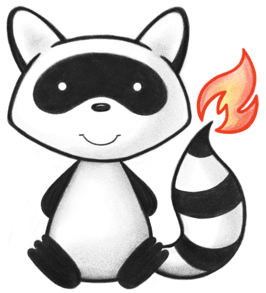
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The MeasureReport resource contains the results of the calculation of a 048 * measure; and optionally a reference to the resources involved in that 049 * calculation. 050 */ 051@ResourceDef(name = "MeasureReport", profile = "http://hl7.org/fhir/StructureDefinition/MeasureReport") 052public class MeasureReport extends DomainResource { 053 054 public enum MeasureReportStatus { 055 /** 056 * The report is complete and ready for use. 057 */ 058 COMPLETE, 059 /** 060 * The report is currently being generated. 061 */ 062 PENDING, 063 /** 064 * An error occurred attempting to generate the report. 065 */ 066 ERROR, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static MeasureReportStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("complete".equals(codeString)) 076 return COMPLETE; 077 if ("pending".equals(codeString)) 078 return PENDING; 079 if ("error".equals(codeString)) 080 return ERROR; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown MeasureReportStatus code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case COMPLETE: 090 return "complete"; 091 case PENDING: 092 return "pending"; 093 case ERROR: 094 return "error"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 switch (this) { 104 case COMPLETE: 105 return "http://hl7.org/fhir/measure-report-status"; 106 case PENDING: 107 return "http://hl7.org/fhir/measure-report-status"; 108 case ERROR: 109 return "http://hl7.org/fhir/measure-report-status"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case COMPLETE: 120 return "The report is complete and ready for use."; 121 case PENDING: 122 return "The report is currently being generated."; 123 case ERROR: 124 return "An error occurred attempting to generate the report."; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case COMPLETE: 135 return "Complete"; 136 case PENDING: 137 return "Pending"; 138 case ERROR: 139 return "Error"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 } 147 148 public static class MeasureReportStatusEnumFactory implements EnumFactory<MeasureReportStatus> { 149 public MeasureReportStatus fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("complete".equals(codeString)) 154 return MeasureReportStatus.COMPLETE; 155 if ("pending".equals(codeString)) 156 return MeasureReportStatus.PENDING; 157 if ("error".equals(codeString)) 158 return MeasureReportStatus.ERROR; 159 throw new IllegalArgumentException("Unknown MeasureReportStatus code '" + codeString + "'"); 160 } 161 162 public Enumeration<MeasureReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.NULL, code); 167 String codeString = code.asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.NULL, code); 170 if ("complete".equals(codeString)) 171 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.COMPLETE, code); 172 if ("pending".equals(codeString)) 173 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.PENDING, code); 174 if ("error".equals(codeString)) 175 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.ERROR, code); 176 throw new FHIRException("Unknown MeasureReportStatus code '" + codeString + "'"); 177 } 178 179 public String toCode(MeasureReportStatus code) { 180 if (code == MeasureReportStatus.NULL) 181 return null; 182 if (code == MeasureReportStatus.COMPLETE) 183 return "complete"; 184 if (code == MeasureReportStatus.PENDING) 185 return "pending"; 186 if (code == MeasureReportStatus.ERROR) 187 return "error"; 188 return "?"; 189 } 190 191 public String toSystem(MeasureReportStatus code) { 192 return code.getSystem(); 193 } 194 } 195 196 public enum MeasureReportType { 197 /** 198 * An individual report that provides information on the performance for a given 199 * measure with respect to a single subject. 200 */ 201 INDIVIDUAL, 202 /** 203 * A subject list report that includes a listing of subjects that satisfied each 204 * population criteria in the measure. 205 */ 206 SUBJECTLIST, 207 /** 208 * A summary report that returns the number of members in each population 209 * criteria for the measure. 210 */ 211 SUMMARY, 212 /** 213 * A data collection report that contains data-of-interest for the measure. 214 */ 215 DATACOLLECTION, 216 /** 217 * added to help the parsers with the generic types 218 */ 219 NULL; 220 221 public static MeasureReportType fromCode(String codeString) throws FHIRException { 222 if (codeString == null || "".equals(codeString)) 223 return null; 224 if ("individual".equals(codeString)) 225 return INDIVIDUAL; 226 if ("subject-list".equals(codeString)) 227 return SUBJECTLIST; 228 if ("summary".equals(codeString)) 229 return SUMMARY; 230 if ("data-collection".equals(codeString)) 231 return DATACOLLECTION; 232 if (Configuration.isAcceptInvalidEnums()) 233 return null; 234 else 235 throw new FHIRException("Unknown MeasureReportType code '" + codeString + "'"); 236 } 237 238 public String toCode() { 239 switch (this) { 240 case INDIVIDUAL: 241 return "individual"; 242 case SUBJECTLIST: 243 return "subject-list"; 244 case SUMMARY: 245 return "summary"; 246 case DATACOLLECTION: 247 return "data-collection"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 255 public String getSystem() { 256 switch (this) { 257 case INDIVIDUAL: 258 return "http://hl7.org/fhir/measure-report-type"; 259 case SUBJECTLIST: 260 return "http://hl7.org/fhir/measure-report-type"; 261 case SUMMARY: 262 return "http://hl7.org/fhir/measure-report-type"; 263 case DATACOLLECTION: 264 return "http://hl7.org/fhir/measure-report-type"; 265 case NULL: 266 return null; 267 default: 268 return "?"; 269 } 270 } 271 272 public String getDefinition() { 273 switch (this) { 274 case INDIVIDUAL: 275 return "An individual report that provides information on the performance for a given measure with respect to a single subject."; 276 case SUBJECTLIST: 277 return "A subject list report that includes a listing of subjects that satisfied each population criteria in the measure."; 278 case SUMMARY: 279 return "A summary report that returns the number of members in each population criteria for the measure."; 280 case DATACOLLECTION: 281 return "A data collection report that contains data-of-interest for the measure."; 282 case NULL: 283 return null; 284 default: 285 return "?"; 286 } 287 } 288 289 public String getDisplay() { 290 switch (this) { 291 case INDIVIDUAL: 292 return "Individual"; 293 case SUBJECTLIST: 294 return "Subject List"; 295 case SUMMARY: 296 return "Summary"; 297 case DATACOLLECTION: 298 return "Data Collection"; 299 case NULL: 300 return null; 301 default: 302 return "?"; 303 } 304 } 305 } 306 307 public static class MeasureReportTypeEnumFactory implements EnumFactory<MeasureReportType> { 308 public MeasureReportType fromCode(String codeString) throws IllegalArgumentException { 309 if (codeString == null || "".equals(codeString)) 310 if (codeString == null || "".equals(codeString)) 311 return null; 312 if ("individual".equals(codeString)) 313 return MeasureReportType.INDIVIDUAL; 314 if ("subject-list".equals(codeString)) 315 return MeasureReportType.SUBJECTLIST; 316 if ("summary".equals(codeString)) 317 return MeasureReportType.SUMMARY; 318 if ("data-collection".equals(codeString)) 319 return MeasureReportType.DATACOLLECTION; 320 throw new IllegalArgumentException("Unknown MeasureReportType code '" + codeString + "'"); 321 } 322 323 public Enumeration<MeasureReportType> fromType(PrimitiveType<?> code) throws FHIRException { 324 if (code == null) 325 return null; 326 if (code.isEmpty()) 327 return new Enumeration<MeasureReportType>(this, MeasureReportType.NULL, code); 328 String codeString = code.asStringValue(); 329 if (codeString == null || "".equals(codeString)) 330 return new Enumeration<MeasureReportType>(this, MeasureReportType.NULL, code); 331 if ("individual".equals(codeString)) 332 return new Enumeration<MeasureReportType>(this, MeasureReportType.INDIVIDUAL, code); 333 if ("subject-list".equals(codeString)) 334 return new Enumeration<MeasureReportType>(this, MeasureReportType.SUBJECTLIST, code); 335 if ("summary".equals(codeString)) 336 return new Enumeration<MeasureReportType>(this, MeasureReportType.SUMMARY, code); 337 if ("data-collection".equals(codeString)) 338 return new Enumeration<MeasureReportType>(this, MeasureReportType.DATACOLLECTION, code); 339 throw new FHIRException("Unknown MeasureReportType code '" + codeString + "'"); 340 } 341 342 public String toCode(MeasureReportType code) { 343 if (code == MeasureReportType.NULL) 344 return null; 345 if (code == MeasureReportType.INDIVIDUAL) 346 return "individual"; 347 if (code == MeasureReportType.SUBJECTLIST) 348 return "subject-list"; 349 if (code == MeasureReportType.SUMMARY) 350 return "summary"; 351 if (code == MeasureReportType.DATACOLLECTION) 352 return "data-collection"; 353 return "?"; 354 } 355 356 public String toSystem(MeasureReportType code) { 357 return code.getSystem(); 358 } 359 } 360 361 @Block() 362 public static class MeasureReportGroupComponent extends BackboneElement implements IBaseBackboneElement { 363 /** 364 * The meaning of the population group as defined in the measure definition. 365 */ 366 @Child(name = "code", type = { 367 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 368 @Description(shortDefinition = "Meaning of the group", formalDefinition = "The meaning of the population group as defined in the measure definition.") 369 protected CodeableConcept code; 370 371 /** 372 * The populations that make up the population group, one for each type of 373 * population appropriate for the measure. 374 */ 375 @Child(name = "population", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 376 @Description(shortDefinition = "The populations in the group", formalDefinition = "The populations that make up the population group, one for each type of population appropriate for the measure.") 377 protected List<MeasureReportGroupPopulationComponent> population; 378 379 /** 380 * The measure score for this population group, calculated as appropriate for 381 * the measure type and scoring method, and based on the contents of the 382 * populations defined in the group. 383 */ 384 @Child(name = "measureScore", type = { 385 Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 386 @Description(shortDefinition = "What score this group achieved", formalDefinition = "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.") 387 protected Quantity measureScore; 388 389 /** 390 * When a measure includes multiple stratifiers, there will be a stratifier 391 * group for each stratifier defined by the measure. 392 */ 393 @Child(name = "stratifier", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 394 @Description(shortDefinition = "Stratification results", formalDefinition = "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.") 395 protected List<MeasureReportGroupStratifierComponent> stratifier; 396 397 private static final long serialVersionUID = 1744426009L; 398 399 /** 400 * Constructor 401 */ 402 public MeasureReportGroupComponent() { 403 super(); 404 } 405 406 /** 407 * @return {@link #code} (The meaning of the population group as defined in the 408 * measure definition.) 409 */ 410 public CodeableConcept getCode() { 411 if (this.code == null) 412 if (Configuration.errorOnAutoCreate()) 413 throw new Error("Attempt to auto-create MeasureReportGroupComponent.code"); 414 else if (Configuration.doAutoCreate()) 415 this.code = new CodeableConcept(); // cc 416 return this.code; 417 } 418 419 public boolean hasCode() { 420 return this.code != null && !this.code.isEmpty(); 421 } 422 423 /** 424 * @param value {@link #code} (The meaning of the population group as defined in 425 * the measure definition.) 426 */ 427 public MeasureReportGroupComponent setCode(CodeableConcept value) { 428 this.code = value; 429 return this; 430 } 431 432 /** 433 * @return {@link #population} (The populations that make up the population 434 * group, one for each type of population appropriate for the measure.) 435 */ 436 public List<MeasureReportGroupPopulationComponent> getPopulation() { 437 if (this.population == null) 438 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 439 return this.population; 440 } 441 442 /** 443 * @return Returns a reference to <code>this</code> for easy method chaining 444 */ 445 public MeasureReportGroupComponent setPopulation(List<MeasureReportGroupPopulationComponent> thePopulation) { 446 this.population = thePopulation; 447 return this; 448 } 449 450 public boolean hasPopulation() { 451 if (this.population == null) 452 return false; 453 for (MeasureReportGroupPopulationComponent item : this.population) 454 if (!item.isEmpty()) 455 return true; 456 return false; 457 } 458 459 public MeasureReportGroupPopulationComponent addPopulation() { // 3 460 MeasureReportGroupPopulationComponent t = new MeasureReportGroupPopulationComponent(); 461 if (this.population == null) 462 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 463 this.population.add(t); 464 return t; 465 } 466 467 public MeasureReportGroupComponent addPopulation(MeasureReportGroupPopulationComponent t) { // 3 468 if (t == null) 469 return this; 470 if (this.population == null) 471 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 472 this.population.add(t); 473 return this; 474 } 475 476 /** 477 * @return The first repetition of repeating field {@link #population}, creating 478 * it if it does not already exist 479 */ 480 public MeasureReportGroupPopulationComponent getPopulationFirstRep() { 481 if (getPopulation().isEmpty()) { 482 addPopulation(); 483 } 484 return getPopulation().get(0); 485 } 486 487 /** 488 * @return {@link #measureScore} (The measure score for this population group, 489 * calculated as appropriate for the measure type and scoring method, 490 * and based on the contents of the populations defined in the group.) 491 */ 492 public Quantity getMeasureScore() { 493 if (this.measureScore == null) 494 if (Configuration.errorOnAutoCreate()) 495 throw new Error("Attempt to auto-create MeasureReportGroupComponent.measureScore"); 496 else if (Configuration.doAutoCreate()) 497 this.measureScore = new Quantity(); // cc 498 return this.measureScore; 499 } 500 501 public boolean hasMeasureScore() { 502 return this.measureScore != null && !this.measureScore.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #measureScore} (The measure score for this population 507 * group, calculated as appropriate for the measure type and 508 * scoring method, and based on the contents of the populations 509 * defined in the group.) 510 */ 511 public MeasureReportGroupComponent setMeasureScore(Quantity value) { 512 this.measureScore = value; 513 return this; 514 } 515 516 /** 517 * @return {@link #stratifier} (When a measure includes multiple stratifiers, 518 * there will be a stratifier group for each stratifier defined by the 519 * measure.) 