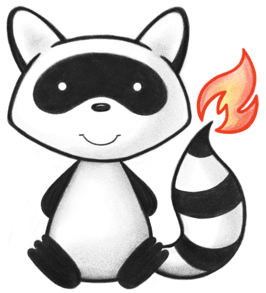
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A photo, video, or audio recording acquired or used in healthcare. The actual 049 * content may be inline or provided by direct reference. 050 */ 051@ResourceDef(name = "Media", profile = "http://hl7.org/fhir/StructureDefinition/Media") 052public class Media extends DomainResource { 053 054 public enum MediaStatus { 055 /** 056 * The core event has not started yet, but some staging activities have begun 057 * (e.g. surgical suite preparation). Preparation stages may be tracked for 058 * billing purposes. 059 */ 060 PREPARATION, 061 /** 062 * The event is currently occurring. 063 */ 064 INPROGRESS, 065 /** 066 * The event was terminated prior to any activity beyond preparation. I.e. The 067 * 'main' activity has not yet begun. The boundary between preparatory and the 068 * 'main' activity is context-specific. 069 */ 070 NOTDONE, 071 /** 072 * The event has been temporarily stopped but is expected to resume in the 073 * future. 074 */ 075 ONHOLD, 076 /** 077 * The event was terminated prior to the full completion of the intended 078 * activity but after at least some of the 'main' activity (beyond preparation) 079 * has occurred. 080 */ 081 STOPPED, 082 /** 083 * The event has now concluded. 084 */ 085 COMPLETED, 086 /** 087 * This electronic record should never have existed, though it is possible that 088 * real-world decisions were based on it. (If real-world activity has occurred, 089 * the status should be "stopped" rather than "entered-in-error".). 090 */ 091 ENTEREDINERROR, 092 /** 093 * The authoring/source system does not know which of the status values 094 * currently applies for this event. Note: This concept is not to be used for 095 * "other" - one of the listed statuses is presumed to apply, but the 096 * authoring/source system does not know which. 097 */ 098 UNKNOWN, 099 /** 100 * added to help the parsers with the generic types 101 */ 102 NULL; 103 104 public static MediaStatus fromCode(String codeString) throws FHIRException { 105 if (codeString == null || "".equals(codeString)) 106 return null; 107 if ("preparation".equals(codeString)) 108 return PREPARATION; 109 if ("in-progress".equals(codeString)) 110 return INPROGRESS; 111 if ("not-done".equals(codeString)) 112 return NOTDONE; 113 if ("on-hold".equals(codeString)) 114 return ONHOLD; 115 if ("stopped".equals(codeString)) 116 return STOPPED; 117 if ("completed".equals(codeString)) 118 return COMPLETED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 if ("unknown".equals(codeString)) 122 return UNKNOWN; 123 if (Configuration.isAcceptInvalidEnums()) 124 return null; 125 else 126 throw new FHIRException("Unknown MediaStatus code '" + codeString + "'"); 127 } 128 129 public String toCode() { 130 switch (this) { 131 case PREPARATION: 132 return "preparation"; 133 case INPROGRESS: 134 return "in-progress"; 135 case NOTDONE: 136 return "not-done"; 137 case ONHOLD: 138 return "on-hold"; 139 case STOPPED: 140 return "stopped"; 141 case COMPLETED: 142 return "completed"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case UNKNOWN: 146 return "unknown"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PREPARATION: 157 return "http://hl7.org/fhir/event-status"; 158 case INPROGRESS: 159 return "http://hl7.org/fhir/event-status"; 160 case NOTDONE: 161 return "http://hl7.org/fhir/event-status"; 162 case ONHOLD: 163 return "http://hl7.org/fhir/event-status"; 164 case STOPPED: 165 return "http://hl7.org/fhir/event-status"; 166 case COMPLETED: 167 return "http://hl7.org/fhir/event-status"; 168 case ENTEREDINERROR: 169 return "http://hl7.org/fhir/event-status"; 170 case UNKNOWN: 171 return "http://hl7.org/fhir/event-status"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDefinition() { 180 switch (this) { 181 case PREPARATION: 182 return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 183 case INPROGRESS: 184 return "The event is currently occurring."; 185 case NOTDONE: 186 return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 187 case ONHOLD: 188 return "The event has been temporarily stopped but is expected to resume in the future."; 189 case STOPPED: 190 return "The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred."; 191 case COMPLETED: 192 return "The event has now concluded."; 193 case ENTEREDINERROR: 194 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 195 case UNKNOWN: 196 return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204 public String getDisplay() { 205 switch (this) { 206 case PREPARATION: 207 return "Preparation"; 208 case INPROGRESS: 209 return "In Progress"; 210 case NOTDONE: 211 return "Not Done"; 212 case ONHOLD: 213 return "On Hold"; 214 case STOPPED: 215 return "Stopped"; 216 case COMPLETED: 217 return "Completed"; 218 case ENTEREDINERROR: 219 return "Entered in Error"; 220 case UNKNOWN: 221 return "Unknown"; 222 case NULL: 223 return null; 224 default: 225 return "?"; 226 } 227 } 228 } 229 230 public static class MediaStatusEnumFactory implements EnumFactory<MediaStatus> { 231 public MediaStatus fromCode(String codeString) throws IllegalArgumentException { 232 if (codeString == null || "".equals(codeString)) 233 if (codeString == null || "".equals(codeString)) 234 return null; 235 if ("preparation".equals(codeString)) 236 return MediaStatus.PREPARATION; 237 if ("in-progress".equals(codeString)) 238 return MediaStatus.INPROGRESS; 239 if ("not-done".equals(codeString)) 240 return MediaStatus.NOTDONE; 241 if ("on-hold".equals(codeString)) 242 return MediaStatus.ONHOLD; 243 if ("stopped".equals(codeString)) 244 return MediaStatus.STOPPED; 245 if ("completed".equals(codeString)) 246 return MediaStatus.COMPLETED; 247 if ("entered-in-error".equals(codeString)) 248 return MediaStatus.ENTEREDINERROR; 249 if ("unknown".equals(codeString)) 250 return MediaStatus.UNKNOWN; 251 throw new IllegalArgumentException("Unknown MediaStatus code '" + codeString + "'"); 252 } 253 254 public Enumeration<MediaStatus> fromType(PrimitiveType<?> code) throws FHIRException { 255 if (code == null) 256 return null; 257 if (code.isEmpty()) 258 return new Enumeration<MediaStatus>(this, MediaStatus.NULL, code); 259 String codeString = code.asStringValue(); 260 if (codeString == null || "".equals(codeString)) 261 return new Enumeration<MediaStatus>(this, MediaStatus.NULL, code); 262 if ("preparation".equals(codeString)) 263 return new Enumeration<MediaStatus>(this, MediaStatus.PREPARATION, code); 264 if ("in-progress".equals(codeString)) 265 return new Enumeration<MediaStatus>(this, MediaStatus.INPROGRESS, code); 266 if ("not-done".equals(codeString)) 267 return new Enumeration<MediaStatus>(this, MediaStatus.NOTDONE, code); 268 if ("on-hold".equals(codeString)) 269 return new Enumeration<MediaStatus>(this, MediaStatus.ONHOLD, code); 270 if ("stopped".equals(codeString)) 271 return new Enumeration<MediaStatus>(this, MediaStatus.STOPPED, code); 272 if ("completed".equals(codeString)) 273 return new Enumeration<MediaStatus>(this, MediaStatus.COMPLETED, code); 274 if ("entered-in-error".equals(codeString)) 275 return new Enumeration<MediaStatus>(this, MediaStatus.ENTEREDINERROR, code); 276 if ("unknown".equals(codeString)) 277 return new Enumeration<MediaStatus>(this, MediaStatus.UNKNOWN, code); 278 throw new FHIRException("Unknown MediaStatus code '" + codeString + "'"); 279 } 280 281 public String toCode(MediaStatus code) { 282 if (code == MediaStatus.NULL) 283 return null; 284 if (code == MediaStatus.PREPARATION) 285 return "preparation"; 286 if (code == MediaStatus.INPROGRESS) 287 return "in-progress"; 288 if (code == MediaStatus.NOTDONE) 289 return "not-done"; 290 if (code == MediaStatus.ONHOLD) 291 return "on-hold"; 292 if (code == MediaStatus.STOPPED) 293 return "stopped"; 294 if (code == MediaStatus.COMPLETED) 295 return "completed"; 296 if (code == MediaStatus.ENTEREDINERROR) 297 return "entered-in-error"; 298 if (code == MediaStatus.UNKNOWN) 299 return "unknown"; 300 return "?"; 301 } 302 303 public String toSystem(MediaStatus code) { 304 return code.getSystem(); 305 } 306 } 307 308 /** 309 * Identifiers associated with the image - these may include identifiers for the 310 * image itself, identifiers for the context of its collection (e.g. series ids) 311 * and context ids such as accession numbers or other workflow identifiers. 312 */ 313 @Child(name = "identifier", type = { 314 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 315 @Description(shortDefinition = "Identifier(s) for the image", formalDefinition = "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.") 316 protected List<Identifier> identifier; 317 318 /** 319 * A procedure that is fulfilled in whole or in part by the creation of this 320 * media. 321 */ 322 @Child(name = "basedOn", type = { ServiceRequest.class, 323 CarePlan.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 324 @Description(shortDefinition = "Procedure that caused this media to be created", formalDefinition = "A procedure that is fulfilled in whole or in part by the creation of this media.") 325 protected List<Reference> basedOn; 326 /** 327 * The actual objects that are the target of the reference (A procedure that is 328 * fulfilled in whole or in part by the creation of this media.) 329 */ 330 protected List<Resource> basedOnTarget; 331 332 /** 333 * A larger event of which this particular event is a component or step. 334 */ 335 @Child(name = "partOf", type = { 336 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 337 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 338 protected List<Reference> partOf; 339 /** 340 * The actual objects that are the target of the reference (A larger event of 341 * which this particular event is a component or step.) 342 */ 343 protected List<Resource> partOfTarget; 344 345 /** 346 * The current state of the {{title}}. 347 */ 348 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 349 @Description(shortDefinition = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition = "The current state of the {{title}}.") 350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-status") 351 protected Enumeration<MediaStatus> status; 352 353 /** 354 * A code that classifies whether the media is an image, video or audio 355 * recording or some other media category. 356 */ 357 @Child(name = "type", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 358 @Description(shortDefinition = "Classification of media as image, video, or audio", formalDefinition = "A code that classifies whether the media is an image, video or audio recording or some other media category.") 359 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/media-type") 360 protected CodeableConcept type; 361 362 /** 363 * Details of the type of the media - usually, how it was acquired (what type of 364 * device). If images sourced from a DICOM system, are wrapped in a Media 365 * resource, then this is the modality. 366 */ 367 @Child(name = "modality", type = { 368 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 369 @Description(shortDefinition = "The type of acquisition equipment/process", formalDefinition = "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.") 370 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/media-modality") 371 protected CodeableConcept modality; 372 373 /** 374 * The name of the imaging view e.g. Lateral or Antero-posterior (AP). 