTML Tidy License"; 7438 case IBM_PIBS: 7439 return "IBM PowerPC Initialization and Boot Software"; 7440 case ICU: 7441 return "ICU License"; 7442 case IEC_CODE_COMPONENTS_EULA: 7443 return "IEC Code Components End-user licence agreement"; 7444 case IJG: 7445 return "Independent JPEG Group License"; 7446 case IJG_SHORT: 7447 return "Independent JPEG Group License - short"; 7448 case IMAGEMAGICK: 7449 return "ImageMagick License"; 7450 case IMATIX: 7451 return "iMatix Standard Function Library Agreement"; 7452 case IMLIB2: 7453 return "Imlib2 License"; 7454 case INFO_ZIP: 7455 return "Info-ZIP License"; 7456 case INNER_NET_2_0: 7457 return "Inner Net License v2.0"; 7458 case INTEL: 7459 return "Intel Open Source License"; 7460 case INTEL_ACPI: 7461 return "Intel ACPI Software License Agreement"; 7462 case INTERBASE_1_0: 7463 return "Interbase Public License v1.0"; 7464 case IPA: 7465 return "IPA Font License"; 7466 case IPL_1_0: 7467 return "IBM Public License v1.0"; 7468 case ISC: 7469 return "ISC License"; 7470 case JAM: 7471 return "Jam License"; 7472 case JASPER_2_0: 7473 return "JasPer License"; 7474 case JPL_IMAGE: 7475 return "JPL Image Use Policy"; 7476 case JPNIC: 7477 return "Japan Network Information Center License"; 7478 case JSON: 7479 return "JSON License"; 7480 case KAZLIB: 7481 return "Kazlib License"; 7482 case KNUTH_CTAN: 7483 return "Knuth CTAN License"; 7484 case LAL_1_2: 7485 return "Licence Art Libre 1.2"; 7486 case LAL_1_3: 7487 return "Licence Art Libre 1.3"; 7488 case LATEX2E: 7489 return "Latex2e License"; 7490 case LATEX2E_TRANSLATED_NOTICE: 7491 return "Latex2e with translated notice permission"; 7492 case LEPTONICA: 7493 return "Leptonica License"; 7494 case LGPL_2_0: 7495 return "GNU Library General Public License v2 only"; 7496 case LGPL_2_0PLUS: 7497 return "GNU Library General Public License v2 or later"; 7498 case LGPL_2_0_ONLY: 7499 return "GNU Library General Public License v2 only"; 7500 case LGPL_2_0_OR_LATER: 7501 return "GNU Library General Public License v2 or later"; 7502 case LGPL_2_1: 7503 return "GNU Lesser General Public License v2.1 only"; 7504 case LGPL_2_1PLUS: 7505 return "GNU Lesser General Public License v2.1 or later"; 7506 case LGPL_2_1_ONLY: 7507 return "GNU Lesser General Public License v2.1 only"; 7508 case LGPL_2_1_OR_LATER: 7509 return "GNU Lesser General Public License v2.1 or later"; 7510 case LGPL_3_0: 7511 return "GNU Lesser General Public License v3.0 only"; 7512 case LGPL_3_0PLUS: 7513 return "GNU Lesser General Public License v3.0 or later"; 7514 case LGPL_3_0_ONLY: 7515 return "GNU Lesser General Public License v3.0 only"; 7516 case LGPL_3_0_OR_LATER: 7517 return "GNU Lesser General Public License v3.0 or later"; 7518 case LGPLLR: 7519 return "Lesser General Public License For Linguistic Resources"; 7520 case LIBPNG: 7521 return "libpng License"; 7522 case LIBPNG_2_0: 7523 return "PNG Reference Library version 2"; 7524 case LIBSELINUX_1_0: 7525 return "libselinux public domain notice"; 7526 case LIBTIFF: 7527 return "libtiff License"; 7528 case LIBUTIL_DAVID_NUGENT: 7529 return "libutil David Nugent License"; 7530 case LILIQ_P_1_1: 7531 return "Licence Libre du Québec ? Permissive version 1.1"; 7532 case LILIQ_R_1_1: 7533 return "Licence Libre du Québec ? Réciprocité version 1.1"; 7534 case LILIQ_RPLUS_1_1: 7535 return "Licence Libre du Québec ? Réciprocité forte version 1.1"; 7536 case LINUX_MAN_PAGES_1_PARA: 7537 return "Linux man-pages - 1 paragraph"; 7538 case LINUX_MAN_PAGES_COPYLEFT: 7539 return "Linux man-pages Copyleft"; 7540 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: 7541 return "Linux man-pages Copyleft - 2 paragraphs"; 7542 case LINUX_MAN_PAGES_COPYLEFT_VAR: 7543 return "Linux man-pages Copyleft Variant"; 7544 case LINUX_OPENIB: 7545 return "Linux Kernel Variant of OpenIB.org license"; 7546 case LOOP: 7547 return "Common Lisp LOOP License"; 7548 case LPL_1_0: 7549 return "Lucent Public License Version 1.0"; 7550 case LPL_1_02: 7551 return "Lucent Public License v1.02"; 7552 case LPPL_1_0: 7553 return "LaTeX Project Public License v1.0"; 7554 case LPPL_1_1: 7555 return "LaTeX Project Public License v1.1"; 7556 case LPPL_1_2: 7557 return "LaTeX Project Public License v1.2"; 7558 case LPPL_1_3A: 7559 return "LaTeX Project Public License v1.3a"; 7560 case LPPL_1_3C: 7561 return "LaTeX Project Public License v1.3c"; 7562 case LZMA_SDK_9_11_TO_9_20: 7563 return "LZMA SDK License (versions 9.11 to 9.20)"; 7564 case LZMA_SDK_9_22: 7565 return "LZMA SDK License (versions 9.22 and beyond)"; 7566 case MAKEINDEX: 7567 return "MakeIndex License"; 7568 case MARTIN_BIRGMEIER: 7569 return "Martin Birgmeier License"; 7570 case METAMAIL: 7571 return "metamail License"; 7572 case MINPACK: 7573 return "Minpack License"; 7574 case MIROS: 7575 return "The MirOS Licence"; 7576 case MIT: 7577 return "MIT License"; 7578 case MIT_0: 7579 return "MIT No Attribution"; 7580 case MIT_ADVERTISING: 7581 return "Enlightenment License (e16)"; 7582 case MIT_CMU: 7583 return "CMU License"; 7584 case MIT_ENNA: 7585 return "enna License"; 7586 case MIT_FEH: 7587 return "feh License"; 7588 case MIT_FESTIVAL: 7589 return "MIT Festival Variant"; 7590 case MIT_MODERN_VARIANT: 7591 return "MIT License Modern Variant"; 7592 case MIT_OPEN_GROUP: 7593 return "MIT Open Group variant"; 7594 case MIT_WU: 7595 return "MIT Tom Wu Variant"; 7596 case MITNFA: 7597 return "MIT +no-false-attribs license"; 7598 case MOTOSOTO: 7599 return "Motosoto License"; 7600 case MPI_PERMISSIVE: 7601 return "mpi Permissive License"; 7602 case MPICH2: 7603 return "mpich2 License"; 7604 case MPL_1_0: 7605 return "Mozilla Public License 1.0"; 7606 case MPL_1_1: 7607 return "Mozilla Public License 1.1"; 7608 case MPL_2_0: 7609 return "Mozilla Public License 2.0"; 7610 case MPL_2_0_NO_COPYLEFT_EXCEPTION: 7611 return "Mozilla Public License 2.0 (no copyleft exception)"; 7612 case MPLUS: 7613 return "mplus Font License"; 7614 case MS_LPL: 7615 return "Microsoft Limited Public License"; 7616 case MS_PL: 7617 return "Microsoft Public License"; 7618 case MS_RL: 7619 return "Microsoft Reciprocal License"; 7620 case MTLL: 7621 return "Matrix Template Library License"; 7622 case MULANPSL_1_0: 7623 return "Mulan Permissive Software License, Version 1"; 7624 case MULANPSL_2_0: 7625 return "Mulan Permissive Software License, Version 2"; 7626 case MULTICS: 7627 return "Multics License"; 7628 case MUP: 7629 return "Mup License"; 7630 case NAIST_2003: 7631 return "Nara Institute of Science and Technology License (2003)"; 7632 case NASA_1_3: 7633 return "NASA Open Source Agreement 1.3"; 7634 case NAUMEN: 7635 return "Naumen Public License"; 7636 case NBPL_1_0: 7637 return "Net Boolean Public License v1"; 7638 case NCGL_UK_2_0: 7639 return "Non-Commercial Government Licence"; 7640 case NCSA: 7641 return "University of Illinois/NCSA Open Source License"; 7642 case NET_SNMP: 7643 return "Net-SNMP License"; 7644 case NETCDF: 7645 return "NetCDF license"; 7646 case NEWSLETR: 7647 return "Newsletr License"; 7648 case NGPL: 7649 return "Nethack General Public License"; 7650 case NICTA_1_0: 7651 return "NICTA Public Software License, Version 1.0"; 7652 case NIST_PD: 7653 return "NIST Public Domain Notice"; 7654 case NIST_PD_FALLBACK: 7655 return "NIST Public Domain Notice with license fallback"; 7656 case NIST_SOFTWARE: 7657 return "NIST Software License"; 7658 case NLOD_1_0: 7659 return "Norwegian Licence for Open Government Data (NLOD) 1.0"; 7660 case NLOD_2_0: 7661 return "Norwegian Licence for Open Government Data (NLOD) 2.0"; 7662 case NLPL: 7663 return "No Limit Public License"; 7664 case NOKIA: 7665 return "Nokia Open Source License"; 7666 case NOSL: 7667 return "Netizen Open Source License"; 7668 case NOT_OPEN_SOURCE: 7669 return "Not open source"; 7670 case NOWEB: 7671 return "Noweb License"; 7672 case NPL_1_0: 7673 return "Netscape Public License v1.0"; 7674 case NPL_1_1: 7675 return "Netscape Public License v1.1"; 7676 case NPOSL_3_0: 7677 return "Non-Profit Open Software License 3.0"; 7678 case NRL: 7679 return "NRL License"; 7680 case NTP: 7681 return "NTP License"; 7682 case NTP_0: 7683 return "NTP No Attribution"; 7684 case NUNIT: 7685 return "Nunit License"; 7686 case O_UDA_1_0: 7687 return "Open Use of Data Agreement v1.0"; 7688 case OCCT_PL: 7689 return "Open CASCADE Technology Public License"; 7690 case OCLC_2_0: 7691 return "OCLC Research Public License 2.0"; 7692 case ODBL_1_0: 7693 return "Open Data Commons Open Database License v1.0"; 7694 case ODC_BY_1_0: 7695 return "Open Data Commons Attribution License v1.0"; 7696 case OFFIS: 7697 return "OFFIS License"; 7698 case OFL_1_0: 7699 return "SIL Open Font License 1.0"; 7700 case OFL_1_0_NO_RFN: 7701 return "SIL Open Font License 1.0 with no Reserved Font Name"; 7702 case OFL_1_0_RFN: 7703 return "SIL Open Font License 1.0 with Reserved Font Name"; 7704 case OFL_1_1: 7705 return "SIL Open Font License 1.1"; 7706 case OFL_1_1_NO_RFN: 7707 return "SIL Open Font License 1.1 with no Reserved Font Name"; 7708 case OFL_1_1_RFN: 7709 return "SIL Open Font License 1.1 with Reserved Font Name"; 7710 case OGC_1_0: 7711 return "OGC Software License, Version 1.0"; 7712 case OGDL_TAIWAN_1_0: 7713 return "Taiwan Open Government Data License, version 1.0"; 7714 case OGL_CANADA_2_0: 7715 return "Open Government Licence - Canada"; 7716 case OGL_UK_1_0: 7717 return "Open Government Licence v1.0"; 7718 case OGL_UK_2_0: 7719 return "Open Government Licence v2.0"; 7720 case OGL_UK_3_0: 7721 return "Open Government Licence v3.0"; 7722 case OGTSL: 7723 return "Open Group Test Suite License"; 7724 case OLDAP_1_1: 7725 return "Open LDAP Public License v1.1"; 7726 case OLDAP_1_2: 7727 return "Open LDAP Public License v1.2"; 7728 case OLDAP_1_3: 7729 return "Open LDAP Public License v1.3"; 7730 case OLDAP_1_4: 7731 return "Open LDAP Public License v1.4"; 7732 case OLDAP_2_0: 7733 return "Open LDAP Public License v2.0 (or possibly 2.0A and 2.0B)"; 7734 case OLDAP_2_0_1: 7735 return "Open LDAP Public License v2.0.1"; 7736 case OLDAP_2_1: 7737 return "Open LDAP Public License v2.1"; 7738 case OLDAP_2_2: 7739 return "Open LDAP Public License v2.2"; 7740 case OLDAP_2_2_1: 7741 return "Open LDAP Public License v2.2.1"; 7742 case OLDAP_2_2_2: 7743 return "Open LDAP Public License 2.2.2"; 7744 case OLDAP_2_3: 7745 return "Open LDAP Public License v2.3"; 7746 case OLDAP_2_4: 7747 return "Open LDAP Public License v2.4"; 7748 case OLDAP_2_5: 7749 return "Open LDAP Public License v2.5"; 7750 case OLDAP_2_6: 7751 return "Open LDAP Public License v2.6"; 7752 case OLDAP_2_7: 7753 return "Open LDAP Public License v2.7"; 7754 case OLDAP_2_8: 7755 return "Open LDAP Public License v2.8"; 7756 case OLFL_1_3: 7757 return "Open Logistics Foundation License Version 1.3"; 7758 case OML: 7759 return "Open Market License"; 7760 case OPENPBS_2_3: 7761 return "OpenPBS v2.3 Software License"; 7762 case OPENSSL: 7763 return "OpenSSL License"; 7764 case OPL_1_0: 7765 return "Open Public License v1.0"; 7766 case OPL_UK_3_0: 7767 return "United Kingdom Open Parliament Licence v3.0"; 7768 case OPUBL_1_0: 7769 return "Open Publication License v1.0"; 7770 case OSET_PL_2_1: 7771 return "OSET Public License version 2.1"; 7772 case OSL_1_0: 7773 return "Open Software License 1.0"; 7774 case OSL_1_1: 7775 return "Open Software License 1.1"; 7776 case OSL_2_0: 7777 return "Open Software License 2.0"; 7778 case OSL_2_1: 7779 return "Open Software License 2.1"; 7780 case OSL_3_0: 7781 return "Open Software License 3.0"; 7782 case PARITY_6_0_0: 7783 return "The Parity Public License 6.0.0"; 7784 case PARITY_7_0_0: 7785 return "The Parity Public License 7.0.0"; 7786 case PDDL_1_0: 7787 return "Open Data Commons Public Domain Dedication & License 1.0"; 7788 case PHP_3_0: 7789 return "PHP License v3.0"; 7790 case PHP_3_01: 7791 return "PHP License v3.01"; 7792 case PLEXUS: 7793 return "Plexus Classworlds License"; 7794 case POLYFORM_NONCOMMERCIAL_1_0_0: 7795 return "PolyForm Noncommercial License 1.0.0"; 7796 case POLYFORM_SMALL_BUSINESS_1_0_0: 7797 return "PolyForm Small Business License 1.0.0"; 7798 case POSTGRESQL: 7799 return "PostgreSQL License"; 7800 case PSF_2_0: 7801 return "Python Software Foundation License 2.0"; 7802 case PSFRAG: 7803 return "psfrag License"; 7804 case PSUTILS: 7805 return "psutils License"; 7806 case PYTHON_2_0: 7807 return "Python License 2.0"; 7808 case PYTHON_2_0_1: 7809 return "Python License 2.0.1"; 7810 case QHULL: 7811 return "Qhull License"; 7812 case QPL_1_0: 7813 return "Q Public License 1.0"; 7814 case QPL_1_0_INRIA_2004: 7815 return "Q Public License 1.0 - INRIA 2004 variant"; 7816 case RDISC: 7817 return "Rdisc License"; 7818 case RHECOS_1_1: 7819 return "Red Hat eCos Public License v1.1"; 7820 case RPL_1_1: 7821 return "Reciprocal Public License 1.1"; 7822 case RPL_1_5: 7823 return "Reciprocal Public License 1.5"; 7824 case RPSL_1_0: 7825 return "RealNetworks Public Source License v1.0"; 7826 case RSA_MD: 7827 return "RSA Message-Digest License"; 7828 case RSCPL: 7829 return "Ricoh Source Code Public License"; 7830 case RUBY: 7831 return "Ruby License"; 7832 case SAX_PD: 7833 return "Sax Public Domain Notice"; 7834 case SAXPATH: 7835 return "Saxpath License"; 7836 case SCEA: 7837 return "SCEA Shared Source License"; 7838 case SCHEMEREPORT: 7839 return "Scheme Language Report License"; 7840 case SENDMAIL: 7841 return "Sendmail License"; 7842 case SENDMAIL_8_23: 7843 return "Sendmail License 8.23"; 7844 case SGI_B_1_0: 7845 return "SGI Free Software License B v1.0"; 7846 case SGI_B_1_1: 7847 return "SGI Free Software License B v1.1"; 7848 case SGI_B_2_0: 7849 return "SGI Free Software License B v2.0"; 7850 case SGP4: 7851 return "SGP4 Permission Notice"; 7852 case SHL_0_5: 7853 return "Solderpad Hardware License v0.5"; 7854 case SHL_0_51: 7855 return "Solderpad Hardware License, Version 0.51"; 7856 case SIMPL_2_0: 7857 return "Simple Public License 2.0"; 7858 case SISSL: 7859 return "Sun Industry Standards Source License v1.1"; 7860 case SISSL_1_2: 7861 return "Sun Industry Standards Source License v1.2"; 7862 case SLEEPYCAT: 7863 return "Sleepycat License"; 7864 case SMLNJ: 7865 return "Standard ML of New Jersey License"; 7866 case SMPPL: 7867 return "Secure Messaging Protocol Public License"; 7868 case SNIA: 7869 return "SNIA Public License 1.1"; 7870 case SNPRINTF: 7871 return "snprintf License"; 7872 case SPENCER_86: 7873 return "Spencer License 86"; 7874 case SPENCER_94: 7875 return "Spencer License 94"; 7876 case SPENCER_99: 7877 return "Spencer License 99"; 7878 case SPL_1_0: 7879 return "Sun Public License v1.0"; 7880 case SSH_OPENSSH: 7881 return "SSH OpenSSH license"; 7882 case SSH_SHORT: 7883 return "SSH short notice"; 7884 case SSPL_1_0: 7885 return "Server Side Public License, v 1"; 7886 case STANDARDML_NJ: 7887 return "Standard ML of New Jersey License"; 7888 case SUGARCRM_1_1_3: 7889 return "SugarCRM Public License v1.1.3"; 7890 case SUNPRO: 7891 return "SunPro License"; 7892 case SWL: 7893 return "Scheme Widget Library (SWL) Software License Agreement"; 7894 case SYMLINKS: 7895 return "Symlinks License"; 7896 case TAPR_OHL_1_0: 7897 return "TAPR Open Hardware License v1.0"; 7898 case TCL: 7899 return "TCL/TK License"; 7900 case TCP_WRAPPERS: 7901 return "TCP Wrappers License"; 7902 case TERMREADKEY: 7903 return "TermReadKey License"; 7904 case TMATE: 7905 return "TMate Open Source License"; 7906 case TORQUE_1_1: 7907 return "TORQUE v2.5+ Software License v1.1"; 7908 case TOSL: 7909 return "Trusster Open Source License"; 7910 case TPDL: 7911 return "Time::ParseDate License"; 7912 case TPL_1_0: 7913 return "THOR Public License 1.0"; 7914 case TTWL: 7915 return "Text-Tabs+Wrap License"; 7916 case TU_BERLIN_1_0: 7917 return "Technische Universitaet Berlin License 1.0"; 7918 case TU_BERLIN_2_0: 7919 return "Technische Universitaet Berlin License 2.0"; 7920 case UCAR: 7921 return "UCAR License"; 7922 case UCL_1_0: 7923 return "Upstream Compatibility License v1.0"; 7924 case UNICODE_DFS_2015: 7925 return "Unicode License Agreement - Data Files and Software (2015)"; 7926 case UNICODE_DFS_2016: 7927 return "Unicode License Agreement - Data Files and Software (2016)"; 7928 case UNICODE_TOU: 7929 return "Unicode Terms of Use"; 7930 case UNIXCRYPT: 7931 return "UnixCrypt License"; 7932 case UNLICENSE: 7933 return "The Unlicense"; 7934 case UPL_1_0: 7935 return "Universal Permissive License v1.0"; 7936 case VIM: 7937 return "Vim License"; 7938 case VOSTROM: 7939 return "VOSTROM Public License for Open Source"; 7940 case VSL_1_0: 7941 return "Vovida Software License v1.0"; 7942 case W3C: 7943 return "W3C Software Notice and License (2002-12-31)"; 7944 case W3C_19980720: 7945 return "W3C Software Notice and License (1998-07-20)"; 7946 case W3C_20150513: 7947 return "W3C Software Notice and Document License (2015-05-13)"; 7948 case W3M: 7949 return "w3m License"; 7950 case WATCOM_1_0: 7951 return "Sybase Open Watcom Public License 1.0"; 7952 case WIDGET_WORKSHOP: 7953 return "Widget Workshop License"; 7954 case WSUIPA: 7955 return "Wsuipa License"; 7956 case WTFPL: 7957 return "Do What The F*ck You Want To Public License"; 7958 case WXWINDOWS: 7959 return "wxWindows Library License"; 7960 case X11: 7961 return "X11 License"; 7962 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: 7963 return "X11 License Distribution Modification Variant"; 7964 case XDEBUG_1_03: 7965 return "Xdebug License v 1.03"; 7966 case XEROX: 7967 return "Xerox License"; 7968 case XFIG: 7969 return "Xfig License"; 7970 case XFREE86_1_1: 7971 return "XFree86 License 1.1"; 7972 case XINETD: 7973 return "xinetd License"; 7974 case XLOCK: 7975 return "xlock License"; 7976 case XNET: 7977 return "X.Net License"; 7978 case XPP: 7979 return "XPP License"; 7980 case XSKAT: 7981 return "XSkat License"; 7982 case YPL_1_0: 7983 return "Yahoo! Public License v1.0"; 7984 case YPL_1_1: 7985 return "Yahoo! Public License v1.1"; 7986 case ZED: 7987 return "Zed License"; 7988 case ZEND_2_0: 7989 return "Zend License v2.0"; 7990 case ZIMBRA_1_3: 7991 return "Zimbra Public License v1.3"; 7992 case ZIMBRA_1_4: 7993 return "Zimbra Public License v1.4"; 7994 case ZLIB: 7995 return "zlib License"; 7996 case ZLIB_ACKNOWLEDGEMENT: 7997 return "zlib/libpng License with Acknowledgement"; 7998 case ZPL_1_1: 7999 return "Zope Public License 1.1"; 8000 case ZPL_2_0: 8001 return "Zope Public License 2.0"; 8002 case ZPL_2_1: 8003 return "Zope Public License 2.1"; 8004 case NULL: 8005 return null; 8006 default: 8007 return "?"; 8008 } 8009 } 8010 } 8011 8012 public static class SPDXLicenseEnumFactory implements EnumFactory<SPDXLicense> { 8013 public SPDXLicense fromCode(String codeString) throws IllegalArgumentException { 8014 if (codeString == null || "".equals(codeString)) 8015 if (codeString == null || "".equals(codeString)) 8016 return null; 8017 if ("0BSD".equals(codeString)) 8018 return SPDXLicense._0BSD; 8019 if ("AAL".equals(codeString)) 8020 return SPDXLicense.AAL; 8021 if ("Abstyles".equals(codeString)) 8022 return SPDXLicense.ABSTYLES; 8023 if ("AdaCore-doc".equals(codeString)) 8024 return SPDXLicense.ADACORE_DOC; 8025 if ("Adobe-2006".equals(codeString)) 8026 return SPDXLicense.ADOBE_2006; 8027 if ("Adobe-Glyph".equals(codeString)) 8028 return SPDXLicense.ADOBE_GLYPH; 8029 if ("ADSL".equals(codeString)) 8030 return SPDXLicense.ADSL; 8031 if ("AFL-1.1".equals(codeString)) 8032 return SPDXLicense.AFL_1_1; 8033 if ("AFL-1.2".equals(codeString)) 8034 return SPDXLicense.AFL_1_2; 8035 if ("AFL-2.0".equals(codeString)) 8036 return SPDXLicense.AFL_2_0; 8037 if ("AFL-2.1".equals(codeString)) 8038 return SPDXLicense.AFL_2_1; 8039 if ("AFL-3.0".equals(codeString)) 8040 return SPDXLicense.AFL_3_0; 8041 if ("Afmparse".equals(codeString)) 8042 return SPDXLicense.AFMPARSE; 8043 if ("AGPL-1.0".equals(codeString)) 8044 return SPDXLicense.AGPL_1_0; 8045 if ("AGPL-1.0-only".equals(codeString)) 8046 return SPDXLicense.AGPL_1_0_ONLY; 8047 if ("AGPL-1.0-or-later".equals(codeString)) 8048 return SPDXLicense.AGPL_1_0_OR_LATER; 8049 if ("AGPL-3.0".equals(codeString)) 8050 return SPDXLicense.AGPL_3_0; 8051 if ("AGPL-3.0-only".equals(codeString)) 8052 return SPDXLicense.AGPL_3_0_ONLY; 8053 if ("AGPL-3.0-or-later".equals(codeString)) 8054 return SPDXLicense.AGPL_3_0_OR_LATER; 8055 if ("Aladdin".equals(codeString)) 8056 return SPDXLicense.ALADDIN; 8057 if ("AMDPLPA".equals(codeString)) 8058 return SPDXLicense.AMDPLPA; 8059 if ("AML".equals(codeString)) 8060 return SPDXLicense.AML; 8061 if ("AMPAS".equals(codeString)) 8062 return SPDXLicense.AMPAS; 8063 if ("ANTLR-PD".equals(codeString)) 8064 return SPDXLicense.ANTLR_PD; 8065 if ("ANTLR-PD-fallback".equals(codeString)) 8066 return SPDXLicense.ANTLR_PD_FALLBACK; 8067 if ("Apache-1.0".equals(codeString)) 8068 return SPDXLicense.APACHE_1_0; 8069 if ("Apache-1.1".equals(codeString)) 8070 return SPDXLicense.APACHE_1_1; 8071 if ("Apache-2.0".equals(codeString)) 8072 return SPDXLicense.APACHE_2_0; 8073 if ("APAFML".equals(codeString)) 8074 return SPDXLicense.APAFML; 8075 if ("APL-1.0".equals(codeString)) 8076 return SPDXLicense.APL_1_0; 8077 if ("App-s2p".equals(codeString)) 8078 return SPDXLicense.APP_S2P; 8079 if ("APSL-1.0".equals(codeString)) 8080 return SPDXLicense.APSL_1_0; 8081 if ("APSL-1.1".equals(codeString)) 8082 return SPDXLicense.APSL_1_1; 8083 if ("APSL-1.2".equals(codeString)) 8084 return SPDXLicense.APSL_1_2; 8085 if ("APSL-2.0".equals(codeString)) 8086 return SPDXLicense.APSL_2_0; 8087 if ("Arphic-1999".equals(codeString)) 8088 return SPDXLicense.ARPHIC_1999; 8089 if ("Artistic-1.0".equals(codeString)) 8090 return SPDXLicense.ARTISTIC_1_0; 8091 if ("Artistic-1.0-cl8".equals(codeString)) 8092 return SPDXLicense.ARTISTIC_1_0_CL8; 8093 if ("Artistic-1.0-Perl".equals(codeString)) 8094 return SPDXLicense.ARTISTIC_1_0_PERL; 8095 if ("Artistic-2.0".equals(codeString)) 8096 return SPDXLicense.ARTISTIC_2_0; 8097 if ("ASWF-Digital-Assets-1.0".equals(codeString)) 8098 return SPDXLicense.ASWF_DIGITAL_ASSETS_1_0; 8099 if ("ASWF-Digital-Assets-1.1".equals(codeString)) 8100 return SPDXLicense.ASWF_DIGITAL_ASSETS_1_1; 8101 if ("Baekmuk".equals(codeString)) 8102 return SPDXLicense.BAEKMUK; 8103 if ("Bahyph".equals(codeString)) 8104 return SPDXLicense.BAHYPH; 8105 if ("Barr".equals(codeString)) 8106 return SPDXLicense.BARR; 8107 if ("Beerware".equals(codeString)) 8108 return SPDXLicense.BEERWARE; 8109 if ("Bitstream-Charter".equals(codeString)) 8110 return SPDXLicense.BITSTREAM_CHARTER; 8111 if ("Bitstream-Vera".equals(codeString)) 8112 return SPDXLicense.BITSTREAM_VERA; 8113 if ("BitTorrent-1.0".equals(codeString)) 8114 return SPDXLicense.BITTORRENT_1_0; 8115 if ("BitTorrent-1.1".equals(codeString)) 8116 return SPDXLicense.BITTORRENT_1_1; 8117 if ("blessing".equals(codeString)) 8118 return SPDXLicense.BLESSING; 8119 if ("BlueOak-1.0.0".equals(codeString)) 8120 return SPDXLicense.BLUEOAK_1_0_0; 8121 if ("Boehm-GC".equals(codeString)) 8122 return SPDXLicense.BOEHM_GC; 8123 if ("Borceux".equals(codeString)) 8124 return SPDXLicense.BORCEUX; 8125 if ("Brian-Gladman-3-Clause".equals(codeString)) 8126 return SPDXLicense.BRIAN_GLADMAN_3_CLAUSE; 8127 if ("BSD-1-Clause".equals(codeString)) 8128 return SPDXLicense.BSD_1_CLAUSE; 8129 if ("BSD-2-Clause".equals(codeString)) 8130 return SPDXLicense.BSD_2_CLAUSE; 8131 if ("BSD-2-Clause-FreeBSD".equals(codeString)) 8132 return SPDXLicense.BSD_2_CLAUSE_FREEBSD; 8133 if ("BSD-2-Clause-NetBSD".equals(codeString)) 8134 return SPDXLicense.BSD_2_CLAUSE_NETBSD; 8135 if ("BSD-2-Clause-Patent".equals(codeString)) 8136 return SPDXLicense.BSD_2_CLAUSE_PATENT; 8137 if ("BSD-2-Clause-Views".equals(codeString)) 8138 return SPDXLicense.BSD_2_CLAUSE_VIEWS; 8139 if ("BSD-3-Clause".equals(codeString)) 8140 return SPDXLicense.BSD_3_CLAUSE; 8141 if ("BSD-3-Clause-Attribution".equals(codeString)) 8142 return SPDXLicense.BSD_3_CLAUSE_ATTRIBUTION; 8143 if ("BSD-3-Clause-Clear".equals(codeString)) 8144 return SPDXLicense.BSD_3_CLAUSE_CLEAR; 8145 if ("BSD-3-Clause-LBNL".equals(codeString)) 8146 return SPDXLicense.BSD_3_CLAUSE_LBNL; 8147 if ("BSD-3-Clause-Modification".equals(codeString)) 8148 return SPDXLicense.BSD_3_CLAUSE_MODIFICATION; 8149 if ("BSD-3-Clause-No-Military-License".equals(codeString)) 8150 return SPDXLicense.BSD_3_CLAUSE_NO_MILITARY_LICENSE; 8151 if ("BSD-3-Clause-No-Nuclear-License".equals(codeString)) 8152 return SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE; 8153 if ("BSD-3-Clause-No-Nuclear-License-2014".equals(codeString)) 8154 return SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014; 8155 if ("BSD-3-Clause-No-Nuclear-Warranty".equals(codeString)) 8156 return SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY; 8157 if ("BSD-3-Clause-Open-MPI".equals(codeString)) 8158 return SPDXLicense.BSD_3_CLAUSE_OPEN_MPI; 8159 if ("BSD-4-Clause".equals(codeString)) 8160 return SPDXLicense.BSD_4_CLAUSE; 8161 if ("BSD-4-Clause-Shortened".equals(codeString)) 8162 return SPDXLicense.BSD_4_CLAUSE_SHORTENED; 8163 if ("BSD-4-Clause-UC".equals(codeString)) 8164 return SPDXLicense.BSD_4_CLAUSE_UC; 8165 if ("BSD-4.3RENO".equals(codeString)) 8166 return SPDXLicense.BSD_4_3RENO; 8167 if ("BSD-4.3TAHOE".equals(codeString)) 8168 return SPDXLicense.BSD_4_3TAHOE; 8169 if ("BSD-Advertising-Acknowledgement".equals(codeString)) 8170 return SPDXLicense.BSD_ADVERTISING_ACKNOWLEDGEMENT; 8171 if ("BSD-Attribution-HPND-disclaimer".equals(codeString)) 8172 return SPDXLicense.BSD_ATTRIBUTION_HPND_DISCLAIMER; 8173 if ("BSD-Protection".equals(codeString)) 8174 return SPDXLicense.BSD_PROTECTION; 8175 if ("BSD-Source-Code".equals(codeString)) 8176 return SPDXLicense.BSD_SOURCE_CODE; 8177 if ("BSL-1.0".equals(codeString)) 8178 return SPDXLicense.BSL_1_0; 8179 if ("BUSL-1.1".equals(codeString)) 8180 return SPDXLicense.BUSL_1_1; 8181 if ("bzip2-1.0.5".equals(codeString)) 8182 return SPDXLicense.BZIP2_1_0_5; 8183 if ("bzip2-1.0.6".equals(codeString)) 8184 return SPDXLicense.BZIP2_1_0_6; 8185 if ("C-UDA-1.0".equals(codeString)) 8186 return SPDXLicense.C_UDA_1_0; 8187 if ("CAL-1.0".equals(codeString)) 8188 return SPDXLicense.CAL_1_0; 8189 if ("CAL-1.0-Combined-Work-Exception".equals(codeString)) 8190 return SPDXLicense.CAL_1_0_COMBINED_WORK_EXCEPTION; 8191 if ("Caldera".equals(codeString)) 8192 return SPDXLicense.CALDERA; 8193 if ("CATOSL-1.1".equals(codeString)) 8194 return SPDXLicense.CATOSL_1_1; 8195 if ("CC-BY-1.0".equals(codeString)) 8196 return SPDXLicense.CC_BY_1_0; 8197 if ("CC-BY-2.0".equals(codeString)) 8198 return SPDXLicense.CC_BY_2_0; 8199 if ("CC-BY-2.5".equals(codeString)) 8200 return SPDXLicense.CC_BY_2_5; 8201 if ("CC-BY-2.5-AU".equals(codeString)) 8202 return SPDXLicense.CC_BY_2_5_AU; 8203 if ("CC-BY-3.0".equals(codeString)) 8204 return SPDXLicense.CC_BY_3_0; 8205 if ("CC-BY-3.0-AT".equals(codeString)) 8206 return SPDXLicense.CC_BY_3_0_AT; 8207 if ("CC-BY-3.0-DE".equals(codeString)) 8208 return SPDXLicense.CC_BY_3_0_DE; 8209 if ("CC-BY-3.0-IGO".equals(codeString)) 8210 return SPDXLicense.CC_BY_3_0_IGO; 8211 if ("CC-BY-3.0-NL".equals(codeString)) 8212 return SPDXLicense.CC_BY_3_0_NL; 8213 if ("CC-BY-3.0-US".equals(codeString)) 8214 return SPDXLicense.CC_BY_3_0_US; 8215 if ("CC-BY-4.0".equals(codeString)) 8216 return SPDXLicense.CC_BY_4_0; 8217 if ("CC-BY-NC-1.0".equals(codeString)) 8218 return SPDXLicense.CC_BY_NC_1_0; 8219 if ("CC-BY-NC-2.0".equals(codeString)) 8220 return SPDXLicense.CC_BY_NC_2_0; 8221 if ("CC-BY-NC-2.5".equals(codeString)) 8222 return SPDXLicense.CC_BY_NC_2_5; 8223 if ("CC-BY-NC-3.0".equals(codeString)) 8224 return SPDXLicense.CC_BY_NC_3_0; 8225 if ("CC-BY-NC-3.0-DE".equals(codeString)) 8226 return SPDXLicense.CC_BY_NC_3_0_DE; 8227 if ("CC-BY-NC-4.0".equals(codeString)) 8228 return SPDXLicense.CC_BY_NC_4_0; 8229 if ("CC-BY-NC-ND-1.0".equals(codeString)) 8230 return SPDXLicense.CC_BY_NC_ND_1_0; 8231 if ("CC-BY-NC-ND-2.0".equals(codeString)) 8232 return SPDXLicense.CC_BY_NC_ND_2_0; 8233 if ("CC-BY-NC-ND-2.5".equals(codeString)) 8234 return SPDXLicense.CC_BY_NC_ND_2_5; 8235 if ("CC-BY-NC-ND-3.0".equals(codeString)) 8236 return SPDXLicense.CC_BY_NC_ND_3_0; 8237 if ("CC-BY-NC-ND-3.0-DE".equals(codeString)) 8238 return SPDXLicense.CC_BY_NC_ND_3_0_DE; 8239 if ("CC-BY-NC-ND-3.0-IGO".equals(codeString)) 8240 return SPDXLicense.CC_BY_NC_ND_3_0_IGO; 8241 if ("CC-BY-NC-ND-4.0".equals(codeString)) 8242 return SPDXLicense.CC_BY_NC_ND_4_0; 8243 if ("CC-BY-NC-SA-1.0".equals(codeString)) 8244 return SPDXLicense.CC_BY_NC_SA_1_0; 8245 if ("CC-BY-NC-SA-2.0".equals(codeString)) 8246 return SPDXLicense.CC_BY_NC_SA_2_0; 8247 if ("CC-BY-NC-SA-2.0-DE".equals(codeString)) 8248 return SPDXLicense.CC_BY_NC_SA_2_0_DE; 8249 if ("CC-BY-NC-SA-2.0-FR".equals(codeString)) 8250 return SPDXLicense.CC_BY_NC_SA_2_0_FR; 8251 if ("CC-BY-NC-SA-2.0-UK".equals(codeString)) 8252 return SPDXLicense.CC_BY_NC_SA_2_0_UK; 8253 if ("CC-BY-NC-SA-2.5".equals(codeString)) 8254 return SPDXLicense.CC_BY_NC_SA_2_5; 8255 if ("CC-BY-NC-SA-3.0".equals(codeString)) 8256 return SPDXLicense.CC_BY_NC_SA_3_0; 8257 if ("CC-BY-NC-SA-3.0-DE".equals(codeString)) 8258 return SPDXLicense.CC_BY_NC_SA_3_0_DE; 8259 if ("CC-BY-NC-SA-3.0-IGO".equals(codeString)) 8260 return SPDXLicense.CC_BY_NC_SA_3_0_IGO; 8261 if ("CC-BY-NC-SA-4.0".equals(codeString)) 8262 return SPDXLicense.CC_BY_NC_SA_4_0; 8263 if ("CC-BY-ND-1.0".equals(codeString)) 8264 return SPDXLicense.CC_BY_ND_1_0; 8265 if ("CC-BY-ND-2.0".equals(codeString)) 8266 return SPDXLicense.CC_BY_ND_2_0; 8267 if ("CC-BY-ND-2.5".equals(codeString)) 8268 return SPDXLicense.CC_BY_ND_2_5; 8269 if ("CC-BY-ND-3.0".equals(codeString)) 8270 return SPDXLicense.CC_BY_ND_3_0; 8271 if ("CC-BY-ND-3.0-DE".equals(codeString)) 8272 return SPDXLicense.CC_BY_ND_3_0_DE; 8273 if ("CC-BY-ND-4.0".equals(codeString)) 8274 return SPDXLicense.CC_BY_ND_4_0; 8275 if ("CC-BY-SA-1.0".equals(codeString)) 8276 return SPDXLicense.CC_BY_SA_1_0; 8277 if ("CC-BY-SA-2.0".equals(codeString)) 8278 return SPDXLicense.CC_BY_SA_2_0; 8279 if ("CC-BY-SA-2.0-UK".equals(codeString)) 8280 return SPDXLicense.CC_BY_SA_2_0_UK; 8281 if ("CC-BY-SA-2.1-JP".equals(codeString)) 8282 return SPDXLicense.CC_BY_SA_2_1_JP; 8283 if ("CC-BY-SA-2.5".equals(codeString)) 8284 return SPDXLicense.CC_BY_SA_2_5; 8285 if ("CC-BY-SA-3.0".equals(codeString)) 8286 return SPDXLicense.CC_BY_SA_3_0; 8287 if ("CC-BY-SA-3.0-AT".equals(codeString)) 8288 return SPDXLicense.CC_BY_SA_3_0_AT; 8289 if ("CC-BY-SA-3.0-DE".equals(codeString)) 8290 return SPDXLicense.CC_BY_SA_3_0_DE; 8291 if ("CC-BY-SA-3.0-IGO".equals(codeString)) 8292 return SPDXLicense.CC_BY_SA_3_0_IGO; 8293 if ("CC-BY-SA-4.0".equals(codeString)) 8294 return SPDXLicense.CC_BY_SA_4_0; 8295 if ("CC-PDDC".equals(codeString)) 8296 return SPDXLicense.CC_PDDC; 8297 if ("CC0-1.0".equals(codeString)) 8298 return SPDXLicense.CC0_1_0; 8299 if ("CDDL-1.0".equals(codeString)) 8300 return SPDXLicense.CDDL_1_0; 8301 if ("CDDL-1.1".equals(codeString)) 8302 return SPDXLicense.CDDL_1_1; 8303 if ("CDL-1.0".equals(codeString)) 8304 return SPDXLicense.CDL_1_0; 8305 if ("CDLA-Permissive-1.0".equals(codeString)) 8306 return SPDXLicense.CDLA_PERMISSIVE_1_0; 8307 if ("CDLA-Permissive-2.0".equals(codeString)) 8308 return SPDXLicense.CDLA_PERMISSIVE_2_0; 8309 if ("CDLA-Sharing-1.0".equals(codeString)) 8310 return SPDXLicense.CDLA_SHARING_1_0; 8311 if ("CECILL-1.0".equals(codeString)) 8312 return SPDXLicense.CECILL_1_0; 8313 if ("CECILL-1.1".equals(codeString)) 8314 return SPDXLicense.CECILL_1_1; 8315 if ("CECILL-2.0".equals(codeString)) 8316 return SPDXLicense.CECILL_2_0; 8317 if ("CECILL-2.1".equals(codeString)) 8318 return SPDXLicense.CECILL_2_1; 8319 if ("CECILL-B".equals(codeString)) 8320 return SPDXLicense.CECILL_B; 8321 if ("CECILL-C".equals(codeString)) 8322 return SPDXLicense.CECILL_C; 8323 if ("CERN-OHL-1.1".equals(codeString)) 8324 return SPDXLicense.CERN_OHL_1_1; 8325 if ("CERN-OHL-1.2".equals(codeString)) 8326 return SPDXLicense.CERN_OHL_1_2; 8327 if ("CERN-OHL-P-2.0".equals(codeString)) 8328 return SPDXLicense.CERN_OHL_P_2_0; 8329 if ("CERN-OHL-S-2.0".equals(codeString)) 8330 return SPDXLicense.CERN_OHL_S_2_0; 8331 if ("CERN-OHL-W-2.0".equals(codeString)) 8332 return SPDXLicense.CERN_OHL_W_2_0; 8333 if ("CFITSIO".equals(codeString)) 8334 return SPDXLicense.CFITSIO; 8335 if ("checkmk".equals(codeString)) 8336 return SPDXLicense.CHECKMK; 8337 if ("ClArtistic".equals(codeString)) 8338 return SPDXLicense.CLARTISTIC; 8339 if ("Clips".equals(codeString)) 8340 return SPDXLicense.CLIPS; 8341 if ("CMU-Mach".equals(codeString)) 8342 return SPDXLicense.CMU_MACH; 8343 if ("CNRI-Jython".equals(codeString)) 8344 return SPDXLicense.CNRI_JYTHON; 8345 if ("CNRI-Python".equals(codeString)) 8346 return SPDXLicense.CNRI_PYTHON; 8347 if ("CNRI-Python-GPL-Compatible".equals(codeString)) 8348 return SPDXLicense.CNRI_PYTHON_GPL_COMPATIBLE; 8349 if ("COIL-1.0".equals(codeString)) 8350 return SPDXLicense.COIL_1_0; 8351 if ("Community-Spec-1.0".equals(codeString)) 8352 return SPDXLicense.COMMUNITY_SPEC_1_0; 8353 if ("Condor-1.1".equals(codeString)) 8354 return SPDXLicense.CONDOR_1_1; 8355 if ("copyleft-next-0.3.0".equals(codeString)) 8356 return SPDXLicense.COPYLEFT_NEXT_0_3_0; 8357 if ("copyleft-next-0.3.1".equals(codeString)) 8358 return SPDXLicense.COPYLEFT_NEXT_0_3_1; 8359 if ("Cornell-Lossless-JPEG".equals(codeString)) 8360 return SPDXLicense.CORNELL_LOSSLESS_JPEG; 8361 if ("CPAL-1.0".equals(codeString)) 8362 return SPDXLicense.CPAL_1_0; 8363 if ("CPL-1.0".equals(codeString)) 8364 return SPDXLicense.CPL_1_0; 8365 if ("CPOL-1.02".equals(codeString)) 8366 return SPDXLicense.CPOL_1_02; 8367 if ("Crossword".equals(codeString)) 8368 return SPDXLicense.CROSSWORD; 8369 if ("CrystalStacker".equals(codeString)) 8370 return SPDXLicense.CRYSTALSTACKER; 8371 if ("CUA-OPL-1.0".equals(codeString)) 8372 return SPDXLicense.CUA_OPL_1_0; 8373 if ("Cube".equals(codeString)) 8374 return SPDXLicense.CUBE; 8375 if ("curl".equals(codeString)) 8376 return SPDXLicense.CURL; 8377 if ("D-FSL-1.0".equals(codeString)) 8378 return SPDXLicense.D_FSL_1_0; 8379 if ("diffmark".equals(codeString)) 8380 return SPDXLicense.DIFFMARK; 8381 if ("DL-DE-BY-2.0".equals(codeString)) 8382 return SPDXLicense.DL_DE_BY_2_0; 8383 if ("DOC".equals(codeString)) 8384 return SPDXLicense.DOC; 8385 if ("Dotseqn".equals(codeString)) 8386 return SPDXLicense.DOTSEQN; 8387 if ("DRL-1.0".equals(codeString)) 8388 return SPDXLicense.DRL_1_0; 8389 if ("DSDP".equals(codeString)) 8390 return SPDXLicense.DSDP; 8391 if ("dtoa".equals(codeString)) 8392 return SPDXLicense.DTOA; 8393 if ("dvipdfm".equals(codeString)) 8394 return SPDXLicense.DVIPDFM; 8395 if ("ECL-1.0".equals(codeString)) 8396 return SPDXLicense.ECL_1_0; 8397 if ("ECL-2.0".equals(codeString)) 8398 return SPDXLicense.ECL_2_0; 8399 if ("eCos-2.0".equals(codeString)) 8400 return SPDXLicense.ECOS_2_0; 8401 if ("EFL-1.0".equals(codeString)) 8402 return SPDXLicense.EFL_1_0; 8403 if ("EFL-2.0".equals(codeString)) 8404 return SPDXLicense.EFL_2_0; 8405 if ("eGenix".equals(codeString)) 8406 return SPDXLicense.EGENIX; 8407 if ("Elastic-2.0".equals(codeString)) 8408 return SPDXLicense.ELASTIC_2_0; 8409 if ("Entessa".equals(codeString)) 8410 return SPDXLicense.ENTESSA; 8411 if ("EPICS".equals(codeString)) 8412 return SPDXLicense.EPICS; 8413 if ("EPL-1.0".equals(codeString)) 8414 return SPDXLicense.EPL_1_0; 8415 if ("EPL-2.0".equals(codeString)) 8416 return SPDXLicense.EPL_2_0; 8417 if ("ErlPL-1.1".equals(codeString)) 8418 return SPDXLicense.ERLPL_1_1; 8419 if ("etalab-2.0".equals(codeString)) 8420 return SPDXLicense.ETALAB_2_0; 8421 if ("EUDatagrid".equals(codeString)) 8422 return SPDXLicense.EUDATAGRID; 8423 if ("EUPL-1.0".equals(codeString)) 8424 return SPDXLicense.EUPL_1_0; 8425 if ("EUPL-1.1".equals(codeString)) 8426 return SPDXLicense.EUPL_1_1; 8427 if ("EUPL-1.2".equals(codeString)) 8428 return SPDXLicense.EUPL_1_2; 8429 if ("Eurosym".equals(codeString)) 8430 return SPDXLicense.EUROSYM; 8431 if ("Fair".equals(codeString)) 8432 return SPDXLicense.FAIR; 8433 if ("FDK-AAC".equals(codeString)) 8434 return SPDXLicense.FDK_AAC; 8435 if ("Frameworx-1.0".equals(codeString)) 8436 return SPDXLicense.FRAMEWORX_1_0; 8437 if ("FreeBSD-DOC".equals(codeString)) 8438 return SPDXLicense.FREEBSD_DOC; 8439 if ("FreeImage".equals(codeString)) 8440 return SPDXLicense.FREEIMAGE; 8441 if ("FSFAP".equals(codeString)) 8442 return SPDXLicense.FSFAP; 8443 if ("FSFUL".equals(codeString)) 8444 return SPDXLicense.FSFUL; 8445 if ("FSFULLR".equals(codeString)) 8446 return SPDXLicense.FSFULLR; 8447 if ("FSFULLRWD".equals(codeString)) 8448 return SPDXLicense.FSFULLRWD; 8449 if ("FTL".equals(codeString)) 8450 return SPDXLicense.FTL; 8451 if ("GD".equals(codeString)) 8452 return SPDXLicense.GD; 8453 if ("GFDL-1.1".equals(codeString)) 8454 return SPDXLicense.GFDL_1_1; 8455 if ("GFDL-1.1-invariants-only".equals(codeString)) 8456 return SPDXLicense.GFDL_1_1_INVARIANTS_ONLY; 8457 if ("GFDL-1.1-invariants-or-later".equals(codeString)) 8458 return SPDXLicense.GFDL_1_1_INVARIANTS_OR_LATER; 8459 if ("GFDL-1.1-no-invariants-only".equals(codeString)) 8460 return SPDXLicense.GFDL_1_1_NO_INVARIANTS_ONLY; 8461 if ("GFDL-1.1-no-invariants-or-later".equals(codeString)) 8462 return SPDXLicense.GFDL_1_1_NO_INVARIANTS_OR_LATER; 8463 if ("GFDL-1.1-only".equals(codeString)) 8464 return SPDXLicense.GFDL_1_1_ONLY; 8465 if ("GFDL-1.1-or-later".equals(codeString)) 8466 return SPDXLicense.GFDL_1_1_OR_LATER; 8467 if ("GFDL-1.2".equals(codeString)) 8468 return SPDXLicense.GFDL_1_2; 8469 if ("GFDL-1.2-invariants-only".equals(codeString)) 8470 return SPDXLicense.GFDL_1_2_INVARIANTS_ONLY; 8471 if ("GFDL-1.2-invariants-or-later".equals(codeString)) 8472 return SPDXLicense.GFDL_1_2_INVARIANTS_OR_LATER; 8473 if ("GFDL-1.2-no-invariants-only".equals(codeString)) 8474 return SPDXLicense.GFDL_1_2_NO_INVARIANTS_ONLY; 8475 if ("GFDL-1.2-no-invariants-or-later".equals(codeString)) 8476 return SPDXLicense.GFDL_1_2_NO_INVARIANTS_OR_LATER; 8477 if ("GFDL-1.2-only".equals(codeString)) 8478 return SPDXLicense.GFDL_1_2_ONLY; 8479 if ("GFDL-1.2-or-later".equals(codeString)) 8480 return SPDXLicense.GFDL_1_2_OR_LATER; 8481 if ("GFDL-1.3".equals(codeString)) 8482 return SPDXLicense.GFDL_1_3; 8483 if ("GFDL-1.3-invariants-only".equals(codeString)) 8484 return SPDXLicense.GFDL_1_3_INVARIANTS_ONLY; 8485 if ("GFDL-1.3-invariants-or-later".equals(codeString)) 8486 return SPDXLicense.GFDL_1_3_INVARIANTS_OR_LATER; 8487 if ("GFDL-1.3-no-invariants-only".equals(codeString)) 8488 return SPDXLicense.GFDL_1_3_NO_INVARIANTS_ONLY; 8489 if ("GFDL-1.3-no-invariants-or-later".equals(codeString)) 8490 return SPDXLicense.GFDL_1_3_NO_INVARIANTS_OR_LATER; 8491 if ("GFDL-1.3-only".equals(codeString)) 8492 return SPDXLicense.GFDL_1_3_ONLY; 8493 if ("GFDL-1.3-or-later".equals(codeString)) 8494 return SPDXLicense.GFDL_1_3_OR_LATER; 8495 if ("Giftware".equals(codeString)) 8496 return SPDXLicense.GIFTWARE; 8497 if ("GL2PS".equals(codeString)) 8498 return SPDXLicense.GL2PS; 8499 if ("Glide".equals(codeString)) 8500 return SPDXLicense.GLIDE; 8501 if ("Glulxe".equals(codeString)) 8502 return SPDXLicense.GLULXE; 8503 if ("GLWTPL".equals(codeString)) 8504 return SPDXLicense.GLWTPL; 8505 if ("gnuplot".equals(codeString)) 8506 return SPDXLicense.GNUPLOT; 8507 if ("GPL-1.0".equals(codeString)) 8508 return SPDXLicense.GPL_1_0; 8509 if ("GPL-1.0+".equals(codeString)) 8510 return SPDXLicense.GPL_1_0PLUS; 8511 if ("GPL-1.0-only".equals(codeString)) 8512 return SPDXLicense.GPL_1_0_ONLY; 8513 if ("GPL-1.0-or-later".equals(codeString)) 8514 return SPDXLicense.GPL_1_0_OR_LATER; 8515 if ("GPL-2.0".equals(codeString)) 8516 return SPDXLicense.GPL_2_0; 8517 if ("GPL-2.0+".equals(codeString)) 8518 return SPDXLicense.GPL_2_0PLUS; 8519 if ("GPL-2.0-only".equals(codeString)) 8520 return SPDXLicense.GPL_2_0_ONLY; 8521 if ("GPL-2.0-or-later".equals(codeString)) 8522 return SPDXLicense.GPL_2_0_OR_LATER; 8523 if ("GPL-2.0-with-autoconf-exception".equals(codeString)) 8524 return SPDXLicense.GPL_2_0_WITH_AUTOCONF_EXCEPTION; 8525 if ("GPL-2.0-with-bison-exception".equals(codeString)) 8526 return SPDXLicense.GPL_2_0_WITH_BISON_EXCEPTION; 8527 if ("GPL-2.0-with-classpath-exception".equals(codeString)) 8528 return SPDXLicense.GPL_2_0_WITH_CLASSPATH_EXCEPTION; 8529 if ("GPL-2.0-with-font-exception".equals(codeString)) 8530 return SPDXLicense.GPL_2_0_WITH_FONT_EXCEPTION; 8531 if ("GPL-2.0-with-GCC-exception".equals(codeString)) 8532 return SPDXLicense.GPL_2_0_WITH_GCC_EXCEPTION; 8533 if ("GPL-3.0".equals(codeString)) 8534 return SPDXLicense.GPL_3_0; 8535 if ("GPL-3.0+".equals(codeString)) 8536 return SPDXLicense.GPL_3_0PLUS; 8537 if ("GPL-3.0-only".equals(codeString)) 8538 return SPDXLicense.GPL_3_0_ONLY; 8539 if ("GPL-3.0-or-later".equals(codeString)) 8540 return SPDXLicense.GPL_3_0_OR_LATER; 8541 if ("GPL-3.0-with-autoconf-exception".equals(codeString)) 8542 return SPDXLicense.GPL_3_0_WITH_AUTOCONF_EXCEPTION; 8543 if ("GPL-3.0-with-GCC-exception".equals(codeString)) 8544 return SPDXLicense.GPL_3_0_WITH_GCC_EXCEPTION; 8545 if ("Graphics-Gems".equals(codeString)) 8546 return SPDXLicense.GRAPHICS_GEMS; 8547 if ("gSOAP-1.3b".equals(codeString)) 8548 return SPDXLicense.GSOAP_1_3B; 8549 if ("HaskellReport".equals(codeString)) 8550 return SPDXLicense.HASKELLREPORT; 8551 if ("Hippocratic-2.1".equals(codeString)) 8552 return SPDXLicense.HIPPOCRATIC_2_1; 8553 if ("HP-1986".equals(codeString)) 8554 return SPDXLicense.HP_1986; 8555 if ("HPND".equals(codeString)) 8556 return SPDXLicense.HPND; 8557 if ("HPND-export-US".equals(codeString)) 8558 return SPDXLicense.HPND_EXPORT_US; 8559 if ("HPND-Markus-Kuhn".equals(codeString)) 8560 return SPDXLicense.HPND_MARKUS_KUHN; 8561 if ("HPND-sell-variant".equals(codeString)) 8562 return SPDXLicense.HPND_SELL_VARIANT; 8563 if ("HPND-sell-variant-MIT-disclaimer".equals(codeString)) 8564 return SPDXLicense.HPND_SELL_VARIANT_MIT_DISCLAIMER; 8565 if ("HTMLTIDY".equals(codeString)) 8566 return SPDXLicense.HTMLTIDY; 8567 if ("IBM-pibs".equals(codeString)) 8568 return SPDXLicense.IBM_PIBS; 8569 if ("ICU".equals(codeString)) 8570 return SPDXLicense.ICU; 8571 if ("IEC-Code-Components-EULA".equals(codeString)) 8572 return SPDXLicense.IEC_CODE_COMPONENTS_EULA; 8573 if ("IJG".equals(codeString)) 8574 return SPDXLicense.IJG; 8575 if ("IJG-short".equals(codeString)) 8576 return SPDXLicense.IJG_SHORT; 8577 if ("ImageMagick".equals(codeString)) 8578 return SPDXLicense.IMAGEMAGICK; 8579 if ("iMatix".equals(codeString)) 8580 return SPDXLicense.IMATIX; 8581 if ("Imlib2".equals(codeString)) 8582 return SPDXLicense.IMLIB2; 8583 if ("Info-ZIP".equals(codeString)) 8584 return SPDXLicense.INFO_ZIP; 8585 if ("Inner-Net-2.0".equals(codeString)) 8586 return SPDXLicense.INNER_NET_2_0; 8587 if ("Intel".equals(codeString)) 8588 return SPDXLicense.INTEL; 8589 if ("Intel-ACPI".equals(codeString)) 8590 return SPDXLicense.INTEL_ACPI; 8591 if ("Interbase-1.0".equals(codeString)) 8592 return SPDXLicense.INTERBASE_1_0; 8593 if ("IPA".equals(codeString)) 8594 return SPDXLicense.IPA; 8595 if ("IPL-1.0".equals(codeString)) 8596 return SPDXLicense.IPL_1_0; 8597 if ("ISC".equals(codeString)) 8598 return SPDXLicense.ISC; 8599 if ("Jam".equals(codeString)) 8600 return SPDXLicense.JAM; 8601 if ("JasPer-2.0".equals(codeString)) 8602 return SPDXLicense.JASPER_2_0; 8603 if ("JPL-image".equals(codeString)) 8604 return SPDXLicense.JPL_IMAGE; 8605 if ("JPNIC".equals(codeString)) 8606 return SPDXLicense.JPNIC; 8607 if ("JSON".equals(codeString)) 8608 return SPDXLicense.JSON; 8609 if ("Kazlib".equals(codeString)) 8610 return SPDXLicense.KAZLIB; 8611 if ("Knuth-CTAN".equals(codeString)) 8612 return SPDXLicense.KNUTH_CTAN; 8613 if ("LAL-1.2".equals(codeString)) 8614 return SPDXLicense.LAL_1_2; 8615 if ("LAL-1.3".equals(codeString)) 8616 return SPDXLicense.LAL_1_3; 8617 if ("Latex2e".equals(codeString)) 8618 return SPDXLicense.LATEX2E; 8619 if ("Latex2e-translated-notice".equals(codeString)) 8620 return SPDXLicense.LATEX2E_TRANSLATED_NOTICE; 8621 if ("Leptonica".equals(codeString)) 8622 return SPDXLicense.LEPTONICA; 8623 if ("LGPL-2.0".equals(codeString)) 8624 return SPDXLicense.LGPL_2_0; 8625 if ("LGPL-2.0+".equals(codeString)) 8626 return SPDXLicense.LGPL_2_0PLUS; 8627 if ("LGPL-2.0-only".equals(codeString)) 8628 return SPDXLicense.LGPL_2_0_ONLY; 8629 if ("LGPL-2.0-or-later".equals(codeString)) 8630 return SPDXLicense.LGPL_2_0_OR_LATER; 8631 if ("LGPL-2.1".equals(codeString)) 8632 return SPDXLicense.LGPL_2_1; 8633 if ("LGPL-2.1+".equals(codeString)) 8634 return SPDXLicense.LGPL_2_1PLUS; 8635 if ("LGPL-2.1-only".equals(codeString)) 8636 return SPDXLicense.LGPL_2_1_ONLY; 8637 if ("LGPL-2.1-or-later".equals(codeString)) 8638 return SPDXLicense.LGPL_2_1_OR_LATER; 8639 if ("LGPL-3.0".equals(codeString)) 8640 return SPDXLicense.LGPL_3_0; 8641 if ("LGPL-3.0+".equals(codeString)) 8642 return SPDXLicense.LGPL_3_0PLUS; 8643 if ("LGPL-3.0-only".equals(codeString)) 8644 return SPDXLicense.LGPL_3_0_ONLY; 8645 if ("LGPL-3.0-or-later".equals(codeString)) 8646 return SPDXLicense.LGPL_3_0_OR_LATER; 8647 if ("LGPLLR".equals(codeString)) 8648 return SPDXLicense.LGPLLR; 8649 if ("Libpng".equals(codeString)) 8650 return SPDXLicense.LIBPNG; 8651 if ("libpng-2.0".equals(codeString)) 8652 return SPDXLicense.LIBPNG_2_0; 8653 if ("libselinux-1.0".equals(codeString)) 8654 return SPDXLicense.LIBSELINUX_1_0; 8655 if ("libtiff".equals(codeString)) 8656 return SPDXLicense.LIBTIFF; 8657 if ("libutil-David-Nugent".equals(codeString)) 8658 return SPDXLicense.LIBUTIL_DAVID_NUGENT; 8659 if ("LiLiQ-P-1.1".equals(codeString)) 8660 return SPDXLicense.LILIQ_P_1_1; 8661 if ("LiLiQ-R-1.1".equals(codeString)) 8662 return SPDXLicense.LILIQ_R_1_1; 8663 if ("LiLiQ-Rplus-1.1".equals(codeString)) 8664 return SPDXLicense.LILIQ_RPLUS_1_1; 8665 if ("Linux-man-pages-1-para".equals(codeString)) 8666 return SPDXLicense.LINUX_MAN_PAGES_1_PARA; 8667 if ("Linux-man-pages-copyleft".equals(codeString)) 8668 return SPDXLicense.LINUX_MAN_PAGES_COPYLEFT; 8669 if ("Linux-man-pages-copyleft-2-para".equals(codeString)) 8670 return SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_2_PARA; 8671 if ("Linux-man-pages-copyleft-var".equals(codeString)) 8672 return SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_VAR; 8673 if ("Linux-OpenIB".equals(codeString)) 8674 return SPDXLicense.LINUX_OPENIB; 8675 if ("LOOP".equals(codeString)) 8676 return SPDXLicense.LOOP; 8677 if ("LPL-1.0".equals(codeString)) 8678 return SPDXLicense.LPL_1_0; 8679 if ("LPL-1.02".equals(codeString)) 8680 return SPDXLicense.LPL_1_02; 8681 if ("LPPL-1.0".equals(codeString)) 8682 return SPDXLicense.LPPL_1_0; 8683 if ("LPPL-1.1".equals(codeString)) 8684 return SPDXLicense.LPPL_1_1; 8685 if ("LPPL-1.2".equals(codeString)) 8686 return SPDXLicense.LPPL_1_2; 8687 if ("LPPL-1.3a".equals(codeString)) 8688 return SPDXLicense.LPPL_1_3A; 8689 if ("LPPL-1.3c".equals(codeString)) 8690 return SPDXLicense.LPPL_1_3C; 8691 if ("LZMA-SDK-9.11-to-9.20".equals(codeString)) 8692 return SPDXLicense.LZMA_SDK_9_11_TO_9_20; 8693 if ("LZMA-SDK-9.22".equals(codeString)) 8694 return SPDXLicense.LZMA_SDK_9_22; 8695 if ("MakeIndex".equals(codeString)) 8696 return SPDXLicense.MAKEINDEX; 8697 if ("Martin-Birgmeier".equals(codeString)) 8698 return SPDXLicense.MARTIN_BIRGMEIER; 8699 if ("metamail".equals(codeString)) 8700 return SPDXLicense.METAMAIL; 8701 if ("Minpack".equals(codeString)) 8702 return SPDXLicense.MINPACK; 8703 if ("MirOS".equals(codeString)) 8704 return SPDXLicense.MIROS; 8705 if ("MIT".equals(codeString)) 8706 return SPDXLicense.MIT; 8707 if ("MIT-0".equals(codeString)) 8708 return SPDXLicense.MIT_0; 8709 if ("MIT-advertising".equals(codeString)) 8710 return SPDXLicense.MIT_ADVERTISING; 8711 if ("MIT-CMU".equals(codeString)) 8712 return SPDXLicense.MIT_CMU; 8713 if ("MIT-enna".equals(codeString)) 8714 return SPDXLicense.MIT_ENNA; 8715 if ("MIT-feh".equals(codeString)) 8716 return SPDXLicense.MIT_FEH; 8717 if ("MIT-Festival".equals(codeString)) 8718 return SPDXLicense.MIT_FESTIVAL; 8719 if ("MIT-Modern-Variant".equals(codeString)) 8720 return SPDXLicense.MIT_MODERN_VARIANT; 8721 if ("MIT-open-group".equals(codeString)) 8722 return SPDXLicense.MIT_OPEN_GROUP; 8723 if ("MIT-Wu".equals(codeString)) 8724 return SPDXLicense.MIT_WU; 8725 if ("MITNFA".equals(codeString)) 8726 return SPDXLicense.MITNFA; 8727 if ("Motosoto".equals(codeString)) 8728 return SPDXLicense.MOTOSOTO; 8729 if ("mpi-permissive".equals(codeString)) 8730 return SPDXLicense.MPI_PERMISSIVE; 8731 if ("mpich2".equals(codeString)) 8732 return SPDXLicense.MPICH2; 8733 if ("MPL-1.0".equals(codeString)) 8734 return SPDXLicense.MPL_1_0; 8735 if ("MPL-1.1".equals(codeString)) 8736 return SPDXLicense.MPL_1_1; 8737 if ("MPL-2.0".equals(codeString)) 8738 return SPDXLicense.MPL_2_0; 8739 if ("MPL-2.0-no-copyleft-exception".equals(codeString)) 8740 return SPDXLicense.MPL_2_0_NO_COPYLEFT_EXCEPTION; 8741 if ("mplus".equals(codeString)) 8742 return SPDXLicense.MPLUS; 8743 if ("MS-LPL".equals(codeString)) 8744 return SPDXLicense.MS_LPL; 8745 if ("MS-PL".equals(codeString)) 8746 return SPDXLicense.MS_PL; 8747 if ("MS-RL".equals(codeString)) 8748 return SPDXLicense.MS_RL; 8749 if ("MTLL".equals(codeString)) 8750 return SPDXLicense.MTLL; 8751 if ("MulanPSL-1.0".equals(codeString)) 8752 return SPDXLicense.MULANPSL_1_0; 8753 if ("MulanPSL-2.0".equals(codeString)) 8754 return SPDXLicense.MULANPSL_2_0; 8755 if ("Multics".equals(codeString)) 8756 return SPDXLicense.MULTICS; 8757 if ("Mup".equals(codeString)) 8758 return SPDXLicense.MUP; 8759 if ("NAIST-2003".equals(codeString)) 8760 return SPDXLicense.NAIST_2003; 8761 if ("NASA-1.3".equals(codeString)) 8762 return SPDXLicense.NASA_1_3; 8763 if ("Naumen".equals(codeString)) 8764 return SPDXLicense.NAUMEN; 8765 if ("NBPL-1.0".equals(codeString)) 8766 return SPDXLicense.NBPL_1_0; 8767 if ("NCGL-UK-2.0".equals(codeString)) 8768 return SPDXLicense.NCGL_UK_2_0; 8769 if ("NCSA".equals(codeString)) 8770 return SPDXLicense.NCSA; 8771 if ("Net-SNMP".equals(codeString)) 8772 return SPDXLicense.NET_SNMP; 8773 if ("NetCDF".equals(codeString)) 8774 return SPDXLicense.NETCDF; 8775 if ("Newsletr".equals(codeString)) 8776 return SPDXLicense.NEWSLETR; 8777 if ("NGPL".equals(codeString)) 8778 return SPDXLicense.NGPL; 8779 if ("NICTA-1.0".equals(codeString)) 8780 return SPDXLicense.NICTA_1_0; 8781 if ("NIST-PD".equals(codeString)) 8782 return SPDXLicense.NIST_PD; 8783 if ("NIST-PD-fallback".equals(codeString)) 8784 return SPDXLicense.NIST_PD_FALLBACK; 8785 if ("NIST-Software".equals(codeString)) 8786 return SPDXLicense.NIST_SOFTWARE; 8787 if ("NLOD-1.0".equals(codeString)) 8788 return SPDXLicense.NLOD_1_0; 8789 if ("NLOD-2.0".equals(codeString)) 8790 return SPDXLicense.NLOD_2_0; 8791 if ("NLPL".equals(codeString)) 8792 return SPDXLicense.NLPL; 8793 if ("Nokia".equals(codeString)) 8794 return SPDXLicense.NOKIA; 8795 if ("NOSL".equals(codeString)) 8796 return SPDXLicense.NOSL; 8797 if ("not-open-source".equals(codeString)) 8798 return SPDXLicense.NOT_OPEN_SOURCE; 8799 if ("Noweb".equals(codeString)) 8800 return SPDXLicense.NOWEB; 8801 if ("NPL-1.0".equals(codeString)) 8802 return SPDXLicense.NPL_1_0; 8803 if ("NPL-1.1".equals(codeString)) 8804 return SPDXLicense.NPL_1_1; 8805 if ("NPOSL-3.0".equals(codeString)) 8806 return SPDXLicense.NPOSL_3_0; 8807 if ("NRL".equals(codeString)) 8808 return SPDXLicense.NRL; 8809 if ("NTP".equals(codeString)) 8810 return SPDXLicense.NTP; 8811 if ("NTP-0".equals(codeString)) 8812 return SPDXLicense.NTP_0; 8813 if ("Nunit".equals(codeString)) 8814 return SPDXLicense.NUNIT; 8815 if ("O-UDA-1.0".equals(codeString)) 8816 return SPDXLicense.O_UDA_1_0; 8817 if ("OCCT-PL".equals(codeString)) 8818 return SPDXLicense.OCCT_PL; 8819 if ("OCLC-2.0".equals(codeString)) 8820 return SPDXLicense.OCLC_2_0; 8821 if ("ODbL-1.0".equals(codeString)) 8822 return SPDXLicense.ODBL_1_0; 8823 if ("ODC-By-1.0".equals(codeString)) 8824 return SPDXLicense.ODC_BY_1_0; 8825 if ("OFFIS".equals(codeString)) 8826 return SPDXLicense.OFFIS; 8827 if ("OFL-1.0".equals(codeString)) 8828 return SPDXLicense.OFL_1_0; 8829 if ("OFL-1.0-no-RFN".equals(codeString)) 8830 return SPDXLicense.OFL_1_0_NO_RFN; 8831 if ("OFL-1.0-RFN".equals(codeString)) 8832 return SPDXLicense.OFL_1_0_RFN; 8833 if ("OFL-1.1".equals(codeString)) 8834 return SPDXLicense.OFL_1_1; 8835 if ("OFL-1.1-no-RFN".equals(codeString)) 8836 return SPDXLicense.OFL_1_1_NO_RFN; 8837 if ("OFL-1.1-RFN".equals(codeString)) 8838 return SPDXLicense.OFL_1_1_RFN; 8839 if ("OGC-1.0".equals(codeString)) 8840 return SPDXLicense.OGC_1_0; 8841 if ("OGDL-Taiwan-1.0".equals(codeString)) 8842 return SPDXLicense.OGDL_TAIWAN_1_0; 8843 if ("OGL-Canada-2.0".equals(codeString)) 8844 return SPDXLicense.OGL_CANADA_2_0; 8845 if ("OGL-UK-1.0".equals(codeString)) 8846 return SPDXLicense.OGL_UK_1_0; 8847 if ("OGL-UK-2.0".equals(codeString)) 8848 return SPDXLicense.OGL_UK_2_0; 8849 if ("OGL-UK-3.0".equals(codeString)) 8850 return SPDXLicense.OGL_UK_3_0; 8851 if ("OGTSL".equals(codeString)) 8852 return SPDXLicense.OGTSL; 8853 if ("OLDAP-1.1".equals(codeString)) 8854 return SPDXLicense.OLDAP_1_1; 8855 if ("OLDAP-1.2".equals(codeString)) 8856 return SPDXLicense.OLDAP_1_2; 8857 if ("OLDAP-1.3".equals(codeString)) 8858 return SPDXLicense.OLDAP_1_3; 8859 if ("OLDAP-1.4".equals(codeString)) 8860 return SPDXLicense.OLDAP_1_4; 8861 if ("OLDAP-2.0".equals(codeString)) 8862 return SPDXLicense.OLDAP_2_0; 8863 if ("OLDAP-2.0.1".equals(codeString)) 8864 return SPDXLicense.OLDAP_2_0_1; 8865 if ("OLDAP-2.1".equals(codeString)) 8866 return SPDXLicense.OLDAP_2_1; 8867 if ("OLDAP-2.2".equals(codeString)) 8868 return SPDXLicense.OLDAP_2_2; 8869 if ("OLDAP-2.2.1".equals(codeString)) 8870 return SPDXLicense.OLDAP_2_2_1; 8871 if ("OLDAP-2.2.2".equals(codeString)) 8872 return SPDXLicense.OLDAP_2_2_2; 8873 if ("OLDAP-2.3".equals(codeString)) 8874 return SPDXLicense.OLDAP_2_3; 8875 if ("OLDAP-2.4".equals(codeString)) 8876 return SPDXLicense.OLDAP_2_4; 8877 if ("OLDAP-2.5".equals(codeString)) 8878 return SPDXLicense.OLDAP_2_5; 8879 if ("OLDAP-2.6".equals(codeString)) 8880 return SPDXLicense.OLDAP_2_6; 8881 if ("OLDAP-2.7".equals(codeString)) 8882 return SPDXLicense.OLDAP_2_7; 8883 if ("OLDAP-2.8".equals(codeString)) 8884 return SPDXLicense.OLDAP_2_8; 8885 if ("OLFL-1.3".equals(codeString)) 8886 return SPDXLicense.OLFL_1_3; 8887 if ("OML".equals(codeString)) 8888 return SPDXLicense.OML; 8889 if ("OpenPBS-2.3".equals(codeString)) 8890 return SPDXLicense.OPENPBS_2_3; 8891 if ("OpenSSL".equals(codeString)) 8892 return SPDXLicense.OPENSSL; 8893 if ("OPL-1.0".equals(codeString)) 8894 return SPDXLicense.OPL_1_0; 8895 if ("OPL-UK-3.0".equals(codeString)) 8896 return SPDXLicense.OPL_UK_3_0; 8897 if ("OPUBL-1.0".equals(codeString)) 8898 return SPDXLicense.OPUBL_1_0; 8899 if ("OSET-PL-2.1".equals(codeString)) 8900 return SPDXLicense.OSET_PL_2_1; 8901 if ("OSL-1.0".equals(codeString)) 8902 return SPDXLicense.OSL_1_0; 8903 if ("OSL-1.1".equals(codeString)) 8904 return SPDXLicense.OSL_1_1; 8905 if ("OSL-2.0".equals(codeString)) 8906 return SPDXLicense.OSL_2_0; 8907 if ("OSL-2.1".equals(codeString)) 8908 return SPDXLicense.OSL_2_1; 8909 if ("OSL-3.0".equals(codeString)) 8910 return SPDXLicense.OSL_3_0; 8911 if ("Parity-6.0.0".equals(codeString)) 8912 return SPDXLicense.PARITY_6_0_0; 8913 if ("Parity-7.0.0".equals(codeString)) 8914 return SPDXLicense.PARITY_7_0_0; 8915 if ("PDDL-1.0".equals(codeString)) 8916 return SPDXLicense.PDDL_1_0; 8917 if ("PHP-3.0".equals(codeString)) 8918 return SPDXLicense.PHP_3_0; 8919 if ("PHP-3.01".equals(codeString)) 8920 return SPDXLicense.PHP_3_01; 8921 if ("Plexus".equals(codeString)) 8922 return SPDXLicense.PLEXUS; 8923 if ("PolyForm-Noncommercial-1.0.0".equals(codeString)) 8924 return SPDXLicense.POLYFORM_NONCOMMERCIAL_1_0_0; 8925 if ("PolyForm-Small-Business-1.0.0".equals(codeString)) 8926 return SPDXLicense.POLYFORM_SMALL_BUSINESS_1_0_0; 8927 if ("PostgreSQL".equals(codeString)) 8928 return SPDXLicense.POSTGRESQL; 8929 if ("PSF-2.0".equals(codeString)) 8930 return SPDXLicense.PSF_2_0; 8931 if ("psfrag".equals(codeString)) 8932 return SPDXLicense.PSFRAG; 8933 if ("psutils".equals(codeString)) 8934 return SPDXLicense.PSUTILS; 8935 if ("Python-2.0".equals(codeString)) 8936 return SPDXLicense.PYTHON_2_0; 8937 if ("Python-2.0.1".equals(codeString)) 8938 return SPDXLicense.PYTHON_2_0_1; 8939 if ("Qhull".equals(codeString)) 8940 return SPDXLicense.QHULL; 8941 if ("QPL-1.0".equals(codeString)) 8942 return SPDXLicense.QPL_1_0; 8943 if ("QPL-1.0-INRIA-2004".equals(codeString)) 8944 return SPDXLicense.QPL_1_0_INRIA_2004; 8945 if ("Rdisc".equals(codeString)) 8946 return SPDXLicense.RDISC; 8947 if ("RHeCos-1.1".equals(codeString)) 8948 return SPDXLicense.RHECOS_1_1; 8949 if ("RPL-1.1".equals(codeString)) 8950 return SPDXLicense.RPL_1_1; 8951 if ("RPL-1.5".equals(codeString)) 8952 return SPDXLicense.RPL_1_5; 8953 if ("RPSL-1.0".equals(codeString)) 8954 return SPDXLicense.RPSL_1_0; 8955 if ("RSA-MD".equals(codeString)) 8956 return SPDXLicense.RSA_MD; 8957 if ("RSCPL".equals(codeString)) 8958 return SPDXLicense.RSCPL; 8959 if ("Ruby".equals(codeString)) 8960 return SPDXLicense.RUBY; 8961 if ("SAX-PD".equals(codeString)) 8962 return SPDXLicense.SAX_PD; 8963 if ("Saxpath".equals(codeString)) 8964 return SPDXLicense.SAXPATH; 8965 if ("SCEA".equals(codeString)) 8966 return SPDXLicense.SCEA; 8967 if ("SchemeReport".equals(codeString)) 8968 return SPDXLicense.SCHEMEREPORT; 8969 if ("Sendmail".equals(codeString)) 8970 return SPDXLicense.SENDMAIL; 8971 if ("Sendmail-8.23".equals(codeString)) 8972 return SPDXLicense.SENDMAIL_8_23; 8973 if ("SGI-B-1.0".equals(codeString)) 8974 return SPDXLicense.SGI_B_1_0; 8975 if ("SGI-B-1.1".equals(codeString)) 8976 return SPDXLicense.SGI_B_1_1; 8977 if ("SGI-B-2.0".equals(codeString)) 8978 return SPDXLicense.SGI_B_2_0; 8979 if ("SGP4".equals(codeString)) 8980 return SPDXLicense.SGP4; 8981 if ("SHL-0.5".equals(codeString)) 8982 return SPDXLicense.SHL_0_5; 8983 if ("SHL-0.51".equals(codeString)) 8984 return SPDXLicense.SHL_0_51; 8985 if ("SimPL-2.0".equals(codeString)) 8986 return SPDXLicense.SIMPL_2_0; 8987 if ("SISSL".equals(codeString)) 8988 return SPDXLicense.SISSL; 8989 if ("SISSL-1.2".equals(codeString)) 8990 return SPDXLicense.SISSL_1_2; 8991 if ("Sleepycat".equals(codeString)) 8992 return SPDXLicense.SLEEPYCAT; 8993 if ("SMLNJ".equals(codeString)) 8994 return SPDXLicense.SMLNJ; 8995 if ("SMPPL".equals(codeString)) 8996 return SPDXLicense.SMPPL; 8997 if ("SNIA".equals(codeString)) 8998 return SPDXLicense.SNIA; 8999 if ("snprintf".equals(codeString)) 9000 return SPDXLicense.SNPRINTF; 9001 if ("Spencer-86".equals(codeString)) 9002 return SPDXLicense.SPENCER_86; 9003 if ("Spencer-94".equals(codeString)) 9004 return SPDXLicense.SPENCER_94; 9005 if ("Spencer-99".equals(codeString)) 9006 return SPDXLicense.SPENCER_99; 9007 if ("SPL-1.0".equals(codeString)) 9008 return SPDXLicense.SPL_1_0; 9009 if ("SSH-OpenSSH".equals(codeString)) 9010 return SPDXLicense.SSH_OPENSSH; 9011 if ("SSH-short".equals(codeString)) 9012 return SPDXLicense.SSH_SHORT; 9013 if ("SSPL-1.0".equals(codeString)) 9014 return SPDXLicense.SSPL_1_0; 9015 if ("StandardML-NJ".equals(codeString)) 9016 return SPDXLicense.STANDARDML_NJ; 9017 if ("SugarCRM-1.1.3".equals(codeString)) 9018 return SPDXLicense.SUGARCRM_1_1_3; 9019 if ("SunPro".equals(codeString)) 9020 return SPDXLicense.SUNPRO; 9021 if ("SWL".equals(codeString)) 9022 return SPDXLicense.SWL; 9023 if ("Symlinks".equals(codeString)) 9024 return SPDXLicense.SYMLINKS; 9025 if ("TAPR-OHL-1.0".equals(codeString)) 9026 return SPDXLicense.TAPR_OHL_1_0; 9027 if ("TCL".equals(codeString)) 9028 return SPDXLicense.TCL; 9029 if ("TCP-wrappers".equals(codeString)) 9030 return SPDXLicense.TCP_WRAPPERS; 9031 if ("TermReadKey".equals(codeString)) 9032 return SPDXLicense.TERMREADKEY; 9033 if ("TMate".equals(codeString)) 9034 return SPDXLicense.TMATE; 9035 if ("TORQUE-1.1".equals(codeString)) 9036 return SPDXLicense.TORQUE_1_1; 9037 if ("TOSL".equals(codeString)) 9038 return SPDXLicense.TOSL; 9039 if ("TPDL".equals(codeString)) 9040 return SPDXLicense.TPDL; 9041 if ("TPL-1.0".equals(codeString)) 9042 return SPDXLicense.TPL_1_0; 9043 if ("TTWL".equals(codeString)) 9044 return SPDXLicense.TTWL; 9045 if ("TU-Berlin-1.0".equals(codeString)) 9046 return SPDXLicense.TU_BERLIN_1_0; 9047 if ("TU-Berlin-2.0".equals(codeString)) 9048 return SPDXLicense.TU_BERLIN_2_0; 9049 if ("UCAR".equals(codeString)) 9050 return SPDXLicense.UCAR; 9051 if ("UCL-1.0".equals(codeString)) 9052 return SPDXLicense.UCL_1_0; 9053 if ("Unicode-DFS-2015".equals(codeString)) 9054 return SPDXLicense.UNICODE_DFS_2015; 9055 if ("Unicode-DFS-2016".equals(codeString)) 9056 return SPDXLicense.UNICODE_DFS_2016; 9057 if ("Unicode-TOU".equals(codeString)) 9058 return SPDXLicense.UNICODE_TOU; 9059 if ("UnixCrypt".equals(codeString)) 9060 return SPDXLicense.UNIXCRYPT; 9061 if ("Unlicense".equals(codeString)) 9062 return SPDXLicense.UNLICENSE; 9063 if ("UPL-1.0".equals(codeString)) 9064 return SPDXLicense.UPL_1_0; 9065 if ("Vim".equals(codeString)) 9066 return SPDXLicense.VIM; 9067 if ("VOSTROM".equals(codeString)) 9068 return SPDXLicense.VOSTROM; 9069 if ("VSL-1.0".equals(codeString)) 9070 return SPDXLicense.VSL_1_0; 9071 if ("W3C".equals(codeString)) 9072 return SPDXLicense.W3C; 9073 if ("W3C-19980720".equals(codeString)) 9074 return SPDXLicense.W3C_19980720; 9075 if ("W3C-20150513".equals(codeString)) 9076 return SPDXLicense.W3C_20150513; 9077 if ("w3m".equals(codeString)) 9078 return SPDXLicense.W3M; 9079 if ("Watcom-1.0".equals(codeString)) 9080 return SPDXLicense.WATCOM_1_0; 9081 if ("Widget-Workshop".equals(codeString)) 9082 return SPDXLicense.WIDGET_WORKSHOP; 9083 if ("Wsuipa".equals(codeString)) 9084 return SPDXLicense.WSUIPA; 9085 if ("WTFPL".equals(codeString)) 9086 return SPDXLicense.WTFPL; 9087 if ("wxWindows".equals(codeString)) 9088 return SPDXLicense.WXWINDOWS; 9089 if ("X11".equals(codeString)) 9090 return SPDXLicense.X11; 9091 if ("X11-distribute-modifications-variant".equals(codeString)) 9092 return SPDXLicense.X11_DISTRIBUTE_MODIFICATIONS_VARIANT; 9093 if ("Xdebug-1.03".equals(codeString)) 9094 return SPDXLicense.XDEBUG_1_03; 9095 if ("Xerox".equals(codeString)) 9096 return SPDXLicense.XEROX; 9097 if ("Xfig".equals(codeString)) 9098 return SPDXLicense.XFIG; 9099 if ("XFree86-1.1".equals(codeString)) 9100 return SPDXLicense.XFREE86_1_1; 9101 if ("xinetd".equals(codeString)) 9102 return SPDXLicense.XINETD; 9103 if ("xlock".equals(codeString)) 9104 return SPDXLicense.XLOCK; 9105 if ("Xnet".equals(codeString)) 9106 return SPDXLicense.XNET; 9107 if ("xpp".equals(codeString)) 9108 return SPDXLicense.XPP; 9109 if ("XSkat".equals(codeString)) 9110 return SPDXLicense.XSKAT; 9111 if ("YPL-1.0".equals(codeString)) 9112 return SPDXLicense.YPL_1_0; 9113 if ("YPL-1.1".equals(codeString)) 9114 return SPDXLicense.YPL_1_1; 9115 if ("Zed".equals(codeString)) 9116 return SPDXLicense.ZED; 9117 if ("Zend-2.0".equals(codeString)) 9118 return SPDXLicense.ZEND_2_0; 9119 if ("Zimbra-1.3".equals(codeString)) 9120 return SPDXLicense.ZIMBRA_1_3; 9121 if ("Zimbra-1.4".equals(codeString)) 9122 return SPDXLicense.ZIMBRA_1_4; 9123 if ("Zlib".equals(codeString)) 9124 return SPDXLicense.ZLIB; 9125 if ("zlib-acknowledgement".equals(codeString)) 9126 return SPDXLicense.ZLIB_ACKNOWLEDGEMENT; 9127 if ("ZPL-1.1".equals(codeString)) 9128 return SPDXLicense.ZPL_1_1; 9129 if ("ZPL-2.0".equals(codeString)) 9130 return SPDXLicense.ZPL_2_0; 9131 if ("ZPL-2.1".equals(codeString)) 9132 return SPDXLicense.ZPL_2_1; 9133 throw new IllegalArgumentException("Unknown SPDXLicense code '" + codeString + "'"); 9134 } 9135 9136 public Enumeration<SPDXLicense> fromType(PrimitiveType<?