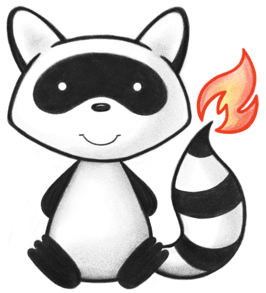
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * The Library resource is a general-purpose container for knowledge asset 050 * definitions. It can be used to describe and expose existing knowledge assets 051 * such as logic libraries and information model descriptions, as well as to 052 * describe a collection of knowledge assets. 053 */ 054@ResourceDef(name = "Library", profile = "http://hl7.org/fhir/StructureDefinition/Library") 055@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "status", "experimental", "type", 056 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 057 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 058 "endorser", "relatedArtifact", "parameter", "dataRequirement", "content" }) 059public class Library extends MetadataResource { 060 061 /** 062 * A formal identifier that is used to identify this library when it is 063 * represented in other formats, or referenced in a specification, model, design 064 * or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that 065 * at least one identifier is required for non-experimental active artifacts. 066 */ 067 @Child(name = "identifier", type = { 068 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 069 @Description(shortDefinition = "Additional identifier for the library", formalDefinition = "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.") 070 protected List<Identifier> identifier; 071 072 /** 073 * An explanatory or alternate title for the library giving additional 074 * information about its content. 075 */ 076 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 077 @Description(shortDefinition = "Subordinate title of the library", formalDefinition = "An explanatory or alternate title for the library giving additional information about its content.") 078 protected StringType subtitle; 079 080 /** 081 * Identifies the type of library such as a Logic Library, Model Definition, 082 * Asset Collection, or Module Definition. 083 */ 084 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 085 @Description(shortDefinition = "logic-library | model-definition | asset-collection | module-definition", formalDefinition = "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.") 086 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/library-type") 087 protected CodeableConcept type; 088 089 /** 090 * A code or group definition that describes the intended subject of the 091 * contents of the library. 092 */ 093 @Child(name = "subject", type = { CodeableConcept.class, 094 Group.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 095 @Description(shortDefinition = "Type of individual the library content is focused on", formalDefinition = "A code or group definition that describes the intended subject of the contents of the library.") 096 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 097 protected Type subject; 098 099 /** 100 * Explanation of why this library is needed and why it has been designed as it 101 * has. 102 */ 103 @Child(name = "purpose", type = { 104 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 105 @Description(shortDefinition = "Why this library is defined", formalDefinition = "Explanation of why this library is needed and why it has been designed as it has.") 106 protected MarkdownType purpose; 107 108 /** 109 * A detailed description of how the library is used from a clinical 110 * perspective. 111 */ 112 @Child(name = "usage", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 113 @Description(shortDefinition = "Describes the clinical usage of the library", formalDefinition = "A detailed description of how the library is used from a clinical perspective.") 114 protected StringType usage; 115 116 /** 117 * A copyright statement relating to the library and/or its contents. Copyright 118 * statements are generally legal restrictions on the use and publishing of the 119 * library. 120 */ 121 @Child(name = "copyright", type = { 122 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 123 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.") 124 protected MarkdownType copyright; 125 126 /** 127 * The date on which the resource content was approved by the publisher. 128 * Approval happens once when the content is officially approved for usage. 129 */ 130 @Child(name = "approvalDate", type = { 131 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 132 @Description(shortDefinition = "When the library was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 133 protected DateType approvalDate; 134 135 /** 136 * The date on which the resource content was last reviewed. Review happens 137 * periodically after approval but does not change the original approval date. 138 */ 139 @Child(name = "lastReviewDate", type = { 140 DateType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 141 @Description(shortDefinition = "When the library was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 142 protected DateType lastReviewDate; 143 144 /** 145 * The period during which the library content was or is planned to be in active 146 * use. 147 */ 148 @Child(name = "effectivePeriod", type = { 149 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 150 @Description(shortDefinition = "When the library is expected to be used", formalDefinition = "The period during which the library content was or is planned to be in active use.") 151 protected Period effectivePeriod; 152 153 /** 154 * Descriptive topics related to the content of the library. Topics provide a 155 * high-level categorization of the library that can be useful for filtering and 156 * searching. 157 */ 158 @Child(name = "topic", type = { 159 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 160 @Description(shortDefinition = "E.g. Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.") 161 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 162 protected List<CodeableConcept> topic; 163 164 /** 165 * An individiual or organization primarily involved in the creation and 166 * maintenance of the content. 167 */ 168 @Child(name = "author", type = { 169 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 170 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 171 protected List<ContactDetail> author; 172 173 /** 174 * An individual or organization primarily responsible for internal coherence of 175 * the content. 176 */ 177 @Child(name = "editor", type = { 178 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 179 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 180 protected List<ContactDetail> editor; 181 182 /** 183 * An individual or organization primarily responsible for review of some aspect 184 * of the content. 185 */ 186 @Child(name = "reviewer", type = { 187 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 188 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 189 protected List<ContactDetail> reviewer; 190 191 /** 192 * An individual or organization responsible for officially endorsing the 193 * content for use in some setting. 194 */ 195 @Child(name = "endorser", type = { 196 ContactDetail.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 197 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 198 protected List<ContactDetail> endorser; 199 200 /** 201 * Related artifacts such as additional documentation, justification, or 202 * bibliographic references. 203 */ 204 @Child(name = "relatedArtifact", type = { 205 RelatedArtifact.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 206 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 207 protected List<RelatedArtifact> relatedArtifact; 208 209 /** 210 * The parameter element defines parameters used by the library. 211 */ 212 @Child(name = "parameter", type = { 213 ParameterDefinition.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 214 @Description(shortDefinition = "Parameters defined by the library", formalDefinition = "The parameter element defines parameters used by the library.") 215 protected List<ParameterDefinition> parameter; 216 217 /** 218 * Describes a set of data that must be provided in order to be able to 219 * successfully perform the computations defined by the library. 220 */ 221 @Child(name = "dataRequirement", type = { 222 DataRequirement.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 223 @Description(shortDefinition = "What data is referenced by this library", formalDefinition = "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.") 224 protected List<DataRequirement> dataRequirement; 225 226 /** 227 * The content of the library as an Attachment. The content may be a reference 228 * to a url, or may be directly embedded as a base-64 string. Either way, the 229 * contentType of the attachment determines how to interpret the content. 230 */ 231 @Child(name = "content", type = { 232 Attachment.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 233 @Description(shortDefinition = "Contents of the library, either embedded or referenced", formalDefinition = "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.") 234 protected List<Attachment> content; 235 236 private static final long serialVersionUID = -796686369L; 237 238 /** 239 * Constructor 240 */ 241 public Library() { 242 super(); 243 } 244 245 /** 246 * Constructor 247 */ 248 public Library(Enumeration<PublicationStatus> status, CodeableConcept type) { 249 super(); 250 this.status = status; 251 this.type = type; 252 } 253 254 /** 255 * @return {@link #url} (An absolute URI that is used to identify this library 256 * when it is referenced in a specification, model, design or an 257 * instance; also called its canonical identifier. This SHOULD be 258 * globally unique and SHOULD be a literal address at which at which an 259 * authoritative instance of this library is (or will be) published. 260 * This URL can be the target of a canonical reference. It SHALL remain 261 * the same when the library is stored on different servers.). This is 262 * the underlying object with id, value and extensions. The accessor 263 * "getUrl" gives direct access to the value 264 */ 265 public UriType getUrlElement() { 266 if (this.url == null) 267 if (Configuration.errorOnAutoCreate()) 268 throw new Error("Attempt to auto-create Library.url"); 269 else if (Configuration.doAutoCreate()) 270 this.url = new UriType(); // bb 271 return this.url; 272 } 273 274 public boolean hasUrlElement() { 275 return this.url != null && !this.url.isEmpty(); 276 } 277 278 public boolean hasUrl() { 279 return this.url != null && !this.url.isEmpty(); 280 } 281 282 /** 283 * @param value {@link #url} (An absolute URI that is used to identify this 284 * library when it is referenced in a specification, model, design 285 * or an instance; also called its canonical identifier. This 286 * SHOULD be globally unique and SHOULD be a literal address at 287 * which at which an authoritative instance of this library is (or 288 * will be) published. This URL can be the target of a canonical 289 * reference. It SHALL remain the same when the library is stored 290 * on different servers.). This is the underlying object with id, 291 * value and extensions. The accessor "getUrl" gives direct access 292 * to the value 293 */ 294 public Library setUrlElement(UriType value) { 295 this.url = value; 296 return this; 297 } 298 299 /** 300 * @return An absolute URI that is used to identify this library when it is 301 * referenced in a specification, model, design or an instance; also 302 * called its canonical identifier. This SHOULD be globally unique and 303 * SHOULD be a literal address at which at which an authoritative 304 * instance of this library is (or will be) published. This URL can be 305 * the target of a canonical reference. It SHALL remain the same when 306 * the library is stored on different servers. 307 */ 308 public String getUrl() { 309 return this.url == null ? null : this.url.getValue(); 310 } 311 312 /** 313 * @param value An absolute URI that is used to identify this library when it is 314 * referenced in a specification, model, design or an instance; 315 * also called its canonical identifier. This SHOULD be globally 316 * unique and SHOULD be a literal address at which at which an 317 * authoritative instance of this library is (or will be) 318 * published. This URL can be the target of a canonical reference. 319 * It SHALL remain the same when the library is stored on different 320 * servers. 321 */ 322 public Library setUrl(String value) { 323 if (Utilities.noString(value)) 324 this.url = null; 325 else { 326 if (this.url == null) 327 this.url = new UriType(); 328 this.url.setValue(value); 329 } 330 return this; 331 } 332 333 /** 334 * @return {@link #identifier} (A formal identifier that is used to identify 335 * this library when it is represented in other formats, or referenced 336 * in a specification, model, design or an instance. e.g. CMS or NQF 337 * identifiers for a measure artifact. Note that at least one identifier 338 * is required for non-experimental active artifacts.) 339 */ 340 public List<Identifier> getIdentifier() { 341 if (this.identifier == null) 342 this.identifier = new ArrayList<Identifier>(); 343 return this.identifier; 344 } 345 346 /** 347 * @return Returns a reference to <code>this</code> for easy method chaining 348 */ 349 public Library setIdentifier(List<Identifier> theIdentifier) { 350 this.identifier = theIdentifier; 351 return this; 352 } 353 354 public boolean hasIdentifier() { 355 if (this.identifier == null) 356 return false; 357 for (Identifier item : this.identifier) 358 if (!item.isEmpty()) 359 return true; 360 return false; 361 } 362 363 public Identifier addIdentifier() { // 3 364 Identifier t = new Identifier(); 365 if (this.identifier == null) 366 this.identifier = new ArrayList<Identifier>(); 367 this.identifier.add(t); 368 return t; 369 } 370 371 public Library addIdentifier(Identifier t) { // 3 372 if (t == null) 373 return this; 374 if (this.identifier == null) 375 this.identifier = new ArrayList<Identifier>(); 376 this.identifier.add(t); 377 return this; 378 } 379 380 /** 381 * @return The first repetition of repeating field {@link #identifier}, creating 382 * it if it does not already exist 383 */ 384 public Identifier getIdentifierFirstRep() { 385 if (getIdentifier().isEmpty()) { 386 addIdentifier(); 387 } 388 return getIdentifier().get(0); 389 } 390 391 /** 392 * @return {@link #version} (The identifier that is used to identify this 393 * version of the library when it is referenced in a specification, 394 * model, design or instance. This is an arbitrary value managed by the 395 * library author and is not expected to be globally unique. For 396 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 397 * is not available. There is also no expectation that versions can be 398 * placed in a lexicographical sequence. To provide a version consistent 399 * with the Decision Support Service specification, use the format 400 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 401 * knowledge assets, refer to the Decision Support Service 402 * specification. Note that a version is required for non-experimental 403 * active artifacts.). This is the underlying object with id, value and 404 * extensions. The accessor "getVersion" gives direct access to the 405 * value 406 */ 407 public StringType getVersionElement() { 408 if (this.version == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create Library.version"); 411 else if (Configuration.doAutoCreate()) 412 this.version = new StringType(); // bb 413 return this.version; 414 } 415 416 public boolean hasVersionElement() { 417 return this.version != null && !this.version.isEmpty(); 418 } 419 420 public boolean hasVersion() { 421 return this.version != null && !this.version.