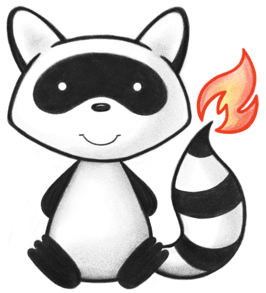
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Details and position information for a physical place where services are 050 * provided and resources and participants may be stored, found, contained, or 051 * accommodated. 052 */ 053@ResourceDef(name = "Location", profile = "http://hl7.org/fhir/StructureDefinition/Location") 054public class Location extends DomainResource { 055 056 public enum LocationStatus { 057 /** 058 * The location is operational. 059 */ 060 ACTIVE, 061 /** 062 * The location is temporarily closed. 063 */ 064 SUSPENDED, 065 /** 066 * The location is no longer used. 067 */ 068 INACTIVE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 074 public static LocationStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("active".equals(codeString)) 078 return ACTIVE; 079 if ("suspended".equals(codeString)) 080 return SUSPENDED; 081 if ("inactive".equals(codeString)) 082 return INACTIVE; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown LocationStatus code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case ACTIVE: 092 return "active"; 093 case SUSPENDED: 094 return "suspended"; 095 case INACTIVE: 096 return "inactive"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case ACTIVE: 107 return "http://hl7.org/fhir/location-status"; 108 case SUSPENDED: 109 return "http://hl7.org/fhir/location-status"; 110 case INACTIVE: 111 return "http://hl7.org/fhir/location-status"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case ACTIVE: 122 return "The location is operational."; 123 case SUSPENDED: 124 return "The location is temporarily closed."; 125 case INACTIVE: 126 return "The location is no longer used."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case ACTIVE: 137 return "Active"; 138 case SUSPENDED: 139 return "Suspended"; 140 case INACTIVE: 141 return "Inactive"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class LocationStatusEnumFactory implements EnumFactory<LocationStatus> { 151 public LocationStatus fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return LocationStatus.ACTIVE; 157 if ("suspended".equals(codeString)) 158 return LocationStatus.SUSPENDED; 159 if ("inactive".equals(codeString)) 160 return LocationStatus.INACTIVE; 161 throw new IllegalArgumentException("Unknown LocationStatus code '" + codeString + "'"); 162 } 163 164 public Enumeration<LocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 165 if (code == null) 166 return null; 167 if (code.isEmpty()) 168 return new Enumeration<LocationStatus>(this, LocationStatus.NULL, code); 169 String codeString = code.asStringValue(); 170 if (codeString == null || "".equals(codeString)) 171 return new Enumeration<LocationStatus>(this, LocationStatus.NULL, code); 172 if ("active".equals(codeString)) 173 return new Enumeration<LocationStatus>(this, LocationStatus.ACTIVE, code); 174 if ("suspended".equals(codeString)) 175 return new Enumeration<LocationStatus>(this, LocationStatus.SUSPENDED, code); 176 if ("inactive".equals(codeString)) 177 return new Enumeration<LocationStatus>(this, LocationStatus.INACTIVE, code); 178 throw new FHIRException("Unknown LocationStatus code '" + codeString + "'"); 179 } 180 181 public String toCode(LocationStatus code) { 182 if (code == LocationStatus.NULL) 183 return null; 184 if (code == LocationStatus.ACTIVE) 185 return "active"; 186 if (code == LocationStatus.SUSPENDED) 187 return "suspended"; 188 if (code == LocationStatus.INACTIVE) 189 return "inactive"; 190 return "?"; 191 } 192 193 public String toSystem(LocationStatus code) { 194 return code.getSystem(); 195 } 196 } 197 198 public enum LocationMode { 199 /** 200 * The Location resource represents a specific instance of a location (e.g. 201 * Operating Theatre 1A). 202 */ 203 INSTANCE, 204 /** 205 * The Location represents a class of locations (e.g. Any Operating Theatre) 206 * although this class of locations could be constrained within a specific 207 * boundary (such as organization, or parent location, address etc.). 208 */ 209 KIND, 210 /** 211 * added to help the parsers with the generic types 212 */ 213 NULL; 214 215 public static LocationMode fromCode(String codeString) throws FHIRException { 216 if (codeString == null || "".equals(codeString)) 217 return null; 218 if ("instance".equals(codeString)) 219 return INSTANCE; 220 if ("kind".equals(codeString)) 221 return KIND; 222 if (Configuration.isAcceptInvalidEnums()) 223 return null; 224 else 225 throw new FHIRException("Unknown LocationMode code '" + codeString + "'"); 226 } 227 228 public String toCode() { 229 switch (this) { 230 case INSTANCE: 231 return "instance"; 232 case KIND: 233 return "kind"; 234 case NULL: 235 return null; 236 default: 237 return "?"; 238 } 239 } 240 241 public String getSystem() { 242 switch (this) { 243 case INSTANCE: 244 return "http://hl7.org/fhir/location-mode"; 245 case KIND: 246 return "http://hl7.org/fhir/location-mode"; 247 case NULL: 248 return null; 249 default: 250 return "?"; 251 } 252 } 253 254 public String getDefinition() { 255 switch (this) { 256 case INSTANCE: 257 return "The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A)."; 258 case KIND: 259 return "The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.)."; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getDisplay() { 268 switch (this) { 269 case INSTANCE: 270 return "Instance"; 271 case KIND: 272 return "Kind"; 273 case NULL: 274 return null; 275 default: 276 return "?"; 277 } 278 } 279 } 280 281 public static class LocationModeEnumFactory implements EnumFactory<LocationMode> { 282 public LocationMode fromCode(String codeString) throws IllegalArgumentException { 283 if (codeString == null || "".equals(codeString)) 284 if (codeString == null || "".equals(codeString)) 285 return null; 286 if ("instance".equals(codeString)) 287 return LocationMode.INSTANCE; 288 if ("kind".equals(codeString)) 289 return LocationMode.KIND; 290 throw new IllegalArgumentException("Unknown LocationMode code '" + codeString + "'"); 291 } 292 293 public Enumeration<LocationMode> fromType(PrimitiveType<?> code) throws FHIRException { 294 if (code == null) 295 return null; 296 if (code.isEmpty()) 297 return new Enumeration<LocationMode>(this, LocationMode.NULL, code); 298 String codeString = code.asStringValue(); 299 if (codeString == null || "".equals(codeString)) 300 return new Enumeration<LocationMode>(this, LocationMode.NULL, code); 301 if ("instance".equals(codeString)) 302 return new Enumeration<LocationMode>(this, LocationMode.INSTANCE, code); 303 if ("kind".equals(codeString)) 304 return new Enumeration<LocationMode>(this, LocationMode.KIND, code); 305 throw new FHIRException("Unknown LocationMode code '" + codeString + "'"); 306 } 307 308 public String toCode(LocationMode code) { 309 if (code == LocationMode.NULL) 310 return null; 311 if (code == LocationMode.INSTANCE) 312 return "instance"; 313 if (code == LocationMode.KIND) 314 return "kind"; 315 return "?"; 316 } 317 318 public String toSystem(LocationMode code) { 319 return code.getSystem(); 320 } 321 } 322 323 public enum DaysOfWeek { 324 /** 325 * Monday. 326 */ 327 MON, 328 /** 329 * Tuesday. 330 */ 331 TUE, 332 /** 333 * Wednesday. 334 */ 335 WED, 336 /** 337 * Thursday. 338 */ 339 THU, 340 /** 341 * Friday. 342 */ 343 FRI, 344 /** 345 * Saturday. 346 */ 347 SAT, 348 /** 349 * Sunday. 350 */ 351 SUN, 352 /** 353 * added to help the parsers with the generic types 354 */ 355 NULL; 356 357 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 358 if (codeString == null || "".equals(codeString)) 359 return null; 360 if ("mon".equals(codeString)) 361 return MON; 362 if ("tue".equals(codeString)) 363 return TUE; 364 if ("wed".equals(codeString)) 365 return WED; 366 if ("thu".equals(codeString)) 367 return THU; 368 if ("fri".equals(codeString)) 369 return FRI; 370 if ("sat".equals(codeString)) 371 return SAT; 372 if ("sun".equals(codeString)) 373 return SUN; 374 if (Configuration.isAcceptInvalidEnums()) 375 return null; 376 else 377 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 378 } 379 380 public String toCode() { 381 switch (this) { 382 case MON: 383 return "mon"; 384 case TUE: 385 return "tue"; 386 case WED: 387 return "wed"; 388 case THU: 389 return "thu"; 390 case FRI: 391 return "fri"; 392 case SAT: 393 return "sat"; 394 case SUN: 395 return "sun"; 396 case NULL: 397 return null; 398 default: 399 return "?"; 400 } 401 } 402 403 public String getSystem() { 404 switch (this) { 405 case MON: 406 return "http://hl7.org/fhir/days-of-week"; 407 case TUE: 408 return "http://hl7.org/fhir/days-of-week"; 409 case WED: 410 return "http://hl7.org/fhir/days-of-week"; 411 case THU: 412 return "http://hl7.org/fhir/days-of-week"; 413 case FRI: 414 return "http://hl7.org/fhir/days-of-week"; 415 case SAT: 416 return "http://hl7.org/fhir/days-of-week"; 417 case SUN: 418 return "http://hl7.org/fhir/days-of-week"; 419 case NULL: 420 return null; 421 default: 422 return "?"; 423 } 424 } 425 426 public String getDefinition() { 427 switch (this) { 428 case MON: 429 return "Monday."; 430 case TUE: 431 return "Tuesday."; 432 case WED: 433 return "Wednesday."; 434 case THU: 435 return "Thursday."; 436 case FRI: 437 return "Friday."; 438 case SAT: 439 return "Saturday."; 440 case SUN: 441 return "Sunday."; 442 case NULL: 443 return null; 444 default: 445 return "?"; 446 } 447 } 448 449 public String getDisplay() { 450 switch (this) { 451 case MON: 452 return "Monday"; 453 case TUE: 454 return "Tuesday"; 455 case WED: 456 return "Wednesday"; 457 case THU: 458 return "Thursday"; 459 case FRI: 460 return "Friday"; 461 case SAT: 462 return "Saturday"; 463 case SUN: 464 return "Sunday"; 465 case NULL: 466 return null; 467 default: 468 return "?"; 469 } 470 } 471 } 472 473 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 474 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 475 if (codeString == null || "".equals(codeString)) 476 if (codeString == null || "".equals(codeString)) 477 return null; 478 if ("mon".equals(codeString)) 479 return DaysOfWeek.MON; 480 if ("tue".equals(codeString)) 481 return DaysOfWeek.TUE; 482 if ("wed".equals(codeString)) 483 return DaysOfWeek.WED; 484 if ("thu".equals(codeString)) 485 return DaysOfWeek.THU; 486 if ("fri".equals(codeString)) 487 return DaysOfWeek.FRI; 488 if ("sat".equals(codeString)) 489 return DaysOfWeek.SAT; 490 if ("sun".equals(codeString)) 491 return DaysOfWeek.SUN; 492 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 493 } 494 495 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 496 if (code == null) 497 return null; 498 if (code.isEmpty()) 499 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 500 String codeString = code.asStringValue(); 501 if (codeString == null || "".equals(codeString)) 502 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 503 if ("mon".equals(codeString)) 504 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON, code); 505 if ("tue".equals(codeString)) 506 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE, code); 507 if ("wed".equals(codeString)) 508 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED, code); 509 if ("thu".equals(codeString)) 510 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU, code); 511 if ("fri".equals(codeString)) 512 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI, code); 513 if ("sat".equals(codeString)) 514 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT, code); 515 if ("sun".equals(codeString)) 516 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN, code); 517 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 518 } 519 520 public String toCode(DaysOfWeek code) { 521 if (code == DaysOfWeek.NULL) 522 return null; 523 if (code == DaysOfWeek.MON) 524 return "mon"; 525 if (code == DaysOfWeek.TUE) 526 return "tue"; 527 if (code == DaysOfWeek.WED) 528 return "wed"; 529 if (code == DaysOfWeek.THU) 530 return "thu"; 531 if (code == DaysOfWeek.FRI) 532 return "fri"; 533 if (code == DaysOfWeek.SAT) 534 return "sat"; 535 if (code == DaysOfWeek.SUN) 536 return "sun"; 537 return "?"; 538 } 539 540 public String toSystem(DaysOfWeek code) { 541 return code.getSystem(); 542 } 543 } 544 545 @Block() 546 public static class LocationPositionComponent extends BackboneElement implements IBaseBackboneElement { 547 /** 548 * Longitude. The value domain and the interpretation are the same as for the 549 * text of the longitude element in KML (see notes below). 550 */ 551 @Child(name = "longitude", type = { 552 DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 553 @Description(shortDefinition = "Longitude with WGS84 datum", formalDefinition = "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).") 554 protected DecimalType longitude; 555 556 /** 557 * Latitude. The value domain and the interpretation are the same as for the 558 * text of the latitude element in KML (see notes below). 559 */ 560 @Child(name = "latitude", type = { 561 DecimalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 562 @Description(shortDefinition = "Latitude with WGS84 datum", formalDefinition = "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).") 563 protected DecimalType latitude; 564 565 /** 566 * Altitude. The value domain and the interpretation are the same as for the 567 * text of the altitude element in KML (see notes below). 568 */ 569 @Child(name = "altitude", type = { 570 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 571 @Description(shortDefinition = "Altitude with WGS84 datum", formalDefinition = "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).") 572 protected DecimalType altitude; 573 574 private static final long serialVersionUID = -74276134L; 575 576 /** 577 * Constructor 578 */ 579 public LocationPositionComponent() { 580 super(); 581 } 582 583 /** 584 * Constructor 585 */ 586 public LocationPositionComponent(DecimalType longitude, DecimalType latitude) { 587 super(); 588 this.longitude = longitude; 589 this.latitude = latitude; 590 } 591 592 /** 593 * @return {@link #longitude} (Longitude. The value domain and the 594 * interpretation are the same as for the text of the longitude element 595 * in KML (see notes below).). This is the underlying object with id, 596 * value and extensions. The accessor "getLongitude" gives direct access 597 * to the value 598 */ 599 public DecimalType getLongitudeElement() { 600 if (this.longitude == null) 601 if (Configuration.errorOnAutoCreate()) 602 throw new Error("Attempt to auto-create LocationPositionComponent.longitude"); 603 else if (Configuration.doAutoCreate()) 604 this.longitude = new DecimalType(); // bb 605 return this.longitude; 606 } 607 608 public boolean hasLongitudeElement() { 609 return this.longitude != null && !this.longitude.isEmpty(); 610 } 611 612 public boolean hasLongitude() { 613 return this.longitude != null && !this.longitude.