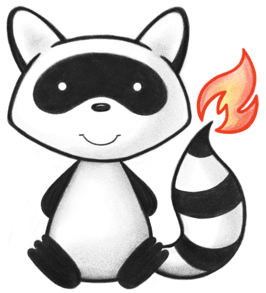
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * The Measure resource provides the definition of a quality measure. 052 */ 053@ResourceDef(name = "Measure", profile = "http://hl7.org/fhir/StructureDefinition/Measure") 054@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "status", "experimental", 055 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 056 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 057 "endorser", "relatedArtifact", "library", "disclaimer", "scoring", "compositeScoring", "type", "riskAdjustment", 058 "rateAggregation", "rationale", "clinicalRecommendationStatement", "improvementNotation", "definition", "guidance", 059 "group", "supplementalData" }) 060public class Measure extends MetadataResource { 061 062 @Block() 063 public static class MeasureGroupComponent extends BackboneElement implements IBaseBackboneElement { 064 /** 065 * Indicates a meaning for the group. This can be as simple as a unique 066 * identifier, or it can establish meaning in a broader context by drawing from 067 * a terminology, allowing groups to be correlated across measures. 068 */ 069 @Child(name = "code", type = { 070 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 071 @Description(shortDefinition = "Meaning of the group", formalDefinition = "Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.") 072 protected CodeableConcept code; 073 074 /** 075 * The human readable description of this population group. 076 */ 077 @Child(name = "description", type = { 078 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 079 @Description(shortDefinition = "Summary description", formalDefinition = "The human readable description of this population group.") 080 protected StringType description; 081 082 /** 083 * A population criteria for the measure. 084 */ 085 @Child(name = "population", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 086 @Description(shortDefinition = "Population criteria", formalDefinition = "A population criteria for the measure.") 087 protected List<MeasureGroupPopulationComponent> population; 088 089 /** 090 * The stratifier criteria for the measure report, specified as either the name 091 * of a valid CQL expression defined within a referenced library or a valid FHIR 092 * Resource Path. 093 */ 094 @Child(name = "stratifier", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 095 @Description(shortDefinition = "Stratifier criteria for the measure", formalDefinition = "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.") 096 protected List<MeasureGroupStratifierComponent> stratifier; 097 098 private static final long serialVersionUID = -1797567579L; 099 100 /** 101 * Constructor 102 */ 103 public MeasureGroupComponent() { 104 super(); 105 } 106 107 /** 108 * @return {@link #code} (Indicates a meaning for the group. This can be as 109 * simple as a unique identifier, or it can establish meaning in a 110 * broader context by drawing from a terminology, allowing groups to be 111 * correlated across measures.) 112 */ 113 public CodeableConcept getCode() { 114 if (this.code == null) 115 if (Configuration.errorOnAutoCreate()) 116 throw new Error("Attempt to auto-create MeasureGroupComponent.code"); 117 else if (Configuration.doAutoCreate()) 118 this.code = new CodeableConcept(); // cc 119 return this.code; 120 } 121 122 public boolean hasCode() { 123 return this.code != null && !this.code.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #code} (Indicates a meaning for the group. This can be as 128 * simple as a unique identifier, or it can establish meaning in a 129 * broader context by drawing from a terminology, allowing groups 130 * to be correlated across measures.) 131 */ 132 public MeasureGroupComponent setCode(CodeableConcept value) { 133 this.code = value; 134 return this; 135 } 136 137 /** 138 * @return {@link #description} (The human readable description of this 139 * population group.). This is the underlying object with id, value and 140 * extensions. The accessor "getDescription" gives direct access to the 141 * value 142 */ 143 public StringType getDescriptionElement() { 144 if (this.description == null) 145 if (Configuration.errorOnAutoCreate()) 146 throw new Error("Attempt to auto-create MeasureGroupComponent.description"); 147 else if (Configuration.doAutoCreate()) 148 this.description = new StringType(); // bb 149 return this.description; 150 } 151 152 public boolean hasDescriptionElement() { 153 return this.description != null && !this.description.isEmpty(); 154 } 155 156 public boolean hasDescription() { 157 return this.description != null && !this.description.isEmpty(); 158 } 159 160 /** 161 * @param value {@link #description} (The human readable description of this 162 * population group.). This is the underlying object with id, value 163 * and extensions. The accessor "getDescription" gives direct 164 * access to the value 165 */ 166 public MeasureGroupComponent setDescriptionElement(StringType value) { 167 this.description = value; 168 return this; 169 } 170 171 /** 172 * @return The human readable description of this population group. 173 */ 174 public String getDescription() { 175 return this.description == null ? null : this.description.getValue(); 176 } 177 178 /** 179 * @param value The human readable description of this population group. 180 */ 181 public MeasureGroupComponent setDescription(String value) { 182 if (Utilities.noString(value)) 183 this.description = null; 184 else { 185 if (this.description == null) 186 this.description = new StringType(); 187 this.description.setValue(value); 188 } 189 return this; 190 } 191 192 /** 193 * @return {@link #population} (A population criteria for the measure.) 194 */ 195 public List<MeasureGroupPopulationComponent> getPopulation() { 196 if (this.population == null) 197 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 198 return this.population; 199 } 200 201 /** 202 * @return Returns a reference to <code>this</code> for easy method chaining 203 */ 204 public MeasureGroupComponent setPopulation(List<MeasureGroupPopulationComponent> thePopulation) { 205 this.population = thePopulation; 206 return this; 207 } 208 209 public boolean hasPopulation() { 210 if (this.population == null) 211 return false; 212 for (MeasureGroupPopulationComponent item : this.population) 213 if (!item.isEmpty()) 214 return true; 215 return false; 216 } 217 218 public MeasureGroupPopulationComponent addPopulation() { // 3 219 MeasureGroupPopulationComponent t = new MeasureGroupPopulationComponent(); 220 if (this.population == null) 221 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 222 this.population.add(t); 223 return t; 224 } 225 226 public MeasureGroupComponent addPopulation(MeasureGroupPopulationComponent t) { // 3 227 if (t == null) 228 return this; 229 if (this.population == null) 230 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 231 this.population.add(t); 232 return this; 233 } 234 235 /** 236 * @return The first repetition of repeating field {@link #population}, creating 237 * it if it does not already exist 238 */ 239 public MeasureGroupPopulationComponent getPopulationFirstRep() { 240 if (getPopulation().isEmpty()) { 241 addPopulation(); 242 } 243 return getPopulation().get(0); 244 } 245 246 /** 247 * @return {@link #stratifier} (The stratifier criteria for the measure report, 248 * specified as either the name of a valid CQL expression defined within 249 * a referenced library or a valid FHIR Resource Path.) 250 */ 251 public List<MeasureGroupStratifierComponent> getStratifier() { 252 if (this.stratifier == null) 253 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 254 return this.stratifier; 255 } 256 257 /** 258 * @return Returns a reference to <code>this</code> for easy method chaining 259 */ 260 public MeasureGroupComponent setStratifier(List<MeasureGroupStratifierComponent> theStratifier) { 261 this.stratifier = theStratifier; 262 return this; 263 } 264 265 public boolean hasStratifier() { 266 if (this.stratifier == null) 267 return false; 268 for (MeasureGroupStratifierComponent item : this.stratifier) 269 if (!item.isEmpty()) 270 return true; 271 return false; 272 } 273 274 public MeasureGroupStratifierComponent addStratifier() { // 3 275 MeasureGroupStratifierComponent t = new MeasureGroupStratifierComponent(); 276 if (this.stratifier == null) 277 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 278 this.stratifier.add(t); 279 return t; 280 } 281 282 public MeasureGroupComponent addStratifier(MeasureGroupStratifierComponent t) { // 3 283 if (t == null) 284 return this; 285 if (this.stratifier == null) 286 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 287 this.stratifier.add(t); 288 return this; 289 } 290 291 /** 292 * @return The first repetition of repeating field {@link #stratifier}, creating 293 * it if it does not already exist 294 */ 295 public MeasureGroupStratifierComponent getStratifierFirstRep() { 296 if (getStratifier().isEmpty()) { 297 addStratifier(); 298 } 299 return getStratifier().get(0); 300 } 301 302 protected void listChildren(List<Property> children) { 303 super.listChildren(children); 304 children.add(new Property("code", "CodeableConcept", 305 "Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.", 306 0, 1, code)); 307 children.add(new Property("description", "string", "The human readable description of this population group.", 0, 308 1, description)); 309 children.add(new Property("population", "", "A population criteria for the measure.", 0, 310 java.lang.Integer.MAX_VALUE, population)); 311 children.add(new Property("stratifier", "", 312 "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 313 0, java.lang.Integer.MAX_VALUE, stratifier)); 314 } 315 316 @Override 317 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 318 switch (_hash) { 319 case 3059181: 320 /* code */ return new Property("code", "CodeableConcept", 321 "Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.", 322 0, 1, code); 323 case -1724546052: 324 /* description */ return new Property("description", "string", 325 "The human readable description of this population group.", 0, 1, description); 326 case -2023558323: 327 /* population */ return new Property("population", "", "A population criteria for the measure.", 0, 328 java.lang.Integer.MAX_VALUE, population); 329 case 90983669: 330 /* stratifier */ return new Property("stratifier", "", 331 "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 332 0, java.lang.Integer.MAX_VALUE, stratifier); 333 default: 334 return super.getNamedProperty(_hash, _name, _checkValid); 335 } 336 337 } 338 339 @Override 340 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 341 switch (hash) { 342 case 3059181: 343 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 344 case -1724546052: 345 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 346 case -2023558323: 347 /* population */ return this.population == null ? new Base[0] 348 : this.population.toArray(new Base[this.population.size()]); // MeasureGroupPopulationComponent 349 case 90983669: 350 /* stratifier */ return this.stratifier == null ? new Base[0] 351 : this.stratifier.toArray(new Base[this.stratifier.size()]); // MeasureGroupStratifierComponent 352 default: 353 return super.getProperty(hash, name, checkValid); 354 } 355 356 } 357 358 @Override 359 public Base setProperty(int hash, String name, Base value) throws FHIRException { 360 switch (hash) { 361 case 3059181: // code 362 this.code = castToCodeableConcept(value); // CodeableConcept 363 return value; 364 case -1724546052: // description 365 this.description = castToString(value); // StringType 366 return value; 367 case -2023558323: // population 368 this.getPopulation().add((MeasureGroupPopulationComponent) value); // MeasureGroupPopulationComponent 369 return value; 370 case 90983669: // stratifier 371 this.getStratifier().add((MeasureGroupStratifierComponent) value); // MeasureGroupStratifierComponent 372 return value; 373 default: 374 return super.setProperty(hash, name, value); 375 } 376 377 } 378 379 @Override 380 public Base setProperty(String name, Base value) throws FHIRException { 381 if (name.equals("code")) { 382 this.code = castToCodeableConcept(value); // CodeableConcept 383 } else if (name.equals("description")) { 384 this.description = castToString(value); // StringType 385 } else if (name.equals("population")) { 386 this.getPopulation().add((MeasureGroupPopulationComponent) value); 387 } else if (name.equals("stratifier")) { 388 this.getStratifier().add((MeasureGroupStratifierComponent) value); 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public void removeChild(String name, Base value) throws FHIRException { 396 if (name.equals("code")) { 397 this.code = null; 398 } else if (name.equals("description")) { 399 this.description = null; 400 } else if (name.equals("population")) { 401 this.getPopulation().remove((MeasureGroupPopulationComponent) value); 402 } else if (name.equals("stratifier")) { 403 this.getStratifier().remove((MeasureGroupStratifierComponent) value); 404 } else 405 super.removeChild(name, value); 406 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case 3059181: 413 return getCode(); 414 case -1724546052: 415 return getDescriptionElement(); 416 case -2023558323: 417 return addPopulation(); 418 case 90983669: 419 return addStratifier(); 420 default: 421 return super.makeProperty(hash, name); 422 } 423 424 } 425 426 @Override 427 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 428 switch (hash) { 429 case 3059181: 430 /* code */ return new String[] { "CodeableConcept" }; 431 case -1724546052: 432 /* description */ return new String[] { "string" }; 433 case -2023558323: 434 /* population */ return new String[] {}; 435 case 90983669: 436 /* stratifier */ return new String[] {}; 437 default: 438 return super.getTypesForProperty(hash, name); 439 } 440 441 } 442 443 @Override 444 public Base addChild(String name) throws FHIRException { 445 if (name.equals("code")) { 446 this.code = new CodeableConcept(); 447 return this.code; 448 } else if (name.equals("description")) { 449 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 450 } else if (name.equals("population")) { 451 return addPopulation(); 452 } else if (name.equals("stratifier")) { 453 return addStratifier(); 454 } else 455 return super.addChild(name); 456 } 457 458 public MeasureGroupComponent copy() { 459 MeasureGroupComponent dst = new MeasureGroupComponent(); 460 copyValues(dst); 461 return dst; 462 } 463 464 public void copyValues(MeasureGroupComponent dst) { 465 super.copyValues(dst); 466 dst.code = code == null ? null : code.copy(); 467 dst.description = description == null ? null : description.copy(); 468 if (population != null) { 469 dst.population = new ArrayList<MeasureGroupPopulationComponent>(); 470 for (MeasureGroupPopulationComponent i : population) 471 dst.population.add(i.copy()); 472 } 473 ; 474 if (stratifier != null) { 475 dst.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 476 for (MeasureGroupStratifierComponent i : stratifier) 477 dst.stratifier.add(i.copy()); 478 } 479 ; 480 } 481 482 @Override 483 public boolean equalsDeep(Base other_) { 484 if (!super.equalsDeep(other_)) 485 return false; 486 if (!(other_ instanceof MeasureGroupComponent)) 487 return false; 488 MeasureGroupComponent o = (MeasureGroupComponent) other_; 489 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 490 && compareDeep(population, o.population, true) && compareDeep(stratifier, o.stratifier, true); 491 } 492 493 @Override 494 public boolean equalsShallow(Base other_) { 495 if (!super.equalsShallow(other_)) 496 return false; 497 if (!(other_ instanceof MeasureGroupComponent)) 498 return false; 499 MeasureGroupComponent o = (MeasureGroupComponent) other_; 500 return compareValues(description, o.description, true); 501 } 502 503 public boolean isEmpty() { 504 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, population, stratifier); 505 } 506 507 public String fhirType() { 508 return "Measure.group"; 509 510 } 511 512 } 513 514 @Block() 515 public static class MeasureGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 516 /** 517 * The type of population criteria. 518 */ 519 @Child(name = "code", type = { 520 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 521 @Description(shortDefinition = "initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition = "The type of population criteria.") 522 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-population") 523 protected CodeableConcept code; 524 525 /** 526 * The human readable description of this population criteria. 527 */ 528 @Child(name = "description", type = { 529 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 530 @Description(shortDefinition = "The human readable description of this population criteria", formalDefinition = "The human readable description of this population criteria.") 531 protected StringType description; 532 533 /** 534 * An expression that specifies the criteria for the population, typically the 535 * name of an expression in a library. 536 */ 537 @Child(name = "criteria", type = { 538 Expression.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 539 @Description(shortDefinition = "The criteria that defines this population", formalDefinition = "An expression that specifies the criteria for the population, typically the name of an expression in a library.") 540 protected Expression criteria; 541 542 private static final long serialVersionUID = 2107514056L; 543 544 /** 545 * Constructor 546 */ 547 public MeasureGroupPopulationComponent() { 548 super(); 549 } 550 551 /** 552 * Constructor 553 */ 554 public MeasureGroupPopulationComponent(Expression criteria) { 555 super(); 556 this.criteria = criteria; 557 } 558 559 /** 560 * @return {@link #code} (The type of population criteria.) 561 */ 562 public CodeableConcept getCode() { 563 if (this.code == null) 564 if (Configuration.errorOnAutoCreate()) 565 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.code"); 566 else if (Configuration.doAutoCreate()) 567 this.code = new CodeableConcept(); // cc 568 return this.code; 569 } 570 571 public boolean hasCode() { 572 return this.code != null && !this.code.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #code} (The type of population criteria.) 577 */ 578 public MeasureGroupPopulationComponent setCode(CodeableConcept value) { 579 this.code = value; 580 return this; 581 } 582 583 /** 584 * @return {@link #description} (The human readable description of this 585 * population criteria.). This is the underlying object with id, value 586 * and extensions. The accessor "getDescription" gives direct access to 587 * the value 588 */ 589 public StringType getDescriptionElement() { 590 if (this.description == null) 591 if (Configuration.errorOnAutoCreate()) 592 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.description"); 593 else if (Configuration.doAutoCreate()) 594 this.description = new StringType(); // bb 595 return this.description; 596 } 597 598 public boolean hasDescriptionElement() { 599 return this.description != null && !this.description.isEmpty(); 600 } 601 602 public boolean hasDescription() { 603 return this.description != null && !this.description.isEmpty(); 604 } 605 606 /** 607 * @param value {@link #description} (The human readable description of this 608 * population criteria.). This is the underlying object with id, 609 * value and extensions. The accessor "getDescription" gives direct 610 * access to the value 611 */ 612 public MeasureGroupPopulationComponent setDescriptionElement(StringType value) { 613 this.description = value; 614 return this; 615 } 616 617 /** 618 * @return The human readable description of this population criteria. 619 */ 620 public String getDescription() { 621 return this.description == null ? null : this.description.getValue(); 622 } 623 624 /** 625 * @param value The human readable description of this population criteria. 626 */ 627 public MeasureGroupPopulationComponent setDescription(String value) { 628 if (Utilities.noString(value)) 629 this.description = null; 630 else { 631 if (this.description == null) 632 this.description = new StringType(); 633 this.description.setValue(value); 634 } 635 return this; 636 } 637 638 /** 639 * @return {@link #criteria} (An expression that specifies the criteria for the 640 * population, typically the name of an expression in a library.) 641 */ 642 public Expression getCriteria() { 643 if (this.criteria == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.criteria"); 646 else if (Configuration.doAutoCreate()) 647 this.criteria = new Expression(); // cc 648 return this.criteria; 649 } 650 651 public boolean hasCriteria() { 652 return this.criteria != null && !this.criteria.isEmpty(); 653 } 654 655 /** 656 * @param value {@link #criteria} (An expression that specifies the criteria for 657 * the population, typically the name of an expression in a 658 * library.) 659 */ 660 public MeasureGroupPopulationComponent setCriteria(Expression value) { 661 this.criteria = value; 662 return this; 663 } 664 665 protected void listChildren(List<Property> children) { 666 super.listChildren(children); 667 children.add(new Property("code", "CodeableConcept", "The type of population criteria.", 0, 1, code)); 668 children.add(new Property("description", "string", "The human readable description of this population criteria.", 669 0, 1, description)); 670 children.add(new Property("criteria", "Expression", 671 "An expression that specifies the criteria for the population, typically the name of an expression in a library.", 672 0, 1, criteria)); 673 } 674 675 @Override 676 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 677 switch (_hash) { 678 case 3059181: 679 /* code */ return new Property("code", "CodeableConcept", "The type of population criteria.", 0, 1, code); 680 case -1724546052: 681 /* description */ return new Property("description", "string", 682 "The human readable description of this population criteria.", 0, 1, description); 683 case 1952046943: 684 /* criteria */ return new Property("criteria", "Expression", 685 "An expression that specifies the criteria for the population, typically the name of an expression in a library.", 686 0, 1, criteria); 687 default: 688 return super.getNamedProperty(_hash, _name, _checkValid); 689 } 690 691 } 692 693 @Override 694 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 695 switch (hash) { 696 case 3059181: 697 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 698 case -1724546052: 699 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 700 case 1952046943: 701 /* criteria */ return this.criteria == null ? new Base[0] : new Base[] { this.criteria }; // Expression 702 default: 703 return super.getProperty(hash, name, checkValid); 704 } 705 706 } 707 708 @Override 709 public Base setProperty(int hash, String name, Base value) throws FHIRException { 710 switch (hash) { 711 case 3059181: // code 712 this.code = castToCodeableConcept(value); // CodeableConcept 713 return value; 714 case -1724546052: // description 715 this.description = castToString(value); // StringType 716 return value; 717 case 1952046943: // criteria 718 this.criteria = castToExpression(value); // Expression 719 return value; 720 default: 721 return super.setProperty(hash, name, value); 722 } 723 724 } 725 726 @Override 727 public Base setProperty(String name, Base value) throws FHIRException { 728 if (name.equals("code")) { 729 this.code = castToCodeableConcept(value); // CodeableConcept 730 } else if (name.equals("description")) { 731 this.description = castToString(value); // StringType 732 } else if (name.equals("criteria")) { 733 this.criteria = castToExpression(value); // Expression 734 } else 735 return super.setProperty(name, value); 736 return value; 737 } 738 739 @Override 740 public void removeChild(String name, Base value) throws FHIRException { 741 if (name.equals("code")) { 742 this.code = null; 743 } else if (name.equals("description")) { 744 this.description = null; 745 } else if (name.equals("criteria")) { 746 this.criteria = null; 747 } else 748 super.removeChild(name, value); 749 750 } 751 752 @Override 753 public Base makeProperty(int hash, String name) throws FHIRException { 754 switch (hash) { 755 case 3059181: 756 return getCode(); 757 case -1724546052: 758 return getDescriptionElement(); 759 case 1952046943: 760 return getCriteria(); 761 default: 762 return super.makeProperty(hash, name); 763 } 764 765 } 766 767 @Override 768 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 769 switch (hash) { 770 case 3059181: 771 /* code */ return new String[] { "CodeableConcept" }; 772 case -1724546052: 773 /* description */ return new String[] { "string" }; 774 case 1952046943: 775 /* criteria */ return new String[] { "Expression" }; 776 default: 777 return super.getTypesForProperty(hash, name); 778 } 779 780 } 781 782 @Override 783 public Base addChild(String name) throws FHIRException { 784 if (name.equals("code")) { 785 this.code = new CodeableConcept(); 786 return this.code; 787 } else if (name.equals("description")) { 788 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 789 } else if (name.equals("criteria")) { 790 this.criteria = new Expression(); 791 return this.criteria; 792 } else 793 return super.addChild(name); 794 } 795 796 public MeasureGroupPopulationComponent copy() { 797 MeasureGroupPopulationComponent dst = new MeasureGroupPopulationComponent(); 798 copyValues(dst); 799 return dst; 800 } 801 802 public void copyValues(MeasureGroupPopulationComponent dst) { 803 super.copyValues(dst); 804 dst.code = code == null ? null : code.copy(); 805 dst.description = description == null ? null : description.copy(); 806 dst.criteria = criteria == null ? null : criteria.copy(); 807 } 808 809 @Override 810 public boolean equalsDeep(Base other_) { 811 if (!super.equalsDeep(other_)) 812 return false; 813 if (!(other_ instanceof MeasureGroupPopulationComponent)) 814 return false; 815 MeasureGroupPopulationComponent o = (MeasureGroupPopulationComponent) other_; 816 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 817 && compareDeep(criteria, o.criteria, true); 818 } 819 820 @Override 821 public boolean equalsShallow(Base other_) { 822 if (!super.equalsShallow(other_)) 823 return false; 824 if (!(other_ instanceof MeasureGroupPopulationComponent)) 825 return false; 826 MeasureGroupPopulationComponent o = (MeasureGroupPopulationComponent) other_; 827 return compareValues(description, o.description, true); 828 } 829 830 public boolean isEmpty() { 831 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, criteria); 832 } 833 834 public String fhirType() { 835 return "Measure.group.population"; 836 837 } 838 839 } 840 841 @Block() 842 public static class MeasureGroupStratifierComponent extends BackboneElement implements IBaseBackboneElement { 843 /** 844 * Indicates a meaning for the stratifier. This can be as simple as a unique 845 * identifier, or it can establish meaning in a broader context by drawing from 846 * a terminology, allowing stratifiers to be correlated across measures. 847 */ 848 @Child(name = "code", type = { 849 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 850 @Description(shortDefinition = "Meaning of the stratifier", formalDefinition = "Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.") 851 protected CodeableConcept code; 852 853 /** 854 * The human readable description of this stratifier criteria. 855 */ 856 @Child(name = "description", type = { 857 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 858 @Description(shortDefinition = "The human readable description of this stratifier", formalDefinition = "The human readable description of this stratifier criteria.") 859 protected StringType description; 860 861 /** 862 * An expression that specifies the criteria for the stratifier. This is 863 * typically the name of an expression defined within a referenced library, but 864 * it may also be a path to a stratifier element. 865 */ 866 @Child(name = "criteria", type = { 867 Expression.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 868 @Description(shortDefinition = "How the measure should be stratified", formalDefinition = "An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.") 869 protected Expression criteria; 870 871 /** 872 * A component of the stratifier criteria for the measure report, specified as 873 * either the name of a valid CQL expression defined within a referenced library 874 * or a valid FHIR Resource Path. 875 */ 876 @Child(name = "component", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 877 @Description(shortDefinition = "Stratifier criteria component for the measure", formalDefinition = "A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.") 878 protected List<MeasureGroupStratifierComponentComponent> component; 879 880 private static final long serialVersionUID = -1706793609L; 881 882 /** 883 * Constructor 884 */ 885 public MeasureGroupStratifierComponent() { 886 super(); 887 } 888 889 /** 890 * @return {@link #code} (Indicates a meaning for the stratifier. This can be as 891 * simple as a unique identifier, or it can establish meaning in a 892 * broader context by drawing from a terminology, allowing stratifiers 893 * to be correlated across measures.) 