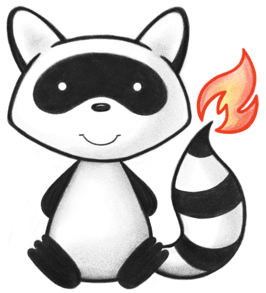
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Describes the event of a patient consuming or otherwise being administered a 048 * medication. This may be as simple as swallowing a tablet or it may be a long 049 * running infusion. Related resources tie this event to the authorizing 050 * prescription, and the specific encounter between patient and health care 051 * practitioner. 052 */ 053@ResourceDef(name = "MedicationAdministration", profile = "http://hl7.org/fhir/StructureDefinition/MedicationAdministration") 054public class MedicationAdministration extends DomainResource { 055 056 public enum MedicationAdministrationStatus { 057 /** 058 * The administration has started but has not yet completed. 059 */ 060 INPROGRESS, 061 /** 062 * The administration was terminated prior to any impact on the subject (though 063 * preparatory actions may have been taken) 064 */ 065 NOTDONE, 066 /** 067 * Actions implied by the administration have been temporarily halted, but are 068 * expected to continue later. May also be called 'suspended'. 069 */ 070 ONHOLD, 071 /** 072 * All actions that are implied by the administration have occurred. 073 */ 074 COMPLETED, 075 /** 076 * The administration was entered in error and therefore nullified. 077 */ 078 ENTEREDINERROR, 079 /** 080 * Actions implied by the administration have been permanently halted, before 081 * all of them occurred. 082 */ 083 STOPPED, 084 /** 085 * The authoring system does not know which of the status values currently 086 * applies for this request. Note: This concept is not to be used for 'other' - 087 * one of the listed statuses is presumed to apply, it's just not known which 088 * one. 089 */ 090 UNKNOWN, 091 /** 092 * added to help the parsers with the generic types 093 */ 094 NULL; 095 096 public static MedicationAdministrationStatus fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("in-progress".equals(codeString)) 100 return INPROGRESS; 101 if ("not-done".equals(codeString)) 102 return NOTDONE; 103 if ("on-hold".equals(codeString)) 104 return ONHOLD; 105 if ("completed".equals(codeString)) 106 return COMPLETED; 107 if ("entered-in-error".equals(codeString)) 108 return ENTEREDINERROR; 109 if ("stopped".equals(codeString)) 110 return STOPPED; 111 if ("unknown".equals(codeString)) 112 return UNKNOWN; 113 if (Configuration.isAcceptInvalidEnums()) 114 return null; 115 else 116 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case INPROGRESS: 122 return "in-progress"; 123 case NOTDONE: 124 return "not-done"; 125 case ONHOLD: 126 return "on-hold"; 127 case COMPLETED: 128 return "completed"; 129 case ENTEREDINERROR: 130 return "entered-in-error"; 131 case STOPPED: 132 return "stopped"; 133 case UNKNOWN: 134 return "unknown"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getSystem() { 143 switch (this) { 144 case INPROGRESS: 145 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 146 case NOTDONE: 147 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 148 case ONHOLD: 149 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 150 case COMPLETED: 151 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 152 case ENTEREDINERROR: 153 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 154 case STOPPED: 155 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 156 case UNKNOWN: 157 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDefinition() { 166 switch (this) { 167 case INPROGRESS: 168 return "The administration has started but has not yet completed."; 169 case NOTDONE: 170 return "The administration was terminated prior to any impact on the subject (though preparatory actions may have been taken)"; 171 case ONHOLD: 172 return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called 'suspended'."; 173 case COMPLETED: 174 return "All actions that are implied by the administration have occurred."; 175 case ENTEREDINERROR: 176 return "The administration was entered in error and therefore nullified."; 177 case STOPPED: 178 return "Actions implied by the administration have been permanently halted, before all of them occurred."; 179 case UNKNOWN: 180 return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, it's just not known which one."; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188 public String getDisplay() { 189 switch (this) { 190 case INPROGRESS: 191 return "In Progress"; 192 case NOTDONE: 193 return "Not Done"; 194 case ONHOLD: 195 return "On Hold"; 196 case COMPLETED: 197 return "Completed"; 198 case ENTEREDINERROR: 199 return "Entered in Error"; 200 case STOPPED: 201 return "Stopped"; 202 case UNKNOWN: 203 return "Unknown"; 204 case NULL: 205 return null; 206 default: 207 return "?"; 208 } 209 } 210 } 211 212 public static class MedicationAdministrationStatusEnumFactory implements EnumFactory<MedicationAdministrationStatus> { 213 public MedicationAdministrationStatus fromCode(String codeString) throws IllegalArgumentException { 214 if (codeString == null || "".equals(codeString)) 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("in-progress".equals(codeString)) 218 return MedicationAdministrationStatus.INPROGRESS; 219 if ("not-done".equals(codeString)) 220 return MedicationAdministrationStatus.NOTDONE; 221 if ("on-hold".equals(codeString)) 222 return MedicationAdministrationStatus.ONHOLD; 223 if ("completed".equals(codeString)) 224 return MedicationAdministrationStatus.COMPLETED; 225 if ("entered-in-error".equals(codeString)) 226 return MedicationAdministrationStatus.ENTEREDINERROR; 227 if ("stopped".equals(codeString)) 228 return MedicationAdministrationStatus.STOPPED; 229 if ("unknown".equals(codeString)) 230 return MedicationAdministrationStatus.UNKNOWN; 231 throw new IllegalArgumentException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 232 } 233 234 public Enumeration<MedicationAdministrationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.NULL, code); 239 String codeString = code.asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.NULL, code); 242 if ("in-progress".equals(codeString)) 243 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.INPROGRESS, code); 244 if ("not-done".equals(codeString)) 245 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.NOTDONE, code); 246 if ("on-hold".equals(codeString)) 247 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ONHOLD, code); 248 if ("completed".equals(codeString)) 249 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.COMPLETED, code); 250 if ("entered-in-error".equals(codeString)) 251 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ENTEREDINERROR, 252 code); 253 if ("stopped".equals(codeString)) 254 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.STOPPED, code); 255 if ("unknown".equals(codeString)) 256 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.UNKNOWN, code); 257 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 258 } 259 260 public String toCode(MedicationAdministrationStatus code) { 261 if (code == MedicationAdministrationStatus.NULL) 262 return null; 263 if (code == MedicationAdministrationStatus.INPROGRESS) 264 return "in-progress"; 265 if (code == MedicationAdministrationStatus.NOTDONE) 266 return "not-done"; 267 if (code == MedicationAdministrationStatus.ONHOLD) 268 return "on-hold"; 269 if (code == MedicationAdministrationStatus.COMPLETED) 270 return "completed"; 271 if (code == MedicationAdministrationStatus.ENTEREDINERROR) 272 return "entered-in-error"; 273 if (code == MedicationAdministrationStatus.STOPPED) 274 return "stopped"; 275 if (code == MedicationAdministrationStatus.UNKNOWN) 276 return "unknown"; 277 return "?"; 278 } 279 280 public String toSystem(MedicationAdministrationStatus code) { 281 return code.getSystem(); 282 } 283 } 284 285 @Block() 286 public static class MedicationAdministrationPerformerComponent extends BackboneElement 287 implements IBaseBackboneElement { 288 /** 289 * Distinguishes the type of involvement of the performer in the medication 290 * administration. 291 */ 292 @Child(name = "function", type = { 293 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 294 @Description(shortDefinition = "Type of performance", formalDefinition = "Distinguishes the type of involvement of the performer in the medication administration.") 295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/med-admin-perform-function") 296 protected CodeableConcept function; 297 298 /** 299 * Indicates who or what performed the medication administration. 300 */ 301 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, 302 Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 303 @Description(shortDefinition = "Who performed the medication administration", formalDefinition = "Indicates who or what performed the medication administration.") 304 protected Reference actor; 305 306 /** 307 * The actual object that is the target of the reference (Indicates who or what 308 * performed the medication administration.) 309 */ 310 protected Resource actorTarget; 311 312 private static final long serialVersionUID = 1424001049L; 313 314 /** 315 * Constructor 316 */ 317 public MedicationAdministrationPerformerComponent() { 318 super(); 319 } 320 321 /** 322 * Constructor 323 */ 324 public MedicationAdministrationPerformerComponent(Reference actor) { 325 super(); 326 this.actor = actor; 327 } 328 329 /** 330 * @return {@link #function} (Distinguishes the type of involvement of the 331 * performer in the medication administration.) 332 */ 333 public CodeableConcept getFunction() { 334 if (this.function == null) 335 if (Configuration.errorOnAutoCreate()) 336 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.function"); 337 else if (Configuration.doAutoCreate()) 338 this.function = new CodeableConcept(); // cc 339 return this.function; 340 } 341 342 public boolean hasFunction() { 343 return this.function != null && !this.function.isEmpty(); 344 } 345 346 /** 347 * @param value {@link #function} (Distinguishes the type of involvement of the 348 * performer in the medication administration.) 349 */ 350 public MedicationAdministrationPerformerComponent setFunction(CodeableConcept value) { 351 this.function = value; 352 return this; 353 } 354 355 /** 356 * @return {@link #actor} (Indicates who or what performed the medication 357 * administration.) 358 */ 359 public Reference getActor() { 360 if (this.actor == null) 361 if (Configuration.errorOnAutoCreate()) 362 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.actor"); 363 else if (Configuration.doAutoCreate()) 364 this.actor = new Reference(); // cc 365 return this.actor; 366 } 367 368 public boolean hasActor() { 369 return this.actor != null && !this.actor.isEmpty(); 370 } 371 372 /** 373 * @param value {@link #actor} (Indicates who or what performed the medication 374 * administration.) 375 */ 376 public MedicationAdministrationPerformerComponent setActor(Reference value) { 377 this.actor = value; 378 return this; 379 } 380 381 /** 382 * @return {@link #actor} The actual object that is the target of the reference. 383 * The reference library doesn't populate this, but you can use it to 384 * hold the resource if you resolve it. (Indicates who or what performed 385 * the medication administration.) 386 */ 387 public Resource getActorTarget() { 388 return this.actorTarget; 389 } 390 391 /** 392 * @param value {@link #actor} The actual object that is the target of the 393 * reference. The reference library doesn't use these, but you can 394 * use it to hold the resource if you resolve it. (Indicates who or 395 * what performed the medication administration.) 396 */ 397 public MedicationAdministrationPerformerComponent setActorTarget(Resource value) { 398 this.actorTarget = value; 399 return this; 400 } 401 402 protected void listChildren(List<Property> children) { 403 super.listChildren(children); 404 children.add(new Property("function", "CodeableConcept", 405 "Distinguishes the type of involvement of the performer in the medication administration.", 0, 1, function)); 406 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device)", 407 "Indicates who or what performed the medication administration.", 0, 1, actor)); 408 } 409 410 @Override 411 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 412 switch (_hash) { 413 case 1380938712: 414 /* function */ return new Property("function", "CodeableConcept", 415 "Distinguishes the type of involvement of the performer in the medication administration.", 0, 1, function); 416 case 92645877: 417 /* actor */ return new Property("actor", 418 "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device)", 419 "Indicates who or what performed the medication administration.", 0, 1, actor); 420 default: 421 return super.getNamedProperty(_hash, _name, _checkValid); 422 } 423 424 } 425 426 @Override 427 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 428 switch (hash) { 429 case 1380938712: 430 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 431 case 92645877: 432 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 433 default: 434 return super.getProperty(hash, name, checkValid); 435 } 436 437 } 438 439 @Override 440 public Base setProperty(int hash, String name, Base value) throws FHIRException { 441 switch (hash) { 442 case 1380938712: // function 443 this.function = castToCodeableConcept(value); // CodeableConcept 444 return value; 445 case 92645877: // actor 446 this.actor = castToReference(value); // Reference 447 return value; 448 default: 449 return super.setProperty(hash, name, value); 450 } 451 452 } 453 454 @Override 455 public Base setProperty(String name, Base value) throws FHIRException { 456 if (name.equals("function")) { 457 this.function = castToCodeableConcept(value); // CodeableConcept 458 } else if (name.equals("actor")) { 459 this.actor = castToReference(value); // Reference 460 } else 461 return super.setProperty(name, value); 462 return value; 463 } 464 465 @Override 466 public void removeChild(String name, Base value) throws FHIRException { 467 if (name.equals("function")) { 468 this.function = null; 469 } else if (name.equals("actor")) { 470 this.actor = null; 471 } else 472 super.removeChild(name, value); 473 474 } 475 476 @Override 477 public Base makeProperty(int hash, String name) throws FHIRException { 478 switch (hash) { 479 case 1380938712: 480 return getFunction(); 481 case 92645877: 482 return getActor(); 483 default: 484 return super.makeProperty(hash, name); 485 } 486 487 } 488 489 @Override 490 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 491 switch (hash) { 492 case 1380938712: 493 /* function */ return new String[] { "CodeableConcept" }; 494 case 92645877: 495 /* actor */ return new String[] { "Reference" }; 496 default: 497 return super.getTypesForProperty(hash, name); 498 } 499 500 } 501 502 @Override 503 public Base addChild(String name) throws FHIRException { 504 if (name.equals("function")) { 505 this.function = new CodeableConcept(); 506 return this.function; 507 } else if (name.equals("actor")) { 508 this.actor = new Reference(); 509 return this.actor; 510 } else 511 return super.addChild(name); 512 } 513 514 public MedicationAdministrationPerformerComponent copy() { 515 MedicationAdministrationPerformerComponent dst = new MedicationAdministrationPerformerComponent(); 516 copyValues(dst); 517 return dst; 518 } 519 520 public void copyValues(MedicationAdministrationPerformerComponent dst) { 521 super.copyValues(dst); 522 dst.function = function == null ? null : function.copy(); 523 dst.actor = actor == null ? null : actor.copy(); 524 } 525 526 @Override 527 public boolean equalsDeep(Base other_) { 528 if (!super.equalsDeep(other_)) 529 return false; 530 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 531 return false; 532 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 533 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 534 } 535 536 @Override 537 public boolean equalsShallow(Base other_) { 538 if (!super.equalsShallow(other_)) 539 return false; 540 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 541 return false; 542 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 543 return true; 544 } 545 546 public boolean isEmpty() { 547 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 548 } 549 550 public String fhirType() { 551 return "MedicationAdministration.performer"; 552 553 } 554 555 } 556 557 @Block() 558 public static class MedicationAdministrationDosageComponent extends BackboneElement implements IBaseBackboneElement { 559 /** 560 * Free text dosage can be used for cases where the dosage administered is too 561 * complex to code. When coded dosage is present, the free text dosage may still 562 * be present for display to humans. 