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #longitude} (Longitude. The value domain and the 618 * interpretation are the same as for the text of the longitude 619 * element in KML (see notes below).). This is the underlying 620 * object with id, value and extensions. The accessor 621 * "getLongitude" gives direct access to the value 622 */ 623 public LocationPositionComponent setLongitudeElement(DecimalType value) { 624 this.longitude = value; 625 return this; 626 } 627 628 /** 629 * @return Longitude. The value domain and the interpretation are the same as 630 * for the text of the longitude element in KML (see notes below). 631 */ 632 public BigDecimal getLongitude() { 633 return this.longitude == null ? null : this.longitude.getValue(); 634 } 635 636 /** 637 * @param value Longitude. The value domain and the interpretation are the same 638 * as for the text of the longitude element in KML (see notes 639 * below). 640 */ 641 public LocationPositionComponent setLongitude(BigDecimal value) { 642 if (this.longitude == null) 643 this.longitude = new DecimalType(); 644 this.longitude.setValue(value); 645 return this; 646 } 647 648 /** 649 * @param value Longitude. The value domain and the interpretation are the same 650 * as for the text of the longitude element in KML (see notes 651 * below). 652 */ 653 public LocationPositionComponent setLongitude(long value) { 654 this.longitude = new DecimalType(); 655 this.longitude.setValue(value); 656 return this; 657 } 658 659 /** 660 * @param value Longitude. The value domain and the interpretation are the same 661 * as for the text of the longitude element in KML (see notes 662 * below). 663 */ 664 public LocationPositionComponent setLongitude(double value) { 665 this.longitude = new DecimalType(); 666 this.longitude.setValue(value); 667 return this; 668 } 669 670 /** 671 * @return {@link #latitude} (Latitude. The value domain and the interpretation 672 * are the same as for the text of the latitude element in KML (see 673 * notes below).). This is the underlying object with id, value and 674 * extensions. The accessor "getLatitude" gives direct access to the 675 * value 676 */ 677 public DecimalType getLatitudeElement() { 678 if (this.latitude == null) 679 if (Configuration.errorOnAutoCreate()) 680 throw new Error("Attempt to auto-create LocationPositionComponent.latitude"); 681 else if (Configuration.doAutoCreate()) 682 this.latitude = new DecimalType(); // bb 683 return this.latitude; 684 } 685 686 public boolean hasLatitudeElement() { 687 return this.latitude != null && !this.latitude.isEmpty(); 688 } 689 690 public boolean hasLatitude() { 691 return this.latitude != null && !this.latitude.isEmpty(); 692 } 693 694 /** 695 * @param value {@link #latitude} (Latitude. The value domain and the 696 * interpretation are the same as for the text of the latitude 697 * element in KML (see notes below).). This is the underlying 698 * object with id, value and extensions. The accessor "getLatitude" 699 * gives direct access to the value 700 */ 701 public LocationPositionComponent setLatitudeElement(DecimalType value) { 702 this.latitude = value; 703 return this; 704 } 705 706 /** 707 * @return Latitude. The value domain and the interpretation are the same as for 708 * the text of the latitude element in KML (see notes below). 709 */ 710 public BigDecimal getLatitude() { 711 return this.latitude == null ? null : this.latitude.getValue(); 712 } 713 714 /** 715 * @param value Latitude. The value domain and the interpretation are the same 716 * as for the text of the latitude element in KML (see notes 717 * below). 718 */ 719 public LocationPositionComponent setLatitude(BigDecimal value) { 720 if (this.latitude == null) 721 this.latitude = new DecimalType(); 722 this.latitude.setValue(value); 723 return this; 724 } 725 726 /** 727 * @param value Latitude. The value domain and the interpretation are the same 728 * as for the text of the latitude element in KML (see notes 729 * below). 730 */ 731 public LocationPositionComponent setLatitude(long value) { 732 this.latitude = new DecimalType(); 733 this.latitude.setValue(value); 734 return this; 735 } 736 737 /** 738 * @param value Latitude. The value domain and the interpretation are the same 739 * as for the text of the latitude element in KML (see notes 740 * below). 741 */ 742 public LocationPositionComponent setLatitude(double value) { 743 this.latitude = new DecimalType(); 744 this.latitude.setValue(value); 745 return this; 746 } 747 748 /** 749 * @return {@link #altitude} (Altitude. The value domain and the interpretation 750 * are the same as for the text of the altitude element in KML (see 751 * notes below).). This is the underlying object with id, value and 752 * extensions. The accessor "getAltitude" gives direct access to the 753 * value 754 */ 755 public DecimalType getAltitudeElement() { 756 if (this.altitude == null) 757 if (Configuration.errorOnAutoCreate()) 758 throw new Error("Attempt to auto-create LocationPositionComponent.altitude"); 759 else if (Configuration.doAutoCreate()) 760 this.altitude = new DecimalType(); // bb 761 return this.altitude; 762 } 763 764 public boolean hasAltitudeElement() { 765 return this.altitude != null && !this.altitude.isEmpty(); 766 } 767 768 public boolean hasAltitude() { 769 return this.altitude != null && !this.altitude.isEmpty(); 770 } 771 772 /** 773 * @param value {@link #altitude} (Altitude. The value domain and the 774 * interpretation are the same as for the text of the altitude 775 * element in KML (see notes below).). This is the underlying 776 * object with id, value and extensions. The accessor "getAltitude" 777 * gives direct access to the value 778 */ 779 public LocationPositionComponent setAltitudeElement(DecimalType value) { 780 this.altitude = value; 781 return this; 782 } 783 784 /** 785 * @return Altitude. The value domain and the interpretation are the same as for 786 * the text of the altitude element in KML (see notes below). 787 */ 788 public BigDecimal getAltitude() { 789 return this.altitude == null ? null : this.altitude.getValue(); 790 } 791 792 /** 793 * @param value Altitude. The value domain and the interpretation are the same 794 * as for the text of the altitude element in KML (see notes 795 * below). 796 */ 797 public LocationPositionComponent setAltitude(BigDecimal value) { 798 if (value == null) 799 this.altitude = null; 800 else { 801 if (this.altitude == null) 802 this.altitude = new DecimalType(); 803 this.altitude.setValue(value); 804 } 805 return this; 806 } 807 808 /** 809 * @param value Altitude. The value domain and the interpretation are the same 810 * as for the text of the altitude element in KML (see notes 811 * below). 812 */ 813 public LocationPositionComponent setAltitude(long value) { 814 this.altitude = new DecimalType(); 815 this.altitude.setValue(value); 816 return this; 817 } 818 819 /** 820 * @param value Altitude. The value domain and the interpretation are the same 821 * as for the text of the altitude element in KML (see notes 822 * below). 823 */ 824 public LocationPositionComponent setAltitude(double value) { 825 this.altitude = new DecimalType(); 826 this.altitude.setValue(value); 827 return this; 828 } 829 830 protected void listChildren(List<Property> children) { 831 super.listChildren(children); 832 children.add(new Property("longitude", "decimal", 833 "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 834 0, 1, longitude)); 835 children.add(new Property("latitude", "decimal", 836 "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 837 0, 1, latitude)); 838 children.add(new Property("altitude", "decimal", 839 "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 840 0, 1, altitude)); 841 } 842 843 @Override 844 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 845 switch (_hash) { 846 case 137365935: 847 /* longitude */ return new Property("longitude", "decimal", 848 "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 849 0, 1, longitude); 850 case -1439978388: 851 /* latitude */ return new Property("latitude", "decimal", 852 "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 853 0, 1, latitude); 854 case 2036550306: 855 /* altitude */ return new Property("altitude", "decimal", 856 "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 857 0, 1, altitude); 858 default: 859 return super.getNamedProperty(_hash, _name, _checkValid); 860 } 861 862 } 863 864 @Override 865 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 866 switch (hash) { 867 case 137365935: 868 /* longitude */ return this.longitude == null ? new Base[0] : new Base[] { this.longitude }; // DecimalType 869 case -1439978388: 870 /* latitude */ return this.latitude == null ? new Base[0] : new Base[] { this.latitude }; // DecimalType 871 case 2036550306: 872 /* altitude */ return this.altitude == null ? new Base[0] : new Base[] { this.altitude }; // DecimalType 873 default: 874 return super.getProperty(hash, name, checkValid); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 881 switch (hash) { 882 case 137365935: // longitude 883 this.longitude = castToDecimal(value); // DecimalType 884 return value; 885 case -1439978388: // latitude 886 this.latitude = castToDecimal(value); // DecimalType 887 return value; 888 case 2036550306: // altitude 889 this.altitude = castToDecimal(value); // DecimalType 890 return value; 891 default: 892 return super.setProperty(hash, name, value); 893 } 894 895 } 896 897 @Override 898 public Base setProperty(String name, Base value) throws FHIRException { 899 if (name.equals("longitude")) { 900 this.longitude = castToDecimal(value); // DecimalType 901 } else if (name.equals("latitude")) { 902 this.latitude = castToDecimal(value); // DecimalType 903 } else if (name.equals("altitude")) { 904 this.altitude = castToDecimal(value); // DecimalType 905 } else 906 return super.setProperty(name, value); 907 return value; 908 } 909 910 @Override 911 public void removeChild(String name, Base value) throws FHIRException { 912 if (name.equals("longitude")) { 913 this.longitude = null; 914 } else if (name.equals("latitude")) { 915 this.latitude = null; 916 } else if (name.equals("altitude")) { 917 this.altitude = null; 918 } else 919 super.removeChild(name, value); 920 921 } 922 923 @Override 924 public Base makeProperty(int hash, String name) throws FHIRException { 925 switch (hash) { 926 case 137365935: 927 return getLongitudeElement(); 928 case -1439978388: 929 return getLatitudeElement(); 930 case 2036550306: 931 return getAltitudeElement(); 932 default: 933 return super.makeProperty(hash, name); 934 } 935 936 } 937 938 @Override 939 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 940 switch (hash) { 941 case 137365935: 942 /* longitude */ return new String[] { "decimal" }; 943 case -1439978388: 944 /* latitude */ return new String[] { "decimal" }; 945 case 2036550306: 946 /* altitude */ return new String[] { "decimal" }; 947 default: 948 return super.getTypesForProperty(hash, name); 949 } 950 951 } 952 953 @Override 954 public Base addChild(String name) throws FHIRException { 955 if (name.equals("longitude")) { 956 throw new FHIRException("Cannot call addChild on a singleton property Location.longitude"); 957 } else if (name.equals("latitude")) { 958 throw new FHIRException("Cannot call addChild on a singleton property Location.latitude"); 959 } else if (name.equals("altitude")) { 960 throw new FHIRException("Cannot call addChild on a singleton property Location.altitude"); 961 } else 962 return super.addChild(name); 963 } 964 965 public LocationPositionComponent copy() { 966 LocationPositionComponent dst = new LocationPositionComponent(); 967 copyValues(dst); 968 return dst; 969 } 970 971 public void copyValues(LocationPositionComponent dst) { 972 super.copyValues(dst); 973 dst.longitude = longitude == null ? null : longitude.copy(); 974 dst.latitude = latitude == null ? null : latitude.copy(); 975 dst.altitude = altitude == null ? null : altitude.copy(); 976 } 977 978 @Override 979 public boolean equalsDeep(Base other_) { 980 if (!super.equalsDeep(other_)) 981 return false; 982 if (!(other_ instanceof LocationPositionComponent)) 983 return false; 984 LocationPositionComponent o = (LocationPositionComponent) other_; 985 return compareDeep(longitude, o.longitude, true) && compareDeep(latitude, o.latitude, true) 986 && compareDeep(altitude, o.altitude, true); 987 } 988 989 @Override 990 public boolean equalsShallow(Base other_) { 991 if (!super.equalsShallow(other_)) 992 return false; 993 if (!(other_ instanceof LocationPositionComponent)) 994 return false; 995 LocationPositionComponent o = (LocationPositionComponent) other_; 996 return compareValues(longitude, o.longitude, true) && compareValues(latitude, o.latitude, true) 997 && compareValues(altitude, o.altitude, true); 998 } 999 1000 public boolean isEmpty() { 1001 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(longitude, latitude, altitude); 1002 } 1003 1004 public String fhirType() { 1005 return "Location.position"; 1006 1007 } 1008 1009 } 1010 1011 @Block() 1012 public static class LocationHoursOfOperationComponent extends BackboneElement implements IBaseBackboneElement { 1013 /** 1014 * Indicates which days of the week are available between the start and end 1015 * Times. 1016 */ 1017 @Child(name = "daysOfWeek", type = { 1018 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1019 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 1020 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 1021 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 1022 1023 /** 1024 * The Location is open all day. 1025 */ 1026 @Child(name = "allDay", type = { 1027 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1028 @Description(shortDefinition = "The Location is open all day", formalDefinition = "The Location is open all day.") 1029 protected BooleanType allDay; 1030 1031 /** 1032 * Time that the Location opens. 1033 */ 1034 @Child(name = "openingTime", type = { 1035 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1036 @Description(shortDefinition = "Time that the Location opens", formalDefinition = "Time that the Location opens.") 1037 protected TimeType openingTime; 1038 1039 /** 1040 * Time that the Location closes. 1041 */ 1042 @Child(name = "closingTime", type = { 1043 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1044 @Description(shortDefinition = "Time that the Location closes", formalDefinition = "Time that the Location closes.") 1045 protected TimeType closingTime; 1046 1047 private static final long serialVersionUID = -932551849L; 1048 1049 /** 1050 * Constructor 1051 */ 1052 public LocationHoursOfOperationComponent() { 1053 super(); 1054 } 1055 1056 /** 1057 * @return {@link #daysOfWeek} (Indicates which days of the week are available 1058 * between the start and end Times.) 