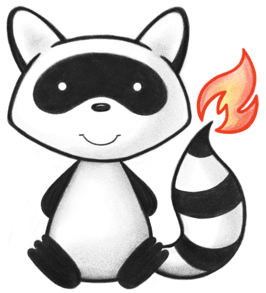
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A list is a curated collection of resources. 049 */ 050@ResourceDef(name = "List", profile = "http://hl7.org/fhir/StructureDefinition/List") 051public class ListResource extends DomainResource { 052 053 public enum ListStatus { 054 /** 055 * The list is considered to be an active part of the patient's record. 056 */ 057 CURRENT, 058 /** 059 * The list is "old" and should no longer be considered accurate or relevant. 060 */ 061 RETIRED, 062 /** 063 * The list was never accurate. It is retained for medico-legal purposes only. 064 */ 065 ENTEREDINERROR, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 071 public static ListStatus fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("current".equals(codeString)) 075 return CURRENT; 076 if ("retired".equals(codeString)) 077 return RETIRED; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if (Configuration.isAcceptInvalidEnums()) 081 return null; 082 else 083 throw new FHIRException("Unknown ListStatus code '" + codeString + "'"); 084 } 085 086 public String toCode() { 087 switch (this) { 088 case CURRENT: 089 return "current"; 090 case RETIRED: 091 return "retired"; 092 case ENTEREDINERROR: 093 return "entered-in-error"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getSystem() { 102 switch (this) { 103 case CURRENT: 104 return "http://hl7.org/fhir/list-status"; 105 case RETIRED: 106 return "http://hl7.org/fhir/list-status"; 107 case ENTEREDINERROR: 108 return "http://hl7.org/fhir/list-status"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getDefinition() { 117 switch (this) { 118 case CURRENT: 119 return "The list is considered to be an active part of the patient's record."; 120 case RETIRED: 121 return "The list is \"old\" and should no longer be considered accurate or relevant."; 122 case ENTEREDINERROR: 123 return "The list was never accurate. It is retained for medico-legal purposes only."; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDisplay() { 132 switch (this) { 133 case CURRENT: 134 return "Current"; 135 case RETIRED: 136 return "Retired"; 137 case ENTEREDINERROR: 138 return "Entered In Error"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 } 146 147 public static class ListStatusEnumFactory implements EnumFactory<ListStatus> { 148 public ListStatus fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("current".equals(codeString)) 153 return ListStatus.CURRENT; 154 if ("retired".equals(codeString)) 155 return ListStatus.RETIRED; 156 if ("entered-in-error".equals(codeString)) 157 return ListStatus.ENTEREDINERROR; 158 throw new IllegalArgumentException("Unknown ListStatus code '" + codeString + "'"); 159 } 160 161 public Enumeration<ListStatus> fromType(PrimitiveType<?> code) throws FHIRException { 162 if (code == null) 163 return null; 164 if (code.isEmpty()) 165 return new Enumeration<ListStatus>(this, ListStatus.NULL, code); 166 String codeString = code.asStringValue(); 167 if (codeString == null || "".equals(codeString)) 168 return new Enumeration<ListStatus>(this, ListStatus.NULL, code); 169 if ("current".equals(codeString)) 170 return new Enumeration<ListStatus>(this, ListStatus.CURRENT, code); 171 if ("retired".equals(codeString)) 172 return new Enumeration<ListStatus>(this, ListStatus.RETIRED, code); 173 if ("entered-in-error".equals(codeString)) 174 return new Enumeration<ListStatus>(this, ListStatus.ENTEREDINERROR, code); 175 throw new FHIRException("Unknown ListStatus code '" + codeString + "'"); 176 } 177 178 public String toCode(ListStatus code) { 179 if (code == ListStatus.NULL) 180 return null; 181 if (code == ListStatus.CURRENT) 182 return "current"; 183 if (code == ListStatus.RETIRED) 184 return "retired"; 185 if (code == ListStatus.ENTEREDINERROR) 186 return "entered-in-error"; 187 return "?"; 188 } 189 190 public String toSystem(ListStatus code) { 191 return code.getSystem(); 192 } 193 } 194 195 public enum ListMode { 196 /** 197 * This list is the master list, maintained in an ongoing fashion with regular 198 * updates as the real world list it is tracking changes. 199 */ 200 WORKING, 201 /** 202 * This list was prepared as a snapshot. It should not be assumed to be current. 203 */ 204 SNAPSHOT, 205 /** 206 * A point-in-time list that shows what changes have been made or recommended. 207 * E.g. a discharge medication list showing what was added and removed during an 208 * encounter. 209 */ 210 CHANGES, 211 /** 212 * added to help the parsers with the generic types 213 */ 214 NULL; 215 216 public static ListMode fromCode(String codeString) throws FHIRException { 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("working".equals(codeString)) 220 return WORKING; 221 if ("snapshot".equals(codeString)) 222 return SNAPSHOT; 223 if ("changes".equals(codeString)) 224 return CHANGES; 225 if (Configuration.isAcceptInvalidEnums()) 226 return null; 227 else 228 throw new FHIRException("Unknown ListMode code '" + codeString + "'"); 229 } 230 231 public String toCode() { 232 switch (this) { 233 case WORKING: 234 return "working"; 235 case SNAPSHOT: 236 return "snapshot"; 237 case CHANGES: 238 return "changes"; 239 case NULL: 240 return null; 241 default: 242 return "?"; 243 } 244 } 245 246 public String getSystem() { 247 switch (this) { 248 case WORKING: 249 return "http://hl7.org/fhir/list-mode"; 250 case SNAPSHOT: 251 return "http://hl7.org/fhir/list-mode"; 252 case CHANGES: 253 return "http://hl7.org/fhir/list-mode"; 254 case NULL: 255 return null; 256 default: 257 return "?"; 258 } 259 } 260 261 public String getDefinition() { 262 switch (this) { 263 case WORKING: 264 return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes."; 265 case SNAPSHOT: 266 return "This list was prepared as a snapshot. It should not be assumed to be current."; 267 case CHANGES: 268 return "A point-in-time list that shows what changes have been made or recommended. E.g. a discharge medication list showing what was added and removed during an encounter."; 269 case NULL: 270 return null; 271 default: 272 return "?"; 273 } 274 } 275 276 public String getDisplay() { 277 switch (this) { 278 case WORKING: 279 return "Working List"; 280 case SNAPSHOT: 281 return "Snapshot List"; 282 case CHANGES: 283 return "Change List"; 284 case NULL: 285 return null; 286 default: 287 return "?"; 288 } 289 } 290 } 291 292 public static class ListModeEnumFactory implements EnumFactory<ListMode> { 293 public ListMode fromCode(String codeString) throws IllegalArgumentException { 294 if (codeString == null || "".equals(codeString)) 295 if (codeString == null || "".equals(codeString)) 296 return null; 297 if ("working".equals(codeString)) 298 return ListMode.WORKING; 299 if ("snapshot".equals(codeString)) 300 return ListMode.SNAPSHOT; 301 if ("changes".equals(codeString)) 302 return ListMode.CHANGES; 303 throw new IllegalArgumentException("Unknown ListMode code '" + codeString + "'"); 304 } 305 306 public Enumeration<ListMode> fromType(PrimitiveType<?> code) throws FHIRException { 307 if (code == null) 308 return null; 309 if (code.isEmpty()) 310 return new Enumeration<ListMode>(this, ListMode.NULL, code); 311 String codeString = code.asStringValue(); 312 if (codeString == null || "".equals(codeString)) 313 return new Enumeration<ListMode>(this, ListMode.NULL, code); 314 if ("working".equals(codeString)) 315 return new Enumeration<ListMode>(this, ListMode.WORKING, code); 316 if ("snapshot".equals(codeString)) 317 return new Enumeration<ListMode>(this, ListMode.SNAPSHOT, code); 318 if ("changes".equals(codeString)) 319 return new Enumeration<ListMode>(this, ListMode.CHANGES, code); 320 throw new FHIRException("Unknown ListMode code '" + codeString + "'"); 321 } 322 323 public String toCode(ListMode code) { 324 if (code == ListMode.NULL) 325 return null; 326 if (code == ListMode.WORKING) 327 return "working"; 328 if (code == ListMode.SNAPSHOT) 329 return "snapshot"; 330 if (code == ListMode.CHANGES) 331 return "changes"; 332 return "?"; 333 } 334 335 public String toSystem(ListMode code) { 336 return code.getSystem(); 337 } 338 } 339 340 @Block() 341 public static class ListEntryComponent extends BackboneElement implements IBaseBackboneElement { 342 /** 343 * The flag allows the system constructing the list to indicate the role and 344 * significance of the item in the list. 345 */ 346 @Child(name = "flag", type = { 347 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 348 @Description(shortDefinition = "Status/Workflow information about this item", formalDefinition = "The flag allows the system constructing the list to indicate the role and significance of the item in the list.") 349 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-item-flag") 350 protected CodeableConcept flag; 351 352 /** 353 * True if this item is marked as deleted in the list. 354 */ 355 @Child(name = "deleted", type = { 356 BooleanType.class }, order = 2, min = 0, max = 1, modifier = true, summary = false) 357 @Description(shortDefinition = "If this item is actually marked as deleted", formalDefinition = "True if this item is marked as deleted in the list.") 358 protected BooleanType deleted; 359 360 /** 361 * When this item was added to the list. 362 */ 363 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 364 @Description(shortDefinition = "When item added to list", formalDefinition = "When this item was added to the list.") 365 protected DateTimeType date; 366 367 /** 368 * A reference to the actual resource from which data was derived. 