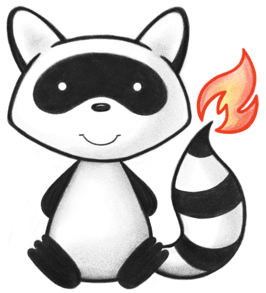
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * This resource is primarily used for the identification and definition of a 049 * medication for the purposes of prescribing, dispensing, and administering a 050 * medication as well as for making statements about medication use. 051 */ 052@ResourceDef(name = "Medication", profile = "http://hl7.org/fhir/StructureDefinition/Medication") 053public class Medication extends DomainResource { 054 055 public enum MedicationStatus { 056 /** 057 * The medication is available for use. 058 */ 059 ACTIVE, 060 /** 061 * The medication is not available for use. 062 */ 063 INACTIVE, 064 /** 065 * The medication was entered in error. 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 073 public static MedicationStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MedicationStatus code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case ACTIVE: 091 return "active"; 092 case INACTIVE: 093 return "inactive"; 094 case ENTEREDINERROR: 095 return "entered-in-error"; 096 case NULL: 097 return null; 098 default: 099 return "?"; 100 } 101 } 102 103 public String getSystem() { 104 switch (this) { 105 case ACTIVE: 106 return "http://hl7.org/fhir/CodeSystem/medication-status"; 107 case INACTIVE: 108 return "http://hl7.org/fhir/CodeSystem/medication-status"; 109 case ENTEREDINERROR: 110 return "http://hl7.org/fhir/CodeSystem/medication-status"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getDefinition() { 119 switch (this) { 120 case ACTIVE: 121 return "The medication is available for use."; 122 case INACTIVE: 123 return "The medication is not available for use."; 124 case ENTEREDINERROR: 125 return "The medication was entered in error."; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getDisplay() { 134 switch (this) { 135 case ACTIVE: 136 return "Active"; 137 case INACTIVE: 138 return "Inactive"; 139 case ENTEREDINERROR: 140 return "Entered in Error"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 } 148 149 public static class MedicationStatusEnumFactory implements EnumFactory<MedicationStatus> { 150 public MedicationStatus fromCode(String codeString) throws IllegalArgumentException { 151 if (codeString == null || "".equals(codeString)) 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("active".equals(codeString)) 155 return MedicationStatus.ACTIVE; 156 if ("inactive".equals(codeString)) 157 return MedicationStatus.INACTIVE; 158 if ("entered-in-error".equals(codeString)) 159 return MedicationStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown MedicationStatus code '" + codeString + "'"); 161 } 162 163 public Enumeration<MedicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<MedicationStatus>(this, MedicationStatus.NULL, code); 168 String codeString = code.asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return new Enumeration<MedicationStatus>(this, MedicationStatus.NULL, code); 171 if ("active".equals(codeString)) 172 return new Enumeration<MedicationStatus>(this, MedicationStatus.ACTIVE, code); 173 if ("inactive".equals(codeString)) 174 return new Enumeration<MedicationStatus>(this, MedicationStatus.INACTIVE, code); 175 if ("entered-in-error".equals(codeString)) 176 return new Enumeration<MedicationStatus>(this, MedicationStatus.ENTEREDINERROR, code); 177 throw new FHIRException("Unknown MedicationStatus code '" + codeString + "'"); 178 } 179 180 public String toCode(MedicationStatus code) { 181 if (code == MedicationStatus.NULL) 182 return null; 183 if (code == MedicationStatus.ACTIVE) 184 return "active"; 185 if (code == MedicationStatus.INACTIVE) 186 return "inactive"; 187 if (code == MedicationStatus.ENTEREDINERROR) 188 return "entered-in-error"; 189 return "?"; 190 } 191 192 public String toSystem(MedicationStatus code) { 193 return code.getSystem(); 194 } 195 } 196 197 @Block() 198 public static class MedicationIngredientComponent extends BackboneElement implements IBaseBackboneElement { 199 /** 200 * The actual ingredient - either a substance (simple ingredient) or another 201 * medication of a medication. 202 */ 203 @Child(name = "item", type = { CodeableConcept.class, Substance.class, 204 Medication.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 205 @Description(shortDefinition = "The actual ingredient or content", formalDefinition = "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.") 206 protected Type item; 207 208 /** 209 * Indication of whether this ingredient affects the therapeutic action of the 210 * drug. 211 */ 212 @Child(name = "isActive", type = { 213 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 214 @Description(shortDefinition = "Active ingredient indicator", formalDefinition = "Indication of whether this ingredient affects the therapeutic action of the drug.") 215 protected BooleanType isActive; 216 217 /** 218 * Specifies how many (or how much) of the items there are in this Medication. 219 * For example, 250 mg per tablet. This is expressed as a ratio where the 220 * numerator is 250mg and the denominator is 1 tablet. 221 */ 222 @Child(name = "strength", type = { Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 223 @Description(shortDefinition = "Quantity of ingredient present", formalDefinition = "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.") 224 protected Ratio strength; 225 226 private static final long serialVersionUID = 1365103497L; 227 228 /** 229 * Constructor 230 */ 231 public MedicationIngredientComponent() { 232 super(); 233 } 234 235 /** 236 * Constructor 237 */ 238 public MedicationIngredientComponent(Type item) { 239 super(); 240 this.item = item; 241 } 242 243 /** 244 * @return {@link #item} (The actual ingredient - either a substance (simple 245 * ingredient) or another medication of a medication.) 246 */ 247 public Type getItem() { 248 return this.item; 249 } 250 251 /** 252 * @return {@link #item} (The actual ingredient - either a substance (simple 253 * ingredient) or another medication of a medication.) 254 */ 255 public CodeableConcept getItemCodeableConcept() throws FHIRException { 256 if (this.item == null) 257 this.item = new CodeableConcept(); 258 if (!(this.item instanceof CodeableConcept)) 259 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 260 + this.item.getClass().getName() + " was encountered"); 261 return (CodeableConcept) this.item; 262 } 263 264 public boolean hasItemCodeableConcept() { 265 return this != null && this.item instanceof CodeableConcept; 266 } 267 268 /** 269 * @return {@link #item} (The actual ingredient - either a substance (simple 270 * ingredient) or another medication of a medication.) 271 */ 272 public Reference getItemReference() throws FHIRException { 273 if (this.item == null) 274 this.item = new Reference(); 275 if (!(this.item instanceof Reference)) 276 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 277 + " was encountered"); 278 return (Reference) this.item; 279 } 280 281 public boolean hasItemReference() { 282 return this != null && this.item instanceof Reference; 283 } 284 285 public boolean hasItem() { 286 return this.item != null && !this.item.isEmpty(); 287 } 288 289 /** 290 * @param value {@link #item} (The actual ingredient - either a substance 291 * (simple ingredient) or another medication of a medication.) 