> code) throws FHIRException { 9137 if (code == null) 9138 return null; 9139 if (code.isEmpty()) 9140 return new Enumeration<SPDXLicense>(this, SPDXLicense.NULL, code); 9141 String codeString = ((PrimitiveType) code).asStringValue(); 9142 if (codeString == null || "".equals(codeString)) 9143 return new Enumeration<SPDXLicense>(this, SPDXLicense.NULL, code); 9144 if ("0BSD".equals(codeString)) 9145 return new Enumeration<SPDXLicense>(this, SPDXLicense._0BSD, code); 9146 if ("AAL".equals(codeString)) 9147 return new Enumeration<SPDXLicense>(this, SPDXLicense.AAL, code); 9148 if ("Abstyles".equals(codeString)) 9149 return new Enumeration<SPDXLicense>(this, SPDXLicense.ABSTYLES, code); 9150 if ("AdaCore-doc".equals(codeString)) 9151 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADACORE_DOC, code); 9152 if ("Adobe-2006".equals(codeString)) 9153 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADOBE_2006, code); 9154 if ("Adobe-Glyph".equals(codeString)) 9155 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADOBE_GLYPH, code); 9156 if ("ADSL".equals(codeString)) 9157 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADSL, code); 9158 if ("AFL-1.1".equals(codeString)) 9159 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_1_1, code); 9160 if ("AFL-1.2".equals(codeString)) 9161 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_1_2, code); 9162 if ("AFL-2.0".equals(codeString)) 9163 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_2_0, code); 9164 if ("AFL-2.1".equals(codeString)) 9165 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_2_1, code); 9166 if ("AFL-3.0".equals(codeString)) 9167 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_3_0, code); 9168 if ("Afmparse".equals(codeString)) 9169 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFMPARSE, code); 9170 if ("AGPL-1.0".equals(codeString)) 9171 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_1_0, code); 9172 if ("AGPL-1.0-only".equals(codeString)) 9173 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_1_0_ONLY, code); 9174 if ("AGPL-1.0-or-later".equals(codeString)) 9175 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_1_0_OR_LATER, code); 9176 if ("AGPL-3.0".equals(codeString)) 9177 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_3_0, code); 9178 if ("AGPL-3.0-only".equals(codeString)) 9179 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_3_0_ONLY, code); 9180 if ("AGPL-3.0-or-later".equals(codeString)) 9181 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_3_0_OR_LATER, code); 9182 if ("Aladdin".equals(codeString)) 9183 return new Enumeration<SPDXLicense>(this, SPDXLicense.ALADDIN, code); 9184 if ("AMDPLPA".equals(codeString)) 9185 return new Enumeration<SPDXLicense>(this, SPDXLicense.AMDPLPA, code); 9186 if ("AML".equals(codeString)) 9187 return new Enumeration<SPDXLicense>(this, SPDXLicense.AML, code); 9188 if ("AMPAS".equals(codeString)) 9189 return new Enumeration<SPDXLicense>(this, SPDXLicense.AMPAS, code); 9190 if ("ANTLR-PD".equals(codeString)) 9191 return new Enumeration<SPDXLicense>(this, SPDXLicense.ANTLR_PD, code); 9192 if ("ANTLR-PD-fallback".equals(codeString)) 9193 return new Enumeration<SPDXLicense>(this, SPDXLicense.ANTLR_PD_FALLBACK, code); 9194 if ("Apache-1.0".equals(codeString)) 9195 return new Enumeration<SPDXLicense>(this, SPDXLicense.APACHE_1_0, code); 9196 if ("Apache-1.1".equals(codeString)) 9197 return new Enumeration<SPDXLicense>(this, SPDXLicense.APACHE_1_1, code); 9198 if ("Apache-2.0".equals(codeString)) 9199 return new Enumeration<SPDXLicense>(this, SPDXLicense.APACHE_2_0, code); 9200 if ("APAFML".equals(codeString)) 9201 return new Enumeration<SPDXLicense>(this, SPDXLicense.APAFML, code); 9202 if ("APL-1.0".equals(codeString)) 9203 return new Enumeration<SPDXLicense>(this, SPDXLicense.APL_1_0, code); 9204 if ("App-s2p".equals(codeString)) 9205 return new Enumeration<SPDXLicense>(this, SPDXLicense.APP_S2P, code); 9206 if ("APSL-1.0".equals(codeString)) 9207 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_1_0, code); 9208 if ("APSL-1.1".equals(codeString)) 9209 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_1_1, code); 9210 if ("APSL-1.2".equals(codeString)) 9211 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_1_2, code); 9212 if ("APSL-2.0".equals(codeString)) 9213 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_2_0, code); 9214 if ("Arphic-1999".equals(codeString)) 9215 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARPHIC_1999, code); 9216 if ("Artistic-1.0".equals(codeString)) 9217 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_1_0, code); 9218 if ("Artistic-1.0-cl8".equals(codeString)) 9219 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_1_0_CL8, code); 9220 if ("Artistic-1.0-Perl".equals(codeString)) 9221 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_1_0_PERL, code); 9222 if ("Artistic-2.0".equals(codeString)) 9223 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_2_0, code); 9224 if ("ASWF-Digital-Assets-1.0".equals(codeString)) 9225 return new Enumeration<SPDXLicense>(this, SPDXLicense.ASWF_DIGITAL_ASSETS_1_0, code); 9226 if ("ASWF-Digital-Assets-1.1".equals(codeString)) 9227 return new Enumeration<SPDXLicense>(this, SPDXLicense.ASWF_DIGITAL_ASSETS_1_1, code); 9228 if ("Baekmuk".equals(codeString)) 9229 return new Enumeration<SPDXLicense>(this, SPDXLicense.BAEKMUK, code); 9230 if ("Bahyph".equals(codeString)) 9231 return new Enumeration<SPDXLicense>(this, SPDXLicense.BAHYPH, code); 9232 if ("Barr".equals(codeString)) 9233 return new Enumeration<SPDXLicense>(this, SPDXLicense.BARR, code); 9234 if ("Beerware".equals(codeString)) 9235 return new Enumeration<SPDXLicense>(this, SPDXLicense.BEERWARE, code); 9236 if ("Bitstream-Charter".equals(codeString)) 9237 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITSTREAM_CHARTER, code); 9238 if ("Bitstream-Vera".equals(codeString)) 9239 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITSTREAM_VERA, code); 9240 if ("BitTorrent-1.0".equals(codeString)) 9241 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITTORRENT_1_0, code); 9242 if ("BitTorrent-1.1".equals(codeString)) 9243 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITTORRENT_1_1, code); 9244 if ("blessing".equals(codeString)) 9245 return new Enumeration<SPDXLicense>(this, SPDXLicense.BLESSING, code); 9246 if ("BlueOak-1.0.0".equals(codeString)) 9247 return new Enumeration<SPDXLicense>(this, SPDXLicense.BLUEOAK_1_0_0, code); 9248 if ("Boehm-GC".equals(codeString)) 9249 return new Enumeration<SPDXLicense>(this, SPDXLicense.BOEHM_GC, code); 9250 if ("Borceux".equals(codeString)) 9251 return new Enumeration<SPDXLicense>(this, SPDXLicense.BORCEUX, code); 9252 if ("Brian-Gladman-3-Clause".equals(codeString)) 9253 return new Enumeration<SPDXLicense>(this, SPDXLicense.BRIAN_GLADMAN_3_CLAUSE, code); 9254 if ("BSD-1-Clause".equals(codeString)) 9255 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_1_CLAUSE, code); 9256 if ("BSD-2-Clause".equals(codeString)) 9257 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE, code); 9258 if ("BSD-2-Clause-FreeBSD".equals(codeString)) 9259 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_FREEBSD, code); 9260 if ("BSD-2-Clause-NetBSD".equals(codeString)) 9261 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_NETBSD, code); 9262 if ("BSD-2-Clause-Patent".equals(codeString)) 9263 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_PATENT, code); 9264 if ("BSD-2-Clause-Views".equals(codeString)) 9265 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_VIEWS, code); 9266 if ("BSD-3-Clause".equals(codeString)) 9267 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE, code); 9268 if ("BSD-3-Clause-Attribution".equals(codeString)) 9269 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_ATTRIBUTION, code); 9270 if ("BSD-3-Clause-Clear".equals(codeString)) 9271 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_CLEAR, code); 9272 if ("BSD-3-Clause-LBNL".equals(codeString)) 9273 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_LBNL, code); 9274 if ("BSD-3-Clause-Modification".equals(codeString)) 9275 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_MODIFICATION, code); 9276 if ("BSD-3-Clause-No-Military-License".equals(codeString)) 9277 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_MILITARY_LICENSE, code); 9278 if ("BSD-3-Clause-No-Nuclear-License".equals(codeString)) 9279 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE, code); 9280 if ("BSD-3-Clause-No-Nuclear-License-2014".equals(codeString)) 9281 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014, code); 9282 if ("BSD-3-Clause-No-Nuclear-Warranty".equals(codeString)) 9283 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY, code); 9284 if ("BSD-3-Clause-Open-MPI".equals(codeString)) 9285 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_OPEN_MPI, code); 9286 if ("BSD-4-Clause".equals(codeString)) 9287 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_CLAUSE, code); 9288 if ("BSD-4-Clause-Shortened".equals(codeString)) 9289 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_CLAUSE_SHORTENED, code); 9290 if ("BSD-4-Clause-UC".equals(codeString)) 9291 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_CLAUSE_UC, code); 9292 if ("BSD-4.3RENO".equals(codeString)) 9293 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_3RENO, code); 9294 if ("BSD-4.3TAHOE".equals(codeString)) 9295 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_3TAHOE, code); 9296 if ("BSD-Advertising-Acknowledgement".equals(codeString)) 9297 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_ADVERTISING_ACKNOWLEDGEMENT, code); 9298 if ("BSD-Attribution-HPND-disclaimer".equals(codeString)) 9299 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_ATTRIBUTION_HPND_DISCLAIMER, code); 9300 if ("BSD-Protection".equals(codeString)) 9301 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_PROTECTION, code); 9302 if ("BSD-Source-Code".equals(codeString)) 9303 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_SOURCE_CODE, code); 9304 if ("BSL-1.0".equals(codeString)) 9305 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSL_1_0, code); 9306 if ("BUSL-1.1".equals(codeString)) 9307 return new Enumeration<SPDXLicense>(this, SPDXLicense.BUSL_1_1, code); 9308 if ("bzip2-1.0.5".equals(codeString)) 9309 return new Enumeration<SPDXLicense>(this, SPDXLicense.BZIP2_1_0_5, code); 9310 if ("bzip2-1.0.6".equals(codeString)) 9311 return new Enumeration<SPDXLicense>(this, SPDXLicense.BZIP2_1_0_6, code); 9312 if ("C-UDA-1.0".equals(codeString)) 9313 return new Enumeration<SPDXLicense>(this, SPDXLicense.C_UDA_1_0, code); 9314 if ("CAL-1.0".equals(codeString)) 9315 return new Enumeration<SPDXLicense>(this, SPDXLicense.CAL_1_0, code); 9316 if ("CAL-1.0-Combined-Work-Exception".equals(codeString)) 9317 return new Enumeration<SPDXLicense>(this, SPDXLicense.CAL_1_0_COMBINED_WORK_EXCEPTION, code); 9318 if ("Caldera".equals(codeString)) 9319 return new Enumeration<SPDXLicense>(this, SPDXLicense.CALDERA, code); 9320 if ("CATOSL-1.1".equals(codeString)) 9321 return new Enumeration<SPDXLicense>(this, SPDXLicense.CATOSL_1_1, code); 9322 if ("CC-BY-1.0".equals(codeString)) 9323 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_1_0, code); 9324 if ("CC-BY-2.0".equals(codeString)) 9325 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_2_0, code); 9326 if ("CC-BY-2.5".equals(codeString)) 9327 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_2_5, code); 9328 if ("CC-BY-2.5-AU".equals(codeString)) 9329 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_2_5_AU, code); 9330 if ("CC-BY-3.0".equals(codeString)) 9331 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0, code); 9332 if ("CC-BY-3.0-AT".equals(codeString)) 9333 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_AT, code); 9334 if ("CC-BY-3.0-DE".equals(codeString)) 9335 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_DE, code); 9336 if ("CC-BY-3.0-IGO".equals(codeString)) 9337 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_IGO, code); 9338 if ("CC-BY-3.0-NL".equals(codeString)) 9339 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_NL, code); 9340 if ("CC-BY-3.0-US".equals(codeString)) 9341 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_US, code); 9342 if ("CC-BY-4.0".equals(codeString)) 9343 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_4_0, code); 9344 if ("CC-BY-NC-1.0".equals(codeString)) 9345 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_1_0, code); 9346 if ("CC-BY-NC-2.0".equals(codeString)) 9347 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_2_0, code); 9348 if ("CC-BY-NC-2.5".equals(codeString)) 9349 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_2_5, code); 9350 if ("CC-BY-NC-3.0".equals(codeString)) 9351 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_3_0, code); 9352 if ("CC-BY-NC-3.0-DE".equals(codeString)) 9353 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_3_0_DE, code); 9354 if ("CC-BY-NC-4.0".equals(codeString)) 9355 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_4_0, code); 9356 if ("CC-BY-NC-ND-1.0".equals(codeString)) 9357 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_1_0, code); 9358 if ("CC-BY-NC-ND-2.0".equals(codeString)) 9359 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_2_0, code); 9360 if ("CC-BY-NC-ND-2.5".equals(codeString)) 9361 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_2_5, code); 9362 if ("CC-BY-NC-ND-3.0".equals(codeString)) 9363 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_3_0, code); 9364 if ("CC-BY-NC-ND-3.0-DE".equals(codeString)) 9365 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_3_0_DE, code); 9366 if ("CC-BY-NC-ND-3.0-IGO".equals(codeString)) 9367 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_3_0_IGO, code); 9368 if ("CC-BY-NC-ND-4.0".equals(codeString)) 9369 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_4_0, code); 9370 if ("CC-BY-NC-SA-1.0".equals(codeString)) 9371 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_1_0, code); 9372 if ("CC-BY-NC-SA-2.0".equals(codeString)) 9373 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0, code); 9374 if ("CC-BY-NC-SA-2.0-DE".equals(codeString)) 9375 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0_DE, code); 9376 if ("CC-BY-NC-SA-2.0-FR".equals(codeString)) 9377 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0_FR, code); 9378 if ("CC-BY-NC-SA-2.0-UK".equals(codeString)) 9379 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0_UK, code); 9380 if ("CC-BY-NC-SA-2.5".equals(codeString)) 9381 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_5, code); 9382 if ("CC-BY-NC-SA-3.0".equals(codeString)) 9383 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_3_0, code); 9384 if ("CC-BY-NC-SA-3.0-DE".equals(codeString)) 9385 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_3_0_DE, code); 9386 if ("CC-BY-NC-SA-3.0-IGO".equals(codeString)) 9387 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_3_0_IGO, code); 9388 if ("CC-BY-NC-SA-4.0".equals(codeString)) 9389 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_4_0, code); 9390 if ("CC-BY-ND-1.0".equals(codeString)) 9391 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_1_0, code); 9392 if ("CC-BY-ND-2.0".equals(codeString)) 9393 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_2_0, code); 9394 if ("CC-BY-ND-2.5".equals(codeString)) 9395 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_2_5, code); 9396 if ("CC-BY-ND-3.0".equals(codeString)) 9397 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_3_0, code); 9398 if ("CC-BY-ND-3.0-DE".equals(codeString)) 9399 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_3_0_DE, code); 9400 if ("CC-BY-ND-4.0".equals(codeString)) 9401 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_4_0, code); 9402 if ("CC-BY-SA-1.0".equals(codeString)) 9403 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_1_0, code); 9404 if ("CC-BY-SA-2.0".equals(codeString)) 9405 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_0, code); 9406 if ("CC-BY-SA-2.0-UK".equals(codeString)) 9407 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_0_UK, code); 9408 if ("CC-BY-SA-2.1-JP".equals(codeString)) 9409 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_1_JP, code); 9410 if ("CC-BY-SA-2.5".equals(codeString)) 9411 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_5, code); 9412 if ("CC-BY-SA-3.0".equals(codeString)) 9413 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0, code); 9414 if ("CC-BY-SA-3.0-AT".equals(codeString)) 9415 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0_AT, code); 9416 if ("CC-BY-SA-3.0-DE".equals(codeString)) 9417 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0_DE, code); 9418 if ("CC-BY-SA-3.0-IGO".equals(codeString)) 9419 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0_IGO, code); 9420 if ("CC-BY-SA-4.0".equals(codeString)) 9421 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_4_0, code); 9422 if ("CC-PDDC".equals(codeString)) 9423 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_PDDC, code); 9424 if ("CC0-1.0".equals(codeString)) 9425 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC0_1_0, code); 9426 if ("CDDL-1.0".equals(codeString)) 9427 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDDL_1_0, code); 9428 if ("CDDL-1.1".equals(codeString)) 9429 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDDL_1_1, code); 9430 if ("CDL-1.0".equals(codeString)) 9431 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDL_1_0, code); 9432 if ("CDLA-Permissive-1.0".equals(codeString)) 9433 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDLA_PERMISSIVE_1_0, code); 9434 if ("CDLA-Permissive-2.0".equals(codeString)) 9435 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDLA_PERMISSIVE_2_0, code); 9436 if ("CDLA-Sharing-1.0".equals(codeString)) 9437 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDLA_SHARING_1_0, code); 9438 if ("CECILL-1.0".equals(codeString)) 9439 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_1_0, code); 9440 if ("CECILL-1.1".equals(codeString)) 9441 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_1_1, code); 9442 if ("CECILL-2.0".equals(codeString)) 9443 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_2_0, code); 9444 if ("CECILL-2.1".equals(codeString)) 9445 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_2_1, code); 9446 if ("CECILL-B".equals(codeString)) 9447 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_B, code); 9448 if ("CECILL-C".equals(codeString)) 9449 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_C, code); 9450 if ("CERN-OHL-1.1".equals(codeString)) 9451 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_1_1, code); 9452 if ("CERN-OHL-1.2".equals(codeString)) 9453 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_1_2, code); 9454 if ("CERN-OHL-P-2.0".equals(codeString)) 9455 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_P_2_0, code); 9456 if ("CERN-OHL-S-2.0".equals(codeString)) 9457 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_S_2_0, code); 9458 if ("CERN-OHL-W-2.0".equals(codeString)) 9459 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_W_2_0, code); 9460 if ("CFITSIO".equals(codeString)) 9461 return new Enumeration<SPDXLicense>(this, SPDXLicense.CFITSIO, code); 9462 if ("checkmk".equals(codeString)) 9463 return new Enumeration<SPDXLicense>(this, SPDXLicense.CHECKMK, code); 9464 if ("ClArtistic".equals(codeString)) 9465 return new Enumeration<SPDXLicense>(this, SPDXLicense.CLARTISTIC, code); 9466 if ("Clips".equals(codeString)) 9467 return new Enumeration<SPDXLicense>(this, SPDXLicense.CLIPS, code); 9468 if ("CMU-Mach".equals(codeString)) 9469 return new Enumeration<SPDXLicense>(this, SPDXLicense.CMU_MACH, code); 9470 if ("CNRI-Jython".equals(codeString)) 9471 return new Enumeration<SPDXLicense>(this, SPDXLicense.CNRI_JYTHON, code); 9472 if ("CNRI-Python".equals(codeString)) 9473 return new Enumeration<SPDXLicense>(this, SPDXLicense.CNRI_PYTHON, code); 9474 if ("CNRI-Python-GPL-Compatible".equals(codeString)) 9475 return new Enumeration<SPDXLicense>(this, SPDXLicense.CNRI_PYTHON_GPL_COMPATIBLE, code); 9476 if ("COIL-1.0".equals(codeString)) 9477 return new Enumeration<SPDXLicense>(this, SPDXLicense.COIL_1_0, code); 9478 if ("Community-Spec-1.0".equals(codeString)) 9479 return new Enumeration<SPDXLicense>(this, SPDXLicense.COMMUNITY_SPEC_1_0, code); 9480 if ("Condor-1.1".equals(codeString)) 9481 return new Enumeration<SPDXLicense>(this, SPDXLicense.CONDOR_1_1, code); 9482 if ("copyleft-next-0.3.0".equals(codeString)) 9483 return new Enumeration<SPDXLicense>(this, SPDXLicense.COPYLEFT_NEXT_0_3_0, code); 9484 if ("copyleft-next-0.3.1".equals(codeString)) 9485 return new Enumeration<SPDXLicense>(this, SPDXLicense.COPYLEFT_NEXT_0_3_1, code); 9486 if ("Cornell-Lossless-JPEG".equals(codeString)) 9487 return new Enumeration<SPDXLicense>(this, SPDXLicense.CORNELL_LOSSLESS_JPEG, code); 9488 if ("CPAL-1.0".equals(codeString)) 9489 return new Enumeration<SPDXLicense>(this, SPDXLicense.CPAL_1_0, code); 9490 if ("CPL-1.0".equals(codeString)) 9491 return new Enumeration<SPDXLicense>(this, SPDXLicense.CPL_1_0, code); 9492 if ("CPOL-1.02".equals(codeString)) 9493 return new Enumeration<SPDXLicense>(this, SPDXLicense.CPOL_1_02, code); 9494 if ("Crossword".equals(codeString)) 9495 return new Enumeration<SPDXLicense>(this, SPDXLicense.CROSSWORD, code); 9496 if ("CrystalStacker".equals(codeString)) 9497 return new Enumeration<SPDXLicense>(this, SPDXLicense.CRYSTALSTACKER, code); 9498 if ("CUA-OPL-1.0".equals(codeString)) 9499 return new Enumeration<SPDXLicense>(this, SPDXLicense.CUA_OPL_1_0, code); 9500 if ("Cube".equals(codeString)) 9501 return new Enumeration<SPDXLicense>(this, SPDXLicense.CUBE, code); 9502 if ("curl".equals(codeString)) 9503 return new Enumeration<SPDXLicense>(this, SPDXLicense.CURL, code); 9504 if ("D-FSL-1.0".equals(codeString)) 9505 return new Enumeration<SPDXLicense>(this, SPDXLicense.D_FSL_1_0, code); 9506 if ("diffmark".equals(codeString)) 9507 return new Enumeration<SPDXLicense>(this, SPDXLicense.DIFFMARK, code); 9508 if ("DL-DE-BY-2.0".equals(codeString)) 9509 return new Enumeration<SPDXLicense>(this, SPDXLicense.DL_DE_BY_2_0, code); 9510 if ("DOC".equals(codeString)) 9511 return new Enumeration<SPDXLicense>(this, SPDXLicense.DOC, code); 9512 if ("Dotseqn".equals(codeString)) 9513 return new Enumeration<SPDXLicense>(this, SPDXLicense.DOTSEQN, code); 9514 if ("DRL-1.0".equals(codeString)) 9515 return new Enumeration<SPDXLicense>(this, SPDXLicense.DRL_1_0, code); 9516 if ("DSDP".equals(codeString)) 9517 return new Enumeration<SPDXLicense>(this, SPDXLicense.DSDP, code); 9518 if ("dtoa".equals(codeString)) 9519 return new Enumeration<SPDXLicense>(this, SPDXLicense.DTOA, code); 9520 if ("dvipdfm".equals(codeString)) 9521 return new Enumeration<SPDXLicense>(this, SPDXLicense.DVIPDFM, code); 9522 if ("ECL-1.0".equals(codeString)) 9523 return new Enumeration<SPDXLicense>(this, SPDXLicense.ECL_1_0, code); 9524 if ("ECL-2.0".equals(codeString)) 9525 return new Enumeration<SPDXLicense>(this, SPDXLicense.ECL_2_0, code); 9526 if ("eCos-2.0".equals(codeString)) 9527 return new Enumeration<SPDXLicense>(this, SPDXLicense.ECOS_2_0, code); 9528 if ("EFL-1.0".equals(codeString)) 9529 return new Enumeration<SPDXLicense>(this, SPDXLicense.EFL_1_0, code); 9530 if ("EFL-2.0".equals(codeString)) 9531 return new Enumeration<SPDXLicense>(this, SPDXLicense.EFL_2_0, code); 9532 if ("eGenix".equals(codeString)) 9533 return new Enumeration<SPDXLicense>(this, SPDXLicense.EGENIX, code); 9534 if ("Elastic-2.0".equals(codeString)) 9535 return new Enumeration<SPDXLicense>(this, SPDXLicense.ELASTIC_2_0, code); 9536 if ("Entessa".equals(codeString)) 9537 return new Enumeration<SPDXLicense>(this, SPDXLicense.ENTESSA, code); 9538 if ("EPICS".equals(codeString)) 9539 return new Enumeration<SPDXLicense>(this, SPDXLicense.EPICS, code); 9540 if ("EPL-1.0".equals(codeString)) 9541 return new Enumeration<SPDXLicense>(this, SPDXLicense.EPL_1_0, code); 9542 if ("EPL-2.0".equals(codeString)) 9543 return new Enumeration<SPDXLicense>(this, SPDXLicense.EPL_2_0, code); 9544 if ("ErlPL-1.1".equals(codeString)) 9545 return new Enumeration<SPDXLicense>(this, SPDXLicense.ERLPL_1_1, code); 9546 if ("etalab-2.0".equals(codeString)) 9547 return new Enumeration<SPDXLicense>(this, SPDXLicense.ETALAB_2_0, code); 9548 if ("EUDatagrid".equals(codeString)) 9549 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUDATAGRID, code); 9550 if ("EUPL-1.0".equals(codeString)) 9551 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUPL_1_0, code); 9552 if ("EUPL-1.1".equals(codeString)) 9553 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUPL_1_1, code); 9554 if ("EUPL-1.2".equals(codeString)) 9555 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUPL_1_2, code); 9556 if ("Eurosym".equals(codeString)) 9557 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUROSYM, code); 9558 if ("Fair".equals(codeString)) 9559 return new Enumeration<SPDXLicense>(this, SPDXLicense.FAIR, code); 9560 if ("FDK-AAC".equals(codeString)) 9561 return new Enumeration<SPDXLicense>(this, SPDXLicense.FDK_AAC, code); 9562 if ("Frameworx-1.0".equals(codeString)) 9563 return new Enumeration<SPDXLicense>(this, SPDXLicense.FRAMEWORX_1_0, code); 9564 if ("FreeBSD-DOC".equals(codeString)) 9565 return new Enumeration<SPDXLicense>(this, SPDXLicense.FREEBSD_DOC, code); 9566 if ("FreeImage".equals(codeString)) 9567 return new Enumeration<SPDXLicense>(this, SPDXLicense.FREEIMAGE, code); 9568 if ("FSFAP".equals(codeString)) 9569 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFAP, code); 9570 if ("FSFUL".equals(codeString)) 9571 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFUL, code); 9572 if ("FSFULLR".equals(codeString)) 9573 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFULLR, code); 9574 if ("FSFULLRWD".equals(codeString)) 9575 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFULLRWD, code); 9576 if ("FTL".equals(codeString)) 9577 return new Enumeration<SPDXLicense>(this, SPDXLicense.FTL, code); 9578 if ("GD".equals(codeString)) 9579 return new Enumeration<SPDXLicense>(this, SPDXLicense.GD, code); 9580 if ("GFDL-1.1".equals(codeString)) 9581 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1, code); 9582 if ("GFDL-1.1-invariants-only".equals(codeString)) 9583 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_INVARIANTS_ONLY, code); 9584 if ("GFDL-1.1-invariants-or-later".equals(codeString)) 9585 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_INVARIANTS_OR_LATER, code); 9586 if ("GFDL-1.1-no-invariants-only".equals(codeString)) 9587 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_NO_INVARIANTS_ONLY, code); 9588 if ("GFDL-1.1-no-invariants-or-later".equals(codeString)) 9589 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_NO_INVARIANTS_OR_LATER, code); 9590 if ("GFDL-1.1-only".equals(codeString)) 9591 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_ONLY, code); 9592 if ("GFDL-1.1-or-later".equals(codeString)) 9593 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_OR_LATER, code); 9594 if ("GFDL-1.2".equals(codeString)) 9595 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2, code); 9596 if ("GFDL-1.2-invariants-only".equals(codeString)) 9597 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_INVARIANTS_ONLY, code); 9598 if ("GFDL-1.2-invariants-or-later".equals(codeString)) 9599 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_INVARIANTS_OR_LATER, code); 9600 if ("GFDL-1.2-no-invariants-only".equals(codeString)) 9601 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_NO_INVARIANTS_ONLY, code); 9602 if ("GFDL-1.2-no-invariants-or-later".equals(codeString)) 9603 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_NO_INVARIANTS_OR_LATER, code); 9604 if ("GFDL-1.2-only".equals(codeString)) 9605 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_ONLY, code); 9606 if ("GFDL-1.2-or-later".equals(codeString)) 9607 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_OR_LATER, code); 9608 if ("GFDL-1.3".equals(codeString)) 9609 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3, code); 9610 if ("GFDL-1.3-invariants-only".equals(codeString)) 9611 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_INVARIANTS_ONLY, code); 9612 if ("GFDL-1.3-invariants-or-later".equals(codeString)) 9613 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_INVARIANTS_OR_LATER, code); 9614 if ("GFDL-1.3-no-invariants-only".equals(codeString)) 9615 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_NO_INVARIANTS_ONLY, code); 9616 if ("GFDL-1.3-no-invariants-or-later".equals(codeString)) 9617 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_NO_INVARIANTS_OR_LATER, code); 9618 if ("GFDL-1.3-only".equals(codeString)) 9619 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_ONLY, code); 9620 if ("GFDL-1.3-or-later".equals(codeString)) 9621 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_OR_LATER, code); 9622 if ("Giftware".equals(codeString)) 9623 return new Enumeration<SPDXLicense>(this, SPDXLicense.GIFTWARE, code); 9624 if ("GL2PS".equals(codeString)) 9625 return new Enumeration<SPDXLicense>(this, SPDXLicense.GL2PS, code); 9626 if ("Glide".equals(codeString)) 9627 return new Enumeration<SPDXLicense>(this, SPDXLicense.GLIDE, code); 9628 if ("Glulxe".equals(codeString)) 9629 return new Enumeration<SPDXLicense>(this, SPDXLicense.GLULXE, code); 9630 if ("GLWTPL".equals(codeString)) 9631 return new Enumeration<SPDXLicense>(this, SPDXLicense.GLWTPL, code); 9632 if ("gnuplot".equals(codeString)) 9633 return new Enumeration<SPDXLicense>(this, SPDXLicense.GNUPLOT, code); 9634 if ("GPL-1.0".equals(codeString)) 9635 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0, code); 9636 if ("GPL-1.0+".equals(codeString)) 9637 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0PLUS, code); 9638 if ("GPL-1.0-only".equals(codeString)) 9639 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0_ONLY, code); 9640 if ("GPL-1.0-or-later".equals(codeString)) 9641 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0_OR_LATER, code); 9642 if ("GPL-2.0".equals(codeString)) 9643 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0, code); 9644 if ("GPL-2.0+".equals(codeString)) 9645 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0PLUS, code); 9646 if ("GPL-2.0-only".equals(codeString)) 9647 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_ONLY, code); 9648 if ("GPL-2.0-or-later".equals(codeString)) 9649 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_OR_LATER, code); 9650 if ("GPL-2.0-with-autoconf-exception".equals(codeString)) 9651 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_AUTOCONF_EXCEPTION, code); 9652 if ("GPL-2.0-with-bison-exception".equals(codeString)) 9653 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_BISON_EXCEPTION, code); 9654 if ("GPL-2.0-with-classpath-exception".equals(codeString)) 9655 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_CLASSPATH_EXCEPTION, code); 9656 if ("GPL-2.0-with-font-exception".equals(codeString)) 9657 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_FONT_EXCEPTION, code); 9658 if ("GPL-2.0-with-GCC-exception".equals(codeString)) 9659 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_GCC_EXCEPTION, code); 9660 if ("GPL-3.0".equals(codeString)) 9661 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0, code); 9662 if ("GPL-3.0+".equals(codeString)) 9663 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0PLUS, code); 9664 if ("GPL-3.0-only".equals(codeString)) 9665 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_ONLY, code); 9666 if ("GPL-3.0-or-later".equals(codeString)) 9667 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_OR_LATER, code); 9668 if ("GPL-3.0-with-autoconf-exception".equals(codeString)) 9669 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_WITH_AUTOCONF_EXCEPTION, code); 9670 if ("GPL-3.0-with-GCC-exception".equals(codeString)) 9671 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_WITH_GCC_EXCEPTION, code); 9672 if ("Graphics-Gems".equals(codeString)) 9673 return new Enumeration<SPDXLicense>(this, SPDXLicense.GRAPHICS_GEMS, code); 9674 if ("gSOAP-1.3b".equals(codeString)) 9675 return new Enumeration<SPDXLicense>(this, SPDXLicense.GSOAP_1_3B, code); 9676 if ("HaskellReport".equals(codeString)) 9677 return new Enumeration<SPDXLicense>(this, SPDXLicense.HASKELLREPORT, code); 9678 if ("Hippocratic-2.1".equals(codeString)) 9679 return new Enumeration<SPDXLicense>(this, SPDXLicense.HIPPOCRATIC_2_1, code); 9680 if ("HP-1986".equals(codeString)) 9681 return new Enumeration<SPDXLicense>(this, SPDXLicense.HP_1986, code); 9682 if ("HPND".equals(codeString)) 9683 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND, code); 9684 if ("HPND-export-US".equals(codeString)) 9685 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_EXPORT_US, code); 9686 if ("HPND-Markus-Kuhn".equals(codeString)) 9687 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_MARKUS_KUHN, code); 9688 if ("HPND-sell-variant".equals(codeString)) 9689 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_SELL_VARIANT, code); 9690 if ("HPND-sell-variant-MIT-disclaimer".equals(codeString)) 9691 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_SELL_VARIANT_MIT_DISCLAIMER, code); 9692 if ("HTMLTIDY".equals(codeString)) 9693 return new Enumeration<SPDXLicense>(this, SPDXLicense.HTMLTIDY, code); 9694 if ("IBM-pibs".equals(codeString)) 9695 return new Enumeration<SPDXLicense>(this, SPDXLicense.IBM_PIBS, code); 9696 if ("ICU".equals(codeString)) 9697 return new Enumeration<SPDXLicense>(this, SPDXLicense.ICU, code); 9698 if ("IEC-Code-Components-EULA".equals(codeString)) 9699 return new Enumeration<SPDXLicense>(this, SPDXLicense.IEC_CODE_COMPONENTS_EULA, code); 9700 if ("IJG".equals(codeString)) 9701 return new Enumeration<SPDXLicense>(this, SPDXLicense.IJG, code); 9702 if ("IJG-short".equals(codeString)) 9703 return new Enumeration<SPDXLicense>(this, SPDXLicense.IJG_SHORT, code); 9704 if ("ImageMagick".equals(codeString)) 9705 return new Enumeration<SPDXLicense>(this, SPDXLicense.IMAGEMAGICK, code); 9706 if ("iMatix".equals(codeString)) 9707 return new Enumeration<SPDXLicense>(this, SPDXLicense.IMATIX, code); 9708 if ("Imlib2".equals(codeString)) 9709 return new Enumeration<SPDXLicense>(this, SPDXLicense.IMLIB2, code); 9710 if ("Info-ZIP".equals(codeString)) 9711 return new Enumeration<SPDXLicense>(this, SPDXLicense.INFO_ZIP, code); 9712 if ("Inner-Net-2.0".equals(codeString)) 9713 return new Enumeration<SPDXLicense>(this, SPDXLicense.INNER_NET_2_0, code); 9714 if ("Intel".equals(codeString)) 9715 return new Enumeration<SPDXLicense>(this, SPDXLicense.INTEL, code); 9716 if ("Intel-ACPI".equals(codeString)) 9717 return new Enumeration<SPDXLicense>(this, SPDXLicense.INTEL_ACPI, code); 9718 if ("Interbase-1.0".equals(codeString)) 9719 return new Enumeration<SPDXLicense>(this, SPDXLicense.INTERBASE_1_0, code); 9720 if ("IPA".equals(codeString)) 9721 return new Enumeration<SPDXLicense>(this, SPDXLicense.IPA, code); 9722 if ("IPL-1.0".equals(codeString)) 9723 return new Enumeration<SPDXLicense>(this, SPDXLicense.IPL_1_0, code); 9724 if ("ISC".equals(codeString)) 9725 return new Enumeration<SPDXLicense>(this, SPDXLicense.ISC, code); 9726 if ("Jam".equals(codeString)) 9727 return new Enumeration<SPDXLicense>(this, SPDXLicense.JAM, code); 9728 if ("JasPer-2.0".equals(codeString)) 9729 return new Enumeration<SPDXLicense>(this, SPDXLicense.JASPER_2_0, code); 9730 if ("JPL-image".equals(codeString)) 9731 return new Enumeration<SPDXLicense>(this, SPDXLicense.JPL_IMAGE, code); 9732 if ("JPNIC".equals(codeString)) 9733 return new Enumeration<SPDXLicense>(this, SPDXLicense.JPNIC, code); 9734 if ("JSON".equals(codeString)) 9735 return new Enumeration<SPDXLicense>(this, SPDXLicense.JSON, code); 9736 if ("Kazlib".equals(codeString)) 9737 return new Enumeration<SPDXLicense>(this, SPDXLicense.KAZLIB, code); 9738 if ("Knuth-CTAN".equals(codeString)) 9739 return new Enumeration<SPDXLicense>(this, SPDXLicense.KNUTH_CTAN, code); 9740 if ("LAL-1.2".equals(codeString)) 9741 return new Enumeration<SPDXLicense>(this, SPDXLicense.LAL_1_2, code); 9742 if ("LAL-1.3".equals(codeString)) 9743 return new Enumeration<SPDXLicense>(this, SPDXLicense.LAL_1_3, code); 9744 if ("Latex2e".equals(codeString)) 9745 return new Enumeration<SPDXLicense>(this, SPDXLicense.LATEX2E, code); 9746 if ("Latex2e-translated-notice".equals(codeString)) 9747 return new Enumeration<SPDXLicense>(this, SPDXLicense.LATEX2E_TRANSLATED_NOTICE, code); 9748 if ("Leptonica".equals(codeString)) 9749 return new Enumeration<SPDXLicense>(this, SPDXLicense.LEPTONICA, code); 9750 if ("LGPL-2.0".equals(codeString)) 9751 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0, code); 9752 if ("LGPL-2.0+".equals(codeString)) 9753 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0PLUS, code); 9754 if ("LGPL-2.0-only".equals(codeString)) 9755 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0_ONLY, code); 9756 if ("LGPL-2.0-or-later".equals(codeString)) 9757 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0_OR_LATER, code); 9758 if ("LGPL-2.1".equals(codeString)) 9759 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1, code); 9760 if ("LGPL-2.1+".equals(codeString)) 9761 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1PLUS, code); 9762 if ("LGPL-2.1-only".equals(codeString)) 9763 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1_ONLY, code); 9764 if ("LGPL-2.1-or-later".equals(codeString)) 9765 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1_OR_LATER, code); 9766 if ("LGPL-3.0".equals(codeString)) 9767 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0, code); 9768 if ("LGPL-3.0+".equals(codeString)) 9769 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0PLUS, code); 9770 if ("LGPL-3.0-only".equals(codeString)) 9771 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0_ONLY, code); 9772 if ("LGPL-3.0-or-later".equals(codeString)) 9773 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0_OR_LATER, code); 9774 if ("LGPLLR".equals(codeString)) 9775 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPLLR, code); 9776 if ("Libpng".equals(codeString)) 9777 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBPNG, code); 9778 if ("libpng-2.0".equals(codeString)) 9779 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBPNG_2_0, code); 9780 if ("libselinux-1.0".equals(codeString)) 9781 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBSELINUX_1_0, code); 9782 if ("libtiff".equals(codeString)) 9783 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBTIFF, code); 9784 if ("libutil-David-Nugent".equals(codeString)) 9785 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBUTIL_DAVID_NUGENT, code); 9786 if ("LiLiQ-P-1.1".equals(codeString)) 9787 return new Enumeration<SPDXLicense>(this, SPDXLicense.LILIQ_P_1_1, code); 9788 if ("LiLiQ-R-1.1".equals(codeString)) 9789 return new Enumeration<SPDXLicense>(this, SPDXLicense.LILIQ_R_1_1, code); 9790 if ("LiLiQ-Rplus-1.1".equals(codeString)) 9791 return new Enumeration<SPDXLicense>(this, SPDXLicense.LILIQ_RPLUS_1_1, code); 9792 if ("Linux-man-pages-1-para".equals(codeString)) 9793 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_1_PARA, code); 9794 if ("Linux-man-pages-copyleft".equals(codeString)) 9795 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_COPYLEFT, code); 9796 if ("Linux-man-pages-copyleft-2-para".equals(codeString)) 9797 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_2_PARA, code); 9798 if ("Linux-man-pages-copyleft-var".equals(codeString)) 9799 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_VAR, code); 9800 if ("Linux-OpenIB".equals(codeString)) 9801 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_OPENIB, code); 9802 if ("LOOP".equals(codeString)) 9803 return new Enumeration<SPDXLicense>(this, SPDXLicense.LOOP, code); 9804 if ("LPL-1.0".equals(codeString)) 9805 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPL_1_0, code); 9806 if ("LPL-1.02".equals(codeString)) 9807 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPL_1_02, code); 9808 if ("LPPL-1.0".equals(codeString)) 9809 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_0, code); 9810 if ("LPPL-1.1".equals(codeString)) 9811 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_1, code); 9812 if ("LPPL-1.2".equals(codeString)) 9813 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_2, code); 9814 if ("LPPL-1.3a".equals(codeString)) 9815 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_3A, code); 9816 if ("LPPL-1.3c".equals(codeString)) 9817 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_3C, code); 9818 if ("LZMA-SDK-9.11-to-9.20".equals(codeString)) 9819 return new Enumeration<SPDXLicense>(this, SPDXLicense.LZMA_SDK_9_11_TO_9_20, code); 9820 if ("LZMA-SDK-9.22".equals(codeString)) 9821 return new Enumeration<SPDXLicense>(this, SPDXLicense.LZMA_SDK_9_22, code); 9822 if ("MakeIndex".equals(codeString)) 9823 return new Enumeration<SPDXLicense>(this, SPDXLicense.MAKEINDEX, code); 9824 if ("Martin-Birgmeier".equals(codeString)) 9825 return new Enumeration<SPDXLicense>(this, SPDXLicense.MARTIN_BIRGMEIER, code); 9826 if ("metamail".equals(codeString)) 9827 return new Enumeration<SPDXLicense>(this, SPDXLicense.METAMAIL, code); 9828 if ("Minpack".equals(codeString)) 9829 return new Enumeration<SPDXLicense>(this, SPDXLicense.MINPACK, code); 9830 if ("MirOS".equals(codeString)) 9831 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIROS, code); 9832 if ("MIT".equals(codeString)) 9833 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT, code); 9834 if ("MIT-0".equals(codeString)) 9835 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_0, code); 9836 if ("MIT-advertising".equals(codeString)) 9837 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_ADVERTISING, code); 9838 if ("MIT-CMU".equals(codeString)) 9839 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_CMU, code); 9840 if ("MIT-enna".equals(codeString)) 9841 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_ENNA, code); 9842 if ("MIT-feh".equals(codeString)) 9843 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_FEH, code); 9844 if ("MIT-Festival".equals(codeString)) 9845 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_FESTIVAL, code); 9846 if ("MIT-Modern-Variant".equals(codeString)) 9847 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_MODERN_VARIANT, code); 9848 if ("MIT-open-group".equals(codeString)) 9849 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_OPEN_GROUP, code); 9850 if ("MIT-Wu".equals(codeString)) 9851 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_WU, code); 9852 if ("MITNFA".equals(codeString)) 9853 return new Enumeration<SPDXLicense>(this, SPDXLicense.MITNFA, code); 9854 if ("Motosoto".equals(codeString)) 9855 return new Enumeration<SPDXLicense>(this, SPDXLicense.MOTOSOTO, code); 9856 if ("mpi-permissive".equals(codeString)) 9857 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPI_PERMISSIVE, code); 9858 if ("mpich2".equals(codeString)) 9859 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPICH2, code); 9860 if ("MPL-1.0".equals(codeString)) 9861 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_1_0, code); 9862 if ("MPL-1.1".equals(codeString)) 9863 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_1_1, code); 9864 if ("MPL-2.0".equals(codeString)) 9865 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_2_0, code); 9866 if ("MPL-2.0-no-copyleft-exception".equals(codeString)) 9867 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_2_0_NO_COPYLEFT_EXCEPTION, code); 9868 if ("mplus".equals(codeString)) 9869 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPLUS, code); 9870 if ("MS-LPL".equals(codeString)) 9871 return new Enumeration<SPDXLicense>(this, SPDXLicense.MS_LPL, code); 9872 if ("MS-PL".equals(codeString)) 9873 return new Enumeration<SPDXLicense>(this, SPDXLicense.MS_PL, code); 9874 if ("MS-RL".equals(codeString)) 9875 return new Enumeration<SPDXLicense>(this, SPDXLicense.MS_RL, code); 9876 if ("MTLL".equals(codeString)) 9877 return new Enumeration<SPDXLicense>(this, SPDXLicense.MTLL, code); 9878 if ("MulanPSL-1.0".equals(codeString)) 9879 return new Enumeration<SPDXLicense>(this, SPDXLicense.MULANPSL_1_0, code); 9880 if ("MulanPSL-2.0".equals(codeString)) 9881 return new Enumeration<SPDXLicense>(this, SPDXLicense.MULANPSL_2_0, code); 9882 if ("Multics".equals(codeString)) 9883 return new Enumeration<SPDXLicense>(this, SPDXLicense.MULTICS, code); 9884 if ("Mup".equals(codeString)) 9885 return new Enumeration<SPDXLicense>(this, SPDXLicense.MUP, code); 9886 if ("NAIST-2003".equals(codeString)) 9887 return new Enumeration<SPDXLicense>(this, SPDXLicense.NAIST_2003, code); 9888 if ("NASA-1.3".equals(codeString)) 9889 return new Enumeration<SPDXLicense>(this, SPDXLicense.NASA_1_3, code); 9890 if ("Naumen".equals(codeString)) 9891 return new Enumeration<SPDXLicense>(this, SPDXLicense.NAUMEN, code); 9892 if ("NBPL-1.0".equals(codeString)) 9893 return new Enumeration<SPDXLicense>(this, SPDXLicense.NBPL_1_0, code); 9894 if ("NCGL-UK-2.0".equals(codeString)) 9895 return new Enumeration<SPDXLicense>(this, SPDXLicense.NCGL_UK_2_0, code); 9896 if ("NCSA".equals(codeString)) 9897 return new Enumeration<SPDXLicense>(this, SPDXLicense.NCSA, code); 9898 if ("Net-SNMP".equals(codeString)) 9899 return new Enumeration<SPDXLicense>(this, SPDXLicense.NET_SNMP, code); 9900 if ("NetCDF".equals(codeString)) 9901 return new Enumeration<SPDXLicense>(this, SPDXLicense.NETCDF, code); 9902 if ("Newsletr".equals(codeString)) 9903 return new Enumeration<SPDXLicense>(this, SPDXLicense.NEWSLETR, code); 9904 if ("NGPL".equals(codeString)) 9905 return new Enumeration<SPDXLicense>(this, SPDXLicense.NGPL, code); 9906 if ("NICTA-1.0".equals(codeString)) 9907 return new Enumeration<SPDXLicense>(this, SPDXLicense.NICTA_1_0, code); 9908 if ("NIST-PD".equals(codeString)) 9909 return new Enumeration<SPDXLicense>(this, SPDXLicense.NIST_PD, code); 9910 if ("NIST-PD-fallback".equals(codeString)) 9911 return new Enumeration<SPDXLicense>(this, SPDXLicense.NIST_PD_FALLBACK, code); 9912 if ("NIST-Software".equals(codeString)) 9913 return new Enumeration<SPDXLicense>(this, SPDXLicense.NIST_SOFTWARE, code); 9914 if ("NLOD-1.0".equals(codeString)) 9915 return new Enumeration<SPDXLicense>(this, SPDXLicense.NLOD_1_0, code); 9916 if ("NLOD-2.0".equals(codeString)) 9917 return new Enumeration<SPDXLicense>(this, SPDXLicense.NLOD_2_0, code); 9918 if ("NLPL".equals(codeString)) 9919 return new Enumeration<SPDXLicense>(this, SPDXLicense.NLPL, code); 9920 if ("Nokia".equals(codeString)) 9921 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOKIA, code); 9922 if ("NOSL".equals(codeString)) 9923 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOSL, code); 9924 if ("not-open-source".equals(codeString)) 9925 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOT_OPEN_SOURCE, code); 9926 if ("Noweb".equals(codeString)) 9927 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOWEB, code); 9928 if ("NPL-1.0".equals(codeString)) 9929 return new Enumeration<SPDXLicense>(this, SPDXLicense.NPL_1_0, code); 9930 if ("NPL-1.1".equals(codeString)) 9931 return new Enumeration<SPDXLicense>(this, SPDXLicense.NPL_1_1, code); 9932 if ("NPOSL-3.0".equals(codeString)) 9933 return new Enumeration<SPDXLicense>(this, SPDXLicense.NPOSL_3_0, code); 9934 if ("NRL".equals(codeString)) 9935 return new Enumeration<SPDXLicense>(this, SPDXLicense.NRL, code); 9936 if ("NTP".equals(codeString)) 9937 return new Enumeration<SPDXLicense>(this, SPDXLicense.NTP, code); 9938 if ("NTP-0".equals(codeString)) 9939 return new Enumeration<SPDXLicense>(this, SPDXLicense.NTP_0, code); 9940 if ("Nunit".equals(codeString)) 9941 return new Enumeration<SPDXLicense>(this, SPDXLicense.NUNIT, code); 9942 if ("O-UDA-1.0".equals(codeString)) 9943 return new Enumeration<SPDXLicense>(this, SPDXLicense.O_UDA_1_0, code); 9944 if ("OCCT-PL".equals(codeString)) 9945 return new Enumeration<SPDXLicense>(this, SPDXLicense.OCCT_PL, code); 9946 if ("OCLC-2.0".equals(codeString)) 9947 return new Enumeration<SPDXLicense>(this, SPDXLicense.OCLC_2_0, code); 9948 if ("ODbL-1.0".equals(codeString)) 9949 return new Enumeration<SPDXLicense>(this, SPDXLicense.ODBL_1_0, code); 9950 if ("ODC-By-1.0".equals(codeString)) 9951 return new Enumeration<SPDXLicense>(this, SPDXLicense.ODC_BY_1_0, code); 9952 if ("OFFIS".equals(codeString)) 9953 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFFIS, code); 9954 if ("OFL-1.0".equals(codeString)) 9955 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_0, code); 9956 if ("OFL-1.0-no-RFN".equals(codeString)) 9957 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_0_NO_RFN, code); 9958 if ("OFL-1.0-RFN".equals(codeString)) 9959 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_0_RFN, code); 9960 if ("OFL-1.1".equals(codeString)) 9961 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_1, code); 