520 */ 521 public List<MeasureReportGroupStratifierComponent> getStratifier() { 522 if (this.stratifier == null) 523 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 524 return this.stratifier; 525 } 526 527 /** 528 * @return Returns a reference to <code>this</code> for easy method chaining 529 */ 530 public MeasureReportGroupComponent setStratifier(List<MeasureReportGroupStratifierComponent> theStratifier) { 531 this.stratifier = theStratifier; 532 return this; 533 } 534 535 public boolean hasStratifier() { 536 if (this.stratifier == null) 537 return false; 538 for (MeasureReportGroupStratifierComponent item : this.stratifier) 539 if (!item.isEmpty()) 540 return true; 541 return false; 542 } 543 544 public MeasureReportGroupStratifierComponent addStratifier() { // 3 545 MeasureReportGroupStratifierComponent t = new MeasureReportGroupStratifierComponent(); 546 if (this.stratifier == null) 547 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 548 this.stratifier.add(t); 549 return t; 550 } 551 552 public MeasureReportGroupComponent addStratifier(MeasureReportGroupStratifierComponent t) { // 3 553 if (t == null) 554 return this; 555 if (this.stratifier == null) 556 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 557 this.stratifier.add(t); 558 return this; 559 } 560 561 /** 562 * @return The first repetition of repeating field {@link #stratifier}, creating 563 * it if it does not already exist 564 */ 565 public MeasureReportGroupStratifierComponent getStratifierFirstRep() { 566 if (getStratifier().isEmpty()) { 567 addStratifier(); 568 } 569 return getStratifier().get(0); 570 } 571 572 protected void listChildren(List<Property> children) { 573 super.listChildren(children); 574 children.add(new Property("code", "CodeableConcept", 575 "The meaning of the population group as defined in the measure definition.", 0, 1, code)); 576 children.add(new Property("population", "", 577 "The populations that make up the population group, one for each type of population appropriate for the measure.", 578 0, java.lang.Integer.MAX_VALUE, population)); 579 children.add(new Property("measureScore", "Quantity", 580 "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 581 0, 1, measureScore)); 582 children.add(new Property("stratifier", "", 583 "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.", 584 0, java.lang.Integer.MAX_VALUE, stratifier)); 585 } 586 587 @Override 588 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 589 switch (_hash) { 590 case 3059181: 591 /* code */ return new Property("code", "CodeableConcept", 592 "The meaning of the population group as defined in the measure definition.", 0, 1, code); 593 case -2023558323: 594 /* population */ return new Property("population", "", 595 "The populations that make up the population group, one for each type of population appropriate for the measure.", 596 0, java.lang.Integer.MAX_VALUE, population); 597 case -386313260: 598 /* measureScore */ return new Property("measureScore", "Quantity", 599 "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 600 0, 1, measureScore); 601 case 90983669: 602 /* stratifier */ return new Property("stratifier", "", 603 "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.", 604 0, java.lang.Integer.MAX_VALUE, stratifier); 605 default: 606 return super.getNamedProperty(_hash, _name, _checkValid); 607 } 608 609 } 610 611 @Override 612 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 613 switch (hash) { 614 case 3059181: 615 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 616 case -2023558323: 617 /* population */ return this.population == null ? new Base[0] 618 : this.population.toArray(new Base[this.population.size()]); // MeasureReportGroupPopulationComponent 619 case -386313260: 620 /* measureScore */ return this.measureScore == null ? new Base[0] : new Base[] { this.measureScore }; // Quantity 621 case 90983669: 622 /* stratifier */ return this.stratifier == null ? new Base[0] 623 : this.stratifier.toArray(new Base[this.stratifier.size()]); // MeasureReportGroupStratifierComponent 624 default: 625 return super.getProperty(hash, name, checkValid); 626 } 627 628 } 629 630 @Override 631 public Base setProperty(int hash, String name, Base value) throws FHIRException { 632 switch (hash) { 633 case 3059181: // code 634 this.code = castToCodeableConcept(value); // CodeableConcept 635 return value; 636 case -2023558323: // population 637 this.getPopulation().add((MeasureReportGroupPopulationComponent) value); // MeasureReportGroupPopulationComponent 638 return value; 639 case -386313260: // measureScore 640 this.measureScore = castToQuantity(value); // Quantity 641 return value; 642 case 90983669: // stratifier 643 this.getStratifier().add((MeasureReportGroupStratifierComponent) value); // MeasureReportGroupStratifierComponent 644 return value; 645 default: 646 return super.setProperty(hash, name, value); 647 } 648 649 } 650 651 @Override 652 public Base setProperty(String name, Base value) throws FHIRException { 653 if (name.equals("code")) { 654 this.code = castToCodeableConcept(value); // CodeableConcept 655 } else if (name.equals("population")) { 656 this.getPopulation().add((MeasureReportGroupPopulationComponent) value); 657 } else if (name.equals("measureScore")) { 658 this.measureScore = castToQuantity(value); // Quantity 659 } else if (name.equals("stratifier")) { 660 this.getStratifier().add((MeasureReportGroupStratifierComponent) value); 661 } else 662 return super.setProperty(name, value); 663 return value; 664 } 665 666 @Override 667 public void removeChild(String name, Base value) throws FHIRException { 668 if (name.equals("code")) { 669 this.code = null; 670 } else if (name.equals("population")) { 671 this.getPopulation().remove((MeasureReportGroupPopulationComponent) value); 672 } else if (name.equals("measureScore")) { 673 this.measureScore = null; 674 } else if (name.equals("stratifier")) { 675 this.getStratifier().remove((MeasureReportGroupStratifierComponent) value); 676 } else 677 super.removeChild(name, value); 678 679 } 680 681 @Override 682 public Base makeProperty(int hash, String name) throws FHIRException { 683 switch (hash) { 684 case 3059181: 685 return getCode(); 686 case -2023558323: 687 return addPopulation(); 688 case -386313260: 689 return getMeasureScore(); 690 case 90983669: 691 return addStratifier(); 692 default: 693 return super.makeProperty(hash, name); 694 } 695 696 } 697 698 @Override 699 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 700 switch (hash) { 701 case 3059181: 702 /* code */ return new String[] { "CodeableConcept" }; 703 case -2023558323: 704 /* population */ return new String[] {}; 705 case -386313260: 706 /* measureScore */ return new String[] { "Quantity" }; 707 case 90983669: 708 /* stratifier */ return new String[] {}; 709 default: 710 return super.getTypesForProperty(hash, name); 711 } 712 713 } 714 715 @Override 716 public Base addChild(String name) throws FHIRException { 717 if (name.equals("code")) { 718 this.code = new CodeableConcept(); 719 return this.code; 720 } else if (name.equals("population")) { 721 return addPopulation(); 722 } else if (name.equals("measureScore")) { 723 this.measureScore = new Quantity(); 724 return this.measureScore; 725 } else if (name.equals("stratifier")) { 726 return addStratifier(); 727 } else 728 return super.addChild(name); 729 } 730 731 public MeasureReportGroupComponent copy() { 732 MeasureReportGroupComponent dst = new MeasureReportGroupComponent(); 733 copyValues(dst); 734 return dst; 735 } 736 737 public void copyValues(MeasureReportGroupComponent dst) { 738 super.copyValues(dst); 739 dst.code = code == null ? null : code.copy(); 740 if (population != null) { 741 dst.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 742 for (MeasureReportGroupPopulationComponent i : population) 743 dst.population.add(i.copy()); 744 } 745 ; 746 dst.measureScore = measureScore == null ? null : measureScore.copy(); 747 if (stratifier != null) { 748 dst.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 749 for (MeasureReportGroupStratifierComponent i : stratifier) 750 dst.stratifier.add(i.copy()); 751 } 752 ; 753 } 754 755 @Override 756 public boolean equalsDeep(Base other_) { 757 if (!super.equalsDeep(other_)) 758 return false; 759 if (!(other_ instanceof MeasureReportGroupComponent)) 760 return false; 761 MeasureReportGroupComponent o = (MeasureReportGroupComponent) other_; 762 return compareDeep(code, o.code, true) && compareDeep(population, o.population, true) 763 && compareDeep(measureScore, o.measureScore, true) && compareDeep(stratifier, o.stratifier, true); 764 } 765 766 @Override 767 public boolean equalsShallow(Base other_) { 768 if (!super.equalsShallow(other_)) 769 return false; 770 if (!(other_ instanceof MeasureReportGroupComponent)) 771 return false; 772 MeasureReportGroupComponent o = (MeasureReportGroupComponent) other_; 773 return true; 774 } 775 776 public boolean isEmpty() { 777 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, population, measureScore, stratifier); 778 } 779 780 public String fhirType() { 781 return "MeasureReport.group"; 782 783 } 784 785 } 786 787 @Block() 788 public static class MeasureReportGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 789 /** 790 * The type of the population. 791 */ 792 @Child(name = "code", type = { 793 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 794 @Description(shortDefinition = "initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition = "The type of the population.") 795 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-population") 796 protected CodeableConcept code; 797 798 /** 799 * The number of members of the population. 800 */ 801 @Child(name = "count", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 802 @Description(shortDefinition = "Size of the population", formalDefinition = "The number of members of the population.") 803 protected IntegerType count; 804 805 /** 806 * This element refers to a List of subject level MeasureReport resources, one 807 * for each subject in this population. 808 */ 809 @Child(name = "subjectResults", type = { 810 ListResource.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 811 @Description(shortDefinition = "For subject-list reports, the subject results in this population", formalDefinition = "This element refers to a List of subject level MeasureReport resources, one for each subject in this population.") 812 protected Reference subjectResults; 813 814 /** 815 * The actual object that is the target of the reference (This element refers to 816 * a List of subject level MeasureReport resources, one for each subject in this 817 * population.) 818 */ 819 protected ListResource subjectResultsTarget; 820 821 private static final long serialVersionUID = 210461445L; 822 823 /** 824 * Constructor 825 */ 826 public MeasureReportGroupPopulationComponent() { 827 super(); 828 } 829 830 /** 831 * @return {@link #code} (The type of the population.) 832 */ 833 public CodeableConcept getCode() { 834 if (this.code == null) 835 if (Configuration.errorOnAutoCreate()) 836 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.code"); 837 else if (Configuration.doAutoCreate()) 838 this.code = new CodeableConcept(); // cc 839 return this.code; 840 } 841 842 public boolean hasCode() { 843 return this.code != null && !this.code.isEmpty(); 844 } 845 846 /** 847 * @param value {@link #code} (The type of the population.) 848 */ 849 public MeasureReportGroupPopulationComponent setCode(CodeableConcept value) { 850 this.code = value; 851 return this; 852 } 853 854 /** 855 * @return {@link #count} (The number of members of the population.). This is 856 * the underlying object with id, value and extensions. The accessor 857 * "getCount" gives direct access to the value 858 */ 859 public IntegerType getCountElement() { 860 if (this.count == null) 861 if (Configuration.errorOnAutoCreate()) 862 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.count"); 863 else if (Configuration.doAutoCreate()) 864 this.count = new IntegerType(); // bb 865 return this.count; 866 } 867 868 public boolean hasCountElement() { 869 return this.count != null && !this.count.isEmpty(); 870 } 871 872 public boolean hasCount() { 873 return this.count != null && !this.count.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #count} (The number of members of the population.). This 878 * is the underlying object with id, value and extensions. The 879 * accessor "getCount" gives direct access to the value 880 */ 881 public MeasureReportGroupPopulationComponent setCountElement(IntegerType value) { 882 this.count = value; 883 return this; 884 } 885 886 /** 887 * @return The number of members of the population. 888 */ 889 public int getCount() { 890 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 891 } 892 893 /** 894 * @param value The number of members of the population. 895 */ 896 public MeasureReportGroupPopulationComponent setCount(int value) { 897 if (this.count == null) 898 this.count = new IntegerType(); 899 this.count.setValue(value); 900 return this; 901 } 902 903 /** 904 * @return {@link #subjectResults} (This element refers to a List of subject 905 * level MeasureReport resources, one for each subject in this 906 * population.) 907 */ 908 public Reference getSubjectResults() { 909 if (this.subjectResults == null) 910 if (Configuration.errorOnAutoCreate()) 911 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.subjectResults"); 912 else if (Configuration.doAutoCreate()) 913 this.subjectResults = new Reference(); // cc 914 return this.subjectResults; 915 } 916 917 public boolean hasSubjectResults() { 918 return this.subjectResults != null && !this.subjectResults.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #subjectResults} (This element refers to a List of 923 * subject level MeasureReport resources, one for each subject in 924 * this population.) 925 */ 926 public MeasureReportGroupPopulationComponent setSubjectResults(Reference value) { 927 this.subjectResults = value; 928 return this; 929 } 930 931 /** 932 * @return {@link #subjectResults} The actual object that is the target of the 933 * reference. The reference library doesn't populate this, but you can 934 * use it to hold the resource if you resolve it. (This element refers 935 * to a List of subject level MeasureReport resources, one for each 936 * subject in this population.) 937 */ 938 public ListResource getSubjectResultsTarget() { 939 if (this.subjectResultsTarget == null) 940 if (Configuration.errorOnAutoCreate()) 941 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.subjectResults"); 942 else if (Configuration.doAutoCreate()) 943 this.subjectResultsTarget = new ListResource(); // aa 944 return this.subjectResultsTarget; 945 } 946 947 /** 948 * @param value {@link #subjectResults} The actual object that is the target of 949 * the reference. The reference library doesn't use these, but you 950 * can use it to hold the resource if you resolve it. (This element 951 * refers to a List of subject level MeasureReport resources, one 952 * for each subject in this population.) 953 */ 954 public MeasureReportGroupPopulationComponent setSubjectResultsTarget(ListResource value) { 955 this.subjectResultsTarget = value; 956 return this; 957 } 958 959 protected void listChildren(List<Property> children) { 960 super.listChildren(children); 961 children.add(new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code)); 962 children.add(new Property("count", "integer", "The number of members of the population.", 0, 1, count)); 963 children.add(new Property("subjectResults", "Reference(List)", 964 "This element refers to a List of subject level MeasureReport resources, one for each subject in this population.", 965 0, 1, subjectResults)); 966 } 967 968 @Override 969 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 970 switch (_hash) { 971 case 3059181: 972 /* code */ return new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code); 973 case 94851343: 974 /* count */ return new Property("count", "integer", "The number of members of the population.", 0, 1, count); 975 case 2136184106: 976 /* subjectResults */ return new Property("subjectResults", "Reference(List)", 977 "This element refers to a List of subject level MeasureReport resources, one for each subject in this population.", 978 0, 1, subjectResults); 979 default: 980 return super.getNamedProperty(_hash, _name, _checkValid); 981 } 982 983 } 984 985 @Override 986 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 987 switch (hash) { 988 case 3059181: 989 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 990 case 94851343: 991 /* count */ return this.count == null ? new Base[0] : new Base[] { this.count }; // IntegerType 992 case 2136184106: 993 /* subjectResults */ return this.subjectResults == null ? new Base[0] : new Base[] { this.subjectResults }; // Reference 994 default: 995 return super.getProperty(hash, name, checkValid); 996 } 997 998 } 999 1000 @Override 1001 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1002 switch (hash) { 1003 case 3059181: // code 1004 this.code = castToCodeableConcept(value); // CodeableConcept 1005 return value; 1006 case 94851343: // count 1007 this.count = castToInteger(value); // IntegerType 1008 return value; 1009 case 2136184106: // subjectResults 1010 this.subjectResults = castToReference(value); // Reference 1011 return value; 1012 default: 1013 return super.setProperty(hash, name, value); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(String name, Base value) throws FHIRException { 1020 if (name.equals("code")) { 1021 this.code = castToCodeableConcept(value); // CodeableConcept 1022 } else if (name.equals("count")) { 1023 this.count = castToInteger(value); // IntegerType 1024 } else if (name.equals("subjectResults")) { 1025 this.subjectResults = castToReference(value); // Reference 1026 } else 1027 return super.setProperty(name, value); 1028 return value; 1029 } 1030 1031 @Override 1032 public void removeChild(String name, Base value) throws FHIRException { 1033 if (name.equals("code")) { 1034 this.code = null; 1035 } else if (name.equals("count")) { 1036 this.count = null; 1037 } else if (name.equals("subjectResults")) { 1038 this.subjectResults = null; 1039 } else 1040 super.removeChild(name, value); 1041 1042 } 1043 1044 @Override 1045 public Base makeProperty(int hash, String name) throws FHIRException { 1046 switch (hash) { 1047 case 3059181: 1048 return getCode(); 1049 case 94851343: 1050 return getCountElement(); 1051 case 2136184106: 1052 return getSubjectResults(); 1053 default: 1054 return super.makeProperty(hash, name); 1055 } 1056 1057 } 1058 1059 @Override 1060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1061 switch (hash) { 1062 case 3059181: 1063 /* code */ return new String[] { "CodeableConcept" }; 1064 case 94851343: 1065 /* count */ return new String[] { "integer" }; 1066 case 2136184106: 1067 /* subjectResults */ return new String[] { "Reference" }; 1068 default: 1069 return super.getTypesForProperty(hash, name); 1070 } 1071 1072 } 1073 1074 @Override 1075 public Base addChild(String name) throws FHIRException { 1076 if (name.equals("code")) { 1077 this.code = new CodeableConcept(); 1078 return this.code; 1079 } else if (name.equals("count")) { 1080 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.count"); 1081 } else if (name.equals("subjectResults")) { 1082 this.subjectResults = new Reference(); 1083 return this.subjectResults; 1084 } else 1085 return super.addChild(name); 1086 } 1087 1088 public MeasureReportGroupPopulationComponent copy() { 1089 MeasureReportGroupPopulationComponent dst = new MeasureReportGroupPopulationComponent(); 1090 copyValues(dst); 1091 return dst; 1092 } 1093 1094 public void copyValues(MeasureReportGroupPopulationComponent dst) { 1095 super.copyValues(dst); 1096 dst.code = code == null ? null : code.copy(); 1097 dst.count = count == null ? null : count.copy(); 1098 dst.subjectResults = subjectResults == null ? null : subjectResults.copy(); 1099 } 1100 1101 @Override 1102 public boolean equalsDeep(Base other_) { 1103 if (!super.equalsDeep(other_)) 1104 return false; 1105 if (!