292 */ 293 public MedicationIngredientComponent setItem(Type value) { 294 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 295 throw new Error("Not the right type for Medication.ingredient.item[x]: " + value.fhirType()); 296 this.item = value; 297 return this; 298 } 299 300 /** 301 * @return {@link #isActive} (Indication of whether this ingredient affects the 302 * therapeutic action of the drug.). This is the underlying object with 303 * id, value and extensions. The accessor "getIsActive" gives direct 304 * access to the value 305 */ 306 public BooleanType getIsActiveElement() { 307 if (this.isActive == null) 308 if (Configuration.errorOnAutoCreate()) 309 throw new Error("Attempt to auto-create MedicationIngredientComponent.isActive"); 310 else if (Configuration.doAutoCreate()) 311 this.isActive = new BooleanType(); // bb 312 return this.isActive; 313 } 314 315 public boolean hasIsActiveElement() { 316 return this.isActive != null && !this.isActive.isEmpty(); 317 } 318 319 public boolean hasIsActive() { 320 return this.isActive != null && !this.isActive.isEmpty(); 321 } 322 323 /** 324 * @param value {@link #isActive} (Indication of whether this ingredient affects 325 * the therapeutic action of the drug.). This is the underlying 326 * object with id, value and extensions. The accessor "getIsActive" 327 * gives direct access to the value 328 */ 329 public MedicationIngredientComponent setIsActiveElement(BooleanType value) { 330 this.isActive = value; 331 return this; 332 } 333 334 /** 335 * @return Indication of whether this ingredient affects the therapeutic action 336 * of the drug. 337 */ 338 public boolean getIsActive() { 339 return this.isActive == null || this.isActive.isEmpty() ? false : this.isActive.getValue(); 340 } 341 342 /** 343 * @param value Indication of whether this ingredient affects the therapeutic 344 * action of the drug. 345 */ 346 public MedicationIngredientComponent setIsActive(boolean value) { 347 if (this.isActive == null) 348 this.isActive = new BooleanType(); 349 this.isActive.setValue(value); 350 return this; 351 } 352 353 /** 354 * @return {@link #strength} (Specifies how many (or how much) of the items 355 * there are in this Medication. For example, 250 mg per tablet. This is 356 * expressed as a ratio where the numerator is 250mg and the denominator 357 * is 1 tablet.) 358 */ 359 public Ratio getStrength() { 360 if (this.strength == null) 361 if (Configuration.errorOnAutoCreate()) 362 throw new Error("Attempt to auto-create MedicationIngredientComponent.strength"); 363 else if (Configuration.doAutoCreate()) 364 this.strength = new Ratio(); // cc 365 return this.strength; 366 } 367 368 public boolean hasStrength() { 369 return this.strength != null && !this.strength.isEmpty(); 370 } 371 372 /** 373 * @param value {@link #strength} (Specifies how many (or how much) of the items 374 * there are in this Medication. For example, 250 mg per tablet. 375 * This is expressed as a ratio where the numerator is 250mg and 376 * the denominator is 1 tablet.) 377 */ 378 public MedicationIngredientComponent setStrength(Ratio value) { 379 this.strength = value; 380 return this; 381 } 382 383 protected void listChildren(List<Property> children) { 384 super.listChildren(children); 385 children.add(new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 386 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 1, 387 item)); 388 children.add(new Property("isActive", "boolean", 389 "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive)); 390 children.add(new Property("strength", "Ratio", 391 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 392 0, 1, strength)); 393 } 394 395 @Override 396 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 397 switch (_hash) { 398 case 2116201613: 399 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 400 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 401 1, item); 402 case 3242771: 403 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 404 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 405 1, item); 406 case 106644494: 407 /* itemCodeableConcept */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 408 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 409 1, item); 410 case 1376364920: 411 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 412 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 413 1, item); 414 case -748916528: 415 /* isActive */ return new Property("isActive", "boolean", 416 "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive); 417 case 1791316033: 418 /* strength */ return new Property("strength", "Ratio", 419 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 420 0, 1, strength); 421 default: 422 return super.getNamedProperty(_hash, _name, _checkValid); 423 } 424 425 } 426 427 @Override 428 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 429 switch (hash) { 430 case 3242771: 431 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 432 case -748916528: 433 /* isActive */ return this.isActive == null ? new Base[0] : new Base[] { this.isActive }; // BooleanType 434 case 1791316033: 435 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Ratio 436 default: 437 return super.getProperty(hash, name, checkValid); 438 } 439 440 } 441 442 @Override 443 public Base setProperty(int hash, String name, Base value) throws FHIRException { 444 switch (hash) { 445 case 3242771: // item 446 this.item = castToType(value); // Type 447 return value; 448 case -748916528: // isActive 449 this.isActive = castToBoolean(value); // BooleanType 450 return value; 451 case 1791316033: // strength 452 this.strength = castToRatio(value); // Ratio 453 return value; 454 default: 455 return super.setProperty(hash, name, value); 456 } 457 458 } 459 460 @Override 461 public Base setProperty(String name, Base value) throws FHIRException { 462 if (name.equals("item[x]")) { 463 this.item = castToType(value); // Type 464 } else if (name.equals("isActive")) { 465 this.isActive = castToBoolean(value); // BooleanType 466 } else if (name.equals("strength")) { 467 this.strength = castToRatio(value); // Ratio 468 } else 469 return super.setProperty(name, value); 470 return value; 471 } 472 473 @Override 474 public void removeChild(String name, Base value) throws FHIRException { 475 if (name.equals("item[x]")) { 476 this.item = null; 477 } else if (name.equals("isActive")) { 478 this.isActive = null; 479 } else if (name.equals("strength")) { 480 this.strength = null; 481 } else 482 super.removeChild(name, value); 483 484 } 485 486 @Override 487 public Base makeProperty(int hash, String name) throws FHIRException { 488 switch (hash) { 489 case 2116201613: 490 return getItem(); 491 case 3242771: 492 return getItem(); 493 case -748916528: 494 return getIsActiveElement(); 495 case 1791316033: 496 return getStrength(); 497 default: 498 return super.makeProperty(hash, name); 499 } 500 501 } 502 503 @Override 504 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 505 switch (hash) { 506 case 3242771: 507 /* item */ return new String[] { "CodeableConcept", "Reference" }; 508 case -748916528: 509 /* isActive */ return new String[] { "boolean" }; 510 case 1791316033: 511 /* strength */ return new String[] { "Ratio" }; 512 default: 513 return super.getTypesForProperty(hash, name); 514 } 515 516 } 517 518 @Override 519 public Base addChild(String name) throws FHIRException { 520 if (name.equals("itemCodeableConcept")) { 521 this.item = new CodeableConcept(); 522 return this.item; 523 } else if (name.equals("itemReference")) { 524 this.item = new Reference(); 525 return this.item; 526 } else if (name.equals("isActive")) { 527 throw new FHIRException("Cannot call addChild on a singleton property Medication.isActive"); 528 } else if (name.equals("strength")) { 529 this.strength = new Ratio(); 530 return this.strength; 531 } else 532 return super.addChild(name); 533 } 534 535 public MedicationIngredientComponent copy() { 536 MedicationIngredientComponent dst = new MedicationIngredientComponent(); 537 copyValues(dst); 538 return dst; 539 } 540 541 public void copyValues(MedicationIngredientComponent dst) { 542 super.copyValues(dst); 543 dst.item = item == null ? null : item.copy(); 544 dst.isActive = isActive == null ? null : isActive.copy(); 545 dst.strength = strength == null ? null : strength.copy(); 546 } 547 548 @Override 549 public boolean equalsDeep(Base other_) { 550 if (!super.equalsDeep(other_)) 551 return false; 552 if (!