9962 if ("OFL-1.1-no-RFN".equals(codeString)) 9963 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_1_NO_RFN, code); 9964 if ("OFL-1.1-RFN".equals(codeString)) 9965 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_1_RFN, code); 9966 if ("OGC-1.0".equals(codeString)) 9967 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGC_1_0, code); 9968 if ("OGDL-Taiwan-1.0".equals(codeString)) 9969 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGDL_TAIWAN_1_0, code); 9970 if ("OGL-Canada-2.0".equals(codeString)) 9971 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_CANADA_2_0, code); 9972 if ("OGL-UK-1.0".equals(codeString)) 9973 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_UK_1_0, code); 9974 if ("OGL-UK-2.0".equals(codeString)) 9975 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_UK_2_0, code); 9976 if ("OGL-UK-3.0".equals(codeString)) 9977 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_UK_3_0, code); 9978 if ("OGTSL".equals(codeString)) 9979 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGTSL, code); 9980 if ("OLDAP-1.1".equals(codeString)) 9981 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_1, code); 9982 if ("OLDAP-1.2".equals(codeString)) 9983 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_2, code); 9984 if ("OLDAP-1.3".equals(codeString)) 9985 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_3, code); 9986 if ("OLDAP-1.4".equals(codeString)) 9987 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_4, code); 9988 if ("OLDAP-2.0".equals(codeString)) 9989 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_0, code); 9990 if ("OLDAP-2.0.1".equals(codeString)) 9991 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_0_1, code); 9992 if ("OLDAP-2.1".equals(codeString)) 9993 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_1, code); 9994 if ("OLDAP-2.2".equals(codeString)) 9995 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_2, code); 9996 if ("OLDAP-2.2.1".equals(codeString)) 9997 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_2_1, code); 9998 if ("OLDAP-2.2.2".equals(codeString)) 9999 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_2_2, code); 10000 if ("OLDAP-2.3".equals(codeString)) 10001 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_3, code); 10002 if ("OLDAP-2.4".equals(codeString)) 10003 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_4, code); 10004 if ("OLDAP-2.5".equals(codeString)) 10005 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_5, code); 10006 if ("OLDAP-2.6".equals(codeString)) 10007 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_6, code); 10008 if ("OLDAP-2.7".equals(codeString)) 10009 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_7, code); 10010 if ("OLDAP-2.8".equals(codeString)) 10011 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_8, code); 10012 if ("OLFL-1.3".equals(codeString)) 10013 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLFL_1_3, code); 10014 if ("OML".equals(codeString)) 10015 return new Enumeration<SPDXLicense>(this, SPDXLicense.OML, code); 10016 if ("OpenPBS-2.3".equals(codeString)) 10017 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPENPBS_2_3, code); 10018 if ("OpenSSL".equals(codeString)) 10019 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPENSSL, code); 10020 if ("OPL-1.0".equals(codeString)) 10021 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPL_1_0, code); 10022 if ("OPL-UK-3.0".equals(codeString)) 10023 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPL_UK_3_0, code); 10024 if ("OPUBL-1.0".equals(codeString)) 10025 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPUBL_1_0, code); 10026 if ("OSET-PL-2.1".equals(codeString)) 10027 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSET_PL_2_1, code); 10028 if ("OSL-1.0".equals(codeString)) 10029 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_1_0, code); 10030 if ("OSL-1.1".equals(codeString)) 10031 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_1_1, code); 10032 if ("OSL-2.0".equals(codeString)) 10033 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_2_0, code); 10034 if ("OSL-2.1".equals(codeString)) 10035 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_2_1, code); 10036 if ("OSL-3.0".equals(codeString)) 10037 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_3_0, code); 10038 if ("Parity-6.0.0".equals(codeString)) 10039 return new Enumeration<SPDXLicense>(this, SPDXLicense.PARITY_6_0_0, code); 10040 if ("Parity-7.0.0".equals(codeString)) 10041 return new Enumeration<SPDXLicense>(this, SPDXLicense.PARITY_7_0_0, code); 10042 if ("PDDL-1.0".equals(codeString)) 10043 return new Enumeration<SPDXLicense>(this, SPDXLicense.PDDL_1_0, code); 10044 if ("PHP-3.0".equals(codeString)) 10045 return new Enumeration<SPDXLicense>(this, SPDXLicense.PHP_3_0, code); 10046 if ("PHP-3.01".equals(codeString)) 10047 return new Enumeration<SPDXLicense>(this, SPDXLicense.PHP_3_01, code); 10048 if ("Plexus".equals(codeString)) 10049 return new Enumeration<SPDXLicense>(this, SPDXLicense.PLEXUS, code); 10050 if ("PolyForm-Noncommercial-1.0.0".equals(codeString)) 10051 return new Enumeration<SPDXLicense>(this, SPDXLicense.POLYFORM_NONCOMMERCIAL_1_0_0, code); 10052 if ("PolyForm-Small-Business-1.0.0".equals(codeString)) 10053 return new Enumeration<SPDXLicense>(this, SPDXLicense.POLYFORM_SMALL_BUSINESS_1_0_0, code); 10054 if ("PostgreSQL".equals(codeString)) 10055 return new Enumeration<SPDXLicense>(this, SPDXLicense.POSTGRESQL, code); 10056 if ("PSF-2.0".equals(codeString)) 10057 return new Enumeration<SPDXLicense>(this, SPDXLicense.PSF_2_0, code); 10058 if ("psfrag".equals(codeString)) 10059 return new Enumeration<SPDXLicense>(this, SPDXLicense.PSFRAG, code); 10060 if ("psutils".equals(codeString)) 10061 return new Enumeration<SPDXLicense>(this, SPDXLicense.PSUTILS, code); 10062 if ("Python-2.0".equals(codeString)) 10063 return new Enumeration<SPDXLicense>(this, SPDXLicense.PYTHON_2_0, code); 10064 if ("Python-2.0.1".equals(codeString)) 10065 return new Enumeration<SPDXLicense>(this, SPDXLicense.PYTHON_2_0_1, code); 10066 if ("Qhull".equals(codeString)) 10067 return new Enumeration<SPDXLicense>(this, SPDXLicense.QHULL, code); 10068 if ("QPL-1.0".equals(codeString)) 10069 return new Enumeration<SPDXLicense>(this, SPDXLicense.QPL_1_0, code); 10070 if ("QPL-1.0-INRIA-2004".equals(codeString)) 10071 return new Enumeration<SPDXLicense>(this, SPDXLicense.QPL_1_0_INRIA_2004, code); 10072 if ("Rdisc".equals(codeString)) 10073 return new Enumeration<SPDXLicense>(this, SPDXLicense.RDISC, code); 10074 if ("RHeCos-1.1".equals(codeString)) 10075 return new Enumeration<SPDXLicense>(this, SPDXLicense.RHECOS_1_1, code); 10076 if ("RPL-1.1".equals(codeString)) 10077 return new Enumeration<SPDXLicense>(this, SPDXLicense.RPL_1_1, code); 10078 if ("RPL-1.5".equals(codeString)) 10079 return new Enumeration<SPDXLicense>(this, SPDXLicense.RPL_1_5, code); 10080 if ("RPSL-1.0".equals(codeString)) 10081 return new Enumeration<SPDXLicense>(this, SPDXLicense.RPSL_1_0, code); 10082 if ("RSA-MD".equals(codeString)) 10083 return new Enumeration<SPDXLicense>(this, SPDXLicense.RSA_MD, code); 10084 if ("RSCPL".equals(codeString)) 10085 return new Enumeration<SPDXLicense>(this, SPDXLicense.RSCPL, code); 10086 if ("Ruby".equals(codeString)) 10087 return new Enumeration<SPDXLicense>(this, SPDXLicense.RUBY, code); 10088 if ("SAX-PD".equals(codeString)) 10089 return new Enumeration<SPDXLicense>(this, SPDXLicense.SAX_PD, code); 10090 if ("Saxpath".equals(codeString)) 10091 return new Enumeration<SPDXLicense>(this, SPDXLicense.SAXPATH, code); 10092 if ("SCEA".equals(codeString)) 10093 return new Enumeration<SPDXLicense>(this, SPDXLicense.SCEA, code); 10094 if ("SchemeReport".equals(codeString)) 10095 return new Enumeration<SPDXLicense>(this, SPDXLicense.SCHEMEREPORT, code); 10096 if ("Sendmail".equals(codeString)) 10097 return new Enumeration<SPDXLicense>(this, SPDXLicense.SENDMAIL, code); 10098 if ("Sendmail-8.23".equals(codeString)) 10099 return new Enumeration<SPDXLicense>(this, SPDXLicense.SENDMAIL_8_23, code); 10100 if ("SGI-B-1.0".equals(codeString)) 10101 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGI_B_1_0, code); 10102 if ("SGI-B-1.1".equals(codeString)) 10103 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGI_B_1_1, code); 10104 if ("SGI-B-2.0".equals(codeString)) 10105 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGI_B_2_0, code); 10106 if ("SGP4".equals(codeString)) 10107 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGP4, code); 10108 if ("SHL-0.5".equals(codeString)) 10109 return new Enumeration<SPDXLicense>(this, SPDXLicense.SHL_0_5, code); 10110 if ("SHL-0.51".equals(codeString)) 10111 return new Enumeration<SPDXLicense>(this, SPDXLicense.SHL_0_51, code); 10112 if ("SimPL-2.0".equals(codeString)) 10113 return new Enumeration<SPDXLicense>(this, SPDXLicense.SIMPL_2_0, code); 10114 if ("SISSL".equals(codeString)) 10115 return new Enumeration<SPDXLicense>(this, SPDXLicense.SISSL, code); 10116 if ("SISSL-1.2".equals(codeString)) 10117 return new Enumeration<SPDXLicense>(this, SPDXLicense.SISSL_1_2, code); 10118 if ("Sleepycat".equals(codeString)) 10119 return new Enumeration<SPDXLicense>(this, SPDXLicense.SLEEPYCAT, code); 10120 if ("SMLNJ".equals(codeString)) 10121 return new Enumeration<SPDXLicense>(this, SPDXLicense.SMLNJ, code); 10122 if ("SMPPL".equals(codeString)) 10123 return new Enumeration<SPDXLicense>(this, SPDXLicense.SMPPL, code); 10124 if ("SNIA".equals(codeString)) 10125 return new Enumeration<SPDXLicense>(this, SPDXLicense.SNIA, code); 10126 if ("snprintf".equals(codeString)) 10127 return new Enumeration<SPDXLicense>(this, SPDXLicense.SNPRINTF, code); 10128 if ("Spencer-86".equals(codeString)) 10129 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPENCER_86, code); 10130 if ("Spencer-94".equals(codeString)) 10131 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPENCER_94, code); 10132 if ("Spencer-99".equals(codeString)) 10133 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPENCER_99, code); 10134 if ("SPL-1.0".equals(codeString)) 10135 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPL_1_0, code); 10136 if ("SSH-OpenSSH".equals(codeString)) 10137 return new Enumeration<SPDXLicense>(this, SPDXLicense.SSH_OPENSSH, code); 10138 if ("SSH-short".equals(codeString)) 10139 return new Enumeration<SPDXLicense>(this, SPDXLicense.SSH_SHORT, code); 10140 if ("SSPL-1.0".equals(codeString)) 10141 return new Enumeration<SPDXLicense>(this, SPDXLicense.SSPL_1_0, code); 10142 if ("StandardML-NJ".equals(codeString)) 10143 return new Enumeration<SPDXLicense>(this, SPDXLicense.STANDARDML_NJ, code); 10144 if ("SugarCRM-1.1.3".equals(codeString)) 10145 return new Enumeration<SPDXLicense>(this, SPDXLicense.SUGARCRM_1_1_3, code); 10146 if ("SunPro".equals(codeString)) 10147 return new Enumeration<SPDXLicense>(this, SPDXLicense.SUNPRO, code); 10148 if ("SWL".equals(codeString)) 10149 return new Enumeration<SPDXLicense>(this, SPDXLicense.SWL, code); 10150 if ("Symlinks".equals(codeString)) 10151 return new Enumeration<SPDXLicense>(this, SPDXLicense.SYMLINKS, code); 10152 if ("TAPR-OHL-1.0".equals(codeString)) 10153 return new Enumeration<SPDXLicense>(this, SPDXLicense.TAPR_OHL_1_0, code); 10154 if ("TCL".equals(codeString)) 10155 return new Enumeration<SPDXLicense>(this, SPDXLicense.TCL, code); 10156 if ("TCP-wrappers".equals(codeString)) 10157 return new Enumeration<SPDXLicense>(this, SPDXLicense.TCP_WRAPPERS, code); 10158 if ("TermReadKey".equals(codeString)) 10159 return new Enumeration<SPDXLicense>(this, SPDXLicense.TERMREADKEY, code); 10160 if ("TMate".equals(codeString)) 10161 return new Enumeration<SPDXLicense>(this, SPDXLicense.TMATE, code); 10162 if ("TORQUE-1.1".equals(codeString)) 10163 return new Enumeration<SPDXLicense>(this, SPDXLicense.TORQUE_1_1, code); 10164 if ("TOSL".equals(codeString)) 10165 return new Enumeration<SPDXLicense>(this, SPDXLicense.TOSL, code); 10166 if ("TPDL".equals(codeString)) 10167 return new Enumeration<SPDXLicense>(this, SPDXLicense.TPDL, code); 10168 if ("TPL-1.0".equals(codeString)) 10169 return new Enumeration<SPDXLicense>(this, SPDXLicense.TPL_1_0, code); 10170 if ("TTWL".equals(codeString)) 10171 return new Enumeration<SPDXLicense>(this, SPDXLicense.TTWL, code); 10172 if ("TU-Berlin-1.0".equals(codeString)) 10173 return new Enumeration<SPDXLicense>(this, SPDXLicense.TU_BERLIN_1_0, code); 10174 if ("TU-Berlin-2.0".equals(codeString)) 10175 return new Enumeration<SPDXLicense>(this, SPDXLicense.TU_BERLIN_2_0, code); 10176 if ("UCAR".equals(codeString)) 10177 return new Enumeration<SPDXLicense>(this, SPDXLicense.UCAR, code); 10178 if ("UCL-1.0".equals(codeString)) 10179 return new Enumeration<SPDXLicense>(this, SPDXLicense.UCL_1_0, code); 10180 if ("Unicode-DFS-2015".equals(codeString)) 10181 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNICODE_DFS_2015, code); 10182 if ("Unicode-DFS-2016".equals(codeString)) 10183 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNICODE_DFS_2016, code); 10184 if ("Unicode-TOU".equals(codeString)) 10185 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNICODE_TOU, code); 10186 if ("UnixCrypt".equals(codeString)) 10187 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNIXCRYPT, code); 10188 if ("Unlicense".equals(codeString)) 10189 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNLICENSE, code); 10190 if ("UPL-1.0".equals(codeString)) 10191 return new Enumeration<SPDXLicense>(this, SPDXLicense.UPL_1_0, code); 10192 if ("Vim".equals(codeString)) 10193 return new Enumeration<SPDXLicense>(this, SPDXLicense.VIM, code); 10194 if ("VOSTROM".equals(codeString)) 10195 return new Enumeration<SPDXLicense>(this, SPDXLicense.VOSTROM, code); 10196 if ("VSL-1.0".equals(codeString)) 10197 return new Enumeration<SPDXLicense>(this, SPDXLicense.VSL_1_0, code); 10198 if ("W3C".equals(codeString)) 10199 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3C, code); 10200 if ("W3C-19980720".equals(codeString)) 10201 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3C_19980720, code); 10202 if ("W3C-20150513".equals(codeString)) 10203 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3C_20150513, code); 10204 if ("w3m".equals(codeString)) 10205 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3M, code); 10206 if ("Watcom-1.0".equals(codeString)) 10207 return new Enumeration<SPDXLicense>(this, SPDXLicense.WATCOM_1_0, code); 10208 if ("Widget-Workshop".equals(codeString)) 10209 return new Enumeration<SPDXLicense>(this, SPDXLicense.WIDGET_WORKSHOP, code); 10210 if ("Wsuipa".equals(codeString)) 10211 return new Enumeration<SPDXLicense>(this, SPDXLicense.WSUIPA, code); 10212 if ("WTFPL".equals(codeString)) 10213 return new Enumeration<SPDXLicense>(this, SPDXLicense.WTFPL, code); 10214 if ("wxWindows".equals(codeString)) 10215 return new Enumeration<SPDXLicense>(this, SPDXLicense.WXWINDOWS, code); 10216 if ("X11".equals(codeString)) 10217 return new Enumeration<SPDXLicense>(this, SPDXLicense.X11, code); 10218 if ("X11-distribute-modifications-variant".equals(codeString)) 10219 return new Enumeration<SPDXLicense>(this, SPDXLicense.X11_DISTRIBUTE_MODIFICATIONS_VARIANT, code); 10220 if ("Xdebug-1.03".equals(codeString)) 10221 return new Enumeration<SPDXLicense>(this, SPDXLicense.XDEBUG_1_03, code); 10222 if ("Xerox".equals(codeString)) 10223 return new Enumeration<SPDXLicense>(this, SPDXLicense.XEROX, code); 10224 if ("Xfig".equals(codeString)) 10225 return new Enumeration<SPDXLicense>(this, SPDXLicense.XFIG, code); 10226 if ("XFree86-1.1".equals(codeString)) 10227 return new Enumeration<SPDXLicense>(this, SPDXLicense.XFREE86_1_1, code); 10228 if ("xinetd".equals(codeString)) 10229 return new Enumeration<SPDXLicense>(this, SPDXLicense.XINETD, code); 10230 if ("xlock".equals(codeString)) 10231 return new Enumeration<SPDXLicense>(this, SPDXLicense.XLOCK, code); 10232 if ("Xnet".equals(codeString)) 10233 return new Enumeration<SPDXLicense>(this, SPDXLicense.XNET, code); 10234 if ("xpp".equals(codeString)) 10235 return new Enumeration<SPDXLicense>(this, SPDXLicense.XPP, code); 10236 if ("XSkat".equals(codeString)) 10237 return new Enumeration<SPDXLicense>(this, SPDXLicense.XSKAT, code); 10238 if ("YPL-1.0".equals(codeString)) 10239 return new Enumeration<SPDXLicense>(this, SPDXLicense.YPL_1_0, code); 10240 if ("YPL-1.1".equals(codeString)) 10241 return new Enumeration<SPDXLicense>(this, SPDXLicense.YPL_1_1, code); 10242 if ("Zed".equals(codeString)) 10243 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZED, code); 10244 if ("Zend-2.0".equals(codeString)) 10245 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZEND_2_0, code); 10246 if ("Zimbra-1.3".equals(codeString)) 10247 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZIMBRA_1_3, code); 10248 if ("Zimbra-1.4".equals(codeString)) 10249 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZIMBRA_1_4, code); 10250 if ("Zlib".equals(codeString)) 10251 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZLIB, code); 10252 if ("zlib-acknowledgement".equals(codeString)) 10253 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZLIB_ACKNOWLEDGEMENT, code); 10254 if ("ZPL-1.1".equals(codeString)) 10255 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZPL_1_1, code); 10256 if ("ZPL-2.0".equals(codeString)) 10257 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZPL_2_0, code); 10258 if ("ZPL-2.1".equals(codeString)) 10259 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZPL_2_1, code); 10260 throw new FHIRException("Unknown SPDXLicense code '" + codeString + "'"); 10261 } 10262 10263 public String toCode(SPDXLicense code) { 10264 if (code == SPDXLicense.NULL) 10265 return null; 10266 if (code == SPDXLicense._0BSD) 10267 return "0BSD"; 10268 if (code == SPDXLicense.AAL) 10269 return "AAL"; 10270 if (code == SPDXLicense.ABSTYLES) 10271 return "Abstyles"; 10272 if (code == SPDXLicense.ADACORE_DOC) 10273 return "AdaCore-doc"; 10274 if (code == SPDXLicense.ADOBE_2006) 10275 return "Adobe-2006"; 10276 if (code == SPDXLicense.ADOBE_GLYPH) 10277 return "Adobe-Glyph"; 10278 if (code == SPDXLicense.ADSL) 10279 return "ADSL"; 10280 if (code == SPDXLicense.AFL_1_1) 10281 return "AFL-1.1"; 10282 if (code == SPDXLicense.AFL_1_2) 10283 return "AFL-1.2"; 10284 if (code == SPDXLicense.AFL_2_0) 10285 return "AFL-2.0"; 10286 if (code == SPDXLicense.AFL_2_1) 10287 return "AFL-2.1"; 10288 if (code == SPDXLicense.AFL_3_0) 10289 return "AFL-3.0"; 10290 if (code == SPDXLicense.AFMPARSE) 10291 return "Afmparse"; 10292 if (code == SPDXLicense.AGPL_1_0) 10293 return "AGPL-1.0"; 10294 if (code == SPDXLicense.AGPL_1_0_ONLY) 10295 return "AGPL-1.0-only"; 10296 if (code == SPDXLicense.AGPL_1_0_OR_LATER) 10297 return "AGPL-1.0-or-later"; 10298 if (code == SPDXLicense.AGPL_3_0) 10299 return "AGPL-3.0"; 10300 if (code == SPDXLicense.AGPL_3_0_ONLY) 10301 return "AGPL-3.0-only"; 10302 if (code == SPDXLicense.AGPL_3_0_OR_LATER) 10303 return "AGPL-3.0-or-later"; 10304 if (code == SPDXLicense.ALADDIN) 10305 return "Aladdin"; 10306 if (code == SPDXLicense.AMDPLPA) 10307 return "AMDPLPA"; 10308 if (code == SPDXLicense.AML) 10309 return "AML"; 10310 if (code == SPDXLicense.AMPAS) 10311 return "AMPAS"; 10312 if (code == SPDXLicense.ANTLR_PD) 10313 return "ANTLR-PD"; 10314 if (code == SPDXLicense.ANTLR_PD_FALLBACK) 10315 return "ANTLR-PD-fallback"; 10316 if (code == SPDXLicense.APACHE_1_0) 10317 return "Apache-1.0"; 10318 if (code == SPDXLicense.APACHE_1_1) 10319 return "Apache-1.1"; 10320 if (code == SPDXLicense.APACHE_2_0) 10321 return "Apache-2.0"; 10322 if (code == SPDXLicense.APAFML) 10323 return "APAFML"; 10324 if (code == SPDXLicense.APL_1_0) 10325 return "APL-1.0"; 10326 if (code == SPDXLicense.APP_S2P) 10327 return "App-s2p"; 10328 if (code == SPDXLicense.APSL_1_0) 10329 return "APSL-1.0"; 10330 if (code == SPDXLicense.APSL_1_1) 10331 return "APSL-1.1"; 10332 if (code == SPDXLicense.APSL_1_2) 10333 return "APSL-1.2"; 10334 if (code == SPDXLicense.APSL_2_0) 10335 return "APSL-2.0"; 10336 if (code == SPDXLicense.ARPHIC_1999) 10337 return "Arphic-1999"; 10338 if (code == SPDXLicense.ARTISTIC_1_0) 10339 return "Artistic-1.0"; 10340 if (code == SPDXLicense.ARTISTIC_1_0_CL8) 10341 return "Artistic-1.0-cl8"; 10342 if (code == SPDXLicense.ARTISTIC_1_0_PERL) 10343 return "Artistic-1.0-Perl"; 10344 if (code == SPDXLicense.ARTISTIC_2_0) 10345 return "Artistic-2.0"; 10346 if (code == SPDXLicense.ASWF_DIGITAL_ASSETS_1_0) 10347 return "ASWF-Digital-Assets-1.0"; 10348 if (code == SPDXLicense.ASWF_DIGITAL_ASSETS_1_1) 10349 return "ASWF-Digital-Assets-1.1"; 10350 if (code == SPDXLicense.BAEKMUK) 10351 return "Baekmuk"; 10352 if (code == SPDXLicense.BAHYPH) 10353 return "Bahyph"; 10354 if (code == SPDXLicense.BARR) 10355 return "Barr"; 10356 if (code == SPDXLicense.BEERWARE) 10357 return "Beerware"; 10358 if (code == SPDXLicense.BITSTREAM_CHARTER) 10359 return "Bitstream-Charter"; 10360 if (code == SPDXLicense.BITSTREAM_VERA) 10361 return "Bitstream-Vera"; 10362 if (code == SPDXLicense.BITTORRENT_1_0) 10363 return "BitTorrent-1.0"; 10364 if (code == SPDXLicense.BITTORRENT_1_1) 10365 return "BitTorrent-1.1"; 10366 if (code == SPDXLicense.BLESSING) 10367 return "blessing"; 10368 if (code == SPDXLicense.BLUEOAK_1_0_0) 10369 return "BlueOak-1.0.0"; 10370 if (code == SPDXLicense.BOEHM_GC) 10371 return "Boehm-GC"; 10372 if (code == SPDXLicense.BORCEUX) 10373 return "Borceux"; 10374 if (code == SPDXLicense.BRIAN_GLADMAN_3_CLAUSE) 10375 return "Brian-Gladman-3-Clause"; 10376 if (code == SPDXLicense.BSD_1_CLAUSE) 10377 return "BSD-1-Clause"; 10378 if (code == SPDXLicense.BSD_2_CLAUSE) 10379 return "BSD-2-Clause"; 10380 if (code == SPDXLicense.BSD_2_CLAUSE_FREEBSD) 10381 return "BSD-2-Clause-FreeBSD"; 10382 if (code == SPDXLicense.BSD_2_CLAUSE_NETBSD) 10383 return "BSD-2-Clause-NetBSD"; 10384 if (code == SPDXLicense.BSD_2_CLAUSE_PATENT) 10385 return "BSD-2-Clause-Patent"; 10386 if (code == SPDXLicense.BSD_2_CLAUSE_VIEWS) 10387 return "BSD-2-Clause-Views"; 10388 if (code == SPDXLicense.BSD_3_CLAUSE) 10389 return "BSD-3-Clause"; 10390 if (code == SPDXLicense.BSD_3_CLAUSE_ATTRIBUTION) 10391 return "BSD-3-Clause-Attribution"; 10392 if (code == SPDXLicense.BSD_3_CLAUSE_CLEAR) 10393 return "BSD-3-Clause-Clear"; 10394 if (code == SPDXLicense.BSD_3_CLAUSE_LBNL) 10395 return "BSD-3-Clause-LBNL"; 10396 if (code == SPDXLicense.BSD_3_CLAUSE_MODIFICATION) 10397 return "BSD-3-Clause-Modification"; 10398 if (code == SPDXLicense.BSD_3_CLAUSE_NO_MILITARY_LICENSE) 10399 return "BSD-3-Clause-No-Military-License"; 10400 if (code == SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE) 10401 return "BSD-3-Clause-No-Nuclear-License"; 10402 if (code == SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014) 10403 return "BSD-3-Clause-No-Nuclear-License-2014"; 10404 if (code == SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY) 10405 return "BSD-3-Clause-No-Nuclear-Warranty"; 10406 if (code == SPDXLicense.BSD_3_CLAUSE_OPEN_MPI) 10407 return "BSD-3-Clause-Open-MPI"; 10408 if (code == SPDXLicense.BSD_4_CLAUSE) 10409 return "BSD-4-Clause"; 10410 if (code == SPDXLicense.BSD_4_CLAUSE_SHORTENED) 10411 return "BSD-4-Clause-Shortened"; 10412 if (code == SPDXLicense.BSD_4_CLAUSE_UC) 10413 return "BSD-4-Clause-UC"; 10414 if (code == SPDXLicense.BSD_4_3RENO) 10415 return "BSD-4.3RENO"; 10416 if (code == SPDXLicense.BSD_4_3TAHOE) 10417 return "BSD-4.3TAHOE"; 10418 if (code == SPDXLicense.BSD_ADVERTISING_ACKNOWLEDGEMENT) 10419 return "BSD-Advertising-Acknowledgement"; 10420 if (code == SPDXLicense.BSD_ATTRIBUTION_HPND_DISCLAIMER) 10421 return "BSD-Attribution-HPND-disclaimer"; 10422 if (code == SPDXLicense.BSD_PROTECTION) 10423 return "BSD-Protection"; 10424 if (code == SPDXLicense.BSD_SOURCE_CODE) 10425 return "BSD-Source-Code"; 10426 if (code == SPDXLicense.BSL_1_0) 10427 return "BSL-1.0"; 10428 if (code == SPDXLicense.BUSL_1_1) 10429 return "BUSL-1.1"; 10430 if (code == SPDXLicense.BZIP2_1_0_5) 10431 return "bzip2-1.0.5"; 10432 if (code == SPDXLicense.BZIP2_1_0_6) 10433 return "bzip2-1.0.6"; 10434 if (code == SPDXLicense.C_UDA_1_0) 10435 return "C-UDA-1.0"; 10436 if (code == SPDXLicense.CAL_1_0) 10437 return "CAL-1.0"; 10438 if (code == SPDXLicense.CAL_1_0_COMBINED_WORK_EXCEPTION) 10439 return "CAL-1.0-Combined-Work-Exception"; 10440 if (code == SPDXLicense.CALDERA) 10441 return "Caldera"; 10442 if (code == SPDXLicense.CATOSL_1_1) 10443 return "CATOSL-1.1"; 10444 if (code == SPDXLicense.CC_BY_1_0) 10445 return "CC-BY-1.0"; 10446 if (code == SPDXLicense.CC_BY_2_0) 10447 return "CC-BY-2.0"; 10448 if (code == SPDXLicense.CC_BY_2_5) 10449 return "CC-BY-2.5"; 10450 if (code == SPDXLicense.CC_BY_2_5_AU) 10451 return "CC-BY-2.5-AU"; 10452 if (code == SPDXLicense.CC_BY_3_0) 10453 return "CC-BY-3.0"; 10454 if (code == SPDXLicense.CC_BY_3_0_AT) 10455 return "CC-BY-3.0-AT"; 10456 if (code == SPDXLicense.CC_BY_3_0_DE) 10457 return "CC-BY-3.0-DE"; 10458 if (code == SPDXLicense.CC_BY_3_0_IGO) 10459 return "CC-BY-3.0-IGO"; 10460 if (code == SPDXLicense.CC_BY_3_0_NL) 10461 return "CC-BY-3.0-NL"; 10462 if (code == SPDXLicense.CC_BY_3_0_US) 10463 return "CC-BY-3.0-US"; 10464 if (code == SPDXLicense.CC_BY_4_0) 10465 return "CC-BY-4.0"; 10466 if (code == SPDXLicense.CC_BY_NC_1_0) 10467 return "CC-BY-NC-1.0"; 10468 if (code == SPDXLicense.CC_BY_NC_2_0) 10469 return "CC-BY-NC-2.0"; 10470 if (code == SPDXLicense.CC_BY_NC_2_5) 10471 return "CC-BY-NC-2.5"; 10472 if (code == SPDXLicense.CC_BY_NC_3_0) 10473 return "CC-BY-NC-3.0"; 10474 if (code == SPDXLicense.CC_BY_NC_3_0_DE) 10475 return "CC-BY-NC-3.0-DE"; 10476 if (code == SPDXLicense.CC_BY_NC_4_0) 10477 return "CC-BY-NC-4.0"; 10478 if (code == SPDXLicense.CC_BY_NC_ND_1_0) 10479 return "CC-BY-NC-ND-1.0"; 10480 if (code == SPDXLicense.CC_BY_NC_ND_2_0) 10481 return "CC-BY-NC-ND-2.0"; 10482 if (code == SPDXLicense.CC_BY_NC_ND_2_5) 10483 return "CC-BY-NC-ND-2.5"; 10484 if (code == SPDXLicense.CC_BY_NC_ND_3_0) 10485 return "CC-BY-NC-ND-3.0"; 10486 if (code == SPDXLicense.CC_BY_NC_ND_3_0_DE) 10487 return "CC-BY-NC-ND-3.0-DE"; 10488 if (code == SPDXLicense.CC_BY_NC_ND_3_0_IGO) 10489 return "CC-BY-NC-ND-3.0-IGO"; 10490 if (code == SPDXLicense.CC_BY_NC_ND_4_0) 10491 return "CC-BY-NC-ND-4.0"; 10492 if (code == SPDXLicense.CC_BY_NC_SA_1_0) 10493 return "CC-BY-NC-SA-1.0"; 10494 if (code == SPDXLicense.CC_BY_NC_SA_2_0) 10495 return "CC-BY-NC-SA-2.0"; 10496 if (code == SPDXLicense.CC_BY_NC_SA_2_0_DE) 10497 return "CC-BY-NC-SA-2.0-DE"; 10498 if (code == SPDXLicense.CC_BY_NC_SA_2_0_FR) 10499 return "CC-BY-NC-SA-2.0-FR"; 10500 if (code == SPDXLicense.CC_BY_NC_SA_2_0_UK) 10501 return "CC-BY-NC-SA-2.0-UK"; 10502 if (code == SPDXLicense.CC_BY_NC_SA_2_5) 10503 return "CC-BY-NC-SA-2.5"; 10504 if (code == SPDXLicense.CC_BY_NC_SA_3_0) 10505 return "CC-BY-NC-SA-3.0"; 10506 if (code == SPDXLicense.CC_BY_NC_SA_3_0_DE) 10507 return "CC-BY-NC-SA-3.0-DE"; 10508 if (code == SPDXLicense.CC_BY_NC_SA_3_0_IGO) 10509 return "CC-BY-NC-SA-3.0-IGO"; 10510 if (code == SPDXLicense.CC_BY_NC_SA_4_0) 10511 return "CC-BY-NC-SA-4.0"; 10512 if (code == SPDXLicense.CC_BY_ND_1_0) 10513 return "CC-BY-ND-1.0"; 10514 if (code == SPDXLicense.CC_BY_ND_2_0) 10515 return "CC-BY-ND-2.0"; 10516 if (code == SPDXLicense.CC_BY_ND_2_5) 10517 return "CC-BY-ND-2.5"; 10518 if (code == SPDXLicense.CC_BY_ND_3_0) 10519 return "CC-BY-ND-3.0"; 10520 if (code == SPDXLicense.CC_BY_ND_3_0_DE) 10521 return "CC-BY-ND-3.0-DE"; 10522 if (code == SPDXLicense.CC_BY_ND_4_0) 10523 return "CC-BY-ND-4.0"; 10524 if (code == SPDXLicense.CC_BY_SA_1_0) 10525 return "CC-BY-SA-1.0"; 10526 if (code == SPDXLicense.CC_BY_SA_2_0) 10527 return "CC-BY-SA-2.0"; 10528 if (code == SPDXLicense.CC_BY_SA_2_0_UK) 10529 return "CC-BY-SA-2.0-UK"; 10530 if (code == SPDXLicense.CC_BY_SA_2_1_JP) 10531 return "CC-BY-SA-2.1-JP"; 10532 if (code == SPDXLicense.CC_BY_SA_2_5) 10533 return "CC-BY-SA-2.5"; 10534 if (code == SPDXLicense.CC_BY_SA_3_0) 10535 return "CC-BY-SA-3.0"; 10536 if (code == SPDXLicense.CC_BY_SA_3_0_AT) 10537 return "CC-BY-SA-3.0-AT"; 10538 if (code == SPDXLicense.CC_BY_SA_3_0_DE) 10539 return "CC-BY-SA-3.0-DE"; 10540 if (code == SPDXLicense.CC_BY_SA_3_0_IGO) 10541 return "CC-BY-SA-3.0-IGO"; 10542 if (code == SPDXLicense.CC_BY_SA_4_0) 10543 return "CC-BY-SA-4.0"; 10544 if (code == SPDXLicense.CC_PDDC) 10545 return "CC-PDDC"; 10546 if (code == SPDXLicense.CC0_1_0) 10547 return "CC0-1.0"; 10548 if (code == SPDXLicense.CDDL_1_0) 10549 return "CDDL-1.0"; 10550 if (code == SPDXLicense.CDDL_1_1) 10551 return "CDDL-1.1"; 10552 if (code == SPDXLicense.CDL_1_0) 10553 return "CDL-1.0"; 10554 if (code == SPDXLicense.CDLA_PERMISSIVE_1_0) 10555 return "CDLA-Permissive-1.0"; 10556 if (code == SPDXLicense.CDLA_PERMISSIVE_2_0) 10557 return "CDLA-Permissive-2.0"; 10558 if (code == SPDXLicense.CDLA_SHARING_1_0) 10559 return "CDLA-Sharing-1.0"; 10560 if (code == SPDXLicense.CECILL_1_0) 10561 return "CECILL-1.0"; 10562 if (code == SPDXLicense.CECILL_1_1) 10563 return "CECILL-1.1"; 10564 if (code == SPDXLicense.CECILL_2_0) 10565 return "CECILL-2.0"; 10566 if (code == SPDXLicense.CECILL_2_1) 10567 return "CECILL-2.1"; 10568 if (code == SPDXLicense.CECILL_B) 10569 return "CECILL-B"; 10570 if (code == SPDXLicense.CECILL_C) 10571 return "CECILL-C"; 10572 if (code == SPDXLicense.CERN_OHL_1_1) 10573 return "CERN-OHL-1.1"; 10574 if (code == SPDXLicense.CERN_OHL_1_2) 10575 return "CERN-OHL-1.2"; 10576 if (code == SPDXLicense.CERN_OHL_P_2_0) 10577 return "CERN-OHL-P-2.0"; 10578 if (code == SPDXLicense.CERN_OHL_S_2_0) 10579 return "CERN-OHL-S-2.0"; 10580 if (code == SPDXLicense.CERN_OHL_W_2_0) 10581 return "CERN-OHL-W-2.0"; 10582 if (code == SPDXLicense.CFITSIO) 10583 return "CFITSIO"; 10584 if (code == SPDXLicense.CHECKMK) 10585 return "checkmk"; 10586 if (code == SPDXLicense.CLARTISTIC) 10587 return "ClArtistic"; 10588 if (code == SPDXLicense.CLIPS) 10589 return "Clips"; 10590 if (code == SPDXLicense.CMU_MACH) 10591 return "CMU-Mach"; 10592 if (code == SPDXLicense.CNRI_JYTHON) 10593 return "CNRI-Jython"; 10594 if (code == SPDXLicense.CNRI_PYTHON) 10595 return "CNRI-Python"; 10596 if (code == SPDXLicense.CNRI_PYTHON_GPL_COMPATIBLE) 10597 return "CNRI-Python-GPL-Compatible"; 10598 if (code == SPDXLicense.COIL_1_0) 10599 return "COIL-1.0"; 10600 if (code == SPDXLicense.COMMUNITY_SPEC_1_0) 10601 return "Community-Spec-1.0"; 10602 if (code == SPDXLicense.CONDOR_1_1) 10603 return "Condor-1.1"; 10604 if (code == SPDXLicense.COPYLEFT_NEXT_0_3_0) 10605 return "copyleft-next-0.3.0"; 10606 if (code == SPDXLicense.COPYLEFT_NEXT_0_3_1) 10607 return "copyleft-next-0.3.1"; 10608 if (code == SPDXLicense.CORNELL_LOSSLESS_JPEG) 10609 return "Cornell-Lossless-JPEG"; 10610 if (code == SPDXLicense.CPAL_1_0) 10611 return "CPAL-1.0"; 10612 if (code == SPDXLicense.CPL_1_0) 10613 return "CPL-1.0"; 10614 if (code == SPDXLicense.CPOL_1_02) 10615 return "CPOL-1.02"; 10616 if (code == SPDXLicense.CROSSWORD) 10617 return "Crossword"; 10618 if (code == SPDXLicense.CRYSTALSTACKER) 10619 return "CrystalStacker"; 10620 if (code == SPDXLicense.CUA_OPL_1_0) 10621 return "CUA-OPL-1.0"; 10622 if (code == SPDXLicense.CUBE) 10623 return "Cube"; 10624 if (code == SPDXLicense.CURL) 10625 return "curl"; 10626 if (code == SPDXLicense.D_FSL_1_0) 10627 return "D-FSL-1.0"; 10628 if (code == SPDXLicense.DIFFMARK) 10629 return "diffmark"; 10630 if (code == SPDXLicense.DL_DE_BY_2_0) 10631 return "DL-DE-BY-2.0"; 10632 if (code == SPDXLicense.DOC) 10633 return "DOC"; 10634 if (code == SPDXLicense.DOTSEQN) 10635 return "Dotseqn"; 10636 if (code == SPDXLicense.DRL_1_0) 10637 return "DRL-1.0"; 10638 if (code == SPDXLicense.DSDP) 10639 return "DSDP"; 10640 if (code == SPDXLicense.DTOA) 10641 return "dtoa"; 10642 if (code == SPDXLicense.DVIPDFM) 10643 return "dvipdfm"; 10644 if (code == SPDXLicense.ECL_1_0) 10645 return "ECL-1.0"; 10646 if (code == SPDXLicense.ECL_2_0) 10647 return "ECL-2.0"; 10648 if (code == SPDXLicense.ECOS_2_0) 10649 return "eCos-2.0"; 10650 if (code == SPDXLicense.EFL_1_0) 10651 return "EFL-1.0"; 10652 if (code == SPDXLicense.EFL_2_0) 10653 return "EFL-2.0"; 10654 if (code == SPDXLicense.EGENIX) 10655 return "eGenix"; 10656 if (code == SPDXLicense.ELASTIC_2_0) 10657 return "Elastic-2.0"; 10658 if (code == SPDXLicense.ENTESSA) 10659 return "Entessa"; 10660 if (code == SPDXLicense.EPICS) 10661 return "EPICS"; 10662 if (code == SPDXLicense.EPL_1_0) 10663 return "EPL-1.0"; 10664 if (code == SPDXLicense.EPL_2_0) 10665 return "EPL-2.0"; 10666 if (code == SPDXLicense.ERLPL_1_1) 10667 return "ErlPL-1.1"; 10668 if (code == SPDXLicense.ETALAB_2_0) 10669 return "etalab-2.0"; 10670 if (code == SPDXLicense.EUDATAGRID) 10671 return "EUDatagrid"; 10672 if (code == SPDXLicense.EUPL_1_0) 10673 return "EUPL-1.0"; 10674 if (code == SPDXLicense.EUPL_1_1) 10675 return "EUPL-1.1"; 10676 if (code == SPDXLicense.EUPL_1_2) 10677 return "EUPL-1.2"; 10678 if (code == SPDXLicense.EUROSYM) 10679 return "Eurosym"; 10680 if (code == SPDXLicense.FAIR) 10681 return "Fair"; 10682 if (code == SPDXLicense.FDK_AAC) 10683 return "FDK-AAC"; 10684 if (code == SPDXLicense.FRAMEWORX_1_0) 10685 return "Frameworx-1.0"; 10686 if (code == SPDXLicense.FREEBSD_DOC) 10687 return "FreeBSD-DOC"; 10688 if (code == SPDXLicense.FREEIMAGE) 10689 return "FreeImage"; 10690 if (code == SPDXLicense.FSFAP) 10691 return "FSFAP"; 10692 if (code == SPDXLicense.FSFUL) 10693 return "FSFUL"; 10694 if (code == SPDXLicense.FSFULLR) 10695 return "FSFULLR"; 10696 if (code == SPDXLicense.FSFULLRWD) 10697 return "FSFULLRWD"; 10698 if (code == SPDXLicense.FTL) 10699 return "FTL"; 10700 if (code == SPDXLicense.GD) 10701 return "GD"; 10702 if (code == SPDXLicense.GFDL_1_1) 10703 return "GFDL-1.1"; 10704 if (code == SPDXLicense.GFDL_1_1_INVARIANTS_ONLY) 10705 return "GFDL-1.1-invariants-only"; 10706 if (code == SPDXLicense.GFDL_1_1_INVARIANTS_OR_LATER) 10707 return "GFDL-1.1-invariants-or-later"; 10708 if (code == SPDXLicense.GFDL_1_1_NO_INVARIANTS_ONLY) 10709 return "GFDL-1.1-no-invariants-only"; 10710 if (code == SPDXLicense.GFDL_1_1_NO_INVARIANTS_OR_LATER) 10711 return "GFDL-1.1-no-invariants-or-later"; 10712 if (code == SPDXLicense.GFDL_1_1_ONLY) 10713 return "GFDL-1.1-only"; 10714 if (code == SPDXLicense.GFDL_1_1_OR_LATER) 10715 return "GFDL-1.1-or-later"; 10716 if (code == SPDXLicense.GFDL_1_2) 10717 return "GFDL-1.2"; 10718 if (code == SPDXLicense.GFDL_1_2_INVARIANTS_ONLY) 10719 return "GFDL-1.2-invariants-only"; 10720 if (code == SPDXLicense.GFDL_1_2_INVARIANTS_OR_LATER) 10721 return "GFDL-1.2-invariants-or-later"; 10722 if (code == SPDXLicense.GFDL_1_2_NO_INVARIANTS_ONLY) 10723 return "GFDL-1.2-no-invariants-only"; 10724 if (code == SPDXLicense.GFDL_1_2_NO_INVARIANTS_OR_LATER) 10725 return "GFDL-1.2-no-invariants-or-later"; 10726 if (code == SPDXLicense.GFDL_1_2_ONLY) 10727 return "GFDL-1.2-only"; 10728 if (code == SPDXLicense.GFDL_1_2_OR_LATER) 10729 return "GFDL-1.2-or-later"; 10730 if (code == SPDXLicense.GFDL_1_3) 10731 return "GFDL-1.3"; 10732 if (code == SPDXLicense.GFDL_1_3_INVARIANTS_ONLY) 10733 return "GFDL-1.3-invariants-only"; 10734 if (code == SPDXLicense.GFDL_1_3_INVARIANTS_OR_LATER) 10735 return "GFDL-1.3-invariants-or-later"; 10736 if (code == SPDXLicense.GFDL_1_3_NO_INVARIANTS_ONLY) 10737 return "GFDL-1.3-no-invariants-only"; 10738 if (code == SPDXLicense.GFDL_1_3_NO_INVARIANTS_OR_LATER) 10739 return "GFDL-1.3-no-invariants-or-later"; 10740 if (code == SPDXLicense.GFDL_1_3_ONLY) 10741 return "GFDL-1.3-only"; 10742 if (code == SPDXLicense.GFDL_1_3_OR_LATER) 10743 return "GFDL-1.3-or-later"; 10744 if (code == SPDXLicense.GIFTWARE) 10745 return "Giftware"; 10746 if (code == SPDXLicense.GL2PS) 10747 return "GL2PS"; 10748 if (code == SPDXLicense.GLIDE) 10749 return "Glide"; 10750 if (code == SPDXLicense.GLULXE) 10751 return "Glulxe"; 10752 if (code == SPDXLicense.GLWTPL) 10753 return "GLWTPL"; 10754 if (code == SPDXLicense.GNUPLOT) 10755 return "gnuplot"; 10756 if (code == SPDXLicense.GPL_1_0) 10757 return "GPL-1.0"; 10758 if (code == SPDXLicense.GPL_1_0PLUS) 10759 return "GPL-1.0+"; 10760 if (code == SPDXLicense.GPL_1_0_ONLY) 10761 return "GPL-1.0-only"; 10762 if (code == SPDXLicense.GPL_1_0_OR_LATER) 10763 return "GPL-1.0-or-later"; 10764 if (code == SPDXLicense.GPL_2_0) 10765 return "GPL-2.0"; 10766 if (code == SPDXLicense.GPL_2_0PLUS) 10767 return "GPL-2.0+"; 10768 if (code == SPDXLicense.GPL_2_0_ONLY) 10769 return "GPL-2.0-only"; 10770 if (code == SPDXLicense.GPL_2_0_OR_LATER) 10771 return "GPL-2.0-or-later"; 10772 if (code == SPDXLicense.GPL_2_0_WITH_AUTOCONF_EXCEPTION) 10773 return "GPL-2.0-with-autoconf-exception"; 10774 if (code == SPDXLicense.GPL_2_0_WITH_BISON_EXCEPTION) 10775 return "GPL-2.0-with-bison-exception"; 10776 if (code == SPDXLicense.GPL_2_0_WITH_CLASSPATH_EXCEPTION) 10777 return "GPL-2.0-with-classpath-exception"; 10778 if (code == SPDXLicense.GPL_2_0_WITH_FONT_EXCEPTION) 10779 return "GPL-2.0-with-font-exception"; 10780 if (code == SPDXLicense.GPL_2_0_WITH_GCC_EXCEPTION) 10781 return "GPL-2.0-with-GCC-exception"; 10782 if (code == SPDXLicense.GPL_3_0) 10783 return "GPL-3.0"; 10784 if (code == SPDXLicense.GPL_3_0PLUS) 10785 return "GPL-3.0+"; 10786 if (code == SPDXLicense.GPL_3_0_ONLY) 10787 return "GPL-3.0-only"; 10788 if (code == SPDXLicense.GPL_3_0_OR_LATER) 10789 return "GPL-3.0-or-later"; 10790 if (code == SPDXLicense.GPL_3_0_WITH_AUTOCONF_EXCEPTION) 10791 return "GPL-3.0-with-autoconf-exception"; 10792 if (code == SPDXLicense.GPL_3_0_WITH_GCC_EXCEPTION) 10793 return "GPL-3.0-with-GCC-exception"; 10794 if (code == SPDXLicense.GRAPHICS_GEMS) 10795 return "Graphics-Gems"; 10796 if (code == SPDXLicense.GSOAP_1_3B) 10797 return "gSOAP-1.3b"; 10798 if (code == SPDXLicense.HASKELLREPORT) 10799 return "HaskellReport"; 10800 if (code == SPDXLicense.HIPPOCRATIC_2_1) 10801 return "Hippocratic-2.1"; 10802 if (code == SPDXLicense.HP_1986) 10803 return "HP-1986"; 10804 if (code == SPDXLicense.HPND) 10805 return "HPND"; 10806 if (code == SPDXLicense.HPND_EXPORT_US) 10807 return "HPND-export-US"; 10808 if (code == SPDXLicense.HPND_MARKUS_KUHN) 10809 return "HPND-Markus-Kuhn"; 10810 if (code == SPDXLicense.HPND_SELL_VARIANT) 10811 return "HPND-sell-variant"; 10812 if (code == SPDXLicense.HPND_SELL_VARIANT_MIT_DISCLAIMER) 10813 return "HPND-sell-variant-MIT-disclaimer"; 10814 if (code == SPDXLicense.HTMLTIDY) 10815 return "HTMLTIDY"; 10816 if (code == SPDXLicense.IBM_PIBS) 10817 return "IBM-pibs"; 10818 if (code == SPDXLicense.ICU) 10819 return "ICU"; 10820 if (code == SPDXLicense.IEC_CODE_COMPONENTS_EULA) 10821 return "IEC-Code-Components-EULA"; 10822 if (code == SPDXLicense.IJG) 10823 return "IJG"; 10824 if (code == SPDXLicense.IJG_SHORT) 10825 return "IJG-short"; 10826 if (code == SPDXLicense.IMAGEMAGICK) 10827 return "ImageMagick"; 10828 if (code == SPDXLicense.IMATIX) 10829 return "iMatix"; 10830 if (code == SPDXLicense.IMLIB2) 10831 return "Imlib2"; 10832 if (code == SPDXLicense.INFO_ZIP) 10833 return "Info-ZIP"; 10834 if (code == SPDXLicense.INNER_NET_2_0) 10835 return "Inner-Net-2.0"; 10836 if (code == SPDXLicense.INTEL) 10837 return "Intel"; 10838 if (code == SPDXLicense.INTEL_ACPI) 10839 return "Intel-ACPI"; 10840 if (code == SPDXLicense.INTERBASE_1_0) 10841 return "Interbase-1.0"; 10842 if (code == SPDXLicense.IPA) 10843 return "IPA"; 10844 if (code == SPDXLicense.IPL_1_0) 10845 return "IPL-1.0"; 10846 if (code == SPDXLicense.ISC) 10847 return "ISC"; 10848 if (code == SPDXLicense.JAM) 10849 return "Jam"; 10850 if (code == SPDXLicense.JASPER_2_0) 10851 return "JasPer-2.0"; 10852 if (code == SPDXLicense.JPL_IMAGE) 10853 return "JPL-image"; 10854 if (code == SPDXLicense.JPNIC) 10855 return "JPNIC"; 10856 if (code == SPDXLicense.JSON) 10857 return "JSON"; 10858 if (code == SPDXLicense.KAZLIB) 10859 return "Kazlib"; 10860 if (code == SPDXLicense.KNUTH_CTAN) 10861 return "Knuth-CTAN"; 10862 if (code == SPDXLicense.LAL_1_2) 10863 return "LAL-1.2"; 10864 if (code == SPDXLicense.LAL_1_3) 10865 return "LAL-1.3"; 10866 if (code == SPDXLicense.LATEX2E) 10867 return "Latex2e"; 10868 if (code == SPDXLicense.LATEX2E_TRANSLATED_NOTICE) 10869 return "Latex2e-translated-notice"; 10870 if (code == SPDXLicense.LEPTONICA) 10871 return "Leptonica"; 10872 if (code == SPDXLicense.LGPL_2_0) 10873 return "LGPL-2.0"; 10874 if (code == SPDXLicense.LGPL_2_0PLUS) 10875 return "LGPL-2.0+"; 10876 if (code == SPDXLicense.LGPL_2_0_ONLY) 10877 return "LGPL-2.0-only"; 10878 if (code == SPDXLicense.LGPL_2_0_OR_LATER) 10879 return "LGPL-2.0-or-later"; 10880 if (code == SPDXLicense.LGPL_2_1) 10881 return "LGPL-2.1"; 10882 if (code == SPDXLicense.LGPL_2_1PLUS) 10883 return "LGPL-2.1+"; 10884 if (code == SPDXLicense.LGPL_2_1_ONLY) 10885 return "LGPL-2.1-only"; 10886 if (code == SPDXLicense.LGPL_2_1_OR_LATER) 10887 return "LGPL-2.1-or-later"; 10888 if (code == SPDXLicense.LGPL_3_0) 10889 return "LGPL-3.0"; 10890 if (code == SPDXLicense.LGPL_3_0PLUS) 10891 return "LGPL-3.0+"; 10892 if (code == SPDXLicense.LGPL_3_0_ONLY) 10893 return "LGPL-3.0-only"; 10894 if (code == SPDXLicense.LGPL_3_0_OR_LATER) 10895 return "LGPL-3.0-or-later"; 10896 if (code == SPDXLicense.LGPLLR) 10897 return "LGPLLR"; 10898 if (code == SPDXLicense.LIBPNG) 10899 return "Libpng"; 10900 if (code == SPDXLicense.LIBPNG_2_0) 10901 return "libpng-2.0"; 10902 if (code == SPDXLicense.LIBSELINUX_1_0) 10903 return "libselinux-1.0"; 10904 if (code == SPDXLicense.LIBTIFF) 10905 return "libtiff"; 10906 if (code == SPDXLicense.LIBUTIL_DAVID_NUGENT) 10907 return "libutil-David-Nugent"; 10908 if (code == SPDXLicense.LILIQ_P_1_1) 10909 return "LiLiQ-P-1.1"; 10910 if (code == SPDXLicense.LILIQ_R_1_1) 10911 return "LiLiQ-R-1.1"; 10912 if (code == SPDXLicense.LILIQ_RPLUS_1_1) 10913 return "LiLiQ-Rplus-1.1"; 10914 if (code == SPDXLicense.LINUX_MAN_PAGES_1_PARA) 10915 return "Linux-man-pages-1-para"; 10916 if (code == SPDXLicense.LINUX_MAN_PAGES_COPYLEFT) 10917 return "Linux-man-pages-copyleft"; 10918 if (code == SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_2_PARA) 10919 return "Linux-man-pages-copyleft-2-para"; 10920 if (code == SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_VAR) 10921 return "Linux-man-pages-copyleft-var"; 10922 if (code == SPDXLicense.LINUX_OPENIB) 10923 return "Linux-OpenIB"; 10924 if (code == SPDXLicense.LOOP) 10925 return "LOOP"; 10926 if (code == SPDXLicense.LPL_1_0) 10927 return "LPL-1.0"; 10928 if (code == SPDXLicense.LPL_1_02) 10929 return "LPL-1.02"; 10930 if (code == SPDXLicense.LPPL_1_0) 10931 return "LPPL-1.0"; 10932 if (code == SPDXLicense.LPPL_1_1) 10933 return "LPPL-1.1"; 10934 if (code == SPDXLicense.LPPL_1_2) 10935 return "LPPL-1.2"; 10936 if (code == SPDXLicense.LPPL_1_3A) 10937 return "LPPL-1.3a"; 10938 if (code == SPDXLicense.LPPL_1_3C) 10939 return "LPPL-1.3c"; 10940 if (code == SPDXLicense.LZMA_SDK_9_11_TO_9_20) 10941 return "LZMA-SDK-9.11-to-9.20"; 10942 if (code == SPDXLicense.LZMA_SDK_9_22) 10943 return "LZMA-SDK-9.22"; 10944 if (code == SPDXLicense.MAKEINDEX) 10945 return "MakeIndex"; 10946 if (code == SPDXLicense.MARTIN_BIRGMEIER) 10947 return "Martin-Birgmeier"; 10948 if (code == SPDXLicense.METAMAIL) 10949 return "metamail"; 10950 if (code == SPDXLicense.MINPACK) 10951 return "Minpack"; 10952 if (code == SPDXLicense.MIROS) 10953 return "MirOS"; 10954 if (code == SPDXLicense.MIT) 10955 return "MIT"; 10956 if (code == SPDXLicense.MIT_0) 10957 return "MIT-0"; 10958 if (code == SPDXLicense.MIT_ADVERTISING) 10959 return "MIT-advertising"; 10960 if (code == SPDXLicense.MIT_CMU) 10961 return "MIT-CMU"; 10962 if (code == SPDXLicense.MIT_ENNA) 10963 return "MIT-enna"; 10964 if (code == SPDXLicense.MIT_FEH) 10965 return "MIT-feh"; 10966 if (code == SPDXLicense.MIT_FESTIVAL) 10967 return "MIT-Festival"; 10968 if (code == SPDXLicense.MIT_MODERN_VARIANT) 10969 return "MIT-Modern-Variant"; 10970 if (code == SPDXLicense.MIT_OPEN_GROUP) 10971 return "MIT-open-group"; 10972 if (code == SPDXLicense.MIT_WU) 10973 return "MIT-Wu"; 10974 if (code == SPDXLicense.MITNFA) 10975 return "MITNFA"; 10976 if (code == SPDXLicense.MOTOSOTO) 10977 return "Motosoto"; 10978 if (code == SPDXLicense.MPI_PERMISSIVE) 10979 return "mpi-permissive"; 10980 if (code == SPDXLicense.MPICH2) 10981 return "mpich2"; 10982 if (code == SPDXLicense.MPL_1_0) 10983 return "MPL-1.0"; 10984 if (code == SPDXLicense.MPL_1_1) 10985 return "MPL-1.1"; 10986 if (code == SPDXLicense.MPL_2_0) 10987 return "MPL-2.0"; 10988 if (code == SPDXLicense.MPL_2_0_NO_COPYLEFT_EXCEPTION) 10989 return "MPL-2.0-no-copyleft-exception"; 10990 if (code == SPDXLicense.MPLUS) 10991 return "mplus"; 10992 if (code == SPDXLicense.MS_LPL) 10993 return "MS-LPL"; 10994 if (code == SPDXLicense.MS_PL) 10995 return "MS-PL"; 10996 if (code == SPDXLicense.MS_RL) 10997 return "MS-RL"; 10998 if (code == SPDXLicense.MTLL) 10999 return "MTLL"; 11000 if (code == SPDXLicense.MULANPSL_1_0) 11001 return "MulanPSL-1.0"; 11002 if (code == SPDXLicense.MULANPSL_2_0) 11003 return "MulanPSL-2.0"; 11004 if (code == SPDXLicense.MULTICS) 11005 return "Multics"; 11006 if (code == SPDXLicense.MUP) 11007 return "Mup"; 11008 if (code == SPDXLicense.NAIST_2003) 11009 return "NAIST-2003"; 11010 if (code == SPDXLicense.NASA_1_3) 11011 return "NASA-1.3"; 11012 if (code == SPDXLicense.NAUMEN) 11013 return "Naumen"; 11014 if (code == SPDXLicense.NBPL_1_0) 11015 return "NBPL-1.0"; 11016 if (code == SPDXLicense.NCGL_UK_2_0) 11017 return "NCGL-UK-2.0"; 11018 if (code == SPDXLicense.NCSA) 11019 return "NCSA"; 11020 if (code == SPDXLicense.NET_SNMP) 11021 return "Net-SNMP"; 11022 if (code == SPDXLicense.NETCDF) 11023 return "NetCDF"; 11024 if (code == SPDXLicense.NEWSLETR) 11025 return "Newsletr"; 11026 if (code == SPDXLicense.NGPL) 11027 return "NGPL"; 11028 if (code == SPDXLicense.NICTA_1_0) 11029 return "NICTA-1.0"; 11030 if (code == SPDXLicense.NIST_PD) 11031 return "NIST-PD"; 11032 if (code == SPDXLicense.NIST_PD_FALLBACK) 11033 return "NIST-PD-fallback"; 11034 if (code == SPDXLicense.NIST_SOFTWARE) 11035 return "NIST-Software"; 11036 if (code == SPDXLicense.NLOD_1_0) 11037 return "NLOD-1.0"; 11038 if (code == SPDXLicense.NLOD_2_0) 11039 return "NLOD-2.0"; 11040 if (code == SPDXLicense.NLPL) 11041 return "NLPL"; 11042 if (code == SPDXLicense.NOKIA) 11043 return "Nokia"; 11044 if (code == SPDXLicense.NOSL) 11045 return "NOSL"; 11046 if (code == SPDXLicense.NOT_OPEN_SOURCE) 11047 return "not-open-source"; 11048 if (code == SPDXLicense.NOWEB) 11049 return "Noweb"; 11050 if (code == SPDXLicense.NPL_1_0) 11051 return "NPL-1.0"; 11052 if (code == SPDXLicense.NPL_1_1) 11053 return "NPL-1.1"; 11054 if (code == SPDXLicense.NPOSL_3_0) 11055 return "NPOSL-3.0"; 11056 if (code == SPDXLicense.NRL) 11057 return "NRL"; 11058 if (code == SPDXLicense.NTP) 11059 return "NTP"; 11060 if (code == SPDXLicense.NTP_0) 11061 return "NTP-0"; 11062 if (code == SPDXLicense.NUNIT) 11063 return "Nunit"; 11064 if (code == SPDXLicense.O_UDA_1_0) 11065 return "O-UDA-1.0"; 11066 if (code == SPDXLicense.OCCT_PL) 11067 return "OCCT-PL"; 11068 if (code == SPDXLicense.OCLC_2_0) 11069 return "OCLC-2.0"; 11070 if (code == SPDXLicense.ODBL_1_0) 11071 return "ODbL-1.0"; 11072 if (code == SPDXLicense.ODC_BY_1_0) 11073 return "ODC-By-1.0"; 11074 if (code == SPDXLicense.OFFIS) 11075 return "OFFIS"; 11076 if (code == SPDXLicense.OFL_1_0) 11077 return "OFL-1.0"; 11078 if (code == SPDXLicense.OFL_1_0_NO_RFN) 11079 return "OFL-1.0-no-RFN"; 11080 if (code == SPDXLicense.OFL_1_0_RFN) 11081 return "OFL-1.0-RFN"; 11082 if (code == SPDXLicense.OFL_1_1) 11083 return "OFL-1.1"; 11084 if (code == SPDXLicense.OFL_1_1_NO_RFN) 11085 return "OFL-1.1-no-RFN"; 11086 if (code == SPDXLicense.OFL_1_1_RFN) 11087 return "OFL-1.1-RFN"; 11088 if (code == SPDXLicense.OGC_1_0) 11089 return "OGC-1.0"; 11090 if (code == SPDXLicense.OGDL_TAIWAN_1_0) 11091 return "OGDL-Taiwan-1.0"; 11092 if (code == SPDXLicense.OGL_CANADA_2_0) 11093 return "OGL-Canada-2.0"; 11094 if (code == SPDXLicense.OGL_UK_1_0) 11095 return "OGL-UK-1.0"; 11096 if (code == SPDXLicense.OGL_UK_2_0) 11097 return "OGL-UK-2.0"; 11098 if (code == SPDXLicense.OGL_UK_3_0) 11099 return "OGL-UK-3.0"; 11100 if (code == SPDXLicense.OGTSL) 11101 return "OGTSL"; 11102 if (code == SPDXLicense.OLDAP_1_1) 11103 return "OLDAP-1.1"; 11104 if (code == SPDXLicense.OLDAP_1_2) 11105 return "OLDAP-1.2"; 11106 if (code == SPDXLicense.OLDAP_1_3) 11107 return "OLDAP-1.3"; 11108 if (code == SPDXLicense.OLDAP_1_4) 11109 return "OLDAP-1.4"; 11110 if (code == SPDXLicense.OLDAP_2_0) 11111 return "OLDAP-2.0"; 11112 if (code == SPDXLicense.OLDAP_2_0_1) 11113 return "OLDAP-2.0.1"; 11114 if (code == SPDXLicense.OLDAP_2_1) 11115 return "OLDAP-2.1"; 11116 if (code == SPDXLicense.OLDAP_2_2) 11117 return "OLDAP-2.2"; 11118 if (code == SPDXLicense.OLDAP_2_2_1) 11119 return "OLDAP-2.2.1"; 11120 if (code == SPDXLicense.OLDAP_2_2_2) 11121 return "OLDAP-2.2.2"; 11122 if (code == SPDXLicense.OLDAP_2_3) 11123 return "OLDAP-2.3"; 11124 if (code == SPDXLicense.OLDAP_2_4) 11125 return "OLDAP-2.4"; 11126 if (code == SPDXLicense.OLDAP_2_5) 11127 return "OLDAP-2.5"; 11128 if (code == SPDXLicense.OLDAP_2_6) 11129 return "OLDAP-2.6"; 11130 if (code == SPDXLicense.OLDAP_2_7) 11131 return "OLDAP-2.7"; 11132 if (code == SPDXLicense.OLDAP_2_8) 11133 return "OLDAP-2.8"; 11134 if (code == SPDXLicense.OLFL_1_3) 11135 return "OLFL-1.3"; 11136 if (code == SPDXLicense.OML) 11137 return "OML"; 11138 if (code == SPDXLicense.OPENPBS_2_3) 11139 return "OpenPBS-2.3"; 11140 if (code == SPDXLicense.OPENSSL) 11141 return "OpenSSL"; 11142 if (code == SPDXLicense.OPL_1_0) 11143 return "OPL-1.0"; 11144 if (code == SPDXLicense.OPL_UK_3_0) 11145 return "OPL-UK-3.0"; 11146 if (code == SPDXLicense.OPUBL_1_0) 11147 return "OPUBL-1.0"; 11148 if (code == SPDXLicense.OSET_PL_2_1) 11149 return "OSET-PL-2.1"; 11150 if (code == SPDXLicense.OSL_1_0) 11151 return "OSL-1.0"; 11152 if (code == SPDXLicense.OSL_1_1) 11153 return "OSL-1.1"; 11154 if (code == SPDXLicense.OSL_2_0) 11155 return "OSL-2.0"; 11156 if (code == SPDXLicense.OSL_2_1) 11157 return "OSL-2.1"; 11158 if (code == SPDXLicense.OSL_3_0) 11159 return "OSL-3.0"; 11160 if (code == SPDXLicense.PARITY_6_0_0) 11161 return "Parity-6.0.0"; 11162 if (code == SPDXLicense.PARITY_7_0_0) 11163 return "Parity-7.0.0"; 11164 if (code == SPDXLicense.PDDL_1_0) 11165 return "PDDL-1.0"; 11166 if (code == SPDXLicense.PHP_3_0) 11167 return "PHP-3.0"; 11168 if (code == SPDXLicense.PHP_3_01) 11169 return "PHP-3.01"; 11170 if (code == SPDXLicense.PLEXUS) 11171 return "Plexus"; 11172 if (code == SPDXLicense.POLYFORM_NONCOMMERCIAL_1_0_0) 11173 return "PolyForm-Noncommercial-1.0.0"; 11174 if (code == SPDXLicense.POLYFORM_SMALL_BUSINESS_1_0_0) 11175 return "PolyForm-Small-Business-1.0.0"; 11176 if (code == SPDXLicense.POSTGRESQL) 11177 return "PostgreSQL"; 11178 if (code == SPDXLicense.PSF_2_0) 11179 return "PSF-2.0"; 11180 if (code == SPDXLicense.PSFRAG) 11181 return "psfrag"; 11182 if (code == SPDXLicense.PSUTILS) 11183 return "psutils"; 11184 if (code == SPDXLicense.PYTHON_2_0) 11185 return "Python-2.0"; 11186 if (code == SPDXLicense.PYTHON_2_0_1) 11187 return "Python-2.0.1"; 11188 if (code == SPDXLicense.QHULL) 11189 return "Qhull"; 11190 if (code == SPDXLicense.QPL_1_0) 11191 return "QPL-1.0"; 11192 if (code == SPDXLicense.QPL_1_0_INRIA_2004) 11193 return "QPL-1.0-INRIA-2004"; 11194 if (code == SPDXLicense.RDISC) 11195 return "Rdisc"; 11196 if (code == SPDXLicense.RHECOS_1_1) 11197 return "RHeCos-1.1"; 11198 if (code == SPDXLicense.RPL_1_1) 11199 return "RPL-1.1"; 11200 if (code == SPDXLicense.RPL_1_5) 11201 return "RPL-1.5"; 11202 if (code == SPDXLicense.RPSL_1_0) 11203 return "RPSL-1.0"; 11204 if (code == SPDXLicense.RSA_MD) 11205 return "RSA-MD"; 11206 if (code == SPDXLicense.RSCPL) 11207 return "RSCPL"; 11208 if (code == SPDXLicense.RUBY) 11209 return "Ruby"; 11210 if (code == SPDXLicense.SAX_PD) 11211 return "SAX-PD"; 11212 if (code == SPDXLicense.SAXPATH) 11213 return "Saxpath"; 11214 if (code == SPDXLicense.SCEA) 11215 return "SCEA"; 11216 if (code == SPDXLicense.SCHEMEREPORT) 11217 return "SchemeReport"; 11218 if (code == SPDXLicense.SENDMAIL) 11219 return "Sendmail"; 11220 if (code == SPDXLicense.SENDMAIL_8_23) 11221 return "Sendmail-8.23"; 11222 if (code == SPDXLicense.SGI_B_1_0) 11223 return "SGI-B-1.0"; 11224 if (code == SPDXLicense.SGI_B_1_1) 11225 return "SGI-B-1.1"; 11226 if (code == SPDXLicense.SGI_B_2_0) 11227 return "SGI-B-2.0"; 11228 if (code == SPDXLicense.SGP4) 11229 return "SGP4"; 11230 if (code == SPDXLicense.SHL_0_5) 11231 return "SHL-0.5"; 11232 if (code == SPDXLicense.SHL_0_51) 11233 return "SHL-0.51"; 11234 if (code == SPDXLicense.SIMPL_2_0) 11235 return "SimPL-2.0"; 11236 if (code == SPDXLicense.SISSL) 11237 return "SISSL"; 11238 if (code == SPDXLicense.SISSL_1_2) 11239 return "SISSL-1.2"; 11240 if (code == SPDXLicense.SLEEPYCAT) 11241 return "Sleepycat"; 11242 if (code == SPDXLicense.SMLNJ) 11243 return "SMLNJ"; 11244 if (code == SPDXLicense.SMPPL) 11245 return "SMPPL"; 11246 if (code == SPDXLicense.SNIA) 11247 return "SNIA"; 11248 if (code == SPDXLicense.SNPRINTF) 11249 return "snprintf"; 11250 if (code == SPDXLicense.SPENCER_86) 11251 return "Spencer-86"; 11252 if (code == SPDXLicense.SPENCER_94) 11253 return "Spencer-94"; 11254 if (code == SPDXLicense.SPENCER_99) 11255 return "Spencer-99"; 11256 if (code == SPDXLicense.SPL_1_0) 11257 return "SPL-1.0"; 11258 if (code == SPDXLicense.SSH_OPENSSH) 11259 return "SSH-OpenSSH"; 11260 if (code == SPDXLicense.SSH_SHORT) 11261 return "SSH-short"; 11262 if (code == SPDXLicense.SSPL_1_0) 11263 return "SSPL-1.0"; 11264 if (code == SPDXLicense.STANDARDML_NJ) 11265 return "StandardML-NJ"; 11266 if (code == SPDXLicense.SUGARCRM_1_1_3) 11267 return "SugarCRM-1.1.3"; 11268 if (code == SPDXLicense.SUNPRO) 11269 return "SunPro"; 11270 if (code == SPDXLicense.SWL) 11271 return "SWL"; 11272 if (code == SPDXLicense.SYMLINKS) 11273 return "Symlinks"; 11274 if (code == SPDXLicense.TAPR_OHL_1_0) 11275 return "TAPR-OHL-1.0"; 11276 if (code == SPDXLicense.TCL) 11277 return "TCL"; 11278 if (code == SPDXLicense.TCP_WRAPPERS) 11279 return "TCP-wrappers"; 11280 if (code == SPDXLicense.TERMREADKEY) 11281 return "TermReadKey"; 11282 if (code == SPDXLicense.TMATE) 11283 return "TMate"; 11284 if (code == SPDXLicense.TORQUE_1_1) 11285 return "TORQUE-1.1"; 11286 if (code == SPDXLicense.TOSL) 11287 return "TOSL"; 11288 if (code == SPDXLicense.TPDL) 11289 return "TPDL"; 11290 if (code == SPDXLicense.TPL_1_0) 11291 return "TPL-1.0"; 11292 if (code == SPDXLicense.TTWL) 11293 return "TTWL"; 11294 if (code == SPDXLicense.TU_BERLIN_1_0) 11295 return "TU-Berlin-1.0"; 11296 if (code == SPDXLicense.TU_BERLIN_2_0) 11297 return "TU-Berlin-2.0"; 11298 if (code == SPDXLicense.UCAR) 11299 return "UCAR"; 11300 if (code == SPDXLicense.UCL_1_0) 11301 return "UCL-1.0"; 11302 if (code == SPDXLicense.UNICODE_DFS_2015) 11303 return "Unicode-DFS-2015"; 11304 if (code == SPDXLicense.UNICODE_DFS_2016) 11305 return "Unicode-DFS-2016"; 11306 if (code == SPDXLicense.UNICODE_TOU) 11307 return "Unicode-TOU"; 11308 if (code == SPDXLicense.UNIXCRYPT) 11309 return "UnixCrypt"; 11310 if (code == SPDXLicense.UNLICENSE) 11311 return "Unlicense"; 11312 if (code == SPDXLicense.UPL_1_0) 11313 return "UPL-1.0"; 11314 if (code == SPDXLicense.VIM) 11315 return "Vim"; 11316 if (code == SPDXLicense.VOSTROM) 11317 return "VOSTROM"; 11318 if (code == SPDXLicense.VSL_1_0) 11319 return "VSL-1.0"; 11320 if (code == SPDXLicense.W3C) 11321 return "W3C"; 11322 if (code == SPDXLicense.W3C_19980720) 11323 return "W3C-19980720"; 11324 if (code == SPDXLicense.W3C_20150513) 11325 return "W3C-20150513"; 11326 if (code == SPDXLicense.W3M) 11327 return "w3m"; 11328 if (code == SPDXLicense.WATCOM_1_0) 11329 return "Watcom-1.0"; 11330 if (code == SPDXLicense.WIDGET_WORKSHOP) 11331 return "Widget-Workshop"; 11332 if (code == SPDXLicense.WSUIPA) 11333 return "Wsuipa"; 11334 if (code == SPDXLicense.WTFPL) 11335 return "WTFPL"; 11336 if (code == SPDXLicense.WXWINDOWS) 11337 return "wxWindows"; 11338 if (code == SPDXLicense.X11) 11339 return "X11"; 11340 if (code == SPDXLicense.X11_DISTRIBUTE_MODIFICATIONS_VARIANT) 11341 return "X11-distribute-modifications-variant"; 11342 if (code == SPDXLicense.XDEBUG_1_03) 11343 return "Xdebug-1.03"; 11344 if (code == SPDXLicense.XEROX) 11345 return "Xerox"; 11346 if (code == SPDXLicense.XFIG) 11347 return "Xfig"; 11348 if (code == SPDXLicense.XFREE86_1_1) 11349 return "XFree86-1.1"; 11350 if (code == SPDXLicense.XINETD) 11351 return "xinetd"; 11352 if (code == SPDXLicense.XLOCK) 11353 return "xlock"; 11354 if (code == SPDXLicense.XNET) 11355 return "Xnet"; 11356 if (code == SPDXLicense.XPP) 11357 return "xpp"; 11358 if (code == SPDXLicense.XSKAT) 11359 return "XSkat"; 11360 if (code == SPDXLicense.YPL_1_0) 11361 return "YPL-1.0"; 11362 if (code == SPDXLicense.YPL_1_1) 11363 return "YPL-1.1"; 11364 if (code == SPDXLicense.ZED) 11365 return "Zed"; 11366 if (code == SPDXLicense.ZEND_2_0) 11367 return "Zend-2.0"; 11368 if (code == SPDXLicense.ZIMBRA_1_3) 11369 return "Zimbra-1.3"; 11370 if (code == SPDXLicense.ZIMBRA_1_4) 11371 return "Zimbra-1.4"; 11372 if (code == SPDXLicense.ZLIB) 11373 return "Zlib"; 11374 if (code == SPDXLicense.ZLIB_ACKNOWLEDGEMENT) 11375 return "zlib-acknowledgement"; 11376 if (code == SPDXLicense.ZPL_1_1) 11377 return "ZPL-1.1"; 11378 if (code == SPDXLicense.ZPL_2_0) 11379 return "ZPL-2.0"; 11380 if (code == SPDXLicense.ZPL_2_1) 11381 return "ZPL-2.1"; 11382 return "?"; 11383 } 11384 11385 public String toSystem(SPDXLicense code) { 11386 return code.getSystem(); 11387 } 11388 } 11389 11390 public enum GuidePageGeneration { 11391 /** 11392 * Page is proper xhtml with no templating. Will be brought across unchanged for 11393 * standard post-processing. 