369 */ 370 @Child(name = "item", type = { Reference.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 371 @Description(shortDefinition = "Actual entry", formalDefinition = "A reference to the actual resource from which data was derived.") 372 protected Reference item; 373 374 /** 375 * The actual object that is the target of the reference (A reference to the 376 * actual resource from which data was derived.) 377 */ 378 protected Resource itemTarget; 379 380 private static final long serialVersionUID = -758164425L; 381 382 /** 383 * Constructor 384 */ 385 public ListEntryComponent() { 386 super(); 387 } 388 389 /** 390 * Constructor 391 */ 392 public ListEntryComponent(Reference item) { 393 super(); 394 this.item = item; 395 } 396 397 /** 398 * @return {@link #flag} (The flag allows the system constructing the list to 399 * indicate the role and significance of the item in the list.) 400 */ 401 public CodeableConcept getFlag() { 402 if (this.flag == null) 403 if (Configuration.errorOnAutoCreate()) 404 throw new Error("Attempt to auto-create ListEntryComponent.flag"); 405 else if (Configuration.doAutoCreate()) 406 this.flag = new CodeableConcept(); // cc 407 return this.flag; 408 } 409 410 public boolean hasFlag() { 411 return this.flag != null && !this.flag.isEmpty(); 412 } 413 414 /** 415 * @param value {@link #flag} (The flag allows the system constructing the list 416 * to indicate the role and significance of the item in the list.) 417 */ 418 public ListEntryComponent setFlag(CodeableConcept value) { 419 this.flag = value; 420 return this; 421 } 422 423 /** 424 * @return {@link #deleted} (True if this item is marked as deleted in the 425 * list.). This is the underlying object with id, value and extensions. 426 * The accessor "getDeleted" gives direct access to the value 427 */ 428 public BooleanType getDeletedElement() { 429 if (this.deleted == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create ListEntryComponent.deleted"); 432 else if (Configuration.doAutoCreate()) 433 this.deleted = new BooleanType(); // bb 434 return this.deleted; 435 } 436 437 public boolean hasDeletedElement() { 438 return this.deleted != null && !this.deleted.isEmpty(); 439 } 440 441 public boolean hasDeleted() { 442 return this.deleted != null && !this.deleted.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #deleted} (True if this item is marked as deleted in the 447 * list.). This is the underlying object with id, value and 448 * extensions. The accessor "getDeleted" gives direct access to the 449 * value 450 */ 451 public ListEntryComponent setDeletedElement(BooleanType value) { 452 this.deleted = value; 453 return this; 454 } 455 456 /** 457 * @return True if this item is marked as deleted in the list. 458 */ 459 public boolean getDeleted() { 460 return this.deleted == null || this.deleted.isEmpty() ? false : this.deleted.getValue(); 461 } 462 463 /** 464 * @param value True if this item is marked as deleted in the list. 465 */ 466 public ListEntryComponent setDeleted(boolean value) { 467 if (this.deleted == null) 468 this.deleted = new BooleanType(); 469 this.deleted.setValue(value); 470 return this; 471 } 472 473 /** 474 * @return {@link #date} (When this item was added to the list.). This is the 475 * underlying object with id, value and extensions. The accessor 476 * "getDate" gives direct access to the value 477 */ 478 public DateTimeType getDateElement() { 479 if (this.date == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create ListEntryComponent.date"); 482 else if (Configuration.doAutoCreate()) 483 this.date = new DateTimeType(); // bb 484 return this.date; 485 } 486 487 public boolean hasDateElement() { 488 return this.date != null && !this.date.isEmpty(); 489 } 490 491 public boolean hasDate() { 492 return this.date != null && !this.date.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #date} (When this item was added to the list.). This is 497 * the underlying object with id, value and extensions. The 498 * accessor "getDate" gives direct access to the value 499 */ 500 public ListEntryComponent setDateElement(DateTimeType value) { 501 this.date = value; 502 return this; 503 } 504 505 /** 506 * @return When this item was added to the list. 507 */ 508 public Date getDate() { 509 return this.date == null ? null : this.date.getValue(); 510 } 511 512 /** 513 * @param value When this item was added to the list. 514 */ 515 public ListEntryComponent setDate(Date value) { 516 if (value == null) 517 this.date = null; 518 else { 519 if (this.date == null) 520 this.date = new DateTimeType(); 521 this.date.setValue(value); 522 } 523 return this; 524 } 525 526 /** 527 * @return {@link #item} (A reference to the actual resource from which data was 528 * derived.) 529 */ 530 public Reference getItem() { 531 if (this.item == null) 532 if (Configuration.errorOnAutoCreate()) 533 throw new Error("Attempt to auto-create ListEntryComponent.item"); 534 else if (Configuration.doAutoCreate()) 535 this.item = new Reference(); // cc 536 return this.item; 537 } 538 539 public boolean hasItem() { 540 return this.item != null && !this.item.isEmpty(); 541 } 542 543 /** 544 * @param value {@link #item} (A reference to the actual resource from which 545 * data was derived.) 546 */ 547 public ListEntryComponent setItem(Reference value) { 548 this.item = value; 549 return this; 550 } 551 552 /** 553 * @return {@link #item} The actual object that is the target of the reference. 554 * The reference library doesn't populate this, but you can use it to 555 * hold the resource if you resolve it. (A reference to the actual 556 * resource from which data was derived.) 557 */ 558 public Resource getItemTarget() { 559 return this.itemTarget; 560 } 561 562 /** 563 * @param value {@link #item} The actual object that is the target of the 564 * reference. The reference library doesn't use these, but you can 565 * use it to hold the resource if you resolve it. (A reference to 566 * the actual resource from which data was derived.) 567 */ 568 public ListEntryComponent setItemTarget(Resource value) { 569 this.itemTarget = value; 570 return this; 571 } 572 573 protected void listChildren(List<Property> children) { 574 super.listChildren(children); 575 children.add(new Property("flag", "CodeableConcept", 576 "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 577 0, 1, flag)); 578 children.add( 579 new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 1, deleted)); 580 children.add(new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date)); 581 children.add(new Property("item", "Reference(Any)", 582 "A reference to the actual resource from which data was derived.", 0, 1, item)); 583 } 584 585 @Override 586 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 587 switch (_hash) { 588 case 3145580: 589 /* flag */ return new Property("flag", "CodeableConcept", 590 "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 591 0, 1, flag); 592 case 1550463001: 593 /* deleted */ return new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 594 0, 1, deleted); 595 case 3076014: 596 /* date */ return new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date); 597 case 3242771: 598 /* item */ return new Property("item", "Reference(Any)", 599 "A reference to the actual resource from which data was derived.", 0, 1, item); 600 default: 601 return super.getNamedProperty(_hash, _name, _checkValid); 602 } 603 604 } 605 606 @Override 607 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 608 switch (hash) { 609 case 3145580: 610 /* flag */ return this.flag == null ? new Base[0] : new Base[] { this.flag }; // CodeableConcept 611 case 1550463001: 612 /* deleted */ return this.deleted == null ? new Base[0] : new Base[] { this.deleted }; // BooleanType 613 case 3076014: 614 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 615 case 3242771: 616 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Reference 617 default: 618 return super.getProperty(hash, name, checkValid); 619 } 620 621 } 622 623 @Override 624 public Base setProperty(int hash, String name, Base value) throws FHIRException { 625 switch (hash) { 626 case 3145580: // flag 627 this.flag = castToCodeableConcept(value); // CodeableConcept 628 return value; 629 case 1550463001: // deleted 630 this.deleted = castToBoolean(value); // BooleanType 631 return value; 632 case 3076014: // date 633 this.date = castToDateTime(value); // DateTimeType 634 return value; 635 case 3242771: // item 636 this.item = castToReference(value); // Reference 637 return value; 638 default: 639 return super.setProperty(hash, name, value); 640 } 641 642 } 643 644 @Override 645 public Base setProperty(String name, Base value) throws FHIRException { 646 if (name.equals("flag")) { 647 this.flag = castToCodeableConcept(value); // CodeableConcept 648 } else if (name.equals("deleted")) { 649 this.deleted = castToBoolean(value); // BooleanType 650 } else if (name.equals("date")) { 651 this.date = castToDateTime(value); // DateTimeType 652 } else if (name.equals("item")) { 653 this.item = castToReference(value); // Reference 654 } else 655 return super.setProperty(name, value); 656 return value; 657 } 658 659 @Override 660 public void removeChild(String name, Base value) throws FHIRException { 661 if (name.equals("flag")) { 662 this.flag = null; 663 } else if (name.equals("deleted")) { 664 this.deleted = null; 665 } else if (name.equals("date")) { 666 this.date = null; 667 } else if (name.equals("item")) { 668 this.item = null; 669 } else 670 super.removeChild(name, value); 671 672 } 673 674 @Override 675 public Base makeProperty(int hash, String name) throws FHIRException { 676 switch (hash) { 677 case 3145580: 678 return getFlag(); 679 case 1550463001: 680 return getDeletedElement(); 681 case 3076014: 682 return getDateElement(); 683 case 3242771: 684 return getItem(); 685 default: 686 return super.makeProperty(hash, name); 687 } 688 689 } 690 691 @Override 692 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 693 switch (hash) { 694 case 3145580: 695 /* flag */ return new String[] { "CodeableConcept" }; 696 case 1550463001: 697 /* deleted */ return new String[] { "boolean" }; 698 case 3076014: 699 /* date */ return new String[] { "dateTime" }; 700 case 3242771: 701 /* item */ return new String[] { "Reference" }; 702 default: 703 return super.getTypesForProperty(hash, name); 704 } 705 706 } 707 708 @Override 709 public Base addChild(String name) throws FHIRException { 710 if (name.equals("flag")) { 711 this.flag = new CodeableConcept(); 712 return this.flag; 713 } else if (name.equals("deleted")) { 714 throw new FHIRException("Cannot call addChild on a singleton property List.deleted"); 715 } else if (name.equals("date")) { 716 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 717 } else if (name.equals("item")) { 718 this.item = new Reference(); 719 return this.item; 720 } else 721 return super.addChild(name); 722 } 723 724 public ListEntryComponent copy() { 725 ListEntryComponent dst = new ListEntryComponent(); 726 copyValues(dst); 727 return dst; 728 } 729 730 public void copyValues(ListEntryComponent dst) { 731 super.copyValues(dst); 732 dst.flag = flag == null ? null : flag.copy(); 733 dst.deleted = deleted == null ? null : deleted.copy(); 734 dst.date = date == null ? null : date.copy(); 735 dst.item = item == null ? null : item.copy(); 736 } 737 738 @Override 739 public boolean equalsDeep(Base other_) { 740 if (!super.equalsDeep(other_)) 741 return false; 742 if (!(other_ instanceof ListEntryComponent)) 743 return false; 744 ListEntryComponent o = (ListEntryComponent) other_; 745 return compareDeep(flag, o.flag, true) && compareDeep(deleted, o.deleted, true) && compareDeep(date, o.date, true) 746 && compareDeep(item, o.item, true); 747 } 748 749 @Override 750 public boolean equalsShallow(Base other_) { 751 if (!super.equalsShallow(other_)) 752 return false; 753 if (!(other_ instanceof ListEntryComponent)) 754 return false; 755 ListEntryComponent o = (ListEntryComponent) other_; 756 return compareValues(deleted, o.deleted, true) && compareValues(date, o.date, true); 757 } 758 759 public boolean isEmpty() { 760 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(flag, deleted, date, item); 761 } 762 763 public String fhirType() { 764 return "List.entry"; 765 766 } 767 768 } 769 770 /** 771 * Identifier for the List assigned for business purposes outside the context of 772 * FHIR. 773 */ 774 @Child(name = "identifier", type = { 775 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 776 @Description(shortDefinition = "Business identifier", formalDefinition = "Identifier for the List assigned for business purposes outside the context of FHIR.") 777 protected List<Identifier> identifier; 778 779 /** 780 * Indicates the current state of this list. 781 */ 782 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 783 @Description(shortDefinition = "current | retired | entered-in-error", formalDefinition = "Indicates the current state of this list.") 784 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-status") 785 protected Enumeration<ListStatus> status; 786 787 /** 788 * How this list was prepared - whether it is a working list that is suitable 789 * for being maintained on an ongoing basis, or if it represents a snapshot of a 790 * list of items from another source, or whether it is a prepared list where 791 * items may be marked as added, modified or deleted. 792 */ 793 @Child(name = "mode", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 794 @Description(shortDefinition = "working | snapshot | changes", formalDefinition = "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.") 795 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-mode") 796 protected Enumeration<ListMode> mode; 797 798 /** 799 * A label for the list assigned by the author. 800 */ 801 @Child(name = "title", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 802 @Description(shortDefinition = "Descriptive name for the list", formalDefinition = "A label for the list assigned by the author.") 803 protected StringType title; 804 805 /** 806 * This code defines the purpose of the list - why it was created. 807 */ 808 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 809 @Description(shortDefinition = "What the purpose of this list is", formalDefinition = "This code defines the purpose of the list - why it was created.") 810 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-example-codes") 811 protected CodeableConcept code; 812 813 /** 814 * The common subject (or patient) of the resources that are in the list if 815 * there is one. 816 */ 817 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 818 Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 819 @Description(shortDefinition = "If all resources have the same subject", formalDefinition = "The common subject (or patient) of the resources that are in the list if there is one.") 820 protected Reference subject; 821 822 /** 823 * The actual object that is the target of the reference (The common subject (or 824 * patient) of the resources that are in the list if there is one.) 825 */ 826 protected Resource subjectTarget; 827 828 /** 829 * The encounter that is the context in which this list was created. 830 */ 831 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 832 @Description(shortDefinition = "Context in which list created", formalDefinition = "The encounter that is the context in which this list was created.") 833 protected Reference encounter; 834 835 /** 836 * The actual object that is the target of the reference (The encounter that is 837 * the context in which this list was created.) 838 */ 839 protected Encounter encounterTarget; 840 841 /** 842 * The date that the list was prepared. 843 */ 844 @Child(name = "date", type = { DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 845 @Description(shortDefinition = "When the list was prepared", formalDefinition = "The date that the list was prepared.") 846 protected DateTimeType date; 847 848 /** 849 * The entity responsible for deciding what the contents of the list were. Where 850 * the list was created by a human, this is the same as the author of the list. 851 */ 852 @Child(name = "source", type = { Practitioner.class, PractitionerRole.class, Patient.class, 853 Device.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 854 @Description(shortDefinition = "Who and/or what defined the list contents (aka Author)", formalDefinition = "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.") 855 protected Reference source; 856 857 /** 858 * The actual object that is the target of the reference (The entity responsible 859 * for deciding what the contents of the list were. Where the list was created 860 * by a human, this is the same as the author of the list.) 861 */ 862 protected Resource sourceTarget; 863 864 /** 865 * What order applies to the items in the list. 866 */ 867 @Child(name = "orderedBy", type = { 868 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 869 @Description(shortDefinition = "What order the list has", formalDefinition = "What order applies to the items in the list.") 870 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-order") 871 protected CodeableConcept orderedBy; 872 873 /** 874 * Comments that apply to the overall list. 875 */ 876 @Child(name = "note", type = { 877 Annotation.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 878 @Description(shortDefinition = "Comments about the list", formalDefinition = "Comments that apply to the overall list.") 879 protected List<Annotation> note; 880 881 /** 882 * Entries in this list. 883 */ 884 @Child(name = "entry", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 885 @Description(shortDefinition = "Entries in the list", formalDefinition = "Entries in this list.") 886 protected List<ListEntryComponent> entry; 887 888 /** 889 * If the list is empty, why the list is empty. 890 */ 891 @Child(name = "emptyReason", type = { 892 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 893 @Description(shortDefinition = "Why list is empty", formalDefinition = "If the list is empty, why the list is empty.") 