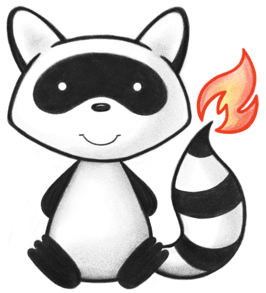
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Details of a Health Insurance product/plan provided by an organization. 050 */ 051@ResourceDef(name = "InsurancePlan", profile = "http://hl7.org/fhir/StructureDefinition/InsurancePlan") 052public class InsurancePlan extends DomainResource { 053 054 @Block() 055 public static class InsurancePlanContactComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * Indicates a purpose for which the contact can be reached. 058 */ 059 @Child(name = "purpose", type = { 060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 061 @Description(shortDefinition = "The type of contact", formalDefinition = "Indicates a purpose for which the contact can be reached.") 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contactentity-type") 063 protected CodeableConcept purpose; 064 065 /** 066 * A name associated with the contact. 067 */ 068 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 069 @Description(shortDefinition = "A name associated with the contact", formalDefinition = "A name associated with the contact.") 070 protected HumanName name; 071 072 /** 073 * A contact detail (e.g. a telephone number or an email address) by which the 074 * party may be contacted. 075 */ 076 @Child(name = "telecom", type = { 077 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 078 @Description(shortDefinition = "Contact details (telephone, email, etc.) for a contact", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.") 079 protected List<ContactPoint> telecom; 080 081 /** 082 * Visiting or postal addresses for the contact. 083 */ 084 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 085 @Description(shortDefinition = "Visiting or postal addresses for the contact", formalDefinition = "Visiting or postal addresses for the contact.") 086 protected Address address; 087 088 private static final long serialVersionUID = 1831121305L; 089 090 /** 091 * Constructor 092 */ 093 public InsurancePlanContactComponent() { 094 super(); 095 } 096 097 /** 098 * @return {@link #purpose} (Indicates a purpose for which the contact can be 099 * reached.) 100 */ 101 public CodeableConcept getPurpose() { 102 if (this.purpose == null) 103 if (Configuration.errorOnAutoCreate()) 104 throw new Error("Attempt to auto-create InsurancePlanContactComponent.purpose"); 105 else if (Configuration.doAutoCreate()) 106 this.purpose = new CodeableConcept(); // cc 107 return this.purpose; 108 } 109 110 public boolean hasPurpose() { 111 return this.purpose != null && !this.purpose.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #purpose} (Indicates a purpose for which the contact can 116 * be reached.) 117 */ 118 public InsurancePlanContactComponent setPurpose(CodeableConcept value) { 119 this.purpose = value; 120 return this; 121 } 122 123 /** 124 * @return {@link #name} (A name associated with the contact.) 125 */ 126 public HumanName getName() { 127 if (this.name == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create InsurancePlanContactComponent.name"); 130 else if (Configuration.doAutoCreate()) 131 this.name = new HumanName(); // cc 132 return this.name; 133 } 134 135 public boolean hasName() { 136 return this.name != null && !this.name.isEmpty(); 137 } 138 139 /** 140 * @param value {@link #name} (A name associated with the contact.) 141 */ 142 public InsurancePlanContactComponent setName(HumanName value) { 143 this.name = value; 144 return this; 145 } 146 147 /** 148 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 149 * email address) by which the party may be contacted.) 150 */ 151 public List<ContactPoint> getTelecom() { 152 if (this.telecom == null) 153 this.telecom = new ArrayList<ContactPoint>(); 154 return this.telecom; 155 } 156 157 /** 158 * @return Returns a reference to <code>this</code> for easy method chaining 159 */ 160 public InsurancePlanContactComponent setTelecom(List<ContactPoint> theTelecom) { 161 this.telecom = theTelecom; 162 return this; 163 } 164 165 public boolean hasTelecom() { 166 if (this.telecom == null) 167 return false; 168 for (ContactPoint item : this.telecom) 169 if (!item.isEmpty()) 170 return true; 171 return false; 172 } 173 174 public ContactPoint addTelecom() { // 3 175 ContactPoint t = new ContactPoint(); 176 if (this.telecom == null) 177 this.telecom = new ArrayList<ContactPoint>(); 178 this.telecom.add(t); 179 return t; 180 } 181 182 public InsurancePlanContactComponent addTelecom(ContactPoint t) { // 3 183 if (t == null) 184 return this; 185 if (this.telecom == null) 186 this.telecom = new ArrayList<ContactPoint>(); 187 this.telecom.add(t); 188 return this; 189 } 190 191 /** 192 * @return The first repetition of repeating field {@link #telecom}, creating it 193 * if it does not already exist 194 */ 195 public ContactPoint getTelecomFirstRep() { 196 if (getTelecom().isEmpty()) { 197 addTelecom(); 198 } 199 return getTelecom().get(0); 200 } 201 202 /** 203 * @return {@link #address} (Visiting or postal addresses for the contact.) 204 */ 205 public Address getAddress() { 206 if (this.address == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create InsurancePlanContactComponent.address"); 209 else if (Configuration.doAutoCreate()) 210 this.address = new Address(); // cc 211 return this.address; 212 } 213 214 public boolean hasAddress() { 215 return this.address != null && !this.address.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #address} (Visiting or postal addresses for the contact.) 220 */ 221 public InsurancePlanContactComponent setAddress(Address value) { 222 this.address = value; 223 return this; 224 } 225 226 protected void listChildren(List<Property> children) { 227 super.listChildren(children); 228 children.add(new Property("purpose", "CodeableConcept", 229 "Indicates a purpose for which the contact can be reached.", 0, 1, purpose)); 230 children.add(new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name)); 231 children.add(new Property("telecom", "ContactPoint", 232 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 233 java.lang.Integer.MAX_VALUE, telecom)); 234 children.add(new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, address)); 235 } 236 237 @Override 238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 239 switch (_hash) { 240 case -220463842: 241 /* purpose */ return new Property("purpose", "CodeableConcept", 242 "Indicates a purpose for which the contact can be reached.", 0, 1, purpose); 243 case 3373707: 244 /* name */ return new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name); 245 case -1429363305: 246 /* telecom */ return new Property("telecom", "ContactPoint", 247 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 248 java.lang.Integer.MAX_VALUE, telecom); 249 case -1147692044: 250 /* address */ return new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, 251 address); 252 default: 253 return super.getNamedProperty(_hash, _name, _checkValid); 254 } 255 256 } 257 258 @Override 259 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 260 switch (hash) { 261 case -220463842: 262 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // CodeableConcept 263 case 3373707: 264 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // HumanName 265 case -1429363305: 266 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 267 case -1147692044: 268 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 269 default: 270 return super.getProperty(hash, name, checkValid); 271 } 272 273 } 274 275 @Override 276 public Base setProperty(int hash, String name, Base value) throws FHIRException { 277 switch (hash) { 278 case -220463842: // purpose 279 this.purpose = castToCodeableConcept(value); // CodeableConcept 280 return value; 281 case 3373707: // name 282 this.name = castToHumanName(value); // HumanName 283 return value; 284 case -1429363305: // telecom 285 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 286 return value; 287 case -1147692044: // address 288 this.address = castToAddress(value); // Address 289 return value; 290 default: 291 return super.setProperty(hash, name, value); 292 } 293 294 } 295 296 @Override 297 public Base setProperty(String name, Base value) throws FHIRException { 298 if (name.equals("purpose")) { 299 this.purpose = castToCodeableConcept(value); // CodeableConcept 300 } else if (name.equals("name")) { 301 this.name = castToHumanName(value); // HumanName 302 } else if (name.equals("telecom")) { 303 this.getTelecom().add(castToContactPoint(value)); 304 } else if (name.equals("address")) { 305 this.address = castToAddress(value); // Address 306 } else 307 return super.setProperty(name, value); 308 return value; 309 } 310 311 @Override 312 public Base makeProperty(int hash, String name) throws FHIRException { 313 switch (hash) { 314 case -220463842: 315 return getPurpose(); 316 case 3373707: 317 return getName(); 318 case -1429363305: 319 return addTelecom(); 320 case -1147692044: 321 return getAddress(); 322 default: 323 return super.makeProperty(hash, name); 324 } 325 326 } 327 328 @Override 329 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 330 switch (hash) { 331 case -220463842: 332 /* purpose */ return new String[] { "CodeableConcept" }; 333 case 3373707: 334 /* name */ return new String[] { "HumanName" }; 335 case -1429363305: 336 /* telecom */ return new String[] { "ContactPoint" }; 337 case -1147692044: 338 /* address */ return new String[] { "Address" }; 339 default: 340 return super.getTypesForProperty(hash, name); 341 } 342 343 } 344 345 @Override 346 public Base addChild(String name) throws FHIRException { 347 if (name.equals("purpose")) { 348 this.purpose = new CodeableConcept(); 349 return this.purpose; 350 } else if (name.equals("name")) { 351 this.name = new HumanName(); 352 return this.name; 353 } else if (name.equals("telecom")) { 354 return addTelecom(); 355 } else if (name.equals("address")) { 356 this.address = new Address(); 357 return this.address; 358 } else 359 return super.addChild(name); 360 } 361 362 public InsurancePlanContactComponent copy() { 363 InsurancePlanContactComponent dst = new InsurancePlanContactComponent(); 364 copyValues(dst); 365 return dst; 366 } 367 368 public void copyValues(InsurancePlanContactComponent dst) { 369 super.copyValues(dst); 370 dst.purpose = purpose == null ? null : purpose.copy(); 371 dst.name = name == null ? null : name.copy(); 372 if (telecom != null) { 373 dst.telecom = new ArrayList<ContactPoint>(); 374 for (ContactPoint i : telecom) 375 dst.telecom.add(i.copy()); 376 } 377 ; 378 dst.address = address == null ? null : address.copy(); 379 } 380 381 @Override 382 public boolean equalsDeep(Base other_) { 383 if (!super.equalsDeep(other_)) 384 return false; 385 if (!(other_ instanceof InsurancePlanContactComponent)) 386 return false; 387 InsurancePlanContactComponent o = (InsurancePlanContactComponent) other_; 388 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) 389 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true); 390 } 391 392 @Override 393 public boolean equalsShallow(Base other_) { 394 if (!super.equalsShallow(other_)) 395 return false; 396 if (!(other_ instanceof InsurancePlanContactComponent)) 397 return false; 398 InsurancePlanContactComponent o = (InsurancePlanContactComponent) other_; 399 return true; 400 } 401 402 public boolean isEmpty() { 403 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, name, telecom, address); 404 } 405 406 public String fhirType() { 407 return "InsurancePlan.contact"; 408 409 } 410 411 } 412 413 @Block() 414 public static class InsurancePlanCoverageComponent extends BackboneElement implements IBaseBackboneElement { 415 /** 416 * Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; 417 * Drug; Short Term; Long Term Care; Hospice; Home Health). 418 */ 419 @Child(name = "type", type = { 420 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 421 @Description(shortDefinition = "Type of coverage", formalDefinition = "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).") 422 protected CodeableConcept type; 423 424 /** 425 * Reference to the network that providing the type of coverage. 426 */ 427 @Child(name = "network", type = { 428 Organization.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 429 @Description(shortDefinition = "What networks provide coverage", formalDefinition = "Reference to the network that providing the type of coverage.") 430 protected List<Reference> network; 431 /** 432 * The actual objects that are the target of the reference (Reference to the 433 * network that providing the type of coverage.) 434 */ 435 protected List<Organization> networkTarget; 436 437 /** 438 * Specific benefits under this type of coverage. 439 */ 440 @Child(name = "benefit", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 441 @Description(shortDefinition = "List of benefits", formalDefinition = "Specific benefits under this type of coverage.") 442 protected List<CoverageBenefitComponent> benefit; 443 444 private static final long serialVersionUID = -1186191877L; 445 446 /** 447 * Constructor 448 */ 449 public InsurancePlanCoverageComponent() { 450 super(); 451 } 452 453 /** 454 * Constructor 455 */ 456 public InsurancePlanCoverageComponent(CodeableConcept type) { 457 super(); 458 this.type = type; 459 } 460 461 /** 462 * @return {@link #type} (Type of coverage (Medical; Dental; Mental Health; 463 * Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; 464 * Home Health).) 465 */ 466 public CodeableConcept getType() { 467 if (this.type == null) 468 if (Configuration.errorOnAutoCreate()) 469 throw new Error("Attempt to auto-create InsurancePlanCoverageComponent.type"); 470 else if (Configuration.doAutoCreate()) 471 this.type = new CodeableConcept(); // cc 472 return this.type; 473 } 474 475 public boolean hasType() { 476 return this.type != null && !this.type.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #type} (Type of coverage (Medical; Dental; Mental Health; 481 * Substance Abuse; Vision; Drug; Short Term; Long Term Care; 482 * Hospice; Home Health).) 483 */ 484 public InsurancePlanCoverageComponent setType(CodeableConcept value) { 485 this.type = value; 486 return this; 487 } 488 489 /** 490 * @return {@link #network} (Reference to the network that providing the type of 491 * coverage.) 492 */ 493 public List<Reference> getNetwork() { 494 if (this.network == null) 495 this.network = new ArrayList<Reference>(); 496 return this.network; 497 } 498 499 /** 500 * @return Returns a reference to <code>this</code> for easy method chaining 501 */ 502 public InsurancePlanCoverageComponent setNetwork(List<Reference> theNetwork) { 503 this.network = theNetwork; 504 return this; 505 } 506 507 public boolean hasNetwork() { 508 if (this.network == null) 509 return false; 510 for (Reference item : this.network) 511 if (!item.isEmpty()) 512 return true; 513 return false; 514 } 515 516 public Reference addNetwork() { // 3 517 Reference t = new Reference(); 518 if (this.network == null) 519 this.network = new ArrayList<Reference>(); 520 this.network.add(t); 521 return t; 522 } 523 524 public InsurancePlanCoverageComponent addNetwork(Reference t) { // 3 525 if (t == null) 526 return this; 527 if (this.network == null) 528 this.network = new ArrayList<Reference>(); 529 this.network.add(t); 530 return this; 531 } 532 533 /** 534 * @return The first repetition of repeating field {@link #network}, creating it 535 * if it does not already exist 536 */ 537 public Reference getNetworkFirstRep() { 538 if (getNetwork().isEmpty()) { 539 addNetwork(); 540 } 541 return getNetwork().get(0); 542 } 543 544 /** 545 * @deprecated Use Reference#setResource(IBaseResource) instead 546 */ 547 @Deprecated 548 public List<Organization> getNetworkTarget() { 549 if (this.networkTarget == null) 550 this.networkTarget = new ArrayList<Organization>(); 551 return this.networkTarget; 552 } 553 554 /** 555 * @deprecated Use Reference#setResource(IBaseResource) instead 556 */ 557 @Deprecated 558 public Organization addNetworkTarget() { 559 Organization r = new Organization(); 560 if (this.networkTarget == null) 561 this.networkTarget = new ArrayList<Organization>(); 562 this.networkTarget.add(r); 563 return r; 564 } 565 566 /** 567 * @return {@link #benefit} (Specific benefits under this type of coverage.) 568 */ 569 public List<CoverageBenefitComponent> getBenefit() { 570 if (this.benefit == null) 571 this.benefit = new ArrayList<CoverageBenefitComponent>(); 572 return this.benefit; 573 } 574 575 /** 576 * @return Returns a reference to <code>this</code> for easy method chaining 577 */ 578 public InsurancePlanCoverageComponent setBenefit(List<CoverageBenefitComponent> theBenefit) { 579 this.benefit = theBenefit; 580 return this; 581 } 582 583 public boolean hasBenefit() { 584 if (this.benefit == null) 585 return false; 586 for (CoverageBenefitComponent item : this.benefit) 587 if (!item.isEmpty()) 588 return true; 589 return false; 590 } 591 592 public CoverageBenefitComponent addBenefit() { // 3 593 CoverageBenefitComponent t = new CoverageBenefitComponent(); 594 if (this.benefit == null) 595 this.benefit = new ArrayList<CoverageBenefitComponent>(); 596 this.benefit.add(t); 597 return t; 598 } 599 600 public InsurancePlanCoverageComponent addBenefit(CoverageBenefitComponent t) { // 3 601 if (t == null) 602 return this; 603 if (this.benefit == null) 604 this.benefit = new ArrayList<CoverageBenefitComponent>(); 605 this.benefit.add(t); 606 return this; 607 } 608 609 /** 610 * @return The first repetition of repeating field {@link #benefit}, creating it 611 * if it does not already exist 612 */ 613 public CoverageBenefitComponent getBenefitFirstRep() { 614 if (getBenefit().isEmpty()) { 615 addBenefit(); 616 } 617 return getBenefit().get(0); 618 } 619 620 protected void listChildren(List<Property> children) { 621 super.listChildren(children); 622 children.add(new Property("type", "CodeableConcept", 623 "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).", 624 0, 1, type)); 625 children.add(new Property("network", "Reference(Organization)", 626 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network)); 627 children.add(new Property("benefit", "", "Specific benefits under this type of coverage.", 0, 628 java.lang.Integer.MAX_VALUE, benefit)); 629 } 630 631 @Override 632 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 633 switch (_hash) { 634 case 3575610: 635 /* type */ return new Property("type", "CodeableConcept", 636 "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).", 637 0, 1, type); 638 case 1843485230: 639 /* network */ return new Property("network", "Reference(Organization)", 640 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network); 641 case -222710633: 642 /* benefit */ return new Property("benefit", "", "Specific benefits under this type of coverage.", 0, 643 java.lang.Integer.MAX_VALUE, benefit); 644 default: 645 return super.getNamedProperty(_hash, _name, _checkValid); 646 } 647 648 } 649 650 @Override 651 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 652 switch (hash) { 653 case 3575610: 654 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 655 case 1843485230: 656 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 657 case -222710633: 658 /* benefit */ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // CoverageBenefitComponent 659 default: 660 return super.getProperty(hash, name, checkValid); 661 } 662 663 } 664 665 @Override 666 public Base setProperty(int hash, String name, Base value) throws FHIRException { 667 switch (hash) { 668 case 3575610: // type 669 this.type = castToCodeableConcept(value); // CodeableConcept 670 return value; 671 case 1843485230: // network 672 this.getNetwork().add(castToReference(value)); // Reference 673 return value; 674 case -222710633: // benefit 675 this.getBenefit().add((CoverageBenefitComponent) value); // CoverageBenefitComponent 676 return value; 677 default: 678 return super.setProperty(hash, name, value); 679 } 680 681 } 682 683 @Override 684 public Base setProperty(String name, Base value) throws FHIRException { 685 if (name.equals("type")) { 686 this.type = castToCodeableConcept(value); // CodeableConcept 687 } else if (name.equals("network")) { 688 this.getNetwork().add(castToReference(value)); 689 } else if (name.equals("benefit")) { 690 this.getBenefit().add((CoverageBenefitComponent) value); 691 } else 692 return super.setProperty(name, value); 693 return value; 694 } 695 696 @Override 697 public Base makeProperty(int hash, String name) throws FHIRException { 698 switch (hash) { 699 case 3575610: 700 return getType(); 701 case 1843485230: 702 return addNetwork(); 703 case -222710633: 704 return addBenefit(); 705 default: 706 return super.makeProperty(hash, name); 707 } 708 709 } 710 711 @Override 712 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 713 switch (hash) { 714 case 3575610: 715 /* type */ return new String[] { "CodeableConcept" }; 716 case 1843485230: 717 /* network */ return new String[] { "Reference" }; 718 case -222710633: 719 /* benefit */ return new String[] {}; 720 default: 721 return super.getTypesForProperty(hash, name); 722 } 723 724 } 725 726 @Override 727 public Base addChild(String name) throws FHIRException { 728 if (name.equals("type")) { 729 this.type = new CodeableConcept(); 730 return this.type; 731 } else if (name.equals("network")) { 732 return addNetwork(); 733 } else if (name.equals("benefit")) { 734 return addBenefit(); 735 } else 736 return super.addChild(name); 737 } 738 739 public InsurancePlanCoverageComponent copy() { 740 InsurancePlanCoverageComponent dst = new InsurancePlanCoverageComponent(); 741 copyValues(dst); 742 return dst; 743 } 744 745 public void copyValues(InsurancePlanCoverageComponent dst) { 746 super.copyValues(dst); 747 dst.type = type == null ? null : type.copy(); 748 if (network != null) { 749 dst.network = new ArrayList<Reference>(); 750 for (Reference i : network) 751 dst.network.add(i.copy()); 752 } 753 ; 754 if (benefit != null) { 755 dst.benefit = new ArrayList<CoverageBenefitComponent>(); 756 for (CoverageBenefitComponent i : benefit) 757 dst.benefit.add(i.copy()); 758 } 759 ; 760 } 761 762 @Override 763 public boolean equalsDeep(Base other_) { 764 if (!super.equalsDeep(other_)) 765 return false; 766 if (!