11394 */ 11395 HTML, 11396 /** 11397 * Page is markdown with templating. Will use the template to create a file that 11398 * imports the markdown file prior to post-processing. 11399 */ 11400 MARKDOWN, 11401 /** 11402 * Page is xml with templating. Will use the template to create a file that 11403 * imports the source file and run the nominated XSLT transform (see parameters) 11404 * if present prior to post-processing. 11405 */ 11406 XML, 11407 /** 11408 * Page will be generated by the publication process - no source to bring 11409 * across. 11410 */ 11411 GENERATED, 11412 /** 11413 * added to help the parsers with the generic types 11414 */ 11415 NULL; 11416 11417 public static GuidePageGeneration fromCode(String codeString) throws FHIRException { 11418 if (codeString == null || "".equals(codeString)) 11419 return null; 11420 if ("html".equals(codeString)) 11421 return HTML; 11422 if ("markdown".equals(codeString)) 11423 return MARKDOWN; 11424 if ("xml".equals(codeString)) 11425 return XML; 11426 if ("generated".equals(codeString)) 11427 return GENERATED; 11428 if (Configuration.isAcceptInvalidEnums()) 11429 return null; 11430 else 11431 throw new FHIRException("Unknown GuidePageGeneration code '" + codeString + "'"); 11432 } 11433 11434 public String toCode() { 11435 switch (this) { 11436 case HTML: 11437 return "html"; 11438 case MARKDOWN: 11439 return "markdown"; 11440 case XML: 11441 return "xml"; 11442 case GENERATED: 11443 return "generated"; 11444 case NULL: 11445 return null; 11446 default: 11447 return "?"; 11448 } 11449 } 11450 11451 public String getSystem() { 11452 switch (this) { 11453 case HTML: 11454 return "http://hl7.org/fhir/guide-page-generation"; 11455 case MARKDOWN: 11456 return "http://hl7.org/fhir/guide-page-generation"; 11457 case XML: 11458 return "http://hl7.org/fhir/guide-page-generation"; 11459 case GENERATED: 11460 return "http://hl7.org/fhir/guide-page-generation"; 11461 case NULL: 11462 return null; 11463 default: 11464 return "?"; 11465 } 11466 } 11467 11468 public String getDefinition() { 11469 switch (this) { 11470 case HTML: 11471 return "Page is proper xhtml with no templating. Will be brought across unchanged for standard post-processing."; 11472 case MARKDOWN: 11473 return "Page is markdown with templating. Will use the template to create a file that imports the markdown file prior to post-processing."; 11474 case XML: 11475 return "Page is xml with templating. Will use the template to create a file that imports the source file and run the nominated XSLT transform (see parameters) if present prior to post-processing."; 11476 case GENERATED: 11477 return "Page will be generated by the publication process - no source to bring across."; 11478 case NULL: 11479 return null; 11480 default: 11481 return "?"; 11482 } 11483 } 11484 11485 public String getDisplay() { 11486 switch (this) { 11487 case HTML: 11488 return "HTML"; 11489 case MARKDOWN: 11490 return "Markdown"; 11491 case XML: 11492 return "XML"; 11493 case GENERATED: 11494 return "Generated"; 11495 case NULL: 11496 return null; 11497 default: 11498 return "?"; 11499 } 11500 } 11501 } 11502 11503 public static class GuidePageGenerationEnumFactory implements EnumFactory<GuidePageGeneration> { 11504 public GuidePageGeneration fromCode(String codeString) throws IllegalArgumentException { 11505 if (codeString == null || "".equals(codeString)) 11506 if (codeString == null || "".equals(codeString)) 11507 return null; 11508 if ("html".equals(codeString)) 11509 return GuidePageGeneration.HTML; 11510 if ("markdown".equals(codeString)) 11511 return GuidePageGeneration.MARKDOWN; 11512 if ("xml".equals(codeString)) 11513 return GuidePageGeneration.XML; 11514 if ("generated".equals(codeString)) 11515 return GuidePageGeneration.GENERATED; 11516 throw new IllegalArgumentException("Unknown GuidePageGeneration code '" + codeString + "'"); 11517 } 11518 11519 public Enumeration<GuidePageGeneration> fromType(PrimitiveType<?> code) throws FHIRException { 11520 if (code == null) 11521 return null; 11522 if (code.isEmpty()) 11523 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.NULL, code); 11524 String codeString = code.asStringValue(); 11525 if (codeString == null || "".equals(codeString)) 11526 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.NULL, code); 11527 if ("html".equals(codeString)) 11528 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.HTML, code); 11529 if ("markdown".equals(codeString)) 11530 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.MARKDOWN, code); 11531 if ("xml".equals(codeString)) 11532 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.XML, code); 11533 if ("generated".equals(codeString)) 11534 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.GENERATED, code); 11535 throw new FHIRException("Unknown GuidePageGeneration code '" + codeString + "'"); 11536 } 11537 11538 public String toCode(GuidePageGeneration code) { 11539 if (code == GuidePageGeneration.NULL) 11540 return null; 11541 if (code == GuidePageGeneration.HTML) 11542 return "html"; 11543 if (code == GuidePageGeneration.MARKDOWN) 11544 return "markdown"; 11545 if (code == GuidePageGeneration.XML) 11546 return "xml"; 11547 if (code == GuidePageGeneration.GENERATED) 11548 return "generated"; 11549 return "?"; 11550 } 11551 11552 public String toSystem(GuidePageGeneration code) { 11553 return code.getSystem(); 11554 } 11555 } 11556 11557 public enum GuideParameterCode { 11558 /** 11559 * If the value of this string 0..* parameter is one of the metadata fields then 11560 * all conformance resources will have any specified [Resource].[field] 11561 * overwritten with the ImplementationGuide.[field], where field is one of: 11562 * version, date, status, publisher, contact, copyright, experimental, 11563 * jurisdiction, useContext. 11564 */ 11565 APPLY, 11566 /** 11567 * The value of this string 0..* parameter is a subfolder of the build context's 11568 * location that is to be scanned to load resources. Scope is (if present) a 11569 * particular resource type. 11570 */ 11571 PATHRESOURCE, 11572 /** 11573 * The value of this string 0..1 parameter is a subfolder of the build context's 11574 * location that contains files that are part of the html content processed by 11575 * the builder. 11576 */ 11577 PATHPAGES, 11578 /** 11579 * The value of this string 0..1 parameter is a subfolder of the build context's 11580 * location that is used as the terminology cache. If this is not present, the 11581 * terminology cache is on the local system, not under version control. 11582 */ 11583 PATHTXCACHE, 11584 /** 11585 * The value of this string 0..* parameter is a parameter (name=value) when 11586 * expanding value sets for this implementation guide. This is particularly used 11587 * to specify the versions of published terminologies such as SNOMED CT. 11588 */ 11589 EXPANSIONPARAMETER, 11590 /** 11591 * The value of this string 0..1 parameter is either "warning" or "error" 11592 * (default = "error"). If the value is "warning" then IG build tools allow the 11593 * IG to be considered successfully build even when there is no internal broken 11594 * links. 11595 */ 11596 RULEBROKENLINKS, 11597 /** 11598 * The value of this boolean 0..1 parameter specifies whether the IG publisher 11599 * creates examples in XML format. If not present, the Publication Tool decides 11600 * whether to generate XML. 11601 */ 11602 GENERATEXML, 11603 /** 11604 * The value of this boolean 0..1 parameter specifies whether the IG publisher 11605 * creates examples in JSON format. If not present, the Publication Tool decides 11606 * whether to generate JSON. 11607 */ 11608 GENERATEJSON, 11609 /** 11610 * The value of this boolean 0..1 parameter specifies whether the IG publisher 11611 * creates examples in Turtle format. If not present, the Publication Tool 11612 * decides whether to generate Turtle. 11613 */ 11614 GENERATETURTLE, 11615 /** 11616 * The value of this string singleton parameter is the name of the file to use 11617 * as the builder template for each generated page (see templating). 11618 */ 11619 HTMLTEMPLATE, 11620 /** 11621 * added to help the parsers with the generic types 11622 */ 11623 NULL; 11624 11625 public static GuideParameterCode fromCode(String codeString) throws FHIRException { 11626 if (codeString == null || "".equals(codeString)) 11627 return null; 11628 if ("apply".equals(codeString)) 11629 return APPLY; 11630 if ("path-resource".equals(codeString)) 11631 return PATHRESOURCE; 11632 if ("path-pages".equals(codeString)) 11633 return PATHPAGES; 11634 if ("path-tx-cache".equals(codeString)) 11635 return PATHTXCACHE; 11636 if ("expansion-parameter".equals(codeString)) 11637 return EXPANSIONPARAMETER; 11638 if ("rule-broken-links".equals(codeString)) 11639 return RULEBROKENLINKS; 11640 if ("generate-xml".equals(codeString)) 11641 return GENERATEXML; 11642 if ("generate-json".equals(codeString)) 11643 return GENERATEJSON; 11644 if ("generate-turtle".equals(codeString)) 11645 return GENERATETURTLE; 11646 if ("html-template".equals(codeString)) 11647 return HTMLTEMPLATE; 11648 if (Configuration.isAcceptInvalidEnums()) 11649 return null; 11650 else 11651 throw new FHIRException("Unknown GuideParameterCode code '" + codeString + "'"); 11652 } 11653 11654 public String toCode() { 11655 switch (this) { 11656 case APPLY: 11657 return "apply"; 11658 case PATHRESOURCE: 11659 return "path-resource"; 11660 case PATHPAGES: 11661 return "path-pages"; 11662 case PATHTXCACHE: 11663 return "path-tx-cache"; 11664 case EXPANSIONPARAMETER: 11665 return "expansion-parameter"; 11666 case RULEBROKENLINKS: 11667 return "rule-broken-links"; 11668 case GENERATEXML: 11669 return "generate-xml"; 11670 case GENERATEJSON: 11671 return "generate-json"; 11672 case GENERATETURTLE: 11673 return "generate-turtle"; 11674 case HTMLTEMPLATE: 11675 return "html-template"; 11676 case NULL: 11677 return null; 11678 default: 11679 return "?"; 11680 } 11681 } 11682 11683 public String getSystem() { 11684 switch (this) { 11685 case APPLY: 11686 return "http://hl7.org/fhir/guide-parameter-code"; 11687 case PATHRESOURCE: 11688 return "http://hl7.org/fhir/guide-parameter-code"; 11689 case PATHPAGES: 11690 return "http://hl7.org/fhir/guide-parameter-code"; 11691 case PATHTXCACHE: 11692 return "http://hl7.org/fhir/guide-parameter-code"; 11693 case EXPANSIONPARAMETER: 11694 return "http://hl7.org/fhir/guide-parameter-code"; 11695 case RULEBROKENLINKS: 11696 return "http://hl7.org/fhir/guide-parameter-code"; 11697 case GENERATEXML: 11698 return "http://hl7.org/fhir/guide-parameter-code"; 11699 case GENERATEJSON: 11700 return "http://hl7.org/fhir/guide-parameter-code"; 11701 case GENERATETURTLE: 11702 return "http://hl7.org/fhir/guide-parameter-code"; 11703 case HTMLTEMPLATE: 11704 return "http://hl7.org/fhir/guide-parameter-code"; 11705 case NULL: 11706 return null; 11707 default: 11708 return "?"; 11709 } 11710 } 11711 11712 public String getDefinition() { 11713 switch (this) { 11714 case APPLY: 11715 return "If the value of this string 0..* parameter is one of the metadata fields then all conformance resources will have any specified [Resource].[field] overwritten with the ImplementationGuide.[field], where field is one of: version, date, status, publisher, contact, copyright, experimental, jurisdiction, useContext."; 11716 case PATHRESOURCE: 11717 return "The value of this string 0..* parameter is a subfolder of the build context's location that is to be scanned to load resources. Scope is (if present) a particular resource type."; 11718 case PATHPAGES: 11719 return "The value of this string 0..1 parameter is a subfolder of the build context's location that contains files that are part of the html content processed by the builder."; 11720 case PATHTXCACHE: 11721 return "The value of this string 0..1 parameter is a subfolder of the build context's location that is used as the terminology cache. If this is not present, the terminology cache is on the local system, not under version control."; 11722 case EXPANSIONPARAMETER: 11723 return "The value of this string 0..* parameter is a parameter (name=value) when expanding value sets for this implementation guide. This is particularly used to specify the versions of published terminologies such as SNOMED CT."; 11724 case RULEBROKENLINKS: 11725 return "The value of this string 0..1 parameter is either \"warning\" or \"error\" (default = \"error\"). If the value is \"warning\" then IG build tools allow the IG to be considered successfully build even when there is no internal broken links."; 11726 case GENERATEXML: 11727 return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in XML format. If not present, the Publication Tool decides whether to generate XML."; 11728 case GENERATEJSON: 11729 return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in JSON format. If not present, the Publication Tool decides whether to generate JSON."; 11730 case GENERATETURTLE: 11731 return "The value of this boolean 0..1 parameter specifies whether the IG publisher creates examples in Turtle format. If not present, the Publication Tool decides whether to generate Turtle."; 11732 case HTMLTEMPLATE: 11733 return "The value of this string singleton parameter is the name of the file to use as the builder template for each generated page (see templating)."; 11734 case NULL: 11735 return null; 11736 default: 11737 return "?"; 11738 } 11739 } 11740 11741 public String getDisplay() { 11742 switch (this) { 11743 case APPLY: 11744 return "Apply Metadata Value"; 11745 case PATHRESOURCE: 11746 return "Resource Path"; 11747 case PATHPAGES: 11748 return "Pages Path"; 11749 case PATHTXCACHE: 11750 return "Terminology Cache Path"; 11751 case EXPANSIONPARAMETER: 11752 return "Expansion Profile"; 11753 case RULEBROKENLINKS: 11754 return "Broken Links Rule"; 11755 case GENERATEXML: 11756 return "Generate XML"; 11757 case GENERATEJSON: 11758 return "Generate JSON"; 11759 case GENERATETURTLE: 11760 return "Generate Turtle"; 11761 case HTMLTEMPLATE: 11762 return "HTML Template"; 11763 case NULL: 11764 return null; 11765 default: 11766 return "?"; 11767 } 11768 } 11769 } 11770 11771 public static class GuideParameterCodeEnumFactory implements EnumFactory<GuideParameterCode> { 11772 public GuideParameterCode fromCode(String codeString) throws IllegalArgumentException { 11773 if (codeString == null || "".equals(codeString)) 11774 if (codeString == null || "".equals(codeString)) 11775 return null; 11776 if ("apply".equals(codeString)) 11777 return GuideParameterCode.APPLY; 11778 if ("path-resource".equals(codeString)) 11779 return GuideParameterCode.PATHRESOURCE; 11780 if ("path-pages".equals(codeString)) 11781 return GuideParameterCode.PATHPAGES; 11782 if ("path-tx-cache".equals(codeString)) 11783 return GuideParameterCode.PATHTXCACHE; 11784 if ("expansion-parameter".equals(codeString)) 11785 return GuideParameterCode.EXPANSIONPARAMETER; 11786 if ("rule-broken-links".equals(codeString)) 11787 return GuideParameterCode.RULEBROKENLINKS; 11788 if ("generate-xml".equals(codeString)) 11789 return GuideParameterCode.GENERATEXML; 11790 if ("generate-json".equals(codeString)) 11791 return GuideParameterCode.GENERATEJSON; 11792 if ("generate-turtle".equals(codeString)) 11793 return GuideParameterCode.GENERATETURTLE; 11794 if ("html-template".equals(codeString)) 11795 return GuideParameterCode.HTMLTEMPLATE; 11796 throw new IllegalArgumentException("Unknown GuideParameterCode code '" + codeString + "'"); 11797 } 11798 11799 public Enumeration<GuideParameterCode> fromType(PrimitiveType<?> code) throws FHIRException { 11800 if (code == null) 11801 return null; 11802 if (code.isEmpty()) 11803 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.NULL, code); 11804 String codeString = code.asStringValue(); 11805 if (codeString == null || "".equals(codeString)) 11806 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.NULL, code); 11807 if ("apply".equals(codeString)) 11808 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.APPLY, code); 11809 if ("path-resource".equals(codeString)) 11810 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHRESOURCE, code); 11811 if ("path-pages".equals(codeString)) 11812 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHPAGES, code); 11813 if ("path-tx-cache".equals(codeString)) 11814 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.PATHTXCACHE, code); 11815 if ("expansion-parameter".equals(codeString)) 11816 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.EXPANSIONPARAMETER, code); 11817 if ("rule-broken-links".equals(codeString)) 11818 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.RULEBROKENLINKS, code); 11819 if ("generate-xml".equals(codeString)) 11820 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATEXML, code); 11821 if ("generate-json".equals(codeString)) 11822 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATEJSON, code); 11823 if ("generate-turtle".equals(codeString)) 11824 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.GENERATETURTLE, code); 11825 if ("html-template".equals(codeString)) 11826 return new Enumeration<GuideParameterCode>(this, GuideParameterCode.HTMLTEMPLATE, code); 11827 throw new FHIRException("Unknown GuideParameterCode code '" + codeString + "'"); 11828 } 11829 11830 public String toCode(GuideParameterCode code) { 11831 if (code == GuideParameterCode.NULL) 11832 return null; 11833 if (code == GuideParameterCode.APPLY) 11834 return "apply"; 11835 if (code == GuideParameterCode.PATHRESOURCE) 11836 return "path-resource"; 11837 if (code == GuideParameterCode.PATHPAGES) 11838 return "path-pages"; 11839 if (code == GuideParameterCode.PATHTXCACHE) 11840 return "path-tx-cache"; 11841 if (code == GuideParameterCode.EXPANSIONPARAMETER) 11842 return "expansion-parameter"; 11843 if (code == GuideParameterCode.RULEBROKENLINKS) 11844 return "rule-broken-links"; 11845 if (code == GuideParameterCode.GENERATEXML) 11846 return "generate-xml"; 11847 if (code == GuideParameterCode.GENERATEJSON) 11848 return "generate-json"; 11849 if (code == GuideParameterCode.GENERATETURTLE) 11850 return "generate-turtle"; 11851 if (code == GuideParameterCode.HTMLTEMPLATE) 11852 return "html-template"; 11853 return "?"; 11854 } 11855 11856 public String toSystem(GuideParameterCode code) { 11857 return code.getSystem(); 11858 } 11859 } 11860 11861 @Block() 11862 public static class ImplementationGuideDependsOnComponent extends BackboneElement implements IBaseBackboneElement { 11863 /** 11864 * A canonical reference to the Implementation guide for the dependency. 11865 */ 11866 @Child(name = "uri", type = { CanonicalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 11867 @Description(shortDefinition = "Identity of the IG that this depends on", formalDefinition = "A canonical reference to the Implementation guide for the dependency.") 11868 protected CanonicalType uri; 11869 11870 /** 11871 * The NPM package name for the Implementation Guide that this IG depends on. 11872 */ 11873 @Child(name = "packageId", type = { IdType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 11874 @Description(shortDefinition = "NPM Package name for IG this depends on", formalDefinition = "The NPM package name for the Implementation Guide that this IG depends on.") 11875 protected IdType packageId; 11876 11877 /** 11878 * The version of the IG that is depended on, when the correct version is 11879 * required to understand the IG correctly. 11880 */ 11881 @Child(name = "version", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 11882 @Description(shortDefinition = "Version of the IG", formalDefinition = "The version of the IG that is depended on, when the correct version is required to understand the IG correctly.") 11883 protected StringType version; 11884 11885 private static final long serialVersionUID = -215808797L; 11886 11887 /** 11888 * Constructor 11889 */ 11890 public ImplementationGuideDependsOnComponent() { 11891 super(); 11892 } 11893 11894 /** 11895 * Constructor 11896 */ 11897 public ImplementationGuideDependsOnComponent(CanonicalType uri) { 11898 super(); 11899 this.uri = uri; 11900 } 11901 11902 /** 11903 * @return {@link #uri} (A canonical reference to the Implementation guide for 11904 * the dependency.). This is the underlying object with id, value and 11905 * extensions. The accessor "getUri" gives direct access to the value 11906 */ 11907 public CanonicalType getUriElement() { 11908 if (this.uri == null) 11909 if (Configuration.errorOnAutoCreate()) 11910 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.uri"); 11911 else if (Configuration.doAutoCreate()) 11912 this.uri = new CanonicalType(); // bb 11913 return this.uri; 11914 } 11915 11916 public boolean hasUriElement() { 11917 return this.uri != null && !this.uri.isEmpty(); 11918 } 11919 11920 public boolean hasUri() { 11921 return this.uri != null && !this.uri.isEmpty(); 11922 } 11923 11924 /** 11925 * @param value {@link #uri} (A canonical reference to the Implementation guide 11926 * for the dependency.). This is the underlying object with id, 11927 * value and extensions. The accessor "getUri" gives direct access 11928 * to the value 11929 */ 11930 public ImplementationGuideDependsOnComponent setUriElement(CanonicalType value) { 11931 this.uri = value; 11932 return this; 11933 } 11934 11935 /** 11936 * @return A canonical reference to the Implementation guide for the dependency. 11937 */ 11938 public String getUri() { 11939 return this.uri == null ? null : this.uri.getValue(); 11940 } 11941 11942 /** 11943 * @param value A canonical reference to the Implementation guide for the 11944 * dependency. 11945 */ 11946 public ImplementationGuideDependsOnComponent setUri(String value) { 11947 if (this.uri == null) 11948 this.uri = new CanonicalType(); 11949 this.uri.setValue(value); 11950 return this; 11951 } 11952 11953 /** 11954 * @return {@link #packageId} (The NPM package name for the Implementation Guide 11955 * that this IG depends on.). This is the underlying object with id, 11956 * value and extensions. The accessor "getPackageId" gives direct access 11957 * to the value 11958 */ 11959 public IdType getPackageIdElement() { 11960 if (this.packageId == null) 11961 if (Configuration.errorOnAutoCreate()) 11962 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.packageId"); 11963 else if (Configuration.doAutoCreate()) 11964 this.packageId = new IdType(); // bb 11965 return this.packageId; 11966 } 11967 11968 public boolean hasPackageIdElement() { 11969 return this.packageId != null && !this.packageId.isEmpty(); 11970 } 11971 11972 public boolean hasPackageId() { 11973 return this.packageId != null && !this.packageId.isEmpty(); 11974 } 11975 11976 /** 11977 * @param value {@link #packageId} (The NPM package name for the Implementation 11978 * Guide that this IG depends on.). This is the underlying object 11979 * with id, value and extensions. The accessor "getPackageId" gives 11980 * direct access to the value 11981 */ 11982 public ImplementationGuideDependsOnComponent setPackageIdElement(IdType value) { 11983 this.packageId = value; 11984 return this; 11985 } 11986 11987 /** 11988 * @return The NPM package name for the Implementation Guide that this IG 11989 * depends on. 11990 */ 11991 public String getPackageId() { 11992 return this.packageId == null ? null : this.packageId.getValue(); 11993 } 11994 11995 /** 11996 * @param value The NPM package name for the Implementation Guide that this IG 11997 * depends on. 11998 */ 11999 public ImplementationGuideDependsOnComponent setPackageId(String value) { 12000 if (Utilities.noString(value)) 12001 this.packageId = null; 12002 else { 12003 if (this.packageId == null) 12004 this.packageId = new IdType(); 12005 this.packageId.setValue(value); 12006 } 12007 return this; 12008 } 12009 12010 /** 12011 * @return {@link #version} (The version of the IG that is depended on, when the 12012 * correct version is required to understand the IG correctly.). This is 12013 * the underlying object with id, value and extensions. The accessor 12014 * "getVersion" gives direct access to the value 12015 */ 12016 public StringType getVersionElement() { 12017 if (this.version == null) 12018 if (Configuration.errorOnAutoCreate()) 12019 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.version"); 12020 else if (Configuration.doAutoCreate()) 12021 this.version = new StringType(); // bb 12022 return this.version; 12023 } 12024 12025 public boolean hasVersionElement() { 12026 return this.version != null && !this.version.isEmpty(); 12027 } 12028 12029 public boolean hasVersion() { 12030 return this.version != null && !this.version.isEmpty(); 12031 } 12032 12033 /** 12034 * @param value {@link #version} (The version of the IG that is depended on, 12035 * when the correct version is required to understand the IG 12036 * correctly.). This is the underlying object with id, value and 12037 * extensions. The accessor "getVersion" gives direct access to the 12038 * value 12039 */ 12040 public ImplementationGuideDependsOnComponent setVersionElement(StringType value) { 12041 this.version = value; 12042 return this; 12043 } 12044 12045 /** 12046 * @return The version of the IG that is depended on, when the correct version 12047 * is required to understand the IG correctly. 12048 */ 12049 public String getVersion() { 12050 return this.version == null ? null : this.version.getValue(); 12051 } 12052 12053 /** 12054 * @param value The version of the IG that is depended on, when the correct 12055 * version is required to understand the IG correctly. 12056 */ 12057 public ImplementationGuideDependsOnComponent setVersion(String value) { 12058 if (Utilities.noString(value)) 12059 this.version = null; 12060 else { 12061 if (this.version == null) 12062 this.version = new StringType(); 12063 this.version.setValue(value); 12064 } 12065 return this; 12066 } 12067 12068 protected void listChildren(List<Property> children) { 12069 super.listChildren(children); 12070 children.add(new Property("uri", "canonical(ImplementationGuide)", 12071 "A canonical reference to the Implementation guide for the dependency.", 0, 1, uri)); 12072 children.add(new Property("packageId", "id", 12073 "The NPM package name for the Implementation Guide that this IG depends on.", 0, 1, packageId)); 12074 children.add(new Property("version", "string", 12075 "The version of the IG that is depended on, when the correct version is required to understand the IG correctly.", 12076 0, 1, version)); 12077 } 12078 12079 @Override 12080 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12081 switch (_hash) { 12082 case 116076: 12083 /* uri */ return new Property("uri", "canonical(ImplementationGuide)", 12084 "A canonical reference to the Implementation guide for the dependency.", 0, 1, uri); 12085 case 1802060801: 12086 /* packageId */ return new Property("packageId", "id", 12087 "The NPM package name for the Implementation Guide that this IG depends on.", 0, 1, packageId); 12088 case 351608024: 12089 /* version */ return new Property("version", "string", 12090 "The version of the IG that is depended on, when the correct version is required to understand the IG correctly.", 12091 0, 1, version); 12092 default: 12093 return super.getNamedProperty(_hash, _name, _checkValid); 12094 } 12095 12096 } 12097 12098 @Override 12099 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12100 switch (hash) { 12101 case 116076: 12102 /* uri */ return this.uri == null ? new Base[0] : new Base[] { this.uri }; // CanonicalType 12103 case 1802060801: 12104 /* packageId */ return this.packageId == null ? new Base[0] : new Base[] { this.packageId }; // IdType 12105 case 351608024: 12106 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 12107 default: 12108 return super.getProperty(hash, name, checkValid); 12109 } 12110 12111 } 12112 12113 @Override 12114 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12115 switch (hash) { 12116 case 116076: // uri 12117 this.uri = castToCanonical(value); // CanonicalType 12118 return value; 12119 case 1802060801: // packageId 12120 this.packageId = castToId(value); // IdType 12121 return value; 12122 case 351608024: // version 12123 this.version = castToString(value); // StringType 12124 return value; 12125 default: 12126 return super.setProperty(hash, name, value); 12127 } 12128 12129 } 12130 12131 @Override 12132 public Base setProperty(String name, Base value) throws FHIRException { 12133 if (name.equals("uri")) { 12134 this.uri = castToCanonical(value); // CanonicalType 12135 } else if (name.equals("packageId")) { 12136 this.packageId = castToId(value); // IdType 12137 } else if (name.equals("version")) { 12138 this.version = castToString(value); // StringType 12139 } else 12140 return super.setProperty(name, value); 12141 return value; 12142 } 12143 12144 @Override 12145 public void removeChild(String name, Base value) throws FHIRException { 12146 if (name.equals("uri")) { 12147 this.uri = null; 12148 } else if (name.equals("packageId")) { 12149 this.packageId = null; 12150 } else if (name.equals("version")) { 12151 this.version = null; 12152 } else 12153 super.removeChild(name, value); 12154 12155 } 12156 12157 @Override 12158 public Base makeProperty(int hash, String name) throws FHIRException { 12159 switch (hash) { 12160 case 116076: 12161 return getUriElement(); 12162 case 1802060801: 12163 return getPackageIdElement(); 12164 case 351608024: 12165 return getVersionElement(); 12166 default: 12167 return super.makeProperty(hash, name); 12168 } 12169 12170 } 12171 12172 @Override 12173 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12174 switch (hash) { 12175 case 116076: 12176 /* uri */ return new String[] { "canonical" }; 12177 case 1802060801: 12178 /* packageId */ return new String[] { "id" }; 12179 case 351608024: 12180 /* version */ return new String[] { "string" }; 12181 default: 12182 return super.getTypesForProperty(hash, name); 12183 } 12184 12185 } 12186 12187 @Override 12188 public Base addChild(String name) throws FHIRException { 12189 if (name.equals("uri")) { 12190 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.uri"); 12191 } else if (name.equals("packageId")) { 12192 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.packageId"); 12193 } else if (name.equals("version")) { 12194 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.version"); 12195 } else 12196 return super.addChild(name); 12197 } 12198 12199 public ImplementationGuideDependsOnComponent copy() { 12200 ImplementationGuideDependsOnComponent dst = new ImplementationGuideDependsOnComponent(); 12201 copyValues(dst); 12202 return dst; 12203 } 12204 12205 public void copyValues(ImplementationGuideDependsOnComponent dst) { 12206 super.copyValues(dst); 12207 dst.uri = uri == null ? null : uri.copy(); 12208 dst.packageId = packageId == null ? null : packageId.copy(); 12209 dst.version = version == null ? null : version.copy(); 12210 } 12211 12212 @Override 12213 public boolean equalsDeep(Base other_) { 12214 if (!super.equalsDeep(other_)) 12215 return false; 12216 if (!(other_ instanceof ImplementationGuideDependsOnComponent)) 12217 return false; 12218 ImplementationGuideDependsOnComponent o = (ImplementationGuideDependsOnComponent) other_; 12219 return compareDeep(uri, o.uri, true) && compareDeep(packageId, o.packageId, true) 12220 && compareDeep(version, o.version, true); 12221 } 12222 12223 @Override 12224 public boolean equalsShallow(Base other_) { 12225 if (!super.equalsShallow(other_)) 12226 return false; 12227 if (!(other_ instanceof ImplementationGuideDependsOnComponent)) 12228 return false; 12229 ImplementationGuideDependsOnComponent o = (ImplementationGuideDependsOnComponent) other_; 12230 return compareValues(packageId, o.packageId, true) && compareValues(version, o.version, true); 12231 } 12232 12233 public boolean isEmpty() { 12234 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uri, packageId, version); 12235 } 12236 12237 public String fhirType() { 12238 return "ImplementationGuide.dependsOn"; 12239 12240 } 12241 12242 } 12243 12244 @Block() 12245 public static class ImplementationGuideGlobalComponent extends BackboneElement implements IBaseBackboneElement { 12246 /** 12247 * The type of resource that all instances must conform to. 12248 */ 12249 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 12250 @Description(shortDefinition = "Type this profile applies to", formalDefinition = "The type of resource that all instances must conform to.") 12251 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 12252 protected CodeType type; 12253 12254 /** 12255 * A reference to the profile that all instances must conform to. 12256 */ 12257 @Child(name = "profile", type = { 12258 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 12259 @Description(shortDefinition = "Profile that all resources must conform to", formalDefinition = "A reference to the profile that all instances must conform to.") 12260 protected CanonicalType profile; 12261 12262 private static final long serialVersionUID = 33894666L; 12263 12264 /** 12265 * Constructor 12266 */ 12267 public ImplementationGuideGlobalComponent() { 12268 super(); 12269 } 12270 12271 /** 12272 * Constructor 12273 */ 12274 public ImplementationGuideGlobalComponent(CodeType type, CanonicalType profile) { 12275 super(); 12276 this.type = type; 12277 this.profile = profile; 12278 } 12279 12280 /** 12281 * @return {@link #type} (The type of resource that all instances must conform 12282 * to.). This is the underlying object with id, value and extensions. 12283 * The accessor "getType" gives direct access to the value 12284 */ 12285 public CodeType getTypeElement() { 12286 if (this.type == null) 12287 if (Configuration.errorOnAutoCreate()) 12288 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.type"); 12289 else if (Configuration.doAutoCreate()) 12290 this.type = new CodeType(); // bb 12291 return this.type; 12292 } 12293 12294 public boolean hasTypeElement() { 12295 return this.type != null && !this.type.isEmpty(); 12296 } 12297 12298 public boolean hasType() { 12299 return this.type != null && !this.type.isEmpty(); 12300 } 12301 12302 /** 12303 * @param value {@link #type} (The type of resource that all instances must 12304 * conform to.). This is the underlying object with id, value and 12305 * extensions. The accessor "getType" gives direct access to the 12306 * value 12307 */ 12308 public ImplementationGuideGlobalComponent setTypeElement(CodeType value) { 12309 this.type = value; 12310 return this; 12311 } 12312 12313 /** 12314 * @return The type of resource that all instances must conform to. 12315 */ 12316 public String getType() { 12317 return this.type == null ? null : this.type.getValue(); 12318 } 12319 12320 /** 12321 * @param value The type of resource that all instances must conform to. 12322 */ 12323 public ImplementationGuideGlobalComponent setType(String value) { 12324 if (this.type == null) 12325 this.type = new CodeType(); 12326 this.type.setValue(value); 12327 return this; 12328 } 12329 12330 /** 12331 * @return {@link #profile} (A reference to the profile that all instances must 12332 * conform to.). This is the underlying object with id, value and 12333 * extensions. The accessor "getProfile" gives direct access to the 12334 * value 12335 */ 12336 public CanonicalType getProfileElement() { 12337 if (this.profile == null) 12338 if (Configuration.errorOnAutoCreate()) 12339 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.profile"); 12340 else if (Configuration.doAutoCreate()) 12341 this.profile = new CanonicalType(); // bb 12342 return this.profile; 12343 } 12344 12345 public boolean hasProfileElement() { 12346 return this.profile != null && !this.profile.isEmpty(); 12347 } 12348 12349 public boolean hasProfile() { 12350 return this.profile != null && !this.profile.isEmpty(); 12351 } 12352 12353 /** 12354 * @param value {@link #profile} (A reference to the profile that all instances 12355 * must conform to.). This is the underlying object with id, value 12356 * and extensions. The accessor "getProfile" gives direct access to 12357 * the value 12358 */ 12359 public ImplementationGuideGlobalComponent setProfileElement(CanonicalType value) { 12360 this.profile = value; 12361 return this; 12362 } 12363 12364 /** 12365 * @return A reference to the profile that all instances must conform to. 12366 */ 12367 public String getProfile() { 12368 return this.profile == null ? null : this.profile.getValue(); 12369 } 12370 12371 /** 12372 * @param value A reference to the profile that all instances must conform to. 12373 */ 12374 public ImplementationGuideGlobalComponent setProfile(String value) { 12375 if (this.profile == null) 12376 this.profile = new CanonicalType(); 12377 this.profile.setValue(value); 12378 return this; 12379 } 12380 12381 protected void listChildren(List<Property> children) { 12382 super.listChildren(children); 12383 children 12384 .add(new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1, type)); 12385 children.add(new Property("profile", "canonical(StructureDefinition)", 12386 "A reference to the profile that all instances must conform to.", 0, 1, profile)); 12387 } 12388 12389 @Override 12390 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12391 switch (_hash) { 12392 case 3575610: 12393 /* type */ return new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1, 12394 type); 12395 case -309425751: 12396 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 12397 "A reference to the profile that all instances must conform to.", 0, 1, profile); 12398 default: 12399 return super.getNamedProperty(_hash, _name, _checkValid); 12400 } 12401 12402 } 12403 12404 @Override 12405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12406 switch (hash) { 12407 case 3575610: 12408 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 12409 case -309425751: 12410 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 12411 default: 12412 return super.getProperty(hash, name, checkValid); 12413 } 12414 12415 } 12416 12417 @Override 12418 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12419 switch (hash) { 12420 case 3575610: // type 12421 this.type = castToCode(value); // CodeType 12422 return value; 12423 case -309425751: // profile 12424 this.profile = castToCanonical(value); // CanonicalType 12425 return value; 12426 default: 12427 return super.setProperty(hash, name, value); 12428 } 12429 12430 } 12431 12432 @Override 12433 public Base setProperty(String name, Base value) throws FHIRException { 12434 if (name.equals("type")) { 12435 this.type = castToCode(value); // CodeType 12436 } else if (name.equals("profile")) { 12437 this.profile = castToCanonical(value); // CanonicalType 12438 } else 12439 return super.setProperty(name, value); 12440 return value; 12441 } 12442 12443 @Override 12444 public void removeChild(String name, Base value) throws FHIRException { 12445 if (name.equals("type")) { 12446 this.type = null; 12447 } else if (name.equals("profile")) { 12448 this.profile = null; 12449 } else 12450 super.removeChild(name, value); 12451 12452 } 12453 12454 @Override 12455 public Base makeProperty(int hash, String name) throws FHIRException { 12456 switch (hash) { 12457 case 3575610: 12458 return getTypeElement(); 12459 case -309425751: 12460 return getProfileElement(); 12461 default: 12462 return super.makeProperty(hash, name); 12463 } 12464 12465 } 12466 12467 @Override 12468 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12469 switch (hash) { 12470 case 3575610: 12471 /* type */ return new String[] { "code" }; 12472 case -309425751: 12473 /* profile */ return new String[] { "canonical" }; 12474 default: 12475 return super.getTypesForProperty(hash, name); 12476 } 12477 12478 } 12479 12480 @Override 12481 public Base addChild(String name) throws FHIRException { 12482 if (name.equals("type")) { 12483 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 12484 } else if (name.equals("profile")) { 12485 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.profile"); 12486 } else 12487 return super.addChild(name); 12488 } 12489 12490 public ImplementationGuideGlobalComponent copy() { 12491 ImplementationGuideGlobalComponent dst = new ImplementationGuideGlobalComponent(); 12492 copyValues(dst); 12493 return dst; 12494 } 12495 12496 public void copyValues(ImplementationGuideGlobalComponent dst) { 12497 super.copyValues(dst); 12498 dst.type = type == null ? null : type.copy(); 12499 dst.profile = profile == null ? null : profile.copy(); 12500 } 12501 12502 @Override 12503 public boolean equalsDeep(Base other_) { 12504 if (!super.equalsDeep(other_)) 12505 return false; 12506 if (!(other_ instanceof ImplementationGuideGlobalComponent)) 12507 return false; 12508 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other_; 12509 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true); 12510 } 12511 12512 @Override 12513 public boolean equalsShallow(Base other_) { 12514 if (!super.equalsShallow(other_)) 12515 return false; 12516 if (!(other_ instanceof ImplementationGuideGlobalComponent)) 12517 return false; 12518 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other_; 12519 return compareValues(type, o.type, true); 12520 } 12521 12522 public boolean isEmpty() { 12523 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile); 12524 } 12525 12526 public String fhirType() { 12527 return "ImplementationGuide.global"; 12528 12529 } 12530 12531 } 12532 12533 @Block() 12534 public static class ImplementationGuideDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 12535 /** 12536 * A logical group of resources. Logical groups can be used when building pages. 12537 */ 12538 @Child(name = "grouping", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 12539 @Description(shortDefinition = "Grouping used to present related resources in the IG", formalDefinition = "A logical group of resources. Logical groups can be used when building pages.") 12540 protected List<ImplementationGuideDefinitionGroupingComponent> grouping; 12541 12542 /** 12543 * A resource that is part of the implementation guide. Conformance resources 12544 * (value set, structure definition, capability statements etc.) are obvious 12545 * candidates for inclusion, but any kind of resource can be included as an 12546 * example resource. 12547 */ 12548 @Child(name = "resource", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 12549 @Description(shortDefinition = "Resource in the implementation guide", formalDefinition = "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.") 12550 protected List<ImplementationGuideDefinitionResourceComponent> resource; 12551 12552 /** 12553 * A page / section in the implementation guide. The root page is the 12554 * implementation guide home page. 12555 */ 12556 @Child(name = "page", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 12557 @Description(shortDefinition = "Page/Section in the Guide", formalDefinition = "A page / section in the implementation guide. The root page is the implementation guide home page.") 12558 protected ImplementationGuideDefinitionPageComponent page; 12559 12560 /** 12561 * Defines how IG is built by tools. 12562 */ 12563 @Child(name = "parameter", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 12564 @Description(shortDefinition = "Defines how IG is built by tools", formalDefinition = "Defines how IG is built by tools.") 12565 protected List<ImplementationGuideDefinitionParameterComponent> parameter; 12566 12567 /** 12568 * A template for building resources. 12569 */ 12570 @Child(name = "template", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 12571 @Description(shortDefinition = "A template for building resources", formalDefinition = "A template for building resources.") 12572 protected List<ImplementationGuideDefinitionTemplateComponent> template; 12573 12574 private static final long serialVersionUID = 179051968L; 12575 12576 /** 12577 * Constructor 12578 */ 12579 public ImplementationGuideDefinitionComponent() { 12580 super(); 12581 } 12582 12583 /** 12584 * @return {@link #grouping} (A logical group of resources. Logical groups can 12585 * be used when building pages.) 12586 */ 12587 public List<ImplementationGuideDefinitionGroupingComponent> getGrouping() { 12588 if (this.grouping == null) 12589 this.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 12590 return this.grouping; 12591 } 12592 12593 /** 12594 * @return Returns a reference to <code>this</code> for easy method chaining 12595 */ 12596 public ImplementationGuideDefinitionComponent setGrouping( 12597 List<ImplementationGuideDefinitionGroupingComponent> theGrouping) { 12598 this.grouping = theGrouping; 12599 return this; 12600 } 12601 12602 public boolean hasGrouping() { 12603 if (this.grouping == null) 12604 return false; 12605 for (ImplementationGuideDefinitionGroupingComponent item : this.grouping) 12606 if (!item.isEmpty()) 12607 return true; 12608 return false; 12609 } 12610 12611 public ImplementationGuideDefinitionGroupingComponent addGrouping() { // 3 12612 ImplementationGuideDefinitionGroupingComponent t = new ImplementationGuideDefinitionGroupingComponent(); 12613 if (this.grouping == null) 12614 this.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 12615 this.grouping.add(t); 12616 return t; 12617 } 12618 12619 public ImplementationGuideDefinitionComponent addGrouping(ImplementationGuideDefinitionGroupingComponent t) { // 3 12620 if (t == null) 12621 return this; 12622 if (this.grouping == null) 12623 this.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 12624 this.grouping.add(t); 12625 return this; 12626 } 12627 12628 /** 12629 * @return The first repetition of repeating field {@link #grouping}, creating 12630 * it if it does not already exist 12631 */ 12632 public ImplementationGuideDefinitionGroupingComponent getGroupingFirstRep() { 12633 if (getGrouping().isEmpty()) { 12634 addGrouping(); 12635 } 12636 return getGrouping().get(0); 12637 } 12638 12639 /** 12640 * @return {@link #resource} (A resource that is part of the implementation 12641 * guide. Conformance resources (value set, structure definition, 12642 * capability statements etc.) are obvious candidates for inclusion, but 12643 * any kind of resource can be included as an example resource.) 12644 */ 12645 public List<ImplementationGuideDefinitionResourceComponent> getResource() { 12646 if (this.resource == null) 12647 this.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 12648 return this.resource; 12649 } 12650 12651 /** 12652 * @return Returns a reference to <code>this</code> for easy method chaining 12653 */ 12654 public ImplementationGuideDefinitionComponent setResource( 12655 List<ImplementationGuideDefinitionResourceComponent> theResource) { 12656 this.resource = theResource; 12657 return this; 12658 } 12659 12660 public boolean hasResource() { 12661 if (this.resource == null) 12662 return false; 12663 for (ImplementationGuideDefinitionResourceComponent item : this.resource) 12664 if (!item.isEmpty()) 12665 return true; 12666 return false; 12667 } 12668 12669 public ImplementationGuideDefinitionResourceComponent addResource() { // 3 12670 ImplementationGuideDefinitionResourceComponent t = new ImplementationGuideDefinitionResourceComponent(); 12671 if (this.resource == null) 12672 this.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 12673 this.resource.add(t); 12674 return t; 12675 } 12676 12677 public ImplementationGuideDefinitionComponent addResource(ImplementationGuideDefinitionResourceComponent t) { // 3 12678 if (t == null) 12679 return this; 12680 if (this.resource == null) 12681 this.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 12682 this.resource.add(t); 12683 return this; 12684 } 12685 12686 /** 12687 * @return The first repetition of repeating field {@link #resource}, creating 12688 * it if it does not already exist 12689 */ 12690 public ImplementationGuideDefinitionResourceComponent getResourceFirstRep() { 12691 if (getResource().isEmpty()) { 12692 addResource(); 12693 } 12694 return getResource().get(0); 12695 } 12696 12697 /** 12698 * @return {@link #page} (A page / section in the implementation guide. The root 12699 * page is the implementation guide home page.) 12700 */ 12701 public ImplementationGuideDefinitionPageComponent getPage() { 12702 if (this.page == null) 12703 if (Configuration.errorOnAutoCreate()) 12704 throw new Error("Attempt to auto-create ImplementationGuideDefinitionComponent.page"); 12705 else if (Configuration.doAutoCreate()) 12706 this.page = new ImplementationGuideDefinitionPageComponent(); // cc 12707 return this.page; 12708 } 12709 12710 public boolean hasPage() { 12711 return this.page != null && !this.page.isEmpty(); 12712 } 12713 12714 /** 12715 * @param value {@link #page} (A page / section in the implementation guide. The 12716 * root page is the implementation guide home page.) 12717 */ 12718 public ImplementationGuideDefinitionComponent setPage(ImplementationGuideDefinitionPageComponent value) { 12719 this.page = value; 12720 return this; 12721 } 12722 12723 /** 12724 * @return {@link #parameter} (Defines how IG is built by tools.) 12725 */ 12726 public List<ImplementationGuideDefinitionParameterComponent> getParameter() { 12727 if (this.parameter == null) 12728 this.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 12729 return this.parameter; 12730 } 12731 12732 /** 12733 * @return Returns a reference to <code>this</code> for easy method chaining 12734 */ 12735 public ImplementationGuideDefinitionComponent setParameter( 12736 List<ImplementationGuideDefinitionParameterComponent> theParameter) { 12737 this.parameter = theParameter; 12738 return this; 12739 } 12740 12741 public boolean hasParameter() { 12742 if (this.parameter == null) 12743 return false; 12744 for (ImplementationGuideDefinitionParameterComponent item : this.parameter) 12745 if (!item.isEmpty()) 12746 return true; 12747 return false; 12748 } 12749 12750 public ImplementationGuideDefinitionParameterComponent addParameter() { // 3 12751 ImplementationGuideDefinitionParameterComponent t = new ImplementationGuideDefinitionParameterComponent(); 12752 if (this.parameter == null) 12753 this.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 12754 this.parameter.add(t); 12755 return t; 12756 } 12757 12758 public ImplementationGuideDefinitionComponent addParameter(ImplementationGuideDefinitionParameterComponent t) { // 3 12759 if (t == null) 12760 return this; 12761 if (this.parameter == null) 12762 this.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 12763 this.parameter.add(t); 12764 return this; 12765 } 12766 12767 /** 12768 * @return The first repetition of repeating field {@link #parameter}, creating 12769 * it if it does not already exist 12770 */ 12771 public ImplementationGuideDefinitionParameterComponent getParameterFirstRep() { 12772 if (getParameter().isEmpty()) { 12773 addParameter(); 12774 } 12775 return getParameter().get(0); 12776 } 12777 12778 /** 12779 * @return {@link #template} (A template for building resources.) 12780 */ 12781 public List<ImplementationGuideDefinitionTemplateComponent> getTemplate() { 12782 if (this.template == null) 12783 this.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 12784 return this.template; 12785 } 12786 12787 /** 12788 * @return Returns a reference to <code>this</code> for easy method chaining 12789 */ 12790 public ImplementationGuideDefinitionComponent setTemplate( 12791 List<ImplementationGuideDefinitionTemplateComponent> theTemplate) { 12792 this.template = theTemplate; 12793 return this; 12794 } 12795 12796 public boolean hasTemplate() { 12797 if (this.template == null) 12798 return false; 12799 for (ImplementationGuideDefinitionTemplateComponent item : this.template) 12800 if (!item.isEmpty()) 12801 return true; 12802 return false; 12803 } 12804 12805 public ImplementationGuideDefinitionTemplateComponent addTemplate() { // 3 12806 ImplementationGuideDefinitionTemplateComponent t = new ImplementationGuideDefinitionTemplateComponent(); 12807 if (this.template == null) 12808 this.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 12809 this.template.add(t); 12810 return t; 12811 } 12812 12813 public ImplementationGuideDefinitionComponent addTemplate(ImplementationGuideDefinitionTemplateComponent t) { // 3 12814 if (t == null) 12815 return this; 12816 if (this.template == null) 12817 this.