1059 */ 1060 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 1061 if (this.daysOfWeek == null) 1062 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1063 return this.daysOfWeek; 1064 } 1065 1066 /** 1067 * @return Returns a reference to <code>this</code> for easy method chaining 1068 */ 1069 public LocationHoursOfOperationComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 1070 this.daysOfWeek = theDaysOfWeek; 1071 return this; 1072 } 1073 1074 public boolean hasDaysOfWeek() { 1075 if (this.daysOfWeek == null) 1076 return false; 1077 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 1078 if (!item.isEmpty()) 1079 return true; 1080 return false; 1081 } 1082 1083 /** 1084 * @return {@link #daysOfWeek} (Indicates which days of the week are available 1085 * between the start and end Times.) 1086 */ 1087 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 1088 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 1089 if (this.daysOfWeek == null) 1090 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1091 this.daysOfWeek.add(t); 1092 return t; 1093 } 1094 1095 /** 1096 * @param value {@link #daysOfWeek} (Indicates which days of the week are 1097 * available between the start and end Times.) 1098 */ 1099 public LocationHoursOfOperationComponent addDaysOfWeek(DaysOfWeek value) { // 1 1100 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 1101 t.setValue(value); 1102 if (this.daysOfWeek == null) 1103 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1104 this.daysOfWeek.add(t); 1105 return this; 1106 } 1107 1108 /** 1109 * @param value {@link #daysOfWeek} (Indicates which days of the week are 1110 * available between the start and end Times.) 1111 */ 1112 public boolean hasDaysOfWeek(DaysOfWeek value) { 1113 if (this.daysOfWeek == null) 1114 return false; 1115 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 1116 if (v.getValue().equals(value)) // code 1117 return true; 1118 return false; 1119 } 1120 1121 /** 1122 * @return {@link #allDay} (The Location is open all day.). This is the 1123 * underlying object with id, value and extensions. The accessor 1124 * "getAllDay" gives direct access to the value 1125 */ 1126 public BooleanType getAllDayElement() { 1127 if (this.allDay == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create LocationHoursOfOperationComponent.allDay"); 1130 else if (Configuration.doAutoCreate()) 1131 this.allDay = new BooleanType(); // bb 1132 return this.allDay; 1133 } 1134 1135 public boolean hasAllDayElement() { 1136 return this.allDay != null && !this.allDay.isEmpty(); 1137 } 1138 1139 public boolean hasAllDay() { 1140 return this.allDay != null && !this.allDay.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #allDay} (The Location is open all day.). This is the 1145 * underlying object with id, value and extensions. The accessor 1146 * "getAllDay" gives direct access to the value 1147 */ 1148 public LocationHoursOfOperationComponent setAllDayElement(BooleanType value) { 1149 this.allDay = value; 1150 return this; 1151 } 1152 1153 /** 1154 * @return The Location is open all day. 1155 */ 1156 public boolean getAllDay() { 1157 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 1158 } 1159 1160 /** 1161 * @param value The Location is open all day. 1162 */ 1163 public LocationHoursOfOperationComponent setAllDay(boolean value) { 1164 if (this.allDay == null) 1165 this.allDay = new BooleanType(); 1166 this.allDay.setValue(value); 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #openingTime} (Time that the Location opens.). This is the 1172 * underlying object with id, value and extensions. The accessor 1173 * "getOpeningTime" gives direct access to the value 1174 */ 1175 public TimeType getOpeningTimeElement() { 1176 if (this.openingTime == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create LocationHoursOfOperationComponent.openingTime"); 1179 else if (Configuration.doAutoCreate()) 1180 this.openingTime = new TimeType(); // bb 1181 return this.openingTime; 1182 } 1183 1184 public boolean hasOpeningTimeElement() { 1185 return this.openingTime != null && !this.openingTime.isEmpty(); 1186 } 1187 1188 public boolean hasOpeningTime() { 1189 return this.openingTime != null && !this.openingTime.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #openingTime} (Time that the Location opens.). This is 1194 * the underlying object with id, value and extensions. The 1195 * accessor "getOpeningTime" gives direct access to the value 1196 */ 1197 public LocationHoursOfOperationComponent setOpeningTimeElement(TimeType value) { 1198 this.openingTime = value; 1199 return this; 1200 } 1201 1202 /** 1203 * @return Time that the Location opens. 1204 */ 1205 public String getOpeningTime() { 1206 return this.openingTime == null ? null : this.openingTime.getValue(); 1207 } 1208 1209 /** 1210 * @param value Time that the Location opens. 1211 */ 1212 public LocationHoursOfOperationComponent setOpeningTime(String value) { 1213 if (value == null) 1214 this.openingTime = null; 1215 else { 1216 if (this.openingTime == null) 1217 this.openingTime = new TimeType(); 1218 this.openingTime.setValue(value); 1219 } 1220 return this; 1221 } 1222 1223 /** 1224 * @return {@link #closingTime} (Time that the Location closes.). This is the 1225 * underlying object with id, value and extensions. The accessor 1226 * "getClosingTime" gives direct access to the value 1227 */ 1228 public TimeType getClosingTimeElement() { 1229 if (this.closingTime == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create LocationHoursOfOperationComponent.closingTime"); 1232 else if (Configuration.doAutoCreate()) 1233 this.closingTime = new TimeType(); // bb 1234 return this.closingTime; 1235 } 1236 1237 public boolean hasClosingTimeElement() { 1238 return this.closingTime != null && !this.closingTime.isEmpty(); 1239 } 1240 1241 public boolean hasClosingTime() { 1242 return this.closingTime != null && !this.closingTime.isEmpty(); 1243 } 1244 1245 /** 1246 * @param value {@link #closingTime} (Time that the Location closes.). This is 1247 * the underlying object with id, value and extensions. The 1248 * accessor "getClosingTime" gives direct access to the value 1249 */ 1250 public LocationHoursOfOperationComponent setClosingTimeElement(TimeType value) { 1251 this.closingTime = value; 1252 return this; 1253 } 1254 1255 /** 1256 * @return Time that the Location closes. 1257 */ 1258 public String getClosingTime() { 1259 return this.closingTime == null ? null : this.closingTime.getValue(); 1260 } 1261 1262 /** 1263 * @param value Time that the Location closes. 1264 */ 1265 public LocationHoursOfOperationComponent setClosingTime(String value) { 1266 if (value == null) 1267 this.closingTime = null; 1268 else { 1269 if (this.closingTime == null) 1270 this.closingTime = new TimeType(); 1271 this.closingTime.setValue(value); 1272 } 1273 return this; 1274 } 1275 1276 protected void listChildren(List<Property> children) { 1277 super.listChildren(children); 1278 children.add(new Property("daysOfWeek", "code", 1279 "Indicates which days of the week are available between the start and end Times.", 0, 1280 java.lang.Integer.MAX_VALUE, daysOfWeek)); 1281 children.add(new Property("allDay", "boolean", "The Location is open all day.", 0, 1, allDay)); 1282 children.add(new Property("openingTime", "time", "Time that the Location opens.", 0, 1, openingTime)); 1283 children.add(new Property("closingTime", "time", "Time that the Location closes.", 0, 1, closingTime)); 1284 } 1285 1286 @Override 1287 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1288 switch (_hash) { 1289 case 68050338: 1290 /* daysOfWeek */ return new Property("daysOfWeek", "code", 1291 "Indicates which days of the week are available between the start and end Times.", 0, 1292 java.lang.Integer.MAX_VALUE, daysOfWeek); 1293 case -1414913477: 1294 /* allDay */ return new Property("allDay", "boolean", "The Location is open all day.", 0, 1, allDay); 1295 case 84062277: 1296 /* openingTime */ return new Property("openingTime", "time", "Time that the Location opens.", 0, 1, 1297 openingTime); 1298 case 188137762: 1299 /* closingTime */ return new Property("closingTime", "time", "Time that the Location closes.", 0, 1, 1300 closingTime); 1301 default: 1302 return super.getNamedProperty(_hash, _name, _checkValid); 1303 } 1304 1305 } 1306 1307 @Override 1308 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1309 switch (hash) { 1310 case 68050338: 1311 /* daysOfWeek */ return this.daysOfWeek == null ? new Base[0] 1312 : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 1313 case -1414913477: 1314 /* allDay */ return this.allDay == null ? new Base[0] : new Base[] { this.allDay }; // BooleanType 1315 case 84062277: 1316 /* openingTime */ return this.openingTime == null ? new Base[0] : new Base[] { this.openingTime }; // TimeType 1317 case 188137762: 1318 /* closingTime */ return this.closingTime == null ? new Base[0] : new Base[] { this.closingTime }; // TimeType 1319 default: 1320 return super.getProperty(hash, name, checkValid); 1321 } 1322 1323 } 1324 1325 @Override 1326 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1327 switch (hash) { 1328 case 68050338: // daysOfWeek 1329 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 1330 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 1331 return value; 1332 case -1414913477: // allDay 1333 this.allDay = castToBoolean(value); // BooleanType 1334 return value; 1335 case 84062277: // openingTime 1336 this.openingTime = castToTime(value); // TimeType 1337 return value; 1338 case 188137762: // closingTime 1339 this.closingTime = castToTime(value); // TimeType 1340 return value; 1341 default: 1342 return super.setProperty(hash, name, value); 1343 } 1344 1345 } 1346 1347 @Override 1348 public Base setProperty(String name, Base value) throws FHIRException { 1349 if (name.equals("daysOfWeek")) { 1350 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 1351 this.getDaysOfWeek().add((Enumeration) value); 1352 } else if (name.equals("allDay")) { 1353 this.allDay = castToBoolean(value); // BooleanType 1354 } else if (name.equals("openingTime")) { 1355 this.openingTime = castToTime(value); // TimeType 1356 } else if (name.equals("closingTime")) { 1357 this.closingTime = castToTime(value); // TimeType 1358 } else 1359 return super.setProperty(name, value); 1360 return value; 1361 } 1362 1363 @Override 1364 public void removeChild(String name, Base value) throws FHIRException { 1365 if (name.equals("daysOfWeek")) { 1366 this.getDaysOfWeek().remove((Enumeration) value); 1367 } else if (name.equals("allDay")) { 1368 this.allDay = null; 1369 } else if (name.equals("openingTime")) { 1370 this.openingTime = null; 1371 } else if (name.equals("closingTime")) { 1372 this.closingTime = null; 1373 } else 1374 super.removeChild(name, value); 1375 1376 } 1377 1378 @Override 1379 public Base makeProperty(int hash, String name) throws FHIRException { 1380 switch (hash) { 1381 case 68050338: 1382 return addDaysOfWeekElement(); 1383 case -1414913477: 1384 return getAllDayElement(); 1385 case 84062277: 1386 return getOpeningTimeElement(); 1387 case 188137762: 1388 return getClosingTimeElement(); 1389 default: 1390 return super.makeProperty(hash, name); 1391 } 1392 1393 } 1394 1395 @Override 1396 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1397 switch (hash) { 1398 case 68050338: 1399 /* daysOfWeek */ return new String[] { "code" }; 1400 case -1414913477: 1401 /* allDay */ return new String[] { "boolean" }; 1402 case 84062277: 1403 /* openingTime */ return new String[] { "time" }; 1404 case 188137762: 1405 /* closingTime */ return new String[] { "time" }; 1406 default: 1407 return super.getTypesForProperty(hash, name); 1408 } 1409 1410 } 1411 1412 @Override 1413 public Base addChild(String name) throws FHIRException { 1414 if (name.equals("daysOfWeek")) { 1415 throw new FHIRException("Cannot call addChild on a singleton property Location.daysOfWeek"); 1416 } else if (name.equals("allDay")) { 1417 throw new FHIRException("Cannot call addChild on a singleton property Location.allDay"); 1418 } else if (name.equals("openingTime")) { 1419 throw new FHIRException("Cannot call addChild on a singleton property Location.openingTime"); 1420 } else if (name.equals("closingTime")) { 1421 throw new FHIRException("Cannot call addChild on a singleton property Location.closingTime"); 1422 } else 1423 return super.addChild(name); 1424 } 1425 1426 public LocationHoursOfOperationComponent copy() { 1427 LocationHoursOfOperationComponent dst = new LocationHoursOfOperationComponent(); 1428 copyValues(dst); 1429 return dst; 1430 } 1431 1432 public void copyValues(LocationHoursOfOperationComponent dst) { 1433 super.copyValues(dst); 1434 if (daysOfWeek != null) { 1435 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1436 for (Enumeration<DaysOfWeek> i : daysOfWeek) 1437 dst.daysOfWeek.add(i.copy()); 1438 } 1439 ; 1440 dst.allDay = allDay == null ? null : allDay.copy(); 1441 dst.openingTime = openingTime == null ? null : openingTime.copy(); 1442 dst.closingTime = closingTime == null ? null : closingTime.copy(); 1443 } 1444 1445 @Override 1446 public boolean equalsDeep(Base other_) { 1447 if (!super.equalsDeep(other_)) 1448 return false; 1449 if (!(other_ instanceof LocationHoursOfOperationComponent)) 1450 return false; 1451 LocationHoursOfOperationComponent o = (LocationHoursOfOperationComponent) other_; 1452 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 1453 && compareDeep(openingTime, o.openingTime, true) && compareDeep(closingTime, o.closingTime, true); 1454 } 1455 1456 @Override 1457 public boolean equalsShallow(Base other_) { 1458 if (!super.equalsShallow(other_)) 1459 return false; 1460 if (!(other_ instanceof LocationHoursOfOperationComponent)) 1461 return false; 1462 LocationHoursOfOperationComponent o = (LocationHoursOfOperationComponent) other_; 1463 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 1464 && compareValues(openingTime, o.openingTime, true) && compareValues(closingTime, o.closingTime, true); 1465 } 1466 1467 public boolean isEmpty() { 1468 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, openingTime, closingTime); 1469 } 1470 1471 public String fhirType() { 1472 return "Location.hoursOfOperation"; 1473 1474 } 1475 1476 } 1477 1478 /** 1479 * Unique code or number identifying the location to its users. 1480 */ 1481 @Child(name = "identifier", type = { 1482 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1483 @Description(shortDefinition = "Unique code or number identifying the location to its users", formalDefinition = "Unique code or number identifying the location to its users.") 1484 protected List<Identifier> identifier; 1485 1486 /** 1487 * The status property covers the general availability of the resource, not the 1488 * current value which may be covered by the operationStatus, or by a 1489 * schedule/slots if they are configured for the location. 