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #version} (The identifier that is used to identify this 426 * version of the library when it is referenced in a specification, 427 * model, design or instance. This is an arbitrary value managed by 428 * the library author and is not expected to be globally unique. 429 * For example, it might be a timestamp (e.g. yyyymmdd) if a 430 * managed version is not available. There is also no expectation 431 * that versions can be placed in a lexicographical sequence. To 432 * provide a version consistent with the Decision Support Service 433 * specification, use the format Major.Minor.Revision (e.g. 1.0.0). 434 * For more information on versioning knowledge assets, refer to 435 * the Decision Support Service specification. Note that a version 436 * is required for non-experimental active artifacts.). This is the 437 * underlying object with id, value and extensions. The accessor 438 * "getVersion" gives direct access to the value 439 */ 440 public Library setVersionElement(StringType value) { 441 this.version = value; 442 return this; 443 } 444 445 /** 446 * @return The identifier that is used to identify this version of the library 447 * when it is referenced in a specification, model, design or instance. 448 * This is an arbitrary value managed by the library author and is not 449 * expected to be globally unique. For example, it might be a timestamp 450 * (e.g. yyyymmdd) if a managed version is not available. There is also 451 * no expectation that versions can be placed in a lexicographical 452 * sequence. To provide a version consistent with the Decision Support 453 * Service specification, use the format Major.Minor.Revision (e.g. 454 * 1.0.0). For more information on versioning knowledge assets, refer to 455 * the Decision Support Service specification. Note that a version is 456 * required for non-experimental active artifacts. 457 */ 458 public String getVersion() { 459 return this.version == null ? null : this.version.getValue(); 460 } 461 462 /** 463 * @param value The identifier that is used to identify this version of the 464 * library when it is referenced in a specification, model, design 465 * or instance. This is an arbitrary value managed by the library 466 * author and is not expected to be globally unique. For example, 467 * it might be a timestamp (e.g. yyyymmdd) if a managed version is 468 * not available. There is also no expectation that versions can be 469 * placed in a lexicographical sequence. To provide a version 470 * consistent with the Decision Support Service specification, use 471 * the format Major.Minor.Revision (e.g. 1.0.0). For more 472 * information on versioning knowledge assets, refer to the 473 * Decision Support Service specification. Note that a version is 474 * required for non-experimental active artifacts. 475 */ 476 public Library setVersion(String value) { 477 if (Utilities.noString(value)) 478 this.version = null; 479 else { 480 if (this.version == null) 481 this.version = new StringType(); 482 this.version.setValue(value); 483 } 484 return this; 485 } 486 487 /** 488 * @return {@link #name} (A natural language name identifying the library. This 489 * name should be usable as an identifier for the module by machine 490 * processing applications such as code generation.). This is the 491 * underlying object with id, value and extensions. The accessor 492 * "getName" gives direct access to the value 493 */ 494 public StringType getNameElement() { 495 if (this.name == null) 496 if (Configuration.errorOnAutoCreate()) 497 throw new Error("Attempt to auto-create Library.name"); 498 else if (Configuration.doAutoCreate()) 499 this.name = new StringType(); // bb 500 return this.name; 501 } 502 503 public boolean hasNameElement() { 504 return this.name != null && !this.name.isEmpty(); 505 } 506 507 public boolean hasName() { 508 return this.name != null && !this.name.isEmpty(); 509 } 510 511 /** 512 * @param value {@link #name} (A natural language name identifying the library. 513 * This name should be usable as an identifier for the module by 514 * machine processing applications such as code generation.). This 515 * is the underlying object with id, value and extensions. The 516 * accessor "getName" gives direct access to the value 517 */ 518 public Library setNameElement(StringType value) { 519 this.name = value; 520 return this; 521 } 522 523 /** 524 * @return A natural language name identifying the library. This name should be 525 * usable as an identifier for the module by machine processing 526 * applications such as code generation. 527 */ 528 public String getName() { 529 return this.name == null ? null : this.name.getValue(); 530 } 531 532 /** 533 * @param value A natural language name identifying the library. This name 534 * should be usable as an identifier for the module by machine 535 * processing applications such as code generation. 536 */ 537 public Library setName(String value) { 538 if (Utilities.noString(value)) 539 this.name = null; 540 else { 541 if (this.name == null) 542 this.name = new StringType(); 543 this.name.setValue(value); 544 } 545 return this; 546 } 547 548 /** 549 * @return {@link #title} (A short, descriptive, user-friendly title for the 550 * library.). This is the underlying object with id, value and 551 * extensions. The accessor "getTitle" gives direct access to the value 552 */ 553 public StringType getTitleElement() { 554 if (this.title == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create Library.title"); 557 else if (Configuration.doAutoCreate()) 558 this.title = new StringType(); // bb 559 return this.title; 560 } 561 562 public boolean hasTitleElement() { 563 return this.title != null && !this.title.isEmpty(); 564 } 565 566 public boolean hasTitle() { 567 return this.title != null && !this.title.isEmpty(); 568 } 569 570 /** 571 * @param value {@link #title} (A short, descriptive, user-friendly title for 572 * the library.). This is the underlying object with id, value and 573 * extensions. The accessor "getTitle" gives direct access to the 574 * value 575 */ 576 public Library setTitleElement(StringType value) { 577 this.title = value; 578 return this; 579 } 580 581 /** 582 * @return A short, descriptive, user-friendly title for the library. 583 */ 584 public String getTitle() { 585 return this.title == null ? null : this.title.getValue(); 586 } 587 588 /** 589 * @param value A short, descriptive, user-friendly title for the library. 590 */ 591 public Library setTitle(String value) { 592 if (Utilities.noString(value)) 593 this.title = null; 594 else { 595 if (this.title == null) 596 this.title = new StringType(); 597 this.title.setValue(value); 598 } 599 return this; 600 } 601 602 /** 603 * @return {@link #subtitle} (An explanatory or alternate title for the library 604 * giving additional information about its content.). This is the 605 * underlying object with id, value and extensions. The accessor 606 * "getSubtitle" gives direct access to the value 607 */ 608 public StringType getSubtitleElement() { 609 if (this.subtitle == null) 610 if (Configuration.errorOnAutoCreate()) 611 throw new Error("Attempt to auto-create Library.subtitle"); 612 else if (Configuration.doAutoCreate()) 613 this.subtitle = new StringType(); // bb 614 return this.subtitle; 615 } 616 617 public boolean hasSubtitleElement() { 618 return this.subtitle != null && !this.subtitle.isEmpty(); 619 } 620 621 public boolean hasSubtitle() { 622 return this.subtitle != null && !this.subtitle.isEmpty(); 623 } 624 625 /** 626 * @param value {@link #subtitle} (An explanatory or alternate title for the 627 * library giving additional information about its content.). This 628 * is the underlying object with id, value and extensions. The 629 * accessor "getSubtitle" gives direct access to the value 630 */ 631 public Library setSubtitleElement(StringType value) { 632 this.subtitle = value; 633 return this; 634 } 635 636 /** 637 * @return An explanatory or alternate title for the library giving additional 638 * information about its content. 639 */ 640 public String getSubtitle() { 641 return this.subtitle == null ? null : this.subtitle.getValue(); 642 } 643 644 /** 645 * @param value An explanatory or alternate title for the library giving 646 * additional information about its content. 647 */ 648 public Library setSubtitle(String value) { 649 if (Utilities.noString(value)) 650 this.subtitle = null; 651 else { 652 if (this.subtitle == null) 653 this.subtitle = new StringType(); 654 this.subtitle.setValue(value); 655 } 656 return this; 657 } 658 659 /** 660 * @return {@link #status} (The status of this library. Enables tracking the 661 * life-cycle of the content.). This is the underlying object with id, 662 * value and extensions. The accessor "getStatus" gives direct access to 663 * the value 664 */ 665 public Enumeration<PublicationStatus> getStatusElement() { 666 if (this.status == null) 667 if (Configuration.errorOnAutoCreate()) 668 throw new Error("Attempt to auto-create Library.status"); 669 else if (Configuration.doAutoCreate()) 670 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 671 return this.status; 672 } 673 674 public boolean hasStatusElement() { 675 return this.status != null && !this.status.isEmpty(); 676 } 677 678 public boolean hasStatus() { 679 return this.status != null && !this.status.isEmpty(); 680 } 681 682 /** 683 * @param value {@link #status} (The status of this library. Enables tracking 684 * the life-cycle of the content.). This is the underlying object 685 * with id, value and extensions. The accessor "getStatus" gives 686 * direct access to the value 687 */ 688 public Library setStatusElement(Enumeration<PublicationStatus> value) { 689 this.status = value; 690 return this; 691 } 692 693 /** 694 * @return The status of this library. Enables tracking the life-cycle of the 695 * content. 696 */ 697 public PublicationStatus getStatus() { 698 return this.status == null ? null : this.status.getValue(); 699 } 700 701 /** 702 * @param value The status of this library. Enables tracking the life-cycle of 703 * the content. 704 */ 705 public Library setStatus(PublicationStatus value) { 706 if (this.status == null) 707 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 708 this.status.setValue(value); 709 return this; 710 } 711 712 /** 713 * @return {@link #experimental} (A Boolean value to indicate that this library 714 * is authored for testing purposes (or education/evaluation/marketing) 715 * and is not intended to be used for genuine usage.). This is the 716 * underlying object with id, value and extensions. The accessor 717 * "getExperimental" gives direct access to the value 718 */ 719 public BooleanType getExperimentalElement() { 720 if (this.experimental == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create Library.experimental"); 723 else if (Configuration.doAutoCreate()) 724 this.experimental = new BooleanType(); // bb 725 return this.experimental; 726 } 727 728 public boolean hasExperimentalElement() { 729 return this.experimental != null && !this.experimental.isEmpty(); 730 } 731 732 public boolean hasExperimental() { 733 return this.experimental != null && !this.experimental.isEmpty(); 734 } 735 736 /** 737 * @param value {@link #experimental} (A Boolean value to indicate that this 738 * library is authored for testing purposes (or 739 * education/evaluation/marketing) and is not intended to be used 740 * for genuine usage.). This is the underlying object with id, 741 * value and extensions. The accessor "getExperimental" gives 742 * direct access to the value 743 */ 744 public Library setExperimentalElement(BooleanType value) { 745 this.experimental = value; 746 return this; 747 } 748 749 /** 750 * @return A Boolean value to indicate that this library is authored for testing 751 * purposes (or education/evaluation/marketing) and is not intended to 752 * be used for genuine usage. 753 */ 754 public boolean getExperimental() { 755 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 756 } 757 758 /** 759 * @param value A Boolean value to indicate that this library is authored for 760 * testing purposes (or education/evaluation/marketing) and is not 761 * intended to be used for genuine usage. 762 */ 763 public Library setExperimental(boolean value) { 764 if (this.experimental == null) 765 this.experimental = new BooleanType(); 766 this.experimental.setValue(value); 767 return this; 768 } 769 770 /** 771 * @return {@link #type} (Identifies the type of library such as a Logic 772 * Library, Model Definition, Asset Collection, or Module Definition.) 773 */ 774 public CodeableConcept getType() { 775 if (this.type == null) 776 if (Configuration.errorOnAutoCreate()) 777 throw new Error("Attempt to auto-create Library.type"); 778 else if (Configuration.doAutoCreate()) 779 this.type = new CodeableConcept(); // cc 780 return this.type; 781 } 782 783 public boolean hasType() { 784 return this.type != null && !this.type.isEmpty(); 785 } 786 787 /** 788 * @param value {@link #type} (Identifies the type of library such as a Logic 789 * Library, Model Definition, Asset Collection, or Module 790 * Definition.) 791 */ 792 public Library setType(CodeableConcept value) { 793 this.type = value; 794 return this; 795 } 796 797 /** 798 * @return {@link #subject} (A code or group definition that describes the 799 * intended subject of the contents of the library.) 800 */ 801 public Type getSubject() { 802 return this.subject; 803 } 804 805 /** 806 * @return {@link #subject} (A code or group definition that describes the 807 * intended subject of the contents of the library.) 808 */ 809 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 810 if (this.subject == null) 811 this.subject = new CodeableConcept(); 812 if (!(this.subject instanceof CodeableConcept)) 813 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 814 + this.subject.getClass().getName() + " was encountered"); 815 return (CodeableConcept) this.subject; 816 } 817 818 public boolean hasSubjectCodeableConcept() { 819 return this != null && this.subject instanceof CodeableConcept; 820 } 821 822 /** 823 * @return {@link #subject} (A code or group definition that describes the 824 * intended subject of the contents of the library.) 825 */ 826 public Reference getSubjectReference() throws FHIRException { 827 if (this.subject == null) 828 this.subject = new Reference(); 829 if (!(this.subject instanceof Reference)) 830 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 831 + " was encountered"); 832 return (Reference) this.subject; 833 } 834 835 public boolean hasSubjectReference() { 836 return this != null && this.subject instanceof Reference; 837 } 838 839 public boolean hasSubject() { 840 return this.subject != null && !this.subject.isEmpty(); 841 } 842 843 /** 844 * @param value {@link #subject} (A code or group definition that describes the 845 * intended subject of the contents of the library.) 