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 12818 this.template.add(t); 12819 return this; 12820 } 12821 12822 /** 12823 * @return The first repetition of repeating field {@link #template}, creating 12824 * it if it does not already exist 12825 */ 12826 public ImplementationGuideDefinitionTemplateComponent getTemplateFirstRep() { 12827 if (getTemplate().isEmpty()) { 12828 addTemplate(); 12829 } 12830 return getTemplate().get(0); 12831 } 12832 12833 protected void listChildren(List<Property> children) { 12834 super.listChildren(children); 12835 children.add( 12836 new Property("grouping", "", "A logical group of resources. Logical groups can be used when building pages.", 12837 0, java.lang.Integer.MAX_VALUE, grouping)); 12838 children.add(new Property("resource", "", 12839 "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 12840 0, java.lang.Integer.MAX_VALUE, resource)); 12841 children.add(new Property("page", "", 12842 "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 1, 12843 page)); 12844 children.add(new Property("parameter", "", "Defines how IG is built by tools.", 0, java.lang.Integer.MAX_VALUE, 12845 parameter)); 12846 children.add( 12847 new Property("template", "", "A template for building resources.", 0, java.lang.Integer.MAX_VALUE, template)); 12848 } 12849 12850 @Override 12851 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12852 switch (_hash) { 12853 case 506371331: 12854 /* grouping */ return new Property("grouping", "", 12855 "A logical group of resources. Logical groups can be used when building pages.", 0, 12856 java.lang.Integer.MAX_VALUE, grouping); 12857 case -341064690: 12858 /* resource */ return new Property("resource", "", 12859 "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 12860 0, java.lang.Integer.MAX_VALUE, resource); 12861 case 3433103: 12862 /* page */ return new Property("page", "", 12863 "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 1, 12864 page); 12865 case 1954460585: 12866 /* parameter */ return new Property("parameter", "", "Defines how IG is built by tools.", 0, 12867 java.lang.Integer.MAX_VALUE, parameter); 12868 case -1321546630: 12869 /* template */ return new Property("template", "", "A template for building resources.", 0, 12870 java.lang.Integer.MAX_VALUE, template); 12871 default: 12872 return super.getNamedProperty(_hash, _name, _checkValid); 12873 } 12874 12875 } 12876 12877 @Override 12878 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12879 switch (hash) { 12880 case 506371331: 12881 /* grouping */ return this.grouping == null ? new Base[0] 12882 : this.grouping.toArray(new Base[this.grouping.size()]); // ImplementationGuideDefinitionGroupingComponent 12883 case -341064690: 12884 /* resource */ return this.resource == null ? new Base[0] 12885 : this.resource.toArray(new Base[this.resource.size()]); // ImplementationGuideDefinitionResourceComponent 12886 case 3433103: 12887 /* page */ return this.page == null ? new Base[0] : new Base[] { this.page }; // ImplementationGuideDefinitionPageComponent 12888 case 1954460585: 12889 /* parameter */ return this.parameter == null ? new Base[0] 12890 : this.parameter.toArray(new Base[this.parameter.size()]); // ImplementationGuideDefinitionParameterComponent 12891 case -1321546630: 12892 /* template */ return this.template == null ? new Base[0] 12893 : this.template.toArray(new Base[this.template.size()]); // ImplementationGuideDefinitionTemplateComponent 12894 default: 12895 return super.getProperty(hash, name, checkValid); 12896 } 12897 12898 } 12899 12900 @Override 12901 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12902 switch (hash) { 12903 case 506371331: // grouping 12904 this.getGrouping().add((ImplementationGuideDefinitionGroupingComponent) value); // ImplementationGuideDefinitionGroupingComponent 12905 return value; 12906 case -341064690: // resource 12907 this.getResource().add((ImplementationGuideDefinitionResourceComponent) value); // ImplementationGuideDefinitionResourceComponent 12908 return value; 12909 case 3433103: // page 12910 this.page = (ImplementationGuideDefinitionPageComponent) value; // ImplementationGuideDefinitionPageComponent 12911 return value; 12912 case 1954460585: // parameter 12913 this.getParameter().add((ImplementationGuideDefinitionParameterComponent) value); // ImplementationGuideDefinitionParameterComponent 12914 return value; 12915 case -1321546630: // template 12916 this.getTemplate().add((ImplementationGuideDefinitionTemplateComponent) value); // ImplementationGuideDefinitionTemplateComponent 12917 return value; 12918 default: 12919 return super.setProperty(hash, name, value); 12920 } 12921 12922 } 12923 12924 @Override 12925 public Base setProperty(String name, Base value) throws FHIRException { 12926 if (name.equals("grouping")) { 12927 this.getGrouping().add((ImplementationGuideDefinitionGroupingComponent) value); 12928 } else if (name.equals("resource")) { 12929 this.getResource().add((ImplementationGuideDefinitionResourceComponent) value); 12930 } else if (name.equals("page")) { 12931 this.page = (ImplementationGuideDefinitionPageComponent) value; // ImplementationGuideDefinitionPageComponent 12932 } else if (name.equals("parameter")) { 12933 this.getParameter().add((ImplementationGuideDefinitionParameterComponent) value); 12934 } else if (name.equals("template")) { 12935 this.getTemplate().add((ImplementationGuideDefinitionTemplateComponent) value); 12936 } else 12937 return super.setProperty(name, value); 12938 return value; 12939 } 12940 12941 @Override 12942 public void removeChild(String name, Base value) throws FHIRException { 12943 if (name.equals("grouping")) { 12944 this.getGrouping().remove((ImplementationGuideDefinitionGroupingComponent) value); 12945 } else if (name.equals("resource")) { 12946 this.getResource().remove((ImplementationGuideDefinitionResourceComponent) value); 12947 } else if (name.equals("page")) { 12948 this.page = (ImplementationGuideDefinitionPageComponent) value; // ImplementationGuideDefinitionPageComponent 12949 } else if (name.equals("parameter")) { 12950 this.getParameter().remove((ImplementationGuideDefinitionParameterComponent) value); 12951 } else if (name.equals("template")) { 12952 this.getTemplate().remove((ImplementationGuideDefinitionTemplateComponent) value); 12953 } else 12954 super.removeChild(name, value); 12955 12956 } 12957 12958 @Override 12959 public Base makeProperty(int hash, String name) throws FHIRException { 12960 switch (hash) { 12961 case 506371331: 12962 return addGrouping(); 12963 case -341064690: 12964 return addResource(); 12965 case 3433103: 12966 return getPage(); 12967 case 1954460585: 12968 return addParameter(); 12969 case -1321546630: 12970 return addTemplate(); 12971 default: 12972 return super.makeProperty(hash, name); 12973 } 12974 12975 } 12976 12977 @Override 12978 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12979 switch (hash) { 12980 case 506371331: 12981 /* grouping */ return new String[] {}; 12982 case -341064690: 12983 /* resource */ return new String[] {}; 12984 case 3433103: 12985 /* page */ return new String[] {}; 12986 case 1954460585: 12987 /* parameter */ return new String[] {}; 12988 case -1321546630: 12989 /* template */ return new String[] {}; 12990 default: 12991 return super.getTypesForProperty(hash, name); 12992 } 12993 12994 } 12995 12996 @Override 12997 public Base addChild(String name) throws FHIRException { 12998 if (name.equals("grouping")) { 12999 return addGrouping(); 13000 } else if (name.equals("resource")) { 13001 return addResource(); 13002 } else if (name.equals("page")) { 13003 this.page = new ImplementationGuideDefinitionPageComponent(); 13004 return this.page; 13005 } else if (name.equals("parameter")) { 13006 return addParameter(); 13007 } else if (name.equals("template")) { 13008 return addTemplate(); 13009 } else 13010 return super.addChild(name); 13011 } 13012 13013 public ImplementationGuideDefinitionComponent copy() { 13014 ImplementationGuideDefinitionComponent dst = new ImplementationGuideDefinitionComponent(); 13015 copyValues(dst); 13016 return dst; 13017 } 13018 13019 public void copyValues(ImplementationGuideDefinitionComponent dst) { 13020 super.copyValues(dst); 13021 if (grouping != null) { 13022 dst.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 13023 for (ImplementationGuideDefinitionGroupingComponent i : grouping) 13024 dst.grouping.add(i.copy()); 13025 } 13026 ; 13027 if (resource != null) { 13028 dst.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 13029 for (ImplementationGuideDefinitionResourceComponent i : resource) 13030 dst.resource.add(i.copy()); 13031 } 13032 ; 13033 dst.page = page == null ? null : page.copy(); 13034 if (parameter != null) { 13035 dst.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 13036 for (ImplementationGuideDefinitionParameterComponent i : parameter) 13037 dst.parameter.add(i.copy()); 13038 } 13039 ; 13040 if (template != null) { 13041 dst.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 13042 for (ImplementationGuideDefinitionTemplateComponent i : template) 13043 dst.template.add(i.copy()); 13044 } 13045 ; 13046 } 13047 13048 @Override 13049 public boolean equalsDeep(Base other_) { 13050 if (!super.equalsDeep(other_)) 13051 return false; 13052 if (!(other_ instanceof ImplementationGuideDefinitionComponent)) 13053 return false; 13054 ImplementationGuideDefinitionComponent o = (ImplementationGuideDefinitionComponent) other_; 13055 return compareDeep(grouping, o.grouping, true) && compareDeep(resource, o.resource, true) 13056 && compareDeep(page, o.page, true) && compareDeep(parameter, o.parameter, true) 13057 && compareDeep(template, o.template, true); 13058 } 13059 13060 @Override 13061 public boolean equalsShallow(Base other_) { 13062 if (!super.equalsShallow(other_)) 13063 return false; 13064 if (!(other_ instanceof ImplementationGuideDefinitionComponent)) 13065 return false; 13066 ImplementationGuideDefinitionComponent o = (ImplementationGuideDefinitionComponent) other_; 13067 return true; 13068 } 13069 13070 public boolean isEmpty() { 13071 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(grouping, resource, page, parameter, template); 13072 } 13073 13074 public String fhirType() { 13075 return "ImplementationGuide.definition"; 13076 13077 } 13078 13079 } 13080 13081 @Block() 13082 public static class ImplementationGuideDefinitionGroupingComponent extends BackboneElement 13083 implements IBaseBackboneElement { 13084 /** 13085 * The human-readable title to display for the package of resources when 13086 * rendering the implementation guide. 13087 */ 13088 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 13089 @Description(shortDefinition = "Descriptive name for the package", formalDefinition = "The human-readable title to display for the package of resources when rendering the implementation guide.") 13090 protected StringType name; 13091 13092 /** 13093 * Human readable text describing the package. 13094 */ 13095 @Child(name = "description", type = { 13096 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 13097 @Description(shortDefinition = "Human readable text describing the package", formalDefinition = "Human readable text describing the package.") 13098 protected StringType description; 13099 13100 private static final long serialVersionUID = -1105523499L; 13101 13102 /** 13103 * Constructor 13104 */ 13105 public ImplementationGuideDefinitionGroupingComponent() { 13106 super(); 13107 } 13108 13109 /** 13110 * Constructor 13111 */ 13112 public ImplementationGuideDefinitionGroupingComponent(StringType name) { 13113 super(); 13114 this.name = name; 13115 } 13116 13117 /** 13118 * @return {@link #name} (The human-readable title to display for the package of 13119 * resources when rendering the implementation guide.). This is the 13120 * underlying object with id, value and extensions. The accessor 13121 * "getName" gives direct access to the value 13122 */ 13123 public StringType getNameElement() { 13124 if (this.name == null) 13125 if (Configuration.errorOnAutoCreate()) 13126 throw new Error("Attempt to auto-create ImplementationGuideDefinitionGroupingComponent.name"); 13127 else if (Configuration.doAutoCreate()) 13128 this.name = new StringType(); // bb 13129 return this.name; 13130 } 13131 13132 public boolean hasNameElement() { 13133 return this.name != null && !this.name.isEmpty(); 13134 } 13135 13136 public boolean hasName() { 13137 return this.name != null && !this.name.isEmpty(); 13138 } 13139 13140 /** 13141 * @param value {@link #name} (The human-readable title to display for the 13142 * package of resources when rendering the implementation guide.). 13143 * This is the underlying object with id, value and extensions. The 13144 * accessor "getName" gives direct access to the value 13145 */ 13146 public ImplementationGuideDefinitionGroupingComponent setNameElement(StringType value) { 13147 this.name = value; 13148 return this; 13149 } 13150 13151 /** 13152 * @return The human-readable title to display for the package of resources when 13153 * rendering the implementation guide. 13154 */ 13155 public String getName() { 13156 return this.name == null ? null : this.name.getValue(); 13157 } 13158 13159 /** 13160 * @param value The human-readable title to display for the package of resources 13161 * when rendering the implementation guide. 13162 */ 13163 public ImplementationGuideDefinitionGroupingComponent setName(String value) { 13164 if (this.name == null) 13165 this.name = new StringType(); 13166 this.name.setValue(value); 13167 return this; 13168 } 13169 13170 /** 13171 * @return {@link #description} (Human readable text describing the package.). 13172 * This is the underlying object with id, value and extensions. The 13173 * accessor "getDescription" gives direct access to the value 13174 */ 13175 public StringType getDescriptionElement() { 13176 if (this.description == null) 13177 if (Configuration.errorOnAutoCreate()) 13178 throw new Error("Attempt to auto-create ImplementationGuideDefinitionGroupingComponent.description"); 13179 else if (Configuration.doAutoCreate()) 13180 this.description = new StringType(); // bb 13181 return this.description; 13182 } 13183 13184 public boolean hasDescriptionElement() { 13185 return this.description != null && !this.description.isEmpty(); 13186 } 13187 13188 public boolean hasDescription() { 13189 return this.description != null && !this.description.isEmpty(); 13190 } 13191 13192 /** 13193 * @param value {@link #description} (Human readable text describing the 13194 * package.). This is the underlying object with id, value and 13195 * extensions. The accessor "getDescription" gives direct access to 13196 * the value 13197 */ 13198 public ImplementationGuideDefinitionGroupingComponent setDescriptionElement(StringType value) { 13199 this.description = value; 13200 return this; 13201 } 13202 13203 /** 13204 * @return Human readable text describing the package. 13205 */ 13206 public String getDescription() { 13207 return this.description == null ? null : this.description.getValue(); 13208 } 13209 13210 /** 13211 * @param value Human readable text describing the package. 13212 */ 13213 public ImplementationGuideDefinitionGroupingComponent setDescription(String value) { 13214 if (Utilities.noString(value)) 13215 this.description = null; 13216 else { 13217 if (this.description == null) 13218 this.description = new StringType(); 13219 this.description.setValue(value); 13220 } 13221 return this; 13222 } 13223 13224 protected void listChildren(List<Property> children) { 13225 super.listChildren(children); 13226 children.add(new Property("name", "string", 13227 "The human-readable title to display for the package of resources when rendering the implementation guide.", 13228 0, 1, name)); 13229 children 13230 .add(new Property("description", "string", "Human readable text describing the package.", 0, 1, description)); 13231 } 13232 13233 @Override 13234 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13235 switch (_hash) { 13236 case 3373707: 13237 /* name */ return new Property("name", "string", 13238 "The human-readable title to display for the package of resources when rendering the implementation guide.", 13239 0, 1, name); 13240 case -1724546052: 13241 /* description */ return new Property("description", "string", "Human readable text describing the package.", 0, 13242 1, description); 13243 default: 13244 return super.getNamedProperty(_hash, _name, _checkValid); 13245 } 13246 13247 } 13248 13249 @Override 13250 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13251 switch (hash) { 13252 case 3373707: 13253 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 13254 case -1724546052: 13255 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 13256 default: 13257 return super.getProperty(hash, name, checkValid); 13258 } 13259 13260 } 13261 13262 @Override 13263 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13264 switch (hash) { 13265 case 3373707: // name 13266 this.name = castToString(value); // StringType 13267 return value; 13268 case -1724546052: // description 13269 this.description = castToString(value); // StringType 13270 return value; 13271 default: 13272 return super.setProperty(hash, name, value); 13273 } 13274 13275 } 13276 13277 @Override 13278 public Base setProperty(String name, Base value) throws FHIRException { 13279 if (name.equals("name")) { 13280 this.name = castToString(value); // StringType 13281 } else if (name.equals("description")) { 13282 this.description = castToString(value); // StringType 13283 } else 13284 return super.setProperty(name, value); 13285 return value; 13286 } 13287 13288 @Override 13289 public void removeChild(String name, Base value) throws FHIRException { 13290 if (name.equals("name")) { 13291 this.name = null; 13292 } else if (name.equals("description")) { 13293 this.description = null; 13294 } else 13295 super.removeChild(name, value); 13296 13297 } 13298 13299 @Override 13300 public Base makeProperty(int hash, String name) throws FHIRException { 13301 switch (hash) { 13302 case 3373707: 13303 return getNameElement(); 13304 case -1724546052: 13305 return getDescriptionElement(); 13306 default: 13307 return super.makeProperty(hash, name); 13308 } 13309 13310 } 13311 13312 @Override 13313 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13314 switch (hash) { 13315 case 3373707: 13316 /* name */ return new String[] { "string" }; 13317 case -1724546052: 13318 /* description */ return new String[] { "string" }; 13319 default: 13320 return super.getTypesForProperty(hash, name); 13321 } 13322 13323 } 13324 13325 @Override 13326 public Base addChild(String name) throws FHIRException { 13327 if (name.equals("name")) { 13328 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 13329 } else if (name.equals("description")) { 13330 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 13331 } else 13332 return super.addChild(name); 13333 } 13334 13335 public ImplementationGuideDefinitionGroupingComponent copy() { 13336 ImplementationGuideDefinitionGroupingComponent dst = new ImplementationGuideDefinitionGroupingComponent(); 13337 copyValues(dst); 13338 return dst; 13339 } 13340 13341 public void copyValues(ImplementationGuideDefinitionGroupingComponent dst) { 13342 super.copyValues(dst); 13343 dst.name = name == null ? null : name.copy(); 13344 dst.description = description == null ? null : description.copy(); 13345 } 13346 13347 @Override 13348 public boolean equalsDeep(Base other_) { 13349 if (!super.equalsDeep(other_)) 13350 return false; 13351 if (!(other_ instanceof ImplementationGuideDefinitionGroupingComponent)) 13352 return false; 13353 ImplementationGuideDefinitionGroupingComponent o = (ImplementationGuideDefinitionGroupingComponent) other_; 13354 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true); 13355 } 13356 13357 @Override 13358 public boolean equalsShallow(Base other_) { 13359 if (!super.equalsShallow(other_)) 13360 return false; 13361 if (!(other_ instanceof ImplementationGuideDefinitionGroupingComponent)) 13362 return false; 13363 ImplementationGuideDefinitionGroupingComponent o = (ImplementationGuideDefinitionGroupingComponent) other_; 13364 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 13365 } 13366 13367 public boolean isEmpty() { 13368 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description); 13369 } 13370 13371 public String fhirType() { 13372 return "ImplementationGuide.definition.grouping"; 13373 13374 } 13375 13376 } 13377 13378 @Block() 13379 public static class ImplementationGuideDefinitionResourceComponent extends BackboneElement 13380 implements IBaseBackboneElement { 13381 /** 13382 * Where this resource is found. 13383 */ 13384 @Child(name = "reference", type = { 13385 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 13386 @Description(shortDefinition = "Location of the resource", formalDefinition = "Where this resource is found.") 13387 protected Reference reference; 13388 13389 /** 13390 * The actual object that is the target of the reference (Where this resource is 13391 * found.) 13392 */ 13393 protected Resource referenceTarget; 13394 13395 /** 13396 * Indicates the FHIR Version(s) this artifact is intended to apply to. If no 13397 * versions are specified, the resource is assumed to apply to all the versions 13398 * stated in ImplementationGuide.fhirVersion. 13399 */ 13400 @Child(name = "fhirVersion", type = { 13401 CodeType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 13402 @Description(shortDefinition = "Versions this applies to (if different to IG)", formalDefinition = "Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.") 13403 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/FHIR-version") 13404 protected List<Enumeration<FHIRVersion>> fhirVersion; 13405 13406 /** 13407 * A human assigned name for the resource. All resources SHOULD have a name, but 13408 * the name may be extracted from the resource (e.g. ValueSet.name). 13409 */ 13410 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 13411 @Description(shortDefinition = "Human Name for the resource", formalDefinition = "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).") 13412 protected StringType name; 13413 13414 /** 13415 * A description of the reason that a resource has been included in the 13416 * implementation guide. 13417 */ 13418 @Child(name = "description", type = { 13419 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 13420 @Description(shortDefinition = "Reason why included in guide", formalDefinition = "A description of the reason that a resource has been included in the implementation guide.") 13421 protected StringType description; 13422 13423 /** 13424 * If true or a reference, indicates the resource is an example instance. If a 13425 * reference is present, indicates that the example is an example of the 13426 * specified profile. 13427 */ 13428 @Child(name = "example", type = { BooleanType.class, 13429 CanonicalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 13430 @Description(shortDefinition = "Is an example/What is this an example of?", formalDefinition = "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.") 13431 protected Type example; 13432 13433 /** 13434 * Reference to the id of the grouping this resource appears in. 13435 */ 13436 @Child(name = "groupingId", type = { IdType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 13437 @Description(shortDefinition = "Grouping this is part of", formalDefinition = "Reference to the id of the grouping this resource appears in.") 13438 protected IdType groupingId; 13439 13440 private static final long serialVersionUID = 1840689093L; 13441 13442 /** 13443 * Constructor 13444 */ 13445 public ImplementationGuideDefinitionResourceComponent() { 13446 super(); 13447 } 13448 13449 /** 13450 * Constructor 13451 */ 13452 public ImplementationGuideDefinitionResourceComponent(Reference reference) { 13453 super(); 13454 this.reference = reference; 13455 } 13456 13457 /** 13458 * @return {@link #reference} (Where this resource is found.) 13459 */ 13460 public Reference getReference() { 13461 if (this.reference == null) 13462 if (Configuration.errorOnAutoCreate()) 13463 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.reference"); 13464 else if (Configuration.doAutoCreate()) 13465 this.reference = new Reference(); // cc 13466 return this.reference; 13467 } 13468 13469 public boolean hasReference() { 13470 return this.reference != null && !this.reference.isEmpty(); 13471 } 13472 13473 /** 13474 * @param value {@link #reference} (Where this resource is found.) 13475 */ 13476 public ImplementationGuideDefinitionResourceComponent setReference(Reference value) { 13477 this.reference = value; 13478 return this; 13479 } 13480 13481 /** 13482 * @return {@link #reference} The actual object that is the target of the 13483 * reference. The reference library doesn't populate this, but you can 13484 * use it to hold the resource if you resolve it. (Where this resource 13485 * is found.) 13486 */ 13487 public Resource getReferenceTarget() { 13488 return this.referenceTarget; 13489 } 13490 13491 /** 13492 * @param value {@link #reference} The actual object that is the target of the 13493 * reference. The reference library doesn't use these, but you can 13494 * use it to hold the resource if you resolve it. (Where this 13495 * resource is found.) 13496 */ 13497 public ImplementationGuideDefinitionResourceComponent setReferenceTarget(Resource value) { 13498 this.referenceTarget = value; 13499 return this; 13500 } 13501 13502 /** 13503 * @return {@link #fhirVersion} (Indicates the FHIR Version(s) this artifact is 13504 * intended to apply to. If no versions are specified, the resource is 13505 * assumed to apply to all the versions stated in 13506 * ImplementationGuide.fhirVersion.) 13507 */ 13508 public List<Enumeration<FHIRVersion>> getFhirVersion() { 13509 if (this.fhirVersion == null) 13510 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 13511 return this.fhirVersion; 13512 } 13513 13514 /** 13515 * @return Returns a reference to <code>this</code> for easy method chaining 13516 */ 13517 public ImplementationGuideDefinitionResourceComponent setFhirVersion( 13518 List<Enumeration<FHIRVersion>> theFhirVersion) { 13519 this.fhirVersion = theFhirVersion; 13520 return this; 13521 } 13522 13523 public boolean hasFhirVersion() { 13524 if (this.fhirVersion == null) 13525 return false; 13526 for (Enumeration<FHIRVersion> item : this.fhirVersion) 13527 if (!item.isEmpty()) 13528 return true; 13529 return false; 13530 } 13531 13532 /** 13533 * @return {@link #fhirVersion} (Indicates the FHIR Version(s) this artifact is 13534 * intended to apply to. If no versions are specified, the resource is 13535 * assumed to apply to all the versions stated in 13536 * ImplementationGuide.fhirVersion.) 13537 */ 13538 public Enumeration<FHIRVersion> addFhirVersionElement() {// 2 13539 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 13540 if (this.fhirVersion == null) 13541 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 13542 this.fhirVersion.add(t); 13543 return t; 13544 } 13545 13546 /** 13547 * @param value {@link #fhirVersion} (Indicates the FHIR Version(s) this 13548 * artifact is intended to apply to. If no versions are specified, 13549 * the resource is assumed to apply to all the versions stated in 13550 * ImplementationGuide.fhirVersion.) 13551 */ 13552 public ImplementationGuideDefinitionResourceComponent addFhirVersion(FHIRVersion value) { // 1 13553 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 13554 t.setValue(value); 13555 if (this.fhirVersion == null) 13556 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 13557 this.fhirVersion.add(t); 13558 return this; 13559 } 13560 13561 /** 13562 * @param value {@link #fhirVersion} (Indicates the FHIR Version(s) this 13563 * artifact is intended to apply to. If no versions are specified, 13564 * the resource is assumed to apply to all the versions stated in 13565 * ImplementationGuide.fhirVersion.) 13566 */ 13567 public boolean hasFhirVersion(FHIRVersion value) { 13568 if (this.fhirVersion == null) 13569 return false; 13570 for (Enumeration<FHIRVersion> v : this.fhirVersion) 13571 if (v.getValue().equals(value)) // code 13572 return true; 13573 return false; 13574 } 13575 13576 /** 13577 * @return {@link #name} (A human assigned name for the resource. All resources 13578 * SHOULD have a name, but the name may be extracted from the resource 13579 * (e.g. ValueSet.name).). This is the underlying object with id, value 13580 * and extensions. The accessor "getName" gives direct access to the 13581 * value 13582 */ 13583 public StringType getNameElement() { 13584 if (this.name == null) 13585 if (Configuration.errorOnAutoCreate()) 13586 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.name"); 13587 else if (Configuration.doAutoCreate()) 13588 this.name = new StringType(); // bb 13589 return this.name; 13590 } 13591 13592 public boolean hasNameElement() { 13593 return this.name != null && !this.name.isEmpty(); 13594 } 13595 13596 public boolean hasName() { 13597 return this.name != null && !this.name.isEmpty(); 13598 } 13599 13600 /** 13601 * @param value {@link #name} (A human assigned name for the resource. All 13602 * resources SHOULD have a name, but the name may be extracted from 13603 * the resource (e.g. ValueSet.name).). This is the underlying 13604 * object with id, value and extensions. The accessor "getName" 13605 * gives direct access to the value 13606 */ 13607 public ImplementationGuideDefinitionResourceComponent setNameElement(StringType value) { 13608 this.name = value; 13609 return this; 13610 } 13611 13612 /** 13613 * @return A human assigned name for the resource. All resources SHOULD have a 13614 * name, but the name may be extracted from the resource (e.g. 13615 * ValueSet.name). 13616 */ 13617 public String getName() { 13618 return this.name == null ? null : this.name.getValue(); 13619 } 13620 13621 /** 13622 * @param value A human assigned name for the resource. All resources SHOULD 13623 * have a name, but the name may be extracted from the resource 13624 * (e.g. ValueSet.name). 13625 */ 13626 public ImplementationGuideDefinitionResourceComponent setName(String value) { 13627 if (Utilities.noString(value)) 13628 this.name = null; 13629 else { 13630 if (this.name == null) 13631 this.name = new StringType(); 13632 this.name.setValue(value); 13633 } 13634 return this; 13635 } 13636 13637 /** 13638 * @return {@link #description} (A description of the reason that a resource has 13639 * been included in the implementation guide.). This is the underlying 13640 * object with id, value and extensions. The accessor "getDescription" 13641 * gives direct access to the value 13642 */ 13643 public StringType getDescriptionElement() { 13644 if (this.description == null) 13645 if (Configuration.errorOnAutoCreate()) 13646 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.description"); 13647 else if (Configuration.doAutoCreate()) 13648 this.description = new StringType(); // bb 13649 return this.description; 13650 } 13651 13652 public boolean hasDescriptionElement() { 13653 return this.description != null && !this.description.isEmpty(); 13654 } 13655 13656 public boolean hasDescription() { 13657 return this.description != null && !this.description.isEmpty(); 13658 } 13659 13660 /** 13661 * @param value {@link #description} (A description of the reason that a 13662 * resource has been included in the implementation guide.). This 13663 * is the underlying object with id, value and extensions. The 13664 * accessor "getDescription" gives direct access to the value 13665 */ 13666 public ImplementationGuideDefinitionResourceComponent setDescriptionElement(StringType value) { 13667 this.description = value; 13668 return this; 13669 } 13670 13671 /** 13672 * @return A description of the reason that a resource has been included in the 13673 * implementation guide. 13674 */ 13675 public String getDescription() { 13676 return this.description == null ? null : this.description.getValue(); 13677 } 13678 13679 /** 13680 * @param value A description of the reason that a resource has been included in 13681 * the implementation guide. 13682 */ 13683 public ImplementationGuideDefinitionResourceComponent setDescription(String value) { 13684 if (Utilities.noString(value)) 13685 this.description = null; 13686 else { 13687 if (this.description == null) 13688 this.description = new StringType(); 13689 this.description.setValue(value); 13690 } 13691 return this; 13692 } 13693 13694 /** 13695 * @return {@link #example} (If true or a reference, indicates the resource is 13696 * an example instance. If a reference is present, indicates that the 13697 * example is an example of the specified profile.) 13698 */ 13699 public Type getExample() { 13700 return this.example; 13701 } 13702 13703 /** 13704 * @return {@link #example} (If true or a reference, indicates the resource is 13705 * an example instance. If a reference is present, indicates that the 13706 * example is an example of the specified profile.) 13707 */ 13708 public BooleanType getExampleBooleanType() throws FHIRException { 13709 if (this.example == null) 13710 this.example = new BooleanType(); 13711 if (!(this.example instanceof BooleanType)) 13712 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 13713 + this.example.getClass().getName() + " was encountered"); 13714 return (BooleanType) this.example; 13715 } 13716 13717 public boolean hasExampleBooleanType() { 13718 return this.example instanceof BooleanType; 13719 } 13720 13721 /** 13722 * @return {@link #example} (If true or a reference, indicates the resource is 13723 * an example instance. If a reference is present, indicates that the 13724 * example is an example of the specified profile.) 13725 */ 13726 public CanonicalType getExampleCanonicalType() throws FHIRException { 13727 if (this.example == null) 13728 this.example = new CanonicalType(); 13729 if (!(this.example instanceof CanonicalType)) 13730 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 13731 + this.example.getClass().getName() + " was encountered"); 13732 return (CanonicalType) this.example; 13733 } 13734 13735 public boolean hasExampleCanonicalType() { 13736 return this.example instanceof CanonicalType; 13737 } 13738 13739 public boolean hasExample() { 13740 return this.example != null && !this.example.isEmpty(); 13741 } 13742 13743 /** 13744 * @param value {@link #example} (If true or a reference, indicates the resource 13745 * is an example instance. If a reference is present, indicates 13746 * that the example is an example of the specified profile.) 13747 */ 13748 public ImplementationGuideDefinitionResourceComponent setExample(Type value) { 13749 if (value != null && !(value instanceof BooleanType || value instanceof CanonicalType)) 13750 throw new Error( 13751 "Not the right type for ImplementationGuide.definition.resource.example[x]: " + value.fhirType()); 13752 this.example = value; 13753 return this; 13754 } 13755 13756 /** 13757 * @return {@link #groupingId} (Reference to the id of the grouping this 13758 * resource appears in.). This is the underlying object with id, value 13759 * and extensions. The accessor "getGroupingId" gives direct access to 13760 * the value 13761 */ 13762 public IdType getGroupingIdElement() { 13763 if (this.groupingId == null) 13764 if (Configuration.errorOnAutoCreate()) 13765 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.groupingId"); 13766 else if (Configuration.doAutoCreate()) 13767 this.groupingId = new IdType(); // bb 13768 return this.groupingId; 13769 } 13770 13771 public boolean hasGroupingIdElement() { 13772 return this.groupingId != null && !this.groupingId.isEmpty(); 13773 } 13774 13775 public boolean hasGroupingId() { 13776 return this.groupingId != null && !this.groupingId.isEmpty(); 13777 } 13778 13779 /** 13780 * @param value {@link #groupingId} (Reference to the id of the grouping this 13781 * resource appears in.). This is the underlying object with id, 13782 * value and extensions. The accessor "getGroupingId" gives direct 13783 * access to the value 13784 */ 13785 public ImplementationGuideDefinitionResourceComponent setGroupingIdElement(IdType value) { 13786 this.groupingId = value; 13787 return this; 13788 } 13789 13790 /** 13791 * @return Reference to the id of the grouping this resource appears in. 13792 */ 13793 public String getGroupingId() { 13794 return this.groupingId == null ? null : this.groupingId.getValue(); 13795 } 13796 13797 /** 13798 * @param value Reference to the id of the grouping this resource appears in. 13799 */ 13800 public ImplementationGuideDefinitionResourceComponent setGroupingId(String value) { 13801 if (Utilities.noString(value)) 13802 this.groupingId = null; 13803 else { 13804 if (this.groupingId == null) 13805 this.groupingId = new IdType(); 13806 this.groupingId.setValue(value); 13807 } 13808 return this; 13809 } 13810 13811 protected void listChildren(List<Property> children) { 13812 super.listChildren(children); 13813 children.add(new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, reference)); 13814 children.add(new Property("fhirVersion", "code", 13815 "Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.", 13816 0, java.lang.Integer.MAX_VALUE, fhirVersion)); 13817 children.add(new Property("name", "string", 13818 "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 13819 0, 1, name)); 13820 children.add(new Property("description", "string", 13821 "A description of the reason that a resource has been included in the implementation guide.", 0, 1, 13822 description)); 13823 children.add(new Property("example[x]", "boolean|canonical(StructureDefinition)", 13824 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 13825 0, 1, example)); 13826 children.add(new Property("groupingId", "id", "Reference to the id of the grouping this resource appears in.", 0, 13827 1, groupingId)); 13828 } 13829 13830 @Override 13831 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13832 switch (_hash) { 13833 case -925155509: 13834 /* reference */ return new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, 13835 reference); 13836 case 461006061: 13837 /* fhirVersion */ return new Property("fhirVersion", "code", 13838 "Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.", 13839 0, java.lang.Integer.MAX_VALUE, fhirVersion); 13840 case 3373707: 13841 /* name */ return new Property("name", "string", 13842 "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 13843 0, 1, name); 13844 case -1724546052: 13845 /* description */ return new Property("description", "string", 13846 "A description of the reason that a resource has been included in the implementation guide.", 0, 1, 13847 description); 13848 case -2002328874: 13849 /* example[x] */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 13850 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 13851 0, 1, example); 13852 case -1322970774: 13853 /* example */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 13854 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 13855 0, 1, example); 13856 case 159803230: 13857 /* exampleBoolean */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 13858 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 13859 0, 1, example); 13860 case 2016979626: 13861 /* exampleCanonical */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 13862 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 13863 0, 1, example); 13864 case 1291547006: 13865 /* groupingId */ return new Property("groupingId", "id", 13866 "Reference to the id of the grouping this resource appears in.", 0, 1, groupingId); 13867 default: 13868 return super.getNamedProperty(_hash, _name, _checkValid); 13869 } 13870 13871 } 13872 13873 @Override 13874 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13875 switch (hash) { 13876 case -925155509: 13877 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 13878 case 461006061: 13879 /* fhirVersion */ return this.fhirVersion == null ? new Base[0] 13880 : this.fhirVersion.toArray(new Base[this.fhirVersion.size()]); // Enumeration<FHIRVersion> 13881 case 3373707: 13882 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 13883 case -1724546052: 13884 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 13885 case -1322970774: 13886 /* example */ return this.example == null ? new Base[0] : new Base[] { this.example }; // Type 13887 case 1291547006: 13888 /* groupingId */ return this.groupingId == null ? new Base[0] : new Base[] { this.groupingId }; // IdType 13889 default: 13890 return super.getProperty(hash, name, checkValid); 13891 } 13892 13893 } 13894 13895 @Override 13896 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13897 switch (hash) { 13898 case -925155509: // reference 13899 this.reference = castToReference(value); // Reference 13900 return value; 13901 case 461006061: // fhirVersion 13902 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 13903 this.getFhirVersion().add((Enumeration) value); // Enumeration<FHIRVersion> 13904 return value; 13905 case 3373707: // name 13906 this.name = castToString(value); // StringType 13907 return value; 13908 case -1724546052: // description 13909 this.description = castToString(value); // StringType 13910 return value; 13911 case -1322970774: // example 13912 this.example = castToType(value); // Type 13913 return value; 13914 case 1291547006: // groupingId 13915 this.groupingId = castToId(value); // IdType 13916 return value; 13917 default: 13918 return super.setProperty(hash, name, value); 13919 } 13920 13921 } 13922 13923 @Override 13924 public Base setProperty(String name, Base value) throws FHIRException { 13925 if (name.equals("reference")) { 13926 this.reference = castToReference(value); // Reference 13927 } else if (name.equals("fhirVersion")) { 13928 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 13929 this.getFhirVersion().add((Enumeration) value); 13930 } else if (name.equals("name")) { 13931 this.name = castToString(value); // StringType 13932 } else if (name.equals("description")) { 13933 this.description = castToString(value); // StringType 13934 } else if (name.equals("example[x]")) { 13935 this.example = castToType(value); // Type 13936 } else if (name.equals("groupingId")) { 13937 this.groupingId = castToId(value); // IdType 13938 } else 13939 return super.setProperty(name, value); 13940 return value; 13941 } 13942 13943 @Override 13944 public void removeChild(String name, Base value) throws FHIRException { 13945 if (name.equals("reference")) { 13946 this.reference = null; 13947 } else if (name.equals("fhirVersion")) { 13948 this.getFhirVersion().remove((Enumeration) value); 13949 } else if (name.equals("name")) { 13950 this.name = null; 13951 } else if (name.equals("description")) { 13952 this.description = null; 13953 } else if (name.equals("example[x]")) { 13954 this.example = null; 13955 } else if (name.equals("groupingId")) { 13956 this.groupingId = null; 13957 } else 13958 super.removeChild(name, value); 13959 13960 } 13961 13962 @Override 13963 public Base makeProperty(int hash, String name) throws FHIRException { 13964 switch (hash) { 13965 case -925155509: 13966 return getReference(); 13967 case 461006061: 13968 return addFhirVersionElement(); 13969 case 3373707: 13970 return getNameElement(); 13971 case -1724546052: 13972 return getDescriptionElement(); 13973 case -2002328874: 13974 return getExample(); 13975 case -1322970774: 13976 return getExample(); 13977 case 1291547006: 13978 return getGroupingIdElement(); 13979 default: 13980 return super.makeProperty(hash, name); 13981 } 13982 13983 } 13984 13985 @Override 13986 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13987 switch (hash) { 13988 case -925155509: 13989 /* reference */ return new String[] { "Reference" }; 13990 case 461006061: 13991 /* fhirVersion */ return new String[] { "code" }; 13992 case 3373707: 13993 /* name */ return new String[] { "string" }; 13994 case -1724546052: 13995 /* description */ return new String[] { "string" }; 13996 case -1322970774: 13997 /* example */ return new String[] { "boolean", "canonical" }; 13998 case 1291547006: 13999 /* groupingId */ return new String[] { "id" }; 14000 default: 14001 return super.getTypesForProperty(hash, name); 14002 } 14003 14004 } 14005 14006 @Override 14007 public Base addChild(String name) throws FHIRException { 14008 if (name.equals("reference")) { 14009 this.reference = new Reference(); 14010 return this.reference; 14011 } else if (name.equals("fhirVersion")) { 14012 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.fhirVersion"); 14013 } else if (name.equals("name")) { 14014 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 14015 } else if (name.equals("description")) { 14016 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 14017 } else if (name.equals("exampleBoolean")) { 14018 this.example = new BooleanType(); 14019 return this.example; 14020 } else if (name.equals("exampleCanonical")) { 14021 this.example = new CanonicalType(); 14022 return this.example; 14023 } else if (name.equals("groupingId")) { 14024 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.groupingId"); 14025 } else 14026 return super.addChild(name); 14027 } 14028 14029 public ImplementationGuideDefinitionResourceComponent copy() { 14030 ImplementationGuideDefinitionResourceComponent dst = new ImplementationGuideDefinitionResourceComponent(); 14031 copyValues(dst); 14032 return dst; 14033 } 14034 14035 public void copyValues(ImplementationGuideDefinitionResourceComponent dst) { 14036 super.copyValues(dst); 14037 dst.reference = reference == null ? null : reference.copy(); 14038 if (fhirVersion != null) { 14039 dst.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 14040 for (Enumeration<FHIRVersion> i : fhirVersion) 14041 dst.fhirVersion.add(i.copy()); 14042 } 14043 ; 14044 dst.name = name == null ? null : name.copy(); 14045 dst.description = description == null ? null : description.copy(); 14046 dst.example = example == null ? null : example.copy(); 14047 dst.groupingId = groupingId == null ? null : groupingId.copy(); 14048 } 14049 14050 @Override 14051 public boolean equalsDeep(Base other_) { 14052 if (!super.equalsDeep(other_)) 14053 return false; 14054 if (!(other_ instanceof ImplementationGuideDefinitionResourceComponent)) 14055 return false; 14056 ImplementationGuideDefinitionResourceComponent o = (ImplementationGuideDefinitionResourceComponent) other_; 14057 return compareDeep(reference, o.reference, true) && compareDeep(fhirVersion, o.fhirVersion, true) 14058 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 14059 && compareDeep(example, o.example, true) && compareDeep(groupingId, o.groupingId, true); 14060 } 14061 14062 @Override 14063 public boolean equalsShallow(Base other_) { 14064 if (!super.equalsShallow(other_)) 14065 return false; 14066 if (!(other_ instanceof ImplementationGuideDefinitionResourceComponent)) 14067 return false; 14068 ImplementationGuideDefinitionResourceComponent o = (ImplementationGuideDefinitionResourceComponent) other_; 14069 return compareValues(fhirVersion, o.fhirVersion, true) && compareValues(name, o.name, true) 14070 && compareValues(description, o.description, true) && compareValues(groupingId, o.groupingId, true); 14071 } 14072 14073 public boolean isEmpty() { 14074 return super.isEmpty() 14075 && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, fhirVersion, name, description, example, groupingId); 14076 } 14077 14078 public String fhirType() { 14079 return "ImplementationGuide.definition.resource"; 14080 14081 } 14082 14083 } 14084 14085 @Block() 14086 public static class ImplementationGuideDefinitionPageComponent extends BackboneElement 14087 implements IBaseBackboneElement { 14088 /** 14089 * The source address for the page. 14090 */ 14091 @Child(name = "name", type = { UrlType.class, 14092 Binary.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 14093 @Description(shortDefinition = "Where to find that page", formalDefinition = "The source address for the page.") 14094 protected Type name; 14095 14096 /** 14097 * A short title used to represent this page in navigational structures such as 14098 * table of contents, bread crumbs, etc. 14099 */ 14100 @Child(name = "title", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 14101 @Description(shortDefinition = "Short title shown for navigational assistance", formalDefinition = "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.") 14102 protected StringType title; 14103 14104 /** 14105 * A code that indicates how the page is generated. 14106 */ 14107 @Child(name = "generation", type = { 14108 CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 14109 @Description(shortDefinition = "html | markdown | xml | generated", formalDefinition = "A code that indicates how the page is generated.") 14110 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/guide-page-generation") 14111 protected Enumeration<GuidePageGeneration> generation; 14112 14113 /** 14114 * Nested Pages/Sections under this page. 14115 */ 14116 @Child(name = "page", type = { 14117 ImplementationGuideDefinitionPageComponent.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 14118 @Description(shortDefinition = "Nested Pages / Sections", formalDefinition = "Nested Pages/Sections under this page.") 14119 protected List<ImplementationGuideDefinitionPageComponent> page; 14120 14121 private static final long serialVersionUID = -365655658L; 14122 14123 /** 14124 * Constructor 14125 */ 14126 public ImplementationGuideDefinitionPageComponent() { 14127 super(); 14128 } 14129 14130 /** 14131 * Constructor 14132 */ 14133 public ImplementationGuideDefinitionPageComponent(Type name, StringType title, 14134 Enumeration<GuidePageGeneration> generation) { 14135 super(); 14136 this.name = name; 14137 this.title = title; 14138 this.generation = generation; 14139 } 14140 14141 /** 14142 * @return {@link #name} (The source address for the page.) 14143 */ 14144 public Type getName() { 14145 return this.name; 14146 } 14147 14148 /** 14149 * @return {@link #name} (The source address for the page.) 14150 */ 14151 public UrlType getNameUrlType() throws FHIRException { 14152 if (this.name == null) 14153 this.name = new UrlType(); 14154 if (!(this.name instanceof UrlType)) 14155 throw new FHIRException( 14156 "Type mismatch: the type UrlType was expected, but " + this.name.getClass().getName() + " was encountered"); 14157 return (UrlType) this.name; 14158 } 14159 14160 public boolean hasNameUrlType() { 14161 return this.name instanceof UrlType; 14162 } 14163 14164 /** 14165 * @return {@link #name} (The source address for the page.) 14166 */ 14167 public Reference getNameReference() throws FHIRException { 14168 if (this.name == null) 14169 this.name = new Reference(); 14170 if (!(this.name instanceof Reference)) 14171 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.name.getClass().getName() 14172 + " was encountered"); 14173 return (Reference) this.name; 14174 } 14175 14176 public boolean hasNameReference() { 14177 return this.name instanceof Reference; 14178 } 14179 14180 public boolean hasName() { 14181 return this.name != null && !this.name.isEmpty(); 14182 } 14183 14184 /** 14185 * @param value {@link #name} (The source address for the page.) 14186 */ 14187 public ImplementationGuideDefinitionPageComponent setName(Type value) { 14188 if (value != null && !(value instanceof UrlType || value instanceof Reference)) 14189 throw new Error("Not the right type for ImplementationGuide.definition.page.name[x]: " + value.fhirType()); 14190 this.name = value; 14191 return this; 14192 } 14193 14194 /** 14195 * @return {@link #title} (A short title used to represent this page in 14196 * navigational structures such as table of contents, bread crumbs, 14197 * etc.). This is the underlying object with id, value and extensions. 14198 * The accessor "getTitle" gives direct access to the value 14199 */ 14200 public StringType getTitleElement() { 14201 if (this.title == null) 14202 if (Configuration.errorOnAutoCreate()) 14203 throw new Error("Attempt to auto-create ImplementationGuideDefinitionPageComponent.title"); 14204 else if (Configuration.doAutoCreate()) 14205 this.title = new StringType(); // bb 14206 return this.title; 14207 } 14208 14209 public boolean hasTitleElement() { 14210 return this.title != null && !this.title.isEmpty(); 14211 } 14212 14213 public boolean hasTitle() { 14214 return this.title != null && !this.title.isEmpty(); 14215 } 14216 14217 /** 14218 * @param value {@link #title} (A short title used to represent this page in 14219 * navigational structures such as table of contents, bread crumbs, 14220 * etc.). This is the underlying object with id, value and 14221 * extensions. The accessor "getTitle" gives direct access to the 14222 * value 14223 */ 14224 public ImplementationGuideDefinitionPageComponent setTitleElement(StringType value) { 14225 this.title = value; 14226 return this; 14227 } 14228 14229 /** 14230 * @return A short title used to represent this page in navigational structures 14231 * such as table of contents, bread crumbs, etc. 14232 */ 14233 public String getTitle() { 14234 return this.title == null ? null : this.title.getValue(); 14235 } 14236 14237 /** 14238 * @param value A short title used to represent this page in navigational 14239 * structures such as table of contents, bread crumbs, etc. 14240 */ 14241 public ImplementationGuideDefinitionPageComponent setTitle(String value) { 14242 if (this.title == null) 14243 this.title = new StringType(); 14244 this.title.setValue(value); 14245 return this; 14246 } 14247 14248 /** 14249 * @return {@link #generation} (A code that indicates how the page is 14250 * generated.). This is the underlying object with id, value and 14251 * extensions. The accessor "getGeneration" gives direct access to the 14252 * value 14253 */ 14254 public Enumeration<GuidePageGeneration> getGenerationElement() { 14255 if (this.generation == null) 14256 if (Configuration.errorOnAutoCreate()) 14257 throw new Error("Attempt to auto-create ImplementationGuideDefinitionPageComponent.generation"); 14258 else if (Configuration.doAutoCreate()) 14259 this.generation = new Enumeration<GuidePageGeneration>(new GuidePageGenerationEnumFactory()); // bb 14260 return this.generation; 14261 } 14262 14263 public boolean hasGenerationElement() { 14264 return this.generation != null && !this.generation.isEmpty(); 14265 } 14266 14267 public boolean hasGeneration() { 14268 return this.generation != null && !this.generation.isEmpty(); 14269 } 14270 14271 /** 14272 * @param value {@link #generation} (A code that indicates how the page is 14273 * generated.). This is the underlying object with id, value and 14274 * extensions. The accessor "getGeneration" gives direct access to 14275 * the value 14276 */ 14277 public ImplementationGuideDefinitionPageComponent setGenerationElement(Enumeration<GuidePageGeneration> value) { 14278 this.generation = value; 14279 return this; 14280 } 14281 14282 /** 14283 * @return A code that indicates how the page is generated. 14284 */ 14285 public GuidePageGeneration getGeneration() { 14286 return this.generation == null ? null : this.generation.getValue(); 14287 } 14288 14289 /** 14290 * @param value A code that indicates how the page is generated. 14291 */ 14292 public ImplementationGuideDefinitionPageComponent setGeneration(GuidePageGeneration value) { 14293 if (this.generation == null) 14294 this.generation = new Enumeration<GuidePageGeneration>(new GuidePageGenerationEnumFactory()); 14295 this.generation.setValue(value); 14296 return this; 14297 } 14298 14299 /** 14300 * @return {@link #page} (Nested Pages/Sections under this page.) 14301 */ 14302 public List<ImplementationGuideDefinitionPageComponent> getPage() { 14303 if (this.page == null) 14304 this.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 14305 return this.page; 14306 } 14307 14308 /** 14309 * @return Returns a reference to <code>this</code> for easy method chaining 14310 */ 14311 public ImplementationGuideDefinitionPageComponent setPage( 14312 List<ImplementationGuideDefinitionPageComponent> thePage) { 14313 this.page = thePage; 14314 return this; 14315 } 14316 14317 public boolean hasPage() { 14318 if (this.page == null) 14319 return false; 14320 for (ImplementationGuideDefinitionPageComponent item : this.page) 14321 if (!item.isEmpty()) 14322 return true; 14323 return false; 14324 } 14325 14326 public ImplementationGuideDefinitionPageComponent addPage() { // 3 14327 ImplementationGuideDefinitionPageComponent t = new ImplementationGuideDefinitionPageComponent(); 14328 if (this.page == null) 14329 this.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 14330 this.page.add(t); 14331 return t; 14332 } 14333 14334 public ImplementationGuideDefinitionPageComponent addPage(ImplementationGuideDefinitionPageComponent t) { // 3 14335 if (t == null) 14336 return this; 14337 if (this.page == null) 14338 this.