1490 */ 1491 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1492 @Description(shortDefinition = "active | suspended | inactive", formalDefinition = "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.") 1493 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-status") 1494 protected Enumeration<LocationStatus> status; 1495 1496 /** 1497 * The operational status covers operation values most relevant to beds (but can 1498 * also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis 1499 * chair). This typically covers concepts such as contamination, housekeeping, 1500 * and other activities like maintenance. 1501 */ 1502 @Child(name = "operationalStatus", type = { 1503 Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1504 @Description(shortDefinition = "The operational status of the location (typically only for a bed/room)", formalDefinition = "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.") 1505 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0116") 1506 protected Coding operationalStatus; 1507 1508 /** 1509 * Name of the location as used by humans. Does not need to be unique. 1510 */ 1511 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1512 @Description(shortDefinition = "Name of the location as used by humans", formalDefinition = "Name of the location as used by humans. Does not need to be unique.") 1513 protected StringType name; 1514 1515 /** 1516 * A list of alternate names that the location is known as, or was known as, in 1517 * the past. 1518 */ 1519 @Child(name = "alias", type = { 1520 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1521 @Description(shortDefinition = "A list of alternate names that the location is known as, or was known as, in the past", formalDefinition = "A list of alternate names that the location is known as, or was known as, in the past.") 1522 protected List<StringType> alias; 1523 1524 /** 1525 * Description of the Location, which helps in finding or referencing the place. 1526 */ 1527 @Child(name = "description", type = { 1528 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1529 @Description(shortDefinition = "Additional details about the location that could be displayed as further information to identify the location beyond its name", formalDefinition = "Description of the Location, which helps in finding or referencing the place.") 1530 protected StringType description; 1531 1532 /** 1533 * Indicates whether a resource instance represents a specific location or a 1534 * class of locations. 1535 */ 1536 @Child(name = "mode", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1537 @Description(shortDefinition = "instance | kind", formalDefinition = "Indicates whether a resource instance represents a specific location or a class of locations.") 1538 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-mode") 1539 protected Enumeration<LocationMode> mode; 1540 1541 /** 1542 * Indicates the type of function performed at the location. 1543 */ 1544 @Child(name = "type", type = { 1545 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1546 @Description(shortDefinition = "Type of function performed", formalDefinition = "Indicates the type of function performed at the location.") 1547 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType") 1548 protected List<CodeableConcept> type; 1549 1550 /** 1551 * The contact details of communication devices available at the location. This 1552 * can include phone numbers, fax numbers, mobile numbers, email addresses and 1553 * web sites. 1554 */ 1555 @Child(name = "telecom", type = { 1556 ContactPoint.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1557 @Description(shortDefinition = "Contact details of the location", formalDefinition = "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.") 1558 protected List<ContactPoint> telecom; 1559 1560 /** 1561 * Physical location. 1562 */ 1563 @Child(name = "address", type = { Address.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1564 @Description(shortDefinition = "Physical location", formalDefinition = "Physical location.") 1565 protected Address address; 1566 1567 /** 1568 * Physical form of the location, e.g. building, room, vehicle, road. 1569 */ 1570 @Child(name = "physicalType", type = { 1571 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1572 @Description(shortDefinition = "Physical form of the location", formalDefinition = "Physical form of the location, e.g. building, room, vehicle, road.") 1573 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-physical-type") 1574 protected CodeableConcept physicalType; 1575 1576 /** 1577 * The absolute geographic location of the Location, expressed using the WGS84 1578 * datum (This is the same co-ordinate system used in KML). 1579 */ 1580 @Child(name = "position", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 1581 @Description(shortDefinition = "The absolute geographic location", formalDefinition = "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).") 1582 protected LocationPositionComponent position; 1583 1584 /** 1585 * The organization responsible for the provisioning and upkeep of the location. 1586 */ 1587 @Child(name = "managingOrganization", type = { 1588 Organization.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1589 @Description(shortDefinition = "Organization responsible for provisioning and upkeep", formalDefinition = "The organization responsible for the provisioning and upkeep of the location.") 1590 protected Reference managingOrganization; 1591 1592 /** 1593 * The actual object that is the target of the reference (The organization 1594 * responsible for the provisioning and upkeep of the location.) 1595 */ 1596 protected Organization managingOrganizationTarget; 1597 1598 /** 1599 * Another Location of which this Location is physically a part of. 1600 */ 1601 @Child(name = "partOf", type = { Location.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1602 @Description(shortDefinition = "Another Location this one is physically a part of", formalDefinition = "Another Location of which this Location is physically a part of.") 1603 protected Reference partOf; 1604 1605 /** 1606 * The actual object that is the target of the reference (Another Location of 1607 * which this Location is physically a part of.) 1608 */ 1609 protected Location partOfTarget; 1610 1611 /** 1612 * What days/times during a week is this location usually open. 1613 */ 1614 @Child(name = "hoursOfOperation", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1615 @Description(shortDefinition = "What days/times during a week is this location usually open", formalDefinition = "What days/times during a week is this location usually open.") 1616 protected List<LocationHoursOfOperationComponent> hoursOfOperation; 1617 1618 /** 1619 * A description of when the locations opening ours are different to normal, 1620 * e.g. public holiday availability. Succinctly describing all possible 1621 * exceptions to normal site availability as detailed in the opening hours 1622 * Times. 1623 */ 1624 @Child(name = "availabilityExceptions", type = { 1625 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1626 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of when the locations opening ours are different to normal, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as detailed in the opening hours Times.") 1627 protected StringType availabilityExceptions; 1628 1629 /** 1630 * Technical endpoints providing access to services operated for the location. 1631 */ 1632 @Child(name = "endpoint", type = { 1633 Endpoint.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1634 @Description(shortDefinition = "Technical endpoints providing access to services operated for the location", formalDefinition = "Technical endpoints providing access to services operated for the location.") 1635 protected List<Reference> endpoint; 1636 /** 1637 * The actual objects that are the target of the reference (Technical endpoints 1638 * providing access to services operated for the location.) 1639 */ 1640 protected List<Endpoint> endpointTarget; 1641 1642 private static final long serialVersionUID = -2126621333L; 1643 1644 /** 1645 * Constructor 1646 */ 1647 public Location() { 1648 super(); 1649 } 1650 1651 /** 1652 * @return {@link #identifier} (Unique code or number identifying the location 1653 * to its users.) 1654 */ 1655 public List<Identifier> getIdentifier() { 1656 if (this.identifier == null) 1657 this.identifier = new ArrayList<Identifier>(); 1658 return this.identifier; 1659 } 1660 1661 /** 1662 * @return Returns a reference to <code>this</code> for easy method chaining 1663 */ 1664 public Location setIdentifier(List<Identifier> theIdentifier) { 1665 this.identifier = theIdentifier; 1666 return this; 1667 } 1668 1669 public boolean hasIdentifier() { 1670 if (this.identifier == null) 1671 return false; 1672 for (Identifier item : this.identifier) 1673 if (!item.isEmpty()) 1674 return true; 1675 return false; 1676 } 1677 1678 public Identifier addIdentifier() { // 3 1679 Identifier t = new Identifier(); 1680 if (this.identifier == null) 1681 this.identifier = new ArrayList<Identifier>(); 1682 this.identifier.add(t); 1683 return t; 1684 } 1685 1686 public Location addIdentifier(Identifier t) { // 3 1687 if (t == null) 1688 return this; 1689 if (this.identifier == null) 1690 this.identifier = new ArrayList<Identifier>(); 1691 this.identifier.add(t); 1692 return this; 1693 } 1694 1695 /** 1696 * @return The first repetition of repeating field {@link #identifier}, creating 1697 * it if it does not already exist 1698 */ 1699 public Identifier getIdentifierFirstRep() { 1700 if (getIdentifier().isEmpty()) { 1701 addIdentifier(); 1702 } 1703 return getIdentifier().get(0); 1704 } 1705 1706 /** 1707 * @return {@link #status} (The status property covers the general availability 1708 * of the resource, not the current value which may be covered by the 1709 * operationStatus, or by a schedule/slots if they are configured for 1710 * the location.). This is the underlying object with id, value and 1711 * extensions. The accessor "getStatus" gives direct access to the value 1712 */ 1713 public Enumeration<LocationStatus> getStatusElement() { 1714 if (this.status == null) 1715 if (Configuration.errorOnAutoCreate()) 1716 throw new Error("Attempt to auto-create Location.status"); 1717 else if (Configuration.doAutoCreate()) 1718 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); // bb 1719 return this.status; 1720 } 1721 1722 public boolean hasStatusElement() { 1723 return this.status != null && !this.status.isEmpty(); 1724 } 1725 1726 public boolean hasStatus() { 1727 return this.status != null && !this.status.isEmpty(); 1728 } 1729 1730 /** 1731 * @param value {@link #status} (The status property covers the general 1732 * availability of the resource, not the current value which may be 1733 * covered by the operationStatus, or by a schedule/slots if they 1734 * are configured for the location.). This is the underlying object 1735 * with id, value and extensions. The accessor "getStatus" gives 1736 * direct access to the value 1737 */ 1738 public Location setStatusElement(Enumeration<LocationStatus> value) { 1739 this.status = value; 1740 return this; 1741 } 1742 1743 /** 1744 * @return The status property covers the general availability of the resource, 1745 * not the current value which may be covered by the operationStatus, or 1746 * by a schedule/slots if they are configured for the location. 1747 */ 1748 public LocationStatus getStatus() { 1749 return this.status == null ? null : this.status.getValue(); 1750 } 1751 1752 /** 1753 * @param value The status property covers the general availability of the 1754 * resource, not the current value which may be covered by the 1755 * operationStatus, or by a schedule/slots if they are configured 1756 * for the location. 1757 */ 1758 public Location setStatus(LocationStatus value) { 1759 if (value == null) 1760 this.status = null; 1761 else { 1762 if (this.status == null) 1763 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); 1764 this.status.setValue(value); 1765 } 1766 return this; 1767 } 1768 1769 /** 1770 * @return {@link #operationalStatus} (The operational status covers operation 1771 * values most relevant to beds (but can also apply to 1772 * rooms/units/chairs/etc. such as an isolation unit/dialysis chair). 1773 * This typically covers concepts such as contamination, housekeeping, 1774 * and other activities like maintenance.) 1775 */ 1776 public Coding getOperationalStatus() { 1777 if (this.operationalStatus == null) 1778 if (Configuration.errorOnAutoCreate()) 1779 throw new Error("Attempt to auto-create Location.operationalStatus"); 1780 else if (Configuration.doAutoCreate()) 1781 this.operationalStatus = new Coding(); // cc 1782 return this.operationalStatus; 1783 } 1784 1785 public boolean hasOperationalStatus() { 1786 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1787 } 1788 1789 /** 1790 * @param value {@link #operationalStatus} (The operational status covers 1791 * operation values most relevant to beds (but can also apply to 1792 * rooms/units/chairs/etc. such as an isolation unit/dialysis 1793 * chair). This typically covers concepts such as contamination, 1794 * housekeeping, and other activities like maintenance.) 1795 */ 1796 public Location setOperationalStatus(Coding value) { 1797 this.operationalStatus = value; 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #name} (Name of the location as used by humans. Does not need 1803 * to be unique.). This is the underlying object with id, value and 1804 * extensions. The accessor "getName" gives direct access to the value 1805 */ 1806 public StringType getNameElement() { 1807 if (this.name == null) 1808 if (Configuration.errorOnAutoCreate()) 1809 throw new Error("Attempt to auto-create Location.name"); 1810 else if (Configuration.doAutoCreate()) 1811 this.name = new StringType(); // bb 1812 return this.name; 1813 } 1814 1815 public boolean hasNameElement() { 1816 return this.name != null && !this.name.isEmpty(); 1817 } 1818 1819 public boolean hasName() { 1820 return this.name != null && !this.name.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #name} (Name of the location as used by humans. Does not 1825 * need to be unique.). This is the underlying object with id, 1826 * value and extensions. The accessor "getName" gives direct access 1827 * to the value 1828 */ 1829 public Location setNameElement(StringType value) { 1830 this.name = value; 1831 return this; 1832 } 1833 1834 /** 1835 * @return Name of the location as used by humans. Does not need to be unique. 