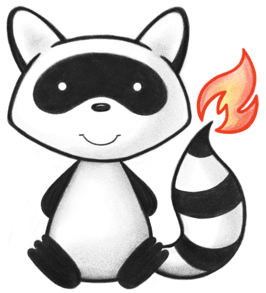
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * Identifies two or more records (resource instances) that refer to the same 047 * real-world "occurrence". 048 */ 049@ResourceDef(name = "Linkage", profile = "http://hl7.org/fhir/StructureDefinition/Linkage") 050public class Linkage extends DomainResource { 051 052 public enum LinkageType { 053 /** 054 * The resource represents the "source of truth" (from the perspective of this 055 * Linkage resource) for the underlying event/condition/etc. 056 */ 057 SOURCE, 058 /** 059 * The resource represents an alternative view of the underlying 060 * event/condition/etc. The resource may still be actively maintained, even 061 * though it is not considered to be the source of truth. 062 */ 063 ALTERNATE, 064 /** 065 * The resource represents an obsolete record of the underlying 066 * event/condition/etc. It is not expected to be actively maintained. 067 */ 068 HISTORICAL, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 074 public static LinkageType fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("source".equals(codeString)) 078 return SOURCE; 079 if ("alternate".equals(codeString)) 080 return ALTERNATE; 081 if ("historical".equals(codeString)) 082 return HISTORICAL; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown LinkageType code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case SOURCE: 092 return "source"; 093 case ALTERNATE: 094 return "alternate"; 095 case HISTORICAL: 096 return "historical"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case SOURCE: 107 return "http://hl7.org/fhir/linkage-type"; 108 case ALTERNATE: 109 return "http://hl7.org/fhir/linkage-type"; 110 case HISTORICAL: 111 return "http://hl7.org/fhir/linkage-type"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case SOURCE: 122 return "The resource represents the \"source of truth\" (from the perspective of this Linkage resource) for the underlying event/condition/etc."; 123 case ALTERNATE: 124 return "The resource represents an alternative view of the underlying event/condition/etc. The resource may still be actively maintained, even though it is not considered to be the source of truth."; 125 case HISTORICAL: 126 return "The resource represents an obsolete record of the underlying event/condition/etc. It is not expected to be actively maintained."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case SOURCE: 137 return "Source of Truth"; 138 case ALTERNATE: 139 return "Alternate Record"; 140 case HISTORICAL: 141 return "Historical/Obsolete Record"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class LinkageTypeEnumFactory implements EnumFactory<LinkageType> { 151 public LinkageType fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("source".equals(codeString)) 156 return LinkageType.SOURCE; 157 if ("alternate".equals(codeString)) 158 return LinkageType.ALTERNATE; 159 if ("historical".equals(codeString)) 160 return LinkageType.HISTORICAL; 161 throw new IllegalArgumentException("Unknown LinkageType code '" + codeString + "'"); 162 } 163 164 public Enumeration<LinkageType> fromType(PrimitiveType<?> code) throws FHIRException { 165 if (code == null) 166 return null; 167 if (code.isEmpty()) 168 return new Enumeration<LinkageType>(this, LinkageType.NULL, code); 169 String codeString = code.asStringValue(); 170 if (codeString == null || "".equals(codeString)) 171 return new Enumeration<LinkageType>(this, LinkageType.NULL, code); 172 if ("source".equals(codeString)) 173 return new Enumeration<LinkageType>(this, LinkageType.SOURCE, code); 174 if ("alternate".equals(codeString)) 175 return new Enumeration<LinkageType>(this, LinkageType.ALTERNATE, code); 176 if ("historical".equals(codeString)) 177 return new Enumeration<LinkageType>(this, LinkageType.HISTORICAL, code); 178 throw new FHIRException("Unknown LinkageType code '" + codeString + "'"); 179 } 180 181 public String toCode(LinkageType code) { 182 if (code == LinkageType.NULL) 183 return null; 184 if (code == LinkageType.SOURCE) 185 return "source"; 186 if (code == LinkageType.ALTERNATE) 187 return "alternate"; 188 if (code == LinkageType.HISTORICAL) 189 return "historical"; 190 return "?"; 191 } 192 193 public String toSystem(LinkageType code) { 194 return code.getSystem(); 195 } 196 } 197 198 @Block() 199 public static class LinkageItemComponent extends BackboneElement implements IBaseBackboneElement { 200 /** 201 * Distinguishes which item is "source of truth" (if any) and which items are no 202 * longer considered to be current representations. 