894 */ 895 public CodeableConcept getCode() { 896 if (this.code == null) 897 if (Configuration.errorOnAutoCreate()) 898 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.code"); 899 else if (Configuration.doAutoCreate()) 900 this.code = new CodeableConcept(); // cc 901 return this.code; 902 } 903 904 public boolean hasCode() { 905 return this.code != null && !this.code.isEmpty(); 906 } 907 908 /** 909 * @param value {@link #code} (Indicates a meaning for the stratifier. This can 910 * be as simple as a unique identifier, or it can establish meaning 911 * in a broader context by drawing from a terminology, allowing 912 * stratifiers to be correlated across measures.) 913 */ 914 public MeasureGroupStratifierComponent setCode(CodeableConcept value) { 915 this.code = value; 916 return this; 917 } 918 919 /** 920 * @return {@link #description} (The human readable description of this 921 * stratifier criteria.). This is the underlying object with id, value 922 * and extensions. The accessor "getDescription" gives direct access to 923 * the value 924 */ 925 public StringType getDescriptionElement() { 926 if (this.description == null) 927 if (Configuration.errorOnAutoCreate()) 928 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.description"); 929 else if (Configuration.doAutoCreate()) 930 this.description = new StringType(); // bb 931 return this.description; 932 } 933 934 public boolean hasDescriptionElement() { 935 return this.description != null && !this.description.isEmpty(); 936 } 937 938 public boolean hasDescription() { 939 return this.description != null && !this.description.isEmpty(); 940 } 941 942 /** 943 * @param value {@link #description} (The human readable description of this 944 * stratifier criteria.). This is the underlying object with id, 945 * value and extensions. The accessor "getDescription" gives direct 946 * access to the value 947 */ 948 public MeasureGroupStratifierComponent setDescriptionElement(StringType value) { 949 this.description = value; 950 return this; 951 } 952 953 /** 954 * @return The human readable description of this stratifier criteria. 955 */ 956 public String getDescription() { 957 return this.description == null ? null : this.description.getValue(); 958 } 959 960 /** 961 * @param value The human readable description of this stratifier criteria. 962 */ 963 public MeasureGroupStratifierComponent setDescription(String value) { 964 if (Utilities.noString(value)) 965 this.description = null; 966 else { 967 if (this.description == null) 968 this.description = new StringType(); 969 this.description.setValue(value); 970 } 971 return this; 972 } 973 974 /** 975 * @return {@link #criteria} (An expression that specifies the criteria for the 976 * stratifier. This is typically the name of an expression defined 977 * within a referenced library, but it may also be a path to a 978 * stratifier element.) 979 */ 980 public Expression getCriteria() { 981 if (this.criteria == null) 982 if (Configuration.errorOnAutoCreate()) 983 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.criteria"); 984 else if (Configuration.doAutoCreate()) 985 this.criteria = new Expression(); // cc 986 return this.criteria; 987 } 988 989 public boolean hasCriteria() { 990 return this.criteria != null && !this.criteria.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #criteria} (An expression that specifies the criteria for 995 * the stratifier. This is typically the name of an expression 996 * defined within a referenced library, but it may also be a path 997 * to a stratifier element.) 998 */ 999 public MeasureGroupStratifierComponent setCriteria(Expression value) { 1000 this.criteria = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return {@link #component} (A component of the stratifier criteria for the 1006 * measure report, specified as either the name of a valid CQL 1007 * expression defined within a referenced library or a valid FHIR 1008 * Resource Path.) 1009 */ 1010 public List<MeasureGroupStratifierComponentComponent> getComponent() { 1011 if (this.component == null) 1012 this.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 1013 return this.component; 1014 } 1015 1016 /** 1017 * @return Returns a reference to <code>this</code> for easy method chaining 1018 */ 1019 public MeasureGroupStratifierComponent setComponent(List<MeasureGroupStratifierComponentComponent> theComponent) { 1020 this.component = theComponent; 1021 return this; 1022 } 1023 1024 public boolean hasComponent() { 1025 if (this.component == null) 1026 return false; 1027 for (MeasureGroupStratifierComponentComponent item : this.component) 1028 if (!item.isEmpty()) 1029 return true; 1030 return false; 1031 } 1032 1033 public MeasureGroupStratifierComponentComponent addComponent() { // 3 1034 MeasureGroupStratifierComponentComponent t = new MeasureGroupStratifierComponentComponent(); 1035 if (this.component == null) 1036 this.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 1037 this.component.add(t); 1038 return t; 1039 } 1040 1041 public MeasureGroupStratifierComponent addComponent(MeasureGroupStratifierComponentComponent t) { // 3 1042 if (t == null) 1043 return this; 1044 if (this.component == null) 1045 this.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 1046 this.component.add(t); 1047 return this; 1048 } 1049 1050 /** 1051 * @return The first repetition of repeating field {@link #component}, creating 1052 * it if it does not already exist 1053 */ 1054 public MeasureGroupStratifierComponentComponent getComponentFirstRep() { 1055 if (getComponent().isEmpty()) { 1056 addComponent(); 1057 } 1058 return getComponent().get(0); 1059 } 1060 1061 protected void listChildren(List<Property> children) { 1062 super.listChildren(children); 1063 children.add(new Property("code", "CodeableConcept", 1064 "Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 1065 0, 1, code)); 1066 children.add(new Property("description", "string", "The human readable description of this stratifier criteria.", 1067 0, 1, description)); 1068 children.add(new Property("criteria", "Expression", 1069 "An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 1070 0, 1, criteria)); 1071 children.add(new Property("component", "", 1072 "A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 1073 0, java.lang.Integer.MAX_VALUE, component)); 1074 } 1075 1076 @Override 1077 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1078 switch (_hash) { 1079 case 3059181: 1080 /* code */ return new Property("code", "CodeableConcept", 1081 "Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 1082 0, 1, code); 1083 case -1724546052: 1084 /* description */ return new Property("description", "string", 1085 "The human readable description of this stratifier criteria.", 0, 1, description); 1086 case 1952046943: 1087 /* criteria */ return new Property("criteria", "Expression", 1088 "An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 1089 0, 1, criteria); 1090 case -1399907075: 1091 /* component */ return new Property("component", "", 1092 "A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 1093 0, java.lang.Integer.MAX_VALUE, component); 1094 default: 1095 return super.getNamedProperty(_hash, _name, _checkValid); 1096 } 1097 1098 } 1099 1100 @Override 1101 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1102 switch (hash) { 1103 case 3059181: 1104 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1105 case -1724546052: 1106 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1107 case 1952046943: 1108 /* criteria */ return this.criteria == null ? new Base[0] : new Base[] { this.criteria }; // Expression 1109 case -1399907075: 1110 /* component */ return this.component == null ? new Base[0] 1111 : this.component.toArray(new Base[this.component.size()]); // MeasureGroupStratifierComponentComponent 1112 default: 1113 return super.getProperty(hash, name, checkValid); 1114 } 1115 1116 } 1117 1118 @Override 1119 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1120 switch (hash) { 1121 case 3059181: // code 1122 this.code = castToCodeableConcept(value); // CodeableConcept 1123 return value; 1124 case -1724546052: // description 1125 this.description = castToString(value); // StringType 1126 return value; 1127 case 1952046943: // criteria 1128 this.criteria = castToExpression(value); // Expression 1129 return value; 1130 case -1399907075: // component 1131 this.getComponent().add((MeasureGroupStratifierComponentComponent) value); // MeasureGroupStratifierComponentComponent 1132 return value; 1133 default: 1134 return super.setProperty(hash, name, value); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base setProperty(String name, Base value) throws FHIRException { 1141 if (name.equals("code")) { 1142 this.code = castToCodeableConcept(value); // CodeableConcept 1143 } else if (name.equals("description")) { 1144 this.description = castToString(value); // StringType 1145 } else if (name.equals("criteria")) { 1146 this.criteria = castToExpression(value); // Expression 1147 } else if (name.equals("component")) { 1148 this.getComponent().add((MeasureGroupStratifierComponentComponent) value); 1149 } else 1150 return super.setProperty(name, value); 1151 return value; 1152 } 1153 1154 @Override 1155 public void removeChild(String name, Base value) throws FHIRException { 1156 if (name.equals("code")) { 1157 this.code = null; 1158 } else if (name.equals("description")) { 1159 this.description = null; 1160 } else if (name.equals("criteria")) { 1161 this.criteria = null; 1162 } else if (name.equals("component")) { 1163 this.getComponent().remove((MeasureGroupStratifierComponentComponent) value); 1164 } else 1165 super.removeChild(name, value); 1166 1167 } 1168 1169 @Override 1170 public Base makeProperty(int hash, String name) throws FHIRException { 1171 switch (hash) { 1172 case 3059181: 1173 return getCode(); 1174 case -1724546052: 1175 return getDescriptionElement(); 1176 case 1952046943: 1177 return getCriteria(); 1178 case -1399907075: 1179 return addComponent(); 1180 default: 1181 return super.makeProperty(hash, name); 1182 } 1183 1184 } 1185 1186 @Override 1187 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1188 switch (hash) { 1189 case 3059181: 1190 /* code */ return new String[] { "CodeableConcept" }; 1191 case -1724546052: 1192 /* description */ return new String[] { "string" }; 1193 case 1952046943: 1194 /* criteria */ return new String[] { "Expression" }; 1195 case -1399907075: 1196 /* component */ return new String[] {}; 1197 default: 1198 return super.getTypesForProperty(hash, name); 1199 } 1200 1201 } 1202 1203 @Override 1204 public Base addChild(String name) throws FHIRException { 1205 if (name.equals("code")) { 1206 this.code = new CodeableConcept(); 1207 return this.code; 1208 } else if (name.equals("description")) { 1209 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 1210 } else if (name.equals("criteria")) { 1211 this.criteria = new Expression(); 1212 return this.criteria; 1213 } else if (name.equals("component")) { 1214 return addComponent(); 1215 } else 1216 return super.addChild(name); 1217 } 1218 1219 public MeasureGroupStratifierComponent copy() { 1220 MeasureGroupStratifierComponent dst = new MeasureGroupStratifierComponent(); 1221 copyValues(dst); 1222 return dst; 1223 } 1224 1225 public void copyValues(MeasureGroupStratifierComponent dst) { 1226 super.copyValues(dst); 1227 dst.code = code == null ? null : code.copy(); 1228 dst.description = description == null ? null : description.copy(); 1229 dst.criteria = criteria == null ? null : criteria.copy(); 1230 if (component != null) { 1231 dst.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 1232 for (MeasureGroupStratifierComponentComponent i : component) 1233 dst.component.add(i.copy()); 1234 } 1235 ; 1236 } 1237 1238 @Override 1239 public boolean equalsDeep(Base other_) { 1240 if (!super.equalsDeep(other_)) 1241 return false; 1242 if (!(other_ instanceof MeasureGroupStratifierComponent)) 1243 return false; 1244 MeasureGroupStratifierComponent o = (MeasureGroupStratifierComponent) other_; 1245 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 1246 && compareDeep(criteria, o.criteria, true) && compareDeep(component, o.component, true); 1247 } 1248 1249 @Override 1250 public boolean equalsShallow(Base other_) { 1251 if (!super.equalsShallow(other_)) 1252 return false; 1253 if (!(other_ instanceof MeasureGroupStratifierComponent)) 1254 return false; 1255 MeasureGroupStratifierComponent o = (MeasureGroupStratifierComponent) other_; 1256 return compareValues(description, o.description, true); 1257 } 1258 1259 public boolean isEmpty() { 1260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, criteria, component); 1261 } 1262 1263 public String fhirType() { 1264 return "Measure.group.stratifier"; 1265 1266 } 1267 1268 } 1269 1270 @Block() 1271 public static class MeasureGroupStratifierComponentComponent extends BackboneElement implements IBaseBackboneElement { 1272 /** 1273 * Indicates a meaning for the stratifier component. This can be as simple as a 1274 * unique identifier, or it can establish meaning in a broader context by 1275 * drawing from a terminology, allowing stratifiers to be correlated across 1276 * measures. 1277 */ 1278 @Child(name = "code", type = { 1279 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1280 @Description(shortDefinition = "Meaning of the stratifier component", formalDefinition = "Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.") 1281 protected CodeableConcept code; 1282 1283 /** 1284 * The human readable description of this stratifier criteria component. 1285 */ 1286 @Child(name = "description", type = { 1287 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1288 @Description(shortDefinition = "The human readable description of this stratifier component", formalDefinition = "The human readable description of this stratifier criteria component.") 1289 protected StringType description; 1290 1291 /** 1292 * An expression that specifies the criteria for this component of the 1293 * stratifier. This is typically the name of an expression defined within a 1294 * referenced library, but it may also be a path to a stratifier element. 1295 */ 1296 @Child(name = "criteria", type = { 1297 Expression.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1298 @Description(shortDefinition = "Component of how the measure should be stratified", formalDefinition = "An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.") 1299 protected Expression criteria; 1300 1301 private static final long serialVersionUID = 2107514056L; 1302 1303 /** 1304 * Constructor 1305 */ 1306 public MeasureGroupStratifierComponentComponent() { 1307 super(); 1308 } 1309 1310 /** 1311 * Constructor 1312 */ 1313 public MeasureGroupStratifierComponentComponent(Expression criteria) { 1314 super(); 1315 this.criteria = criteria; 1316 } 1317 1318 /** 1319 * @return {@link #code} (Indicates a meaning for the stratifier component. This 1320 * can be as simple as a unique identifier, or it can establish meaning 1321 * in a broader context by drawing from a terminology, allowing 1322 * stratifiers to be correlated across measures.) 1323 */ 1324 public CodeableConcept getCode() { 1325 if (this.code == null) 1326 if (Configuration.errorOnAutoCreate()) 1327 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.code"); 1328 else if (Configuration.doAutoCreate()) 1329 this.code = new CodeableConcept(); // cc 1330 return this.code; 1331 } 1332 1333 public boolean hasCode() { 1334 return this.code != null && !this.code.isEmpty(); 1335 } 1336 1337 /** 1338 * @param value {@link #code} (Indicates a meaning for the stratifier component. 1339 * This can be as simple as a unique identifier, or it can 1340 * establish meaning in a broader context by drawing from a 1341 * terminology, allowing stratifiers to be correlated across 1342 * measures.) 1343 */ 1344 public MeasureGroupStratifierComponentComponent setCode(CodeableConcept value) { 1345 this.code = value; 1346 return this; 1347 } 1348 1349 /** 1350 * @return {@link #description} (The human readable description of this 1351 * stratifier criteria component.). This is the underlying object with 1352 * id, value and extensions. The accessor "getDescription" gives direct 1353 * access to the value 1354 */ 1355 public StringType getDescriptionElement() { 1356 if (this.description == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.description"); 1359 else if (Configuration.doAutoCreate()) 1360 this.description = new StringType(); // bb 1361 return this.description; 1362 } 1363 1364 public boolean hasDescriptionElement() { 1365 return this.description != null && !this.description.isEmpty(); 1366 } 1367 1368 public boolean hasDescription() { 1369 return this.description != null && !this.description.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #description} (The human readable description of this 1374 * stratifier criteria component.). This is the underlying object 1375 * with id, value and extensions. The accessor "getDescription" 1376 * gives direct access to the value 1377 */ 1378 public MeasureGroupStratifierComponentComponent setDescriptionElement(StringType value) { 1379 this.description = value; 1380 return this; 1381 } 1382 1383 /** 1384 * @return The human readable description of this stratifier criteria component. 1385 */ 1386 public String getDescription() { 1387 return this.description == null ? null : this.description.getValue(); 1388 } 1389 1390 /** 1391 * @param value The human readable description of this stratifier criteria 1392 * component. 1393 */ 1394 public MeasureGroupStratifierComponentComponent setDescription(String value) { 1395 if (Utilities.noString(value)) 1396 this.description = null; 1397 else { 1398 if (this.description == null) 1399 this.description = new StringType(); 1400 this.description.setValue(value); 1401 } 1402 return this; 1403 } 1404 1405 /** 1406 * @return {@link #criteria} (An expression that specifies the criteria for this 1407 * component of the stratifier. This is typically the name of an 1408 * expression defined within a referenced library, but it may also be a 1409 * path to a stratifier element.) 1410 */ 1411 public Expression getCriteria() { 1412 if (this.criteria == null) 1413 if (Configuration.errorOnAutoCreate()) 1414 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.criteria"); 1415 else if (Configuration.doAutoCreate()) 1416 this.criteria = new Expression(); // cc 1417 return this.criteria; 1418 } 1419 1420 public boolean hasCriteria() { 1421 return this.criteria != null && !this.criteria.isEmpty(); 1422 } 1423 1424 /** 1425 * @param value {@link #criteria} (An expression that specifies the criteria for 1426 * this component of the stratifier. This is typically the name of 1427 * an expression defined within a referenced library, but it may 1428 * also be a path to a stratifier element.) 1429 */ 1430 public MeasureGroupStratifierComponentComponent setCriteria(Expression value) { 1431 this.criteria = value; 1432 return this; 1433 } 1434 1435 protected void listChildren(List<Property> children) { 1436 super.listChildren(children); 1437 children.add(new Property("code", "CodeableConcept", 1438 "Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 1439 0, 1, code)); 1440 children.add(new Property("description", "string", 1441 "The human readable description of this stratifier criteria component.", 0, 1, description)); 1442 children.add(new Property("criteria", "Expression", 1443 "An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 1444 0, 1, criteria)); 1445 } 1446 1447 @Override 1448 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1449 switch (_hash) { 1450 case 3059181: 1451 /* code */ return new Property("code", "CodeableConcept", 1452 "Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 1453 0, 1, code); 1454 case -1724546052: 1455 /* description */ return new Property("description", "string", 1456 "The human readable description of this stratifier criteria component.", 0, 1, description); 1457 case 1952046943: 1458 /* criteria */ return new Property("criteria", "Expression", 1459 "An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 1460 0, 1, criteria); 1461 default: 1462 return super.getNamedProperty(_hash, _name, _checkValid); 1463 } 1464 1465 } 1466 1467 @Override 1468 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1469 switch (hash) { 1470 case 3059181: 1471 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1472 case -1724546052: 1473 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1474 case 1952046943: 1475 /* criteria */ return this.criteria == null ? new Base[0] : new Base[] { this.criteria }; // Expression 1476 default: 1477 return super.getProperty(hash, name, checkValid); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1484 switch (hash) { 1485 case 3059181: // code 1486 this.code = castToCodeableConcept(value); // CodeableConcept 1487 return value; 1488 case -1724546052: // description 1489 this.description = castToString(value); // StringType 1490 return value; 1491 case 1952046943: // criteria 1492 this.criteria = castToExpression(value); // Expression 1493 return value; 1494 default: 1495 return super.setProperty(hash, name, value); 1496 } 1497 1498 } 1499 1500 @Override 1501 public Base setProperty(String name, Base value) throws FHIRException { 1502 if (name.equals("code")) { 1503 this.code = castToCodeableConcept(value); // CodeableConcept 1504 } else if (name.equals("description")) { 1505 this.description = castToString(value); // StringType 1506 } else if (name.equals("criteria")) { 1507 this.criteria = castToExpression(value); // Expression 1508 } else 1509 return super.setProperty(name, value); 1510 return value; 1511 } 1512 1513 @Override 1514 public void removeChild(String name, Base value) throws FHIRException { 1515 if (name.equals("code")) { 1516 this.code = null; 1517 } else if (name.equals("description")) { 1518 this.description = null; 1519 } else if (name.equals("criteria")) { 1520 this.criteria = null; 1521 } else 1522 super.removeChild(name, value); 1523 1524 } 1525 1526 @Override 1527 public Base makeProperty(int hash, String name) throws FHIRException { 1528 switch (hash) { 1529 case 3059181: 1530 return getCode(); 1531 case -1724546052: 1532 return getDescriptionElement(); 1533 case 1952046943: 1534 return getCriteria(); 1535 default: 1536 return super.makeProperty(hash, name); 1537 } 1538 1539 } 1540 1541 @Override 1542 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1543 switch (hash) { 1544 case 3059181: 1545 /* code */ return new String[] { "CodeableConcept" }; 1546 case -1724546052: 1547 /* description */ return new String[] { "string" }; 1548 case 1952046943: 1549 /* criteria */ return new String[] { "Expression" }; 1550 default: 1551 return super.getTypesForProperty(hash, name); 1552 } 1553 1554 } 1555 1556 @Override 1557 public Base addChild(String name) throws FHIRException { 1558 if (name.equals("code")) { 1559 this.code = new CodeableConcept(); 1560 return this.code; 1561 } else if (name.equals("description")) { 1562 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 1563 } else if (name.equals("criteria")) { 1564 this.criteria = new Expression(); 1565 return this.criteria; 1566 } else 1567 return super.addChild(name); 1568 } 1569 1570 public MeasureGroupStratifierComponentComponent copy() { 1571 MeasureGroupStratifierComponentComponent dst = new MeasureGroupStratifierComponentComponent(); 1572 copyValues(dst); 1573 return dst; 1574 } 1575 1576 public void copyValues(MeasureGroupStratifierComponentComponent dst) { 1577 super.copyValues(dst); 1578 dst.code = code == null ? null : code.copy(); 1579 dst.description = description == null ? null : description.copy(); 1580 dst.criteria = criteria == null ? null : criteria.copy(); 1581 } 1582 1583 @Override 1584 public boolean equalsDeep(Base other_) { 1585 if (!super.equalsDeep(other_)) 1586 return false; 1587 if (!(other_ instanceof MeasureGroupStratifierComponentComponent)) 1588 return false; 1589 MeasureGroupStratifierComponentComponent o = (MeasureGroupStratifierComponentComponent) other_; 1590 return compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 1591 && compareDeep(criteria, o.criteria, true); 1592 } 1593 1594 @Override 1595 public boolean equalsShallow(Base other_) { 1596 if (!super.equalsShallow(other_)) 1597 return false; 1598 if (!(other_ instanceof MeasureGroupStratifierComponentComponent)) 1599 return false; 1600 MeasureGroupStratifierComponentComponent o = (MeasureGroupStratifierComponentComponent) other_; 1601 return compareValues(description, o.description, true); 1602 } 1603 1604 public boolean isEmpty() { 1605 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, description, criteria); 1606 } 1607 1608 public String fhirType() { 1609 return "Measure.group.stratifier.component"; 1610 1611 } 1612 1613 } 1614 1615 @Block() 1616 public static class MeasureSupplementalDataComponent extends BackboneElement implements IBaseBackboneElement { 1617 /** 1618 * Indicates a meaning for the supplemental data. This can be as simple as a 1619 * unique identifier, or it can establish meaning in a broader context by 1620 * drawing from a terminology, allowing supplemental data to be correlated 1621 * across measures. 1622 */ 1623 @Child(name = "code", type = { 1624 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1625 @Description(shortDefinition = "Meaning of the supplemental data", formalDefinition = "Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.") 1626 protected CodeableConcept code; 1627 1628 /** 1629 * An indicator of the intended usage for the supplemental data element. 1630 * Supplemental data indicates the data is additional information requested to 1631 * augment the measure information. Risk adjustment factor indicates the data is 1632 * additional information used to calculate risk adjustment factors when 1633 * applying a risk model to the measure calculation. 1634 */ 1635 @Child(name = "usage", type = { 1636 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1637 @Description(shortDefinition = "supplemental-data | risk-adjustment-factor", formalDefinition = "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.") 1638 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-data-usage") 1639 protected List<CodeableConcept> usage; 1640 1641 /** 1642 * The human readable description of this supplemental data. 1643 */ 1644 @Child(name = "description", type = { 1645 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1646 @Description(shortDefinition = "The human readable description of this supplemental data", formalDefinition = "The human readable description of this supplemental data.") 1647 protected StringType description; 1648 1649 /** 1650 * The criteria for the supplemental data. This is typically the name of a valid 1651 * expression defined within a referenced library, but it may also be a path to 1652 * a specific data element. The criteria defines the data to be returned for 1653 * this element. 1654 */ 1655 @Child(name = "criteria", type = { 1656 Expression.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 1657 @Description(shortDefinition = "Expression describing additional data to be reported", formalDefinition = "The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.") 1658 protected Expression criteria; 1659 1660 private static final long serialVersionUID = -1897021670L; 1661 1662 /** 1663 * Constructor 1664 */ 1665 public MeasureSupplementalDataComponent() { 1666 super(); 1667 } 1668 1669 /** 1670 * Constructor 1671 */ 1672 public MeasureSupplementalDataComponent(Expression criteria) { 1673 super(); 1674 this.criteria = criteria; 1675 } 1676 1677 /** 1678 * @return {@link #code} (Indicates a meaning for the supplemental data. This 1679 * can be as simple as a unique identifier, or it can establish meaning 1680 * in a broader context by drawing from a terminology, allowing 1681 * supplemental data to be correlated across measures.) 1682 */ 1683 public CodeableConcept getCode() { 1684 if (this.code == null) 1685 if (Configuration.errorOnAutoCreate()) 1686 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.code"); 1687 else if (Configuration.doAutoCreate()) 1688 this.code = new CodeableConcept(); // cc 1689 return this.code; 1690 } 1691 1692 public boolean hasCode() { 1693 return this.code != null && !this.code.isEmpty(); 1694 } 1695 1696 /** 1697 * @param value {@link #code} (Indicates a meaning for the supplemental data. 1698 * This can be as simple as a unique identifier, or it can 1699 * establish meaning in a broader context by drawing from a 1700 * terminology, allowing supplemental data to be correlated across 1701 * measures.) 