846 */ 847 public Library setSubject(Type value) { 848 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 849 throw new Error("Not the right type for Library.subject[x]: " + value.fhirType()); 850 this.subject = value; 851 return this; 852 } 853 854 /** 855 * @return {@link #date} (The date (and optionally time) when the library was 856 * published. The date must change when the business version changes and 857 * it must change if the status code changes. In addition, it should 858 * change when the substantive content of the library changes.). This is 859 * the underlying object with id, value and extensions. The accessor 860 * "getDate" gives direct access to the value 861 */ 862 public DateTimeType getDateElement() { 863 if (this.date == null) 864 if (Configuration.errorOnAutoCreate()) 865 throw new Error("Attempt to auto-create Library.date"); 866 else if (Configuration.doAutoCreate()) 867 this.date = new DateTimeType(); // bb 868 return this.date; 869 } 870 871 public boolean hasDateElement() { 872 return this.date != null && !this.date.isEmpty(); 873 } 874 875 public boolean hasDate() { 876 return this.date != null && !this.date.isEmpty(); 877 } 878 879 /** 880 * @param value {@link #date} (The date (and optionally time) when the library 881 * was published. The date must change when the business version 882 * changes and it must change if the status code changes. In 883 * addition, it should change when the substantive content of the 884 * library changes.). This is the underlying object with id, value 885 * and extensions. The accessor "getDate" gives direct access to 886 * the value 887 */ 888 public Library setDateElement(DateTimeType value) { 889 this.date = value; 890 return this; 891 } 892 893 /** 894 * @return The date (and optionally time) when the library was published. The 895 * date must change when the business version changes and it must change 896 * if the status code changes. In addition, it should change when the 897 * substantive content of the library changes. 898 */ 899 public Date getDate() { 900 return this.date == null ? null : this.date.getValue(); 901 } 902 903 /** 904 * @param value The date (and optionally time) when the library was published. 905 * The date must change when the business version changes and it 906 * must change if the status code changes. In addition, it should 907 * change when the substantive content of the library changes. 908 */ 909 public Library setDate(Date value) { 910 if (value == null) 911 this.date = null; 912 else { 913 if (this.date == null) 914 this.date = new DateTimeType(); 915 this.date.setValue(value); 916 } 917 return this; 918 } 919 920 /** 921 * @return {@link #publisher} (The name of the organization or individual that 922 * published the library.). This is the underlying object with id, value 923 * and extensions. The accessor "getPublisher" gives direct access to 924 * the value 925 */ 926 public StringType getPublisherElement() { 927 if (this.publisher == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create Library.publisher"); 930 else if (Configuration.doAutoCreate()) 931 this.publisher = new StringType(); // bb 932 return this.publisher; 933 } 934 935 public boolean hasPublisherElement() { 936 return this.publisher != null && !this.publisher.isEmpty(); 937 } 938 939 public boolean hasPublisher() { 940 return this.publisher != null && !this.publisher.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #publisher} (The name of the organization or individual 945 * that published the library.). This is the underlying object with 946 * id, value and extensions. The accessor "getPublisher" gives 947 * direct access to the value 948 */ 949 public Library setPublisherElement(StringType value) { 950 this.publisher = value; 951 return this; 952 } 953 954 /** 955 * @return The name of the organization or individual that published the 956 * library. 957 */ 958 public String getPublisher() { 959 return this.publisher == null ? null : this.publisher.getValue(); 960 } 961 962 /** 963 * @param value The name of the organization or individual that published the 964 * library. 965 */ 966 public Library setPublisher(String value) { 967 if (Utilities.noString(value)) 968 this.publisher = null; 969 else { 970 if (this.publisher == null) 971 this.publisher = new StringType(); 972 this.publisher.setValue(value); 973 } 974 return this; 975 } 976 977 /** 978 * @return {@link #contact} (Contact details to assist a user in finding and 979 * communicating with the publisher.) 980 */ 981 public List<ContactDetail> getContact() { 982 if (this.contact == null) 983 this.contact = new ArrayList<ContactDetail>(); 984 return this.contact; 985 } 986 987 /** 988 * @return Returns a reference to <code>this</code> for easy method chaining 989 */ 990 public Library setContact(List<ContactDetail> theContact) { 991 this.contact = theContact; 992 return this; 993 } 994 995 public boolean hasContact() { 996 if (this.contact == null) 997 return false; 998 for (ContactDetail item : this.contact) 999 if (!item.isEmpty()) 1000 return true; 1001 return false; 1002 } 1003 1004 public ContactDetail addContact() { // 3 1005 ContactDetail t = new ContactDetail(); 1006 if (this.contact == null) 1007 this.contact = new ArrayList<ContactDetail>(); 1008 this.contact.add(t); 1009 return t; 1010 } 1011 1012 public Library addContact(ContactDetail t) { // 3 1013 if (t == null) 1014 return this; 1015 if (this.contact == null) 1016 this.contact = new ArrayList<ContactDetail>(); 1017 this.contact.add(t); 1018 return this; 1019 } 1020 1021 /** 1022 * @return The first repetition of repeating field {@link #contact}, creating it 1023 * if it does not already exist 1024 */ 1025 public ContactDetail getContactFirstRep() { 1026 if (getContact().isEmpty()) { 1027 addContact(); 1028 } 1029 return getContact().get(0); 1030 } 1031 1032 /** 1033 * @return {@link #description} (A free text natural language description of the 1034 * library from a consumer's perspective.). This is the underlying 1035 * object with id, value and extensions. The accessor "getDescription" 1036 * gives direct access to the value 1037 */ 1038 public MarkdownType getDescriptionElement() { 1039 if (this.description == null) 1040 if (Configuration.errorOnAutoCreate()) 1041 throw new Error("Attempt to auto-create Library.description"); 1042 else if (Configuration.doAutoCreate()) 1043 this.description = new MarkdownType(); // bb 1044 return this.description; 1045 } 1046 1047 public boolean hasDescriptionElement() { 1048 return this.description != null && !this.description.isEmpty(); 1049 } 1050 1051 public boolean hasDescription() { 1052 return this.description != null && !this.description.isEmpty(); 1053 } 1054 1055 /** 1056 * @param value {@link #description} (A free text natural language description 1057 * of the library from a consumer's perspective.). This is the 1058 * underlying object with id, value and extensions. The accessor 1059 * "getDescription" gives direct access to the value 1060 */ 1061 public Library setDescriptionElement(MarkdownType value) { 1062 this.description = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return A free text natural language description of the library from a 1068 * consumer's perspective. 1069 */ 1070 public String getDescription() { 1071 return this.description == null ? null : this.description.getValue(); 1072 } 1073 1074 /** 1075 * @param value A free text natural language description of the library from a 1076 * consumer's perspective. 1077 */ 1078 public Library setDescription(String value) { 1079 if (value == null) 1080 this.description = null; 1081 else { 1082 if (this.description == null) 1083 this.description = new MarkdownType(); 1084 this.description.setValue(value); 1085 } 1086 return this; 1087 } 1088 1089 /** 1090 * @return {@link #useContext} (The content was developed with a focus and 1091 * intent of supporting the contexts that are listed. These contexts may 1092 * be general categories (gender, age, ...) or may be references to 1093 * specific programs (insurance plans, studies, ...) and may be used to 1094 * assist with indexing and searching for appropriate library 1095 * instances.) 1096 */ 1097 public List<UsageContext> getUseContext() { 1098 if (this.useContext == null) 1099 this.useContext = new ArrayList<UsageContext>(); 1100 return this.useContext; 1101 } 1102 1103 /** 1104 * @return Returns a reference to <code>this</code> for easy method chaining 1105 */ 1106 public Library setUseContext(List<UsageContext> theUseContext) { 1107 this.useContext = theUseContext; 1108 return this; 1109 } 1110 1111 public boolean hasUseContext() { 1112 if (this.useContext == null) 1113 return false; 1114 for (UsageContext item : this.useContext) 1115 if (!item.isEmpty()) 1116 return true; 1117 return false; 1118 } 1119 1120 public UsageContext addUseContext() { // 3 1121 UsageContext t = new UsageContext(); 1122 if (this.useContext == null) 1123 this.useContext = new ArrayList<UsageContext>(); 1124 this.useContext.add(t); 1125 return t; 1126 } 1127 1128 public Library addUseContext(UsageContext t) { // 3 1129 if (t == null) 1130 return this; 1131 if (this.useContext == null) 1132 this.useContext = new ArrayList<UsageContext>(); 1133 this.useContext.add(t); 1134 return this; 1135 } 1136 1137 /** 1138 * @return The first repetition of repeating field {@link #useContext}, creating 1139 * it if it does not already exist 1140 */ 1141 public UsageContext getUseContextFirstRep() { 1142 if (getUseContext().isEmpty()) { 1143 addUseContext(); 1144 } 1145 return getUseContext().get(0); 1146 } 1147 1148 /** 1149 * @return {@link #jurisdiction} (A legal or geographic region in which the 1150 * library is intended to be used.) 1151 */ 1152 public List<CodeableConcept> getJurisdiction() { 1153 if (this.jurisdiction == null) 1154 this.jurisdiction = new ArrayList<CodeableConcept>(); 1155 return this.jurisdiction; 1156 } 1157 1158 /** 1159 * @return Returns a reference to <code>this</code> for easy method chaining 1160 */ 1161 public Library setJurisdiction(List<CodeableConcept> theJurisdiction) { 1162 this.jurisdiction = theJurisdiction; 1163 return this; 1164 } 1165 1166 public boolean hasJurisdiction() { 1167 if (this.jurisdiction == null) 1168 return false; 1169 for (CodeableConcept item : this.jurisdiction) 1170 if (!item.isEmpty()) 1171 return true; 1172 return false; 1173 } 1174 1175 public CodeableConcept addJurisdiction() { // 3 1176 CodeableConcept t = new CodeableConcept(); 1177 if (this.jurisdiction == null) 1178 this.jurisdiction = new ArrayList<CodeableConcept>(); 1179 this.jurisdiction.add(t); 1180 return t; 1181 } 1182 1183 public Library addJurisdiction(CodeableConcept t) { // 3 1184 if (t == null) 1185 return this; 1186 if (this.jurisdiction == null) 1187 this.jurisdiction = new ArrayList<CodeableConcept>(); 1188 this.jurisdiction.add(t); 1189 return this; 1190 } 1191 1192 /** 1193 * @return The first repetition of repeating field {@link #jurisdiction}, 1194 * creating it if it does not already exist 1195 */ 1196 public CodeableConcept getJurisdictionFirstRep() { 1197 if (getJurisdiction().isEmpty()) { 1198 addJurisdiction(); 1199 } 1200 return getJurisdiction().get(0); 1201 } 1202 1203 /** 1204 * @return {@link #purpose} (Explanation of why this library is needed and why 1205 * it has been designed as it has.). This is the underlying object with 1206 * id, value and extensions. The accessor "getPurpose" gives direct 1207 * access to the value 1208 */ 1209 public MarkdownType getPurposeElement() { 1210 if (this.purpose == null) 1211 if (Configuration.errorOnAutoCreate()) 1212 throw new Error("Attempt to auto-create Library.purpose"); 1213 else if (Configuration.doAutoCreate()) 1214 this.purpose = new MarkdownType(); // bb 1215 return this.purpose; 1216 } 1217 1218 public boolean hasPurposeElement() { 1219 return this.purpose != null && !this.purpose.isEmpty(); 1220 } 1221 1222 public boolean hasPurpose() { 1223 return this.purpose != null && !this.purpose.isEmpty(); 1224 } 1225 1226 /** 1227 * @param value {@link #purpose} (Explanation of why this library is needed and 1228 * why it has been designed as it has.). This is the underlying 1229 * object with id, value and extensions. The accessor "getPurpose" 1230 * gives direct access to the value 1231 */ 1232 public Library setPurposeElement(MarkdownType value) { 1233 this.purpose = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return Explanation of why this library is needed and why it has been 1239 * designed as it has. 1240 */ 1241 public String getPurpose() { 1242 return this.purpose == null ? null : this.purpose.getValue(); 1243 } 1244 1245 /** 1246 * @param value Explanation of why this library is needed and why it has been 1247 * designed as it has. 1248 */ 1249 public Library setPurpose(String value) { 1250 if (value == null) 1251 this.purpose = null; 1252 else { 1253 if (this.purpose == null) 1254 this.purpose = new MarkdownType(); 1255 this.purpose.setValue(value); 1256 } 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #usage} (A detailed description of how the library is used 1262 * from a clinical perspective.). This is the underlying object with id, 1263 * value and extensions. The accessor "getUsage" gives direct access to 1264 * the value 1265 */ 1266 public StringType getUsageElement() { 1267 if (this.usage == null) 1268 if (Configuration.errorOnAutoCreate()) 1269 throw new Error("Attempt to auto-create Library.usage"); 1270 else if (Configuration.doAutoCreate()) 1271 this.usage = new StringType(); // bb 1272 return this.usage; 1273 } 1274 1275 public boolean hasUsageElement() { 1276 return this.usage != null && !this.usage.isEmpty(); 1277 } 1278 1279 public boolean hasUsage() { 1280 return this.usage != null && !this.usage.isEmpty(); 1281 } 1282 1283 /** 1284 * @param value {@link #usage} (A detailed description of how the library is 1285 * used from a clinical perspective.). This is the underlying 1286 * object with id, value and extensions. The accessor "getUsage" 1287 * gives direct access to the value 1288 */ 1289 public Library setUsageElement(StringType value) { 1290 this.usage = value; 1291 return this; 1292 } 1293 1294 /** 1295 * @return A detailed description of how the library is used from a clinical 1296 * perspective. 1297 */ 1298 public String getUsage() { 1299 return this.usage == null ? null : this.usage.getValue(); 1300 } 1301 1302 /** 1303 * @param value A detailed description of how the library is used from a 1304 * clinical perspective. 1305 */ 1306 public Library setUsage(String value) { 1307 if (Utilities.noString(value)) 1308 this.usage = null; 1309 else { 1310 if (this.usage == null) 1311 this.usage = new StringType(); 1312 this.usage.setValue(value); 1313 } 1314 return this; 1315 } 1316 1317 /** 1318 * @return {@link #copyright} (A copyright statement relating to the library 1319 * and/or its contents. Copyright statements are generally legal 1320 * restrictions on the use and publishing of the library.). This is the 1321 * underlying object with id, value and extensions. The accessor 1322 * "getCopyright" gives direct access to the value 1323 */ 1324 public MarkdownType getCopyrightElement() { 1325 if (this.copyright == null) 1326 if (Configuration.errorOnAutoCreate()) 1327 throw new Error("Attempt to auto-create Library.copyright"); 1328 else if (Configuration.doAutoCreate()) 1329 this.copyright = new MarkdownType(); // bb 1330 return this.copyright; 1331 } 1332 1333 public boolean hasCopyrightElement() { 1334 return this.copyright != null && !this.copyright.isEmpty(); 1335 } 1336 1337 public boolean hasCopyright() { 1338 return this.copyright != null && !this.copyright.isEmpty(); 1339 } 1340 1341 /** 1342 * @param value {@link #copyright} (A copyright statement relating to the 1343 * library and/or its contents. Copyright statements are generally 1344 * legal restrictions on the use and publishing of the library.). 1345 * This is the underlying object with id, value and extensions. The 1346 * accessor "getCopyright" gives direct access to the value 1347 */ 1348 public Library setCopyrightElement(MarkdownType value) { 1349 this.copyright = value; 1350 return this; 1351 } 1352 1353 /** 1354 * @return A copyright statement relating to the library and/or its contents. 