203 */ 204 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 205 @Description(shortDefinition = "source | alternate | historical", formalDefinition = "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.") 206 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/linkage-type") 207 protected Enumeration<LinkageType> type; 208 209 /** 210 * The resource instance being linked as part of the group. 211 */ 212 @Child(name = "resource", type = { Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 213 @Description(shortDefinition = "Resource being linked", formalDefinition = "The resource instance being linked as part of the group.") 214 protected Reference resource; 215 216 /** 217 * The actual object that is the target of the reference (The resource instance 218 * being linked as part of the group.) 219 */ 220 protected Resource resourceTarget; 221 222 private static final long serialVersionUID = -209332008L; 223 224 /** 225 * Constructor 226 */ 227 public LinkageItemComponent() { 228 super(); 229 } 230 231 /** 232 * Constructor 233 */ 234 public LinkageItemComponent(Enumeration<LinkageType> type, Reference resource) { 235 super(); 236 this.type = type; 237 this.resource = resource; 238 } 239 240 /** 241 * @return {@link #type} (Distinguishes which item is "source of truth" (if any) 242 * and which items are no longer considered to be current 243 * representations.). This is the underlying object with id, value and 244 * extensions. The accessor "getType" gives direct access to the value 245 */ 246 public Enumeration<LinkageType> getTypeElement() { 247 if (this.type == null) 248 if (Configuration.errorOnAutoCreate()) 249 throw new Error("Attempt to auto-create LinkageItemComponent.type"); 250 else if (Configuration.doAutoCreate()) 251 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); // bb 252 return this.type; 253 } 254 255 public boolean hasTypeElement() { 256 return this.type != null && !this.type.isEmpty(); 257 } 258 259 public boolean hasType() { 260 return this.type != null && !this.type.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #type} (Distinguishes which item is "source of truth" (if 265 * any) and which items are no longer considered to be current 266 * representations.). This is the underlying object with id, value 267 * and extensions. The accessor "getType" gives direct access to 268 * the value 269 */ 270 public LinkageItemComponent setTypeElement(Enumeration<LinkageType> value) { 271 this.type = value; 272 return this; 273 } 274 275 /** 276 * @return Distinguishes which item is "source of truth" (if any) and which 277 * items are no longer considered to be current representations. 278 */ 279 public LinkageType getType() { 280 return this.type == null ? null : this.type.getValue(); 281 } 282 283 /** 284 * @param value Distinguishes which item is "source of truth" (if any) and which 285 * items are no longer considered to be current representations. 286 */ 287 public LinkageItemComponent setType(LinkageType value) { 288 if (this.type == null) 289 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); 290 this.type.setValue(value); 291 return this; 292 } 293 294 /** 295 * @return {@link #resource} (The resource instance being linked as part of the 296 * group.) 297 */ 298 public Reference getResource() { 299 if (this.resource == null) 300 if (Configuration.errorOnAutoCreate()) 301 throw new Error("Attempt to auto-create LinkageItemComponent.resource"); 302 else if (Configuration.doAutoCreate()) 303 this.resource = new Reference(); // cc 304 return this.resource; 305 } 306 307 public boolean hasResource() { 308 return this.resource != null && !this.resource.isEmpty(); 309 } 310 311 /** 312 * @param value {@link #resource} (The resource instance being linked as part of 313 * the group.) 