563 * 564 * The dosage instructions should reflect the dosage of the medication that was 565 * administered. 566 */ 567 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 568 @Description(shortDefinition = "Free text dosage instructions e.g. SIG", formalDefinition = "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.") 569 protected StringType text; 570 571 /** 572 * A coded specification of the anatomic site where the medication first entered 573 * the body. For example, "left arm". 574 */ 575 @Child(name = "site", type = { 576 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 577 @Description(shortDefinition = "Body site administered to", formalDefinition = "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".") 578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/approach-site-codes") 579 protected CodeableConcept site; 580 581 /** 582 * A code specifying the route or physiological path of administration of a 583 * therapeutic agent into or onto the patient. For example, topical, 584 * intravenous, etc. 585 */ 586 @Child(name = "route", type = { 587 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 588 @Description(shortDefinition = "Path of substance into body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.") 589 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/route-codes") 590 protected CodeableConcept route; 591 592 /** 593 * A coded value indicating the method by which the medication is intended to be 594 * or was introduced into or on the body. This attribute will most often NOT be 595 * populated. It is most commonly used for injections. For example, Slow Push, 596 * Deep IV. 597 */ 598 @Child(name = "method", type = { 599 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 600 @Description(shortDefinition = "How drug was administered", formalDefinition = "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.") 601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administration-method-codes") 602 protected CodeableConcept method; 603 604 /** 605 * The amount of the medication given at one administration event. Use this 606 * value when the administration is essentially an instantaneous event such as a 607 * swallowing a tablet or giving an injection. 608 */ 609 @Child(name = "dose", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 610 @Description(shortDefinition = "Amount of medication per dose", formalDefinition = "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.") 611 protected Quantity dose; 612 613 /** 614 * Identifies the speed with which the medication was or will be introduced into 615 * the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 616 * 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 617 * 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours. 618 */ 619 @Child(name = "rate", type = { Ratio.class, 620 Quantity.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 621 @Description(shortDefinition = "Dose quantity per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 622 protected Type rate; 623 624 private static final long serialVersionUID = 947835626L; 625 626 /** 627 * Constructor 628 */ 629 public MedicationAdministrationDosageComponent() { 630 super(); 631 } 632 633 /** 634 * @return {@link #text} (Free text dosage can be used for cases where the 635 * dosage administered is too complex to code. When coded dosage is 636 * present, the free text dosage may still be present for display to 637 * humans. 638 * 639 * The dosage instructions should reflect the dosage of the medication 640 * that was administered.). This is the underlying object with id, value 641 * and extensions. The accessor "getText" gives direct access to the 642 * value 643 */ 644 public StringType getTextElement() { 645 if (this.text == null) 646 if (Configuration.errorOnAutoCreate()) 647 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.text"); 648 else if (Configuration.doAutoCreate()) 649 this.text = new StringType(); // bb 650 return this.text; 651 } 652 653 public boolean hasTextElement() { 654 return this.text != null && !this.text.isEmpty(); 655 } 656 657 public boolean hasText() { 658 return this.text != null && !this.text.isEmpty(); 659 } 660 661 /** 662 * @param value {@link #text} (Free text dosage can be used for cases where the 663 * dosage administered is too complex to code. When coded dosage is 664 * present, the free text dosage may still be present for display 665 * to humans. 666 * 667 * The dosage instructions should reflect the dosage of the 668 * medication that was administered.). This is the underlying 669 * object with id, value and extensions. The accessor "getText" 670 * gives direct access to the value 671 */ 672 public MedicationAdministrationDosageComponent setTextElement(StringType value) { 673 this.text = value; 674 return this; 675 } 676 677 /** 678 * @return Free text dosage can be used for cases where the dosage administered 679 * is too complex to code. When coded dosage is present, the free text 680 * dosage may still be present for display to humans. 681 * 682 * The dosage instructions should reflect the dosage of the medication 683 * that was administered. 684 */ 685 public String getText() { 686 return this.text == null ? null : this.text.getValue(); 687 } 688 689 /** 690 * @param value Free text dosage can be used for cases where the dosage 691 * administered is too complex to code. When coded dosage is 692 * present, the free text dosage may still be present for display 693 * to humans. 694 * 695 * The dosage instructions should reflect the dosage of the 696 * medication that was administered. 697 */ 698 public MedicationAdministrationDosageComponent setText(String value) { 699 if (Utilities.noString(value)) 700 this.text = null; 701 else { 702 if (this.text == null) 703 this.text = new StringType(); 704 this.text.setValue(value); 705 } 706 return this; 707 } 708 709 /** 710 * @return {@link #site} (A coded specification of the anatomic site where the 711 * medication first entered the body. For example, "left arm".) 712 */ 713 public CodeableConcept getSite() { 714 if (this.site == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.site"); 717 else if (Configuration.doAutoCreate()) 718 this.site = new CodeableConcept(); // cc 719 return this.site; 720 } 721 722 public boolean hasSite() { 723 return this.site != null && !this.site.isEmpty(); 724 } 725 726 /** 727 * @param value {@link #site} (A coded specification of the anatomic site where 728 * the medication first entered the body. For example, "left arm".) 729 */ 730 public MedicationAdministrationDosageComponent setSite(CodeableConcept value) { 731 this.site = value; 732 return this; 733 } 734 735 /** 736 * @return {@link #route} (A code specifying the route or physiological path of 737 * administration of a therapeutic agent into or onto the patient. For 738 * example, topical, intravenous, etc.) 739 */ 740 public CodeableConcept getRoute() { 741 if (this.route == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.route"); 744 else if (Configuration.doAutoCreate()) 745 this.route = new CodeableConcept(); // cc 746 return this.route; 747 } 748 749 public boolean hasRoute() { 750 return this.route != null && !this.route.isEmpty(); 751 } 752 753 /** 754 * @param value {@link #route} (A code specifying the route or physiological 755 * path of administration of a therapeutic agent into or onto the 756 * patient. For example, topical, intravenous, etc.) 757 */ 758 public MedicationAdministrationDosageComponent setRoute(CodeableConcept value) { 759 this.route = value; 760 return this; 761 } 762 763 /** 764 * @return {@link #method} (A coded value indicating the method by which the 765 * medication is intended to be or was introduced into or on the body. 766 * This attribute will most often NOT be populated. It is most commonly 767 * used for injections. For example, Slow Push, Deep IV.) 768 */ 769 public CodeableConcept getMethod() { 770 if (this.method == null) 771 if (Configuration.errorOnAutoCreate()) 772 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.method"); 773 else if (Configuration.doAutoCreate()) 774 this.method = new CodeableConcept(); // cc 775 return this.method; 776 } 777 778 public boolean hasMethod() { 779 return this.method != null && !this.method.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #method} (A coded value indicating the method by which 784 * the medication is intended to be or was introduced into or on 785 * the body. This attribute will most often NOT be populated. It is 786 * most commonly used for injections. For example, Slow Push, Deep 787 * IV.) 788 */ 789 public MedicationAdministrationDosageComponent setMethod(CodeableConcept value) { 790 this.method = value; 791 return this; 792 } 793 794 /** 795 * @return {@link #dose} (The amount of the medication given at one 796 * administration event. Use this value when the administration is 797 * essentially an instantaneous event such as a swallowing a tablet or 798 * giving an injection.) 799 */ 800 public Quantity getDose() { 801 if (this.dose == null) 802 if (Configuration.errorOnAutoCreate()) 803 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.dose"); 804 else if (Configuration.doAutoCreate()) 805 this.dose = new Quantity(); // cc 806 return this.dose; 807 } 808 809 public boolean hasDose() { 810 return this.dose != null && !this.dose.isEmpty(); 811 } 812 813 /** 814 * @param value {@link #dose} (The amount of the medication given at one 815 * administration event. Use this value when the administration is 816 * essentially an instantaneous event such as a swallowing a tablet 817 * or giving an injection.) 818 */ 819 public MedicationAdministrationDosageComponent setDose(Quantity value) { 820 this.dose = value; 821 return this; 822 } 823 824 /** 825 * @return {@link #rate} (Identifies the speed with which the medication was or 826 * will be introduced into the patient. Typically, the rate for an 827 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 828 * as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 829 * 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 830 */ 831 public Type getRate() { 832 return this.rate; 833 } 834 835 /** 836 * @return {@link #rate} (Identifies the speed with which the medication was or 837 * will be introduced into the patient. Typically, the rate for an 838 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 839 * as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 840 * 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 841 */ 842 public Ratio getRateRatio() throws FHIRException { 843 if (this.rate == null) 844 this.rate = new Ratio(); 845 if (!(this.rate instanceof Ratio)) 846 throw new FHIRException( 847 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 848 return (Ratio) this.rate; 849 } 850 851 public boolean hasRateRatio() { 852 return this != null && this.rate instanceof Ratio; 853 } 854 855 /** 856 * @return {@link #rate} (Identifies the speed with which the medication was or 857 * will be introduced into the patient. Typically, the rate for an 858 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 859 * as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 860 * 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 861 */ 862 public Quantity getRateQuantity() throws FHIRException { 863 if (this.rate == null) 864 this.rate = new Quantity(); 865 if (!(this.rate instanceof Quantity)) 866 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.rate.getClass().getName() 867 + " was encountered"); 868 return (Quantity) this.rate; 869 } 870 871 public boolean hasRateQuantity() { 872 return this != null && this.rate instanceof Quantity; 873 } 874 875 public boolean hasRate() { 876 return this.rate != null && !this.rate.isEmpty(); 877 } 878 879 /** 880 * @param value {@link #rate} (Identifies the speed with which the medication 881 * was or will be introduced into the patient. Typically, the rate 882 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 883 * expressed as a rate per unit of time, e.g. 500 ml per 2 hours. 884 * Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 885 * hours.) 886 */ 887 public MedicationAdministrationDosageComponent setRate(Type value) { 888 if (value != null && !(value instanceof Ratio || value instanceof Quantity)) 889 throw new Error("Not the right type for MedicationAdministration.dosage.rate[x]: " + value.fhirType()); 890 this.rate = value; 891 return this; 892 } 893 894 protected void listChildren(List<Property> children) { 895 super.listChildren(children); 896 children.add(new Property("text", "string", 897 "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 898 0, 1, text)); 899 children.add(new Property("site", "CodeableConcept", 900 "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 901 0, 1, site)); 902 children.add(new Property("route", "CodeableConcept", 903 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 904 0, 1, route)); 905 children.add(new Property("method", "CodeableConcept", 906 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 907 0, 1, method)); 908 children.add(new Property("dose", "SimpleQuantity", 909 "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 910 0, 1, dose)); 911 children.add(new Property("rate[x]", "Ratio|SimpleQuantity", 912 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 913 0, 1, rate)); 914 } 915 916 @Override 917 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 918 switch (_hash) { 919 case 3556653: 920 /* text */ return new Property("text", "string", 921 "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 922 0, 1, text); 923 case 3530567: 924 /* site */ return new Property("site", "CodeableConcept", 925 "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 926 0, 1, site); 927 case 108704329: 928 /* route */ return new Property("route", "CodeableConcept", 929 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 930 0, 1, route); 931 case -1077554975: 932 /* method */ return new Property("method", "CodeableConcept", 933 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 934 0, 1, method); 935 case 3089437: 936 /* dose */ return new Property("dose", "SimpleQuantity", 937 "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 938 0, 1, dose); 939 case 983460768: 940 /* rate[x] */ return new Property("rate[x]", "Ratio|SimpleQuantity", 941 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 942 0, 1, rate); 943 case 3493088: 944 /* rate */ return new Property("rate[x]", "Ratio|SimpleQuantity", 945 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 946 0, 1, rate); 947 case 204021515: 948 /* rateRatio */ return new Property("rate[x]", "Ratio|SimpleQuantity", 949 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 950 0, 1, rate); 951 case -1085459061: 952 /* rateQuantity */ return new Property("rate[x]", "Ratio|SimpleQuantity", 953 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 954 0, 1, rate); 955 default: 956 return super.getNamedProperty(_hash, _name, _checkValid); 957 } 958 959 } 960 961 @Override 962 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 963 switch (hash) { 964 case 3556653: 965 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 966 case 3530567: 967 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // CodeableConcept 968 case 