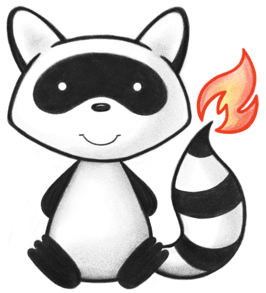
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.Date; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * The marketing status describes the date when a medicinal product is actually 045 * put on the market or the date as of which it is no longer available. 046 */ 047@DatatypeDef(name = "MarketingStatus") 048public class MarketingStatus extends BackboneType implements ICompositeType { 049 050 /** 051 * The country in which the marketing authorisation has been granted shall be 052 * specified It should be specified using the ISO 3166 ? 1 alpha-2 code 053 * elements. 054 */ 055 @Child(name = "country", type = { 056 CodeableConcept.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 057 @Description(shortDefinition = "The country in which the marketing authorisation has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements", formalDefinition = "The country in which the marketing authorisation has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.") 058 protected CodeableConcept country; 059 060 /** 061 * Where a Medicines Regulatory Agency has granted a marketing authorisation for 062 * which specific provisions within a jurisdiction apply, the jurisdiction can 063 * be specified using an appropriate controlled terminology The controlled term 064 * and the controlled term identifier shall be specified. 065 */ 066 @Child(name = "jurisdiction", type = { 067 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Where a Medicines Regulatory Agency has granted a marketing authorisation for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified", formalDefinition = "Where a Medicines Regulatory Agency has granted a marketing authorisation for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.") 069 protected CodeableConcept jurisdiction; 070 071 /** 072 * This attribute provides information on the status of the marketing of the 073 * medicinal product See ISO/TS 20443 for more information and examples. 074 */ 075 @Child(name = "status", type = { 076 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 077 @Description(shortDefinition = "This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples", formalDefinition = "This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.") 078 protected CodeableConcept status; 079 080 /** 081 * The date when the Medicinal Product is placed on the market by the Marketing 082 * Authorisation Holder (or where applicable, the manufacturer/distributor) in a 083 * country and/or jurisdiction shall be provided A complete date consisting of 084 * day, month and year shall be specified using the ISO 8601 date format NOTE 085 * ?Placed on the market? refers to the release of the Medicinal Product into 086 * the distribution chain. 087 */ 088 @Child(name = "dateRange", type = { Period.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 089 @Description(shortDefinition = "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain", formalDefinition = "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.") 090 protected Period dateRange; 091 092 /** 093 * The date when the Medicinal Product is placed on the market by the Marketing 094 * Authorisation Holder (or where applicable, the manufacturer/distributor) in a 095 * country and/or jurisdiction shall be provided A complete date consisting of 096 * day, month and year shall be specified using the ISO 8601 date format NOTE 097 * ?Placed on the market? refers to the release of the Medicinal Product into 098 * the distribution chain. 099 */ 100 @Child(name = "restoreDate", type = { 101 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 102 @Description(shortDefinition = "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain", formalDefinition = "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.") 103 protected DateTimeType restoreDate; 104 105 private static final long serialVersionUID = -1445736863L; 106 107 /** 108 * Constructor 109 */ 110 public MarketingStatus() { 111 super(); 112 } 113 114 /** 115 * Constructor 116 */ 117 public MarketingStatus(CodeableConcept country, CodeableConcept status, Period dateRange) { 118 super(); 119 this.country = country; 120 this.status = status; 121 this.dateRange = dateRange; 122 } 123 124 /** 125 * @return {@link #country} (The country in which the marketing authorisation 126 * has been granted shall be specified It should be specified using the 127 * ISO 3166 ? 1 alpha-2 code elements.) 128 */ 129 public CodeableConcept getCountry() { 130 if (this.country == null) 131 if (Configuration.errorOnAutoCreate()) 132 throw new Error("Attempt to auto-create MarketingStatus.country"); 133 else if (Configuration.doAutoCreate()) 134 this.country = new CodeableConcept(); // cc 135 return this.country; 136 } 137 138 public boolean hasCountry() { 139 return this.country != null && !this.country.isEmpty(); 140 } 141 142 /** 143 * @param value {@link #country} (The country in which the marketing 144 * authorisation has been granted shall be specified It should be 145 * specified using the ISO 3166 ? 1 alpha-2 code elements.) 