1836 */ 1837 public String getName() { 1838 return this.name == null ? null : this.name.getValue(); 1839 } 1840 1841 /** 1842 * @param value Name of the location as used by humans. Does not need to be 1843 * unique. 1844 */ 1845 public Location setName(String value) { 1846 if (Utilities.noString(value)) 1847 this.name = null; 1848 else { 1849 if (this.name == null) 1850 this.name = new StringType(); 1851 this.name.setValue(value); 1852 } 1853 return this; 1854 } 1855 1856 /** 1857 * @return {@link #alias} (A list of alternate names that the location is known 1858 * as, or was known as, in the past.) 1859 */ 1860 public List<StringType> getAlias() { 1861 if (this.alias == null) 1862 this.alias = new ArrayList<StringType>(); 1863 return this.alias; 1864 } 1865 1866 /** 1867 * @return Returns a reference to <code>this</code> for easy method chaining 1868 */ 1869 public Location setAlias(List<StringType> theAlias) { 1870 this.alias = theAlias; 1871 return this; 1872 } 1873 1874 public boolean hasAlias() { 1875 if (this.alias == null) 1876 return false; 1877 for (StringType item : this.alias) 1878 if (!item.isEmpty()) 1879 return true; 1880 return false; 1881 } 1882 1883 /** 1884 * @return {@link #alias} (A list of alternate names that the location is known 1885 * as, or was known as, in the past.) 1886 */ 1887 public StringType addAliasElement() {// 2 1888 StringType t = new StringType(); 1889 if (this.alias == null) 1890 this.alias = new ArrayList<StringType>(); 1891 this.alias.add(t); 1892 return t; 1893 } 1894 1895 /** 1896 * @param value {@link #alias} (A list of alternate names that the location is 1897 * known as, or was known as, in the past.) 1898 */ 1899 public Location addAlias(String value) { // 1 1900 StringType t = new StringType(); 1901 t.setValue(value); 1902 if (this.alias == null) 1903 this.alias = new ArrayList<StringType>(); 1904 this.alias.add(t); 1905 return this; 1906 } 1907 1908 /** 1909 * @param value {@link #alias} (A list of alternate names that the location is 1910 * known as, or was known as, in the past.) 1911 */ 1912 public boolean hasAlias(String value) { 1913 if (this.alias == null) 1914 return false; 1915 for (StringType v : this.alias) 1916 if (v.getValue().equals(value)) // string 1917 return true; 1918 return false; 1919 } 1920 1921 /** 1922 * @return {@link #description} (Description of the Location, which helps in 1923 * finding or referencing the place.). This is the underlying object 1924 * with id, value and extensions. The accessor "getDescription" gives 1925 * direct access to the value 1926 */ 1927 public StringType getDescriptionElement() { 1928 if (this.description == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create Location.description"); 1931 else if (Configuration.doAutoCreate()) 1932 this.description = new StringType(); // bb 1933 return this.description; 1934 } 1935 1936 public boolean hasDescriptionElement() { 1937 return this.description != null && !this.description.isEmpty(); 1938 } 1939 1940 public boolean hasDescription() { 1941 return this.description != null && !this.description.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #description} (Description of the Location, which helps 1946 * in finding or referencing the place.). This is the underlying 1947 * object with id, value and extensions. The accessor 1948 * "getDescription" gives direct access to the value 1949 */ 1950 public Location setDescriptionElement(StringType value) { 1951 this.description = value; 1952 return this; 1953 } 1954 1955 /** 1956 * @return Description of the Location, which helps in finding or referencing 1957 * the place. 1958 */ 1959 public String getDescription() { 1960 return this.description == null ? null : this.description.getValue(); 1961 } 1962 1963 /** 1964 * @param value Description of the Location, which helps in finding or 1965 * referencing the place. 1966 */ 1967 public Location setDescription(String value) { 1968 if (Utilities.noString(value)) 1969 this.description = null; 1970 else { 1971 if (this.description == null) 1972 this.description = new StringType(); 1973 this.description.setValue(value); 1974 } 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #mode} (Indicates whether a resource instance represents a 1980 * specific location or a class of locations.). This is the underlying 1981 * object with id, value and extensions. The accessor "getMode" gives 1982 * direct access to the value 1983 */ 1984 public Enumeration<LocationMode> getModeElement() { 1985 if (this.mode == null) 1986 if (Configuration.errorOnAutoCreate()) 1987 throw new Error("Attempt to auto-create Location.mode"); 1988 else if (Configuration.doAutoCreate()) 1989 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); // bb 1990 return this.mode; 1991 } 1992 1993 public boolean hasModeElement() { 1994 return this.mode != null && !this.mode.isEmpty(); 1995 } 1996 1997 public boolean hasMode() { 1998 return this.mode != null && !this.mode.isEmpty(); 1999 } 2000 2001 /** 2002 * @param value {@link #mode} (Indicates whether a resource instance represents 2003 * a specific location or a class of locations.). This is the 2004 * underlying object with id, value and extensions. The accessor 2005 * "getMode" gives direct access to the value 2006 */ 2007 public Location setModeElement(Enumeration<LocationMode> value) { 2008 this.mode = value; 2009 return this; 2010 } 2011 2012 /** 2013 * @return Indicates whether a resource instance represents a specific location 2014 * or a class of locations. 2015 */ 2016 public LocationMode getMode() { 2017 return this.mode == null ? null : this.mode.getValue(); 2018 } 2019 2020 /** 2021 * @param value Indicates whether a resource instance represents a specific 2022 * location or a class of locations. 2023 */ 2024 public Location setMode(LocationMode value) { 2025 if (value == null) 2026 this.mode = null; 2027 else { 2028 if (this.mode == null) 2029 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); 2030 this.mode.setValue(value); 2031 } 2032 return this; 2033 } 2034 2035 /** 2036 * @return {@link #type} (Indicates the type of function performed at the 2037 * location.) 2038 */ 2039 public List<CodeableConcept> getType() { 2040 if (this.type == null) 2041 this.type = new ArrayList<CodeableConcept>(); 2042 return this.type; 2043 } 2044 2045 /** 2046 * @return Returns a reference to <code>this</code> for easy method chaining 2047 */ 2048 public Location setType(List<CodeableConcept> theType) { 2049 this.type = theType; 2050 return this; 2051 } 2052 2053 public boolean hasType() { 2054 if (this.type == null) 2055 return false; 2056 for (CodeableConcept item : this.type) 2057 if (!item.isEmpty()) 2058 return true; 2059 return false; 2060 } 2061 2062 public CodeableConcept addType() { // 3 2063 CodeableConcept t = new CodeableConcept(); 2064 if (this.type == null) 2065 this.type = new ArrayList<CodeableConcept>(); 2066 this.type.add(t); 2067 return t; 2068 } 2069 2070 public Location addType(CodeableConcept t) { // 3 2071 if (t == null) 2072 return this; 2073 if (this.type == null) 2074 this.type = new ArrayList<CodeableConcept>(); 2075 this.type.add(t); 2076 return this; 2077 } 2078 2079 /** 2080 * @return The first repetition of repeating field {@link #type}, creating it if 2081 * it does not already exist 2082 */ 2083 public CodeableConcept getTypeFirstRep() { 2084 if (getType().isEmpty()) { 2085 addType(); 2086 } 2087 return getType().get(0); 2088 } 2089 2090 /** 2091 * @return {@link #telecom} (The contact details of communication devices 2092 * available at the location. This can include phone numbers, fax 2093 * numbers, mobile numbers, email addresses and web sites.) 2094 */ 2095 public List<ContactPoint> getTelecom() { 2096 if (this.telecom == null) 2097 this.telecom = new ArrayList<ContactPoint>(); 2098 return this.telecom; 2099 } 2100 2101 /** 2102 * @return Returns a reference to <code>this</code> for easy method chaining 2103 */ 2104 public Location setTelecom(List<ContactPoint> theTelecom) { 2105 this.telecom = theTelecom; 2106 return this; 2107 } 2108 2109 public boolean hasTelecom() { 2110 if (this.telecom == null) 2111 return false; 2112 for (ContactPoint item : this.telecom) 2113 if (!item.isEmpty()) 2114 return true; 2115 return false; 2116 } 2117 2118 public ContactPoint addTelecom() { // 3 2119 ContactPoint t = new ContactPoint(); 2120 if (this.telecom == null) 2121 this.telecom = new ArrayList<ContactPoint>(); 2122 this.telecom.add(t); 2123 return t; 2124 } 2125 2126 public Location addTelecom(ContactPoint t) { // 3 2127 if (t == null) 2128 return this; 2129 if (this.telecom == null) 2130 this.telecom = new ArrayList<ContactPoint>(); 2131 this.telecom.add(t); 2132 return this; 2133 } 2134 2135 /** 2136 * @return The first repetition of repeating field {@link #telecom}, creating it 2137 * if it does not already exist 2138 */ 2139 public ContactPoint getTelecomFirstRep() { 2140 if (getTelecom().isEmpty()) { 2141 addTelecom(); 2142 } 2143 return getTelecom().get(0); 2144 } 2145 2146 /** 2147 * @return {@link #address} (Physical location.) 2148 */ 2149 public Address getAddress() { 2150 if (this.address == null) 2151 if (Configuration.errorOnAutoCreate()) 2152 throw new Error("Attempt to auto-create Location.address"); 2153 else if (Configuration.doAutoCreate()) 2154 this.address = new Address(); // cc 2155 return this.address; 2156 } 2157 2158 public boolean hasAddress() { 2159 return this.address != null && !this.address.isEmpty(); 2160 } 2161 2162 /** 2163 * @param value {@link #address} (Physical location.) 2164 */ 2165 public Location setAddress(Address value) { 2166 this.address = value; 2167 return this; 2168 } 2169 2170 /** 2171 * @return {@link #physicalType} (Physical form of the location, e.g. building, 2172 * room, vehicle, road.) 2173 */ 2174 public CodeableConcept getPhysicalType() { 2175 if (this.physicalType == null) 2176 if (Configuration.errorOnAutoCreate()) 2177 throw new Error("Attempt to auto-create Location.physicalType"); 2178 else if (Configuration.doAutoCreate()) 2179 this.physicalType = new CodeableConcept(); // cc 2180 return this.physicalType; 2181 } 2182 2183 public boolean hasPhysicalType() { 2184 return this.physicalType != null && !this.physicalType.isEmpty(); 2185 } 2186 2187 /** 2188 * @param value {@link #physicalType} (Physical form of the location, e.g. 2189 * building, room, vehicle, road.) 2190 */ 2191 public Location setPhysicalType(CodeableConcept value) { 2192 this.physicalType = value; 2193 return this; 2194 } 2195 2196 /** 2197 * @return {@link #position} (The absolute geographic location of the Location, 2198 * expressed using the WGS84 datum (This is the same co-ordinate system 2199 * used in KML).) 2200 */ 2201 public LocationPositionComponent getPosition() { 2202 if (this.position == null) 2203 if (Configuration.errorOnAutoCreate()) 2204 throw new Error("Attempt to auto-create Location.position"); 2205 else if (Configuration.doAutoCreate()) 2206 this.position = new LocationPositionComponent(); // cc 2207 return this.position; 2208 } 2209 2210 public boolean hasPosition() { 2211 return this.position != null && !this.position.isEmpty(); 2212 } 2213 2214 /** 2215 * @param value {@link #position} (The absolute geographic location of the 2216 * Location, expressed using the WGS84 datum (This is the same 2217 * co-ordinate system used in KML).) 2218 */ 2219 public Location setPosition(LocationPositionComponent value) { 2220 this.position = value; 2221 return this; 2222 } 2223 2224 /** 2225 * @return {@link #managingOrganization} (The organization responsible for the 2226 * provisioning and upkeep of the location.) 2227 */ 2228 public Reference getManagingOrganization() { 2229 if (this.managingOrganization == null) 2230 if (Configuration.errorOnAutoCreate()) 2231 throw new Error("Attempt to auto-create Location.managingOrganization"); 2232 else if (Configuration.doAutoCreate()) 2233 this.managingOrganization = new Reference(); // cc 2234 return this.managingOrganization; 2235 } 2236 2237 public boolean hasManagingOrganization() { 2238 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2239 } 2240 2241 /** 2242 * @param value {@link #managingOrganization} (The organization responsible for 2243 * the provisioning and upkeep of the location.) 2244 */ 2245 public Location setManagingOrganization(Reference value) { 2246 this.managingOrganization = value; 2247 return this; 2248 } 2249 2250 /** 2251 * @return {@link #managingOrganization} The actual object that is the target of 2252 * the reference. The reference library doesn't populate this, but you 2253 * can use it to hold the resource if you resolve it. (The organization 2254 * responsible for the provisioning and upkeep of the location.) 2255 */ 2256 public Organization getManagingOrganizationTarget() { 2257 if (this.managingOrganizationTarget == null) 2258 if (Configuration.errorOnAutoCreate()) 2259 throw new Error("Attempt to auto-create Location.managingOrganization"); 2260 else if (Configuration.doAutoCreate()) 2261 this.managingOrganizationTarget = new Organization(); // aa 2262 return this.managingOrganizationTarget; 2263 } 2264 2265 /** 2266 * @param value {@link #managingOrganization} The actual object that is the 2267 * target of the reference. The reference library doesn't use 2268 * these, but you can use it to hold the resource if you resolve 2269 * it. (The organization responsible for the provisioning and 2270 * upkeep of the location.) 2271 */ 2272 public Location setManagingOrganizationTarget(Organization value) { 2273 this.managingOrganizationTarget = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return {@link #partOf} (Another Location of which this Location is 2279 * physically a part of.) 2280 */ 2281 public Reference getPartOf() { 2282 if (this.partOf == null) 2283 if (Configuration.errorOnAutoCreate()) 2284 throw new Error("Attempt to auto-create Location.partOf"); 2285 else if (Configuration.doAutoCreate()) 2286 this.partOf = new Reference(); // cc 2287 return this.partOf; 2288 } 2289 2290 public boolean hasPartOf() { 2291 return this.partOf != null && !this.partOf.isEmpty(); 2292 } 2293 2294 /** 2295 * @param value {@link #partOf} (Another Location of which this Location is 2296 * physically a part of.) 2297 */ 2298 public Location setPartOf(Reference value) { 2299 this.partOf = value; 2300 return this; 2301 } 2302 2303 /** 2304 * @return {@link #partOf} The actual object that is the target of the 2305 * reference. The reference library doesn't populate this, but you can 2306 * use it to hold the resource if you resolve it. (Another Location of 2307 * which this Location is physically a part of.) 2308 */ 2309 public Location getPartOfTarget() { 2310 if (this.partOfTarget == null) 2311 if (Configuration.errorOnAutoCreate()) 2312 throw new Error("Attempt to auto-create Location.partOf"); 2313 else if (Configuration.doAutoCreate()) 2314 this.partOfTarget = new Location(); // aa 2315 return this.partOfTarget; 2316 } 2317 2318 /** 2319 * @param value {@link #partOf} The actual object that is the target of the 2320 * reference. The reference library doesn't use these, but you can 2321 * use it to hold the resource if you resolve it. (Another Location 2322 * of which this Location is physically a part of.) 2323 */ 2324 public Location setPartOfTarget(Location value) { 2325 this.partOfTarget = value; 2326 return this; 2327 } 2328 2329 /** 2330 * @return {@link #hoursOfOperation} (What days/times during a week is this 2331 * location usually open.) 2332 */ 2333 public List<LocationHoursOfOperationComponent> getHoursOfOperation() { 2334 if (this.hoursOfOperation == null) 2335 this.