108704329: 969 /* route */ return this.route == null ? new Base[0] : new Base[] { this.route }; // CodeableConcept 970 case -1077554975: 971 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 972 case 3089437: 973 /* dose */ return this.dose == null ? new Base[0] : new Base[] { this.dose }; // Quantity 974 case 3493088: 975 /* rate */ return this.rate == null ? new Base[0] : new Base[] { this.rate }; // Type 976 default: 977 return super.getProperty(hash, name, checkValid); 978 } 979 980 } 981 982 @Override 983 public Base setProperty(int hash, String name, Base value) throws FHIRException { 984 switch (hash) { 985 case 3556653: // text 986 this.text = castToString(value); // StringType 987 return value; 988 case 3530567: // site 989 this.site = castToCodeableConcept(value); // CodeableConcept 990 return value; 991 case 108704329: // route 992 this.route = castToCodeableConcept(value); // CodeableConcept 993 return value; 994 case -1077554975: // method 995 this.method = castToCodeableConcept(value); // CodeableConcept 996 return value; 997 case 3089437: // dose 998 this.dose = castToQuantity(value); // Quantity 999 return value; 1000 case 3493088: // rate 1001 this.rate = castToType(value); // Type 1002 return value; 1003 default: 1004 return super.setProperty(hash, name, value); 1005 } 1006 1007 } 1008 1009 @Override 1010 public Base setProperty(String name, Base value) throws FHIRException { 1011 if (name.equals("text")) { 1012 this.text = castToString(value); // StringType 1013 } else if (name.equals("site")) { 1014 this.site = castToCodeableConcept(value); // CodeableConcept 1015 } else if (name.equals("route")) { 1016 this.route = castToCodeableConcept(value); // CodeableConcept 1017 } else if (name.equals("method")) { 1018 this.method = castToCodeableConcept(value); // CodeableConcept 1019 } else if (name.equals("dose")) { 1020 this.dose = castToQuantity(value); // Quantity 1021 } else if (name.equals("rate[x]")) { 1022 this.rate = castToType(value); // Type 1023 } else 1024 return super.setProperty(name, value); 1025 return value; 1026 } 1027 1028 @Override 1029 public void removeChild(String name, Base value) throws FHIRException { 1030 if (name.equals("text")) { 1031 this.text = null; 1032 } else if (name.equals("site")) { 1033 this.site = null; 1034 } else if (name.equals("route")) { 1035 this.route = null; 1036 } else if (name.equals("method")) { 1037 this.method = null; 1038 } else if (name.equals("dose")) { 1039 this.dose = null; 1040 } else if (name.equals("rate[x]")) { 1041 this.rate = null; 1042 } else 1043 super.removeChild(name, value); 1044 1045 } 1046 1047 @Override 1048 public Base makeProperty(int hash, String name) throws FHIRException { 1049 switch (hash) { 1050 case 3556653: 1051 return getTextElement(); 1052 case 3530567: 1053 return getSite(); 1054 case 108704329: 1055 return getRoute(); 1056 case -1077554975: 1057 return getMethod(); 1058 case 3089437: 1059 return getDose(); 1060 case 983460768: 1061 return getRate(); 1062 case 3493088: 1063 return getRate(); 1064 default: 1065 return super.makeProperty(hash, name); 1066 } 1067 1068 } 1069 1070 @Override 1071 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1072 switch (hash) { 1073 case 3556653: 1074 /* text */ return new String[] { "string" }; 1075 case 3530567: 1076 /* site */ return new String[] { "CodeableConcept" }; 1077 case 108704329: 1078 /* route */ return new String[] { "CodeableConcept" }; 1079 case -1077554975: 1080 /* method */ return new String[] { "CodeableConcept" }; 1081 case 3089437: 1082 /* dose */ return new String[] { "SimpleQuantity" }; 1083 case 3493088: 1084 /* rate */ return new String[] { "Ratio", "SimpleQuantity" }; 1085 default: 1086 return super.getTypesForProperty(hash, name); 1087 } 1088 1089 } 1090 1091 @Override 1092 public Base addChild(String name) throws FHIRException { 1093 if (name.equals("text")) { 1094 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.text"); 1095 } else if (name.equals("site")) { 1096 this.site = new CodeableConcept(); 1097 return this.site; 1098 } else if (name.equals("route")) { 1099 this.route = new CodeableConcept(); 1100 return this.route; 1101 } else if (name.equals("method")) { 1102 this.method = new CodeableConcept(); 1103 return this.method; 1104 } else if (name.equals("dose")) { 1105 this.dose = new Quantity(); 1106 return this.dose; 1107 } else if (name.equals("rateRatio")) { 1108 this.rate = new Ratio(); 1109 return this.rate; 1110 } else if (name.equals("rateQuantity")) { 1111 this.rate = new Quantity(); 1112 return this.rate; 1113 } else 1114 return super.addChild(name); 1115 } 1116 1117 public MedicationAdministrationDosageComponent copy() { 1118 MedicationAdministrationDosageComponent dst = new MedicationAdministrationDosageComponent(); 1119 copyValues(dst); 1120 return dst; 1121 } 1122 1123 public void copyValues(MedicationAdministrationDosageComponent dst) { 1124 super.copyValues(dst); 1125 dst.text = text == null ? null : text.copy(); 1126 dst.site = site == null ? null : site.copy(); 1127 dst.route = route == null ? null : route.copy(); 1128 dst.method = method == null ? null : method.copy(); 1129 dst.dose = dose == null ? null : dose.copy(); 1130 dst.rate = rate == null ? null : rate.copy(); 1131 } 1132 1133 @Override 1134 public boolean equalsDeep(Base other_) { 1135 if (!super.equalsDeep(other_)) 1136 return false; 1137 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 1138 return false; 1139 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 1140 return compareDeep(text, o.text, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 1141 && compareDeep(method, o.method, true) && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true); 1142 } 1143 1144 @Override 1145 public boolean equalsShallow(Base other_) { 1146 if (!super.equalsShallow(other_)) 1147 return false; 1148 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 1149 return false; 1150 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 1151 return compareValues(text, o.text, true); 1152 } 1153 1154 public boolean isEmpty() { 1155 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, site, route, method, dose, rate); 1156 } 1157 1158 public String fhirType() { 1159 return "MedicationAdministration.dosage"; 1160 1161 } 1162 1163 } 1164 1165 /** 1166 * Identifiers associated with this Medication Administration that are defined 1167 * by business processes and/or used to refer to it when a direct URL reference 1168 * to the resource itself is not appropriate. They are business identifiers 1169 * assigned to this resource by the performer or other systems and remain 1170 * constant as the resource is updated and propagates from server to server. 1171 */ 1172 @Child(name = "identifier", type = { 1173 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1174 @Description(shortDefinition = "External identifier", formalDefinition = "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.") 1175 protected List<Identifier> identifier; 1176 1177 /** 1178 * A protocol, guideline, orderset, or other definition that was adhered to in 1179 * whole or in part by this event. 1180 */ 1181 @Child(name = "instantiates", type = { 1182 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1183 @Description(shortDefinition = "Instantiates protocol or definition", formalDefinition = "A protocol, guideline, orderset, or other definition that was adhered to in whole or in part by this event.") 1184 protected List<UriType> instantiates; 1185 1186 /** 1187 * A larger event of which this particular event is a component or step. 1188 */ 1189 @Child(name = "partOf", type = { MedicationAdministration.class, 1190 Procedure.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1191 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 1192 protected List<Reference> partOf; 1193 /** 1194 * The actual objects that are the target of the reference (A larger event of 1195 * which this particular event is a component or step.) 1196 */ 1197 protected List<Resource> partOfTarget; 1198 1199 /** 1200 * Will generally be set to show that the administration has been completed. For 1201 * some long running administrations such as infusions, it is possible for an 1202 * administration to be started but not completed or it may be paused while some 1203 * other process is under way. 1204 */ 1205 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 1206 @Description(shortDefinition = "in-progress | not-done | on-hold | completed | entered-in-error | stopped | unknown", formalDefinition = "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.") 1207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-admin-status") 1208 protected Enumeration<MedicationAdministrationStatus> status; 1209 1210 /** 1211 * A code indicating why the administration was not performed. 1212 */ 1213 @Child(name = "statusReason", type = { 1214 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1215 @Description(shortDefinition = "Reason administration not performed", formalDefinition = "A code indicating why the administration was not performed.") 1216 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reason-medication-not-given-codes") 1217 protected List<CodeableConcept> statusReason; 1218 1219 /** 1220 * Indicates where the medication is expected to be consumed or administered. 1221 */ 1222 @Child(name = "category", type = { 1223 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1224 @Description(shortDefinition = "Type of medication usage", formalDefinition = "Indicates where the medication is expected to be consumed or administered.") 1225 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-admin-category") 1226 protected CodeableConcept category; 1227 1228 /** 1229 * Identifies the medication that was administered. This is either a link to a 1230 * resource representing the details of the medication or a simple attribute 1231 * carrying a code that identifies the medication from a known list of 1232 * medications. 1233 */ 1234 @Child(name = "medication", type = { CodeableConcept.class, 1235 Medication.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1236 @Description(shortDefinition = "What was administered", formalDefinition = "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 1237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1238 protected Type medication; 1239 1240 /** 1241 * The person or animal or group receiving the medication. 1242 */ 1243 @Child(name = "subject", type = { Patient.class, 1244 Group.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1245 @Description(shortDefinition = "Who received medication", formalDefinition = "The person or animal or group receiving the medication.") 1246 protected Reference subject; 1247 1248 /** 1249 * The actual object that is the target of the reference (The person or animal 1250 * or group receiving the medication.) 1251 */ 1252 protected Resource subjectTarget; 1253 1254 /** 1255 * The visit, admission, or other contact between patient and health care 1256 * provider during which the medication administration was performed. 1257 */ 1258 @Child(name = "context", type = { Encounter.class, 1259 EpisodeOfCare.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1260 @Description(shortDefinition = "Encounter or Episode of Care administered as part of", formalDefinition = "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.") 1261 protected Reference context; 1262 1263 /** 1264 * The actual object that is the target of the reference (The visit, admission, 1265 * or other contact between patient and health care provider during which the 1266 * medication administration was performed.) 1267 */ 1268 protected Resource contextTarget; 1269 1270 /** 1271 * Additional information (for example, patient height and weight) that supports 1272 * the administration of the medication. 1273 */ 1274 @Child(name = "supportingInformation", type = { 1275 Reference.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1276 @Description(shortDefinition = "Additional information to support administration", formalDefinition = "Additional information (for example, patient height and weight) that supports the administration of the medication.") 1277 protected List<Reference> supportingInformation; 1278 /** 1279 * The actual objects that are the target of the reference (Additional 1280 * information (for example, patient height and weight) that supports the 1281 * administration of the medication.) 1282 */ 1283 protected List<Resource> supportingInformationTarget; 1284 1285 /** 1286 * A specific date/time or interval of time during which the administration took 1287 * place (or did not take place, when the 'notGiven' attribute is true). For 1288 * many administrations, such as swallowing a tablet the use of dateTime is more 1289 * appropriate. 1290 */ 1291 @Child(name = "effective", type = { DateTimeType.class, 1292 Period.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 1293 @Description(shortDefinition = "Start and end time of administration", formalDefinition = "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.") 1294 protected Type effective; 1295 1296 /** 1297 * Indicates who or what performed the medication administration and how they 1298 * were involved. 1299 */ 1300 @Child(name = "performer", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1301 @Description(shortDefinition = "Who performed the medication administration and what they did", formalDefinition = "Indicates who or what performed the medication administration and how they were involved.") 1302 protected List<MedicationAdministrationPerformerComponent> performer; 1303 1304 /** 1305 * A code indicating why the medication was given. 1306 */ 1307 @Child(name = "reasonCode", type = { 1308 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1309 @Description(shortDefinition = "Reason administration performed", formalDefinition = "A code indicating why the medication was given.") 1310 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reason-medication-given-codes") 1311 protected List<CodeableConcept> reasonCode; 1312 1313 /** 1314 * Condition or observation that supports why the medication was administered. 1315 */ 1316 @Child(name = "reasonReference", type = { Condition.class, Observation.class, 1317 DiagnosticReport.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1318 @Description(shortDefinition = "Condition or observation that supports why the medication was administered", formalDefinition = "Condition or observation that supports why the medication was administered.") 1319 protected List<Reference> reasonReference; 1320 /** 1321 * The actual objects that are the target of the reference (Condition or 1322 * observation that supports why the medication was administered.) 1323 */ 1324 protected List<Resource> reasonReferenceTarget; 1325 1326 /** 1327 * The original request, instruction or authority to perform the administration. 1328 */ 1329 @Child(name = "request", type = { 1330 MedicationRequest.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1331 @Description(shortDefinition = "Request administration performed against", formalDefinition = "The original request, instruction or authority to perform the administration.") 1332 protected Reference request; 1333 1334 /** 1335 * The actual object that is the target of the reference (The original request, 1336 * instruction or authority to perform the administration.) 1337 */ 1338 protected MedicationRequest requestTarget; 1339 1340 /** 1341 * The device used in administering the medication to the patient. For example, 1342 * a particular infusion pump. 1343 */ 1344 @Child(name = "device", type = { 1345 Device.