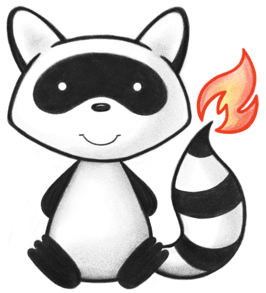
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Indicates that a medication product is to be or has been dispensed for a 048 * named person/patient. This includes a description of the medication product 049 * (supply) provided and the instructions for administering the medication. The 050 * medication dispense is the result of a pharmacy system responding to a 051 * medication order. 052 */ 053@ResourceDef(name = "MedicationDispense", profile = "http://hl7.org/fhir/StructureDefinition/MedicationDispense") 054public class MedicationDispense extends DomainResource { 055 056 public enum MedicationDispenseStatus { 057 /** 058 * The core event has not started yet, but some staging activities have begun 059 * (e.g. initial compounding or packaging of medication). Preparation stages may 060 * be tracked for billing purposes. 061 */ 062 PREPARATION, 063 /** 064 * The dispensed product is ready for pickup. 065 */ 066 INPROGRESS, 067 /** 068 * The dispensed product was not and will never be picked up by the patient. 069 */ 070 CANCELLED, 071 /** 072 * The dispense process is paused while waiting for an external event to 073 * reactivate the dispense. For example, new stock has arrived or the prescriber 074 * has called. 075 */ 076 ONHOLD, 077 /** 078 * The dispensed product has been picked up. 079 */ 080 COMPLETED, 081 /** 082 * The dispense was entered in error and therefore nullified. 083 */ 084 ENTEREDINERROR, 085 /** 086 * Actions implied by the dispense have been permanently halted, before all of 087 * them occurred. 088 */ 089 STOPPED, 090 /** 091 * The dispense was declined and not performed. 092 */ 093 DECLINED, 094 /** 095 * The authoring system does not know which of the status values applies for 096 * this medication dispense. Note: this concept is not to be used for other - 097 * one of the listed statuses is presumed to apply, it's just now known which 098 * one. 099 */ 100 UNKNOWN, 101 /** 102 * added to help the parsers with the generic types 103 */ 104 NULL; 105 106 public static MedicationDispenseStatus fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("preparation".equals(codeString)) 110 return PREPARATION; 111 if ("in-progress".equals(codeString)) 112 return INPROGRESS; 113 if ("cancelled".equals(codeString)) 114 return CANCELLED; 115 if ("on-hold".equals(codeString)) 116 return ONHOLD; 117 if ("completed".equals(codeString)) 118 return COMPLETED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 if ("stopped".equals(codeString)) 122 return STOPPED; 123 if ("declined".equals(codeString)) 124 return DECLINED; 125 if ("unknown".equals(codeString)) 126 return UNKNOWN; 127 if (Configuration.isAcceptInvalidEnums()) 128 return null; 129 else 130 throw new FHIRException("Unknown MedicationDispenseStatus code '" + codeString + "'"); 131 } 132 133 public String toCode() { 134 switch (this) { 135 case PREPARATION: 136 return "preparation"; 137 case INPROGRESS: 138 return "in-progress"; 139 case CANCELLED: 140 return "cancelled"; 141 case ONHOLD: 142 return "on-hold"; 143 case COMPLETED: 144 return "completed"; 145 case ENTEREDINERROR: 146 return "entered-in-error"; 147 case STOPPED: 148 return "stopped"; 149 case DECLINED: 150 return "declined"; 151 case UNKNOWN: 152 return "unknown"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getSystem() { 161 switch (this) { 162 case PREPARATION: 163 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 164 case INPROGRESS: 165 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 166 case CANCELLED: 167 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 168 case ONHOLD: 169 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 170 case COMPLETED: 171 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 172 case ENTEREDINERROR: 173 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 174 case STOPPED: 175 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 176 case DECLINED: 177 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 178 case UNKNOWN: 179 return "http://terminology.hl7.org/CodeSystem/medicationdispense-status"; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 187 public String getDefinition() { 188 switch (this) { 189 case PREPARATION: 190 return "The core event has not started yet, but some staging activities have begun (e.g. initial compounding or packaging of medication). Preparation stages may be tracked for billing purposes."; 191 case INPROGRESS: 192 return "The dispensed product is ready for pickup."; 193 case CANCELLED: 194 return "The dispensed product was not and will never be picked up by the patient."; 195 case ONHOLD: 196 return "The dispense process is paused while waiting for an external event to reactivate the dispense. For example, new stock has arrived or the prescriber has called."; 197 case COMPLETED: 198 return "The dispensed product has been picked up."; 199 case ENTEREDINERROR: 200 return "The dispense was entered in error and therefore nullified."; 201 case STOPPED: 202 return "Actions implied by the dispense have been permanently halted, before all of them occurred."; 203 case DECLINED: 204 return "The dispense was declined and not performed."; 205 case UNKNOWN: 206 return "The authoring system does not know which of the status values applies for this medication dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just now known which one."; 207 case NULL: 208 return null; 209 default: 210 return "?"; 211 } 212 } 213 214 public String getDisplay() { 215 switch (this) { 216 case PREPARATION: 217 return "Preparation"; 218 case INPROGRESS: 219 return "In Progress"; 220 case CANCELLED: 221 return "Cancelled"; 222 case ONHOLD: 223 return "On Hold"; 224 case COMPLETED: 225 return "Completed"; 226 case ENTEREDINERROR: 227 return "Entered in Error"; 228 case STOPPED: 229 return "Stopped"; 230 case DECLINED: 231 return "Declined"; 232 case UNKNOWN: 233 return "Unknown"; 234 case NULL: 235 return null; 236 default: 237 return "?"; 238 } 239 } 240 } 241 242 public static class MedicationDispenseStatusEnumFactory implements EnumFactory<MedicationDispenseStatus> { 243 public MedicationDispenseStatus fromCode(String codeString) throws IllegalArgumentException { 244 if (codeString == null || "".equals(codeString)) 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("preparation".equals(codeString)) 248 return MedicationDispenseStatus.PREPARATION; 249 if ("in-progress".equals(codeString)) 250 return MedicationDispenseStatus.INPROGRESS; 251 if ("cancelled".equals(codeString)) 252 return MedicationDispenseStatus.CANCELLED; 253 if ("on-hold".equals(codeString)) 254 return MedicationDispenseStatus.ONHOLD; 255 if ("completed".equals(codeString)) 256 return MedicationDispenseStatus.COMPLETED; 257 if ("entered-in-error".equals(codeString)) 258 return MedicationDispenseStatus.ENTEREDINERROR; 259 if ("stopped".equals(codeString)) 260 return MedicationDispenseStatus.STOPPED; 261 if ("declined".equals(codeString)) 262 return MedicationDispenseStatus.DECLINED; 263 if ("unknown".equals(codeString)) 264 return MedicationDispenseStatus.UNKNOWN; 265 throw new IllegalArgumentException("Unknown MedicationDispenseStatus code '" + codeString + "'"); 266 } 267 268 public Enumeration<MedicationDispenseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 269 if (code == null) 270 return null; 271 if (code.isEmpty()) 272 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.NULL, code); 273 String codeString = code.asStringValue(); 274 if (codeString == null || "".equals(codeString)) 275 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.NULL, code); 276 if ("preparation".equals(codeString)) 277 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.PREPARATION, code); 278 if ("in-progress".equals(codeString)) 279 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.INPROGRESS, code); 280 if ("cancelled".equals(codeString)) 281 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.CANCELLED, code); 282 if ("on-hold".equals(codeString)) 283 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.ONHOLD, code); 284 if ("completed".equals(codeString)) 285 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.COMPLETED, code); 286 if ("entered-in-error".equals(codeString)) 287 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.ENTEREDINERROR, code); 288 if ("stopped".equals(codeString)) 289 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.STOPPED, code); 290 if ("declined".equals(codeString)) 291 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.DECLINED, code); 292 if ("unknown".equals(codeString)) 293 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.UNKNOWN, code); 294 throw new FHIRException("Unknown MedicationDispenseStatus code '" + codeString + "'"); 295 } 296 297 public String toCode(MedicationDispenseStatus code) { 298 if (code == MedicationDispenseStatus.NULL) 299 return null; 300 if (code == MedicationDispenseStatus.PREPARATION) 301 return "preparation"; 302 if (code == MedicationDispenseStatus.INPROGRESS) 303 return "in-progress"; 304 if (code == MedicationDispenseStatus.CANCELLED) 305 return "cancelled"; 306 if (code == MedicationDispenseStatus.ONHOLD) 307 return "on-hold"; 308 if (code == MedicationDispenseStatus.COMPLETED) 309 return "completed"; 310 if (code == MedicationDispenseStatus.ENTEREDINERROR) 311 return "entered-in-error"; 312 if (code == MedicationDispenseStatus.STOPPED) 313 return "stopped"; 314 if (code == MedicationDispenseStatus.DECLINED) 315 return "declined"; 316 if (code == MedicationDispenseStatus.UNKNOWN) 317 return "unknown"; 318 return "?"; 319 } 320 321 public String toSystem(MedicationDispenseStatus code) { 322 return code.getSystem(); 323 } 324 } 325 326 @Block() 327 public static class MedicationDispensePerformerComponent extends BackboneElement implements IBaseBackboneElement { 328 /** 329 * Distinguishes the type of performer in the dispense. For example, date 330 * enterer, packager, final checker. 