1355 * Copyright statements are generally legal restrictions on the use and 1356 * publishing of the library. 1357 */ 1358 public String getCopyright() { 1359 return this.copyright == null ? null : this.copyright.getValue(); 1360 } 1361 1362 /** 1363 * @param value A copyright statement relating to the library and/or its 1364 * contents. Copyright statements are generally legal restrictions 1365 * on the use and publishing of the library. 1366 */ 1367 public Library setCopyright(String value) { 1368 if (value == null) 1369 this.copyright = null; 1370 else { 1371 if (this.copyright == null) 1372 this.copyright = new MarkdownType(); 1373 this.copyright.setValue(value); 1374 } 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #approvalDate} (The date on which the resource content was 1380 * approved by the publisher. Approval happens once when the content is 1381 * officially approved for usage.). This is the underlying object with 1382 * id, value and extensions. The accessor "getApprovalDate" gives direct 1383 * access to the value 1384 */ 1385 public DateType getApprovalDateElement() { 1386 if (this.approvalDate == null) 1387 if (Configuration.errorOnAutoCreate()) 1388 throw new Error("Attempt to auto-create Library.approvalDate"); 1389 else if (Configuration.doAutoCreate()) 1390 this.approvalDate = new DateType(); // bb 1391 return this.approvalDate; 1392 } 1393 1394 public boolean hasApprovalDateElement() { 1395 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1396 } 1397 1398 public boolean hasApprovalDate() { 1399 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1400 } 1401 1402 /** 1403 * @param value {@link #approvalDate} (The date on which the resource content 1404 * was approved by the publisher. Approval happens once when the 1405 * content is officially approved for usage.). This is the 1406 * underlying object with id, value and extensions. The accessor 1407 * "getApprovalDate" gives direct access to the value 1408 */ 1409 public Library setApprovalDateElement(DateType value) { 1410 this.approvalDate = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return The date on which the resource content was approved by the publisher. 1416 * Approval happens once when the content is officially approved for 1417 * usage. 1418 */ 1419 public Date getApprovalDate() { 1420 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1421 } 1422 1423 /** 1424 * @param value The date on which the resource content was approved by the 1425 * publisher. Approval happens once when the content is officially 1426 * approved for usage. 1427 */ 1428 public Library setApprovalDate(Date value) { 1429 if (value == null) 1430 this.approvalDate = null; 1431 else { 1432 if (this.approvalDate == null) 1433 this.approvalDate = new DateType(); 1434 this.approvalDate.setValue(value); 1435 } 1436 return this; 1437 } 1438 1439 /** 1440 * @return {@link #lastReviewDate} (The date on which the resource content was 1441 * last reviewed. Review happens periodically after approval but does 1442 * not change the original approval date.). This is the underlying 1443 * object with id, value and extensions. The accessor 1444 * "getLastReviewDate" gives direct access to the value 1445 */ 1446 public DateType getLastReviewDateElement() { 1447 if (this.lastReviewDate == null) 1448 if (Configuration.errorOnAutoCreate()) 1449 throw new Error("Attempt to auto-create Library.lastReviewDate"); 1450 else if (Configuration.doAutoCreate()) 1451 this.lastReviewDate = new DateType(); // bb 1452 return this.lastReviewDate; 1453 } 1454 1455 public boolean hasLastReviewDateElement() { 1456 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1457 } 1458 1459 public boolean hasLastReviewDate() { 1460 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1461 } 1462 1463 /** 1464 * @param value {@link #lastReviewDate} (The date on which the resource content 1465 * was last reviewed. Review happens periodically after approval 1466 * but does not change the original approval date.). This is the 1467 * underlying object with id, value and extensions. The accessor 1468 * "getLastReviewDate" gives direct access to the value 1469 */ 1470 public Library setLastReviewDateElement(DateType value) { 1471 this.lastReviewDate = value; 1472 return this; 1473 } 1474 1475 /** 1476 * @return The date on which the resource content was last reviewed. Review 1477 * happens periodically after approval but does not change the original 1478 * approval date. 1479 */ 1480 public Date getLastReviewDate() { 1481 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1482 } 1483 1484 /** 1485 * @param value The date on which the resource content was last reviewed. Review 1486 * happens periodically after approval but does not change the 1487 * original approval date. 1488 */ 1489 public Library setLastReviewDate(Date value) { 1490 if (value == null) 1491 this.lastReviewDate = null; 1492 else { 1493 if (this.lastReviewDate == null) 1494 this.lastReviewDate = new DateType(); 1495 this.lastReviewDate.setValue(value); 1496 } 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #effectivePeriod} (The period during which the library content 1502 * was or is planned to be in active use.) 1503 */ 1504 public Period getEffectivePeriod() { 1505 if (this.effectivePeriod == null) 1506 if (Configuration.errorOnAutoCreate()) 1507 throw new Error("Attempt to auto-create Library.effectivePeriod"); 1508 else if (Configuration.doAutoCreate()) 1509 this.effectivePeriod = new Period(); // cc 1510 return this.effectivePeriod; 1511 } 1512 1513 public boolean hasEffectivePeriod() { 1514 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 1515 } 1516 1517 /** 1518 * @param value {@link #effectivePeriod} (The period during which the library 1519 * content was or is planned to be in active use.) 1520 */ 1521 public Library setEffectivePeriod(Period value) { 1522 this.effectivePeriod = value; 1523 return this; 1524 } 1525 1526 /** 1527 * @return {@link #topic} (Descriptive topics related to the content of the 1528 * library. Topics provide a high-level categorization of the library 1529 * that can be useful for filtering and searching.) 1530 */ 1531 public List<CodeableConcept> getTopic() { 1532 if (this.topic == null) 1533 this.topic = new ArrayList<CodeableConcept>(); 1534 return this.topic; 1535 } 1536 1537 /** 1538 * @return Returns a reference to <code>this</code> for easy method chaining 1539 */ 1540 public Library setTopic(List<CodeableConcept> theTopic) { 1541 this.topic = theTopic; 1542 return this; 1543 } 1544 1545 public boolean hasTopic() { 1546 if (this.topic == null) 1547 return false; 1548 for (CodeableConcept item : this.topic) 1549 if (!item.isEmpty()) 1550 return true; 1551 return false; 1552 } 1553 1554 public CodeableConcept addTopic() { // 3 1555 CodeableConcept t = new CodeableConcept(); 1556 if (this.topic == null) 1557 this.topic = new ArrayList<CodeableConcept>(); 1558 this.topic.add(t); 1559 return t; 1560 } 1561 1562 public Library addTopic(CodeableConcept t) { // 3 1563 if (t == null) 1564 return this; 1565 if (this.topic == null) 1566 this.topic = new ArrayList<CodeableConcept>(); 1567 this.topic.add(t); 1568 return this; 1569 } 1570 1571 /** 1572 * @return The first repetition of repeating field {@link #topic}, creating it 1573 * if it does not already exist 1574 */ 1575 public CodeableConcept getTopicFirstRep() { 1576 if (getTopic().isEmpty()) { 1577 addTopic(); 1578 } 1579 return getTopic().get(0); 1580 } 1581 1582 /** 1583 * @return {@link #author} (An individiual or organization primarily involved in 1584 * the creation and maintenance of the content.) 1585 */ 1586 public List<ContactDetail> getAuthor() { 1587 if (this.author == null) 1588 this.author = new ArrayList<ContactDetail>(); 1589 return this.author; 1590 } 1591 1592 /** 1593 * @return Returns a reference to <code>this</code> for easy method chaining 1594 */ 1595 public Library setAuthor(List<ContactDetail> theAuthor) { 1596 this.author = theAuthor; 1597 return this; 1598 } 1599 1600 public boolean hasAuthor() { 1601 if (this.author == null) 1602 return false; 1603 for (ContactDetail item : this.author) 1604 if (!item.isEmpty()) 1605 return true; 1606 return false; 1607 } 1608 1609 public ContactDetail addAuthor() { // 3 1610 ContactDetail t = new ContactDetail(); 1611 if (this.author == null) 1612 this.author = new ArrayList<ContactDetail>(); 1613 this.author.add(t); 1614 return t; 1615 } 1616 1617 public Library addAuthor(ContactDetail t) { // 3 1618 if (t == null) 1619 return this; 1620 if (this.author == null) 1621 this.author = new ArrayList<ContactDetail>(); 1622 this.author.add(t); 1623 return this; 1624 } 1625 1626 /** 1627 * @return The first repetition of repeating field {@link #author}, creating it 1628 * if it does not already exist 1629 */ 1630 public ContactDetail getAuthorFirstRep() { 1631 if (getAuthor().isEmpty()) { 1632 addAuthor(); 1633 } 1634 return getAuthor().get(0); 1635 } 1636 1637 /** 1638 * @return {@link #editor} (An individual or organization primarily responsible 1639 * for internal coherence of the content.) 1640 */ 1641 public List<ContactDetail> getEditor() { 1642 if (this.editor == null) 1643 this.editor = new ArrayList<ContactDetail>(); 1644 return this.editor; 1645 } 1646 1647 /** 1648 * @return Returns a reference to <code>this</code> for easy method chaining 1649 */ 1650 public Library setEditor(List<ContactDetail> theEditor) { 1651 this.editor = theEditor; 1652 return this; 1653 } 1654 1655 public boolean hasEditor() { 1656 if (this.editor == null) 1657 return false; 1658 for (ContactDetail item : this.editor) 1659 if (!item.isEmpty()) 1660 return true; 1661 return false; 1662 } 1663 1664 public ContactDetail addEditor() { // 3 1665 ContactDetail t = new ContactDetail(); 1666 if (this.editor == null) 1667 this.editor = new ArrayList<ContactDetail>(); 1668 this.editor.add(t); 1669 return t; 1670 } 1671 1672 public Library addEditor(ContactDetail t) { // 3 1673 if (t == null) 1674 return this; 1675 if (this.editor == null) 1676 this.editor = new ArrayList<ContactDetail>(); 1677 this.editor.add(t); 1678 return this; 1679 } 1680 1681 /** 1682 * @return The first repetition of repeating field {@link #editor}, creating it 1683 * if it does not already exist 1684 */ 1685 public ContactDetail getEditorFirstRep() { 1686 if (getEditor().isEmpty()) { 1687 addEditor(); 1688 } 1689 return getEditor().get(0); 1690 } 1691 1692 /** 1693 * @return {@link #reviewer} (An individual or organization primarily 1694 * responsible for review of some aspect of the content.) 1695 */ 1696 public List<ContactDetail> getReviewer() { 1697 if (this.reviewer == null) 1698 this.reviewer = new ArrayList<ContactDetail>(); 1699 return this.reviewer; 1700 } 1701 1702 /** 1703 * @return Returns a reference to <code>this</code> for easy method chaining 1704 */ 1705 public Library setReviewer(List<ContactDetail> theReviewer) { 1706 this.reviewer = theReviewer; 1707 return this; 1708 } 1709 1710 public boolean hasReviewer() { 1711 if (this.reviewer == null) 1712 return false; 1713 for (ContactDetail item : this.reviewer) 1714 if (!item.isEmpty()) 1715 return true; 1716 return false; 1717 } 1718 1719 public ContactDetail addReviewer() { // 3 1720 ContactDetail t = new ContactDetail(); 1721 if (this.reviewer == null) 1722 this.reviewer = new ArrayList<ContactDetail>(); 1723 this.reviewer.add(t); 1724 return t; 1725 } 1726 1727 public Library addReviewer(ContactDetail t) { // 3 1728 if (t == null) 1729 return this; 1730 if (this.reviewer == null) 1731 this.reviewer = new ArrayList<ContactDetail>(); 1732 this.reviewer.add(t); 1733 return this; 1734 } 1735 1736 /** 1737 * @return The first repetition of repeating field {@link #reviewer}, creating 1738 * it if it does not already exist 1739 */ 1740 public ContactDetail getReviewerFirstRep() { 1741 if (getReviewer().isEmpty()) { 1742 addReviewer(); 1743 } 1744 return getReviewer().get(0); 1745 } 1746 1747 /** 1748 * @return {@link #endorser} (An individual or organization responsible for 1749 * officially endorsing the content for use in some setting.) 1750 */ 1751 public List<ContactDetail> getEndorser() { 1752 if (this.endorser == null) 1753 this.endorser = new ArrayList<ContactDetail>(); 1754 return this.endorser; 1755 } 1756 1757 /** 1758 * @return Returns a reference to <code>this</code> for easy method chaining 1759 */ 1760 public Library setEndorser(List<ContactDetail> theEndorser) { 1761 this.endorser = theEndorser; 1762 return this; 1763 } 1764 1765 public boolean hasEndorser() { 1766 if (this.endorser == null) 1767 return false; 1768 for (ContactDetail item : this.endorser) 1769 if (!item.isEmpty()) 1770 return true; 1771 return false; 1772 } 1773 1774 public ContactDetail addEndorser() { // 3 1775 ContactDetail t = new ContactDetail(); 1776 if (this.endorser == null) 1777 this.endorser = new ArrayList<ContactDetail>(); 1778 this.endorser.add(t); 1779 return t; 1780 } 1781 1782 public Library addEndorser(ContactDetail t) { // 3 1783 if (t == null) 1784 return this; 1785 if (this.endorser == null) 1786 this.endorser = new ArrayList<ContactDetail>(); 1787 this.endorser.add(t); 1788 return this; 1789 } 1790 1791 /** 1792 * @return The first repetition of repeating field {@link #endorser}, creating 1793 * it if it does not already exist 1794 */ 1795 public ContactDetail getEndorserFirstRep() { 1796 if (getEndorser().isEmpty()) { 1797 addEndorser(); 1798 } 1799 return getEndorser().get(0); 1800 } 1801 1802 /** 1803 * @return {@link #relatedArtifact} (Related artifacts such as additional 1804 * documentation, justification, or bibliographic references.) 1805 */ 1806 public List<RelatedArtifact> getRelatedArtifact() { 1807 if (this.relatedArtifact == null) 1808 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1809 return this.relatedArtifact; 1810 } 1811 1812 /** 1813 * @return Returns a reference to <code>this</code> for easy method chaining 1814 */ 1815 public Library setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1816 this.relatedArtifact = theRelatedArtifact; 1817 return this; 1818 } 1819 1820 public boolean hasRelatedArtifact() { 1821 if (this.relatedArtifact == null) 1822 return false; 1823 for (RelatedArtifact item : this.relatedArtifact) 1824 if (!item.isEmpty()) 1825 return true; 1826 return false; 1827 } 1828 1829 public RelatedArtifact addRelatedArtifact() { // 3 1830 RelatedArtifact t = new RelatedArtifact(); 1831 if (this.relatedArtifact == null) 1832 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1833 this.relatedArtifact.add(t); 1834 return t; 1835 } 1836 1837 public Library addRelatedArtifact(RelatedArtifact t) { // 3 1838 if (t == null) 1839 return this; 1840 if (this.relatedArtifact == null) 1841 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1842 this.relatedArtifact.add(t); 1843 return this; 1844 } 1845 1846 /** 1847 * @return The first repetition of repeating field {@link #relatedArtifact}, 1848 * creating it if it does not already exist 1849 */ 1850 public RelatedArtifact getRelatedArtifactFirstRep() { 1851 if (getRelatedArtifact().isEmpty()) { 1852 addRelatedArtifact(); 1853 } 1854 return getRelatedArtifact().get(0); 1855 } 1856 1857 /** 1858 * @return {@link #parameter} (The parameter element defines parameters used by 1859 * the library.) 