314 */ 315 public LinkageItemComponent setResource(Reference value) { 316 this.resource = value; 317 return this; 318 } 319 320 /** 321 * @return {@link #resource} The actual object that is the target of the 322 * reference. The reference library doesn't populate this, but you can 323 * use it to hold the resource if you resolve it. (The resource instance 324 * being linked as part of the group.) 325 */ 326 public Resource getResourceTarget() { 327 return this.resourceTarget; 328 } 329 330 /** 331 * @param value {@link #resource} The actual object that is the target of the 332 * reference. The reference library doesn't use these, but you can 333 * use it to hold the resource if you resolve it. (The resource 334 * instance being linked as part of the group.) 335 */ 336 public LinkageItemComponent setResourceTarget(Resource value) { 337 this.resourceTarget = value; 338 return this; 339 } 340 341 protected void listChildren(List<Property> children) { 342 super.listChildren(children); 343 children.add(new Property("type", "code", 344 "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 345 0, 1, type)); 346 children.add(new Property("resource", "Reference(Any)", 347 "The resource instance being linked as part of the group.", 0, 1, resource)); 348 } 349 350 @Override 351 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 352 switch (_hash) { 353 case 3575610: 354 /* type */ return new Property("type", "code", 355 "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 356 0, 1, type); 357 case -341064690: 358 /* resource */ return new Property("resource", "Reference(Any)", 359 "The resource instance being linked as part of the group.", 0, 1, resource); 360 default: 361 return super.getNamedProperty(_hash, _name, _checkValid); 362 } 363 364 } 365 366 @Override 367 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 368 switch (hash) { 369 case 3575610: 370 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<LinkageType> 371 case -341064690: 372 /* resource */ return this.resource == null ? new Base[0] : new Base[] { this.resource }; // Reference 373 default: 374 return super.getProperty(hash, name, checkValid); 375 } 376 377 } 378 379 @Override 380 public Base setProperty(int hash, String name, Base value) throws FHIRException { 381 switch (hash) { 382 case 3575610: // type 383 value = new LinkageTypeEnumFactory().fromType(castToCode(value)); 384 this.type = (Enumeration) value; // Enumeration<LinkageType> 385 return value; 386 case -341064690: // resource 387 this.resource = castToReference(value); // Reference 388 return value; 389 default: 390 return super.setProperty(hash, name, value); 391 } 392 393 } 394 395 @Override 396 public Base setProperty(String name, Base value) throws FHIRException { 397 if (name.equals("type")) { 398 value = new LinkageTypeEnumFactory().fromType(castToCode(value)); 399 this.type = (Enumeration) value; // Enumeration<LinkageType> 400 } else if (name.equals("resource")) { 401 this.resource = castToReference(value); // Reference 402 } else 403 return super.setProperty(name, value); 404 return value; 405 } 406 407 @Override 408 public void removeChild(String name, Base value) throws FHIRException { 409 if (name.equals("type")) { 410 this.type = null; 411 } else if (name.equals("resource")) { 412 this.resource = null; 413 } else 414 super.removeChild(name, value); 415 416 } 417 418 @Override 419 public Base makeProperty(int hash, String name) throws FHIRException { 420 switch (hash) { 421 case 3575610: 422 return getTypeElement(); 423 case -341064690: 424 return getResource(); 425 default: 426 return super.makeProperty(hash, name); 427 } 428 429 } 430 431 @Override 432 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 433 switch (hash) { 434 case 3575610: 435 /* type */ return new String[] { "code" }; 436 case -341064690: 437 /* resource */ return new String[] { "Reference" }; 438 default: 439 return super.getTypesForProperty(hash, name); 440 } 441 442 } 443 444 @Override 445 public Base addChild(String name) throws FHIRException { 446 if (name.equals("type")) { 447 throw new FHIRException("Cannot call addChild on a singleton property Linkage.type"); 448 } else if (name.equals("resource")) { 449 this.resource = new Reference(); 450 return this.resource; 451 } else 452 return super.addChild(name); 453 } 454 455 public LinkageItemComponent copy() { 456 LinkageItemComponent dst = new LinkageItemComponent(); 457 copyValues(dst); 458 return dst; 459 } 460 461 public void copyValues(LinkageItemComponent dst) { 462 super.copyValues(dst); 463 dst.type = type == null ? null : type.copy(); 464 dst.resource = resource == null ? null : resource.copy(); 465 } 466 467 @Override 468 public boolean equalsDeep(Base other_) { 469 if (!super.equalsDeep(other_)) 470 return false; 471 if (!