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1346 @Description(shortDefinition = "Device used to administer", formalDefinition = "The device used in administering the medication to the patient. For example, a particular infusion pump.") 1347 protected List<Reference> device; 1348 /** 1349 * The actual objects that are the target of the reference (The device used in 1350 * administering the medication to the patient. For example, a particular 1351 * infusion pump.) 1352 */ 1353 protected List<Device> deviceTarget; 1354 1355 /** 1356 * Extra information about the medication administration that is not conveyed by 1357 * the other attributes. 1358 */ 1359 @Child(name = "note", type = { 1360 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1361 @Description(shortDefinition = "Information about the administration", formalDefinition = "Extra information about the medication administration that is not conveyed by the other attributes.") 1362 protected List<Annotation> note; 1363 1364 /** 1365 * Describes the medication dosage information details e.g. dose, rate, site, 1366 * route, etc. 1367 */ 1368 @Child(name = "dosage", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = false) 1369 @Description(shortDefinition = "Details of how medication was taken", formalDefinition = "Describes the medication dosage information details e.g. dose, rate, site, route, etc.") 1370 protected MedicationAdministrationDosageComponent dosage; 1371 1372 /** 1373 * A summary of the events of interest that have occurred, such as when the 1374 * administration was verified. 1375 */ 1376 @Child(name = "eventHistory", type = { 1377 Provenance.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1378 @Description(shortDefinition = "A list of events of interest in the lifecycle", formalDefinition = "A summary of the events of interest that have occurred, such as when the administration was verified.") 1379 protected List<Reference> eventHistory; 1380 /** 1381 * The actual objects that are the target of the reference (A summary of the 1382 * events of interest that have occurred, such as when the administration was 1383 * verified.) 1384 */ 1385 protected List<Provenance> eventHistoryTarget; 1386 1387 private static final long serialVersionUID = 463158971L; 1388 1389 /** 1390 * Constructor 1391 */ 1392 public MedicationAdministration() { 1393 super(); 1394 } 1395 1396 /** 1397 * Constructor 1398 */ 1399 public MedicationAdministration(Enumeration<MedicationAdministrationStatus> status, Type medication, 1400 Reference subject, Type effective) { 1401 super(); 1402 this.status = status; 1403 this.medication = medication; 1404 this.subject = subject; 1405 this.effective = effective; 1406 } 1407 1408 /** 1409 * @return {@link #identifier} (Identifiers associated with this Medication 1410 * Administration that are defined by business processes and/or used to 1411 * refer to it when a direct URL reference to the resource itself is not 1412 * appropriate. They are business identifiers assigned to this resource 1413 * by the performer or other systems and remain constant as the resource 1414 * is updated and propagates from server to server.) 1415 */ 1416 public List<Identifier> getIdentifier() { 1417 if (this.identifier == null) 1418 this.identifier = new ArrayList<Identifier>(); 1419 return this.identifier; 1420 } 1421 1422 /** 1423 * @return Returns a reference to <code>this</code> for easy method chaining 1424 */ 1425 public MedicationAdministration setIdentifier(List<Identifier> theIdentifier) { 1426 this.identifier = theIdentifier; 1427 return this; 1428 } 1429 1430 public boolean hasIdentifier() { 1431 if (this.identifier == null) 1432 return false; 1433 for (Identifier item : this.identifier) 1434 if (!item.isEmpty()) 1435 return true; 1436 return false; 1437 } 1438 1439 public Identifier addIdentifier() { // 3 1440 Identifier t = new Identifier(); 1441 if (this.identifier == null) 1442 this.identifier = new ArrayList<Identifier>(); 1443 this.identifier.add(t); 1444 return t; 1445 } 1446 1447 public MedicationAdministration addIdentifier(Identifier t) { // 3 1448 if (t == null) 1449 return this; 1450 if (this.identifier == null) 1451 this.identifier = new ArrayList<Identifier>(); 1452 this.identifier.add(t); 1453 return this; 1454 } 1455 1456 /** 1457 * @return The first repetition of repeating field {@link #identifier}, creating 1458 * it if it does not already exist 1459 */ 1460 public Identifier getIdentifierFirstRep() { 1461 if (getIdentifier().isEmpty()) { 1462 addIdentifier(); 1463 } 1464 return getIdentifier().get(0); 1465 } 1466 1467 /** 1468 * @return {@link #instantiates} (A protocol, guideline, orderset, or other 1469 * definition that was adhered to in whole or in part by this event.) 1470 */ 1471 public List<UriType> getInstantiates() { 1472 if (this.instantiates == null) 1473 this.instantiates = new ArrayList<UriType>(); 1474 return this.instantiates; 1475 } 1476 1477 /** 1478 * @return Returns a reference to <code>this</code> for easy method chaining 1479 */ 1480 public MedicationAdministration setInstantiates(List<UriType> theInstantiates) { 1481 this.instantiates = theInstantiates; 1482 return this; 1483 } 1484 1485 public boolean hasInstantiates() { 1486 if (this.instantiates == null) 1487 return false; 1488 for (UriType item : this.instantiates) 1489 if (!item.isEmpty()) 1490 return true; 1491 return false; 1492 } 1493 1494 /** 1495 * @return {@link #instantiates} (A protocol, guideline, orderset, or other 1496 * definition that was adhered to in whole or in part by this event.) 1497 */ 1498 public UriType addInstantiatesElement() {// 2 1499 UriType t = new UriType(); 1500 if (this.instantiates == null) 1501 this.instantiates = new ArrayList<UriType>(); 1502 this.instantiates.add(t); 1503 return t; 1504 } 1505 1506 /** 1507 * @param value {@link #instantiates} (A protocol, guideline, orderset, or other 1508 * definition that was adhered to in whole or in part by this 1509 * event.) 1510 */ 1511 public MedicationAdministration addInstantiates(String value) { // 1 1512 UriType t = new UriType(); 1513 t.setValue(value); 1514 if (this.instantiates == null) 1515 this.instantiates = new ArrayList<UriType>(); 1516 this.instantiates.add(t); 1517 return this; 1518 } 1519 1520 /** 1521 * @param value {@link #instantiates} (A protocol, guideline, orderset, or other 1522 * definition that was adhered to in whole or in part by this 1523 * event.) 1524 */ 1525 public boolean hasInstantiates(String value) { 1526 if (this.instantiates == null) 1527 return false; 1528 for (UriType v : this.instantiates) 1529 if (v.getValue().equals(value)) // uri 1530 return true; 1531 return false; 1532 } 1533 1534 /** 1535 * @return {@link #partOf} (A larger event of which this particular event is a 1536 * component or step.) 1537 */ 1538 public List<Reference> getPartOf() { 1539 if (this.partOf == null) 1540 this.partOf = new ArrayList<Reference>(); 1541 return this.partOf; 1542 } 1543 1544 /** 1545 * @return Returns a reference to <code>this</code> for easy method chaining 1546 */ 1547 public MedicationAdministration setPartOf(List<Reference> thePartOf) { 1548 this.partOf = thePartOf; 1549 return this; 1550 } 1551 1552 public boolean hasPartOf() { 1553 if (this.partOf == null) 1554 return false; 1555 for (Reference item : this.partOf) 1556 if (!item.isEmpty()) 1557 return true; 1558 return false; 1559 } 1560 1561 public Reference addPartOf() { // 3 1562 Reference t = new Reference(); 1563 if (this.partOf == null) 1564 this.partOf = new ArrayList<Reference>(); 1565 this.partOf.add(t); 1566 return t; 1567 } 1568 1569 public MedicationAdministration addPartOf(Reference t) { // 3 1570 if (t == null) 1571 return this; 1572 if (this.partOf == null) 1573 this.partOf = new ArrayList<Reference>(); 1574 this.partOf.add(t); 1575 return this; 1576 } 1577 1578 /** 1579 * @return The first repetition of repeating field {@link #partOf}, creating it 1580 * if it does not already exist 1581 */ 1582 public Reference getPartOfFirstRep() { 1583 if (getPartOf().isEmpty()) { 1584 addPartOf(); 1585 } 1586 return getPartOf().get(0); 1587 } 1588 1589 /** 1590 * @deprecated Use Reference#setResource(IBaseResource) instead 1591 */ 1592 @Deprecated 1593 public List<Resource> getPartOfTarget() { 1594 if (this.partOfTarget == null) 1595 this.partOfTarget = new ArrayList<Resource>(); 1596 return this.partOfTarget; 1597 } 1598 1599 /** 1600 * @return {@link #status} (Will generally be set to show that the 1601 * administration has been completed. For some long running 1602 * administrations such as infusions, it is possible for an 1603 * administration to be started but not completed or it may be paused 1604 * while some other process is under way.). This is the underlying 1605 * object with id, value and extensions. The accessor "getStatus" gives 1606 * direct access to the value 1607 */ 1608 public Enumeration<MedicationAdministrationStatus> getStatusElement() { 1609 if (this.status == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create MedicationAdministration.status"); 1612 else if (Configuration.doAutoCreate()) 1613 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); // bb 1614 return this.status; 1615 } 1616 1617 public boolean hasStatusElement() { 1618 return this.status != null && !this.status.isEmpty(); 1619 } 1620 1621 public boolean hasStatus() { 1622 return this.status != null && !this.status.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #status} (Will generally be set to show that the 1627 * administration has been completed. For some long running 1628 * administrations such as infusions, it is possible for an 1629 * administration to be started but not completed or it may be 1630 * paused while some other process is under way.). This is the 1631 * underlying object with id, value and extensions. The accessor 1632 * "getStatus" gives direct access to the value 1633 */ 1634 public MedicationAdministration setStatusElement(Enumeration<MedicationAdministrationStatus> value) { 1635 this.status = value; 1636 return this; 1637 } 1638 1639 /** 1640 * @return Will generally be set to show that the administration has been 1641 * completed. For some long running administrations such as infusions, 1642 * it is possible for an administration to be started but not completed 1643 * or it may be paused while some other process is under way. 1644 */ 1645 public MedicationAdministrationStatus getStatus() { 1646 return this.status == null ? null : this.status.getValue(); 1647 } 1648 1649 /** 1650 * @param value Will generally be set to show that the administration has been 1651 * completed. For some long running administrations such as 1652 * infusions, it is possible for an administration to be started 1653 * but not completed or it may be paused while some other process 1654 * is under way. 1655 */ 1656 public MedicationAdministration setStatus(MedicationAdministrationStatus value) { 1657 if (this.status == null) 1658 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); 1659 this.status.setValue(value); 1660 return this; 1661 } 1662 1663 /** 1664 * @return {@link #statusReason} (A code indicating why the administration was 1665 * not performed.) 1666 */ 1667 public List<CodeableConcept> getStatusReason() { 1668 if (this.statusReason == null) 1669 this.statusReason = new ArrayList<CodeableConcept>(); 1670 return this.statusReason; 1671 } 1672 1673 /** 1674 * @return Returns a reference to <code>this</code> for easy method chaining 1675 */ 1676 public MedicationAdministration setStatusReason(List<CodeableConcept> theStatusReason) { 1677 this.statusReason = theStatusReason; 1678 return this; 1679 } 1680 1681 public boolean hasStatusReason() { 1682 if (this.statusReason == null) 1683 return false; 1684 for (CodeableConcept item : this.statusReason) 1685 if (!item.isEmpty()) 1686 return true; 1687 return false; 1688 } 1689 1690 public CodeableConcept addStatusReason() { // 3 1691 CodeableConcept t = new CodeableConcept(); 1692 if (this.statusReason == null) 1693 this.statusReason = new ArrayList<CodeableConcept>(); 1694 this.statusReason.add(t); 1695 return t; 1696 } 1697 1698 public MedicationAdministration addStatusReason(CodeableConcept t) { // 3 1699 if (t == null) 1700 return this; 1701 if (this.statusReason == null) 1702 this.statusReason = new ArrayList<CodeableConcept>(); 1703 this.statusReason.add(t); 1704 return this; 1705 } 1706 1707 /** 1708 * @return The first repetition of repeating field {@link #statusReason}, 1709 * creating it if it does not already exist 1710 */ 1711 public CodeableConcept getStatusReasonFirstRep() { 1712 if (getStatusReason().isEmpty()) { 1713 addStatusReason(); 1714 } 1715 return getStatusReason().get(0); 1716 } 1717 1718 /** 1719 * @return {@link #category} (Indicates where the medication is expected to be 1720 * consumed or administered.) 1721 */ 1722 public CodeableConcept getCategory() { 1723 if (this.category == null) 1724 if (Configuration.errorOnAutoCreate()) 1725 throw new Error("Attempt to auto-create MedicationAdministration.category"); 1726 else if (Configuration.doAutoCreate()) 1727 this.category = new CodeableConcept(); // cc 1728 return this.category; 1729 } 1730 1731 public boolean hasCategory() { 1732 return this.category != null && !this.category.isEmpty(); 1733 } 1734 1735 /** 1736 * @param value {@link #category} (Indicates where the medication is expected to 1737 * be consumed or administered.) 1738 */ 1739 public MedicationAdministration setCategory(CodeableConcept value) { 1740 this.category = value; 1741 return this; 1742 } 1743 1744 /** 1745 * @return {@link #medication} (Identifies the medication that was administered. 1746 * This is either a link to a resource representing the details of the 1747 * medication or a simple attribute carrying a code that identifies the 1748 * medication from a known list of medications.) 1749 */ 1750 public Type getMedication() { 1751 return this.medication; 1752 } 1753 1754 /** 1755 * @return {@link #medication} (Identifies the medication that was administered. 1756 * This is either a link to a resource representing the details of the 1757 * medication or a simple attribute carrying a code that identifies the 1758 * medication from a known list of medications.) 1759 */ 1760 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1761 if (this.medication == null) 1762 this.medication = new CodeableConcept(); 1763 if (!(this.medication instanceof CodeableConcept)) 1764 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1765 + this.medication.getClass().getName() + " was encountered"); 1766 return (CodeableConcept) this.medication; 1767 } 1768 1769 public boolean hasMedicationCodeableConcept() { 1770 return this != null && this.medication instanceof CodeableConcept; 1771 } 1772 1773 /** 1774 * @return {@link #medication} (Identifies the medication that was administered. 1775 * This is either a link to a resource representing the details of the 1776 * medication or a simple attribute carrying a code that identifies the 1777 * medication from a known list of medications.) 1778 */ 1779 public Reference getMedicationReference() throws FHIRException { 1780 if (this.medication == null) 1781 this.medication = new Reference(); 1782 if (!(this.medication instanceof Reference)) 1783 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1784 + this.medication.getClass().getName() + " was encountered"); 1785 return (Reference) this.medication; 1786 } 1787 1788 public boolean hasMedicationReference() { 1789 return this != null && this.medication instanceof Reference; 1790 } 1791 1792 public boolean hasMedication() { 1793 return this.medication != null && !this.medication.isEmpty(); 1794 } 1795 1796 /** 1797 * @param value {@link #medication} (Identifies the medication that was 1798 * administered. This is either a link to a resource representing 1799 * the details of the medication or a simple attribute carrying a 1800 * code that identifies the medication from a known list of 1801 * medications.) 1802 */ 1803 public MedicationAdministration setMedication(Type value) { 1804 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1805 throw new Error("Not the right type for MedicationAdministration.medication[x]: " + value.fhirType()); 1806 this.medication = value; 1807 return this; 1808 } 1809 1810 /** 1811 * @return {@link #subject} (The person or animal or group receiving the 1812 * medication.) 