(other_ instanceof MeasureReportGroupPopulationComponent)) 1106 return false; 1107 MeasureReportGroupPopulationComponent o = (MeasureReportGroupPopulationComponent) other_; 1108 return compareDeep(code, o.code, true) && compareDeep(count, o.count, true) 1109 && compareDeep(subjectResults, o.subjectResults, true); 1110 } 1111 1112 @Override 1113 public boolean equalsShallow(Base other_) { 1114 if (!super.equalsShallow(other_)) 1115 return false; 1116 if (!(other_ instanceof MeasureReportGroupPopulationComponent)) 1117 return false; 1118 MeasureReportGroupPopulationComponent o = (MeasureReportGroupPopulationComponent) other_; 1119 return compareValues(count, o.count, true); 1120 } 1121 1122 public boolean isEmpty() { 1123 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, count, subjectResults); 1124 } 1125 1126 public String fhirType() { 1127 return "MeasureReport.group.population"; 1128 1129 } 1130 1131 } 1132 1133 @Block() 1134 public static class MeasureReportGroupStratifierComponent extends BackboneElement implements IBaseBackboneElement { 1135 /** 1136 * The meaning of this stratifier, as defined in the measure definition. 1137 */ 1138 @Child(name = "code", type = { 1139 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1140 @Description(shortDefinition = "What stratifier of the group", formalDefinition = "The meaning of this stratifier, as defined in the measure definition.") 1141 protected List<CodeableConcept> code; 1142 1143 /** 1144 * This element contains the results for a single stratum within the stratifier. 1145 * For example, when stratifying on administrative gender, there will be four 1146 * strata, one for each possible gender value. 1147 */ 1148 @Child(name = "stratum", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1149 @Description(shortDefinition = "Stratum results, one for each unique value, or set of values, in the stratifier, or stratifier components", formalDefinition = "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.") 1150 protected List<StratifierGroupComponent> stratum; 1151 1152 private static final long serialVersionUID = 259550185L; 1153 1154 /** 1155 * Constructor 1156 */ 1157 public MeasureReportGroupStratifierComponent() { 1158 super(); 1159 } 1160 1161 /** 1162 * @return {@link #code} (The meaning of this stratifier, as defined in the 1163 * measure definition.) 1164 */ 1165 public List<CodeableConcept> getCode() { 1166 if (this.code == null) 1167 this.code = new ArrayList<CodeableConcept>(); 1168 return this.code; 1169 } 1170 1171 /** 1172 * @return Returns a reference to <code>this</code> for easy method chaining 1173 */ 1174 public MeasureReportGroupStratifierComponent setCode(List<CodeableConcept> theCode) { 1175 this.code = theCode; 1176 return this; 1177 } 1178 1179 public boolean hasCode() { 1180 if (this.code == null) 1181 return false; 1182 for (CodeableConcept item : this.code) 1183 if (!item.isEmpty()) 1184 return true; 1185 return false; 1186 } 1187 1188 public CodeableConcept addCode() { // 3 1189 CodeableConcept t = new CodeableConcept(); 1190 if (this.code == null) 1191 this.code = new ArrayList<CodeableConcept>(); 1192 this.code.add(t); 1193 return t; 1194 } 1195 1196 public MeasureReportGroupStratifierComponent addCode(CodeableConcept t) { // 3 1197 if (t == null) 1198 return this; 1199 if (this.code == null) 1200 this.code = new ArrayList<CodeableConcept>(); 1201 this.code.add(t); 1202 return this; 1203 } 1204 1205 /** 1206 * @return The first repetition of repeating field {@link #code}, creating it if 1207 * it does not already exist 1208 */ 1209 public CodeableConcept getCodeFirstRep() { 1210 if (getCode().isEmpty()) { 1211 addCode(); 1212 } 1213 return getCode().get(0); 1214 } 1215 1216 /** 1217 * @return {@link #stratum} (This element contains the results for a single 1218 * stratum within the stratifier. For example, when stratifying on 1219 * administrative gender, there will be four strata, one for each 1220 * possible gender value.) 1221 */ 1222 public List<StratifierGroupComponent> getStratum() { 1223 if (this.stratum == null) 1224 this.stratum = new ArrayList<StratifierGroupComponent>(); 1225 return this.stratum; 1226 } 1227 1228 /** 1229 * @return Returns a reference to <code>this</code> for easy method chaining 1230 */ 1231 public MeasureReportGroupStratifierComponent setStratum(List<StratifierGroupComponent> theStratum) { 1232 this.stratum = theStratum; 1233 return this; 1234 } 1235 1236 public boolean hasStratum() { 1237 if (this.stratum == null) 1238 return false; 1239 for (StratifierGroupComponent item : this.stratum) 1240 if (!item.isEmpty()) 1241 return true; 1242 return false; 1243 } 1244 1245 public StratifierGroupComponent addStratum() { // 3 1246 StratifierGroupComponent t = new StratifierGroupComponent(); 1247 if (this.stratum == null) 1248 this.stratum = new ArrayList<StratifierGroupComponent>(); 1249 this.stratum.add(t); 1250 return t; 1251 } 1252 1253 public MeasureReportGroupStratifierComponent addStratum(StratifierGroupComponent t) { // 3 1254 if (t == null) 1255 return this; 1256 if (this.stratum == null) 1257 this.stratum = new ArrayList<StratifierGroupComponent>(); 1258 this.stratum.add(t); 1259 return this; 1260 } 1261 1262 /** 1263 * @return The first repetition of repeating field {@link #stratum}, creating it 1264 * if it does not already exist 1265 */ 1266 public StratifierGroupComponent getStratumFirstRep() { 1267 if (getStratum().isEmpty()) { 1268 addStratum(); 1269 } 1270 return getStratum().get(0); 1271 } 1272 1273 protected void listChildren(List<Property> children) { 1274 super.listChildren(children); 1275 children.add(new Property("code", "CodeableConcept", 1276 "The meaning of this stratifier, as defined in the measure definition.", 0, java.lang.Integer.MAX_VALUE, 1277 code)); 1278 children.add(new Property("stratum", "", 1279 "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.", 1280 0, java.lang.Integer.MAX_VALUE, stratum)); 1281 } 1282 1283 @Override 1284 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1285 switch (_hash) { 1286 case 3059181: 1287 /* code */ return new Property("code", "CodeableConcept", 1288 "The meaning of this stratifier, as defined in the measure definition.", 0, java.lang.Integer.MAX_VALUE, 1289 code); 1290 case -1881991236: 1291 /* stratum */ return new Property("stratum", "", 1292 "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.", 1293 0, java.lang.Integer.MAX_VALUE, stratum); 1294 default: 1295 return super.getNamedProperty(_hash, _name, _checkValid); 1296 } 1297 1298 } 1299 1300 @Override 1301 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1302 switch (hash) { 1303 case 3059181: 1304 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 1305 case -1881991236: 1306 /* stratum */ return this.stratum == null ? new Base[0] : this.stratum.toArray(new Base[this.stratum.size()]); // StratifierGroupComponent 1307 default: 1308 return super.getProperty(hash, name, checkValid); 1309 } 1310 1311 } 1312 1313 @Override 1314 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1315 switch (hash) { 1316 case 3059181: // code 1317 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 1318 return value; 1319 case -1881991236: // stratum 1320 this.getStratum().add((StratifierGroupComponent) value); // StratifierGroupComponent 1321 return value; 1322 default: 1323 return super.setProperty(hash, name, value); 1324 } 1325 1326 } 1327 1328 @Override 1329 public Base setProperty(String name, Base value) throws FHIRException { 1330 if (name.equals("code")) { 1331 this.getCode().add(castToCodeableConcept(value)); 1332 } else if (name.equals("stratum")) { 1333 this.getStratum().add((StratifierGroupComponent) value); 1334 } else 1335 return super.setProperty(name, value); 1336 return value; 1337 } 1338 1339 @Override 1340 public void removeChild(String name, Base value) throws FHIRException { 1341 if (name.equals("code")) { 1342 this.getCode().remove(castToCodeableConcept(value)); 1343 } else if (name.equals("stratum")) { 1344 this.getStratum().remove((StratifierGroupComponent) value); 1345 } else 1346 super.removeChild(name, value); 1347 1348 } 1349 1350 @Override 1351 public Base makeProperty(int hash, String name) throws FHIRException { 1352 switch (hash) { 1353 case 3059181: 1354 return addCode(); 1355 case -1881991236: 1356 return addStratum(); 1357 default: 1358 return super.makeProperty(hash, name); 1359 } 1360 1361 } 1362 1363 @Override 1364 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1365 switch (hash) { 1366 case 3059181: 1367 /* code */ return new String[] { "CodeableConcept" }; 1368 case -1881991236: 1369 /* stratum */ return new String[] {}; 1370 default: 1371 return super.getTypesForProperty(hash, name); 1372 } 1373 1374 } 1375 1376 @Override 1377 public Base addChild(String name) throws FHIRException { 1378 if (name.equals("code")) { 1379 return addCode(); 1380 } else if (name.equals("stratum")) { 1381 return addStratum(); 1382 } else 1383 return super.addChild(name); 1384 } 1385 1386 public MeasureReportGroupStratifierComponent copy() { 1387 MeasureReportGroupStratifierComponent dst = new MeasureReportGroupStratifierComponent(); 1388 copyValues(dst); 1389 return dst; 1390 } 1391 1392 public void copyValues(MeasureReportGroupStratifierComponent dst) { 1393 super.copyValues(dst); 1394 if (code != null) { 1395 dst.code = new ArrayList<CodeableConcept>(); 1396 for (CodeableConcept i : code) 1397 dst.code.add(i.copy()); 1398 } 1399 ; 1400 if (stratum != null) { 1401 dst.stratum = new ArrayList<StratifierGroupComponent>(); 1402 for (StratifierGroupComponent i : stratum) 1403 dst.stratum.add(i.copy()); 1404 } 1405 ; 1406 } 1407 1408 @Override 1409 public boolean equalsDeep(Base other_) { 1410 if (!super.equalsDeep(other_)) 1411 return false; 1412 if (!(other_ instanceof MeasureReportGroupStratifierComponent)) 1413 return false; 1414 MeasureReportGroupStratifierComponent o = (MeasureReportGroupStratifierComponent) other_; 1415 return compareDeep(code, o.code, true) && compareDeep(stratum, o.stratum, true); 1416 } 1417 1418 @Override 1419 public boolean equalsShallow(Base other_) { 1420 if (!super.equalsShallow(other_)) 1421 return false; 1422 if (!(other_ instanceof MeasureReportGroupStratifierComponent)) 1423 return false; 1424 MeasureReportGroupStratifierComponent o = (MeasureReportGroupStratifierComponent) other_; 1425 return true; 1426 } 1427 1428 public boolean isEmpty() { 1429 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, stratum); 1430 } 1431 1432 public String fhirType() { 1433 return "MeasureReport.group.stratifier"; 1434 1435 } 1436 1437 } 1438 1439 @Block() 1440 public static class StratifierGroupComponent extends BackboneElement implements IBaseBackboneElement { 1441 /** 1442 * The value for this stratum, expressed as a CodeableConcept. When defining 1443 * stratifiers on complex values, the value must be rendered such that the value 1444 * for each stratum within the stratifier is unique. 1445 */ 1446 @Child(name = "value", type = { 1447 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1448 @Description(shortDefinition = "The stratum value, e.g. male", formalDefinition = "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.") 1449 protected CodeableConcept value; 1450 1451 /** 1452 * A stratifier component value. 1453 */ 1454 @Child(name = "component", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1455 @Description(shortDefinition = "Stratifier component values", formalDefinition = "A stratifier component value.") 1456 protected List<StratifierGroupComponentComponent> component; 1457 1458 /** 1459 * The populations that make up the stratum, one for each type of population 1460 * appropriate to the measure. 1461 */ 1462 @Child(name = "population", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1463 @Description(shortDefinition = "Population results in this stratum", formalDefinition = "The populations that make up the stratum, one for each type of population appropriate to the measure.") 1464 protected List<StratifierGroupPopulationComponent> population; 1465 1466 /** 1467 * The measure score for this stratum, calculated as appropriate for the measure 1468 * type and scoring method, and based on only the members of this stratum. 1469 */ 1470 @Child(name = "measureScore", type = { 1471 Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1472 @Description(shortDefinition = "What score this stratum achieved", formalDefinition = "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.") 1473 protected Quantity measureScore; 1474 1475 private static final long serialVersionUID = 892251179L; 1476 1477 /** 1478 * Constructor 1479 */ 1480 public StratifierGroupComponent() { 1481 super(); 1482 } 1483 1484 /** 1485 * @return {@link #value} (The value for this stratum, expressed as a 1486 * CodeableConcept. When defining stratifiers on complex values, the 1487 * value must be rendered such that the value for each stratum within 1488 * the stratifier is unique.) 1489 */ 1490 public CodeableConcept getValue() { 1491 if (this.value == null) 1492 if (Configuration.errorOnAutoCreate()) 1493 throw new Error("Attempt to auto-create StratifierGroupComponent.value"); 1494 else if (Configuration.doAutoCreate()) 1495 this.value = new CodeableConcept(); // cc 1496 return this.value; 1497 } 1498 1499 public boolean hasValue() { 1500 return this.value != null && !this.value.isEmpty(); 1501 } 1502 1503 /** 1504 * @param value {@link #value} (The value for this stratum, expressed as a 1505 * CodeableConcept. When defining stratifiers on complex values, 1506 * the value must be rendered such that the value for each stratum 1507 * within the stratifier is unique.) 1508 */ 1509 public StratifierGroupComponent setValue(CodeableConcept value) { 1510 this.value = value; 1511 return this; 1512 } 1513 1514 /** 1515 * @return {@link #component} (A stratifier component value.) 