375 */ 376 @Child(name = "view", type = { CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 377 @Description(shortDefinition = "Imaging view, e.g. Lateral or Antero-posterior", formalDefinition = "The name of the imaging view e.g. Lateral or Antero-posterior (AP).") 378 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/media-view") 379 protected CodeableConcept view; 380 381 /** 382 * Who/What this Media is a record of. 383 */ 384 @Child(name = "subject", type = { Patient.class, Practitioner.class, PractitionerRole.class, Group.class, 385 Device.class, Specimen.class, Location.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 386 @Description(shortDefinition = "Who/What this Media is a record of", formalDefinition = "Who/What this Media is a record of.") 387 protected Reference subject; 388 389 /** 390 * The actual object that is the target of the reference (Who/What this Media is 391 * a record of.) 392 */ 393 protected Resource subjectTarget; 394 395 /** 396 * The encounter that establishes the context for this media. 397 */ 398 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 399 @Description(shortDefinition = "Encounter associated with media", formalDefinition = "The encounter that establishes the context for this media.") 400 protected Reference encounter; 401 402 /** 403 * The actual object that is the target of the reference (The encounter that 404 * establishes the context for this media.) 405 */ 406 protected Encounter encounterTarget; 407 408 /** 409 * The date and time(s) at which the media was collected. 410 */ 411 @Child(name = "created", type = { DateTimeType.class, 412 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 413 @Description(shortDefinition = "When Media was collected", formalDefinition = "The date and time(s) at which the media was collected.") 414 protected Type created; 415 416 /** 417 * The date and time this version of the media was made available to providers, 418 * typically after having been reviewed. 419 */ 420 @Child(name = "issued", type = { InstantType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 421 @Description(shortDefinition = "Date/Time this version was made available", formalDefinition = "The date and time this version of the media was made available to providers, typically after having been reviewed.") 422 protected InstantType issued; 423 424 /** 425 * The person who administered the collection of the image. 426 */ 427 @Child(name = "operator", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 428 Patient.class, Device.class, 429 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 430 @Description(shortDefinition = "The person who generated the image", formalDefinition = "The person who administered the collection of the image.") 431 protected Reference operator; 432 433 /** 434 * The actual object that is the target of the reference (The person who 435 * administered the collection of the image.) 436 */ 437 protected Resource operatorTarget; 438 439 /** 440 * Describes why the event occurred in coded or textual form. 441 */ 442 @Child(name = "reasonCode", type = { 443 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 444 @Description(shortDefinition = "Why was event performed?", formalDefinition = "Describes why the event occurred in coded or textual form.") 445 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-reason") 446 protected List<CodeableConcept> reasonCode; 447 448 /** 449 * Indicates the site on the subject's body where the observation was made (i.e. 450 * the target site). 451 */ 452 @Child(name = "bodySite", type = { 453 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 454 @Description(shortDefinition = "Observed body part", formalDefinition = "Indicates the site on the subject's body where the observation was made (i.e. the target site).") 455 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 456 protected CodeableConcept bodySite; 457 458 /** 459 * The name of the device / manufacturer of the device that was used to make the 460 * recording. 461 */ 462 @Child(name = "deviceName", type = { 463 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 464 @Description(shortDefinition = "Name of the device/manufacturer", formalDefinition = "The name of the device / manufacturer of the device that was used to make the recording.") 465 protected StringType deviceName; 466 467 /** 468 * The device used to collect the media. 469 */ 470 @Child(name = "device", type = { Device.class, DeviceMetric.class, 471 Device.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 472 @Description(shortDefinition = "Observing Device", formalDefinition = "The device used to collect the media.") 473 protected Reference device; 474 475 /** 476 * The actual object that is the target of the reference (The device used to 477 * collect the media.) 478 */ 479 protected Resource deviceTarget; 480 481 /** 482 * Height of the image in pixels (photo/video). 483 */ 484 @Child(name = "height", type = { 485 PositiveIntType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 486 @Description(shortDefinition = "Height of the image in pixels (photo/video)", formalDefinition = "Height of the image in pixels (photo/video).") 487 protected PositiveIntType height; 488 489 /** 490 * Width of the image in pixels (photo/video). 491 */ 492 @Child(name = "width", type = { 493 PositiveIntType.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 494 @Description(shortDefinition = "Width of the image in pixels (photo/video)", formalDefinition = "Width of the image in pixels (photo/video).") 495 protected PositiveIntType width; 496 497 /** 498 * The number of frames in a photo. This is used with a multi-page fax, or an 499 * imaging acquisition context that takes multiple slices in a single image, or 500 * an animated gif. If there is more than one frame, this SHALL have a value in 501 * order to alert interface software that a multi-frame capable rendering widget 502 * is required. 503 */ 504 @Child(name = "frames", type = { 505 PositiveIntType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 506 @Description(shortDefinition = "Number of frames if > 1 (photo)", formalDefinition = "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.") 507 protected PositiveIntType frames; 508 509 /** 510 * The duration of the recording in seconds - for audio and video. 511 */ 512 @Child(name = "duration", type = { 513 DecimalType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 514 @Description(shortDefinition = "Length in seconds (audio / video)", formalDefinition = "The duration of the recording in seconds - for audio and video.") 515 protected DecimalType duration; 516 517 /** 518 * The actual content of the media - inline or by direct reference to the media 519 * source file. 520 */ 521 @Child(name = "content", type = { Attachment.class }, order = 20, min = 1, max = 1, modifier = false, summary = true) 522 @Description(shortDefinition = "Actual Media - reference or data", formalDefinition = "The actual content of the media - inline or by direct reference to the media source file.") 523 protected Attachment content; 524 525 /** 526 * Comments made about the media by the performer, subject or other 527 * participants. 528 */ 529 @Child(name = "note", type = { 530 Annotation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 531 @Description(shortDefinition = "Comments made about the media", formalDefinition = "Comments made about the media by the performer, subject or other participants.") 532 protected List<Annotation> note; 533 534 private static final long serialVersionUID = 2069980126L; 535 536 /** 537 * Constructor 538 */ 539 public Media() { 540 super(); 541 } 542 543 /** 544 * Constructor 545 */ 546 public Media(Enumeration<MediaStatus> status, Attachment content) { 547 super(); 548 this.status = status; 549 this.content = content; 550 } 551 552 /** 553 * @return {@link #identifier} (Identifiers associated with the image - these 554 * may include identifiers for the image itself, identifiers for the 555 * context of its collection (e.g. series ids) and context ids such as 556 * accession numbers or other workflow identifiers.) 557 */ 558 public List<Identifier> getIdentifier() { 559 if (this.identifier == null) 560 this.identifier = new ArrayList<Identifier>(); 561 return this.identifier; 562 } 563 564 /** 565 * @return Returns a reference to <code>this</code> for easy method chaining 566 */ 567 public Media setIdentifier(List<Identifier> theIdentifier) { 568 this.identifier = theIdentifier; 569 return this; 570 } 571 572 public boolean hasIdentifier() { 573 if (this.identifier == null) 574 return false; 575 for (Identifier item : this.identifier) 576 if (!item.isEmpty()) 577 return true; 578 return false; 579 } 580 581 public Identifier addIdentifier() { // 3 582 Identifier t = new Identifier(); 583 if (this.identifier == null) 584 this.identifier = new ArrayList<Identifier>(); 585 this.identifier.add(t); 586 return t; 587 } 588 589 public Media addIdentifier(Identifier t) { // 3 590 if (t == null) 591 return this; 592 if (this.identifier == null) 593 this.identifier = new ArrayList<Identifier>(); 594 this.identifier.add(t); 595 return this; 596 } 597 598 /** 599 * @return The first repetition of repeating field {@link #identifier}, creating 600 * it if it does not already exist 601 */ 602 public Identifier getIdentifierFirstRep() { 603 if (getIdentifier().isEmpty()) { 604 addIdentifier(); 605 } 606 return getIdentifier().get(0); 607 } 608 609 /** 610 * @return {@link #basedOn} (A procedure that is fulfilled in whole or in part 611 * by the creation of this media.) 612 */ 613 public List<Reference> getBasedOn() { 614 if (this.basedOn == null) 615 this.basedOn = new ArrayList<Reference>(); 616 return this.basedOn; 617 } 618 619 /** 620 * @return Returns a reference to <code>this</code> for easy method chaining 621 */ 622 public Media setBasedOn(List<Reference> theBasedOn) { 623 this.basedOn = theBasedOn; 624 return this; 625 } 626 627 public boolean hasBasedOn() { 628 if (this.basedOn == null) 629 return false; 630 for (Reference item : this.basedOn) 631 if (!item.isEmpty()) 632 return true; 633 return false; 634 } 635 636 public Reference addBasedOn() { // 3 637 Reference t = new Reference(); 638 if (this.basedOn == null) 639 this.basedOn = new ArrayList<Reference>(); 640 this.basedOn.add(t); 641 return t; 642 } 643 644 public Media addBasedOn(Reference t) { // 3 645 if (t == null) 646 return this; 647 if (this.basedOn == null) 648 this.basedOn = new ArrayList<Reference>(); 649 this.basedOn.add(t); 650 return this; 651 } 652 653 /** 654 * @return The first repetition of repeating field {@link #basedOn}, creating it 655 * if it does not already exist 656 */ 657 public Reference getBasedOnFirstRep() { 658 if (getBasedOn().isEmpty()) { 659 addBasedOn(); 660 } 661 return getBasedOn().get(0); 662 } 663 664 /** 665 * @return {@link #partOf} (A larger event of which this particular event is a 666 * component or step.) 667 */ 668 public List<Reference> getPartOf() { 669 if (this.partOf == null) 670 this.partOf = new ArrayList<Reference>(); 671 return this.partOf; 672 } 673 674 /** 675 * @return Returns a reference to <code>this</code> for easy method chaining 676 */ 677 public Media setPartOf(List<Reference> thePartOf) { 678 this.partOf = thePartOf; 679 return this; 680 } 681 682 public boolean hasPartOf() { 683 if (this.partOf == null) 684 return false; 685 for (Reference item : this.partOf) 686 if (!item.isEmpty()) 687 return true; 688 return false; 689 } 690 691 public Reference addPartOf() { // 3 692 Reference t = new Reference(); 693 if (this.partOf == null) 694 this.partOf = new ArrayList<Reference>(); 695 this.partOf.add(t); 696 return t; 697 } 698 699 public Media addPartOf(Reference t) { // 3 700 if (t == null) 701 return this; 702 if (this.partOf == null) 703 this.partOf = new ArrayList<Reference>(); 704 this.partOf.add(t); 705 return this; 706 } 707 708 /** 709 * @return The first repetition of repeating field {@link #partOf}, creating it 710 * if it does not already exist 711 */ 712 public Reference getPartOfFirstRep() { 713 if (getPartOf().isEmpty()) { 714 addPartOf(); 715 } 716 return getPartOf().get(0); 717 } 718 719 /** 720 * @return {@link #status} (The current state of the {{title}}.). This is the 721 * underlying object with id, value and extensions. The accessor 722 * "getStatus" gives direct access to the value 723 */ 724 public Enumeration<MediaStatus> getStatusElement() { 725 if (this.status == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create Media.status"); 728 else if (Configuration.doAutoCreate()) 729 this.status = new Enumeration<MediaStatus>(new MediaStatusEnumFactory()); // bb 730 return this.status; 731 } 732 733 public boolean hasStatusElement() { 734 return this.status != null && !this.status.isEmpty(); 735 } 736 737 public boolean hasStatus() { 738 return this.status != null && !this.status.