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2336 return this.hoursOfOperation; 2337 } 2338 2339 /** 2340 * @return Returns a reference to <code>this</code> for easy method chaining 2341 */ 2342 public Location setHoursOfOperation(List<LocationHoursOfOperationComponent> theHoursOfOperation) { 2343 this.hoursOfOperation = theHoursOfOperation; 2344 return this; 2345 } 2346 2347 public boolean hasHoursOfOperation() { 2348 if (this.hoursOfOperation == null) 2349 return false; 2350 for (LocationHoursOfOperationComponent item : this.hoursOfOperation) 2351 if (!item.isEmpty()) 2352 return true; 2353 return false; 2354 } 2355 2356 public LocationHoursOfOperationComponent addHoursOfOperation() { // 3 2357 LocationHoursOfOperationComponent t = new LocationHoursOfOperationComponent(); 2358 if (this.hoursOfOperation == null) 2359 this.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2360 this.hoursOfOperation.add(t); 2361 return t; 2362 } 2363 2364 public Location addHoursOfOperation(LocationHoursOfOperationComponent t) { // 3 2365 if (t == null) 2366 return this; 2367 if (this.hoursOfOperation == null) 2368 this.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2369 this.hoursOfOperation.add(t); 2370 return this; 2371 } 2372 2373 /** 2374 * @return The first repetition of repeating field {@link #hoursOfOperation}, 2375 * creating it if it does not already exist 2376 */ 2377 public LocationHoursOfOperationComponent getHoursOfOperationFirstRep() { 2378 if (getHoursOfOperation().isEmpty()) { 2379 addHoursOfOperation(); 2380 } 2381 return getHoursOfOperation().get(0); 2382 } 2383 2384 /** 2385 * @return {@link #availabilityExceptions} (A description of when the locations 2386 * opening ours are different to normal, e.g. public holiday 2387 * availability. Succinctly describing all possible exceptions to normal 2388 * site availability as detailed in the opening hours Times.). This is 2389 * the underlying object with id, value and extensions. The accessor 2390 * "getAvailabilityExceptions" gives direct access to the value 2391 */ 2392 public StringType getAvailabilityExceptionsElement() { 2393 if (this.availabilityExceptions == null) 2394 if (Configuration.errorOnAutoCreate()) 2395 throw new Error("Attempt to auto-create Location.availabilityExceptions"); 2396 else if (Configuration.doAutoCreate()) 2397 this.availabilityExceptions = new StringType(); // bb 2398 return this.availabilityExceptions; 2399 } 2400 2401 public boolean hasAvailabilityExceptionsElement() { 2402 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2403 } 2404 2405 public boolean hasAvailabilityExceptions() { 2406 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2407 } 2408 2409 /** 2410 * @param value {@link #availabilityExceptions} (A description of when the 2411 * locations opening ours are different to normal, e.g. public 2412 * holiday availability. Succinctly describing all possible 2413 * exceptions to normal site availability as detailed in the 2414 * opening hours Times.). This is the underlying object with id, 2415 * value and extensions. The accessor "getAvailabilityExceptions" 2416 * gives direct access to the value 2417 */ 2418 public Location setAvailabilityExceptionsElement(StringType value) { 2419 this.availabilityExceptions = value; 2420 return this; 2421 } 2422 2423 /** 2424 * @return A description of when the locations opening ours are different to 2425 * normal, e.g. public holiday availability. Succinctly describing all 2426 * possible exceptions to normal site availability as detailed in the 2427 * opening hours Times. 2428 */ 2429 public String getAvailabilityExceptions() { 2430 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 2431 } 2432 2433 /** 2434 * @param value A description of when the locations opening ours are different 2435 * to normal, e.g. public holiday availability. Succinctly 2436 * describing all possible exceptions to normal site availability 2437 * as detailed in the opening hours Times. 2438 */ 2439 public Location setAvailabilityExceptions(String value) { 2440 if (Utilities.noString(value)) 2441 this.availabilityExceptions = null; 2442 else { 2443 if (this.availabilityExceptions == null) 2444 this.availabilityExceptions = new StringType(); 2445 this.availabilityExceptions.setValue(value); 2446 } 2447 return this; 2448 } 2449 2450 /** 2451 * @return {@link #endpoint} (Technical endpoints providing access to services 2452 * operated for the location.) 2453 */ 2454 public List<Reference> getEndpoint() { 2455 if (this.endpoint == null) 2456 this.endpoint = new ArrayList<Reference>(); 2457 return this.endpoint; 2458 } 2459 2460 /** 2461 * @return Returns a reference to <code>this</code> for easy method chaining 2462 */ 2463 public Location setEndpoint(List<Reference> theEndpoint) { 2464 this.endpoint = theEndpoint; 2465 return this; 2466 } 2467 2468 public boolean hasEndpoint() { 2469 if (this.endpoint == null) 2470 return false; 2471 for (Reference item : this.endpoint) 2472 if (!item.isEmpty()) 2473 return true; 2474 return false; 2475 } 2476 2477 public Reference addEndpoint() { // 3 2478 Reference t = new Reference(); 2479 if (this.endpoint == null) 2480 this.endpoint = new ArrayList<Reference>(); 2481 this.endpoint.add(t); 2482 return t; 2483 } 2484 2485 public Location addEndpoint(Reference t) { // 3 2486 if (t == null) 2487 return this; 2488 if (this.endpoint == null) 2489 this.endpoint = new ArrayList<Reference>(); 2490 this.endpoint.add(t); 2491 return this; 2492 } 2493 2494 /** 2495 * @return The first repetition of repeating field {@link #endpoint}, creating 2496 * it if it does not already exist 2497 */ 2498 public Reference getEndpointFirstRep() { 2499 if (getEndpoint().isEmpty()) { 2500 addEndpoint(); 2501 } 2502 return getEndpoint().get(0); 2503 } 2504 2505 /** 2506 * @deprecated Use Reference#setResource(IBaseResource) instead 2507 */ 2508 @Deprecated 2509 public List<Endpoint> getEndpointTarget() { 2510 if (this.endpointTarget == null) 2511 this.endpointTarget = new ArrayList<Endpoint>(); 2512 return this.endpointTarget; 2513 } 2514 2515 /** 2516 * @deprecated Use Reference#setResource(IBaseResource) instead 2517 */ 2518 @Deprecated 2519 public Endpoint addEndpointTarget() { 2520 Endpoint r = new Endpoint(); 2521 if (this.endpointTarget == null) 2522 this.endpointTarget = new ArrayList<Endpoint>(); 2523 this.endpointTarget.add(r); 2524 return r; 2525 } 2526 2527 protected void listChildren(List<Property> children) { 2528 super.listChildren(children); 2529 children.add(new Property("identifier", "Identifier", 2530 "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2531 children.add(new Property("status", "code", 2532 "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 2533 0, 1, status)); 2534 children.add(new Property("operationalStatus", "Coding", 2535 "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.", 2536 0, 1, operationalStatus)); 2537 children.add(new Property("name", "string", "Name of the location as used by humans. Does not need to be unique.", 2538 0, 1, name)); 2539 children.add(new Property("alias", "string", 2540 "A list of alternate names that the location is known as, or was known as, in the past.", 0, 2541 java.lang.Integer.MAX_VALUE, alias)); 2542 children.add(new Property("description", "string", 2543 "Description of the Location, which helps in finding or referencing the place.", 0, 1, description)); 2544 children.add(new Property("mode", "code", 2545 "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode)); 2546 children.add(new Property("type", "CodeableConcept", "Indicates the type of function performed at the location.", 0, 2547 java.lang.Integer.MAX_VALUE, type)); 2548 children.add(new Property("telecom", "ContactPoint", 2549 "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 2550 0, java.lang.Integer.MAX_VALUE, telecom)); 2551 children.add(new Property("address", "Address", "Physical location.", 0, 1, address)); 2552 children.add(new Property("physicalType", "CodeableConcept", 2553 "Physical form of the location, e.g. building, room, vehicle, road.", 0, 1, physicalType)); 2554 children.add(new Property("position", "", 2555 "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 2556 0, 1, position)); 2557 children.add(new Property("managingOrganization", "Reference(Organization)", 2558 "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization)); 2559 children.add(new Property("partOf", "Reference(Location)", 2560 "Another Location of which this Location is physically a part of.", 0, 1, partOf)); 2561 children.add(new Property("hoursOfOperation", "", "What days/times during a week is this location usually open.", 0, 2562 java.lang.Integer.MAX_VALUE, hoursOfOperation)); 2563 children.add(new Property("availabilityExceptions", "string", 2564 "A description of when the locations opening ours are different to normal, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as detailed in the opening hours Times.", 2565 0, 1, availabilityExceptions)); 2566 children.add(new Property("endpoint", "Reference(Endpoint)", 2567 "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, 2568 endpoint)); 2569 } 2570 2571 @Override 2572 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2573 switch (_hash) { 2574 case -1618432855: 2575 /* identifier */ return new Property("identifier", "Identifier", 2576 "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier); 2577 case -892481550: 2578 /* status */ return new Property("status", "code", 2579 "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 2580 0, 1, status); 2581 case -2103166364: 2582 /* operationalStatus */ return new Property("operationalStatus", "Coding", 2583 "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.", 2584 0, 1, operationalStatus); 2585 case 3373707: 2586 /* name */ return new Property("name", "string", 2587 "Name of the location as used by humans. Does not need to be unique.", 0, 1, name); 2588 case 92902992: 2589 /* alias */ return new Property("alias", "string", 2590 "A list of alternate names that the location is known as, or was known as, in the past.", 0, 2591 java.lang.Integer.MAX_VALUE, alias); 2592 case -1724546052: 2593 /* description */ return new Property("description", "string", 2594 "Description of the Location, which helps in finding or referencing the place.", 0, 1, description); 2595 case 3357091: 2596 /* mode */ return new Property("mode", "code", 2597 "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode); 2598 case 3575610: 2599 /* type */ return new Property("type", "CodeableConcept", 2600 "Indicates the type of function performed at the location.", 0, java.lang.Integer.MAX_VALUE, type); 2601 case -1429363305: 2602 /* telecom */ return new Property("telecom", "ContactPoint", 2603 "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 2604 0, java.lang.Integer.MAX_VALUE, telecom); 2605 case -1147692044: 2606 /* address */ return new Property("address", "Address", "Physical location.", 0, 1, address); 2607 case -1474715471: 2608 /* physicalType */ return new Property("physicalType", "CodeableConcept", 2609 "Physical form of the location, e.g. building, room, vehicle, road.", 0, 1, physicalType); 2610 case 747804969: 2611 /* position */ return new Property("position", "", 2612 "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 2613 0, 1, position); 2614 case -2058947787: 2615 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 2616 "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization); 2617 case -995410646: 2618 /* partOf */ return new Property("partOf", "Reference(Location)", 2619 "Another Location of which this Location is physically a part of.", 0, 1, partOf); 2620 case -1588872511: 2621 /* hoursOfOperation */ return new Property("hoursOfOperation", "", 2622 "What days/times during a week is this location usually open.", 0, java.lang.Integer.MAX_VALUE, 2623 hoursOfOperation); 2624 case -1149143617: 2625 /* availabilityExceptions */ return new Property("availabilityExceptions", "string", 2626 "A description of when the locations opening ours are different to normal, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as detailed in the opening hours Times.", 2627 0, 1, availabilityExceptions); 2628 case 1741102485: 2629 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 2630 "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, 2631 endpoint); 2632 default: 2633 return super.getNamedProperty(_hash, _name, _checkValid); 2634 } 2635 2636 } 2637 2638 @Override 2639 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2640 switch (hash) { 2641 case -1618432855: 2642 /* identifier */ return this.identifier == null ? new Base[0] 2643 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2644 case -892481550: 2645 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<LocationStatus> 2646 case -2103166364: 2647 /* operationalStatus */ return this.operationalStatus == null ? new Base[0] 2648 : new Base[] { this.operationalStatus }; // Coding 2649 case 3373707: 2650 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2651 case 92902992: 2652 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 2653 case -1724546052: 2654 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2655 case 3357091: 2656 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<LocationMode> 2657 case 3575610: 2658 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2659 case -1429363305: 2660 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2661 case -1147692044: 2662 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 2663 case -1474715471: 2664 /* physicalType */ return this.physicalType == null ? new Base[0] : new Base[] { this.physicalType }; // CodeableConcept 2665 case 747804969: 2666 /* position */ return this.position == null ? new Base[0] : new Base[] { this.position }; // LocationPositionComponent 2667 case -2058947787: 2668 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 2669 : new Base[] { this.managingOrganization }; // Reference 2670 case -995410646: 2671 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 2672 case -1588872511: 2673 /* hoursOfOperation */ return this.hoursOfOperation == null ? new