894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-empty-reason") 895 protected CodeableConcept emptyReason; 896 897 private static final long serialVersionUID = 2071342704L; 898 899 /** 900 * Constructor 901 */ 902 public ListResource() { 903 super(); 904 } 905 906 /** 907 * Constructor 908 */ 909 public ListResource(Enumeration<ListStatus> status, Enumeration<ListMode> mode) { 910 super(); 911 this.status = status; 912 this.mode = mode; 913 } 914 915 /** 916 * @return {@link #identifier} (Identifier for the List assigned for business 917 * purposes outside the context of FHIR.) 918 */ 919 public List<Identifier> getIdentifier() { 920 if (this.identifier == null) 921 this.identifier = new ArrayList<Identifier>(); 922 return this.identifier; 923 } 924 925 /** 926 * @return Returns a reference to <code>this</code> for easy method chaining 927 */ 928 public ListResource setIdentifier(List<Identifier> theIdentifier) { 929 this.identifier = theIdentifier; 930 return this; 931 } 932 933 public boolean hasIdentifier() { 934 if (this.identifier == null) 935 return false; 936 for (Identifier item : this.identifier) 937 if (!item.isEmpty()) 938 return true; 939 return false; 940 } 941 942 public Identifier addIdentifier() { // 3 943 Identifier t = new Identifier(); 944 if (this.identifier == null) 945 this.identifier = new ArrayList<Identifier>(); 946 this.identifier.add(t); 947 return t; 948 } 949 950 public ListResource addIdentifier(Identifier t) { // 3 951 if (t == null) 952 return this; 953 if (this.identifier == null) 954 this.identifier = new ArrayList<Identifier>(); 955 this.identifier.add(t); 956 return this; 957 } 958 959 /** 960 * @return The first repetition of repeating field {@link #identifier}, creating 961 * it if it does not already exist 962 */ 963 public Identifier getIdentifierFirstRep() { 964 if (getIdentifier().isEmpty()) { 965 addIdentifier(); 966 } 967 return getIdentifier().get(0); 968 } 969 970 /** 971 * @return {@link #status} (Indicates the current state of this list.). This is 972 * the underlying object with id, value and extensions. The accessor 973 * "getStatus" gives direct access to the value 974 */ 975 public Enumeration<ListStatus> getStatusElement() { 976 if (this.status == null) 977 if (Configuration.errorOnAutoCreate()) 978 throw new Error("Attempt to auto-create List.status"); 979 else if (Configuration.doAutoCreate()) 980 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); // bb 981 return this.status; 982 } 983 984 public boolean hasStatusElement() { 985 return this.status != null && !this.status.isEmpty(); 986 } 987 988 public boolean hasStatus() { 989 return this.status != null && !this.status.isEmpty(); 990 } 991 992 /** 993 * @param value {@link #status} (Indicates the current state of this list.). 994 * This is the underlying object with id, value and extensions. The 995 * accessor "getStatus" gives direct access to the value 996 */ 997 public ListResource setStatusElement(Enumeration<ListStatus> value) { 998 this.status = value; 999 return this; 1000 } 1001 1002 /** 1003 * @return Indicates the current state of this list. 1004 */ 1005 public ListStatus getStatus() { 1006 return this.status == null ? null : this.status.getValue(); 1007 } 1008 1009 /** 1010 * @param value Indicates the current state of this list. 1011 */ 1012 public ListResource setStatus(ListStatus value) { 1013 if (this.status == null) 1014 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); 1015 this.status.setValue(value); 1016 return this; 1017 } 1018 1019 /** 1020 * @return {@link #mode} (How this list was prepared - whether it is a working 1021 * list that is suitable for being maintained on an ongoing basis, or if 1022 * it represents a snapshot of a list of items from another source, or 1023 * whether it is a prepared list where items may be marked as added, 1024 * modified or deleted.). This is the underlying object with id, value 1025 * and extensions. The accessor "getMode" gives direct access to the 1026 * value 1027 */ 1028 public Enumeration<ListMode> getModeElement() { 1029 if (this.mode == null) 1030 if (Configuration.errorOnAutoCreate()) 1031 throw new Error("Attempt to auto-create List.mode"); 1032 else if (Configuration.doAutoCreate()) 1033 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 1034 return this.mode; 1035 } 1036 1037 public boolean hasModeElement() { 1038 return this.mode != null && !this.mode.isEmpty(); 1039 } 1040 1041 public boolean hasMode() { 1042 return this.mode != null && !this.mode.isEmpty(); 1043 } 1044 1045 /** 1046 * @param value {@link #mode} (How this list was prepared - whether it is a 1047 * working list that is suitable for being maintained on an ongoing 1048 * basis, or if it represents a snapshot of a list of items from 1049 * another source, or whether it is a prepared list where items may 1050 * be marked as added, modified or deleted.). This is the 1051 * underlying object with id, value and extensions. The accessor 1052 * "getMode" gives direct access to the value 1053 */ 1054 public ListResource setModeElement(Enumeration<ListMode> value) { 1055 this.mode = value; 1056 return this; 1057 } 1058 1059 /** 1060 * @return How this list was prepared - whether it is a working list that is 1061 * suitable for being maintained on an ongoing basis, or if it 1062 * represents a snapshot of a list of items from another source, or 1063 * whether it is a prepared list where items may be marked as added, 1064 * modified or deleted. 1065 */ 1066 public ListMode getMode() { 1067 return this.mode == null ? null : this.mode.getValue(); 1068 } 1069 1070 /** 1071 * @param value How this list was prepared - whether it is a working list that 1072 * is suitable for being maintained on an ongoing basis, or if it 1073 * represents a snapshot of a list of items from another source, or 1074 * whether it is a prepared list where items may be marked as 1075 * added, modified or deleted. 1076 */ 1077 public ListResource setMode(ListMode value) { 1078 if (this.mode == null) 1079 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 1080 this.mode.setValue(value); 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #title} (A label for the list assigned by the author.). This 1086 * is the underlying object with id, value and extensions. The accessor 1087 * "getTitle" gives direct access to the value 1088 */ 1089 public StringType getTitleElement() { 1090 if (this.title == null) 1091 if (Configuration.errorOnAutoCreate()) 1092 throw new Error("Attempt to auto-create List.title"); 1093 else if (Configuration.doAutoCreate()) 1094 this.title = new StringType(); // bb 1095 return this.title; 1096 } 1097 1098 public boolean hasTitleElement() { 1099 return this.title != null && !this.title.isEmpty(); 1100 } 1101 1102 public boolean hasTitle() { 1103 return this.title != null && !this.title.isEmpty(); 1104 } 1105 1106 /** 1107 * @param value {@link #title} (A label for the list assigned by the author.). 1108 * This is the underlying object with id, value and extensions. The 1109 * accessor "getTitle" gives direct access to the value 1110 */ 1111 public ListResource setTitleElement(StringType value) { 1112 this.title = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return A label for the list assigned by the author. 1118 */ 1119 public String getTitle() { 1120 return this.title == null ? null : this.title.getValue(); 1121 } 1122 1123 /** 1124 * @param value A label for the list assigned by the author. 1125 */ 1126 public ListResource setTitle(String value) { 1127 if (Utilities.noString(value)) 1128 this.title = null; 1129 else { 1130 if (this.title == null) 1131 this.title = new StringType(); 1132 this.title.setValue(value); 1133 } 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #code} (This code defines the purpose of the list - why it was 1139 * created.) 1140 */ 1141 public CodeableConcept getCode() { 1142 if (this.code == null) 1143 if (Configuration.errorOnAutoCreate()) 1144 throw new Error("Attempt to auto-create List.code"); 1145 else if (Configuration.doAutoCreate()) 1146 this.code = new CodeableConcept(); // cc 1147 return this.code; 1148 } 1149 1150 public boolean hasCode() { 1151 return this.code != null && !this.code.isEmpty(); 1152 } 1153 1154 /** 1155 * @param value {@link #code} (This code defines the purpose of the list - why 1156 * it was created.) 1157 */ 1158 public ListResource setCode(CodeableConcept value) { 1159 this.code = value; 1160 return this; 1161 } 1162 1163 /** 1164 * @return {@link #subject} (The common subject (or patient) of the resources 1165 * that are in the list if there is one.) 1166 */ 1167 public Reference getSubject() { 1168 if (this.subject == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create List.subject"); 1171 else if (Configuration.doAutoCreate()) 1172 this.subject = new Reference(); // cc 1173 return this.subject; 1174 } 1175 1176 public boolean hasSubject() { 1177 return this.subject != null && !this.subject.