331 */ 332 @Child(name = "function", type = { 333 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 334 @Description(shortDefinition = "Who performed the dispense and what they did", formalDefinition = "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.") 335 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationdispense-performer-function") 336 protected CodeableConcept function; 337 338 /** 339 * The device, practitioner, etc. who performed the action. It should be assumed 340 * that the actor is the dispenser of the medication. 341 */ 342 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 343 Device.class, RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 344 @Description(shortDefinition = "Individual who was performing", formalDefinition = "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.") 345 protected Reference actor; 346 347 /** 348 * The actual object that is the target of the reference (The device, 349 * practitioner, etc. who performed the action. It should be assumed that the 350 * actor is the dispenser of the medication.) 351 */ 352 protected Resource actorTarget; 353 354 private static final long serialVersionUID = 1424001049L; 355 356 /** 357 * Constructor 358 */ 359 public MedicationDispensePerformerComponent() { 360 super(); 361 } 362 363 /** 364 * Constructor 365 */ 366 public MedicationDispensePerformerComponent(Reference actor) { 367 super(); 368 this.actor = actor; 369 } 370 371 /** 372 * @return {@link #function} (Distinguishes the type of performer in the 373 * dispense. For example, date enterer, packager, final checker.) 374 */ 375 public CodeableConcept getFunction() { 376 if (this.function == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.function"); 379 else if (Configuration.doAutoCreate()) 380 this.function = new CodeableConcept(); // cc 381 return this.function; 382 } 383 384 public boolean hasFunction() { 385 return this.function != null && !this.function.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #function} (Distinguishes the type of performer in the 390 * dispense. For example, date enterer, packager, final checker.) 391 */ 392 public MedicationDispensePerformerComponent setFunction(CodeableConcept value) { 393 this.function = value; 394 return this; 395 } 396 397 /** 398 * @return {@link #actor} (The device, practitioner, etc. who performed the 399 * action. It should be assumed that the actor is the dispenser of the 400 * medication.) 401 */ 402 public Reference getActor() { 403 if (this.actor == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.actor"); 406 else if (Configuration.doAutoCreate()) 407 this.actor = new Reference(); // cc 408 return this.actor; 409 } 410 411 public boolean hasActor() { 412 return this.actor != null && !this.actor.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #actor} (The device, practitioner, etc. who performed the 417 * action. It should be assumed that the actor is the dispenser of 418 * the medication.) 419 */ 420 public MedicationDispensePerformerComponent setActor(Reference value) { 421 this.actor = value; 422 return this; 423 } 424 425 /** 426 * @return {@link #actor} The actual object that is the target of the reference. 427 * The reference library doesn't populate this, but you can use it to 428 * hold the resource if you resolve it. (The device, practitioner, etc. 429 * who performed the action. It should be assumed that the actor is the 430 * dispenser of the medication.) 431 */ 432 public Resource getActorTarget() { 433 return this.actorTarget; 434 } 435 436 /** 437 * @param value {@link #actor} The actual object that is the target of the 438 * reference. The reference library doesn't use these, but you can 439 * use it to hold the resource if you resolve it. (The device, 440 * practitioner, etc. who performed the action. It should be 441 * assumed that the actor is the dispenser of the medication.) 442 */ 443 public MedicationDispensePerformerComponent setActorTarget(Resource value) { 444 this.actorTarget = value; 445 return this; 446 } 447 448 protected void listChildren(List<Property> children) { 449 super.listChildren(children); 450 children.add(new Property("function", "CodeableConcept", 451 "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.", 452 0, 1, function)); 453 children.add(new Property("actor", 454 "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", 455 "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.", 456 0, 1, actor)); 457 } 458 459 @Override 460 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 461 switch (_hash) { 462 case 1380938712: 463 /* function */ return new Property("function", "CodeableConcept", 464 "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.", 465 0, 1, function); 466 case 92645877: 467 /* actor */ return new Property("actor", 468 "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson)", 469 "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.", 470 0, 1, actor); 471 default: 472 return super.getNamedProperty(_hash, _name, _checkValid); 473 } 474 475 } 476 477 @Override 478 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 479 switch (hash) { 480 case 1380938712: 481 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 482 case 92645877: 483 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 484 default: 485 return super.getProperty(hash, name, checkValid); 486 } 487 488 } 489 490 @Override 491 public Base setProperty(int hash, String name, Base value) throws FHIRException { 492 switch (hash) { 493 case 1380938712: // function 494 this.function = castToCodeableConcept(value); // CodeableConcept 495 return value; 496 case 92645877: // actor 497 this.actor = castToReference(value); // Reference 498 return value; 499 default: 500 return super.setProperty(hash, name, value); 501 } 502 503 } 504 505 @Override 506 public Base setProperty(String name, Base value) throws FHIRException { 507 if (name.equals("function")) { 508 this.function = castToCodeableConcept(value); // CodeableConcept 509 } else if (name.equals("actor")) { 510 this.actor = castToReference(value); // Reference 511 } else 512 return super.setProperty(name, value); 513 return value; 514 } 515 516 @Override 517 public void removeChild(String name, Base value) throws FHIRException { 518 if (name.equals("function")) { 519 this.function = null; 520 } else if (name.equals("actor")) { 521 this.actor = null; 522 } else 523 super.removeChild(name, value); 524 525 } 526 527 @Override 528 public Base makeProperty(int hash, String name) throws FHIRException { 529 switch (hash) { 530 case 1380938712: 531 return getFunction(); 532 case 92645877: 533 return getActor(); 534 default: 535 return super.makeProperty(hash, name); 536 } 537 538 } 539 540 @Override 541 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 542 switch (hash) { 543 case 1380938712: 544 /* function */ return new String[] { "CodeableConcept" }; 545 case 92645877: 546 /* actor */ return new String[] { "Reference" }; 547 default: 548 return super.getTypesForProperty(hash, name); 549 } 550 551 } 552 553 @Override 554 public Base addChild(String name) throws FHIRException { 555 if (name.equals("function")) { 556 this.function = new CodeableConcept(); 557 return this.function; 558 } else if (name.equals("actor")) { 559 this.actor = new Reference(); 560 return this.actor; 561 } else 562 return super.addChild(name); 563 } 564 565 public MedicationDispensePerformerComponent copy() { 566 MedicationDispensePerformerComponent dst = new MedicationDispensePerformerComponent(); 567 copyValues(dst); 568 return dst; 569 } 570 571 public void copyValues(MedicationDispensePerformerComponent dst) { 572 super.copyValues(dst); 573 dst.function = function == null ? null : function.copy(); 574 dst.actor = actor == null ? null : actor.copy(); 575 } 576 577 @Override 578 public boolean equalsDeep(Base other_) { 579 if (!super.equalsDeep(other_)) 580 return false; 581 if (!(other_ instanceof MedicationDispensePerformerComponent)) 582 return false; 583 MedicationDispensePerformerComponent o = (MedicationDispensePerformerComponent) other_; 584 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 585 } 586 587 @Override 588 public boolean equalsShallow(Base other_) { 589 if (!super.equalsShallow(other_)) 590 return false; 591 if (!(other_ instanceof MedicationDispensePerformerComponent)) 592 return false; 593 MedicationDispensePerformerComponent o = (MedicationDispensePerformerComponent) other_; 594 return true; 595 } 596 597 public boolean isEmpty() { 598 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 599 } 600 601 public String fhirType() { 602 return "MedicationDispense.performer"; 603 604 } 605 606 } 607 608 @Block() 609 public static class MedicationDispenseSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 610 /** 611 * True if the dispenser dispensed a different drug or product from what was 612 * prescribed. 