(other_ instanceof LinkageItemComponent)) 472 return false; 473 LinkageItemComponent o = (LinkageItemComponent) other_; 474 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true); 475 } 476 477 @Override 478 public boolean equalsShallow(Base other_) { 479 if (!super.equalsShallow(other_)) 480 return false; 481 if (!(other_ instanceof LinkageItemComponent)) 482 return false; 483 LinkageItemComponent o = (LinkageItemComponent) other_; 484 return compareValues(type, o.type, true); 485 } 486 487 public boolean isEmpty() { 488 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource); 489 } 490 491 public String fhirType() { 492 return "Linkage.item"; 493 494 } 495 496 } 497 498 /** 499 * Indicates whether the asserted set of linkages are considered to be "in 500 * effect". 501 */ 502 @Child(name = "active", type = { BooleanType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 503 @Description(shortDefinition = "Whether this linkage assertion is active or not", formalDefinition = "Indicates whether the asserted set of linkages are considered to be \"in effect\".") 504 protected BooleanType active; 505 506 /** 507 * Identifies the user or organization responsible for asserting the linkages as 508 * well as the user or organization who establishes the context in which the 509 * nature of each linkage is evaluated. 510 */ 511 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, 512 Organization.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 513 @Description(shortDefinition = "Who is responsible for linkages", formalDefinition = "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.") 514 protected Reference author; 515 516 /** 517 * The actual object that is the target of the reference (Identifies the user or 518 * organization responsible for asserting the linkages as well as the user or 519 * organization who establishes the context in which the nature of each linkage 520 * is evaluated.) 521 */ 522 protected Resource authorTarget; 523 524 /** 525 * Identifies which record considered as the reference to the same real-world 526 * occurrence as well as how the items should be evaluated within the collection 527 * of linked items. 528 */ 529 @Child(name = "item", type = {}, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 530 @Description(shortDefinition = "Item to be linked", formalDefinition = "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.") 531 protected List<LinkageItemComponent> item; 532 533 private static final long serialVersionUID = 25900306L; 534 535 /** 536 * Constructor 537 */ 538 public Linkage() { 539 super(); 540 } 541 542 /** 543 * @return {@link #active} (Indicates whether the asserted set of linkages are 544 * considered to be "in effect".). This is the underlying object with 545 * id, value and extensions. The accessor "getActive" gives direct 546 * access to the value 547 */ 548 public BooleanType getActiveElement() { 549 if (this.active == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create Linkage.active"); 552 else if (Configuration.doAutoCreate()) 553 this.active = new BooleanType(); // bb 554 return this.active; 555 } 556 557 public boolean hasActiveElement() { 558 return this.active != null && !this.active.isEmpty(); 559 } 560 561 public boolean hasActive() { 562 return this.active != null && !this.active.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #active} (Indicates whether the asserted set of linkages 567 * are considered to be "in effect".). This is the underlying 568 * object with id, value and extensions. The accessor "getActive" 569 * gives direct access to the value 570 */ 571 public Linkage setActiveElement(BooleanType value) { 572 this.active = value; 573 return this; 574 } 575 576 /** 577 * @return Indicates whether the asserted set of linkages are considered to be 578 * "in effect". 