(other_ instanceof InsurancePlanCoverageComponent)) 767 return false; 768 InsurancePlanCoverageComponent o = (InsurancePlanCoverageComponent) other_; 769 return compareDeep(type, o.type, true) && compareDeep(network, o.network, true) 770 && compareDeep(benefit, o.benefit, true); 771 } 772 773 @Override 774 public boolean equalsShallow(Base other_) { 775 if (!super.equalsShallow(other_)) 776 return false; 777 if (!(other_ instanceof InsurancePlanCoverageComponent)) 778 return false; 779 InsurancePlanCoverageComponent o = (InsurancePlanCoverageComponent) other_; 780 return true; 781 } 782 783 public boolean isEmpty() { 784 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, network, benefit); 785 } 786 787 public String fhirType() { 788 return "InsurancePlan.coverage"; 789 790 } 791 792 } 793 794 @Block() 795 public static class CoverageBenefitComponent extends BackboneElement implements IBaseBackboneElement { 796 /** 797 * Type of benefit (primary care; speciality care; inpatient; outpatient). 798 */ 799 @Child(name = "type", type = { 800 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 801 @Description(shortDefinition = "Type of benefit", formalDefinition = "Type of benefit (primary care; speciality care; inpatient; outpatient).") 802 protected CodeableConcept type; 803 804 /** 805 * The referral requirements to have access/coverage for this benefit. 806 */ 807 @Child(name = "requirement", type = { 808 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 809 @Description(shortDefinition = "Referral requirements", formalDefinition = "The referral requirements to have access/coverage for this benefit.") 810 protected StringType requirement; 811 812 /** 813 * The specific limits on the benefit. 814 */ 815 @Child(name = "limit", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 816 @Description(shortDefinition = "Benefit limits", formalDefinition = "The specific limits on the benefit.") 817 protected List<CoverageBenefitLimitComponent> limit; 818 819 private static final long serialVersionUID = -113658449L; 820 821 /** 822 * Constructor 823 */ 824 public CoverageBenefitComponent() { 825 super(); 826 } 827 828 /** 829 * Constructor 830 */ 831 public CoverageBenefitComponent(CodeableConcept type) { 832 super(); 833 this.type = type; 834 } 835 836 /** 837 * @return {@link #type} (Type of benefit (primary care; speciality care; 838 * inpatient; outpatient).) 839 */ 840 public CodeableConcept getType() { 841 if (this.type == null) 842 if (Configuration.errorOnAutoCreate()) 843 throw new Error("Attempt to auto-create CoverageBenefitComponent.type"); 844 else if (Configuration.doAutoCreate()) 845 this.type = new CodeableConcept(); // cc 846 return this.type; 847 } 848 849 public boolean hasType() { 850 return this.type != null && !this.type.isEmpty(); 851 } 852 853 /** 854 * @param value {@link #type} (Type of benefit (primary care; speciality care; 855 * inpatient; outpatient).) 856 */ 857 public CoverageBenefitComponent setType(CodeableConcept value) { 858 this.type = value; 859 return this; 860 } 861 862 /** 863 * @return {@link #requirement} (The referral requirements to have 864 * access/coverage for this benefit.). This is the underlying object 865 * with id, value and extensions. The accessor "getRequirement" gives 866 * direct access to the value 867 */ 868 public StringType getRequirementElement() { 869 if (this.requirement == null) 870 if (Configuration.errorOnAutoCreate()) 871 throw new Error("Attempt to auto-create CoverageBenefitComponent.requirement"); 872 else if (Configuration.doAutoCreate()) 873 this.requirement = new StringType(); // bb 874 return this.requirement; 875 } 876 877 public boolean hasRequirementElement() { 878 return this.requirement != null && !this.requirement.isEmpty(); 879 } 880 881 public boolean hasRequirement() { 882 return this.requirement != null && !this.requirement.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #requirement} (The referral requirements to have 887 * access/coverage for this benefit.). This is the underlying 888 * object with id, value and extensions. The accessor 889 * "getRequirement" gives direct access to the value 890 */ 891 public CoverageBenefitComponent setRequirementElement(StringType value) { 892 this.requirement = value; 893 return this; 894 } 895 896 /** 897 * @return The referral requirements to have access/coverage for this benefit. 898 */ 899 public String getRequirement() { 900 return this.requirement == null ? null : this.requirement.getValue(); 901 } 902 903 /** 904 * @param value The referral requirements to have access/coverage for this 905 * benefit. 906 */ 907 public CoverageBenefitComponent setRequirement(String value) { 908 if (Utilities.noString(value)) 909 this.requirement = null; 910 else { 911 if (this.requirement == null) 912 this.requirement = new StringType(); 913 this.requirement.setValue(value); 914 } 915 return this; 916 } 917 918 /** 919 * @return {@link #limit} (The specific limits on the benefit.) 920 */ 921 public List<CoverageBenefitLimitComponent> getLimit() { 922 if (this.limit == null) 923 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 924 return this.limit; 925 } 926 927 /** 928 * @return Returns a reference to <code>this</code> for easy method chaining 929 */ 930 public CoverageBenefitComponent setLimit(List<CoverageBenefitLimitComponent> theLimit) { 931 this.limit = theLimit; 932 return this; 933 } 934 935 public boolean hasLimit() { 936 if (this.limit == null) 937 return false; 938 for (CoverageBenefitLimitComponent item : this.limit) 939 if (!item.isEmpty()) 940 return true; 941 return false; 942 } 943 944 public CoverageBenefitLimitComponent addLimit() { // 3 945 CoverageBenefitLimitComponent t = new CoverageBenefitLimitComponent(); 946 if (this.limit == null) 947 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 948 this.limit.add(t); 949 return t; 950 } 951 952 public CoverageBenefitComponent addLimit(CoverageBenefitLimitComponent t) { // 3 953 if (t == null) 954 return this; 955 if (this.limit == null) 956 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 957 this.limit.add(t); 958 return this; 959 } 960 961 /** 962 * @return The first repetition of repeating field {@link #limit}, creating it 963 * if it does not already exist 964 */ 965 public CoverageBenefitLimitComponent getLimitFirstRep() { 966 if (getLimit().isEmpty()) { 967 addLimit(); 968 } 969 return getLimit().get(0); 970 } 971 972 protected void listChildren(List<Property> children) { 973 super.listChildren(children); 974 children.add(new Property("type", "CodeableConcept", 975 "Type of benefit (primary care; speciality care; inpatient; outpatient).", 0, 1, type)); 976 children.add(new Property("requirement", "string", 977 "The referral requirements to have access/coverage for this benefit.", 0, 1, requirement)); 978 children 979 .add(new Property("limit", "", "The specific limits on the benefit.", 0, java.lang.Integer.MAX_VALUE, limit)); 980 } 981 982 @Override 983 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 984 switch (_hash) { 985 case 3575610: 986 /* type */ return new Property("type", "CodeableConcept", 987 "Type of benefit (primary care; speciality care; inpatient; outpatient).", 0, 1, type); 988 case 363387971: 989 /* requirement */ return new Property("requirement", "string", 990 "The referral requirements to have access/coverage for this benefit.", 0, 1, requirement); 991 case 102976443: 992 /* limit */ return new Property("limit", "", "The specific limits on the benefit.", 0, 993 java.lang.Integer.MAX_VALUE, limit); 994 default: 995 return super.getNamedProperty(_hash, _name, _checkValid); 996 } 997 998 } 999 1000 @Override 1001 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1002 switch (hash) { 1003 case 3575610: 1004 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1005 case 363387971: 1006 /* requirement */ return this.requirement == null ? new Base[0] : new Base[] { this.requirement }; // StringType 1007 case 102976443: 1008 /* limit */ return this.limit == null ? new Base[0] : this.limit.toArray(new Base[this.limit.size()]); // CoverageBenefitLimitComponent 1009 default: 1010 return super.getProperty(hash, name, checkValid); 1011 } 1012 1013 } 1014 1015 @Override 1016 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1017 switch (hash) { 1018 case 3575610: // type 1019 this.type = castToCodeableConcept(value); // CodeableConcept 1020 return value; 1021 case 363387971: // requirement 1022 this.requirement = castToString(value); // StringType 1023 return value; 1024 case 102976443: // limit 1025 this.getLimit().add((CoverageBenefitLimitComponent) value); // CoverageBenefitLimitComponent 1026 return value; 1027 default: 1028 return super.setProperty(hash, name, value); 1029 } 1030 1031 } 1032 1033 @Override 1034 public Base setProperty(String name, Base value) throws FHIRException { 1035 if (name.equals("type")) { 1036 this.type = castToCodeableConcept(value); // CodeableConcept 1037 } else if (name.equals("requirement")) { 1038 this.requirement = castToString(value); // StringType 1039 } else if (name.equals("limit")) { 1040 this.getLimit().add((CoverageBenefitLimitComponent) value); 1041 } else 1042 return super.setProperty(name, value); 1043 return value; 1044 } 1045 1046 @Override 1047 public Base makeProperty(int hash, String name) throws FHIRException { 1048 switch (hash) { 1049 case 3575610: 1050 return getType(); 1051 case 363387971: 1052 return getRequirementElement(); 1053 case 102976443: 1054 return addLimit(); 1055 default: 1056 return super.makeProperty(hash, name); 1057 } 1058 1059 } 1060 1061 @Override 1062 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1063 switch (hash) { 1064 case 3575610: 1065 /* type */ return new String[] { "CodeableConcept" }; 1066 case 363387971: 1067 /* requirement */ return new String[] { "string" }; 1068 case 102976443: 1069 /* limit */ return new String[] {}; 1070 default: 1071 return super.getTypesForProperty(hash, name); 1072 } 1073 1074 } 1075 1076 @Override 1077 public Base addChild(String name) throws FHIRException { 1078 if (name.equals("type")) { 1079 this.type = new CodeableConcept(); 1080 return this.type; 1081 } else if (name.equals("requirement")) { 1082 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.requirement"); 1083 } else if (name.equals("limit")) { 1084 return addLimit(); 1085 } else 1086 return super.addChild(name); 1087 } 1088 1089 public CoverageBenefitComponent copy() { 1090 CoverageBenefitComponent dst = new CoverageBenefitComponent(); 1091 copyValues(dst); 1092 return dst; 1093 } 1094 1095 public void copyValues(CoverageBenefitComponent dst) { 1096 super.copyValues(dst); 1097 dst.type = type == null ? null : type.copy(); 1098 dst.requirement = requirement == null ? null : requirement.copy(); 1099 if (limit != null) { 1100 dst.limit = new ArrayList<CoverageBenefitLimitComponent>(); 1101 for (CoverageBenefitLimitComponent i : limit) 1102 dst.limit.add(i.copy()); 1103 } 1104 ; 1105 } 1106 1107 @Override 1108 public boolean equalsDeep(Base other_) { 1109 if (!super.equalsDeep(other_)) 1110 return false; 1111 if (!(other_ instanceof CoverageBenefitComponent)) 1112 return false; 1113 CoverageBenefitComponent o = (CoverageBenefitComponent) other_; 1114 return compareDeep(type, o.type, true) && compareDeep(requirement, o.requirement, true) 1115 && compareDeep(limit, o.limit, true); 1116 } 1117 1118 @Override 1119 public boolean equalsShallow(Base other_) { 1120 if (!super.equalsShallow(other_)) 1121 return false; 1122 if (!(other_ instanceof CoverageBenefitComponent)) 1123 return false; 1124 CoverageBenefitComponent o = (CoverageBenefitComponent) other_; 1125 return compareValues(requirement, o.requirement, true); 1126 } 1127 1128 public boolean isEmpty() { 1129 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, requirement, limit); 1130 } 1131 1132 public String fhirType() { 1133 return "InsurancePlan.coverage.benefit"; 1134 1135 } 1136 1137 } 1138 1139 @Block() 1140 public static class CoverageBenefitLimitComponent extends BackboneElement implements IBaseBackboneElement { 1141 /** 1142 * The maximum amount of a service item a plan will pay for a covered benefit. 1143 * For examples. wellness visits, or eyeglasses. 1144 */ 1145 @Child(name = "value", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1146 @Description(shortDefinition = "Maximum value allowed", formalDefinition = "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.") 1147 protected Quantity value; 1148 1149 /** 1150 * The specific limit on the benefit. 1151 */ 1152 @Child(name = "code", type = { 1153 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1154 @Description(shortDefinition = "Benefit limit details", formalDefinition = "The specific limit on the benefit.") 1155 protected CodeableConcept code; 1156 1157 private static final long serialVersionUID = -304318128L; 1158 1159 /** 1160 * Constructor 1161 */ 1162 public CoverageBenefitLimitComponent() { 1163 super(); 1164 } 1165 1166 /** 1167 * @return {@link #value} (The maximum amount of a service item a plan will pay 1168 * for a covered benefit. For examples. wellness visits, or eyeglasses.) 1169 */ 1170 public Quantity getValue() { 1171 if (this.value == null) 1172 if (Configuration.errorOnAutoCreate()) 1173 throw new Error("Attempt to auto-create CoverageBenefitLimitComponent.value"); 1174 else if (Configuration.doAutoCreate()) 1175 this.value = new Quantity(); // cc 1176 return this.value; 1177 } 1178 1179 public boolean hasValue() { 1180 return this.value != null && !this.value.isEmpty(); 1181 } 1182 1183 /** 1184 * @param value {@link #value} (The maximum amount of a service item a plan will 1185 * pay for a covered benefit. For examples. wellness visits, or 1186 * eyeglasses.) 1187 */ 1188 public CoverageBenefitLimitComponent setValue(Quantity value) { 1189 this.value = value; 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #code} (The specific limit on the benefit.) 1195 */ 1196 public CodeableConcept getCode() { 1197 if (this.code == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create CoverageBenefitLimitComponent.code"); 1200 else if (Configuration.doAutoCreate()) 1201 this.code = new CodeableConcept(); // cc 1202 return this.code; 1203 } 1204 1205 public boolean hasCode() { 1206 return this.code != null && !this.code.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #code} (The specific limit on the benefit.) 1211 */ 1212 public CoverageBenefitLimitComponent setCode(CodeableConcept value) { 1213 this.code = value; 1214 return this; 1215 } 1216 1217 protected void listChildren(List<Property> children) { 1218 super.listChildren(children); 1219 children.add(new Property("value", "Quantity", 1220 "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.", 1221 0, 1, value)); 1222 children.add(new Property("code", "CodeableConcept", "The specific limit on the benefit.", 0, 1, code)); 1223 } 1224 1225 @Override 1226 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1227 switch (_hash) { 1228 case 111972721: 1229 /* value */ return new Property("value", "Quantity", 1230 "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.", 1231 0, 1, value); 1232 case 3059181: 1233 /* code */ return new Property("code", "CodeableConcept", "The specific limit on the benefit.", 0, 1, code); 1234 default: 1235 return super.getNamedProperty(_hash, _name, _checkValid); 1236 } 1237 1238 } 1239 1240 @Override 1241 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1242 switch (hash) { 1243 case 111972721: 1244 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Quantity 1245 case 3059181: 1246 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1247 default: 1248 return super.getProperty(hash, name, checkValid); 1249 } 1250 1251 } 1252 1253 @Override 1254 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1255 switch (hash) { 1256 case 111972721: // value 1257 this.value = castToQuantity(value); // Quantity 1258 return value; 1259 case 3059181: // code 1260 this.code = castToCodeableConcept(value); // CodeableConcept 1261 return value; 1262 default: 1263 return super.setProperty(hash, name, value); 1264 } 1265 1266 } 1267 1268 @Override 1269 public Base setProperty(String name, Base value) throws FHIRException { 1270 if (name.equals("value")) { 1271 this.value = castToQuantity(value); // Quantity 1272 } else if (name.equals("code")) { 1273 this.code = castToCodeableConcept(value); // CodeableConcept 1274 } else 1275 return super.setProperty(name, value); 1276 return value; 1277 } 1278 1279 @Override 1280 public Base makeProperty(int hash, String name) throws FHIRException { 1281 switch (hash) { 1282 case 111972721: 1283 return getValue(); 1284 case 3059181: 1285 return getCode(); 1286 default: 1287 return super.makeProperty(hash, name); 1288 } 1289 1290 } 1291 1292 @Override 1293 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1294 switch (hash) { 1295 case 111972721: 1296 /* value */ return new String[] { "Quantity" }; 1297 case 3059181: 1298 /* code */ return new String[] { "CodeableConcept" }; 1299 default: 1300 return super.getTypesForProperty(hash, name); 1301 } 1302 1303 } 1304 1305 @Override 1306 public Base addChild(String name) throws FHIRException { 1307 if (name.equals("value")) { 1308 this.value = new Quantity(); 1309 return this.value; 1310 } else if (name.equals("code")) { 1311 this.code = new CodeableConcept(); 1312 return this.code; 1313 } else 1314 return super.addChild(name); 1315 } 1316 1317 public CoverageBenefitLimitComponent copy() { 1318 CoverageBenefitLimitComponent dst = new CoverageBenefitLimitComponent(); 1319 copyValues(dst); 1320 return dst; 1321 } 1322 1323 public void copyValues(CoverageBenefitLimitComponent dst) { 1324 super.copyValues(dst); 1325 dst.value = value == null ? null : value.copy(); 1326 dst.code = code == null ? null : code.copy(); 1327 } 1328 1329 @Override 1330 public boolean equalsDeep(Base other_) { 1331 if (!super.equalsDeep(other_)) 1332 return false; 1333 if (!(other_ instanceof CoverageBenefitLimitComponent)) 1334 return false; 1335 CoverageBenefitLimitComponent o = (CoverageBenefitLimitComponent) other_; 1336 return compareDeep(value, o.value, true) && compareDeep(code, o.code, true); 1337 } 1338 1339 @Override 1340 public boolean equalsShallow(Base other_) { 1341 if (!super.equalsShallow(other_)) 1342 return false; 1343 if (!(other_ instanceof CoverageBenefitLimitComponent)) 1344 return false; 1345 CoverageBenefitLimitComponent o = (CoverageBenefitLimitComponent) other_; 1346 return true; 1347 } 1348 1349 public boolean isEmpty() { 1350 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, code); 1351 } 1352 1353 public String fhirType() { 1354 return "InsurancePlan.coverage.benefit.limit"; 1355 1356 } 1357 1358 } 1359 1360 @Block() 1361 public static class InsurancePlanPlanComponent extends BackboneElement implements IBaseBackboneElement { 1362 /** 1363 * Business identifiers assigned to this health insurance plan which remain 1364 * constant as the resource is updated and propagates from server to server. 1365 */ 1366 @Child(name = "identifier", type = { 1367 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1368 @Description(shortDefinition = "Business Identifier for Product", formalDefinition = "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.") 1369 protected List<Identifier> identifier; 1370 1371 /** 1372 * Type of plan. For example, "Platinum" or "High Deductable". 1373 */ 1374 @Child(name = "type", type = { 1375 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1376 @Description(shortDefinition = "Type of plan", formalDefinition = "Type of plan. For example, \"Platinum\" or \"High Deductable\".") 1377 protected CodeableConcept type; 1378 1379 /** 1380 * The geographic region in which a health insurance plan's benefits apply. 1381 */ 1382 @Child(name = "coverageArea", type = { 1383 Location.