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #status} (The current state of the {{title}}.). This is 743 * the underlying object with id, value and extensions. The 744 * accessor "getStatus" gives direct access to the value 745 */ 746 public Media setStatusElement(Enumeration<MediaStatus> value) { 747 this.status = value; 748 return this; 749 } 750 751 /** 752 * @return The current state of the {{title}}. 753 */ 754 public MediaStatus getStatus() { 755 return this.status == null ? null : this.status.getValue(); 756 } 757 758 /** 759 * @param value The current state of the {{title}}. 760 */ 761 public Media setStatus(MediaStatus value) { 762 if (this.status == null) 763 this.status = new Enumeration<MediaStatus>(new MediaStatusEnumFactory()); 764 this.status.setValue(value); 765 return this; 766 } 767 768 /** 769 * @return {@link #type} (A code that classifies whether the media is an image, 770 * video or audio recording or some other media category.) 771 */ 772 public CodeableConcept getType() { 773 if (this.type == null) 774 if (Configuration.errorOnAutoCreate()) 775 throw new Error("Attempt to auto-create Media.type"); 776 else if (Configuration.doAutoCreate()) 777 this.type = new CodeableConcept(); // cc 778 return this.type; 779 } 780 781 public boolean hasType() { 782 return this.type != null && !this.type.isEmpty(); 783 } 784 785 /** 786 * @param value {@link #type} (A code that classifies whether the media is an 787 * image, video or audio recording or some other media category.) 788 */ 789 public Media setType(CodeableConcept value) { 790 this.type = value; 791 return this; 792 } 793 794 /** 795 * @return {@link #modality} (Details of the type of the media - usually, how it 796 * was acquired (what type of device). If images sourced from a DICOM 797 * system, are wrapped in a Media resource, then this is the modality.) 798 */ 799 public CodeableConcept getModality() { 800 if (this.modality == null) 801 if (Configuration.errorOnAutoCreate()) 802 throw new Error("Attempt to auto-create Media.modality"); 803 else if (Configuration.doAutoCreate()) 804 this.modality = new CodeableConcept(); // cc 805 return this.modality; 806 } 807 808 public boolean hasModality() { 809 return this.modality != null && !this.modality.isEmpty(); 810 } 811 812 /** 813 * @param value {@link #modality} (Details of the type of the media - usually, 814 * how it was acquired (what type of device). If images sourced 815 * from a DICOM system, are wrapped in a Media resource, then this 816 * is the modality.) 817 */ 818 public Media setModality(CodeableConcept value) { 819 this.modality = value; 820 return this; 821 } 822 823 /** 824 * @return {@link #view} (The name of the imaging view e.g. Lateral or 825 * Antero-posterior (AP).) 826 */ 827 public CodeableConcept getView() { 828 if (this.view == null) 829 if (Configuration.errorOnAutoCreate()) 830 throw new Error("Attempt to auto-create Media.view"); 831 else if (Configuration.doAutoCreate()) 832 this.view = new CodeableConcept(); // cc 833 return this.view; 834 } 835 836 public boolean hasView() { 837 return this.view != null && !this.view.isEmpty(); 838 } 839 840 /** 841 * @param value {@link #view} (The name of the imaging view e.g. Lateral or 842 * Antero-posterior (AP).) 843 */ 844 public Media setView(CodeableConcept value) { 845 this.view = value; 846 return this; 847 } 848 849 /** 850 * @return {@link #subject} (Who/What this Media is a record of.) 851 */ 852 public Reference getSubject() { 853 if (this.subject == null) 854 if (Configuration.errorOnAutoCreate()) 855 throw new Error("Attempt to auto-create Media.subject"); 856 else if (Configuration.doAutoCreate()) 857 this.subject = new Reference(); // cc 858 return this.subject; 859 } 860 861 public boolean hasSubject() { 862 return this.subject != null && !this.subject.isEmpty(); 863 } 864 865 /** 866 * @param value {@link #subject} (Who/What this Media is a record of.) 867 */ 868 public Media setSubject(Reference value) { 869 this.subject = value; 870 return this; 871 } 872 873 /** 874 * @return {@link #subject} The actual object that is the target of the 875 * reference. The reference library doesn't populate this, but you can 876 * use it to hold the resource if you resolve it. (Who/What this Media 877 * is a record of.) 878 */ 879 public Resource getSubjectTarget() { 880 return this.subjectTarget; 881 } 882 883 /** 884 * @param value {@link #subject} The actual object that is the target of the 885 * reference. The reference library doesn't use these, but you can 886 * use it to hold the resource if you resolve it. (Who/What this 887 * Media is a record of.) 888 */ 889 public Media setSubjectTarget(Resource value) { 890 this.subjectTarget = value; 891 return this; 892 } 893 894 /** 895 * @return {@link #encounter} (The encounter that establishes the context for 896 * this media.) 897 */ 898 public Reference getEncounter() { 899 if (this.encounter == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create Media.encounter"); 902 else if (Configuration.doAutoCreate()) 903 this.encounter = new Reference(); // cc 904 return this.encounter; 905 } 906 907 public boolean hasEncounter() { 908 return this.encounter != null && !this.encounter.isEmpty(); 909 } 910 911 /** 912 * @param value {@link #encounter} (The encounter that establishes the context 913 * for this media.) 914 */ 915 public Media setEncounter(Reference value) { 916 this.encounter = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #encounter} The actual object that is the target of the 922 * reference. The reference library doesn't populate this, but you can 923 * use it to hold the resource if you resolve it. (The encounter that 924 * establishes the context for this media.) 925 */ 926 public Encounter getEncounterTarget() { 927 if (this.encounterTarget == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create Media.encounter"); 930 else if (Configuration.doAutoCreate()) 931 this.encounterTarget = new Encounter(); // aa 932 return this.encounterTarget; 933 } 934 935 /** 936 * @param value {@link #encounter} The actual object that is the target of the 937 * reference. The reference library doesn't use these, but you can 938 * use it to hold the resource if you resolve it. (The encounter 939 * that establishes the context for this media.) 940 */ 941 public Media setEncounterTarget(Encounter value) { 942 this.encounterTarget = value; 943 return this; 944 } 945 946 /** 947 * @return {@link #created} (The date and time(s) at which the media was 948 * collected.) 949 */ 950 public Type getCreated() { 951 return this.created; 952 } 953 954 /** 955 * @return {@link #created} (The date and time(s) at which the media was 956 * collected.) 957 */ 958 public DateTimeType getCreatedDateTimeType() throws FHIRException { 959 if (this.created == null) 960 this.created = new DateTimeType(); 961 if (!(this.created instanceof DateTimeType)) 962 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 963 + this.created.getClass().getName() + " was encountered"); 964 return (DateTimeType) this.created; 965 } 966 967 public boolean hasCreatedDateTimeType() { 968 return this.created instanceof DateTimeType; 969 } 970 971 /** 972 * @return {@link #created} (The date and time(s) at which the media was 973 * collected.) 974 */ 975 public Period getCreatedPeriod() throws FHIRException { 976 if (this.created == null) 977 this.created = new Period(); 978 if (!(this.created instanceof Period)) 979 throw new FHIRException( 980 "Type mismatch: the type Period was expected, but " + this.created.getClass().getName() + " was encountered"); 981 return (Period) this.created; 982 } 983 984 public boolean hasCreatedPeriod() { 985 return this.created instanceof Period; 986 } 987 988 public boolean hasCreated() { 989 return this.created != null && !this.created.isEmpty(); 990 } 991 992 /** 993 * @param value {@link #created} (The date and time(s) at which the media was 994 * collected.) 995 */ 996 public Media setCreated(Type value) { 997 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 998 throw new Error("Not the right type for Media.created[x]: " + value.fhirType()); 999 this.created = value; 1000 return this; 1001 } 1002 1003 /** 1004 * @return {@link #issued} (The date and time this version of the media was made 1005 * available to providers, typically after having been reviewed.). This 1006 * is the underlying object with id, value and extensions. The accessor 1007 * "getIssued" gives direct access to the value 1008 */ 1009 public InstantType getIssuedElement() { 1010 if (this.issued == null) 1011 if (Configuration.errorOnAutoCreate()) 1012 throw new Error("Attempt to auto-create Media.issued"); 1013 else if (Configuration.doAutoCreate()) 1014 this.issued = new InstantType(); // bb 1015 return this.issued; 1016 } 1017 1018 public boolean hasIssuedElement() { 1019 return this.issued != null && !this.issued.isEmpty(); 1020 } 1021 1022 public boolean hasIssued() { 1023 return this.issued != null && !this.issued.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #issued} (The date and time this version of the media was 1028 * made available to providers, typically after having been 1029 * reviewed.). This is the underlying object with id, value and 1030 * extensions. The accessor "getIssued" gives direct access to the 1031 * value 1032 */ 1033 public Media setIssuedElement(InstantType value) { 1034 this.issued = value; 1035 return this; 1036 } 1037 1038 /** 1039 * @return The date and time this version of the media was made available to 1040 * providers, typically after having been reviewed. 1041 */ 1042 public Date getIssued() { 1043 return this.issued == null ? null : this.issued.getValue(); 1044 } 1045 1046 /** 1047 * @param value The date and time this version of the media was made available 1048 * to providers, typically after having been reviewed. 