579 */ 580 public boolean getActive() { 581 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 582 } 583 584 /** 585 * @param value Indicates whether the asserted set of linkages are considered to 586 * be "in effect". 587 */ 588 public Linkage setActive(boolean value) { 589 if (this.active == null) 590 this.active = new BooleanType(); 591 this.active.setValue(value); 592 return this; 593 } 594 595 /** 596 * @return {@link #author} (Identifies the user or organization responsible for 597 * asserting the linkages as well as the user or organization who 598 * establishes the context in which the nature of each linkage is 599 * evaluated.) 600 */ 601 public Reference getAuthor() { 602 if (this.author == null) 603 if (Configuration.errorOnAutoCreate()) 604 throw new Error("Attempt to auto-create Linkage.author"); 605 else if (Configuration.doAutoCreate()) 606 this.author = new Reference(); // cc 607 return this.author; 608 } 609 610 public boolean hasAuthor() { 611 return this.author != null && !this.author.isEmpty(); 612 } 613 614 /** 615 * @param value {@link #author} (Identifies the user or organization responsible 616 * for asserting the linkages as well as the user or organization 617 * who establishes the context in which the nature of each linkage 618 * is evaluated.) 619 */ 620 public Linkage setAuthor(Reference value) { 621 this.author = value; 622 return this; 623 } 624 625 /** 626 * @return {@link #author} The actual object that is the target of the 627 * reference. The reference library doesn't populate this, but you can 628 * use it to hold the resource if you resolve it. (Identifies the user 629 * or organization responsible for asserting the linkages as well as the 630 * user or organization who establishes the context in which the nature 631 * of each linkage is evaluated.) 632 */ 633 public Resource getAuthorTarget() { 634 return this.authorTarget; 635 } 636 637 /** 638 * @param value {@link #author} The actual object that is the target of the 639 * reference. The reference library doesn't use these, but you can 640 * use it to hold the resource if you resolve it. (Identifies the 641 * user or organization responsible for asserting the linkages as 642 * well as the user or organization who establishes the context in 643 * which the nature of each linkage is evaluated.) 644 */ 645 public Linkage setAuthorTarget(Resource value) { 646 this.authorTarget = value; 647 return this; 648 } 649 650 /** 651 * @return {@link #item} (Identifies which record considered as the reference to 652 * the same real-world occurrence as well as how the items should be 653 * evaluated within the collection of linked items.) 654 */ 655 public List<LinkageItemComponent> getItem() { 656 if (this.item == null) 657 this.item = new ArrayList<LinkageItemComponent>(); 658 return this.item; 659 } 660 661 /** 662 * @return Returns a reference to <code>this</code> for easy method chaining 663 */ 664 public Linkage setItem(List<LinkageItemComponent> theItem) { 665 this.item = theItem; 666 return this; 667 } 668 669 public boolean hasItem() { 670 if (this.item == null) 671 return false; 672 for (LinkageItemComponent item : this.item) 673 if (!item.isEmpty()) 674 return true; 675 return false; 676 } 677 678 public LinkageItemComponent addItem() { // 3 679 LinkageItemComponent t = new LinkageItemComponent(); 680 if (this.item == null) 681 this.item = new ArrayList<LinkageItemComponent>(); 682 this.item.add(t); 683 return t; 684 } 685 686 public Linkage addItem(LinkageItemComponent t) { // 3 687 if (t == null) 688 return this; 689 if (this.item == null) 690 this.item = new ArrayList<LinkageItemComponent>(); 691 this.item.add(t); 692 return this; 693 } 694 695 /** 696 * @return The first repetition of repeating field {@link #item}, creating it if 697 * it does not already exist 698 */ 699 public LinkageItemComponent getItemFirstRep() { 700 if (getItem().isEmpty()) { 701 addItem(); 702 } 703 return getItem().get(0); 704 } 705 706 protected void listChildren(List<Property> children) { 707 super.listChildren(children); 708 children.add(new Property("active", "boolean", 709 "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active)); 