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #subject} (The common subject (or patient) of the 1182 * resources that are in the list if there is one.) 1183 */ 1184 public ListResource setSubject(Reference value) { 1185 this.subject = value; 1186 return this; 1187 } 1188 1189 /** 1190 * @return {@link #subject} The actual object that is the target of the 1191 * reference. The reference library doesn't populate this, but you can 1192 * use it to hold the resource if you resolve it. (The common subject 1193 * (or patient) of the resources that are in the list if there is one.) 1194 */ 1195 public Resource getSubjectTarget() { 1196 return this.subjectTarget; 1197 } 1198 1199 /** 1200 * @param value {@link #subject} The actual object that is the target of the 1201 * reference. The reference library doesn't use these, but you can 1202 * use it to hold the resource if you resolve it. (The common 1203 * subject (or patient) of the resources that are in the list if 1204 * there is one.) 1205 */ 1206 public ListResource setSubjectTarget(Resource value) { 1207 this.subjectTarget = value; 1208 return this; 1209 } 1210 1211 /** 1212 * @return {@link #encounter} (The encounter that is the context in which this 1213 * list was created.) 1214 */ 1215 public Reference getEncounter() { 1216 if (this.encounter == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create List.encounter"); 1219 else if (Configuration.doAutoCreate()) 1220 this.encounter = new Reference(); // cc 1221 return this.encounter; 1222 } 1223 1224 public boolean hasEncounter() { 1225 return this.encounter != null && !this.encounter.isEmpty(); 1226 } 1227 1228 /** 1229 * @param value {@link #encounter} (The encounter that is the context in which 1230 * this list was created.) 1231 */ 1232 public ListResource setEncounter(Reference value) { 1233 this.encounter = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return {@link #encounter} The actual object that is the target of the 1239 * reference. The reference library doesn't populate this, but you can 1240 * use it to hold the resource if you resolve it. (The encounter that is 1241 * the context in which this list was created.) 1242 */ 1243 public Encounter getEncounterTarget() { 1244 if (this.encounterTarget == null) 1245 if (Configuration.errorOnAutoCreate()) 1246 throw new Error("Attempt to auto-create List.encounter"); 1247 else if (Configuration.doAutoCreate()) 1248 this.encounterTarget = new Encounter(); // aa 1249 return this.encounterTarget; 1250 } 1251 1252 /** 1253 * @param value {@link #encounter} The actual object that is the target of the 1254 * reference. The reference library doesn't use these, but you can 1255 * use it to hold the resource if you resolve it. (The encounter 1256 * that is the context in which this list was created.) 1257 */ 1258 public ListResource setEncounterTarget(Encounter value) { 1259 this.encounterTarget = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #date} (The date that the list was prepared.). This is the 1265 * underlying object with id, value and extensions. The accessor 1266 * "getDate" gives direct access to the value 1267 */ 1268 public DateTimeType getDateElement() { 1269 if (this.date == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create List.date"); 1272 else if (Configuration.doAutoCreate()) 1273 this.date = new DateTimeType(); // bb 1274 return this.date; 1275 } 1276 1277 public boolean hasDateElement() { 1278 return this.date != null && !this.date.isEmpty(); 1279 } 1280 1281 public boolean hasDate() { 1282 return this.date != null && !this.date.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #date} (The date that the list was prepared.). This is 1287 * the underlying object with id, value and extensions. The 1288 * accessor "getDate" gives direct access to the value 1289 */ 1290 public ListResource setDateElement(DateTimeType value) { 1291 this.date = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return The date that the list was prepared. 1297 */ 1298 public Date getDate() { 1299 return this.date == null ? null : this.date.getValue(); 1300 } 1301 1302 /** 1303 * @param value The date that the list was prepared. 1304 */ 1305 public ListResource setDate(Date value) { 1306 if (value == null) 1307 this.date = null; 1308 else { 1309 if (this.date == null) 1310 this.date = new DateTimeType(); 1311 this.date.setValue(value); 1312 } 1313 return this; 1314 } 1315 1316 /** 1317 * @return {@link #source} (The entity responsible for deciding what the 1318 * contents of the list were. Where the list was created by a human, 1319 * this is the same as the author of the list.) 1320 */ 1321 public Reference getSource() { 1322 if (this.source == null) 1323 if (Configuration.errorOnAutoCreate()) 1324 throw new Error("Attempt to auto-create List.source"); 1325 else if (Configuration.doAutoCreate()) 1326 this.source = new Reference(); // cc 1327 return this.source; 1328 } 1329 1330 public boolean hasSource() { 1331 return this.source != null && !this.source.isEmpty(); 1332 } 1333 1334 /** 1335 * @param value {@link #source} (The entity responsible for deciding what the 1336 * contents of the list were. Where the list was created by a 1337 * human, this is the same as the author of the list.) 1338 */ 1339 public ListResource setSource(Reference value) { 1340 this.source = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return {@link #source} The actual object that is the target of the 1346 * reference. The reference library doesn't populate this, but you can 1347 * use it to hold the resource if you resolve it. (The entity 1348 * responsible for deciding what the contents of the list were. Where 1349 * the list was created by a human, this is the same as the author of 1350 * the list.) 1351 */ 1352 public Resource getSourceTarget() { 1353 return this.sourceTarget; 1354 } 1355 1356 /** 1357 * @param value {@link #source} The actual object that is the target of the 1358 * reference. The reference library doesn't use these, but you can 1359 * use it to hold the resource if you resolve it. (The entity 1360 * responsible for deciding what the contents of the list were. 1361 * Where the list was created by a human, this is the same as the 1362 * author of the list.) 1363 */ 1364 public ListResource setSourceTarget(Resource value) { 1365 this.sourceTarget = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return {@link #orderedBy} (What order applies to the items in the list.) 1371 */ 1372 public CodeableConcept getOrderedBy() { 1373 if (this.orderedBy == null) 1374 if (Configuration.errorOnAutoCreate()) 1375 throw new Error("Attempt to auto-create List.orderedBy"); 1376 else if (Configuration.doAutoCreate()) 1377 this.orderedBy = new CodeableConcept(); // cc 1378 return this.orderedBy; 1379 } 1380 1381 public boolean hasOrderedBy() { 1382 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1383 } 1384 1385 /** 1386 * @param value {@link #orderedBy} (What order applies to the items in the 1387 * list.) 1388 */ 1389 public ListResource setOrderedBy(CodeableConcept value) { 1390 this.orderedBy = value; 1391 return this; 1392 } 1393 1394 /** 1395 * @return {@link #note} (Comments that apply to the overall list.) 1396 */ 1397 public List<Annotation> getNote() { 1398 if (this.note == null) 1399 this.note = new ArrayList<Annotation>(); 1400 return this.note; 1401 } 1402 1403 /** 1404 * @return Returns a reference to <code>this</code> for easy method chaining 1405 */ 1406 public ListResource setNote(List<Annotation> theNote) { 1407 this.note = theNote; 1408 return this; 1409 } 1410 1411 public boolean hasNote() { 1412 if (this.note == null) 1413 return false; 1414 for (Annotation item : this.note) 1415 if (!item.isEmpty()) 1416 return true; 1417 return false; 1418 } 1419 1420 public Annotation addNote() { // 3 1421 Annotation t = new Annotation(); 1422 if (this.note == null) 1423 this.note = new ArrayList<Annotation>(); 1424 this.note.add(t); 1425 return t; 1426 } 1427 1428 public ListResource addNote(Annotation t) { // 3 1429 if (t == null) 1430 return this; 1431 if (this.note == null) 1432 this.note = new ArrayList<Annotation>(); 1433 this.note.add(t); 1434 return this; 1435 } 1436 1437 /** 1438 * @return The first repetition of repeating field {@link #note}, creating it if 1439 * it does not already exist 1440 */ 1441 public Annotation getNoteFirstRep() { 1442 if (getNote().isEmpty()) { 1443 addNote(); 1444 } 1445 return getNote().get(0); 1446 } 1447 1448 /** 1449 * @return {@link #entry} (Entries in this list.) 1450 */ 1451 public List<ListEntryComponent> getEntry() { 1452 if (this.entry == null) 1453 this.entry = new ArrayList<ListEntryComponent>(); 1454 return this.entry; 1455 } 1456 1457 /** 1458 * @return Returns a reference to <code>this</code> for easy method chaining 1459 */ 1460 public ListResource setEntry(List<ListEntryComponent> theEntry) { 1461 this.entry = theEntry; 1462 return this; 1463 } 1464 1465 public boolean hasEntry() { 1466 if (this.entry == null) 1467 return false; 1468 for (ListEntryComponent item : this.entry) 1469 if (!item.isEmpty()) 1470 return true; 1471 return false; 1472 } 1473 1474 public ListEntryComponent addEntry() { // 3 1475 ListEntryComponent t = new ListEntryComponent(); 1476 if (this.entry == null) 1477 this.entry = new ArrayList<ListEntryComponent>(); 1478 this.entry.add(t); 1479 return t; 1480 } 1481 1482 public ListResource addEntry(ListEntryComponent t) { // 3 1483 if (t == null) 1484 return this; 1485 if (this.entry == null) 1486 this.entry = new ArrayList<ListEntryComponent>(); 1487 this.entry.add(t); 1488 return this; 1489 } 1490 1491 /** 1492 * @return The first repetition of repeating field {@link #entry}, creating it 1493 * if it does not already exist 1494 */ 1495 public ListEntryComponent getEntryFirstRep() { 1496 if (getEntry().isEmpty()) { 1497 addEntry(); 1498 } 1499 return getEntry().get(0); 1500 } 1501 1502 /** 1503 * @return {@link #emptyReason} (If the list is empty, why the list is empty.) 