1049 */ 1050 public Media setIssued(Date value) { 1051 if (value == null) 1052 this.issued = null; 1053 else { 1054 if (this.issued == null) 1055 this.issued = new InstantType(); 1056 this.issued.setValue(value); 1057 } 1058 return this; 1059 } 1060 1061 /** 1062 * @return {@link #operator} (The person who administered the collection of the 1063 * image.) 1064 */ 1065 public Reference getOperator() { 1066 if (this.operator == null) 1067 if (Configuration.errorOnAutoCreate()) 1068 throw new Error("Attempt to auto-create Media.operator"); 1069 else if (Configuration.doAutoCreate()) 1070 this.operator = new Reference(); // cc 1071 return this.operator; 1072 } 1073 1074 public boolean hasOperator() { 1075 return this.operator != null && !this.operator.isEmpty(); 1076 } 1077 1078 /** 1079 * @param value {@link #operator} (The person who administered the collection of 1080 * the image.) 1081 */ 1082 public Media setOperator(Reference value) { 1083 this.operator = value; 1084 return this; 1085 } 1086 1087 /** 1088 * @return {@link #operator} The actual object that is the target of the 1089 * reference. The reference library doesn't populate this, but you can 1090 * use it to hold the resource if you resolve it. (The person who 1091 * administered the collection of the image.) 1092 */ 1093 public Resource getOperatorTarget() { 1094 return this.operatorTarget; 1095 } 1096 1097 /** 1098 * @param value {@link #operator} The actual object that is the target of the 1099 * reference. The reference library doesn't use these, but you can 1100 * use it to hold the resource if you resolve it. (The person who 1101 * administered the collection of the image.) 1102 */ 1103 public Media setOperatorTarget(Resource value) { 1104 this.operatorTarget = value; 1105 return this; 1106 } 1107 1108 /** 1109 * @return {@link #reasonCode} (Describes why the event occurred in coded or 1110 * textual form.) 1111 */ 1112 public List<CodeableConcept> getReasonCode() { 1113 if (this.reasonCode == null) 1114 this.reasonCode = new ArrayList<CodeableConcept>(); 1115 return this.reasonCode; 1116 } 1117 1118 /** 1119 * @return Returns a reference to <code>this</code> for easy method chaining 1120 */ 1121 public Media setReasonCode(List<CodeableConcept> theReasonCode) { 1122 this.reasonCode = theReasonCode; 1123 return this; 1124 } 1125 1126 public boolean hasReasonCode() { 1127 if (this.reasonCode == null) 1128 return false; 1129 for (CodeableConcept item : this.reasonCode) 1130 if (!item.isEmpty()) 1131 return true; 1132 return false; 1133 } 1134 1135 public CodeableConcept addReasonCode() { // 3 1136 CodeableConcept t = new CodeableConcept(); 1137 if (this.reasonCode == null) 1138 this.reasonCode = new ArrayList<CodeableConcept>(); 1139 this.reasonCode.add(t); 1140 return t; 1141 } 1142 1143 public Media addReasonCode(CodeableConcept t) { // 3 1144 if (t == null) 1145 return this; 1146 if (this.reasonCode == null) 1147 this.reasonCode = new ArrayList<CodeableConcept>(); 1148 this.reasonCode.add(t); 1149 return this; 1150 } 1151 1152 /** 1153 * @return The first repetition of repeating field {@link #reasonCode}, creating 1154 * it if it does not already exist 1155 */ 1156 public CodeableConcept getReasonCodeFirstRep() { 1157 if (getReasonCode().isEmpty()) { 1158 addReasonCode(); 1159 } 1160 return getReasonCode().get(0); 1161 } 1162 1163 /** 1164 * @return {@link #bodySite} (Indicates the site on the subject's body where the 1165 * observation was made (i.e. the target site).) 1166 */ 1167 public CodeableConcept getBodySite() { 1168 if (this.bodySite == null) 1169 if (Configuration.errorOnAutoCreate()) 1170 throw new Error("Attempt to auto-create Media.bodySite"); 1171 else if (Configuration.doAutoCreate()) 1172 this.bodySite = new CodeableConcept(); // cc 1173 return this.bodySite; 1174 } 1175 1176 public boolean hasBodySite() { 1177 return this.bodySite != null && !this.bodySite.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #bodySite} (Indicates the site on the subject's body 1182 * where the observation was made (i.e. the target site).) 1183 */ 1184 public Media setBodySite(CodeableConcept value) { 1185 this.bodySite = value; 1186 return this; 1187 } 1188 1189 /** 1190 * @return {@link #deviceName} (The name of the device / manufacturer of the 1191 * device that was used to make the recording.). This is the underlying 1192 * object with id, value and extensions. The accessor "getDeviceName" 1193 * gives direct access to the value 1194 */ 1195 public StringType getDeviceNameElement() { 1196 if (this.deviceName == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create Media.deviceName"); 1199 else if (Configuration.doAutoCreate()) 1200 this.deviceName = new StringType(); // bb 1201 return this.deviceName; 1202 } 1203 1204 public boolean hasDeviceNameElement() { 1205 return this.deviceName != null && !this.deviceName.isEmpty(); 1206 } 1207 1208 public boolean hasDeviceName() { 1209 return this.deviceName != null && !this.deviceName.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #deviceName} (The name of the device / manufacturer of 1214 * the device that was used to make the recording.). This is the 1215 * underlying object with id, value and extensions. The accessor 1216 * "getDeviceName" gives direct access to the value 1217 */ 1218 public Media setDeviceNameElement(StringType value) { 1219 this.deviceName = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return The name of the device / manufacturer of the device that was used to 1225 * make the recording. 1226 */ 1227 public String getDeviceName() { 1228 return this.deviceName == null ? null : this.deviceName.getValue(); 1229 } 1230 1231 /** 1232 * @param value The name of the device / manufacturer of the device that was 1233 * used to make the recording. 1234 */ 1235 public Media setDeviceName(String value) { 1236 if (Utilities.noString(value)) 1237 this.deviceName = null; 1238 else { 1239 if (this.deviceName == null) 1240 this.deviceName = new StringType(); 1241 this.deviceName.setValue(value); 1242 } 1243 return this; 1244 } 1245 1246 /** 1247 * @return {@link #device} (The device used to collect the media.) 1248 */ 1249 public Reference getDevice() { 1250 if (this.device == null) 1251 if (Configuration.errorOnAutoCreate()) 1252 throw new Error("Attempt to auto-create Media.device"); 1253 else if (Configuration.doAutoCreate()) 1254 this.device = new Reference(); // cc 1255 return this.device; 1256 } 1257 1258 public boolean hasDevice() { 1259 return this.device != null && !this.device.isEmpty(); 1260 } 1261 1262 /** 1263 * @param value {@link #device} (The device used to collect the media.) 1264 */ 1265 public Media setDevice(Reference value) { 1266 this.device = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return {@link #device} The actual object that is the target of the 1272 * reference. The reference library doesn't populate this, but you can 1273 * use it to hold the resource if you resolve it. (The device used to 1274 * collect the media.) 1275 */ 1276 public Resource getDeviceTarget() { 1277 return this.deviceTarget; 1278 } 1279 1280 /** 1281 * @param value {@link #device} The actual object that is the target of the 1282 * reference. The reference library doesn't use these, but you can 1283 * use it to hold the resource if you resolve it. (The device used 1284 * to collect the media.) 1285 */ 1286 public Media setDeviceTarget(Resource value) { 1287 this.deviceTarget = value; 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #height} (Height of the image in pixels (photo/video).). This 1293 * is the underlying object with id, value and extensions. The accessor 1294 * "getHeight" gives direct access to the value 1295 */ 1296 public PositiveIntType getHeightElement() { 1297 if (this.height == null) 1298 if (Configuration.errorOnAutoCreate()) 1299 throw new Error("Attempt to auto-create Media.height"); 1300 else if (Configuration.doAutoCreate()) 1301 this.height = new PositiveIntType(); // bb 1302 return this.height; 1303 } 1304 1305 public boolean hasHeightElement() { 1306 return this.height != null && !this.height.isEmpty(); 1307 } 1308 1309 public boolean hasHeight() { 1310 return this.height != null && !this.height.isEmpty(); 1311 } 1312 1313 /** 1314 * @param value {@link #height} (Height of the image in pixels (photo/video).). 1315 * This is the underlying object with id, value and extensions. The 1316 * accessor "getHeight" gives direct access to the value 1317 */ 1318 public Media setHeightElement(PositiveIntType value) { 1319 this.height = value; 1320 return this; 1321 } 1322 1323 /** 1324 * @return Height of the image in pixels (photo/video). 1325 */ 1326 public int getHeight() { 1327 return this.height == null || this.height.isEmpty() ? 0 : this.height.getValue(); 1328 } 1329 1330 /** 1331 * @param value Height of the image in pixels (photo/video). 1332 */ 1333 public Media setHeight(int value) { 1334 if (this.height == null) 1335 this.height = new PositiveIntType(); 1336 this.height.setValue(value); 1337 return this; 1338 } 1339 1340 /** 1341 * @return {@link #width} (Width of the image in pixels (photo/video).). This is 1342 * the underlying object with id, value and extensions. The accessor 1343 * "getWidth" gives direct access to the value 1344 */ 1345 public PositiveIntType getWidthElement() { 1346 if (this.width == null) 1347 if (Configuration.errorOnAutoCreate()) 1348 throw new Error("Attempt to auto-create Media.width"); 1349 else if (Configuration.doAutoCreate()) 1350 this.width = new PositiveIntType(); // bb 1351 return this.width; 1352 } 1353 1354 public boolean hasWidthElement() { 1355 return this.width != null && !this.width.isEmpty(); 1356 } 1357 1358 public boolean hasWidth() { 1359 return this.width != null && !this.width.isEmpty(); 1360 } 1361 1362 /** 1363 * @param value {@link #width} (Width of the image in pixels (photo/video).). 1364 * This is the underlying object with id, value and extensions. The 1365 * accessor "getWidth" gives direct access to the value 1366 */ 1367 public Media setWidthElement(PositiveIntType value) { 1368 this.width = value; 1369 return this; 1370 } 1371 1372 /** 1373 * @return Width of the image in pixels (photo/video). 1374 */ 1375 public int getWidth() { 1376 return this.width == null || this.width.isEmpty() ? 0 : this.width.getValue(); 1377 } 1378 1379 /** 1380 * @param value Width of the image in pixels (photo/video). 1381 */ 1382 public Media setWidth(int value) { 1383 if (this.width == null) 1384 this.width = new PositiveIntType(); 1385 this.width.setValue(value); 1386 return this; 1387 } 1388 1389 /** 1390 * @return {@link #frames} (The number of frames in a photo. This is used with a 1391 * multi-page fax, or an imaging acquisition context that takes multiple 1392 * slices in a single image, or an animated gif. If there is more than 1393 * one frame, this SHALL have a value in order to alert interface 1394 * software that a multi-frame capable rendering widget is required.). 1395 * This is the underlying object with id, value and extensions. The 1396 * accessor "getFrames" gives direct access to the value 1397 */ 1398 public PositiveIntType getFramesElement() { 1399 if (this.frames == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create Media.frames"); 1402 else if (Configuration.doAutoCreate()) 1403 this.frames = new PositiveIntType(); // bb 1404 return this.frames; 1405 } 1406 1407 public boolean hasFramesElement() { 1408 return this.frames != null && !this.frames.isEmpty(); 1409 } 1410 1411 public boolean hasFrames() { 1412 return this.frames != null && !this.frames.