1516 */ 1517 public List<StratifierGroupComponentComponent> getComponent() { 1518 if (this.component == null) 1519 this.component = new ArrayList<StratifierGroupComponentComponent>(); 1520 return this.component; 1521 } 1522 1523 /** 1524 * @return Returns a reference to <code>this</code> for easy method chaining 1525 */ 1526 public StratifierGroupComponent setComponent(List<StratifierGroupComponentComponent> theComponent) { 1527 this.component = theComponent; 1528 return this; 1529 } 1530 1531 public boolean hasComponent() { 1532 if (this.component == null) 1533 return false; 1534 for (StratifierGroupComponentComponent item : this.component) 1535 if (!item.isEmpty()) 1536 return true; 1537 return false; 1538 } 1539 1540 public StratifierGroupComponentComponent addComponent() { // 3 1541 StratifierGroupComponentComponent t = new StratifierGroupComponentComponent(); 1542 if (this.component == null) 1543 this.component = new ArrayList<StratifierGroupComponentComponent>(); 1544 this.component.add(t); 1545 return t; 1546 } 1547 1548 public StratifierGroupComponent addComponent(StratifierGroupComponentComponent t) { // 3 1549 if (t == null) 1550 return this; 1551 if (this.component == null) 1552 this.component = new ArrayList<StratifierGroupComponentComponent>(); 1553 this.component.add(t); 1554 return this; 1555 } 1556 1557 /** 1558 * @return The first repetition of repeating field {@link #component}, creating 1559 * it if it does not already exist 1560 */ 1561 public StratifierGroupComponentComponent getComponentFirstRep() { 1562 if (getComponent().isEmpty()) { 1563 addComponent(); 1564 } 1565 return getComponent().get(0); 1566 } 1567 1568 /** 1569 * @return {@link #population} (The populations that make up the stratum, one 1570 * for each type of population appropriate to the measure.) 1571 */ 1572 public List<StratifierGroupPopulationComponent> getPopulation() { 1573 if (this.population == null) 1574 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 1575 return this.population; 1576 } 1577 1578 /** 1579 * @return Returns a reference to <code>this</code> for easy method chaining 1580 */ 1581 public StratifierGroupComponent setPopulation(List<StratifierGroupPopulationComponent> thePopulation) { 1582 this.population = thePopulation; 1583 return this; 1584 } 1585 1586 public boolean hasPopulation() { 1587 if (this.population == null) 1588 return false; 1589 for (StratifierGroupPopulationComponent item : this.population) 1590 if (!item.isEmpty()) 1591 return true; 1592 return false; 1593 } 1594 1595 public StratifierGroupPopulationComponent addPopulation() { // 3 1596 StratifierGroupPopulationComponent t = new StratifierGroupPopulationComponent(); 1597 if (this.population == null) 1598 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 1599 this.population.add(t); 1600 return t; 1601 } 1602 1603 public StratifierGroupComponent addPopulation(StratifierGroupPopulationComponent t) { // 3 1604 if (t == null) 1605 return this; 1606 if (this.population == null) 1607 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 1608 this.population.add(t); 1609 return this; 1610 } 1611 1612 /** 1613 * @return The first repetition of repeating field {@link #population}, creating 1614 * it if it does not already exist 1615 */ 1616 public StratifierGroupPopulationComponent getPopulationFirstRep() { 1617 if (getPopulation().isEmpty()) { 1618 addPopulation(); 1619 } 1620 return getPopulation().get(0); 1621 } 1622 1623 /** 1624 * @return {@link #measureScore} (The measure score for this stratum, calculated 1625 * as appropriate for the measure type and scoring method, and based on 1626 * only the members of this stratum.) 1627 */ 1628 public Quantity getMeasureScore() { 1629 if (this.measureScore == null) 1630 if (Configuration.errorOnAutoCreate()) 1631 throw new Error("Attempt to auto-create StratifierGroupComponent.measureScore"); 1632 else if (Configuration.doAutoCreate()) 1633 this.measureScore = new Quantity(); // cc 1634 return this.measureScore; 1635 } 1636 1637 public boolean hasMeasureScore() { 1638 return this.measureScore != null && !this.measureScore.isEmpty(); 1639 } 1640 1641 /** 1642 * @param value {@link #measureScore} (The measure score for this stratum, 1643 * calculated as appropriate for the measure type and scoring 1644 * method, and based on only the members of this stratum.) 1645 */ 1646 public StratifierGroupComponent setMeasureScore(Quantity value) { 1647 this.measureScore = value; 1648 return this; 1649 } 1650 1651 protected void listChildren(List<Property> children) { 1652 super.listChildren(children); 1653 children.add(new Property("value", "CodeableConcept", 1654 "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 1655 0, 1, value)); 1656 children.add( 1657 new Property("component", "", "A stratifier component value.", 0, java.lang.Integer.MAX_VALUE, component)); 1658 children.add(new Property("population", "", 1659 "The populations that make up the stratum, one for each type of population appropriate to the measure.", 0, 1660 java.lang.Integer.MAX_VALUE, population)); 1661 children.add(new Property("measureScore", "Quantity", 1662 "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 1663 0, 1, measureScore)); 1664 } 1665 1666 @Override 1667 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1668 switch (_hash) { 1669 case 111972721: 1670 /* value */ return new Property("value", "CodeableConcept", 1671 "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 1672 0, 1, value); 1673 case -1399907075: 1674 /* component */ return new Property("component", "", "A stratifier component value.", 0, 1675 java.lang.Integer.MAX_VALUE, component); 1676 case -2023558323: 1677 /* population */ return new Property("population", "", 1678 "The populations that make up the stratum, one for each type of population appropriate to the measure.", 0, 1679 java.lang.Integer.MAX_VALUE, population); 1680 case -386313260: 1681 /* measureScore */ return new Property("measureScore", "Quantity", 1682 "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 1683 0, 1, measureScore); 1684 default: 1685 return super.getNamedProperty(_hash, _name, _checkValid); 1686 } 1687 1688 } 1689 1690 @Override 1691 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1692 switch (hash) { 1693 case 111972721: 1694 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // CodeableConcept 1695 case -1399907075: 1696 /* component */ return this.component == null ? new Base[0] 1697 : this.component.toArray(new Base[this.component.size()]); // StratifierGroupComponentComponent 1698 case -2023558323: 1699 /* population */ return this.population == null ? new Base[0] 1700 : this.population.toArray(new Base[this.population.size()]); // StratifierGroupPopulationComponent 1701 case -386313260: 1702 /* measureScore */ return this.measureScore == null ? new Base[0] : new Base[] { this.measureScore }; // Quantity 1703 default: 1704 return super.getProperty(hash, name, checkValid); 1705 } 1706 1707 } 1708 1709 @Override 1710 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1711 switch (hash) { 1712 case 111972721: // value 1713 this.value = castToCodeableConcept(value); // CodeableConcept 1714 return value; 1715 case -1399907075: // component 1716 this.getComponent().add((StratifierGroupComponentComponent) value); // StratifierGroupComponentComponent 1717 return value; 1718 case -2023558323: // population 1719 this.getPopulation().add((StratifierGroupPopulationComponent) value); // StratifierGroupPopulationComponent 1720 return value; 1721 case -386313260: // measureScore 1722 this.measureScore = castToQuantity(value); // Quantity 1723 return value; 1724 default: 1725 return super.setProperty(hash, name, value); 1726 } 1727 1728 } 1729 1730 @Override 1731 public Base setProperty(String name, Base value) throws FHIRException { 1732 if (name.equals("value")) { 1733 this.value = castToCodeableConcept(value); // CodeableConcept 1734 } else if (name.equals("component")) { 1735 this.getComponent().add((StratifierGroupComponentComponent) value); 1736 } else if (name.equals("population")) { 1737 this.getPopulation().add((StratifierGroupPopulationComponent) value); 1738 } else if (name.equals("measureScore")) { 1739 this.measureScore = castToQuantity(value); // Quantity 1740 } else 1741 return super.setProperty(name, value); 1742 return value; 1743 } 1744 1745 @Override 1746 public void removeChild(String name, Base value) throws FHIRException { 1747 if (name.equals("value")) { 1748 this.value = null; 1749 } else if (name.equals("component")) { 1750 this.getComponent().remove((StratifierGroupComponentComponent) value); 1751 } else if (name.equals("population")) { 1752 this.getPopulation().remove((StratifierGroupPopulationComponent) value); 1753 } else if (name.equals("measureScore")) { 1754 this.measureScore = null; 1755 } else 1756 super.removeChild(name, value); 1757 1758 } 1759 1760 @Override 1761 public Base makeProperty(int hash, String name) throws FHIRException { 1762 switch (hash) { 1763 case 111972721: 1764 return getValue(); 1765 case -1399907075: 1766 return addComponent(); 1767 case -2023558323: 1768 return addPopulation(); 1769 case -386313260: 1770 return getMeasureScore(); 1771 default: 1772 return super.makeProperty(hash, name); 1773 } 1774 1775 } 1776 1777 @Override 1778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1779 switch (hash) { 1780 case 111972721: 1781 /* value */ return new String[] { "CodeableConcept" }; 1782 case -1399907075: 1783 /* component */ return new String[] {}; 1784 case -2023558323: 1785 /* population */ return new String[] {}; 1786 case -386313260: 1787 /* measureScore */ return new String[] { "Quantity" }; 1788 default: 1789 return super.getTypesForProperty(hash, name); 1790 } 1791 1792 } 1793 1794 @Override 1795 public Base addChild(String name) throws FHIRException { 1796 if (name.equals("value")) { 1797 this.value = new CodeableConcept(); 1798 return this.value; 1799 } else if (name.equals("component")) { 1800 return addComponent(); 1801 } else if (name.equals("population")) { 1802 return addPopulation(); 1803 } else if (name.equals("measureScore")) { 1804 this.measureScore = new Quantity(); 1805 return this.measureScore; 1806 } else 1807 return super.addChild(name); 1808 } 1809 1810 public StratifierGroupComponent copy() { 1811 StratifierGroupComponent dst = new StratifierGroupComponent(); 1812 copyValues(dst); 1813 return dst; 1814 } 1815 1816 public void copyValues(StratifierGroupComponent dst) { 1817 super.copyValues(dst); 1818 dst.value = value == null ? null : value.copy(); 1819 if (component != null) { 1820 dst.component = new ArrayList<StratifierGroupComponentComponent>(); 1821 for (StratifierGroupComponentComponent i : component) 1822 dst.component.add(i.copy()); 1823 } 1824 ; 1825 if (population != null) { 1826 dst.population = new ArrayList<StratifierGroupPopulationComponent>(); 1827 for (StratifierGroupPopulationComponent i : population) 1828 dst.population.add(i.copy()); 1829 } 1830 ; 1831 dst.measureScore = measureScore == null ? null : measureScore.copy(); 1832 } 1833 1834 @Override 1835 public boolean equalsDeep(Base other_) { 1836 if (!super.equalsDeep(other_)) 1837 return false; 1838 if (!(other_ instanceof StratifierGroupComponent)) 1839 return false; 1840 StratifierGroupComponent o = (StratifierGroupComponent) other_; 1841 return compareDeep(value, o.value, true) && compareDeep(component, o.component, true) 1842 && compareDeep(population, o.population, true) && compareDeep(measureScore, o.measureScore, true); 1843 } 1844 1845 @Override 1846 public boolean equalsShallow(Base other_) { 1847 if (!super.equalsShallow(other_)) 1848 return false; 1849 if (!(other_ instanceof StratifierGroupComponent)) 1850 return false; 1851 StratifierGroupComponent o = (StratifierGroupComponent) other_; 1852 return true; 1853 } 1854 1855 public boolean isEmpty() { 1856 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, component, population, measureScore); 1857 } 1858 1859 public String fhirType() { 1860 return "MeasureReport.group.stratifier.stratum"; 1861 1862 } 1863 1864 } 1865 1866 @Block() 1867 public static class StratifierGroupComponentComponent extends BackboneElement implements IBaseBackboneElement { 1868 /** 1869 * The code for the stratum component value. 1870 */ 1871 @Child(name = "code", type = { 1872 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1873 @Description(shortDefinition = "What stratifier component of the group", formalDefinition = "The code for the stratum component value.") 1874 protected CodeableConcept code; 1875 1876 /** 1877 * The stratum component value. 1878 */ 1879 @Child(name = "value", type = { 1880 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1881 @Description(shortDefinition = "The stratum component value, e.g. male", formalDefinition = "The stratum component value.") 1882 protected CodeableConcept value; 1883 1884 private static final long serialVersionUID = 1750253426L; 1885 1886 /** 1887 * Constructor 1888 */ 1889 public StratifierGroupComponentComponent() { 1890 super(); 1891 } 1892 1893 /** 1894 * Constructor 1895 */ 1896 public StratifierGroupComponentComponent(CodeableConcept code, CodeableConcept value) { 1897 super(); 1898 this.code = code; 1899 this.value = value; 1900 } 1901 1902 /** 1903 * @return {@link #code} (The code for the stratum component value.) 1904 */ 1905 public CodeableConcept getCode() { 1906 if (this.code == null) 1907 if (Configuration.errorOnAutoCreate()) 1908 throw new Error("Attempt to auto-create StratifierGroupComponentComponent.code"); 1909 else if (Configuration.doAutoCreate()) 1910 this.code = new CodeableConcept(); // cc 1911 return this.code; 1912 } 1913 1914 public boolean hasCode() { 1915 return this.code != null && !this.code.isEmpty(); 1916 } 1917 1918 /** 1919 * @param value {@link #code} (The code for the stratum component value.) 1920 */ 1921 public StratifierGroupComponentComponent setCode(CodeableConcept value) { 1922 this.code = value; 1923 return this; 1924 } 1925 1926 /** 1927 * @return {@link #value} (The stratum component value.) 1928 */ 1929 public CodeableConcept getValue() { 1930 if (this.value == null) 1931 if (Configuration.errorOnAutoCreate()) 1932 throw new Error("Attempt to auto-create StratifierGroupComponentComponent.value"); 1933 else if (Configuration.doAutoCreate()) 1934 this.value = new CodeableConcept(); // cc 1935 return this.value; 1936 } 1937 1938 public boolean hasValue() { 1939 return this.value != null && !this.value.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #value} (The stratum component value.) 1944 */ 1945 public StratifierGroupComponentComponent setValue(CodeableConcept value) { 1946 this.value = value; 1947 return this; 1948 } 1949 1950 protected void listChildren(List<Property> children) { 1951 super.listChildren(children); 1952 children.add(new Property("code", "CodeableConcept", "The code for the stratum component value.", 0, 1, code)); 1953 children.add(new Property("value", "CodeableConcept", "The stratum component value.", 0, 1, value)); 1954 } 1955 1956 @Override 1957 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1958 switch (_hash) { 1959 case 3059181: 1960 /* code */ return new Property("code", "CodeableConcept", "The code for the stratum component value.", 0, 1, 1961 code); 1962 case 111972721: 1963 /* value */ return new Property("value", "CodeableConcept", "The stratum component value.", 0, 1, value); 1964 default: 1965 return super.getNamedProperty(_hash, _name, _checkValid); 1966 } 1967 1968 } 1969 1970 @Override 1971 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1972 switch (hash) { 1973 case 3059181: 1974 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1975 case 111972721: 1976 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // CodeableConcept 1977 default: 1978 return super.getProperty(hash, name, checkValid); 1979 } 1980 1981 } 1982 1983 @Override 1984 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1985 switch (hash) { 1986 case 3059181: // code 1987 this.code = castToCodeableConcept(value); // CodeableConcept 1988 return value; 1989 case 111972721: // value 1990 this.value = castToCodeableConcept(value); // CodeableConcept 1991 return value; 1992 default: 1993 return super.setProperty(hash, name, value); 1994 } 1995 1996 } 1997 1998 @Override 1999 public Base setProperty(String name, Base value) throws FHIRException { 2000 if (name.equals("code")) { 2001 this.code = castToCodeableConcept(value); // CodeableConcept 2002 } else if (name.equals("value")) { 2003 this.value = castToCodeableConcept(value); // CodeableConcept 2004 } else 2005 return super.setProperty(name, value); 2006 return value; 2007 } 2008 2009 @Override 2010 public void removeChild(String name, Base value) throws FHIRException { 2011 if (name.equals("code")) { 2012 this.code = null; 2013 } else if (name.equals("value")) { 2014 this.value = null; 2015 } else 2016 super.removeChild(name, value); 2017 2018 } 2019 2020 @Override 2021 public Base makeProperty(int hash, String name) throws FHIRException { 2022 switch (hash) { 2023 case 3059181: 2024 return getCode(); 2025 case 111972721: 2026 return getValue(); 2027 default: 2028 return super.makeProperty(hash, name); 2029 } 2030 2031 } 2032 2033 @Override 2034 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2035 switch (hash) { 2036 case 3059181: 2037 /* code */ return new String[] { "CodeableConcept" }; 2038 case 111972721: 2039 /* value */ return new String[] { "CodeableConcept" }; 2040 default: 2041 return super.getTypesForProperty(hash, name); 2042 } 2043 2044 } 2045 2046 @Override 2047 public Base addChild(String name) throws FHIRException { 2048 if (name.equals("code")) { 2049 this.code = new CodeableConcept(); 2050 return this.code; 2051 } else if (name.equals("value")) { 2052 this.value = new CodeableConcept(); 2053 return this.value; 2054 } else 2055 return super.addChild(name); 2056 } 2057 2058 public StratifierGroupComponentComponent copy() { 2059 StratifierGroupComponentComponent dst = new StratifierGroupComponentComponent(); 2060 copyValues(dst); 2061 return dst; 2062 } 2063 2064 public void copyValues(StratifierGroupComponentComponent dst) { 2065 super.copyValues(dst); 2066 dst.code = code == null ? null : code.copy(); 2067 dst.value = value == null ? null : value.copy(); 2068 } 2069 2070 @Override 2071 public boolean equalsDeep(Base other_) { 2072 if (!super.equalsDeep(other_)) 2073 return false; 2074 if (!