1702 */ 1703 public MeasureSupplementalDataComponent setCode(CodeableConcept value) { 1704 this.code = value; 1705 return this; 1706 } 1707 1708 /** 1709 * @return {@link #usage} (An indicator of the intended usage for the 1710 * supplemental data element. Supplemental data indicates the data is 1711 * additional information requested to augment the measure information. 1712 * Risk adjustment factor indicates the data is additional information 1713 * used to calculate risk adjustment factors when applying a risk model 1714 * to the measure calculation.) 1715 */ 1716 public List<CodeableConcept> getUsage() { 1717 if (this.usage == null) 1718 this.usage = new ArrayList<CodeableConcept>(); 1719 return this.usage; 1720 } 1721 1722 /** 1723 * @return Returns a reference to <code>this</code> for easy method chaining 1724 */ 1725 public MeasureSupplementalDataComponent setUsage(List<CodeableConcept> theUsage) { 1726 this.usage = theUsage; 1727 return this; 1728 } 1729 1730 public boolean hasUsage() { 1731 if (this.usage == null) 1732 return false; 1733 for (CodeableConcept item : this.usage) 1734 if (!item.isEmpty()) 1735 return true; 1736 return false; 1737 } 1738 1739 public CodeableConcept addUsage() { // 3 1740 CodeableConcept t = new CodeableConcept(); 1741 if (this.usage == null) 1742 this.usage = new ArrayList<CodeableConcept>(); 1743 this.usage.add(t); 1744 return t; 1745 } 1746 1747 public MeasureSupplementalDataComponent addUsage(CodeableConcept t) { // 3 1748 if (t == null) 1749 return this; 1750 if (this.usage == null) 1751 this.usage = new ArrayList<CodeableConcept>(); 1752 this.usage.add(t); 1753 return this; 1754 } 1755 1756 /** 1757 * @return The first repetition of repeating field {@link #usage}, creating it 1758 * if it does not already exist 1759 */ 1760 public CodeableConcept getUsageFirstRep() { 1761 if (getUsage().isEmpty()) { 1762 addUsage(); 1763 } 1764 return getUsage().get(0); 1765 } 1766 1767 /** 1768 * @return {@link #description} (The human readable description of this 1769 * supplemental data.). This is the underlying object with id, value and 1770 * extensions. The accessor "getDescription" gives direct access to the 1771 * value 1772 */ 1773 public StringType getDescriptionElement() { 1774 if (this.description == null) 1775 if (Configuration.errorOnAutoCreate()) 1776 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.description"); 1777 else if (Configuration.doAutoCreate()) 1778 this.description = new StringType(); // bb 1779 return this.description; 1780 } 1781 1782 public boolean hasDescriptionElement() { 1783 return this.description != null && !this.description.isEmpty(); 1784 } 1785 1786 public boolean hasDescription() { 1787 return this.description != null && !this.description.isEmpty(); 1788 } 1789 1790 /** 1791 * @param value {@link #description} (The human readable description of this 1792 * supplemental data.). This is the underlying object with id, 1793 * value and extensions. The accessor "getDescription" gives direct 1794 * access to the value 1795 */ 1796 public MeasureSupplementalDataComponent setDescriptionElement(StringType value) { 1797 this.description = value; 1798 return this; 1799 } 1800 1801 /** 1802 * @return The human readable description of this supplemental data. 1803 */ 1804 public String getDescription() { 1805 return this.description == null ? null : this.description.getValue(); 1806 } 1807 1808 /** 1809 * @param value The human readable description of this supplemental data. 1810 */ 1811 public MeasureSupplementalDataComponent setDescription(String value) { 1812 if (Utilities.noString(value)) 1813 this.description = null; 1814 else { 1815 if (this.description == null) 1816 this.description = new StringType(); 1817 this.description.setValue(value); 1818 } 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #criteria} (The criteria for the supplemental data. This is 1824 * typically the name of a valid expression defined within a referenced 1825 * library, but it may also be a path to a specific data element. The 1826 * criteria defines the data to be returned for this element.) 1827 */ 1828 public Expression getCriteria() { 1829 if (this.criteria == null) 1830 if (Configuration.errorOnAutoCreate()) 1831 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.criteria"); 1832 else if (Configuration.doAutoCreate()) 1833 this.criteria = new Expression(); // cc 1834 return this.criteria; 1835 } 1836 1837 public boolean hasCriteria() { 1838 return this.criteria != null && !this.criteria.isEmpty(); 1839 } 1840 1841 /** 1842 * @param value {@link #criteria} (The criteria for the supplemental data. This 1843 * is typically the name of a valid expression defined within a 1844 * referenced library, but it may also be a path to a specific data 1845 * element. The criteria defines the data to be returned for this 1846 * element.) 1847 */ 1848 public MeasureSupplementalDataComponent setCriteria(Expression value) { 1849 this.criteria = value; 1850 return this; 1851 } 1852 1853 protected void listChildren(List<Property> children) { 1854 super.listChildren(children); 1855 children.add(new Property("code", "CodeableConcept", 1856 "Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.", 1857 0, 1, code)); 1858 children.add(new Property("usage", "CodeableConcept", 1859 "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.", 1860 0, java.lang.Integer.MAX_VALUE, usage)); 1861 children.add(new Property("description", "string", "The human readable description of this supplemental data.", 0, 1862 1, description)); 1863 children.add(new Property("criteria", "Expression", 1864 "The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.", 1865 0, 1, criteria)); 1866 } 1867 1868 @Override 1869 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1870 switch (_hash) { 1871 case 3059181: 1872 /* code */ return new Property("code", "CodeableConcept", 1873 "Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.", 1874 0, 1, code); 1875 case 111574433: 1876 /* usage */ return new Property("usage", "CodeableConcept", 1877 "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.", 1878 0, java.lang.Integer.MAX_VALUE, usage); 1879 case -1724546052: 1880 /* description */ return new Property("description", "string", 1881 "The human readable description of this supplemental data.", 0, 1, description); 1882 case 1952046943: 1883 /* criteria */ return new Property("criteria", "Expression", 1884 "The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.", 1885 0, 1, criteria); 1886 default: 1887 return super.getNamedProperty(_hash, _name, _checkValid); 1888 } 1889 1890 } 1891 1892 @Override 1893 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1894 switch (hash) { 1895 case 3059181: 1896 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1897 case 111574433: 1898 /* usage */ return this.usage == null ? new Base[0] : this.usage.toArray(new Base[this.usage.size()]); // CodeableConcept 1899 case -1724546052: 1900 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1901 case 1952046943: 1902 /* criteria */ return this.criteria == null ? new Base[0] : new Base[] { this.criteria }; // Expression 1903 default: 1904 return super.getProperty(hash, name, checkValid); 1905 } 1906 1907 } 1908 1909 @Override 1910 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1911 switch (hash) { 1912 case 3059181: // code 1913 this.code = castToCodeableConcept(value); // CodeableConcept 1914 return value; 1915 case 111574433: // usage 1916 this.getUsage().add(castToCodeableConcept(value)); // CodeableConcept 1917 return value; 1918 case -1724546052: // description 1919 this.description = castToString(value); // StringType 1920 return value; 1921 case 1952046943: // criteria 1922 this.criteria = castToExpression(value); // Expression 1923 return value; 1924 default: 1925 return super.setProperty(hash, name, value); 1926 } 1927 1928 } 1929 1930 @Override 1931 public Base setProperty(String name, Base value) throws FHIRException { 1932 if (name.equals("code")) { 1933 this.code = castToCodeableConcept(value); // CodeableConcept 1934 } else if (name.equals("usage")) { 1935 this.getUsage().add(castToCodeableConcept(value)); 1936 } else if (name.equals("description")) { 1937 this.description = castToString(value); // StringType 1938 } else if (name.equals("criteria")) { 1939 this.criteria = castToExpression(value); // Expression 1940 } else 1941 return super.setProperty(name, value); 1942 return value; 1943 } 1944 1945 @Override 1946 public void removeChild(String name, Base value) throws FHIRException { 1947 if (name.equals("code")) { 1948 this.code = null; 1949 } else if (name.equals("usage")) { 1950 this.getUsage().remove(castToCodeableConcept(value)); 1951 } else if (name.equals("description")) { 1952 this.description = null; 1953 } else if (name.equals("criteria")) { 1954 this.criteria = null; 1955 } else 1956 super.removeChild(name, value); 1957 1958 } 1959 1960 @Override 1961 public Base makeProperty(int hash, String name) throws FHIRException { 1962 switch (hash) { 1963 case 3059181: 1964 return getCode(); 1965 case 111574433: 1966 return addUsage(); 1967 case -1724546052: 1968 return getDescriptionElement(); 1969 case 1952046943: 1970 return getCriteria(); 1971 default: 1972 return super.makeProperty(hash, name); 1973 } 1974 1975 } 1976 1977 @Override 1978 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1979 switch (hash) { 1980 case 3059181: 1981 /* code */ return new String[] { "CodeableConcept" }; 1982 case 111574433: 1983 /* usage */ return new String[] { "CodeableConcept" }; 1984 case -1724546052: 1985 /* description */ return new String[] { "string" }; 1986 case 1952046943: 1987 /* criteria */ return new String[] { "Expression" }; 1988 default: 1989 return super.getTypesForProperty(hash, name); 1990 } 1991 1992 } 1993 1994 @Override 1995 public Base addChild(String name) throws FHIRException { 1996 if (name.equals("code")) { 1997 this.code = new CodeableConcept(); 1998 return this.code; 1999 } else if (name.equals("usage")) { 2000 return addUsage(); 2001 } else if (name.equals("description")) { 2002 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 2003 } else if (name.equals("criteria")) { 2004 this.criteria = new Expression(); 2005 return this.criteria; 2006 } else 2007 return super.addChild(name); 2008 } 2009 2010 public MeasureSupplementalDataComponent copy() { 2011 MeasureSupplementalDataComponent dst = new MeasureSupplementalDataComponent(); 2012 copyValues(dst); 2013 return dst; 2014 } 2015 2016 public void copyValues(MeasureSupplementalDataComponent dst) { 2017 super.copyValues(dst); 2018 dst.code = code == null ? null : code.copy(); 2019 if (usage != null) { 2020 dst.usage = new ArrayList<CodeableConcept>(); 2021 for (CodeableConcept i : usage) 2022 dst.usage.add(i.copy()); 2023 } 2024 ; 2025 dst.description = description == null ? null : description.copy(); 2026 dst.criteria = criteria == null ? null : criteria.copy(); 2027 } 2028 2029 @Override 2030 public boolean equalsDeep(Base other_) { 2031 if (!super.equalsDeep(other_)) 2032 return false; 2033 if (!(other_ instanceof MeasureSupplementalDataComponent)) 2034 return false; 2035 MeasureSupplementalDataComponent o = (MeasureSupplementalDataComponent) other_; 2036 return compareDeep(code, o.code, true) && compareDeep(usage, o.usage, true) 2037 && compareDeep(description, o.description, true) && compareDeep(criteria, o.criteria, true); 2038 } 2039 2040 @Override 2041 public boolean equalsShallow(Base other_) { 2042 if (!super.equalsShallow(other_)) 2043 return false; 2044 if (!(other_ instanceof MeasureSupplementalDataComponent)) 2045 return false; 2046 MeasureSupplementalDataComponent o = (MeasureSupplementalDataComponent) other_; 2047 return compareValues(description, o.description, true); 2048 } 2049 2050 public boolean isEmpty() { 2051 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, usage, description, criteria); 2052 } 2053 2054 public String fhirType() { 2055 return "Measure.supplementalData"; 2056 2057 } 2058 2059 } 2060 2061 /** 2062 * A formal identifier that is used to identify this measure when it is 2063 * represented in other formats, or referenced in a specification, model, design 2064 * or an instance. 2065 */ 2066 @Child(name = "identifier", type = { 2067 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2068 @Description(shortDefinition = "Additional identifier for the measure", formalDefinition = "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.") 2069 protected List<Identifier> identifier; 2070 2071 /** 2072 * An explanatory or alternate title for the measure giving additional 2073 * information about its content. 2074 */ 2075 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2076 @Description(shortDefinition = "Subordinate title of the measure", formalDefinition = "An explanatory or alternate title for the measure giving additional information about its content.") 2077 protected StringType subtitle; 2078 2079 /** 2080 * The intended subjects for the measure. If this element is not provided, a 2081 * Patient subject is assumed, but the subject of the measure can be anything. 2082 */ 2083 @Child(name = "subject", type = { CodeableConcept.class, 2084 Group.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2085 @Description(shortDefinition = "E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition = "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.") 2086 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 2087 protected Type subject; 2088 2089 /** 2090 * Explanation of why this measure is needed and why it has been designed as it 2091 * has. 2092 */ 2093 @Child(name = "purpose", type = { 2094 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2095 @Description(shortDefinition = "Why this measure is defined", formalDefinition = "Explanation of why this measure is needed and why it has been designed as it has.") 2096 protected MarkdownType purpose; 2097 2098 /** 2099 * A detailed description, from a clinical perspective, of how the measure is 2100 * used. 2101 */ 2102 @Child(name = "usage", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2103 @Description(shortDefinition = "Describes the clinical usage of the measure", formalDefinition = "A detailed description, from a clinical perspective, of how the measure is used.") 2104 protected StringType usage; 2105 2106 /** 2107 * A copyright statement relating to the measure and/or its contents. Copyright 2108 * statements are generally legal restrictions on the use and publishing of the 2109 * measure. 2110 */ 2111 @Child(name = "copyright", type = { 2112 MarkdownType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2113 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.") 2114 protected MarkdownType copyright; 2115 2116 /** 2117 * The date on which the resource content was approved by the publisher. 2118 * Approval happens once when the content is officially approved for usage. 2119 */ 2120 @Child(name = "approvalDate", type = { 2121 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2122 @Description(shortDefinition = "When the measure was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 2123 protected DateType approvalDate; 2124 2125 /** 2126 * The date on which the resource content was last reviewed. Review happens 2127 * periodically after approval but does not change the original approval date. 2128 */ 2129 @Child(name = "lastReviewDate", type = { 2130 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2131 @Description(shortDefinition = "When the measure was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 2132 protected DateType lastReviewDate; 2133 2134 /** 2135 * The period during which the measure content was or is planned to be in active 2136 * use. 2137 */ 2138 @Child(name = "effectivePeriod", type = { 2139 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2140 @Description(shortDefinition = "When the measure is expected to be used", formalDefinition = "The period during which the measure content was or is planned to be in active use.") 2141 protected Period effectivePeriod; 2142 2143 /** 2144 * Descriptive topics related to the content of the measure. Topics provide a 2145 * high-level categorization grouping types of measures that can be useful for 2146 * filtering and searching. 2147 */ 2148 @Child(name = "topic", type = { 2149 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2150 @Description(shortDefinition = "The category of the measure, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching.") 2151 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 2152 protected List<CodeableConcept> topic; 2153 2154 /** 2155 * An individiual or organization primarily involved in the creation and 2156 * maintenance of the content. 2157 */ 2158 @Child(name = "author", type = { 2159 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2160 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 2161 protected List<ContactDetail> author; 2162 2163 /** 2164 * An individual or organization primarily responsible for internal coherence of 2165 * the content. 2166 */ 2167 @Child(name = "editor", type = { 2168 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2169 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 2170 protected List<ContactDetail> editor; 2171 2172 /** 2173 * An individual or organization primarily responsible for review of some aspect 2174 * of the content. 2175 */ 2176 @Child(name = "reviewer", type = { 2177 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2178 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 2179 protected List<ContactDetail> reviewer; 2180 2181 /** 2182 * An individual or organization responsible for officially endorsing the 2183 * content for use in some setting. 2184 */ 2185 @Child(name = "endorser", type = { 2186 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2187 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 2188 protected List<ContactDetail> endorser; 2189 2190 /** 2191 * Related artifacts such as additional documentation, justification, or 2192 * bibliographic references. 2193 */ 2194 @Child(name = "relatedArtifact", type = { 2195 RelatedArtifact.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2196 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 2197 protected List<RelatedArtifact> relatedArtifact; 2198 2199 /** 2200 * A reference to a Library resource containing the formal logic used by the 2201 * measure. 2202 */ 2203 @Child(name = "library", type = { 2204 CanonicalType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2205 @Description(shortDefinition = "Logic used by the measure", formalDefinition = "A reference to a Library resource containing the formal logic used by the measure.") 2206 protected List<CanonicalType> library; 2207 2208 /** 2209 * Notices and disclaimers regarding the use of the measure or related to 2210 * intellectual property (such as code systems) referenced by the measure. 2211 */ 2212 @Child(name = "disclaimer", type = { 2213 MarkdownType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 2214 @Description(shortDefinition = "Disclaimer for use of the measure or its referenced content", formalDefinition = "Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.") 2215 protected MarkdownType disclaimer; 2216 2217 /** 2218 * Indicates how the calculation is performed for the measure, including 2219 * proportion, ratio, continuous-variable, and cohort. The value set is 2220 * extensible, allowing additional measure scoring types to be represented. 2221 */ 2222 @Child(name = "scoring", type = { 2223 CodeableConcept.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 2224 @Description(shortDefinition = "proportion | ratio | continuous-variable | cohort", formalDefinition = "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.") 2225 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-scoring") 2226 protected CodeableConcept scoring; 2227 2228 /** 2229 * If this is a composite measure, the scoring method used to combine the 2230 * component measures to determine the composite score. 2231 */ 2232 @Child(name = "compositeScoring", type = { 2233 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 2234 @Description(shortDefinition = "opportunity | all-or-nothing | linear | weighted", formalDefinition = "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.") 2235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/composite-measure-scoring") 2236 protected CodeableConcept compositeScoring; 2237 2238 /** 2239 * Indicates whether the measure is used to examine a process, an outcome over 2240 * time, a patient-reported outcome, or a structure measure such as utilization. 2241 */ 2242 @Child(name = "type", type = { 2243 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2244 @Description(shortDefinition = "process | outcome | structure | patient-reported-outcome | composite", formalDefinition = "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.") 2245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-type") 2246 protected List<CodeableConcept> type; 2247 2248 /** 2249 * A description of the risk adjustment factors that may impact the resulting 2250 * score for the measure and how they may be accounted for when computing and 2251 * reporting measure results. 2252 */ 2253 @Child(name = "riskAdjustment", type = { 2254 StringType.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 2255 @Description(shortDefinition = "How risk adjustment is applied for this measure", formalDefinition = "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.") 2256 protected StringType riskAdjustment; 2257 2258 /** 2259 * Describes how to combine the information calculated, based on logic in each 2260 * of several populations, into one summarized result. 2261 */ 2262 @Child(name = "rateAggregation", type = { 2263 StringType.class }, order = 21, min = 0, max = 1, modifier = false, summary = true) 2264 @Description(shortDefinition = "How is rate aggregation performed for this measure", formalDefinition = "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.") 2265 protected StringType rateAggregation; 2266 2267 /** 2268 * Provides a succinct statement of the need for the measure. Usually includes 2269 * statements pertaining to importance criterion: impact, gap in care, and 2270 * evidence. 2271 */ 2272 @Child(name = "rationale", type = { 2273 MarkdownType.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 2274 @Description(shortDefinition = "Detailed description of why the measure exists", formalDefinition = "Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.") 2275 protected MarkdownType rationale; 2276 2277 /** 2278 * Provides a summary of relevant clinical guidelines or other clinical 2279 * recommendations supporting the measure. 2280 */ 2281 @Child(name = "clinicalRecommendationStatement", type = { 2282 MarkdownType.class }, order = 23, min = 0, max = 1, modifier = false, summary = true) 2283 @Description(shortDefinition = "Summary of clinical guidelines", formalDefinition = "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.") 2284 protected MarkdownType clinicalRecommendationStatement; 2285 2286 /** 2287 * Information on whether an increase or decrease in score is the preferred 2288 * result (e.g., a higher score indicates better quality OR a lower score 2289 * indicates better quality OR quality is within a range). 2290 */ 2291 @Child(name = "improvementNotation", type = { 2292 CodeableConcept.class }, order = 24, min = 0, max = 1, modifier = false, summary = true) 2293 @Description(shortDefinition = "increase | decrease", formalDefinition = "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).") 2294 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-improvement-notation") 2295 protected CodeableConcept improvementNotation; 2296 2297 /** 2298 * Provides a description of an individual term used within the measure. 2299 */ 2300 @Child(name = "definition", type = { 2301 MarkdownType.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2302 @Description(shortDefinition = "Defined terms used in the measure documentation", formalDefinition = "Provides a description of an individual term used within the measure.") 2303 protected List<MarkdownType> definition; 2304 2305 /** 2306 * Additional guidance for the measure including how it can be used in a 2307 * clinical context, and the intent of the measure. 2308 */ 2309 @Child(name = "guidance", type = { 2310 MarkdownType.class }, order = 26, min = 0, max = 1, modifier = false, summary = true) 2311 @Description(shortDefinition = "Additional guidance for implementers", formalDefinition = "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.") 2312 protected MarkdownType guidance; 2313 2314 /** 2315 * A group of population criteria for the measure. 2316 */ 2317 @Child(name = "group", type = {}, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2318 @Description(shortDefinition = "Population criteria group", formalDefinition = "A group of population criteria for the measure.") 2319 protected List<MeasureGroupComponent> group; 2320 2321 /** 2322 * The supplemental data criteria for the measure report, specified as either 2323 * the name of a valid CQL expression within a referenced library, or a valid 2324 * FHIR Resource Path. 2325 */ 2326 @Child(name = "supplementalData", type = {}, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2327 @Description(shortDefinition = "What other data should be reported with the measure", formalDefinition = "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.") 2328 protected List<MeasureSupplementalDataComponent> supplementalData; 2329 2330 private static final long serialVersionUID = 341731972L; 2331 2332 /** 2333 * Constructor 2334 */ 2335 public Measure() { 2336 super(); 2337 } 2338 2339 /** 2340 * Constructor 2341 */ 2342 public Measure(Enumeration<PublicationStatus> status) { 2343 super(); 2344 this.status = status; 2345 } 2346 2347 /** 2348 * @return {@link #url} (An absolute URI that is used to identify this measure 2349 * when it is referenced in a specification, model, design or an 2350 * instance; also called its canonical identifier. This SHOULD be 2351 * globally unique and SHOULD be a literal address at which at which an 2352 * authoritative instance of this measure is (or will be) published. 2353 * This URL can be the target of a canonical reference. It SHALL remain 2354 * the same when the measure is stored on different servers.). This is 2355 * the underlying object with id, value and extensions. The accessor 2356 * "getUrl" gives direct access to the value 2357 */ 2358 public UriType getUrlElement() { 2359 if (this.url == null) 2360 if (Configuration.errorOnAutoCreate()) 2361 throw new Error("Attempt to auto-create Measure.url"); 2362 else if (Configuration.doAutoCreate()) 2363 this.url = new UriType(); // bb 2364 return this.url; 2365 } 2366 2367 public boolean hasUrlElement() { 2368 return this.url != null && !this.url.isEmpty(); 2369 } 2370 2371 public boolean hasUrl() { 2372 return this.url != null && !this.url.isEmpty(); 2373 } 2374 2375 /** 2376 * @param value {@link #url} (An absolute URI that is used to identify this 2377 * measure when it is referenced in a specification, model, design 2378 * or an instance; also called its canonical identifier. This 2379 * SHOULD be globally unique and SHOULD be a literal address at 2380 * which at which an authoritative instance of this measure is (or 2381 * will be) published. This URL can be the target of a canonical 2382 * reference. It SHALL remain the same when the measure is stored 2383 * on different servers.). This is the underlying object with id, 2384 * value and extensions. The accessor "getUrl" gives direct access 2385 * to the value 2386 */ 2387 public Measure setUrlElement(UriType value) { 2388 this.url = value; 2389 return this; 2390 } 2391 2392 /** 2393 * @return An absolute URI that is used to identify this measure when it is 2394 * referenced in a specification, model, design or an instance; also 2395 * called its canonical identifier. This SHOULD be globally unique and 2396 * SHOULD be a literal address at which at which an authoritative 2397 * instance of this measure is (or will be) published. This URL can be 2398 * the target of a canonical reference. It SHALL remain the same when 2399 * the measure is stored on different servers. 2400 */ 2401 public String getUrl() { 2402 return this.url == null ? null : this.url.getValue(); 2403 } 2404 2405 /** 2406 * @param value An absolute URI that is used to identify this measure when it is 2407 * referenced in a specification, model, design or an instance; 2408 * also called its canonical identifier. This SHOULD be globally 2409 * unique and SHOULD be a literal address at which at which an 2410 * authoritative instance of this measure is (or will be) 2411 * published. This URL can be the target of a canonical reference. 2412 * It SHALL remain the same when the measure is stored on different 2413 * servers. 