(other_ instanceof MedicationIngredientComponent)) 553 return false; 554 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 555 return compareDeep(item, o.item, true) && compareDeep(isActive, o.isActive, true) 556 && compareDeep(strength, o.strength, true); 557 } 558 559 @Override 560 public boolean equalsShallow(Base other_) { 561 if (!super.equalsShallow(other_)) 562 return false; 563 if (!(other_ instanceof MedicationIngredientComponent)) 564 return false; 565 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 566 return compareValues(isActive, o.isActive, true); 567 } 568 569 public boolean isEmpty() { 570 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, isActive, strength); 571 } 572 573 public String fhirType() { 574 return "Medication.ingredient"; 575 576 } 577 578 } 579 580 @Block() 581 public static class MedicationBatchComponent extends BackboneElement implements IBaseBackboneElement { 582 /** 583 * The assigned lot number of a batch of the specified product. 584 */ 585 @Child(name = "lotNumber", type = { 586 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 587 @Description(shortDefinition = "Identifier assigned to batch", formalDefinition = "The assigned lot number of a batch of the specified product.") 588 protected StringType lotNumber; 589 590 /** 591 * When this specific batch of product will expire. 592 */ 593 @Child(name = "expirationDate", type = { 594 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 595 @Description(shortDefinition = "When batch will expire", formalDefinition = "When this specific batch of product will expire.") 596 protected DateTimeType expirationDate; 597 598 private static final long serialVersionUID = 1982738755L; 599 600 /** 601 * Constructor 602 */ 603 public MedicationBatchComponent() { 604 super(); 605 } 606 607 /** 608 * @return {@link #lotNumber} (The assigned lot number of a batch of the 609 * specified product.). This is the underlying object with id, value and 610 * extensions. The accessor "getLotNumber" gives direct access to the 611 * value 612 */ 613 public StringType getLotNumberElement() { 614 if (this.lotNumber == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create MedicationBatchComponent.lotNumber"); 617 else if (Configuration.doAutoCreate()) 618 this.lotNumber = new StringType(); // bb 619 return this.lotNumber; 620 } 621 622 public boolean hasLotNumberElement() { 623 return this.lotNumber != null && !this.lotNumber.isEmpty(); 624 } 625 626 public boolean hasLotNumber() { 627 return this.lotNumber != null && !this.lotNumber.isEmpty(); 628 } 629 630 /** 631 * @param value {@link #lotNumber} (The assigned lot number of a batch of the 632 * specified product.). This is the underlying object with id, 633 * value and extensions. The accessor "getLotNumber" gives direct 634 * access to the value 635 */ 636 public MedicationBatchComponent setLotNumberElement(StringType value) { 637 this.lotNumber = value; 638 return this; 639 } 640 641 /** 642 * @return The assigned lot number of a batch of the specified product. 643 */ 644 public String getLotNumber() { 645 return this.lotNumber == null ? null : this.lotNumber.getValue(); 646 } 647 648 /** 649 * @param value The assigned lot number of a batch of the specified product. 650 */ 651 public MedicationBatchComponent setLotNumber(String value) { 652 if (Utilities.noString(value)) 653 this.lotNumber = null; 654 else { 655 if (this.lotNumber == null) 656 this.lotNumber = new StringType(); 657 this.lotNumber.setValue(value); 658 } 659 return this; 660 } 661 662 /** 663 * @return {@link #expirationDate} (When this specific batch of product will 664 * expire.). This is the underlying object with id, value and 665 * extensions. The accessor "getExpirationDate" gives direct access to 666 * the value 667 */ 668 public DateTimeType getExpirationDateElement() { 669 if (this.expirationDate == null) 670 if (Configuration.errorOnAutoCreate()) 671 throw new Error("Attempt to auto-create MedicationBatchComponent.expirationDate"); 672 else if (Configuration.doAutoCreate()) 673 this.expirationDate = new DateTimeType(); // bb 674 return this.expirationDate; 675 } 676 677 public boolean hasExpirationDateElement() { 678 return this.expirationDate != null && !this.expirationDate.isEmpty(); 679 } 680 681 public boolean hasExpirationDate() { 682 return this.expirationDate != null && !this.expirationDate.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #expirationDate} (When this specific batch of product 687 * will expire.). This is the underlying object with id, value and 688 * extensions. The accessor "getExpirationDate" gives direct access 689 * to the value 690 */ 691 public MedicationBatchComponent setExpirationDateElement(DateTimeType value) { 692 this.expirationDate = value; 693 return this; 694 } 695 696 /** 697 * @return When this specific batch of product will expire. 698 */ 699 public Date getExpirationDate() { 700 return this.expirationDate == null ? null : this.expirationDate.getValue(); 701 } 702 703 /** 704 * @param value When this specific batch of product will expire. 705 */ 706 public MedicationBatchComponent setExpirationDate(Date value) { 707 if (value == null) 708 this.expirationDate = null; 709 else { 710 if (this.expirationDate == null) 711 this.expirationDate = new DateTimeType(); 712 this.expirationDate.setValue(value); 713 } 714 return this; 715 } 716 717 protected void listChildren(List<Property> children) { 718 super.listChildren(children); 719 children.add(new Property("lotNumber", "string", "The assigned lot number of a batch of the specified product.", 720 0, 1, lotNumber)); 721 children.add(new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 1, 722 expirationDate)); 723 } 724 725 @Override 726 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 727 switch (_hash) { 728 case 462547450: 729 /* lotNumber */ return new Property("lotNumber", "string", 730 "The assigned lot number of a batch of the specified product.", 0, 1, lotNumber); 731 case -668811523: 732 /* expirationDate */ return new Property("expirationDate", "dateTime", 733 "When this specific batch of product will expire.", 0, 1, expirationDate); 734 default: 735 return super.getNamedProperty(_hash, _name, _checkValid); 736 } 737 738 } 739 740 @Override 741 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 742 switch (hash) { 743 case 462547450: 744 /* lotNumber */ return this.lotNumber == null ? new Base[0] : new Base[] { this.lotNumber }; // StringType 745 case -668811523: 746 /* expirationDate */ return this.expirationDate == null ? new Base[0] : new Base[] { this.expirationDate }; // DateTimeType 747 default: 748 return super.getProperty(hash, name, checkValid); 749 } 750 751 } 752 753 @Override 754 public Base setProperty(int hash, String name, Base value) throws FHIRException { 755 switch (hash) { 756 case 462547450: // lotNumber 757 this.lotNumber = castToString(value); // StringType 