1813 */ 1814 public Reference getSubject() { 1815 if (this.subject == null) 1816 if (Configuration.errorOnAutoCreate()) 1817 throw new Error("Attempt to auto-create MedicationAdministration.subject"); 1818 else if (Configuration.doAutoCreate()) 1819 this.subject = new Reference(); // cc 1820 return this.subject; 1821 } 1822 1823 public boolean hasSubject() { 1824 return this.subject != null && !this.subject.isEmpty(); 1825 } 1826 1827 /** 1828 * @param value {@link #subject} (The person or animal or group receiving the 1829 * medication.) 1830 */ 1831 public MedicationAdministration setSubject(Reference value) { 1832 this.subject = value; 1833 return this; 1834 } 1835 1836 /** 1837 * @return {@link #subject} The actual object that is the target of the 1838 * reference. The reference library doesn't populate this, but you can 1839 * use it to hold the resource if you resolve it. (The person or animal 1840 * or group receiving the medication.) 1841 */ 1842 public Resource getSubjectTarget() { 1843 return this.subjectTarget; 1844 } 1845 1846 /** 1847 * @param value {@link #subject} The actual object that is the target of the 1848 * reference. The reference library doesn't use these, but you can 1849 * use it to hold the resource if you resolve it. (The person or 1850 * animal or group receiving the medication.) 1851 */ 1852 public MedicationAdministration setSubjectTarget(Resource value) { 1853 this.subjectTarget = value; 1854 return this; 1855 } 1856 1857 /** 1858 * @return {@link #context} (The visit, admission, or other contact between 1859 * patient and health care provider during which the medication 1860 * administration was performed.) 1861 */ 1862 public Reference getContext() { 1863 if (this.context == null) 1864 if (Configuration.errorOnAutoCreate()) 1865 throw new Error("Attempt to auto-create MedicationAdministration.context"); 1866 else if (Configuration.doAutoCreate()) 1867 this.context = new Reference(); // cc 1868 return this.context; 1869 } 1870 1871 public boolean hasContext() { 1872 return this.context != null && !this.context.isEmpty(); 1873 } 1874 1875 /** 1876 * @param value {@link #context} (The visit, admission, or other contact between 1877 * patient and health care provider during which the medication 1878 * administration was performed.) 1879 */ 1880 public MedicationAdministration setContext(Reference value) { 1881 this.context = value; 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #context} The actual object that is the target of the 1887 * reference. The reference library doesn't populate this, but you can 1888 * use it to hold the resource if you resolve it. (The visit, admission, 1889 * or other contact between patient and health care provider during 1890 * which the medication administration was performed.) 1891 */ 1892 public Resource getContextTarget() { 1893 return this.contextTarget; 1894 } 1895 1896 /** 1897 * @param value {@link #context} The actual object that is the target of the 1898 * reference. The reference library doesn't use these, but you can 1899 * use it to hold the resource if you resolve it. (The visit, 1900 * admission, or other contact between patient and health care 1901 * provider during which the medication administration was 1902 * performed.) 1903 */ 1904 public MedicationAdministration setContextTarget(Resource value) { 1905 this.contextTarget = value; 1906 return this; 1907 } 1908 1909 /** 1910 * @return {@link #supportingInformation} (Additional information (for example, 1911 * patient height and weight) that supports the administration of the 1912 * medication.) 1913 */ 1914 public List<Reference> getSupportingInformation() { 1915 if (this.supportingInformation == null) 1916 this.supportingInformation = new ArrayList<Reference>(); 1917 return this.supportingInformation; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public MedicationAdministration setSupportingInformation(List<Reference> theSupportingInformation) { 1924 this.supportingInformation = theSupportingInformation; 1925 return this; 1926 } 1927 1928 public boolean hasSupportingInformation() { 1929 if (this.supportingInformation == null) 1930 return false; 1931 for (Reference item : this.supportingInformation) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public Reference addSupportingInformation() { // 3 1938 Reference t = new Reference(); 1939 if (this.supportingInformation == null) 1940 this.supportingInformation = new ArrayList<Reference>(); 1941 this.supportingInformation.add(t); 1942 return t; 1943 } 1944 1945 public MedicationAdministration addSupportingInformation(Reference t) { // 3 1946 if (t == null) 1947 return this; 1948 if (this.supportingInformation == null) 1949 this.supportingInformation = new ArrayList<Reference>(); 1950 this.supportingInformation.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field 1956 * {@link #supportingInformation}, creating it if it does not already 1957 * exist 1958 */ 1959 public Reference getSupportingInformationFirstRep() { 1960 if (getSupportingInformation().isEmpty()) { 1961 addSupportingInformation(); 1962 } 1963 return getSupportingInformation().get(0); 1964 } 1965 1966 /** 1967 * @deprecated Use Reference#setResource(IBaseResource) instead 1968 */ 1969 @Deprecated 1970 public List<Resource> getSupportingInformationTarget() { 1971 if (this.supportingInformationTarget == null) 1972 this.supportingInformationTarget = new ArrayList<Resource>(); 1973 return this.supportingInformationTarget; 1974 } 1975 1976 /** 1977 * @return {@link #effective} (A specific date/time or interval of time during 1978 * which the administration took place (or did not take place, when the 1979 * 'notGiven' attribute is true). For many administrations, such as 1980 * swallowing a tablet the use of dateTime is more appropriate.) 1981 */ 1982 public Type getEffective() { 1983 return this.effective; 1984 } 1985 1986 /** 1987 * @return {@link #effective} (A specific date/time or interval of time during 1988 * which the administration took place (or did not take place, when the 1989 * 'notGiven' attribute is true). For many administrations, such as 1990 * swallowing a tablet the use of dateTime is more appropriate.) 1991 */ 1992 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1993 if (this.effective == null) 1994 this.effective = new DateTimeType(); 1995 if (!(this.effective instanceof DateTimeType)) 1996 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1997 + this.effective.getClass().getName() + " was encountered"); 1998 return (DateTimeType) this.effective; 1999 } 2000 2001 public boolean hasEffectiveDateTimeType() { 2002 return this != null && this.effective instanceof DateTimeType; 2003 } 2004 2005 /** 2006 * @return {@link #effective} (A specific date/time or interval of time during 2007 * which the administration took place (or did not take place, when the 2008 * 'notGiven' attribute is true). For many administrations, such as 2009 * swallowing a tablet the use of dateTime is more appropriate.) 2010 */ 2011 public Period getEffectivePeriod() throws FHIRException { 2012 if (this.effective == null) 2013 this.effective = new Period(); 2014 if (!(this.effective instanceof Period)) 2015 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 2016 + " was encountered"); 2017 return (Period) this.effective; 2018 } 2019 2020 public boolean hasEffectivePeriod() { 2021 return this != null && this.effective instanceof Period; 2022 } 2023 2024 public boolean hasEffective() { 2025 return this.effective != null && !this.effective.isEmpty(); 2026 } 2027 2028 /** 2029 * @param value {@link #effective} (A specific date/time or interval of time 2030 * during which the administration took place (or did not take 2031 * place, when the 'notGiven' attribute is true). For many 2032 * administrations, such as swallowing a tablet the use of dateTime 2033 * is more appropriate.) 2034 */ 2035 public MedicationAdministration setEffective(Type value) { 2036 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 2037 throw new Error("Not the right type for MedicationAdministration.effective[x]: " + value.fhirType()); 2038 this.effective = value; 2039 return this; 2040 } 2041 2042 /** 2043 * @return {@link #performer} (Indicates who or what performed the medication 2044 * administration and how they were involved.) 2045 */ 2046 public List<MedicationAdministrationPerformerComponent> getPerformer() { 2047 if (this.performer == null) 2048 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2049 return this.performer; 2050 } 2051 2052 /** 2053 * @return Returns a reference to <code>this</code> for easy method chaining 2054 */ 2055 public MedicationAdministration setPerformer(List<MedicationAdministrationPerformerComponent> thePerformer) { 2056 this.performer = thePerformer; 2057 return this; 2058 } 2059 2060 public boolean hasPerformer() { 2061 if (this.performer == null) 2062 return false; 2063 for (MedicationAdministrationPerformerComponent item : this.performer) 2064 if (!item.isEmpty()) 2065 return true; 2066 return false; 2067 } 2068 2069 public MedicationAdministrationPerformerComponent addPerformer() { // 3 2070 MedicationAdministrationPerformerComponent t = new MedicationAdministrationPerformerComponent(); 2071 if (this.performer == null) 2072 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2073 this.performer.add(t); 2074 return t; 2075 } 2076 2077 public MedicationAdministration addPerformer(MedicationAdministrationPerformerComponent t) { // 3 2078 if (t == null) 2079 return this; 2080 if (this.performer == null) 2081 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2082 this.performer.add(t); 2083 return this; 2084 } 2085 2086 /** 2087 * @return The first repetition of repeating field {@link #performer}, creating 2088 * it if it does not already exist 2089 */ 2090 public MedicationAdministrationPerformerComponent getPerformerFirstRep() { 2091 if (getPerformer().isEmpty()) { 2092 addPerformer(); 2093 } 2094 return getPerformer().get(0); 2095 } 2096 2097 /** 2098 * @return {@link #reasonCode} (A code indicating why the medication was given.) 2099 */ 2100 public List<CodeableConcept> getReasonCode() { 2101 if (this.reasonCode == null) 2102 this.reasonCode = new ArrayList<CodeableConcept>(); 2103 return this.reasonCode; 2104 } 2105 2106 /** 2107 * @return Returns a reference to <code>this</code> for easy method chaining 2108 */ 2109 public MedicationAdministration setReasonCode(List<CodeableConcept> theReasonCode) { 2110 this.reasonCode = theReasonCode; 2111 return this; 2112 } 2113 2114 public boolean hasReasonCode() { 2115 if (this.reasonCode == null) 2116 return false; 2117 for (CodeableConcept item : this.reasonCode) 2118 if (!item.isEmpty()) 2119 return true; 2120 return false; 2121 } 2122 2123 public CodeableConcept addReasonCode() { // 3 2124 CodeableConcept t = new CodeableConcept(); 2125 if (this.reasonCode == null) 2126 this.reasonCode = new ArrayList<CodeableConcept>(); 2127 this.reasonCode.add(t); 2128 return t; 2129 } 2130 2131 public MedicationAdministration addReasonCode(CodeableConcept t) { // 3 2132 if (t == null) 2133 return this; 2134 if (this.reasonCode == null) 2135 this.reasonCode = new ArrayList<CodeableConcept>(); 2136 this.reasonCode.add(t); 2137 return this; 2138 } 2139 2140 /** 2141 * @return The first repetition of repeating field {@link #reasonCode}, creating 2142 * it if it does not already exist 2143 */ 2144 public CodeableConcept getReasonCodeFirstRep() { 2145 if (getReasonCode().isEmpty()) { 2146 addReasonCode(); 2147 } 2148 return getReasonCode().get(0); 2149 } 2150 2151 /** 2152 * @return {@link #reasonReference} (Condition or observation that supports why 2153 * the medication was administered.) 2154 */ 2155 public List<Reference> getReasonReference() { 2156 if (this.reasonReference == null) 2157 this.reasonReference = new ArrayList<Reference>(); 2158 return this.reasonReference; 2159 } 2160 2161 /** 2162 * @return Returns a reference to <code>this</code> for easy method chaining 2163 */ 2164 public MedicationAdministration setReasonReference(List<Reference> theReasonReference) { 2165 this.reasonReference = theReasonReference; 2166 return this; 2167 } 2168 2169 public boolean hasReasonReference() { 2170 if (this.reasonReference == null) 2171 return false; 2172 for (Reference item : this.reasonReference) 2173 if (!item.isEmpty()) 2174 return true; 2175 return false; 2176 } 2177 2178 public Reference addReasonReference() { // 3 2179 Reference t = new Reference(); 2180 if (this.reasonReference == null) 2181 this.reasonReference = new ArrayList<Reference>(); 2182 this.reasonReference.add(t); 2183 return t; 2184 } 2185 2186 public MedicationAdministration addReasonReference(Reference t) { // 3 2187 if (t == null) 2188 return this; 2189 if (this.reasonReference == null) 2190 this.reasonReference = new ArrayList<Reference>(); 2191 this.reasonReference.add(t); 2192 return this; 2193 } 2194 2195 /** 2196 * @return The first repetition of repeating field {@link #reasonReference}, 2197 * creating it if it does not already exist 2198 */ 2199 public Reference getReasonReferenceFirstRep() { 2200 if (getReasonReference().isEmpty()) { 2201 addReasonReference(); 2202 } 2203 return getReasonReference().get(0); 2204 } 2205 2206 /** 2207 * @deprecated Use Reference#setResource(IBaseResource) instead 2208 */ 2209 @Deprecated 2210 public List<Resource> getReasonReferenceTarget() { 2211 if (this.reasonReferenceTarget == null) 2212 this.reasonReferenceTarget = new ArrayList<Resource>(); 2213 return this.reasonReferenceTarget; 2214 } 2215 2216 /** 2217 * @return {@link #request} (The original request, instruction or authority to 2218 * perform the administration.) 2219 */ 2220 public Reference getRequest() { 2221 if (this.request == null) 2222 if (Configuration.errorOnAutoCreate()) 2223 throw new Error("Attempt to auto-create MedicationAdministration.request"); 2224 else if (Configuration.doAutoCreate()) 2225 this.request = new Reference(); // cc 2226 return this.request; 2227 } 2228 2229 public boolean hasRequest() { 2230 return this.request != null && !this.request.isEmpty(); 2231 } 2232 2233 /** 2234 * @param value {@link #request} (The original request, instruction or authority 2235 * to perform the administration.) 2236 */ 2237 public MedicationAdministration setRequest(Reference value) { 2238 this.request = value; 2239 return this; 2240 } 2241 2242 /** 2243 * @return {@link #request} The actual object that is the target of the 2244 * reference. The reference library doesn't populate this, but you can 2245 * use it to hold the resource if you resolve it. (The original request, 2246 * instruction or authority to perform the administration.) 2247 */ 2248 public MedicationRequest getRequestTarget() { 2249 if (this.requestTarget == null) 2250 if (Configuration.errorOnAutoCreate()) 2251 throw new Error("Attempt to auto-create MedicationAdministration.request"); 2252 else if (Configuration.doAutoCreate()) 2253 this.requestTarget = new MedicationRequest(); // aa 2254 return this.requestTarget; 2255 } 2256 2257 /** 2258 * @param value {@link #request} The actual object that is the target of the 2259 * reference. The reference library doesn't use these, but you can 2260 * use it to hold the resource if you resolve it. (The original 2261 * request, instruction or authority to perform the 2262 * administration.) 