2414 */ 2415 public Measure setUrl(String value) { 2416 if (Utilities.noString(value)) 2417 this.url = null; 2418 else { 2419 if (this.url == null) 2420 this.url = new UriType(); 2421 this.url.setValue(value); 2422 } 2423 return this; 2424 } 2425 2426 /** 2427 * @return {@link #identifier} (A formal identifier that is used to identify 2428 * this measure when it is represented in other formats, or referenced 2429 * in a specification, model, design or an instance.) 2430 */ 2431 public List<Identifier> getIdentifier() { 2432 if (this.identifier == null) 2433 this.identifier = new ArrayList<Identifier>(); 2434 return this.identifier; 2435 } 2436 2437 /** 2438 * @return Returns a reference to <code>this</code> for easy method chaining 2439 */ 2440 public Measure setIdentifier(List<Identifier> theIdentifier) { 2441 this.identifier = theIdentifier; 2442 return this; 2443 } 2444 2445 public boolean hasIdentifier() { 2446 if (this.identifier == null) 2447 return false; 2448 for (Identifier item : this.identifier) 2449 if (!item.isEmpty()) 2450 return true; 2451 return false; 2452 } 2453 2454 public Identifier addIdentifier() { // 3 2455 Identifier t = new Identifier(); 2456 if (this.identifier == null) 2457 this.identifier = new ArrayList<Identifier>(); 2458 this.identifier.add(t); 2459 return t; 2460 } 2461 2462 public Measure addIdentifier(Identifier t) { // 3 2463 if (t == null) 2464 return this; 2465 if (this.identifier == null) 2466 this.identifier = new ArrayList<Identifier>(); 2467 this.identifier.add(t); 2468 return this; 2469 } 2470 2471 /** 2472 * @return The first repetition of repeating field {@link #identifier}, creating 2473 * it if it does not already exist 2474 */ 2475 public Identifier getIdentifierFirstRep() { 2476 if (getIdentifier().isEmpty()) { 2477 addIdentifier(); 2478 } 2479 return getIdentifier().get(0); 2480 } 2481 2482 /** 2483 * @return {@link #version} (The identifier that is used to identify this 2484 * version of the measure when it is referenced in a specification, 2485 * model, design or instance. This is an arbitrary value managed by the 2486 * measure author and is not expected to be globally unique. For 2487 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 2488 * is not available. There is also no expectation that versions can be 2489 * placed in a lexicographical sequence. To provide a version consistent 2490 * with the Decision Support Service specification, use the format 2491 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 2492 * knowledge assets, refer to the Decision Support Service 2493 * specification. Note that a version is required for non-experimental 2494 * active artifacts.). This is the underlying object with id, value and 2495 * extensions. The accessor "getVersion" gives direct access to the 2496 * value 2497 */ 2498 public StringType getVersionElement() { 2499 if (this.version == null) 2500 if (Configuration.errorOnAutoCreate()) 2501 throw new Error("Attempt to auto-create Measure.version"); 2502 else if (Configuration.doAutoCreate()) 2503 this.version = new StringType(); // bb 2504 return this.version; 2505 } 2506 2507 public boolean hasVersionElement() { 2508 return this.version != null && !this.version.isEmpty(); 2509 } 2510 2511 public boolean hasVersion() { 2512 return this.version != null && !this.version.isEmpty(); 2513 } 2514 2515 /** 2516 * @param value {@link #version} (The identifier that is used to identify this 2517 * version of the measure when it is referenced in a specification, 2518 * model, design or instance. This is an arbitrary value managed by 2519 * the measure author and is not expected to be globally unique. 2520 * For example, it might be a timestamp (e.g. yyyymmdd) if a 2521 * managed version is not available. There is also no expectation 2522 * that versions can be placed in a lexicographical sequence. To 2523 * provide a version consistent with the Decision Support Service 2524 * specification, use the format Major.Minor.Revision (e.g. 1.0.0). 2525 * For more information on versioning knowledge assets, refer to 2526 * the Decision Support Service specification. Note that a version 2527 * is required for non-experimental active artifacts.). This is the 2528 * underlying object with id, value and extensions. The accessor 2529 * "getVersion" gives direct access to the value 2530 */ 2531 public Measure setVersionElement(StringType value) { 2532 this.version = value; 2533 return this; 2534 } 2535 2536 /** 2537 * @return The identifier that is used to identify this version of the measure 2538 * when it is referenced in a specification, model, design or instance. 2539 * This is an arbitrary value managed by the measure author and is not 2540 * expected to be globally unique. For example, it might be a timestamp 2541 * (e.g. yyyymmdd) if a managed version is not available. There is also 2542 * no expectation that versions can be placed in a lexicographical 2543 * sequence. To provide a version consistent with the Decision Support 2544 * Service specification, use the format Major.Minor.Revision (e.g. 2545 * 1.0.0). For more information on versioning knowledge assets, refer to 2546 * the Decision Support Service specification. Note that a version is 2547 * required for non-experimental active artifacts. 2548 */ 2549 public String getVersion() { 2550 return this.version == null ? null : this.version.getValue(); 2551 } 2552 2553 /** 2554 * @param value The identifier that is used to identify this version of the 2555 * measure when it is referenced in a specification, model, design 2556 * or instance. This is an arbitrary value managed by the measure 2557 * author and is not expected to be globally unique. For example, 2558 * it might be a timestamp (e.g. yyyymmdd) if a managed version is 2559 * not available. There is also no expectation that versions can be 2560 * placed in a lexicographical sequence. To provide a version 2561 * consistent with the Decision Support Service specification, use 2562 * the format Major.Minor.Revision (e.g. 1.0.0). For more 2563 * information on versioning knowledge assets, refer to the 2564 * Decision Support Service specification. Note that a version is 2565 * required for non-experimental active artifacts. 2566 */ 2567 public Measure setVersion(String value) { 2568 if (Utilities.noString(value)) 2569 this.version = null; 2570 else { 2571 if (this.version == null) 2572 this.version = new StringType(); 2573 this.version.setValue(value); 2574 } 2575 return this; 2576 } 2577 2578 /** 2579 * @return {@link #name} (A natural language name identifying the measure. This 2580 * name should be usable as an identifier for the module by machine 2581 * processing applications such as code generation.). This is the 2582 * underlying object with id, value and extensions. The accessor 2583 * "getName" gives direct access to the value 2584 */ 2585 public StringType getNameElement() { 2586 if (this.name == null) 2587 if (Configuration.errorOnAutoCreate()) 2588 throw new Error("Attempt to auto-create Measure.name"); 2589 else if (Configuration.doAutoCreate()) 2590 this.name = new StringType(); // bb 2591 return this.name; 2592 } 2593 2594 public boolean hasNameElement() { 2595 return this.name != null && !this.name.isEmpty(); 2596 } 2597 2598 public boolean hasName() { 2599 return this.name != null && !this.name.isEmpty(); 2600 } 2601 2602 /** 2603 * @param value {@link #name} (A natural language name identifying the measure. 2604 * This name should be usable as an identifier for the module by 2605 * machine processing applications such as code generation.). This 2606 * is the underlying object with id, value and extensions. The 2607 * accessor "getName" gives direct access to the value 2608 */ 2609 public Measure setNameElement(StringType value) { 2610 this.name = value; 2611 return this; 2612 } 2613 2614 /** 2615 * @return A natural language name identifying the measure. This name should be 2616 * usable as an identifier for the module by machine processing 2617 * applications such as code generation. 2618 */ 2619 public String getName() { 2620 return this.name == null ? null : this.name.getValue(); 2621 } 2622 2623 /** 2624 * @param value A natural language name identifying the measure. This name 2625 * should be usable as an identifier for the module by machine 2626 * processing applications such as code generation. 2627 */ 2628 public Measure setName(String value) { 2629 if (Utilities.noString(value)) 2630 this.name = null; 2631 else { 2632 if (this.name == null) 2633 this.name = new StringType(); 2634 this.name.setValue(value); 2635 } 2636 return this; 2637 } 2638 2639 /** 2640 * @return {@link #title} (A short, descriptive, user-friendly title for the 2641 * measure.). This is the underlying object with id, value and 2642 * extensions. The accessor "getTitle" gives direct access to the value 2643 */ 2644 public StringType getTitleElement() { 2645 if (this.title == null) 2646 if (Configuration.errorOnAutoCreate()) 2647 throw new Error("Attempt to auto-create Measure.title"); 2648 else if (Configuration.doAutoCreate()) 2649 this.title = new StringType(); // bb 2650 return this.title; 2651 } 2652 2653 public boolean hasTitleElement() { 2654 return this.title != null && !this.title.isEmpty(); 2655 } 2656 2657 public boolean hasTitle() { 2658 return this.title != null && !this.title.isEmpty(); 2659 } 2660 2661 /** 2662 * @param value {@link #title} (A short, descriptive, user-friendly title for 2663 * the measure.). This is the underlying object with id, value and 2664 * extensions. The accessor "getTitle" gives direct access to the 2665 * value 2666 */ 2667 public Measure setTitleElement(StringType value) { 2668 this.title = value; 2669 return this; 2670 } 2671 2672 /** 2673 * @return A short, descriptive, user-friendly title for the measure. 2674 */ 2675 public String getTitle() { 2676 return this.title == null ? null : this.title.getValue(); 2677 } 2678 2679 /** 2680 * @param value A short, descriptive, user-friendly title for the measure. 2681 */ 2682 public Measure setTitle(String value) { 2683 if (Utilities.noString(value)) 2684 this.title = null; 2685 else { 2686 if (this.title == null) 2687 this.title = new StringType(); 2688 this.title.setValue(value); 2689 } 2690 return this; 2691 } 2692 2693 /** 2694 * @return {@link #subtitle} (An explanatory or alternate title for the measure 2695 * giving additional information about its content.). This is the 2696 * underlying object with id, value and extensions. The accessor 2697 * "getSubtitle" gives direct access to the value 2698 */ 2699 public StringType getSubtitleElement() { 2700 if (this.subtitle == null) 2701 if (Configuration.errorOnAutoCreate()) 2702 throw new Error("Attempt to auto-create Measure.subtitle"); 2703 else if (Configuration.doAutoCreate()) 2704 this.subtitle = new StringType(); // bb 2705 return this.subtitle; 2706 } 2707 2708 public boolean hasSubtitleElement() { 2709 return this.subtitle != null && !this.subtitle.isEmpty(); 2710 } 2711 2712 public boolean hasSubtitle() { 2713 return this.subtitle != null && !this.subtitle.isEmpty(); 2714 } 2715 2716 /** 2717 * @param value {@link #subtitle} (An explanatory or alternate title for the 2718 * measure giving additional information about its content.). This 2719 * is the underlying object with id, value and extensions. The 2720 * accessor "getSubtitle" gives direct access to the value 2721 */ 2722 public Measure setSubtitleElement(StringType value) { 2723 this.subtitle = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return An explanatory or alternate title for the measure giving additional 2729 * information about its content. 2730 */ 2731 public String getSubtitle() { 2732 return this.subtitle == null ? null : this.subtitle.getValue(); 2733 } 2734 2735 /** 2736 * @param value An explanatory or alternate title for the measure giving 2737 * additional information about its content. 2738 */ 2739 public Measure setSubtitle(String value) { 2740 if (Utilities.noString(value)) 2741 this.subtitle = null; 2742 else { 2743 if (this.subtitle == null) 2744 this.subtitle = new StringType(); 2745 this.subtitle.setValue(value); 2746 } 2747 return this; 2748 } 2749 2750 /** 2751 * @return {@link #status} (The status of this measure. Enables tracking the 2752 * life-cycle of the content.). This is the underlying object with id, 2753 * value and extensions. The accessor "getStatus" gives direct access to 2754 * the value 2755 */ 2756 public Enumeration<PublicationStatus> getStatusElement() { 2757 if (this.status == null) 2758 if (Configuration.errorOnAutoCreate()) 2759 throw new Error("Attempt to auto-create Measure.status"); 2760 else if (Configuration.doAutoCreate()) 2761 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2762 return this.status; 2763 } 2764 2765 public boolean hasStatusElement() { 2766 return this.status != null && !this.status.isEmpty(); 2767 } 2768 2769 public boolean hasStatus() { 2770 return this.status != null && !this.status.isEmpty(); 2771 } 2772 2773 /** 2774 * @param value {@link #status} (The status of this measure. Enables tracking 2775 * the life-cycle of the content.). This is the underlying object 2776 * with id, value and extensions. The accessor "getStatus" gives 2777 * direct access to the value 2778 */ 2779 public Measure setStatusElement(Enumeration<PublicationStatus> value) { 2780 this.status = value; 2781 return this; 2782 } 2783 2784 /** 2785 * @return The status of this measure. Enables tracking the life-cycle of the 2786 * content. 2787 */ 2788 public PublicationStatus getStatus() { 2789 return this.status == null ? null : this.status.getValue(); 2790 } 2791 2792 /** 2793 * @param value The status of this measure. Enables tracking the life-cycle of 2794 * the content. 2795 */ 2796 public Measure setStatus(PublicationStatus value) { 2797 if (this.status == null) 2798 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2799 this.status.setValue(value); 2800 return this; 2801 } 2802 2803 /** 2804 * @return {@link #experimental} (A Boolean value to indicate that this measure 2805 * is authored for testing purposes (or education/evaluation/marketing) 2806 * and is not intended to be used for genuine usage.). This is the 2807 * underlying object with id, value and extensions. The accessor 2808 * "getExperimental" gives direct access to the value 2809 */ 2810 public BooleanType getExperimentalElement() { 2811 if (this.experimental == null) 2812 if (Configuration.errorOnAutoCreate()) 2813 throw new Error("Attempt to auto-create Measure.experimental"); 2814 else if (Configuration.doAutoCreate()) 2815 this.experimental = new BooleanType(); // bb 2816 return this.experimental; 2817 } 2818 2819 public boolean hasExperimentalElement() { 2820 return this.experimental != null && !this.experimental.isEmpty(); 2821 } 2822 2823 public boolean hasExperimental() { 2824 return this.experimental != null && !this.experimental.isEmpty(); 2825 } 2826 2827 /** 2828 * @param value {@link #experimental} (A Boolean value to indicate that this 2829 * measure is authored for testing purposes (or 2830 * education/evaluation/marketing) and is not intended to be used 2831 * for genuine usage.). This is the underlying object with id, 2832 * value and extensions. The accessor "getExperimental" gives 2833 * direct access to the value 2834 */ 2835 public Measure setExperimentalElement(BooleanType value) { 2836 this.experimental = value; 2837 return this; 2838 } 2839 2840 /** 2841 * @return A Boolean value to indicate that this measure is authored for testing 2842 * purposes (or education/evaluation/marketing) and is not intended to 2843 * be used for genuine usage. 2844 */ 2845 public boolean getExperimental() { 2846 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2847 } 2848 2849 /** 2850 * @param value A Boolean value to indicate that this measure is authored for 2851 * testing purposes (or education/evaluation/marketing) and is not 2852 * intended to be used for genuine usage. 2853 */ 2854 public Measure setExperimental(boolean value) { 2855 if (this.experimental == null) 2856 this.experimental = new BooleanType(); 2857 this.experimental.setValue(value); 2858 return this; 2859 } 2860 2861 /** 2862 * @return {@link #subject} (The intended subjects for the measure. If this 2863 * element is not provided, a Patient subject is assumed, but the 2864 * subject of the measure can be anything.) 2865 */ 2866 public Type getSubject() { 2867 return this.subject; 2868 } 2869 2870 /** 2871 * @return {@link #subject} (The intended subjects for the measure. If this 2872 * element is not provided, a Patient subject is assumed, but the 2873 * subject of the measure can be anything.) 2874 */ 2875 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 2876 if (this.subject == null) 2877 this.subject = new CodeableConcept(); 2878 if (!(this.subject instanceof CodeableConcept)) 2879 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2880 + this.subject.getClass().getName() + " was encountered"); 2881 return (CodeableConcept) this.subject; 2882 } 2883 2884 public boolean hasSubjectCodeableConcept() { 2885 return this != null && this.subject instanceof CodeableConcept; 2886 } 2887 2888 /** 2889 * @return {@link #subject} (The intended subjects for the measure. If this 2890 * element is not provided, a Patient subject is assumed, but the 2891 * subject of the measure can be anything.) 2892 */ 2893 public Reference getSubjectReference() throws FHIRException { 2894 if (this.subject == null) 2895 this.subject = new Reference(); 2896 if (!(this.subject instanceof Reference)) 2897 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 2898 + " was encountered"); 2899 return (Reference) this.subject; 2900 } 2901 2902 public boolean hasSubjectReference() { 2903 return this != null && this.subject instanceof Reference; 2904 } 2905 2906 public boolean hasSubject() { 2907 return this.subject != null && !this.subject.isEmpty(); 2908 } 2909 2910 /** 2911 * @param value {@link #subject} (The intended subjects for the measure. If this 2912 * element is not provided, a Patient subject is assumed, but the 2913 * subject of the measure can be anything.) 2914 */ 2915 public Measure setSubject(Type value) { 2916 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2917 throw new Error("Not the right type for Measure.subject[x]: " + value.fhirType()); 2918 this.subject = value; 2919 return this; 2920 } 2921 2922 /** 2923 * @return {@link #date} (The date (and optionally time) when the measure was 2924 * published. The date must change when the business version changes and 2925 * it must change if the status code changes. In addition, it should 2926 * change when the substantive content of the measure changes.). This is 2927 * the underlying object with id, value and extensions. The accessor 2928 * "getDate" gives direct access to the value 2929 */ 2930 public DateTimeType getDateElement() { 2931 if (this.date == null) 2932 if (Configuration.errorOnAutoCreate()) 2933 throw new Error("Attempt to auto-create Measure.date"); 2934 else if (Configuration.doAutoCreate()) 2935 this.date = new DateTimeType(); // bb 2936 return this.date; 2937 } 2938 2939 public boolean hasDateElement() { 2940 return this.date != null && !this.date.isEmpty(); 2941 } 2942 2943 public boolean hasDate() { 2944 return this.date != null && !this.date.isEmpty(); 2945 } 2946 2947 /** 2948 * @param value {@link #date} (The date (and optionally time) when the measure 2949 * was published. The date must change when the business version 2950 * changes and it must change if the status code changes. In 2951 * addition, it should change when the substantive content of the 2952 * measure changes.). This is the underlying object with id, value 2953 * and extensions. The accessor "getDate" gives direct access to 2954 * the value 2955 */ 2956 public Measure setDateElement(DateTimeType value) { 2957 this.date = value; 2958 return this; 2959 } 2960 2961 /** 2962 * @return The date (and optionally time) when the measure was published. The 2963 * date must change when the business version changes and it must change 2964 * if the status code changes. In addition, it should change when the 2965 * substantive content of the measure changes. 2966 */ 2967 public Date getDate() { 2968 return this.date == null ? null : this.date.getValue(); 2969 } 2970 2971 /** 2972 * @param value The date (and optionally time) when the measure was published. 2973 * The date must change when the business version changes and it 2974 * must change if the status code changes. In addition, it should 2975 * change when the substantive content of the measure changes. 2976 */ 2977 public Measure setDate(Date value) { 2978 if (value == null) 2979 this.date = null; 2980 else { 2981 if (this.date == null) 2982 this.date = new DateTimeType(); 2983 this.date.setValue(value); 2984 } 2985 return this; 2986 } 2987 2988 /** 2989 * @return {@link #publisher} (The name of the organization or individual that 2990 * published the measure.). This is the underlying object with id, value 2991 * and extensions. The accessor "getPublisher" gives direct access to 2992 * the value 2993 */ 2994 public StringType getPublisherElement() { 2995 if (this.publisher == null) 2996 if (Configuration.errorOnAutoCreate()) 2997 throw new Error("Attempt to auto-create Measure.publisher"); 2998 else if (Configuration.doAutoCreate()) 2999 this.publisher = new StringType(); // bb 3000 return this.publisher; 3001 } 3002 3003 public boolean hasPublisherElement() { 3004 return this.publisher != null && !this.publisher.isEmpty(); 3005 } 3006 3007 public boolean hasPublisher() { 3008 return this.publisher != null && !this.publisher.isEmpty(); 3009 } 3010 3011 /** 3012 * @param value {@link #publisher} (The name of the organization or individual 3013 * that published the measure.). This is the underlying object with 3014 * id, value and extensions. The accessor "getPublisher" gives 3015 * direct access to the value 3016 */ 3017 public Measure setPublisherElement(StringType value) { 3018 this.publisher = value; 3019 return this; 3020 } 3021 3022 /** 3023 * @return The name of the organization or individual that published the 3024 * measure. 3025 */ 3026 public String getPublisher() { 3027 return this.publisher == null ? null : this.publisher.getValue(); 3028 } 3029 3030 /** 3031 * @param value The name of the organization or individual that published the 3032 * measure. 3033 */ 3034 public Measure setPublisher(String value) { 3035 if (Utilities.noString(value)) 3036 this.publisher = null; 3037 else { 3038 if (this.publisher == null) 3039 this.publisher = new StringType(); 3040 this.publisher.setValue(value); 3041 } 3042 return this; 3043 } 3044 3045 /** 3046 * @return {@link #contact} (Contact details to assist a user in finding and 3047 * communicating with the publisher.) 3048 */ 3049 public List<ContactDetail> getContact() { 3050 if (this.contact == null) 3051 this.contact = new ArrayList<ContactDetail>(); 3052 return this.contact; 3053 } 3054 3055 /** 3056 * @return Returns a reference to <code>this</code> for easy method chaining 3057 */ 3058 public Measure setContact(List<ContactDetail> theContact) { 3059 this.contact = theContact; 3060 return this; 3061 } 3062 3063 public boolean hasContact() { 3064 if (this.contact == null) 3065 return false; 3066 for (ContactDetail item : this.contact) 3067 if (!item.isEmpty()) 3068 return true; 3069 return false; 3070 } 3071 3072 public ContactDetail addContact() { // 3 3073 ContactDetail t = new ContactDetail(); 3074 if (this.contact == null) 3075 this.contact = new ArrayList<ContactDetail>(); 3076 this.contact.add(t); 3077 return t; 3078 } 3079 3080 public Measure addContact(ContactDetail t) { // 3 3081 if (t == null) 3082 return this; 3083 if (this.contact == null) 3084 this.contact = new ArrayList<ContactDetail>(); 3085 this.contact.add(t); 3086 return this; 3087 } 3088 3089 /** 3090 * @return The first repetition of repeating field {@link #contact}, creating it 3091 * if it does not already exist 3092 */ 3093 public ContactDetail getContactFirstRep() { 3094 if (getContact().isEmpty()) { 3095 addContact(); 3096 } 3097 return getContact().get(0); 3098 } 3099 3100 /** 3101 * @return {@link #description} (A free text natural language description of the 3102 * measure from a consumer's perspective.). This is the underlying 3103 * object with id, value and extensions. The accessor "getDescription" 3104 * gives direct access to the value 3105 */ 3106 public MarkdownType getDescriptionElement() { 3107 if (this.description == null) 3108 if (Configuration.errorOnAutoCreate()) 3109 throw new Error("Attempt to auto-create Measure.description"); 3110 else if (Configuration.doAutoCreate()) 3111 this.description = new MarkdownType(); // bb 3112 return this.description; 3113 } 3114 3115 public boolean hasDescriptionElement() { 3116 return this.description != null && !this.description.isEmpty(); 3117 } 3118 3119 public boolean hasDescription() { 3120 return this.description != null && !this.description.isEmpty(); 3121 } 3122 3123 /** 3124 * @param value {@link #description} (A free text natural language description 3125 * of the measure from a consumer's perspective.). This is the 3126 * underlying object with id, value and extensions. The accessor 3127 * "getDescription" gives direct access to the value 3128 */ 3129 public Measure setDescriptionElement(MarkdownType value) { 3130 this.description = value; 3131 return this; 3132 } 3133 3134 /** 3135 * @return A free text natural language description of the measure from a 3136 * consumer's perspective. 3137 */ 3138 public String getDescription() { 3139 return this.description == null ? null : this.description.getValue(); 3140 } 3141 3142 /** 3143 * @param value A free text natural language description of the measure from a 3144 * consumer's perspective. 3145 */ 3146 public Measure setDescription(String value) { 3147 if (value == null) 3148 this.description = null; 3149 else { 3150 if (this.description == null) 3151 this.description = new MarkdownType(); 3152 this.description.setValue(value); 3153 } 3154 return this; 3155 } 3156 3157 /** 3158 * @return {@link #useContext} (The content was developed with a focus and 3159 * intent of supporting the contexts that are listed. These contexts may 3160 * be general categories (gender, age, ...) or may be references to 3161 * specific programs (insurance plans, studies, ...) and may be used to 3162 * assist with indexing and searching for appropriate measure 3163 * instances.) 