758 return value; 759 case -668811523: // expirationDate 760 this.expirationDate = castToDateTime(value); // DateTimeType 761 return value; 762 default: 763 return super.setProperty(hash, name, value); 764 } 765 766 } 767 768 @Override 769 public Base setProperty(String name, Base value) throws FHIRException { 770 if (name.equals("lotNumber")) { 771 this.lotNumber = castToString(value); // StringType 772 } else if (name.equals("expirationDate")) { 773 this.expirationDate = castToDateTime(value); // DateTimeType 774 } else 775 return super.setProperty(name, value); 776 return value; 777 } 778 779 @Override 780 public void removeChild(String name, Base value) throws FHIRException { 781 if (name.equals("lotNumber")) { 782 this.lotNumber = null; 783 } else if (name.equals("expirationDate")) { 784 this.expirationDate = null; 785 } else 786 super.removeChild(name, value); 787 788 } 789 790 @Override 791 public Base makeProperty(int hash, String name) throws FHIRException { 792 switch (hash) { 793 case 462547450: 794 return getLotNumberElement(); 795 case -668811523: 796 return getExpirationDateElement(); 797 default: 798 return super.makeProperty(hash, name); 799 } 800 801 } 802 803 @Override 804 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 805 switch (hash) { 806 case 462547450: 807 /* lotNumber */ return new String[] { "string" }; 808 case -668811523: 809 /* expirationDate */ return new String[] { "dateTime" }; 810 default: 811 return super.getTypesForProperty(hash, name); 812 } 813 814 } 815 816 @Override 817 public Base addChild(String name) throws FHIRException { 818 if (name.equals("lotNumber")) { 819 throw new FHIRException("Cannot call addChild on a singleton property Medication.lotNumber"); 820 } else if (name.equals("expirationDate")) { 821 throw new FHIRException("Cannot call addChild on a singleton property Medication.expirationDate"); 822 } else 823 return super.addChild(name); 824 } 825 826 public MedicationBatchComponent copy() { 827 MedicationBatchComponent dst = new MedicationBatchComponent(); 828 copyValues(dst); 829 return dst; 830 } 831 832 public void copyValues(MedicationBatchComponent dst) { 833 super.copyValues(dst); 834 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 835 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 836 } 837 838 @Override 839 public boolean equalsDeep(Base other_) { 840 if (!super.equalsDeep(other_)) 841 return false; 842 if (!(other_ instanceof MedicationBatchComponent)) 843 return false; 844 MedicationBatchComponent o = (MedicationBatchComponent) other_; 845 return compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true); 846 } 847 848 @Override 849 public boolean equalsShallow(Base other_) { 850 if (!super.equalsShallow(other_)) 851 return false; 852 if (!(other_ instanceof MedicationBatchComponent)) 853 return false; 854 MedicationBatchComponent o = (MedicationBatchComponent) other_; 855 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true); 856 } 857 858 public boolean isEmpty() { 859 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lotNumber, expirationDate); 860 } 861 862 public String fhirType() { 863 return "Medication.batch"; 864 865 } 866 867 } 868 869 /** 870 * Business identifier for this medication. 871 */ 872 @Child(name = "identifier", type = { 873 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 874 @Description(shortDefinition = "Business identifier for this medication", formalDefinition = "Business identifier for this medication.") 875 protected List<Identifier> identifier; 876 877 /** 878 * A code (or set of codes) that specify this medication, or a textual 879 * description if no code is available. Usage note: This could be a standard 880 * medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could 881 * also be a national or local formulary code, optionally with translations to 882 * other code systems. 883 */ 884 @Child(name = "code", type = { CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 885 @Description(shortDefinition = "Codes that identify this medication", formalDefinition = "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.") 886 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 887 protected CodeableConcept code; 888 889 /** 890 * A code to indicate if the medication is in active use. 891 */ 892 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 893 @Description(shortDefinition = "active | inactive | entered-in-error", formalDefinition = "A code to indicate if the medication is in active use.") 894 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-status") 895 protected Enumeration<MedicationStatus> status; 896 897 /** 898 * Describes the details of the manufacturer of the medication product. This is 899 * not intended to represent the distributor of a medication product. 900 */ 901 @Child(name = "manufacturer", type = { 902 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 903 @Description(shortDefinition = "Manufacturer of the item", formalDefinition = "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.") 904 protected Reference manufacturer; 905 906 /** 907 * The actual object that is the target of the reference (Describes the details 908 * of the manufacturer of the medication product. This is not intended to 909 * represent the distributor of a medication product.) 910 */ 911 protected Organization manufacturerTarget; 912 913 /** 914 * Describes the form of the item. Powder; tablets; capsule. 915 */ 916 @Child(name = "form", type = { 917 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 918 @Description(shortDefinition = "powder | tablets | capsule +", formalDefinition = "Describes the form of the item. Powder; tablets; capsule.") 919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-form-codes") 920 protected CodeableConcept form; 921 922 /** 923 * Specific amount of the drug in the packaged product. For example, when 924 * specifying a product that has the same strength (For example, Insulin 925 * glargine 100 unit per mL solution for injection), this attribute provides 926 * additional clarification of the package amount (For example, 3 mL, 10mL, 927 * etc.). 928 */ 929 @Child(name = "amount", type = { Ratio.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 930 @Description(shortDefinition = "Amount of drug in package", formalDefinition = "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).") 931 protected Ratio amount; 932 933 /** 934 * Identifies a particular constituent of interest in the product. 935 */ 936 @Child(name = "ingredient", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 937 @Description(shortDefinition = "Active or inactive ingredient", formalDefinition = "Identifies a particular constituent of interest in the product.") 938 protected List<MedicationIngredientComponent> ingredient; 939 940 /** 941 * Information that only applies to packages (not products). 942 */ 943 @Child(name = "batch", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 944 @Description(shortDefinition = "Details about packaged medications", formalDefinition = "Information that only applies to packages (not products).") 945 protected MedicationBatchComponent batch; 946 947 private static final long serialVersionUID = 781229373L; 948 949 /** 950 * Constructor 951 */ 952 public Medication() { 953 super(); 954 } 955 956 /** 957 * @return {@link #identifier} (Business identifier for this medication.) 