(other_ instanceof StratifierGroupComponentComponent)) 2075 return false; 2076 StratifierGroupComponentComponent o = (StratifierGroupComponentComponent) other_; 2077 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 2078 } 2079 2080 @Override 2081 public boolean equalsShallow(Base other_) { 2082 if (!super.equalsShallow(other_)) 2083 return false; 2084 if (!(other_ instanceof StratifierGroupComponentComponent)) 2085 return false; 2086 StratifierGroupComponentComponent o = (StratifierGroupComponentComponent) other_; 2087 return true; 2088 } 2089 2090 public boolean isEmpty() { 2091 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 2092 } 2093 2094 public String fhirType() { 2095 return "MeasureReport.group.stratifier.stratum.component"; 2096 2097 } 2098 2099 } 2100 2101 @Block() 2102 public static class StratifierGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 2103 /** 2104 * The type of the population. 2105 */ 2106 @Child(name = "code", type = { 2107 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2108 @Description(shortDefinition = "initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition = "The type of the population.") 2109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-population") 2110 protected CodeableConcept code; 2111 2112 /** 2113 * The number of members of the population in this stratum. 2114 */ 2115 @Child(name = "count", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2116 @Description(shortDefinition = "Size of the population", formalDefinition = "The number of members of the population in this stratum.") 2117 protected IntegerType count; 2118 2119 /** 2120 * This element refers to a List of subject level MeasureReport resources, one 2121 * for each subject in this population in this stratum. 2122 */ 2123 @Child(name = "subjectResults", type = { 2124 ListResource.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2125 @Description(shortDefinition = "For subject-list reports, the subject results in this population", formalDefinition = "This element refers to a List of subject level MeasureReport resources, one for each subject in this population in this stratum.") 2126 protected Reference subjectResults; 2127 2128 /** 2129 * The actual object that is the target of the reference (This element refers to 2130 * a List of subject level MeasureReport resources, one for each subject in this 2131 * population in this stratum.) 2132 */ 2133 protected ListResource subjectResultsTarget; 2134 2135 private static final long serialVersionUID = 210461445L; 2136 2137 /** 2138 * Constructor 2139 */ 2140 public StratifierGroupPopulationComponent() { 2141 super(); 2142 } 2143 2144 /** 2145 * @return {@link #code} (The type of the population.) 2146 */ 2147 public CodeableConcept getCode() { 2148 if (this.code == null) 2149 if (Configuration.errorOnAutoCreate()) 2150 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.code"); 2151 else if (Configuration.doAutoCreate()) 2152 this.code = new CodeableConcept(); // cc 2153 return this.code; 2154 } 2155 2156 public boolean hasCode() { 2157 return this.code != null && !this.code.isEmpty(); 2158 } 2159 2160 /** 2161 * @param value {@link #code} (The type of the population.) 2162 */ 2163 public StratifierGroupPopulationComponent setCode(CodeableConcept value) { 2164 this.code = value; 2165 return this; 2166 } 2167 2168 /** 2169 * @return {@link #count} (The number of members of the population in this 2170 * stratum.). This is the underlying object with id, value and 2171 * extensions. The accessor "getCount" gives direct access to the value 2172 */ 2173 public IntegerType getCountElement() { 2174 if (this.count == null) 2175 if (Configuration.errorOnAutoCreate()) 2176 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.count"); 2177 else if (Configuration.doAutoCreate()) 2178 this.count = new IntegerType(); // bb 2179 return this.count; 2180 } 2181 2182 public boolean hasCountElement() { 2183 return this.count != null && !this.count.isEmpty(); 2184 } 2185 2186 public boolean hasCount() { 2187 return this.count != null && !this.count.isEmpty(); 2188 } 2189 2190 /** 2191 * @param value {@link #count} (The number of members of the population in this 2192 * stratum.). This is the underlying object with id, value and 2193 * extensions. The accessor "getCount" gives direct access to the 2194 * value 2195 */ 2196 public StratifierGroupPopulationComponent setCountElement(IntegerType value) { 2197 this.count = value; 2198 return this; 2199 } 2200 2201 /** 2202 * @return The number of members of the population in this stratum. 2203 */ 2204 public int getCount() { 2205 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 2206 } 2207 2208 /** 2209 * @param value The number of members of the population in this stratum. 2210 */ 2211 public StratifierGroupPopulationComponent setCount(int value) { 2212 if (this.count == null) 2213 this.count = new IntegerType(); 2214 this.count.setValue(value); 2215 return this; 2216 } 2217 2218 /** 2219 * @return {@link #subjectResults} (This element refers to a List of subject 2220 * level MeasureReport resources, one for each subject in this 2221 * population in this stratum.) 2222 */ 2223 public Reference getSubjectResults() { 2224 if (this.subjectResults == null) 2225 if (Configuration.errorOnAutoCreate()) 2226 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.subjectResults"); 2227 else if (Configuration.doAutoCreate()) 2228 this.subjectResults = new Reference(); // cc 2229 return this.subjectResults; 2230 } 2231 2232 public boolean hasSubjectResults() { 2233 return this.subjectResults != null && !this.subjectResults.isEmpty(); 2234 } 2235 2236 /** 2237 * @param value {@link #subjectResults} (This element refers to a List of 2238 * subject level MeasureReport resources, one for each subject in 2239 * this population in this stratum.) 2240 */ 2241 public StratifierGroupPopulationComponent setSubjectResults(Reference value) { 2242 this.subjectResults = value; 2243 return this; 2244 } 2245 2246 /** 2247 * @return {@link #subjectResults} The actual object that is the target of the 2248 * reference. The reference library doesn't populate this, but you can 2249 * use it to hold the resource if you resolve it. (This element refers 2250 * to a List of subject level MeasureReport resources, one for each 2251 * subject in this population in this stratum.) 2252 */ 2253 public ListResource getSubjectResultsTarget() { 2254 if (this.subjectResultsTarget == null) 2255 if (Configuration.errorOnAutoCreate()) 2256 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.subjectResults"); 2257 else if (Configuration.doAutoCreate()) 2258 this.subjectResultsTarget = new ListResource(); // aa 2259 return this.subjectResultsTarget; 2260 } 2261 2262 /** 2263 * @param value {@link #subjectResults} The actual object that is the target of 2264 * the reference. The reference library doesn't use these, but you 2265 * can use it to hold the resource if you resolve it. (This element 2266 * refers to a List of subject level MeasureReport resources, one 2267 * for each subject in this population in this stratum.) 2268 */ 2269 public StratifierGroupPopulationComponent setSubjectResultsTarget(ListResource value) { 2270 this.subjectResultsTarget = value; 2271 return this; 2272 } 2273 2274 protected void listChildren(List<Property> children) { 2275 super.listChildren(children); 2276 children.add(new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code)); 2277 children.add( 2278 new Property("count", "integer", "The number of members of the population in this stratum.", 0, 1, count)); 2279 children.add(new Property("subjectResults", "Reference(List)", 2280 "This element refers to a List of subject level MeasureReport resources, one for each subject in this population in this stratum.", 2281 0, 1, subjectResults)); 2282 } 2283 2284 @Override 2285 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2286 switch (_hash) { 2287 case 3059181: 2288 /* code */ return new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code); 2289 case 94851343: 2290 /* count */ return new Property("count", "integer", "The number of members of the population in this stratum.", 2291 0, 1, count); 2292 case 2136184106: 2293 /* subjectResults */ return new Property("subjectResults", "Reference(List)", 2294 "This element refers to a List of subject level MeasureReport resources, one for each subject in this population in this stratum.", 2295 0, 1, subjectResults); 2296 default: 2297 return super.getNamedProperty(_hash, _name, _checkValid); 2298 } 2299 2300 } 2301 2302 @Override 2303 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2304 switch (hash) { 2305 case 3059181: 2306 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2307 case 94851343: 2308 /* count */ return this.count == null ? new Base[0] : new Base[] { this.count }; // IntegerType 2309 case 2136184106: 2310 /* subjectResults */ return this.subjectResults == null ? new Base[0] : new Base[] { this.subjectResults }; // Reference 2311 default: 2312 return super.getProperty(hash, name, checkValid); 2313 } 2314 2315 } 2316 2317 @Override 2318 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2319 switch (hash) { 2320 case 3059181: // code 2321 this.code = castToCodeableConcept(value); // CodeableConcept 2322 return value; 2323 case 94851343: // count 2324 this.count = castToInteger(value); // IntegerType 2325 return value; 2326 case 2136184106: // subjectResults 2327 this.subjectResults = castToReference(value); // Reference 2328 return value; 2329 default: 2330 return super.setProperty(hash, name, value); 2331 } 2332 2333 } 2334 2335 @Override 2336 public Base setProperty(String name, Base value) throws FHIRException { 2337 if (name.equals("code")) { 2338 this.code = castToCodeableConcept(value); // CodeableConcept 2339 } else if (name.equals("count")) { 2340 this.count = castToInteger(value); // IntegerType 2341 } else if (name.equals("subjectResults")) { 2342 this.subjectResults = castToReference(value); // Reference 2343 } else 2344 return super.setProperty(name, value); 2345 return value; 2346 } 2347 2348 @Override 2349 public void removeChild(String name, Base value) throws FHIRException { 2350 if (name.equals("code")) { 2351 this.code = null; 2352 } else if (name.equals("count")) { 2353 this.count = null; 2354 } else if (name.equals("subjectResults")) { 2355 this.subjectResults = null; 2356 } else 2357 super.removeChild(name, value); 2358 2359 } 2360 2361 @Override 2362 public Base makeProperty(int hash, String name) throws FHIRException { 2363 switch (hash) { 2364 case 3059181: 2365 return getCode(); 2366 case 94851343: 2367 return getCountElement(); 2368 case 2136184106: 2369 return getSubjectResults(); 2370 default: 2371 return super.makeProperty(hash, name); 2372 } 2373 2374 } 2375 2376 @Override 2377 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2378 switch (hash) { 2379 case 3059181: 2380 /* code */ return new String[] { "CodeableConcept" }; 2381 case 94851343: 2382 /* count */ return new String[] { "integer" }; 2383 case 2136184106: 2384 /* subjectResults */ return new String[] { "Reference" }; 2385 default: 2386 return super.getTypesForProperty(hash, name); 2387 } 2388 2389 } 2390 2391 @Override 2392 public Base addChild(String name) throws FHIRException { 2393 if (name.equals("code")) { 2394 this.code = new CodeableConcept(); 2395 return this.code; 2396 } else if (name.equals("count")) { 2397 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.count"); 2398 } else if (name.equals("subjectResults")) { 2399 this.subjectResults = new Reference(); 2400 return this.subjectResults; 2401 } else 2402 return super.addChild(name); 2403 } 2404 2405 public StratifierGroupPopulationComponent copy() { 2406 StratifierGroupPopulationComponent dst = new StratifierGroupPopulationComponent(); 2407 copyValues(dst); 2408 return dst; 2409 } 2410 2411 public void copyValues(StratifierGroupPopulationComponent dst) { 2412 super.copyValues(dst); 2413 dst.code = code == null ? null : code.copy(); 2414 dst.count = count == null ? null : count.copy(); 2415 dst.subjectResults = subjectResults == null ? null : subjectResults.copy(); 2416 } 2417 2418 @Override 2419 public boolean equalsDeep(Base other_) { 2420 if (!super.equalsDeep(other_)) 2421 return false; 2422 if (!(other_ instanceof StratifierGroupPopulationComponent)) 2423 return false; 2424 StratifierGroupPopulationComponent o = (StratifierGroupPopulationComponent) other_; 2425 return compareDeep(code, o.code, true) && compareDeep(count, o.count, true) 2426 && compareDeep(subjectResults, o.subjectResults, true); 2427 } 2428 2429 @Override 2430 public boolean equalsShallow(Base other_) { 2431 if (!super.equalsShallow(other_)) 2432 return false; 2433 if (!(other_ instanceof StratifierGroupPopulationComponent)) 2434 return false; 2435 StratifierGroupPopulationComponent o = (StratifierGroupPopulationComponent) other_; 2436 return compareValues(count, o.count, true); 2437 } 2438 2439 public boolean isEmpty() { 2440 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, count, subjectResults); 2441 } 2442 2443 public String fhirType() { 2444 return "MeasureReport.group.stratifier.stratum.population"; 2445 2446 } 2447 2448 } 2449 2450 /** 2451 * A formal identifier that is used to identify this MeasureReport when it is 2452 * represented in other formats or referenced in a specification, model, design 2453 * or an instance. 2454 */ 2455 @Child(name = "identifier", type = { 2456 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2457 @Description(shortDefinition = "Additional identifier for the MeasureReport", formalDefinition = "A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance.") 2458 protected List<Identifier> identifier; 2459 2460 /** 2461 * The MeasureReport status. No data will be available until the MeasureReport 2462 * status is complete. 2463 */ 2464 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2465 @Description(shortDefinition = "complete | pending | error", formalDefinition = "The MeasureReport status. No data will be available until the MeasureReport status is complete.") 2466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-report-status") 2467 protected Enumeration<MeasureReportStatus> status; 2468 2469 /** 2470 * The type of measure report. This may be an individual report, which provides 2471 * the score for the measure for an individual member of the population; a 2472 * subject-listing, which returns the list of members that meet the various 2473 * criteria in the measure; a summary report, which returns a population count 2474 * for each of the criteria in the measure; or a data-collection, which enables 2475 * the MeasureReport to be used to exchange the data-of-interest for a quality 2476 * measure. 