3164 */ 3165 public List<UsageContext> getUseContext() { 3166 if (this.useContext == null) 3167 this.useContext = new ArrayList<UsageContext>(); 3168 return this.useContext; 3169 } 3170 3171 /** 3172 * @return Returns a reference to <code>this</code> for easy method chaining 3173 */ 3174 public Measure setUseContext(List<UsageContext> theUseContext) { 3175 this.useContext = theUseContext; 3176 return this; 3177 } 3178 3179 public boolean hasUseContext() { 3180 if (this.useContext == null) 3181 return false; 3182 for (UsageContext item : this.useContext) 3183 if (!item.isEmpty()) 3184 return true; 3185 return false; 3186 } 3187 3188 public UsageContext addUseContext() { // 3 3189 UsageContext t = new UsageContext(); 3190 if (this.useContext == null) 3191 this.useContext = new ArrayList<UsageContext>(); 3192 this.useContext.add(t); 3193 return t; 3194 } 3195 3196 public Measure addUseContext(UsageContext t) { // 3 3197 if (t == null) 3198 return this; 3199 if (this.useContext == null) 3200 this.useContext = new ArrayList<UsageContext>(); 3201 this.useContext.add(t); 3202 return this; 3203 } 3204 3205 /** 3206 * @return The first repetition of repeating field {@link #useContext}, creating 3207 * it if it does not already exist 3208 */ 3209 public UsageContext getUseContextFirstRep() { 3210 if (getUseContext().isEmpty()) { 3211 addUseContext(); 3212 } 3213 return getUseContext().get(0); 3214 } 3215 3216 /** 3217 * @return {@link #jurisdiction} (A legal or geographic region in which the 3218 * measure is intended to be used.) 3219 */ 3220 public List<CodeableConcept> getJurisdiction() { 3221 if (this.jurisdiction == null) 3222 this.jurisdiction = new ArrayList<CodeableConcept>(); 3223 return this.jurisdiction; 3224 } 3225 3226 /** 3227 * @return Returns a reference to <code>this</code> for easy method chaining 3228 */ 3229 public Measure setJurisdiction(List<CodeableConcept> theJurisdiction) { 3230 this.jurisdiction = theJurisdiction; 3231 return this; 3232 } 3233 3234 public boolean hasJurisdiction() { 3235 if (this.jurisdiction == null) 3236 return false; 3237 for (CodeableConcept item : this.jurisdiction) 3238 if (!item.isEmpty()) 3239 return true; 3240 return false; 3241 } 3242 3243 public CodeableConcept addJurisdiction() { // 3 3244 CodeableConcept t = new CodeableConcept(); 3245 if (this.jurisdiction == null) 3246 this.jurisdiction = new ArrayList<CodeableConcept>(); 3247 this.jurisdiction.add(t); 3248 return t; 3249 } 3250 3251 public Measure addJurisdiction(CodeableConcept t) { // 3 3252 if (t == null) 3253 return this; 3254 if (this.jurisdiction == null) 3255 this.jurisdiction = new ArrayList<CodeableConcept>(); 3256 this.jurisdiction.add(t); 3257 return this; 3258 } 3259 3260 /** 3261 * @return The first repetition of repeating field {@link #jurisdiction}, 3262 * creating it if it does not already exist 3263 */ 3264 public CodeableConcept getJurisdictionFirstRep() { 3265 if (getJurisdiction().isEmpty()) { 3266 addJurisdiction(); 3267 } 3268 return getJurisdiction().get(0); 3269 } 3270 3271 /** 3272 * @return {@link #purpose} (Explanation of why this measure is needed and why 3273 * it has been designed as it has.). This is the underlying object with 3274 * id, value and extensions. The accessor "getPurpose" gives direct 3275 * access to the value 3276 */ 3277 public MarkdownType getPurposeElement() { 3278 if (this.purpose == null) 3279 if (Configuration.errorOnAutoCreate()) 3280 throw new Error("Attempt to auto-create Measure.purpose"); 3281 else if (Configuration.doAutoCreate()) 3282 this.purpose = new MarkdownType(); // bb 3283 return this.purpose; 3284 } 3285 3286 public boolean hasPurposeElement() { 3287 return this.purpose != null && !this.purpose.isEmpty(); 3288 } 3289 3290 public boolean hasPurpose() { 3291 return this.purpose != null && !this.purpose.isEmpty(); 3292 } 3293 3294 /** 3295 * @param value {@link #purpose} (Explanation of why this measure is needed and 3296 * why it has been designed as it has.). This is the underlying 3297 * object with id, value and extensions. The accessor "getPurpose" 3298 * gives direct access to the value 3299 */ 3300 public Measure setPurposeElement(MarkdownType value) { 3301 this.purpose = value; 3302 return this; 3303 } 3304 3305 /** 3306 * @return Explanation of why this measure is needed and why it has been 3307 * designed as it has. 3308 */ 3309 public String getPurpose() { 3310 return this.purpose == null ? null : this.purpose.getValue(); 3311 } 3312 3313 /** 3314 * @param value Explanation of why this measure is needed and why it has been 3315 * designed as it has. 3316 */ 3317 public Measure setPurpose(String value) { 3318 if (value == null) 3319 this.purpose = null; 3320 else { 3321 if (this.purpose == null) 3322 this.purpose = new MarkdownType(); 3323 this.purpose.setValue(value); 3324 } 3325 return this; 3326 } 3327 3328 /** 3329 * @return {@link #usage} (A detailed description, from a clinical perspective, 3330 * of how the measure is used.). This is the underlying object with id, 3331 * value and extensions. The accessor "getUsage" gives direct access to 3332 * the value 3333 */ 3334 public StringType getUsageElement() { 3335 if (this.usage == null) 3336 if (Configuration.errorOnAutoCreate()) 3337 throw new Error("Attempt to auto-create Measure.usage"); 3338 else if (Configuration.doAutoCreate()) 3339 this.usage = new StringType(); // bb 3340 return this.usage; 3341 } 3342 3343 public boolean hasUsageElement() { 3344 return this.usage != null && !this.usage.isEmpty(); 3345 } 3346 3347 public boolean hasUsage() { 3348 return this.usage != null && !this.usage.isEmpty(); 3349 } 3350 3351 /** 3352 * @param value {@link #usage} (A detailed description, from a clinical 3353 * perspective, of how the measure is used.). This is the 3354 * underlying object with id, value and extensions. The accessor 3355 * "getUsage" gives direct access to the value 3356 */ 3357 public Measure setUsageElement(StringType value) { 3358 this.usage = value; 3359 return this; 3360 } 3361 3362 /** 3363 * @return A detailed description, from a clinical perspective, of how the 3364 * measure is used. 3365 */ 3366 public String getUsage() { 3367 return this.usage == null ? null : this.usage.getValue(); 3368 } 3369 3370 /** 3371 * @param value A detailed description, from a clinical perspective, of how the 3372 * measure is used. 3373 */ 3374 public Measure setUsage(String value) { 3375 if (Utilities.noString(value)) 3376 this.usage = null; 3377 else { 3378 if (this.usage == null) 3379 this.usage = new StringType(); 3380 this.usage.setValue(value); 3381 } 3382 return this; 3383 } 3384 3385 /** 3386 * @return {@link #copyright} (A copyright statement relating to the measure 3387 * and/or its contents. Copyright statements are generally legal 3388 * restrictions on the use and publishing of the measure.). This is the 3389 * underlying object with id, value and extensions. The accessor 3390 * "getCopyright" gives direct access to the value 3391 */ 3392 public MarkdownType getCopyrightElement() { 3393 if (this.copyright == null) 3394 if (Configuration.errorOnAutoCreate()) 3395 throw new Error("Attempt to auto-create Measure.copyright"); 3396 else if (Configuration.doAutoCreate()) 3397 this.copyright = new MarkdownType(); // bb 3398 return this.copyright; 3399 } 3400 3401 public boolean hasCopyrightElement() { 3402 return this.copyright != null && !this.copyright.isEmpty(); 3403 } 3404 3405 public boolean hasCopyright() { 3406 return this.copyright != null && !this.copyright.isEmpty(); 3407 } 3408 3409 /** 3410 * @param value {@link #copyright} (A copyright statement relating to the 3411 * measure and/or its contents. Copyright statements are generally 3412 * legal restrictions on the use and publishing of the measure.). 3413 * This is the underlying object with id, value and extensions. The 3414 * accessor "getCopyright" gives direct access to the value 3415 */ 3416 public Measure setCopyrightElement(MarkdownType value) { 3417 this.copyright = value; 3418 return this; 3419 } 3420 3421 /** 3422 * @return A copyright statement relating to the measure and/or its contents. 3423 * Copyright statements are generally legal restrictions on the use and 3424 * publishing of the measure. 3425 */ 3426 public String getCopyright() { 3427 return this.copyright == null ? null : this.copyright.getValue(); 3428 } 3429 3430 /** 3431 * @param value A copyright statement relating to the measure and/or its 3432 * contents. Copyright statements are generally legal restrictions 3433 * on the use and publishing of the measure. 3434 */ 3435 public Measure setCopyright(String value) { 3436 if (value == null) 3437 this.copyright = null; 3438 else { 3439 if (this.copyright == null) 3440 this.copyright = new MarkdownType(); 3441 this.copyright.setValue(value); 3442 } 3443 return this; 3444 } 3445 3446 /** 3447 * @return {@link #approvalDate} (The date on which the resource content was 3448 * approved by the publisher. Approval happens once when the content is 3449 * officially approved for usage.). This is the underlying object with 3450 * id, value and extensions. The accessor "getApprovalDate" gives direct 3451 * access to the value 3452 */ 3453 public DateType getApprovalDateElement() { 3454 if (this.approvalDate == null) 3455 if (Configuration.errorOnAutoCreate()) 3456 throw new Error("Attempt to auto-create Measure.approvalDate"); 3457 else if (Configuration.doAutoCreate()) 3458 this.approvalDate = new DateType(); // bb 3459 return this.approvalDate; 3460 } 3461 3462 public boolean hasApprovalDateElement() { 3463 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3464 } 3465 3466 public boolean hasApprovalDate() { 3467 return this.approvalDate != null && !this.approvalDate.isEmpty(); 3468 } 3469 3470 /** 3471 * @param value {@link #approvalDate} (The date on which the resource content 3472 * was approved by the publisher. Approval happens once when the 3473 * content is officially approved for usage.). This is the 3474 * underlying object with id, value and extensions. The accessor 3475 * "getApprovalDate" gives direct access to the value 3476 */ 3477 public Measure setApprovalDateElement(DateType value) { 3478 this.approvalDate = value; 3479 return this; 3480 } 3481 3482 /** 3483 * @return The date on which the resource content was approved by the publisher. 3484 * Approval happens once when the content is officially approved for 3485 * usage. 3486 */ 3487 public Date getApprovalDate() { 3488 return this.approvalDate == null ? null : this.approvalDate.getValue(); 3489 } 3490 3491 /** 3492 * @param value The date on which the resource content was approved by the 3493 * publisher. Approval happens once when the content is officially 3494 * approved for usage. 3495 */ 3496 public Measure setApprovalDate(Date value) { 3497 if (value == null) 3498 this.approvalDate = null; 3499 else { 3500 if (this.approvalDate == null) 3501 this.approvalDate = new DateType(); 3502 this.approvalDate.setValue(value); 3503 } 3504 return this; 3505 } 3506 3507 /** 3508 * @return {@link #lastReviewDate} (The date on which the resource content was 3509 * last reviewed. Review happens periodically after approval but does 3510 * not change the original approval date.). This is the underlying 3511 * object with id, value and extensions. The accessor 3512 * "getLastReviewDate" gives direct access to the value 3513 */ 3514 public DateType getLastReviewDateElement() { 3515 if (this.lastReviewDate == null) 3516 if (Configuration.errorOnAutoCreate()) 3517 throw new Error("Attempt to auto-create Measure.lastReviewDate"); 3518 else if (Configuration.doAutoCreate()) 3519 this.lastReviewDate = new DateType(); // bb 3520 return this.lastReviewDate; 3521 } 3522 3523 public boolean hasLastReviewDateElement() { 3524 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3525 } 3526 3527 public boolean hasLastReviewDate() { 3528 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 3529 } 3530 3531 /** 3532 * @param value {@link #lastReviewDate} (The date on which the resource content 3533 * was last reviewed. Review happens periodically after approval 3534 * but does not change the original approval date.). This is the 3535 * underlying object with id, value and extensions. The accessor 3536 * "getLastReviewDate" gives direct access to the value 3537 */ 3538 public Measure setLastReviewDateElement(DateType value) { 3539 this.lastReviewDate = value; 3540 return this; 3541 } 3542 3543 /** 3544 * @return The date on which the resource content was last reviewed. Review 3545 * happens periodically after approval but does not change the original 3546 * approval date. 3547 */ 3548 public Date getLastReviewDate() { 3549 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 3550 } 3551 3552 /** 3553 * @param value The date on which the resource content was last reviewed. Review 3554 * happens periodically after approval but does not change the 3555 * original approval date. 3556 */ 3557 public Measure setLastReviewDate(Date value) { 3558 if (value == null) 3559 this.lastReviewDate = null; 3560 else { 3561 if (this.lastReviewDate == null) 3562 this.lastReviewDate = new DateType(); 3563 this.lastReviewDate.setValue(value); 3564 } 3565 return this; 3566 } 3567 3568 /** 3569 * @return {@link #effectivePeriod} (The period during which the measure content 3570 * was or is planned to be in active use.) 3571 */ 3572 public Period getEffectivePeriod() { 3573 if (this.effectivePeriod == null) 3574 if (Configuration.errorOnAutoCreate()) 3575 throw new Error("Attempt to auto-create Measure.effectivePeriod"); 3576 else if (Configuration.doAutoCreate()) 3577 this.effectivePeriod = new Period(); // cc 3578 return this.effectivePeriod; 3579 } 3580 3581 public boolean hasEffectivePeriod() { 3582 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 3583 } 3584 3585 /** 3586 * @param value {@link #effectivePeriod} (The period during which the measure 3587 * content was or is planned to be in active use.) 3588 */ 3589 public Measure setEffectivePeriod(Period value) { 3590 this.effectivePeriod = value; 3591 return this; 3592 } 3593 3594 /** 3595 * @return {@link #topic} (Descriptive topics related to the content of the 3596 * measure. Topics provide a high-level categorization grouping types of 3597 * measures that can be useful for filtering and searching.) 3598 */ 3599 public List<CodeableConcept> getTopic() { 3600 if (this.topic == null) 3601 this.topic = new ArrayList<CodeableConcept>(); 3602 return this.topic; 3603 } 3604 3605 /** 3606 * @return Returns a reference to <code>this</code> for easy method chaining 3607 */ 3608 public Measure setTopic(List<CodeableConcept> theTopic) { 3609 this.topic = theTopic; 3610 return this; 3611 } 3612 3613 public boolean hasTopic() { 3614 if (this.topic == null) 3615 return false; 3616 for (CodeableConcept item : this.topic) 3617 if (!item.isEmpty()) 3618 return true; 3619 return false; 3620 } 3621 3622 public CodeableConcept addTopic() { // 3 3623 CodeableConcept t = new CodeableConcept(); 3624 if (this.topic == null) 3625 this.topic = new ArrayList<CodeableConcept>(); 3626 this.topic.add(t); 3627 return t; 3628 } 3629 3630 public Measure addTopic(CodeableConcept t) { // 3 3631 if (t == null) 3632 return this; 3633 if (this.topic == null) 3634 this.topic = new ArrayList<CodeableConcept>(); 3635 this.topic.add(t); 3636 return this; 3637 } 3638 3639 /** 3640 * @return The first repetition of repeating field {@link #topic}, creating it 3641 * if it does not already exist 3642 */ 3643 public CodeableConcept getTopicFirstRep() { 3644 if (getTopic().isEmpty()) { 3645 addTopic(); 3646 } 3647 return getTopic().get(0); 3648 } 3649 3650 /** 3651 * @return {@link #author} (An individiual or organization primarily involved in 3652 * the creation and maintenance of the content.) 3653 */ 3654 public List<ContactDetail> getAuthor() { 3655 if (this.author == null) 3656 this.author = new ArrayList<ContactDetail>(); 3657 return this.author; 3658 } 3659 3660 /** 3661 * @return Returns a reference to <code>this</code> for easy method chaining 3662 */ 3663 public Measure setAuthor(List<ContactDetail> theAuthor) { 3664 this.author = theAuthor; 3665 return this; 3666 } 3667 3668 public boolean hasAuthor() { 3669 if (this.author == null) 3670 return false; 3671 for (ContactDetail item : this.author) 3672 if (!item.isEmpty()) 3673 return true; 3674 return false; 3675 } 3676 3677 public ContactDetail addAuthor() { // 3 3678 ContactDetail t = new ContactDetail(); 3679 if (this.author == null) 3680 this.author = new ArrayList<ContactDetail>(); 3681 this.author.add(t); 3682 return t; 3683 } 3684 3685 public Measure addAuthor(ContactDetail t) { // 3 3686 if (t == null) 3687 return this; 3688 if (this.author == null) 3689 this.author = new ArrayList<ContactDetail>(); 3690 this.author.add(t); 3691 return this; 3692 } 3693 3694 /** 3695 * @return The first repetition of repeating field {@link #author}, creating it 3696 * if it does not already exist 3697 */ 3698 public ContactDetail getAuthorFirstRep() { 3699 if (getAuthor().isEmpty()) { 3700 addAuthor(); 3701 } 3702 return getAuthor().get(0); 3703 } 3704 3705 /** 3706 * @return {@link #editor} (An individual or organization primarily responsible 3707 * for internal coherence of the content.) 3708 */ 3709 public List<ContactDetail> getEditor() { 3710 if (this.editor == null) 3711 this.editor = new ArrayList<ContactDetail>(); 3712 return this.editor; 3713 } 3714 3715 /** 3716 * @return Returns a reference to <code>this</code> for easy method chaining 3717 */ 3718 public Measure setEditor(List<ContactDetail> theEditor) { 3719 this.editor = theEditor; 3720 return this; 3721 } 3722 3723 public boolean hasEditor() { 3724 if (this.editor == null) 3725 return false; 3726 for (ContactDetail item : this.editor) 3727 if (!item.isEmpty()) 3728 return true; 3729 return false; 3730 } 3731 3732 public ContactDetail addEditor() { // 3 3733 ContactDetail t = new ContactDetail(); 3734 if (this.editor == null) 3735 this.editor = new ArrayList<ContactDetail>(); 3736 this.editor.add(t); 3737 return t; 3738 } 3739 3740 public Measure addEditor(ContactDetail t) { // 3 3741 if (t == null) 3742 return this; 3743 if (this.editor == null) 3744 this.editor = new ArrayList<ContactDetail>(); 3745 this.editor.add(t); 3746 return this; 3747 } 3748 3749 /** 3750 * @return The first repetition of repeating field {@link #editor}, creating it 3751 * if it does not already exist 3752 */ 3753 public ContactDetail getEditorFirstRep() { 3754 if (getEditor().isEmpty()) { 3755 addEditor(); 3756 } 3757 return getEditor().get(0); 3758 } 3759 3760 /** 3761 * @return {@link #reviewer} (An individual or organization primarily 3762 * responsible for review of some aspect of the content.) 3763 */ 3764 public List<ContactDetail> getReviewer() { 3765 if (this.reviewer == null) 3766 this.reviewer = new ArrayList<ContactDetail>(); 3767 return this.reviewer; 3768 } 3769 3770 /** 3771 * @return Returns a reference to <code>this</code> for easy method chaining 3772 */ 3773 public Measure setReviewer(List<ContactDetail> theReviewer) { 3774 this.reviewer = theReviewer; 3775 return this; 3776 } 3777 3778 public boolean hasReviewer() { 3779 if (this.reviewer == null) 3780 return false; 3781 for (ContactDetail item : this.reviewer) 3782 if (!item.isEmpty()) 3783 return true; 3784 return false; 3785 } 3786 3787 public ContactDetail addReviewer() { // 3 3788 ContactDetail t = new ContactDetail(); 3789 if (this.reviewer == null) 3790 this.reviewer = new ArrayList<ContactDetail>(); 3791 this.reviewer.add(t); 3792 return t; 3793 } 3794 3795 public Measure addReviewer(ContactDetail t) { // 3 3796 if (t == null) 3797 return this; 3798 if (this.reviewer == null) 3799 this.reviewer = new ArrayList<ContactDetail>(); 3800 this.reviewer.add(t); 3801 return this; 3802 } 3803 3804 /** 3805 * @return The first repetition of repeating field {@link #reviewer}, creating 3806 * it if it does not already exist 3807 */ 3808 public ContactDetail getReviewerFirstRep() { 3809 if (getReviewer().isEmpty()) { 3810 addReviewer(); 3811 } 3812 return getReviewer().get(0); 3813 } 3814 3815 /** 3816 * @return {@link #endorser} (An individual or organization responsible for 3817 * officially endorsing the content for use in some setting.) 3818 */ 3819 public List<ContactDetail> getEndorser() { 3820 if (this.endorser == null) 3821 this.endorser = new ArrayList<ContactDetail>(); 3822 return this.endorser; 3823 } 3824 3825 /** 3826 * @return Returns a reference to <code>this</code> for easy method chaining 3827 */ 3828 public Measure setEndorser(List<ContactDetail> theEndorser) { 3829 this.endorser = theEndorser; 3830 return this; 3831 } 3832 3833 public boolean hasEndorser() { 3834 if (this.endorser == null) 3835 return false; 3836 for (ContactDetail item : this.endorser) 3837 if (!item.isEmpty()) 3838 return true; 3839 return false; 3840 } 3841 3842 public ContactDetail addEndorser() { // 3 3843 ContactDetail t = new ContactDetail(); 3844 if (this.endorser == null) 3845 this.endorser = new ArrayList<ContactDetail>(); 3846 this.endorser.add(t); 3847 return t; 3848 } 3849 3850 public Measure addEndorser(ContactDetail t) { // 3 3851 if (t == null) 3852 return this; 3853 if (this.endorser == null) 3854 this.endorser = new ArrayList<ContactDetail>(); 3855 this.endorser.add(t); 3856 return this; 3857 } 3858 3859 /** 3860 * @return The first repetition of repeating field {@link #endorser}, creating 3861 * it if it does not already exist 3862 */ 3863 public ContactDetail getEndorserFirstRep() { 3864 if (getEndorser().isEmpty()) { 3865 addEndorser(); 3866 } 3867 return getEndorser().get(0); 3868 } 3869 3870 /** 3871 * @return {@link #relatedArtifact} (Related artifacts such as additional 3872 * documentation, justification, or bibliographic references.) 3873 */ 3874 public List<RelatedArtifact> getRelatedArtifact() { 3875 if (this.relatedArtifact == null) 3876 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3877 return this.relatedArtifact; 3878 } 3879 3880 /** 3881 * @return Returns a reference to <code>this</code> for easy method chaining 3882 */ 3883 public Measure setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 3884 this.relatedArtifact = theRelatedArtifact; 3885 return this; 3886 } 3887 3888 public boolean hasRelatedArtifact() { 3889 if (this.relatedArtifact == null) 3890 return false; 3891 for (RelatedArtifact item : this.relatedArtifact) 3892 if (!item.isEmpty()) 3893 return true; 3894 return false; 3895 } 3896 3897 public RelatedArtifact addRelatedArtifact() { // 3 3898 RelatedArtifact t = new RelatedArtifact(); 3899 if (this.relatedArtifact == null) 3900 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3901 this.relatedArtifact.add(t); 3902 return t; 3903 } 3904 3905 public Measure addRelatedArtifact(RelatedArtifact t) { // 3 3906 if (t == null) 3907 return this; 3908 if (this.relatedArtifact == null) 3909 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 3910 this.relatedArtifact.add(t); 3911 return this; 3912 } 3913 3914 /** 3915 * @return The first repetition of repeating field {@link #relatedArtifact}, 3916 * creating it if it does not already exist 3917 */ 3918 public RelatedArtifact getRelatedArtifactFirstRep() { 3919 if (getRelatedArtifact().isEmpty()) { 3920 addRelatedArtifact(); 3921 } 3922 return getRelatedArtifact().get(0); 3923 } 3924 3925 /** 3926 * @return {@link #library} (A reference to a Library resource containing the 3927 * formal logic used by the measure.) 3928 */ 3929 public List<CanonicalType> getLibrary() { 3930 if (this.library == null) 3931 this.library = new ArrayList<CanonicalType>(); 3932 return this.library; 3933 } 3934 3935 /** 3936 * @return Returns a reference to <code>this</code> for easy method chaining 3937 */ 3938 public Measure setLibrary(List<CanonicalType> theLibrary) { 3939 this.library = theLibrary; 3940 return this; 3941 } 3942 3943 public boolean hasLibrary() { 3944 if (this.library == null) 3945 return false; 3946 for (CanonicalType item : this.library) 3947 if (!item.isEmpty()) 3948 return true; 3949 return false; 3950 } 3951 3952 /** 3953 * @return {@link #library} (A reference to a Library resource containing the 3954 * formal logic used by the measure.) 3955 */ 3956 public CanonicalType addLibraryElement() {// 2 3957 CanonicalType t = new CanonicalType(); 3958 if (this.library == null) 3959 this.library = new ArrayList<CanonicalType>(); 3960 this.library.add(t); 3961 return t; 3962 } 3963 3964 /** 3965 * @param value {@link #library} (A reference to a Library resource containing 3966 * the formal logic used by the measure.) 3967 */ 3968 public Measure addLibrary(String value) { // 1 3969 CanonicalType t = new CanonicalType(); 3970 t.setValue(value); 3971 if (this.library == null) 3972 this.library = new ArrayList<CanonicalType>(); 3973 this.library.add(t); 3974 return this; 3975 } 3976 3977 /** 3978 * @param value {@link #library} (A reference to a Library resource containing 3979 * the formal logic used by the measure.) 3980 */ 3981 public boolean hasLibrary(String value) { 3982 if (this.library == null) 3983 return false; 3984 for (CanonicalType v : this.library) 3985 if (v.getValue().equals(value)) // canonical(Library) 3986 return true; 3987 return false; 3988 } 3989 3990 /** 3991 * @return {@link #disclaimer} (Notices and disclaimers regarding the use of the 3992 * measure or related to intellectual property (such as code systems) 3993 * referenced by the measure.). This is the underlying object with id, 3994 * value and extensions. The accessor "getDisclaimer" gives direct 3995 * access to the value 3996 */ 3997 public MarkdownType getDisclaimerElement() { 3998 if (this.disclaimer == null) 3999 if (Configuration.errorOnAutoCreate()) 4000 throw new Error("Attempt to auto-create Measure.disclaimer"); 4001 else if (Configuration.doAutoCreate()) 4002 this.disclaimer = new MarkdownType(); // bb 4003 return this.disclaimer; 4004 } 4005 4006 public boolean hasDisclaimerElement() { 4007 return this.disclaimer != null && !this.disclaimer.isEmpty(); 4008 } 4009 4010 public boolean hasDisclaimer() { 4011 return this.disclaimer != null && !this.disclaimer.isEmpty(); 4012 } 4013 4014 /** 4015 * @param value {@link #disclaimer} (Notices and disclaimers regarding the use 4016 * of the measure or related to intellectual property (such as code 4017 * systems) referenced by the measure.). This is the underlying 4018 * object with id, value and extensions. The accessor 4019 * "getDisclaimer" gives direct access to the value 4020 */ 4021 public Measure setDisclaimerElement(MarkdownType value) { 4022 this.disclaimer = value; 4023 return this; 4024 } 4025 4026 /** 4027 * @return Notices and disclaimers regarding the use of the measure or related 4028 * to intellectual property (such as code systems) referenced by the 4029 * measure. 4030 */ 4031 public String getDisclaimer() { 4032 return this.disclaimer == null ? null : this.disclaimer.getValue(); 4033 } 4034 4035 /** 4036 * @param value Notices and disclaimers regarding the use of the measure or 4037 * related to intellectual property (such as code systems) 4038 * referenced by the measure. 4039 */ 4040 public Measure setDisclaimer(String value) { 4041 if (value == null) 4042 this.disclaimer = null; 4043 else { 4044 if (this.disclaimer == null) 4045 this.disclaimer = new MarkdownType(); 4046 this.disclaimer.setValue(value); 4047 } 4048 return this; 4049 } 4050 4051 /** 4052 * @return {@link #scoring} (Indicates how the calculation is performed for the 4053 * measure, including proportion, ratio, continuous-variable, and 4054 * cohort. The value set is extensible, allowing additional measure 4055 * scoring types to be represented.) 4056 */ 4057 public CodeableConcept getScoring() { 4058 if (this.scoring == null) 4059 if (Configuration.errorOnAutoCreate()) 4060 throw new Error("Attempt to auto-create Measure.scoring"); 4061 else if (Configuration.doAutoCreate()) 4062 this.scoring = new CodeableConcept(); // cc 4063 return this.scoring; 4064 } 4065 4066 public boolean hasScoring() { 4067 return this.scoring != null && !this.scoring.isEmpty(); 4068 } 4069 4070 /** 4071 * @param value {@link #scoring} (Indicates how the calculation is performed for 4072 * the measure, including proportion, ratio, continuous-variable, 4073 * and cohort. The value set is extensible, allowing additional 4074 * measure scoring types to be represented.) 4075 */ 4076 public Measure setScoring(CodeableConcept value) { 4077 this.scoring = value; 4078 return this; 4079 } 4080 4081 /** 4082 * @return {@link #compositeScoring} (If this is a composite measure, the 4083 * scoring method used to combine the component measures to determine 4084 * the composite score.) 4085 */ 4086 public CodeableConcept getCompositeScoring() { 4087 if (this.compositeScoring == null) 4088 if (Configuration.errorOnAutoCreate()) 4089 throw new Error("Attempt to auto-create Measure.compositeScoring"); 4090 else if (Configuration.doAutoCreate()) 4091 this.compositeScoring = new CodeableConcept(); // cc 4092 return this.compositeScoring; 4093 } 4094 4095 public boolean hasCompositeScoring() { 4096 return this.compositeScoring != null && !this.compositeScoring.isEmpty(); 4097 } 4098 4099 /** 4100 * @param value {@link #compositeScoring} (If this is a composite measure, the 4101 * scoring method used to combine the component measures to 4102 * determine the composite score.) 4103 */ 4104 public Measure setCompositeScoring(CodeableConcept value) { 4105 this.compositeScoring = value; 4106 return this; 4107 } 4108 4109 /** 4110 * @return {@link #type} (Indicates whether the measure is used to examine a 4111 * process, an outcome over time, a patient-reported outcome, or a 4112 * structure measure such as utilization.) 4113 */ 4114 public List<CodeableConcept> getType() { 4115 if (this.type == null) 4116 this.type = new ArrayList<CodeableConcept>(); 4117 return this.type; 4118 } 4119 4120 /** 4121 * @return Returns a reference to <code>this</code> for easy method chaining 4122 */ 4123 public Measure setType(List<CodeableConcept> theType) { 4124 this.type = theType; 4125 return this; 4126 } 4127 4128 public boolean hasType() { 4129 if (this.type == null) 4130 return false; 4131 for (CodeableConcept item : this.type) 4132 if (!item.isEmpty()) 4133 return true; 4134 return false; 4135 } 4136 4137 public CodeableConcept addType() { // 3 4138 CodeableConcept t = new CodeableConcept(); 4139 if (this.type == null) 4140 this.type = new ArrayList<CodeableConcept>(); 4141 this.type.add(t); 4142 return t; 4143 } 4144 4145 public Measure addType(CodeableConcept t) { // 3 4146 if (t == null) 4147 return this; 4148 if (this.type == null) 4149 this.type = new ArrayList<CodeableConcept>(); 4150 this.type.add(t); 4151 return this; 4152 } 4153 4154 /** 4155 * @return The first repetition of repeating field {@link #type}, creating it if 4156 * it does not already exist 4157 */ 4158 public CodeableConcept getTypeFirstRep() { 4159 if (getType().isEmpty()) { 4160 addType(); 4161 } 4162 return getType().get(0); 4163 } 4164 4165 /** 4166 * @return {@link #riskAdjustment} (A description of the risk adjustment factors 4167 * that may impact the resulting score for the measure and how they may 4168 * be accounted for when computing and reporting measure results.). This 4169 * is the underlying object with id, value and extensions. The accessor 4170 * "getRiskAdjustment" gives direct access to the value 4171 */ 4172 public StringType getRiskAdjustmentElement() { 4173 if (this.riskAdjustment == null) 4174 if (Configuration.errorOnAutoCreate()) 4175 throw new Error("Attempt to auto-create Measure.riskAdjustment"); 4176 else if (Configuration.doAutoCreate()) 4177 this.riskAdjustment = new StringType(); // bb 4178 return this.riskAdjustment; 4179 } 4180 4181 public boolean hasRiskAdjustmentElement() { 4182 return this.riskAdjustment != null && !this.riskAdjustment.isEmpty(); 4183 } 4184 4185 public boolean hasRiskAdjustment() { 4186 return this.riskAdjustment != null && !this.riskAdjustment.isEmpty(); 4187 } 4188 4189 /** 4190 * @param value {@link #riskAdjustment} (A description of the risk adjustment 4191 * factors that may impact the resulting score for the measure and 4192 * how they may be accounted for when computing and reporting 4193 * measure results.). This is the underlying object with id, value 4194 * and extensions. The accessor "getRiskAdjustment" gives direct 4195 * access to the value 4196 */ 4197 public Measure setRiskAdjustmentElement(StringType value) { 4198 this.riskAdjustment = value; 4199 return this; 4200 } 4201 4202 /** 4203 * @return A description of the risk adjustment factors that may impact the 4204 * resulting score for the measure and how they may be accounted for 4205 * when computing and reporting measure results. 