958 */ 959 public List<Identifier> getIdentifier() { 960 if (this.identifier == null) 961 this.identifier = new ArrayList<Identifier>(); 962 return this.identifier; 963 } 964 965 /** 966 * @return Returns a reference to <code>this</code> for easy method chaining 967 */ 968 public Medication setIdentifier(List<Identifier> theIdentifier) { 969 this.identifier = theIdentifier; 970 return this; 971 } 972 973 public boolean hasIdentifier() { 974 if (this.identifier == null) 975 return false; 976 for (Identifier item : this.identifier) 977 if (!item.isEmpty()) 978 return true; 979 return false; 980 } 981 982 public Identifier addIdentifier() { // 3 983 Identifier t = new Identifier(); 984 if (this.identifier == null) 985 this.identifier = new ArrayList<Identifier>(); 986 this.identifier.add(t); 987 return t; 988 } 989 990 public Medication addIdentifier(Identifier t) { // 3 991 if (t == null) 992 return this; 993 if (this.identifier == null) 994 this.identifier = new ArrayList<Identifier>(); 995 this.identifier.add(t); 996 return this; 997 } 998 999 /** 1000 * @return The first repetition of repeating field {@link #identifier}, creating 1001 * it if it does not already exist 1002 */ 1003 public Identifier getIdentifierFirstRep() { 1004 if (getIdentifier().isEmpty()) { 1005 addIdentifier(); 1006 } 1007 return getIdentifier().get(0); 1008 } 1009 1010 /** 1011 * @return {@link #code} (A code (or set of codes) that specify this medication, 1012 * or a textual description if no code is available. Usage note: This 1013 * could be a standard medication code such as a code from RxNorm, 1014 * SNOMED CT, IDMP etc. It could also be a national or local formulary 1015 * code, optionally with translations to other code systems.) 1016 */ 1017 public CodeableConcept getCode() { 1018 if (this.code == null) 1019 if (Configuration.errorOnAutoCreate()) 1020 throw new Error("Attempt to auto-create Medication.code"); 1021 else if (Configuration.doAutoCreate()) 1022 this.code = new CodeableConcept(); // cc 1023 return this.code; 1024 } 1025 1026 public boolean hasCode() { 1027 return this.code != null && !this.code.isEmpty(); 1028 } 1029 1030 /** 1031 * @param value {@link #code} (A code (or set of codes) that specify this 1032 * medication, or a textual description if no code is available. 1033 * Usage note: This could be a standard medication code such as a 1034 * code from RxNorm, SNOMED CT, IDMP etc. It could also be a 1035 * national or local formulary code, optionally with translations 1036 * to other code systems.) 1037 */ 1038 public Medication setCode(CodeableConcept value) { 1039 this.code = value; 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #status} (A code to indicate if the medication is in active 1045 * use.). This is the underlying object with id, value and extensions. 1046 * The accessor "getStatus" gives direct access to the value 1047 */ 1048 public Enumeration<MedicationStatus> getStatusElement() { 1049 if (this.status == null) 1050 if (Configuration.errorOnAutoCreate()) 1051 throw new Error("Attempt to auto-create Medication.status"); 1052 else if (Configuration.doAutoCreate()) 1053 this.status = new Enumeration<MedicationStatus>(new MedicationStatusEnumFactory()); // bb 1054 return this.status; 1055 } 1056 1057 public boolean hasStatusElement() { 1058 return this.status != null && !this.status.isEmpty(); 1059 } 1060 1061 public boolean hasStatus() { 1062 return this.status != null && !this.status.isEmpty(); 1063 } 1064 1065 /** 1066 * @param value {@link #status} (A code to indicate if the medication is in 1067 * active use.). This is the underlying object with id, value and 1068 * extensions. The accessor "getStatus" gives direct access to the 1069 * value 1070 */ 1071 public Medication setStatusElement(Enumeration<MedicationStatus> value) { 1072 this.status = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return A code to indicate if the medication is in active use. 1078 */ 1079 public MedicationStatus getStatus() { 1080 return this.status == null ? null : this.status.getValue(); 1081 } 1082 1083 /** 1084 * @param value A code to indicate if the medication is in active use. 1085 */ 1086 public Medication setStatus(MedicationStatus value) { 1087 if (value == null) 1088 this.status = null; 1089 else { 1090 if (this.status == null) 1091 this.status = new Enumeration<MedicationStatus>(new MedicationStatusEnumFactory()); 1092 this.status.setValue(value); 1093 } 1094 return this; 1095 } 1096 1097 /** 1098 * @return {@link #manufacturer} (Describes the details of the manufacturer of 1099 * the medication product. This is not intended to represent the 1100 * distributor of a medication product.) 1101 */ 1102 public Reference getManufacturer() { 1103 if (this.manufacturer == null) 1104 if (Configuration.errorOnAutoCreate()) 1105 throw new Error("Attempt to auto-create Medication.manufacturer"); 1106 else if (Configuration.doAutoCreate()) 1107 this.manufacturer = new Reference(); // cc 1108 return this.manufacturer; 1109 } 1110 1111 public boolean hasManufacturer() { 1112 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1113 } 1114 1115 /** 1116 * @param value {@link #manufacturer} (Describes the details of the manufacturer 1117 * of the medication product. This is not intended to represent the 1118 * distributor of a medication product.) 1119 */ 1120 public Medication setManufacturer(Reference value) { 1121 this.manufacturer = value; 1122 return this; 1123 } 1124 1125 /** 1126 * @return {@link #manufacturer} The actual object that is the target of the 1127 * reference. The reference library doesn't populate this, but you can 1128 * use it to hold the resource if you resolve it. (Describes the details 1129 * of the manufacturer of the medication product. This is not intended 1130 * to represent the distributor of a medication product.) 1131 */ 1132 public Organization getManufacturerTarget() { 1133 if (this.manufacturerTarget == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create Medication.manufacturer"); 1136 else if (Configuration.doAutoCreate()) 1137 this.manufacturerTarget = new Organization(); // aa 1138 return this.manufacturerTarget; 1139 } 1140 1141 /** 1142 * @param value {@link #manufacturer} The actual object that is the target of 1143 * the reference. The reference library doesn't use these, but you 1144 * can use it to hold the resource if you resolve it. (Describes 1145 * the details of the manufacturer of the medication product. This 1146 * is not intended to represent the distributor of a medication 1147 * product.) 1148 */ 1149 public Medication setManufacturerTarget(Organization value) { 1150 this.manufacturerTarget = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return {@link #form} (Describes the form of the item. Powder; tablets; 1156 * capsule.) 1157 */ 1158 public CodeableConcept getForm() { 1159 if (this.form == null) 1160 if (Configuration.errorOnAutoCreate()) 1161 throw new Error("Attempt to auto-create Medication.form"); 1162 else if (Configuration.doAutoCreate()) 1163 this.form = new CodeableConcept(); // cc 1164 return this.form; 1165 } 1166 1167 public boolean hasForm() { 1168 return this.form != null && !this.form.isEmpty(); 1169 } 1170 1171 /** 1172 * @param value {@link #form} (Describes the form of the item. Powder; tablets; 1173 * capsule.) 1174 */ 1175 public Medication setForm(CodeableConcept value) { 1176 this.form = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #amount} (Specific amount of the drug in the packaged product. 1182 * For example, when specifying a product that has the same strength 1183 * (For example, Insulin glargine 100 unit per mL solution for 1184 * injection), this attribute provides additional clarification of the 1185 * package amount (For example, 3 mL, 10mL, etc.).) 