Base[0] 2674 : this.hoursOfOperation.toArray(new Base[this.hoursOfOperation.size()]); // LocationHoursOfOperationComponent 2675 case -1149143617: 2676 /* availabilityExceptions */ return this.availabilityExceptions == null ? new Base[0] 2677 : new Base[] { this.availabilityExceptions }; // StringType 2678 case 1741102485: 2679 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2680 default: 2681 return super.getProperty(hash, name, checkValid); 2682 } 2683 2684 } 2685 2686 @Override 2687 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2688 switch (hash) { 2689 case -1618432855: // identifier 2690 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2691 return value; 2692 case -892481550: // status 2693 value = new LocationStatusEnumFactory().fromType(castToCode(value)); 2694 this.status = (Enumeration) value; // Enumeration<LocationStatus> 2695 return value; 2696 case -2103166364: // operationalStatus 2697 this.operationalStatus = castToCoding(value); // Coding 2698 return value; 2699 case 3373707: // name 2700 this.name = castToString(value); // StringType 2701 return value; 2702 case 92902992: // alias 2703 this.getAlias().add(castToString(value)); // StringType 2704 return value; 2705 case -1724546052: // description 2706 this.description = castToString(value); // StringType 2707 return value; 2708 case 3357091: // mode 2709 value = new LocationModeEnumFactory().fromType(castToCode(value)); 2710 this.mode = (Enumeration) value; // Enumeration<LocationMode> 2711 return value; 2712 case 3575610: // type 2713 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2714 return value; 2715 case -1429363305: // telecom 2716 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2717 return value; 2718 case -1147692044: // address 2719 this.address = castToAddress(value); // Address 2720 return value; 2721 case -1474715471: // physicalType 2722 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2723 return value; 2724 case 747804969: // position 2725 this.position = (LocationPositionComponent) value; // LocationPositionComponent 2726 return value; 2727 case -2058947787: // managingOrganization 2728 this.managingOrganization = castToReference(value); // Reference 2729 return value; 2730 case -995410646: // partOf 2731 this.partOf = castToReference(value); // Reference 2732 return value; 2733 case -1588872511: // hoursOfOperation 2734 this.getHoursOfOperation().add((LocationHoursOfOperationComponent) value); // LocationHoursOfOperationComponent 2735 return value; 2736 case -1149143617: // availabilityExceptions 2737 this.availabilityExceptions = castToString(value); // StringType 2738 return value; 2739 case 1741102485: // endpoint 2740 this.getEndpoint().add(castToReference(value)); // Reference 2741 return value; 2742 default: 2743 return super.setProperty(hash, name, value); 2744 } 2745 2746 } 2747 2748 @Override 2749 public Base setProperty(String name, Base value) throws FHIRException { 2750 if (name.equals("identifier")) { 2751 this.getIdentifier().add(castToIdentifier(value)); 2752 } else if (name.equals("status")) { 2753 value = new LocationStatusEnumFactory().fromType(castToCode(value)); 2754 this.status = (Enumeration) value; // Enumeration<LocationStatus> 2755 } else if (name.equals("operationalStatus")) { 2756 this.operationalStatus = castToCoding(value); // Coding 2757 } else if (name.equals("name")) { 2758 this.name = castToString(value); // StringType 2759 } else if (name.equals("alias")) { 2760 this.getAlias().add(castToString(value)); 2761 } else if (name.equals("description")) { 2762 this.description = castToString(value); // StringType 2763 } else if (name.equals("mode")) { 2764 value = new LocationModeEnumFactory().fromType(castToCode(value)); 2765 this.mode = (Enumeration) value; // Enumeration<LocationMode> 2766 } else if (name.equals("type")) { 2767 this.getType().add(castToCodeableConcept(value)); 2768 } else if (name.equals("telecom")) { 2769 this.getTelecom().add(castToContactPoint(value)); 2770 } else if (name.equals("address")) { 2771 this.address = castToAddress(value); // Address 2772 } else if (name.equals("physicalType")) { 2773 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2774 } else if (name.equals("position")) { 2775 this.position = (LocationPositionComponent) value; // LocationPositionComponent 2776 } else if (name.equals("managingOrganization")) { 2777 this.managingOrganization = castToReference(value); // Reference 2778 } else if (name.equals("partOf")) { 2779 this.partOf = castToReference(value); // Reference 2780 } else if (name.equals("hoursOfOperation")) { 2781 this.getHoursOfOperation().add((LocationHoursOfOperationComponent) value); 2782 } else if (name.equals("availabilityExceptions")) { 2783 this.availabilityExceptions = castToString(value); // StringType 2784 } else if (name.equals("endpoint")) { 2785 this.getEndpoint().add(castToReference(value)); 2786 } else 2787 return super.setProperty(name, value); 2788 return value; 2789 } 2790 2791 @Override 2792 public void removeChild(String name, Base value) throws FHIRException { 2793 if (name.equals("identifier")) { 2794 this.getIdentifier().remove(castToIdentifier(value)); 2795 } else if (name.equals("status")) { 2796 this.status = null; 2797 } else if (name.equals("operationalStatus")) { 2798 this.operationalStatus = null; 2799 } else if (name.equals("name")) { 2800 this.name = null; 2801 } else if (name.equals("alias")) { 2802 this.getAlias().remove(castToString(value)); 2803 } else if (name.equals("description")) { 2804 this.description = null; 2805 } else if (name.equals("mode")) { 2806 this.mode = null; 2807 } else if (name.equals("type")) { 2808 this.getType().remove(castToCodeableConcept(value)); 2809 } else if (name.equals("telecom")) { 2810 this.getTelecom().remove(castToContactPoint(value)); 2811 } else if (name.equals("address")) { 2812 this.address = null; 2813 } else if (name.equals("physicalType")) { 2814 this.physicalType = null; 2815 } else if (name.equals("position")) { 2816 this.position = (LocationPositionComponent) value; // LocationPositionComponent 2817 } else if (name.equals("managingOrganization")) { 2818 this.managingOrganization = null; 2819 } else if (name.equals("partOf")) { 2820 this.partOf = null; 2821 } else if (name.equals("hoursOfOperation")) { 2822 this.getHoursOfOperation().remove((LocationHoursOfOperationComponent) value); 2823 } else if (name.equals("availabilityExceptions")) { 2824 this.availabilityExceptions = null; 2825 } else if (name.equals("endpoint")) { 2826 this.getEndpoint().remove(castToReference(value)); 2827 } else 2828 super.removeChild(name, value); 2829 2830 } 2831 2832 @Override 2833 public Base makeProperty(int hash, String name) throws FHIRException { 2834 switch (hash) { 2835 case -1618432855: 2836 return addIdentifier(); 2837 case -892481550: 2838 return getStatusElement(); 2839 case -2103166364: 2840 return getOperationalStatus(); 2841 case 3373707: 2842 return getNameElement(); 2843 case 92902992: 2844 return addAliasElement(); 2845 case -1724546052: 2846 return getDescriptionElement(); 2847 case 3357091: 2848 return getModeElement(); 2849 case 3575610: 2850 return addType(); 2851 case -1429363305: 2852 return addTelecom(); 2853 case -1147692044: 2854 return getAddress(); 2855 case -1474715471: 2856 return getPhysicalType(); 2857 case 747804969: 2858 return getPosition(); 2859 case -2058947787: 2860 return getManagingOrganization(); 2861 case -995410646: 2862 return getPartOf(); 2863 case -1588872511: 2864 return addHoursOfOperation(); 2865 case -1149143617: 2866 return getAvailabilityExceptionsElement(); 2867 case 1741102485: 2868 return addEndpoint(); 2869 default: 2870 return super.makeProperty(hash, name); 2871 } 2872 2873 } 2874 2875 @Override 2876 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2877 switch (hash) { 2878 case -1618432855: 2879 /* identifier */ return new String[] { "Identifier" }; 2880 case -892481550: 2881 /* status */ return new String[] { "code" }; 2882 case -2103166364: 2883 /* operationalStatus */ return new String[] { "Coding" }; 2884 case 3373707: 2885 /* name */ return new String[] { "string" }; 2886 case 92902992: 2887 /* alias */ return new String[] { "string" }; 2888 case -1724546052: 2889 /* description */ return new String[] { "string" }; 2890 case 3357091: 2891 /* mode */ return new String[] { "code" }; 2892 case 3575610: 2893 /* type */ return new String[] { "CodeableConcept" }; 2894 case -1429363305: 2895 /* telecom */ return new String[] { "ContactPoint" }; 2896 case -1147692044: 2897 /* address */ return new String[] { "Address" }; 2898 case -1474715471: 2899 /* physicalType */ return new String[] { "CodeableConcept" }; 2900 case 747804969: 2901 /* position */ return new String[] {}; 2902 case -2058947787: 2903 /* managingOrganization */ return new String[] { "Reference" }; 2904 case -995410646: 2905 /* partOf */ return new String[] { "Reference" }; 2906 case -1588872511: 2907 /* hoursOfOperation */ return new String[] {}; 2908 case -1149143617: 2909 /* availabilityExceptions */ return new String[] { "string" }; 2910 case 1741102485: 2911 /* endpoint */ return new String[] { "Reference" }; 2912 default: 2913 return super.getTypesForProperty(hash, name); 2914 } 2915 2916 } 2917 2918 @Override 2919 public Base addChild(String name) throws FHIRException { 2920 if (name.equals("identifier")) { 2921 return addIdentifier(); 2922 } else if (name.equals("status")) { 2923 throw new FHIRException("Cannot call addChild on a singleton property Location.status"); 2924 } else if (name.equals("operationalStatus")) { 2925 this.operationalStatus = new Coding(); 2926 return this.operationalStatus; 2927 } else if (name.equals("name")) { 2928 throw new FHIRException("Cannot call addChild on a singleton property Location.name"); 2929 } else if (name.equals("alias")) { 2930 throw new FHIRException("Cannot call addChild on a singleton property Location.alias"); 2931 } else if (name.equals("description")) { 2932 throw new FHIRException("Cannot call addChild on a singleton property Location.description"); 2933 } else if (name.equals("mode")) { 2934 throw new FHIRException("Cannot call addChild on a singleton property Location.mode"); 2935 } else if (name.equals("type")) { 2936 return addType(); 2937 } else if (name.equals("telecom")) { 2938 return addTelecom(); 2939 } else if (name.equals("address")) { 2940 this.address = new Address(); 2941 return this.address; 2942 } else if (name.equals("physicalType")) { 2943 this.physicalType = new CodeableConcept(); 2944 return this.physicalType; 2945 } else if (name.equals("position")) { 2946 this.position = new LocationPositionComponent(); 2947 return this.position; 2948 } else if (name.equals("managingOrganization")) { 2949 this.managingOrganization = new Reference(); 2950 return this.managingOrganization; 2951 } else if (name.equals("partOf")) { 2952 this.partOf = new Reference(); 2953 return this.partOf; 2954 } else if (name.equals("hoursOfOperation")) { 2955 return addHoursOfOperation(); 2956 } else if (name.equals("availabilityExceptions")) { 2957 throw new FHIRException("Cannot call addChild on a singleton property Location.availabilityExceptions"); 2958 } else if (name.equals("endpoint")) { 2959 return addEndpoint(); 2960 } else 2961 return super.addChild(name); 2962 } 2963 2964 public String fhirType() { 2965 return "Location"; 2966 2967 } 2968 2969 public Location copy() { 2970 Location dst = new Location(); 2971 copyValues(dst); 2972 return dst; 2973 } 2974 2975 public void copyValues(Location dst) { 2976 super.copyValues(dst); 2977 if (identifier != null) { 2978 dst.identifier = new ArrayList<Identifier>(); 2979 for (Identifier i : identifier) 2980 dst.identifier.add(i.copy()); 2981 } 2982 ; 2983 dst.status = status == null ? null : status.copy(); 2984 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 2985 dst.name = name == null ? null : name.copy(); 2986 if (alias != null) { 2987 dst.alias = new ArrayList<StringType>(); 2988 for (StringType i : alias) 2989 dst.alias.add(i.copy()); 2990 } 2991 ; 2992 dst.description = description == null ? null : description.copy(); 2993 dst.mode = mode == null ? null : mode.copy(); 2994 if (type != null) { 2995 dst.type = new ArrayList<CodeableConcept>(); 2996 for (CodeableConcept i : type) 2997 dst.type.add(i.copy()); 2998 } 2999 ; 3000 if (telecom != null) { 3001 dst.telecom = new ArrayList<ContactPoint>(); 3002 for (ContactPoint i : telecom) 3003 dst.telecom.add(i.copy()); 3004 } 3005 ; 3006 dst.address = address == null ? null : address.copy(); 3007 dst.physicalType = physicalType == null ? null : physicalType.copy(); 3008 dst.position = position == null ? null : position.copy(); 3009 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 3010 dst.partOf = partOf == null ? null : partOf.copy(); 3011 if (hoursOfOperation != null) { 3012 dst.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 3013 for (LocationHoursOfOperationComponent i : hoursOfOperation) 3014 dst.hoursOfOperation.add(i.copy()); 3015 } 3016 ; 3017 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 3018 if (endpoint != null) { 3019 dst.endpoint = new ArrayList<Reference>(); 3020 for (Reference i : endpoint) 3021 dst.endpoint.add(i.copy()); 3022 } 3023 ; 3024 } 3025 3026 protected Location typedCopy() { 3027 return copy(); 3028 } 3029 3030 @Override 3031 public boolean equalsDeep(Base other_) { 3032 if (!super.equalsDeep(other_)) 3033 return false; 3034 if (!(other_ instanceof Location)) 3035 return false; 3036 Location o = (Location) other_; 3037 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 3038 && compareDeep(operationalStatus, o.operationalStatus, true) && compareDeep(name, o.name, true) 3039 && compareDeep(alias, o.alias, true) && compareDeep(description, o.description, true) 3040 && compareDeep(mode, o.mode, true) && compareDeep(type, o.type, true) && compareDeep(telecom, o.telecom, true) 3041 && compareDeep(address, o.address, true) && compareDeep(physicalType, o.physicalType, true) 3042 && compareDeep(position, o.position, true) && compareDeep(managingOrganization, o.managingOrganization, true) 3043 && compareDeep(partOf, o.partOf, true) && compareDeep(hoursOfOperation, o.hoursOfOperation, true) 3044 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) 3045 && compareDeep(endpoint, o.endpoint, true); 3046 } 3047 3048 @Override 3049 public boolean equalsShallow(Base other_) { 3050 if (!super.equalsShallow(other_)) 3051 return false; 3052 if (!