2477 */ 2478 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2479 @Description(shortDefinition = "individual | subject-list | summary | data-collection", formalDefinition = "The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.") 2480 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-report-type") 2481 protected Enumeration<MeasureReportType> type; 2482 2483 /** 2484 * A reference to the Measure that was calculated to produce this report. 2485 */ 2486 @Child(name = "measure", type = { 2487 CanonicalType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2488 @Description(shortDefinition = "What measure was calculated", formalDefinition = "A reference to the Measure that was calculated to produce this report.") 2489 protected CanonicalType measure; 2490 2491 /** 2492 * Optional subject identifying the individual or individuals the report is for. 2493 */ 2494 @Child(name = "subject", type = { Patient.class, Practitioner.class, PractitionerRole.class, Location.class, 2495 Device.class, RelatedPerson.class, Group.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2496 @Description(shortDefinition = "What individual(s) the report is for", formalDefinition = "Optional subject identifying the individual or individuals the report is for.") 2497 protected Reference subject; 2498 2499 /** 2500 * The actual object that is the target of the reference (Optional subject 2501 * identifying the individual or individuals the report is for.) 2502 */ 2503 protected Resource subjectTarget; 2504 2505 /** 2506 * The date this measure report was generated. 2507 */ 2508 @Child(name = "date", type = { DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2509 @Description(shortDefinition = "When the report was generated", formalDefinition = "The date this measure report was generated.") 2510 protected DateTimeType date; 2511 2512 /** 2513 * The individual, location, or organization that is reporting the data. 2514 */ 2515 @Child(name = "reporter", type = { Practitioner.class, PractitionerRole.class, Location.class, 2516 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2517 @Description(shortDefinition = "Who is reporting the data", formalDefinition = "The individual, location, or organization that is reporting the data.") 2518 protected Reference reporter; 2519 2520 /** 2521 * The actual object that is the target of the reference (The individual, 2522 * location, or organization that is reporting the data.) 2523 */ 2524 protected Resource reporterTarget; 2525 2526 /** 2527 * The reporting period for which the report was calculated. 2528 */ 2529 @Child(name = "period", type = { Period.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 2530 @Description(shortDefinition = "What period the report covers", formalDefinition = "The reporting period for which the report was calculated.") 2531 protected Period period; 2532 2533 /** 2534 * Whether improvement in the measure is noted by an increase or decrease in the 2535 * measure score. 2536 */ 2537 @Child(name = "improvementNotation", type = { 2538 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 2539 @Description(shortDefinition = "increase | decrease", formalDefinition = "Whether improvement in the measure is noted by an increase or decrease in the measure score.") 2540 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/measure-improvement-notation") 2541 protected CodeableConcept improvementNotation; 2542 2543 /** 2544 * The results of the calculation, one for each population group in the measure. 2545 */ 2546 @Child(name = "group", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2547 @Description(shortDefinition = "Measure results for each group", formalDefinition = "The results of the calculation, one for each population group in the measure.") 2548 protected List<MeasureReportGroupComponent> group; 2549 2550 /** 2551 * A reference to a Bundle containing the Resources that were used in the 2552 * calculation of this measure. 2553 */ 2554 @Child(name = "evaluatedResource", type = { 2555 Reference.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2556 @Description(shortDefinition = "What data was used to calculate the measure score", formalDefinition = "A reference to a Bundle containing the Resources that were used in the calculation of this measure.") 2557 protected List<Reference> evaluatedResource; 2558 /** 2559 * The actual objects that are the target of the reference (A reference to a 2560 * Bundle containing the Resources that were used in the calculation of this 2561 * measure.) 2562 */ 2563 protected List<Resource> evaluatedResourceTarget; 2564 2565 private static final long serialVersionUID = 355307999L; 2566 2567 /** 2568 * Constructor 2569 */ 2570 public MeasureReport() { 2571 super(); 2572 } 2573 2574 /** 2575 * Constructor 2576 */ 2577 public MeasureReport(Enumeration<MeasureReportStatus> status, Enumeration<MeasureReportType> type, 2578 CanonicalType measure, Period period) { 2579 super(); 2580 this.status = status; 2581 this.type = type; 2582 this.measure = measure; 2583 this.period = period; 2584 } 2585 2586 /** 2587 * @return {@link #identifier} (A formal identifier that is used to identify 2588 * this MeasureReport when it is represented in other formats or 2589 * referenced in a specification, model, design or an instance.) 2590 */ 2591 public List<Identifier> getIdentifier() { 2592 if (this.identifier == null) 2593 this.identifier = new ArrayList<Identifier>(); 2594 return this.identifier; 2595 } 2596 2597 /** 2598 * @return Returns a reference to <code>this</code> for easy method chaining 2599 */ 2600 public MeasureReport setIdentifier(List<Identifier> theIdentifier) { 2601 this.identifier = theIdentifier; 2602 return this; 2603 } 2604 2605 public boolean hasIdentifier() { 2606 if (this.identifier == null) 2607 return false; 2608 for (Identifier item : this.identifier) 2609 if (!item.isEmpty()) 2610 return true; 2611 return false; 2612 } 2613 2614 public Identifier addIdentifier() { // 3 2615 Identifier t = new Identifier(); 2616 if (this.identifier == null) 2617 this.identifier = new ArrayList<Identifier>(); 2618 this.identifier.add(t); 2619 return t; 2620 } 2621 2622 public MeasureReport addIdentifier(Identifier t) { // 3 2623 if (t == null) 2624 return this; 2625 if (this.identifier == null) 2626 this.identifier = new ArrayList<Identifier>(); 2627 this.identifier.add(t); 2628 return this; 2629 } 2630 2631 /** 2632 * @return The first repetition of repeating field {@link #identifier}, creating 2633 * it if it does not already exist 2634 */ 2635 public Identifier getIdentifierFirstRep() { 2636 if (getIdentifier().isEmpty()) { 2637 addIdentifier(); 2638 } 2639 return getIdentifier().get(0); 2640 } 2641 2642 /** 2643 * @return {@link #status} (The MeasureReport status. No data will be available 2644 * until the MeasureReport status is complete.). This is the underlying 2645 * object with id, value and extensions. The accessor "getStatus" gives 2646 * direct access to the value 2647 */ 2648 public Enumeration<MeasureReportStatus> getStatusElement() { 2649 if (this.status == null) 2650 if (Configuration.errorOnAutoCreate()) 2651 throw new Error("Attempt to auto-create MeasureReport.status"); 2652 else if (Configuration.doAutoCreate()) 2653 this.status = new Enumeration<MeasureReportStatus>(new MeasureReportStatusEnumFactory()); // bb 2654 return this.status; 2655 } 2656 2657 public boolean hasStatusElement() { 2658 return this.status != null && !this.status.isEmpty(); 2659 } 2660 2661 public boolean hasStatus() { 2662 return this.status != null && !this.status.isEmpty(); 2663 } 2664 2665 /** 2666 * @param value {@link #status} (The MeasureReport status. No data will be 2667 * available until the MeasureReport status is complete.). This is 2668 * the underlying object with id, value and extensions. The 2669 * accessor "getStatus" gives direct access to the value 2670 */ 2671 public MeasureReport setStatusElement(Enumeration<MeasureReportStatus> value) { 2672 this.status = value; 2673 return this; 2674 } 2675 2676 /** 2677 * @return The MeasureReport status. No data will be available until the 2678 * MeasureReport status is complete. 2679 */ 2680 public MeasureReportStatus getStatus() { 2681 return this.status == null ? null : this.status.getValue(); 2682 } 2683 2684 /** 2685 * @param value The MeasureReport status. No data will be available until the 2686 * MeasureReport status is complete. 2687 */ 2688 public MeasureReport setStatus(MeasureReportStatus value) { 2689 if (this.status == null) 2690 this.status = new Enumeration<MeasureReportStatus>(new MeasureReportStatusEnumFactory()); 2691 this.status.setValue(value); 2692 return this; 2693 } 2694 2695 /** 2696 * @return {@link #type} (The type of measure report. This may be an individual 2697 * report, which provides the score for the measure for an individual 2698 * member of the population; a subject-listing, which returns the list 2699 * of members that meet the various criteria in the measure; a summary 2700 * report, which returns a population count for each of the criteria in 2701 * the measure; or a data-collection, which enables the MeasureReport to 2702 * be used to exchange the data-of-interest for a quality measure.). 2703 * This is the underlying object with id, value and extensions. The 2704 * accessor "getType" gives direct access to the value 2705 */ 2706 public Enumeration<MeasureReportType> getTypeElement() { 2707 if (this.type == null) 2708 if (Configuration.errorOnAutoCreate()) 2709 throw new Error("Attempt to auto-create MeasureReport.type"); 2710 else if (Configuration.doAutoCreate()) 2711 this.type = new Enumeration<MeasureReportType>(new MeasureReportTypeEnumFactory()); // bb 2712 return this.type; 2713 } 2714 2715 public boolean hasTypeElement() { 2716 return this.type != null && !this.type.isEmpty(); 2717 } 2718 2719 public boolean hasType() { 2720 return this.type != null && !this.type.isEmpty(); 2721 } 2722 2723 /** 2724 * @param value {@link #type} (The type of measure report. This may be an 2725 * individual report, which provides the score for the measure for 2726 * an individual member of the population; a subject-listing, which 2727 * returns the list of members that meet the various criteria in 2728 * the measure; a summary report, which returns a population count 2729 * for each of the criteria in the measure; or a data-collection, 2730 * which enables the MeasureReport to be used to exchange the 2731 * data-of-interest for a quality measure.). This is the underlying 2732 * object with id, value and extensions. The accessor "getType" 2733 * gives direct access to the value 2734 */ 2735 public MeasureReport setTypeElement(Enumeration<MeasureReportType> value) { 2736 this.type = value; 2737 return this; 2738 } 2739 2740 /** 2741 * @return The type of measure report. This may be an individual report, which 2742 * provides the score for the measure for an individual member of the 2743 * population; a subject-listing, which returns the list of members that 2744 * meet the various criteria in the measure; a summary report, which 2745 * returns a population count for each of the criteria in the measure; 2746 * or a data-collection, which enables the MeasureReport to be used to 2747 * exchange the data-of-interest for a quality measure. 2748 */ 2749 public MeasureReportType getType() { 2750 return this.type == null ? null : this.type.getValue(); 2751 } 2752 2753 /** 2754 * @param value The type of measure report. This may be an individual report, 2755 * which provides the score for the measure for an individual 2756 * member of the population; a subject-listing, which returns the 2757 * list of members that meet the various criteria in the measure; a 2758 * summary report, which returns a population count for each of the 2759 * criteria in the measure; or a data-collection, which enables the 2760 * MeasureReport to be used to exchange the data-of-interest for a 2761 * quality measure. 2762 */ 2763 public MeasureReport setType(MeasureReportType value) { 2764 if (this.type == null) 2765 this.type = new Enumeration<MeasureReportType>(new MeasureReportTypeEnumFactory()); 2766 this.type.setValue(value); 2767 return this; 2768 } 2769 2770 /** 2771 * @return {@link #measure} (A reference to the Measure that was calculated to 2772 * produce this report.). This is the underlying object with id, value 2773 * and extensions. The accessor "getMeasure" gives direct access to the 2774 * value 2775 */ 2776 public CanonicalType getMeasureElement() { 2777 if (this.measure == null) 2778 if (Configuration.errorOnAutoCreate()) 2779 throw new Error("Attempt to auto-create MeasureReport.measure"); 2780 else if (Configuration.doAutoCreate()) 2781 this.measure = new CanonicalType(); // bb 2782 return this.measure; 2783 } 2784 2785 public boolean hasMeasureElement() { 2786 return this.measure != null && !this.measure.isEmpty(); 2787 } 2788 2789 public boolean hasMeasure() { 2790 return this.measure != null && !this.measure.isEmpty(); 2791 } 2792 2793 /** 2794 * @param value {@link #measure} (A reference to the Measure that was calculated 2795 * to produce this report.). This is the underlying object with id, 2796 * value and extensions. The accessor "getMeasure" gives direct 2797 * access to the value 2798 */ 2799 public MeasureReport setMeasureElement(CanonicalType value) { 2800 this.measure = value; 2801 return this; 2802 } 2803 2804 /** 2805 * @return A reference to the Measure that was calculated to produce this 2806 * report. 2807 */ 2808 public String getMeasure() { 2809 return this.measure == null ? null : this.measure.getValue(); 2810 } 2811 2812 /** 2813 * @param value A reference to the Measure that was calculated to produce this 2814 * report. 2815 */ 2816 public MeasureReport setMeasure(String value) { 2817 if (this.measure == null) 2818 this.measure = new CanonicalType(); 2819 this.measure.setValue(value); 2820 return this; 2821 } 2822 2823 /** 2824 * @return {@link #subject} (Optional subject identifying the individual or 2825 * individuals the report is for.) 2826 */ 2827 public Reference getSubject() { 2828 if (this.subject == null) 2829 if (Configuration.errorOnAutoCreate()) 2830 throw new Error("Attempt to auto-create MeasureReport.subject"); 2831 else if (Configuration.doAutoCreate()) 2832 this.subject = new Reference(); // cc 2833 return this.subject; 2834 } 2835 2836 public boolean hasSubject() { 2837 return this.subject != null && !this.subject.isEmpty(); 2838 } 2839 2840 /** 2841 * @param value {@link #subject} (Optional subject identifying the individual or 2842 * individuals the report is for.) 2843 */ 2844 public MeasureReport setSubject(Reference value) { 2845 this.subject = value; 2846 return this; 2847 } 2848 2849 /** 2850 * @return {@link #subject} The actual object that is the target of the 2851 * reference. The reference library doesn't populate this, but you can 2852 * use it to hold the resource if you resolve it. (Optional subject 2853 * identifying the individual or individuals the report is for.) 2854 */ 2855 public Resource getSubjectTarget() { 2856 return this.subjectTarget; 2857 } 2858 2859 /** 2860 * @param value {@link #subject} The actual object that is the target of the 2861 * reference. The reference library doesn't use these, but you can 2862 * use it to hold the resource if you resolve it. (Optional subject 2863 * identifying the individual or individuals the report is for.) 2864 */ 2865 public MeasureReport setSubjectTarget(Resource value) { 2866 this.subjectTarget = value; 2867 return this; 2868 } 2869 2870 /** 2871 * @return {@link #date} (The date this measure report was generated.). This is 2872 * the underlying object with id, value and extensions. The accessor 2873 * "getDate" gives direct access to the value 2874 */ 2875 public DateTimeType getDateElement() { 2876 if (this.date == null) 2877 if (Configuration.errorOnAutoCreate()) 2878 throw new Error("Attempt to auto-create MeasureReport.date"); 2879 else if (Configuration.doAutoCreate()) 2880 this.date = new DateTimeType(); // bb 2881 return this.date; 2882 } 2883 2884 public boolean hasDateElement() { 2885 return this.date != null && !this.date.isEmpty(); 2886 } 2887 2888 public boolean hasDate() { 2889 return this.date != null && !this.date.isEmpty(); 2890 } 2891 2892 /** 2893 * @param value {@link #date} (The date this measure report was generated.). 