4206 */ 4207 public String getRiskAdjustment() { 4208 return this.riskAdjustment == null ? null : this.riskAdjustment.getValue(); 4209 } 4210 4211 /** 4212 * @param value A description of the risk adjustment factors that may impact the 4213 * resulting score for the measure and how they may be accounted 4214 * for when computing and reporting measure results. 4215 */ 4216 public Measure setRiskAdjustment(String value) { 4217 if (Utilities.noString(value)) 4218 this.riskAdjustment = null; 4219 else { 4220 if (this.riskAdjustment == null) 4221 this.riskAdjustment = new StringType(); 4222 this.riskAdjustment.setValue(value); 4223 } 4224 return this; 4225 } 4226 4227 /** 4228 * @return {@link #rateAggregation} (Describes how to combine the information 4229 * calculated, based on logic in each of several populations, into one 4230 * summarized result.). This is the underlying object with id, value and 4231 * extensions. The accessor "getRateAggregation" gives direct access to 4232 * the value 4233 */ 4234 public StringType getRateAggregationElement() { 4235 if (this.rateAggregation == null) 4236 if (Configuration.errorOnAutoCreate()) 4237 throw new Error("Attempt to auto-create Measure.rateAggregation"); 4238 else if (Configuration.doAutoCreate()) 4239 this.rateAggregation = new StringType(); // bb 4240 return this.rateAggregation; 4241 } 4242 4243 public boolean hasRateAggregationElement() { 4244 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 4245 } 4246 4247 public boolean hasRateAggregation() { 4248 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 4249 } 4250 4251 /** 4252 * @param value {@link #rateAggregation} (Describes how to combine the 4253 * information calculated, based on logic in each of several 4254 * populations, into one summarized result.). This is the 4255 * underlying object with id, value and extensions. The accessor 4256 * "getRateAggregation" gives direct access to the value 4257 */ 4258 public Measure setRateAggregationElement(StringType value) { 4259 this.rateAggregation = value; 4260 return this; 4261 } 4262 4263 /** 4264 * @return Describes how to combine the information calculated, based on logic 4265 * in each of several populations, into one summarized result. 4266 */ 4267 public String getRateAggregation() { 4268 return this.rateAggregation == null ? null : this.rateAggregation.getValue(); 4269 } 4270 4271 /** 4272 * @param value Describes how to combine the information calculated, based on 4273 * logic in each of several populations, into one summarized 4274 * result. 4275 */ 4276 public Measure setRateAggregation(String value) { 4277 if (Utilities.noString(value)) 4278 this.rateAggregation = null; 4279 else { 4280 if (this.rateAggregation == null) 4281 this.rateAggregation = new StringType(); 4282 this.rateAggregation.setValue(value); 4283 } 4284 return this; 4285 } 4286 4287 /** 4288 * @return {@link #rationale} (Provides a succinct statement of the need for the 4289 * measure. Usually includes statements pertaining to importance 4290 * criterion: impact, gap in care, and evidence.). This is the 4291 * underlying object with id, value and extensions. The accessor 4292 * "getRationale" gives direct access to the value 4293 */ 4294 public MarkdownType getRationaleElement() { 4295 if (this.rationale == null) 4296 if (Configuration.errorOnAutoCreate()) 4297 throw new Error("Attempt to auto-create Measure.rationale"); 4298 else if (Configuration.doAutoCreate()) 4299 this.rationale = new MarkdownType(); // bb 4300 return this.rationale; 4301 } 4302 4303 public boolean hasRationaleElement() { 4304 return this.rationale != null && !this.rationale.isEmpty(); 4305 } 4306 4307 public boolean hasRationale() { 4308 return this.rationale != null && !this.rationale.isEmpty(); 4309 } 4310 4311 /** 4312 * @param value {@link #rationale} (Provides a succinct statement of the need 4313 * for the measure. Usually includes statements pertaining to 4314 * importance criterion: impact, gap in care, and evidence.). This 4315 * is the underlying object with id, value and extensions. The 4316 * accessor "getRationale" gives direct access to the value 4317 */ 4318 public Measure setRationaleElement(MarkdownType value) { 4319 this.rationale = value; 4320 return this; 4321 } 4322 4323 /** 4324 * @return Provides a succinct statement of the need for the measure. Usually 4325 * includes statements pertaining to importance criterion: impact, gap 4326 * in care, and evidence. 4327 */ 4328 public String getRationale() { 4329 return this.rationale == null ? null : this.rationale.getValue(); 4330 } 4331 4332 /** 4333 * @param value Provides a succinct statement of the need for the measure. 4334 * Usually includes statements pertaining to importance criterion: 4335 * impact, gap in care, and evidence. 4336 */ 4337 public Measure setRationale(String value) { 4338 if (value == null) 4339 this.rationale = null; 4340 else { 4341 if (this.rationale == null) 4342 this.rationale = new MarkdownType(); 4343 this.rationale.setValue(value); 4344 } 4345 return this; 4346 } 4347 4348 /** 4349 * @return {@link #clinicalRecommendationStatement} (Provides a summary of 4350 * relevant clinical guidelines or other clinical recommendations 4351 * supporting the measure.). This is the underlying object with id, 4352 * value and extensions. The accessor 4353 * "getClinicalRecommendationStatement" gives direct access to the value 4354 */ 4355 public MarkdownType getClinicalRecommendationStatementElement() { 4356 if (this.clinicalRecommendationStatement == null) 4357 if (Configuration.errorOnAutoCreate()) 4358 throw new Error("Attempt to auto-create Measure.clinicalRecommendationStatement"); 4359 else if (Configuration.doAutoCreate()) 4360 this.clinicalRecommendationStatement = new MarkdownType(); // bb 4361 return this.clinicalRecommendationStatement; 4362 } 4363 4364 public boolean hasClinicalRecommendationStatementElement() { 4365 return this.clinicalRecommendationStatement != null && !this.clinicalRecommendationStatement.isEmpty(); 4366 } 4367 4368 public boolean hasClinicalRecommendationStatement() { 4369 return this.clinicalRecommendationStatement != null && !this.clinicalRecommendationStatement.isEmpty(); 4370 } 4371 4372 /** 4373 * @param value {@link #clinicalRecommendationStatement} (Provides a summary of 4374 * relevant clinical guidelines or other clinical recommendations 4375 * supporting the measure.). This is the underlying object with id, 4376 * value and extensions. The accessor 4377 * "getClinicalRecommendationStatement" gives direct access to the 4378 * value 4379 */ 4380 public Measure setClinicalRecommendationStatementElement(MarkdownType value) { 4381 this.clinicalRecommendationStatement = value; 4382 return this; 4383 } 4384 4385 /** 4386 * @return Provides a summary of relevant clinical guidelines or other clinical 4387 * recommendations supporting the measure. 4388 */ 4389 public String getClinicalRecommendationStatement() { 4390 return this.clinicalRecommendationStatement == null ? null : this.clinicalRecommendationStatement.getValue(); 4391 } 4392 4393 /** 4394 * @param value Provides a summary of relevant clinical guidelines or other 4395 * clinical recommendations supporting the measure. 4396 */ 4397 public Measure setClinicalRecommendationStatement(String value) { 4398 if (value == null) 4399 this.clinicalRecommendationStatement = null; 4400 else { 4401 if (this.clinicalRecommendationStatement == null) 4402 this.clinicalRecommendationStatement = new MarkdownType(); 4403 this.clinicalRecommendationStatement.setValue(value); 4404 } 4405 return this; 4406 } 4407 4408 /** 4409 * @return {@link #improvementNotation} (Information on whether an increase or 4410 * decrease in score is the preferred result (e.g., a higher score 4411 * indicates better quality OR a lower score indicates better quality OR 4412 * quality is within a range).) 4413 */ 4414 public CodeableConcept getImprovementNotation() { 4415 if (this.improvementNotation == null) 4416 if (Configuration.errorOnAutoCreate()) 4417 throw new Error("Attempt to auto-create Measure.improvementNotation"); 4418 else if (Configuration.doAutoCreate()) 4419 this.improvementNotation = new CodeableConcept(); // cc 4420 return this.improvementNotation; 4421 } 4422 4423 public boolean hasImprovementNotation() { 4424 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 4425 } 4426 4427 /** 4428 * @param value {@link #improvementNotation} (Information on whether an increase 4429 * or decrease in score is the preferred result (e.g., a higher 4430 * score indicates better quality OR a lower score indicates better 4431 * quality OR quality is within a range).) 4432 */ 4433 public Measure setImprovementNotation(CodeableConcept value) { 4434 this.improvementNotation = value; 4435 return this; 4436 } 4437 4438 /** 4439 * @return {@link #definition} (Provides a description of an individual term 4440 * used within the measure.) 4441 */ 4442 public List<MarkdownType> getDefinition() { 4443 if (this.definition == null) 4444 this.definition = new ArrayList<MarkdownType>(); 4445 return this.definition; 4446 } 4447 4448 /** 4449 * @return Returns a reference to <code>this</code> for easy method chaining 4450 */ 4451 public Measure setDefinition(List<MarkdownType> theDefinition) { 4452 this.definition = theDefinition; 4453 return this; 4454 } 4455 4456 public boolean hasDefinition() { 4457 if (this.definition == null) 4458 return false; 4459 for (MarkdownType item : this.definition) 4460 if (!item.isEmpty()) 4461 return true; 4462 return false; 4463 } 4464 4465 /** 4466 * @return {@link #definition} (Provides a description of an individual term 4467 * used within the measure.) 4468 */ 4469 public MarkdownType addDefinitionElement() {// 2 4470 MarkdownType t = new MarkdownType(); 4471 if (this.definition == null) 4472 this.definition = new ArrayList<MarkdownType>(); 4473 this.definition.add(t); 4474 return t; 4475 } 4476 4477 /** 4478 * @param value {@link #definition} (Provides a description of an individual 4479 * term used within the measure.) 4480 */ 4481 public Measure addDefinition(String value) { // 1 4482 MarkdownType t = new MarkdownType(); 4483 t.setValue(value); 4484 if (this.definition == null) 4485 this.definition = new ArrayList<MarkdownType>(); 4486 this.definition.add(t); 4487 return this; 4488 } 4489 4490 /** 4491 * @param value {@link #definition} (Provides a description of an individual 4492 * term used within the measure.) 4493 */ 4494 public boolean hasDefinition(String value) { 4495 if (this.definition == null) 4496 return false; 4497 for (MarkdownType v : this.definition) 4498 if (v.getValue().equals(value)) // markdown 4499 return true; 4500 return false; 4501 } 4502 4503 /** 4504 * @return {@link #guidance} (Additional guidance for the measure including how 4505 * it can be used in a clinical context, and the intent of the 4506 * measure.). This is the underlying object with id, value and 4507 * extensions. The accessor "getGuidance" gives direct access to the 4508 * value 4509 */ 4510 public MarkdownType getGuidanceElement() { 4511 if (this.guidance == null) 4512 if (Configuration.errorOnAutoCreate()) 4513 throw new Error("Attempt to auto-create Measure.guidance"); 4514 else if (Configuration.doAutoCreate()) 4515 this.guidance = new MarkdownType(); // bb 4516 return this.guidance; 4517 } 4518 4519 public boolean hasGuidanceElement() { 4520 return this.guidance != null && !this.guidance.isEmpty(); 4521 } 4522 4523 public boolean hasGuidance() { 4524 return this.guidance != null && !this.guidance.isEmpty(); 4525 } 4526 4527 /** 4528 * @param value {@link #guidance} (Additional guidance for the measure including 4529 * how it can be used in a clinical context, and the intent of the 4530 * measure.). This is the underlying object with id, value and 4531 * extensions. The accessor "getGuidance" gives direct access to 4532 * the value 4533 */ 4534 public Measure setGuidanceElement(MarkdownType value) { 4535 this.guidance = value; 4536 return this; 4537 } 4538 4539 /** 4540 * @return Additional guidance for the measure including how it can be used in a 4541 * clinical context, and the intent of the measure. 4542 */ 4543 public String getGuidance() { 4544 return this.guidance == null ? null : this.guidance.getValue(); 4545 } 4546 4547 /** 4548 * @param value Additional guidance for the measure including how it can be used 4549 * in a clinical context, and the intent of the measure. 4550 */ 4551 public Measure setGuidance(String value) { 4552 if (value == null) 4553 this.guidance = null; 4554 else { 4555 if (this.guidance == null) 4556 this.guidance = new MarkdownType(); 4557 this.guidance.setValue(value); 4558 } 4559 return this; 4560 } 4561 4562 /** 4563 * @return {@link #group} (A group of population criteria for the measure.) 4564 */ 4565 public List<MeasureGroupComponent> getGroup() { 4566 if (this.group == null) 4567 this.group = new ArrayList<MeasureGroupComponent>(); 4568 return this.group; 4569 } 4570 4571 /** 4572 * @return Returns a reference to <code>this</code> for easy method chaining 4573 */ 4574 public Measure setGroup(List<MeasureGroupComponent> theGroup) { 4575 this.group = theGroup; 4576 return this; 4577 } 4578 4579 public boolean hasGroup() { 4580 if (this.group == null) 4581 return false; 4582 for (MeasureGroupComponent item : this.group) 4583 if (!item.isEmpty()) 4584 return true; 4585 return false; 4586 } 4587 4588 public MeasureGroupComponent addGroup() { // 3 4589 MeasureGroupComponent t = new MeasureGroupComponent(); 4590 if (this.group == null) 4591 this.group = new ArrayList<MeasureGroupComponent>(); 4592 this.group.add(t); 4593 return t; 4594 } 4595 4596 public Measure addGroup(MeasureGroupComponent t) { // 3 4597 if (t == null) 4598 return this; 4599 if (this.group == null) 4600 this.group = new ArrayList<MeasureGroupComponent>(); 4601 this.group.add(t); 4602 return this; 4603 } 4604 4605 /** 4606 * @return The first repetition of repeating field {@link #group}, creating it 4607 * if it does not already exist 4608 */ 4609 public MeasureGroupComponent getGroupFirstRep() { 4610 if (getGroup().isEmpty()) { 4611 addGroup(); 4612 } 4613 return getGroup().get(0); 4614 } 4615 4616 /** 4617 * @return {@link #supplementalData} (The supplemental data criteria for the 4618 * measure report, specified as either the name of a valid CQL 4619 * expression within a referenced library, or a valid FHIR Resource 4620 * Path.) 4621 */ 4622 public List<MeasureSupplementalDataComponent> getSupplementalData() { 4623 if (this.supplementalData == null) 4624 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 4625 return this.supplementalData; 4626 } 4627 4628 /** 4629 * @return Returns a reference to <code>this</code> for easy method chaining 4630 */ 4631 public Measure setSupplementalData(List<MeasureSupplementalDataComponent> theSupplementalData) { 4632 this.supplementalData = theSupplementalData; 4633 return this; 4634 } 4635 4636 public boolean hasSupplementalData() { 4637 if (this.supplementalData == null) 4638 return false; 4639 for (MeasureSupplementalDataComponent item : this.supplementalData) 4640 if (!item.isEmpty()) 4641 return true; 4642 return false; 4643 } 4644 4645 public MeasureSupplementalDataComponent addSupplementalData() { // 3 4646 MeasureSupplementalDataComponent t = new MeasureSupplementalDataComponent(); 4647 if (this.supplementalData == null) 4648 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 4649 this.supplementalData.add(t); 4650 return t; 4651 } 4652 4653 public Measure addSupplementalData(MeasureSupplementalDataComponent t) { // 3 4654 if (t == null) 4655 return this; 4656 if (this.supplementalData == null) 4657 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 4658 this.supplementalData.add(t); 4659 return this; 4660 } 4661 4662 /** 4663 * @return The first repetition of repeating field {@link #supplementalData}, 4664 * creating it if it does not already exist 4665 */ 4666 public MeasureSupplementalDataComponent getSupplementalDataFirstRep() { 4667 if (getSupplementalData().isEmpty()) { 4668 addSupplementalData(); 4669 } 4670 return getSupplementalData().get(0); 4671 } 4672 4673 protected void listChildren(List<Property> children) { 4674 super.listChildren(children); 4675 children.add(new Property("url", "uri", 4676 "An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers.", 4677 0, 1, url)); 4678 children.add(new Property("identifier", "Identifier", 4679 "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4680 0, java.lang.Integer.MAX_VALUE, identifier)); 4681 children.add(new Property("version", "string", 4682 "The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 4683 0, 1, version)); 4684 children.add(new Property("name", "string", 4685 "A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4686 0, 1, name)); 4687 children.add( 4688 new Property("title", "string", "A short, descriptive, user-friendly title for the measure.", 0, 1, title)); 4689 children.add(new Property("subtitle", "string", 4690 "An explanatory or alternate title for the measure giving additional information about its content.", 0, 1, 4691 subtitle)); 4692 children.add(new Property("status", "code", 4693 "The status of this measure. Enables tracking the life-cycle of the content.", 0, 1, status)); 4694 children.add(new Property("experimental", "boolean", 4695 "A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4696 0, 1, experimental)); 4697 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4698 "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 4699 0, 1, subject)); 4700 children.add(new Property("date", "dateTime", 4701 "The date (and optionally time) when the measure was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.", 4702 0, 1, date)); 4703 children.add(new Property("publisher", "string", 4704 "The name of the organization or individual that published the measure.", 0, 1, publisher)); 4705 children.add(new Property("contact", "ContactDetail", 4706 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4707 java.lang.Integer.MAX_VALUE, contact)); 4708 children.add(new Property("description", "markdown", 4709 "A free text natural language description of the measure from a consumer's perspective.", 0, 1, description)); 4710 children.add(new Property("useContext", "UsageContext", 4711 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances.", 4712 0, java.lang.Integer.MAX_VALUE, useContext)); 4713 children.add(new Property("jurisdiction", "CodeableConcept", 4714 "A legal or geographic region in which the measure is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 4715 jurisdiction)); 4716 children.add(new Property("purpose", "markdown", 4717 "Explanation of why this measure is needed and why it has been designed as it has.", 0, 1, purpose)); 4718 children.add(new Property("usage", "string", 4719 "A detailed description, from a clinical perspective, of how the measure is used.", 0, 1, usage)); 4720 children.add(new Property("copyright", "markdown", 4721 "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.", 4722 0, 1, copyright)); 4723 children.add(new Property("approvalDate", "date", 4724 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4725 0, 1, approvalDate)); 4726 children.add(new Property("lastReviewDate", "date", 4727 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4728 0, 1, lastReviewDate)); 4729 children.add(new Property("effectivePeriod", "Period", 4730 "The period during which the measure content was or is planned to be in active use.", 0, 1, effectivePeriod)); 4731 children.add(new Property("topic", "CodeableConcept", 4732 "Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching.", 4733 0, java.lang.Integer.MAX_VALUE, topic)); 4734 children.add(new Property("author", "ContactDetail", 4735 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4736 java.lang.Integer.MAX_VALUE, author)); 4737 children.add(new Property("editor", "ContactDetail", 4738 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4739 java.lang.Integer.MAX_VALUE, editor)); 4740 children.add(new Property("reviewer", "ContactDetail", 4741 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4742 java.lang.Integer.MAX_VALUE, reviewer)); 4743 children.add(new Property("endorser", "ContactDetail", 4744 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4745 java.lang.Integer.MAX_VALUE, endorser)); 4746 children.add(new Property("relatedArtifact", "RelatedArtifact", 4747 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4748 java.lang.Integer.MAX_VALUE, relatedArtifact)); 4749 children.add(new Property("library", "canonical(Library)", 4750 "A reference to a Library resource containing the formal logic used by the measure.", 0, 4751 java.lang.Integer.MAX_VALUE, library)); 4752 children.add(new Property("disclaimer", "markdown", 4753 "Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.", 4754 0, 1, disclaimer)); 4755 children.add(new Property("scoring", "CodeableConcept", 4756 "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 4757 0, 1, scoring)); 4758 children.add(new Property("compositeScoring", "CodeableConcept", 4759 "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.", 4760 0, 1, compositeScoring)); 4761 children.add(new Property("type", "CodeableConcept", 4762 "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 4763 0, java.lang.Integer.MAX_VALUE, type)); 4764 children.add(new Property("riskAdjustment", "string", 4765 "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.", 4766 0, 1, riskAdjustment)); 4767 children.add(new Property("rateAggregation", "string", 4768 "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 4769 0, 1, rateAggregation)); 4770 children.add(new Property("rationale", "markdown", 4771 "Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.", 4772 0, 1, rationale)); 4773 children.add(new Property("clinicalRecommendationStatement", "markdown", 4774 "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.", 4775 0, 1, clinicalRecommendationStatement)); 4776 children.add(new Property("improvementNotation", "CodeableConcept", 4777 "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).", 4778 0, 1, improvementNotation)); 4779 children.add( 4780 new Property("definition", "markdown", "Provides a description of an individual term used within the measure.", 4781 0, java.lang.Integer.MAX_VALUE, definition)); 4782 children.add(new Property("guidance", "markdown", 4783 "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.", 4784 0, 1, guidance)); 4785 children.add(new Property("group", "", "A group of population criteria for the measure.", 0, 4786 java.lang.Integer.MAX_VALUE, group)); 4787 children.add(new Property("supplementalData", "", 4788 "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.", 4789 0, java.lang.Integer.MAX_VALUE, supplementalData)); 4790 } 4791 4792 @Override 4793 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4794 switch (_hash) { 4795 case 116079: 4796 /* url */ return new Property("url", "uri", 4797 "An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers.", 4798 0, 1, url); 4799 case -1618432855: 4800 /* identifier */ return new Property("identifier", "Identifier", 4801 "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.", 4802 0, java.lang.Integer.MAX_VALUE, identifier); 4803 case 351608024: 4804 /* version */ return new Property("version", "string", 4805 "The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 4806 0, 1, version); 4807 case 3373707: 4808 /* name */ return new Property("name", "string", 4809 "A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4810 0, 1, name); 4811 case 110371416: 4812 /* title */ return new Property("title", "string", "A short, descriptive, user-friendly title for the measure.", 4813 0, 1, title); 4814 case -2060497896: 4815 /* subtitle */ return new Property("subtitle", "string", 4816 "An explanatory or alternate title for the measure giving additional information about its content.", 0, 1, 4817 subtitle); 4818 case -892481550: 4819 /* status */ return new Property("status", "code", 4820 "The status of this measure. Enables tracking the life-cycle of the content.", 0, 1, status); 4821 case -404562712: 4822 /* experimental */ return new Property("experimental", "boolean", 4823 "A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4824 0, 1, experimental); 4825 case -573640748: 4826 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4827 "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 4828 0, 1, subject); 4829 case -1867885268: 4830 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4831 "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 4832 0, 1, subject); 4833 case -1257122603: 4834 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4835 "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 4836 0, 1, subject); 4837 case 772938623: 4838 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4839 "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 4840 0, 1, subject); 4841 case 3076014: 4842 /* date */ return new Property("date", "dateTime", 4843 "The date (and optionally time) when the measure was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.", 4844 0, 1, date); 4845 case 1447404028: 4846 /* publisher */ return new Property("publisher", "string", 4847 "The name of the organization or individual that published the measure.", 0, 1, publisher); 4848 case 951526432: 4849 /* contact */ return new Property("contact", "ContactDetail", 4850 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4851 java.lang.Integer.MAX_VALUE, contact); 4852 case -1724546052: 4853 /* description */ return new Property("description", "markdown", 4854 "A free text natural language description of the measure from a consumer's perspective.", 0, 1, description); 4855 case -669707736: 4856 /* useContext */ return new Property("useContext", "UsageContext", 4857 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances.", 4858 0, java.lang.Integer.MAX_VALUE, useContext); 4859 case -507075711: 4860 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4861 "A legal or geographic region in which the measure is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 4862 jurisdiction); 4863 case -220463842: 4864 /* purpose */ return new Property("purpose", "markdown", 4865 "Explanation of why this measure is needed and why it has been designed as it has.", 0, 1, purpose); 4866 case 111574433: 4867 /* usage */ return new Property("usage", "string", 4868 "A detailed description, from a clinical perspective, of how the measure is used.", 0, 1, usage); 4869 case 1522889671: 4870 /* copyright */ return new Property("copyright", "markdown", 4871 "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.", 4872 0, 1, copyright); 4873 case 223539345: 4874 /* approvalDate */ return new Property("approvalDate", "date", 4875 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 4876 0, 1, approvalDate); 4877 case -1687512484: 4878 /* lastReviewDate */ return new Property("lastReviewDate", "date", 4879 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 4880 0, 1, lastReviewDate); 4881 case -403934648: 4882 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 4883 "The period during which the measure content was or is planned to be in active use.", 0, 1, effectivePeriod); 4884 case 110546223: 4885 /* topic */ return new Property("topic", "CodeableConcept", 4886 "Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching.", 4887 0, java.lang.Integer.MAX_VALUE, topic); 4888 case -1406328437: 4889 /* author */ return new Property("author", "ContactDetail", 4890 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 4891 java.lang.Integer.MAX_VALUE, author); 4892 case -1307827859: 4893 /* editor */ return new Property("editor", "ContactDetail", 4894 "An individual or organization primarily responsible for internal coherence of the content.", 0, 4895 java.lang.Integer.MAX_VALUE, editor); 4896 case -261190139: 4897 /* reviewer */ return new Property("reviewer", "ContactDetail", 4898 