1504 */ 1505 public CodeableConcept getEmptyReason() { 1506 if (this.emptyReason == null) 1507 if (Configuration.errorOnAutoCreate()) 1508 throw new Error("Attempt to auto-create List.emptyReason"); 1509 else if (Configuration.doAutoCreate()) 1510 this.emptyReason = new CodeableConcept(); // cc 1511 return this.emptyReason; 1512 } 1513 1514 public boolean hasEmptyReason() { 1515 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1516 } 1517 1518 /** 1519 * @param value {@link #emptyReason} (If the list is empty, why the list is 1520 * empty.) 1521 */ 1522 public ListResource setEmptyReason(CodeableConcept value) { 1523 this.emptyReason = value; 1524 return this; 1525 } 1526 1527 protected void listChildren(List<Property> children) { 1528 super.listChildren(children); 1529 children.add(new Property("identifier", "Identifier", 1530 "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, 1531 java.lang.Integer.MAX_VALUE, identifier)); 1532 children.add(new Property("status", "code", "Indicates the current state of this list.", 0, 1, status)); 1533 children.add(new Property("mode", "code", 1534 "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 1535 0, 1, mode)); 1536 children.add(new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title)); 1537 children.add(new Property("code", "CodeableConcept", 1538 "This code defines the purpose of the list - why it was created.", 0, 1, code)); 1539 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 1540 "The common subject (or patient) of the resources that are in the list if there is one.", 0, 1, subject)); 1541 children.add(new Property("encounter", "Reference(Encounter)", 1542 "The encounter that is the context in which this list was created.", 0, 1, encounter)); 1543 children.add(new Property("date", "dateTime", "The date that the list was prepared.", 0, 1, date)); 1544 children.add(new Property("source", "Reference(Practitioner|PractitionerRole|Patient|Device)", 1545 "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 1546 0, 1, source)); 1547 children.add( 1548 new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1, orderedBy)); 1549 children.add(new Property("note", "Annotation", "Comments that apply to the overall list.", 0, 1550 java.lang.Integer.MAX_VALUE, note)); 1551 children.add(new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry)); 1552 children.add(new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1, 1553 emptyReason)); 1554 } 1555 1556 @Override 1557 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1558 switch (_hash) { 1559 case -1618432855: 1560 /* identifier */ return new Property("identifier", "Identifier", 1561 "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, 1562 java.lang.Integer.MAX_VALUE, identifier); 1563 case -892481550: 1564 /* status */ return new Property("status", "code", "Indicates the current state of this list.", 0, 1, status); 1565 case 3357091: 1566 /* mode */ return new Property("mode", "code", 1567 "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 1568 0, 1, mode); 1569 case 110371416: 1570 /* title */ return new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title); 1571 case 3059181: 1572 /* code */ return new Property("code", "CodeableConcept", 1573 "This code defines the purpose of the list - why it was created.", 0, 1, code); 1574 case -1867885268: 1575 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Location)", 1576 "The common subject (or patient) of the resources that are in the list if there is one.", 0, 1, subject); 1577 case 1524132147: 1578 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1579 "The encounter that is the context in which this list was created.", 0, 1, encounter); 1580 case 3076014: 1581 /* date */ return new Property("date", "dateTime", "The date that the list was prepared.", 0, 1, date); 1582 case -896505829: 1583 /* source */ return new Property("source", "Reference(Practitioner|PractitionerRole|Patient|Device)", 1584 "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 1585 0, 1, source); 1586 case -391079516: 1587 /* orderedBy */ return new Property("orderedBy", "CodeableConcept", 1588 "What order applies to the items in the list.", 0, 1, orderedBy); 1589 case 3387378: 1590 /* note */ return new Property("note", "Annotation", "Comments that apply to the overall list.", 0, 1591 java.lang.Integer.MAX_VALUE, note); 1592 case 96667762: 1593 /* entry */ return new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry); 1594 case 1140135409: 1595 /* emptyReason */ return new Property("emptyReason", "CodeableConcept", 1596 "If the list is empty, why the list is empty.", 0, 1, emptyReason); 1597 default: 1598 return super.getNamedProperty(_hash, _name, _checkValid); 1599 } 1600 1601 } 1602 1603 @Override 1604 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1605 switch (hash) { 1606 case -1618432855: 1607 /* identifier */ return this.identifier == null ? new Base[0] 1608 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1609 case -892481550: 1610 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ListStatus> 1611 case 3357091: 1612 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<ListMode> 1613 case 110371416: 1614 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 1615 case 3059181: 1616 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1617 case -1867885268: 1618 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1619 case 1524132147: 1620 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1621 case 3076014: 1622 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1623 case -896505829: 1624 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 1625 case -391079516: 1626 /* orderedBy */ return this.orderedBy == null ? new Base[0] : new Base[] { this.orderedBy }; // CodeableConcept 1627 case 3387378: 1628 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1629 case 96667762: 1630 /* entry */ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // ListEntryComponent 1631 case 1140135409: 1632 /* emptyReason */ return this.emptyReason == null ? new Base[0] : new Base[] { this.emptyReason }; // CodeableConcept 1633 default: 1634 return super.getProperty(hash, name, checkValid); 1635 } 1636 1637 } 1638 1639 @Override 1640 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1641 switch (hash) { 1642 case -1618432855: // identifier 1643 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1644 return value; 1645 case -892481550: // status 1646 value = new ListStatusEnumFactory().fromType(castToCode(value)); 1647 this.status = (Enumeration) value; // Enumeration<ListStatus> 1648 return value; 1649 case 3357091: // mode 1650 value = new ListModeEnumFactory().fromType(castToCode(value)); 1651 this.mode = (Enumeration) value; // Enumeration<ListMode> 1652 return value; 1653 case 110371416: // title 1654 this.title = castToString(value); // StringType 1655 return value; 1656 case 3059181: // code 1657 this.code = castToCodeableConcept(value); // CodeableConcept 1658 return value; 1659 case -1867885268: // subject 1660 this.subject = castToReference(value); // Reference 1661 return value; 1662 case 1524132147: // encounter 1663 this.encounter = castToReference(value); // Reference 1664 return value; 1665 case 3076014: // date 1666 this.date = castToDateTime(value); // DateTimeType 1667 return value; 1668 case -896505829: // source 1669 this.source = castToReference(value); // Reference 1670 return value; 1671 case -391079516: // orderedBy 1672 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1673 return value; 1674 case 3387378: // note 1675 this.getNote().add(castToAnnotation(value)); // Annotation 1676 return value; 1677 case 96667762: // entry 1678 this.getEntry().add((ListEntryComponent) value); // ListEntryComponent 1679 return value; 1680 case 1140135409: // emptyReason 1681 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1682 return value; 1683 default: 1684 return super.setProperty(hash, name, value); 1685 } 1686 1687 } 1688 1689 @Override 1690 public Base setProperty(String name, Base value) throws FHIRException { 1691 if (name.equals("identifier")) { 1692 this.getIdentifier().add(castToIdentifier(value)); 1693 } else if (name.equals("status")) { 1694 value = new ListStatusEnumFactory().fromType(castToCode(value)); 1695 this.status = (Enumeration) value; // Enumeration<ListStatus> 1696 } else if (name.equals("mode")) { 1697 value = new ListModeEnumFactory().fromType(castToCode(value)); 1698 this.mode = (Enumeration) value; // Enumeration<ListMode> 1699 } else if (name.equals("title")) { 1700 this.title = castToString(value); // StringType 1701 } else if (name.equals("code")) { 1702 this.code = castToCodeableConcept(value); // CodeableConcept 1703 } else if (name.equals("subject")) { 1704 this.subject = castToReference(value); // Reference 1705 } else if (name.equals("encounter")) { 1706 this.encounter = castToReference(value); // Reference 1707 } else if (name.equals("date")) { 1708 this.date = castToDateTime(value); // DateTimeType 1709 } else if (name.equals("source")) { 