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1384 @Description(shortDefinition = "Where product applies", formalDefinition = "The geographic region in which a health insurance plan's benefits apply.") 1385 protected List<Reference> coverageArea; 1386 /** 1387 * The actual objects that are the target of the reference (The geographic 1388 * region in which a health insurance plan's benefits apply.) 1389 */ 1390 protected List<Location> coverageAreaTarget; 1391 1392 /** 1393 * Reference to the network that providing the type of coverage. 1394 */ 1395 @Child(name = "network", type = { 1396 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1397 @Description(shortDefinition = "What networks provide coverage", formalDefinition = "Reference to the network that providing the type of coverage.") 1398 protected List<Reference> network; 1399 /** 1400 * The actual objects that are the target of the reference (Reference to the 1401 * network that providing the type of coverage.) 1402 */ 1403 protected List<Organization> networkTarget; 1404 1405 /** 1406 * Overall costs associated with the plan. 1407 */ 1408 @Child(name = "generalCost", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1409 @Description(shortDefinition = "Overall costs", formalDefinition = "Overall costs associated with the plan.") 1410 protected List<InsurancePlanPlanGeneralCostComponent> generalCost; 1411 1412 /** 1413 * Costs associated with the coverage provided by the product. 1414 */ 1415 @Child(name = "specificCost", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1416 @Description(shortDefinition = "Specific costs", formalDefinition = "Costs associated with the coverage provided by the product.") 1417 protected List<InsurancePlanPlanSpecificCostComponent> specificCost; 1418 1419 private static final long serialVersionUID = -2063324071L; 1420 1421 /** 1422 * Constructor 1423 */ 1424 public InsurancePlanPlanComponent() { 1425 super(); 1426 } 1427 1428 /** 1429 * @return {@link #identifier} (Business identifiers assigned to this health 1430 * insurance plan which remain constant as the resource is updated and 1431 * propagates from server to server.) 1432 */ 1433 public List<Identifier> getIdentifier() { 1434 if (this.identifier == null) 1435 this.identifier = new ArrayList<Identifier>(); 1436 return this.identifier; 1437 } 1438 1439 /** 1440 * @return Returns a reference to <code>this</code> for easy method chaining 1441 */ 1442 public InsurancePlanPlanComponent setIdentifier(List<Identifier> theIdentifier) { 1443 this.identifier = theIdentifier; 1444 return this; 1445 } 1446 1447 public boolean hasIdentifier() { 1448 if (this.identifier == null) 1449 return false; 1450 for (Identifier item : this.identifier) 1451 if (!item.isEmpty()) 1452 return true; 1453 return false; 1454 } 1455 1456 public Identifier addIdentifier() { // 3 1457 Identifier t = new Identifier(); 1458 if (this.identifier == null) 1459 this.identifier = new ArrayList<Identifier>(); 1460 this.identifier.add(t); 1461 return t; 1462 } 1463 1464 public InsurancePlanPlanComponent addIdentifier(Identifier t) { // 3 1465 if (t == null) 1466 return this; 1467 if (this.identifier == null) 1468 this.identifier = new ArrayList<Identifier>(); 1469 this.identifier.add(t); 1470 return this; 1471 } 1472 1473 /** 1474 * @return The first repetition of repeating field {@link #identifier}, creating 1475 * it if it does not already exist 1476 */ 1477 public Identifier getIdentifierFirstRep() { 1478 if (getIdentifier().isEmpty()) { 1479 addIdentifier(); 1480 } 1481 return getIdentifier().get(0); 1482 } 1483 1484 /** 1485 * @return {@link #type} (Type of plan. For example, "Platinum" or "High 1486 * Deductable".) 1487 */ 1488 public CodeableConcept getType() { 1489 if (this.type == null) 1490 if (Configuration.errorOnAutoCreate()) 1491 throw new Error("Attempt to auto-create InsurancePlanPlanComponent.type"); 1492 else if (Configuration.doAutoCreate()) 1493 this.type = new CodeableConcept(); // cc 1494 return this.type; 1495 } 1496 1497 public boolean hasType() { 1498 return this.type != null && !this.type.isEmpty(); 1499 } 1500 1501 /** 1502 * @param value {@link #type} (Type of plan. For example, "Platinum" or "High 1503 * Deductable".) 1504 */ 1505 public InsurancePlanPlanComponent setType(CodeableConcept value) { 1506 this.type = value; 1507 return this; 1508 } 1509 1510 /** 1511 * @return {@link #coverageArea} (The geographic region in which a health 1512 * insurance plan's benefits apply.) 1513 */ 1514 public List<Reference> getCoverageArea() { 1515 if (this.coverageArea == null) 1516 this.coverageArea = new ArrayList<Reference>(); 1517 return this.coverageArea; 1518 } 1519 1520 /** 1521 * @return Returns a reference to <code>this</code> for easy method chaining 1522 */ 1523 public InsurancePlanPlanComponent setCoverageArea(List<Reference> theCoverageArea) { 1524 this.coverageArea = theCoverageArea; 1525 return this; 1526 } 1527 1528 public boolean hasCoverageArea() { 1529 if (this.coverageArea == null) 1530 return false; 1531 for (Reference item : this.coverageArea) 1532 if (!item.isEmpty()) 1533 return true; 1534 return false; 1535 } 1536 1537 public Reference addCoverageArea() { // 3 1538 Reference t = new Reference(); 1539 if (this.coverageArea == null) 1540 this.coverageArea = new ArrayList<Reference>(); 1541 this.coverageArea.add(t); 1542 return t; 1543 } 1544 1545 public InsurancePlanPlanComponent addCoverageArea(Reference t) { // 3 1546 if (t == null) 1547 return this; 1548 if (this.coverageArea == null) 1549 this.coverageArea = new ArrayList<Reference>(); 1550 this.coverageArea.add(t); 1551 return this; 1552 } 1553 1554 /** 1555 * @return The first repetition of repeating field {@link #coverageArea}, 1556 * creating it if it does not already exist 1557 */ 1558 public Reference getCoverageAreaFirstRep() { 1559 if (getCoverageArea().isEmpty()) { 1560 addCoverageArea(); 1561 } 1562 return getCoverageArea().get(0); 1563 } 1564 1565 /** 1566 * @deprecated Use Reference#setResource(IBaseResource) instead 1567 */ 1568 @Deprecated 1569 public List<Location> getCoverageAreaTarget() { 1570 if (this.coverageAreaTarget == null) 1571 this.coverageAreaTarget = new ArrayList<Location>(); 1572 return this.coverageAreaTarget; 1573 } 1574 1575 /** 1576 * @deprecated Use Reference#setResource(IBaseResource) instead 1577 */ 1578 @Deprecated 1579 public Location addCoverageAreaTarget() { 1580 Location r = new Location(); 1581 if (this.coverageAreaTarget == null) 1582 this.coverageAreaTarget = new ArrayList<Location>(); 1583 this.coverageAreaTarget.add(r); 1584 return r; 1585 } 1586 1587 /** 1588 * @return {@link #network} (Reference to the network that providing the type of 1589 * coverage.) 1590 */ 1591 public List<Reference> getNetwork() { 1592 if (this.network == null) 1593 this.network = new ArrayList<Reference>(); 1594 return this.network; 1595 } 1596 1597 /** 1598 * @return Returns a reference to <code>this</code> for easy method chaining 1599 */ 1600 public InsurancePlanPlanComponent setNetwork(List<Reference> theNetwork) { 1601 this.network = theNetwork; 1602 return this; 1603 } 1604 1605 public boolean hasNetwork() { 1606 if (this.network == null) 1607 return false; 1608 for (Reference item : this.network) 1609 if (!item.isEmpty()) 1610 return true; 1611 return false; 1612 } 1613 1614 public Reference addNetwork() { // 3 1615 Reference t = new Reference(); 1616 if (this.network == null) 1617 this.network = new ArrayList<Reference>(); 1618 this.network.add(t); 1619 return t; 1620 } 1621 1622 public InsurancePlanPlanComponent addNetwork(Reference t) { // 3 1623 if (t == null) 1624 return this; 1625 if (this.network == null) 1626 this.network = new ArrayList<Reference>(); 1627 this.network.add(t); 1628 return this; 1629 } 1630 1631 /** 1632 * @return The first repetition of repeating field {@link #network}, creating it 1633 * if it does not already exist 1634 */ 1635 public Reference getNetworkFirstRep() { 1636 if (getNetwork().isEmpty()) { 1637 addNetwork(); 1638 } 1639 return getNetwork().get(0); 1640 } 1641 1642 /** 1643 * @deprecated Use Reference#setResource(IBaseResource) instead 1644 */ 1645 @Deprecated 1646 public List<Organization> getNetworkTarget() { 1647 if (this.networkTarget == null) 1648 this.networkTarget = new ArrayList<Organization>(); 1649 return this.networkTarget; 1650 } 1651 1652 /** 1653 * @deprecated Use Reference#setResource(IBaseResource) instead 1654 */ 1655 @Deprecated 1656 public Organization addNetworkTarget() { 1657 Organization r = new Organization(); 1658 if (this.networkTarget == null) 1659 this.networkTarget = new ArrayList<Organization>(); 1660 this.networkTarget.add(r); 1661 return r; 1662 } 1663 1664 /** 1665 * @return {@link #generalCost} (Overall costs associated with the plan.) 1666 */ 1667 public List<InsurancePlanPlanGeneralCostComponent> getGeneralCost() { 1668 if (this.generalCost == null) 1669 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1670 return this.generalCost; 1671 } 1672 1673 /** 1674 * @return Returns a reference to <code>this</code> for easy method chaining 1675 */ 1676 public InsurancePlanPlanComponent setGeneralCost(List<InsurancePlanPlanGeneralCostComponent> theGeneralCost) { 1677 this.generalCost = theGeneralCost; 1678 return this; 1679 } 1680 1681 public boolean hasGeneralCost() { 1682 if (this.generalCost == null) 1683 return false; 1684 for (InsurancePlanPlanGeneralCostComponent item : this.generalCost) 1685 if (!item.isEmpty()) 1686 return true; 1687 return false; 1688 } 1689 1690 public InsurancePlanPlanGeneralCostComponent addGeneralCost() { // 3 1691 InsurancePlanPlanGeneralCostComponent t = new InsurancePlanPlanGeneralCostComponent(); 1692 if (this.generalCost == null) 1693 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1694 this.generalCost.add(t); 1695 return t; 1696 } 1697 1698 public InsurancePlanPlanComponent addGeneralCost(InsurancePlanPlanGeneralCostComponent t) { // 3 1699 if (t == null) 1700 return this; 1701 if (this.generalCost == null) 1702 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1703 this.generalCost.add(t); 1704 return this; 1705 } 1706 1707 /** 1708 * @return The first repetition of repeating field {@link #generalCost}, 1709 * creating it if it does not already exist 1710 */ 1711 public InsurancePlanPlanGeneralCostComponent getGeneralCostFirstRep() { 1712 if (getGeneralCost().isEmpty()) { 1713 addGeneralCost(); 1714 } 1715 return getGeneralCost().get(0); 1716 } 1717 1718 /** 1719 * @return {@link #specificCost} (Costs associated with the coverage provided by 1720 * the product.) 1721 */ 1722 public List<InsurancePlanPlanSpecificCostComponent> getSpecificCost() { 1723 if (this.specificCost == null) 1724 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1725 return this.specificCost; 1726 } 1727 1728 /** 1729 * @return Returns a reference to <code>this</code> for easy method chaining 1730 */ 1731 public InsurancePlanPlanComponent setSpecificCost(List<InsurancePlanPlanSpecificCostComponent> theSpecificCost) { 1732 this.specificCost = theSpecificCost; 1733 return this; 1734 } 1735 1736 public boolean hasSpecificCost() { 1737 if (this.specificCost == null) 1738 return false; 1739 for (InsurancePlanPlanSpecificCostComponent item : this.specificCost) 1740 if (!item.isEmpty()) 1741 return true; 1742 return false; 1743 } 1744 1745 public InsurancePlanPlanSpecificCostComponent addSpecificCost() { // 3 1746 InsurancePlanPlanSpecificCostComponent t = new InsurancePlanPlanSpecificCostComponent(); 1747 if (this.specificCost == null) 1748 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1749 this.specificCost.add(t); 1750 return t; 1751 } 1752 1753 public InsurancePlanPlanComponent addSpecificCost(InsurancePlanPlanSpecificCostComponent t) { // 3 1754 if (t == null) 1755 return this; 1756 if (this.specificCost == null) 1757 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1758 this.specificCost.add(t); 1759 return this; 1760 } 1761 1762 /** 1763 * @return The first repetition of repeating field {@link #specificCost}, 1764 * creating it if it does not already exist 1765 */ 1766 public InsurancePlanPlanSpecificCostComponent getSpecificCostFirstRep() { 1767 if (getSpecificCost().isEmpty()) { 1768 addSpecificCost(); 1769 } 1770 return getSpecificCost().get(0); 1771 } 1772 1773 protected void listChildren(List<Property> children) { 1774 super.listChildren(children); 1775 children.add(new Property("identifier", "Identifier", 1776 "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.", 1777 0, java.lang.Integer.MAX_VALUE, identifier)); 1778 children.add(new Property("type", "CodeableConcept", 1779 "Type of plan. For example, \"Platinum\" or \"High Deductable\".", 0, 1, type)); 1780 children.add(new Property("coverageArea", "Reference(Location)", 1781 "The geographic region in which a health insurance plan's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 1782 coverageArea)); 1783 children.add(new Property("network", "Reference(Organization)", 1784 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network)); 1785 children.add(new Property("generalCost", "", "Overall costs associated with the plan.", 0, 1786 java.lang.Integer.MAX_VALUE, generalCost)); 1787 children.add(new Property("specificCost", "", "Costs associated with the coverage provided by the product.", 0, 1788 java.lang.Integer.MAX_VALUE, specificCost)); 1789 } 1790 1791 @Override 1792 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1793 switch (_hash) { 1794 case -1618432855: 1795 /* identifier */ return new Property("identifier", "Identifier", 1796 "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.", 1797 0, java.lang.Integer.MAX_VALUE, identifier); 1798 case 3575610: 1799 /* type */ return new Property("type", "CodeableConcept", 1800 "Type of plan. For example, \"Platinum\" or \"High Deductable\".", 0, 1, type); 1801 case -1532328299: 1802 /* coverageArea */ return new Property("coverageArea", "Reference(Location)", 1803 "The geographic region in which a health insurance plan's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 1804 coverageArea); 1805 case 1843485230: 1806 /* network */ return new Property("network", "Reference(Organization)", 1807 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network); 1808 case 878344405: 1809 /* generalCost */ return new Property("generalCost", "", "Overall costs associated with the plan.", 0, 1810 java.lang.Integer.MAX_VALUE, generalCost); 1811 case -1205656545: 1812 /* specificCost */ return new Property("specificCost", "", 1813 "Costs associated with the coverage provided by the product.", 0, java.lang.Integer.MAX_VALUE, 1814 specificCost); 1815 default: 1816 return super.getNamedProperty(_hash, _name, _checkValid); 1817 } 1818 1819 } 1820 1821 @Override 1822 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1823 switch (hash) { 1824 case -1618432855: 1825 /* identifier */ return this.identifier == null ? new Base[0] 1826 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1827 case 3575610: 1828 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1829 case -1532328299: 1830 /* coverageArea */ return this.coverageArea == null ? new Base[0] 1831 : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 1832 case 1843485230: 1833 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 1834 case 878344405: 1835 /* generalCost */ return this.generalCost == null ? new Base[0] 1836 : this.generalCost.toArray(new Base[this.generalCost.size()]); // InsurancePlanPlanGeneralCostComponent 1837 case -1205656545: 1838 /* specificCost */ return this.specificCost == null ? new Base[0] 1839 : this.specificCost.toArray(new Base[this.specificCost.size()]); // InsurancePlanPlanSpecificCostComponent 1840 default: 1841 return super.getProperty(hash, name, checkValid); 1842 } 1843 1844 } 1845 1846 @Override 1847 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1848 switch (hash) { 1849 case -1618432855: // identifier 1850 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1851 return value; 1852 case 3575610: // type 1853 this.type = castToCodeableConcept(value); // CodeableConcept 1854 return value; 1855 case -1532328299: // coverageArea 1856 this.getCoverageArea().add(castToReference(value)); // Reference 1857 return value; 1858 case 1843485230: // network 1859 this.getNetwork().add(castToReference(value)); // Reference 1860 return value; 1861 case 878344405: // generalCost 1862 this.getGeneralCost().add((InsurancePlanPlanGeneralCostComponent) value); // InsurancePlanPlanGeneralCostComponent 1863 return value; 1864 case -1205656545: // specificCost 1865 this.getSpecificCost().add((InsurancePlanPlanSpecificCostComponent) value); // InsurancePlanPlanSpecificCostComponent 1866 return value; 1867 default: 1868 return super.setProperty(hash, name, value); 1869 } 1870 1871 } 1872 1873 @Override 1874 public Base setProperty(String name, Base value) throws FHIRException { 1875 if (name.equals("identifier")) { 1876 this.getIdentifier().add(castToIdentifier(value)); 1877 } else if (name.equals("type")) { 1878 this.type = castToCodeableConcept(value); // CodeableConcept 1879 } else if (name.equals("coverageArea")) { 1880 this.getCoverageArea().add(castToReference(value)); 1881 } else if (name.equals("network")) { 1882 this.getNetwork().add(castToReference(value)); 1883 } else if (name.equals("generalCost")) { 1884 this.getGeneralCost().add((InsurancePlanPlanGeneralCostComponent) value); 1885 } else if (name.equals("specificCost")) { 1886 this.getSpecificCost().add((InsurancePlanPlanSpecificCostComponent) value); 1887 } else 1888 return super.setProperty(name, value); 1889 return value; 1890 } 1891 1892 @Override 1893 public Base makeProperty(int hash, String name) throws FHIRException { 1894 switch (hash) { 1895 case -1618432855: 1896 return addIdentifier(); 1897 case 3575610: 1898 return getType(); 1899 case -1532328299: 1900 return addCoverageArea(); 1901 case 1843485230: 1902 return addNetwork(); 1903 case 878344405: 1904 return addGeneralCost(); 1905 case -1205656545: 1906 return addSpecificCost(); 1907 default: 1908 return super.makeProperty(hash, name); 1909 } 1910 1911 } 1912 1913 @Override 1914 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1915 switch (hash) { 1916 case -1618432855: 1917 /* identifier */ return new String[] { "Identifier" }; 1918 case 3575610: 1919 /* type */ return new String[] { "CodeableConcept" }; 1920 case -1532328299: 1921 /* coverageArea */ return new String[] { "Reference" }; 1922 case 1843485230: 1923 /* network */ return new String[] { "Reference" }; 1924 case 878344405: 1925 /* generalCost */ return new String[] {}; 1926 case -1205656545: 1927 /* specificCost */ return new String[] {}; 1928 default: 1929 return super.getTypesForProperty(hash, name); 1930 } 1931 1932 } 1933 1934 @Override 1935 public Base addChild(String name) throws FHIRException { 1936 if (name.equals("identifier")) { 1937 return addIdentifier(); 1938 } else if (name.equals("type")) { 1939 this.type = new CodeableConcept(); 1940 return this.type; 1941 } else if (name.equals("coverageArea")) { 1942 return addCoverageArea(); 1943 } else if (name.equals("network")) { 1944 return addNetwork(); 1945 } else if (name.equals("generalCost")) { 1946 return addGeneralCost(); 1947 } else if (name.equals("specificCost")) { 1948 return addSpecificCost(); 1949 } else 1950 return super.addChild(name); 1951 } 1952 1953 public InsurancePlanPlanComponent copy() { 1954 InsurancePlanPlanComponent dst = new InsurancePlanPlanComponent(); 1955 copyValues(dst); 1956 return dst; 1957 } 1958 1959 public void copyValues(InsurancePlanPlanComponent dst) { 1960 super.copyValues(dst); 1961 if (identifier != null) { 1962 dst.identifier = new ArrayList<Identifier>(); 1963 for (Identifier i : identifier) 1964 dst.identifier.add(i.copy()); 1965 } 1966 ; 1967 dst.type = type == null ? null : type.copy(); 1968 if (coverageArea != null) { 1969 dst.coverageArea = new ArrayList<Reference>(); 1970 for (Reference i : coverageArea) 1971 dst.coverageArea.add(i.copy()); 1972 } 1973 ; 1974 if (network != null) { 1975 dst.network = new ArrayList<Reference>(); 1976 for (Reference i : network) 1977 dst.network.add(i.copy()); 1978 } 1979 ; 1980 if (generalCost != null) { 1981 dst.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1982 for (InsurancePlanPlanGeneralCostComponent i : generalCost) 1983 dst.generalCost.add(i.copy()); 1984 } 1985 ; 1986 if (specificCost != null) { 1987 dst.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1988 for (InsurancePlanPlanSpecificCostComponent i : specificCost) 1989 dst.specificCost.add(i.copy()); 1990 } 1991 ; 1992 } 1993 1994 @Override 1995 public boolean equalsDeep(Base other_) { 1996 if (!super.equalsDeep(other_)) 1997 return false; 1998 if (!(other_ instanceof InsurancePlanPlanComponent)) 1999 return false; 2000 InsurancePlanPlanComponent o = (InsurancePlanPlanComponent) other_; 2001 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 2002 && compareDeep(coverageArea, o.coverageArea, true) && compareDeep(network, o.network, true) 2003 && compareDeep(generalCost, o.generalCost, true) && compareDeep(specificCost, o.specificCost, true); 2004 } 2005 2006 @Override 2007 public boolean equalsShallow(Base other_) { 2008 if (!super.equalsShallow(other_)) 2009 return false; 2010 if (!