1186 */ 1187 public Ratio getAmount() { 1188 if (this.amount == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create Medication.amount"); 1191 else if (Configuration.doAutoCreate()) 1192 this.amount = new Ratio(); // cc 1193 return this.amount; 1194 } 1195 1196 public boolean hasAmount() { 1197 return this.amount != null && !this.amount.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #amount} (Specific amount of the drug in the packaged 1202 * product. For example, when specifying a product that has the 1203 * same strength (For example, Insulin glargine 100 unit per mL 1204 * solution for injection), this attribute provides additional 1205 * clarification of the package amount (For example, 3 mL, 10mL, 1206 * etc.).) 1207 */ 1208 public Medication setAmount(Ratio value) { 1209 this.amount = value; 1210 return this; 1211 } 1212 1213 /** 1214 * @return {@link #ingredient} (Identifies a particular constituent of interest 1215 * in the product.) 1216 */ 1217 public List<MedicationIngredientComponent> getIngredient() { 1218 if (this.ingredient == null) 1219 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1220 return this.ingredient; 1221 } 1222 1223 /** 1224 * @return Returns a reference to <code>this</code> for easy method chaining 1225 */ 1226 public Medication setIngredient(List<MedicationIngredientComponent> theIngredient) { 1227 this.ingredient = theIngredient; 1228 return this; 1229 } 1230 1231 public boolean hasIngredient() { 1232 if (this.ingredient == null) 1233 return false; 1234 for (MedicationIngredientComponent item : this.ingredient) 1235 if (!item.isEmpty()) 1236 return true; 1237 return false; 1238 } 1239 1240 public MedicationIngredientComponent addIngredient() { // 3 1241 MedicationIngredientComponent t = new MedicationIngredientComponent(); 1242 if (this.ingredient == null) 1243 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1244 this.ingredient.add(t); 1245 return t; 1246 } 1247 1248 public Medication addIngredient(MedicationIngredientComponent t) { // 3 1249 if (t == null) 1250 return this; 1251 if (this.ingredient == null) 1252 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1253 this.ingredient.add(t); 1254 return this; 1255 } 1256 1257 /** 1258 * @return The first repetition of repeating field {@link #ingredient}, creating 1259 * it if it does not already exist 1260 */ 1261 public MedicationIngredientComponent getIngredientFirstRep() { 1262 if (getIngredient().isEmpty()) { 1263 addIngredient(); 1264 } 1265 return getIngredient().get(0); 1266 } 1267 1268 /** 1269 * @return {@link #batch} (Information that only applies to packages (not 1270 * products).) 1271 */ 1272 public MedicationBatchComponent getBatch() { 1273 if (this.batch == null) 1274 if (Configuration.errorOnAutoCreate()) 1275 throw new Error("Attempt to auto-create Medication.batch"); 1276 else if (Configuration.doAutoCreate()) 1277 this.batch = new MedicationBatchComponent(); // cc 1278 return this.batch; 1279 } 1280 1281 public boolean hasBatch() { 1282 return this.batch != null && !this.batch.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #batch} (Information that only applies to packages (not 1287 * products).) 1288 */ 1289 public Medication setBatch(MedicationBatchComponent value) { 1290 this.batch = value; 1291 return this; 1292 } 1293 1294 protected void listChildren(List<Property> children) { 1295 super.listChildren(children); 1296 children.add(new Property("identifier", "Identifier", "Business identifier for this medication.", 0, 1297 java.lang.Integer.MAX_VALUE, identifier)); 1298 children.add(new Property("code", "CodeableConcept", 1299 "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 1300 0, 1, code)); 1301 children 1302 .add(new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, status)); 1303 children.add(new Property("manufacturer", "Reference(Organization)", 1304 "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 1305 0, 1, manufacturer)); 1306 children.add(new Property("form", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 1307 0, 1, form)); 1308 children.add(new Property("amount", "Ratio", 1309 "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 1310 0, 1, amount)); 1311 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, 1312 java.lang.Integer.MAX_VALUE, ingredient)); 1313 children.add(new Property("batch", "", "Information that only applies to packages (not products).", 0, 1, batch)); 1314 } 1315 1316 @Override 1317 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1318 switch (_hash) { 1319 case -1618432855: 1320 /* identifier */ return new Property("identifier", "Identifier", "Business identifier for this medication.", 0, 1321 java.lang.Integer.MAX_VALUE, identifier); 1322 case 3059181: 1323 /* code */ return new Property("code", "CodeableConcept", 1324 "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 1325 0, 1, code); 1326 case -892481550: 1327 /* status */ return new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, 1328 status); 1329 case -1969347631: 1330 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 1331 "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 1332 0, 1, manufacturer); 1333 case 3148996: 1334 /* form */ return new Property("form", "CodeableConcept", 1335 "Describes the form of the item. Powder; tablets; capsule.", 0, 1, form); 1336 case -1413853096: 1337 /* amount */ return new Property("amount", "Ratio", 1338 "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 1339 0, 1, amount); 1340 case -206409263: 1341 /* ingredient */ return new Property("ingredient", "", 1342 "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, 1343 ingredient); 1344 case 93509434: 1345 /* batch */ return new Property("batch", "", "Information that only applies to packages (not products).", 0, 1, 1346 batch); 1347 default: 1348 return super.getNamedProperty(_hash, _name, _checkValid); 1349 } 1350 1351 } 1352 1353 @Override 1354 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1355 switch (hash) { 1356 case -1618432855: 1357 /* identifier */ return this.identifier == null ? new Base[0] 1358 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1359 case 3059181: 1360 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1361 case -892481550: 1362 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationStatus> 1363 case -1969347631: 1364 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // Reference 1365 case 3148996: 1366 /* form */ return this.form == null ? new Base[0] : new Base[] { this.form }; // CodeableConcept 1367 case -1413853096: 1368 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Ratio 1369 case -206409263: 1370 /* ingredient */ return this.ingredient == null ? new Base[0] 1371 : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationIngredientComponent 1372 case 93509434: 1373 /* batch */ return this.batch == null ? new Base[0] : new Base[] { this.batch }; // MedicationBatchComponent 1374 default: 1375 return super.getProperty(hash, name, checkValid); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1382 switch (hash) { 1383 case -1618432855: // identifier 1384 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1385 return value; 1386 case 3059181: // code 1387 this.code = castToCodeableConcept(value); // CodeableConcept 1388 return value; 1389 case -892481550: // status 1390 value = new MedicationStatusEnumFactory().fromType(castToCode(value)); 1391 this.status = (Enumeration) value; // Enumeration<MedicationStatus> 1392 return