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #frames} (The number of frames in a photo. This is used 1417 * with a multi-page fax, or an imaging acquisition context that 1418 * takes multiple slices in a single image, or an animated gif. If 1419 * there is more than one frame, this SHALL have a value in order 1420 * to alert interface software that a multi-frame capable rendering 1421 * widget is required.). This is the underlying object with id, 1422 * value and extensions. The accessor "getFrames" gives direct 1423 * access to the value 1424 */ 1425 public Media setFramesElement(PositiveIntType value) { 1426 this.frames = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return The number of frames in a photo. This is used with a multi-page fax, 1432 * or an imaging acquisition context that takes multiple slices in a 1433 * single image, or an animated gif. If there is more than one frame, 1434 * this SHALL have a value in order to alert interface software that a 1435 * multi-frame capable rendering widget is required. 1436 */ 1437 public int getFrames() { 1438 return this.frames == null || this.frames.isEmpty() ? 0 : this.frames.getValue(); 1439 } 1440 1441 /** 1442 * @param value The number of frames in a photo. This is used with a multi-page 1443 * fax, or an imaging acquisition context that takes multiple 1444 * slices in a single image, or an animated gif. If there is more 1445 * than one frame, this SHALL have a value in order to alert 1446 * interface software that a multi-frame capable rendering widget 1447 * is required. 1448 */ 1449 public Media setFrames(int value) { 1450 if (this.frames == null) 1451 this.frames = new PositiveIntType(); 1452 this.frames.setValue(value); 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #duration} (The duration of the recording in seconds - for 1458 * audio and video.). This is the underlying object with id, value and 1459 * extensions. The accessor "getDuration" gives direct access to the 1460 * value 1461 */ 1462 public DecimalType getDurationElement() { 1463 if (this.duration == null) 1464 if (Configuration.errorOnAutoCreate()) 1465 throw new Error("Attempt to auto-create Media.duration"); 1466 else if (Configuration.doAutoCreate()) 1467 this.duration = new DecimalType(); // bb 1468 return this.duration; 1469 } 1470 1471 public boolean hasDurationElement() { 1472 return this.duration != null && !this.duration.isEmpty(); 1473 } 1474 1475 public boolean hasDuration() { 1476 return this.duration != null && !this.duration.isEmpty(); 1477 } 1478 1479 /** 1480 * @param value {@link #duration} (The duration of the recording in seconds - 1481 * for audio and video.). This is the underlying object with id, 1482 * value and extensions. The accessor "getDuration" gives direct 1483 * access to the value 1484 */ 1485 public Media setDurationElement(DecimalType value) { 1486 this.duration = value; 1487 return this; 1488 } 1489 1490 /** 1491 * @return The duration of the recording in seconds - for audio and video. 1492 */ 1493 public BigDecimal getDuration() { 1494 return this.duration == null ? null : this.duration.getValue(); 1495 } 1496 1497 /** 1498 * @param value The duration of the recording in seconds - for audio and video. 1499 */ 1500 public Media setDuration(BigDecimal value) { 1501 if (value == null) 1502 this.duration = null; 1503 else { 1504 if (this.duration == null) 1505 this.duration = new DecimalType(); 1506 this.duration.setValue(value); 1507 } 1508 return this; 1509 } 1510 1511 /** 1512 * @param value The duration of the recording in seconds - for audio and video. 1513 */ 1514 public Media setDuration(long value) { 1515 this.duration = new DecimalType(); 1516 this.duration.setValue(value); 1517 return this; 1518 } 1519 1520 /** 1521 * @param value The duration of the recording in seconds - for audio and video. 1522 */ 1523 public Media setDuration(double value) { 1524 this.duration = new DecimalType(); 1525 this.duration.setValue(value); 1526 return this; 1527 } 1528 1529 /** 1530 * @return {@link #content} (The actual content of the media - inline or by 1531 * direct reference to the media source file.) 1532 */ 1533 public Attachment getContent() { 1534 if (this.content == null) 1535 if (Configuration.errorOnAutoCreate()) 1536 throw new Error("Attempt to auto-create Media.content"); 1537 else if (Configuration.doAutoCreate()) 1538 this.content = new Attachment(); // cc 1539 return this.content; 1540 } 1541 1542 public boolean hasContent() { 1543 return this.content != null && !this.content.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #content} (The actual content of the media - inline or by 1548 * direct reference to the media source file.) 1549 */ 1550 public Media setContent(Attachment value) { 1551 this.content = value; 1552 return this; 1553 } 1554 1555 /** 1556 * @return {@link #note} (Comments made about the media by the performer, 1557 * subject or other participants.) 1558 */ 1559 public List<Annotation> getNote() { 1560 if (this.note == null) 1561 this.note = new ArrayList<Annotation>(); 1562 return this.note; 1563 } 1564 1565 /** 1566 * @return Returns a reference to <code>this</code> for easy method chaining 1567 */ 1568 public Media setNote(List<Annotation> theNote) { 1569 this.note = theNote; 1570 return this; 1571 } 1572 1573 public boolean hasNote() { 1574 if (this.note == null) 1575 return false; 1576 for (Annotation item : this.note) 1577 if (!item.isEmpty()) 1578 return true; 1579 return false; 1580 } 1581 1582 public Annotation addNote() { // 3 1583 Annotation t = new Annotation(); 1584 if (this.note == null) 1585 this.note = new ArrayList<Annotation>(); 1586 this.note.add(t); 1587 return t; 1588 } 1589 1590 public Media addNote(Annotation t) { // 3 1591 if (t == null) 1592 return this; 1593 if (this.note == null) 1594 this.note = new ArrayList<Annotation>(); 1595 this.note.add(t); 1596 return this; 1597 } 1598 1599 /** 1600 * @return The first repetition of repeating field {@link #note}, creating it if 1601 * it does not already exist 1602 */ 1603 public Annotation getNoteFirstRep() { 1604 if (getNote().isEmpty()) { 1605 addNote(); 1606 } 1607 return getNote().get(0); 1608 } 1609 1610 protected void listChildren(List<Property> children) { 1611 super.listChildren(children); 1612 children.add(new Property("identifier", "Identifier", 1613 "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 1614 0, java.lang.Integer.MAX_VALUE, identifier)); 1615 children.add(new Property("basedOn", "Reference(ServiceRequest|CarePlan)", 1616 "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, 1617 java.lang.Integer.MAX_VALUE, basedOn)); 1618 children.add(new Property("partOf", "Reference(Any)", 1619 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1620 partOf)); 1621 children.add(new Property("status", "code", "The current state of the {{title}}.", 0, 1, status)); 1622 children.add(new Property("type", "CodeableConcept", 1623 "A code that classifies whether the media is an image, video or audio recording or some other media category.", 1624 0, 1, type)); 1625 children.add(new Property("modality", "CodeableConcept", 1626 "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 1627 0, 1, modality)); 1628 children.add(new Property("view", "CodeableConcept", 1629 "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, 1, view)); 1630 children 1631 .add(new Property("subject", "Reference(Patient|Practitioner|PractitionerRole|Group|Device|Specimen|Location)", 1632 "Who/What this Media is a record of.", 0, 1, subject)); 1633 children.add(new Property("encounter", "Reference(Encounter)", 1634 "The encounter that establishes the context for this media.", 0, 1, encounter)); 1635 children.add(new Property("created[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 1636 0, 1, created)); 1637 children.add(new Property("issued", "instant", 1638 "The date and time this version of the media was made available to providers, typically after having been reviewed.", 1639 0, 1, issued)); 1640 children.add(new Property("operator", 1641 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 1642 "The person who administered the collection of the image.", 0, 1, operator)); 1643 children.add(new Property("reasonCode", "CodeableConcept", 1644 "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1645 children.add(new Property("bodySite", "CodeableConcept", 1646 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 1647 bodySite)); 1648 children.add(new Property("deviceName", "string", 1649 "The name of the device / manufacturer of the device that was used to make the recording.", 0, 1, deviceName)); 1650 children.add(new Property("device", "Reference(Device|DeviceMetric|Device)", 1651 "The device used to collect the media.", 0, 1, device)); 1652 children.add(new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, height)); 1653 children.add(new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, width)); 1654 children.add(new Property("frames", "positiveInt", 1655 "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 1656 0, 1, frames)); 1657 children.add(new Property("duration", "decimal", "The duration of the recording in seconds - for audio and video.", 1658 0, 1, duration)); 1659 children.add(new Property("content", "Attachment", 1660 "The actual content of the media - inline or by direct reference to the media source file.", 0, 1, content)); 1661 children.add(new Property("note", "Annotation", 1662 "Comments made about the media by the performer, subject or other participants.", 0, 1663 java.lang.Integer.MAX_VALUE, note)); 1664 } 1665 1666 @Override 1667 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1668 switch (_hash) { 1669 case -1618432855: 1670 /* identifier */ return new Property("identifier", "Identifier", 1671 "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 1672 0, java.lang.Integer.MAX_VALUE, identifier); 1673 case -332612366: 1674 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest|CarePlan)", 1675 "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, 1676 java.lang.Integer.MAX_VALUE, basedOn); 1677 case -995410646: 1678 /* partOf */ return new Property("partOf", "Reference(Any)", 1679 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1680 partOf); 1681 case -892481550: 1682 /* status */ return new Property("status", "code", "The current state of the {{title}}.", 0, 1, status); 1683 case 3575610: 1684 /* type */ return new Property("type", "CodeableConcept", 1685 "A code that classifies whether the media is an image, video or audio recording or some other media category.", 1686 0, 1, type); 1687 case -622722335: 1688 /* modality */ return new Property("modality", "CodeableConcept", 1689 "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 1690 0, 1, modality); 1691 case 3619493: 1692 /* view */ return new Property("view", "CodeableConcept", 1693 "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, 1, view); 1694 case -1867885268: 1695 /* subject */ return new Property("subject", 1696 "Reference(Patient|Practitioner|PractitionerRole|Group|Device|Specimen|Location)", 1697 "Who/What this Media is a record of.", 0, 1, subject); 1698 case 1524132147: 1699 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1700 "The encounter that establishes the context for this media.", 0, 1, encounter); 