(other_ instanceof InsurancePlanPlanComponent)) 2011 return false; 2012 InsurancePlanPlanComponent o = (InsurancePlanPlanComponent) other_; 2013 return true; 2014 } 2015 2016 public boolean isEmpty() { 2017 return super.isEmpty() 2018 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coverageArea, network, generalCost, specificCost); 2019 } 2020 2021 public String fhirType() { 2022 return "InsurancePlan.plan"; 2023 2024 } 2025 2026 } 2027 2028 @Block() 2029 public static class InsurancePlanPlanGeneralCostComponent extends BackboneElement implements IBaseBackboneElement { 2030 /** 2031 * Type of cost. 2032 */ 2033 @Child(name = "type", type = { 2034 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2035 @Description(shortDefinition = "Type of cost", formalDefinition = "Type of cost.") 2036 protected CodeableConcept type; 2037 2038 /** 2039 * Number of participants enrolled in the plan. 2040 */ 2041 @Child(name = "groupSize", type = { 2042 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2043 @Description(shortDefinition = "Number of enrollees", formalDefinition = "Number of participants enrolled in the plan.") 2044 protected PositiveIntType groupSize; 2045 2046 /** 2047 * Value of the cost. 2048 */ 2049 @Child(name = "cost", type = { Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2050 @Description(shortDefinition = "Cost value", formalDefinition = "Value of the cost.") 2051 protected Money cost; 2052 2053 /** 2054 * Additional information about the general costs associated with this plan. 2055 */ 2056 @Child(name = "comment", type = { 2057 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2058 @Description(shortDefinition = "Additional cost information", formalDefinition = "Additional information about the general costs associated with this plan.") 2059 protected StringType comment; 2060 2061 private static final long serialVersionUID = 1563949866L; 2062 2063 /** 2064 * Constructor 2065 */ 2066 public InsurancePlanPlanGeneralCostComponent() { 2067 super(); 2068 } 2069 2070 /** 2071 * @return {@link #type} (Type of cost.) 2072 */ 2073 public CodeableConcept getType() { 2074 if (this.type == null) 2075 if (Configuration.errorOnAutoCreate()) 2076 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.type"); 2077 else if (Configuration.doAutoCreate()) 2078 this.type = new CodeableConcept(); // cc 2079 return this.type; 2080 } 2081 2082 public boolean hasType() { 2083 return this.type != null && !this.type.isEmpty(); 2084 } 2085 2086 /** 2087 * @param value {@link #type} (Type of cost.) 2088 */ 2089 public InsurancePlanPlanGeneralCostComponent setType(CodeableConcept value) { 2090 this.type = value; 2091 return this; 2092 } 2093 2094 /** 2095 * @return {@link #groupSize} (Number of participants enrolled in the plan.). 2096 * This is the underlying object with id, value and extensions. The 2097 * accessor "getGroupSize" gives direct access to the value 2098 */ 2099 public PositiveIntType getGroupSizeElement() { 2100 if (this.groupSize == null) 2101 if (Configuration.errorOnAutoCreate()) 2102 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.groupSize"); 2103 else if (Configuration.doAutoCreate()) 2104 this.groupSize = new PositiveIntType(); // bb 2105 return this.groupSize; 2106 } 2107 2108 public boolean hasGroupSizeElement() { 2109 return this.groupSize != null && !this.groupSize.isEmpty(); 2110 } 2111 2112 public boolean hasGroupSize() { 2113 return this.groupSize != null && !this.groupSize.isEmpty(); 2114 } 2115 2116 /** 2117 * @param value {@link #groupSize} (Number of participants enrolled in the 2118 * plan.). This is the underlying object with id, value and 2119 * extensions. The accessor "getGroupSize" gives direct access to 2120 * the value 2121 */ 2122 public InsurancePlanPlanGeneralCostComponent setGroupSizeElement(PositiveIntType value) { 2123 this.groupSize = value; 2124 return this; 2125 } 2126 2127 /** 2128 * @return Number of participants enrolled in the plan. 2129 */ 2130 public int getGroupSize() { 2131 return this.groupSize == null || this.groupSize.isEmpty() ? 0 : this.groupSize.getValue(); 2132 } 2133 2134 /** 2135 * @param value Number of participants enrolled in the plan. 2136 */ 2137 public InsurancePlanPlanGeneralCostComponent setGroupSize(int value) { 2138 if (this.groupSize == null) 2139 this.groupSize = new PositiveIntType(); 2140 this.groupSize.setValue(value); 2141 return this; 2142 } 2143 2144 /** 2145 * @return {@link #cost} (Value of the cost.) 2146 */ 2147 public Money getCost() { 2148 if (this.cost == null) 2149 if (Configuration.errorOnAutoCreate()) 2150 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.cost"); 2151 else if (Configuration.doAutoCreate()) 2152 this.cost = new Money(); // cc 2153 return this.cost; 2154 } 2155 2156 public boolean hasCost() { 2157 return this.cost != null && !this.cost.isEmpty(); 2158 } 2159 2160 /** 2161 * @param value {@link #cost} (Value of the cost.) 2162 */ 2163 public InsurancePlanPlanGeneralCostComponent setCost(Money value) { 2164 this.cost = value; 2165 return this; 2166 } 2167 2168 /** 2169 * @return {@link #comment} (Additional information about the general costs 2170 * associated with this plan.). This is the underlying object with id, 2171 * value and extensions. The accessor "getComment" gives direct access 2172 * to the value 2173 */ 2174 public StringType getCommentElement() { 2175 if (this.comment == null) 2176 if (Configuration.errorOnAutoCreate()) 2177 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.comment"); 2178 else if (Configuration.doAutoCreate()) 2179 this.comment = new StringType(); // bb 2180 return this.comment; 2181 } 2182 2183 public boolean hasCommentElement() { 2184 return this.comment != null && !this.comment.isEmpty(); 2185 } 2186 2187 public boolean hasComment() { 2188 return this.comment != null && !this.comment.isEmpty(); 2189 } 2190 2191 /** 2192 * @param value {@link #comment} (Additional information about the general costs 2193 * associated with this plan.). This is the underlying object with 2194 * id, value and extensions. The accessor "getComment" gives direct 2195 * access to the value 2196 */ 2197 public InsurancePlanPlanGeneralCostComponent setCommentElement(StringType value) { 2198 this.comment = value; 2199 return this; 2200 } 2201 2202 /** 2203 * @return Additional information about the general costs associated with this 2204 * plan. 2205 */ 2206 public String getComment() { 2207 return this.comment == null ? null : this.comment.getValue(); 2208 } 2209 2210 /** 2211 * @param value Additional information about the general costs associated with 2212 * this plan. 2213 */ 2214 public InsurancePlanPlanGeneralCostComponent setComment(String value) { 2215 if (Utilities.noString(value)) 2216 this.comment = null; 2217 else { 2218 if (this.comment == null) 2219 this.comment = new StringType(); 2220 this.comment.setValue(value); 2221 } 2222 return this; 2223 } 2224 2225 protected void listChildren(List<Property> children) { 2226 super.listChildren(children); 2227 children.add(new Property("type", "CodeableConcept", "Type of cost.", 0, 1, type)); 2228 children.add( 2229 new Property("groupSize", "positiveInt", "Number of participants enrolled in the plan.", 0, 1, groupSize)); 2230 children.add(new Property("cost", "Money", "Value of the cost.", 0, 1, cost)); 2231 children.add(new Property("comment", "string", 2232 "Additional information about the general costs associated with this plan.", 0, 1, comment)); 2233 } 2234 2235 @Override 2236 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2237 switch (_hash) { 2238 case 3575610: 2239 /* type */ return new Property("type", "CodeableConcept", "Type of cost.", 0, 1, type); 2240 case -1483017440: 2241 /* groupSize */ return new Property("groupSize", "positiveInt", "Number of participants enrolled in the plan.", 2242 0, 1, groupSize); 2243 case 3059661: 2244 /* cost */ return new Property("cost", "Money", "Value of the cost.", 0, 1, cost); 2245 case 950398559: 2246 /* comment */ return new Property("comment", "string", 2247 "Additional information about the general costs associated with this plan.", 0, 1, comment); 2248 default: 2249 return super.getNamedProperty(_hash, _name, _checkValid); 2250 } 2251 2252 } 2253 2254 @Override 2255 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2256 switch (hash) { 2257 case 3575610: 2258 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2259 case -1483017440: 2260 /* groupSize */ return this.groupSize == null ? new Base[0] : new Base[] { this.groupSize }; // PositiveIntType 2261 case 3059661: 2262 /* cost */ return this.cost == null ? new Base[0] : new Base[] { this.cost }; // Money 2263 case 950398559: 2264 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2265 default: 2266 return super.getProperty(hash, name, checkValid); 2267 } 2268 2269 } 2270 2271 @Override 2272 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2273 switch (hash) { 2274 case 3575610: // type 2275 this.type = castToCodeableConcept(value); // CodeableConcept 2276 return value; 2277 case -1483017440: // groupSize 2278 this.groupSize = castToPositiveInt(value); // PositiveIntType 2279 return value; 2280 case 3059661: // cost 2281 this.cost = castToMoney(value); // Money 2282 return value; 2283 case 950398559: // comment 2284 this.comment = castToString(value); // StringType 2285 return value; 2286 default: 2287 return super.setProperty(hash, name, value); 2288 } 2289 2290 } 2291 2292 @Override 2293 public Base setProperty(String name, Base value) throws FHIRException { 2294 if (name.equals("type")) { 2295 this.type = castToCodeableConcept(value); // CodeableConcept 2296 } else if (name.equals("groupSize")) { 2297 this.groupSize = castToPositiveInt(value); // PositiveIntType 2298 } else if (name.equals("cost")) { 2299 this.cost = castToMoney(value); // Money 2300 } else if (name.equals("comment")) { 2301 this.comment = castToString(value); // StringType 2302 } else 2303 return super.setProperty(name, value); 2304 return value; 2305 } 2306 2307 @Override 2308 public Base makeProperty(int hash, String name) throws FHIRException { 2309 switch (hash) { 2310 case 3575610: 2311 return getType(); 2312 case -1483017440: 2313 return getGroupSizeElement(); 2314 case 3059661: 2315 return getCost(); 2316 case 950398559: 2317 return getCommentElement(); 2318 default: 2319 return super.makeProperty(hash, name); 2320 } 2321 2322 } 2323 2324 @Override 2325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2326 switch (hash) { 2327 case 3575610: 2328 /* type */ return new String[] { "CodeableConcept" }; 2329 case -1483017440: 2330 /* groupSize */ return new String[] { "positiveInt" }; 2331 case 3059661: 2332 /* cost */ return new String[] { "Money" }; 2333 case 950398559: 2334 /* comment */ return new String[] { "string" }; 2335 default: 2336 return super.getTypesForProperty(hash, name); 2337 } 2338 2339 } 2340 2341 @Override 2342 public Base addChild(String name) throws FHIRException { 2343 if (name.equals("type")) { 2344 this.type = new CodeableConcept(); 2345 return this.type; 2346 } else if (name.equals("groupSize")) { 2347 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.groupSize"); 2348 } else if (name.equals("cost")) { 2349 this.cost = new Money(); 2350 return this.cost; 2351 } else if (name.equals("comment")) { 2352 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.comment"); 2353 } else 2354 return super.addChild(name); 2355 } 2356 2357 public InsurancePlanPlanGeneralCostComponent copy() { 2358 InsurancePlanPlanGeneralCostComponent dst = new InsurancePlanPlanGeneralCostComponent(); 2359 copyValues(dst); 2360 return dst; 2361 } 2362 2363 public void copyValues(InsurancePlanPlanGeneralCostComponent dst) { 2364 super.copyValues(dst); 2365 dst.type = type == null ? null : type.copy(); 2366 dst.groupSize = groupSize == null ? null : groupSize.copy(); 2367 dst.cost = cost == null ? null : cost.copy(); 2368 dst.comment = comment == null ? null : comment.copy(); 2369 } 2370 2371 @Override 2372 public boolean equalsDeep(Base other_) { 2373 if (!super.equalsDeep(other_)) 2374 return false; 2375 if (!(other_ instanceof InsurancePlanPlanGeneralCostComponent)) 2376 return false; 2377 InsurancePlanPlanGeneralCostComponent o = (InsurancePlanPlanGeneralCostComponent) other_; 2378 return compareDeep(type, o.type, true) && compareDeep(groupSize, o.groupSize, true) 2379 && compareDeep(cost, o.cost, true) && compareDeep(comment, o.comment, true); 2380 } 2381 2382 @Override 2383 public boolean equalsShallow(Base other_) { 2384 if (!super.equalsShallow(other_)) 2385 return false; 2386 if (!(other_ instanceof InsurancePlanPlanGeneralCostComponent)) 2387 return false; 2388 InsurancePlanPlanGeneralCostComponent o = (InsurancePlanPlanGeneralCostComponent) other_; 2389 return compareValues(groupSize, o.groupSize, true) && compareValues(comment, o.comment, true); 2390 } 2391 2392 public boolean isEmpty() { 2393 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, groupSize, cost, comment); 2394 } 2395 2396 public String fhirType() { 2397 return "InsurancePlan.plan.generalCost"; 2398 2399 } 2400 2401 } 2402 2403 @Block() 2404 public static class InsurancePlanPlanSpecificCostComponent extends BackboneElement implements IBaseBackboneElement { 2405 /** 2406 * General category of benefit (Medical; Dental; Vision; Drug; Mental Health; 2407 * Substance Abuse; Hospice, Home Health). 2408 */ 2409 @Child(name = "category", type = { 2410 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2411 @Description(shortDefinition = "General category of benefit", formalDefinition = "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).") 2412 protected CodeableConcept category; 2413 2414 /** 2415 * List of the specific benefits under this category of benefit. 2416 */ 2417 @Child(name = "benefit", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2418 @Description(shortDefinition = "Benefits list", formalDefinition = "List of the specific benefits under this category of benefit.") 2419 protected List<PlanBenefitComponent> benefit; 2420 2421 private static final long serialVersionUID = 922585525L; 2422 2423 /** 2424 * Constructor 2425 */ 2426 public InsurancePlanPlanSpecificCostComponent() { 2427 super(); 2428 } 2429 2430 /** 2431 * Constructor 2432 */ 2433 public InsurancePlanPlanSpecificCostComponent(CodeableConcept category) { 2434 super(); 2435 this.category = category; 2436 } 2437 2438 /** 2439 * @return {@link #category} (General category of benefit (Medical; Dental; 2440 * Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).) 2441 */ 2442 public CodeableConcept getCategory() { 2443 if (this.category == null) 2444 if (Configuration.errorOnAutoCreate()) 2445 throw new Error("Attempt to auto-create InsurancePlanPlanSpecificCostComponent.category"); 2446 else if (Configuration.doAutoCreate()) 2447 this.category = new CodeableConcept(); // cc 2448 return this.category; 2449 } 2450 2451 public boolean hasCategory() { 2452 return this.category != null && !this.category.isEmpty(); 2453 } 2454 2455 /** 2456 * @param value {@link #category} (General category of benefit (Medical; Dental; 2457 * Vision; Drug; Mental Health; Substance Abuse; Hospice, Home 2458 * Health).) 2459 */ 2460 public InsurancePlanPlanSpecificCostComponent setCategory(CodeableConcept value) { 2461 this.category = value; 2462 return this; 2463 } 2464 2465 /** 2466 * @return {@link #benefit} (List of the specific benefits under this category 2467 * of benefit.) 2468 */ 2469 public List<PlanBenefitComponent> getBenefit() { 2470 if (this.benefit == null) 2471 this.benefit = new ArrayList<PlanBenefitComponent>(); 2472 return this.benefit; 2473 } 2474 2475 /** 2476 * @return Returns a reference to <code>this</code> for easy method chaining 2477 */ 2478 public InsurancePlanPlanSpecificCostComponent setBenefit(List<PlanBenefitComponent> theBenefit) { 2479 this.benefit = theBenefit; 2480 return this; 2481 } 2482 2483 public boolean hasBenefit() { 2484 if (this.benefit == null) 2485 return false; 2486 for (PlanBenefitComponent item : this.benefit) 2487 if (!item.isEmpty()) 2488 return true; 2489 return false; 2490 } 2491 2492 public PlanBenefitComponent addBenefit() { // 3 2493 PlanBenefitComponent t = new PlanBenefitComponent(); 2494 if (this.benefit == null) 2495 this.benefit = new ArrayList<PlanBenefitComponent>(); 2496 this.benefit.add(t); 2497 return t; 2498 } 2499 2500 public InsurancePlanPlanSpecificCostComponent addBenefit(PlanBenefitComponent t) { // 3 2501 if (t == null) 2502 return this; 2503 if (this.benefit == null) 2504 this.benefit = new ArrayList<PlanBenefitComponent>(); 2505 this.benefit.add(t); 2506 return this; 2507 } 2508 2509 /** 2510 * @return The first repetition of repeating field {@link #benefit}, creating it 2511 * if it does not already exist 2512 */ 2513 public PlanBenefitComponent getBenefitFirstRep() { 2514 if (getBenefit().isEmpty()) { 2515 addBenefit(); 2516 } 2517 return getBenefit().get(0); 2518 } 2519 2520 protected void listChildren(List<Property> children) { 2521 super.listChildren(children); 2522 children.add(new Property("category", "CodeableConcept", 2523 "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).", 2524 0, 1, category)); 2525 children.add(new Property("benefit", "", "List of the specific benefits under this category of benefit.", 0, 2526 java.lang.Integer.MAX_VALUE, benefit)); 2527 } 2528 2529 @Override 2530 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2531 switch (_hash) { 2532 case 50511102: 2533 /* category */ return new Property("category", "CodeableConcept", 2534 "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).", 2535 0, 1, category); 2536 case -222710633: 2537 /* benefit */ return new Property("benefit", "", 2538 "List of the specific benefits under this category of benefit.", 0, java.lang.Integer.MAX_VALUE, benefit); 2539 default: 2540 return super.getNamedProperty(_hash, _name, _checkValid); 2541 } 2542 2543 } 2544 2545 @Override 2546 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2547 switch (hash) { 2548 case 50511102: 2549 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 2550 case -222710633: 2551 /* benefit */ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // PlanBenefitComponent 2552 default: 2553 return super.getProperty(hash, name, checkValid); 2554 } 2555 2556 } 2557 2558 @Override 2559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2560 switch (hash) { 2561 case 50511102: // category 2562 this.category = castToCodeableConcept(value); // CodeableConcept 2563 return value; 2564 case -222710633: // benefit 2565 this.getBenefit().add((PlanBenefitComponent) value); // PlanBenefitComponent 2566 return value; 2567 default: 2568 return super.setProperty(hash, name, value); 2569 } 2570 2571 } 2572 2573 @Override 2574 public Base setProperty(String name, Base value) throws FHIRException { 2575 if (name.equals("category")) { 2576 this.category = castToCodeableConcept(value); // CodeableConcept 2577 } else if (name.equals("benefit")) { 2578 this.getBenefit().add((PlanBenefitComponent) value); 2579 } else 2580 return super.setProperty(name, value); 2581 return value; 2582 } 2583 2584 @Override 2585 public Base makeProperty(int hash, String name) throws FHIRException { 2586 switch (hash) { 2587 case 50511102: 2588 return getCategory(); 2589 case -222710633: 2590 return addBenefit(); 2591 default: 2592 return super.makeProperty(hash, name); 2593 } 2594 2595 } 2596 2597 @Override 2598 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2599 switch (hash) { 2600 case 50511102: 2601 /* category */ return new String[] { "CodeableConcept" }; 2602 case -222710633: 2603 /* benefit */ return new String[] {}; 2604 default: 2605 return super.getTypesForProperty(hash, name); 2606 } 2607 2608 } 2609 2610 @Override 2611 public Base addChild(String name) throws FHIRException { 2612 if (name.equals("category")) { 2613 this.category = new CodeableConcept(); 2614 return this.category; 2615 } else if (name.equals("benefit")) { 2616 return addBenefit(); 2617 } else 2618 return super.addChild(name); 2619 } 2620 2621 public InsurancePlanPlanSpecificCostComponent copy() { 2622 InsurancePlanPlanSpecificCostComponent dst = new InsurancePlanPlanSpecificCostComponent(); 2623 copyValues(dst); 2624 return dst; 2625 } 2626 2627 public void copyValues(InsurancePlanPlanSpecificCostComponent dst) { 2628 super.copyValues(dst); 2629 dst.category = category == null ? null : category.copy(); 2630 if (benefit != null) { 2631 dst.benefit = new ArrayList<PlanBenefitComponent>(); 2632 for (PlanBenefitComponent i : benefit) 2633 dst.benefit.add(i.copy()); 2634 } 2635 ; 2636 } 2637 2638 @Override 2639 public boolean equalsDeep(Base other_) { 2640 if (!super.equalsDeep(other_)) 2641 return false; 2642 if (!(other_ instanceof InsurancePlanPlanSpecificCostComponent)) 2643 return false; 2644 InsurancePlanPlanSpecificCostComponent o = (InsurancePlanPlanSpecificCostComponent) other_; 2645 return compareDeep(category, o.category, true) && compareDeep(benefit, o.benefit, true); 2646 } 2647 2648 @Override 2649 public boolean equalsShallow(Base other_) { 2650 if (!super.equalsShallow(other_)) 2651 return false; 2652 if (!