1860 */ 1861 public List<ParameterDefinition> getParameter() { 1862 if (this.parameter == null) 1863 this.parameter = new ArrayList<ParameterDefinition>(); 1864 return this.parameter; 1865 } 1866 1867 /** 1868 * @return Returns a reference to <code>this</code> for easy method chaining 1869 */ 1870 public Library setParameter(List<ParameterDefinition> theParameter) { 1871 this.parameter = theParameter; 1872 return this; 1873 } 1874 1875 public boolean hasParameter() { 1876 if (this.parameter == null) 1877 return false; 1878 for (ParameterDefinition item : this.parameter) 1879 if (!item.isEmpty()) 1880 return true; 1881 return false; 1882 } 1883 1884 public ParameterDefinition addParameter() { // 3 1885 ParameterDefinition t = new ParameterDefinition(); 1886 if (this.parameter == null) 1887 this.parameter = new ArrayList<ParameterDefinition>(); 1888 this.parameter.add(t); 1889 return t; 1890 } 1891 1892 public Library addParameter(ParameterDefinition t) { // 3 1893 if (t == null) 1894 return this; 1895 if (this.parameter == null) 1896 this.parameter = new ArrayList<ParameterDefinition>(); 1897 this.parameter.add(t); 1898 return this; 1899 } 1900 1901 /** 1902 * @return The first repetition of repeating field {@link #parameter}, creating 1903 * it if it does not already exist 1904 */ 1905 public ParameterDefinition getParameterFirstRep() { 1906 if (getParameter().isEmpty()) { 1907 addParameter(); 1908 } 1909 return getParameter().get(0); 1910 } 1911 1912 /** 1913 * @return {@link #dataRequirement} (Describes a set of data that must be 1914 * provided in order to be able to successfully perform the computations 1915 * defined by the library.) 1916 */ 1917 public List<DataRequirement> getDataRequirement() { 1918 if (this.dataRequirement == null) 1919 this.dataRequirement = new ArrayList<DataRequirement>(); 1920 return this.dataRequirement; 1921 } 1922 1923 /** 1924 * @return Returns a reference to <code>this</code> for easy method chaining 1925 */ 1926 public Library setDataRequirement(List<DataRequirement> theDataRequirement) { 1927 this.dataRequirement = theDataRequirement; 1928 return this; 1929 } 1930 1931 public boolean hasDataRequirement() { 1932 if (this.dataRequirement == null) 1933 return false; 1934 for (DataRequirement item : this.dataRequirement) 1935 if (!item.isEmpty()) 1936 return true; 1937 return false; 1938 } 1939 1940 public DataRequirement addDataRequirement() { // 3 1941 DataRequirement t = new DataRequirement(); 1942 if (this.dataRequirement == null) 1943 this.dataRequirement = new ArrayList<DataRequirement>(); 1944 this.dataRequirement.add(t); 1945 return t; 1946 } 1947 1948 public Library addDataRequirement(DataRequirement t) { // 3 1949 if (t == null) 1950 return this; 1951 if (this.dataRequirement == null) 1952 this.dataRequirement = new ArrayList<DataRequirement>(); 1953 this.dataRequirement.add(t); 1954 return this; 1955 } 1956 1957 /** 1958 * @return The first repetition of repeating field {@link #dataRequirement}, 1959 * creating it if it does not already exist 1960 */ 1961 public DataRequirement getDataRequirementFirstRep() { 1962 if (getDataRequirement().isEmpty()) { 1963 addDataRequirement(); 1964 } 1965 return getDataRequirement().get(0); 1966 } 1967 1968 /** 1969 * @return {@link #content} (The content of the library as an Attachment. The 1970 * content may be a reference to a url, or may be directly embedded as a 1971 * base-64 string. Either way, the contentType of the attachment 1972 * determines how to interpret the content.) 1973 */ 1974 public List<Attachment> getContent() { 1975 if (this.content == null) 1976 this.content = new ArrayList<Attachment>(); 1977 return this.content; 1978 } 1979 1980 /** 1981 * @return Returns a reference to <code>this</code> for easy method chaining 1982 */ 1983 public Library setContent(List<Attachment> theContent) { 1984 this.content = theContent; 1985 return this; 1986 } 1987 1988 public boolean hasContent() { 1989 if (this.content == null) 1990 return false; 1991 for (Attachment item : this.content) 1992 if (!item.isEmpty()) 1993 return true; 1994 return false; 1995 } 1996 1997 public Attachment addContent() { // 3 1998 Attachment t = new Attachment(); 1999 if (this.content == null) 2000 this.content = new ArrayList<Attachment>(); 2001 this.content.add(t); 2002 return t; 2003 } 2004 2005 public Library addContent(Attachment t) { // 3 2006 if (t == null) 2007 return this; 2008 if (this.content == null) 2009 this.content = new ArrayList<Attachment>(); 2010 this.content.add(t); 2011 return this; 2012 } 2013 2014 /** 2015 * @return The first repetition of repeating field {@link #content}, creating it 2016 * if it does not already exist 2017 */ 2018 public Attachment getContentFirstRep() { 2019 if (getContent().isEmpty()) { 2020 addContent(); 2021 } 2022 return getContent().get(0); 2023 } 2024 2025 protected void listChildren(List<Property> children) { 2026 super.listChildren(children); 2027 children.add(new Property("url", "uri", 2028 "An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers.", 2029 0, 1, url)); 2030 children.add(new Property("identifier", "Identifier", 2031 "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 2032 0, java.lang.Integer.MAX_VALUE, identifier)); 2033 children.add(new Property("version", "string", 2034 "The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 2035 0, 1, version)); 2036 children.add(new Property("name", "string", 2037 "A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2038 0, 1, name)); 2039 children.add( 2040 new Property("title", "string", "A short, descriptive, user-friendly title for the library.", 0, 1, title)); 2041 children.add(new Property("subtitle", "string", 2042 "An explanatory or alternate title for the library giving additional information about its content.", 0, 1, 2043 subtitle)); 2044 children.add(new Property("status", "code", 2045 "The status of this library. Enables tracking the life-cycle of the content.", 0, 1, status)); 2046 children.add(new Property("experimental", "boolean", 2047 "A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2048 0, 1, experimental)); 2049 children.add(new Property("type", "CodeableConcept", 2050 "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.", 2051 0, 1, type)); 2052 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 2053 "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, 2054 subject)); 2055 children.add(new Property("date", "dateTime", 2056 "The date (and optionally time) when the library was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.", 2057 0, 1, date)); 2058 children.add(new Property("publisher", "string", 2059 "The name of the organization or individual that published the library.", 0, 1, publisher)); 2060 children.add(new Property("contact", "ContactDetail", 2061 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2062 java.lang.Integer.MAX_VALUE, contact)); 2063 children.add(new Property("description", "markdown", 2064 "A free text natural language description of the library from a consumer's perspective.", 0, 1, description)); 2065 children.add(new Property("useContext", "UsageContext", 2066 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances.", 2067 0, java.lang.Integer.MAX_VALUE, useContext)); 2068 children.add(new Property("jurisdiction", "CodeableConcept", 2069 "A legal or geographic region in which the library is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 2070 jurisdiction)); 2071 children.add(new Property("purpose", "markdown", 2072 "Explanation of why this library is needed and why it has been designed as it has.", 0, 1, purpose)); 2073 children.add(new Property("usage", "string", 2074 "A detailed description of how the library is used from a clinical perspective.", 0, 1, usage)); 2075 children.add(new Property("copyright", "markdown", 2076 "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.", 2077 0, 1, copyright)); 2078 children.add(new Property("approvalDate", "date", 2079 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2080 0, 1, approvalDate)); 2081 children.add(new Property("lastReviewDate", "date", 2082 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2083 0, 1, lastReviewDate)); 2084 children.add(new Property("effectivePeriod", "Period", 2085 "The period during which the library content was or is planned to be in active use.", 0, 1, effectivePeriod)); 2086 children.add(new Property("topic", "CodeableConcept", 2087 "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.", 2088 0, java.lang.Integer.MAX_VALUE, topic)); 2089 children.add(new Property("author", "ContactDetail", 2090 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 2091 java.lang.Integer.MAX_VALUE, author)); 2092 children.add(new Property("editor", "ContactDetail", 2093 "An individual or organization primarily responsible for internal coherence of the content.", 0, 2094 java.lang.Integer.MAX_VALUE, editor)); 2095 children.add(new Property("reviewer", "ContactDetail", 2096 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 2097 java.lang.Integer.MAX_VALUE, reviewer)); 2098 children.add(new Property("endorser", "ContactDetail", 2099 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 2100 java.lang.Integer.MAX_VALUE, endorser)); 2101 children.add(new Property("relatedArtifact", "RelatedArtifact", 2102 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 2103 java.lang.Integer.MAX_VALUE, relatedArtifact)); 2104 children.add(new Property("parameter", "ParameterDefinition", 2105 "The parameter element defines parameters used by the library.", 0, java.lang.Integer.MAX_VALUE, parameter)); 2106 children.add(new Property("dataRequirement", "DataRequirement", 2107 "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.", 2108 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 2109 children.add(new Property("content", "Attachment", 2110 "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.", 2111 0, java.lang.Integer.MAX_VALUE, content)); 2112 } 2113 2114 @Override 2115 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2116 switch (_hash) { 2117 case 116079: 2118 /* url */ return new Property("url", "uri", 2119 "An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers.", 2120 0, 1, url); 2121 case -1618432855: 2122 /* identifier */ return new Property("identifier", "Identifier", 2123 "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 2124 0, java.lang.Integer.MAX_VALUE, identifier); 2125 case 351608024: 2126 /* version */ return new Property("version", "string", 2127 "The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 2128 0, 1, version); 2129 case 3373707: 2130 /* name */ return new Property("name", "string", 2131 "A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2132 0, 1, name); 2133 case 110371416: 2134 /* title */ return new Property("title", "string", "A short, descriptive, user-friendly title for the library.", 2135 0, 1, title); 2136 case -2060497896: 2137 /* subtitle */ return new Property("subtitle", "string", 2138 "An explanatory or alternate title for the library giving additional information about its content.", 0, 1, 2139 subtitle); 2140 case -892481550: 2141 /* status */ return new Property("status", "code", 2142 "The status of this library. Enables tracking the life-cycle of the content.", 0, 1, status); 2143 case -404562712: 2144 /* experimental */ return new Property("experimental", "boolean", 2145 "A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2146 0, 1, experimental); 2147 case 3575610: 2148 /* type */ return new Property("type", "CodeableConcept", 2149 "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.", 2150 0, 1, type); 2151 case -573640748: 2152 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2153 "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, 2154 subject); 2155 case -1867885268: 2156 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2157 "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, 2158 subject); 2159 case -1257122603: 2160 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2161 "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, 2162 subject); 2163 case 772938623: 2164 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2165 "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, 2166 subject); 2167 case 3076014: 2168 /* date */ return new Property("date", "dateTime", 2169 "The date (and optionally time) when the library was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.", 2170 0, 1, date); 2171 case 1447404028: 2172 /* publisher */ return new Property("publisher", "string", 2173 "The name of the organization or individual that published the library.", 0, 1, publisher); 2174 case 951526432: 2175 /* contact */ return new Property("contact", "ContactDetail", 2176 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2177 java.lang.Integer.MAX_VALUE, contact); 2178 case -1724546052: 2179 /* description */ return new Property("description", "markdown", 2180 "A free text natural language description of the library from a consumer's perspective.", 0, 1, description); 2181 case -669707736: 2182 /* useContext */ return new Property("useContext", "UsageContext", 2183 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances.", 2184 0, java.lang.Integer.MAX_VALUE, useContext); 2185 case -507075711: 2186 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 2187 "A legal or geographic region in which the library is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 2188 jurisdiction); 2189 case -220463842: 2190 /* purpose */ return new Property("purpose", "markdown", 2191 "Explanation of why this library is needed and why it has been designed as it has.", 0, 1, purpose); 2192 case 111574433: 2193 /* usage */ return new Property("usage", "string", 2194 "A detailed description of how the library is used from a clinical perspective.", 0, 1, usage); 2195 case 1522889671: 2196 /* copyright */ return new Property("copyright", "markdown", 2197 "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.", 2198 0, 1, copyright); 2199 case 223539345: 2200 /* approvalDate */ return new Property("approvalDate", "date", 2201 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2202 0, 1, approvalDate); 2203 case -1687512484: 2204 /* lastReviewDate */ return new Property("lastReviewDate", "date", 2205 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2206 0, 1, lastReviewDate); 2207 case -403934648: 2208 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 2209 "The period during which the library content was or is planned to be in active use.", 0, 1, effectivePeriod); 2210 case 110546223: 2211 /* topic */ return new Property("topic", "CodeableConcept", 2212 "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.", 2213 0, java.lang.Integer.MAX_VALUE, topic); 2214 case -1406328437: 2215 /* author */ return new Property("author", "ContactDetail", 2216 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 2217 java.lang.Integer.MAX_VALUE, author); 2218 case -1307827859: 2219 /* editor */ return new Property("editor", "ContactDetail", 2220 "An individual or organization primarily responsible for internal coherence of the content.", 0, 2221 java.lang.Integer.MAX_VALUE, editor); 2222 case -261190139: 2223 /* reviewer */ return new Property("reviewer", "ContactDetail", 2224 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 2225 java.lang.Integer.MAX_VALUE, reviewer); 2226 case 1740277666: 2227 /* endorser */ return new Property("endorser", "ContactDetail", 2228 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 2229 java.lang.Integer.MAX_VALUE, endorser); 2230 case 666807069: 2231 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 2232 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 2233 java.lang.Integer.MAX_VALUE, relatedArtifact); 2234 case 1954460585: 2235 /* parameter */ return new Property("parameter", "ParameterDefinition", 2236 "The parameter element defines parameters used by the library.", 0, java.lang.Integer.MAX_VALUE, parameter); 2237 case 629147193: 2238 /* dataRequirement */ return new