1701 case 1369676952: 1702 /* created[x] */ return new Property("created[x]", "dateTime|Period", 1703 "The date and time(s) at which the media was collected.", 0, 1, created); 1704 case 1028554472: 1705 /* created */ return new Property("created[x]", "dateTime|Period", 1706 "The date and time(s) at which the media was collected.", 0, 1, created); 1707 case -1968526685: 1708 /* createdDateTime */ return new Property("created[x]", "dateTime|Period", 1709 "The date and time(s) at which the media was collected.", 0, 1, created); 1710 case 1525027529: 1711 /* createdPeriod */ return new Property("created[x]", "dateTime|Period", 1712 "The date and time(s) at which the media was collected.", 0, 1, created); 1713 case -1179159893: 1714 /* issued */ return new Property("issued", "instant", 1715 "The date and time this version of the media was made available to providers, typically after having been reviewed.", 1716 0, 1, issued); 1717 case -500553564: 1718 /* operator */ return new Property("operator", 1719 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 1720 "The person who administered the collection of the image.", 0, 1, operator); 1721 case 722137681: 1722 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1723 "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1724 case 1702620169: 1725 /* bodySite */ return new Property("bodySite", "CodeableConcept", 1726 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 1727 bodySite); 1728 case 780988929: 1729 /* deviceName */ return new Property("deviceName", "string", 1730 "The name of the device / manufacturer of the device that was used to make the recording.", 0, 1, 1731 deviceName); 1732 case -1335157162: 1733 /* device */ return new Property("device", "Reference(Device|DeviceMetric|Device)", 1734 "The device used to collect the media.", 0, 1, device); 1735 case -1221029593: 1736 /* height */ return new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, 1737 height); 1738 case 113126854: 1739 /* width */ return new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, 1740 width); 1741 case -1266514778: 1742 /* frames */ return new Property("frames", "positiveInt", 1743 "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 1744 0, 1, frames); 1745 case -1992012396: 1746 /* duration */ return new Property("duration", "decimal", 1747 "The duration of the recording in seconds - for audio and video.", 0, 1, duration); 1748 case 951530617: 1749 /* content */ return new Property("content", "Attachment", 1750 "The actual content of the media - inline or by direct reference to the media source file.", 0, 1, content); 1751 case 3387378: 1752 /* note */ return new Property("note", "Annotation", 1753 "Comments made about the media by the performer, subject or other participants.", 0, 1754 java.lang.Integer.MAX_VALUE, note); 1755 default: 1756 return super.getNamedProperty(_hash, _name, _checkValid); 1757 } 1758 1759 } 1760 1761 @Override 1762 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1763 switch (hash) { 1764 case -1618432855: 1765 /* identifier */ return this.identifier == null ? new Base[0] 1766 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1767 case -332612366: 1768 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1769 case -995410646: 1770 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1771 case -892481550: 1772 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MediaStatus> 1773 case 3575610: 1774 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1775 case -622722335: 1776 /* modality */ return this.modality == null ? new Base[0] : new Base[] { this.modality }; // CodeableConcept 1777 case 3619493: 1778 /* view */ return this.view == null ? new Base[0] : new Base[] { this.view }; // CodeableConcept 1779 case -1867885268: 1780 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1781 case 1524132147: 1782 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1783 case 1028554472: 1784 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // Type 1785 case -1179159893: 1786 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // InstantType 1787 case -500553564: 1788 /* operator */ return this.operator == null ? new Base[0] : new Base[] { this.operator }; // Reference 1789 case 722137681: 1790 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1791 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1792 case 1702620169: 1793 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 1794 case 780988929: 1795 /* deviceName */ return this.deviceName == null ? new Base[0] : new Base[] { this.deviceName }; // StringType 1796 case -1335157162: 1797 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 1798 case -1221029593: 1799 /* height */ return this.height == null ? new Base[0] : new Base[] { this.height }; // PositiveIntType 1800 case 113126854: 1801 /* width */ return this.width == null ? new Base[0] : new Base[] { this.width }; // PositiveIntType 1802 case -1266514778: 1803 /* frames */ return this.frames == null ? new Base[0] : new Base[] { this.frames }; // PositiveIntType 1804 case -1992012396: 1805 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // DecimalType 1806 case 951530617: 1807 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Attachment 1808 case 3387378: 1809 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1810 default: 1811 return super.getProperty(hash, name, checkValid); 1812 } 1813 1814 } 1815 1816 @Override 1817 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1818 switch (hash) { 1819 case -1618432855: // identifier 1820 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1821 return value; 1822 case -332612366: // basedOn 1823 this.getBasedOn().add(castToReference(value)); // Reference 1824 return value; 1825 case -995410646: // partOf 1826 this.getPartOf().add(castToReference(value)); // Reference 1827 return value; 1828 case -892481550: // status 1829 value = new MediaStatusEnumFactory().fromType(castToCode(value)); 1830 this.status = (Enumeration) value; // Enumeration<MediaStatus> 1831 return value; 1832 case 3575610: // type 1833 this.type = castToCodeableConcept(value); // CodeableConcept 1834 return value; 1835 case -622722335: // modality 1836 this.modality = castToCodeableConcept(value); // CodeableConcept 1837 return value; 1838 case 3619493: // view 1839 this.view = castToCodeableConcept(value); // CodeableConcept 1840 return value; 1841 case -1867885268: // subject 1842 this.subject = castToReference(value); // Reference 1843 return value; 1844 case 1524132147: // encounter 1845 this.encounter = castToReference(value); // Reference 1846 return value; 1847 case 1028554472: // created 1848 this.created = castToType(value); // Type 1849 return value; 1850 case -1179159893: // issued 1851 this.issued = castToInstant(value); // InstantType 1852 return value; 1853 case -500553564: // operator 1854 this.operator = castToReference(value); // Reference 1855 return value; 1856 case 722137681: // reasonCode 1857 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1858 return value; 1859 case 1702620169: // bodySite 1860 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1861 return value; 1862 case 780988929: // deviceName 1863 this.deviceName = castToString(value); // StringType 1864 return value; 1865 case -1335157162: // device 1866 this.device = castToReference(value); // Reference 1867 return value; 1868 case -1221029593: // height 1869 this.height = castToPositiveInt(value); // PositiveIntType 1870 return value; 1871 case 113126854: // width 1872 this.width = castToPositiveInt(value); // PositiveIntType 1873 return value; 1874 case -1266514778: // frames 1875 this.frames = castToPositiveInt(value); // PositiveIntType 1876 return value; 1877 case -1992012396: // duration 1878 this.duration = castToDecimal(value); // DecimalType 1879 return value; 1880 case 951530617: // content 1881 this.content = castToAttachment(value); // Attachment 1882 return value; 1883 case 3387378: // note 1884 this.getNote().add(castToAnnotation(value)); // Annotation 1885 return value; 1886 default: 1887 return super.setProperty(hash, name, value); 1888 } 1889 1890 } 1891 1892 @Override 1893 public Base setProperty(String name, Base value) throws FHIRException { 1894 if (name.equals("identifier")) { 1895 this.getIdentifier().add(castToIdentifier(value)); 1896 } else if (name.equals("basedOn")) { 1897 this.getBasedOn().add(castToReference(value)); 1898 } else if (name.equals("partOf")) { 1899 this.getPartOf().add(castToReference(value)); 1900 } else if (name.equals("status")) { 1901 value = new MediaStatusEnumFactory().fromType(castToCode(value)); 1902 this.status = (Enumeration) value; // Enumeration<MediaStatus> 1903 } else if (name.equals("type")) { 1904 this.type = castToCodeableConcept(value); // CodeableConcept 1905 } else if (name.equals("modality")) { 1906 this.modality = castToCodeableConcept(value); // CodeableConcept 1907 } else if (name.equals("view")) { 1908 this.view = castToCodeableConcept(value); // CodeableConcept 1909 } else if (name.equals("subject")) { 1910 this.subject = castToReference(value); // Reference 1911 } else if (name.equals("encounter")) { 1912 this.encounter = castToReference(value); // Reference 1913 } else if (name.equals("created[x]")) { 1914 this.created = castToType(value); // Type 1915 } else if (name.equals("issued")) { 1916 this.issued = castToInstant(value); // InstantType 1917 } else if (name.equals("operator")) { 1918 this.operator = castToReference(value); // Reference 1919 } else if (name.equals("reasonCode")) { 1920 this.getReasonCode().add(castToCodeableConcept(value)); 1921 } else if (name.equals("bodySite")) { 1922 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1923 } else if (name.equals("deviceName")) { 1924 this.deviceName = castToString(value); // StringType 1925 } else if (name.equals("device")) { 1926 this.device = castToReference(value); // Reference 1927 } else if (name.equals("height")) { 1928 this.height = castToPositiveInt(value); // PositiveIntType 1929 } else if (name.equals("width")) { 1930 this.width = castToPositiveInt(value); // PositiveIntType 1931 } else if (name.equals("frames")) { 1932 this.frames = castToPositiveInt(value); // PositiveIntType 1933 } else if (name.equals("duration")) { 1934 this.duration = castToDecimal(value); // DecimalType 1935 } else if (name.equals("content")) { 1936 this.content = castToAttachment(value); // Attachment 1937 } else if (name.equals("note")) { 1938 this.getNote().add(castToAnnotation(value)); 1939 } else 1940 return super.setProperty(name, value); 1941 return value; 1942 } 1943 1944 @Override 1945 public void removeChild(String name, Base value) throws FHIRException { 1946 if (name.equals("identifier")) { 1947 this.getIdentifier().remove(castToIdentifier(value)); 1948 } else if (name.equals("basedOn")) { 1949 this.getBasedOn().remove(castToReference(value)); 1950 } else if (name.equals("partOf")) { 1951 this.getPartOf().remove(castToReference(value)); 1952 } else if (name.equals("status")) { 1953 this.status = null; 1954 } else if (name.equals("type")) { 1955 this.type = null; 1956 } else if (name.equals("modality")) { 1957 this.modality = null; 1958 } else if (name.equals("view")) { 1959 this.view = null; 1960 } else if (name.equals("subject")) { 1961 this.subject = null; 1962 } else if (name.equals("encounter")) { 1963 this.encounter = null; 1964 } else if (name.equals("created[x]")) { 1965 