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 14339 this.page.add(t); 14340 return this; 14341 } 14342 14343 /** 14344 * @return The first repetition of repeating field {@link #page}, creating it if 14345 * it does not already exist 14346 */ 14347 public ImplementationGuideDefinitionPageComponent getPageFirstRep() { 14348 if (getPage().isEmpty()) { 14349 addPage(); 14350 } 14351 return getPage().get(0); 14352 } 14353 14354 protected void listChildren(List<Property> children) { 14355 super.listChildren(children); 14356 children.add(new Property("name[x]", "url|Reference(Binary)", "The source address for the page.", 0, 1, name)); 14357 children.add(new Property("title", "string", 14358 "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 14359 0, 1, title)); 14360 children.add( 14361 new Property("generation", "code", "A code that indicates how the page is generated.", 0, 1, generation)); 14362 children.add(new Property("page", "@ImplementationGuide.definition.page", 14363 "Nested Pages/Sections under this page.", 0, java.lang.Integer.MAX_VALUE, page)); 14364 } 14365 14366 @Override 14367 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14368 switch (_hash) { 14369 case 1721948693: 14370 /* name[x] */ return new Property("name[x]", "url|Reference(Binary)", "The source address for the page.", 0, 1, 14371 name); 14372 case 3373707: 14373 /* name */ return new Property("name[x]", "url|Reference(Binary)", "The source address for the page.", 0, 1, 14374 name); 14375 case 1721942756: 14376 /* nameUrl */ return new Property("name[x]", "url|Reference(Binary)", "The source address for the page.", 0, 1, 14377 name); 14378 case 1833144576: 14379 /* nameReference */ return new Property("name[x]", "url|Reference(Binary)", "The source address for the page.", 14380 0, 1, name); 14381 case 110371416: 14382 /* title */ return new Property("title", "string", 14383 "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 14384 0, 1, title); 14385 case 305703192: 14386 /* generation */ return new Property("generation", "code", "A code that indicates how the page is generated.", 14387 0, 1, generation); 14388 case 3433103: 14389 /* page */ return new Property("page", "@ImplementationGuide.definition.page", 14390 "Nested Pages/Sections under this page.", 0, java.lang.Integer.MAX_VALUE, page); 14391 default: 14392 return super.getNamedProperty(_hash, _name, _checkValid); 14393 } 14394 14395 } 14396 14397 @Override 14398 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14399 switch (hash) { 14400 case 3373707: 14401 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // Type 14402 case 110371416: 14403 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 14404 case 305703192: 14405 /* generation */ return this.generation == null ? new Base[0] : new Base[] { this.generation }; // Enumeration<GuidePageGeneration> 14406 case 3433103: 14407 /* page */ return this.page == null ? new Base[0] : this.page.toArray(new Base[this.page.size()]); // ImplementationGuideDefinitionPageComponent 14408 default: 14409 return super.getProperty(hash, name, checkValid); 14410 } 14411 14412 } 14413 14414 @Override 14415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14416 switch (hash) { 14417 case 3373707: // name 14418 this.name = castToType(value); // Type 14419 return value; 14420 case 110371416: // title 14421 this.title = castToString(value); // StringType 14422 return value; 14423 case 305703192: // generation 14424 value = new GuidePageGenerationEnumFactory().fromType(castToCode(value)); 14425 this.generation = (Enumeration) value; // Enumeration<GuidePageGeneration> 14426 return value; 14427 case 3433103: // page 14428 this.getPage().add((ImplementationGuideDefinitionPageComponent) value); // ImplementationGuideDefinitionPageComponent 14429 return value; 14430 default: 14431 return super.setProperty(hash, name, value); 14432 } 14433 14434 } 14435 14436 @Override 14437 public Base setProperty(String name, Base value) throws FHIRException { 14438 if (name.equals("name[x]")) { 14439 this.name = castToType(value); // Type 14440 } else if (name.equals("title")) { 14441 this.title = castToString(value); // StringType 14442 } else if (name.equals("generation")) { 14443 value = new GuidePageGenerationEnumFactory().fromType(castToCode(value)); 14444 this.generation = (Enumeration) value; // Enumeration<GuidePageGeneration> 14445 } else if (name.equals("page")) { 14446 this.getPage().add((ImplementationGuideDefinitionPageComponent) value); 14447 } else 14448 return super.setProperty(name, value); 14449 return value; 14450 } 14451 14452 @Override 14453 public void removeChild(String name, Base value) throws FHIRException { 14454 if (name.equals("name[x]")) { 14455 this.name = null; 14456 } else if (name.equals("title")) { 14457 this.title = null; 14458 } else if (name.equals("generation")) { 14459 this.generation = null; 14460 } else if (name.equals("page")) { 14461 this.getPage().remove((ImplementationGuideDefinitionPageComponent) value); 14462 } else 14463 super.removeChild(name, value); 14464 14465 } 14466 14467 @Override 14468 public Base makeProperty(int hash, String name) throws FHIRException { 14469 switch (hash) { 14470 case 1721948693: 14471 return getName(); 14472 case 3373707: 14473 return getName(); 14474 case 110371416: 14475 return getTitleElement(); 14476 case 305703192: 14477 return getGenerationElement(); 14478 case 3433103: 14479 return addPage(); 14480 default: 14481 return super.makeProperty(hash, name); 14482 } 14483 14484 } 14485 14486 @Override 14487 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14488 switch (hash) { 14489 case 3373707: 14490 /* name */ return new String[] { "url", "Reference" }; 14491 case 110371416: 14492 /* title */ return new String[] { "string" }; 14493 case 305703192: 14494 /* generation */ return new String[] { "code" }; 14495 case 3433103: 14496 /* page */ return new String[] { "@ImplementationGuide.definition.page" }; 14497 default: 14498 return super.getTypesForProperty(hash, name); 14499 } 14500 14501 } 14502 14503 @Override 14504 public Base addChild(String name) throws FHIRException { 14505 if (name.equals("nameUrl")) { 14506 this.name = new UrlType(); 14507 return this.name; 14508 } else if (name.equals("nameReference")) { 14509 this.name = new Reference(); 14510 return this.name; 14511 } else if (name.equals("title")) { 14512 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.title"); 14513 } else if (name.equals("generation")) { 14514 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.generation"); 14515 } else if (name.equals("page")) { 14516 return addPage(); 14517 } else 14518 return super.addChild(name); 14519 } 14520 14521 public ImplementationGuideDefinitionPageComponent copy() { 14522 ImplementationGuideDefinitionPageComponent dst = new ImplementationGuideDefinitionPageComponent(); 14523 copyValues(dst); 14524 return dst; 14525 } 14526 14527 public void copyValues(ImplementationGuideDefinitionPageComponent dst) { 14528 super.copyValues(dst); 14529 dst.name = name == null ? null : name.copy(); 14530 dst.title = title == null ? null : title.copy(); 14531 dst.generation = generation == null ? null : generation.copy(); 14532 if (page != null) { 14533 dst.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 14534 for (ImplementationGuideDefinitionPageComponent i : page) 14535 dst.page.add(i.copy()); 14536 } 14537 ; 14538 } 14539 14540 @Override 14541 public boolean equalsDeep(Base other_) { 14542 if (!super.equalsDeep(other_)) 14543 return false; 14544 if (!(other_ instanceof ImplementationGuideDefinitionPageComponent)) 14545 return false; 14546 ImplementationGuideDefinitionPageComponent o = (ImplementationGuideDefinitionPageComponent) other_; 14547 return compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 14548 && compareDeep(generation, o.generation, true) && compareDeep(page, o.page, true); 14549 } 14550 14551 @Override 14552 public boolean equalsShallow(Base other_) { 14553 if (!super.equalsShallow(other_)) 14554 return false; 14555 if (!(other_ instanceof ImplementationGuideDefinitionPageComponent)) 14556 return false; 14557 ImplementationGuideDefinitionPageComponent o = (ImplementationGuideDefinitionPageComponent) other_; 14558 return compareValues(title, o.title, true) && compareValues(generation, o.generation, true); 14559 } 14560 14561 public boolean isEmpty() { 14562 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, title, generation, page); 14563 } 14564 14565 public String fhirType() { 14566 return "ImplementationGuide.definition.page"; 14567 14568 } 14569 14570 } 14571 14572 @Block() 14573 public static class ImplementationGuideDefinitionParameterComponent extends BackboneElement 14574 implements IBaseBackboneElement { 14575 /** 14576 * apply | path-resource | path-pages | path-tx-cache | expansion-parameter | 14577 * rule-broken-links | generate-xml | generate-json | generate-turtle | 14578 * html-template. 14579 */ 14580 @Child(name = "code", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 14581 @Description(shortDefinition = "apply | path-resource | path-pages | path-tx-cache | expansion-parameter | rule-broken-links | generate-xml | generate-json | generate-turtle | html-template", formalDefinition = "apply | path-resource | path-pages | path-tx-cache | expansion-parameter | rule-broken-links | generate-xml | generate-json | generate-turtle | html-template.") 14582 protected StringType code; 14583 14584 /** 14585 * Value for named type. 14586 */ 14587 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 14588 @Description(shortDefinition = "Value for named type", formalDefinition = "Value for named type.") 14589 protected StringType value; 14590 14591 private static final long serialVersionUID = 1188999138L; 14592 14593 /** 14594 * Constructor 14595 */ 14596 public ImplementationGuideDefinitionParameterComponent() { 14597 super(); 14598 } 14599 14600 /** 14601 * Constructor 14602 */ 14603 public ImplementationGuideDefinitionParameterComponent(StringType code, StringType value) { 14604 super(); 14605 this.code = code; 14606 this.value = value; 14607 } 14608 14609 /** 14610 * @return {@link #code} (apply | path-resource | path-pages | path-tx-cache | 14611 * expansion-parameter | rule-broken-links | generate-xml | 14612 * generate-json | generate-turtle | html-template.). This is the 14613 * underlying object with id, value and extensions. The accessor 14614 * "getCode" gives direct access to the value 14615 */ 14616 public StringType getCodeElement() { 14617 if (this.code == null) 14618 if (Configuration.errorOnAutoCreate()) 14619 throw new Error("Attempt to auto-create ImplementationGuideDefinitionParameterComponent.code"); 14620 else if (Configuration.doAutoCreate()) 14621 this.code = new StringType(); // bb 14622 return this.code; 14623 } 14624 14625 public boolean hasCodeElement() { 14626 return this.code != null && !this.code.isEmpty(); 14627 } 14628 14629 public boolean hasCode() { 14630 return this.code != null && !this.code.isEmpty(); 14631 } 14632 14633 /** 14634 * @param value {@link #code} (apply | path-resource | path-pages | 14635 * path-tx-cache | expansion-parameter | rule-broken-links | 14636 * generate-xml | generate-json | generate-turtle | 14637 * html-template.). This is the underlying object with id, value 14638 * and extensions. The accessor "getCode" gives direct access to 14639 * the value 14640 */ 14641 public ImplementationGuideDefinitionParameterComponent setCodeElement(StringType value) { 14642 this.code = value; 14643 return this; 14644 } 14645 14646 /** 14647 * @return apply | path-resource | path-pages | path-tx-cache | 14648 * expansion-parameter | rule-broken-links | generate-xml | 14649 * generate-json | generate-turtle | html-template. 14650 */ 14651 public String getCode() { 14652 return this.code == null ? null : this.code.getValue(); 14653 } 14654 14655 /** 14656 * @param value apply | path-resource | path-pages | path-tx-cache | 14657 * expansion-parameter | rule-broken-links | generate-xml | 14658 * generate-json | generate-turtle | html-template. 14659 */ 14660 public ImplementationGuideDefinitionParameterComponent setCode(String value) { 14661 if (this.code == null) 14662 this.code = new StringType(); 14663 this.code.setValue(value); 14664 return this; 14665 } 14666 14667 /** 14668 * @return {@link #value} (Value for named type.). This is the underlying object 14669 * with id, value and extensions. The accessor "getValue" gives direct 14670 * access to the value 14671 */ 14672 public StringType getValueElement() { 14673 if (this.value == null) 14674 if (Configuration.errorOnAutoCreate()) 14675 throw new Error("Attempt to auto-create ImplementationGuideDefinitionParameterComponent.value"); 14676 else if (Configuration.doAutoCreate()) 14677 this.value = new StringType(); // bb 14678 return this.value; 14679 } 14680 14681 public boolean hasValueElement() { 14682 return this.value != null && !this.value.isEmpty(); 14683 } 14684 14685 public boolean hasValue() { 14686 return this.value != null && !this.value.isEmpty(); 14687 } 14688 14689 /** 14690 * @param value {@link #value} (Value for named type.). This is the underlying 14691 * object with id, value and extensions. The accessor "getValue" 14692 * gives direct access to the value 14693 */ 14694 public ImplementationGuideDefinitionParameterComponent setValueElement(StringType value) { 14695 this.value = value; 14696 return this; 14697 } 14698 14699 /** 14700 * @return Value for named type. 14701 */ 14702 public String getValue() { 14703 return this.value == null ? null : this.value.getValue(); 14704 } 14705 14706 /** 14707 * @param value Value for named type. 14708 */ 14709 public ImplementationGuideDefinitionParameterComponent setValue(String value) { 14710 if (this.value == null) 14711 this.value = new StringType(); 14712 this.value.setValue(value); 14713 return this; 14714 } 14715 14716 protected void listChildren(List<Property> children) { 14717 super.listChildren(children); 14718 children.add(new Property("code", "string", 14719 "apply | path-resource | path-pages | path-tx-cache | expansion-parameter | rule-broken-links | generate-xml | generate-json | generate-turtle | html-template.", 14720 0, 1, code)); 14721 children.add(new Property("value", "string", "Value for named type.", 0, 1, value)); 14722 } 14723 14724 @Override 14725 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14726 switch (_hash) { 14727 case 3059181: 14728 /* code */ return new Property("code", "string", 14729 "apply | path-resource | path-pages | path-tx-cache | expansion-parameter | rule-broken-links | generate-xml | generate-json | generate-turtle | html-template.", 14730 0, 1, code); 14731 case 111972721: 14732 /* value */ return new Property("value", "string", "Value for named type.", 0, 1, value); 14733 default: 14734 return super.getNamedProperty(_hash, _name, _checkValid); 14735 } 14736 14737 } 14738 14739 @Override 14740 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14741 switch (hash) { 14742 case 3059181: 14743 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // StringType 14744 case 111972721: 14745 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 14746 default: 14747 return super.getProperty(hash, name, checkValid); 14748 } 14749 14750 } 14751 14752 @Override 14753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14754 switch (hash) { 14755 case 3059181: // code 14756 this.code = castToString(value); // StringType 14757 return value; 14758 case 111972721: // value 14759 this.value = castToString(value); // StringType 14760 return value; 14761 default: 14762 return super.setProperty(hash, name, value); 14763 } 14764 14765 } 14766 14767 @Override 14768 public Base setProperty(String name, Base value) throws FHIRException { 14769 if (name.equals("code")) { 14770 this.code = castToString(value); // StringType 14771 } else if (name.equals("value")) { 14772 this.value = castToString(value); // StringType 14773 } else 14774 return super.setProperty(name, value); 14775 return value; 14776 } 14777 14778 @Override 14779 public void removeChild(String name, Base value) throws FHIRException { 14780 if (name.equals("code")) { 14781 this.code = null; 14782 } else if (name.equals("value")) { 14783 this.value = null; 14784 } else 14785 super.removeChild(name, value); 14786 14787 } 14788 14789 @Override 14790 public Base makeProperty(int hash, String name) throws FHIRException { 14791 switch (hash) { 14792 case 3059181: 14793 return getCodeElement(); 14794 case 111972721: 14795 return getValueElement(); 14796 default: 14797 return super.makeProperty(hash, name); 14798 } 14799 14800 } 14801 14802 @Override 14803 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14804 switch (hash) { 14805 case 3059181: 14806 /* code */ return new String[] { "string" }; 14807 case 111972721: 14808 /* value */ return new String[] { "string" }; 14809 default: 14810 return super.getTypesForProperty(hash, name); 14811 } 14812 14813 } 14814 14815 @Override 14816 public Base addChild(String name) throws FHIRException { 14817 if (name.equals("code")) { 14818 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.code"); 14819 } else if (name.equals("value")) { 14820 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.value"); 14821 } else 14822 return super.addChild(name); 14823 } 14824 14825 public ImplementationGuideDefinitionParameterComponent copy() { 14826 ImplementationGuideDefinitionParameterComponent dst = new ImplementationGuideDefinitionParameterComponent(); 14827 copyValues(dst); 14828 return dst; 14829 } 14830 14831 public void copyValues(ImplementationGuideDefinitionParameterComponent dst) { 14832 super.copyValues(dst); 14833 dst.code = code == null ? null : code.copy(); 14834 dst.value = value == null ? null : value.copy(); 14835 } 14836 14837 @Override 14838 public boolean equalsDeep(Base other_) { 14839 if (!super.equalsDeep(other_)) 14840 return false; 14841 if (!(other_ instanceof ImplementationGuideDefinitionParameterComponent)) 14842 return false; 14843 ImplementationGuideDefinitionParameterComponent o = (ImplementationGuideDefinitionParameterComponent) other_; 14844 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 14845 } 14846 14847 @Override 14848 public boolean equalsShallow(Base other_) { 14849 if (!super.equalsShallow(other_)) 14850 return false; 14851 if (!(other_ instanceof ImplementationGuideDefinitionParameterComponent)) 14852 return false; 14853 ImplementationGuideDefinitionParameterComponent o = (ImplementationGuideDefinitionParameterComponent) other_; 14854 return compareValues(code, o.code, true) && compareValues(value, o.value, true); 14855 } 14856 14857 public boolean isEmpty() { 14858 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 14859 } 14860 14861 public String fhirType() { 14862 return "ImplementationGuide.definition.parameter"; 14863 14864 } 14865 14866 } 14867 14868 @Block() 14869 public static class ImplementationGuideDefinitionTemplateComponent extends BackboneElement 14870 implements IBaseBackboneElement { 14871 /** 14872 * Type of template specified. 14873 */ 14874 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 14875 @Description(shortDefinition = "Type of template specified", formalDefinition = "Type of template specified.") 14876 protected CodeType code; 14877 14878 /** 14879 * The source location for the template. 14880 */ 14881 @Child(name = "source", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 14882 @Description(shortDefinition = "The source location for the template", formalDefinition = "The source location for the template.") 14883 protected StringType source; 14884 14885 /** 14886 * The scope in which the template applies. 14887 */ 14888 @Child(name = "scope", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 14889 @Description(shortDefinition = "The scope in which the template applies", formalDefinition = "The scope in which the template applies.") 14890 protected StringType scope; 14891 14892 private static final long serialVersionUID = 923832457L; 14893 14894 /** 14895 * Constructor 14896 */ 14897 public ImplementationGuideDefinitionTemplateComponent() { 14898 super(); 14899 } 14900 14901 /** 14902 * Constructor 14903 */ 14904 public ImplementationGuideDefinitionTemplateComponent(CodeType code, StringType source) { 14905 super(); 14906 this.code = code; 14907 this.source = source; 14908 } 14909 14910 /** 14911 * @return {@link #code} (Type of template specified.). This is the underlying 14912 * object with id, value and extensions. The accessor "getCode" gives 14913 * direct access to the value 14914 */ 14915 public CodeType getCodeElement() { 14916 if (this.code == null) 14917 if (Configuration.errorOnAutoCreate()) 14918 throw new Error("Attempt to auto-create ImplementationGuideDefinitionTemplateComponent.code"); 14919 else if (Configuration.doAutoCreate()) 14920 this.code = new CodeType(); // bb 14921 return this.code; 14922 } 14923 14924 public boolean hasCodeElement() { 14925 return this.code != null && !this.code.isEmpty(); 14926 } 14927 14928 public boolean hasCode() { 14929 return this.code != null && !this.code.isEmpty(); 14930 } 14931 14932 /** 14933 * @param value {@link #code} (Type of template specified.). This is the 14934 * underlying object with id, value and extensions. The accessor 14935 * "getCode" gives direct access to the value 14936 */ 14937 public ImplementationGuideDefinitionTemplateComponent setCodeElement(CodeType value) { 14938 this.code = value; 14939 return this; 14940 } 14941 14942 /** 14943 * @return Type of template specified. 14944 */ 14945 public String getCode() { 14946 return this.code == null ? null : this.code.getValue(); 14947 } 14948 14949 /** 14950 * @param value Type of template specified. 14951 */ 14952 public ImplementationGuideDefinitionTemplateComponent setCode(String value) { 14953 if (this.code == null) 14954 this.code = new CodeType(); 14955 this.code.setValue(value); 14956 return this; 14957 } 14958 14959 /** 14960 * @return {@link #source} (The source location for the template.). This is the 14961 * underlying object with id, value and extensions. The accessor 14962 * "getSource" gives direct access to the value 14963 */ 14964 public StringType getSourceElement() { 14965 if (this.source == null) 14966 if (Configuration.errorOnAutoCreate()) 14967 throw new Error("Attempt to auto-create ImplementationGuideDefinitionTemplateComponent.source"); 14968 else if (Configuration.doAutoCreate()) 14969 this.source = new StringType(); // bb 14970 return this.source; 14971 } 14972 14973 public boolean hasSourceElement() { 14974 return this.source != null && !this.source.isEmpty(); 14975 } 14976 14977 public boolean hasSource() { 14978 return this.source != null && !this.source.isEmpty(); 14979 } 14980 14981 /** 14982 * @param value {@link #source} (The source location for the template.). This is 14983 * the underlying object with id, value and extensions. The 14984 * accessor "getSource" gives direct access to the value 14985 */ 14986 public ImplementationGuideDefinitionTemplateComponent setSourceElement(StringType value) { 14987 this.source = value; 14988 return this; 14989 } 14990 14991 /** 14992 * @return The source location for the template. 14993 */ 14994 public String getSource() { 14995 return this.source == null ? null : this.source.getValue(); 14996 } 14997 14998 /** 14999 * @param value The source location for the template. 15000 */ 15001 public ImplementationGuideDefinitionTemplateComponent setSource(String value) { 15002 if (this.source == null) 15003 this.source = new StringType(); 15004 this.source.setValue(value); 15005 return this; 15006 } 15007 15008 /** 15009 * @return {@link #scope} (The scope in which the template applies.). This is 15010 * the underlying object with id, value and extensions. The accessor 15011 * "getScope" gives direct access to the value 15012 */ 15013 public StringType getScopeElement() { 15014 if (this.scope == null) 15015 if (Configuration.errorOnAutoCreate()) 15016 throw new Error("Attempt to auto-create ImplementationGuideDefinitionTemplateComponent.scope"); 15017 else if (Configuration.doAutoCreate()) 15018 this.scope = new StringType(); // bb 15019 return this.scope; 15020 } 15021 15022 public boolean hasScopeElement() { 15023 return this.scope != null && !this.scope.isEmpty(); 15024 } 15025 15026 public boolean hasScope() { 15027 return this.scope != null && !this.scope.isEmpty(); 15028 } 15029 15030 /** 15031 * @param value {@link #scope} (The scope in which the template applies.). This 15032 * is the underlying object with id, value and extensions. The 15033 * accessor "getScope" gives direct access to the value 15034 */ 15035 public ImplementationGuideDefinitionTemplateComponent setScopeElement(StringType value) { 15036 this.scope = value; 15037 return this; 15038 } 15039 15040 /** 15041 * @return The scope in which the template applies. 15042 */ 15043 public String getScope() { 15044 return this.scope == null ? null : this.scope.getValue(); 15045 } 15046 15047 /** 15048 * @param value The scope in which the template applies. 15049 */ 15050 public ImplementationGuideDefinitionTemplateComponent setScope(String value) { 15051 if (Utilities.noString(value)) 15052 this.scope = null; 15053 else { 15054 if (this.scope == null) 15055 this.scope = new StringType(); 15056 this.scope.setValue(value); 15057 } 15058 return this; 15059 } 15060 15061 protected void listChildren(List<Property> children) { 15062 super.listChildren(children); 15063 children.add(new Property("code", "code", "Type of template specified.", 0, 1, code)); 15064 children.add(new Property("source", "string", "The source location for the template.", 0, 1, source)); 15065 children.add(new Property("scope", "string", "The scope in which the template applies.", 0, 1, scope)); 15066 } 15067 15068 @Override 15069 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 15070 switch (_hash) { 15071 case 3059181: 15072 /* code */ return new Property("code", "code", "Type of template specified.", 0, 1, code); 15073 case -896505829: 15074 /* source */ return new Property("source", "string", "The source location for the template.", 0, 1, source); 15075 case 109264468: 15076 /* scope */ return new Property("scope", "string", "The scope in which the template applies.", 0, 1, scope); 15077 default: 15078 return super.getNamedProperty(_hash, _name, _checkValid); 15079 } 15080 15081 } 15082 15083 @Override 15084 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 15085 switch (hash) { 15086 case 3059181: 15087 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 15088 case -896505829: 15089 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // StringType 15090 case 109264468: 15091 /* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // StringType 15092 default: 15093 return super.getProperty(hash, name, checkValid); 15094 } 15095 15096 } 15097 15098 @Override 15099 public Base setProperty(int hash, String name, Base value) throws FHIRException { 15100 switch (hash) { 15101 case 3059181: // code 15102 this.code = castToCode(value); // CodeType 15103 return value; 15104 case -896505829: // source 15105 this.source = castToString(value); // StringType 15106 return value; 15107 case 109264468: // scope 15108 this.scope = castToString(value); // StringType 15109 return value; 15110 default: 15111 return super.setProperty(hash, name, value); 15112 } 15113 15114 } 15115 15116 @Override 15117 public Base setProperty(String name, Base value) throws FHIRException { 15118 if (name.equals("code")) { 15119 this.code = castToCode(value); // CodeType 15120 } else if (name.equals("source")) { 15121 this.source = castToString(value); // StringType 15122 } else if (name.equals("scope")) { 15123 this.scope = castToString(value); // StringType 15124 } else 15125 return super.setProperty(name, value); 15126 return value; 15127 } 15128 15129 @Override 15130 public void removeChild(String name, Base value) throws FHIRException { 15131 if (name.equals("code")) { 15132 this.code = null; 15133 } else if (name.equals("source")) { 15134 this.source = null; 15135 } else if (name.equals("scope")) { 15136 this.scope = null; 15137 } else 15138 super.removeChild(name, value); 15139 15140 } 15141 15142 @Override 15143 public Base makeProperty(int hash, String name) throws FHIRException { 15144 switch (hash) { 15145 case 3059181: 15146 return getCodeElement(); 15147 case -896505829: 15148 return getSourceElement(); 15149 case 109264468: 15150 return getScopeElement(); 15151 default: 15152 return super.makeProperty(hash, name); 15153 } 15154 15155 } 15156 15157 @Override 15158 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 15159 switch (hash) { 15160 case 3059181: 15161 /* code */ return new String[] { "code" }; 15162 case -896505829: 15163 /* source */ return new String[] { "string" }; 15164 case 109264468: 15165 /* scope */ return new String[] { "string" }; 15166 default: 15167 return super.getTypesForProperty(hash, name); 15168 } 15169 15170 } 15171 15172 @Override 15173 public Base addChild(String name) throws FHIRException { 15174 if (name.equals("code")) { 15175 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.code"); 15176 } else if (name.equals("source")) { 15177 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.source"); 15178 } else if (name.equals("scope")) { 15179 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.scope"); 15180 } else 15181 return super.addChild(name); 15182 } 15183 15184 public ImplementationGuideDefinitionTemplateComponent copy() { 15185 ImplementationGuideDefinitionTemplateComponent dst = new ImplementationGuideDefinitionTemplateComponent(); 15186 copyValues(dst); 15187 return dst; 15188 } 15189 15190 public void copyValues(ImplementationGuideDefinitionTemplateComponent dst) { 15191 super.copyValues(dst); 15192 dst.code = code == null ? null : code.copy(); 15193 dst.source = source == null ? null : source.copy(); 15194 dst.scope = scope == null ? null : scope.copy(); 15195 } 15196 15197 @Override 15198 public boolean equalsDeep(Base other_) { 15199 if (!super.equalsDeep(other_)) 15200 return false; 15201 if (!(other_ instanceof ImplementationGuideDefinitionTemplateComponent)) 15202 return false; 15203 ImplementationGuideDefinitionTemplateComponent o = (ImplementationGuideDefinitionTemplateComponent) other_; 15204 return compareDeep(code, o.code, true) && compareDeep(source, o.source, true) 15205 && compareDeep(scope, o.scope, true); 15206 } 15207 15208 @Override 15209 public boolean equalsShallow(Base other_) { 15210 if (!super.equalsShallow(other_)) 15211 return false; 15212 if (!(other_ instanceof ImplementationGuideDefinitionTemplateComponent)) 15213 return false; 15214 ImplementationGuideDefinitionTemplateComponent o = (ImplementationGuideDefinitionTemplateComponent) other_; 15215 return compareValues(code, o.code, true) && compareValues(source, o.source, true) 15216 && compareValues(scope, o.scope, true); 15217 } 15218 15219 public boolean isEmpty() { 15220 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, source, scope); 15221 } 15222 15223 public String fhirType() { 15224 return "ImplementationGuide.definition.template"; 15225 15226 } 15227 15228 } 15229 15230 @Block() 15231 public static class ImplementationGuideManifestComponent extends BackboneElement implements IBaseBackboneElement { 15232 /** 15233 * A pointer to official web page, PDF or other rendering of the implementation 15234 * guide. 15235 */ 15236 @Child(name = "rendering", type = { UrlType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 15237 @Description(shortDefinition = "Location of rendered implementation guide", formalDefinition = "A pointer to official web page, PDF or other rendering of the implementation guide.") 15238 protected UrlType rendering; 15239 15240 /** 15241 * A resource that is part of the implementation guide. Conformance resources 15242 * (value set, structure definition, capability statements etc.) are obvious 15243 * candidates for inclusion, but any kind of resource can be included as an 15244 * example resource. 15245 */ 15246 @Child(name = "resource", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 15247 @Description(shortDefinition = "Resource in the implementation guide", formalDefinition = "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.") 15248 protected List<ManifestResourceComponent> resource; 15249 15250 /** 15251 * Information about a page within the IG. 15252 */ 15253 @Child(name = "page", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15254 @Description(shortDefinition = "HTML page within the parent IG", formalDefinition = "Information about a page within the IG.") 15255 protected List<ManifestPageComponent> page; 15256 15257 /** 15258 * Indicates a relative path to an image that exists within the IG. 15259 */ 15260 @Child(name = "image", type = { 15261 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15262 @Description(shortDefinition = "Image within the IG", formalDefinition = "Indicates a relative path to an image that exists within the IG.") 15263 protected List<StringType> image; 15264 15265 /** 15266 * Indicates the relative path of an additional non-page, non-image file that is 15267 * part of the IG - e.g. zip, jar and similar files that could be the target of 15268 * a hyperlink in a derived IG. 15269 */ 15270 @Child(name = "other", type = { 15271 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 15272 @Description(shortDefinition = "Additional linkable file in IG", formalDefinition = "Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.") 15273 protected List<StringType> other; 15274 15275 private static final long serialVersionUID = 1881327712L; 15276 15277 /** 15278 * Constructor 15279 */ 15280 public ImplementationGuideManifestComponent() { 15281 super(); 15282 } 15283 15284 /** 15285 * @return {@link #rendering} (A pointer to official web page, PDF or other 15286 * rendering of the implementation guide.). This is the underlying 15287 * object with id, value and extensions. The accessor "getRendering" 15288 * gives direct access to the value 15289 */ 15290 public UrlType getRenderingElement() { 15291 if (this.rendering == null) 15292 if (Configuration.errorOnAutoCreate()) 15293 throw new Error("Attempt to auto-create ImplementationGuideManifestComponent.rendering"); 15294 else if (Configuration.doAutoCreate()) 15295 this.rendering = new UrlType(); // bb 15296 return this.rendering; 15297 } 15298 15299 public boolean hasRenderingElement() { 15300 return this.rendering != null && !this.rendering.isEmpty(); 15301 } 15302 15303 public boolean hasRendering() { 15304 return this.rendering != null && !this.rendering.isEmpty(); 15305 } 15306 15307 /** 15308 * @param value {@link #rendering} (A pointer to official web page, PDF or other 15309 * rendering of the implementation guide.). This is the underlying 15310 * object with id, value and extensions. The accessor 15311 * "getRendering" gives direct access to the value 15312 */ 15313 public ImplementationGuideManifestComponent setRenderingElement(UrlType value) { 15314 this.rendering = value; 15315 return this; 15316 } 15317 15318 /** 15319 * @return A pointer to official web page, PDF or other rendering of the 15320 * implementation guide. 15321 */ 15322 public String getRendering() { 15323 return this.rendering == null ? null : this.rendering.getValue(); 15324 } 15325 15326 /** 15327 * @param value A pointer to official web page, PDF or other rendering of the 15328 * implementation guide. 15329 */ 15330 public ImplementationGuideManifestComponent setRendering(String value) { 15331 if (Utilities.noString(value)) 15332 this.rendering = null; 15333 else { 15334 if (this.rendering == null) 15335 this.rendering = new UrlType(); 15336 this.rendering.setValue(value); 15337 } 15338 return this; 15339 } 15340 15341 /** 15342 * @return {@link #resource} (A resource that is part of the implementation 15343 * guide. Conformance resources (value set, structure definition, 15344 * capability statements etc.) are obvious candidates for inclusion, but 15345 * any kind of resource can be included as an example resource.) 15346 */ 15347 public List<ManifestResourceComponent> getResource() { 15348 if (this.resource == null) 15349 this.resource = new ArrayList<ManifestResourceComponent>(); 15350 return this.resource; 15351 } 15352 15353 /** 15354 * @return Returns a reference to <code>this</code> for easy method chaining 15355 */ 15356 public ImplementationGuideManifestComponent setResource(List<ManifestResourceComponent> theResource) { 15357 this.resource = theResource; 15358 return this; 15359 } 15360 15361 public boolean hasResource() { 15362 if (this.resource == null) 15363 return false; 15364 for (ManifestResourceComponent item : this.resource) 15365 if (!item.isEmpty()) 15366 return true; 15367 return false; 15368 } 15369 15370 public ManifestResourceComponent addResource() { // 3 15371 ManifestResourceComponent t = new ManifestResourceComponent(); 15372 if (this.resource == null) 15373 this.resource = new ArrayList<ManifestResourceComponent>(); 15374 this.resource.add(t); 15375 return t; 15376 } 15377 15378 public ImplementationGuideManifestComponent addResource(ManifestResourceComponent t) { // 3 15379 if (t == null) 15380 return this; 15381 if (this.resource == null) 15382 this.resource = new ArrayList<ManifestResourceComponent>(); 15383 this.resource.add(t); 15384 return this; 15385 } 15386 15387 /** 15388 * @return The first repetition of repeating field {@link #resource}, creating 15389 * it if it does not already exist 15390 */ 15391 public ManifestResourceComponent getResourceFirstRep() { 15392 if (getResource().isEmpty()) { 15393 addResource(); 15394 } 15395 return getResource().get(0); 15396 } 15397 15398 /** 15399 * @return {@link #page} (Information about a page within the IG.) 15400 */ 15401 public List<ManifestPageComponent> getPage() { 15402 if (this.page == null) 15403 this.page = new ArrayList<ManifestPageComponent>(); 15404 return this.page; 15405 } 15406 15407 /** 15408 * @return Returns a reference to <code>this</code> for easy method chaining 15409 */ 15410 public ImplementationGuideManifestComponent setPage(List<ManifestPageComponent> thePage) { 15411 this.page = thePage; 15412 return this; 15413 } 15414 15415 public boolean hasPage() { 15416 if (this.page == null) 15417 return false; 15418 for (ManifestPageComponent item : this.page) 15419 if (!item.isEmpty()) 15420 return true; 15421 return false; 15422 } 15423 15424 public ManifestPageComponent addPage() { // 3 15425 ManifestPageComponent t = new ManifestPageComponent(); 15426 if (this.page == null) 15427 this.page = new ArrayList<ManifestPageComponent>(); 15428 this.page.add(t); 15429 return t; 15430 } 15431 15432 public ImplementationGuideManifestComponent addPage(ManifestPageComponent t) { // 3 15433 if (t == null) 15434 return this; 15435 if (this.page == null) 15436 this.page = new ArrayList<ManifestPageComponent>(); 15437 this.page.add(t); 15438 return this; 15439 } 15440 15441 /** 15442 * @return The first repetition of repeating field {@link #page}, creating it if 15443 * it does not already exist 15444 */ 15445 public ManifestPageComponent getPageFirstRep() { 15446 if (getPage().isEmpty()) { 15447 addPage(); 15448 } 15449 return getPage().get(0); 15450 } 15451 15452 /** 15453 * @return {@link #image} (Indicates a relative path to an image that exists 15454 * within the IG.) 15455 */ 15456 public List<StringType> getImage() { 15457 if (this.image == null) 15458 this.image = new ArrayList<StringType>(); 15459 return this.image; 15460 } 15461 15462 /** 15463 * @return Returns a reference to <code>this</code> for easy method chaining 15464 */ 15465 public ImplementationGuideManifestComponent setImage(List<StringType> theImage) { 15466 this.image = theImage; 15467 return this; 15468 } 15469 15470 public boolean hasImage() { 15471 if (this.image == null) 15472 return false; 15473 for (StringType item : this.image) 15474 if (!item.isEmpty()) 15475 return true; 15476 return false; 15477 } 15478 15479 /** 15480 * @return {@link #image} (Indicates a relative path to an image that exists 15481 * within the IG.) 15482 */ 15483 public StringType addImageElement() {// 2 15484 StringType t = new StringType(); 15485 if (this.image == null) 15486 this.image = new ArrayList<StringType>(); 15487 this.image.add(t); 15488 return t; 15489 } 15490 15491 /** 15492 * @param value {@link #image} (Indicates a relative path to an image that 15493 * exists within the IG.) 15494 */ 15495 public ImplementationGuideManifestComponent addImage(String value) { // 1 15496 StringType t = new StringType(); 15497 t.setValue(value); 15498 if (this.image == null) 15499 this.image = new ArrayList<StringType>(); 15500 this.image.add(t); 15501 return this; 15502 } 15503 15504 /** 15505 * @param value {@link #image} (Indicates a relative path to an image that 15506 * exists within the IG.) 15507 */ 15508 public boolean hasImage(String value) { 15509 if (this.image == null) 15510 return false; 15511 for (StringType v : this.image) 15512 if (v.getValue().equals(value)) // string 15513 return true; 15514 return false; 15515 } 15516 15517 /** 15518 * @return {@link #other} (Indicates the relative path of an additional 15519 * non-page, non-image file that is part of the IG - e.g. zip, jar and 15520 * similar files that could be the target of a hyperlink in a derived 15521 * IG.) 15522 */ 15523 public List<StringType> getOther() { 15524 if (this.other == null) 15525 this.other = new ArrayList<StringType>(); 15526 return this.other; 15527 } 15528 15529 /** 15530 * @return Returns a reference to <code>this</code> for easy method chaining 15531 */ 15532 public ImplementationGuideManifestComponent setOther(List<StringType> theOther) { 15533 this.other = theOther; 15534 return this; 15535 } 15536 15537 public boolean hasOther() { 15538 if (this.other == null) 15539 return false; 15540 for (StringType item : this.other) 15541 if (!item.isEmpty()) 15542 return true; 15543 return false; 15544 } 15545 15546 /** 15547 * @return {@link #other} (Indicates the relative path of an additional 15548 * non-page, non-image file that is part of the IG - e.g. zip, jar and 15549 * similar files that could be the target of a hyperlink in a derived 15550 * IG.) 15551 */ 15552 public StringType addOtherElement() {// 2 15553 StringType t = new StringType(); 15554 if (this.other == null) 15555 this.other = new ArrayList<StringType>(); 15556 this.other.add(t); 15557 return t; 15558 } 15559 15560 /** 15561 * @param value {@link #other} (Indicates the relative path of an additional 15562 * non-page, non-image file that is part of the IG - e.g. zip, jar 15563 * and similar files that could be the target of a hyperlink in a 15564 * derived IG.) 15565 */ 15566 public ImplementationGuideManifestComponent addOther(String value) { // 1 15567 StringType t = new StringType(); 15568 t.setValue(value); 15569 if (this.other == null) 15570 this.other = new ArrayList<StringType>(); 15571 this.other.add(t); 15572 return this; 15573 } 15574 15575 /** 15576 * @param value {@link #other} (Indicates the relative path of an additional 15577 * non-page, non-image file that is part of the IG - e.g. zip, jar 15578 * and similar files that could be the target of a hyperlink in a 15579 * derived IG.) 15580 */ 15581 public boolean hasOther(String value) { 15582 if (this.other == null) 15583 return false; 15584 for (StringType v : this.other) 15585 if (v.getValue().equals(value)) // string 15586 return true; 15587 return false; 15588 } 15589 15590 protected void listChildren(List<Property> children) { 15591 super.listChildren(children); 15592 children.add(new Property("rendering", "url", 15593 "A pointer to official web page, PDF or other rendering of the implementation guide.", 0, 1, rendering)); 15594 children.add(new Property("resource", "", 15595 "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 15596 0, java.lang.Integer.MAX_VALUE, resource)); 15597 children.add( 15598 new Property("page", "", "Information about a page within the IG.", 0, java.lang.Integer.MAX_VALUE, page)); 15599 children.add(new Property("image", "string", "Indicates a relative path to an image that exists within the IG.", 15600 0, java.lang.Integer.MAX_VALUE, image)); 15601 children.add(new Property("other", "string", 15602 "Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.", 15603 0, java.lang.Integer.MAX_VALUE, other)); 15604 } 15605 15606 @Override 15607 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 15608 switch (_hash) { 15609 case 1839654540: 15610 /* rendering */ return new Property("rendering", "url", 15611 "A pointer to official web page, PDF or other rendering of the implementation guide.", 0, 1, rendering); 15612 case -341064690: 15613 /* resource */ return new Property("resource", "", 15614 "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 15615 0, java.lang.Integer.MAX_VALUE, resource); 15616 case 3433103: 15617 /* page */ return new Property("page", "", "Information about a page within the IG.", 0, 15618 java.lang.Integer.MAX_VALUE, page); 15619 case 100313435: 15620 /* image */ return new Property("image", "string", 15621 "Indicates a relative path to an image that exists within the IG.", 0, java.lang.Integer.MAX_VALUE, image); 15622 case 106069776: 15623 /* other */ return new Property("other", "string", 15624 "Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.", 15625 0, java.lang.Integer.MAX_VALUE, other); 15626 default: 15627 return super.getNamedProperty(_hash, _name, _checkValid); 15628 } 15629 15630 } 15631 15632 @Override 15633 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 15634 switch (hash) { 15635 case 1839654540: 15636 /* rendering */ return this.rendering == null ? new Base[0] : new Base[] { this.rendering }; // UrlType 15637 case -341064690: 15638 /* resource */ return this.resource == null ? new Base[0] 15639 : this.resource.toArray(new Base[this.resource.size()]); // ManifestResourceComponent 15640 case 3433103: 15641 /* page */ return this.page == null ? new Base[0] : this.page.toArray(new Base[this.page.size()]); // ManifestPageComponent 15642 case 100313435: 15643 /* image */ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // StringType 15644 case 106069776: 15645 /* other */ return this.other == null ? new Base[0] : this.other.toArray(new Base[this.other.size()]); // StringType 15646 default: 15647 return super.getProperty(hash, name, checkValid); 15648 } 15649 15650 } 15651 15652 @Override 15653 public Base setProperty(int hash, String name, Base value) throws FHIRException { 15654 switch (hash) { 15655 case 1839654540: // rendering 15656 this.rendering = castToUrl(value); // UrlType 15657 return value; 15658 case -341064690: // resource 15659 this.getResource().add((ManifestResourceComponent) value); // ManifestResourceComponent 15660 return value; 15661 case 3433103: // page 15662 this.getPage().add((ManifestPageComponent) value); // ManifestPageComponent 15663 return value; 15664 case 100313435: // image 15665 this.getImage().add(castToString(value)); // StringType 15666 return value; 15667 case 106069776: // other 15668 this.getOther().add(castToString(value)); // StringType 15669 return value; 15670 default: 15671 return super.setProperty(hash, name, value); 15672 } 15673 15674 } 15675 15676 @Override 15677 public Base setProperty(String name, Base value) throws FHIRException { 15678 if (name.equals("rendering")) { 15679 this.rendering = castToUrl(value); // UrlType 15680 } else if (name.equals("resource")) { 15681 this.getResource().add((ManifestResourceComponent) value); 15682 } else if (name.equals("page")) { 15683 this.getPage().add((ManifestPageComponent) value); 15684 } else if (name.equals("image")) { 15685 this.getImage().add(castToString(value)); 15686 } else if (name.equals("other")) { 15687 this.getOther().add(castToString(value)); 15688 } else 15689 return super.setProperty(name, value); 15690 return value; 15691 } 15692 15693 @Override 15694 public void removeChild(String name, Base value) throws FHIRException { 15695 if (name.equals("rendering")) { 15696 this.rendering = null; 15697 } else if (name.equals("resource")) { 15698 this.getResource().remove((ManifestResourceComponent) value); 15699 } else if (name.equals("page")) { 15700 this.getPage().remove((ManifestPageComponent) value); 15701 } else if (name.equals("image")) { 15702 this.getImage().remove(castToString(value)); 15703 } else if (name.equals("other")) { 15704 this.getOther().remove(castToString(value)); 15705 } else 15706 super.removeChild(name, value); 15707 15708 } 15709 15710 @Override 15711 public Base makeProperty(int hash, String name) throws FHIRException { 15712 switch (hash) { 15713 case 1839654540: 15714 return getRenderingElement(); 15715 case -341064690: 15716 return addResource(); 15717 case 3433103: 15718 return addPage(); 15719 case 100313435: 15720 return addImageElement(); 15721 case 106069776: 15722 return addOtherElement(); 15723 default: 15724 return super.makeProperty(hash, name); 15725 } 15726 15727 } 15728 15729 @Override 15730 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 15731 switch (hash) { 15732 case 1839654540: 15733 /* rendering */ return new String[] { "url" }; 15734 case -341064690: 15735 /* resource */ return new String[] {}; 15736 case 3433103: 15737 /* page */ return new String[] {}; 15738 case 100313435: 15739 /* image */ return new String[] { "string" }; 15740 case 106069776: 15741 /* other */ return new String[] { "string" }; 15742 default: 15743 return super.getTypesForProperty(hash, name); 15744 } 15745 15746 } 15747 15748 @Override 15749 public Base addChild(String name) throws FHIRException { 15750 if (name.equals("rendering")) { 15751 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.rendering"); 15752 } else if (name.equals("resource")) { 15753 return addResource(); 15754 } else if (name.equals("page")) { 15755 return addPage(); 15756 } else if (name.equals("image")) { 15757 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.image"); 15758 } else if (name.equals("other")) { 15759 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.other"); 15760 } else 15761 return super.addChild(name); 15762 } 15763 15764 public ImplementationGuideManifestComponent copy() { 15765 ImplementationGuideManifestComponent dst = new ImplementationGuideManifestComponent(); 15766 copyValues(dst); 15767 return dst; 15768 } 15769 15770 public void copyValues(ImplementationGuideManifestComponent dst) { 15771 super.copyValues(dst); 15772 dst.rendering = rendering == null ? null : rendering.copy(); 15773 if (resource != null) { 15774 dst.resource = new ArrayList<ManifestResourceComponent>(); 15775 for (ManifestResourceComponent i : resource) 15776 dst.resource.add(i.copy()); 15777 } 15778 ; 15779 if (page != null) { 15780 dst.page = new ArrayList<ManifestPageComponent>(); 15781 for (ManifestPageComponent i : page) 15782 dst.page.add(i.copy()); 15783 } 15784 ; 15785 if (image != null) { 15786 dst.image = new ArrayList<StringType>(); 15787 for (StringType i : image) 15788 dst.image.add(i.copy()); 15789 } 15790 ; 15791 if (other != null) { 15792 dst.other = new ArrayList<StringType>(); 15793 for (StringType i : other) 15794 dst.other.add(i.copy()); 15795 } 15796 ; 15797 } 15798 15799 @Override 15800 public boolean equalsDeep(Base other_) { 15801 if (!super.equalsDeep(other_)) 15802 return false; 15803 if (!(other_ instanceof ImplementationGuideManifestComponent)) 15804 return false; 15805 ImplementationGuideManifestComponent o = (ImplementationGuideManifestComponent) other_; 15806 return compareDeep(rendering, o.rendering, true) && compareDeep(resource, o.resource, true) 15807 && compareDeep(page, o.page, true) && compareDeep(image, o.image, true) && compareDeep(other, o.other, true); 15808 } 15809 15810 @Override 15811 public boolean equalsShallow(Base other_) { 15812 if (!super.equalsShallow(other_)) 15813 return false; 15814 if (!(other_ instanceof ImplementationGuideManifestComponent)) 15815 return false; 15816 ImplementationGuideManifestComponent o = (ImplementationGuideManifestComponent) other_; 15817 return compareValues(rendering, o.rendering, true) && compareValues(image, o.image, true) 15818 && compareValues(other, o.other, true); 15819 } 15820 15821 public boolean isEmpty() { 15822 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rendering, resource, page, image, other); 15823 } 15824 15825 public String fhirType() { 15826 return "ImplementationGuide.manifest"; 15827 15828 } 15829 15830 } 15831 15832 @Block() 15833 public static class ManifestResourceComponent extends BackboneElement implements IBaseBackboneElement { 15834 /** 15835 * Where this resource is found. 15836 */ 15837 @Child(name = "reference", type = { 15838 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 15839 @Description(shortDefinition = "Location of the resource", formalDefinition = "Where this resource is found.") 15840 protected Reference reference; 15841 15842 /** 15843 * The actual object that is the target of the reference (Where this resource is 15844 * found.) 15845 */ 15846 protected Resource referenceTarget; 15847 15848 /** 15849 * If true or a reference, indicates the resource is an example instance. If a 15850 * reference is present, indicates that the example is an example of the 15851 * specified profile. 15852 */ 15853 @Child(name = "example", type = { BooleanType.class, 15854 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 15855 @Description(shortDefinition = "Is an example/What is this an example of?", formalDefinition = "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.") 15856 protected Type example; 15857 15858 /** 15859 * The relative path for primary page for this resource within the IG. 15860 */ 15861 @Child(name = "relativePath", type = { 15862 UrlType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 15863 @Description(shortDefinition = "Relative path for page in IG", formalDefinition = "The relative path for primary page for this resource within the IG.") 15864 protected UrlType relativePath; 15865 15866 private static final long serialVersionUID = 1150095716L; 15867 15868 /** 15869 * Constructor 15870 */ 15871 public ManifestResourceComponent() { 15872 super(); 15873 } 15874 15875 /** 15876 * Constructor 15877 */ 15878 public ManifestResourceComponent(Reference reference) { 15879 super(); 15880 this.reference = reference; 15881 } 15882 15883 /** 15884 * @return {@link #reference} (Where this resource is found.) 15885 */ 15886 public Reference getReference() { 15887 if (this.reference == null) 15888 if (Configuration.errorOnAutoCreate()) 15889 throw new Error("Attempt to auto-create ManifestResourceComponent.reference"); 15890 else if (Configuration.doAutoCreate()) 15891 this.reference = new Reference(); // cc 15892 return this.reference; 15893 } 15894 15895 public boolean hasReference() { 15896 return this.reference != null && !this.reference.isEmpty(); 15897 } 15898 15899 /** 15900 * @param value {@link #reference} (Where this resource is found.) 15901 */ 15902 public ManifestResourceComponent setReference(Reference value) { 15903 this.reference = value; 15904 return this; 15905 } 15906 15907 /** 15908 * @return {@link #reference} The actual object that is the target of the 15909 * reference. The reference library doesn't populate this, but you can 15910 * use it to hold the resource if you resolve it. (Where this resource 15911 * is found.) 15912 */ 15913 public Resource getReferenceTarget() { 15914 return this.referenceTarget; 15915 } 15916 15917 /** 15918 * @param value {@link #reference} The actual object that is the target of the 15919 * reference. The reference library doesn't use these, but you can 15920 * use it to hold the resource if you resolve it. (Where this 15921 * resource is found.) 15922 */ 15923 public ManifestResourceComponent setReferenceTarget(Resource value) { 15924 this.referenceTarget = value; 15925 return this; 15926 } 15927 15928 /** 15929 * @return {@link #example} (If true or a reference, indicates the resource is 15930 * an example instance. If a reference is present, indicates that the 15931 * example is an example of the specified profile.) 15932 */ 15933 public Type getExample() { 15934 return this.example; 15935 } 15936 15937 /** 15938 * @return {@link #example} (If true or a reference, indicates the resource is 15939 * an example instance. If a reference is present, indicates that the 15940 * example is an example of the specified profile.) 15941 */ 15942 public BooleanType getExampleBooleanType() throws FHIRException { 15943 if (this.example == null) 15944 this.example = new BooleanType(); 15945 if (!(this.example instanceof BooleanType)) 15946 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 15947 + this.example.getClass().getName() + " was encountered"); 15948 return (BooleanType) this.example; 15949 } 15950 15951 public boolean hasExampleBooleanType() { 15952 return this.example instanceof BooleanType; 15953 } 15954 15955 /** 15956 * @return {@link #example} (If true or a reference, indicates the resource is 15957 * an example instance. If a reference is present, indicates that the 15958 * example is an example of the specified profile.) 15959 */ 15960 public CanonicalType getExampleCanonicalType() throws FHIRException { 15961 if (this.example == null) 15962 this.example = new CanonicalType(); 15963 if (!(this.example instanceof CanonicalType)) 15964 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 15965 + this.example.getClass().getName() + " was encountered"); 15966 return (CanonicalType) this.example; 15967 } 15968 15969 public boolean hasExampleCanonicalType() { 15970 return this.example instanceof CanonicalType; 15971 } 15972 15973 public boolean hasExample() { 15974 return this.example != null && !this.example.isEmpty(); 15975 } 15976 15977 /** 15978 * @param value {@link #example} (If true or a reference, indicates the resource 15979 * is an example instance. If a reference is present, indicates 15980 * that the example is an example of the specified profile.) 15981 */ 15982 public ManifestResourceComponent setExample(Type value) { 15983 if (value != null && !