Property("dataRequirement", "DataRequirement", 2239 "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.", 2240 0, java.lang.Integer.MAX_VALUE, dataRequirement); 2241 case 951530617: 2242 /* content */ return new Property("content", "Attachment", 2243 "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.", 2244 0, java.lang.Integer.MAX_VALUE, content); 2245 default: 2246 return super.getNamedProperty(_hash, _name, _checkValid); 2247 } 2248 2249 } 2250 2251 @Override 2252 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2253 switch (hash) { 2254 case 116079: 2255 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2256 case -1618432855: 2257 /* identifier */ return this.identifier == null ? new Base[0] 2258 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2259 case 351608024: 2260 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 2261 case 3373707: 2262 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2263 case 110371416: 2264 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2265 case -2060497896: 2266 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 2267 case -892481550: 2268 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 2269 case -404562712: 2270 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 2271 case 3575610: 2272 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2273 case -1867885268: 2274 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 2275 case 3076014: 2276 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2277 case 1447404028: 2278 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 2279 case 951526432: 2280 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2281 case -1724546052: 2282 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2283 case -669707736: 2284 /* useContext */ return this.useContext == null ? new Base[0] 2285 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2286 case -507075711: 2287 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 2288 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2289 case -220463842: 2290 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 2291 case 111574433: 2292 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 2293 case 1522889671: 2294 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 2295 case 223539345: 2296 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 2297 case -1687512484: 2298 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 2299 case -403934648: 2300 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 2301 case 110546223: 2302 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2303 case -1406328437: 2304 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2305 case -1307827859: 2306 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2307 case -261190139: 2308 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2309 case 1740277666: 2310 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2311 case 666807069: 2312 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 2313 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2314 case 1954460585: 2315 /* parameter */ return this.parameter == null ? new Base[0] 2316 : this.parameter.toArray(new Base[this.parameter.size()]); // ParameterDefinition 2317 case 629147193: 2318 /* dataRequirement */ return this.dataRequirement == null ? new Base[0] 2319 : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 2320 case 951530617: 2321 /* content */ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // Attachment 2322 default: 2323 return super.getProperty(hash, name, checkValid); 2324 } 2325 2326 } 2327 2328 @Override 2329 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2330 switch (hash) { 2331 case 116079: // url 2332 this.url = castToUri(value); // UriType 2333 return value; 2334 case -1618432855: // identifier 2335 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2336 return value; 2337 case 351608024: // version 2338 this.version = castToString(value); // StringType 2339 return value; 2340 case 3373707: // name 2341 this.name = castToString(value); // StringType 2342 return value; 2343 case 110371416: // title 2344 this.title = castToString(value); // StringType 2345 return value; 2346 case -2060497896: // subtitle 2347 this.subtitle = castToString(value); // StringType 2348 return value; 2349 case -892481550: // status 2350 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2351 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2352 return value; 2353 case -404562712: // experimental 2354 this.experimental = castToBoolean(value); // BooleanType 2355 return value; 2356 case 3575610: // type 2357 this.type = castToCodeableConcept(value); // CodeableConcept 2358 return value; 2359 case -1867885268: // subject 2360 this.subject = castToType(value); // Type 2361 return value; 2362 case 3076014: // date 2363 this.date = castToDateTime(value); // DateTimeType 2364 return value; 2365 case 1447404028: // publisher 2366 this.publisher = castToString(value); // StringType 2367 return value; 2368 case 951526432: // contact 2369 this.getContact().add(castToContactDetail(value)); // ContactDetail 2370 return value; 2371 case -1724546052: // description 2372 this.description = castToMarkdown(value); // MarkdownType 2373 return value; 2374 case -669707736: // useContext 2375 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2376 return value; 2377 case -507075711: // jurisdiction 2378 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2379 return value; 2380 case -220463842: // purpose 2381 this.purpose = castToMarkdown(value); // MarkdownType 2382 return value; 2383 case 111574433: // usage 2384 this.usage = castToString(value); // StringType 2385 return value; 2386 case 1522889671: // copyright 2387 this.copyright = castToMarkdown(value); // MarkdownType 2388 return value; 2389 case 223539345: // approvalDate 2390 this.approvalDate = castToDate(value); // DateType 2391 return value; 2392 case -1687512484: // lastReviewDate 2393 this.lastReviewDate = castToDate(value); // DateType 2394 return value; 2395 case -403934648: // effectivePeriod 2396 this.effectivePeriod = castToPeriod(value); // Period 2397 return value; 2398 case 110546223: // topic 2399 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 2400 return value; 2401 case -1406328437: // author 2402 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 2403 return value; 2404 case -1307827859: // editor 2405 this.getEditor().add(castToContactDetail(value)); // ContactDetail 2406 return value; 2407 case -261190139: // reviewer 2408 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 2409 return value; 2410 case 1740277666: // endorser 2411 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 2412 return value; 2413 case 666807069: // relatedArtifact 2414 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 2415 return value; 2416 case 1954460585: // parameter 2417 this.getParameter().add(castToParameterDefinition(value)); // ParameterDefinition 2418 return value; 2419 case 629147193: // dataRequirement 2420 this.getDataRequirement().add(castToDataRequirement(value)); // DataRequirement 2421 return value; 2422 case 951530617: // content 2423 this.getContent().add(castToAttachment(value)); // Attachment 2424 return value; 2425 default: 2426 return super.setProperty(hash, name, value); 2427 } 2428 2429 } 2430 2431 @Override 2432 public Base setProperty(String name, Base value) throws FHIRException { 2433 if (name.equals("url")) { 2434 this.url = castToUri(value); // UriType 2435 } else if (name.equals("identifier")) { 2436 this.getIdentifier().add(castToIdentifier(value)); 2437 } else if (name.equals("version")) { 2438 this.version = castToString(value); // StringType 2439 } else if (name.equals("name")) { 2440 this.name = castToString(value); // StringType 2441 } else if (name.equals("title")) { 2442 this.title = castToString(value); // StringType 2443 } else if (name.equals("subtitle")) { 2444 this.subtitle = castToString(value); // StringType 2445 } else if (name.equals("status")) { 2446 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2447 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2448 } else if (name.equals("experimental")) { 2449 this.experimental = castToBoolean(value); // BooleanType 2450 } else if (name.equals("type")) { 2451 this.type = castToCodeableConcept(value); // CodeableConcept 2452 } else if (name.equals("subject[x]")) { 2453 this.subject = castToType(value); // Type 2454 } else if (name.equals("date")) { 2455 this.date = castToDateTime(value); // DateTimeType 2456 } else if (name.equals("publisher")) { 2457 this.publisher = castToString(value); // StringType 2458 } else if (name.equals("contact")) { 2459 this.getContact().add(castToContactDetail(value)); 2460 } else if (name.equals("description")) { 2461 this.description = castToMarkdown(value); // MarkdownType 2462 } else if (name.equals("useContext")) { 2463 this.getUseContext().add(castToUsageContext(value)); 2464 } else if (name.equals("jurisdiction")) { 2465 this.getJurisdiction().add(castToCodeableConcept(value)); 2466 } else if (name.equals("purpose")) { 2467 this.purpose = castToMarkdown(value); // MarkdownType 2468 } else if (name.equals("usage")) { 2469 this.usage = castToString(value); // StringType 2470 } else if (name.equals("copyright")) { 2471 this.copyright = castToMarkdown(value); // MarkdownType 2472 } else if (name.equals("approvalDate")) { 2473 this.approvalDate = castToDate(value); // DateType 2474 } else if (name.equals("lastReviewDate")) { 2475 this.lastReviewDate = castToDate(value); // DateType 2476 } else if (name.equals("effectivePeriod")) { 2477 this.effectivePeriod = castToPeriod(value); // Period 2478 } else if (name.equals("topic")) { 2479 this.getTopic().add(castToCodeableConcept(value)); 2480 } else if (name.equals("author")) { 2481 this.getAuthor().add(castToContactDetail(value)); 2482 } else if (name.equals("editor")) { 2483 this.getEditor().add(castToContactDetail(value)); 2484 } else if (name.equals("reviewer")) { 2485 this.getReviewer().add(castToContactDetail(value)); 2486 } else if (name.equals("endorser")) { 2487 this.getEndorser().add(castToContactDetail(value)); 2488 } else if (name.equals("relatedArtifact")) { 2489 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 2490 } else if (name.equals("parameter")) { 2491 this.getParameter().add(castToParameterDefinition(value)); 2492 } else if (name.equals("dataRequirement")) { 2493 this.getDataRequirement().add(castToDataRequirement(value)); 2494 } else if (name.equals("content")) { 2495 this.getContent().add(castToAttachment(value)); 2496 } else 2497 return super.setProperty(name, value); 2498 return value; 2499 } 2500 2501 @Override 2502 public void removeChild(String name, Base value) throws FHIRException { 2503 if (name.equals("url")) { 2504 this.url = null; 2505 } else if (name.equals("identifier")) { 2506 this.getIdentifier().remove(castToIdentifier(value)); 2507 } else if (name.equals("version")) { 2508 this.version = null; 2509 } else if (name.equals("name")) { 2510 this.name = null; 2511 } else if (name.equals("title")) { 2512 this.title = null; 2513 } else if (name.equals("subtitle")) { 2514 this.subtitle = null; 2515 } else if (name.equals("status")) { 2516 this.status = null; 2517 } else if (name.equals("experimental")) { 2518 this.experimental = null; 2519 } else if (name.equals("type")) { 2520 this.type = null; 2521 } else if (name.equals("subject[x]")) { 2522 this.subject = null; 2523 } else if (name.equals("date")) { 2524 this.date = null; 2525 } else if (name.equals("publisher")) { 2526 this.publisher = null; 2527 } else if (name.equals("contact")) { 2528 this.getContact().remove(castToContactDetail(value)); 2529 } else if (name.equals("description")) { 2530 this.description = null; 2531 } else if (name.equals("useContext")) { 2532 this.getUseContext().remove(castToUsageContext(value)); 2533 } else if (name.equals("jurisdiction")) { 2534 this.getJurisdiction().remove(castToCodeableConcept(value)); 2535 } else if (name.equals("purpose")) { 2536 this.purpose = null; 2537 } else if (name.equals("usage")) { 2538 this.usage = null; 2539 } else if (name.equals("copyright")) { 2540 this.copyright = null; 2541 } else if (name.equals("approvalDate")) { 2542 this.approvalDate = null; 2543 } else if (name.equals("lastReviewDate")) { 2544 this.lastReviewDate = null; 2545 } else if (name.equals("effectivePeriod")) { 2546 this.effectivePeriod = null; 2547 } else if (name.equals("topic")) { 2548 this.getTopic().remove(castToCodeableConcept(value)); 2549 } else if (name.equals("author")) { 2550 this.getAuthor().remove(castToContactDetail(value)); 2551 } else if (name.equals("editor")) { 2552 this.getEditor().remove(castToContactDetail(value)); 2553 } else if (name.equals("reviewer")) { 2554 this.getReviewer().remove(castToContactDetail(value)); 2555 } else if (name.equals("endorser")) { 2556 this.getEndorser().remove(castToContactDetail(value)); 2557 } else if (name.equals("relatedArtifact")) { 2558 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 2559 } else if (name.equals("parameter")) { 2560 this.getParameter().remove(castToParameterDefinition(value)); 2561 } else if (name.equals("dataRequirement")) { 2562 this.getDataRequirement().remove(castToDataRequirement(value)); 2563 } else if (name.equals("content")) { 2564 this.getContent().remove(castToAttachment(value)); 2565 } else 2566 super.removeChild(name, value); 2567 2568 } 2569 2570 @Override 2571 public Base makeProperty(int hash, String name) throws FHIRException { 2572 switch (hash) { 2573 case 116079: 2574 return getUrlElement(); 2575 case -1618432855: 2576 return addIdentifier(); 2577 case 351608024: 2578 return getVersionElement(); 2579 case 3373707: 2580 return getNameElement(); 2581 case 110371416: 2582 return getTitleElement(); 2583 case -2060497896: 2584 return getSubtitleElement(); 2585 case -892481550: 2586 return getStatusElement(); 2587 case -404562712: 2588 return getExperimentalElement(); 2589 case 3575610: 2590 return getType(); 2591 case -573640748: 2592 return getSubject(); 2593 case -1867885268: 2594 return getSubject(); 2595 case 3076014: 2596 return getDateElement(); 2597 case 1447404028: 2598 return getPublisherElement(); 2599 case 951526432: 2600 return addContact(); 2601 case -1724546052: 2602 return getDescriptionElement(); 2603 case -669707736: 2604 return addUseContext(); 2605 case -507075711: 2606 return addJurisdiction(); 2607 case -220463842: 2608 return getPurposeElement(); 2609 case 111574433: 2610 return getUsageElement(); 2611 case 1522889671: 2612 return getCopyrightElement(); 2613 case 223539345: 2614 return getApprovalDateElement(); 2615 case -1687512484: 2616 return getLastReviewDateElement(); 2617 case -403934648: 2618 return getEffectivePeriod(); 2619 case 110546223: 2620 return addTopic(); 2621 case -1406328437: 2622 return addAuthor(); 2623 case -1307827859: 2624 return addEditor(); 2625 case -261190139: 2626 return addReviewer(); 2627 case 1740277666: 2628 return addEndorser(); 2629 case 666807069: 2630 return addRelatedArtifact(); 2631 case 1954460585: 2632 return addParameter(); 2633 case 629147193: 2634 return addDataRequirement(); 2635 case 951530617: 2636 return addContent(); 2637 default: 2638 return super.makeProperty(hash, name); 2639 } 2640 2641 } 2642 2643 @Override 2644 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2645 switch (hash) { 2646 case 116079: 2647 /* url */ return new String[] { "uri" }; 2648 case -1618432855: 2649 /* identifier */ return new String[] { "Identifier" }; 2650 case 351608024: 2651 /* version */ return new String[] { "string" }; 2652 case 3373707: 2653 /* name */ return new