(other_ instanceof Location)) 3053 return false; 3054 Location o = (Location) other_; 3055 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 3056 && compareValues(alias, o.alias, true) && compareValues(description, o.description, true) 3057 && compareValues(mode, o.mode, true) && compareValues(availabilityExceptions, o.availabilityExceptions, true); 3058 } 3059 3060 public boolean isEmpty() { 3061 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, operationalStatus, name, alias, 3062 description, mode, type, telecom, address, physicalType, position, managingOrganization, partOf, 3063 hoursOfOperation, availabilityExceptions, endpoint); 3064 } 3065 3066 @Override 3067 public ResourceType getResourceType() { 3068 return ResourceType.Location; 3069 } 3070 3071 /** 3072 * Search parameter: <b>identifier</b> 3073 * <p> 3074 * Description: <b>An identifier for the location</b><br> 3075 * Type: <b>token</b><br> 3076 * Path: <b>Location.identifier</b><br> 3077 * </p> 3078 */ 3079 @SearchParamDefinition(name = "identifier", path = "Location.identifier", description = "An identifier for the location", type = "token") 3080 public static final String SP_IDENTIFIER = "identifier"; 3081 /** 3082 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3083 * <p> 3084 * Description: <b>An identifier for the location</b><br> 3085 * Type: <b>token</b><br> 3086 * Path: <b>Location.identifier</b><br> 3087 * </p> 3088 */ 3089 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3090 SP_IDENTIFIER); 3091 3092 /** 3093 * Search parameter: <b>partof</b> 3094 * <p> 3095 * Description: <b>A location of which this location is a part</b><br> 3096 * Type: <b>reference</b><br> 3097 * Path: <b>Location.partOf</b><br> 3098 * </p> 3099 */ 3100 @SearchParamDefinition(name = "partof", path = "Location.partOf", description = "A location of which this location is a part", type = "reference", target = { 3101 Location.class }) 3102 public static final String SP_PARTOF = "partof"; 3103 /** 3104 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 3105 * <p> 3106 * Description: <b>A location of which this location is a part</b><br> 3107 * Type: <b>reference</b><br> 3108 * Path: <b>Location.partOf</b><br> 3109 * </p> 3110 */ 3111 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3112 SP_PARTOF); 3113 3114 /** 3115 * Constant for fluent queries to be used to add include statements. Specifies 3116 * the path value of "<b>Location:partof</b>". 3117 */ 3118 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include( 3119 "Location:partof").toLocked(); 3120 3121 /** 3122 * Search parameter: <b>address</b> 3123 * <p> 3124 * Description: <b>A (part of the) address of the location</b><br> 3125 * Type: <b>string</b><br> 3126 * Path: <b>Location.address</b><br> 3127 * </p> 3128 */ 3129 @SearchParamDefinition(name = "address", path = "Location.address", description = "A (part of the) address of the location", type = "string") 3130 public static final String SP_ADDRESS = "address"; 3131 /** 3132 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3133 * <p> 3134 * Description: <b>A (part of the) address of the location</b><br> 3135 * Type: <b>string</b><br> 3136 * Path: <b>Location.address</b><br> 3137 * </p> 3138 */ 3139 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 3140 SP_ADDRESS); 3141 3142 /** 3143 * Search parameter: <b>address-state</b> 3144 * <p> 3145 * Description: <b>A state specified in an address</b><br> 3146 * Type: <b>string</b><br> 3147 * Path: <b>Location.address.state</b><br> 3148 * </p> 3149 */ 3150 @SearchParamDefinition(name = "address-state", path = "Location.address.state", description = "A state specified in an address", type = "string") 3151 public static final String SP_ADDRESS_STATE = "address-state"; 3152 /** 3153 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 3154 * <p> 3155 * Description: <b>A state specified in an address</b><br> 3156 * Type: <b>string</b><br> 3157 * Path: <b>Location.address.state</b><br> 3158 * </p> 3159 */ 3160 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3161 SP_ADDRESS_STATE); 3162 3163 /** 3164 * Search parameter: <b>operational-status</b> 3165 * <p> 3166 * Description: <b>Searches for locations (typically bed/room) that have an 3167 * operational status (e.g. contaminated, housekeeping)</b><br> 3168 * Type: <b>token</b><br> 3169 * Path: <b>Location.operationalStatus</b><br> 3170 * </p> 3171 */ 3172 @SearchParamDefinition(name = "operational-status", path = "Location.operationalStatus", description = "Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)", type = "token") 3173 public static final String SP_OPERATIONAL_STATUS = "operational-status"; 3174 /** 3175 * <b>Fluent Client</b> search parameter constant for <b>operational-status</b> 3176 * <p> 3177 * Description: <b>Searches for locations (typically bed/room) that have an 3178 * operational status (e.g. contaminated, housekeeping)</b><br> 3179 * Type: <b>token</b><br> 3180 * Path: <b>Location.operationalStatus</b><br> 3181 * </p> 3182 */ 3183 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OPERATIONAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3184 SP_OPERATIONAL_STATUS); 3185 3186 /** 3187 * Search parameter: <b>type</b> 3188 * <p> 3189 * Description: <b>A code for the type of location</b><br> 3190 * Type: <b>token</b><br> 3191 * Path: <b>Location.type</b><br> 3192 * </p> 3193 */ 3194 @SearchParamDefinition(name = "type", path = "Location.type", description = "A code for the type of location", type = "token") 3195 public static final String SP_TYPE = "type"; 3196 /** 3197 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3198 * <p> 3199 * Description: <b>A code for the type of location</b><br> 3200 * Type: <b>token</b><br> 3201 * Path: <b>Location.type</b><br> 3202 * </p> 3203 */ 3204 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3205 SP_TYPE); 3206 3207 /** 3208 * Search parameter: <b>address-postalcode</b> 3209 * <p> 3210 * Description: <b>A postal code specified in an address</b><br> 3211 * Type: <b>string</b><br> 3212 * Path: <b>Location.address.postalCode</b><br> 3213 * </p> 3214 */ 3215 @SearchParamDefinition(name = "address-postalcode", path = "Location.address.postalCode", description = "A postal code specified in an address", type = "string") 3216 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3217 /** 3218 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3219 * <p> 3220 * Description: <b>A postal code specified in an address</b><br> 3221 * Type: <b>string</b><br> 3222 * Path: <b>Location.address.postalCode</b><br> 3223 * </p> 3224 */ 3225 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3226 SP_ADDRESS_POSTALCODE); 3227 3228 /** 3229 * Search parameter: <b>address-country</b> 3230 * <p> 3231 * Description: <b>A country specified in an address</b><br> 3232 * Type: <b>string</b><br> 3233 * Path: <b>Location.address.country</b><br> 3234 * </p> 3235 */ 3236 @SearchParamDefinition(name = "address-country", path = "Location.address.country", description = "A country specified in an address", type = "string") 3237 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3238 /** 3239 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3240 * <p> 3241 * Description: <b>A country specified in an address</b><br> 3242 * Type: <b>string</b><br> 3243 * Path: <b>Location.address.country</b><br> 3244 * </p> 3245 */ 3246 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3247 SP_ADDRESS_COUNTRY); 3248 3249 /** 3250 * Search parameter: <b>endpoint</b> 3251 * <p> 3252 * Description: <b>Technical endpoints providing access to services operated for 3253 * the location</b><br> 3254 * Type: <b>reference</b><br> 3255 * Path: <b>Location.endpoint</b><br> 3256 * </p> 3257 */ 3258 @SearchParamDefinition(name = "endpoint", path = "Location.endpoint", description = "Technical endpoints providing access to services operated for the location", type = "reference", target = { 3259 Endpoint.class }) 3260 public static final String SP_ENDPOINT = "endpoint"; 3261 /** 3262 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 3263 * <p> 3264 * Description: <b>Technical endpoints providing access to services operated for 3265 * the location</b><br> 3266 * Type: <b>reference</b><br> 3267 * Path: <b>Location.endpoint</b><br> 3268 * </p> 3269 */ 3270 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3271 SP_ENDPOINT); 3272 3273 /** 3274 * Constant for fluent queries to be used to add include statements. Specifies 3275 * the path value of "<b>Location:endpoint</b>". 3276 */ 3277 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 3278 "Location:endpoint").toLocked(); 3279 3280 /** 3281 * Search parameter: <b>organization</b> 3282 * <p> 3283 * Description: <b>Searches for locations that are managed by the provided 3284 * organization</b><br> 3285 * Type: <b>reference</b><br> 3286 * Path: <b>Location.managingOrganization</b><br> 3287 * </p> 3288 */ 3289 @SearchParamDefinition(name = "organization", path = "Location.managingOrganization", description = "Searches for locations that are managed by the provided organization", type = "reference", target = { 3290 Organization.class }) 3291 public static final String SP_ORGANIZATION = "organization"; 3292 /** 3293 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3294 * <p> 3295 * Description: <b>Searches for locations that are managed by the provided 3296 * organization</b><br> 3297 * Type: <b>reference</b><br> 3298 * Path: <b>Location.managingOrganization</b><br> 3299 * </p> 3300 */ 3301 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3302 SP_ORGANIZATION); 3303 3304 /** 3305 * Constant for fluent queries to be used to add include statements. Specifies 3306 * the path value of "<b>Location:organization</b>". 3307 */ 3308 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3309 "Location:organization").toLocked(); 3310 3311 /** 3312 * Search parameter: <b>name</b> 3313 * <p> 3314 * Description: <b>A portion of the location's name or alias</b><br> 3315 * Type: <b>string</b><br> 3316 * Path: <b>Location.name, Location.alias</b><br> 3317 * </p> 3318 */ 3319 @SearchParamDefinition(name = "name", path = "Location.name | Location.alias", description = "A portion of the location's name or alias", type = "string") 3320 public static final String SP_NAME = "name"; 3321 /** 3322 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3323 * <p> 3324 * Description: <b>A portion of the location's name or alias</b><br> 3325 * Type: <b>string</b><br> 3326 * Path: <b>Location.name, Location.alias</b><br> 3327 * </p> 3328 */ 3329 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3330 SP_NAME); 3331 3332 /** 3333 * Search parameter: <b>address-use</b> 3334 * <p> 3335 * Description: <b>A use code specified in an address</b><br> 3336 * Type: <b>token</b><br> 3337 * Path: <b>Location.address.use</b><br> 3338 * </p> 3339 */ 3340 @SearchParamDefinition(name = "address-use", path = "Location.address.use", description = "A use code specified in an address", type = "token") 3341 public static final String SP_ADDRESS_USE = "address-use"; 3342 /** 3343 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3344 * <p> 3345 * Description: <b>A use code specified in an address</b><br> 3346 * Type: <b>token</b><br> 3347 * Path: <b>Location.address.use</b><br> 3348 * </p> 3349 */ 3350 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3351 SP_ADDRESS_USE); 3352 3353 /** 3354 * Search parameter: <b>near</b> 3355 * <p> 3356 * Description: <b>Search for locations where the location.position is near to, 3357 * or within a specified distance of, the provided coordinates expressed as 3358 * [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes). 3359 * If the units are omitted, then kms should be assumed. If the distance is 3360 * omitted, then the server can use its own discretion as to what distances 3361 * should be considered near (and units are irrelevant) 3362 * 3363 * Servers may search using various techniques that might have differing 3364 * accuracies, depending on implementation efficiency. 3365 * 3366 * Requires the near-distance parameter to be provided also</b><br> 3367 * Type: <b>special</b><br> 3368 * Path: <b>Location.position</b><br> 3369 * </p> 3370 */ 3371 @SearchParamDefinition(name = "near", path = "Location.position", description = "Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes).\nIf the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant)\n\nServers may search using various techniques that might have differing accuracies, depending on implementation efficiency.\n\nRequires the near-distance parameter to be provided also", type = "special") 3372 public static final String SP_NEAR = "near"; 3373 /** 3374 * <b>Fluent Client</b> search parameter constant for <b>near</b> 3375 * <p> 3376 * Description: <b>Search for locations where the location.position is near to, 3377 * or within a specified distance of, the provided coordinates expressed as 3378 * [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes). 3379 * If the units are omitted, then kms should be assumed. If the distance is 3380 * omitted, then the server can use its own discretion as to what distances 3381 * should be considered near (and units are irrelevant) 3382 * 3383 * Servers may search using various techniques that might have differing 3384 * accuracies, depending on implementation efficiency. 3385 * 3386 * Requires the near-distance parameter to be provided also</b><br> 3387 * Type: <b>special</b><br> 3388 * Path: <b>Location.position</b><br> 3389 * </p> 3390 */ 3391 public static final ca.uhn.fhir.rest.gclient.SpecialClientParam NEAR = new ca.uhn.fhir.rest.gclient.SpecialClientParam( 3392 SP_NEAR); 3393 3394 /** 3395 * Search parameter: <b>address-city</b> 3396 * <p> 3397 * Description: <b>A city specified in an address</b><br> 3398 * Type: <b>string</b><br> 3399 * Path: <b>Location.address.city</b><br> 3400 * </p> 3401 */ 3402 @SearchParamDefinition(name = "address-city", path = "Location.address.city", description = "A city specified in an address", type = "string") 3403 public static final String SP_ADDRESS_CITY = "address-city"; 3404 /** 3405 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3406 * <p> 3407 * Description: <b>A city specified in an address</b><br> 3408 * Type: <b>string</b><br> 3409 * Path: <b>Location.address.city</b><br> 3410 * </p> 3411 */ 3412 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3413 SP_ADDRESS_CITY); 3414 3415 /** 3416 * Search parameter: <b>status</b> 3417 * <p> 3418 * Description: <b>Searches for locations with a specific kind of status</b><br> 3419 * Type: <b>token</b><br> 3420 * Path: <b>Location.status</b><br> 3421 * </p> 3422 */ 3423 @SearchParamDefinition(name = "status", path = "Location.status", description = "Searches for locations with a specific kind of status", type = "token") 3424 public static final String SP_STATUS = "status"; 3425 /** 3426 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3427 * <p> 3428 * Description: <b>Searches for locations with a specific kind of status</b><br> 3429 * Type: <b>token</b><br> 3430 * Path: <b>Location.status</b><br> 3431 * </p> 3432 */ 3433 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3434 SP_STATUS); 3435 3436}