710 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Organization)", 711 "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.", 712 0, 1, author)); 713 children.add(new Property("item", "", 714 "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.", 715 0, java.lang.Integer.MAX_VALUE, item)); 716 } 717 718 @Override 719 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 720 switch (_hash) { 721 case -1422950650: 722 /* active */ return new Property("active", "boolean", 723 "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active); 724 case -1406328437: 725 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole|Organization)", 726 "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.", 727 0, 1, author); 728 case 3242771: 729 /* item */ return new Property("item", "", 730 "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.", 731 0, java.lang.Integer.MAX_VALUE, item); 732 default: 733 return super.getNamedProperty(_hash, _name, _checkValid); 734 } 735 736 } 737 738 @Override 739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 740 switch (hash) { 741 case -1422950650: 742 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 743 case -1406328437: 744 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 745 case 3242771: 746 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // LinkageItemComponent 747 default: 748 return super.getProperty(hash, name, checkValid); 749 } 750 751 } 752 753 @Override 754 public Base setProperty(int hash, String name, Base value) throws FHIRException { 755 switch (hash) { 756 case -1422950650: // active 757 this.active = castToBoolean(value); // BooleanType 758 return value; 759 case -1406328437: // author 760 this.author = castToReference(value); // Reference 761 return value; 762 case 3242771: // item 763 this.getItem().add((LinkageItemComponent) value); // LinkageItemComponent 764 return value; 765 default: 766 return super.setProperty(hash, name, value); 767 } 768 769 } 770 771 @Override 772 public Base setProperty(String name, Base value) throws FHIRException { 773 if (name.equals("active")) { 774 this.active = castToBoolean(value); // BooleanType 775 } else if (name.equals("author")) { 776 this.author = castToReference(value); // Reference 777 } else if (name.equals("item")) { 778 this.getItem().add((LinkageItemComponent) value); 779 } else 780 return super.setProperty(name, value); 781 return value; 782 } 783 784 @Override 785 public void removeChild(String name, Base value) throws FHIRException { 786 if (name.equals("active")) { 787 this.active = null; 788 } else if (name.equals("author")) { 789 this.author = null; 790 } else if (name.equals("item")) { 791 this.getItem().remove((LinkageItemComponent) value); 792 } else 793 super.removeChild(name, value); 794 795 } 796 797 @Override 798 public Base makeProperty(int hash, String name) throws FHIRException { 799 switch (hash) { 800 case -1422950650: 801 return getActiveElement(); 802 case -1406328437: 803 return getAuthor(); 804 case 3242771: 805 return addItem(); 806 default: 807 return super.makeProperty(hash, name); 808 } 809 810 } 811 812 @Override 813 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 814 switch (hash) { 815 case -1422950650: 816 /* active */ return new String[] { "boolean" }; 817 case -1406328437: 818 /* author */ return new String[] { "Reference" }; 819 case 3242771: 820 /* item */ return new String[] {}; 821 default: 822 return super.getTypesForProperty(hash, name); 823 } 824 825 } 826 827 @Override 828 public Base addChild(String name) throws FHIRException { 829 if (name.equals("active")) { 830 throw new FHIRException("Cannot call addChild on a singleton property Linkage.active"); 831 } else if (name.equals("author")) { 832 this.author = new Reference(); 833 return this.author; 834 } else if (name.equals("item")) { 835 return addItem(); 836 } else 837 return super.addChild(name); 838 } 839 840 public String fhirType() { 841 return "Linkage"; 842 843 } 844 845 public Linkage copy() { 846 Linkage dst = new Linkage(); 847 copyValues(dst); 848 return dst; 849 } 850 851 public void copyValues(Linkage dst) { 852 super.copyValues(dst); 853 dst.active = active == null ? null : active.copy(); 854 dst.author = author == null ? null : author.copy(); 855 if (item != null) { 856 dst.item = new ArrayList<LinkageItemComponent>(); 857 for (LinkageItemComponent i : item) 858 dst.item.add(i.copy()); 859 } 860 ; 861 } 862 863 protected Linkage typedCopy() { 864 return copy(); 865 } 866 867 @Override 868 public boolean equalsDeep(Base other_) { 869 if (!super.equalsDeep(other_)) 870 return false; 871 if (!