(other_ instanceof InsurancePlanPlanSpecificCostComponent)) 2653 return false; 2654 InsurancePlanPlanSpecificCostComponent o = (InsurancePlanPlanSpecificCostComponent) other_; 2655 return true; 2656 } 2657 2658 public boolean isEmpty() { 2659 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, benefit); 2660 } 2661 2662 public String fhirType() { 2663 return "InsurancePlan.plan.specificCost"; 2664 2665 } 2666 2667 } 2668 2669 @Block() 2670 public static class PlanBenefitComponent extends BackboneElement implements IBaseBackboneElement { 2671 /** 2672 * Type of specific benefit (preventative; primary care office visit; speciality 2673 * office visit; hospitalization; emergency room; urgent care). 2674 */ 2675 @Child(name = "type", type = { 2676 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2677 @Description(shortDefinition = "Type of specific benefit", formalDefinition = "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).") 2678 protected CodeableConcept type; 2679 2680 /** 2681 * List of the costs associated with a specific benefit. 2682 */ 2683 @Child(name = "cost", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2684 @Description(shortDefinition = "List of the costs", formalDefinition = "List of the costs associated with a specific benefit.") 2685 protected List<PlanBenefitCostComponent> cost; 2686 2687 private static final long serialVersionUID = 792296200L; 2688 2689 /** 2690 * Constructor 2691 */ 2692 public PlanBenefitComponent() { 2693 super(); 2694 } 2695 2696 /** 2697 * Constructor 2698 */ 2699 public PlanBenefitComponent(CodeableConcept type) { 2700 super(); 2701 this.type = type; 2702 } 2703 2704 /** 2705 * @return {@link #type} (Type of specific benefit (preventative; primary care 2706 * office visit; speciality office visit; hospitalization; emergency 2707 * room; urgent care).) 2708 */ 2709 public CodeableConcept getType() { 2710 if (this.type == null) 2711 if (Configuration.errorOnAutoCreate()) 2712 throw new Error("Attempt to auto-create PlanBenefitComponent.type"); 2713 else if (Configuration.doAutoCreate()) 2714 this.type = new CodeableConcept(); // cc 2715 return this.type; 2716 } 2717 2718 public boolean hasType() { 2719 return this.type != null && !this.type.isEmpty(); 2720 } 2721 2722 /** 2723 * @param value {@link #type} (Type of specific benefit (preventative; primary 2724 * care office visit; speciality office visit; hospitalization; 2725 * emergency room; urgent care).) 2726 */ 2727 public PlanBenefitComponent setType(CodeableConcept value) { 2728 this.type = value; 2729 return this; 2730 } 2731 2732 /** 2733 * @return {@link #cost} (List of the costs associated with a specific benefit.) 2734 */ 2735 public List<PlanBenefitCostComponent> getCost() { 2736 if (this.cost == null) 2737 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2738 return this.cost; 2739 } 2740 2741 /** 2742 * @return Returns a reference to <code>this</code> for easy method chaining 2743 */ 2744 public PlanBenefitComponent setCost(List<PlanBenefitCostComponent> theCost) { 2745 this.cost = theCost; 2746 return this; 2747 } 2748 2749 public boolean hasCost() { 2750 if (this.cost == null) 2751 return false; 2752 for (PlanBenefitCostComponent item : this.cost) 2753 if (!item.isEmpty()) 2754 return true; 2755 return false; 2756 } 2757 2758 public PlanBenefitCostComponent addCost() { // 3 2759 PlanBenefitCostComponent t = new PlanBenefitCostComponent(); 2760 if (this.cost == null) 2761 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2762 this.cost.add(t); 2763 return t; 2764 } 2765 2766 public PlanBenefitComponent addCost(PlanBenefitCostComponent t) { // 3 2767 if (t == null) 2768 return this; 2769 if (this.cost == null) 2770 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2771 this.cost.add(t); 2772 return this; 2773 } 2774 2775 /** 2776 * @return The first repetition of repeating field {@link #cost}, creating it if 2777 * it does not already exist 2778 */ 2779 public PlanBenefitCostComponent getCostFirstRep() { 2780 if (getCost().isEmpty()) { 2781 addCost(); 2782 } 2783 return getCost().get(0); 2784 } 2785 2786 protected void listChildren(List<Property> children) { 2787 super.listChildren(children); 2788 children.add(new Property("type", "CodeableConcept", 2789 "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).", 2790 0, 1, type)); 2791 children.add(new Property("cost", "", "List of the costs associated with a specific benefit.", 0, 2792 java.lang.Integer.MAX_VALUE, cost)); 2793 } 2794 2795 @Override 2796 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2797 switch (_hash) { 2798 case 3575610: 2799 /* type */ return new Property("type", "CodeableConcept", 2800 "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).", 2801 0, 1, type); 2802 case 3059661: 2803 /* cost */ return new Property("cost", "", "List of the costs associated with a specific benefit.", 0, 2804 java.lang.Integer.MAX_VALUE, cost); 2805 default: 2806 return super.getNamedProperty(_hash, _name, _checkValid); 2807 } 2808 2809 } 2810 2811 @Override 2812 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2813 switch (hash) { 2814 case 3575610: 2815 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2816 case 3059661: 2817 /* cost */ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // PlanBenefitCostComponent 2818 default: 2819 return super.getProperty(hash, name, checkValid); 2820 } 2821 2822 } 2823 2824 @Override 2825 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2826 switch (hash) { 2827 case 3575610: // type 2828 this.type = castToCodeableConcept(value); // CodeableConcept 2829 return value; 2830 case 3059661: // cost 2831 this.getCost().add((PlanBenefitCostComponent) value); // PlanBenefitCostComponent 2832 return value; 2833 default: 2834 return super.setProperty(hash, name, value); 2835 } 2836 2837 } 2838 2839 @Override 2840 public Base setProperty(String name, Base value) throws FHIRException { 2841 if (name.equals("type")) { 2842 this.type = castToCodeableConcept(value); // CodeableConcept 2843 } else if (name.equals("cost")) { 2844 this.getCost().add((PlanBenefitCostComponent) value); 2845 } else 2846 return super.setProperty(name, value); 2847 return value; 2848 } 2849 2850 @Override 2851 public Base makeProperty(int hash, String name) throws FHIRException { 2852 switch (hash) { 2853 case 3575610: 2854 return getType(); 2855 case 3059661: 2856 return addCost(); 2857 default: 2858 return super.makeProperty(hash, name); 2859 } 2860 2861 } 2862 2863 @Override 2864 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2865 switch (hash) { 2866 case 3575610: 2867 /* type */ return new String[] { "CodeableConcept" }; 2868 case 3059661: 2869 /* cost */ return new String[] {}; 2870 default: 2871 return super.getTypesForProperty(hash, name); 2872 } 2873 2874 } 2875 2876 @Override 2877 public Base addChild(String name) throws FHIRException { 2878 if (name.equals("type")) { 2879 this.type = new CodeableConcept(); 2880 return this.type; 2881 } else if (name.equals("cost")) { 2882 return addCost(); 2883 } else 2884 return super.addChild(name); 2885 } 2886 2887 public PlanBenefitComponent copy() { 2888 PlanBenefitComponent dst = new PlanBenefitComponent(); 2889 copyValues(dst); 2890 return dst; 2891 } 2892 2893 public void copyValues(PlanBenefitComponent dst) { 2894 super.copyValues(dst); 2895 dst.type = type == null ? null : type.copy(); 2896 if (cost != null) { 2897 dst.cost = new ArrayList<PlanBenefitCostComponent>(); 2898 for (PlanBenefitCostComponent i : cost) 2899 dst.cost.add(i.copy()); 2900 } 2901 ; 2902 } 2903 2904 @Override 2905 public boolean equalsDeep(Base other_) { 2906 if (!super.equalsDeep(other_)) 2907 return false; 2908 if (!(other_ instanceof PlanBenefitComponent)) 2909 return false; 2910 PlanBenefitComponent o = (PlanBenefitComponent) other_; 2911 return compareDeep(type, o.type, true) && compareDeep(cost, o.cost, true); 2912 } 2913 2914 @Override 2915 public boolean equalsShallow(Base other_) { 2916 if (!super.equalsShallow(other_)) 2917 return false; 2918 if (!(other_ instanceof PlanBenefitComponent)) 2919 return false; 2920 PlanBenefitComponent o = (PlanBenefitComponent) other_; 2921 return true; 2922 } 2923 2924 public boolean isEmpty() { 2925 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, cost); 2926 } 2927 2928 public String fhirType() { 2929 return "InsurancePlan.plan.specificCost.benefit"; 2930 2931 } 2932 2933 } 2934 2935 @Block() 2936 public static class PlanBenefitCostComponent extends BackboneElement implements IBaseBackboneElement { 2937 /** 2938 * Type of cost (copay; individual cap; family cap; coinsurance; deductible). 2939 */ 2940 @Child(name = "type", type = { 2941 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2942 @Description(shortDefinition = "Type of cost", formalDefinition = "Type of cost (copay; individual cap; family cap; coinsurance; deductible).") 2943 protected CodeableConcept type; 2944 2945 /** 2946 * Whether the cost applies to in-network or out-of-network providers 2947 * (in-network; out-of-network; other). 2948 */ 2949 @Child(name = "applicability", type = { 2950 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2951 @Description(shortDefinition = "in-network | out-of-network | other", formalDefinition = "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).") 2952 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/insuranceplan-applicability") 2953 protected CodeableConcept applicability; 2954 2955 /** 2956 * Additional information about the cost, such as information about funding 2957 * sources (e.g. HSA, HRA, FSA, RRA). 2958 */ 2959 @Child(name = "qualifiers", type = { 2960 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2961 @Description(shortDefinition = "Additional information about the cost", formalDefinition = "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).") 2962 protected List<CodeableConcept> qualifiers; 2963 2964 /** 2965 * The actual cost value. (some of the costs may be represented as percentages 2966 * rather than currency, e.g. 10% coinsurance). 2967 */ 2968 @Child(name = "value", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2969 @Description(shortDefinition = "The actual cost value", formalDefinition = "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).") 2970 protected Quantity value; 2971 2972 private static final long serialVersionUID = -340688733L; 2973 2974 /** 2975 * Constructor 2976 */ 2977 public PlanBenefitCostComponent() { 2978 super(); 2979 } 2980 2981 /** 2982 * Constructor 2983 */ 2984 public PlanBenefitCostComponent(CodeableConcept type) { 2985 super(); 2986 this.type = type; 2987 } 2988 2989 /** 2990 * @return {@link #type} (Type of cost (copay; individual cap; family cap; 2991 * coinsurance; deductible).) 2992 */ 2993 public CodeableConcept getType() { 2994 if (this.type == null) 2995 if (Configuration.errorOnAutoCreate()) 2996 throw new Error("Attempt to auto-create PlanBenefitCostComponent.type"); 2997 else if (Configuration.doAutoCreate()) 2998 this.type = new CodeableConcept(); // cc 2999 return this.type; 3000 } 3001 3002 public boolean hasType() { 3003 return this.type != null && !this.type.isEmpty(); 3004 } 3005 3006 /** 3007 * @param value {@link #type} (Type of cost (copay; individual cap; family cap; 3008 * coinsurance; deductible).) 3009 */ 3010 public PlanBenefitCostComponent setType(CodeableConcept value) { 3011 this.type = value; 3012 return this; 3013 } 3014 3015 /** 3016 * @return {@link #applicability} (Whether the cost applies to in-network or 3017 * out-of-network providers (in-network; out-of-network; other).) 3018 */ 3019 public CodeableConcept getApplicability() { 3020 if (this.applicability == null) 3021 if (Configuration.errorOnAutoCreate()) 3022 throw new Error("Attempt to auto-create PlanBenefitCostComponent.applicability"); 3023 else if (Configuration.doAutoCreate()) 3024 this.applicability = new CodeableConcept(); // cc 3025 return this.applicability; 3026 } 3027 3028 public boolean hasApplicability() { 3029 return this.applicability != null && !this.applicability.isEmpty(); 3030 } 3031 3032 /** 3033 * @param value {@link #applicability} (Whether the cost applies to in-network 3034 * or out-of-network providers (in-network; out-of-network; 3035 * other).) 3036 */ 3037 public PlanBenefitCostComponent setApplicability(CodeableConcept value) { 3038 this.applicability = value; 3039 return this; 3040 } 3041 3042 /** 3043 * @return {@link #qualifiers} (Additional information about the cost, such as 3044 * information about funding sources (e.g. HSA, HRA, FSA, RRA).) 3045 */ 3046 public List<CodeableConcept> getQualifiers() { 3047 if (this.qualifiers == null) 3048 this.qualifiers = new ArrayList<CodeableConcept>(); 3049 return this.qualifiers; 3050 } 3051 3052 /** 3053 * @return Returns a reference to <code>this</code> for easy method chaining 3054 */ 3055 public PlanBenefitCostComponent setQualifiers(List<CodeableConcept> theQualifiers) { 3056 this.qualifiers = theQualifiers; 3057 return this; 3058 } 3059 3060 public boolean hasQualifiers() { 3061 if (this.qualifiers == null) 3062 return false; 3063 for (CodeableConcept item : this.qualifiers) 3064 if (!item.isEmpty()) 3065 return true; 3066 return false; 3067 } 3068 3069 public CodeableConcept addQualifiers() { // 3 3070 CodeableConcept t = new CodeableConcept(); 3071 if (this.qualifiers == null) 3072 this.qualifiers = new ArrayList<CodeableConcept>(); 3073 this.qualifiers.add(t); 3074 return t; 3075 } 3076 3077 public PlanBenefitCostComponent addQualifiers(CodeableConcept t) { // 3 3078 if (t == null) 3079 return this; 3080 if (this.qualifiers == null) 3081 this.qualifiers = new ArrayList<CodeableConcept>(); 3082 this.qualifiers.add(t); 3083 return this; 3084 } 3085 3086 /** 3087 * @return The first repetition of repeating field {@link #qualifiers}, creating 3088 * it if it does not already exist 3089 */ 3090 public CodeableConcept getQualifiersFirstRep() { 3091 if (getQualifiers().isEmpty()) { 3092 addQualifiers(); 3093 } 3094 return getQualifiers().get(0); 3095 } 3096 3097 /** 3098 * @return {@link #value} (The actual cost value. (some of the costs may be 3099 * represented as percentages rather than currency, e.g. 10% 3100 * coinsurance).) 3101 */ 3102 public Quantity getValue() { 3103 if (this.value == null) 3104 if (Configuration.errorOnAutoCreate()) 3105 throw new Error("Attempt to auto-create PlanBenefitCostComponent.value"); 3106 else if (Configuration.doAutoCreate()) 3107 this.value = new Quantity(); // cc 3108 return this.value; 3109 } 3110 3111 public boolean hasValue() { 3112 return this.value != null && !this.value.isEmpty(); 3113 } 3114 3115 /** 3116 * @param value {@link #value} (The actual cost value. (some of the costs may be 3117 * represented as percentages rather than currency, e.g. 10% 3118 * coinsurance).) 3119 */ 3120 public PlanBenefitCostComponent setValue(Quantity value) { 3121 this.value = value; 3122 return this; 3123 } 3124 3125 protected void listChildren(List<Property> children) { 3126 super.listChildren(children); 3127 children.add(new Property("type", "CodeableConcept", 3128 "Type of cost (copay; individual cap; family cap; coinsurance; deductible).", 0, 1, type)); 3129 children.add(new Property("applicability", "CodeableConcept", 3130 "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).", 0, 3131 1, applicability)); 3132 children.add(new Property("qualifiers", "CodeableConcept", 3133 "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).", 3134 0, java.lang.Integer.MAX_VALUE, qualifiers)); 3135 children.add(new Property("value", "Quantity", 3136 "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).", 3137 0, 1, value)); 3138 } 3139 3140 @Override 3141 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3142 switch (_hash) { 3143 case 3575610: 3144 /* type */ return new Property("type", "CodeableConcept", 3145 "Type of cost (copay; individual cap; family cap; coinsurance; deductible).", 0, 1, type); 3146 case -1526770491: 3147 /* applicability */ return new Property("applicability", "CodeableConcept", 3148 "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).", 3149 0, 1, applicability); 3150 case -31447799: 3151 /* qualifiers */ return new Property("qualifiers", "CodeableConcept", 3152 "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).", 3153 0, java.lang.Integer.MAX_VALUE, qualifiers); 3154 case 111972721: 3155 /* value */ return new Property("value", "Quantity", 3156 "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).", 3157 0, 1, value); 3158 default: 3159 return super.getNamedProperty(_hash, _name, _checkValid); 3160 } 3161 3162 } 3163 3164 @Override 3165 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3166 switch (hash) { 3167 case 3575610: 3168 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3169 case -1526770491: 3170 /* applicability */ return this.applicability == null ? new Base[0] : new Base[] { this.applicability }; // CodeableConcept 3171 case -31447799: 3172 /* qualifiers */ return this.qualifiers == null ? new Base[0] 3173 : this.qualifiers.toArray(new Base[this.qualifiers.size()]); // CodeableConcept 3174 case 111972721: 3175 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Quantity 3176 default: 3177 return super.getProperty(hash, name, checkValid); 3178 } 3179 3180 } 3181 3182 @Override 3183 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3184 switch (hash) { 3185 case 3575610: // type 3186 this.type = castToCodeableConcept(value); // CodeableConcept 3187 return value; 3188 case -1526770491: // applicability 3189 this.applicability = castToCodeableConcept(value); // CodeableConcept 3190 return value; 3191 case -31447799: // qualifiers 3192 this.getQualifiers().add(castToCodeableConcept(value)); // CodeableConcept 3193 return value; 3194 case 111972721: // value 3195 this.value = castToQuantity(value); // Quantity 3196 return value; 3197 default: 3198 return super.setProperty(hash, name, value); 3199 } 3200 3201 } 3202 3203 @Override 3204 public Base setProperty(String name, Base value) throws FHIRException { 3205 if (name.equals("type")) { 3206 this.type = castToCodeableConcept(value); // CodeableConcept 3207 } else if (name.equals("applicability")) { 3208 this.applicability = castToCodeableConcept(value); // CodeableConcept 3209 } else if (name.equals("qualifiers")) { 3210 this.getQualifiers().add(castToCodeableConcept(value)); 3211 } else if (name.equals("value")) { 3212 this.value = castToQuantity(value); // Quantity 3213 } else 3214 return super.setProperty(name, value); 3215 return value; 3216 } 3217 3218 @Override 3219 public Base makeProperty(int hash, String name) throws FHIRException { 3220 switch (hash) { 3221 case 3575610: 3222 return getType(); 3223 case -1526770491: 3224 return getApplicability(); 3225 case -31447799: 3226 return addQualifiers(); 3227 case 111972721: 3228 return getValue(); 3229 default: 3230 return super.makeProperty(hash, name); 3231 } 3232 3233 } 3234 3235 @Override 3236 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3237 switch (hash) { 3238 case 3575610: 3239 /* type */ return new String[] { "CodeableConcept" }; 3240 case -1526770491: 3241 /* applicability */ return new String[] { "CodeableConcept" }; 3242 case -31447799: 3243 /* qualifiers */ return new String[] { "CodeableConcept" }; 3244 case 111972721: 3245 /* value */ return new String[] { "Quantity" }; 3246 default: 3247 return super.getTypesForProperty(hash, name); 3248 } 3249 3250 } 3251 3252 @Override 3253 public Base addChild(String name) throws FHIRException { 3254 if (name.equals("type")) { 3255 this.type = new CodeableConcept(); 3256 return this.type; 3257 } else if (name.equals("applicability")) { 3258 this.applicability = new CodeableConcept(); 3259 return this.applicability; 3260 } else if (name.equals("qualifiers")) { 3261 return addQualifiers(); 3262 } else if (name.equals("value")) { 3263 this.value = new Quantity(); 3264 return this.value; 3265 } else 3266 return super.addChild(name); 3267 } 3268 3269 public PlanBenefitCostComponent copy() { 3270 PlanBenefitCostComponent dst = new PlanBenefitCostComponent(); 3271 copyValues(dst); 3272 return dst; 3273 } 3274 3275 public void copyValues(PlanBenefitCostComponent dst) { 3276 super.copyValues(dst); 3277 dst.type = type == null ? null : type.copy(); 3278 dst.applicability = applicability == null ? null : applicability.copy(); 3279 if (qualifiers != null) { 3280 dst.qualifiers = new ArrayList<CodeableConcept>(); 3281 for (CodeableConcept i : qualifiers) 3282 dst.qualifiers.add(i.copy()); 3283 } 3284 ; 3285 dst.value = value == null ? null : value.copy(); 3286 } 3287 3288 @Override 3289 public boolean equalsDeep(Base other_) { 3290 if (!super.equalsDeep(other_)) 3291 return false; 3292 if (!(other_ instanceof PlanBenefitCostComponent)) 3293 return false; 3294 PlanBenefitCostComponent o = (PlanBenefitCostComponent) other_; 3295 return compareDeep(type, o.type, true) && compareDeep(applicability, o.applicability, true) 3296 && compareDeep(qualifiers, o.qualifiers, true) && compareDeep(value, o.value, true); 3297 } 3298 3299 @Override 3300 public boolean equalsShallow(Base other_) { 3301 if (!super.equalsShallow(other_)) 3302 return false; 3303 if (!