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 4899 java.lang.Integer.MAX_VALUE, reviewer); 4900 case 1740277666: 4901 /* endorser */ return new Property("endorser", "ContactDetail", 4902 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 4903 java.lang.Integer.MAX_VALUE, endorser); 4904 case 666807069: 4905 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 4906 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 4907 java.lang.Integer.MAX_VALUE, relatedArtifact); 4908 case 166208699: 4909 /* library */ return new Property("library", "canonical(Library)", 4910 "A reference to a Library resource containing the formal logic used by the measure.", 0, 4911 java.lang.Integer.MAX_VALUE, library); 4912 case 432371099: 4913 /* disclaimer */ return new Property("disclaimer", "markdown", 4914 "Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.", 4915 0, 1, disclaimer); 4916 case 1924005583: 4917 /* scoring */ return new Property("scoring", "CodeableConcept", 4918 "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 4919 0, 1, scoring); 4920 case 569347656: 4921 /* compositeScoring */ return new Property("compositeScoring", "CodeableConcept", 4922 "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.", 4923 0, 1, compositeScoring); 4924 case 3575610: 4925 /* type */ return new Property("type", "CodeableConcept", 4926 "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 4927 0, java.lang.Integer.MAX_VALUE, type); 4928 case 93273500: 4929 /* riskAdjustment */ return new Property("riskAdjustment", "string", 4930 "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.", 4931 0, 1, riskAdjustment); 4932 case 1254503906: 4933 /* rateAggregation */ return new Property("rateAggregation", "string", 4934 "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 4935 0, 1, rateAggregation); 4936 case 345689335: 4937 /* rationale */ return new Property("rationale", "markdown", 4938 "Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.", 4939 0, 1, rationale); 4940 case -18631389: 4941 /* clinicalRecommendationStatement */ return new Property("clinicalRecommendationStatement", "markdown", 4942 "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.", 4943 0, 1, clinicalRecommendationStatement); 4944 case -2085456136: 4945 /* improvementNotation */ return new Property("improvementNotation", "CodeableConcept", 4946 "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).", 4947 0, 1, improvementNotation); 4948 case -1014418093: 4949 /* definition */ return new Property("definition", "markdown", 4950 "Provides a description of an individual term used within the measure.", 0, java.lang.Integer.MAX_VALUE, 4951 definition); 4952 case -1314002088: 4953 /* guidance */ return new Property("guidance", "markdown", 4954 "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.", 4955 0, 1, guidance); 4956 case 98629247: 4957 /* group */ return new Property("group", "", "A group of population criteria for the measure.", 0, 4958 java.lang.Integer.MAX_VALUE, group); 4959 case 1447496814: 4960 /* supplementalData */ return new Property("supplementalData", "", 4961 "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.", 4962 0, java.lang.Integer.MAX_VALUE, supplementalData); 4963 default: 4964 return super.getNamedProperty(_hash, _name, _checkValid); 4965 } 4966 4967 } 4968 4969 @Override 4970 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4971 switch (hash) { 4972 case 116079: 4973 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4974 case -1618432855: 4975 /* identifier */ return this.identifier == null ? new Base[0] 4976 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4977 case 351608024: 4978 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4979 case 3373707: 4980 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4981 case 110371416: 4982 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4983 case -2060497896: 4984 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 4985 case -892481550: 4986 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4987 case -404562712: 4988 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4989 case -1867885268: 4990 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 4991 case 3076014: 4992 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4993 case 1447404028: 4994 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4995 case 951526432: 4996 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4997 case -1724546052: 4998 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4999 case -669707736: 5000 /* useContext */ return this.useContext == null ? new Base[0] 5001 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5002 case -507075711: 5003 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 5004 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5005 case -220463842: 5006 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 5007 case 111574433: 5008 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 5009 case 1522889671: 5010 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 5011 case 223539345: 5012 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 5013 case -1687512484: 5014 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 5015 case -403934648: 5016 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 5017 case 110546223: 5018 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 5019 case -1406328437: 5020 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5021 case -1307827859: 5022 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5023 case -261190139: 5024 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5025 case 1740277666: 5026 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5027 case 666807069: 5028 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 5029 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5030 case 166208699: 5031 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 5032 case 432371099: 5033 /* disclaimer */ return this.disclaimer == null ? new Base[0] : new Base[] { this.disclaimer }; // MarkdownType 5034 case 1924005583: 5035 /* scoring */ return this.scoring == null ? new Base[0] : new Base[] { this.scoring }; // CodeableConcept 5036 case 569347656: 5037 /* compositeScoring */ return this.compositeScoring == null ? new Base[0] : new Base[] { this.compositeScoring }; // CodeableConcept 5038 case 3575610: 5039 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 5040 case 93273500: 5041 /* riskAdjustment */ return this.riskAdjustment == null ? new Base[0] : new Base[] { this.riskAdjustment }; // StringType 5042 case 1254503906: 5043 /* rateAggregation */ return this.rateAggregation == null ? new Base[0] : new Base[] { this.rateAggregation }; // StringType 5044 case 345689335: 5045 /* rationale */ return this.rationale == null ? new Base[0] : new Base[] { this.rationale }; // MarkdownType 5046 case -18631389: 5047 /* clinicalRecommendationStatement */ return this.clinicalRecommendationStatement == null ? new Base[0] 5048 : new Base[] { this.clinicalRecommendationStatement }; // MarkdownType 5049 case -2085456136: 5050 /* improvementNotation */ return this.improvementNotation == null ? new Base[0] 5051 : new Base[] { this.improvementNotation }; // CodeableConcept 5052 case -1014418093: 5053 /* definition */ return this.definition == null ? new Base[0] 5054 : this.definition.toArray(new Base[this.definition.size()]); // MarkdownType 5055 case -1314002088: 5056 /* guidance */ return this.guidance == null ? new Base[0] : new Base[] { this.guidance }; // MarkdownType 5057 case 98629247: 5058 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // MeasureGroupComponent 5059 case 1447496814: 5060 /* supplementalData */ return this.supplementalData == null ? new Base[0] 5061 : this.supplementalData.toArray(new Base[this.supplementalData.size()]); // MeasureSupplementalDataComponent 5062 default: 5063 return super.getProperty(hash, name, checkValid); 5064 } 5065 5066 } 5067 5068 @Override 5069 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5070 switch (hash) { 5071 case 116079: // url 5072 this.url = castToUri(value); // UriType 5073 return value; 5074 case -1618432855: // identifier 5075 this.getIdentifier().add(castToIdentifier(value)); // Identifier 5076 return value; 5077 case 351608024: // version 5078 this.version = castToString(value); // StringType 5079 return value; 5080 case 3373707: // name 5081 this.name = castToString(value); // StringType 5082 return value; 5083 case 110371416: // title 5084 this.title = castToString(value); // StringType 5085 return value; 5086 case -2060497896: // subtitle 5087 this.subtitle = castToString(value); // StringType 5088 return value; 5089 case -892481550: // status 5090 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5091 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5092 return value; 5093 case -404562712: // experimental 5094 this.experimental = castToBoolean(value); // BooleanType 5095 return value; 5096 case -1867885268: // subject 5097 this.subject = castToType(value); // Type 5098 return value; 5099 case 3076014: // date 5100 this.date = castToDateTime(value); // DateTimeType 5101 return value; 5102 case 1447404028: // publisher 5103 this.publisher = castToString(value); // StringType 5104 return value; 5105 case 951526432: // contact 5106 this.getContact().add(castToContactDetail(value)); // ContactDetail 5107 return value; 5108 case -1724546052: // description 5109 this.description = castToMarkdown(value); // MarkdownType 5110 return value; 5111 case -669707736: // useContext 5112 this.getUseContext().add(castToUsageContext(value)); // UsageContext 5113 return value; 5114 case -507075711: // jurisdiction 5115 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 5116 return value; 5117 case -220463842: // purpose 5118 this.purpose = castToMarkdown(value); // MarkdownType 5119 return value; 5120 case 111574433: // usage 5121 this.usage = castToString(value); // StringType 5122 return value; 5123 case 1522889671: // copyright 5124 this.copyright = castToMarkdown(value); // MarkdownType 5125 return value; 5126 case 223539345: // approvalDate 5127 this.approvalDate = castToDate(value); // DateType 5128 return value; 5129 case -1687512484: // lastReviewDate 5130 this.lastReviewDate = castToDate(value); // DateType 5131 return value; 5132 case -403934648: // effectivePeriod 5133 this.effectivePeriod = castToPeriod(value); // Period 5134 return value; 5135 case 110546223: // topic 5136 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 5137 return value; 5138 case -1406328437: // author 5139 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 5140 return value; 5141 case -1307827859: // editor 5142 this.getEditor().add(castToContactDetail(value)); // ContactDetail 5143 return value; 5144 case -261190139: // reviewer 5145 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 5146 return value; 5147 case 1740277666: // endorser 5148 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 5149 return value; 5150 case 666807069: // relatedArtifact 5151 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 5152 return value; 5153 case 166208699: // library 5154 this.getLibrary().add(castToCanonical(value)); // CanonicalType 5155 return value; 5156 case 432371099: // disclaimer 5157 this.disclaimer = castToMarkdown(value); // MarkdownType 5158 return value; 5159 case 1924005583: // scoring 5160 this.scoring = castToCodeableConcept(value); // CodeableConcept 5161 return value; 5162 case 569347656: // compositeScoring 5163 this.compositeScoring = castToCodeableConcept(value); // CodeableConcept 5164 return value; 5165 case 3575610: // type 5166 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 5167 return value; 5168 case 93273500: // riskAdjustment 5169 this.riskAdjustment = castToString(value); // StringType 5170 return value; 5171 case 1254503906: // rateAggregation 5172 this.rateAggregation = castToString(value); // StringType 5173 return value; 5174 case 345689335: // rationale 5175 this.rationale = castToMarkdown(value); // MarkdownType 5176 return value; 5177 case -18631389: // clinicalRecommendationStatement 5178 this.clinicalRecommendationStatement = castToMarkdown(value); // MarkdownType 5179 return value; 5180 case -2085456136: // improvementNotation 5181 this.improvementNotation = castToCodeableConcept(value); // CodeableConcept 5182 return value; 5183 case -1014418093: // definition 5184 this.getDefinition().add(castToMarkdown(value)); // MarkdownType 5185 return value; 5186 case -1314002088: // guidance 5187 this.guidance = castToMarkdown(value); // MarkdownType 5188 return value; 5189 case 98629247: // group 5190 this.getGroup().add((MeasureGroupComponent) value); // MeasureGroupComponent 5191 return value; 5192 case 1447496814: // supplementalData 5193 this.getSupplementalData().add((MeasureSupplementalDataComponent) value); // MeasureSupplementalDataComponent 5194 return value; 5195 default: 5196 return super.setProperty(hash, name, value); 5197 } 5198 5199 } 5200 5201 @Override 5202 public Base setProperty(String name, Base value) throws FHIRException { 5203 if (name.equals("url")) { 5204 this.url = castToUri(value); // UriType 5205 } else if (name.equals("identifier")) { 5206 this.getIdentifier().add(castToIdentifier(value)); 5207 } else if (name.equals("version")) { 5208 this.version = castToString(value); // StringType 5209 } else if (name.equals("name")) { 5210 this.name = castToString(value); // StringType 5211 } else if (name.equals("title")) { 5212 this.title = castToString(value); // StringType 5213 } else if (name.equals("subtitle")) { 5214 this.subtitle = castToString(value); // StringType 5215 } else if (name.equals("status")) { 5216 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 5217 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5218 } else if (name.equals("experimental")) { 5219 this.experimental = castToBoolean(value); // BooleanType 5220 } else if (name.equals("subject[x]")) { 5221 this.subject = castToType(value); // Type 5222 } else if (name.equals("date")) { 5223 this.date = castToDateTime(value); // DateTimeType 5224 } else if (name.equals("publisher")) { 5225 this.publisher = castToString(value); // StringType 5226 } else if (name.equals("contact")) { 5227 this.getContact().add(castToContactDetail(value)); 5228 } else if (name.equals("description")) { 5229 this.description = castToMarkdown(value); // MarkdownType 5230 } else if (name.equals("useContext")) { 5231 this.getUseContext().add(castToUsageContext(value)); 5232 } else if (name.equals("jurisdiction")) { 5233 this.getJurisdiction().add(castToCodeableConcept(value)); 5234 } else if (name.equals("purpose")) { 5235 this.purpose = castToMarkdown(value); // MarkdownType 5236 } else if (name.equals("usage")) { 5237 this.usage = castToString(value); // StringType 5238 } else if (name.equals("copyright")) { 5239 this.copyright = castToMarkdown(value); // MarkdownType 5240 } else if (name.equals("approvalDate")) { 5241 this.approvalDate = castToDate(value); // DateType 5242 } else if (name.equals("lastReviewDate")) { 5243 this.lastReviewDate = castToDate(value); // DateType 5244 } else if (name.equals("effectivePeriod")) { 5245 this.effectivePeriod = castToPeriod(value); // Period 5246 } else if (name.equals("topic")) { 5247 this.getTopic().add(castToCodeableConcept(value)); 5248 } else if (name.equals("author")) { 5249 this.getAuthor().add(castToContactDetail(value)); 5250 } else if (name.equals("editor")) { 5251 this.getEditor().add(castToContactDetail(value)); 5252 } else if (name.equals("reviewer")) { 5253 this.getReviewer().add(castToContactDetail(value)); 5254 } else if (name.equals("endorser")) { 5255 this.getEndorser().add(castToContactDetail(value)); 5256 } else if (name.equals("relatedArtifact")) { 5257 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 5258 } else if (name.equals("library")) { 5259 this.getLibrary().add(castToCanonical(value)); 5260 } else if (name.equals("disclaimer")) { 5261 this.disclaimer = castToMarkdown(value); // MarkdownType 5262 } else if (name.equals("scoring")) { 5263 this.scoring = castToCodeableConcept(value); // CodeableConcept 5264 } else if (name.equals("compositeScoring")) { 5265 this.compositeScoring = castToCodeableConcept(value); // CodeableConcept 5266 } else if (name.equals("type")) { 5267 this.getType().add(castToCodeableConcept(value)); 5268 } else if (name.equals("riskAdjustment")) { 5269 this.riskAdjustment = castToString(value); // StringType 5270 } else if (name.equals("rateAggregation")) { 5271 this.rateAggregation = castToString(value); // StringType 5272 } else if (name.equals("rationale")) { 5273 this.rationale = castToMarkdown(value); // MarkdownType 5274 } else if (name.equals("clinicalRecommendationStatement")) { 5275 this.clinicalRecommendationStatement = castToMarkdown(value); // MarkdownType 5276 } else if (name.equals("improvementNotation")) { 5277 this.improvementNotation = castToCodeableConcept(value); // CodeableConcept 5278 } else if (name.equals("definition")) { 5279 this.getDefinition().add(castToMarkdown(value)); 5280 } else if (name.equals("guidance")) { 5281 this.guidance = castToMarkdown(value); // MarkdownType 5282 } else if (name.equals("group")) { 5283 this.getGroup().add((MeasureGroupComponent) value); 5284 } else if (name.equals("supplementalData")) { 5285 this.getSupplementalData().add((MeasureSupplementalDataComponent) value); 5286 } else 5287 return super.setProperty(name, value); 5288 return value; 5289 } 5290 5291 @Override 5292 public void removeChild(String name, Base value) throws FHIRException { 5293 if (name.equals("url")) { 5294 this.url = null; 5295 } else if (name.equals("identifier")) { 5296 this.getIdentifier().remove(castToIdentifier(value)); 5297 } else if (name.equals("version")) { 5298 this.version = null; 5299 } else if (name.equals("name")) { 5300 this.name = null; 5301 } else if (name.equals("title")) { 5302 this.title = null; 5303 } else if (name.equals("subtitle")) { 5304 this.subtitle = null; 5305 } else if (name.equals("status")) { 5306 this.status = null; 5307 } else if (name.equals("experimental")) { 5308 this.experimental = null; 5309 } else if (name.equals("subject[x]")) { 5310 this.subject = null; 5311 } else if (name.equals("date")) { 5312 this.date = null; 5313 } else if (name.equals("publisher")) { 5314 this.publisher = null; 5315 } else if (name.equals("contact")) { 5316 this.getContact().remove(castToContactDetail(value)); 5317 } else if (name.equals("description")) { 5318 this.description = null; 5319 } else if (name.equals("useContext")) { 5320 this.getUseContext().remove(castToUsageContext(value)); 5321 } else if (name.equals("jurisdiction")) { 5322 this.getJurisdiction().remove(castToCodeableConcept(value)); 5323 } else if (name.equals("purpose")) { 5324 this.purpose = null; 5325 } else if (name.equals("usage")) { 5326 this.usage = null; 5327 } else if (name.equals("copyright")) { 5328 this.copyright = null; 5329 } else if (name.equals("approvalDate")) { 5330 this.approvalDate = null; 5331 } else if (name.equals("lastReviewDate")) { 5332 this.lastReviewDate = null; 5333 } else if (name.equals("effectivePeriod")) { 5334 this.effectivePeriod = null; 5335 } else if (name.equals("topic")) { 5336 this.getTopic().remove(castToCodeableConcept(value)); 5337 } else if (name.equals("author")) { 5338 this.getAuthor().remove(castToContactDetail(value)); 5339 } else if (name.equals("editor")) { 5340 this.getEditor().remove(castToContactDetail(value)); 5341 } else if (name.equals("reviewer")) { 5342 this.getReviewer().remove(castToContactDetail(value)); 5343 } else if (name.equals("endorser")) { 5344 this.getEndorser().remove(castToContactDetail(value)); 5345 } else if (name.equals("relatedArtifact")) { 5346 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 5347 } else if (name.equals("library")) { 5348 this.getLibrary().remove(castToCanonical(value)); 5349 } else if (name.equals("disclaimer")) { 5350 this.disclaimer = null; 5351 } else if (name.equals("scoring")) { 5352 this.scoring = null; 5353 } else if (name.equals("compositeScoring")) { 5354 this.compositeScoring = null; 5355 } else if (name.equals("type")) { 5356 this.getType().remove(castToCodeableConcept(value)); 5357 } else if (name.equals("riskAdjustment")) { 5358 this.riskAdjustment = null; 5359 } else if (name.equals("rateAggregation")) { 5360 this.rateAggregation = null; 5361 } else if (name.equals("rationale")) { 5362 this.rationale = null; 5363 } else if (name.equals("clinicalRecommendationStatement")) { 5364 this.clinicalRecommendationStatement = null; 5365 } else if (name.equals("improvementNotation")) { 5366 this.improvementNotation = null; 5367 } else if (name.equals("definition")) { 5368 this.getDefinition().remove(castToMarkdown(value)); 5369 } else if (name.equals("guidance")) { 5370 this.guidance = null; 5371 } else if (name.equals("group")) { 5372 this.getGroup().remove((MeasureGroupComponent) value); 5373 } else if (name.equals("supplementalData")) { 5374 this.getSupplementalData().remove((MeasureSupplementalDataComponent) value); 5375 } else 5376 super.removeChild(name, value); 5377 5378 } 5379 5380 @Override 5381 public Base makeProperty(int hash, String name) throws FHIRException { 5382 switch (hash) { 5383 case 116079: 5384 return getUrlElement(); 5385 case -1618432855: 5386 return addIdentifier(); 5387 case 351608024: 5388 return getVersionElement(); 5389 case 3373707: 5390 return getNameElement(); 5391 case 110371416: 5392 return getTitleElement(); 5393 case -2060497896: 5394 return getSubtitleElement(); 5395 case -892481550: 5396 return getStatusElement(); 5397 case -404562712: 5398 return getExperimentalElement(); 5399 case -573640748: 5400 return getSubject(); 5401 case -1867885268: 5402 return getSubject(); 5403 case 3076014: 5404 return getDateElement(); 5405 case 1447404028: 5406 return getPublisherElement(); 5407 case 951526432: 5408 return addContact(); 5409 case -1724546052: 5410 return getDescriptionElement(); 5411 case -669707736: 5412 return addUseContext(); 5413 case -507075711: 5414 return addJurisdiction(); 5415 case -220463842: 5416 return getPurposeElement(); 5417 case 111574433: 5418 return getUsageElement(); 5419 case 1522889671: 5420 return getCopyrightElement(); 5421 case 223539345: 5422 return getApprovalDateElement(); 5423 case -1687512484: 5424 return getLastReviewDateElement(); 5425 case -403934648: 5426 return getEffectivePeriod(); 5427 case 110546223: 5428 return addTopic(); 5429 case -1406328437: 5430 return addAuthor(); 5431 case -1307827859: 5432 return addEditor(); 5433 case -261190139: 5434 return addReviewer(); 5435 case 1740277666: 5436 return addEndorser(); 5437 case 666807069: 5438 return addRelatedArtifact(); 5439 case 166208699: 5440 return addLibraryElement(); 5441 case 432371099: 5442 return getDisclaimerElement(); 5443 case 1924005583: 5444 return getScoring(); 5445 case 569347656: 5446 return getCompositeScoring(); 5447 case 3575610: 5448 return addType(); 5449 case 93273500: 5450 return getRiskAdjustmentElement(); 5451 case 1254503906: 5452 return getRateAggregationElement(); 5453 case 345689335: 5454 return getRationaleElement(); 5455 case -18631389: 5456 return getClinicalRecommendationStatementElement(); 5457 case -2085456136: 5458 return getImprovementNotation(); 5459 case -1014418093: 5460 return addDefinitionElement(); 5461 case -1314002088: 5462 return getGuidanceElement(); 5463 case 98629247: 5464 return addGroup(); 5465 case 1447496814: 5466 return addSupplementalData(); 5467 default: 5468 return super.makeProperty(hash, name); 5469 } 5470 5471 } 5472 5473 @Override 5474 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5475 switch (hash) { 5476 case 116079: 5477 /* url */ return new String[] { "uri" }; 5478 case -1618432855: 5479 /* identifier */ return new String[] { "Identifier" }; 5480 case 351608024: 5481 /* version */ return new String[] { "string" }; 5482 case 3373707: 5483 /* name */ return new String[] { "string" }; 5484 case 110371416: 5485 /* title */ return new String[] { "string" }; 5486 case -2060497896: 5487 /* subtitle */ return new String[] { "string" }; 5488 case -892481550: 5489 /* status */ return new String[] { "code" }; 5490 case -404562712: 5491 /* experimental */ return new String[] { "boolean" }; 5492 case -1867885268: 5493 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 5494 case 3076014: 5495 /* date */ return new String[] { "dateTime" }; 5496 case 1447404028: 5497 /* publisher */ return new String[] { "string" }; 5498 case 951526432: 5499 /* contact */ return new String[] { "ContactDetail" }; 5500 case -1724546052: 5501 /* description */ return new String[] { "markdown" }; 5502 case -669707736: 5503 /* useContext */ return new String[] { "UsageContext" }; 5504 case -507075711: 5505 /* jurisdiction */ return new String[] { "CodeableConcept" }; 5506 case -220463842: 5507 /* purpose */ return new String[] { "markdown" }; 5508 case 111574433: 5509 /* usage */ return new String[] { "string" }; 5510 case 1522889671: 5511 /* copyright */ return new String[] { "markdown" }; 5512 case 223539345: 5513 /* approvalDate */ return new String[] { "date" }; 5514 case -1687512484: 5515 /* lastReviewDate */ return new String[] { "date" }; 5516 case -403934648: 5517 /* effectivePeriod */ return new String[] { "Period" }; 5518 case 110546223: 5519 /* topic */ return new String[] { "CodeableConcept" }; 5520 case -1406328437: 5521 /* author */ return new String[] { "ContactDetail" }; 5522 case -1307827859: 5523 /* editor */ return new String[] { "ContactDetail" }; 5524 case -261190139: 5525 /* reviewer */ return new String[] { "ContactDetail" }; 5526 case 1740277666: 5527 /* endorser */ return new String[] { "ContactDetail" }; 5528 case 666807069: 5529 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 5530 case 166208699: 5531 /* library */ return new String[] { "canonical" }; 5532 case 432371099: 5533 /* disclaimer */ return new String[] { "markdown" }; 5534 case 1924005583: 5535 /* scoring */ return new String[] { "CodeableConcept" }; 5536 case 569347656: 5537 /* compositeScoring */ return new String[] { "CodeableConcept" }; 5538 case 3575610: 5539 /* type */ return new String[] { "CodeableConcept" }; 5540 case 93273500: 5541 /* riskAdjustment */ return new String[] { "string" }; 5542 case 1254503906: 5543 /* rateAggregation */ return new String[] { "string" }; 5544 case 345689335: 5545 /* rationale */ return new String[] { "markdown" }; 5546 case -18631389: 5547 /* clinicalRecommendationStatement */ return new String[] { "markdown" }; 5548 case -2085456136: 5549 /* improvementNotation */ return new String[] { "CodeableConcept" }; 5550 case -1014418093: 5551 /* definition */ return new String[] { "markdown" }; 5552 case -1314002088: 5553 /* guidance */ return new String[] { "markdown" }; 5554 case 98629247: 5555 /* group */ return new String[] {}; 5556 case 1447496814: 5557 /* supplementalData */ return new String[] {}; 5558 default: 5559 return super.getTypesForProperty(hash, name); 5560 } 5561 5562 } 5563 5564 @Override 5565 public Base addChild(String name) throws FHIRException { 5566 if (name.equals("url")) { 5567 throw new FHIRException("Cannot call addChild on a singleton property Measure.url"); 5568 } else if (name.equals("identifier")) { 5569 return addIdentifier(); 5570 } else if (name.equals("version")) { 5571 throw new FHIRException("Cannot call addChild on a singleton property Measure.version"); 5572 } else if (name.equals("name")) { 5573 throw new FHIRException("Cannot call addChild on a singleton property Measure.name"); 5574 } else if (name.equals("title")) { 5575 throw new FHIRException("Cannot call addChild on a singleton property Measure.title"); 5576 } else if (name.equals("subtitle")) { 5577 throw new FHIRException("Cannot call addChild on a singleton property Measure.subtitle"); 5578 } else if (name.equals("status")) { 5579 throw new FHIRException("Cannot call addChild on a singleton property Measure.status"); 5580 } else if (name.equals("experimental")) { 5581 throw new FHIRException("Cannot call addChild on a singleton property Measure.experimental"); 5582 } else if (name.equals("subjectCodeableConcept")) { 5583 this.subject = new CodeableConcept(); 5584 return this.subject; 5585 } else if (name.equals("subjectReference")) { 5586 this.subject = new Reference(); 5587 return this.subject; 5588 } else if (name.equals("date")) { 5589 throw new FHIRException("Cannot call addChild on a singleton property Measure.date"); 5590 } else if (name.equals("publisher")) { 5591 throw new FHIRException("Cannot call addChild on a singleton property Measure.publisher"); 5592 } else if (name.equals("contact")) { 5593 return addContact(); 5594 } else if (name.equals("description")) { 5595 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 5596 } else if (name.equals("useContext")) { 5597 return addUseContext(); 5598 } else if (name.equals("jurisdiction")) { 5599 return addJurisdiction(); 5600 } else if (name.equals("purpose")) { 5601 throw new FHIRException("Cannot call addChild on a singleton