(other_ instanceof Linkage)) 872 return false; 873 Linkage o = (Linkage) other_; 874 return compareDeep(active, o.active, true) && compareDeep(author, o.author, true) 875 && compareDeep(item, o.item, true); 876 } 877 878 @Override 879 public boolean equalsShallow(Base other_) { 880 if (!super.equalsShallow(other_)) 881 return false; 882 if (!(other_ instanceof Linkage)) 883 return false; 884 Linkage o = (Linkage) other_; 885 return compareValues(active, o.active, true); 886 } 887 888 public boolean isEmpty() { 889 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(active, author, item); 890 } 891 892 @Override 893 public ResourceType getResourceType() { 894 return ResourceType.Linkage; 895 } 896 897 /** 898 * Search parameter: <b>item</b> 899 * <p> 900 * Description: <b>Matches on any item in the Linkage</b><br> 901 * Type: <b>reference</b><br> 902 * Path: <b>Linkage.item.resource</b><br> 903 * </p> 904 */ 905 @SearchParamDefinition(name = "item", path = "Linkage.item.resource", description = "Matches on any item in the Linkage", type = "reference") 906 public static final String SP_ITEM = "item"; 907 /** 908 * <b>Fluent Client</b> search parameter constant for <b>item</b> 909 * <p> 910 * Description: <b>Matches on any item in the Linkage</b><br> 911 * Type: <b>reference</b><br> 912 * Path: <b>Linkage.item.resource</b><br> 913 * </p> 914 */ 915 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 916 SP_ITEM); 917 918 /** 919 * Constant for fluent queries to be used to add include statements. Specifies 920 * the path value of "<b>Linkage:item</b>". 921 */ 922 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("Linkage:item") 923 .toLocked(); 924 925 /** 926 * Search parameter: <b>author</b> 927 * <p> 928 * Description: <b>Author of the Linkage</b><br> 929 * Type: <b>reference</b><br> 930 * Path: <b>Linkage.author</b><br> 931 * </p> 932 */ 933 @SearchParamDefinition(name = "author", path = "Linkage.author", description = "Author of the Linkage", type = "reference", providesMembershipIn = { 934 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 935 Practitioner.class, PractitionerRole.class }) 936 public static final String SP_AUTHOR = "author"; 937 /** 938 * <b>Fluent Client</b> search parameter constant for <b>author</b> 939 * <p> 940 * Description: <b>Author of the Linkage</b><br> 941 * Type: <b>reference</b><br> 942 * Path: <b>Linkage.author</b><br> 943 * </p> 944 */ 945 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 946 SP_AUTHOR); 947 948 /** 949 * Constant for fluent queries to be used to add include statements. Specifies 950 * the path value of "<b>Linkage:author</b>". 951 */ 952 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Linkage:author") 953 .toLocked(); 954 955 /** 956 * Search parameter: <b>source</b> 957 * <p> 958 * Description: <b>Matches on any item in the Linkage with a type of 959 * 'source'</b><br> 960 * Type: <b>reference</b><br> 961 * Path: <b>Linkage.item.resource</b><br> 962 * </p> 963 */ 964 @SearchParamDefinition(name = "source", path = "Linkage.item.resource", description = "Matches on any item in the Linkage with a type of 'source'", type = "reference") 965 public static final String SP_SOURCE = "source"; 966 /** 967 * <b>Fluent Client</b> search parameter constant for <b>source</b> 968 * <p> 969 * Description: <b>Matches on any item in the Linkage with a type of 970 * 'source'</b><br> 971 * Type: <b>reference</b><br> 972 * Path: <b>Linkage.item.resource</b><br> 973 * </p> 974 */ 975 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 976 SP_SOURCE); 977 978 /** 979 * Constant for fluent queries to be used to add include statements. Specifies 980 * the path value of "<b>Linkage:source</b>". 981 */ 982 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("Linkage:source") 983 .toLocked(); 984 985}