(other_ instanceof PlanBenefitCostComponent)) 3304 return false; 3305 PlanBenefitCostComponent o = (PlanBenefitCostComponent) other_; 3306 return true; 3307 } 3308 3309 public boolean isEmpty() { 3310 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, applicability, qualifiers, value); 3311 } 3312 3313 public String fhirType() { 3314 return "InsurancePlan.plan.specificCost.benefit.cost"; 3315 3316 } 3317 3318 } 3319 3320 /** 3321 * Business identifiers assigned to this health insurance product which remain 3322 * constant as the resource is updated and propagates from server to server. 3323 */ 3324 @Child(name = "identifier", type = { 3325 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3326 @Description(shortDefinition = "Business Identifier for Product", formalDefinition = "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.") 3327 protected List<Identifier> identifier; 3328 3329 /** 3330 * The current state of the health insurance product. 3331 */ 3332 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 3333 @Description(shortDefinition = "draft | active | retired | unknown", formalDefinition = "The current state of the health insurance product.") 3334 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/publication-status") 3335 protected Enumeration<PublicationStatus> status; 3336 3337 /** 3338 * The kind of health insurance product. 3339 */ 3340 @Child(name = "type", type = { 3341 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3342 @Description(shortDefinition = "Kind of product", formalDefinition = "The kind of health insurance product.") 3343 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/insuranceplan-type") 3344 protected List<CodeableConcept> type; 3345 3346 /** 3347 * Official name of the health insurance product as designated by the owner. 3348 */ 3349 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3350 @Description(shortDefinition = "Official name", formalDefinition = "Official name of the health insurance product as designated by the owner.") 3351 protected StringType name; 3352 3353 /** 3354 * A list of alternate names that the product is known as, or was known as in 3355 * the past. 3356 */ 3357 @Child(name = "alias", type = { 3358 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3359 @Description(shortDefinition = "Alternate names", formalDefinition = "A list of alternate names that the product is known as, or was known as in the past.") 3360 protected List<StringType> alias; 3361 3362 /** 3363 * The period of time that the health insurance product is available. 3364 */ 3365 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3366 @Description(shortDefinition = "When the product is available", formalDefinition = "The period of time that the health insurance product is available.") 3367 protected Period period; 3368 3369 /** 3370 * The entity that is providing the health insurance product and underwriting 3371 * the risk. This is typically an insurance carriers, other third-party payers, 3372 * or health plan sponsors comonly referred to as 'payers'. 3373 */ 3374 @Child(name = "ownedBy", type = { Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3375 @Description(shortDefinition = "Plan issuer", formalDefinition = "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.") 3376 protected Reference ownedBy; 3377 3378 /** 3379 * The actual object that is the target of the reference (The entity that is 3380 * providing the health insurance product and underwriting the risk. This is 3381 * typically an insurance carriers, other third-party payers, or health plan 3382 * sponsors comonly referred to as 'payers'.) 3383 */ 3384 protected Organization ownedByTarget; 3385 3386 /** 3387 * An organization which administer other services such as underwriting, 3388 * customer service and/or claims processing on behalf of the health insurance 3389 * product owner. 3390 */ 3391 @Child(name = "administeredBy", type = { 3392 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3393 @Description(shortDefinition = "Product administrator", formalDefinition = "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.") 3394 protected Reference administeredBy; 3395 3396 /** 3397 * The actual object that is the target of the reference (An organization which 3398 * administer other services such as underwriting, customer service and/or 3399 * claims processing on behalf of the health insurance product owner.) 3400 */ 3401 protected Organization administeredByTarget; 3402 3403 /** 3404 * The geographic region in which a health insurance product's benefits apply. 3405 */ 3406 @Child(name = "coverageArea", type = { 3407 Location.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3408 @Description(shortDefinition = "Where product applies", formalDefinition = "The geographic region in which a health insurance product's benefits apply.") 3409 protected List<Reference> coverageArea; 3410 /** 3411 * The actual objects that are the target of the reference (The geographic 3412 * region in which a health insurance product's benefits apply.) 3413 */ 3414 protected List<Location> coverageAreaTarget; 3415 3416 /** 3417 * The contact for the health insurance product for a certain purpose. 3418 */ 3419 @Child(name = "contact", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3420 @Description(shortDefinition = "Contact for the product", formalDefinition = "The contact for the health insurance product for a certain purpose.") 3421 protected List<InsurancePlanContactComponent> contact; 3422 3423 /** 3424 * The technical endpoints providing access to services operated for the health 3425 * insurance product. 3426 */ 3427 @Child(name = "endpoint", type = { 3428 Endpoint.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3429 @Description(shortDefinition = "Technical endpoint", formalDefinition = "The technical endpoints providing access to services operated for the health insurance product.") 3430 protected List<Reference> endpoint; 3431 /** 3432 * The actual objects that are the target of the reference (The technical 3433 * endpoints providing access to services operated for the health insurance 3434 * product.) 3435 */ 3436 protected List<Endpoint> endpointTarget; 3437 3438 /** 3439 * Reference to the network included in the health insurance product. 3440 */ 3441 @Child(name = "network", type = { 3442 Organization.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3443 @Description(shortDefinition = "What networks are Included", formalDefinition = "Reference to the network included in the health insurance product.") 3444 protected List<Reference> network; 3445 /** 3446 * The actual objects that are the target of the reference (Reference to the 3447 * network included in the health insurance product.) 3448 */ 3449 protected List<Organization> networkTarget; 3450 3451 /** 3452 * Details about the coverage offered by the insurance product. 3453 */ 3454 @Child(name = "coverage", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3455 @Description(shortDefinition = "Coverage details", formalDefinition = "Details about the coverage offered by the insurance product.") 3456 protected List<InsurancePlanCoverageComponent> coverage; 3457 3458 /** 3459 * Details about an insurance plan. 3460 */ 3461 @Child(name = "plan", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3462 @Description(shortDefinition = "Plan details", formalDefinition = "Details about an insurance plan.") 3463 protected List<InsurancePlanPlanComponent> plan; 3464 3465 private static final long serialVersionUID = -1910594688L; 3466 3467 /** 3468 * Constructor 3469 */ 3470 public InsurancePlan() { 3471 super(); 3472 } 3473 3474 /** 3475 * @return {@link #identifier} (Business identifiers assigned to this health 3476 * insurance product which remain constant as the resource is updated 3477 * and propagates from server to server.) 3478 */ 3479 public List<Identifier> getIdentifier() { 3480 if (this.identifier == null) 3481 this.identifier = new ArrayList<Identifier>(); 3482 return this.identifier; 3483 } 3484 3485 /** 3486 * @return Returns a reference to <code>this</code> for easy method chaining 3487 */ 3488 public InsurancePlan setIdentifier(List<Identifier> theIdentifier) { 3489 this.identifier = theIdentifier; 3490 return this; 3491 } 3492 3493 public boolean hasIdentifier() { 3494 if (this.identifier == null) 3495 return false; 3496 for (Identifier item : this.identifier) 3497 if (!item.isEmpty()) 3498 return true; 3499 return false; 3500 } 3501 3502 public Identifier addIdentifier() { // 3 3503 Identifier t = new Identifier(); 3504 if (this.identifier == null) 3505 this.identifier = new ArrayList<Identifier>(); 3506 this.identifier.add(t); 3507 return t; 3508 } 3509 3510 public InsurancePlan addIdentifier(Identifier t) { // 3 3511 if (t == null) 3512 return this; 3513 if (this.identifier == null) 3514 this.identifier = new ArrayList<Identifier>(); 3515 this.identifier.add(t); 3516 return this; 3517 } 3518 3519 /** 3520 * @return The first repetition of repeating field {@link #identifier}, creating 3521 * it if it does not already exist 3522 */ 3523 public Identifier getIdentifierFirstRep() { 3524 if (getIdentifier().isEmpty()) { 3525 addIdentifier(); 3526 } 3527 return getIdentifier().get(0); 3528 } 3529 3530 /** 3531 * @return {@link #status} (The current state of the health insurance product.). 3532 * This is the underlying object with id, value and extensions. The 3533 * accessor "getStatus" gives direct access to the value 3534 */ 3535 public Enumeration<PublicationStatus> getStatusElement() { 3536 if (this.status == null) 3537 if (Configuration.errorOnAutoCreate()) 3538 throw new Error("Attempt to auto-create InsurancePlan.status"); 3539 else if (Configuration.doAutoCreate()) 3540 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3541 return this.status; 3542 } 3543 3544 public boolean hasStatusElement() { 3545 return this.status != null && !this.status.isEmpty(); 3546 } 3547 3548 public boolean hasStatus() { 3549 return this.status != null && !this.status.isEmpty(); 3550 } 3551 3552 /** 3553 * @param value {@link #status} (The current state of the health insurance 3554 * product.). This is the underlying object with id, value and 3555 * extensions. The accessor "getStatus" gives direct access to the 3556 * value 3557 */ 3558 public InsurancePlan setStatusElement(Enumeration<PublicationStatus> value) { 3559 this.status = value; 3560 return this; 3561 } 3562 3563 /** 3564 * @return The current state of the health insurance product. 3565 */ 3566 public PublicationStatus getStatus() { 3567 return this.status == null ? null : this.status.getValue(); 3568 } 3569 3570 /** 3571 * @param value The current state of the health insurance product. 3572 */ 3573 public InsurancePlan setStatus(PublicationStatus value) { 3574 if (value == null) 3575 this.status = null; 3576 else { 3577 if (this.status == null) 3578 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3579 this.status.setValue(value); 3580 } 3581 return this; 3582 } 3583 3584 /** 3585 * @return {@link #type} (The kind of health insurance product.) 3586 */ 3587 public List<CodeableConcept> getType() { 3588 if (this.type == null) 3589 this.type = new ArrayList<CodeableConcept>(); 3590 return this.type; 3591 } 3592 3593 /** 3594 * @return Returns a reference to <code>this</code> for easy method chaining 3595 */ 3596 public InsurancePlan setType(List<CodeableConcept> theType) { 3597 this.type = theType; 3598 return this; 3599 } 3600 3601 public boolean hasType() { 3602 if (this.type == null) 3603 return false; 3604 for (CodeableConcept item : this.type) 3605 if (!item.isEmpty()) 3606 return true; 3607 return false; 3608 } 3609 3610 public CodeableConcept addType() { // 3 3611 CodeableConcept t = new CodeableConcept(); 3612 if (this.type == null) 3613 this.type = new ArrayList<CodeableConcept>(); 3614 this.type.add(t); 3615 return t; 3616 } 3617 3618 public InsurancePlan addType(CodeableConcept t) { // 3 3619 if (t == null) 3620 return this; 3621 if (this.type == null) 3622 this.type = new ArrayList<CodeableConcept>(); 3623 this.type.add(t); 3624 return this; 3625 } 3626 3627 /** 3628 * @return The first repetition of repeating field {@link #type}, creating it if 3629 * it does not already exist 3630 */ 3631 public CodeableConcept getTypeFirstRep() { 3632 if (getType().isEmpty()) { 3633 addType(); 3634 } 3635 return getType().get(0); 3636 } 3637 3638 /** 3639 * @return {@link #name} (Official name of the health insurance product as 3640 * designated by the owner.). This is the underlying object with id, 3641 * value and extensions. The accessor "getName" gives direct access to 3642 * the value 3643 */ 3644 public StringType getNameElement() { 3645 if (this.name == null) 3646 if (Configuration.errorOnAutoCreate()) 3647 throw new Error("Attempt to auto-create InsurancePlan.name"); 3648 else if (Configuration.doAutoCreate()) 3649 this.name = new StringType(); // bb 3650 return this.name; 3651 } 3652 3653 public boolean hasNameElement() { 3654 return this.name != null && !this.name.isEmpty(); 3655 } 3656 3657 public boolean hasName() { 3658 return this.name != null && !this.name.isEmpty(); 3659 } 3660 3661 /** 3662 * @param value {@link #name} (Official name of the health insurance product as 3663 * designated by the owner.). This is the underlying object with 3664 * id, value and extensions. The accessor "getName" gives direct 3665 * access to the value 3666 */ 3667 public InsurancePlan setNameElement(StringType value) { 3668 this.name = value; 3669 return this; 3670 } 3671 3672 /** 3673 * @return Official name of the health insurance product as designated by the 3674 * owner. 3675 */ 3676 public String getName() { 3677 return this.name == null ? null : this.name.getValue(); 3678 } 3679 3680 /** 3681 * @param value Official name of the health insurance product as designated by 3682 * the owner. 3683 */ 3684 public InsurancePlan setName(String value) { 3685 if (Utilities.noString(value)) 3686 this.name = null; 3687 else { 3688 if (this.name == null) 3689 this.name = new StringType(); 3690 this.name.setValue(value); 3691 } 3692 return this; 3693 } 3694 3695 /** 3696 * @return {@link #alias} (A list of alternate names that the product is known 3697 * as, or was known as in the past.) 3698 */ 3699 public List<StringType> getAlias() { 3700 if (this.alias == null) 3701 this.alias = new ArrayList<StringType>(); 3702 return this.alias; 3703 } 3704 3705 /** 3706 * @return Returns a reference to <code>this</code> for easy method chaining 3707 */ 3708 public InsurancePlan setAlias(List<StringType> theAlias) { 3709 this.alias = theAlias; 3710 return this; 3711 } 3712 3713 public boolean hasAlias() { 3714 if (this.alias == null) 3715 return false; 3716 for (StringType item : this.alias) 3717 if (!item.isEmpty()) 3718 return true; 3719 return false; 3720 } 3721 3722 /** 3723 * @return {@link #alias} (A list of alternate names that the product is known 3724 * as, or was known as in the past.) 3725 */ 3726 public StringType addAliasElement() {// 2 3727 StringType t = new StringType(); 3728 if (this.alias == null) 3729 this.alias = new ArrayList<StringType>(); 3730 this.alias.add(t); 3731 return t; 3732 } 3733 3734 /** 3735 * @param value {@link #alias} (A list of alternate names that the product is 3736 * known as, or was known as in the past.) 3737 */ 3738 public InsurancePlan addAlias(String value) { // 1 3739 StringType t = new StringType(); 3740 t.setValue(value); 3741 if (this.alias == null) 3742 this.alias = new ArrayList<StringType>(); 3743 this.alias.add(t); 3744 return this; 3745 } 3746 3747 /** 3748 * @param value {@link #alias} (A list of alternate names that the product is 3749 * known as, or was known as in the past.) 3750 */ 3751 public boolean hasAlias(String value) { 3752 if (this.alias == null) 3753 return false; 3754 for (StringType v : this.alias) 3755 if (v.getValue().equals(value)) // string 3756 return true; 3757 return false; 3758 } 3759 3760 /** 3761 * @return {@link #period} (The period of time that the health insurance product 3762 * is available.) 3763 */ 3764 public Period getPeriod() { 3765 if (this.period == null) 3766 if (Configuration.errorOnAutoCreate()) 3767 throw new Error("Attempt to auto-create InsurancePlan.period"); 3768 else if (Configuration.doAutoCreate()) 3769 this.period = new Period(); // cc 3770 return this.period; 3771 } 3772 3773 public boolean hasPeriod() { 3774 return this.period != null && !this.period.isEmpty(); 3775 } 3776 3777 /** 3778 * @param value {@link #period} (The period of time that the health insurance 3779 * product is available.) 3780 */ 3781 public InsurancePlan setPeriod(Period value) { 3782 this.period = value; 3783 return this; 3784 } 3785 3786 /** 3787 * @return {@link #ownedBy} (The entity that is providing the health insurance 3788 * product and underwriting the risk. This is typically an insurance 3789 * carriers, other third-party payers, or health plan sponsors comonly 3790 * referred to as 'payers'.) 3791 */ 3792 public Reference getOwnedBy() { 3793 if (this.ownedBy == null) 3794 if (Configuration.errorOnAutoCreate()) 3795 throw new Error("Attempt to auto-create InsurancePlan.ownedBy"); 3796 else if (Configuration.doAutoCreate()) 3797 this.ownedBy = new Reference(); // cc 3798 return this.ownedBy; 3799 } 3800 3801 public boolean hasOwnedBy() { 3802 return this.ownedBy != null && !this.ownedBy.isEmpty(); 3803 } 3804 3805 /** 3806 * @param value {@link #ownedBy} (The entity that is providing the health 3807 * insurance product and underwriting the risk. This is typically 3808 * an insurance carriers, other third-party payers, or health plan 3809 * sponsors comonly referred to as 'payers'.) 3810 */ 3811 public InsurancePlan setOwnedBy(Reference value) { 3812 this.ownedBy = value; 3813 return this; 3814 } 3815 3816 /** 3817 * @return {@link #ownedBy} The actual object that is the target of the 3818 * reference. The reference library doesn't populate this, but you can 3819 * use it to hold the resource if you resolve it. (The entity that is 3820 * providing the health insurance product and underwriting the risk. 3821 * This is typically an insurance carriers, other third-party payers, or 3822 * health plan sponsors comonly referred to as 'payers'.) 3823 */ 3824 public Organization getOwnedByTarget() { 3825 if (this.ownedByTarget == null) 3826 if (Configuration.errorOnAutoCreate()) 3827 throw new Error("Attempt to auto-create InsurancePlan.ownedBy"); 3828 else if (Configuration.doAutoCreate()) 3829 this.ownedByTarget = new Organization(); // aa 3830 return this.ownedByTarget; 3831 } 3832 3833 /** 3834 * @param value {@link #ownedBy} The actual object that is the target of the 3835 * reference. The reference library doesn't use these, but you can 3836 * use it to hold the resource if you resolve it. (The entity that 3837 * is providing the health insurance product and underwriting the 3838 * risk. This is typically an insurance carriers, other third-party 3839 * payers, or health plan sponsors comonly referred to as 3840 * 'payers'.) 3841 */ 3842 public InsurancePlan setOwnedByTarget(Organization value) { 3843 this.ownedByTarget = value; 3844 return this; 3845 } 3846 3847 /** 3848 * @return {@link #administeredBy} (An organization which administer other 3849 * services such as underwriting, customer service and/or claims 3850 * processing on behalf of the health insurance product owner.) 3851 */ 3852 public Reference getAdministeredBy() { 3853 if (this.administeredBy == null) 3854 if (Configuration.errorOnAutoCreate()) 3855 throw new Error("Attempt to auto-create InsurancePlan.administeredBy"); 3856 else if (Configuration.doAutoCreate()) 3857 this.administeredBy = new Reference(); // cc 3858 return this.administeredBy; 3859 } 3860 3861 public boolean hasAdministeredBy() { 3862 return this.administeredBy != null && !this.administeredBy.isEmpty(); 3863 } 3864 3865 /** 3866 * @param value {@link #administeredBy} (An organization which administer other 3867 * services such as underwriting, customer service and/or claims 3868 * processing on behalf of the health insurance product owner.) 3869 */ 3870 public InsurancePlan setAdministeredBy(Reference value) { 3871 this.administeredBy = value; 3872 return this; 3873 } 3874 3875 /** 3876 * @return {@link #administeredBy} The actual object that is the target of the 3877 * reference. The reference library doesn't populate this, but you can 3878 * use it to hold the resource if you resolve it. (An organization which 3879 * administer other services such as underwriting, customer service 3880 * and/or claims processing on behalf of the health insurance product 3881 * owner.) 