this.created = null; 1966 } else if (name.equals("issued")) { 1967 this.issued = null; 1968 } else if (name.equals("operator")) { 1969 this.operator = null; 1970 } else if (name.equals("reasonCode")) { 1971 this.getReasonCode().remove(castToCodeableConcept(value)); 1972 } else if (name.equals("bodySite")) { 1973 this.bodySite = null; 1974 } else if (name.equals("deviceName")) { 1975 this.deviceName = null; 1976 } else if (name.equals("device")) { 1977 this.device = null; 1978 } else if (name.equals("height")) { 1979 this.height = null; 1980 } else if (name.equals("width")) { 1981 this.width = null; 1982 } else if (name.equals("frames")) { 1983 this.frames = null; 1984 } else if (name.equals("duration")) { 1985 this.duration = null; 1986 } else if (name.equals("content")) { 1987 this.content = null; 1988 } else if (name.equals("note")) { 1989 this.getNote().remove(castToAnnotation(value)); 1990 } else 1991 super.removeChild(name, value); 1992 1993 } 1994 1995 @Override 1996 public Base makeProperty(int hash, String name) throws FHIRException { 1997 switch (hash) { 1998 case -1618432855: 1999 return addIdentifier(); 2000 case -332612366: 2001 return addBasedOn(); 2002 case -995410646: 2003 return addPartOf(); 2004 case -892481550: 2005 return getStatusElement(); 2006 case 3575610: 2007 return getType(); 2008 case -622722335: 2009 return getModality(); 2010 case 3619493: 2011 return getView(); 2012 case -1867885268: 2013 return getSubject(); 2014 case 1524132147: 2015 return getEncounter(); 2016 case 1369676952: 2017 return getCreated(); 2018 case 1028554472: 2019 return getCreated(); 2020 case -1179159893: 2021 return getIssuedElement(); 2022 case -500553564: 2023 return getOperator(); 2024 case 722137681: 2025 return addReasonCode(); 2026 case 1702620169: 2027 return getBodySite(); 2028 case 780988929: 2029 return getDeviceNameElement(); 2030 case -1335157162: 2031 return getDevice(); 2032 case -1221029593: 2033 return getHeightElement(); 2034 case 113126854: 2035 return getWidthElement(); 2036 case -1266514778: 2037 return getFramesElement(); 2038 case -1992012396: 2039 return getDurationElement(); 2040 case 951530617: 2041 return getContent(); 2042 case 3387378: 2043 return addNote(); 2044 default: 2045 return super.makeProperty(hash, name); 2046 } 2047 2048 } 2049 2050 @Override 2051 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2052 switch (hash) { 2053 case -1618432855: 2054 /* identifier */ return new String[] { "Identifier" }; 2055 case -332612366: 2056 /* basedOn */ return new String[] { "Reference" }; 2057 case -995410646: 2058 /* partOf */ return new String[] { "Reference" }; 2059 case -892481550: 2060 /* status */ return new String[] { "code" }; 2061 case 3575610: 2062 /* type */ return new String[] { "CodeableConcept" }; 2063 case -622722335: 2064 /* modality */ return new String[] { "CodeableConcept" }; 2065 case 3619493: 2066 /* view */ return new String[] { "CodeableConcept" }; 2067 case -1867885268: 2068 /* subject */ return new String[] { "Reference" }; 2069 case 1524132147: 2070 /* encounter */ return new String[] { "Reference" }; 2071 case 1028554472: 2072 /* created */ return new String[] { "dateTime", "Period" }; 2073 case -1179159893: 2074 /* issued */ return new String[] { "instant" }; 2075 case -500553564: 2076 /* operator */ return new String[] { "Reference" }; 2077 case 722137681: 2078 /* reasonCode */ return new String[] { "CodeableConcept" }; 2079 case 1702620169: 2080 /* bodySite */ return new String[] { "CodeableConcept" }; 2081 case 780988929: 2082 /* deviceName */ return new String[] { "string" }; 2083 case -1335157162: 2084 /* device */ return new String[] { "Reference" }; 2085 case -1221029593: 2086 /* height */ return new String[] { "positiveInt" }; 2087 case 113126854: 2088 /* width */ return new String[] { "positiveInt" }; 2089 case -1266514778: 2090 /* frames */ return new String[] { "positiveInt" }; 2091 case -1992012396: 2092 /* duration */ return new String[] { "decimal" }; 2093 case 951530617: 2094 /* content */ return new String[] { "Attachment" }; 2095 case 3387378: 2096 /* note */ return new String[] { "Annotation" }; 2097 default: 2098 return super.getTypesForProperty(hash, name); 2099 } 2100 2101 } 2102 2103 @Override 2104 public Base addChild(String name) throws FHIRException { 2105 if (name.equals("identifier")) { 2106 return addIdentifier(); 2107 } else if (name.equals("basedOn")) { 2108 return addBasedOn(); 2109 } else if (name.equals("partOf")) { 2110 return addPartOf(); 2111 } else if (name.equals("status")) { 2112 throw new FHIRException("Cannot call addChild on a singleton property Media.status"); 2113 } else if (name.equals("type")) { 2114 this.type = new CodeableConcept(); 2115 return this.type; 2116 } else if (name.equals("modality")) { 2117 this.modality = new CodeableConcept(); 2118 return this.modality; 2119 } else if (name.equals("view")) { 2120 this.view = new CodeableConcept(); 2121 return this.view; 2122 } else if (name.equals("subject")) { 2123 this.subject = new Reference(); 2124 return this.subject; 2125 } else if (name.equals("encounter")) { 2126 this.encounter = new Reference(); 2127 return this.encounter; 2128 } else if (name.equals("createdDateTime")) { 2129 this.created = new DateTimeType(); 2130 return this.created; 2131 } else if (name.equals("createdPeriod")) { 2132 this.created = new Period(); 2133 return this.created; 2134 } else if (name.equals("issued")) { 2135 throw new FHIRException("Cannot call addChild on a singleton property Media.issued"); 2136 } else if (name.equals("operator")) { 2137 this.operator = new Reference(); 2138 return this.operator; 2139 } else if (name.equals("reasonCode")) { 2140 return addReasonCode(); 2141 } else if (name.equals("bodySite")) { 2142 this.bodySite = new CodeableConcept(); 2143 return this.bodySite; 2144 } else if (name.equals("deviceName")) { 2145 throw new FHIRException("Cannot call addChild on a singleton property Media.deviceName"); 2146 } else if (name.equals("device")) { 2147 this.device = new Reference(); 2148 return this.device; 2149 } else if (name.equals("height")) { 2150 throw new FHIRException("Cannot call addChild on a singleton property Media.height"); 2151 } else if (name.equals("width")) { 2152 throw new FHIRException("Cannot call addChild on a singleton property Media.width"); 2153 } else if (name.equals("frames")) { 2154 throw new FHIRException("Cannot call addChild on a singleton property Media.frames"); 2155 } else if (name.equals("duration")) { 2156 throw new FHIRException("Cannot call addChild on a singleton property Media.duration"); 2157 } else if (name.equals("content")) { 2158 this.content = new Attachment(); 2159 return this.content; 2160 } else if (name.equals("note")) { 2161 return addNote(); 2162 } else 2163 return super.addChild(name); 2164 } 2165 2166 public String fhirType() { 2167 return "Media"; 2168 2169 } 2170 2171 public Media copy() { 2172 Media dst = new Media(); 2173 copyValues(dst); 2174 return dst; 2175 } 2176 2177 public void copyValues(Media dst) { 2178 super.copyValues(dst); 2179 if (identifier != null) { 2180 dst.identifier = new ArrayList<Identifier>(); 2181 for (Identifier i : identifier) 2182 dst.identifier.add(i.copy()); 2183 } 2184 ; 2185 if (basedOn != null) { 2186 dst.basedOn = new ArrayList<Reference>(); 2187 for (Reference i : basedOn) 2188 dst.basedOn.add(i.copy()); 2189 } 2190 ; 2191 if (partOf != null) { 2192 dst.partOf = new ArrayList<Reference>(); 2193 for (Reference i : partOf) 2194 dst.partOf.add(i.copy()); 2195 } 2196 ; 2197 dst.status = status == null ? null : status.copy(); 2198 dst.type = type == null ? null : type.copy(); 2199 dst.modality = modality == null ? null : modality.copy(); 2200 dst.view = view == null ? null : view.copy(); 2201 dst.subject = subject == null ? null : subject.copy(); 2202 dst.encounter = encounter == null ? null : encounter.copy(); 2203 dst.created = created == null ? null : created.copy(); 2204 dst.issued = issued == null ? null : issued.copy(); 2205 dst.operator = operator == null ? null : operator.copy(); 2206 if (reasonCode != null) { 2207 dst.reasonCode = new ArrayList<CodeableConcept>(); 2208 for (CodeableConcept i : reasonCode) 2209 dst.reasonCode.add(i.copy()); 2210 } 2211 ; 2212 dst.bodySite = bodySite == null ? null : bodySite.copy(); 2213 dst.deviceName = deviceName == null ? null : deviceName.copy(); 2214 dst.device = device == null ? null : device.copy(); 2215 dst.height = height == null ? null : height.copy(); 2216 dst.width = width == null ? null : width.copy(); 2217 dst.frames = frames == null ? null : frames.copy(); 2218 dst.duration = duration == null ? null : duration.copy(); 2219 dst.content = content == null ? null : content.copy(); 2220 if (note != null) { 2221 dst.note = new ArrayList<Annotation>(); 2222 for (Annotation i : note) 2223 dst.note.add(i.copy()); 2224 } 2225 ; 2226 } 2227 2228 protected Media typedCopy() { 2229 return copy(); 2230 } 2231 2232 @Override 2233 public boolean equalsDeep(Base other_) { 2234 if (!super.equalsDeep(other_)) 2235 return false; 2236 if (!(other_ instanceof Media)) 2237 return false; 2238 Media o = (Media) other_; 2239 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2240 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 2241 && compareDeep(modality, o.modality, true) && compareDeep(view, o.view, true) 2242 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2243 && compareDeep(created, o.created, true) && compareDeep(issued, o.issued, true) 2244 && compareDeep(operator, o.operator, true) && compareDeep(reasonCode, o.reasonCode, true) 2245 && compareDeep(bodySite, o.bodySite, true) && compareDeep(deviceName, o.deviceName, true) 2246 && compareDeep(device, o.device, true) && compareDeep(height, o.height, true) 2247 && compareDeep(width, o.width, true) && compareDeep(frames, o.frames, true) 2248 && compareDeep(duration, o.duration, true) && compareDeep(content, o.content, true) 2249 && compareDeep(note, o.note, true); 2250 } 2251 2252 @Override 2253 public boolean equalsShallow(Base other_) { 2254 if (!super.equalsShallow(other_)) 2255 return false; 2256 if (!