2263 */ 2264 public MedicationAdministration setRequestTarget(MedicationRequest value) { 2265 this.requestTarget = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return {@link #device} (The device used in administering the medication to 2271 * the patient. For example, a particular infusion pump.) 2272 */ 2273 public List<Reference> getDevice() { 2274 if (this.device == null) 2275 this.device = new ArrayList<Reference>(); 2276 return this.device; 2277 } 2278 2279 /** 2280 * @return Returns a reference to <code>this</code> for easy method chaining 2281 */ 2282 public MedicationAdministration setDevice(List<Reference> theDevice) { 2283 this.device = theDevice; 2284 return this; 2285 } 2286 2287 public boolean hasDevice() { 2288 if (this.device == null) 2289 return false; 2290 for (Reference item : this.device) 2291 if (!item.isEmpty()) 2292 return true; 2293 return false; 2294 } 2295 2296 public Reference addDevice() { // 3 2297 Reference t = new Reference(); 2298 if (this.device == null) 2299 this.device = new ArrayList<Reference>(); 2300 this.device.add(t); 2301 return t; 2302 } 2303 2304 public MedicationAdministration addDevice(Reference t) { // 3 2305 if (t == null) 2306 return this; 2307 if (this.device == null) 2308 this.device = new ArrayList<Reference>(); 2309 this.device.add(t); 2310 return this; 2311 } 2312 2313 /** 2314 * @return The first repetition of repeating field {@link #device}, creating it 2315 * if it does not already exist 2316 */ 2317 public Reference getDeviceFirstRep() { 2318 if (getDevice().isEmpty()) { 2319 addDevice(); 2320 } 2321 return getDevice().get(0); 2322 } 2323 2324 /** 2325 * @deprecated Use Reference#setResource(IBaseResource) instead 2326 */ 2327 @Deprecated 2328 public List<Device> getDeviceTarget() { 2329 if (this.deviceTarget == null) 2330 this.deviceTarget = new ArrayList<Device>(); 2331 return this.deviceTarget; 2332 } 2333 2334 /** 2335 * @deprecated Use Reference#setResource(IBaseResource) instead 2336 */ 2337 @Deprecated 2338 public Device addDeviceTarget() { 2339 Device r = new Device(); 2340 if (this.deviceTarget == null) 2341 this.deviceTarget = new ArrayList<Device>(); 2342 this.deviceTarget.add(r); 2343 return r; 2344 } 2345 2346 /** 2347 * @return {@link #note} (Extra information about the medication administration 2348 * that is not conveyed by the other attributes.) 2349 */ 2350 public List<Annotation> getNote() { 2351 if (this.note == null) 2352 this.note = new ArrayList<Annotation>(); 2353 return this.note; 2354 } 2355 2356 /** 2357 * @return Returns a reference to <code>this</code> for easy method chaining 2358 */ 2359 public MedicationAdministration setNote(List<Annotation> theNote) { 2360 this.note = theNote; 2361 return this; 2362 } 2363 2364 public boolean hasNote() { 2365 if (this.note == null) 2366 return false; 2367 for (Annotation item : this.note) 2368 if (!item.isEmpty()) 2369 return true; 2370 return false; 2371 } 2372 2373 public Annotation addNote() { // 3 2374 Annotation t = new Annotation(); 2375 if (this.note == null) 2376 this.note = new ArrayList<Annotation>(); 2377 this.note.add(t); 2378 return t; 2379 } 2380 2381 public MedicationAdministration addNote(Annotation t) { // 3 2382 if (t == null) 2383 return this; 2384 if (this.note == null) 2385 this.note = new ArrayList<Annotation>(); 2386 this.note.add(t); 2387 return this; 2388 } 2389 2390 /** 2391 * @return The first repetition of repeating field {@link #note}, creating it if 2392 * it does not already exist 2393 */ 2394 public Annotation getNoteFirstRep() { 2395 if (getNote().isEmpty()) { 2396 addNote(); 2397 } 2398 return getNote().get(0); 2399 } 2400 2401 /** 2402 * @return {@link #dosage} (Describes the medication dosage information details 2403 * e.g. dose, rate, site, route, etc.) 2404 */ 2405 public MedicationAdministrationDosageComponent getDosage() { 2406 if (this.dosage == null) 2407 if (Configuration.errorOnAutoCreate()) 2408 throw new Error("Attempt to auto-create MedicationAdministration.dosage"); 2409 else if (Configuration.doAutoCreate()) 2410 this.dosage = new MedicationAdministrationDosageComponent(); // cc 2411 return this.dosage; 2412 } 2413 2414 public boolean hasDosage() { 2415 return this.dosage != null && !this.dosage.isEmpty(); 2416 } 2417 2418 /** 2419 * @param value {@link #dosage} (Describes the medication dosage information 2420 * details e.g. dose, rate, site, route, etc.) 2421 */ 2422 public MedicationAdministration setDosage(MedicationAdministrationDosageComponent value) { 2423 this.dosage = value; 2424 return this; 2425 } 2426 2427 /** 2428 * @return {@link #eventHistory} (A summary of the events of interest that have 2429 * occurred, such as when the administration was verified.) 2430 */ 2431 public List<Reference> getEventHistory() { 2432 if (this.eventHistory == null) 2433 this.eventHistory = new ArrayList<Reference>(); 2434 return this.eventHistory; 2435 } 2436 2437 /** 2438 * @return Returns a reference to <code>this</code> for easy method chaining 2439 */ 2440 public MedicationAdministration setEventHistory(List<Reference> theEventHistory) { 2441 this.eventHistory = theEventHistory; 2442 return this; 2443 } 2444 2445 public boolean hasEventHistory() { 2446 if (this.eventHistory == null) 2447 return false; 2448 for (Reference item : this.eventHistory) 2449 if (!item.isEmpty()) 2450 return true; 2451 return false; 2452 } 2453 2454 public Reference addEventHistory() { // 3 2455 Reference t = new Reference(); 2456 if (this.eventHistory == null) 2457 this.eventHistory = new ArrayList<Reference>(); 2458 this.eventHistory.add(t); 2459 return t; 2460 } 2461 2462 public MedicationAdministration addEventHistory(Reference t) { // 3 2463 if (t == null) 2464 return this; 2465 if (this.eventHistory == null) 2466 this.eventHistory = new ArrayList<Reference>(); 2467 this.eventHistory.add(t); 2468 return this; 2469 } 2470 2471 /** 2472 * @return The first repetition of repeating field {@link #eventHistory}, 2473 * creating it if it does not already exist 2474 */ 2475 public Reference getEventHistoryFirstRep() { 2476 if (getEventHistory().isEmpty()) { 2477 addEventHistory(); 2478 } 2479 return getEventHistory().get(0); 2480 } 2481 2482 /** 2483 * @deprecated Use Reference#setResource(IBaseResource) instead 2484 */ 2485 @Deprecated 2486 public List<Provenance> getEventHistoryTarget() { 2487 if (this.eventHistoryTarget == null) 2488 this.eventHistoryTarget = new ArrayList<Provenance>(); 2489 return this.eventHistoryTarget; 2490 } 2491 2492 /** 2493 * @deprecated Use Reference#setResource(IBaseResource) instead 2494 */ 2495 @Deprecated 2496 public Provenance addEventHistoryTarget() { 2497 Provenance r = new Provenance(); 2498 if (this.eventHistoryTarget == null) 2499 this.eventHistoryTarget = new ArrayList<Provenance>(); 2500 this.eventHistoryTarget.add(r); 2501 return r; 2502 } 2503 2504 protected void listChildren(List<Property> children) { 2505 super.listChildren(children); 2506 children.add(new Property("identifier", "Identifier", 2507 "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 2508 0, java.lang.Integer.MAX_VALUE, identifier)); 2509 children.add(new Property("instantiates", "uri", 2510 "A protocol, guideline, orderset, or other definition that was adhered to in whole or in part by this event.", 2511 0, java.lang.Integer.MAX_VALUE, instantiates)); 2512 children.add(new Property("partOf", "Reference(MedicationAdministration|Procedure)", 2513 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 2514 partOf)); 2515 children.add(new Property("status", "code", 2516 "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 2517 0, 1, status)); 2518 children.add(new Property("statusReason", "CodeableConcept", 2519 "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, statusReason)); 2520 children.add(new Property("category", "CodeableConcept", 2521 "Indicates where the medication is expected to be consumed or administered.", 0, 1, category)); 2522 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2523 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2524 0, 1, medication)); 2525 children.add(new Property("subject", "Reference(Patient|Group)", 2526 "The person or animal or group receiving the medication.", 0, 1, subject)); 2527 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 2528 "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.", 2529 0, 1, context)); 2530 children.add(new Property("supportingInformation", "Reference(Any)", 2531 "Additional information (for example, patient height and weight) that supports the administration of the medication.", 2532 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2533 children.add(new Property("effective[x]", "dateTime|Period", 2534 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2535 0, 1, effective)); 2536 children.add(new Property("performer", "", 2537 "Indicates who or what performed the medication administration and how they were involved.", 0, 2538 java.lang.Integer.MAX_VALUE, performer)); 2539 children.add(new Property("reasonCode", "CodeableConcept", "A code indicating why the medication was given.", 0, 2540 java.lang.Integer.MAX_VALUE, reasonCode)); 2541 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 2542 "Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, 2543 reasonReference)); 2544 children.add(new Property("request", "Reference(MedicationRequest)", 2545 "The original request, instruction or authority to perform the administration.", 0, 1, request)); 2546 children.add(new Property("device", "Reference(Device)", 2547 "The device used in administering the medication to the patient. For example, a particular infusion pump.", 0, 2548 java.lang.Integer.MAX_VALUE, device)); 2549 children.add(new Property("note", "Annotation", 2550 "Extra information about the medication administration that is not conveyed by the other attributes.", 0, 2551 java.lang.Integer.MAX_VALUE, note)); 2552 children.add(new Property("dosage", "", 2553 "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage)); 2554 children.add(new Property("eventHistory", "Reference(Provenance)", 2555 "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, 2556 java.lang.Integer.MAX_VALUE, eventHistory)); 2557 } 2558 2559 @Override 2560 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2561 switch (_hash) { 2562 case -1618432855: 2563 /* identifier */ return new Property("identifier", "Identifier", 2564 "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 2565 0, java.lang.Integer.MAX_VALUE, identifier); 2566 case -246883639: 2567 /* instantiates */ return new Property("instantiates", "uri", 2568 "A protocol, guideline, orderset, or other definition that was adhered to in whole or in part by this event.", 2569 0, java.lang.Integer.MAX_VALUE, instantiates); 2570 case -995410646: 2571 /* partOf */ return new Property("partOf", "Reference(MedicationAdministration|Procedure)", 2572 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 2573 partOf); 2574 case -892481550: 2575 /* status */ return new Property("status", "code", 2576 "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 2577 0, 1, status); 2578 case 2051346646: 2579 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2580 "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, statusReason); 2581 case 50511102: 2582 /* category */ return new Property("category", "CodeableConcept", 2583 "Indicates where the medication is expected to be consumed or administered.", 0, 1, category); 2584 case 1458402129: 2585 /* medication[x] */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2586 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2587 0, 1, medication); 2588 case 1998965455: 2589 /* medication */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2590 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2591 0, 1, medication); 2592 case -209845038: 2593 /* medicationCodeableConcept */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2594 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2595 0, 1, medication); 2596 case 2104315196: 2597 /* medicationReference */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2598 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2599 0, 1, medication); 2600 case -1867885268: 2601 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2602 "The person or animal or group receiving the medication.", 0, 1, subject); 2603 case 951530927: 2604 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 2605 "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.", 2606 0, 1, context); 2607 case -1248768647: 2608 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2609 "Additional information (for example, patient height and weight) that supports the administration of the medication.", 2610 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2611 case 247104889: 2612 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 2613 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2614 0, 1, effective); 2615 case -1468651097: 2616 /* effective */ return new Property("effective[x]", "dateTime|Period", 2617 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2618 0, 1, effective); 2619 case -275306910: 2620 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 2621 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2622 0, 1, effective); 2623 case -403934648: 2624 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 2625 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2626 0, 1, effective); 2627 case 481140686: 2628 /* performer */ return new Property("performer", "", 2629 "Indicates who or what performed the medication administration and how they were involved.", 0, 2630 java.lang.Integer.MAX_VALUE, performer); 2631 case 722137681: 2632 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2633 "A code indicating why the medication was given.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2634 case -1146218137: 2635 /* reasonReference */ return new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 2636 "Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, 2637 reasonReference); 2638 case 1095692943: 2639 /* request */ return new Property("request", "Reference(MedicationRequest)", 2640 "The original request, instruction or authority to perform the administration.", 0, 1, request); 2641 case -1335157162: 2642 /* device */ return new Property("device", "Reference(Device)", 2643 "The device used in administering the medication to the patient. For example, a particular infusion pump.", 2644 0, java.lang.Integer.MAX_VALUE, device); 2645 case 3387378: 2646 /* note */ return new Property("note", "Annotation", 2647 "Extra information about the medication administration that is not conveyed by the other attributes.", 0, 2648 java.lang.Integer.MAX_VALUE, note); 2649 case -1326018889: 2650 /* dosage */ return new Property("dosage", "", 2651 "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage); 2652 case 1835190426: 2653 /* eventHistory */ return new Property("eventHistory", "Reference(Provenance)", 2654 "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, 2655 java.lang.Integer.MAX_VALUE, eventHistory); 2656 default: 2657 return super.getNamedProperty(_hash, _name, _checkValid); 2658 } 2659 2660 } 2661 2662 @Override 2663 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2664 switch (hash) { 2665 case -1618432855: 2666 /* identifier */ return this.identifier == null ? new Base[0] 2667 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2668 case -246883639: 2669 /* instantiates */ return this.instantiates == null ? new Base[0] 2670 : this.instantiates.toArray(new Base[this.instantiates.size()]); // UriType 2671 case -995410646: 2672 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2673 case -892481550: 2674 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationAdministrationStatus> 2675 case 2051346646: 2676 /* statusReason */ return this.statusReason == null ? new Base[0] 2677 : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 2678 case 50511102: 2679 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 2680 case 1998965455: 2681 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 2682 case -1867885268: 2683 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2684 case 951530927: 2685 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 2686 case -1248768647: 2687 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2688 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2689 case -1468651097: 2690 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 