2894 * This is the underlying object with id, value and extensions. The 2895 * accessor "getDate" gives direct access to the value 2896 */ 2897 public MeasureReport setDateElement(DateTimeType value) { 2898 this.date = value; 2899 return this; 2900 } 2901 2902 /** 2903 * @return The date this measure report was generated. 2904 */ 2905 public Date getDate() { 2906 return this.date == null ? null : this.date.getValue(); 2907 } 2908 2909 /** 2910 * @param value The date this measure report was generated. 2911 */ 2912 public MeasureReport setDate(Date value) { 2913 if (value == null) 2914 this.date = null; 2915 else { 2916 if (this.date == null) 2917 this.date = new DateTimeType(); 2918 this.date.setValue(value); 2919 } 2920 return this; 2921 } 2922 2923 /** 2924 * @return {@link #reporter} (The individual, location, or organization that is 2925 * reporting the data.) 2926 */ 2927 public Reference getReporter() { 2928 if (this.reporter == null) 2929 if (Configuration.errorOnAutoCreate()) 2930 throw new Error("Attempt to auto-create MeasureReport.reporter"); 2931 else if (Configuration.doAutoCreate()) 2932 this.reporter = new Reference(); // cc 2933 return this.reporter; 2934 } 2935 2936 public boolean hasReporter() { 2937 return this.reporter != null && !this.reporter.isEmpty(); 2938 } 2939 2940 /** 2941 * @param value {@link #reporter} (The individual, location, or organization 2942 * that is reporting the data.) 2943 */ 2944 public MeasureReport setReporter(Reference value) { 2945 this.reporter = value; 2946 return this; 2947 } 2948 2949 /** 2950 * @return {@link #reporter} The actual object that is the target of the 2951 * reference. The reference library doesn't populate this, but you can 2952 * use it to hold the resource if you resolve it. (The individual, 2953 * location, or organization that is reporting the data.) 2954 */ 2955 public Resource getReporterTarget() { 2956 return this.reporterTarget; 2957 } 2958 2959 /** 2960 * @param value {@link #reporter} The actual object that is the target of the 2961 * reference. The reference library doesn't use these, but you can 2962 * use it to hold the resource if you resolve it. (The individual, 2963 * location, or organization that is reporting the data.) 2964 */ 2965 public MeasureReport setReporterTarget(Resource value) { 2966 this.reporterTarget = value; 2967 return this; 2968 } 2969 2970 /** 2971 * @return {@link #period} (The reporting period for which the report was 2972 * calculated.) 2973 */ 2974 public Period getPeriod() { 2975 if (this.period == null) 2976 if (Configuration.errorOnAutoCreate()) 2977 throw new Error("Attempt to auto-create MeasureReport.period"); 2978 else if (Configuration.doAutoCreate()) 2979 this.period = new Period(); // cc 2980 return this.period; 2981 } 2982 2983 public boolean hasPeriod() { 2984 return this.period != null && !this.period.isEmpty(); 2985 } 2986 2987 /** 2988 * @param value {@link #period} (The reporting period for which the report was 2989 * calculated.) 2990 */ 2991 public MeasureReport setPeriod(Period value) { 2992 this.period = value; 2993 return this; 2994 } 2995 2996 /** 2997 * @return {@link #improvementNotation} (Whether improvement in the measure is 2998 * noted by an increase or decrease in the measure score.) 2999 */ 3000 public CodeableConcept getImprovementNotation() { 3001 if (this.improvementNotation == null) 3002 if (Configuration.errorOnAutoCreate()) 3003 throw new Error("Attempt to auto-create MeasureReport.improvementNotation"); 3004 else if (Configuration.doAutoCreate()) 3005 this.improvementNotation = new CodeableConcept(); // cc 3006 return this.improvementNotation; 3007 } 3008 3009 public boolean hasImprovementNotation() { 3010 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 3011 } 3012 3013 /** 3014 * @param value {@link #improvementNotation} (Whether improvement in the measure 3015 * is noted by an increase or decrease in the measure score.) 3016 */ 3017 public MeasureReport setImprovementNotation(CodeableConcept value) { 3018 this.improvementNotation = value; 3019 return this; 3020 } 3021 3022 /** 3023 * @return {@link #group} (The results of the calculation, one for each 3024 * population group in the measure.) 3025 */ 3026 public List<MeasureReportGroupComponent> getGroup() { 3027 if (this.group == null) 3028 this.group = new ArrayList<MeasureReportGroupComponent>(); 3029 return this.group; 3030 } 3031 3032 /** 3033 * @return Returns a reference to <code>this</code> for easy method chaining 3034 */ 3035 public MeasureReport setGroup(List<MeasureReportGroupComponent> theGroup) { 3036 this.group = theGroup; 3037 return this; 3038 } 3039 3040 public boolean hasGroup() { 3041 if (this.group == null) 3042 return false; 3043 for (MeasureReportGroupComponent item : this.group) 3044 if (!item.isEmpty()) 3045 return true; 3046 return false; 3047 } 3048 3049 public MeasureReportGroupComponent addGroup() { // 3 3050 MeasureReportGroupComponent t = new MeasureReportGroupComponent(); 3051 if (this.group == null) 3052 this.group = new ArrayList<MeasureReportGroupComponent>(); 3053 this.group.add(t); 3054 return t; 3055 } 3056 3057 public MeasureReport addGroup(MeasureReportGroupComponent t) { // 3 3058 if (t == null) 3059 return this; 3060 if (this.group == null) 3061 this.group = new ArrayList<MeasureReportGroupComponent>(); 3062 this.group.add(t); 3063 return this; 3064 } 3065 3066 /** 3067 * @return The first repetition of repeating field {@link #group}, creating it 3068 * if it does not already exist 3069 */ 3070 public MeasureReportGroupComponent getGroupFirstRep() { 3071 if (getGroup().isEmpty()) { 3072 addGroup(); 3073 } 3074 return getGroup().get(0); 3075 } 3076 3077 /** 3078 * @return {@link #evaluatedResource} (A reference to a Bundle containing the 3079 * Resources that were used in the calculation of this measure.) 3080 */ 3081 public List<Reference> getEvaluatedResource() { 3082 if (this.evaluatedResource == null) 3083 this.evaluatedResource = new ArrayList<Reference>(); 3084 return this.evaluatedResource; 3085 } 3086 3087 /** 3088 * @return Returns a reference to <code>this</code> for easy method chaining 3089 */ 3090 public MeasureReport setEvaluatedResource(List<Reference> theEvaluatedResource) { 3091 this.evaluatedResource = theEvaluatedResource; 3092 return this; 3093 } 3094 3095 public boolean hasEvaluatedResource() { 3096 if (this.evaluatedResource == null) 3097 return false; 3098 for (Reference item : this.evaluatedResource) 3099 if (!item.isEmpty()) 3100 return true; 3101 return false; 3102 } 3103 3104 public Reference addEvaluatedResource() { // 3 3105 Reference t = new Reference(); 3106 if (this.evaluatedResource == null) 3107 this.evaluatedResource = new ArrayList<Reference>(); 3108 this.evaluatedResource.add(t); 3109 return t; 3110 } 3111 3112 public MeasureReport addEvaluatedResource(Reference t) { // 3 3113 if (t == null) 3114 return this; 3115 if (this.evaluatedResource == null) 3116 this.evaluatedResource = new ArrayList<Reference>(); 3117 this.evaluatedResource.add(t); 3118 return this; 3119 } 3120 3121 /** 3122 * @return The first repetition of repeating field {@link #evaluatedResource}, 3123 * creating it if it does not already exist 3124 */ 3125 public Reference getEvaluatedResourceFirstRep() { 3126 if (getEvaluatedResource().isEmpty()) { 3127 addEvaluatedResource(); 3128 } 3129 return getEvaluatedResource().get(0); 3130 } 3131 3132 protected void listChildren(List<Property> children) { 3133 super.listChildren(children); 3134 children.add(new Property("identifier", "Identifier", 3135 "A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance.", 3136 0, java.lang.Integer.MAX_VALUE, identifier)); 3137 children.add(new Property("status", "code", 3138 "The MeasureReport status. No data will be available until the MeasureReport status is complete.", 0, 1, 3139 status)); 3140 children.add(new Property("type", "code", 3141 "The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.", 3142 0, 1, type)); 3143 children.add(new Property("measure", "canonical(Measure)", 3144 "A reference to the Measure that was calculated to produce this report.", 0, 1, measure)); 3145 children.add( 3146 new Property("subject", "Reference(Patient|Practitioner|PractitionerRole|Location|Device|RelatedPerson|Group)", 3147 "Optional subject identifying the individual or individuals the report is for.", 0, 1, subject)); 3148 children.add(new Property("date", "dateTime", "The date this measure report was generated.", 0, 1, date)); 3149 children.add(new Property("reporter", "Reference(Practitioner|PractitionerRole|Location|Organization)", 3150 "The individual, location, or organization that is reporting the data.", 0, 1, reporter)); 3151 children.add( 3152 new Property("period", "Period", "The reporting period for which the report was calculated.", 0, 1, period)); 3153 children.add(new Property("improvementNotation", "CodeableConcept", 3154 "Whether improvement in the measure is noted by an increase or decrease in the measure score.", 0, 1, 3155 improvementNotation)); 3156 children 3157 .add(new Property("group", "", "The results of the calculation, one for each population group in the measure.", 3158 0, java.lang.Integer.MAX_VALUE, group)); 3159 children.add(new Property("evaluatedResource", "Reference(Any)", 3160 "A reference to a Bundle containing the Resources that were used in the calculation of this measure.", 0, 3161 java.lang.Integer.MAX_VALUE, evaluatedResource)); 3162 } 3163 3164 @Override 3165 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3166 switch (_hash) { 3167 case -1618432855: 3168 /* identifier */ return new Property("identifier", "Identifier", 3169 "A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance.", 3170 0, java.lang.Integer.MAX_VALUE, identifier); 3171 case -892481550: 3172 /* status */ return new Property("status", "code", 3173 "The MeasureReport status. No data will be available until the MeasureReport status is complete.", 0, 1, 3174 status); 3175 case 3575610: 3176 /* type */ return new Property("type", "code", 3177 "The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.", 3178 0, 1, type); 3179 case 938321246: 3180 /* measure */ return new Property("measure", "canonical(Measure)", 3181 "A reference to the Measure that was calculated to produce this report.", 0, 1, measure); 3182 case -1867885268: 3183 /* subject */ return new Property("subject", 3184 "Reference(Patient|Practitioner|PractitionerRole|Location|Device|RelatedPerson|Group)", 3185 "Optional subject identifying the individual or individuals the report is for.", 0, 1, subject); 3186 case 3076014: 3187 /* date */ return new Property("date", "dateTime", "The date this measure report was generated.", 0, 1, date); 3188 case -427039519: 3189 /* reporter */ return new Property("reporter", "Reference(Practitioner|PractitionerRole|Location|Organization)", 3190 "The individual, location, or organization that is reporting the data.", 0, 1, reporter); 3191 case -991726143: 3192 /* period */ return new Property("period", "Period", "The reporting period for which the report was calculated.", 3193 0, 1, period); 3194 case -2085456136: 3195 /* improvementNotation */ return new Property("improvementNotation", "CodeableConcept", 3196 "Whether improvement in the measure is noted by an increase or decrease in the measure score.", 0, 1, 3197 improvementNotation); 3198 case 98629247: 3199 /* group */ return new Property("group", "", 3200 "The results of the calculation, one for each population group in the measure.", 0, 3201 java.lang.Integer.MAX_VALUE, group); 3202 case -1056771047: 3203 /* evaluatedResource */ return new Property("evaluatedResource", "Reference(Any)", 3204 "A reference to a Bundle containing the Resources that were used in the calculation of this measure.", 0, 3205 java.lang.Integer.MAX_VALUE, evaluatedResource); 3206 default: 3207 return super.getNamedProperty(_hash, _name, _checkValid); 3208 } 3209 3210 } 3211 3212 @Override 3213 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3214 switch (hash) { 3215 case -1618432855: 3216 /* identifier */ return this.identifier == null ? new Base[0] 3217 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3218 case -892481550: 3219 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MeasureReportStatus> 3220 case 3575610: 3221 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<MeasureReportType> 3222 case 938321246: 3223 /* measure */ return this.measure == null ? new Base[0] : new Base[] { this.measure }; // CanonicalType 3224 case -1867885268: 3225 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 3226 case 3076014: 3227 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3228 case -427039519: 3229 /* reporter */ return this.reporter == null ? new Base[0] : new Base[] { this.reporter }; // Reference 3230 case -991726143: 3231 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 3232 case -2085456136: 3233 /* improvementNotation */ return this.improvementNotation == null ? new Base[0] 3234 : new Base[] { this.improvementNotation }; // CodeableConcept 3235 case 98629247: 3236 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // MeasureReportGroupComponent 3237 case -1056771047: 3238 /* evaluatedResource */ return this.evaluatedResource == null ? new Base[0] 3239 : this.evaluatedResource.toArray(new Base[this.evaluatedResource.size()]); // Reference 3240 default: 3241 return super.getProperty(hash, name, checkValid); 3242 } 3243 3244 } 3245 3246 @Override 3247 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3248 switch (hash) { 3249 case -1618432855: // identifier 3250 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3251 return value; 3252 case -892481550: // status 3253 value = new MeasureReportStatusEnumFactory().fromType(castToCode(value)); 3254 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 3255 return value; 3256 case 3575610: // type 3257 value = new MeasureReportTypeEnumFactory().fromType(castToCode(value)); 3258 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 3259 return value; 3260 case 938321246: // measure 3261 this.measure = castToCanonical(value); // CanonicalType 3262 return value; 3263 case -1867885268: // subject 3264 this.subject = castToReference(value); // Reference 3265 return value; 3266 case 3076014: // date 3267 this.date = castToDateTime(value); // DateTimeType 3268 return value; 3269 case -427039519: // reporter 3270 this.reporter = castToReference(value); // Reference 3271 return value; 3272 case -991726143: // period 3273 this.period = castToPeriod(value); // Period 3274 return value; 3275 case -2085456136: // improvementNotation 3276 this.improvementNotation = castToCodeableConcept(value); // CodeableConcept 3277 return value; 3278 case 98629247: // group 3279 this.getGroup().add((MeasureReportGroupComponent) value); // MeasureReportGroupComponent 3280 return value; 3281 case -1056771047: // evaluatedResource 3282 this.getEvaluatedResource().add(castToReference(value)); // Reference 3283 return value; 3284 default: 3285 return super.setProperty(hash, name, value); 3286 } 3287 3288 } 3289 3290 @Override 3291 public Base setProperty(String name, Base value) throws FHIRException { 3292 if (name.equals("identifier")) { 3293 this.getIdentifier().add(castToIdentifier(value)); 3294 } else if (name.equals("status")) { 3295 value = new MeasureReportStatusEnumFactory().fromType(castToCode(value)); 3296 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 3297 } else if (name.equals("type")) { 3298 value = new MeasureReportTypeEnumFactory().fromType(castToCode(value)); 3299 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 3300 } else if (name.equals("measure")) { 3301 this.measure = castToCanonical(value); // CanonicalType 3302 } else if (name.equals("subject")) { 3303 this.subject = castToReference(value); // Reference 3304 } else if (name.equals("date")) { 3305 this.date = castToDateTime(value); // DateTimeType 3306 } else if (name.equals("reporter")) { 3307 this.reporter = castToReference(value); // Reference 3308 } else if (name.equals("period")) { 3309 this.period = castToPeriod(value); // Period 3310 } else if (name.equals("improvementNotation")) { 3311 this.improvementNotation = castToCodeableConcept(value); // CodeableConcept 3312 } else if (name.equals("group")) { 3313 this.getGroup().add((MeasureReportGroupComponent) value); 3314 } else if (name.equals("evaluatedResource")) { 3315 