613 */ 614 @Child(name = "wasSubstituted", type = { 615 BooleanType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 616 @Description(shortDefinition = "Whether a substitution was or was not performed on the dispense", formalDefinition = "True if the dispenser dispensed a different drug or product from what was prescribed.") 617 protected BooleanType wasSubstituted; 618 619 /** 620 * A code signifying whether a different drug was dispensed from what was 621 * prescribed. 622 */ 623 @Child(name = "type", type = { 624 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 625 @Description(shortDefinition = "Code signifying whether a different drug was dispensed from what was prescribed", formalDefinition = "A code signifying whether a different drug was dispensed from what was prescribed.") 626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActSubstanceAdminSubstitutionCode") 627 protected CodeableConcept type; 628 629 /** 630 * Indicates the reason for the substitution (or lack of substitution) from what 631 * was prescribed. 632 */ 633 @Child(name = "reason", type = { 634 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 635 @Description(shortDefinition = "Why was substitution made", formalDefinition = "Indicates the reason for the substitution (or lack of substitution) from what was prescribed.") 636 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-SubstanceAdminSubstitutionReason") 637 protected List<CodeableConcept> reason; 638 639 /** 640 * The person or organization that has primary responsibility for the 641 * substitution. 642 */ 643 @Child(name = "responsibleParty", type = { Practitioner.class, 644 PractitionerRole.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 645 @Description(shortDefinition = "Who is responsible for the substitution", formalDefinition = "The person or organization that has primary responsibility for the substitution.") 646 protected List<Reference> responsibleParty; 647 /** 648 * The actual objects that are the target of the reference (The person or 649 * organization that has primary responsibility for the substitution.) 650 */ 651 protected List<Resource> responsiblePartyTarget; 652 653 private static final long serialVersionUID = 357914571L; 654 655 /** 656 * Constructor 657 */ 658 public MedicationDispenseSubstitutionComponent() { 659 super(); 660 } 661 662 /** 663 * Constructor 664 */ 665 public MedicationDispenseSubstitutionComponent(BooleanType wasSubstituted) { 666 super(); 667 this.wasSubstituted = wasSubstituted; 668 } 669 670 /** 671 * @return {@link #wasSubstituted} (True if the dispenser dispensed a different 672 * drug or product from what was prescribed.). This is the underlying 673 * object with id, value and extensions. The accessor 674 * "getWasSubstituted" gives direct access to the value 675 */ 676 public BooleanType getWasSubstitutedElement() { 677 if (this.wasSubstituted == null) 678 if (Configuration.errorOnAutoCreate()) 679 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.wasSubstituted"); 680 else if (Configuration.doAutoCreate()) 681 this.wasSubstituted = new BooleanType(); // bb 682 return this.wasSubstituted; 683 } 684 685 public boolean hasWasSubstitutedElement() { 686 return this.wasSubstituted != null && !this.wasSubstituted.isEmpty(); 687 } 688 689 public boolean hasWasSubstituted() { 690 return this.wasSubstituted != null && !this.wasSubstituted.isEmpty(); 691 } 692 693 /** 694 * @param value {@link #wasSubstituted} (True if the dispenser dispensed a 695 * different drug or product from what was prescribed.). This is 696 * the underlying object with id, value and extensions. The 697 * accessor "getWasSubstituted" gives direct access to the value 698 */ 699 public MedicationDispenseSubstitutionComponent setWasSubstitutedElement(BooleanType value) { 700 this.wasSubstituted = value; 701 return this; 702 } 703 704 /** 705 * @return True if the dispenser dispensed a different drug or product from what 706 * was prescribed. 707 */ 708 public boolean getWasSubstituted() { 709 return this.wasSubstituted == null || this.wasSubstituted.isEmpty() ? false : this.wasSubstituted.getValue(); 710 } 711 712 /** 713 * @param value True if the dispenser dispensed a different drug or product from 714 * what was prescribed. 715 */ 716 public MedicationDispenseSubstitutionComponent setWasSubstituted(boolean value) { 717 if (this.wasSubstituted == null) 718 this.wasSubstituted = new BooleanType(); 719 this.wasSubstituted.setValue(value); 720 return this; 721 } 722 723 /** 724 * @return {@link #type} (A code signifying whether a different drug was 725 * dispensed from what was prescribed.) 726 */ 727 public CodeableConcept getType() { 728 if (this.type == null) 729 if (Configuration.errorOnAutoCreate()) 730 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.type"); 731 else if (Configuration.doAutoCreate()) 732 this.type = new CodeableConcept(); // cc 733 return this.type; 734 } 735 736 public boolean hasType() { 737 return this.type != null && !this.type.isEmpty(); 738 } 739 740 /** 741 * @param value {@link #type} (A code signifying whether a different drug was 742 * dispensed from what was prescribed.) 743 */ 744 public MedicationDispenseSubstitutionComponent setType(CodeableConcept value) { 745 this.type = value; 746 return this; 747 } 748 749 /** 750 * @return {@link #reason} (Indicates the reason for the substitution (or lack 751 * of substitution) from what was prescribed.) 752 */ 753 public List<CodeableConcept> getReason() { 754 if (this.reason == null) 755 this.reason = new ArrayList<CodeableConcept>(); 756 return this.reason; 757 } 758 759 /** 760 * @return Returns a reference to <code>this</code> for easy method chaining 761 */ 762 public MedicationDispenseSubstitutionComponent setReason(List<CodeableConcept> theReason) { 763 this.reason = theReason; 764 return this; 765 } 766 767 public boolean hasReason() { 768 if (this.reason == null) 769 return false; 770 for (CodeableConcept item : this.reason) 771 if (!item.isEmpty()) 772 return true; 773 return false; 774 } 775 776 public CodeableConcept addReason() { // 3 777 CodeableConcept t = new CodeableConcept(); 778 if (this.reason == null) 779 this.reason = new ArrayList<CodeableConcept>(); 780 this.reason.add(t); 781 return t; 782 } 783 784 public MedicationDispenseSubstitutionComponent addReason(CodeableConcept t) { // 3 785 if (t == null) 786 return this; 787 if (this.reason == null) 788 this.reason = new ArrayList<CodeableConcept>(); 789 this.reason.add(t); 790 return this; 791 } 792 793 /** 794 * @return The first repetition of repeating field {@link #reason}, creating it 795 * if it does not already exist 796 */ 797 public CodeableConcept getReasonFirstRep() { 798 if (getReason().isEmpty()) { 799 addReason(); 800 } 801 return getReason().get(0); 802 } 803 804 /** 805 * @return {@link #responsibleParty} (The person or organization that has 806 * primary responsibility for the substitution.) 807 */ 808 public List<Reference> getResponsibleParty() { 809 if (this.responsibleParty == null) 810 this.responsibleParty = new ArrayList<Reference>(); 811 return this.responsibleParty; 812 } 813 814 /** 815 * @return Returns a reference to <code>this</code> for easy method chaining 816 */ 817 public MedicationDispenseSubstitutionComponent setResponsibleParty(List<Reference> theResponsibleParty) { 818 this.responsibleParty = theResponsibleParty; 819 return this; 820 } 821 822 public boolean hasResponsibleParty() { 823 if (this.responsibleParty == null) 824 return false; 825 for (Reference item : this.responsibleParty) 826 if (!item.isEmpty()) 827 return true; 828 return false; 829 } 830 831 public Reference addResponsibleParty() { // 3 832 Reference t = new Reference(); 833 if (this.responsibleParty == null) 834 this.responsibleParty = new ArrayList<Reference>(); 835 this.responsibleParty.add(t); 836 return t; 837 } 838 839 public MedicationDispenseSubstitutionComponent addResponsibleParty(Reference t) { // 3 840 if (t == null) 841 return this; 842 if (this.responsibleParty == null) 843 this.responsibleParty = new ArrayList<Reference>(); 844 this.responsibleParty.add(t); 845 return this; 846 } 847 848 /** 849 * @return The first repetition of repeating field {@link #responsibleParty}, 850 * creating it if it does not already exist 851 */ 852 public Reference getResponsiblePartyFirstRep() { 853 if (getResponsibleParty().isEmpty()) { 854 addResponsibleParty(); 855 } 856 return getResponsibleParty().get(0); 857 } 858 859 /** 860 * @deprecated Use Reference#setResource(IBaseResource) instead 861 */ 862 @Deprecated 863 public List<Resource> getResponsiblePartyTarget() { 864 if (this.responsiblePartyTarget == null) 865 this.responsiblePartyTarget = new ArrayList<Resource>(); 866 return this.responsiblePartyTarget; 867 } 868 869 protected void listChildren(List<Property> children) { 870 super.listChildren(children); 871 children.add(new Property("wasSubstituted", "boolean", 872 "True if the dispenser dispensed a different drug or product from what was prescribed.", 0, 1, 873 wasSubstituted)); 874 children.add(new Property("type", "CodeableConcept", 875 "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1, type)); 876 children.add(new Property("reason", "CodeableConcept", 877 "Indicates the reason for the substitution (or lack of substitution) from what was prescribed.", 0, 878 java.lang.Integer.MAX_VALUE, reason)); 879 children.add(new Property("responsibleParty", "Reference(Practitioner|PractitionerRole)", 880 "The person or organization that has primary responsibility for the substitution.", 0, 881 java.lang.Integer.MAX_VALUE, responsibleParty)); 882 } 883 884 @Override 885 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 886 switch (_hash) { 887 case -592113567: 888 /* wasSubstituted */ return new Property("wasSubstituted", "boolean", 889 "True if the dispenser dispensed a different drug or product from what was prescribed.", 0, 1, 890 wasSubstituted); 891 case 3575610: 892 /* type */ return new Property("type", "CodeableConcept", 893 "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1, type); 894 case -934964668: 895 /* reason */ return new Property("reason", "CodeableConcept", 896 "Indicates the reason for the substitution (or lack of substitution) from what was prescribed.", 0, 897 java.lang.Integer.MAX_VALUE, reason); 898 case 1511509392: 899 /* responsibleParty */ return new Property("responsibleParty", "Reference(Practitioner|PractitionerRole)", 900 "The person or organization that has primary responsibility for the substitution.", 0, 901 java.lang.Integer.MAX_VALUE, responsibleParty); 902 default: 903 return super.getNamedProperty(_hash, _name, _checkValid); 904 } 905 906 } 907 908 @Override 909 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 910 switch (hash) { 911 case -592113567: 912 /* wasSubstituted */ return this.wasSubstituted == null ? new Base[0] : new Base[] { this.wasSubstituted }; // BooleanType 913 case 3575610: 914 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 915 case -934964668: 916 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 917 case 1511509392: 918 /* responsibleParty */ return this.responsibleParty == null ? new Base[0] 919 : this.responsibleParty.toArray(new Base[this.responsibleParty.size()]); // Reference 920 default: 921 return super.getProperty(hash, name, checkValid); 922 } 923 924 } 925 926 @Override 927 public Base setProperty(int hash, String name, Base value) throws FHIRException { 928 switch (hash) { 929 case -592113567: // wasSubstituted 930 this.wasSubstituted = castToBoolean(value); // BooleanType 931 return value; 932 case 3575610: // type 933 this.type = castToCodeableConcept(value); // CodeableConcept 934 return value; 935 case -934964668: // reason 936 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 937 return value; 938 case 1511509392: // responsibleParty 939 this.getResponsibleParty().add(castToReference(value)); // Reference 940 return value; 941 default: 942 return super.setProperty(hash, name, value); 943 } 944 945 } 946 947 @Override 948 public Base setProperty(String name, Base value) throws FHIRException { 949 if (name.equals("wasSubstituted")) { 950 this.wasSubstituted = castToBoolean(value); // BooleanType 951 } else if (name.equals("type")) { 952 this.type = castToCodeableConcept(value); // CodeableConcept 953 } else if (name.equals("reason")) { 954 this.getReason().add(castToCodeableConcept(value)); 955 } else if (name.equals("responsibleParty")) { 956 this.getResponsibleParty().add(castToReference(value)); 957 } else 958 return super.setProperty(name, value); 959 return value; 960 } 961 962 @Override 963 public void removeChild(String name, Base value) throws FHIRException { 964 if (name.equals("wasSubstituted")) { 965 this.wasSubstituted = null; 966 } else if (name.equals("type")) { 967 this.type = null; 968 } else if (name.equals("reason")) { 969 this.getReason().remove(castToCodeableConcept(value)); 970 } else if (name.equals("responsibleParty")) { 971 this.getResponsibleParty().remove(castToReference(value)); 972 } else 973 super.removeChild(name, value); 974 975 } 976 977 @Override 978 public Base makeProperty(int hash, String name) throws FHIRException { 979 switch (hash) { 980 case -592113567: 981 return getWasSubstitutedElement(); 982 case 3575610: 983 return getType(); 984 case -934964668: 985 return addReason(); 986 case 1511509392: 987 return addResponsibleParty(); 988 default: 989 return super.makeProperty(hash, name); 990 } 991 992 } 993 994 @Override 995 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 996 switch (hash) { 997 case -592113567: 998 /* wasSubstituted */ return new String[] { "boolean" }; 999 case 3575610: 1000 /* type */ return new String[] { "CodeableConcept" }; 1001 case -934964668: 1002 /* reason */ return new String[] { "CodeableConcept" }; 1003 case 1511509392: 1004 /* responsibleParty */ return new String[] { "Reference" }; 1005 default: 1006 return super.getTypesForProperty(hash, name); 1007 } 1008 1009 } 1010 1011 @Override 1012 public Base addChild(String name) throws FHIRException { 1013 if (name.equals("wasSubstituted")) { 1014 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.wasSubstituted"); 1015 } else if (name.equals("type")) { 1016 this.type = new CodeableConcept(); 1017 return this.type; 1018 } else if (name.equals("reason")) { 1019 return addReason(); 1020 } else if (name.equals("responsibleParty")) { 1021 return addResponsibleParty(); 1022 } else 1023 return super.addChild(name); 1024 } 1025 1026 public MedicationDispenseSubstitutionComponent copy() { 1027 MedicationDispenseSubstitutionComponent dst = new MedicationDispenseSubstitutionComponent(); 1028 copyValues(dst); 1029 return dst; 1030 } 1031 1032 public void copyValues(MedicationDispenseSubstitutionComponent dst) { 1033 super.copyValues(dst); 1034 dst.wasSubstituted = wasSubstituted == null ? null : wasSubstituted.copy(); 1035 dst.type = type == null ? null : type.copy(); 1036 if (reason != null) { 1037 dst.reason = new ArrayList<CodeableConcept>(); 1038 for (CodeableConcept i : reason) 1039 dst.reason.add(i.copy()); 1040 } 1041 ; 1042 if (responsibleParty != null) { 1043 dst.responsibleParty = new ArrayList<Reference>(); 1044 for (Reference i : responsibleParty) 1045 dst.responsibleParty.add(i.copy()); 1046 } 1047 ; 1048 } 1049 1050 @Override 1051 public boolean equalsDeep(Base other_) { 1052 if (!super.equalsDeep(other_)) 1053 return false; 1054 if (!(other_ instanceof MedicationDispenseSubstitutionComponent)) 1055 return false; 1056 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other_; 1057 return compareDeep(wasSubstituted, o.wasSubstituted, true) && compareDeep(type, o.type, true) 1058 && compareDeep(reason, o.reason, true) && compareDeep(responsibleParty, o.responsibleParty, true); 1059 } 1060 1061 @Override 1062 public boolean equalsShallow(Base other_) { 1063 if (!super.equalsShallow(other_)) 1064 return false; 1065 if (!(other_ instanceof MedicationDispenseSubstitutionComponent)) 1066 return false; 1067 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other_; 1068 return compareValues(wasSubstituted, o.wasSubstituted, true); 1069 } 1070 1071 public boolean isEmpty() { 1072 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(wasSubstituted, type, reason, responsibleParty); 1073 } 1074 1075 public String fhirType() { 1076 return "MedicationDispense.substitution"; 1077 1078 } 1079 1080 } 1081 1082 /** 1083 * Identifiers associated with this Medication Dispense that are defined by 1084 * business processes and/or used to refer to it when a direct URL reference to 1085 * the resource itself is not appropriate. They are business identifiers 1086 * assigned to this resource by the performer or other systems and remain 1087 * constant as the resource is updated and propagates from server to server. 1088 */ 1089 @Child(name = "identifier", type = { 1090 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1091 @Description(shortDefinition = "External identifier", formalDefinition = "Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.") 1092 protected List<Identifier> identifier; 1093 1094 /** 1095 * The procedure that trigger the dispense. 1096 */ 1097 @Child(name = "partOf", type = { 1098 Procedure.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1099 @Description(shortDefinition = "Event that dispense is part of", formalDefinition = "The procedure that trigger the dispense.") 1100 protected List<Reference> partOf; 1101 /** 1102 * The actual objects that are the target of the reference (The procedure that 1103 * trigger the dispense.) 1104 */ 1105 protected List<Procedure> partOfTarget; 1106 1107 /** 1108 * A code specifying the state of the set of dispense events. 1109 */ 1110 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 1111 @Description(shortDefinition = "preparation | in-progress | cancelled | on-hold | completed | entered-in-error | stopped | declined | unknown", formalDefinition = "A code specifying the state of the set of dispense events.") 1112 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationdispense-status") 1113 protected Enumeration<MedicationDispenseStatus> status; 1114 1115 /** 1116 * Indicates the reason why a dispense was not performed. 1117 */ 1118 @Child(name = "statusReason", type = { CodeableConcept.class, 1119 DetectedIssue.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1120 @Description(shortDefinition = "Why a dispense was not performed", formalDefinition = "Indicates the reason why a dispense was not performed.") 