3882 */ 3883 public Organization getAdministeredByTarget() { 3884 if (this.administeredByTarget == null) 3885 if (Configuration.errorOnAutoCreate()) 3886 throw new Error("Attempt to auto-create InsurancePlan.administeredBy"); 3887 else if (Configuration.doAutoCreate()) 3888 this.administeredByTarget = new Organization(); // aa 3889 return this.administeredByTarget; 3890 } 3891 3892 /** 3893 * @param value {@link #administeredBy} The actual object that is the target of 3894 * the reference. The reference library doesn't use these, but you 3895 * can use it to hold the resource if you resolve it. (An 3896 * organization which administer other services such as 3897 * underwriting, customer service and/or claims processing on 3898 * behalf of the health insurance product owner.) 3899 */ 3900 public InsurancePlan setAdministeredByTarget(Organization value) { 3901 this.administeredByTarget = value; 3902 return this; 3903 } 3904 3905 /** 3906 * @return {@link #coverageArea} (The geographic region in which a health 3907 * insurance product's benefits apply.) 3908 */ 3909 public List<Reference> getCoverageArea() { 3910 if (this.coverageArea == null) 3911 this.coverageArea = new ArrayList<Reference>(); 3912 return this.coverageArea; 3913 } 3914 3915 /** 3916 * @return Returns a reference to <code>this</code> for easy method chaining 3917 */ 3918 public InsurancePlan setCoverageArea(List<Reference> theCoverageArea) { 3919 this.coverageArea = theCoverageArea; 3920 return this; 3921 } 3922 3923 public boolean hasCoverageArea() { 3924 if (this.coverageArea == null) 3925 return false; 3926 for (Reference item : this.coverageArea) 3927 if (!item.isEmpty()) 3928 return true; 3929 return false; 3930 } 3931 3932 public Reference addCoverageArea() { // 3 3933 Reference t = new Reference(); 3934 if (this.coverageArea == null) 3935 this.coverageArea = new ArrayList<Reference>(); 3936 this.coverageArea.add(t); 3937 return t; 3938 } 3939 3940 public InsurancePlan addCoverageArea(Reference t) { // 3 3941 if (t == null) 3942 return this; 3943 if (this.coverageArea == null) 3944 this.coverageArea = new ArrayList<Reference>(); 3945 this.coverageArea.add(t); 3946 return this; 3947 } 3948 3949 /** 3950 * @return The first repetition of repeating field {@link #coverageArea}, 3951 * creating it if it does not already exist 3952 */ 3953 public Reference getCoverageAreaFirstRep() { 3954 if (getCoverageArea().isEmpty()) { 3955 addCoverageArea(); 3956 } 3957 return getCoverageArea().get(0); 3958 } 3959 3960 /** 3961 * @deprecated Use Reference#setResource(IBaseResource) instead 3962 */ 3963 @Deprecated 3964 public List<Location> getCoverageAreaTarget() { 3965 if (this.coverageAreaTarget == null) 3966 this.coverageAreaTarget = new ArrayList<Location>(); 3967 return this.coverageAreaTarget; 3968 } 3969 3970 /** 3971 * @deprecated Use Reference#setResource(IBaseResource) instead 3972 */ 3973 @Deprecated 3974 public Location addCoverageAreaTarget() { 3975 Location r = new Location(); 3976 if (this.coverageAreaTarget == null) 3977 this.coverageAreaTarget = new ArrayList<Location>(); 3978 this.coverageAreaTarget.add(r); 3979 return r; 3980 } 3981 3982 /** 3983 * @return {@link #contact} (The contact for the health insurance product for a 3984 * certain purpose.) 3985 */ 3986 public List<InsurancePlanContactComponent> getContact() { 3987 if (this.contact == null) 3988 this.contact = new ArrayList<InsurancePlanContactComponent>(); 3989 return this.contact; 3990 } 3991 3992 /** 3993 * @return Returns a reference to <code>this</code> for easy method chaining 3994 */ 3995 public InsurancePlan setContact(List<InsurancePlanContactComponent> theContact) { 3996 this.contact = theContact; 3997 return this; 3998 } 3999 4000 public boolean hasContact() { 4001 if (this.contact == null) 4002 return false; 4003 for (InsurancePlanContactComponent item : this.contact) 4004 if (!item.isEmpty()) 4005 return true; 4006 return false; 4007 } 4008 4009 public InsurancePlanContactComponent addContact() { // 3 4010 InsurancePlanContactComponent t = new InsurancePlanContactComponent(); 4011 if (this.contact == null) 4012 this.contact = new ArrayList<InsurancePlanContactComponent>(); 4013 this.contact.add(t); 4014 return t; 4015 } 4016 4017 public InsurancePlan addContact(InsurancePlanContactComponent t) { // 3 4018 if (t == null) 4019 return this; 4020 if (this.contact == null) 4021 this.contact = new ArrayList<InsurancePlanContactComponent>(); 4022 this.contact.add(t); 4023 return this; 4024 } 4025 4026 /** 4027 * @return The first repetition of repeating field {@link #contact}, creating it 4028 * if it does not already exist 4029 */ 4030 public InsurancePlanContactComponent getContactFirstRep() { 4031 if (getContact().isEmpty()) { 4032 addContact(); 4033 } 4034 return getContact().get(0); 4035 } 4036 4037 /** 4038 * @return {@link #endpoint} (The technical endpoints providing access to 4039 * services operated for the health insurance product.) 4040 */ 4041 public List<Reference> getEndpoint() { 4042 if (this.endpoint == null) 4043 this.endpoint = new ArrayList<Reference>(); 4044 return this.endpoint; 4045 } 4046 4047 /** 4048 * @return Returns a reference to <code>this</code> for easy method chaining 4049 */ 4050 public InsurancePlan setEndpoint(List<Reference> theEndpoint) { 4051 this.endpoint = theEndpoint; 4052 return this; 4053 } 4054 4055 public boolean hasEndpoint() { 4056 if (this.endpoint == null) 4057 return false; 4058 for (Reference item : this.endpoint) 4059 if (!item.isEmpty()) 4060 return true; 4061 return false; 4062 } 4063 4064 public Reference addEndpoint() { // 3 4065 Reference t = new Reference(); 4066 if (this.endpoint == null) 4067 this.endpoint = new ArrayList<Reference>(); 4068 this.endpoint.add(t); 4069 return t; 4070 } 4071 4072 public InsurancePlan addEndpoint(Reference t) { // 3 4073 if (t == null) 4074 return this; 4075 if (this.endpoint == null) 4076 this.endpoint = new ArrayList<Reference>(); 4077 this.endpoint.add(t); 4078 return this; 4079 } 4080 4081 /** 4082 * @return The first repetition of repeating field {@link #endpoint}, creating 4083 * it if it does not already exist 4084 */ 4085 public Reference getEndpointFirstRep() { 4086 if (getEndpoint().isEmpty()) { 4087 addEndpoint(); 4088 } 4089 return getEndpoint().get(0); 4090 } 4091 4092 /** 4093 * @deprecated Use Reference#setResource(IBaseResource) instead 4094 */ 4095 @Deprecated 4096 public List<Endpoint> getEndpointTarget() { 4097 if (this.endpointTarget == null) 4098 this.endpointTarget = new ArrayList<Endpoint>(); 4099 return this.endpointTarget; 4100 } 4101 4102 /** 4103 * @deprecated Use Reference#setResource(IBaseResource) instead 4104 */ 4105 @Deprecated 4106 public Endpoint addEndpointTarget() { 4107 Endpoint r = new Endpoint(); 4108 if (this.endpointTarget == null) 4109 this.endpointTarget = new ArrayList<Endpoint>(); 4110 this.endpointTarget.add(r); 4111 return r; 4112 } 4113 4114 /** 4115 * @return {@link #network} (Reference to the network included in the health 4116 * insurance product.) 4117 */ 4118 public List<Reference> getNetwork() { 4119 if (this.network == null) 4120 this.network = new ArrayList<Reference>(); 4121 return this.network; 4122 } 4123 4124 /** 4125 * @return Returns a reference to <code>this</code> for easy method chaining 4126 */ 4127 public InsurancePlan setNetwork(List<Reference> theNetwork) { 4128 this.network = theNetwork; 4129 return this; 4130 } 4131 4132 public boolean hasNetwork() { 4133 if (this.network == null) 4134 return false; 4135 for (Reference item : this.network) 4136 if (!item.isEmpty()) 4137 return true; 4138 return false; 4139 } 4140 4141 public Reference addNetwork() { // 3 4142 Reference t = new Reference(); 4143 if (this.network == null) 4144 this.network = new ArrayList<Reference>(); 4145 this.network.add(t); 4146 return t; 4147 } 4148 4149 public InsurancePlan addNetwork(Reference t) { // 3 4150 if (t == null) 4151 return this; 4152 if (this.network == null) 4153 this.network = new ArrayList<Reference>(); 4154 this.network.add(t); 4155 return this; 4156 } 4157 4158 /** 4159 * @return The first repetition of repeating field {@link #network}, creating it 4160 * if it does not already exist 4161 */ 4162 public Reference getNetworkFirstRep() { 4163 if (getNetwork().isEmpty()) { 4164 addNetwork(); 4165 } 4166 return getNetwork().get(0); 4167 } 4168 4169 /** 4170 * @deprecated Use Reference#setResource(IBaseResource) instead 4171 */ 4172 @Deprecated 4173 public List<Organization> getNetworkTarget() { 4174 if (this.networkTarget == null) 4175 this.networkTarget = new ArrayList<Organization>(); 4176 return this.networkTarget; 4177 } 4178 4179 /** 4180 * @deprecated Use Reference#setResource(IBaseResource) instead 4181 */ 4182 @Deprecated 4183 public Organization addNetworkTarget() { 4184 Organization r = new Organization(); 4185 if (this.networkTarget == null) 4186 this.networkTarget = new ArrayList<Organization>(); 4187 this.networkTarget.add(r); 4188 return r; 4189 } 4190 4191 /** 4192 * @return {@link #coverage} (Details about the coverage offered by the 4193 * insurance product.) 4194 */ 4195 public List<InsurancePlanCoverageComponent> getCoverage() { 4196 if (this.coverage == null) 4197 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4198 return this.coverage; 4199 } 4200 4201 /** 4202 * @return Returns a reference to <code>this</code> for easy method chaining 4203 */ 4204 public InsurancePlan setCoverage(List<InsurancePlanCoverageComponent> theCoverage) { 4205 this.coverage = theCoverage; 4206 return this; 4207 } 4208 4209 public boolean hasCoverage() { 4210 if (this.coverage == null) 4211 return false; 4212 for (InsurancePlanCoverageComponent item : this.coverage) 4213 if (!item.isEmpty()) 4214 return true; 4215 return false; 4216 } 4217 4218 public InsurancePlanCoverageComponent addCoverage() { // 3 4219 InsurancePlanCoverageComponent t = new InsurancePlanCoverageComponent(); 4220 if (this.coverage == null) 4221 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4222 this.coverage.add(t); 4223 return t; 4224 } 4225 4226 public InsurancePlan addCoverage(InsurancePlanCoverageComponent t) { // 3 4227 if (t == null) 4228 return this; 4229 if (this.coverage == null) 4230 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4231 this.coverage.add(t); 4232 return this; 4233 } 4234 4235 /** 4236 * @return The first repetition of repeating field {@link #coverage}, creating 4237 * it if it does not already exist 4238 */ 4239 public InsurancePlanCoverageComponent getCoverageFirstRep() { 4240 if (getCoverage().isEmpty()) { 4241 addCoverage(); 4242 } 4243 return getCoverage().get(0); 4244 } 4245 4246 /** 4247 * @return {@link #plan} (Details about an insurance plan.) 4248 */ 4249 public List<InsurancePlanPlanComponent> getPlan() { 4250 if (this.plan == null) 4251 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 4252 return this.plan; 4253 } 4254 4255 /** 4256 * @return Returns a reference to <code>this</code> for easy method chaining 4257 */ 4258 public InsurancePlan setPlan(List<InsurancePlanPlanComponent> thePlan) { 4259 this.plan = thePlan; 4260 return this; 4261 } 4262 4263 public boolean hasPlan() { 4264 if (this.plan == null) 4265 return false; 4266 for (InsurancePlanPlanComponent item : this.plan) 4267 if (!item.isEmpty()) 4268 return true; 4269 return false; 4270 } 4271 4272 public InsurancePlanPlanComponent addPlan() { // 3 4273 InsurancePlanPlanComponent t = new InsurancePlanPlanComponent(); 4274 if (this.plan == null) 4275 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 4276 this.plan.add(t); 4277 return t; 4278 } 4279 4280 public InsurancePlan addPlan(InsurancePlanPlanComponent t) { // 3 4281 if (t == null) 4282 return this; 4283 if (this.plan == null) 4284 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 4285 this.plan.add(t); 4286 return this; 4287 } 4288 4289 /** 4290 * @return The first repetition of repeating field {@link #plan}, creating it if 4291 * it does not already exist 4292 */ 4293 public InsurancePlanPlanComponent getPlanFirstRep() { 4294 if (getPlan().isEmpty()) { 4295 addPlan(); 4296 } 4297 return getPlan().get(0); 4298 } 4299 4300 protected void listChildren(List<Property> children) { 4301 super.listChildren(children); 4302 children.add(new Property("identifier", "Identifier", 4303 "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.", 4304 0, java.lang.Integer.MAX_VALUE, identifier)); 4305 children.add(new Property("status", "code", "The current state of the health insurance product.", 0, 1, status)); 4306 children.add(new Property("type", "CodeableConcept", "The kind of health insurance product.", 0, 4307 java.lang.Integer.MAX_VALUE, type)); 4308 children.add(new Property("name", "string", 4309 "Official name of the health insurance product as designated by the owner.", 0, 1, name)); 4310 children.add(new Property("alias", "string", 4311 "A list of alternate names that the product is known as, or was known as in the past.", 0, 4312 java.lang.Integer.MAX_VALUE, alias)); 4313 children.add(new Property("period", "Period", "The period of time that the health insurance product is available.", 4314 0, 1, period)); 4315 children.add(new Property("ownedBy", "Reference(Organization)", 4316 "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.", 4317 0, 1, ownedBy)); 4318 children.add(new Property("administeredBy", "Reference(Organization)", 4319 "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.", 4320 0, 1, administeredBy)); 4321 children.add(new Property("coverageArea", "Reference(Location)", 4322 "The geographic region in which a health insurance product's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 4323 coverageArea)); 4324 children.add(new Property("contact", "", "The contact for the health insurance product for a certain purpose.", 0, 4325 java.lang.Integer.MAX_VALUE, contact)); 4326 children.add(new Property("endpoint", "Reference(Endpoint)", 4327 "The technical endpoints providing access to services operated for the health insurance product.", 0, 4328 java.lang.Integer.MAX_VALUE, endpoint)); 4329 children.add(new Property("network", "Reference(Organization)", 4330 "Reference to the network included in the health insurance product.", 0, java.lang.Integer.MAX_VALUE, network)); 4331 children.add(new Property("coverage", "", "Details about the coverage offered by the insurance product.", 0, 4332 java.lang.Integer.MAX_VALUE, coverage)); 4333 children.add(new Property("plan", "", "Details about an insurance plan.", 0, java.lang.Integer.MAX_VALUE, plan)); 4334 } 4335 4336 @Override 4337 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4338 switch (_hash) { 4339 case -1618432855: 4340 /* identifier */ return new Property("identifier", "Identifier", 4341 "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.", 4342 0, java.lang.Integer.MAX_VALUE, identifier); 4343 case -892481550: 4344 /* status */ return new Property("status", "code", "The current state of the health insurance product.", 0, 1, 4345 status); 4346 case 3575610: 4347 /* type */ return new Property("type", "CodeableConcept", "The kind of health insurance product.", 0, 4348 java.lang.Integer.MAX_VALUE, type); 4349 case 3373707: 4350 /* name */ return new Property("name", "string", 4351 "Official name of the health insurance product as designated by the owner.", 0, 1, name); 4352 case 92902992: 4353 /* alias */ return new Property("alias", "string", 4354 "A list of alternate names that the product is known as, or was known as in the past.", 0, 4355 java.lang.Integer.MAX_VALUE, alias); 4356 case -991726143: 4357 /* period */ return new Property("period", "Period", 4358 "The period of time that the health insurance product is available.", 0, 1, period); 4359 case -1054743076: 4360 /* ownedBy */ return new Property("ownedBy", "Reference(Organization)", 4361 "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.", 4362 0, 1, ownedBy); 4363 case 898770462: 4364 /* administeredBy */ return new Property("administeredBy", "Reference(Organization)", 4365 "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.", 4366 0, 1, administeredBy); 4367 case -1532328299: 4368 /* coverageArea */ return new Property("coverageArea", "Reference(Location)", 4369 "The geographic region in which a health insurance product's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 4370 coverageArea); 4371 case 951526432: 4372 /* contact */ return new Property("contact", "", 4373 "The contact for the health insurance product for a certain purpose.", 0, java.lang.Integer.MAX_VALUE, 4374 contact); 4375 case 1741102485: 4376 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 4377 "The technical endpoints providing access to services operated for the health insurance product.", 0, 4378 java.lang.Integer.MAX_VALUE, endpoint); 4379 case 1843485230: 4380 /* network */ return new Property("network", "Reference(Organization)", 4381 "Reference to the network included in the health insurance product.", 0, java.lang.Integer.MAX_VALUE, 4382 network); 4383 case -351767064: 4384 /* coverage */ return new Property("coverage", "", "Details about the coverage offered by the insurance product.", 4385 0, java.lang.Integer.MAX_VALUE, coverage); 4386 case 3443497: 4387 /* plan */ return new Property("plan", "", "Details about an insurance plan.", 0, java.lang.Integer.MAX_VALUE, 4388 plan); 4389 default: 4390 return super.getNamedProperty(_hash, _name, _checkValid); 4391 } 4392 4393 } 4394 4395 @Override 4396 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4397 switch (hash) { 4398 case -1618432855: 4399 /* identifier */ return this.identifier == null ? new Base[0] 4400 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4401 case -892481550: 4402 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4403 case 3575610: 4404 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4405 case 3373707: 4406 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4407 case 92902992: 4408 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 4409 case -991726143: 4410 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 4411 case -1054743076: 4412 /* ownedBy */ return this.ownedBy == null ? new Base[0] : new Base[] { this.ownedBy }; // Reference 4413 case 898770462: 4414 /* administeredBy */ return this.administeredBy == null ? new Base[0] : new Base[] { this.administeredBy }; // Reference 4415 case -1532328299: 4416 /* coverageArea */ return this.coverageArea == null ? new Base[0] 4417 : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 4418 case 951526432: 4419 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // InsurancePlanContactComponent 4420 case 1741102485: 4421 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 4422 case 1843485230: 4423 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 4424 case -351767064: 4425 /* coverage */ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // InsurancePlanCoverageComponent 4426 case 3443497: 4427 /* plan */ return this.plan == null ? new Base[0] : this.plan.toArray(new Base[this.plan.size()]); // InsurancePlanPlanComponent 4428 default: 4429 return super.getProperty(hash, name, checkValid); 4430 } 4431 4432 } 4433 4434 @Override 4435 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4436 switch (hash) { 4437 case -1618432855: // identifier 4438 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4439 return value; 4440 case -892481550: // status 4441 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4442 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4443 return value; 4444 case 3575610: // type 4445 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 4446 return value; 4447 case 3373707: // name 4448 this.name = castToString(value); // StringType 4449 return value; 4450 case 92902992: // alias 4451 this.getAlias().add(castToString(value)); // StringType 4452 return value; 4453 case -991726143: // period 4454 this.period = castToPeriod(value); // Period 4455 return value; 4456 case -1054743076: // ownedBy 4457 this.ownedBy = castToReference(value); // Reference 4458 return value; 4459 case 898770462: // administeredBy 4460 this.administeredBy = castToReference(value); // Reference 4461 return value; 4462 case -1532328299: // coverageArea 4463 this.getCoverageArea().add(castToReference(value)); // Reference 4464 return value; 4465 case 951526432: // contact 4466 this.getContact().add((InsurancePlanContactComponent) value); // InsurancePlanContactComponent 4467 return value; 4468 case 1741102485: // endpoint 4469 this.getEndpoint().add(castToReference(value)); // Reference 4470 return value; 4471 case 1843485230: // network 4472 this.getNetwork().add(castToReference(value)); // Reference 4473 return value; 4474 case -351767064: // coverage 4475 this.getCoverage().add((InsurancePlanCoverageComponent) value); // InsurancePlanCoverageComponent 4476 return value; 4477 case 3443497: // plan 4478 this.getPlan().add((InsurancePlanPlanComponent) value); // InsurancePlanPlanComponent 4479 return value; 4480 default: 4481 return super.setProperty(hash, name, value); 4482 } 4483 4484 } 4485 4486 @Override 4487 public Base setProperty(String name, Base value) throws FHIRException { 4488 if (name.equals("identifier")) { 4489 this.getIdentifier().add(castToIdentifier(value)); 4490 } else if (name.equals("status")) { 4491 