value; 1393 case -1969347631: // manufacturer 1394 this.manufacturer = castToReference(value); // Reference 1395 return value; 1396 case 3148996: // form 1397 this.form = castToCodeableConcept(value); // CodeableConcept 1398 return value; 1399 case -1413853096: // amount 1400 this.amount = castToRatio(value); // Ratio 1401 return value; 1402 case -206409263: // ingredient 1403 this.getIngredient().add((MedicationIngredientComponent) value); // MedicationIngredientComponent 1404 return value; 1405 case 93509434: // batch 1406 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1407 return value; 1408 default: 1409 return super.setProperty(hash, name, value); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base setProperty(String name, Base value) throws FHIRException { 1416 if (name.equals("identifier")) { 1417 this.getIdentifier().add(castToIdentifier(value)); 1418 } else if (name.equals("code")) { 1419 this.code = castToCodeableConcept(value); // CodeableConcept 1420 } else if (name.equals("status")) { 1421 value = new MedicationStatusEnumFactory().fromType(castToCode(value)); 1422 this.status = (Enumeration) value; // Enumeration<MedicationStatus> 1423 } else if (name.equals("manufacturer")) { 1424 this.manufacturer = castToReference(value); // Reference 1425 } else if (name.equals("form")) { 1426 this.form = castToCodeableConcept(value); // CodeableConcept 1427 } else if (name.equals("amount")) { 1428 this.amount = castToRatio(value); // Ratio 1429 } else if (name.equals("ingredient")) { 1430 this.getIngredient().add((MedicationIngredientComponent) value); 1431 } else if (name.equals("batch")) { 1432 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1433 } else 1434 return super.setProperty(name, value); 1435 return value; 1436 } 1437 1438 @Override 1439 public void removeChild(String name, Base value) throws FHIRException { 1440 if (name.equals("identifier")) { 1441 this.getIdentifier().remove(castToIdentifier(value)); 1442 } else if (name.equals("code")) { 1443 this.code = null; 1444 } else if (name.equals("status")) { 1445 this.status = null; 1446 } else if (name.equals("manufacturer")) { 1447 this.manufacturer = null; 1448 } else if (name.equals("form")) { 1449 this.form = null; 1450 } else if (name.equals("amount")) { 1451 this.amount = null; 1452 } else if (name.equals("ingredient")) { 1453 this.getIngredient().remove((MedicationIngredientComponent) value); 1454 } else if (name.equals("batch")) { 1455 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1456 } else 1457 super.removeChild(name, value); 1458 1459 } 1460 1461 @Override 1462 public Base makeProperty(int hash, String name) throws FHIRException { 1463 switch (hash) { 1464 case -1618432855: 1465 return addIdentifier(); 1466 case 3059181: 1467 return getCode(); 1468 case -892481550: 1469 return getStatusElement(); 1470 case -1969347631: 1471 return getManufacturer(); 1472 case 3148996: 1473 return getForm(); 1474 case -1413853096: 1475 return getAmount(); 1476 case -206409263: 1477 return addIngredient(); 1478 case 93509434: 1479 return getBatch(); 1480 default: 1481 return super.makeProperty(hash, name); 1482 } 1483 1484 } 1485 1486 @Override 1487 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1488 switch (hash) { 1489 case -1618432855: 1490 /* identifier */ return new String[] { "Identifier" }; 1491 case 3059181: 1492 /* code */ return new String[] { "CodeableConcept" }; 1493 case -892481550: 1494 /* status */ return new String[] { "code" }; 1495 case -1969347631: 1496 /* manufacturer */ return new String[] { "Reference" }; 1497 case 3148996: 1498 /* form */ return new String[] { "CodeableConcept" }; 1499 case -1413853096: 1500 /* amount */ return new String[] { "Ratio" }; 1501 case -206409263: 1502 /* ingredient */ return new String[] {}; 1503 case 93509434: 1504 /* batch */ return new String[] {}; 1505 default: 1506 return super.getTypesForProperty(hash, name); 1507 } 1508 1509 } 1510 1511 @Override 1512 public Base addChild(String name) throws FHIRException { 1513 if (name.equals("identifier")) { 1514 return addIdentifier(); 1515 } else if (name.equals("code")) { 1516 this.code = new CodeableConcept(); 1517 return this.code; 1518 } else if (name.equals("status")) { 1519 throw new FHIRException("Cannot call addChild on a singleton property Medication.status"); 1520 } else if (name.equals("manufacturer")) { 1521 this.manufacturer = new Reference(); 1522 return this.manufacturer; 1523 } else if (name.equals("form")) { 1524 this.form = new CodeableConcept(); 1525 return this.form; 1526 } else if (name.equals("amount")) { 1527 this.amount = new Ratio(); 1528 return this.amount; 1529 } else if (name.equals("ingredient")) { 1530 return addIngredient(); 1531 } else if (name.equals("batch")) { 1532 this.batch = new MedicationBatchComponent(); 1533 return this.batch; 1534 } else 1535 return super.addChild(name); 1536 } 1537 1538 public String fhirType() { 1539 return "Medication"; 1540 1541 } 1542 1543 public Medication copy() { 1544 Medication dst = new Medication(); 1545 copyValues(dst); 1546 return dst; 1547 } 1548 1549 public void copyValues(Medication dst) { 1550 super.copyValues(dst); 1551 if (identifier != null) { 1552 dst.identifier = new ArrayList<Identifier>(); 1553 for (Identifier i : identifier) 1554 dst.identifier.add(i.copy()); 1555 } 1556 ; 1557 dst.code = code == null ? null : code.copy(); 1558 dst.status = status == null ? null : status.copy(); 1559 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 1560 dst.form = form == null ? null : form.copy(); 1561 dst.amount = amount == null ? null : amount.copy(); 1562 if (ingredient != null) { 1563 dst.ingredient = new ArrayList<MedicationIngredientComponent>(); 1564 for (MedicationIngredientComponent i : ingredient) 1565 dst.ingredient.add(i.copy()); 1566 } 1567 ; 1568 dst.batch = batch == null ? null : batch.copy(); 1569 } 1570 1571 protected Medication typedCopy() { 1572 return copy(); 1573 } 1574 1575 @Override 1576 public boolean equalsDeep(Base other_) { 1577 if (!super.equalsDeep(other_)) 1578 return false; 1579 if (!(other_ instanceof Medication)) 1580 return false; 1581 Medication o = (Medication) other_; 1582 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 1583 && compareDeep(status, o.status, true) && compareDeep(manufacturer, o.manufacturer, true) 1584 && compareDeep(form, o.form, true) && compareDeep(amount, o.amount, true) 1585 && compareDeep(ingredient, o.ingredient, true) && compareDeep(batch, o.batch, true); 1586 } 1587 1588 @Override 1589 public boolean equalsShallow(Base other_) { 1590 if (!super.equalsShallow(other_)) 1591 return false; 1592 if (!