1121 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationdispense-status-reason") 1122 protected Type statusReason; 1123 1124 /** 1125 * Indicates the type of medication dispense (for example, where the medication 1126 * is expected to be consumed or administered (i.e. inpatient or outpatient)). 1127 */ 1128 @Child(name = "category", type = { 1129 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1130 @Description(shortDefinition = "Type of medication dispense", formalDefinition = "Indicates the type of medication dispense (for example, where the medication is expected to be consumed or administered (i.e. inpatient or outpatient)).") 1131 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationdispense-category") 1132 protected CodeableConcept category; 1133 1134 /** 1135 * Identifies the medication being administered. This is either a link to a 1136 * resource representing the details of the medication or a simple attribute 1137 * carrying a code that identifies the medication from a known list of 1138 * medications. 1139 */ 1140 @Child(name = "medication", type = { CodeableConcept.class, 1141 Medication.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 1142 @Description(shortDefinition = "What medication was supplied", formalDefinition = "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 1143 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1144 protected Type medication; 1145 1146 /** 1147 * A link to a resource representing the person or the group to whom the 1148 * medication will be given. 1149 */ 1150 @Child(name = "subject", type = { Patient.class, 1151 Group.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1152 @Description(shortDefinition = "Who the dispense is for", formalDefinition = "A link to a resource representing the person or the group to whom the medication will be given.") 1153 protected Reference subject; 1154 1155 /** 1156 * The actual object that is the target of the reference (A link to a resource 1157 * representing the person or the group to whom the medication will be given.) 1158 */ 1159 protected Resource subjectTarget; 1160 1161 /** 1162 * The encounter or episode of care that establishes the context for this event. 1163 */ 1164 @Child(name = "context", type = { Encounter.class, 1165 EpisodeOfCare.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1166 @Description(shortDefinition = "Encounter / Episode associated with event", formalDefinition = "The encounter or episode of care that establishes the context for this event.") 1167 protected Reference context; 1168 1169 /** 1170 * The actual object that is the target of the reference (The encounter or 1171 * episode of care that establishes the context for this event.) 1172 */ 1173 protected Resource contextTarget; 1174 1175 /** 1176 * Additional information that supports the medication being dispensed. 1177 */ 1178 @Child(name = "supportingInformation", type = { 1179 Reference.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1180 @Description(shortDefinition = "Information that supports the dispensing of the medication", formalDefinition = "Additional information that supports the medication being dispensed.") 1181 protected List<Reference> supportingInformation; 1182 /** 1183 * The actual objects that are the target of the reference (Additional 1184 * information that supports the medication being dispensed.) 1185 */ 1186 protected List<Resource> supportingInformationTarget; 1187 1188 /** 1189 * Indicates who or what performed the event. 1190 */ 1191 @Child(name = "performer", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1192 @Description(shortDefinition = "Who performed event", formalDefinition = "Indicates who or what performed the event.") 1193 protected List<MedicationDispensePerformerComponent> performer; 1194 1195 /** 1196 * The principal physical location where the dispense was performed. 1197 */ 1198 @Child(name = "location", type = { Location.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1199 @Description(shortDefinition = "Where the dispense occurred", formalDefinition = "The principal physical location where the dispense was performed.") 1200 protected Reference location; 1201 1202 /** 1203 * The actual object that is the target of the reference (The principal physical 1204 * location where the dispense was performed.) 1205 */ 1206 protected Location locationTarget; 1207 1208 /** 1209 * Indicates the medication order that is being dispensed against. 1210 */ 1211 @Child(name = "authorizingPrescription", type = { 1212 MedicationRequest.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1213 @Description(shortDefinition = "Medication order that authorizes the dispense", formalDefinition = "Indicates the medication order that is being dispensed against.") 1214 protected List<Reference> authorizingPrescription; 1215 /** 1216 * The actual objects that are the target of the reference (Indicates the 1217 * medication order that is being dispensed against.) 1218 */ 1219 protected List<MedicationRequest> authorizingPrescriptionTarget; 1220 1221 /** 1222 * Indicates the type of dispensing event that is performed. For example, Trial 1223 * Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 1224 */ 1225 @Child(name = "type", type = { 1226 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1227 @Description(shortDefinition = "Trial fill, partial fill, emergency fill, etc.", formalDefinition = "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.") 1228 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActPharmacySupplyType") 1229 protected CodeableConcept type; 1230 1231 /** 1232 * The amount of medication that has been dispensed. Includes unit of measure. 1233 */ 1234 @Child(name = "quantity", type = { Quantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1235 @Description(shortDefinition = "Amount dispensed", formalDefinition = "The amount of medication that has been dispensed. Includes unit of measure.") 1236 protected Quantity quantity; 1237 1238 /** 1239 * The amount of medication expressed as a timing amount. 1240 */ 1241 @Child(name = "daysSupply", type = { 1242 Quantity.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1243 @Description(shortDefinition = "Amount of medication expressed as a timing amount", formalDefinition = "The amount of medication expressed as a timing amount.") 1244 protected Quantity daysSupply; 1245 1246 /** 1247 * The time when the dispensed product was packaged and reviewed. 1248 */ 1249 @Child(name = "whenPrepared", type = { 1250 DateTimeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 1251 @Description(shortDefinition = "When product was packaged and reviewed", formalDefinition = "The time when the dispensed product was packaged and reviewed.") 1252 protected DateTimeType whenPrepared; 1253 1254 /** 1255 * The time the dispensed product was provided to the patient or their 1256 * representative. 1257 */ 1258 @Child(name = "whenHandedOver", type = { 1259 DateTimeType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1260 @Description(shortDefinition = "When product was given out", formalDefinition = "The time the dispensed product was provided to the patient or their representative.") 1261 protected DateTimeType whenHandedOver; 1262 1263 /** 1264 * Identification of the facility/location where the medication was shipped to, 1265 * as part of the dispense event. 1266 */ 1267 @Child(name = "destination", type = { 1268 Location.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1269 @Description(shortDefinition = "Where the medication was sent", formalDefinition = "Identification of the facility/location where the medication was shipped to, as part of the dispense event.") 1270 protected Reference destination; 1271 1272 /** 1273 * The actual object that is the target of the reference (Identification of the 1274 * facility/location where the medication was shipped to, as part of the 1275 * dispense event.) 1276 */ 1277 protected Location destinationTarget; 1278 1279 /** 1280 * Identifies the person who picked up the medication. This will usually be a 1281 * patient or their caregiver, but some cases exist where it can be a healthcare 1282 * professional. 1283 */ 1284 @Child(name = "receiver", type = { Patient.class, 1285 Practitioner.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1286 @Description(shortDefinition = "Who collected the medication", formalDefinition = "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.") 1287 protected List<Reference> receiver; 1288 /** 1289 * The actual objects that are the target of the reference (Identifies the 1290 * person who picked up the medication. This will usually be a patient or their 1291 * caregiver, but some cases exist where it can be a healthcare professional.) 1292 */ 1293 protected List<Resource> receiverTarget; 1294 1295 /** 1296 * Extra information about the dispense that could not be conveyed in the other 1297 * attributes. 1298 */ 1299 @Child(name = "note", type = { 1300 Annotation.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1301 @Description(shortDefinition = "Information about the dispense", formalDefinition = "Extra information about the dispense that could not be conveyed in the other attributes.") 1302 protected List<Annotation> note; 1303 1304 /** 1305 * Indicates how the medication is to be used by the patient. 1306 */ 1307 @Child(name = "dosageInstruction", type = { 1308 Dosage.