(value instanceof BooleanType || value instanceof CanonicalType)) 15984 throw new Error("Not the right type for ImplementationGuide.manifest.resource.example[x]: " + value.fhirType()); 15985 this.example = value; 15986 return this; 15987 } 15988 15989 /** 15990 * @return {@link #relativePath} (The relative path for primary page for this 15991 * resource within the IG.). This is the underlying object with id, 15992 * value and extensions. The accessor "getRelativePath" gives direct 15993 * access to the value 15994 */ 15995 public UrlType getRelativePathElement() { 15996 if (this.relativePath == null) 15997 if (Configuration.errorOnAutoCreate()) 15998 throw new Error("Attempt to auto-create ManifestResourceComponent.relativePath"); 15999 else if (Configuration.doAutoCreate()) 16000 this.relativePath = new UrlType(); // bb 16001 return this.relativePath; 16002 } 16003 16004 public boolean hasRelativePathElement() { 16005 return this.relativePath != null && !this.relativePath.isEmpty(); 16006 } 16007 16008 public boolean hasRelativePath() { 16009 return this.relativePath != null && !this.relativePath.isEmpty(); 16010 } 16011 16012 /** 16013 * @param value {@link #relativePath} (The relative path for primary page for 16014 * this resource within the IG.). This is the underlying object 16015 * with id, value and extensions. The accessor "getRelativePath" 16016 * gives direct access to the value 16017 */ 16018 public ManifestResourceComponent setRelativePathElement(UrlType value) { 16019 this.relativePath = value; 16020 return this; 16021 } 16022 16023 /** 16024 * @return The relative path for primary page for this resource within the IG. 16025 */ 16026 public String getRelativePath() { 16027 return this.relativePath == null ? null : this.relativePath.getValue(); 16028 } 16029 16030 /** 16031 * @param value The relative path for primary page for this resource within the 16032 * IG. 16033 */ 16034 public ManifestResourceComponent setRelativePath(String value) { 16035 if (Utilities.noString(value)) 16036 this.relativePath = null; 16037 else { 16038 if (this.relativePath == null) 16039 this.relativePath = new UrlType(); 16040 this.relativePath.setValue(value); 16041 } 16042 return this; 16043 } 16044 16045 protected void listChildren(List<Property> children) { 16046 super.listChildren(children); 16047 children.add(new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, reference)); 16048 children.add(new Property("example[x]", "boolean|canonical(StructureDefinition)", 16049 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 16050 0, 1, example)); 16051 children.add(new Property("relativePath", "url", 16052 "The relative path for primary page for this resource within the IG.", 0, 1, relativePath)); 16053 } 16054 16055 @Override 16056 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 16057 switch (_hash) { 16058 case -925155509: 16059 /* reference */ return new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, 16060 reference); 16061 case -2002328874: 16062 /* example[x] */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 16063 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 16064 0, 1, example); 16065 case -1322970774: 16066 /* example */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 16067 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 16068 0, 1, example); 16069 case 159803230: 16070 /* exampleBoolean */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 16071 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 16072 0, 1, example); 16073 case 2016979626: 16074 /* exampleCanonical */ return new Property("example[x]", "boolean|canonical(StructureDefinition)", 16075 "If true or a reference, indicates the resource is an example instance. If a reference is present, indicates that the example is an example of the specified profile.", 16076 0, 1, example); 16077 case -70808303: 16078 /* relativePath */ return new Property("relativePath", "url", 16079 "The relative path for primary page for this resource within the IG.", 0, 1, relativePath); 16080 default: 16081 return super.getNamedProperty(_hash, _name, _checkValid); 16082 } 16083 16084 } 16085 16086 @Override 16087 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 16088 switch (hash) { 16089 case -925155509: 16090 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 16091 case -1322970774: 16092 /* example */ return this.example == null ? new Base[0] : new Base[] { this.example }; // Type 16093 case -70808303: 16094 /* relativePath */ return this.relativePath == null ? new Base[0] : new Base[] { this.relativePath }; // UrlType 16095 default: 16096 return super.getProperty(hash, name, checkValid); 16097 } 16098 16099 } 16100 16101 @Override 16102 public Base setProperty(int hash, String name, Base value) throws FHIRException { 16103 switch (hash) { 16104 case -925155509: // reference 16105 this.reference = castToReference(value); // Reference 16106 return value; 16107 case -1322970774: // example 16108 this.example = castToType(value); // Type 16109 return value; 16110 case -70808303: // relativePath 16111 this.relativePath = castToUrl(value); // UrlType 16112 return value; 16113 default: 16114 return super.setProperty(hash, name, value); 16115 } 16116 16117 } 16118 16119 @Override 16120 public Base setProperty(String name, Base value) throws FHIRException { 16121 if (name.equals("reference")) { 16122 this.reference = castToReference(value); // Reference 16123 } else if (name.equals("example[x]")) { 16124 this.example = castToType(value); // Type 16125 } else if (name.equals("relativePath")) { 16126 this.relativePath = castToUrl(value); // UrlType 16127 } else 16128 return super.setProperty(name, value); 16129 return value; 16130 } 16131 16132 @Override 16133 public void removeChild(String name, Base value) throws FHIRException { 16134 if (name.equals("reference")) { 16135 this.reference = null; 16136 } else if (name.equals("example[x]")) { 16137 this.example = null; 16138 } else if (name.equals("relativePath")) { 16139 this.relativePath = null; 16140 } else 16141 super.removeChild(name, value); 16142 16143 } 16144 16145 @Override 16146 public Base makeProperty(int hash, String name) throws FHIRException { 16147 switch (hash) { 16148 case -925155509: 16149 return getReference(); 16150 case -2002328874: 16151 return getExample(); 16152 case -1322970774: 16153 return getExample(); 16154 case -70808303: 16155 return getRelativePathElement(); 16156 default: 16157 return super.makeProperty(hash, name); 16158 } 16159 16160 } 16161 16162 @Override 16163 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 16164 switch (hash) { 16165 case -925155509: 16166 /* reference */ return new String[] { "Reference" }; 16167 case -1322970774: 16168 /* example */ return new String[] { "boolean", "canonical" }; 16169 case -70808303: 16170 /* relativePath */ return new String[] { "url" }; 16171 default: 16172 return super.getTypesForProperty(hash, name); 16173 } 16174 16175 } 16176 16177 @Override 16178 public Base addChild(String name) throws FHIRException { 16179 if (name.equals("reference")) { 16180 this.reference = new Reference(); 16181 return this.reference; 16182 } else if (name.equals("exampleBoolean")) { 16183 this.example = new BooleanType(); 16184 return this.example; 16185 } else if (name.equals("exampleCanonical")) { 16186 this.example = new CanonicalType(); 16187 return this.example; 16188 } else if (name.equals("relativePath")) { 16189 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.relativePath"); 16190 } else 16191 return super.addChild(name); 16192 } 16193 16194 public ManifestResourceComponent copy() { 16195 ManifestResourceComponent dst = new ManifestResourceComponent(); 16196 copyValues(dst); 16197 return dst; 16198 } 16199 16200 public void copyValues(ManifestResourceComponent dst) { 16201 super.copyValues(dst); 16202 dst.reference = reference == null ? null : reference.copy(); 16203 dst.example = example == null ? null : example.copy(); 16204 dst.relativePath = relativePath == null ? null : relativePath.copy(); 16205 } 16206 16207 @Override 16208 public boolean equalsDeep(Base other_) { 16209 if (!super.equalsDeep(other_)) 16210 return false; 16211 if (!(other_ instanceof ManifestResourceComponent)) 16212 return false; 16213 ManifestResourceComponent o = (ManifestResourceComponent) other_; 16214 return compareDeep(reference, o.reference, true) && compareDeep(example, o.example, true) 16215 && compareDeep(relativePath, o.relativePath, true); 16216 } 16217 16218 @Override 16219 public boolean equalsShallow(Base other_) { 16220 if (!super.equalsShallow(other_)) 16221 return false; 16222 if (!(other_ instanceof ManifestResourceComponent)) 16223 return false; 16224 ManifestResourceComponent o = (ManifestResourceComponent) other_; 16225 return compareValues(relativePath, o.relativePath, true); 16226 } 16227 16228 public boolean isEmpty() { 16229 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, example, relativePath); 16230 } 16231 16232 public String fhirType() { 16233 return "ImplementationGuide.manifest.resource"; 16234 16235 } 16236 16237 } 16238 16239 @Block() 16240 public static class ManifestPageComponent extends BackboneElement implements IBaseBackboneElement { 16241 /** 16242 * Relative path to the page. 16243 */ 16244 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 16245 @Description(shortDefinition = "HTML page name", formalDefinition = "Relative path to the page.") 16246 protected StringType name; 16247 16248 /** 16249 * Label for the page intended for human display. 16250 */ 16251 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 16252 @Description(shortDefinition = "Title of the page, for references", formalDefinition = "Label for the page intended for human display.") 16253 protected StringType title; 16254 16255 /** 16256 * The name of an anchor available on the page. 16257 */ 16258 @Child(name = "anchor", type = { 16259 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 16260 @Description(shortDefinition = "Anchor available on the page", formalDefinition = "The name of an anchor available on the page.") 16261 protected List<StringType> anchor; 16262 16263 private static final long serialVersionUID = 1920576611L; 16264 16265 /** 16266 * Constructor 16267 */ 16268 public ManifestPageComponent() { 16269 super(); 16270 } 16271 16272 /** 16273 * Constructor 16274 */ 16275 public ManifestPageComponent(StringType name) { 16276 super(); 16277 this.name = name; 16278 } 16279 16280 /** 16281 * @return {@link #name} (Relative path to the page.). This is the underlying 16282 * object with id, value and extensions. The accessor "getName" gives 16283 * direct access to the value 16284 */ 16285 public StringType getNameElement() { 16286 if (this.name == null) 16287 if (Configuration.errorOnAutoCreate()) 16288 throw new Error("Attempt to auto-create ManifestPageComponent.name"); 16289 else if (Configuration.doAutoCreate()) 16290 this.name = new StringType(); // bb 16291 return this.name; 16292 } 16293 16294 public boolean hasNameElement() { 16295 return this.name != null && !this.name.isEmpty(); 16296 } 16297 16298 public boolean hasName() { 16299 return this.name != null && !this.name.isEmpty(); 16300 } 16301 16302 /** 16303 * @param value {@link #name} (Relative path to the page.). This is the 16304 * underlying object with id, value and extensions. The accessor 16305 * "getName" gives direct access to the value 16306 */ 16307 public ManifestPageComponent setNameElement(StringType value) { 16308 this.name = value; 16309 return this; 16310 } 16311 16312 /** 16313 * @return Relative path to the page. 16314 */ 16315 public String getName() { 16316 return this.name == null ? null : this.name.getValue(); 16317 } 16318 16319 /** 16320 * @param value Relative path to the page. 16321 */ 16322 public ManifestPageComponent setName(String value) { 16323 if (this.name == null) 16324 this.name = new StringType(); 16325 this.name.setValue(value); 16326 return this; 16327 } 16328 16329 /** 16330 * @return {@link #title} (Label for the page intended for human display.). This 16331 * is the underlying object with id, value and extensions. The accessor 16332 * "getTitle" gives direct access to the value 16333 */ 16334 public StringType getTitleElement() { 16335 if (this.title == null) 16336 if (Configuration.errorOnAutoCreate()) 16337 throw new Error("Attempt to auto-create ManifestPageComponent.title"); 16338 else if (Configuration.doAutoCreate()) 16339 this.title = new StringType(); // bb 16340 return this.title; 16341 } 16342 16343 public boolean hasTitleElement() { 16344 return this.title != null && !this.title.isEmpty(); 16345 } 16346 16347 public boolean hasTitle() { 16348 return this.title != null && !this.title.isEmpty(); 16349 } 16350 16351 /** 16352 * @param value {@link #title} (Label for the page intended for human display.). 16353 * This is the underlying object with id, value and extensions. The 16354 * accessor "getTitle" gives direct access to the value 16355 */ 16356 public ManifestPageComponent setTitleElement(StringType value) { 16357 this.title = value; 16358 return this; 16359 } 16360 16361 /** 16362 * @return Label for the page intended for human display. 16363 */ 16364 public String getTitle() { 16365 return this.title == null ? null : this.title.getValue(); 16366 } 16367 16368 /** 16369 * @param value Label for the page intended for human display. 16370 */ 16371 public ManifestPageComponent setTitle(String value) { 16372 if (Utilities.noString(value)) 16373 this.title = null; 16374 else { 16375 if (this.title == null) 16376 this.title = new StringType(); 16377 this.title.setValue(value); 16378 } 16379 return this; 16380 } 16381 16382 /** 16383 * @return {@link #anchor} (The name of an anchor available on the page.) 16384 */ 16385 public List<StringType> getAnchor() { 16386 if (this.anchor == null) 16387 this.anchor = new ArrayList<StringType>(); 16388 return this.anchor; 16389 } 16390 16391 /** 16392 * @return Returns a reference to <code>this</code> for easy method chaining 16393 */ 16394 public ManifestPageComponent setAnchor(List<StringType> theAnchor) { 16395 this.anchor = theAnchor; 16396 return this; 16397 } 16398 16399 public boolean hasAnchor() { 16400 if (this.anchor == null) 16401 return false; 16402 for (StringType item : this.anchor) 16403 if (!item.isEmpty()) 16404 return true; 16405 return false; 16406 } 16407 16408 /** 16409 * @return {@link #anchor} (The name of an anchor available on the page.) 16410 */ 16411 public StringType addAnchorElement() {// 2 16412 StringType t = new StringType(); 16413 if (this.anchor == null) 16414 this.anchor = new ArrayList<StringType>(); 16415 this.anchor.add(t); 16416 return t; 16417 } 16418 16419 /** 16420 * @param value {@link #anchor} (The name of an anchor available on the page.) 16421 */ 16422 public ManifestPageComponent addAnchor(String value) { // 1 16423 StringType t = new StringType(); 16424 t.setValue(value); 16425 if (this.anchor == null) 16426 this.anchor = new ArrayList<StringType>(); 16427 this.anchor.add(t); 16428 return this; 16429 } 16430 16431 /** 16432 * @param value {@link #anchor} (The name of an anchor available on the page.) 16433 */ 16434 public boolean hasAnchor(String value) { 16435 if (this.anchor == null) 16436 return false; 16437 for (StringType v : this.anchor) 16438 if (v.getValue().equals(value)) // string 16439 return true; 16440 return false; 16441 } 16442 16443 protected void listChildren(List<Property> children) { 16444 super.listChildren(children); 16445 children.add(new Property("name", "string", "Relative path to the page.", 0, 1, name)); 16446 children.add(new Property("title", "string", "Label for the page intended for human display.", 0, 1, title)); 16447 children.add(new Property("anchor", "string", "The name of an anchor available on the page.", 0, 16448 java.lang.Integer.MAX_VALUE, anchor)); 16449 } 16450 16451 @Override 16452 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 16453 switch (_hash) { 16454 case 3373707: 16455 /* name */ return new Property("name", "string", "Relative path to the page.", 0, 1, name); 16456 case 110371416: 16457 /* title */ return new Property("title", "string", "Label for the page intended for human display.", 0, 1, 16458 title); 16459 case -1413299531: 16460 /* anchor */ return new Property("anchor", "string", "The name of an anchor available on the page.", 0, 16461 java.lang.Integer.MAX_VALUE, anchor); 16462 default: 16463 return super.getNamedProperty(_hash, _name, _checkValid); 16464 } 16465 16466 } 16467 16468 @Override 16469 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 16470 switch (hash) { 16471 case 3373707: 16472 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 16473 case 110371416: 16474 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 16475 case -1413299531: 16476 /* anchor */ return this.anchor == null ? new Base[0] : this.anchor.toArray(new Base[this.anchor.size()]); // StringType 16477 default: 16478 return super.getProperty(hash, name, checkValid); 16479 } 16480 16481 } 16482 16483 @Override 16484 public Base setProperty(int hash, String name, Base value) throws FHIRException { 16485 switch (hash) { 16486 case 3373707: // name 16487 this.name = castToString(value); // StringType 16488 return value; 16489 case 110371416: // title 16490 this.title = castToString(value); // StringType 16491 return value; 16492 case -1413299531: // anchor 16493 this.getAnchor().add(castToString(value)); // StringType 16494 return value; 16495 default: 16496 return super.setProperty(hash, name, value); 16497 } 16498 16499 } 16500 16501 @Override 16502 public Base setProperty(String name, Base value) throws FHIRException { 16503 if (name.equals("name")) { 16504 this.name = castToString(value); // StringType 16505 } else if (name.equals("title")) { 16506 this.title = castToString(value); // StringType 16507 } else if (name.equals("anchor")) { 16508 this.getAnchor().add(castToString(value)); 16509 } else 16510 return super.setProperty(name, value); 16511 return value; 16512 } 16513 16514 @Override 16515 public void removeChild(String name, Base value) throws FHIRException { 16516 if (name.equals("name")) { 16517 this.name = null; 16518 } else if (name.equals("title")) { 16519 this.title = null; 16520 } else if (name.equals("anchor")) { 16521 this.getAnchor().remove(castToString(value)); 16522 } else 16523 super.removeChild(name, value); 16524 16525 } 16526 16527 @Override 16528 public Base makeProperty(int hash, String name) throws FHIRException { 16529 switch (hash) { 16530 case 3373707: 16531 return getNameElement(); 16532 case 110371416: 16533 return getTitleElement(); 16534 case -1413299531: 16535 return addAnchorElement(); 16536 default: 16537 return super.makeProperty(hash, name); 16538 } 16539 16540 } 16541 16542 @Override 16543 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 16544 switch (hash) { 16545 case 3373707: 16546 /* name */ return new String[] { "string" }; 16547 case 110371416: 16548 /* title */ return new String[] { "string" }; 16549 case -1413299531: 16550 /* anchor */ return new String[] { "string" }; 16551 default: 16552 return super.getTypesForProperty(hash, name); 16553 } 16554 16555 } 16556 16557 @Override 16558 public Base addChild(String name) throws FHIRException { 16559 if (name.equals("name")) { 16560 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 16561 } else if (name.equals("title")) { 16562 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.title"); 16563 } else if (name.equals("anchor")) { 16564 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.anchor"); 16565 } else 16566 return super.addChild(name); 16567 } 16568 16569 public ManifestPageComponent copy() { 16570 ManifestPageComponent dst = new ManifestPageComponent(); 16571 copyValues(dst); 16572 return dst; 16573 } 16574 16575 public void copyValues(ManifestPageComponent dst) { 16576 super.copyValues(dst); 16577 dst.name = name == null ? null : name.copy(); 16578 dst.title = title == null ? null : title.copy(); 16579 if (anchor != null) { 16580 dst.anchor = new ArrayList<StringType>(); 16581 for (StringType i : anchor) 16582 dst.anchor.add(i.copy()); 16583 } 16584 ; 16585 } 16586 16587 @Override 16588 public boolean equalsDeep(Base other_) { 16589 if (!super.equalsDeep(other_)) 16590 return false; 16591 if (!(other_ instanceof ManifestPageComponent)) 16592 return false; 16593 ManifestPageComponent o = (ManifestPageComponent) other_; 16594 return compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 16595 && compareDeep(anchor, o.anchor, true); 16596 } 16597 16598 @Override 16599 public boolean equalsShallow(Base other_) { 16600 if (!super.equalsShallow(other_)) 16601 return false; 16602 if (!(other_ instanceof ManifestPageComponent)) 16603 return false; 16604 ManifestPageComponent o = (ManifestPageComponent) other_; 16605 return compareValues(name, o.name, true) && compareValues(title, o.title, true) 16606 && compareValues(anchor, o.anchor, true); 16607 } 16608 16609 public boolean isEmpty() { 16610 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, title, anchor); 16611 } 16612 16613 public String fhirType() { 16614 return "ImplementationGuide.manifest.page"; 16615 16616 } 16617 16618 } 16619 16620 /** 16621 * A copyright statement relating to the implementation guide and/or its 16622 * contents. Copyright statements are generally legal restrictions on the use 16623 * and publishing of the implementation guide. 16624 */ 16625 @Child(name = "copyright", type = { 16626 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 16627 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.") 16628 protected MarkdownType copyright; 16629 16630 /** 16631 * The NPM package name for this Implementation Guide, used in the NPM package 16632 * distribution, which is the primary mechanism by which FHIR based tooling 16633 * manages IG dependencies. This value must be globally unique, and should be 16634 * assigned with care. 16635 */ 16636 @Child(name = "packageId", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 16637 @Description(shortDefinition = "NPM Package name for IG", formalDefinition = "The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.") 16638 protected IdType packageId; 16639 16640 /** 16641 * The license that applies to this Implementation Guide, using an SPDX license 16642 * code, or 'not-open-source'. 16643 */ 16644 @Child(name = "license", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 16645 @Description(shortDefinition = "SPDX license code for this IG (or not-open-source)", formalDefinition = "The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.") 16646 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/spdx-license") 16647 protected Enumeration<SPDXLicense> license; 16648 16649 /** 16650 * The version(s) of the FHIR specification that this ImplementationGuide 16651 * targets - e.g. describes how to use. The value of this element is the formal 16652 * version of the specification, without the revision number, e.g. 16653 * [publication].[major].[minor], which is 4.0.1. for this version. 16654 */ 16655 @Child(name = "fhirVersion", type = { 16656 CodeType.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 16657 @Description(shortDefinition = "FHIR Version(s) this Implementation Guide targets", formalDefinition = "The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.0.1. for this version.") 16658 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/FHIR-version") 16659 protected List<Enumeration<FHIRVersion>> fhirVersion; 16660 16661 /** 16662 * Another implementation guide that this implementation depends on. Typically, 16663 * an implementation guide uses value sets, profiles etc.defined in other 16664 * implementation guides. 16665 */ 16666 @Child(name = "dependsOn", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 16667 @Description(shortDefinition = "Another Implementation guide this depends on", formalDefinition = "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.") 16668 protected List<ImplementationGuideDependsOnComponent> dependsOn; 16669 16670 /** 16671 * A set of profiles that all resources covered by this implementation guide 16672 * must conform to. 16673 */ 16674 @Child(name = "global", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 16675 @Description(shortDefinition = "Profiles that apply globally", formalDefinition = "A set of profiles that all resources covered by this implementation guide must conform to.") 16676 protected List<ImplementationGuideGlobalComponent> global; 16677 16678 /** 16679 * The information needed by an IG publisher tool to publish the whole 16680 * implementation guide. 16681 */ 16682 @Child(name = "definition", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = false) 16683 @Description(shortDefinition = "Information needed to build the IG", formalDefinition = "The information needed by an IG publisher tool to publish the whole implementation guide.") 16684 protected ImplementationGuideDefinitionComponent definition; 16685 16686 /** 16687 * Information about an assembled implementation guide, created by the 16688 * publication tooling. 16689 */ 16690 @Child(name = "manifest", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 16691 @Description(shortDefinition = "Information about an assembled IG", formalDefinition = "Information about an assembled implementation guide, created by the publication tooling.") 16692 protected ImplementationGuideManifestComponent manifest; 16693 16694 private static final long serialVersionUID = 415193005L; 16695 16696 /** 16697 * Constructor 16698 */ 16699 public ImplementationGuide() { 16700 super(); 16701 } 16702 16703 /** 16704 * Constructor 16705 */ 16706 public ImplementationGuide(UriType url, StringType name, Enumeration<PublicationStatus> status, IdType packageId) { 16707 super(); 16708 this.url = url; 16709 this.name = name; 16710 this.status = status; 16711 this.packageId = packageId; 16712 } 16713 16714 /** 16715 * @return {@link #url} (An absolute URI that is used to identify this 16716 * implementation guide when it is referenced in a specification, model, 16717 * design or an instance; also called its canonical identifier. This 16718 * SHOULD be globally unique and SHOULD be a literal address at which at 16719 * which an authoritative instance of this implementation guide is (or 16720 * will be) published. This URL can be the target of a canonical 16721 * reference. It SHALL remain the same when the implementation guide is 16722 * stored on different servers.). This is the underlying object with id, 16723 * value and extensions. The accessor "getUrl" gives direct access to 16724 * the value 16725 */ 16726 public UriType getUrlElement() { 16727 if (this.url == null) 16728 if (Configuration.errorOnAutoCreate()) 16729 throw new Error("Attempt to auto-create ImplementationGuide.url"); 16730 else if (Configuration.doAutoCreate()) 16731 this.url = new UriType(); // bb 16732 return this.url; 16733 } 16734 16735 public boolean hasUrlElement() { 16736 return this.url != null && !this.url.isEmpty(); 16737 } 16738 16739 public boolean hasUrl() { 16740 return this.url != null && !this.url.isEmpty(); 16741 } 16742 16743 /** 16744 * @param value {@link #url} (An absolute URI that is used to identify this 16745 * implementation guide when it is referenced in a specification, 16746 * model, design or an instance; also called its canonical 16747 * identifier. This SHOULD be globally unique and SHOULD be a 16748 * literal address at which at which an authoritative instance of 16749 * this implementation guide is (or will be) published. This URL 16750 * can be the target of a canonical reference. It SHALL remain the 16751 * same when the implementation guide is stored on different 16752 * servers.). This is the underlying object with id, value and 16753 * extensions. The accessor "getUrl" gives direct access to the 16754 * value 16755 */ 16756 public ImplementationGuide setUrlElement(UriType value) { 16757 this.url = value; 16758 return this; 16759 } 16760 16761 /** 16762 * @return An absolute URI that is used to identify this implementation guide 16763 * when it is referenced in a specification, model, design or an 16764 * instance; also called its canonical identifier. This SHOULD be 16765 * globally unique and SHOULD be a literal address at which at which an 16766 * authoritative instance of this implementation guide is (or will be) 16767 * published. This URL can be the target of a canonical reference. It 16768 * SHALL remain the same when the implementation guide is stored on 16769 * different servers. 16770 */ 16771 public String getUrl() { 16772 return this.url == null ? null : this.url.getValue(); 16773 } 16774 16775 /** 16776 * @param value An absolute URI that is used to identify this implementation 16777 * guide when it is referenced in a specification, model, design or 16778 * an instance; also called its canonical identifier. This SHOULD 16779 * be globally unique and SHOULD be a literal address at which at 16780 * which an authoritative instance of this implementation guide is 16781 * (or will be) published. This URL can be the target of a 16782 * canonical reference. It SHALL remain the same when the 16783 * implementation guide is stored on different servers. 16784 */ 16785 public ImplementationGuide setUrl(String value) { 16786 if (this.url == null) 16787 this.url = new UriType(); 16788 this.url.setValue(value); 16789 return this; 16790 } 16791 16792 /** 16793 * @return {@link #version} (The identifier that is used to identify this 16794 * version of the implementation guide when it is referenced in a 16795 * specification, model, design or instance. This is an arbitrary value 16796 * managed by the implementation guide author and is not expected to be 16797 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 16798 * if a managed version is not available. There is also no expectation 16799 * that versions can be placed in a lexicographical sequence.). This is 16800 * the underlying object with id, value and extensions. The accessor 16801 * "getVersion" gives direct access to the value 16802 */ 16803 public StringType getVersionElement() { 16804 if (this.version == null) 16805 if (Configuration.errorOnAutoCreate()) 16806 throw new Error("Attempt to auto-create ImplementationGuide.version"); 16807 else if (Configuration.doAutoCreate()) 16808 this.version = new StringType(); // bb 16809 return this.version; 16810 } 16811 16812 public boolean hasVersionElement() { 16813 return this.version != null && !this.version.isEmpty(); 16814 } 16815 16816 public boolean hasVersion() { 16817 return this.version != null && !this.version.isEmpty(); 16818 } 16819 16820 /** 16821 * @param value {@link #version} (The identifier that is used to identify this 16822 * version of the implementation guide when it is referenced in a 16823 * specification, model, design or instance. This is an arbitrary 16824 * value managed by the implementation guide author and is not 16825 * expected to be globally unique. For example, it might be a 16826 * timestamp (e.g. yyyymmdd) if a managed version is not available. 16827 * There is also no expectation that versions can be placed in a 16828 * lexicographical sequence.). This is the underlying object with 16829 * id, value and extensions. The accessor "getVersion" gives direct 16830 * access to the value 16831 */ 16832 public ImplementationGuide setVersionElement(StringType value) { 16833 this.version = value; 16834 return this; 16835 } 16836 16837 /** 16838 * @return The identifier that is used to identify this version of the 16839 * implementation guide when it is referenced in a specification, model, 16840 * design or instance. This is an arbitrary value managed by the 16841 * implementation guide author and is not expected to be globally 16842 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 16843 * managed version is not available. There is also no expectation that 16844 * versions can be placed in a lexicographical sequence. 16845 */ 16846 public String getVersion() { 16847 return this.version == null ? null : this.version.getValue(); 16848 } 16849 16850 /** 16851 * @param value The identifier that is used to identify this version of the 16852 * implementation guide when it is referenced in a specification, 16853 * model, design or instance. This is an arbitrary value managed by 16854 * the implementation guide author and is not expected to be 16855 * globally unique. For example, it might be a timestamp (e.g. 16856 * yyyymmdd) if a managed version is not available. There is also 16857 * no expectation that versions can be placed in a lexicographical 16858 * sequence. 16859 */ 16860 public ImplementationGuide setVersion(String value) { 16861 if (Utilities.noString(value)) 16862 this.version = null; 16863 else { 16864 if (this.version == null) 16865 this.version = new StringType(); 16866 this.version.setValue(value); 16867 } 16868 return this; 16869 } 16870 16871 /** 16872 * @return {@link #name} (A natural language name identifying the implementation 16873 * guide. This name should be usable as an identifier for the module by 16874 * machine processing applications such as code generation.). This is 16875 * the underlying object with id, value and extensions. The accessor 16876 * "getName" gives direct access to the value 16877 */ 16878 public StringType getNameElement() { 16879 if (this.name == null) 16880 if (Configuration.errorOnAutoCreate()) 16881 throw new Error("Attempt to auto-create ImplementationGuide.name"); 16882 else if (Configuration.doAutoCreate()) 16883 this.name = new StringType(); // bb 16884 return this.name; 16885 } 16886 16887 public boolean hasNameElement() { 16888 return this.name != null && !this.name.isEmpty(); 16889 } 16890 16891 public boolean hasName() { 16892 return this.name != null && !this.name.isEmpty(); 16893 } 16894 16895 /** 16896 * @param value {@link #name} (A natural language name identifying the 16897 * implementation guide. This name should be usable as an 16898 * identifier for the module by machine processing applications 16899 * such as code generation.). This is the underlying object with 16900 * id, value and extensions. The accessor "getName" gives direct 16901 * access to the value 16902 */ 16903 public ImplementationGuide setNameElement(StringType value) { 16904 this.name = value; 16905 return this; 16906 } 16907 16908 /** 16909 * @return A natural language name identifying the implementation guide. This 16910 * name should be usable as an identifier for the module by machine 16911 * processing applications such as code generation. 16912 */ 16913 public String getName() { 16914 return this.name == null ? null : this.name.getValue(); 16915 } 16916 16917 /** 16918 * @param value A natural language name identifying the implementation guide. 16919 * This name should be usable as an identifier for the module by 16920 * machine processing applications such as code generation. 16921 */ 16922 public ImplementationGuide setName(String value) { 16923 if (this.name == null) 16924 this.name = new StringType(); 16925 this.name.setValue(value); 16926 return this; 16927 } 16928 16929 /** 16930 * @return {@link #title} (A short, descriptive, user-friendly title for the 16931 * implementation guide.). This is the underlying object with id, value 16932 * and extensions. The accessor "getTitle" gives direct access to the 16933 * value 16934 */ 16935 public StringType getTitleElement() { 16936 if (this.title == null) 16937 if (Configuration.errorOnAutoCreate()) 16938 throw new Error("Attempt to auto-create ImplementationGuide.title"); 16939 else if (Configuration.doAutoCreate()) 16940 this.title = new StringType(); // bb 16941 return this.title; 16942 } 16943 16944 public boolean hasTitleElement() { 16945 return this.title != null && !this.title.isEmpty(); 16946 } 16947 16948 public boolean hasTitle() { 16949 return this.title != null && !this.title.isEmpty(); 16950 } 16951 16952 /** 16953 * @param value {@link #title} (A short, descriptive, user-friendly title for 16954 * the implementation guide.). This is the underlying object with 16955 * id, value and extensions. The accessor "getTitle" gives direct 16956 * access to the value 16957 */ 16958 public ImplementationGuide setTitleElement(StringType value) { 16959 this.title = value; 16960 return this; 16961 } 16962 16963 /** 16964 * @return A short, descriptive, user-friendly title for the implementation 16965 * guide. 16966 */ 16967 public String getTitle() { 16968 return this.title == null ? null : this.title.getValue(); 16969 } 16970 16971 /** 16972 * @param value A short, descriptive, user-friendly title for the implementation 16973 * guide. 16974 */ 16975 public ImplementationGuide setTitle(String value) { 16976 if (Utilities.noString(value)) 16977 this.title = null; 16978 else { 16979 if (this.title == null) 16980 this.title = new StringType(); 16981 this.title.setValue(value); 16982 } 16983 return this; 16984 } 16985 16986 /** 16987 * @return {@link #status} (The status of this implementation guide. Enables 16988 * tracking the life-cycle of the content.). This is the underlying 16989 * object with id, value and extensions. The accessor "getStatus" gives 16990 * direct access to the value 16991 */ 16992 public Enumeration<PublicationStatus> getStatusElement() { 16993 if (this.status == null) 16994 if (Configuration.errorOnAutoCreate()) 16995 throw new Error("Attempt to auto-create ImplementationGuide.status"); 16996 else if (Configuration.doAutoCreate()) 16997 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 16998 return this.status; 16999 } 17000 17001 public boolean hasStatusElement() { 17002 return this.status != null && !this.status.isEmpty(); 17003 } 17004 17005 public boolean hasStatus() { 17006 return this.status != null && !this.status.isEmpty(); 17007 } 17008 17009 /** 17010 * @param value {@link #status} (The status of this implementation guide. 17011 * Enables tracking the life-cycle of the content.). This is the 17012 * underlying object with id, value and extensions. The accessor 17013 * "getStatus" gives direct access to the value 17014 */ 17015 public ImplementationGuide setStatusElement(Enumeration<PublicationStatus> value) { 17016 this.status = value; 17017 return this; 17018 } 17019 17020 /** 17021 * @return The status of this implementation guide. Enables tracking the 17022 * life-cycle of the content. 17023 */ 17024 public PublicationStatus getStatus() { 17025 return this.status == null ? null : this.status.getValue(); 17026 } 17027 17028 /** 17029 * @param value The status of this implementation guide. Enables tracking the 17030 * life-cycle of the content. 17031 */ 17032 public ImplementationGuide setStatus(PublicationStatus value) { 17033 if (this.status == null) 17034 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 17035 this.status.setValue(value); 17036 return this; 17037 } 17038 17039 /** 17040 * @return {@link #experimental} (A Boolean value to indicate that this 17041 * implementation guide is authored for testing purposes (or 17042 * education/evaluation/marketing) and is not intended to be used for 17043 * genuine usage.). This is the underlying object with id, value and 17044 * extensions. The accessor "getExperimental" gives direct access to the 17045 * value 17046 */ 17047 public BooleanType getExperimentalElement() { 17048 if (this.experimental == null) 17049 if (Configuration.errorOnAutoCreate()) 17050 throw new Error("Attempt to auto-create ImplementationGuide.experimental"); 17051 else if (Configuration.doAutoCreate()) 17052 this.experimental = new BooleanType(); // bb 17053 return this.experimental; 17054 } 17055 17056 public boolean hasExperimentalElement() { 17057 return this.experimental != null && !this.experimental.isEmpty(); 17058 } 17059 17060 public boolean hasExperimental() { 17061 return this.experimental != null && !this.experimental.isEmpty(); 17062 } 17063 17064 /** 17065 * @param value {@link #experimental} (A Boolean value to indicate that this 17066 * implementation guide is authored for testing purposes (or 17067 * education/evaluation/marketing) and is not intended to be used 17068 * for genuine usage.). This is the underlying object with id, 17069 * value and extensions. The accessor "getExperimental" gives 17070 * direct access to the value 17071 */ 17072 public ImplementationGuide setExperimentalElement(BooleanType value) { 17073 this.experimental = value; 17074 return this; 17075 } 17076 17077 /** 17078 * @return A Boolean value to indicate that this implementation guide is 17079 * authored for testing purposes (or education/evaluation/marketing) and 17080 * is not intended to be used for genuine usage. 17081 */ 17082 public boolean getExperimental() { 17083 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 17084 } 17085 17086 /** 17087 * @param value A Boolean value to indicate that this implementation guide is 17088 * authored for testing purposes (or 17089 * education/evaluation/marketing) and is not intended to be used 17090 * for genuine usage. 17091 */ 17092 public ImplementationGuide setExperimental(boolean value) { 17093 if (this.experimental == null) 17094 this.experimental = new BooleanType(); 17095 this.experimental.setValue(value); 17096 return this; 17097 } 17098 17099 /** 17100 * @return {@link #date} (The date (and optionally time) when the implementation 17101 * guide was published. The date must change when the business version 17102 * changes and it must change if the status code changes. In addition, 17103 * it should change when the substantive content of the implementation 17104 * guide changes.). This is the underlying object with id, value and 17105 * extensions. The accessor "getDate" gives direct access to the value 17106 */ 17107 public DateTimeType getDateElement() { 17108 if (this.date == null) 17109 if (Configuration.errorOnAutoCreate()) 17110 throw new Error("Attempt to auto-create ImplementationGuide.date"); 17111 else if (Configuration.doAutoCreate()) 17112 this.date = new DateTimeType(); // bb 17113 return this.date; 17114 } 17115 17116 public boolean hasDateElement() { 17117 return this.date != null && !this.date.isEmpty(); 17118 } 17119 17120 public boolean hasDate() { 17121 return this.date != null && !this.date.isEmpty(); 17122 } 17123 17124 /** 17125 * @param value {@link #date} (The date (and optionally time) when the 17126 * implementation guide was published. The date must change when 17127 * the business version changes and it must change if the status 17128 * code changes. In addition, it should change when the substantive 17129 * content of the implementation guide changes.). This is the 17130 * underlying object with id, value and extensions. The accessor 17131 * "getDate" gives direct access to the value 17132 */ 17133 public ImplementationGuide setDateElement(DateTimeType value) { 17134 this.date = value; 17135 return this; 17136 } 17137 17138 /** 17139 * @return The date (and optionally time) when the implementation guide was 17140 * published. The date must change when the business version changes and 17141 * it must change if the status code changes. In addition, it should 17142 * change when the substantive content of the implementation guide 17143 * changes. 17144 */ 17145 public Date getDate() { 17146 return this.date == null ? null : this.date.getValue(); 17147 } 17148 17149 /** 17150 * @param value The date (and optionally time) when the implementation guide was 17151 * published. The date must change when the business version 17152 * changes and it must change if the status code changes. In 17153 * addition, it should change when the substantive content of the 17154 * implementation guide changes. 17155 */ 17156 public ImplementationGuide setDate(Date value) { 17157 if (value == null) 17158 this.date = null; 17159 else { 17160 if (this.date == null) 17161 this.date = new DateTimeType(); 17162 this.date.setValue(value); 17163 } 17164 return this; 17165 } 17166 17167 /** 17168 * @return {@link #publisher} (The name of the organization or individual that 17169 * published the implementation guide.). This is the underlying object 17170 * with id, value and extensions. The accessor "getPublisher" gives 17171 * direct access to the value 17172 */ 17173 public StringType getPublisherElement() { 17174 if (this.publisher == null) 17175 if (Configuration.errorOnAutoCreate()) 17176 throw new Error("Attempt to auto-create ImplementationGuide.publisher"); 17177 else if (Configuration.doAutoCreate()) 17178 this.publisher = new StringType(); // bb 17179 return this.publisher; 17180 } 17181 17182 public boolean hasPublisherElement() { 17183 return this.publisher != null && !this.publisher.isEmpty(); 17184 } 17185 17186 public boolean hasPublisher() { 17187 return this.publisher != null && !this.publisher.isEmpty(); 17188 } 17189 17190 /** 17191 * @param value {@link #publisher} (The name of the organization or individual 17192 * that published the implementation guide.). This is the 17193 * underlying object with id, value and extensions. The accessor 17194 * "getPublisher" gives direct access to the value 17195 */ 17196 public ImplementationGuide setPublisherElement(StringType value) { 17197 this.publisher = value; 17198 return this; 17199 } 17200 17201 /** 17202 * @return The name of the organization or individual that published the 17203 * implementation guide. 17204 */ 17205 public String getPublisher() { 17206 return this.publisher == null ? null : this.publisher.getValue(); 17207 } 17208 17209 /** 17210 * @param value The name of the organization or individual that published the 17211 * implementation guide. 17212 */ 17213 public ImplementationGuide setPublisher(String value) { 17214 if (Utilities.noString(value)) 17215 this.publisher = null; 17216 else { 17217 if (this.publisher == null) 17218 this.publisher = new StringType(); 17219 this.publisher.setValue(value); 17220 } 17221 return this; 17222 } 17223 17224 /** 17225 * @return {@link #contact} (Contact details to assist a user in finding and 17226 * communicating with the publisher.) 17227 */ 17228 public List<ContactDetail> getContact() { 17229 if (this.contact == null) 17230 this.contact = new ArrayList<ContactDetail>(); 17231 return this.contact; 17232 } 17233 17234 /** 17235 * @return Returns a reference to <code>this</code> for easy method chaining 17236 */ 17237 public ImplementationGuide setContact(List<ContactDetail> theContact) { 17238 this.contact = theContact; 17239 return this; 17240 } 17241 17242 public boolean hasContact() { 17243 if (this.contact == null) 17244 return false; 17245 for (ContactDetail item : this.contact) 17246 if (!item.isEmpty()) 17247 return true; 17248 return false; 17249 } 17250 17251 public ContactDetail addContact() { // 3 17252 ContactDetail t = new ContactDetail(); 17253 if (this.contact == null) 17254 this.contact = new ArrayList<ContactDetail>(); 17255 this.contact.add(t); 17256 return t; 17257 } 17258 17259 public ImplementationGuide addContact(ContactDetail t) { // 3 17260 if (t == null) 17261 return this; 17262 if (this.contact == null) 17263 this.contact = new ArrayList<ContactDetail>(); 17264 this.contact.add(t); 17265 return this; 17266 } 17267 17268 /** 17269 * @return The first repetition of repeating field {@link #contact}, creating it 17270 * if it does not already exist 17271 */ 17272 public ContactDetail getContactFirstRep() { 17273 if (getContact().isEmpty()) { 17274 addContact(); 17275 } 17276 return getContact().get(0); 17277 } 17278 17279 /** 17280 * @return {@link #description} (A free text natural language description of the 17281 * implementation guide from a consumer's perspective.). This is the 17282 * underlying object with id, value and extensions. The accessor 17283 * "getDescription" gives direct access to the value 17284 */ 17285 public MarkdownType getDescriptionElement() { 17286 if (this.description == null) 17287 if (Configuration.errorOnAutoCreate()) 17288 throw new Error("Attempt to auto-create ImplementationGuide.description"); 17289 else if (Configuration.doAutoCreate()) 17290 this.description = new MarkdownType(); // bb 17291 return this.description; 17292 } 17293 17294 public boolean hasDescriptionElement() { 17295 return this.description != null && !this.description.isEmpty(); 17296 } 17297 17298 public boolean hasDescription() { 17299 return this.description != null && !this.description.isEmpty(); 17300 } 17301 17302 /** 17303 * @param value {@link #description} (A free text natural language description 17304 * of the implementation guide from a consumer's perspective.). 17305 * This is the underlying object with id, value and extensions. The 17306 * accessor "getDescription" gives direct access to the value 17307 */ 17308 public ImplementationGuide setDescriptionElement(MarkdownType value) { 17309 this.description = value; 17310 return this; 17311 } 17312 17313 /** 17314 * @return A free text natural language description of the implementation guide 17315 * from a consumer's perspective. 17316 */ 17317 public String getDescription() { 17318 return this.description == null ? null : this.description.getValue(); 17319 } 17320 17321 /** 17322 * @param value A free text natural language description of the implementation 17323 * guide from a consumer's perspective. 17324 */ 17325 public ImplementationGuide setDescription(String value) { 17326 if (value == null) 17327 this.description = null; 17328 else { 17329 if (this.description == null) 17330 this.description = new MarkdownType(); 17331 this.description.setValue(value); 17332 } 17333 return this; 17334 } 17335 17336 /** 17337 * @return {@link #useContext} (The content was developed with a focus and 17338 * intent of supporting the contexts that are listed. These contexts may 17339 * be general categories (gender, age, ...) or may be references to 17340 * specific programs (insurance plans, studies, ...) and may be used to 17341 * assist with indexing and searching for appropriate implementation 17342 * guide instances.) 17343 */ 17344 public List<UsageContext> getUseContext() { 17345 if (this.useContext == null) 17346 this.useContext = new ArrayList<UsageContext>(); 17347 return this.useContext; 17348 } 17349 17350 /** 17351 * @return Returns a reference to <code>this</code> for easy method chaining 17352 */ 17353 public ImplementationGuide setUseContext(List<UsageContext> theUseContext) { 17354 this.useContext = theUseContext; 17355 return this; 17356 } 17357 17358 public boolean hasUseContext() { 17359 if (this.useContext == null) 17360 return false; 17361 for (UsageContext item : this.useContext) 17362 if (!item.isEmpty()) 17363 return true; 17364 return false; 17365 } 17366 17367 public UsageContext addUseContext() { // 3 17368 UsageContext t = new UsageContext(); 17369 if (this.useContext == null) 17370 this.useContext = new ArrayList<UsageContext>(); 17371 this.useContext.add(t); 17372 return t; 17373 } 17374 17375 public ImplementationGuide addUseContext(UsageContext t) { // 3 17376 if (t == null) 17377 return this; 17378 if (this.useContext == null) 17379 this.useContext = new ArrayList<UsageContext>(); 17380 this.useContext.add(t); 17381 return this; 17382 } 17383 17384 /** 17385 * @return The first repetition of repeating field {@link #useContext}, creating 17386 * it if it does not already exist 17387 */ 17388 public UsageContext getUseContextFirstRep() { 17389 if (getUseContext().isEmpty()) { 17390 addUseContext(); 17391 } 17392 return getUseContext().get(0); 17393 } 17394 17395 /** 17396 * @return {@link #jurisdiction} (A legal or geographic region in which the 17397 * implementation guide is intended to be used.) 17398 */ 17399 public List<CodeableConcept> getJurisdiction() { 17400 if (this.jurisdiction == null) 17401 this.jurisdiction = new ArrayList<CodeableConcept>(); 17402 return this.jurisdiction; 17403 } 17404 17405 /** 17406 * @return Returns a reference to <code>this</code> for easy method chaining 17407 */ 17408 public ImplementationGuide setJurisdiction(List<CodeableConcept> theJurisdiction) { 17409 this.jurisdiction = theJurisdiction; 17410 return this; 17411 } 17412 17413 public boolean hasJurisdiction() { 17414 if (this.jurisdiction == null) 17415 return false; 17416 for (CodeableConcept item : this.jurisdiction) 17417 if (!item.isEmpty()) 17418 return true; 17419 return false; 17420 } 17421 17422 public CodeableConcept addJurisdiction() { // 3 17423 CodeableConcept t = new CodeableConcept(); 17424 if (this.jurisdiction == null) 17425 this.jurisdiction = new ArrayList<CodeableConcept>(); 17426 this.jurisdiction.add(t); 17427 return t; 17428 } 17429 17430 public ImplementationGuide addJurisdiction(CodeableConcept t) { // 3 17431 if (t == null) 17432 return this; 17433 if (this.jurisdiction == null) 17434 this.jurisdiction = new ArrayList<CodeableConcept>(); 17435 this.jurisdiction.add(t); 17436 return this; 17437 } 17438 17439 /** 17440 * @return The first repetition of repeating field {@link #jurisdiction}, 17441 * creating it if it does not already exist 17442 */ 17443 public CodeableConcept getJurisdictionFirstRep() { 17444 if (getJurisdiction().isEmpty()) { 17445 addJurisdiction(); 17446 } 17447 return getJurisdiction().get(0); 17448 } 17449 17450 /** 17451 * @return {@link #copyright} (A copyright statement relating to the 17452 * implementation guide and/or its contents. Copyright statements are 17453 * generally legal restrictions on the use and publishing of the 17454 * implementation guide.). This is the underlying object with id, value 17455 * and extensions. The accessor "getCopyright" gives direct access to 17456 * the value 17457 */ 17458 public MarkdownType getCopyrightElement() { 17459 if (this.copyright == null) 17460 if (Configuration.errorOnAutoCreate()) 17461 throw new Error("Attempt to auto-create ImplementationGuide.copyright"); 17462 else if (Configuration.doAutoCreate()) 17463 this.copyright = new MarkdownType(); // bb 17464 return this.copyright; 17465 } 17466 17467 public boolean hasCopyrightElement() { 17468 return this.copyright != null && !this.copyright.isEmpty(); 17469 } 17470 17471 public boolean hasCopyright() { 17472 return this.copyright != null && !this.copyright.isEmpty(); 17473 } 17474 17475 /** 17476 * @param value {@link #copyright} (A copyright statement relating to the 17477 * implementation guide and/or its contents. Copyright statements 17478 * are generally legal restrictions on the use and publishing of 17479 * the implementation guide.). This is the underlying object with 17480 * id, value and extensions. The accessor "getCopyright" gives 17481 * direct access to the value 17482 */ 17483 public ImplementationGuide setCopyrightElement(MarkdownType value) { 17484 this.copyright = value; 17485 return this; 17486 } 17487 17488 /** 17489 * @return A copyright statement relating to the implementation guide and/or its 17490 * contents. Copyright statements are generally legal restrictions on 17491 * the use and publishing of the implementation guide. 17492 */ 17493 public String getCopyright() { 17494 return this.copyright == null ? null : this.copyright.getValue(); 17495 } 17496 17497 /** 17498 * @param value A copyright statement relating to the implementation guide 17499 * and/or its contents. Copyright statements are generally legal 17500 * restrictions on the use and publishing of the implementation 17501 * guide. 17502 */ 17503 public ImplementationGuide setCopyright(String value) { 17504 if (value == null) 17505 this.copyright = null; 17506 else { 17507 if (this.copyright == null) 17508 this.copyright = new MarkdownType(); 17509 this.copyright.setValue(value); 17510 } 17511 return this; 17512 } 17513 17514 /** 17515 * @return {@link #packageId} (The NPM package name for this Implementation 17516 * Guide, used in the NPM package distribution, which is the primary 17517 * mechanism by which FHIR based tooling manages IG dependencies. This 17518 * value must be globally unique, and should be assigned with care.). 