String[] { "string" }; 2654 case 110371416: 2655 /* title */ return new String[] { "string" }; 2656 case -2060497896: 2657 /* subtitle */ return new String[] { "string" }; 2658 case -892481550: 2659 /* status */ return new String[] { "code" }; 2660 case -404562712: 2661 /* experimental */ return new String[] { "boolean" }; 2662 case 3575610: 2663 /* type */ return new String[] { "CodeableConcept" }; 2664 case -1867885268: 2665 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 2666 case 3076014: 2667 /* date */ return new String[] { "dateTime" }; 2668 case 1447404028: 2669 /* publisher */ return new String[] { "string" }; 2670 case 951526432: 2671 /* contact */ return new String[] { "ContactDetail" }; 2672 case -1724546052: 2673 /* description */ return new String[] { "markdown" }; 2674 case -669707736: 2675 /* useContext */ return new String[] { "UsageContext" }; 2676 case -507075711: 2677 /* jurisdiction */ return new String[] { "CodeableConcept" }; 2678 case -220463842: 2679 /* purpose */ return new String[] { "markdown" }; 2680 case 111574433: 2681 /* usage */ return new String[] { "string" }; 2682 case 1522889671: 2683 /* copyright */ return new String[] { "markdown" }; 2684 case 223539345: 2685 /* approvalDate */ return new String[] { "date" }; 2686 case -1687512484: 2687 /* lastReviewDate */ return new String[] { "date" }; 2688 case -403934648: 2689 /* effectivePeriod */ return new String[] { "Period" }; 2690 case 110546223: 2691 /* topic */ return new String[] { "CodeableConcept" }; 2692 case -1406328437: 2693 /* author */ return new String[] { "ContactDetail" }; 2694 case -1307827859: 2695 /* editor */ return new String[] { "ContactDetail" }; 2696 case -261190139: 2697 /* reviewer */ return new String[] { "ContactDetail" }; 2698 case 1740277666: 2699 /* endorser */ return new String[] { "ContactDetail" }; 2700 case 666807069: 2701 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 2702 case 1954460585: 2703 /* parameter */ return new String[] { "ParameterDefinition" }; 2704 case 629147193: 2705 /* dataRequirement */ return new String[] { "DataRequirement" }; 2706 case 951530617: 2707 /* content */ return new String[] { "Attachment" }; 2708 default: 2709 return super.getTypesForProperty(hash, name); 2710 } 2711 2712 } 2713 2714 @Override 2715 public Base addChild(String name) throws FHIRException { 2716 if (name.equals("url")) { 2717 throw new FHIRException("Cannot call addChild on a singleton property Library.url"); 2718 } else if (name.equals("identifier")) { 2719 return addIdentifier(); 2720 } else if (name.equals("version")) { 2721 throw new FHIRException("Cannot call addChild on a singleton property Library.version"); 2722 } else if (name.equals("name")) { 2723 throw new FHIRException("Cannot call addChild on a singleton property Library.name"); 2724 } else if (name.equals("title")) { 2725 throw new FHIRException("Cannot call addChild on a singleton property Library.title"); 2726 } else if (name.equals("subtitle")) { 2727 throw new FHIRException("Cannot call addChild on a singleton property Library.subtitle"); 2728 } else if (name.equals("status")) { 2729 throw new FHIRException("Cannot call addChild on a singleton property Library.status"); 2730 } else if (name.equals("experimental")) { 2731 throw new FHIRException("Cannot call addChild on a singleton property Library.experimental"); 2732 } else if (name.equals("type")) { 2733 this.type = new CodeableConcept(); 2734 return this.type; 2735 } else if (name.equals("subjectCodeableConcept")) { 2736 this.subject = new CodeableConcept(); 2737 return this.subject; 2738 } else if (name.equals("subjectReference")) { 2739 this.subject = new Reference(); 2740 return this.subject; 2741 } else if (name.equals("date")) { 2742 throw new FHIRException("Cannot call addChild on a singleton property Library.date"); 2743 } else if (name.equals("publisher")) { 2744 throw new FHIRException("Cannot call addChild on a singleton property Library.publisher"); 2745 } else if (name.equals("contact")) { 2746 return addContact(); 2747 } else if (name.equals("description")) { 2748 throw new FHIRException("Cannot call addChild on a singleton property Library.description"); 2749 } else if (name.equals("useContext")) { 2750 return addUseContext(); 2751 } else if (name.equals("jurisdiction")) { 2752 return addJurisdiction(); 2753 } else if (name.equals("purpose")) { 2754 throw new FHIRException("Cannot call addChild on a singleton property Library.purpose"); 2755 } else if (name.equals("usage")) { 2756 throw new FHIRException("Cannot call addChild on a singleton property Library.usage"); 2757 } else if (name.equals("copyright")) { 2758 throw new FHIRException("Cannot call addChild on a singleton property Library.copyright"); 2759 } else if (name.equals("approvalDate")) { 2760 throw new FHIRException("Cannot call addChild on a singleton property Library.approvalDate"); 2761 } else if (name.equals("lastReviewDate")) { 2762 throw new FHIRException("Cannot call addChild on a singleton property Library.lastReviewDate"); 2763 } else if (name.equals("effectivePeriod")) { 2764 this.effectivePeriod = new Period(); 2765 return this.effectivePeriod; 2766 } else if (name.equals("topic")) { 2767 return addTopic(); 2768 } else if (name.equals("author")) { 2769 return addAuthor(); 2770 } else if (name.equals("editor")) { 2771 return addEditor(); 2772 } else if (name.equals("reviewer")) { 2773 return addReviewer(); 2774 } else if (name.equals("endorser")) { 2775 return addEndorser(); 2776 } else if (name.equals("relatedArtifact")) { 2777 return addRelatedArtifact(); 2778 } else if (name.equals("parameter")) { 2779 return addParameter(); 2780 } else if (name.equals("dataRequirement")) { 2781 return addDataRequirement(); 2782 } else if (name.equals("content")) { 2783 return addContent(); 2784 } else 2785 return super.addChild(name); 2786 } 2787 2788 public String fhirType() { 2789 return "Library"; 2790 2791 } 2792 2793 public Library copy() { 2794 Library dst = new Library(); 2795 copyValues(dst); 2796 return dst; 2797 } 2798 2799 public void copyValues(Library dst) { 2800 super.copyValues(dst); 2801 dst.url = url == null ? null : url.copy(); 2802 if (identifier != null) { 2803 dst.identifier = new ArrayList<Identifier>(); 2804 for (Identifier i : identifier) 2805 dst.identifier.add(i.copy()); 2806 } 2807 ; 2808 dst.version = version == null ? null : version.copy(); 2809 dst.name = name == null ? null : name.copy(); 2810 dst.title = title == null ? null : title.copy(); 2811 dst.subtitle = subtitle == null ? null : subtitle.copy(); 2812 dst.status = status == null ? null : status.copy(); 2813 dst.experimental = experimental == null ? null : experimental.copy(); 2814 dst.type = type == null ? null : type.copy(); 2815 dst.subject = subject == null ? null : subject.copy(); 2816 dst.date = date == null ? null : date.copy(); 2817 dst.publisher = publisher == null ? null : publisher.copy(); 2818 if (contact != null) { 2819 dst.contact = new ArrayList<ContactDetail>(); 2820 for (ContactDetail i : contact) 2821 dst.contact.add(i.copy()); 2822 } 2823 ; 2824 dst.description = description == null ? null : description.copy(); 2825 if (useContext != null) { 2826 dst.useContext = new ArrayList<UsageContext>(); 2827 for (UsageContext i : useContext) 2828 dst.useContext.add(i.copy()); 2829 } 2830 ; 2831 if (jurisdiction != null) { 2832 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2833 for (CodeableConcept i : jurisdiction) 2834 dst.jurisdiction.add(i.copy()); 2835 } 2836 ; 2837 dst.purpose = purpose == null ? null : purpose.copy(); 2838 dst.usage = usage == null ? null : usage.copy(); 2839 dst.copyright = copyright == null ? null : copyright.copy(); 2840 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2841 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2842 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 2843 if (topic != null) { 2844 dst.topic = new ArrayList<CodeableConcept>(); 2845 for (CodeableConcept i : topic) 2846 dst.topic.add(i.copy()); 2847 } 2848 ; 2849 if (author != null) { 2850 dst.author = new ArrayList<ContactDetail>(); 2851 for (ContactDetail i : author) 2852 dst.author.add(i.copy()); 2853 } 2854 ; 2855 if (editor != null) { 2856 dst.editor = new ArrayList<ContactDetail>(); 2857 for (ContactDetail i : editor) 2858 dst.editor.add(i.copy()); 2859 } 2860 ; 2861 if (reviewer != null) { 2862 dst.reviewer = new ArrayList<ContactDetail>(); 2863 for (ContactDetail i : reviewer) 2864 dst.reviewer.add(i.copy()); 2865 } 2866 ; 2867 if (endorser != null) { 2868 dst.endorser = new ArrayList<ContactDetail>(); 2869 for (ContactDetail i : endorser) 2870 dst.endorser.add(i.copy()); 2871 } 2872 ; 2873 if (relatedArtifact != null) { 2874 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 2875 for (RelatedArtifact i : relatedArtifact) 2876 dst.relatedArtifact.add(i.copy()); 2877 } 2878 ; 2879 if (parameter != null) { 2880 dst.parameter = new ArrayList<ParameterDefinition>(); 2881 for (ParameterDefinition i : parameter) 2882 dst.parameter.add(i.copy()); 2883 } 2884 ; 2885 if (dataRequirement != null) { 2886 dst.dataRequirement = new ArrayList<DataRequirement>(); 2887 for (DataRequirement i : dataRequirement) 2888 dst.dataRequirement.add(i.copy()); 2889 } 2890 ; 2891 if (content != null) { 2892 dst.content = new ArrayList<Attachment>(); 2893 for (Attachment i : content) 2894 dst.content.add(i.copy()); 2895 } 2896 ; 2897 } 2898 2899 protected Library typedCopy() { 2900 return copy(); 2901 } 2902 2903 @Override 2904 public boolean equalsDeep(Base other_) { 2905 if (!super.equalsDeep(other_)) 2906 return false; 2907 if (!(other_ instanceof Library)) 2908 return false; 2909 Library o = (Library) other_; 2910 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 2911 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 2912 && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 2913 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 2914 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 2915 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 2916 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 2917 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 2918 && compareDeep(parameter, o.parameter, true) && compareDeep(dataRequirement, o.dataRequirement, true) 2919 && compareDeep(content, o.content, true); 2920 } 2921 2922 @Override 2923 public boolean equalsShallow(Base other_) { 2924 if (!super.equalsShallow(other_)) 2925 return false; 2926 if (!(other_ instanceof Library)) 2927 return false; 2928 Library o = (Library) other_; 2929 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 2930 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 2931 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 2932 } 2933 2934 public boolean isEmpty() { 2935 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, type, subject, purpose, usage, 2936 copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, 2937 relatedArtifact, parameter, dataRequirement, content); 2938 } 2939 2940 @Override 2941 public ResourceType getResourceType() { 2942 return ResourceType.Library; 2943 } 2944 2945 /** 2946 * Search parameter: <b>date</b> 2947 * <p> 2948 * Description: <b>The library publication date</b><br> 2949 * Type: <b>date</b><br> 2950 * Path: <b>Library.date</b><br> 2951 * </p> 2952 */ 2953 @SearchParamDefinition(name = "date", path = "Library.date", description = "The library publication date", type = "date") 2954 public static final String SP_DATE = "date"; 2955 /** 2956 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2957 * <p> 2958 * Description: <b>The library publication date</b><br> 2959 * Type: <b>date</b><br> 2960 * Path: <b>Library.date</b><br> 2961 * </p> 2962 */ 2963 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2964 SP_DATE); 2965 2966 /** 2967 * Search parameter: <b>identifier</b> 2968 * <p> 2969 * Description: <b>External identifier for the library</b><br> 2970 * Type: <b>token</b><br> 2971 * Path: <b>Library.identifier</b><br> 2972 * </p> 2973 */ 2974 @SearchParamDefinition(name = "identifier", path = "Library.identifier", description = "External identifier for the library", type = "token") 2975 public static final String SP_IDENTIFIER = "identifier"; 2976 /** 2977 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2978 * <p> 2979 * Description: <b>External identifier for the library</b><br> 2980 * Type: <b>token</b><br> 2981 * Path: <b>Library.identifier</b><br> 2982 * </p> 2983 */ 2984 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2985 SP_IDENTIFIER); 2986 2987 /** 2988 * Search parameter: <b>successor</b> 2989 * <p> 2990 * Description: <b>What resource is being referenced</b><br> 2991 * Type: <b>reference</b><br> 2992 * Path: <b>Library.relatedArtifact.resource</b><br> 2993 * </p> 2994 */ 2995 @SearchParamDefinition(name = "successor", path = "Library.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 2996 public static final String SP_SUCCESSOR = "successor"; 2997 /** 2998 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 2999 * <p> 3000 * Description: <b>What resource is being referenced</b><br> 3001 * Type: <b>reference</b><br> 3002 * Path: <b>Library.relatedArtifact.resource</b><br> 3003 * </p> 3004 */ 3005 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3006 SP_SUCCESSOR); 3007 3008 /** 3009 * Constant for fluent queries to be used to add include statements. Specifies 3010 * the path value of "<b>Library:successor</b>". 3011 */ 3012 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 3013 "Library:successor").toLocked(); 3014 3015 /** 3016 * Search parameter: <b>context-type-value</b> 3017 * <p> 3018 * Description: <b>A use context type and value assigned to the library</b><br> 3019 * Type: <b>composite</b><br> 3020 * Path: <b></b><br> 3021 * </p> 3022 */ 3023 @SearchParamDefinition(name = "context-type-value", path = "Library.useContext", description = "A use context type and value assigned to the library", type = "composite", compositeOf = { 3024 "context-type", "context" }) 3025 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3026 /** 3027 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3028 * <p> 3029 * Description: <b>A use context type and value assigned to the library</b><br> 3030 * Type: <b>composite</b><br> 3031 * Path: <b></b><br> 3032 * </p> 3033 */ 3034 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3035 SP_CONTEXT_TYPE_VALUE); 3036 3037 /** 3038 * Search parameter: <b>jurisdiction</b> 3039 * <p> 3040 * Description: <b>Intended jurisdiction for the library</b><br> 3041 * Type: <b>token</b><br> 3042 * Path: <b>Library.jurisdiction</b><br> 3043 * </p> 3044 */ 3045 @SearchParamDefinition(name = "jurisdiction", path = "Library.jurisdiction", description = "Intended jurisdiction for the library", type = "token") 3046 public static final String SP_JURISDICTION = "jurisdiction"; 3047 /** 3048 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3049 * <p> 3050 * Description: <b>Intended jurisdiction for the library</b><br> 3051 * Type: <b>token</b><br> 3052 * Path: <b>Library.jurisdiction</b><br> 3053 * </p> 3054 */ 3055 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3056 SP_JURISDICTION); 3057 3058 /** 3059 * Search parameter: <b>description</b> 3060 * <p> 3061 * Description: <b>The description of the library</b><br> 3062 * Type: <b>string</b><br> 3063 * Path: <b>Library.description</b><br> 3064 * </p> 3065 */ 3066 @SearchParamDefinition(name = "description", path = "Library.description", description = "The description of the library", type = "string") 3067 public static final String SP_DESCRIPTION = "description"; 3068 /** 3069 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3070 * <p> 3071 * Description: <b>The description of the library</b><br> 3072 * Type: <b>string</b><br> 3073 * Path: <b>Library.description</b><br> 3074 * </p> 3075 */ 3076 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3077 SP_DESCRIPTION); 3078 3079 /** 3080 * Search parameter: <b>derived-from</b> 3081 * <p> 3082 * Description: <b>What resource is being referenced</b><br> 3083 * Type: <b>reference</b><br> 3084 * Path: <b>Library.relatedArtifact.resource</b><br> 3085 * </p> 3086 */ 3087 @SearchParamDefinition(name = "derived-from", path = "Library.