146 */ 147 public MarketingStatus setCountry(CodeableConcept value) { 148 this.country = value; 149 return this; 150 } 151 152 /** 153 * @return {@link #jurisdiction} (Where a Medicines Regulatory Agency has 154 * granted a marketing authorisation for which specific provisions 155 * within a jurisdiction apply, the jurisdiction can be specified using 156 * an appropriate controlled terminology The controlled term and the 157 * controlled term identifier shall be specified.) 158 */ 159 public CodeableConcept getJurisdiction() { 160 if (this.jurisdiction == null) 161 if (Configuration.errorOnAutoCreate()) 162 throw new Error("Attempt to auto-create MarketingStatus.jurisdiction"); 163 else if (Configuration.doAutoCreate()) 164 this.jurisdiction = new CodeableConcept(); // cc 165 return this.jurisdiction; 166 } 167 168 public boolean hasJurisdiction() { 169 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 170 } 171 172 /** 173 * @param value {@link #jurisdiction} (Where a Medicines Regulatory Agency has 174 * granted a marketing authorisation for which specific provisions 175 * within a jurisdiction apply, the jurisdiction can be specified 176 * using an appropriate controlled terminology The controlled term 177 * and the controlled term identifier shall be specified.) 178 */ 179 public MarketingStatus setJurisdiction(CodeableConcept value) { 180 this.jurisdiction = value; 181 return this; 182 } 183 184 /** 185 * @return {@link #status} (This attribute provides information on the status of 186 * the marketing of the medicinal product See ISO/TS 20443 for more 187 * information and examples.) 188 */ 189 public CodeableConcept getStatus() { 190 if (this.status == null) 191 if (Configuration.errorOnAutoCreate()) 192 throw new Error("Attempt to auto-create MarketingStatus.status"); 193 else if (Configuration.doAutoCreate()) 194 this.status = new CodeableConcept(); // cc 195 return this.status; 196 } 197 198 public boolean hasStatus() { 199 return this.status != null && !this.status.isEmpty(); 200 } 201 202 /** 203 * @param value {@link #status} (This attribute provides information on the 204 * status of the marketing of the medicinal product See ISO/TS 205 * 20443 for more information and examples.) 206 */ 207 public MarketingStatus setStatus(CodeableConcept value) { 208 this.status = value; 209 return this; 210 } 211 212 /** 213 * @return {@link #dateRange} (The date when the Medicinal Product is placed on 214 * the market by the Marketing Authorisation Holder (or where 215 * applicable, the manufacturer/distributor) in a country and/or 216 * jurisdiction shall be provided A complete date consisting of day, 217 * month and year shall be specified using the ISO 8601 date format NOTE 218 * ?Placed on the market? refers to the release of the Medicinal Product 219 * into the distribution chain.) 220 */ 221 public Period getDateRange() { 222 if (this.dateRange == null) 223 if (Configuration.errorOnAutoCreate()) 224 throw new Error("Attempt to auto-create MarketingStatus.dateRange"); 225 else if (Configuration.doAutoCreate()) 226 this.dateRange = new Period(); // cc 227 return this.dateRange; 228 } 229 230 public boolean hasDateRange() { 231 return this.dateRange != null && !this.dateRange.isEmpty(); 232 } 233 234 /** 235 * @param value {@link #dateRange} (The date when the Medicinal Product is 236 * placed on the market by the Marketing Authorisation Holder (or 237 * where applicable, the manufacturer/distributor) in a country 238 * and/or jurisdiction shall be provided A complete date consisting 239 * of day, month and year shall be specified using the ISO 8601 240 * date format NOTE ?Placed on the market? refers to the release of 241 * the Medicinal Product into the distribution chain.) 242 */ 243 public MarketingStatus setDateRange(Period value) { 244 this.dateRange = value; 245 return this; 246 } 247 248 /** 249 * @return {@link #restoreDate} (The date when the Medicinal Product is placed 250 * on the market by the Marketing Authorisation Holder (or where 251 * applicable, the manufacturer/distributor) in a country and/or 252 * jurisdiction shall be provided A complete date consisting of day, 253 * month and year shall be specified using the ISO 8601 date format NOTE 254 * ?Placed on the market? refers to the release of the Medicinal Product 255 * into the distribution chain.). This is the underlying object with id, 256 * value and extensions. The accessor "getRestoreDate" gives direct 257 * access to the value 258 */ 259 public DateTimeType getRestoreDateElement() { 260 if (this.restoreDate == null) 261 if (Configuration.errorOnAutoCreate()) 262 throw new Error("Attempt to auto-create MarketingStatus.restoreDate"); 263 else if (Configuration.doAutoCreate()) 264 this.restoreDate = new DateTimeType(); // bb 265 return this.restoreDate; 266 } 267 268 public boolean hasRestoreDateElement() { 269 return this.restoreDate != null && !this.restoreDate.isEmpty(); 270 } 271 272 public boolean hasRestoreDate() { 273 return this.restoreDate != null && !this.restoreDate.