2691 case 481140686: 2692 /* performer */ return this.performer == null ? new Base[0] 2693 : this.performer.toArray(new Base[this.performer.size()]); // MedicationAdministrationPerformerComponent 2694 case 722137681: 2695 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2696 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2697 case -1146218137: 2698 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2699 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2700 case 1095692943: 2701 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 2702 case -1335157162: 2703 /* device */ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 2704 case 3387378: 2705 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2706 case -1326018889: 2707 /* dosage */ return this.dosage == null ? new Base[0] : new Base[] { this.dosage }; // MedicationAdministrationDosageComponent 2708 case 1835190426: 2709 /* eventHistory */ return this.eventHistory == null ? new Base[0] 2710 : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2711 default: 2712 return super.getProperty(hash, name, checkValid); 2713 } 2714 2715 } 2716 2717 @Override 2718 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2719 switch (hash) { 2720 case -1618432855: // identifier 2721 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2722 return value; 2723 case -246883639: // instantiates 2724 this.getInstantiates().add(castToUri(value)); // UriType 2725 return value; 2726 case -995410646: // partOf 2727 this.getPartOf().add(castToReference(value)); // Reference 2728 return value; 2729 case -892481550: // status 2730 value = new MedicationAdministrationStatusEnumFactory().fromType(castToCode(value)); 2731 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatus> 2732 return value; 2733 case 2051346646: // statusReason 2734 this.getStatusReason().add(castToCodeableConcept(value)); // CodeableConcept 2735 return value; 2736 case 50511102: // category 2737 this.category = castToCodeableConcept(value); // CodeableConcept 2738 return value; 2739 case 1998965455: // medication 2740 this.medication = castToType(value); // Type 2741 return value; 2742 case -1867885268: // subject 2743 this.subject = castToReference(value); // Reference 2744 return value; 2745 case 951530927: // context 2746 this.context = castToReference(value); // Reference 2747 return value; 2748 case -1248768647: // supportingInformation 2749 this.getSupportingInformation().add(castToReference(value)); // Reference 2750 return value; 2751 case -1468651097: // effective 2752 this.effective = castToType(value); // Type 2753 return value; 2754 case 481140686: // performer 2755 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); // MedicationAdministrationPerformerComponent 2756 return value; 2757 case 722137681: // reasonCode 2758 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2759 return value; 2760 case -1146218137: // reasonReference 2761 this.getReasonReference().add(castToReference(value)); // Reference 2762 return value; 2763 case 1095692943: // request 2764 this.request = castToReference(value); // Reference 2765 return value; 2766 case -1335157162: // device 2767 this.getDevice().add(castToReference(value)); // Reference 2768 return value; 2769 case 3387378: // note 2770 this.getNote().add(castToAnnotation(value)); // Annotation 2771 return value; 2772 case -1326018889: // dosage 2773 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2774 return value; 2775 case 1835190426: // eventHistory 2776 this.getEventHistory().add(castToReference(value)); // Reference 2777 return value; 2778 default: 2779 return super.setProperty(hash, name, value); 2780 } 2781 2782 } 2783 2784 @Override 2785 public Base setProperty(String name, Base value) throws FHIRException { 2786 if (name.equals("identifier")) { 2787 this.getIdentifier().add(castToIdentifier(value)); 2788 } else if (name.equals("instantiates")) { 2789 this.getInstantiates().add(castToUri(value)); 2790 } else if (name.equals("partOf")) { 2791 this.getPartOf().add(castToReference(value)); 2792 } else if (name.equals("status")) { 2793 value = new MedicationAdministrationStatusEnumFactory().fromType(castToCode(value)); 2794 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatus> 2795 } else if (name.equals("statusReason")) { 2796 this.getStatusReason().add(castToCodeableConcept(value)); 2797 } else if (name.equals("category")) { 2798 this.category = castToCodeableConcept(value); // CodeableConcept 2799 } else if (name.equals("medication[x]")) { 2800 this.medication = castToType(value); // Type 2801 } else if (name.equals("subject")) { 2802 this.subject = castToReference(value); // Reference 2803 } else if (name.equals("context")) { 2804 this.context = castToReference(value); // Reference 2805 } else if (name.equals("supportingInformation")) { 2806 this.getSupportingInformation().add(castToReference(value)); 2807 } else if (name.equals("effective[x]")) { 2808 this.effective = castToType(value); // Type 2809 } else if (name.equals("performer")) { 2810 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); 2811 } else if (name.equals("reasonCode")) { 2812 this.getReasonCode().add(castToCodeableConcept(value)); 2813 } else if (name.equals("reasonReference")) { 2814 this.getReasonReference().add(castToReference(value)); 2815 } else if (name.equals("request")) { 2816 this.request = castToReference(value); // Reference 2817 } else if (name.equals("device")) { 2818 this.getDevice().add(castToReference(value)); 2819 } else if (name.equals("note")) { 2820 this.getNote().add(castToAnnotation(value)); 2821 } else if (name.equals("dosage")) { 2822 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2823 } else if (name.equals("eventHistory")) { 2824 this.getEventHistory().add(castToReference(value)); 2825 } else 2826 return super.setProperty(name, value); 2827 return value; 2828 } 2829 2830 @Override 2831 public void removeChild(String name, Base value) throws FHIRException { 2832 if (name.equals("identifier")) { 2833 this.getIdentifier().remove(castToIdentifier(value)); 2834 } else if (name.equals("instantiates")) { 2835 this.getInstantiates().remove(castToUri(value)); 2836 } else if (name.equals("partOf")) { 2837 this.getPartOf().remove(castToReference(value)); 2838 } else if (name.equals("status")) { 2839 this.status = null; 2840 } else if (name.equals("statusReason")) { 2841 this.getStatusReason().remove(castToCodeableConcept(value)); 2842 } else if (name.equals("category")) { 2843 this.category = null; 2844 } else if (name.equals("medication[x]")) { 2845 this.medication = null; 2846 } else if (name.equals("subject")) { 2847 this.subject = null; 2848 } else if (name.equals("context")) { 2849 this.context = null; 2850 } else if (name.equals("supportingInformation")) { 2851 this.getSupportingInformation().remove(castToReference(value)); 2852 } else if (name.equals("effective[x]")) { 2853 this.effective = null; 2854 } else if (name.equals("performer")) { 2855 this.getPerformer().remove((MedicationAdministrationPerformerComponent) value); 2856 } else if (name.equals("reasonCode")) { 2857 this.getReasonCode().remove(castToCodeableConcept(value)); 2858 } else if (name.equals("reasonReference")) { 2859 this.getReasonReference().remove(castToReference(value)); 2860 } else if (name.equals("request")) { 2861 this.request = null; 2862 } else if (name.equals("device")) { 2863 this.getDevice().remove(castToReference(value)); 2864 } else if (name.equals("note")) { 2865 this.getNote().remove(castToAnnotation(value)); 2866 } else if (name.equals("dosage")) { 2867 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2868 } else if (name.equals("eventHistory")) { 2869 this.getEventHistory().remove(castToReference(value)); 2870 } else 2871 super.removeChild(name, value); 2872 2873 } 2874 2875 @Override 2876 public Base makeProperty(int hash, String name) throws FHIRException { 2877 switch (hash) { 2878 case -1618432855: 2879 return addIdentifier(); 2880 case -246883639: 2881 return addInstantiatesElement(); 2882 case -995410646: 2883 return addPartOf(); 2884 case -892481550: 2885 return getStatusElement(); 2886 case 2051346646: 2887 return addStatusReason(); 2888 case 50511102: 2889 return getCategory(); 2890 case 1458402129: 2891 return getMedication(); 2892 case 1998965455: 2893 return getMedication(); 2894 case -1867885268: 2895 return getSubject(); 2896 case 951530927: 2897 return getContext(); 2898 case -1248768647: 2899 return addSupportingInformation(); 2900 case 247104889: 2901 return getEffective(); 2902 case -1468651097: 2903 return getEffective(); 2904 case 481140686: 2905 return addPerformer(); 2906 case 722137681: 2907 return addReasonCode(); 2908 case -1146218137: 2909 return addReasonReference(); 2910 case 1095692943: 2911 return getRequest(); 2912 case -1335157162: 2913 return addDevice(); 2914 case 3387378: 2915 return addNote(); 2916 case -1326018889: 2917 return getDosage(); 2918 case 1835190426: 2919 return addEventHistory(); 2920 default: 2921 return super.makeProperty(hash, name); 2922 } 2923 2924 } 2925 2926 @Override 2927 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2928 switch (hash) { 2929 case -1618432855: 2930 /* identifier */ return new String[] { "Identifier" }; 2931 case -246883639: 2932 /* instantiates */ return new String[] { "uri" }; 2933 case -995410646: 2934 /* partOf */ return new String[] { "Reference" }; 2935 case -892481550: 2936 /* status */ return new String[] { "code" }; 2937 case 2051346646: 2938 /* statusReason */ return new String[] { "CodeableConcept" }; 2939 case 50511102: 2940 /* category */ return new String[] { "CodeableConcept" }; 2941 case 1998965455: 2942 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 2943 case -1867885268: 2944 /* subject */ return new String[] { "Reference" }; 2945 case 951530927: 2946 /* context */ return new String[] { "Reference" }; 2947 case -1248768647: 2948 /* supportingInformation */ return new String[] { "Reference" }; 2949 case -1468651097: 2950 /* effective */ return new String[] { "dateTime", "Period" }; 2951 case 481140686: 2952 /* performer */ return new String[] {}; 2953 case 722137681: 2954 /* reasonCode */ return new String[] { "CodeableConcept" }; 2955 case -1146218137: 2956 /* reasonReference */ return new String[] { "Reference" }; 2957 case 1095692943: 2958 /* request */ return new String[] { "Reference" }; 2959 case -1335157162: 2960 /* device */ return new String[] { "Reference" }; 2961 case 3387378: 2962 /* note */ return new String[] { "Annotation" }; 2963 case -1326018889: 2964 /* dosage */ return new String[] {}; 2965 case 1835190426: 2966 /* eventHistory */ return new String[] { "Reference" }; 2967 default: 2968 return super.getTypesForProperty(hash, name); 2969 } 2970 2971 } 2972 2973 @Override 2974 public Base addChild(String name) throws FHIRException { 2975 if (name.equals("identifier")) { 2976 return addIdentifier(); 2977 } else if (name.equals("instantiates")) { 2978 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.instantiates"); 2979 } else if (name.equals("partOf")) { 2980 return addPartOf(); 2981 } else if (name.equals("status")) { 2982 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.status"); 2983 } else if (name.equals("statusReason")) { 2984 return addStatusReason(); 2985 } else if (name.equals("category")) { 2986 this.category = new CodeableConcept(); 2987 return this.category; 2988 } else if (name.equals("medicationCodeableConcept")) { 2989 this.medication = new CodeableConcept(); 2990 return this.medication; 2991 } else if (name.equals("medicationReference")) { 2992 this.medication = new Reference(); 2993 return this.medication; 2994 } else if (name.equals("subject")) { 2995 this.subject = new Reference(); 2996 return this.subject; 2997 } else if (name.equals("context")) { 2998 this.context = new Reference(); 2999 return this.context; 3000 } else if (name.equals("supportingInformation")) { 3001 return addSupportingInformation(); 3002 } else if (name.equals("effectiveDateTime")) { 3003 this.effective = new DateTimeType(); 3004 return this.effective; 3005 } else if (name.equals("effectivePeriod")) { 3006 this.effective = new Period(); 3007 return this.effective; 3008 } else if (name.equals("performer")) { 3009 return addPerformer(); 3010 } else if (name.equals("reasonCode")) { 3011 return addReasonCode(); 3012 } else if (name.equals("reasonReference")) { 3013 return addReasonReference(); 3014 } else if (name.equals("request")) { 3015 this.request = new Reference(); 3016 return this.request; 3017 } else if (name.equals("device")) { 3018 return addDevice(); 3019 } else if (name.equals("note")) { 3020 return addNote(); 3021 } else if (name.equals("dosage")) { 3022 this.dosage = new MedicationAdministrationDosageComponent(); 3023 return this.dosage; 3024 } else if (name.equals("eventHistory")) { 3025 return addEventHistory(); 3026 } else 3027 return super.addChild(name); 3028 } 3029 3030 public String fhirType() { 3031 return "MedicationAdministration"; 3032 3033 } 3034 3035 public MedicationAdministration copy() { 3036 MedicationAdministration dst = new MedicationAdministration(); 3037 copyValues(dst); 3038 return dst; 3039 } 3040 3041 public void copyValues(MedicationAdministration dst) { 3042 super.copyValues(dst); 3043 if (identifier != null) { 3044 dst.identifier = new ArrayList<Identifier>(); 3045 for (Identifier i : identifier) 3046 dst.identifier.add(i.copy()); 3047 } 3048 ; 3049 if (instantiates != null) { 3050 dst.instantiates = new ArrayList<UriType>(); 3051 for (UriType i : instantiates) 3052 dst.instantiates.add(i.copy()); 3053 } 3054 ; 3055 if (partOf != null) { 3056 dst.partOf = new ArrayList<Reference>(); 3057 for (Reference i : partOf) 3058 dst.partOf.add(i.copy()); 3059 } 3060 ; 3061 dst.status = status == null ? null : status.copy(); 3062 if (statusReason != null) { 3063 dst.statusReason = new ArrayList<CodeableConcept>(); 3064 for (CodeableConcept i : statusReason) 3065 dst.statusReason.add(i.copy()); 3066 } 3067 ; 3068 dst.category = category == null ? null : category.copy(); 3069 dst.medication = medication == null ? null : medication.copy(); 3070 dst.subject = subject == null ? null : subject.copy(); 3071 dst.context = context == null ? null : context.copy(); 3072 if (supportingInformation != null) { 3073 dst.supportingInformation = new ArrayList<Reference>(); 3074 for (Reference i : supportingInformation) 3075 dst.supportingInformation.add(i.copy()); 3076 } 3077 ; 3078 dst.effective = effective == null ? null : effective.copy(); 3079 if (performer != null) { 3080 dst.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 3081 for (MedicationAdministrationPerformerComponent i : performer) 3082 dst.performer.add(i.copy()); 3083 } 3084 ; 3085 if (reasonCode != null) { 3086 dst.reasonCode = new ArrayList<CodeableConcept>(); 3087 for (CodeableConcept i : reasonCode) 3088 dst.reasonCode.add(i.copy()); 3089 } 3090 ; 3091 if (reasonReference != null) { 3092 dst.reasonReference = new ArrayList<Reference>(); 3093 for (Reference i : reasonReference) 3094 dst.reasonReference.add(i.copy()); 3095 } 3096 ; 3097 dst.request = request == null ? null : request.copy(); 3098 if (device != null) { 3099 dst.device = new ArrayList<Reference>(); 3100 for (Reference i : device) 3101 dst.device.add(i.copy()); 3102 } 3103 ; 3104 if (note != null) { 3105 dst.note = new ArrayList<Annotation>(); 3106 for (Annotation i : note) 3107 dst.note.add(i.copy()); 3108 } 3109 ; 3110 dst.dosage = dosage == null ? null : dosage.copy(); 3111 if (eventHistory != null) { 3112 dst.eventHistory = new ArrayList<Reference>(); 3113 for (Reference i : eventHistory) 3114 dst.eventHistory.add(i.copy()); 3115 } 3116 ; 3117 } 3118 3119 protected MedicationAdministration typedCopy() { 3120 return copy(); 3121 } 3122 3123 @Override 3124 public boolean equalsDeep(Base other_) { 3125 if (!super.equalsDeep(other_)) 3126 return false; 3127 if (!