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1309 @Description(shortDefinition = "How the medication is to be used by the patient or administered by the caregiver", formalDefinition = "Indicates how the medication is to be used by the patient.") 1310 protected List<Dosage> dosageInstruction; 1311 1312 /** 1313 * Indicates whether or not substitution was made as part of the dispense. In 1314 * some cases, substitution will be expected but does not happen, in other cases 1315 * substitution is not expected but does happen. This block explains what 1316 * substitution did or did not happen and why. If nothing is specified, 1317 * substitution was not done. 1318 */ 1319 @Child(name = "substitution", type = {}, order = 21, min = 0, max = 1, modifier = false, summary = false) 1320 @Description(shortDefinition = "Whether a substitution was performed on the dispense", formalDefinition = "Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.") 1321 protected MedicationDispenseSubstitutionComponent substitution; 1322 1323 /** 1324 * Indicates an actual or potential clinical issue with or between one or more 1325 * active or proposed clinical actions for a patient; e.g. drug-drug 1326 * interaction, duplicate therapy, dosage alert etc. 1327 */ 1328 @Child(name = "detectedIssue", type = { 1329 DetectedIssue.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1330 @Description(shortDefinition = "Clinical issue with action", formalDefinition = "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. drug-drug interaction, duplicate therapy, dosage alert etc.") 1331 protected List<Reference> detectedIssue; 1332 /** 1333 * The actual objects that are the target of the reference (Indicates an actual 1334 * or potential clinical issue with or between one or more active or proposed 1335 * clinical actions for a patient; e.g. drug-drug interaction, duplicate 1336 * therapy, dosage alert etc.) 1337 */ 1338 protected List<DetectedIssue> detectedIssueTarget; 1339 1340 /** 1341 * A summary of the events of interest that have occurred, such as when the 1342 * dispense was verified. 1343 */ 1344 @Child(name = "eventHistory", type = { 1345 Provenance.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1346 @Description(shortDefinition = "A list of relevant lifecycle events", formalDefinition = "A summary of the events of interest that have occurred, such as when the dispense was verified.") 1347 protected List<Reference> eventHistory; 1348 /** 1349 * The actual objects that are the target of the reference (A summary of the 1350 * events of interest that have occurred, such as when the dispense was 1351 * verified.) 1352 */ 1353 protected List<Provenance> eventHistoryTarget; 1354 1355 private static final long serialVersionUID = -976967238L; 1356 1357 /** 1358 * Constructor 1359 */ 1360 public MedicationDispense() { 1361 super(); 1362 } 1363 1364 /** 1365 * Constructor 1366 */ 1367 public MedicationDispense(Enumeration<MedicationDispenseStatus> status, Type medication) { 1368 super(); 1369 this.status = status; 1370 this.medication = medication; 1371 } 1372 1373 /** 1374 * @return {@link #identifier} (Identifiers associated with this Medication 1375 * Dispense that are defined by business processes and/or used to refer 1376 * to it when a direct URL reference to the resource itself is not 1377 * appropriate. They are business identifiers assigned to this resource 1378 * by the performer or other systems and remain constant as the resource 1379 * is updated and propagates from server to server.) 1380 */ 1381 public List<Identifier> getIdentifier() { 1382 if (this.identifier == null) 1383 this.identifier = new ArrayList<Identifier>(); 1384 return this.identifier; 1385 } 1386 1387 /** 1388 * @return Returns a reference to <code>this</code> for easy method chaining 1389 */ 1390 public MedicationDispense setIdentifier(List<Identifier> theIdentifier) { 1391 this.identifier = theIdentifier; 1392 return this; 1393 } 1394 1395 public boolean hasIdentifier() { 1396 if (this.identifier == null) 1397 return false; 1398 for (Identifier item : this.identifier) 1399 if (!item.isEmpty()) 1400 return true; 1401 return false; 1402 } 1403 1404 public Identifier addIdentifier() { // 3 1405 Identifier t = new Identifier(); 1406 if (this.identifier == null) 1407 this.identifier = new ArrayList<Identifier>(); 1408 this.identifier.add(t); 1409 return t; 1410 } 1411 1412 public MedicationDispense addIdentifier(Identifier t) { // 3 1413 if (t == null) 1414 return this; 1415 if (this.identifier == null) 1416 this.identifier = new ArrayList<Identifier>(); 1417 this.identifier.add(t); 1418 return this; 1419 } 1420 1421 /** 1422 * @return The first repetition of repeating field {@link #identifier}, creating 1423 * it if it does not already exist 1424 */ 1425 public Identifier getIdentifierFirstRep() { 1426 if (getIdentifier().isEmpty()) { 1427 addIdentifier(); 1428 } 1429 return getIdentifier().get(0); 1430 } 1431 1432 /** 1433 * @return {@link #partOf} (The procedure that trigger the dispense.) 1434 */ 1435 public List<Reference> getPartOf() { 1436 if (this.partOf == null) 1437 this.partOf = new ArrayList<Reference>(); 1438 return this.partOf; 1439 } 1440 1441 /** 1442 * @return Returns a reference to <code>this</code> for easy method chaining 1443 */ 1444 public MedicationDispense setPartOf(List<Reference> thePartOf) { 1445 this.partOf = thePartOf; 1446 return this; 1447 } 1448 1449 public boolean hasPartOf() { 1450 if (this.partOf == null) 1451 return false; 1452 for (Reference item : this.partOf) 1453 if (!item.isEmpty()) 1454 return true; 1455 return false; 1456 } 1457 1458 public Reference addPartOf() { // 3 1459 Reference t = new Reference(); 1460 if (this.partOf == null) 1461 this.partOf = new ArrayList<Reference>(); 1462 this.partOf.add(t); 1463 return t; 1464 } 1465 1466 public MedicationDispense addPartOf(Reference t) { // 3 1467 if (t == null) 1468 return this; 1469 if (this.partOf == null) 1470 this.partOf = new ArrayList<Reference>(); 1471 this.partOf.add(t); 1472 return this; 1473 } 1474 1475 /** 1476 * @return The first repetition of repeating field {@link #partOf}, creating it 1477 * if it does not already exist 1478 */ 1479 public Reference getPartOfFirstRep() { 1480 if (getPartOf().isEmpty()) { 1481 addPartOf(); 1482 } 1483 return getPartOf().get(0); 1484 } 1485 1486 /** 1487 * @deprecated Use Reference#setResource(IBaseResource) instead 1488 */ 1489 @Deprecated 1490 public List<Procedure> getPartOfTarget() { 1491 if (this.partOfTarget == null) 1492 this.partOfTarget = new ArrayList<Procedure>(); 1493 return this.partOfTarget; 1494 } 1495 1496 /** 1497 * @deprecated Use Reference#setResource(IBaseResource) instead 1498 */ 1499 @Deprecated 1500 public Procedure addPartOfTarget() { 1501 Procedure r = new Procedure(); 1502 if (this.partOfTarget == null) 1503 this.partOfTarget = new ArrayList<Procedure>(); 1504 this.partOfTarget.add(r); 1505 return r; 1506 } 1507 1508 /** 1509 * @return {@link #status} (A code specifying the state of the set of dispense 1510 * events.). This is the underlying object with id, value and 1511 * extensions. The accessor "getStatus" gives direct access to the value 1512 */ 1513 public Enumeration<MedicationDispenseStatus> getStatusElement() { 1514 if (this.status == null) 1515 if (Configuration.errorOnAutoCreate()) 1516 throw new Error("Attempt to auto-create MedicationDispense.status"); 1517 else if (Configuration.doAutoCreate()) 1518 this.status = new Enumeration<MedicationDispenseStatus>(new MedicationDispenseStatusEnumFactory()); // bb 1519 return this.status; 1520 } 1521 1522 public boolean hasStatusElement() { 1523 return this.status != null && !this.status.isEmpty(); 1524 } 1525 1526 public boolean hasStatus() { 1527 return this.status != null && !this.status.isEmpty(); 1528 } 1529 1530 /** 1531 * @param value {@link #status} (A code specifying the state of the set of 1532 * dispense events.). This is the underlying object with id, value 1533 * and extensions. The accessor "getStatus" gives direct access to 1534 * the value 1535 */ 1536 public MedicationDispense setStatusElement(Enumeration<MedicationDispenseStatus> value) { 1537 this.status = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return A code specifying the state of the set of dispense events. 1543 */ 1544 public MedicationDispenseStatus getStatus() { 1545 return this.status == null ? null : this.status.getValue(); 1546 } 1547 1548 /** 1549 * @param value A code specifying the state of the set of dispense events. 1550 */ 1551 public MedicationDispense setStatus(MedicationDispenseStatus value) { 1552 if (this.status == null) 1553 this.status = new Enumeration<MedicationDispenseStatus>(new MedicationDispenseStatusEnumFactory()); 1554 this.status.setValue(value); 1555 return this; 1556 } 1557 1558 /** 1559 * @return {@link #statusReason} (Indicates the reason why a dispense was not 1560 * performed.) 1561 */ 1562 public Type getStatusReason() { 1563 return this.statusReason; 1564 } 1565 1566 /** 1567 * @return {@link #statusReason} (Indicates the reason why a dispense was not 1568 * performed.) 1569 */ 1570 public CodeableConcept getStatusReasonCodeableConcept() throws FHIRException { 1571 if (this.statusReason == null) 1572 this.statusReason = new CodeableConcept(); 1573 if (!