property Measure.purpose"); 5602 } else if (name.equals("usage")) { 5603 throw new FHIRException("Cannot call addChild on a singleton property Measure.usage"); 5604 } else if (name.equals("copyright")) { 5605 throw new FHIRException("Cannot call addChild on a singleton property Measure.copyright"); 5606 } else if (name.equals("approvalDate")) { 5607 throw new FHIRException("Cannot call addChild on a singleton property Measure.approvalDate"); 5608 } else if (name.equals("lastReviewDate")) { 5609 throw new FHIRException("Cannot call addChild on a singleton property Measure.lastReviewDate"); 5610 } else if (name.equals("effectivePeriod")) { 5611 this.effectivePeriod = new Period(); 5612 return this.effectivePeriod; 5613 } else if (name.equals("topic")) { 5614 return addTopic(); 5615 } else if (name.equals("author")) { 5616 return addAuthor(); 5617 } else if (name.equals("editor")) { 5618 return addEditor(); 5619 } else if (name.equals("reviewer")) { 5620 return addReviewer(); 5621 } else if (name.equals("endorser")) { 5622 return addEndorser(); 5623 } else if (name.equals("relatedArtifact")) { 5624 return addRelatedArtifact(); 5625 } else if (name.equals("library")) { 5626 throw new FHIRException("Cannot call addChild on a singleton property Measure.library"); 5627 } else if (name.equals("disclaimer")) { 5628 throw new FHIRException("Cannot call addChild on a singleton property Measure.disclaimer"); 5629 } else if (name.equals("scoring")) { 5630 this.scoring = new CodeableConcept(); 5631 return this.scoring; 5632 } else if (name.equals("compositeScoring")) { 5633 this.compositeScoring = new CodeableConcept(); 5634 return this.compositeScoring; 5635 } else if (name.equals("type")) { 5636 return addType(); 5637 } else if (name.equals("riskAdjustment")) { 5638 throw new FHIRException("Cannot call addChild on a singleton property Measure.riskAdjustment"); 5639 } else if (name.equals("rateAggregation")) { 5640 throw new FHIRException("Cannot call addChild on a singleton property Measure.rateAggregation"); 5641 } else if (name.equals("rationale")) { 5642 throw new FHIRException("Cannot call addChild on a singleton property Measure.rationale"); 5643 } else if (name.equals("clinicalRecommendationStatement")) { 5644 throw new FHIRException("Cannot call addChild on a singleton property Measure.clinicalRecommendationStatement"); 5645 } else if (name.equals("improvementNotation")) { 5646 this.improvementNotation = new CodeableConcept(); 5647 return this.improvementNotation; 5648 } else if (name.equals("definition")) { 5649 throw new FHIRException("Cannot call addChild on a singleton property Measure.definition"); 5650 } else if (name.equals("guidance")) { 5651 throw new FHIRException("Cannot call addChild on a singleton property Measure.guidance"); 5652 } else if (name.equals("group")) { 5653 return addGroup(); 5654 } else if (name.equals("supplementalData")) { 5655 return addSupplementalData(); 5656 } else 5657 return super.addChild(name); 5658 } 5659 5660 public String fhirType() { 5661 return "Measure"; 5662 5663 } 5664 5665 public Measure copy() { 5666 Measure dst = new Measure(); 5667 copyValues(dst); 5668 return dst; 5669 } 5670 5671 public void copyValues(Measure dst) { 5672 super.copyValues(dst); 5673 dst.url = url == null ? null : url.copy(); 5674 if (identifier != null) { 5675 dst.identifier = new ArrayList<Identifier>(); 5676 for (Identifier i : identifier) 5677 dst.identifier.add(i.copy()); 5678 } 5679 ; 5680 dst.version = version == null ? null : version.copy(); 5681 dst.name = name == null ? null : name.copy(); 5682 dst.title = title == null ? null : title.copy(); 5683 dst.subtitle = subtitle == null ? null : subtitle.copy(); 5684 dst.status = status == null ? null : status.copy(); 5685 dst.experimental = experimental == null ? null : experimental.copy(); 5686 dst.subject = subject == null ? null : subject.copy(); 5687 dst.date = date == null ? null : date.copy(); 5688 dst.publisher = publisher == null ? null : publisher.copy(); 5689 if (contact != null) { 5690 dst.contact = new ArrayList<ContactDetail>(); 5691 for (ContactDetail i : contact) 5692 dst.contact.add(i.copy()); 5693 } 5694 ; 5695 dst.description = description == null ? null : description.copy(); 5696 if (useContext != null) { 5697 dst.useContext = new ArrayList<UsageContext>(); 5698 for (UsageContext i : useContext) 5699 dst.useContext.add(i.copy()); 5700 } 5701 ; 5702 if (jurisdiction != null) { 5703 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5704 for (CodeableConcept i : jurisdiction) 5705 dst.jurisdiction.add(i.copy()); 5706 } 5707 ; 5708 dst.purpose = purpose == null ? null : purpose.copy(); 5709 dst.usage = usage == null ? null : usage.copy(); 5710 dst.copyright = copyright == null ? null : copyright.copy(); 5711 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 5712 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 5713 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 5714 if (topic != null) { 5715 dst.topic = new ArrayList<CodeableConcept>(); 5716 for (CodeableConcept i : topic) 5717 dst.topic.add(i.copy()); 5718 } 5719 ; 5720 if (author != null) { 5721 dst.author = new ArrayList<ContactDetail>(); 5722 for (ContactDetail i : author) 5723 dst.author.add(i.copy()); 5724 } 5725 ; 5726 if (editor != null) { 5727 dst.editor = new ArrayList<ContactDetail>(); 5728 for (ContactDetail i : editor) 5729 dst.editor.add(i.copy()); 5730 } 5731 ; 5732 if (reviewer != null) { 5733 dst.reviewer = new ArrayList<ContactDetail>(); 5734 for (ContactDetail i : reviewer) 5735 dst.reviewer.add(i.copy()); 5736 } 5737 ; 5738 if (endorser != null) { 5739 dst.endorser = new ArrayList<ContactDetail>(); 5740 for (ContactDetail i : endorser) 5741 dst.endorser.add(i.copy()); 5742 } 5743 ; 5744 if (relatedArtifact != null) { 5745 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 5746 for (RelatedArtifact i : relatedArtifact) 5747 dst.relatedArtifact.add(i.copy()); 5748 } 5749 ; 5750 if (library != null) { 5751 dst.library = new ArrayList<CanonicalType>(); 5752 for (CanonicalType i : library) 5753 dst.library.add(i.copy()); 5754 } 5755 ; 5756 dst.disclaimer = disclaimer == null ? null : disclaimer.copy(); 5757 dst.scoring = scoring == null ? null : scoring.copy(); 5758 dst.compositeScoring = compositeScoring == null ? null : compositeScoring.copy(); 5759 if (type != null) { 5760 dst.type = new ArrayList<CodeableConcept>(); 5761 for (CodeableConcept i : type) 5762 dst.type.add(i.copy()); 5763 } 5764 ; 5765 dst.riskAdjustment = riskAdjustment == null ? null : riskAdjustment.copy(); 5766 dst.rateAggregation = rateAggregation == null ? null : rateAggregation.copy(); 5767 dst.rationale = rationale == null ? null : rationale.copy(); 5768 dst.clinicalRecommendationStatement = clinicalRecommendationStatement == null ? null 5769 : clinicalRecommendationStatement.copy(); 5770 dst.improvementNotation = improvementNotation == null ? null : improvementNotation.copy(); 5771 if (definition != null) { 5772 dst.definition = new ArrayList<MarkdownType>(); 5773 for (MarkdownType i : definition) 5774 dst.definition.add(i.copy()); 5775 } 5776 ; 5777 dst.guidance = guidance == null ? null : guidance.copy(); 5778 if (group != null) { 5779 dst.group = new ArrayList<MeasureGroupComponent>(); 5780 for (MeasureGroupComponent i : group) 5781 dst.group.add(i.copy()); 5782 } 5783 ; 5784 if (supplementalData != null) { 5785 dst.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 5786 for (MeasureSupplementalDataComponent i : supplementalData) 5787 dst.supplementalData.add(i.copy()); 5788 } 5789 ; 5790 } 5791 5792 protected Measure typedCopy() { 5793 return copy(); 5794 } 5795 5796 @Override 5797 public boolean equalsDeep(Base other_) { 5798 if (!super.equalsDeep(other_)) 5799 return false; 5800 if (!(other_ instanceof Measure)) 5801 return false; 5802 Measure o = (Measure) other_; 5803 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 5804 && compareDeep(subject, o.subject, true) && compareDeep(purpose, o.purpose, true) 5805 && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 5806 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 5807 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) 5808 && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 5809 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) 5810 && compareDeep(relatedArtifact, o.relatedArtifact, true) && compareDeep(library, o.library, true) 5811 && compareDeep(disclaimer, o.disclaimer, true) && compareDeep(scoring, o.scoring, true) 5812 && compareDeep(compositeScoring, o.compositeScoring, true) && compareDeep(type, o.type, true) 5813 && compareDeep(riskAdjustment, o.riskAdjustment, true) && compareDeep(rateAggregation, o.rateAggregation, true) 5814 && compareDeep(rationale, o.rationale, true) 5815 && compareDeep(clinicalRecommendationStatement, o.clinicalRecommendationStatement, true) 5816 && compareDeep(improvementNotation, o.improvementNotation, true) && compareDeep(definition, o.definition, true) 5817 && compareDeep(guidance, o.guidance, true) && compareDeep(group, o.group, true) 5818 && compareDeep(supplementalData, o.supplementalData, true); 5819 } 5820 5821 @Override 5822 public boolean equalsShallow(Base other_) { 5823 if (!super.equalsShallow(other_)) 5824 return false; 5825 if (!(other_ instanceof Measure)) 5826 return false; 5827 Measure o = (Measure) other_; 5828 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 5829 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 5830 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 5831 && compareValues(disclaimer, o.disclaimer, true) && compareValues(riskAdjustment, o.riskAdjustment, true) 5832 && compareValues(rateAggregation, o.rateAggregation, true) && compareValues(rationale, o.rationale, true) 5833 && compareValues(clinicalRecommendationStatement, o.clinicalRecommendationStatement, true) 5834 && compareValues(definition, o.definition, true) && compareValues(guidance, o.guidance, true); 5835 } 5836 5837 public boolean isEmpty() { 5838 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, subject, purpose, usage, 5839 copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, 5840 relatedArtifact, library, disclaimer, scoring, compositeScoring, type, riskAdjustment, rateAggregation, 5841 rationale, clinicalRecommendationStatement, improvementNotation, definition, guidance, group, supplementalData); 5842 } 5843 5844 @Override 5845 public ResourceType getResourceType() { 5846 return ResourceType.Measure; 5847 } 5848 5849 /** 5850 * Search parameter: <b>date</b> 5851 * <p> 5852 * Description: <b>The measure publication date</b><br> 5853 * Type: <b>date</b><br> 5854 * Path: <b>Measure.date</b><br> 5855 * </p> 5856 */ 5857 @SearchParamDefinition(name = "date", path = "Measure.date", description = "The measure publication date", type = "date") 5858 public static final String SP_DATE = "date"; 5859 /** 5860 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5861 * <p> 5862 * Description: <b>The measure publication date</b><br> 5863 * Type: <b>date</b><br> 5864 * Path: <b>Measure.date</b><br> 5865 * </p> 5866 */ 5867 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5868 SP_DATE); 5869 5870 /** 5871 * Search parameter: <b>identifier</b> 5872 * <p> 5873 * Description: <b>External identifier for the measure</b><br> 5874 * Type: <b>token</b><br> 5875 * Path: <b>Measure.identifier</b><br> 5876 * </p> 5877 */ 5878 @SearchParamDefinition(name = "identifier", path = "Measure.identifier", description = "External identifier for the measure", type = "token") 5879 public static final String SP_IDENTIFIER = "identifier"; 5880 /** 5881 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5882 * <p> 5883 * Description: <b>External identifier for the measure</b><br> 5884 * Type: <b>token</b><br> 5885 * Path: <b>Measure.identifier</b><br> 5886 * </p> 5887 */ 5888 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5889 SP_IDENTIFIER); 5890 5891 /** 5892 * Search parameter: <b>successor</b> 5893 * <p> 5894 * Description: <b>What resource is being referenced</b><br> 5895 * Type: <b>reference</b><br> 5896 * Path: <b>Measure.relatedArtifact.resource</b><br> 5897 * </p> 5898 */ 5899 @SearchParamDefinition(name = "successor", path = "Measure.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 5900 public static final String SP_SUCCESSOR = "successor"; 5901 /** 5902 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 5903 * <p> 5904 * Description: <b>What resource is being referenced</b><br> 5905 * Type: <b>reference</b><br> 5906 * Path: <b>Measure.relatedArtifact.resource</b><br> 5907 * </p> 5908 */ 5909 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5910 SP_SUCCESSOR); 5911 5912 /** 5913 * Constant for fluent queries to be used to add include statements. Specifies 5914 * the path value of "<b>Measure:successor</b>". 5915 */ 5916 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 5917 "Measure:successor").toLocked(); 5918 5919 /** 5920 * Search parameter: <b>context-type-value</b> 5921 * <p> 5922 * Description: <b>A use context type and value assigned to the measure</b><br> 5923 * Type: <b>composite</b><br> 5924 * Path: <b></b><br> 5925 * </p> 5926 */ 5927 @SearchParamDefinition(name = "context-type-value", path = "Measure.useContext", description = "A use context type and value assigned to the measure", type = "composite", compositeOf = { 5928 "context-type", "context" }) 5929 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5930 /** 5931 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5932 * <p> 5933 * Description: <b>A use context type and value assigned to the measure</b><br> 5934 * Type: <b>composite</b><br> 5935 * Path: <b></b><br> 5936 * </p> 5937 */ 5938 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5939 SP_CONTEXT_TYPE_VALUE); 5940 5941 /** 5942 * Search parameter: <b>jurisdiction</b> 5943 * <p> 5944 * Description: <b>Intended jurisdiction for the measure</b><br> 5945 * Type: <b>token</b><br> 5946 * Path: <b>Measure.jurisdiction</b><br> 5947 * </p> 5948 */ 5949 @SearchParamDefinition(name = "jurisdiction", path = "Measure.jurisdiction", description = "Intended jurisdiction for the measure", type = "token") 5950 public static final String SP_JURISDICTION = "jurisdiction"; 5951 /** 5952 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 5953 * <p> 5954 * Description: <b>Intended jurisdiction for the measure</b><br> 5955 * Type: <b>token</b><br> 5956 * Path: <b>Measure.jurisdiction</b><br> 5957 * </p> 5958 */ 5959 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5960 SP_JURISDICTION); 5961 5962 /** 5963 * Search parameter: <b>description</b> 5964 * <p> 5965 * Description: <b>The description of the measure</b><br> 5966 * Type: <b>string</b><br> 5967 * Path: <b>Measure.description</b><br> 5968 * </p> 5969 */ 5970 @SearchParamDefinition(name = "description", path = "Measure.description", description = "The description of the measure", type = "string") 5971 public static final String SP_DESCRIPTION = "description"; 5972 /** 5973 * <b>Fluent Client</b> search parameter constant for <b>description</b> 5974 * <p> 5975 * Description: <b>The description of the measure</b><br> 5976 * Type: <b>string</b><br> 5977 * Path: <b>Measure.description</b><br> 5978 * </p> 5979 */ 5980 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 5981 SP_DESCRIPTION); 5982 5983 /** 5984 * Search parameter: <b>derived-from</b> 5985 * <p> 5986 * Description: <b>What resource is being referenced</b><br> 5987 * Type: <b>reference</b><br> 5988 * Path: <b>Measure.relatedArtifact.resource</b><br> 5989 * </p> 5990 */ 5991 @SearchParamDefinition(name = "derived-from", path = "Measure.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 5992 public static final String SP_DERIVED_FROM = "derived-from"; 5993 /** 5994 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 5995 * <p> 5996 * Description: <b>What resource is being referenced</b><br> 5997 * Type: <b>reference</b><br> 5998 * Path: <b>Measure.relatedArtifact.resource</b><br> 5999 * </p> 6000 */ 6001 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6002 SP_DERIVED_FROM); 6003 6004 /** 6005 * Constant for fluent queries to be used to add include statements. Specifies 6006 * the path value of "<b>Measure:derived-from</b>". 6007 */ 6008 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 6009 "Measure:derived-from").toLocked(); 6010 6011 /** 6012 * Search parameter: <b>context-type</b> 6013 * <p> 6014 * Description: <b>A type of use context assigned to the measure</b><br> 6015 * Type: <b>token</b><br> 6016 * Path: <b>Measure.useContext.code</b><br> 6017 * </p> 6018 */ 6019 @SearchParamDefinition(name = "context-type", path = "Measure.useContext.code", description = "A type of use context assigned to the measure", type = "token") 6020 public static final String SP_CONTEXT_TYPE = "context-type"; 6021 /** 6022 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6023 * <p> 6024 * Description: <b>A type of use context assigned to the measure</b><br> 6025 * Type: <b>token</b><br> 6026 * Path: <b>Measure.useContext.code</b><br> 6027 * </p> 6028 */ 6029 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6030 SP_CONTEXT_TYPE); 6031 6032 /** 6033 * Search parameter: <b>predecessor</b> 6034 * <p> 6035 * Description: <b>What resource is being referenced</b><br> 6036 * Type: <b>reference</b><br> 6037 * Path: <b>Measure.relatedArtifact.resource</b><br> 6038 * </p> 6039 */ 6040 @SearchParamDefinition(name = "predecessor", path = "Measure.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 6041 public static final String SP_PREDECESSOR = "predecessor"; 6042 /** 6043 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 6044 * <p> 6045 * Description: <b>What resource is being referenced</b><br> 6046 * Type: <b>reference</b><br> 6047 * Path: <b>Measure.relatedArtifact.resource</b><br> 6048 * </p> 6049 */ 6050 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6051 SP_PREDECESSOR); 6052 6053 /** 6054 * Constant for fluent queries to be used to add include statements. Specifies 6055 * the path value of "<b>Measure:predecessor</b>". 6056 */ 6057 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 6058 "Measure:predecessor").toLocked(); 6059 6060 /** 6061 * Search parameter: <b>title</b> 6062 * <p> 6063 * Description: <b>The human-friendly name of the measure</b><br> 6064 * Type: <b>string</b><br> 6065 * Path: <b>Measure.title</b><br> 6066 * </p> 6067 */ 6068 @SearchParamDefinition(name = "title", path = "Measure.title", description = "The human-friendly name of the measure", type = "string") 6069 public static final String SP_TITLE = "title"; 6070 /** 6071 * <b>Fluent Client</b> search parameter constant for <b>title</b> 6072 * <p> 6073 * Description: <b>The human-friendly name of the measure</b><br> 6074 * Type: <b>string</b><br> 6075 * Path: <b>Measure.title</b><br> 6076 * </p> 6077 */ 6078 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 6079 SP_TITLE); 6080 6081 /** 6082 * Search parameter: <b>composed-of</b> 6083 * <p> 6084 * Description: <b>What resource is being referenced</b><br> 6085 * Type: <b>reference</b><br> 6086 * Path: <b>Measure.relatedArtifact.resource</b><br> 6087 * </p> 6088 */ 6089 @SearchParamDefinition(name = "composed-of", path = "Measure.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 6090 public static final String SP_COMPOSED_OF = "composed-of"; 6091 /** 6092 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 6093 * <p> 6094 * Description: <b>What resource is being referenced</b><br> 6095 * Type: <b>reference</b><br> 6096 * Path: <b>Measure.relatedArtifact.resource</b><br> 6097 * </p> 6098 */ 6099 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6100 SP_COMPOSED_OF); 6101 6102 /** 6103 * Constant for fluent queries to be used to add include statements. Specifies 6104 * the path value of "<b>Measure:composed-of</b>". 6105 */ 6106 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 6107 "Measure:composed-of").toLocked(); 6108 6109 /** 6110 * Search parameter: <b>version</b> 6111 * <p> 6112 * Description: <b>The business version of the measure</b><br> 6113 * Type: <b>token</b><br> 6114 * Path: <b>Measure.version</b><br> 6115 * </p> 6116 */ 6117 @SearchParamDefinition(name = "version", path = "Measure.version", description = "The business version of the measure", type = "token") 6118 public static final String SP_VERSION = "version"; 6119 /** 6120 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6121 * <p> 6122 * Description: <b>The business version of the measure</b><br> 6123 * Type: <b>token</b><br> 6124 * Path: <b>Measure.version</b><br> 6125 * </p> 6126 */ 6127 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6128 SP_VERSION); 6129 6130 /** 6131 * Search parameter: <b>url</b> 6132 * <p> 6133 * Description: <b>The uri that identifies the measure</b><br> 6134 * Type: <b>uri</b><br> 6135 * Path: <b>Measure.url</b><br> 6136 * </p> 6137 */ 6138 @SearchParamDefinition(name = "url", path = "Measure.url", description = "The uri that identifies the measure", type = "uri") 6139 public static final String SP_URL = "url"; 6140 /** 6141 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6142 * <p> 6143 * Description: <b>The uri that identifies the measure</b><br> 6144 * Type: <b>uri</b><br> 6145 * Path: <b>Measure.url</b><br> 6146 * </p> 6147 */ 6148 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6149 6150 /** 6151 * Search parameter: <b>context-quantity</b> 6152 * <p> 6153 * Description: <b>A quantity- or range-valued use context assigned to the 6154 * measure</b><br> 6155 * Type: <b>quantity</b><br> 6156 * Path: <b>Measure.useContext.valueQuantity, 6157 * Measure.useContext.valueRange</b><br> 6158 * </p> 6159 */ 6160 @SearchParamDefinition(name = "context-quantity", path = "(Measure.useContext.value as Quantity) | (Measure.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the measure", type = "quantity") 6161 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6162 /** 6163 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6164 * <p> 6165 * Description: <b>A quantity- or range-valued use context assigned to the 6166 * measure</b><br> 6167 * Type: <b>quantity</b><br> 6168 * Path: <b>Measure.useContext.valueQuantity, 6169 * Measure.useContext.valueRange</b><br> 6170 * </p> 6171 */ 6172 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 6173 SP_CONTEXT_QUANTITY); 6174 6175 /** 6176 * Search parameter: <b>effective</b> 6177 * <p> 6178 * Description: <b>The time during which the measure is intended to be in 6179 * use</b><br> 6180 * Type: <b>date</b><br> 6181 * Path: <b>Measure.effectivePeriod</b><br> 6182 * </p> 6183 */ 6184 @SearchParamDefinition(name = "effective", path = "Measure.effectivePeriod", description = "The time during which the measure is intended to be in use", type = "date") 6185 public static final String SP_EFFECTIVE = "effective"; 6186 /** 6187 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 6188 * <p> 6189 * Description: <b>The time during which the measure is intended to be in 6190 * use</b><br> 6191 * Type: <b>date</b><br> 6192 * Path: <b>Measure.effectivePeriod</b><br> 6193 * </p> 6194 */ 6195 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 6196 SP_EFFECTIVE); 6197 6198 /** 6199 * Search parameter: <b>depends-on</b> 6200 * <p> 6201 * Description: <b>What resource is being referenced</b><br> 6202 * Type: <b>reference</b><br> 6203 * Path: <b>Measure.relatedArtifact.resource, Measure.library</b><br> 6204 * </p> 6205 */ 6206 @SearchParamDefinition(name = "depends-on", path = "Measure.relatedArtifact.where(type='depends-on').resource | Measure.library", description = "What resource is being referenced", type = "reference") 6207 public static final String SP_DEPENDS_ON = "depends-on"; 6208 /** 6209 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 6210 * <p> 6211 * Description: <b>What resource is being referenced</b><br> 6212 * Type: <b>reference</b><br> 6213 * Path: <b>Measure.relatedArtifact.resource, Measure.library</b><br> 6214 * </p> 6215 */ 6216 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 6217 SP_DEPENDS_ON); 6218 6219 /** 6220 * Constant for fluent queries to be used to add include statements. Specifies 6221 * the path value of "<b>Measure:depends-on</b>". 6222 */ 6223 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 6224 "Measure:depends-on").toLocked(); 6225 6226 /** 6227 * Search parameter: <b>name</b> 6228 * <p> 6229 * Description: <b>Computationally friendly name of the measure</b><br> 6230 * Type: <b>string</b><br> 6231 * Path: <b>Measure.name</b><br> 6232 * </p> 6233 */ 6234 @SearchParamDefinition(name = "name", path = "Measure.name", description = "Computationally friendly name of the measure", type = "string") 6235 public static final String SP_NAME = "name"; 6236 /** 6237 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6238 * <p> 6239 * Description: <b>Computationally friendly name of the measure</b><br> 6240 * Type: <b>string</b><br> 6241 * Path: <b>Measure.name</b><br> 6242 * </p> 6243 */ 6244 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 6245 SP_NAME); 6246 6247 /** 6248 * Search parameter: <b>context</b> 6249 * <p> 6250 * Description: <b>A use context assigned to the measure</b><br> 6251 * Type: <b>token</b><br> 6252 * Path: <b>Measure.useContext.valueCodeableConcept</b><br> 6253 * </p> 6254 */ 6255 @SearchParamDefinition(name = "context", path = "(Measure.useContext.value as CodeableConcept)", description = "A use context assigned to the measure", type = "token") 6256 public static final String SP_CONTEXT = "context"; 6257 /** 6258 * <b>Fluent Client</b> search parameter constant for <b>context</b> 6259 * <p> 6260 * Description: <b>A use context assigned to the measure</b><br> 6261 * Type: <b>token</b><br> 6262 * Path: <b>Measure.useContext.valueCodeableConcept</b><br> 6263 * </p> 6264 */ 6265 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6266 SP_CONTEXT); 6267 6268 /** 6269 * Search parameter: <b>publisher</b> 6270 * <p> 6271 * Description: <b>Name of the publisher of the measure</b><br> 6272 * Type: <b>string</b><br> 6273 * Path: <b>Measure.publisher</b><br> 6274 * </p> 6275 */ 6276 @SearchParamDefinition(name = "publisher", path = "Measure.publisher", description = "Name of the publisher of the measure", type = "string") 6277 public static final String SP_PUBLISHER = "publisher"; 6278 /** 6279 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6280 * <p> 6281 * Description: <b>Name of the publisher of the measure</b><br> 6282 * Type: <b>string</b><br> 6283 * Path: <b>Measure.publisher</b><br> 6284 * </p> 6285 */ 6286 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 6287 SP_PUBLISHER); 6288 6289 /** 6290 * Search parameter: <b>topic</b> 6291 * <p> 6292 * Description: <b>Topics associated with the measure</b><br> 6293 * Type: <b>token</b><br> 6294 * Path: <b>Measure.topic</b><br> 6295 * </p> 6296 */ 6297 @SearchParamDefinition(name = "topic", path = "Measure.topic", description = "Topics associated with the measure", type = "token") 6298 public static final String SP_TOPIC = "topic"; 6299 /** 6300 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 6301 * <p> 6302 * Description: <b>Topics associated with the measure</b><br> 6303 * Type: <b>token</b><br> 6304 * Path: <b>Measure.topic</b><br> 6305 * </p> 6306 */ 6307 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6308 SP_TOPIC); 6309 6310 /** 6311 * Search parameter: <b>context-type-quantity</b> 6312 * <p> 6313 * Description: <b>A use context type and quantity- or range-based value 6314 * assigned to the measure</b><br> 6315 * Type: <b>composite</b><br> 6316 * Path: <b></b><br> 6317 * </p> 6318 */ 6319 @SearchParamDefinition(name = "context-type-quantity", path = "Measure.useContext", description = "A use context type and quantity- or range-based value assigned to the measure", type = "composite", compositeOf = { 6320 "context-type", "context-quantity" }) 6321 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6322 /** 6323 * <b>Fluent Client</b> search parameter constant for 6324 * <b>context-type-quantity</b> 6325 * <p> 6326 * Description: <b>A use context type and quantity- or range-based value 6327 * assigned to the measure</b><br> 6328 * Type: <b>composite</b><br> 6329 * Path: <b></b><br> 6330 * </p> 6331 */ 6332 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 6333 SP_CONTEXT_TYPE_QUANTITY); 6334 6335 /** 6336 * Search parameter: <b>status</b> 6337 * <p> 6338 * Description: <b>The current status of the measure</b><br> 6339 * Type: <b>token</b><br> 6340 * Path: <b>Measure.status</b><br> 6341 * </p> 6342 */ 6343 @SearchParamDefinition(name = "status", path = "Measure.status", description = "The current status of the measure", type = "token") 6344 public static final String SP_STATUS = "status"; 6345 /** 6346 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6347 * <p> 6348 * Description: <b>The current status of the measure</b><br> 6349 * Type: <b>token</b><br> 6350 * Path: <b>Measure.status</b><br> 6351 * </p> 6352 */ 6353 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 6354 SP_STATUS); 6355 6356}