17519 * This is the underlying object with id, value and extensions. The 17520 * accessor "getPackageId" gives direct access to the value 17521 */ 17522 public IdType getPackageIdElement() { 17523 if (this.packageId == null) 17524 if (Configuration.errorOnAutoCreate()) 17525 throw new Error("Attempt to auto-create ImplementationGuide.packageId"); 17526 else if (Configuration.doAutoCreate()) 17527 this.packageId = new IdType(); // bb 17528 return this.packageId; 17529 } 17530 17531 public boolean hasPackageIdElement() { 17532 return this.packageId != null && !this.packageId.isEmpty(); 17533 } 17534 17535 public boolean hasPackageId() { 17536 return this.packageId != null && !this.packageId.isEmpty(); 17537 } 17538 17539 /** 17540 * @param value {@link #packageId} (The NPM package name for this Implementation 17541 * Guide, used in the NPM package distribution, which is the 17542 * primary mechanism by which FHIR based tooling manages IG 17543 * dependencies. This value must be globally unique, and should be 17544 * assigned with care.). This is the underlying object with id, 17545 * value and extensions. The accessor "getPackageId" gives direct 17546 * access to the value 17547 */ 17548 public ImplementationGuide setPackageIdElement(IdType value) { 17549 this.packageId = value; 17550 return this; 17551 } 17552 17553 /** 17554 * @return The NPM package name for this Implementation Guide, used in the NPM 17555 * package distribution, which is the primary mechanism by which FHIR 17556 * based tooling manages IG dependencies. This value must be globally 17557 * unique, and should be assigned with care. 17558 */ 17559 public String getPackageId() { 17560 return this.packageId == null ? null : this.packageId.getValue(); 17561 } 17562 17563 /** 17564 * @param value The NPM package name for this Implementation Guide, used in the 17565 * NPM package distribution, which is the primary mechanism by 17566 * which FHIR based tooling manages IG dependencies. This value 17567 * must be globally unique, and should be assigned with care. 17568 */ 17569 public ImplementationGuide setPackageId(String value) { 17570 if (this.packageId == null) 17571 this.packageId = new IdType(); 17572 this.packageId.setValue(value); 17573 return this; 17574 } 17575 17576 /** 17577 * @return {@link #license} (The license that applies to this Implementation 17578 * Guide, using an SPDX license code, or 'not-open-source'.). This is 17579 * the underlying object with id, value and extensions. The accessor 17580 * "getLicense" gives direct access to the value 17581 */ 17582 public Enumeration<SPDXLicense> getLicenseElement() { 17583 if (this.license == null) 17584 if (Configuration.errorOnAutoCreate()) 17585 throw new Error("Attempt to auto-create ImplementationGuide.license"); 17586 else if (Configuration.doAutoCreate()) 17587 this.license = new Enumeration<SPDXLicense>(new SPDXLicenseEnumFactory()); // bb 17588 return this.license; 17589 } 17590 17591 public boolean hasLicenseElement() { 17592 return this.license != null && !this.license.isEmpty(); 17593 } 17594 17595 public boolean hasLicense() { 17596 return this.license != null && !this.license.isEmpty(); 17597 } 17598 17599 /** 17600 * @param value {@link #license} (The license that applies to this 17601 * Implementation Guide, using an SPDX license code, or 17602 * 'not-open-source'.). This is the underlying object with id, 17603 * value and extensions. The accessor "getLicense" gives direct 17604 * access to the value 17605 */ 17606 public ImplementationGuide setLicenseElement(Enumeration<SPDXLicense> value) { 17607 this.license = value; 17608 return this; 17609 } 17610 17611 /** 17612 * @return The license that applies to this Implementation Guide, using an SPDX 17613 * license code, or 'not-open-source'. 17614 */ 17615 public SPDXLicense getLicense() { 17616 return this.license == null ? null : this.license.getValue(); 17617 } 17618 17619 /** 17620 * @param value The license that applies to this Implementation Guide, using an 17621 * SPDX license code, or 'not-open-source'. 17622 */ 17623 public ImplementationGuide setLicense(SPDXLicense value) { 17624 if (value == null) 17625 this.license = null; 17626 else { 17627 if (this.license == null) 17628 this.license = new Enumeration<SPDXLicense>(new SPDXLicenseEnumFactory()); 17629 this.license.setValue(value); 17630 } 17631 return this; 17632 } 17633 17634 /** 17635 * @return {@link #fhirVersion} (The version(s) of the FHIR specification that 17636 * this ImplementationGuide targets - e.g. describes how to use. The 17637 * value of this element is the formal version of the specification, 17638 * without the revision number, e.g. [publication].[major].[minor], 17639 * which is 4.0.1. for this version.) 17640 */ 17641 public List<Enumeration<FHIRVersion>> getFhirVersion() { 17642 if (this.fhirVersion == null) 17643 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 17644 return this.fhirVersion; 17645 } 17646 17647 /** 17648 * @return Returns a reference to <code>this</code> for easy method chaining 17649 */ 17650 public ImplementationGuide setFhirVersion(List<Enumeration<FHIRVersion>> theFhirVersion) { 17651 this.fhirVersion = theFhirVersion; 17652 return this; 17653 } 17654 17655 public boolean hasFhirVersion() { 17656 if (this.fhirVersion == null) 17657 return false; 17658 for (Enumeration<FHIRVersion> item : this.fhirVersion) 17659 if (!item.isEmpty()) 17660 return true; 17661 return false; 17662 } 17663 17664 /** 17665 * @return {@link #fhirVersion} (The version(s) of the FHIR specification that 17666 * this ImplementationGuide targets - e.g. describes how to use. The 17667 * value of this element is the formal version of the specification, 17668 * without the revision number, e.g. [publication].[major].[minor], 17669 * which is 4.0.1. for this version.) 17670 */ 17671 public Enumeration<FHIRVersion> addFhirVersionElement() {// 2 17672 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 17673 if (this.fhirVersion == null) 17674 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 17675 this.fhirVersion.add(t); 17676 return t; 17677 } 17678 17679 /** 17680 * @param value {@link #fhirVersion} (The version(s) of the FHIR specification 17681 * that this ImplementationGuide targets - e.g. describes how to 17682 * use. The value of this element is the formal version of the 17683 * specification, without the revision number, e.g. 17684 * [publication].[major].[minor], which is 4.0.1. for this 17685 * version.) 17686 */ 17687 public ImplementationGuide addFhirVersion(FHIRVersion value) { // 1 17688 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 17689 t.setValue(value); 17690 if (this.fhirVersion == null) 17691 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 17692 this.fhirVersion.add(t); 17693 return this; 17694 } 17695 17696 /** 17697 * @param value {@link #fhirVersion} (The version(s) of the FHIR specification 17698 * that this ImplementationGuide targets - e.g. describes how to 17699 * use. The value of this element is the formal version of the 17700 * specification, without the revision number, e.g. 17701 * [publication].[major].[minor], which is 4.0.1. for this 17702 * version.) 17703 */ 17704 public boolean hasFhirVersion(FHIRVersion value) { 17705 if (this.fhirVersion == null) 17706 return false; 17707 for (Enumeration<FHIRVersion> v : this.fhirVersion) 17708 if (v.getValue().equals(value)) // code 17709 return true; 17710 return false; 17711 } 17712 17713 /** 17714 * @return {@link #dependsOn} (Another implementation guide that this 17715 * implementation depends on. Typically, an implementation guide uses 17716 * value sets, profiles etc.defined in other implementation guides.) 17717 */ 17718 public List<ImplementationGuideDependsOnComponent> getDependsOn() { 17719 if (this.dependsOn == null) 17720 this.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 17721 return this.dependsOn; 17722 } 17723 17724 /** 17725 * @return Returns a reference to <code>this</code> for easy method chaining 17726 */ 17727 public ImplementationGuide setDependsOn(List<ImplementationGuideDependsOnComponent> theDependsOn) { 17728 this.dependsOn = theDependsOn; 17729 return this; 17730 } 17731 17732 public boolean hasDependsOn() { 17733 if (this.dependsOn == null) 17734 return false; 17735 for (ImplementationGuideDependsOnComponent item : this.dependsOn) 17736 if (!item.isEmpty()) 17737 return true; 17738 return false; 17739 } 17740 17741 public ImplementationGuideDependsOnComponent addDependsOn() { // 3 17742 ImplementationGuideDependsOnComponent t = new ImplementationGuideDependsOnComponent(); 17743 if (this.dependsOn == null) 17744 this.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 17745 this.dependsOn.add(t); 17746 return t; 17747 } 17748 17749 public ImplementationGuide addDependsOn(ImplementationGuideDependsOnComponent t) { // 3 17750 if (t == null) 17751 return this; 17752 if (this.dependsOn == null) 17753 this.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 17754 this.dependsOn.add(t); 17755 return this; 17756 } 17757 17758 /** 17759 * @return The first repetition of repeating field {@link #dependsOn}, creating 17760 * it if it does not already exist 17761 */ 17762 public ImplementationGuideDependsOnComponent getDependsOnFirstRep() { 17763 if (getDependsOn().isEmpty()) { 17764 addDependsOn(); 17765 } 17766 return getDependsOn().get(0); 17767 } 17768 17769 /** 17770 * @return {@link #global} (A set of profiles that all resources covered by this 17771 * implementation guide must conform to.) 17772 */ 17773 public List<ImplementationGuideGlobalComponent> getGlobal() { 17774 if (this.global == null) 17775 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 17776 return this.global; 17777 } 17778 17779 /** 17780 * @return Returns a reference to <code>this</code> for easy method chaining 17781 */ 17782 public ImplementationGuide setGlobal(List<ImplementationGuideGlobalComponent> theGlobal) { 17783 this.global = theGlobal; 17784 return this; 17785 } 17786 17787 public boolean hasGlobal() { 17788 if (this.global == null) 17789 return false; 17790 for (ImplementationGuideGlobalComponent item : this.global) 17791 if (!item.isEmpty()) 17792 return true; 17793 return false; 17794 } 17795 17796 public ImplementationGuideGlobalComponent addGlobal() { // 3 17797 ImplementationGuideGlobalComponent t = new ImplementationGuideGlobalComponent(); 17798 if (this.global == null) 17799 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 17800 this.global.add(t); 17801 return t; 17802 } 17803 17804 public ImplementationGuide addGlobal(ImplementationGuideGlobalComponent t) { // 3 17805 if (t == null) 17806 return this; 17807 if (this.global == null) 17808 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 17809 this.global.add(t); 17810 return this; 17811 } 17812 17813 /** 17814 * @return The first repetition of repeating field {@link #global}, creating it 17815 * if it does not already exist 17816 */ 17817 public ImplementationGuideGlobalComponent getGlobalFirstRep() { 17818 if (getGlobal().isEmpty()) { 17819 addGlobal(); 17820 } 17821 return getGlobal().get(0); 17822 } 17823 17824 /** 17825 * @return {@link #definition} (The information needed by an IG publisher tool 17826 * to publish the whole implementation guide.) 17827 */ 17828 public ImplementationGuideDefinitionComponent getDefinition() { 17829 if (this.definition == null) 17830 if (Configuration.errorOnAutoCreate()) 17831 throw new Error("Attempt to auto-create ImplementationGuide.definition"); 17832 else if (Configuration.doAutoCreate()) 17833 this.definition = new ImplementationGuideDefinitionComponent(); // cc 17834 return this.definition; 17835 } 17836 17837 public boolean hasDefinition() { 17838 return this.definition != null && !this.definition.isEmpty(); 17839 } 17840 17841 /** 17842 * @param value {@link #definition} (The information needed by an IG publisher 17843 * tool to publish the whole implementation guide.) 17844 */ 17845 public ImplementationGuide setDefinition(ImplementationGuideDefinitionComponent value) { 17846 this.definition = value; 17847 return this; 17848 } 17849 17850 /** 17851 * @return {@link #manifest} (Information about an assembled implementation 17852 * guide, created by the publication tooling.) 17853 */ 17854 public ImplementationGuideManifestComponent getManifest() { 17855 if (this.manifest == null) 17856 if (Configuration.errorOnAutoCreate()) 17857 throw new Error("Attempt to auto-create ImplementationGuide.manifest"); 17858 else if (Configuration.doAutoCreate()) 17859 this.manifest = new ImplementationGuideManifestComponent(); // cc 17860 return this.manifest; 17861 } 17862 17863 public boolean hasManifest() { 17864 return this.manifest != null && !this.manifest.isEmpty(); 17865 } 17866 17867 /** 17868 * @param value {@link #manifest} (Information about an assembled implementation 17869 * guide, created by the publication tooling.) 17870 */ 17871 public ImplementationGuide setManifest(ImplementationGuideManifestComponent value) { 17872 this.manifest = value; 17873 return this; 17874 } 17875 17876 protected void listChildren(List<Property> children) { 17877 super.listChildren(children); 17878 children.add(new Property("url", "uri", 17879 "An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers.", 17880 0, 1, url)); 17881 children.add(new Property("version", "string", 17882 "The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 17883 0, 1, version)); 17884 children.add(new Property("name", "string", 17885 "A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 17886 0, 1, name)); 17887 children.add(new Property("title", "string", 17888 "A short, descriptive, user-friendly title for the implementation guide.", 0, 1, title)); 17889 children.add(new Property("status", "code", 17890 "The status of this implementation guide. Enables tracking the life-cycle of the content.", 0, 1, status)); 17891 children.add(new Property("experimental", "boolean", 17892 "A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 17893 0, 1, experimental)); 17894 children.add(new Property("date", "dateTime", 17895 "The date (and optionally time) when the implementation guide was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 17896 0, 1, date)); 17897 children.add(new Property("publisher", "string", 17898 "The name of the organization or individual that published the implementation guide.", 0, 1, publisher)); 17899 children.add(new Property("contact", "ContactDetail", 17900 "Contact details to assist a user in finding and communicating with the publisher.", 0, 17901 java.lang.Integer.MAX_VALUE, contact)); 17902 children.add(new Property("description", "markdown", 17903 "A free text natural language description of the implementation guide from a consumer's perspective.", 0, 1, 17904 description)); 17905 children.add(new Property("useContext", "UsageContext", 17906 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances.", 17907 0, java.lang.Integer.MAX_VALUE, useContext)); 17908 children.add(new Property("jurisdiction", "CodeableConcept", 17909 "A legal or geographic region in which the implementation guide is intended to be used.", 0, 17910 java.lang.Integer.MAX_VALUE, jurisdiction)); 17911 children.add(new Property("copyright", "markdown", 17912 "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.", 17913 0, 1, copyright)); 17914 children.add(new Property("packageId", "id", 17915 "The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.", 17916 0, 1, packageId)); 17917 children.add(new Property("license", "code", 17918 "The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.", 0, 17919 1, license)); 17920 children.add(new Property("fhirVersion", "code", 17921 "The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.0.1. for this version.", 17922 0, java.lang.Integer.MAX_VALUE, fhirVersion)); 17923 children.add(new Property("dependsOn", "", 17924 "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 17925 0, java.lang.Integer.MAX_VALUE, dependsOn)); 17926 children.add(new Property("global", "", 17927 "A set of profiles that all resources covered by this implementation guide must conform to.", 0, 17928 java.lang.Integer.MAX_VALUE, global)); 17929 children.add(new Property("definition", "", 17930 "The information needed by an IG publisher tool to publish the whole implementation guide.", 0, 1, definition)); 17931 children.add(new Property("manifest", "", 17932 "Information about an assembled implementation guide, created by the publication tooling.", 0, 1, manifest)); 17933 } 17934 17935 @Override 17936 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 17937 switch (_hash) { 17938 case 116079: 17939 /* url */ return new Property("url", "uri", 17940 "An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers.", 17941 0, 1, url); 17942 case 351608024: 17943 /* version */ return new Property("version", "string", 17944 "The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 17945 0, 1, version); 17946 case 3373707: 17947 /* name */ return new Property("name", "string", 17948 "A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 17949 0, 1, name); 17950 case 110371416: 17951 /* title */ return new Property("title", "string", 17952 "A short, descriptive, user-friendly title for the implementation guide.", 0, 1, title); 17953 case -892481550: 17954 /* status */ return new Property("status", "code", 17955 "The status of this implementation guide. Enables tracking the life-cycle of the content.", 0, 1, status); 17956 case -404562712: 17957 /* experimental */ return new Property("experimental", "boolean", 17958 "A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 17959 0, 1, experimental); 17960 case 3076014: 17961 /* date */ return new Property("date", "dateTime", 17962 "The date (and optionally time) when the implementation guide was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 17963 0, 1, date); 17964 case 1447404028: 17965 /* publisher */ return new Property("publisher", "string", 17966 "The name of the organization or individual that published the implementation guide.", 0, 1, publisher); 17967 case 951526432: 17968 /* contact */ return new Property("contact", "ContactDetail", 17969 "Contact details to assist a user in finding and communicating with the publisher.", 0, 17970 java.lang.Integer.MAX_VALUE, contact); 17971 case -1724546052: 17972 /* description */ return new Property("description", "markdown", 17973 "A free text natural language description of the implementation guide from a consumer's perspective.", 0, 1, 17974 description); 17975 case -669707736: 17976 /* useContext */ return new Property("useContext", "UsageContext", 17977 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances.", 17978 0, java.lang.Integer.MAX_VALUE, useContext); 17979 case -507075711: 17980 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 17981 "A legal or geographic region in which the implementation guide is intended to be used.", 0, 17982 java.lang.Integer.MAX_VALUE, jurisdiction); 17983 case 1522889671: 17984 /* copyright */ return new Property("copyright", "markdown", 17985 "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.", 17986 0, 1, copyright); 17987 case 1802060801: 17988 /* packageId */ return new Property("packageId", "id", 17989 "The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.", 17990 0, 1, packageId); 17991 case 166757441: 17992 /* license */ return new Property("license", "code", 17993 "The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.", 0, 17994 1, license); 17995 case 461006061: 17996 /* fhirVersion */ return new Property("fhirVersion", "code", 17997 "The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.0.1. for this version.", 17998 0, java.lang.Integer.MAX_VALUE, fhirVersion); 17999 case -1109214266: 18000 /* dependsOn */ return new Property("dependsOn", "", 18001 "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 18002 0, java.lang.Integer.MAX_VALUE, dependsOn); 18003 case -1243020381: 18004 /* global */ return new Property("global", "", 18005 "A set of profiles that all resources covered by this implementation guide must conform to.", 0, 18006 java.lang.Integer.MAX_VALUE, global); 18007 case -1014418093: 18008 /* definition */ return new Property("definition", "", 18009 "The information needed by an IG publisher tool to publish the whole implementation guide.", 0, 1, 18010 definition); 18011 case 130625071: 18012 /* manifest */ return new Property("manifest", "", 18013 "Information about an assembled implementation guide, created by the publication tooling.", 0, 1, manifest); 18014 default: 18015 return super.getNamedProperty(_hash, _name, _checkValid); 18016 } 18017 18018 } 18019 18020 @Override 18021 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 18022 switch (hash) { 18023 case 116079: 18024 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 18025 case 351608024: 18026 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 18027 case 3373707: 18028 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 18029 case 110371416: 18030 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 18031 case -892481550: 18032 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 18033 case -404562712: 18034 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 18035 case 3076014: 18036 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 18037 case 1447404028: 18038 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 18039 case 951526432: 18040 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 18041 case -1724546052: 18042 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 18043 case -669707736: 18044 /* useContext */ return this.useContext == null ? new Base[0] 18045 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 18046 case -507075711: 18047 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 18048 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 18049 case 1522889671: 18050 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 18051 case 1802060801: 18052 /* packageId */ return this.packageId == null ? new Base[0] : new Base[] { this.packageId }; // IdType 18053 case 166757441: 18054 /* license */ return this.license == null ? new Base[0] : new Base[] { this.license }; // Enumeration<SPDXLicense> 18055 case 461006061: 18056 /* fhirVersion */ return this.fhirVersion == null ? new Base[0] 18057 : this.fhirVersion.toArray(new Base[this.fhirVersion.size()]); // Enumeration<FHIRVersion> 18058 case -1109214266: 18059 /* dependsOn */ return this.dependsOn == null ? new Base[0] 18060 : this.dependsOn.toArray(new Base[this.dependsOn.size()]); // ImplementationGuideDependsOnComponent 18061 case -1243020381: 18062 /* global */ return this.global == null ? new Base[0] : this.global.toArray(new Base[this.global.size()]); // ImplementationGuideGlobalComponent 18063 case -1014418093: 18064 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // ImplementationGuideDefinitionComponent 18065 case 130625071: 18066 /* manifest */ return this.manifest == null ? new Base[0] : new Base[] { this.manifest }; // ImplementationGuideManifestComponent 18067 default: 18068 return super.getProperty(hash, name, checkValid); 18069 } 18070 18071 } 18072 18073 @Override 18074 public Base setProperty(int hash, String name, Base value) throws FHIRException { 18075 switch (hash) { 18076 case 116079: // url 18077 this.url = castToUri(value); // UriType 18078 return value; 18079 case 351608024: // version 18080 this.version = castToString(value); // StringType 18081 return value; 18082 case 3373707: // name 18083 this.name = castToString(value); // StringType 18084 return value; 18085 case 110371416: // title 18086 this.title = castToString(value); // StringType 18087 return value; 18088 case -892481550: // status 18089 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 18090 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 18091 return value; 18092 case -404562712: // experimental 18093 this.experimental = castToBoolean(value); // BooleanType 18094 return value; 18095 case 3076014: // date 18096 this.date = castToDateTime(value); // DateTimeType 18097 return value; 18098 case 1447404028: // publisher 18099 this.publisher = castToString(value); // StringType 18100 return value; 18101 case 951526432: // contact 18102 this.getContact().add(castToContactDetail(value)); // ContactDetail 18103 return value; 18104 case -1724546052: // description 18105 this.description = castToMarkdown(value); // MarkdownType 18106 return value; 18107 case -669707736: // useContext 18108 this.getUseContext().add(castToUsageContext(value)); // UsageContext 18109 return value; 18110 case -507075711: // jurisdiction 18111 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 18112 return value; 18113 case 1522889671: // copyright 18114 this.copyright = castToMarkdown(value); // MarkdownType 18115 return value; 18116 case 1802060801: // packageId 18117 this.packageId = castToId(value); // IdType 18118 return value; 18119 case 166757441: // license 18120 value = new SPDXLicenseEnumFactory().fromType(castToCode(value)); 18121 this.license = (Enumeration) value; // Enumeration<SPDXLicense> 18122 return value; 18123 case 461006061: // fhirVersion 18124 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 18125 this.getFhirVersion().add((Enumeration) value); // Enumeration<FHIRVersion> 18126 return value; 18127 case -1109214266: // dependsOn 18128 this.getDependsOn().add((ImplementationGuideDependsOnComponent) value); // ImplementationGuideDependsOnComponent 18129 return value; 18130 case -1243020381: // global 18131 this.getGlobal().add((ImplementationGuideGlobalComponent) value); // ImplementationGuideGlobalComponent 18132 return value; 18133 case -1014418093: // definition 18134 this.definition = (ImplementationGuideDefinitionComponent) value; // ImplementationGuideDefinitionComponent 18135 return value; 18136 case 130625071: // manifest 18137 this.manifest = (ImplementationGuideManifestComponent) value; // ImplementationGuideManifestComponent 18138 return value; 18139 default: 18140 return super.setProperty(hash, name, value); 18141 } 18142 18143 } 18144 18145 @Override 18146 public Base setProperty(String name, Base value) throws FHIRException { 18147 if (name.equals("url")) { 18148 this.url = castToUri(value); // UriType 18149 } else if (name.equals("version")) { 18150 this.version = castToString(value); // StringType 18151 } else if (name.equals("name")) { 18152 this.name = castToString(value); // StringType 18153 } else if (name.equals("title")) { 18154 this.title = castToString(value); // StringType 18155 } else if (name.equals("status")) { 18156 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 18157 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 18158 } else if (name.equals("experimental")) { 18159 this.experimental = castToBoolean(value); // BooleanType 18160 } else if (name.equals("date")) { 18161 this.date = castToDateTime(value); // DateTimeType 18162 } else if (name.equals("publisher")) { 18163 this.publisher = castToString(value); // StringType 18164 } else if (name.equals("contact")) { 18165 this.getContact().add(castToContactDetail(value)); 18166 } else if (name.equals("description")) { 18167 this.description = castToMarkdown(value); // MarkdownType 18168 } else if (name.equals("useContext")) { 18169 this.getUseContext().add(castToUsageContext(value)); 18170 } else if (name.equals("jurisdiction")) { 18171 this.getJurisdiction().add(castToCodeableConcept(value)); 18172 } else if (name.equals("copyright")) { 18173 this.copyright = castToMarkdown(value); // MarkdownType 18174 } else if (name.equals("packageId")) { 18175 this.packageId = castToId(value); // IdType 18176 } else if (name.equals("license")) { 18177 value = new SPDXLicenseEnumFactory().fromType(castToCode(value)); 18178 this.license = (Enumeration) value; // Enumeration<SPDXLicense> 18179 } else if (name.equals("fhirVersion")) { 18180 value = new FHIRVersionEnumFactory().fromType(castToCode(value)); 18181 this.getFhirVersion().add((Enumeration) value); 18182 } else if (name.equals("dependsOn")) { 18183 this.getDependsOn().add((ImplementationGuideDependsOnComponent) value); 18184 } else if (name.equals("global")) { 18185 this.getGlobal().add((ImplementationGuideGlobalComponent) value); 18186 } else if (name.equals("definition")) { 18187 this.definition = (ImplementationGuideDefinitionComponent) value; // ImplementationGuideDefinitionComponent 18188 } else if (name.equals("manifest")) { 18189 this.manifest = (ImplementationGuideManifestComponent) value; // ImplementationGuideManifestComponent 18190 } else 18191 return super.setProperty(name, value); 18192 return value; 18193 } 18194 18195 @Override 18196 public void removeChild(String name, Base value) throws FHIRException { 18197 if (name.equals("url")) { 18198 this.url = null; 18199 } else if (name.equals("version")) { 18200 this.version = null; 18201 } else if (name.equals("name")) { 18202 this.name = null; 18203 } else if (name.equals("title")) { 18204 this.title = null; 18205 } else if (name.equals("status")) { 18206 this.status = null; 18207 } else if (name.equals("experimental")) { 18208 this.experimental = null; 18209 } else if (name.equals("date")) { 18210 this.date = null; 18211 } else if (name.equals("publisher")) { 18212 this.publisher = null; 18213 } else if (name.equals("contact")) { 18214 this.getContact().remove(castToContactDetail(value)); 18215 } else if (name.equals("description")) { 18216 this.description = null; 18217 } else if (name.equals("useContext")) { 18218 this.getUseContext().remove(castToUsageContext(value)); 18219 } else if (name.equals("jurisdiction")) { 18220 this.getJurisdiction().remove(castToCodeableConcept(value)); 18221 } else if (name.equals("copyright")) { 18222 this.copyright = null; 18223 } else if (name.equals("packageId")) { 18224 this.packageId = null; 18225 } else if (name.equals("license")) { 18226 this.license = null; 18227 } else if (name.equals("fhirVersion")) { 18228 this.getFhirVersion().remove((Enumeration) value); 18229 } else if (name.equals("dependsOn")) { 18230 this.getDependsOn().remove((ImplementationGuideDependsOnComponent) value); 18231 } else if (name.equals("global")) { 18232 this.getGlobal().remove((ImplementationGuideGlobalComponent) value); 18233 } else if (name.equals("definition")) { 18234 this.definition = null; 18235 } else if (name.equals("manifest")) { 18236 this.manifest = (ImplementationGuideManifestComponent) value; // ImplementationGuideManifestComponent 18237 } else 18238 super.removeChild(name, value); 18239 18240 } 18241 18242 @Override 18243 public Base makeProperty(int hash, String name) throws FHIRException { 18244 switch (hash) { 18245 case 116079: 18246 return getUrlElement(); 18247 case 351608024: 18248 return getVersionElement(); 18249 case 3373707: 18250 return getNameElement(); 18251 case 110371416: 18252 return getTitleElement(); 18253 case -892481550: 18254 return getStatusElement(); 18255 case -404562712: 18256 return getExperimentalElement(); 18257 case 3076014: 18258 return getDateElement(); 18259 case 1447404028: 18260 return getPublisherElement(); 18261 case 951526432: 18262 return addContact(); 18263 case -1724546052: 18264 return getDescriptionElement(); 18265 case -669707736: 18266 return addUseContext(); 18267 case -507075711: 18268 return addJurisdiction(); 18269 case 1522889671: 18270 return getCopyrightElement(); 18271 case 1802060801: 18272 return getPackageIdElement(); 18273 case 166757441: 18274 return getLicenseElement(); 18275 case 461006061: 18276 return addFhirVersionElement(); 18277 case -1109214266: 18278 return addDependsOn(); 18279 case -1243020381: 18280 return addGlobal(); 18281 case -1014418093: 18282 return getDefinition(); 18283 case 130625071: 18284 return getManifest(); 18285 default: 18286 return super.makeProperty(hash, name); 18287 } 18288 18289 } 18290 18291 @Override 18292 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 18293 switch (hash) { 18294 case 116079: 18295 /* url */ return new String[] { "uri" }; 18296 case 351608024: 18297 /* version */ return new String[] { "string" }; 18298 case 3373707: 18299 /* name */ return new String[] { "string" }; 18300 case 110371416: 18301 /* title */ return new String[] { "string" }; 18302 case -892481550: 18303 /* status */ return new String[] { "code" }; 18304 case -404562712: 18305 /* experimental */ return new String[] { "boolean" }; 18306 case 3076014: 18307 /* date */ return new String[] { "dateTime" }; 18308 case 1447404028: 18309 /* publisher */ return new String[] { "string" }; 18310 case 951526432: 18311 /* contact */ return new String[] { "ContactDetail" }; 18312 case -1724546052: 18313 /* description */ return new String[] { "markdown" }; 18314 case -669707736: 18315 /* useContext */ return new String[] { "UsageContext" }; 18316 case -507075711: 18317 /* jurisdiction */ return new String[] { "CodeableConcept" }; 18318 case 1522889671: 18319 /* copyright */ return new String[] { "markdown" }; 18320 case 1802060801: 18321 /* packageId */ return new String[] { "id" }; 18322 case 166757441: 18323 /* license */ return new String[] { "code" }; 18324 case 461006061: 18325 /* fhirVersion */ return new String[] { "code" }; 18326 case -1109214266: 18327 /* dependsOn */ return new String[] {}; 18328 case -1243020381: 18329 /* global */ return new String[] {}; 18330 case -1014418093: 18331 /* definition */ return new String[] {}; 18332 case 130625071: 18333 /* manifest */ return new String[] {}; 18334 default: 18335 return super.getTypesForProperty(hash, name); 18336 } 18337 18338 } 18339 18340 @Override 18341 public Base addChild(String name) throws FHIRException { 18342 if (name.equals("url")) { 18343 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.url"); 18344 } else if (name.equals("version")) { 18345 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.version"); 18346 } else if (name.equals("name")) { 18347 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 18348 } else if (name.equals("title")) { 18349 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.title"); 18350 } else if (name.equals("status")) { 18351 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.status"); 18352 } else if (name.equals("experimental")) { 18353 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.experimental"); 18354 } else if (name.equals("date")) { 18355 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.date"); 18356 } else if (name.equals("publisher")) { 18357 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.publisher"); 18358 } else if (name.equals("contact")) { 18359 return addContact(); 18360 } else if (name.equals("description")) { 18361 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 18362 } else if (name.equals("useContext")) { 18363 return addUseContext(); 18364 } else if (name.equals("jurisdiction")) { 18365 return addJurisdiction(); 18366 } else if (name.equals("copyright")) { 18367 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.copyright"); 18368 } else if (name.equals("packageId")) { 18369 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.packageId"); 18370 } else if (name.equals("license")) { 18371 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.license"); 18372 } else if (name.equals("fhirVersion")) { 18373 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.fhirVersion"); 18374 } else if (name.equals("dependsOn")) { 18375 return addDependsOn(); 18376 } else if (name.equals("global")) { 18377 return addGlobal(); 18378 } else if (name.equals("definition")) { 18379 this.definition = new ImplementationGuideDefinitionComponent(); 18380 return this.definition; 18381 } else if (name.equals("manifest")) { 18382 this.manifest = new ImplementationGuideManifestComponent(); 18383 return this.manifest; 18384 } else 18385 return super.addChild(name); 18386 } 18387 18388 public String fhirType() { 18389 return "ImplementationGuide"; 18390 18391 } 18392 18393 public ImplementationGuide copy() { 18394 ImplementationGuide dst = new ImplementationGuide(); 18395 copyValues(dst); 18396 return dst; 18397 } 18398 18399 public void copyValues(ImplementationGuide dst) { 18400 super.copyValues(dst); 18401 dst.url = url == null ? null : url.copy(); 18402 dst.version = version == null ? null : version.copy(); 18403 dst.name = name == null ? null : name.copy(); 18404 dst.title = title == null ? null : title.copy(); 18405 dst.status = status == null ? null : status.copy(); 18406 dst.experimental = experimental == null ? null : experimental.copy(); 18407 dst.date = date == null ? null : date.copy(); 18408 dst.publisher = publisher == null ? null : publisher.copy(); 18409 if (contact != null) { 18410 dst.contact = new ArrayList<ContactDetail>(); 18411 for (ContactDetail i : contact) 18412 dst.contact.add(i.copy()); 18413 } 18414 ; 18415 dst.description = description == null ? null : description.copy(); 18416 if (useContext != null) { 18417 dst.useContext = new ArrayList<UsageContext>(); 18418 for (UsageContext i : useContext) 18419 dst.useContext.add(i.copy()); 18420 } 18421 ; 18422 if (jurisdiction != null) { 18423 dst.jurisdiction = new ArrayList<CodeableConcept>(); 18424 for (CodeableConcept i : jurisdiction) 18425 dst.jurisdiction.add(i.copy()); 18426 } 18427 ; 18428 dst.copyright = copyright == null ? null : copyright.copy(); 18429 dst.packageId = packageId == null ? null : packageId.copy(); 18430 dst.license = license == null ? null : license.copy(); 18431 if (fhirVersion != null) { 18432 dst.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 18433 for (Enumeration<FHIRVersion> i : fhirVersion) 18434 dst.fhirVersion.add(i.copy()); 18435 } 18436 ; 18437 if (dependsOn != null) { 18438 dst.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 18439 for (ImplementationGuideDependsOnComponent i : dependsOn) 18440 dst.dependsOn.add(i.copy()); 18441 } 18442 ; 18443 if (global != null) { 18444 dst.global = new ArrayList<ImplementationGuideGlobalComponent>(); 18445 for (ImplementationGuideGlobalComponent i : global) 18446 dst.global.add(i.copy()); 18447 } 18448 ; 18449 dst.definition = definition == null ? null : definition.copy(); 18450 dst.manifest = manifest == null ? null : manifest.copy(); 18451 } 18452 18453 protected ImplementationGuide typedCopy() { 18454 return copy(); 18455 } 18456 18457 @Override 18458 public boolean equalsDeep(Base other_) { 18459 if (!super.equalsDeep(other_)) 18460 return false; 18461 if (!(other_ instanceof ImplementationGuide)) 18462 return false; 18463 ImplementationGuide o = (ImplementationGuide) other_; 18464 return compareDeep(copyright, o.copyright, true) && compareDeep(packageId, o.packageId, true) 18465 && compareDeep(license, o.license, true) && compareDeep(fhirVersion, o.fhirVersion, true) 18466 && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(global, o.global, true) 18467 && compareDeep(definition, o.definition, true) && compareDeep(manifest, o.manifest, true); 18468 } 18469 18470 @Override 18471 public boolean equalsShallow(Base other_) { 18472 if (!super.equalsShallow(other_)) 18473 return false; 18474 if (!(other_ instanceof ImplementationGuide)) 18475 return false; 18476 ImplementationGuide o = (ImplementationGuide) other_; 18477 return compareValues(copyright, o.copyright, true) && compareValues(packageId, o.packageId, true) 18478 && compareValues(license, o.license, true) && compareValues(fhirVersion, o.fhirVersion, true); 18479 } 18480 18481 public boolean isEmpty() { 18482 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(copyright, packageId, license, fhirVersion, 18483 dependsOn, global, definition, manifest); 18484 } 18485 18486 @Override 18487 public ResourceType getResourceType() { 18488 return ResourceType.ImplementationGuide; 18489 } 18490 18491 /** 18492 * Search parameter: <b>date</b> 18493 * <p> 18494 * Description: <b>The implementation guide publication date</b><br> 18495 * Type: <b>date</b><br> 18496 * Path: <b>ImplementationGuide.date</b><br> 18497 * </p> 18498 */ 18499 @SearchParamDefinition(name = "date", path = "ImplementationGuide.date", description = "The implementation guide publication date", type = "date") 18500 public static final String SP_DATE = "date"; 18501 /** 18502 * <b>Fluent Client</b> search parameter constant for <b>date</b> 18503 * <p> 18504 * Description: <b>The implementation guide publication date</b><br> 18505 * Type: <b>date</b><br> 18506 * Path: <b>ImplementationGuide.date</b><br> 18507 * </p> 18508 */ 18509 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 18510 SP_DATE); 18511 18512 /** 18513 * Search parameter: <b>context-type-value</b> 18514 * <p> 18515 * Description: <b>A use context type and value assigned to the implementation 18516 * guide</b><br> 18517 * Type: <b>composite</b><br> 18518 * Path: <b></b><br> 18519 * </p> 18520 */ 18521 @SearchParamDefinition(name = "context-type-value", path = "ImplementationGuide.useContext", description = "A use context type and value assigned to the implementation guide", type = "composite", compositeOf = { 18522 "context-type", "context" }) 18523 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 18524 /** 18525 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 18526 * <p> 18527 * Description: <b>A use context type and value assigned to the implementation 18528 * guide</b><br> 18529 * Type: <b>composite</b><br> 18530 * Path: <b></b><br> 18531 * </p> 18532 */ 18533 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 18534 SP_CONTEXT_TYPE_VALUE); 18535 18536 /** 18537 * Search parameter: <b>resource</b> 18538 * <p> 18539 * Description: <b>Location of the resource</b><br> 18540 * Type: <b>reference</b><br> 18541 * Path: <b>ImplementationGuide.definition.resource.reference</b><br> 18542 * </p> 18543 */ 18544 @SearchParamDefinition(name = "resource", path = "ImplementationGuide.definition.resource.reference", description = "Location of the resource", type = "reference") 18545 public static final String SP_RESOURCE = "resource"; 18546 /** 18547 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 18548 * <p> 18549 * Description: <b>Location of the resource</b><br> 18550 * Type: <b>reference</b><br> 18551 * Path: <b>ImplementationGuide.definition.resource.reference</b><br> 18552 * </p> 18553 */ 18554 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18555 SP_RESOURCE); 18556 18557 /** 18558 * Constant for fluent queries to be used to add include statements. Specifies 18559 * the path value of "<b>ImplementationGuide:resource</b>". 18560 */ 18561 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE = new ca.uhn.fhir.model.api.Include( 18562 "ImplementationGuide:resource").toLocked(); 18563 18564 /** 18565 * Search parameter: <b>jurisdiction</b> 18566 * <p> 18567 * Description: <b>Intended jurisdiction for the implementation guide</b><br> 18568 * Type: <b>token</b><br> 18569 * Path: <b>ImplementationGuide.jurisdiction</b><br> 18570 * </p> 18571 */ 18572 @SearchParamDefinition(name = "jurisdiction", path = "ImplementationGuide.jurisdiction", description = "Intended jurisdiction for the implementation guide", type = "token") 18573 public static final String SP_JURISDICTION = "jurisdiction"; 18574 /** 18575 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 18576 * <p> 18577 * Description: <b>Intended jurisdiction for the implementation guide</b><br> 18578 * Type: <b>token</b><br> 18579 * Path: <b>ImplementationGuide.jurisdiction</b><br> 18580 * </p> 18581 */ 18582 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18583 SP_JURISDICTION); 18584 18585 /** 18586 * Search parameter: <b>description</b> 18587 * <p> 18588 * Description: <b>The description of the implementation guide</b><br> 18589 * Type: <b>string</b><br> 18590 * Path: <b>ImplementationGuide.description</b><br> 18591 * </p> 18592 */ 18593 @SearchParamDefinition(name = "description", path = "ImplementationGuide.description", description = "The description of the implementation guide", type = "string") 18594 public static final String SP_DESCRIPTION = "description"; 18595 /** 18596 * <b>Fluent Client</b> search parameter constant for <b>description</b> 18597 * <p> 18598 * Description: <b>The description of the implementation guide</b><br> 18599 * Type: <b>string</b><br> 18600 * Path: <b>ImplementationGuide.description</b><br> 18601 * </p> 18602 */ 18603 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 18604 SP_DESCRIPTION); 18605 18606 /** 18607 * Search parameter: <b>context-type</b> 18608 * <p> 18609 * Description: <b>A type of use context assigned to the implementation 18610 * guide</b><br> 18611 * Type: <b>token</b><br> 18612 * Path: <b>ImplementationGuide.useContext.code</b><br> 18613 * </p> 18614 */ 18615 @SearchParamDefinition(name = "context-type", path = "ImplementationGuide.useContext.code", description = "A type of use context assigned to the implementation guide", type = "token") 18616 public static final String SP_CONTEXT_TYPE = "context-type"; 18617 /** 18618 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 18619 * <p> 18620 * Description: <b>A type of use context assigned to the implementation 18621 * guide</b><br> 18622 * Type: <b>token</b><br> 18623 * Path: <b>ImplementationGuide.useContext.code</b><br> 18624 * </p> 18625 */ 18626 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18627 SP_CONTEXT_TYPE); 18628 18629 /** 18630 * Search parameter: <b>experimental</b> 18631 * <p> 18632 * Description: <b>For testing purposes, not real usage</b><br> 18633 * Type: <b>token</b><br> 18634 * Path: <b>ImplementationGuide.experimental</b><br> 18635 * </p> 18636 */ 18637 @SearchParamDefinition(name = "experimental", path = "ImplementationGuide.experimental", description = "For testing purposes, not real usage", type = "token") 18638 public static final String SP_EXPERIMENTAL = "experimental"; 18639 /** 18640 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 18641 * <p> 18642 * Description: <b>For testing purposes, not real usage</b><br> 18643 * Type: <b>token</b><br> 18644 * Path: <b>ImplementationGuide.experimental</b><br> 18645 * </p> 18646 */ 18647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18648 SP_EXPERIMENTAL); 18649 18650 /** 18651 * Search parameter: <b>global</b> 18652 * <p> 18653 * Description: <b>Profile that all resources must conform to</b><br> 18654 * Type: <b>reference</b><br> 18655 * Path: <b>ImplementationGuide.global.profile</b><br> 18656 * </p> 18657 */ 18658 @SearchParamDefinition(name = "global", path = "ImplementationGuide.global.profile", description = "Profile that all resources must conform to", type = "reference", target = { 18659 StructureDefinition.class }) 18660 public static final String SP_GLOBAL = "global"; 18661 /** 18662 * <b>Fluent Client</b> search parameter constant for <b>global</b> 18663 * <p> 18664 * Description: <b>Profile that all resources must conform to</b><br> 18665 * Type: <b>reference</b><br> 18666 * Path: <b>ImplementationGuide.global.profile</b><br> 18667 * </p> 18668 */ 18669 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GLOBAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18670 SP_GLOBAL); 18671 18672 /** 18673 * Constant for fluent queries to be used to add include statements. Specifies 18674 * the path value of "<b>ImplementationGuide:global</b>". 18675 */ 18676 public static final ca.uhn.fhir.model.api.Include INCLUDE_GLOBAL = new ca.uhn.fhir.model.api.Include( 18677 "ImplementationGuide:global").toLocked(); 18678 18679 /** 18680 * Search parameter: <b>title</b> 18681 * <p> 18682 * Description: <b>The human-friendly name of the implementation guide</b><br> 18683 * Type: <b>string</b><br> 18684 * Path: <b>ImplementationGuide.title</b><br> 18685 * </p> 18686 */ 18687 @SearchParamDefinition(name = "title", path = "ImplementationGuide.title", description = "The human-friendly name of the implementation guide", type = "string") 18688 public static final String SP_TITLE = "title"; 18689 /** 18690 * <b>Fluent Client</b> search parameter constant for <b>title</b> 18691 * <p> 18692 * Description: <b>The human-friendly name of the implementation guide</b><br> 18693 * Type: <b>string</b><br> 18694 * Path: <b>ImplementationGuide.title</b><br> 18695 * </p> 18696 */ 18697 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 18698 SP_TITLE); 18699 18700 /** 18701 * Search parameter: <b>version</b> 18702 * <p> 18703 * Description: <b>The business version of the implementation guide</b><br> 18704 * Type: <b>token</b><br> 18705 * Path: <b>ImplementationGuide.version</b><br> 18706 * </p> 18707 */ 18708 @SearchParamDefinition(name = "version", path = "ImplementationGuide.version", description = "The business version of the implementation guide", type = "token") 18709 public static final String SP_VERSION = "version"; 18710 /** 18711 * <b>Fluent Client</b> search parameter constant for <b>version</b> 18712 * <p> 18713 * Description: <b>The business version of the implementation guide</b><br> 18714 * Type: <b>token</b><br> 18715 * Path: <b>ImplementationGuide.version</b><br> 18716 * </p> 18717 */ 18718 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18719 SP_VERSION); 18720 18721 /** 18722 * Search parameter: <b>url</b> 18723 * <p> 18724 * Description: <b>The uri that identifies the implementation guide</b><br> 18725 * Type: <b>uri</b><br> 18726 * Path: <b>ImplementationGuide.url</b><br> 18727 * </p> 18728 */ 18729 @SearchParamDefinition(name = "url", path = "ImplementationGuide.url", description = "The uri that identifies the implementation guide", type = "uri") 18730 public static final String SP_URL = "url"; 18731 /** 18732 * <b>Fluent Client</b> search parameter constant for <b>url</b> 18733 * <p> 18734 * Description: <b>The uri that identifies the implementation guide</b><br> 18735 * Type: <b>uri</b><br> 18736 * Path: <b>ImplementationGuide.url</b><br> 18737 * </p> 18738 */ 18739 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 18740 18741 /** 18742 * Search parameter: <b>context-quantity</b> 18743 * <p> 18744 * Description: <b>A quantity- or range-valued use context assigned to the 18745 * implementation guide</b><br> 18746 * Type: <b>quantity</b><br> 18747 * Path: <b>ImplementationGuide.useContext.valueQuantity, 18748 * ImplementationGuide.useContext.valueRange</b><br> 18749 * </p> 18750 */ 18751 @SearchParamDefinition(name = "context-quantity", path = "(ImplementationGuide.useContext.value as Quantity) | (ImplementationGuide.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the implementation guide", type = "quantity") 18752 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 18753 /** 18754 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 18755 * <p> 18756 * Description: <b>A quantity- or range-valued use context assigned to the 18757 * implementation guide</b><br> 18758 * Type: <b>quantity</b><br> 18759 * Path: <b>ImplementationGuide.useContext.valueQuantity, 18760 * ImplementationGuide.useContext.valueRange</b><br> 18761 * </p> 18762 */ 18763 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 18764 SP_CONTEXT_QUANTITY); 18765 18766 /** 18767 * Search parameter: <b>depends-on</b> 18768 * <p> 18769 * Description: <b>Identity of the IG that this depends on</b><br> 18770 * Type: <b>reference</b><br> 18771 * Path: <b>ImplementationGuide.dependsOn.uri</b><br> 18772 * </p> 18773 */ 18774 @SearchParamDefinition(name = "depends-on", path = "ImplementationGuide.dependsOn.uri", description = "Identity of the IG that this depends on", type = "reference", target = { 18775 ImplementationGuide.class }) 18776 public static final String SP_DEPENDS_ON = "depends-on"; 18777 /** 18778 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 18779 * <p> 18780 * Description: <b>Identity of the IG that this depends on</b><br> 18781 * Type: <b>reference</b><br> 18782 * Path: <b>ImplementationGuide.dependsOn.uri</b><br> 18783 * </p> 18784 */ 18785 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 18786 SP_DEPENDS_ON); 18787 18788 /** 18789 * Constant for fluent queries to be used to add include statements. Specifies 18790 * the path value of "<b>ImplementationGuide:depends-on</b>". 18791 */ 18792 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 18793 "ImplementationGuide:depends-on").toLocked(); 18794 18795 /** 18796 * Search parameter: <b>name</b> 18797 * <p> 18798 * Description: <b>Computationally friendly name of the implementation 18799 * guide</b><br> 18800 * Type: <b>string</b><br> 18801 * Path: <b>ImplementationGuide.name</b><br> 18802 * </p> 18803 */ 18804 @SearchParamDefinition(name = "name", path = "ImplementationGuide.name", description = "Computationally friendly name of the implementation guide", type = "string") 18805 public static final String SP_NAME = "name"; 18806 /** 18807 * <b>Fluent Client</b> search parameter constant for <b>name</b> 18808 * <p> 18809 * Description: <b>Computationally friendly name of the implementation 18810 * guide</b><br> 18811 * Type: <b>string</b><br> 18812 * Path: <b>ImplementationGuide.name</b><br> 18813 * </p> 18814 */ 18815 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 18816 SP_NAME); 18817 18818 /** 18819 * Search parameter: <b>context</b> 18820 * <p> 18821 * Description: <b>A use context assigned to the implementation guide</b><br> 18822 * Type: <b>token</b><br> 18823 * Path: <b>ImplementationGuide.useContext.valueCodeableConcept</b><br> 18824 * </p> 18825 */ 18826 @SearchParamDefinition(name = "context", path = "(ImplementationGuide.useContext.value as CodeableConcept)", description = "A use context assigned to the implementation guide", type = "token") 18827 public static final String SP_CONTEXT = "context"; 18828 /** 18829 * <b>Fluent Client</b> search parameter constant for <b>context</b> 18830 * <p> 18831 * Description: <b>A use context assigned to the implementation guide</b><br> 18832 * Type: <b>token</b><br> 18833 * Path: <b>ImplementationGuide.useContext.valueCodeableConcept</b><br> 18834 * </p> 18835 */ 18836 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18837 SP_CONTEXT); 18838 18839 /** 18840 * Search parameter: <b>publisher</b> 18841 * <p> 18842 * Description: <b>Name of the publisher of the implementation guide</b><br> 18843 * Type: <b>string</b><br> 18844 * Path: <b>ImplementationGuide.publisher</b><br> 18845 * </p> 18846 */ 18847 @SearchParamDefinition(name = "publisher", path = "ImplementationGuide.publisher", description = "Name of the publisher of the implementation guide", type = "string") 18848 public static final String SP_PUBLISHER = "publisher"; 18849 /** 18850 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 18851 * <p> 18852 * Description: <b>Name of the publisher of the implementation guide</b><br> 18853 * Type: <b>string</b><br> 18854 * Path: <b>ImplementationGuide.publisher</b><br> 18855 * </p> 18856 */ 18857 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 18858 SP_PUBLISHER); 18859 18860 /** 18861 * Search parameter: <b>context-type-quantity</b> 18862 * <p> 18863 * Description: <b>A use context type and quantity- or range-based value 18864 * assigned to the implementation guide</b><br> 18865 * Type: <b>composite</b><br> 18866 * Path: <b></b><br> 18867 * </p> 18868 */ 18869 @SearchParamDefinition(name = "context-type-quantity", path = "ImplementationGuide.useContext", description = "A use context type and quantity- or range-based value assigned to the implementation guide", type = "composite", compositeOf = { 18870 "context-type", "context-quantity" }) 18871 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 18872 /** 18873 * <b>Fluent Client</b> search parameter constant for 18874 * <b>context-type-quantity</b> 18875 * <p> 18876 * Description: <b>A use context type and quantity- or range-based value 18877 * assigned to the implementation guide</b><br> 18878 * Type: <b>composite</b><br> 18879 * Path: <b></b><br> 18880 * </p> 18881 */ 18882 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 18883 SP_CONTEXT_TYPE_QUANTITY); 18884 18885 /** 18886 * Search parameter: <b>status</b> 18887 * <p> 18888 * Description: <b>The current status of the implementation guide</b><br> 18889 * Type: <b>token</b><br> 18890 * Path: <b>ImplementationGuide.status</b><br> 18891 * </p> 18892 */ 18893 @SearchParamDefinition(name = "status", path = "ImplementationGuide.status", description = "The current status of the implementation guide", type = "token") 18894 public static final String SP_STATUS = "status"; 18895 /** 18896 * <b>Fluent Client</b> search parameter constant for <b>status</b> 18897 * <p> 18898 * Description: <b>The current status of the implementation guide</b><br> 18899 * Type: <b>token</b><br> 18900 * Path: <b>ImplementationGuide.status</b><br> 18901 * </p> 18902 */ 18903 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 18904 SP_STATUS); 18905 18906}