(this.statusReason instanceof CodeableConcept)) 1574 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1575 + this.statusReason.getClass().getName() + " was encountered"); 1576 return (CodeableConcept) this.statusReason; 1577 } 1578 1579 public boolean hasStatusReasonCodeableConcept() { 1580 return this != null && this.statusReason instanceof CodeableConcept; 1581 } 1582 1583 /** 1584 * @return {@link #statusReason} (Indicates the reason why a dispense was not 1585 * performed.) 1586 */ 1587 public Reference getStatusReasonReference() throws FHIRException { 1588 if (this.statusReason == null) 1589 this.statusReason = new Reference(); 1590 if (!(this.statusReason instanceof Reference)) 1591 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1592 + this.statusReason.getClass().getName() + " was encountered"); 1593 return (Reference) this.statusReason; 1594 } 1595 1596 public boolean hasStatusReasonReference() { 1597 return this != null && this.statusReason instanceof Reference; 1598 } 1599 1600 public boolean hasStatusReason() { 1601 return this.statusReason != null && !this.statusReason.isEmpty(); 1602 } 1603 1604 /** 1605 * @param value {@link #statusReason} (Indicates the reason why a dispense was 1606 * not performed.) 1607 */ 1608 public MedicationDispense setStatusReason(Type value) { 1609 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1610 throw new Error("Not the right type for MedicationDispense.statusReason[x]: " + value.fhirType()); 1611 this.statusReason = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #category} (Indicates the type of medication dispense (for 1617 * example, where the medication is expected to be consumed or 1618 * administered (i.e. inpatient or outpatient)).) 1619 */ 1620 public CodeableConcept getCategory() { 1621 if (this.category == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create MedicationDispense.category"); 1624 else if (Configuration.doAutoCreate()) 1625 this.category = new CodeableConcept(); // cc 1626 return this.category; 1627 } 1628 1629 public boolean hasCategory() { 1630 return this.category != null && !this.category.isEmpty(); 1631 } 1632 1633 /** 1634 * @param value {@link #category} (Indicates the type of medication dispense 1635 * (for example, where the medication is expected to be consumed or 1636 * administered (i.e. inpatient or outpatient)).) 1637 */ 1638 public MedicationDispense setCategory(CodeableConcept value) { 1639 this.category = value; 1640 return this; 1641 } 1642 1643 /** 1644 * @return {@link #medication} (Identifies the medication being administered. 1645 * This is either a link to a resource representing the details of the 1646 * medication or a simple attribute carrying a code that identifies the 1647 * medication from a known list of medications.) 1648 */ 1649 public Type getMedication() { 1650 return this.medication; 1651 } 1652 1653 /** 1654 * @return {@link #medication} (Identifies the medication being administered. 1655 * This is either a link to a resource representing the details of the 1656 * medication or a simple attribute carrying a code that identifies the 1657 * medication from a known list of medications.) 1658 */ 1659 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1660 if (this.medication == null) 1661 this.medication = new CodeableConcept(); 1662 if (!(this.medication instanceof CodeableConcept)) 1663 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1664 + this.medication.getClass().getName() + " was encountered"); 1665 return (CodeableConcept) this.medication; 1666 } 1667 1668 public boolean hasMedicationCodeableConcept() { 1669 return this != null && this.medication instanceof CodeableConcept; 1670 } 1671 1672 /** 1673 * @return {@link #medication} (Identifies the medication being administered. 1674 * This is either a link to a resource representing the details of the 1675 * medication or a simple attribute carrying a code that identifies the 1676 * medication from a known list of medications.) 1677 */ 1678 public Reference getMedicationReference() throws FHIRException { 1679 if (this.medication == null) 1680 this.medication = new Reference(); 1681 if (!(this.medication instanceof Reference)) 1682 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1683 + this.medication.getClass().getName() + " was encountered"); 1684 return (Reference) this.medication; 1685 } 1686 1687 public boolean hasMedicationReference() { 1688 return this != null && this.medication instanceof Reference; 1689 } 1690 1691 public boolean hasMedication() { 1692 return this.medication != null && !this.medication.isEmpty(); 1693 } 1694 1695 /** 1696 * @param value {@link #medication} (Identifies the medication being 1697 * administered. This is either a link to a resource representing 1698 * the details of the medication or a simple attribute carrying a 1699 * code that identifies the medication from a known list of 1700 * medications.) 1701 */ 1702 public MedicationDispense setMedication(Type value) { 1703 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1704 throw new Error("Not the right type for MedicationDispense.medication[x]: " + value.fhirType()); 1705 this.medication = value; 1706 return this; 1707 } 1708 1709 /** 1710 * @return {@link #subject} (A link to a resource representing the person or the 1711 * group to whom the medication will be given.) 1712 */ 1713 public Reference getSubject() { 1714 if (this.subject == null) 1715 if (Configuration.errorOnAutoCreate()) 1716 throw new Error("Attempt to auto-create MedicationDispense.subject"); 1717 else if (Configuration.doAutoCreate()) 1718 this.subject = new Reference(); // cc 1719 return this.subject; 1720 } 1721 1722 public boolean hasSubject() { 1723 return this.subject != null && !this.subject.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #subject} (A link to a resource representing the person 1728 * or the group to whom the medication will be given.) 1729 */ 1730 public MedicationDispense setSubject(Reference value) { 1731 this.subject = value; 1732 return this; 1733 } 1734 1735 /** 1736 * @return {@link #subject} The actual object that is the target of the 1737 * reference. The reference library doesn't populate this, but you can 1738 * use it to hold the resource if you resolve it. (A link to a resource 1739 * representing the person or the group to whom the medication will be 1740 * given.) 1741 */ 1742 public Resource getSubjectTarget() { 1743 return this.subjectTarget; 1744 } 1745 1746 /** 1747 * @param value {@link #subject} The actual object that is the target of the 1748 * reference. The reference library doesn't use these, but you can 1749 * use it to hold the resource if you resolve it. (A link to a 1750 * resource representing the person or the group to whom the 1751 * medication will be given.) 1752 */ 1753 public MedicationDispense setSubjectTarget(Resource value) { 1754 this.subjectTarget = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return {@link #context} (The encounter or episode of care that establishes 1760 * the context for this event.) 1761 */ 1762 public Reference getContext() { 1763 if (this.context == null) 1764 if (Configuration.errorOnAutoCreate()) 1765 throw new Error("Attempt to auto-create MedicationDispense.context"); 1766 else if (Configuration.doAutoCreate()) 1767 this.context = new Reference(); // cc 1768 return this.context; 1769 } 1770 1771 public boolean hasContext() { 1772 return this.context != null && !this.context.isEmpty(); 1773 } 1774 1775 /** 1776 * @param value {@link #context} (The encounter or episode of care that 1777 * establishes the context for this event.) 1778 */ 1779 public MedicationDispense setContext(Reference value) { 1780 this.context = value; 1781 return this; 1782 } 1783 1784 /** 1785 * @return {@link #context} The actual object that is the target of the 1786 * reference. The reference library doesn't populate this, but you can 1787 * use it to hold the resource if you resolve it. (The encounter or 1788 * episode of care that establishes the context for this event.) 1789 */ 1790 public Resource getContextTarget() { 1791 return this.contextTarget; 1792 } 1793 1794 /** 1795 * @param value {@link #context} The actual object that is the target of the 1796 * reference. The reference library doesn't use these, but you can 1797 * use it to hold the resource if you resolve it. (The encounter or 1798 * episode of care that establishes the context for this event.) 1799 */ 1800 public MedicationDispense setContextTarget(Resource value) { 1801 this.contextTarget = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #supportingInformation} (Additional information that supports 1807 * the medication being dispensed.) 