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4492 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4493 } else if (name.equals("type")) { 4494 this.getType().add(castToCodeableConcept(value)); 4495 } else if (name.equals("name")) { 4496 this.name = castToString(value); // StringType 4497 } else if (name.equals("alias")) { 4498 this.getAlias().add(castToString(value)); 4499 } else if (name.equals("period")) { 4500 this.period = castToPeriod(value); // Period 4501 } else if (name.equals("ownedBy")) { 4502 this.ownedBy = castToReference(value); // Reference 4503 } else if (name.equals("administeredBy")) { 4504 this.administeredBy = castToReference(value); // Reference 4505 } else if (name.equals("coverageArea")) { 4506 this.getCoverageArea().add(castToReference(value)); 4507 } else if (name.equals("contact")) { 4508 this.getContact().add((InsurancePlanContactComponent) value); 4509 } else if (name.equals("endpoint")) { 4510 this.getEndpoint().add(castToReference(value)); 4511 } else if (name.equals("network")) { 4512 this.getNetwork().add(castToReference(value)); 4513 } else if (name.equals("coverage")) { 4514 this.getCoverage().add((InsurancePlanCoverageComponent) value); 4515 } else if (name.equals("plan")) { 4516 this.getPlan().add((InsurancePlanPlanComponent) value); 4517 } else 4518 return super.setProperty(name, value); 4519 return value; 4520 } 4521 4522 @Override 4523 public Base makeProperty(int hash, String name) throws FHIRException { 4524 switch (hash) { 4525 case -1618432855: 4526 return addIdentifier(); 4527 case -892481550: 4528 return getStatusElement(); 4529 case 3575610: 4530 return addType(); 4531 case 3373707: 4532 return getNameElement(); 4533 case 92902992: 4534 return addAliasElement(); 4535 case -991726143: 4536 return getPeriod(); 4537 case -1054743076: 4538 return getOwnedBy(); 4539 case 898770462: 4540 return getAdministeredBy(); 4541 case -1532328299: 4542 return addCoverageArea(); 4543 case 951526432: 4544 return addContact(); 4545 case 1741102485: 4546 return addEndpoint(); 4547 case 1843485230: 4548 return addNetwork(); 4549 case -351767064: 4550 return addCoverage(); 4551 case 3443497: 4552 return addPlan(); 4553 default: 4554 return super.makeProperty(hash, name); 4555 } 4556 4557 } 4558 4559 @Override 4560 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4561 switch (hash) { 4562 case -1618432855: 4563 /* identifier */ return new String[] { "Identifier" }; 4564 case -892481550: 4565 /* status */ return new String[] { "code" }; 4566 case 3575610: 4567 /* type */ return new String[] { "CodeableConcept" }; 4568 case 3373707: 4569 /* name */ return new String[] { "string" }; 4570 case 92902992: 4571 /* alias */ return new String[] { "string" }; 4572 case -991726143: 4573 /* period */ return new String[] { "Period" }; 4574 case -1054743076: 4575 /* ownedBy */ return new String[] { "Reference" }; 4576 case 898770462: 4577 /* administeredBy */ return new String[] { "Reference" }; 4578 case -1532328299: 4579 /* coverageArea */ return new String[] { "Reference" }; 4580 case 951526432: 4581 /* contact */ return new String[] {}; 4582 case 1741102485: 4583 /* endpoint */ return new String[] { "Reference" }; 4584 case 1843485230: 4585 /* network */ return new String[] { "Reference" }; 4586 case -351767064: 4587 /* coverage */ return new String[] {}; 4588 case 3443497: 4589 /* plan */ return new String[] {}; 4590 default: 4591 return super.getTypesForProperty(hash, name); 4592 } 4593 4594 } 4595 4596 @Override 4597 public Base addChild(String name) throws FHIRException { 4598 if (name.equals("identifier")) { 4599 return addIdentifier(); 4600 } else if (name.equals("status")) { 4601 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.status"); 4602 } else if (name.equals("type")) { 4603 return addType(); 4604 } else if (name.equals("name")) { 4605 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.name"); 4606 } else if (name.equals("alias")) { 4607 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.alias"); 4608 } else if (name.equals("period")) { 4609 this.period = new Period(); 4610 return this.period; 4611 } else if (name.equals("ownedBy")) { 4612 this.ownedBy = new Reference(); 4613 return this.ownedBy; 4614 } else if (name.equals("administeredBy")) { 4615 this.administeredBy = new Reference(); 4616 return this.administeredBy; 4617 } else if (name.equals("coverageArea")) { 4618 return addCoverageArea(); 4619 } else if (name.equals("contact")) { 4620 return addContact(); 4621 } else if (name.equals("endpoint")) { 4622 return addEndpoint(); 4623 } else if (name.equals("network")) { 4624 return addNetwork(); 4625 } else if (name.equals("coverage")) { 4626 return addCoverage(); 4627 } else if (name.equals("plan")) { 4628 return addPlan(); 4629 } else 4630 return super.addChild(name); 4631 } 4632 4633 public String fhirType() { 4634 return "InsurancePlan"; 4635 4636 } 4637 4638 public InsurancePlan copy() { 4639 InsurancePlan dst = new InsurancePlan(); 4640 copyValues(dst); 4641 return dst; 4642 } 4643 4644 public void copyValues(InsurancePlan dst) { 4645 super.copyValues(dst); 4646 if (identifier != null) { 4647 dst.identifier = new ArrayList<Identifier>(); 4648 for (Identifier i : identifier) 4649 dst.identifier.add(i.copy()); 4650 } 4651 ; 4652 dst.status = status == null ? null : status.copy(); 4653 if (type != null) { 4654 dst.type = new ArrayList<CodeableConcept>(); 4655 for (CodeableConcept i : type) 4656 dst.type.add(i.copy()); 4657 } 4658 ; 4659 dst.name = name == null ? null : name.copy(); 4660 if (alias != null) { 4661 dst.alias = new ArrayList<StringType>(); 4662 for (StringType i : alias) 4663 dst.alias.add(i.copy()); 4664 } 4665 ; 4666 dst.period = period == null ? null : period.copy(); 4667 dst.ownedBy = ownedBy == null ? null : ownedBy.copy(); 4668 dst.administeredBy = administeredBy == null ? null : administeredBy.copy(); 4669 if (coverageArea != null) { 4670 dst.coverageArea = new ArrayList<Reference>(); 4671 for (Reference i : coverageArea) 4672 dst.coverageArea.add(i.copy()); 4673 } 4674 ; 4675 if (contact != null) { 4676 dst.contact = new ArrayList<InsurancePlanContactComponent>(); 4677 for (InsurancePlanContactComponent i : contact) 4678 dst.contact.add(i.copy()); 4679 } 4680 ; 4681 if (endpoint != null) { 4682 dst.endpoint = new ArrayList<Reference>(); 4683 for (Reference i : endpoint) 4684 dst.endpoint.add(i.copy()); 4685 } 4686 ; 4687 if (network != null) { 4688 dst.network = new ArrayList<Reference>(); 4689 for (Reference i : network) 4690 dst.network.add(i.copy()); 4691 } 4692 ; 4693 if (coverage != null) { 4694 dst.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4695 for (InsurancePlanCoverageComponent i : coverage) 4696 dst.coverage.add(i.copy()); 4697 } 4698 ; 4699 if (plan != null) { 4700 dst.plan = new ArrayList<InsurancePlanPlanComponent>(); 4701 for (InsurancePlanPlanComponent i : plan) 4702 dst.plan.add(i.copy()); 4703 } 4704 ; 4705 } 4706 4707 protected InsurancePlan typedCopy() { 4708 return copy(); 4709 } 4710 4711 @Override 4712 public boolean equalsDeep(Base other_) { 4713 if (!super.equalsDeep(other_)) 4714 return false; 4715 if (!(other_ instanceof InsurancePlan)) 4716 return false; 4717 InsurancePlan o = (InsurancePlan) other_; 4718 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4719 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) 4720 && compareDeep(period, o.period, true) && compareDeep(ownedBy, o.ownedBy, true) 4721 && compareDeep(administeredBy, o.administeredBy, true) && compareDeep(coverageArea, o.coverageArea, true) 4722 && compareDeep(contact, o.contact, true) && compareDeep(endpoint, o.endpoint, true) 4723 && compareDeep(network, o.network, true) && compareDeep(coverage, o.coverage, true) 4724 && compareDeep(plan, o.plan, true); 4725 } 4726 4727 @Override 4728 public boolean equalsShallow(Base other_) { 4729 if (!super.equalsShallow(other_)) 4730 return false; 4731 if (!(other_ instanceof InsurancePlan)) 4732 return false; 4733 InsurancePlan o = (InsurancePlan) other_; 4734 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 4735 && compareValues(alias, o.alias, true); 4736 } 4737 4738 public boolean isEmpty() { 4739 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, name, alias, period, 4740 ownedBy, administeredBy, coverageArea, contact, endpoint, network, coverage, plan); 4741 } 4742 4743 @Override 4744 public ResourceType getResourceType() { 4745 return ResourceType.InsurancePlan; 4746 } 4747 4748 /** 4749 * Search parameter: <b>identifier</b> 4750 * <p> 4751 * Description: <b>Any identifier for the organization (not the accreditation 4752 * issuer's identifier)</b><br> 4753 * Type: <b>token</b><br> 4754 * Path: <b>InsurancePlan.identifier</b><br> 4755 * </p> 4756 */ 4757 @SearchParamDefinition(name = "identifier", path = "InsurancePlan.identifier", description = "Any identifier for the organization (not the accreditation issuer's identifier)", type = "token") 4758 public static final String SP_IDENTIFIER = "identifier"; 4759 /** 4760 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4761 * <p> 4762 * Description: <b>Any identifier for the organization (not the accreditation 4763 * issuer's identifier)</b><br> 4764 * Type: <b>token</b><br> 4765 * Path: <b>InsurancePlan.identifier</b><br> 4766 * </p> 4767 */ 4768 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4769 SP_IDENTIFIER); 4770 4771 /** 4772 * Search parameter: <b>address</b> 4773 * <p> 4774 * Description: <b>A server defined search that may match any of the string 4775 * fields in the Address, including line, city, district, state, country, 4776 * postalCode, and/or text</b><br> 4777 * Type: <b>string</b><br> 4778 * Path: <b>InsurancePlan.contact.address</b><br> 4779 * </p> 4780 */ 4781 @SearchParamDefinition(name = "address", path = "InsurancePlan.contact.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 4782 public static final String SP_ADDRESS = "address"; 4783 /** 4784 * <b>Fluent Client</b> search parameter constant for <b>address</b> 4785 * <p> 4786 * Description: <b>A server defined search that may match any of the string 4787 * fields in the Address, including line, city, district, state, country, 4788 * postalCode, and/or text</b><br> 4789 * Type: <b>string</b><br> 4790 * Path: <b>InsurancePlan.contact.address</b><br> 4791 * </p> 4792 */ 4793 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 4794 SP_ADDRESS); 4795 4796 /** 4797 * Search parameter: <b>address-state</b> 4798 * <p> 4799 * Description: <b>A state specified in an address</b><br> 4800 * Type: <b>string</b><br> 4801 * Path: <b>InsurancePlan.contact.address.state</b><br> 4802 * </p> 4803 */ 4804 @SearchParamDefinition(name = "address-state", path = "InsurancePlan.contact.address.state", description = "A state specified in an address", type = "string") 4805 public static final String SP_ADDRESS_STATE = "address-state"; 4806 /** 4807 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 4808 * <p> 4809 * Description: <b>A state specified in an address</b><br> 4810 * Type: <b>string</b><br> 4811 * Path: <b>InsurancePlan.contact.address.state</b><br> 4812 * </p> 4813 */ 4814 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4815 SP_ADDRESS_STATE); 4816 4817 /** 4818 * Search parameter: <b>owned-by</b> 4819 * <p> 4820 * Description: <b>An organization of which this organization forms a 4821 * part</b><br> 4822 * Type: <b>reference</b><br> 4823 * Path: <b>InsurancePlan.ownedBy</b><br> 4824 * </p> 4825 */ 4826 @SearchParamDefinition(name = "owned-by", path = "InsurancePlan.ownedBy", description = "An organization of which this organization forms a part", type = "reference", target = { 4827 Organization.class }) 4828 public static final String SP_OWNED_BY = "owned-by"; 4829 /** 4830 * <b>Fluent Client</b> search parameter constant for <b>owned-by</b> 4831 * <p> 4832 * Description: <b>An organization of which this organization forms a 4833 * part</b><br> 4834 * Type: <b>reference</b><br> 4835 * Path: <b>InsurancePlan.ownedBy</b><br> 4836 * </p> 4837 */ 4838 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4839 SP_OWNED_BY); 4840 4841 /** 4842 * Constant for fluent queries to be used to add include statements. Specifies 4843 * the path value of "<b>InsurancePlan:owned-by</b>". 4844 */ 4845 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNED_BY = new ca.uhn.fhir.model.api.Include( 4846 "InsurancePlan:owned-by").toLocked(); 4847 4848 /** 4849 * Search parameter: <b>type</b> 4850 * <p> 4851 * Description: <b>A code for the type of organization</b><br> 4852 * Type: <b>token</b><br> 4853 * Path: <b>InsurancePlan.type</b><br> 4854 * </p> 4855 */ 4856 @SearchParamDefinition(name = "type", path = "InsurancePlan.type", description = "A code for the type of organization", type = "token") 4857 public static final String SP_TYPE = "type"; 4858 /** 4859 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4860 * <p> 4861 * Description: <b>A code for the type of organization</b><br> 4862 * Type: <b>token</b><br> 4863 * Path: <b>InsurancePlan.type</b><br> 4864 * </p> 4865 */ 4866 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4867 SP_TYPE); 4868 4869 /** 4870 * Search parameter: <b>address-postalcode</b> 4871 * <p> 4872 * Description: <b>A postal code specified in an address</b><br> 4873 * Type: <b>string</b><br> 4874 * Path: <b>InsurancePlan.contact.address.postalCode</b><br> 4875 * </p> 4876 */ 4877 @SearchParamDefinition(name = "address-postalcode", path = "InsurancePlan.contact.address.postalCode", description = "A postal code specified in an address", type = "string") 4878 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 4879 /** 4880 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 4881 * <p> 4882 * Description: <b>A postal code specified in an address</b><br> 4883 * Type: <b>string</b><br> 4884 * Path: <b>InsurancePlan.contact.address.postalCode</b><br> 4885 * </p> 4886 */ 4887 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4888 SP_ADDRESS_POSTALCODE); 4889 4890 /** 4891 * Search parameter: <b>administered-by</b> 4892 * <p> 4893 * Description: <b>Product administrator</b><br> 4894 * Type: <b>reference</b><br> 4895 * Path: <b>InsurancePlan.administeredBy</b><br> 4896 * </p> 4897 */ 4898 @SearchParamDefinition(name = "administered-by", path = "InsurancePlan.administeredBy", description = "Product administrator", type = "reference", target = { 4899 Organization.class }) 4900 public static final String SP_ADMINISTERED_BY = "administered-by"; 4901 /** 4902 * <b>Fluent Client</b> search parameter constant for <b>administered-by</b> 4903 * <p> 4904 * Description: <b>Product administrator</b><br> 4905 * Type: <b>reference</b><br> 4906 * Path: <b>InsurancePlan.administeredBy</b><br> 4907 * </p> 4908 */ 4909 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ADMINISTERED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4910 SP_ADMINISTERED_BY); 4911 4912 /** 4913 * Constant for fluent queries to be used to add include statements. Specifies 4914 * the path value of "<b>InsurancePlan:administered-by</b>". 4915 */ 4916 public static final ca.uhn.fhir.model.api.Include INCLUDE_ADMINISTERED_BY = new ca.uhn.fhir.model.api.Include( 4917 "InsurancePlan:administered-by").toLocked(); 4918 4919 /** 4920 * Search parameter: <b>address-country</b> 4921 * <p> 4922 * Description: <b>A country specified in an address</b><br> 4923 * Type: <b>string</b><br> 4924 * Path: <b>InsurancePlan.contact.address.country</b><br> 4925 * </p> 4926 */ 4927 @SearchParamDefinition(name = "address-country", path = "InsurancePlan.contact.address.country", description = "A country specified in an address", type = "string") 4928 public static final String SP_ADDRESS_COUNTRY = "address-country"; 4929 /** 4930 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 4931 * <p> 4932 * Description: <b>A country specified in an address</b><br> 4933 * Type: <b>string</b><br> 4934 * Path: <b>InsurancePlan.contact.address.country</b><br> 4935 * </p> 4936 */ 4937 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 4938 SP_ADDRESS_COUNTRY); 4939 4940 /** 4941 * Search parameter: <b>endpoint</b> 4942 * <p> 4943 * Description: <b>Technical endpoint</b><br> 4944 * Type: <b>reference</b><br> 4945 * Path: <b>InsurancePlan.endpoint</b><br> 4946 * </p> 4947 */ 4948 @SearchParamDefinition(name = "endpoint", path = "InsurancePlan.endpoint", description = "Technical endpoint", type = "reference", target = { 4949 Endpoint.class }) 4950 public static final String SP_ENDPOINT = "endpoint"; 4951 /** 4952 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 4953 * <p> 4954 * Description: <b>Technical endpoint</b><br> 4955 * Type: <b>reference</b><br> 4956 * Path: <b>InsurancePlan.endpoint</b><br> 4957 * </p> 4958 */ 4959 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4960 SP_ENDPOINT); 4961 4962 /** 4963 * Constant for fluent queries to be used to add include statements. Specifies 4964 * the path value of "<b>InsurancePlan:endpoint</b>". 4965 */ 4966 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 4967 "InsurancePlan:endpoint").toLocked(); 4968 4969 /** 4970 * Search parameter: <b>phonetic</b> 4971 * <p> 4972 * Description: <b>A portion of the organization's name using some kind of 4973 * phonetic matching algorithm</b><br> 4974 * Type: <b>string</b><br> 4975 * Path: <b>InsurancePlan.name</b><br> 4976 * </p> 4977 */ 4978 @SearchParamDefinition(name = "phonetic", path = "InsurancePlan.name", description = "A portion of the organization's name using some kind of phonetic matching algorithm", type = "string") 4979 public static final String SP_PHONETIC = "phonetic"; 4980 /** 4981 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 4982 * <p> 4983 * Description: <b>A portion of the organization's name using some kind of 4984 * phonetic matching algorithm</b><br> 4985 * Type: <b>string</b><br> 4986 * Path: <b>InsurancePlan.name</b><br> 4987 * </p> 4988 */ 4989 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 4990 SP_PHONETIC); 4991 4992 /** 4993 * Search parameter: <b>name</b> 4994 * <p> 4995 * Description: <b>A portion of the organization's name or alias</b><br> 4996 * Type: <b>string</b><br> 4997 * Path: <b>InsurancePlan.name, InsurancePlan.alias</b><br> 4998 * </p> 4999 */ 5000 @SearchParamDefinition(name = "name", path = "name | alias", description = "A portion of the organization's name or alias", type = "string") 5001 public static final String SP_NAME = "name"; 5002 /** 5003 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5004 * <p> 5005 * Description: <b>A portion of the organization's name or alias</b><br> 5006 * Type: <b>string</b><br> 5007 * Path: <b>InsurancePlan.name, InsurancePlan.alias</b><br> 5008 * </p> 5009 */ 5010 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5011 SP_NAME); 5012 5013 /** 5014 * Search parameter: <b>address-use</b> 5015 * <p> 5016 * Description: <b>A use code specified in an address</b><br> 5017 * Type: <b>token</b><br> 5018 * Path: <b>InsurancePlan.contact.address.use</b><br> 5019 * </p> 5020 */ 5021 @SearchParamDefinition(name = "address-use", path = "InsurancePlan.contact.address.use", description = "A use code specified in an address", type = "token") 5022 public static final String SP_ADDRESS_USE = "address-use"; 5023 /** 5024 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 5025 * <p> 5026 * Description: <b>A use code specified in an address</b><br> 5027 * Type: <b>token</b><br> 5028 * Path: <b>InsurancePlan.contact.address.use</b><br> 5029 * </p> 5030 */ 5031 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5032 SP_ADDRESS_USE); 5033 5034 /** 5035 * Search parameter: <b>address-city</b> 5036 * <p> 5037 * Description: <b>A city specified in an address</b><br> 5038 * Type: <b>string</b><br> 5039 * Path: <b>InsurancePlan.contact.address.city</b><br> 5040 * </p> 5041 */ 5042 @SearchParamDefinition(name = "address-city", path = "InsurancePlan.contact.address.city", description = "A city specified in an address", type = "string") 5043 public static final String SP_ADDRESS_CITY = "address-city"; 5044 /** 5045 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 5046 * <p> 5047 * Description: <b>A city specified in an address</b><br> 5048 * Type: <b>string</b><br> 5049 * Path: <b>InsurancePlan.contact.address.city</b><br> 5050 * </p> 5051 */ 5052 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 5053 SP_ADDRESS_CITY); 5054 5055 /** 5056 * Search parameter: <b>status</b> 5057 * <p> 5058 * Description: <b>Is the Organization record active</b><br> 5059 * Type: <b>token</b><br> 5060 * Path: <b>InsurancePlan.status</b><br> 5061 * </p> 5062 */ 5063 @SearchParamDefinition(name = "status", path = "InsurancePlan.status", description = "Is the Organization record active", type = "token") 5064 public static final String SP_STATUS = "status"; 5065 /** 5066 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5067 * <p> 5068 * Description: <b>Is the Organization record active</b><br> 5069 * Type: <b>token</b><br> 5070 * Path: <b>InsurancePlan.status</b><br> 5071 * </p> 5072 */ 5073 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5074 SP_STATUS); 5075 5076}