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #restoreDate} (The date when the Medicinal Product is 278 * placed on the market by the Marketing Authorisation Holder (or 279 * where applicable, the manufacturer/distributor) in a country 280 * and/or jurisdiction shall be provided A complete date consisting 281 * of day, month and year shall be specified using the ISO 8601 282 * date format NOTE ?Placed on the market? refers to the release of 283 * the Medicinal Product into the distribution chain.). This is the 284 * underlying object with id, value and extensions. The accessor 285 * "getRestoreDate" gives direct access to the value 286 */ 287 public MarketingStatus setRestoreDateElement(DateTimeType value) { 288 this.restoreDate = value; 289 return this; 290 } 291 292 /** 293 * @return The date when the Medicinal Product is placed on the market by the 294 * Marketing Authorisation Holder (or where applicable, the 295 * manufacturer/distributor) in a country and/or jurisdiction shall be 296 * provided A complete date consisting of day, month and year shall be 297 * specified using the ISO 8601 date format NOTE ?Placed on the market? 298 * refers to the release of the Medicinal Product into the distribution 299 * chain. 300 */ 301 public Date getRestoreDate() { 302 return this.restoreDate == null ? null : this.restoreDate.getValue(); 303 } 304 305 /** 306 * @param value The date when the Medicinal Product is placed on the market by 307 * the Marketing Authorisation Holder (or where applicable, the 308 * manufacturer/distributor) in a country and/or jurisdiction shall 309 * be provided A complete date consisting of day, month and year 310 * shall be specified using the ISO 8601 date format NOTE ?Placed 311 * on the market? refers to the release of the Medicinal Product 312 * into the distribution chain. 313 */ 314 public MarketingStatus setRestoreDate(Date value) { 315 if (value == null) 316 this.restoreDate = null; 317 else { 318 if (this.restoreDate == null) 319 this.restoreDate = new DateTimeType(); 320 this.restoreDate.setValue(value); 321 } 322 return this; 323 } 324 325 protected void listChildren(List<Property> children) { 326 super.listChildren(children); 327 children.add(new Property("country", "CodeableConcept", 328 "The country in which the marketing authorisation has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.", 329 0, 1, country)); 330 children.add(new Property("jurisdiction", "CodeableConcept", 331 "Where a Medicines Regulatory Agency has granted a marketing authorisation for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.", 332 0, 1, jurisdiction)); 333 children.add(new Property("status", "CodeableConcept", 334 "This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.", 335 0, 1, status)); 336 children.add(new Property("dateRange", "Period", 337 "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 338 0, 1, dateRange)); 339 children.add(new Property("restoreDate", "dateTime", 340 "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 341 0, 1, restoreDate)); 342 } 343 344 @Override 345 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 346 switch (_hash) { 347 case 957831062: 348 /* country */ return new Property("country", "CodeableConcept", 349 "The country in which the marketing authorisation has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.", 350 0, 1, country); 351 case -507075711: 352 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 353 "Where a Medicines Regulatory Agency has granted a marketing authorisation for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.", 354 0, 1, jurisdiction); 355 case -892481550: 356 /* status */ return new Property("status", "CodeableConcept", 357 "This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.", 358 0, 1, status); 359 case -261425617: 360 /* dateRange */ return new Property("dateRange", "Period", 361 "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 362 0, 1, dateRange); 363 case 329465692: 364 /* restoreDate */ return new Property("restoreDate", "dateTime", 365 "The date when the Medicinal Product is placed on the market by the Marketing Authorisation Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 366 0, 1, restoreDate); 367 default: 368 return super.getNamedProperty(_hash, _name, _checkValid); 369 } 370 371 } 372 373 @Override 374 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 375 switch (hash) { 376 case 957831062: 377 /* country */ return this.country == null ? new Base[0] : new Base[] { this.country }; // CodeableConcept 378 case -507075711: 379 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] : new Base[] { this.jurisdiction }; // CodeableConcept 380 case -892481550: 381 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 382 case -261425617: 383 /* dateRange */ return this.dateRange == null ? new Base[0] : new Base[] { this.dateRange }; // Period 384 case 329465692: 385 /* restoreDate */ return this.restoreDate == null ? new Base[0] : new Base[] { this.restoreDate }; // DateTimeType 386 default: 387 return super.getProperty(hash, name, checkValid); 388 } 389 390 } 391 392 @Override 393 public Base setProperty(int hash, String name, Base value) throws FHIRException { 394 switch (hash) { 395 case 957831062: // country 396 this.country = castToCodeableConcept(value); // CodeableConcept 397 return value; 398 case -507075711: // jurisdiction 399 this.jurisdiction = castToCodeableConcept(value); // CodeableConcept 400 return value; 401 case -892481550: // status 402 this.status = castToCodeableConcept(value); // CodeableConcept 403 return value; 404 case -261425617: // dateRange 405 this.dateRange = castToPeriod(value); // Period 406 return value; 407 case 329465692: // restoreDate 408 this.restoreDate = castToDateTime(value); // DateTimeType 409 return value; 410 default: 411 return super.setProperty(hash, name, value); 412 } 413 414 } 415 416 @Override 417 public Base setProperty(String name, Base value) throws FHIRException { 418 if (name.equals("country")) { 419 this.country = castToCodeableConcept(value); // CodeableConcept 420 } else if (name.equals("jurisdiction")) { 421 this.jurisdiction = castToCodeableConcept(value); // CodeableConcept 422 } else if (name.equals("status")) { 423 this.status = castToCodeableConcept(value); // CodeableConcept 424 } else if (name.equals("dateRange")) { 425 this.dateRange = castToPeriod(value); // Period 426 } else if (name.equals("restoreDate")) { 427 this.restoreDate = castToDateTime(value); // DateTimeType 428 } else 429 return super.setProperty(name, value); 430 return value; 431 } 432 433 @Override 434 public void removeChild(String name, Base value) throws FHIRException { 435 if (name.equals("country")) { 436 this.country = null; 437 } else if (name.equals("jurisdiction")) { 438 this.jurisdiction = null; 439 } else if (name.equals("status")) { 440 this.status = null; 441 } else if (name.equals("dateRange")) { 442 this.dateRange = null; 443 } else if (name.equals("restoreDate")) { 444 this.restoreDate = null; 445 } else 446 super.removeChild(name, value); 447 448 } 449 450 @Override 451 public Base makeProperty(int hash, String name) throws FHIRException { 452 switch (hash) { 453 case 957831062: 454 return getCountry(); 455 case -507075711: 456 return getJurisdiction(); 457 case -892481550: 458 return getStatus(); 459 case -261425617: 460 return getDateRange(); 461 case 329465692: 462 return getRestoreDateElement(); 463 default: 464 return super.makeProperty(hash, name); 465 } 466 467 } 468 469 @Override 470 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 471 switch (hash) { 472 case 957831062: 473 /* country */ return new String[] { "CodeableConcept" }; 474 case -507075711: 475 /* jurisdiction */ return new String[] { "CodeableConcept" }; 476 case -892481550: 477 /* status */ return new String[] { "CodeableConcept" }; 478 case -261425617: 479 /* dateRange */ return new String[] { "Period" }; 480 case 329465692: 481 /* restoreDate */ return new String[] { "dateTime" }; 482 default: 483 return super.getTypesForProperty(hash, name); 484 } 485 486 } 487 488 @Override 489 public Base addChild(String name) throws FHIRException { 490 if (name.equals("country")) { 491 this.country = new CodeableConcept(); 492 return this.country; 493 } else if (name.equals("jurisdiction")) { 494 this.jurisdiction = new CodeableConcept(); 495 return this.jurisdiction; 496 } else if (name.equals("status")) { 497 this.status = new CodeableConcept(); 498 return this.status; 499 } else if (name.equals("dateRange")) { 500 this.dateRange = new Period(); 501 return this.dateRange; 502 } else if (name.equals("restoreDate")) { 503 throw new FHIRException("Cannot call addChild on a singleton property MarketingStatus.restoreDate"); 504 } else 505 return super.addChild(name); 506 } 507 508 public String fhirType() { 509 return "MarketingStatus"; 510 511 } 512 513 public MarketingStatus copy() { 514 MarketingStatus dst = new MarketingStatus(); 515 copyValues(dst); 516 return dst; 517 } 518 519 public void copyValues(MarketingStatus dst) { 520 super.copyValues(dst); 521 dst.country = country == null ? null : country.copy(); 522 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 523 dst.status = status == null ? null : status.copy(); 524 dst.dateRange = dateRange == null ? null : dateRange.copy(); 525 dst.restoreDate = restoreDate == null ? null : restoreDate.copy(); 526 } 527 528 protected MarketingStatus typedCopy() { 529 return copy(); 530 } 531 532 @Override 533 public boolean equalsDeep(Base other_) { 534 if (!super.equalsDeep(other_)) 535 return false; 536 if (!(other_ instanceof MarketingStatus)) 537 return false; 538 MarketingStatus o = (MarketingStatus) other_; 539 return compareDeep(country, o.country, true) && compareDeep(jurisdiction, o.jurisdiction, true) 540 && compareDeep(status, o.status, true) && compareDeep(dateRange, o.dateRange, true) 541 && compareDeep(restoreDate, o.restoreDate, true); 542 } 543 544 @Override 545 public boolean equalsShallow(Base other_) { 546 if (!super.equalsShallow(other_)) 547 return false; 548 if (!(other_ instanceof MarketingStatus)) 549 return false; 550 MarketingStatus o = (MarketingStatus) other_; 551 return compareValues(restoreDate, o.restoreDate, true); 552 } 553 554 public boolean isEmpty() { 555 return super.isEmpty() 556 && ca.uhn.fhir.util.ElementUtil.isEmpty(country, jurisdiction, status, dateRange, restoreDate); 557 } 558 559}