(other_ instanceof Media)) 2257 return false; 2258 Media o = (Media) other_; 2259 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) 2260 && compareValues(deviceName, o.deviceName, true) && compareValues(height, o.height, true) 2261 && compareValues(width, o.width, true) && compareValues(frames, o.frames, true) 2262 && compareValues(duration, o.duration, true); 2263 } 2264 2265 public boolean isEmpty() { 2266 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, type, modality, 2267 view, subject, encounter, created, issued, operator, reasonCode, bodySite, deviceName, device, height, width, 2268 frames, duration, content, note); 2269 } 2270 2271 @Override 2272 public ResourceType getResourceType() { 2273 return ResourceType.Media; 2274 } 2275 2276 /** 2277 * Search parameter: <b>identifier</b> 2278 * <p> 2279 * Description: <b>Identifier(s) for the image</b><br> 2280 * Type: <b>token</b><br> 2281 * Path: <b>Media.identifier</b><br> 2282 * </p> 2283 */ 2284 @SearchParamDefinition(name = "identifier", path = "Media.identifier", description = "Identifier(s) for the image", type = "token") 2285 public static final String SP_IDENTIFIER = "identifier"; 2286 /** 2287 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2288 * <p> 2289 * Description: <b>Identifier(s) for the image</b><br> 2290 * Type: <b>token</b><br> 2291 * Path: <b>Media.identifier</b><br> 2292 * </p> 2293 */ 2294 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2295 SP_IDENTIFIER); 2296 2297 /** 2298 * Search parameter: <b>modality</b> 2299 * <p> 2300 * Description: <b>The type of acquisition equipment/process</b><br> 2301 * Type: <b>token</b><br> 2302 * Path: <b>Media.modality</b><br> 2303 * </p> 2304 */ 2305 @SearchParamDefinition(name = "modality", path = "Media.modality", description = "The type of acquisition equipment/process", type = "token") 2306 public static final String SP_MODALITY = "modality"; 2307 /** 2308 * <b>Fluent Client</b> search parameter constant for <b>modality</b> 2309 * <p> 2310 * Description: <b>The type of acquisition equipment/process</b><br> 2311 * Type: <b>token</b><br> 2312 * Path: <b>Media.modality</b><br> 2313 * </p> 2314 */ 2315 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2316 SP_MODALITY); 2317 2318 /** 2319 * Search parameter: <b>subject</b> 2320 * <p> 2321 * Description: <b>Who/What this Media is a record of</b><br> 2322 * Type: <b>reference</b><br> 2323 * Path: <b>Media.subject</b><br> 2324 * </p> 2325 */ 2326 @SearchParamDefinition(name = "subject", path = "Media.subject", description = "Who/What this Media is a record of", type = "reference", providesMembershipIn = { 2327 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2328 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2329 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 2330 Location.class, Patient.class, Practitioner.class, PractitionerRole.class, Specimen.class }) 2331 public static final String SP_SUBJECT = "subject"; 2332 /** 2333 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2334 * <p> 2335 * Description: <b>Who/What this Media is a record of</b><br> 2336 * Type: <b>reference</b><br> 2337 * Path: <b>Media.subject</b><br> 2338 * </p> 2339 */ 2340 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2341 SP_SUBJECT); 2342 2343 /** 2344 * Constant for fluent queries to be used to add include statements. Specifies 2345 * the path value of "<b>Media:subject</b>". 2346 */ 2347 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Media:subject") 2348 .toLocked(); 2349 2350 /** 2351 * Search parameter: <b>created</b> 2352 * <p> 2353 * Description: <b>When Media was collected</b><br> 2354 * Type: <b>date</b><br> 2355 * Path: <b>Media.created[x]</b><br> 2356 * </p> 2357 */ 2358 @SearchParamDefinition(name = "created", path = "Media.created", description = "When Media was collected", type = "date") 2359 public static final String SP_CREATED = "created"; 2360 /** 2361 * <b>Fluent Client</b> search parameter constant for <b>created</b> 2362 * <p> 2363 * Description: <b>When Media was collected</b><br> 2364 * Type: <b>date</b><br> 2365 * Path: <b>Media.created[x]</b><br> 2366 * </p> 2367 */ 2368 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2369 SP_CREATED); 2370 2371 /** 2372 * Search parameter: <b>encounter</b> 2373 * <p> 2374 * Description: <b>Encounter associated with media</b><br> 2375 * Type: <b>reference</b><br> 2376 * Path: <b>Media.encounter</b><br> 2377 * </p> 2378 */ 2379 @SearchParamDefinition(name = "encounter", path = "Media.encounter", description = "Encounter associated with media", type = "reference", providesMembershipIn = { 2380 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2381 public static final String SP_ENCOUNTER = "encounter"; 2382 /** 2383 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2384 * <p> 2385 * Description: <b>Encounter associated with media</b><br> 2386 * Type: <b>reference</b><br> 2387 * Path: <b>Media.encounter</b><br> 2388 * </p> 2389 */ 2390 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2391 SP_ENCOUNTER); 2392 2393 /** 2394 * Constant for fluent queries to be used to add include statements. Specifies 2395 * the path value of "<b>Media:encounter</b>". 2396 */ 2397 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2398 "Media:encounter").toLocked(); 2399 2400 /** 2401 * Search parameter: <b>type</b> 2402 * <p> 2403 * Description: <b>Classification of media as image, video, or audio</b><br> 2404 * Type: <b>token</b><br> 2405 * Path: <b>Media.type</b><br> 2406 * </p> 2407 */ 2408 @SearchParamDefinition(name = "type", path = "Media.type", description = "Classification of media as image, video, or audio", type = "token") 2409 public static final String SP_TYPE = "type"; 2410 /** 2411 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2412 * <p> 2413 * Description: <b>Classification of media as image, video, or audio</b><br> 2414 * Type: <b>token</b><br> 2415 * Path: <b>Media.type</b><br> 2416 * </p> 2417 */ 2418 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2419 SP_TYPE); 2420 2421 /** 2422 * Search parameter: <b>operator</b> 2423 * <p> 2424 * Description: <b>The person who generated the image</b><br> 2425 * Type: <b>reference</b><br> 2426 * Path: <b>Media.operator</b><br> 2427 * </p> 2428 */ 2429 @SearchParamDefinition(name = "operator", path = "Media.operator", description = "The person who generated the image", type = "reference", providesMembershipIn = { 2430 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { CareTeam.class, Device.class, 2431 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2432 public static final String SP_OPERATOR = "operator"; 2433 /** 2434 * <b>Fluent Client</b> search parameter constant for <b>operator</b> 2435 * <p> 2436 * Description: <b>The person who generated the image</b><br> 2437 * Type: <b>reference</b><br> 2438 * Path: <b>Media.operator</b><br> 2439 * </p> 2440 */ 2441 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OPERATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2442 SP_OPERATOR); 2443 2444 /** 2445 * Constant for fluent queries to be used to add include statements. Specifies 2446 * the path value of "<b>Media:operator</b>". 2447 */ 2448 public static final ca.uhn.fhir.model.api.Include INCLUDE_OPERATOR = new ca.uhn.fhir.model.api.Include( 2449 "Media:operator").toLocked(); 2450 2451 /** 2452 * Search parameter: <b>view</b> 2453 * <p> 2454 * Description: <b>Imaging view, e.g. Lateral or Antero-posterior</b><br> 2455 * Type: <b>token</b><br> 2456 * Path: <b>Media.view</b><br> 2457 * </p> 2458 */ 2459 @SearchParamDefinition(name = "view", path = "Media.view", description = "Imaging view, e.g. Lateral or Antero-posterior", type = "token") 2460 public static final String SP_VIEW = "view"; 2461 /** 2462 * <b>Fluent Client</b> search parameter constant for <b>view</b> 2463 * <p> 2464 * Description: <b>Imaging view, e.g. Lateral or Antero-posterior</b><br> 2465 * Type: <b>token</b><br> 2466 * Path: <b>Media.view</b><br> 2467 * </p> 2468 */ 2469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VIEW = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2470 SP_VIEW); 2471 2472 /** 2473 * Search parameter: <b>site</b> 2474 * <p> 2475 * Description: <b>Observed body part</b><br> 2476 * Type: <b>token</b><br> 2477 * Path: <b>Media.bodySite</b><br> 2478 * </p> 2479 */ 2480 @SearchParamDefinition(name = "site", path = "Media.bodySite", description = "Observed body part", type = "token") 2481 public static final String SP_SITE = "site"; 2482 /** 2483 * <b>Fluent Client</b> search parameter constant for <b>site</b> 2484 * <p> 2485 * Description: <b>Observed body part</b><br> 2486 * Type: <b>token</b><br> 2487 * Path: <b>Media.bodySite</b><br> 2488 * </p> 2489 */ 2490 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2491 SP_SITE); 2492 2493 /** 2494 * Search parameter: <b>based-on</b> 2495 * <p> 2496 * Description: <b>Procedure that caused this media to be created</b><br> 2497 * Type: <b>reference</b><br> 2498 * Path: <b>Media.basedOn</b><br> 2499 * </p> 2500 */ 2501 @SearchParamDefinition(name = "based-on", path = "Media.basedOn", description = "Procedure that caused this media to be created", type = "reference", target = { 2502 CarePlan.class, ServiceRequest.class }) 2503 public static final String SP_BASED_ON = "based-on"; 2504 /** 2505 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2506 * <p> 2507 * Description: <b>Procedure that caused this media to be created</b><br> 2508 * Type: <b>reference</b><br> 2509 * Path: <b>Media.basedOn</b><br> 2510 * </p> 2511 */ 2512 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2513 SP_BASED_ON); 2514 2515 /** 2516 * Constant for fluent queries to be used to add include statements. Specifies 2517 * the path value of "<b>Media:based-on</b>". 2518 */ 2519 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 2520 "Media:based-on").toLocked(); 2521 2522 /** 2523 * Search parameter: <b>patient</b> 2524 * <p> 2525 * Description: <b>Who/What this Media is a record of</b><br> 2526 * Type: <b>reference</b><br> 2527 * Path: <b>Media.subject</b><br> 2528 * </p> 2529 */ 2530 @SearchParamDefinition(name = "patient", path = "Media.subject.where(resolve() is Patient)", description = "Who/What this Media is a record of", type = "reference", target = { 2531 Patient.class }) 2532 public static final String SP_PATIENT = "patient"; 2533 /** 2534 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2535 * <p> 2536 * Description: <b>Who/What this Media is a record of</b><br> 2537 * Type: <b>reference</b><br> 2538 * Path: <b>Media.subject</b><br> 2539 * </p> 2540 */ 2541 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2542 SP_PATIENT); 2543 2544 /** 2545 * Constant for fluent queries to be used to add include statements. Specifies 2546 * the path value of "<b>Media:patient</b>". 2547 */ 2548 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Media:patient") 2549 .toLocked(); 2550 2551 /** 2552 * Search parameter: <b>device</b> 2553 * <p> 2554 * Description: <b>Observing Device</b><br> 2555 * Type: <b>reference</b><br> 2556 * Path: <b>Media.device</b><br> 2557 * </p> 2558 */ 2559 @SearchParamDefinition(name = "device", path = "Media.device", description = "Observing Device", type = "reference", target = { 2560 Device.class, DeviceMetric.class }) 2561 public static final String SP_DEVICE = "device"; 2562 /** 2563 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2564 * <p> 2565 * Description: <b>Observing Device</b><br> 2566 * Type: <b>reference</b><br> 2567 * Path: <b>Media.device</b><br> 2568 * </p> 2569 */ 2570 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2571 SP_DEVICE); 2572 2573 /** 2574 * Constant for fluent queries to be used to add include statements. Specifies 2575 * the path value of "<b>Media:device</b>". 2576 */ 2577 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("Media:device") 2578 .toLocked(); 2579 2580 /** 2581 * Search parameter: <b>status</b> 2582 * <p> 2583 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 2584 * completed | entered-in-error | unknown</b><br> 2585 * Type: <b>token</b><br> 2586 * Path: <b>Media.status</b><br> 2587 * </p> 2588 */ 2589 @SearchParamDefinition(name = "status", path = "Media.status", description = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type = "token") 2590 public static final String SP_STATUS = "status"; 2591 /** 2592 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2593 * <p> 2594 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 2595 * completed | entered-in-error | unknown</b><br> 2596 * Type: <b>token</b><br> 2597 * Path: <b>Media.status</b><br> 2598 * </p> 2599 */ 2600 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2601 SP_STATUS); 2602 2603}