1710 this.source = castToReference(value); // Reference 1711 } else if (name.equals("orderedBy")) { 1712 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1713 } else if (name.equals("note")) { 1714 this.getNote().add(castToAnnotation(value)); 1715 } else if (name.equals("entry")) { 1716 this.getEntry().add((ListEntryComponent) value); 1717 } else if (name.equals("emptyReason")) { 1718 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1719 } else 1720 return super.setProperty(name, value); 1721 return value; 1722 } 1723 1724 @Override 1725 public void removeChild(String name, Base value) throws FHIRException { 1726 if (name.equals("identifier")) { 1727 this.getIdentifier().remove(castToIdentifier(value)); 1728 } else if (name.equals("status")) { 1729 this.status = null; 1730 } else if (name.equals("mode")) { 1731 this.mode = null; 1732 } else if (name.equals("title")) { 1733 this.title = null; 1734 } else if (name.equals("code")) { 1735 this.code = null; 1736 } else if (name.equals("subject")) { 1737 this.subject = null; 1738 } else if (name.equals("encounter")) { 1739 this.encounter = null; 1740 } else if (name.equals("date")) { 1741 this.date = null; 1742 } else if (name.equals("source")) { 1743 this.source = null; 1744 } else if (name.equals("orderedBy")) { 1745 this.orderedBy = null; 1746 } else if (name.equals("note")) { 1747 this.getNote().remove(castToAnnotation(value)); 1748 } else if (name.equals("entry")) { 1749 this.getEntry().remove((ListEntryComponent) value); 1750 } else if (name.equals("emptyReason")) { 1751 this.emptyReason = null; 1752 } else 1753 super.removeChild(name, value); 1754 1755 } 1756 1757 @Override 1758 public Base makeProperty(int hash, String name) throws FHIRException { 1759 switch (hash) { 1760 case -1618432855: 1761 return addIdentifier(); 1762 case -892481550: 1763 return getStatusElement(); 1764 case 3357091: 1765 return getModeElement(); 1766 case 110371416: 1767 return getTitleElement(); 1768 case 3059181: 1769 return getCode(); 1770 case -1867885268: 1771 return getSubject(); 1772 case 1524132147: 1773 return getEncounter(); 1774 case 3076014: 1775 return getDateElement(); 1776 case -896505829: 1777 return getSource(); 1778 case -391079516: 1779 return getOrderedBy(); 1780 case 3387378: 1781 return addNote(); 1782 case 96667762: 1783 return addEntry(); 1784 case 1140135409: 1785 return getEmptyReason(); 1786 default: 1787 return super.makeProperty(hash, name); 1788 } 1789 1790 } 1791 1792 @Override 1793 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1794 switch (hash) { 1795 case -1618432855: 1796 /* identifier */ return new String[] { "Identifier" }; 1797 case -892481550: 1798 /* status */ return new String[] { "code" }; 1799 case 3357091: 1800 /* mode */ return new String[] { "code" }; 1801 case 110371416: 1802 /* title */ return new String[] { "string" }; 1803 case 3059181: 1804 /* code */ return new String[] { "CodeableConcept" }; 1805 case -1867885268: 1806 /* subject */ return new String[] { "Reference" }; 1807 case 1524132147: 1808 /* encounter */ return new String[] { "Reference" }; 1809 case 3076014: 1810 /* date */ return new String[] { "dateTime" }; 1811 case -896505829: 1812 /* source */ return new String[] { "Reference" }; 1813 case -391079516: 1814 /* orderedBy */ return new String[] { "CodeableConcept" }; 1815 case 3387378: 1816 /* note */ return new String[] { "Annotation" }; 1817 case 96667762: 1818 /* entry */ return new String[] {}; 1819 case 1140135409: 1820 /* emptyReason */ return new String[] { "CodeableConcept" }; 1821 default: 1822 return super.getTypesForProperty(hash, name); 1823 } 1824 1825 } 1826 1827 @Override 1828 public Base addChild(String name) throws FHIRException { 1829 if (name.equals("identifier")) { 1830 return addIdentifier(); 1831 } else if (name.equals("status")) { 1832 throw new FHIRException("Cannot call addChild on a singleton property List.status"); 1833 } else if (name.equals("mode")) { 1834 throw new FHIRException("Cannot call addChild on a singleton property List.mode"); 1835 } else if (name.equals("title")) { 1836 throw new FHIRException("Cannot call addChild on a singleton property List.title"); 1837 } else if (name.equals("code")) { 1838 this.code = new CodeableConcept(); 1839 return this.code; 1840 } else if (name.equals("subject")) { 1841 this.subject = new Reference(); 1842 return this.subject; 1843 } else if (name.equals("encounter")) { 1844 this.encounter = new Reference(); 1845 return this.encounter; 1846 } else if (name.equals("date")) { 1847 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 1848 } else if (name.equals("source")) { 1849 this.source = new Reference(); 1850 return this.source; 1851 } else if (name.equals("orderedBy")) { 1852 this.orderedBy = new CodeableConcept(); 1853 return this.orderedBy; 1854 } else if (name.equals("note")) { 1855 return addNote(); 1856 } else if (name.equals("entry")) { 1857 return addEntry(); 1858 } else if (name.equals("emptyReason")) { 1859 this.emptyReason = new CodeableConcept(); 1860 return this.emptyReason; 1861 } else 1862 return super.addChild(name); 1863 } 1864 1865 public String fhirType() { 1866 return "List"; 1867 1868 } 1869 1870 public ListResource copy() { 1871 ListResource dst = new ListResource(); 1872 copyValues(dst); 1873 return dst; 1874 } 1875 1876 public void copyValues(ListResource dst) { 1877 super.copyValues(dst); 1878 if (identifier != null) { 1879 dst.identifier = new ArrayList<Identifier>(); 1880 for (Identifier i : identifier) 1881 dst.identifier.add(i.copy()); 1882 } 1883 ; 1884 dst.status = status == null ? null : status.copy(); 1885 dst.mode = mode == null ? null : mode.copy(); 1886 dst.title = title == null ? null : title.copy(); 1887 dst.code = code == null ? null : code.copy(); 1888 dst.subject = subject == null ? null : subject.copy(); 1889 dst.encounter = encounter == null ? null : encounter.copy(); 1890 dst.date = date == null ? null : date.copy(); 1891 dst.source = source == null ? null : source.copy(); 1892 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1893 if (note != null) { 1894 dst.note = new ArrayList<Annotation>(); 1895 for (Annotation i : note) 1896 dst.note.add(i.copy()); 1897 } 1898 ; 1899 if (entry != null) { 1900 dst.entry = new ArrayList<ListEntryComponent>(); 1901 for (ListEntryComponent i : entry) 1902 dst.entry.add(i.copy()); 1903 } 1904 ; 1905 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1906 } 1907 1908 protected ListResource typedCopy() { 1909 return copy(); 1910 } 1911 1912 @Override 1913 public boolean equalsDeep(Base other_) { 1914 if (!super.equalsDeep(other_)) 1915 return false; 1916 if (!(other_ instanceof ListResource)) 1917 return false; 1918 ListResource o = (ListResource) other_; 1919 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1920 && compareDeep(mode, o.mode, true) && compareDeep(title, o.title, true) && compareDeep(code, o.code, true) 1921 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1922 && compareDeep(date, o.date, true) && compareDeep(source, o.source, true) 1923 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(note, o.note, true) 1924 && compareDeep(entry, o.entry, true) && compareDeep(emptyReason, o.emptyReason, true); 1925 } 1926 1927 @Override 1928 public boolean equalsShallow(Base other_) { 1929 if (!super.equalsShallow(other_)) 1930 return false; 1931 if (!(other_ instanceof ListResource)) 1932 return false; 1933 ListResource o = (ListResource) other_; 1934 return compareValues(status, o.status, true) && compareValues(mode, o.mode, true) 1935 && compareValues(title, o.title, true) && compareValues(date, o.date, true); 1936 } 1937 1938 public boolean isEmpty() { 1939 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, mode, title, code, subject, 1940 encounter, date, source, orderedBy, note, entry, emptyReason); 1941 } 1942 1943 @Override 1944 public ResourceType getResourceType() { 1945 return ResourceType.List; 1946 } 1947 1948 /** 1949 * Search parameter: <b>date</b> 1950 * <p> 1951 * Description: <b>When the list was prepared</b><br> 1952 * Type: <b>date</b><br> 1953 * Path: <b>List.date</b><br> 1954 * </p> 1955 */ 1956 @SearchParamDefinition(name = "date", path = "List.date", description = "When the list was prepared", type = "date") 1957 public static final String SP_DATE = "date"; 1958 /** 1959 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1960 * <p> 1961 * Description: <b>When the list was prepared</b><br> 1962 * Type: <b>date</b><br> 1963 * Path: <b>List.date</b><br> 1964 * </p> 1965 */ 1966 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1967 SP_DATE); 1968 1969 /** 1970 * Search parameter: <b>identifier</b> 1971 * <p> 1972 * Description: <b>Business identifier</b><br> 1973 * Type: <b>token</b><br> 1974 * Path: <b>List.identifier</b><br> 1975 * </p> 1976 */ 1977 @SearchParamDefinition(name = "identifier", path = "List.identifier", description = "Business identifier", type = "token") 1978 public static final String SP_IDENTIFIER = "identifier"; 1979 /** 1980 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1981 * <p> 1982 * Description: <b>Business identifier</b><br> 1983 * Type: <b>token</b><br> 1984 * Path: <b>List.identifier</b><br> 1985 * </p> 1986 */ 1987 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1988 SP_IDENTIFIER); 1989 1990 /** 1991 * Search parameter: <b>item</b> 1992 * <p> 1993 * Description: <b>Actual entry</b><br> 1994 * Type: <b>reference</b><br> 1995 * Path: <b>List.entry.item</b><br> 1996 * </p> 1997 */ 1998 @SearchParamDefinition(name = "item", path = "List.entry.item", description = "Actual entry", type = "reference") 1999 public static final String SP_ITEM = "item"; 2000 /** 2001 * <b>Fluent Client</b> search parameter constant for <b>item</b> 2002 * <p> 2003 * Description: <b>Actual entry</b><br> 2004 * Type: <b>reference</b><br> 2005 * Path: <b>List.entry.item</b><br> 2006 * </p> 2007 */ 2008 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2009 SP_ITEM); 2010 2011 /** 2012 * Constant for fluent queries to be used to add include statements. Specifies 2013 * the path value of "<b>List:item</b>". 