(other_ instanceof MedicationAdministration)) 3128 return false; 3129 MedicationAdministration o = (MedicationAdministration) other_; 3130 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiates, o.instantiates, true) 3131 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 3132 && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) 3133 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 3134 && compareDeep(context, o.context, true) && compareDeep(supportingInformation, o.supportingInformation, true) 3135 && compareDeep(effective, o.effective, true) && compareDeep(performer, o.performer, true) 3136 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3137 && compareDeep(request, o.request, true) && compareDeep(device, o.device, true) 3138 && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true) 3139 && compareDeep(eventHistory, o.eventHistory, true); 3140 } 3141 3142 @Override 3143 public boolean equalsShallow(Base other_) { 3144 if (!super.equalsShallow(other_)) 3145 return false; 3146 if (!(other_ instanceof MedicationAdministration)) 3147 return false; 3148 MedicationAdministration o = (MedicationAdministration) other_; 3149 return compareValues(instantiates, o.instantiates, true) && compareValues(status, o.status, true); 3150 } 3151 3152 public boolean isEmpty() { 3153 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiates, partOf, status, 3154 statusReason, category, medication, subject, context, supportingInformation, effective, performer, reasonCode, 3155 reasonReference, request, device, note, dosage, eventHistory); 3156 } 3157 3158 @Override 3159 public ResourceType getResourceType() { 3160 return ResourceType.MedicationAdministration; 3161 } 3162 3163 /** 3164 * Search parameter: <b>identifier</b> 3165 * <p> 3166 * Description: <b>Return administrations with this external identifier</b><br> 3167 * Type: <b>token</b><br> 3168 * Path: <b>MedicationAdministration.identifier</b><br> 3169 * </p> 3170 */ 3171 @SearchParamDefinition(name = "identifier", path = "MedicationAdministration.identifier", description = "Return administrations with this external identifier", type = "token") 3172 public static final String SP_IDENTIFIER = "identifier"; 3173 /** 3174 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3175 * <p> 3176 * Description: <b>Return administrations with this external identifier</b><br> 3177 * Type: <b>token</b><br> 3178 * Path: <b>MedicationAdministration.identifier</b><br> 3179 * </p> 3180 */ 3181 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3182 SP_IDENTIFIER); 3183 3184 /** 3185 * Search parameter: <b>request</b> 3186 * <p> 3187 * Description: <b>The identity of a request to list administrations 3188 * from</b><br> 3189 * Type: <b>reference</b><br> 3190 * Path: <b>MedicationAdministration.request</b><br> 3191 * </p> 3192 */ 3193 @SearchParamDefinition(name = "request", path = "MedicationAdministration.request", description = "The identity of a request to list administrations from", type = "reference", target = { 3194 MedicationRequest.class }) 3195 public static final String SP_REQUEST = "request"; 3196 /** 3197 * <b>Fluent Client</b> search parameter constant for <b>request</b> 3198 * <p> 3199 * Description: <b>The identity of a request to list administrations 3200 * from</b><br> 3201 * Type: <b>reference</b><br> 3202 * Path: <b>MedicationAdministration.request</b><br> 3203 * </p> 3204 */ 3205 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3206 SP_REQUEST); 3207 3208 /** 3209 * Constant for fluent queries to be used to add include statements. Specifies 3210 * the path value of "<b>MedicationAdministration:request</b>". 3211 */ 3212 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 3213 "MedicationAdministration:request").toLocked(); 3214 3215 /** 3216 * Search parameter: <b>code</b> 3217 * <p> 3218 * Description: <b>Return administrations of this medication code</b><br> 3219 * Type: <b>token</b><br> 3220 * Path: <b>MedicationAdministration.medicationCodeableConcept</b><br> 3221 * </p> 3222 */ 3223 @SearchParamDefinition(name = "code", path = "(MedicationAdministration.medication as CodeableConcept)", description = "Return administrations of this medication code", type = "token") 3224 public static final String SP_CODE = "code"; 3225 /** 3226 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3227 * <p> 3228 * Description: <b>Return administrations of this medication code</b><br> 3229 * Type: <b>token</b><br> 3230 * Path: <b>MedicationAdministration.medicationCodeableConcept</b><br> 3231 * </p> 3232 */ 3233 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3234 SP_CODE); 3235 3236 /** 3237 * Search parameter: <b>performer</b> 3238 * <p> 3239 * Description: <b>The identity of the individual who administered the 3240 * medication</b><br> 3241 * Type: <b>reference</b><br> 3242 * Path: <b>MedicationAdministration.performer.actor</b><br> 3243 * </p> 3244 */ 3245 @SearchParamDefinition(name = "performer", path = "MedicationAdministration.performer.actor", description = "The identity of the individual who administered the medication", type = "reference", providesMembershipIn = { 3246 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3247 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3248 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, Patient.class, 3249 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3250 public static final String SP_PERFORMER = "performer"; 3251 /** 3252 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3253 * <p> 3254 * Description: <b>The identity of the individual who administered the 3255 * medication</b><br> 3256 * Type: <b>reference</b><br> 3257 * Path: <b>MedicationAdministration.performer.actor</b><br> 3258 * </p> 3259 */ 3260 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3261 SP_PERFORMER); 3262 3263 /** 3264 * Constant for fluent queries to be used to add include statements. Specifies 3265 * the path value of "<b>MedicationAdministration:performer</b>". 3266 */ 3267 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 3268 "MedicationAdministration:performer").toLocked(); 3269 3270 /** 3271 * Search parameter: <b>subject</b> 3272 * <p> 3273 * Description: <b>The identity of the individual or group to list 3274 * administrations for</b><br> 3275 * Type: <b>reference</b><br> 3276 * Path: <b>MedicationAdministration.subject</b><br> 3277 * </p> 3278 */ 3279 @SearchParamDefinition(name = "subject", path = "MedicationAdministration.subject", description = "The identity of the individual or group to list administrations for", type = "reference", providesMembershipIn = { 3280 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3281 public static final String SP_SUBJECT = "subject"; 3282 /** 3283 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3284 * <p> 3285 * Description: <b>The identity of the individual or group to list 3286 * administrations for</b><br> 3287 * Type: <b>reference</b><br> 3288 * Path: <b>MedicationAdministration.subject</b><br> 3289 * </p> 3290 */ 3291 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3292 SP_SUBJECT); 3293 3294 /** 3295 * Constant for fluent queries to be used to add include statements. Specifies 3296 * the path value of "<b>MedicationAdministration:subject</b>". 3297 */ 3298 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3299 "MedicationAdministration:subject").toLocked(); 3300 3301 /** 3302 * Search parameter: <b>medication</b> 3303 * <p> 3304 * Description: <b>Return administrations of this medication resource</b><br> 3305 * Type: <b>reference</b><br> 3306 * Path: <b>MedicationAdministration.medicationReference</b><br> 3307 * </p> 3308 */ 3309 @SearchParamDefinition(name = "medication", path = "(MedicationAdministration.medication as Reference)", description = "Return administrations of this medication resource", type = "reference", target = { 3310 Medication.class }) 3311 public static final String SP_MEDICATION = "medication"; 3312 /** 3313 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3314 * <p> 3315 * Description: <b>Return administrations of this medication resource</b><br> 3316 * Type: <b>reference</b><br> 3317 * Path: <b>MedicationAdministration.medicationReference</b><br> 3318 * </p> 3319 */ 3320 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3321 SP_MEDICATION); 3322 3323 /** 3324 * Constant for fluent queries to be used to add include statements. Specifies 3325 * the path value of "<b>MedicationAdministration:medication</b>". 3326 */ 3327 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include( 3328 "MedicationAdministration:medication").toLocked(); 3329 3330 /** 3331 * Search parameter: <b>reason-given</b> 3332 * <p> 3333 * Description: <b>Reasons for administering the medication</b><br> 3334 * Type: <b>token</b><br> 3335 * Path: <b>MedicationAdministration.reasonCode</b><br> 3336 * </p> 3337 */ 3338 @SearchParamDefinition(name = "reason-given", path = "MedicationAdministration.reasonCode", description = "Reasons for administering the medication", type = "token") 3339 public static final String SP_REASON_GIVEN = "reason-given"; 3340 /** 3341 * <b>Fluent Client</b> search parameter constant for <b>reason-given</b> 3342 * <p> 3343 * Description: <b>Reasons for administering the medication</b><br> 3344 * Type: <b>token</b><br> 3345 * Path: <b>MedicationAdministration.reasonCode</b><br> 3346 * </p> 3347 */ 3348 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3349 SP_REASON_GIVEN); 3350 3351 /** 3352 * Search parameter: <b>patient</b> 3353 * <p> 3354 * Description: <b>The identity of a patient to list administrations for</b><br> 3355 * Type: <b>reference</b><br> 3356 * Path: <b>MedicationAdministration.subject</b><br> 3357 * </p> 3358 */ 3359 @SearchParamDefinition(name = "patient", path = "MedicationAdministration.subject.where(resolve() is Patient)", description = "The identity of a patient to list administrations for", type = "reference", providesMembershipIn = { 3360 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3361 public static final String SP_PATIENT = "patient"; 3362 /** 3363 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3364 * <p> 3365 * Description: <b>The identity of a patient to list administrations for</b><br> 3366 * Type: <b>reference</b><br> 3367 * Path: <b>MedicationAdministration.subject</b><br> 3368 * </p> 3369 */ 3370 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3371 SP_PATIENT); 3372 3373 /** 3374 * Constant for fluent queries to be used to add include statements. Specifies 3375 * the path value of "<b>MedicationAdministration:patient</b>". 3376 */ 3377 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3378 "MedicationAdministration:patient").toLocked(); 3379 3380 /** 3381 * Search parameter: <b>effective-time</b> 3382 * <p> 3383 * Description: <b>Date administration happened (or did not happen)</b><br> 3384 * Type: <b>date</b><br> 3385 * Path: <b>MedicationAdministration.effective[x]</b><br> 3386 * </p> 3387 */ 3388 @SearchParamDefinition(name = "effective-time", path = "MedicationAdministration.effective", description = "Date administration happened (or did not happen)", type = "date") 3389 public static final String SP_EFFECTIVE_TIME = "effective-time"; 3390 /** 3391 * <b>Fluent Client</b> search parameter constant for <b>effective-time</b> 3392 * <p> 3393 * Description: <b>Date administration happened (or did not happen)</b><br> 3394 * Type: <b>date</b><br> 3395 * Path: <b>MedicationAdministration.effective[x]</b><br> 3396 * </p> 3397 */ 3398 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE_TIME = new ca.uhn.fhir.rest.gclient.DateClientParam( 3399 SP_EFFECTIVE_TIME); 3400 3401 /** 3402 * Search parameter: <b>context</b> 3403 * <p> 3404 * Description: <b>Return administrations that share this encounter or episode 3405 * of care</b><br> 3406 * Type: <b>reference</b><br> 3407 * Path: <b>MedicationAdministration.context</b><br> 3408 * </p> 3409 */ 3410 @SearchParamDefinition(name = "context", path = "MedicationAdministration.context", description = "Return administrations that share this encounter or episode of care", type = "reference", providesMembershipIn = { 3411 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3412 EpisodeOfCare.class }) 3413 public static final String SP_CONTEXT = "context"; 3414 /** 3415 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3416 * <p> 3417 * Description: <b>Return administrations that share this encounter or episode 3418 * of care</b><br> 3419 * Type: <b>reference</b><br> 3420 * Path: <b>MedicationAdministration.context</b><br> 3421 * </p> 3422 */ 3423 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3424 SP_CONTEXT); 3425 3426 /** 3427 * Constant for fluent queries to be used to add include statements. Specifies 3428 * the path value of "<b>MedicationAdministration:context</b>". 3429 */ 3430 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include( 3431 "MedicationAdministration:context").toLocked(); 3432 3433 /** 3434 * Search parameter: <b>reason-not-given</b> 3435 * <p> 3436 * Description: <b>Reasons for not administering the medication</b><br> 3437 * Type: <b>token</b><br> 3438 * Path: <b>MedicationAdministration.statusReason</b><br> 3439 * </p> 3440 */ 3441 @SearchParamDefinition(name = "reason-not-given", path = "MedicationAdministration.statusReason", description = "Reasons for not administering the medication", type = "token") 3442 public static final String SP_REASON_NOT_GIVEN = "reason-not-given"; 3443 /** 3444 * <b>Fluent Client</b> search parameter constant for <b>reason-not-given</b> 3445 * <p> 3446 * Description: <b>Reasons for not administering the medication</b><br> 3447 * Type: <b>token</b><br> 3448 * Path: <b>MedicationAdministration.statusReason</b><br> 3449 * </p> 3450 */ 3451 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3452 SP_REASON_NOT_GIVEN); 3453 3454 /** 3455 * Search parameter: <b>device</b> 3456 * <p> 3457 * Description: <b>Return administrations with this administration device 3458 * identity</b><br> 3459 * Type: <b>reference</b><br> 3460 * Path: <b>MedicationAdministration.device</b><br> 3461 * </p> 3462 */ 3463 @SearchParamDefinition(name = "device", path = "MedicationAdministration.device", description = "Return administrations with this administration device identity", type = "reference", providesMembershipIn = { 3464 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 3465 public static final String SP_DEVICE = "device"; 3466 /** 3467 * <b>Fluent Client</b> search parameter constant for <b>device</b> 3468 * <p> 3469 * Description: <b>Return administrations with this administration device 3470 * identity</b><br> 3471 * Type: <b>reference</b><br> 3472 * Path: <b>MedicationAdministration.device</b><br> 3473 * </p> 3474 */ 3475 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3476 SP_DEVICE); 3477 3478 /** 3479 * Constant for fluent queries to be used to add include statements. Specifies 3480 * the path value of "<b>MedicationAdministration:device</b>". 3481 */ 3482 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 3483 "MedicationAdministration:device").toLocked(); 3484 3485 /** 3486 * Search parameter: <b>status</b> 3487 * <p> 3488 * Description: <b>MedicationAdministration event status (for example one of 3489 * active/paused/completed/nullified)</b><br> 3490 * Type: <b>token</b><br> 3491 * Path: <b>MedicationAdministration.status</b><br> 3492 * </p> 3493 */ 3494 @SearchParamDefinition(name = "status", path = "MedicationAdministration.status", description = "MedicationAdministration event status (for example one of active/paused/completed/nullified)", type = "token") 3495 public static final String SP_STATUS = "status"; 3496 /** 3497 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3498 * <p> 3499 * Description: <b>MedicationAdministration event status (for example one of 3500 * active/paused/completed/nullified)</b><br> 3501 * Type: <b>token</b><br> 3502 * Path: <b>MedicationAdministration.status</b><br> 3503 * </p> 3504 */ 3505 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3506 SP_STATUS); 3507 3508}