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 3088 public static final String SP_DERIVED_FROM = "derived-from"; 3089 /** 3090 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3091 * <p> 3092 * Description: <b>What resource is being referenced</b><br> 3093 * Type: <b>reference</b><br> 3094 * Path: <b>Library.relatedArtifact.resource</b><br> 3095 * </p> 3096 */ 3097 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3098 SP_DERIVED_FROM); 3099 3100 /** 3101 * Constant for fluent queries to be used to add include statements. Specifies 3102 * the path value of "<b>Library:derived-from</b>". 3103 */ 3104 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 3105 "Library:derived-from").toLocked(); 3106 3107 /** 3108 * Search parameter: <b>context-type</b> 3109 * <p> 3110 * Description: <b>A type of use context assigned to the library</b><br> 3111 * Type: <b>token</b><br> 3112 * Path: <b>Library.useContext.code</b><br> 3113 * </p> 3114 */ 3115 @SearchParamDefinition(name = "context-type", path = "Library.useContext.code", description = "A type of use context assigned to the library", type = "token") 3116 public static final String SP_CONTEXT_TYPE = "context-type"; 3117 /** 3118 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3119 * <p> 3120 * Description: <b>A type of use context assigned to the library</b><br> 3121 * Type: <b>token</b><br> 3122 * Path: <b>Library.useContext.code</b><br> 3123 * </p> 3124 */ 3125 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3126 SP_CONTEXT_TYPE); 3127 3128 /** 3129 * Search parameter: <b>predecessor</b> 3130 * <p> 3131 * Description: <b>What resource is being referenced</b><br> 3132 * Type: <b>reference</b><br> 3133 * Path: <b>Library.relatedArtifact.resource</b><br> 3134 * </p> 3135 */ 3136 @SearchParamDefinition(name = "predecessor", path = "Library.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 3137 public static final String SP_PREDECESSOR = "predecessor"; 3138 /** 3139 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 3140 * <p> 3141 * Description: <b>What resource is being referenced</b><br> 3142 * Type: <b>reference</b><br> 3143 * Path: <b>Library.relatedArtifact.resource</b><br> 3144 * </p> 3145 */ 3146 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3147 SP_PREDECESSOR); 3148 3149 /** 3150 * Constant for fluent queries to be used to add include statements. Specifies 3151 * the path value of "<b>Library:predecessor</b>". 3152 */ 3153 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 3154 "Library:predecessor").toLocked(); 3155 3156 /** 3157 * Search parameter: <b>title</b> 3158 * <p> 3159 * Description: <b>The human-friendly name of the library</b><br> 3160 * Type: <b>string</b><br> 3161 * Path: <b>Library.title</b><br> 3162 * </p> 3163 */ 3164 @SearchParamDefinition(name = "title", path = "Library.title", description = "The human-friendly name of the library", type = "string") 3165 public static final String SP_TITLE = "title"; 3166 /** 3167 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3168 * <p> 3169 * Description: <b>The human-friendly name of the library</b><br> 3170 * Type: <b>string</b><br> 3171 * Path: <b>Library.title</b><br> 3172 * </p> 3173 */ 3174 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3175 SP_TITLE); 3176 3177 /** 3178 * Search parameter: <b>composed-of</b> 3179 * <p> 3180 * Description: <b>What resource is being referenced</b><br> 3181 * Type: <b>reference</b><br> 3182 * Path: <b>Library.relatedArtifact.resource</b><br> 3183 * </p> 3184 */ 3185 @SearchParamDefinition(name = "composed-of", path = "Library.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 3186 public static final String SP_COMPOSED_OF = "composed-of"; 3187 /** 3188 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 3189 * <p> 3190 * Description: <b>What resource is being referenced</b><br> 3191 * Type: <b>reference</b><br> 3192 * Path: <b>Library.relatedArtifact.resource</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3196 SP_COMPOSED_OF); 3197 3198 /** 3199 * Constant for fluent queries to be used to add include statements. Specifies 3200 * the path value of "<b>Library:composed-of</b>". 3201 */ 3202 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 3203 "Library:composed-of").toLocked(); 3204 3205 /** 3206 * Search parameter: <b>type</b> 3207 * <p> 3208 * Description: <b>The type of the library (e.g. logic-library, 3209 * model-definition, asset-collection, module-definition)</b><br> 3210 * Type: <b>token</b><br> 3211 * Path: <b>Library.type</b><br> 3212 * </p> 3213 */ 3214 @SearchParamDefinition(name = "type", path = "Library.type", description = "The type of the library (e.g. logic-library, model-definition, asset-collection, module-definition)", type = "token") 3215 public static final String SP_TYPE = "type"; 3216 /** 3217 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3218 * <p> 3219 * Description: <b>The type of the library (e.g. logic-library, 3220 * model-definition, asset-collection, module-definition)</b><br> 3221 * Type: <b>token</b><br> 3222 * Path: <b>Library.type</b><br> 3223 * </p> 3224 */ 3225 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3226 SP_TYPE); 3227 3228 /** 3229 * Search parameter: <b>version</b> 3230 * <p> 3231 * Description: <b>The business version of the library</b><br> 3232 * Type: <b>token</b><br> 3233 * Path: <b>Library.version</b><br> 3234 * </p> 3235 */ 3236 @SearchParamDefinition(name = "version", path = "Library.version", description = "The business version of the library", type = "token") 3237 public static final String SP_VERSION = "version"; 3238 /** 3239 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3240 * <p> 3241 * Description: <b>The business version of the library</b><br> 3242 * Type: <b>token</b><br> 3243 * Path: <b>Library.version</b><br> 3244 * </p> 3245 */ 3246 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3247 SP_VERSION); 3248 3249 /** 3250 * Search parameter: <b>url</b> 3251 * <p> 3252 * Description: <b>The uri that identifies the library</b><br> 3253 * Type: <b>uri</b><br> 3254 * Path: <b>Library.url</b><br> 3255 * </p> 3256 */ 3257 @SearchParamDefinition(name = "url", path = "Library.url", description = "The uri that identifies the library", type = "uri") 3258 public static final String SP_URL = "url"; 3259 /** 3260 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3261 * <p> 3262 * Description: <b>The uri that identifies the library</b><br> 3263 * Type: <b>uri</b><br> 3264 * Path: <b>Library.url</b><br> 3265 * </p> 3266 */ 3267 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3268 3269 /** 3270 * Search parameter: <b>context-quantity</b> 3271 * <p> 3272 * Description: <b>A quantity- or range-valued use context assigned to the 3273 * library</b><br> 3274 * Type: <b>quantity</b><br> 3275 * Path: <b>Library.useContext.valueQuantity, 3276 * Library.useContext.valueRange</b><br> 3277 * </p> 3278 */ 3279 @SearchParamDefinition(name = "context-quantity", path = "(Library.useContext.value as Quantity) | (Library.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the library", type = "quantity") 3280 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3281 /** 3282 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3283 * <p> 3284 * Description: <b>A quantity- or range-valued use context assigned to the 3285 * library</b><br> 3286 * Type: <b>quantity</b><br> 3287 * Path: <b>Library.useContext.valueQuantity, 3288 * Library.useContext.valueRange</b><br> 3289 * </p> 3290 */ 3291 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3292 SP_CONTEXT_QUANTITY); 3293 3294 /** 3295 * Search parameter: <b>effective</b> 3296 * <p> 3297 * Description: <b>The time during which the library is intended to be in 3298 * use</b><br> 3299 * Type: <b>date</b><br> 3300 * Path: <b>Library.effectivePeriod</b><br> 3301 * </p> 3302 */ 3303 @SearchParamDefinition(name = "effective", path = "Library.effectivePeriod", description = "The time during which the library is intended to be in use", type = "date") 3304 public static final String SP_EFFECTIVE = "effective"; 3305 /** 3306 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3307 * <p> 3308 * Description: <b>The time during which the library is intended to be in 3309 * use</b><br> 3310 * Type: <b>date</b><br> 3311 * Path: <b>Library.effectivePeriod</b><br> 3312 * </p> 3313 */ 3314 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3315 SP_EFFECTIVE); 3316 3317 /** 3318 * Search parameter: <b>depends-on</b> 3319 * <p> 3320 * Description: <b>What resource is being referenced</b><br> 3321 * Type: <b>reference</b><br> 3322 * Path: <b>Library.relatedArtifact.resource</b><br> 3323 * </p> 3324 */ 3325 @SearchParamDefinition(name = "depends-on", path = "Library.relatedArtifact.where(type='depends-on').resource", description = "What resource is being referenced", type = "reference") 3326 public static final String SP_DEPENDS_ON = "depends-on"; 3327 /** 3328 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 3329 * <p> 3330 * Description: <b>What resource is being referenced</b><br> 3331 * Type: <b>reference</b><br> 3332 * Path: <b>Library.relatedArtifact.resource</b><br> 3333 * </p> 3334 */ 3335 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3336 SP_DEPENDS_ON); 3337 3338 /** 3339 * Constant for fluent queries to be used to add include statements. Specifies 3340 * the path value of "<b>Library:depends-on</b>". 3341 */ 3342 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 3343 "Library:depends-on").toLocked(); 3344 3345 /** 3346 * Search parameter: <b>name</b> 3347 * <p> 3348 * Description: <b>Computationally friendly name of the library</b><br> 3349 * Type: <b>string</b><br> 3350 * Path: <b>Library.name</b><br> 3351 * </p> 3352 */ 3353 @SearchParamDefinition(name = "name", path = "Library.name", description = "Computationally friendly name of the library", type = "string") 3354 public static final String SP_NAME = "name"; 3355 /** 3356 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3357 * <p> 3358 * Description: <b>Computationally friendly name of the library</b><br> 3359 * Type: <b>string</b><br> 3360 * Path: <b>Library.name</b><br> 3361 * </p> 3362 */ 3363 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3364 SP_NAME); 3365 3366 /** 3367 * Search parameter: <b>context</b> 3368 * <p> 3369 * Description: <b>A use context assigned to the library</b><br> 3370 * Type: <b>token</b><br> 3371 * Path: <b>Library.useContext.valueCodeableConcept</b><br> 3372 * </p> 3373 */ 3374 @SearchParamDefinition(name = "context", path = "(Library.useContext.value as CodeableConcept)", description = "A use context assigned to the library", type = "token") 3375 public static final String SP_CONTEXT = "context"; 3376 /** 3377 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3378 * <p> 3379 * Description: <b>A use context assigned to the library</b><br> 3380 * Type: <b>token</b><br> 3381 * Path: <b>Library.useContext.valueCodeableConcept</b><br> 3382 * </p> 3383 */ 3384 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3385 SP_CONTEXT); 3386 3387 /** 3388 * Search parameter: <b>publisher</b> 3389 * <p> 3390 * Description: <b>Name of the publisher of the library</b><br> 3391 * Type: <b>string</b><br> 3392 * Path: <b>Library.publisher</b><br> 3393 * </p> 3394 */ 3395 @SearchParamDefinition(name = "publisher", path = "Library.publisher", description = "Name of the publisher of the library", type = "string") 3396 public static final String SP_PUBLISHER = "publisher"; 3397 /** 3398 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3399 * <p> 3400 * Description: <b>Name of the publisher of the library</b><br> 3401 * Type: <b>string</b><br> 3402 * Path: <b>Library.publisher</b><br> 3403 * </p> 3404 */ 3405 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3406 SP_PUBLISHER); 3407 3408 /** 3409 * Search parameter: <b>topic</b> 3410 * <p> 3411 * Description: <b>Topics associated with the module</b><br> 3412 * Type: <b>token</b><br> 3413 * Path: <b>Library.topic</b><br> 3414 * </p> 3415 */ 3416 @SearchParamDefinition(name = "topic", path = "Library.topic", description = "Topics associated with the module", type = "token") 3417 public static final String SP_TOPIC = "topic"; 3418 /** 3419 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 3420 * <p> 3421 * Description: <b>Topics associated with the module</b><br> 3422 * Type: <b>token</b><br> 3423 * Path: <b>Library.topic</b><br> 3424 * </p> 3425 */ 3426 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3427 SP_TOPIC); 3428 3429 /** 3430 * Search parameter: <b>content-type</b> 3431 * <p> 3432 * Description: <b>The type of content in the library (e.g. text/cql)</b><br> 3433 * Type: <b>token</b><br> 3434 * Path: <b>Library.content.contentType</b><br> 3435 * </p> 3436 */ 3437 @SearchParamDefinition(name = "content-type", path = "Library.content.contentType", description = "The type of content in the library (e.g. text/cql)", type = "token") 3438 public static final String SP_CONTENT_TYPE = "content-type"; 3439 /** 3440 * <b>Fluent Client</b> search parameter constant for <b>content-type</b> 3441 * <p> 3442 * Description: <b>The type of content in the library (e.g. text/cql)</b><br> 3443 * Type: <b>token</b><br> 3444 * Path: <b>Library.content.contentType</b><br> 3445 * </p> 3446 */ 3447 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3448 SP_CONTENT_TYPE); 3449 3450 /** 3451 * Search parameter: <b>context-type-quantity</b> 3452 * <p> 3453 * Description: <b>A use context type and quantity- or range-based value 3454 * assigned to the library</b><br> 3455 * Type: <b>composite</b><br> 3456 * Path: <b></b><br> 3457 * </p> 3458 */ 3459 @SearchParamDefinition(name = "context-type-quantity", path = "Library.useContext", description = "A use context type and quantity- or range-based value assigned to the library", type = "composite", compositeOf = { 3460 "context-type", "context-quantity" }) 3461 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3462 /** 3463 * <b>Fluent Client</b> search parameter constant for 3464 * <b>context-type-quantity</b> 3465 * <p> 3466 * Description: <b>A use context type and quantity- or range-based value 3467 * assigned to the library</b><br> 3468 * Type: <b>composite</b><br> 3469 * Path: <b></b><br> 3470 * </p> 3471 */ 3472 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3473 SP_CONTEXT_TYPE_QUANTITY); 3474 3475 /** 3476 * Search parameter: <b>status</b> 3477 * <p> 3478 * Description: <b>The current status of the library</b><br> 3479 * Type: <b>token</b><br> 3480 * Path: <b>Library.status</b><br> 3481 * </p> 3482 */ 3483 @SearchParamDefinition(name = "status", path = "Library.status", description = "The current status of the library", type = "token") 3484 public static final String SP_STATUS = "status"; 3485 /** 3486 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3487 * <p> 3488 * Description: <b>The current status of the library</b><br> 3489 * Type: <b>token</b><br> 3490 * Path: <b>Library.status</b><br> 3491 * </p> 3492 */ 3493 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3494 SP_STATUS); 3495 3496}