2014 */ 2015 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("List:item") 2016 .toLocked(); 2017 2018 /** 2019 * Search parameter: <b>empty-reason</b> 2020 * <p> 2021 * Description: <b>Why list is empty</b><br> 2022 * Type: <b>token</b><br> 2023 * Path: <b>List.emptyReason</b><br> 2024 * </p> 2025 */ 2026 @SearchParamDefinition(name = "empty-reason", path = "List.emptyReason", description = "Why list is empty", type = "token") 2027 public static final String SP_EMPTY_REASON = "empty-reason"; 2028 /** 2029 * <b>Fluent Client</b> search parameter constant for <b>empty-reason</b> 2030 * <p> 2031 * Description: <b>Why list is empty</b><br> 2032 * Type: <b>token</b><br> 2033 * Path: <b>List.emptyReason</b><br> 2034 * </p> 2035 */ 2036 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMPTY_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2037 SP_EMPTY_REASON); 2038 2039 /** 2040 * Search parameter: <b>code</b> 2041 * <p> 2042 * Description: <b>What the purpose of this list is</b><br> 2043 * Type: <b>token</b><br> 2044 * Path: <b>List.code</b><br> 2045 * </p> 2046 */ 2047 @SearchParamDefinition(name = "code", path = "List.code", description = "What the purpose of this list is", type = "token") 2048 public static final String SP_CODE = "code"; 2049 /** 2050 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2051 * <p> 2052 * Description: <b>What the purpose of this list is</b><br> 2053 * Type: <b>token</b><br> 2054 * Path: <b>List.code</b><br> 2055 * </p> 2056 */ 2057 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2058 SP_CODE); 2059 2060 /** 2061 * Search parameter: <b>notes</b> 2062 * <p> 2063 * Description: <b>The annotation - text content (as markdown)</b><br> 2064 * Type: <b>string</b><br> 2065 * Path: <b>List.note.text</b><br> 2066 * </p> 2067 */ 2068 @SearchParamDefinition(name = "notes", path = "List.note.text", description = "The annotation - text content (as markdown)", type = "string") 2069 public static final String SP_NOTES = "notes"; 2070 /** 2071 * <b>Fluent Client</b> search parameter constant for <b>notes</b> 2072 * <p> 2073 * Description: <b>The annotation - text content (as markdown)</b><br> 2074 * Type: <b>string</b><br> 2075 * Path: <b>List.note.text</b><br> 2076 * </p> 2077 */ 2078 public static final ca.uhn.fhir.rest.gclient.StringClientParam NOTES = new ca.uhn.fhir.rest.gclient.StringClientParam( 2079 SP_NOTES); 2080 2081 /** 2082 * Search parameter: <b>subject</b> 2083 * <p> 2084 * Description: <b>If all resources have the same subject</b><br> 2085 * Type: <b>reference</b><br> 2086 * Path: <b>List.subject</b><br> 2087 * </p> 2088 */ 2089 @SearchParamDefinition(name = "subject", path = "List.subject", description = "If all resources have the same subject", type = "reference", providesMembershipIn = { 2090 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Device"), 2091 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Patient") }, target = { 2092 Device.class, Group.class, Location.class, Patient.class }) 2093 public static final String SP_SUBJECT = "subject"; 2094 /** 2095 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2096 * <p> 2097 * Description: <b>If all resources have the same subject</b><br> 2098 * Type: <b>reference</b><br> 2099 * Path: <b>List.subject</b><br> 2100 * </p> 2101 */ 2102 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2103 SP_SUBJECT); 2104 2105 /** 2106 * Constant for fluent queries to be used to add include statements. Specifies 2107 * the path value of "<b>List:subject</b>". 2108 */ 2109 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("List:subject") 2110 .toLocked(); 2111 2112 /** 2113 * Search parameter: <b>patient</b> 2114 * <p> 2115 * Description: <b>If all resources have the same subject</b><br> 2116 * Type: <b>reference</b><br> 2117 * Path: <b>List.subject</b><br> 2118 * </p> 2119 */ 2120 @SearchParamDefinition(name = "patient", path = "List.subject.where(resolve() is Patient)", description = "If all resources have the same subject", type = "reference", target = { 2121 Patient.class }) 2122 public static final String SP_PATIENT = "patient"; 2123 /** 2124 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2125 * <p> 2126 * Description: <b>If all resources have the same subject</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>List.subject</b><br> 2129 * </p> 2130 */ 2131 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2132 SP_PATIENT); 2133 2134 /** 2135 * Constant for fluent queries to be used to add include statements. Specifies 2136 * the path value of "<b>List:patient</b>". 2137 */ 2138 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("List:patient") 2139 .toLocked(); 2140 2141 /** 2142 * Search parameter: <b>source</b> 2143 * <p> 2144 * Description: <b>Who and/or what defined the list contents (aka 2145 * Author)</b><br> 2146 * Type: <b>reference</b><br> 2147 * Path: <b>List.source</b><br> 2148 * </p> 2149 */ 2150 @SearchParamDefinition(name = "source", path = "List.source", description = "Who and/or what defined the list contents (aka Author)", type = "reference", providesMembershipIn = { 2151 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Device"), 2152 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Patient"), 2153 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Practitioner") }, target = { 2154 Device.class, Patient.class, Practitioner.class, PractitionerRole.class }) 2155 public static final String SP_SOURCE = "source"; 2156 /** 2157 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2158 * <p> 2159 * Description: <b>Who and/or what defined the list contents (aka 2160 * Author)</b><br> 2161 * Type: <b>reference</b><br> 2162 * Path: <b>List.source</b><br> 2163 * </p> 2164 */ 2165 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2166 SP_SOURCE); 2167 2168 /** 2169 * Constant for fluent queries to be used to add include statements. Specifies 2170 * the path value of "<b>List:source</b>". 2171 */ 2172 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("List:source") 2173 .toLocked(); 2174 2175 /** 2176 * Search parameter: <b>encounter</b> 2177 * <p> 2178 * Description: <b>Context in which list created</b><br> 2179 * Type: <b>reference</b><br> 2180 * Path: <b>List.encounter</b><br> 2181 * </p> 2182 */ 2183 @SearchParamDefinition(name = "encounter", path = "List.encounter", description = "Context in which list created", type = "reference", target = { 2184 Encounter.class }) 2185 public static final String SP_ENCOUNTER = "encounter"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2188 * <p> 2189 * Description: <b>Context in which list created</b><br> 2190 * Type: <b>reference</b><br> 2191 * Path: <b>List.encounter</b><br> 2192 * </p> 2193 */ 2194 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2195 SP_ENCOUNTER); 2196 2197 /** 2198 * Constant for fluent queries to be used to add include statements. Specifies 2199 * the path value of "<b>List:encounter</b>". 2200 */ 2201 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2202 "List:encounter").toLocked(); 2203 2204 /** 2205 * Search parameter: <b>title</b> 2206 * <p> 2207 * Description: <b>Descriptive name for the list</b><br> 2208 * Type: <b>string</b><br> 2209 * Path: <b>List.title</b><br> 2210 * </p> 2211 */ 2212 @SearchParamDefinition(name = "title", path = "List.title", description = "Descriptive name for the list", type = "string") 2213 public static final String SP_TITLE = "title"; 2214 /** 2215 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2216 * <p> 2217 * Description: <b>Descriptive name for the list</b><br> 2218 * Type: <b>string</b><br> 2219 * Path: <b>List.title</b><br> 2220 * </p> 2221 */ 2222 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2223 SP_TITLE); 2224 2225 /** 2226 * Search parameter: <b>status</b> 2227 * <p> 2228 * Description: <b>current | retired | entered-in-error</b><br> 2229 * Type: <b>token</b><br> 2230 * Path: <b>List.status</b><br> 2231 * </p> 2232 */ 2233 @SearchParamDefinition(name = "status", path = "List.status", description = "current | retired | entered-in-error", type = "token") 2234 public static final String SP_STATUS = "status"; 2235 /** 2236 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2237 * <p> 2238 * Description: <b>current | retired | entered-in-error</b><br> 2239 * Type: <b>token</b><br> 2240 * Path: <b>List.status</b><br> 2241 * </p> 2242 */ 2243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2244 SP_STATUS); 2245 2246}