this.getEvaluatedResource().add(castToReference(value)); 3316 } else 3317 return super.setProperty(name, value); 3318 return value; 3319 } 3320 3321 @Override 3322 public void removeChild(String name, Base value) throws FHIRException { 3323 if (name.equals("identifier")) { 3324 this.getIdentifier().remove(castToIdentifier(value)); 3325 } else if (name.equals("status")) { 3326 this.status = null; 3327 } else if (name.equals("type")) { 3328 this.type = null; 3329 } else if (name.equals("measure")) { 3330 this.measure = null; 3331 } else if (name.equals("subject")) { 3332 this.subject = null; 3333 } else if (name.equals("date")) { 3334 this.date = null; 3335 } else if (name.equals("reporter")) { 3336 this.reporter = null; 3337 } else if (name.equals("period")) { 3338 this.period = null; 3339 } else if (name.equals("improvementNotation")) { 3340 this.improvementNotation = null; 3341 } else if (name.equals("group")) { 3342 this.getGroup().remove((MeasureReportGroupComponent) value); 3343 } else if (name.equals("evaluatedResource")) { 3344 this.getEvaluatedResource().remove(castToReference(value)); 3345 } else 3346 super.removeChild(name, value); 3347 3348 } 3349 3350 @Override 3351 public Base makeProperty(int hash, String name) throws FHIRException { 3352 switch (hash) { 3353 case -1618432855: 3354 return addIdentifier(); 3355 case -892481550: 3356 return getStatusElement(); 3357 case 3575610: 3358 return getTypeElement(); 3359 case 938321246: 3360 return getMeasureElement(); 3361 case -1867885268: 3362 return getSubject(); 3363 case 3076014: 3364 return getDateElement(); 3365 case -427039519: 3366 return getReporter(); 3367 case -991726143: 3368 return getPeriod(); 3369 case -2085456136: 3370 return getImprovementNotation(); 3371 case 98629247: 3372 return addGroup(); 3373 case -1056771047: 3374 return addEvaluatedResource(); 3375 default: 3376 return super.makeProperty(hash, name); 3377 } 3378 3379 } 3380 3381 @Override 3382 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3383 switch (hash) { 3384 case -1618432855: 3385 /* identifier */ return new String[] { "Identifier" }; 3386 case -892481550: 3387 /* status */ return new String[] { "code" }; 3388 case 3575610: 3389 /* type */ return new String[] { "code" }; 3390 case 938321246: 3391 /* measure */ return new String[] { "canonical" }; 3392 case -1867885268: 3393 /* subject */ return new String[] { "Reference" }; 3394 case 3076014: 3395 /* date */ return new String[] { "dateTime" }; 3396 case -427039519: 3397 /* reporter */ return new String[] { "Reference" }; 3398 case -991726143: 3399 /* period */ return new String[] { "Period" }; 3400 case -2085456136: 3401 /* improvementNotation */ return new String[] { "CodeableConcept" }; 3402 case 98629247: 3403 /* group */ return new String[] {}; 3404 case -1056771047: 3405 /* evaluatedResource */ return new String[] { "Reference" }; 3406 default: 3407 return super.getTypesForProperty(hash, name); 3408 } 3409 3410 } 3411 3412 @Override 3413 public Base addChild(String name) throws FHIRException { 3414 if (name.equals("identifier")) { 3415 return addIdentifier(); 3416 } else if (name.equals("status")) { 3417 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.status"); 3418 } else if (name.equals("type")) { 3419 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.type"); 3420 } else if (name.equals("measure")) { 3421 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.measure"); 3422 } else if (name.equals("subject")) { 3423 this.subject = new Reference(); 3424 return this.subject; 3425 } else if (name.equals("date")) { 3426 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.date"); 3427 } else if (name.equals("reporter")) { 3428 this.reporter = new Reference(); 3429 return this.reporter; 3430 } else if (name.equals("period")) { 3431 this.period = new Period(); 3432 return this.period; 3433 } else if (name.equals("improvementNotation")) { 3434 this.improvementNotation = new CodeableConcept(); 3435 return this.improvementNotation; 3436 } else if (name.equals("group")) { 3437 return addGroup(); 3438 } else if (name.equals("evaluatedResource")) { 3439 return addEvaluatedResource(); 3440 } else 3441 return super.addChild(name); 3442 } 3443 3444 public String fhirType() { 3445 return "MeasureReport"; 3446 3447 } 3448 3449 public MeasureReport copy() { 3450 MeasureReport dst = new MeasureReport(); 3451 copyValues(dst); 3452 return dst; 3453 } 3454 3455 public void copyValues(MeasureReport dst) { 3456 super.copyValues(dst); 3457 if (identifier != null) { 3458 dst.identifier = new ArrayList<Identifier>(); 3459 for (Identifier i : identifier) 3460 dst.identifier.add(i.copy()); 3461 } 3462 ; 3463 dst.status = status == null ? null : status.copy(); 3464 dst.type = type == null ? null : type.copy(); 3465 dst.measure = measure == null ? null : measure.copy(); 3466 dst.subject = subject == null ? null : subject.copy(); 3467 dst.date = date == null ? null : date.copy(); 3468 dst.reporter = reporter == null ? null : reporter.copy(); 3469 dst.period = period == null ? null : period.copy(); 3470 dst.improvementNotation = improvementNotation == null ? null : improvementNotation.copy(); 3471 if (group != null) { 3472 dst.group = new ArrayList<MeasureReportGroupComponent>(); 3473 for (MeasureReportGroupComponent i : group) 3474 dst.group.add(i.copy()); 3475 } 3476 ; 3477 if (evaluatedResource != null) { 3478 dst.evaluatedResource = new ArrayList<Reference>(); 3479 for (Reference i : evaluatedResource) 3480 dst.evaluatedResource.add(i.copy()); 3481 } 3482 ; 3483 } 3484 3485 protected MeasureReport typedCopy() { 3486 return copy(); 3487 } 3488 3489 @Override 3490 public boolean equalsDeep(Base other_) { 3491 if (!super.equalsDeep(other_)) 3492 return false; 3493 if (!(other_ instanceof MeasureReport)) 3494 return false; 3495 MeasureReport o = (MeasureReport) other_; 3496 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 3497 && compareDeep(type, o.type, true) && compareDeep(measure, o.measure, true) 3498 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 3499 && compareDeep(reporter, o.reporter, true) && compareDeep(period, o.period, true) 3500 && compareDeep(improvementNotation, o.improvementNotation, true) && compareDeep(group, o.group, true) 3501 && compareDeep(evaluatedResource, o.evaluatedResource, true); 3502 } 3503 3504 @Override 3505 public boolean equalsShallow(Base other_) { 3506 if (!super.equalsShallow(other_)) 3507 return false; 3508 if (!(other_ instanceof MeasureReport)) 3509 return false; 3510 MeasureReport o = (MeasureReport) other_; 3511 return compareValues(status, o.status, true) && compareValues(type, o.type, true) 3512 && compareValues(date, o.date, true); 3513 } 3514 3515 public boolean isEmpty() { 3516 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, measure, subject, date, 3517 reporter, period, improvementNotation, group, evaluatedResource); 3518 } 3519 3520 @Override 3521 public ResourceType getResourceType() { 3522 return ResourceType.MeasureReport; 3523 } 3524 3525 /** 3526 * Search parameter: <b>date</b> 3527 * <p> 3528 * Description: <b>The date of the measure report</b><br> 3529 * Type: <b>date</b><br> 3530 * Path: <b>MeasureReport.date</b><br> 3531 * </p> 3532 */ 3533 @SearchParamDefinition(name = "date", path = "MeasureReport.date", description = "The date of the measure report", type = "date") 3534 public static final String SP_DATE = "date"; 3535 /** 3536 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3537 * <p> 3538 * Description: <b>The date of the measure report</b><br> 3539 * Type: <b>date</b><br> 3540 * Path: <b>MeasureReport.date</b><br> 3541 * </p> 3542 */ 3543 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3544 SP_DATE); 3545 3546 /** 3547 * Search parameter: <b>identifier</b> 3548 * <p> 3549 * Description: <b>External identifier of the measure report to be 3550 * returned</b><br> 3551 * Type: <b>token</b><br> 3552 * Path: <b>MeasureReport.identifier</b><br> 3553 * </p> 3554 */ 3555 @SearchParamDefinition(name = "identifier", path = "MeasureReport.identifier", description = "External identifier of the measure report to be returned", type = "token") 3556 public static final String SP_IDENTIFIER = "identifier"; 3557 /** 3558 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3559 * <p> 3560 * Description: <b>External identifier of the measure report to be 3561 * returned</b><br> 3562 * Type: <b>token</b><br> 3563 * Path: <b>MeasureReport.identifier</b><br> 3564 * </p> 3565 */ 3566 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3567 SP_IDENTIFIER); 3568 3569 /** 3570 * Search parameter: <b>period</b> 3571 * <p> 3572 * Description: <b>The period of the measure report</b><br> 3573 * Type: <b>date</b><br> 3574 * Path: <b>MeasureReport.period</b><br> 3575 * </p> 3576 */ 3577 @SearchParamDefinition(name = "period", path = "MeasureReport.period", description = "The period of the measure report", type = "date") 3578 public static final String SP_PERIOD = "period"; 3579 /** 3580 * <b>Fluent Client</b> search parameter constant for <b>period</b> 3581 * <p> 3582 * Description: <b>The period of the measure report</b><br> 3583 * Type: <b>date</b><br> 3584 * Path: <b>MeasureReport.period</b><br> 3585 * </p> 3586 */ 3587 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 3588 SP_PERIOD); 3589 3590 /** 3591 * Search parameter: <b>measure</b> 3592 * <p> 3593 * Description: <b>The measure to return measure report results for</b><br> 3594 * Type: <b>reference</b><br> 3595 * Path: <b>MeasureReport.measure</b><br> 3596 * </p> 3597 */ 3598 @SearchParamDefinition(name = "measure", path = "MeasureReport.measure", description = "The measure to return measure report results for", type = "reference", target = { 3599 Measure.class }) 3600 public static final String SP_MEASURE = "measure"; 3601 /** 3602 * <b>Fluent Client</b> search parameter constant for <b>measure</b> 3603 * <p> 3604 * Description: <b>The measure to return measure report results for</b><br> 3605 * Type: <b>reference</b><br> 3606 * Path: <b>MeasureReport.measure</b><br> 3607 * </p> 3608 */ 3609 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEASURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3610 SP_MEASURE); 3611 3612 /** 3613 * Constant for fluent queries to be used to add include statements. Specifies 3614 * the path value of "<b>MeasureReport:measure</b>". 3615 */ 3616 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEASURE = new ca.uhn.fhir.model.api.Include( 3617 "MeasureReport:measure").toLocked(); 3618 3619 /** 3620 * Search parameter: <b>patient</b> 3621 * <p> 3622 * Description: <b>The identity of a patient to search for individual measure 3623 * report results for</b><br> 3624 * Type: <b>reference</b><br> 3625 * Path: <b>MeasureReport.subject</b><br> 3626 * </p> 3627 */ 3628 @SearchParamDefinition(name = "patient", path = "MeasureReport.subject.where(resolve() is Patient)", description = "The identity of a patient to search for individual measure report results for", type = "reference", providesMembershipIn = { 3629 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3630 public static final String SP_PATIENT = "patient"; 3631 /** 3632 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3633 * <p> 3634 * Description: <b>The identity of a patient to search for individual measure 3635 * report results for</b><br> 3636 * Type: <b>reference</b><br> 3637 * Path: <b>MeasureReport.subject</b><br> 3638 * </p> 3639 */ 3640 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3641 SP_PATIENT); 3642 3643 /** 3644 * Constant for fluent queries to be used to add include statements. Specifies 3645 * the path value of "<b>MeasureReport:patient</b>". 3646 */ 3647 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3648 "MeasureReport:patient").toLocked(); 3649 3650 /** 3651 * Search parameter: <b>subject</b> 3652 * <p> 3653 * Description: <b>The identity of a subject to search for individual measure 3654 * report results for</b><br> 3655 * Type: <b>reference</b><br> 3656 * Path: <b>MeasureReport.subject</b><br> 3657 * </p> 3658 */ 3659 @SearchParamDefinition(name = "subject", path = "MeasureReport.subject", description = "The identity of a subject to search for individual measure report results for", type = "reference", target = { 3660 Device.class, Group.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, 3661 RelatedPerson.class }) 3662 public static final String SP_SUBJECT = "subject"; 3663 /** 3664 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3665 * <p> 3666 * Description: <b>The identity of a subject to search for individual measure 3667 * report results for</b><br> 3668 * Type: <b>reference</b><br> 3669 * Path: <b>MeasureReport.subject</b><br> 3670 * </p> 3671 */ 3672 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3673 SP_SUBJECT); 3674 3675 /** 3676 * Constant for fluent queries to be used to add include statements. Specifies 3677 * the path value of "<b>MeasureReport:subject</b>". 3678 */ 3679 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3680 "MeasureReport:subject").toLocked(); 3681 3682 /** 3683 * Search parameter: <b>reporter</b> 3684 * <p> 3685 * Description: <b>The reporter to return measure report results for</b><br> 3686 * Type: <b>reference</b><br> 3687 * Path: <b>MeasureReport.reporter</b><br> 3688 * </p> 3689 */ 3690 @SearchParamDefinition(name = "reporter", path = "MeasureReport.reporter", description = "The reporter to return measure report results for", type = "reference", target = { 3691 Location.class, Organization.class, Practitioner.class, PractitionerRole.class }) 3692 public static final String SP_REPORTER = "reporter"; 3693 /** 3694 * <b>Fluent Client</b> search parameter constant for <b>reporter</b> 3695 * <p> 3696 * Description: <b>The reporter to return measure report results for</b><br> 3697 * Type: <b>reference</b><br> 3698 * Path: <b>MeasureReport.reporter</b><br> 3699 * </p> 3700 */ 3701 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPORTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3702 SP_REPORTER); 3703 3704 /** 3705 * Constant for fluent queries to be used to add include statements. Specifies 3706 * the path value of "<b>MeasureReport:reporter</b>". 3707 */ 3708 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPORTER = new ca.uhn.fhir.model.api.Include( 3709 "MeasureReport:reporter").toLocked(); 3710 3711 /** 3712 * Search parameter: <b>status</b> 3713 * <p> 3714 * Description: <b>The status of the measure report</b><br> 3715 * Type: <b>token</b><br> 3716 * Path: <b>MeasureReport.status</b><br> 3717 * </p> 3718 */ 3719 @SearchParamDefinition(name = "status", path = "MeasureReport.status", description = "The status of the measure report", type = "token") 3720 public static final String SP_STATUS = "status"; 3721 /** 3722 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3723 * <p> 3724 * Description: <b>The status of the measure report</b><br> 3725 * Type: <b>token</b><br> 3726 * Path: <b>MeasureReport.status</b><br> 3727 * </p> 3728 */ 3729 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3730 SP_STATUS); 3731 3732 /** 3733 * Search parameter: <b>evaluated-resource</b> 3734 * <p> 3735 * Description: <b>An evaluated resource referenced by the measure 3736 * report</b><br> 3737 * Type: <b>reference</b><br> 3738 * Path: <b>MeasureReport.evaluatedResource</b><br> 3739 * </p> 3740 */ 3741 @SearchParamDefinition(name = "evaluated-resource", path = "MeasureReport.evaluatedResource", description = "An evaluated resource referenced by the measure report", type = "reference") 3742 public static final String SP_EVALUATED_RESOURCE = "evaluated-resource"; 3743 /** 3744 * <b>Fluent Client</b> search parameter constant for <b>evaluated-resource</b> 3745 * <p> 3746 * Description: <b>An evaluated resource referenced by the measure 3747 * report</b><br> 3748 * Type: <b>reference</b><br> 3749 * Path: <b>MeasureReport.evaluatedResource</b><br> 3750 * </p> 3751 */ 3752 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVALUATED_RESOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3753 SP_EVALUATED_RESOURCE); 3754 3755 /** 3756 * Constant for fluent queries to be used to add include statements. Specifies 3757 * the path value of "<b>MeasureReport:evaluated-resource</b>". 3758 */ 3759 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVALUATED_RESOURCE = new ca.uhn.fhir.model.api.Include( 3760 "MeasureReport:evaluated-resource").toLocked(); 3761 3762}