(other_ instanceof Medication)) 1593 return false; 1594 Medication o = (Medication) other_; 1595 return compareValues(status, o.status, true); 1596 } 1597 1598 public boolean isEmpty() { 1599 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, status, manufacturer, form, amount, 1600 ingredient, batch); 1601 } 1602 1603 @Override 1604 public ResourceType getResourceType() { 1605 return ResourceType.Medication; 1606 } 1607 1608 /** 1609 * Search parameter: <b>ingredient-code</b> 1610 * <p> 1611 * Description: <b>Returns medications for this ingredient code</b><br> 1612 * Type: <b>token</b><br> 1613 * Path: <b>Medication.ingredient.itemCodeableConcept</b><br> 1614 * </p> 1615 */ 1616 @SearchParamDefinition(name = "ingredient-code", path = "(Medication.ingredient.item as CodeableConcept)", description = "Returns medications for this ingredient code", type = "token") 1617 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 1618 /** 1619 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 1620 * <p> 1621 * Description: <b>Returns medications for this ingredient code</b><br> 1622 * Type: <b>token</b><br> 1623 * Path: <b>Medication.ingredient.itemCodeableConcept</b><br> 1624 * </p> 1625 */ 1626 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1627 SP_INGREDIENT_CODE); 1628 1629 /** 1630 * Search parameter: <b>identifier</b> 1631 * <p> 1632 * Description: <b>Returns medications with this external identifier</b><br> 1633 * Type: <b>token</b><br> 1634 * Path: <b>Medication.identifier</b><br> 1635 * </p> 1636 */ 1637 @SearchParamDefinition(name = "identifier", path = "Medication.identifier", description = "Returns medications with this external identifier", type = "token") 1638 public static final String SP_IDENTIFIER = "identifier"; 1639 /** 1640 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1641 * <p> 1642 * Description: <b>Returns medications with this external identifier</b><br> 1643 * Type: <b>token</b><br> 1644 * Path: <b>Medication.identifier</b><br> 1645 * </p> 1646 */ 1647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1648 SP_IDENTIFIER); 1649 1650 /** 1651 * Search parameter: <b>code</b> 1652 * <p> 1653 * Description: <b>Returns medications for a specific code</b><br> 1654 * Type: <b>token</b><br> 1655 * Path: <b>Medication.code</b><br> 1656 * </p> 1657 */ 1658 @SearchParamDefinition(name = "code", path = "Medication.code", description = "Returns medications for a specific code", type = "token") 1659 public static final String SP_CODE = "code"; 1660 /** 1661 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1662 * <p> 1663 * Description: <b>Returns medications for a specific code</b><br> 1664 * Type: <b>token</b><br> 1665 * Path: <b>Medication.code</b><br> 1666 * </p> 1667 */ 1668 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1669 SP_CODE); 1670 1671 /** 1672 * Search parameter: <b>ingredient</b> 1673 * <p> 1674 * Description: <b>Returns medications for this ingredient reference</b><br> 1675 * Type: <b>reference</b><br> 1676 * Path: <b>Medication.ingredient.itemReference</b><br> 1677 * </p> 1678 */ 1679 @SearchParamDefinition(name = "ingredient", path = "(Medication.ingredient.item as Reference)", description = "Returns medications for this ingredient reference", type = "reference", target = { 1680 Medication.class, Substance.class }) 1681 public static final String SP_INGREDIENT = "ingredient"; 1682 /** 1683 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 1684 * <p> 1685 * Description: <b>Returns medications for this ingredient reference</b><br> 1686 * Type: <b>reference</b><br> 1687 * Path: <b>Medication.ingredient.itemReference</b><br> 1688 * </p> 1689 */ 1690 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1691 SP_INGREDIENT); 1692 1693 /** 1694 * Constant for fluent queries to be used to add include statements. Specifies 1695 * the path value of "<b>Medication:ingredient</b>". 1696 */ 1697 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include( 1698 "Medication:ingredient").toLocked(); 1699 1700 /** 1701 * Search parameter: <b>form</b> 1702 * <p> 1703 * Description: <b>Returns medications for a specific dose form</b><br> 1704 * Type: <b>token</b><br> 1705 * Path: <b>Medication.form</b><br> 1706 * </p> 1707 */ 1708 @SearchParamDefinition(name = "form", path = "Medication.form", description = "Returns medications for a specific dose form", type = "token") 1709 public static final String SP_FORM = "form"; 1710 /** 1711 * <b>Fluent Client</b> search parameter constant for <b>form</b> 1712 * <p> 1713 * Description: <b>Returns medications for a specific dose form</b><br> 1714 * Type: <b>token</b><br> 1715 * Path: <b>Medication.form</b><br> 1716 * </p> 1717 */ 1718 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1719 SP_FORM); 1720 1721 /** 1722 * Search parameter: <b>lot-number</b> 1723 * <p> 1724 * Description: <b>Returns medications in a batch with this lot number</b><br> 1725 * Type: <b>token</b><br> 1726 * Path: <b>Medication.batch.lotNumber</b><br> 1727 * </p> 1728 */ 1729 @SearchParamDefinition(name = "lot-number", path = "Medication.batch.lotNumber", description = "Returns medications in a batch with this lot number", type = "token") 1730 public static final String SP_LOT_NUMBER = "lot-number"; 1731 /** 1732 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 1733 * <p> 1734 * Description: <b>Returns medications in a batch with this lot number</b><br> 1735 * Type: <b>token</b><br> 1736 * Path: <b>Medication.batch.lotNumber</b><br> 1737 * </p> 1738 */ 1739 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1740 SP_LOT_NUMBER); 1741 1742 /** 1743 * Search parameter: <b>expiration-date</b> 1744 * <p> 1745 * Description: <b>Returns medications in a batch with this expiration 1746 * date</b><br> 1747 * Type: <b>date</b><br> 1748 * Path: <b>Medication.batch.expirationDate</b><br> 1749 * </p> 1750 */ 1751 @SearchParamDefinition(name = "expiration-date", path = "Medication.batch.expirationDate", description = "Returns medications in a batch with this expiration date", type = "date") 1752 public static final String SP_EXPIRATION_DATE = "expiration-date"; 1753 /** 1754 * <b>Fluent Client</b> search parameter constant for <b>expiration-date</b> 1755 * <p> 1756 * Description: <b>Returns medications in a batch with this expiration 1757 * date</b><br> 1758 * Type: <b>date</b><br> 1759 * Path: <b>Medication.batch.expirationDate</b><br> 1760 * </p> 1761 */ 1762 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRATION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1763 SP_EXPIRATION_DATE); 1764 1765 /** 1766 * Search parameter: <b>manufacturer</b> 1767 * <p> 1768 * Description: <b>Returns medications made or sold for this 1769 * manufacturer</b><br> 1770 * Type: <b>reference</b><br> 1771 * Path: <b>Medication.manufacturer</b><br> 1772 * </p> 1773 */ 1774 @SearchParamDefinition(name = "manufacturer", path = "Medication.manufacturer", description = "Returns medications made or sold for this manufacturer", type = "reference", target = { 1775 Organization.class }) 1776 public static final String SP_MANUFACTURER = "manufacturer"; 1777 /** 1778 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 1779 * <p> 1780 * Description: <b>Returns medications made or sold for this 1781 * manufacturer</b><br> 1782 * Type: <b>reference</b><br> 1783 * Path: <b>Medication.manufacturer</b><br> 1784 * </p> 1785 */ 1786 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1787 SP_MANUFACTURER); 1788 1789 /** 1790 * Constant for fluent queries to be used to add include statements. Specifies 1791 * the path value of "<b>Medication:manufacturer</b>". 1792 */ 1793 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include( 1794 "Medication:manufacturer").toLocked(); 1795 1796 /** 1797 * Search parameter: <b>status</b> 1798 * <p> 1799 * Description: <b>Returns medications for this status</b><br> 1800 * Type: <b>token</b><br> 1801 * Path: <b>Medication.status</b><br> 1802 * </p> 1803 */ 1804 @SearchParamDefinition(name = "status", path = "Medication.status", description = "Returns medications for this status", type = "token") 1805 public static final String SP_STATUS = "status"; 1806 /** 1807 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1808 * <p> 1809 * Description: <b>Returns medications for this status</b><br> 1810 * Type: <b>token</b><br> 1811 * Path: <b>Medication.status</b><br> 1812 * </p> 1813 */ 1814 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1815 SP_STATUS); 1816 1817}