1808 */ 1809 public List<Reference> getSupportingInformation() { 1810 if (this.supportingInformation == null) 1811 this.supportingInformation = new ArrayList<Reference>(); 1812 return this.supportingInformation; 1813 } 1814 1815 /** 1816 * @return Returns a reference to <code>this</code> for easy method chaining 1817 */ 1818 public MedicationDispense setSupportingInformation(List<Reference> theSupportingInformation) { 1819 this.supportingInformation = theSupportingInformation; 1820 return this; 1821 } 1822 1823 public boolean hasSupportingInformation() { 1824 if (this.supportingInformation == null) 1825 return false; 1826 for (Reference item : this.supportingInformation) 1827 if (!item.isEmpty()) 1828 return true; 1829 return false; 1830 } 1831 1832 public Reference addSupportingInformation() { // 3 1833 Reference t = new Reference(); 1834 if (this.supportingInformation == null) 1835 this.supportingInformation = new ArrayList<Reference>(); 1836 this.supportingInformation.add(t); 1837 return t; 1838 } 1839 1840 public MedicationDispense addSupportingInformation(Reference t) { // 3 1841 if (t == null) 1842 return this; 1843 if (this.supportingInformation == null) 1844 this.supportingInformation = new ArrayList<Reference>(); 1845 this.supportingInformation.add(t); 1846 return this; 1847 } 1848 1849 /** 1850 * @return The first repetition of repeating field 1851 * {@link #supportingInformation}, creating it if it does not already 1852 * exist 1853 */ 1854 public Reference getSupportingInformationFirstRep() { 1855 if (getSupportingInformation().isEmpty()) { 1856 addSupportingInformation(); 1857 } 1858 return getSupportingInformation().get(0); 1859 } 1860 1861 /** 1862 * @deprecated Use Reference#setResource(IBaseResource) instead 1863 */ 1864 @Deprecated 1865 public List<Resource> getSupportingInformationTarget() { 1866 if (this.supportingInformationTarget == null) 1867 this.supportingInformationTarget = new ArrayList<Resource>(); 1868 return this.supportingInformationTarget; 1869 } 1870 1871 /** 1872 * @return {@link #performer} (Indicates who or what performed the event.) 1873 */ 1874 public List<MedicationDispensePerformerComponent> getPerformer() { 1875 if (this.performer == null) 1876 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1877 return this.performer; 1878 } 1879 1880 /** 1881 * @return Returns a reference to <code>this</code> for easy method chaining 1882 */ 1883 public MedicationDispense setPerformer(List<MedicationDispensePerformerComponent> thePerformer) { 1884 this.performer = thePerformer; 1885 return this; 1886 } 1887 1888 public boolean hasPerformer() { 1889 if (this.performer == null) 1890 return false; 1891 for (MedicationDispensePerformerComponent item : this.performer) 1892 if (!item.isEmpty()) 1893 return true; 1894 return false; 1895 } 1896 1897 public MedicationDispensePerformerComponent addPerformer() { // 3 1898 MedicationDispensePerformerComponent t = new MedicationDispensePerformerComponent(); 1899 if (this.performer == null) 1900 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1901 this.performer.add(t); 1902 return t; 1903 } 1904 1905 public MedicationDispense addPerformer(MedicationDispensePerformerComponent t) { // 3 1906 if (t == null) 1907 return this; 1908 if (this.performer == null) 1909 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1910 this.performer.add(t); 1911 return this; 1912 } 1913 1914 /** 1915 * @return The first repetition of repeating field {@link #performer}, creating 1916 * it if it does not already exist 1917 */ 1918 public MedicationDispensePerformerComponent getPerformerFirstRep() { 1919 if (getPerformer().isEmpty()) { 1920 addPerformer(); 1921 } 1922 return getPerformer().get(0); 1923 } 1924 1925 /** 1926 * @return {@link #location} (The principal physical location where the dispense 1927 * was performed.) 1928 */ 1929 public Reference getLocation() { 1930 if (this.location == null) 1931 if (Configuration.errorOnAutoCreate()) 1932 throw new Error("Attempt to auto-create MedicationDispense.location"); 1933 else if (Configuration.doAutoCreate()) 1934 this.location = new Reference(); // cc 1935 return this.location; 1936 } 1937 1938 public boolean hasLocation() { 1939 return this.location != null && !this.location.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #location} (The principal physical location where the 1944 * dispense was performed.) 1945 */ 1946 public MedicationDispense setLocation(Reference value) { 1947 this.location = value; 1948 return this; 1949 } 1950 1951 /** 1952 * @return {@link #location} The actual object that is the target of the 1953 * reference. The reference library doesn't populate this, but you can 1954 * use it to hold the resource if you resolve it. (The principal 1955 * physical location where the dispense was performed.) 1956 */ 1957 public Location getLocationTarget() { 1958 if (this.locationTarget == null) 1959 if (Configuration.errorOnAutoCreate()) 1960 throw new Error("Attempt to auto-create MedicationDispense.location"); 1961 else if (Configuration.doAutoCreate()) 1962 this.locationTarget = new Location(); // aa 1963 return this.locationTarget; 1964 } 1965 1966 /** 1967 * @param value {@link #location} The actual object that is the target of the 1968 * reference. The reference library doesn't use these, but you can 1969 * use it to hold the resource if you resolve it. (The principal 1970 * physical location where the dispense was performed.) 1971 */ 1972 public MedicationDispense setLocationTarget(Location value) { 1973 this.locationTarget = value; 1974 return this; 1975 } 1976 1977 /** 1978 * @return {@link #authorizingPrescription} (Indicates the medication order that 1979 * is being dispensed against.) 1980 */ 1981 public List<Reference> getAuthorizingPrescription() { 1982 if (this.authorizingPrescription == null) 1983 this.authorizingPrescription = new ArrayList<Reference>(); 1984 return this.authorizingPrescription; 1985 } 1986 1987 /** 1988 * @return Returns a reference to <code>this</code> for easy method chaining 1989 */ 1990 public MedicationDispense setAuthorizingPrescription(List<Reference> theAuthorizingPrescription) { 1991 this.authorizingPrescription = theAuthorizingPrescription; 1992 return this; 1993 } 1994 1995 public boolean hasAuthorizingPrescription() { 1996 if (this.authorizingPrescription == null) 1997 return false; 1998 for (Reference item : this.authorizingPrescription) 1999 if (!item.isEmpty()) 2000 return true; 2001 return false; 2002 } 2003 2004 public Reference addAuthorizingPrescription() { // 3 2005 Reference t = new Reference(); 2006 if (this.authorizingPrescription == null) 2007 this.authorizingPrescription = new ArrayList<Reference>(); 2008 this.authorizingPrescription.add(t); 2009 return t; 2010 } 2011 2012 public MedicationDispense addAuthorizingPrescription(Reference t) { // 3 2013 if (t == null) 2014 return this; 2015 if (this.authorizingPrescription == null) 2016 this.authorizingPrescription = new ArrayList<Reference>(); 2017 this.authorizingPrescription.add(t); 2018 return this; 2019 } 2020 2021 /** 2022 * @return The first repetition of repeating field 2023 * {@link #authorizingPrescription}, creating it if it does not already 2024 * exist 2025 */ 2026 public Reference getAuthorizingPrescriptionFirstRep() { 2027 if (getAuthorizingPrescription().isEmpty()) { 2028 addAuthorizingPrescription(); 2029 } 2030 return getAuthorizingPrescription().get(0); 2031 } 2032 2033 /** 2034 * @deprecated Use Reference#setResource(IBaseResource) instead 2035 */ 2036 @Deprecated 2037 public List<MedicationRequest> getAuthorizingPrescriptionTarget() { 2038 if (this.authorizingPrescriptionTarget == null) 2039 this.authorizingPrescriptionTarget = new ArrayList<MedicationRequest>(); 2040 return this.authorizingPrescriptionTarget; 2041 } 2042 2043 /** 2044 * @deprecated Use Reference#setResource(IBaseResource) instead 2045 */ 2046 @Deprecated 2047 public MedicationRequest addAuthorizingPrescriptionTarget() { 2048 MedicationRequest r = new MedicationRequest(); 2049 if (this.authorizingPrescriptionTarget == null) 2050 this.authorizingPrescriptionTarget = new ArrayList<MedicationRequest>(); 2051 this.authorizingPrescriptionTarget.add(r); 2052 return r; 2053 } 2054 2055 /** 2056 * @return {@link #type} (Indicates the type of dispensing event that is 2057 * performed. For example, Trial Fill, Completion of Trial, Partial 2058 * Fill, Emergency Fill, Samples, etc.) 2059 */ 2060 public CodeableConcept getType() { 2061 if (this.type == null) 2062 if (Configuration.errorOnAutoCreate()) 2063 throw new Error("Attempt to auto-create MedicationDispense.type"); 2064 else if (Configuration.doAutoCreate()) 2065 this.type = new CodeableConcept(); // cc 2066 return this.type; 2067 } 2068 2069 public boolean hasType() { 2070 return this.type != null && !this.type.isEmpty(); 2071 } 2072 2073 /** 2074 * @param value {@link #type} (Indicates the type of dispensing event that is 2075 * performed. For example, Trial Fill, Completion of Trial, Partial 2076 * Fill, Emergency Fill, Samples, etc.) 2077 */ 2078 public MedicationDispense setType(CodeableConcept value) { 2079 this.type = value; 2080 return this; 2081 } 2082 2083 /** 2084 * @return {@link #quantity} (The amount of medication that has been dispensed. 2085 * Includes unit of measure.) 2086 */ 2087 public Quantity getQuantity() { 2088 if (this.quantity == null) 2089 if (Configuration.errorOnAutoCreate()) 2090 throw new Error("Attempt to auto-create MedicationDispense.quantity"); 2091 else if (Configuration.doAutoCreate()) 2092 this.quantity = new Quantity(); // cc 2093 return this.quantity; 2094 } 2095 2096 public boolean hasQuantity() { 2097 return this.quantity != null && !this.quantity.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #quantity} (The amount of medication that has been 2102 * dispensed. Includes unit of measure.) 2103 */ 2104 public MedicationDispense setQuantity(Quantity value) { 2105 this.quantity = value; 2106 return this; 2107 } 2108 2109 /** 2110 * @return {@link #daysSupply} (The amount of medication expressed as a timing 2111 * amount.) 2112 */ 2113 public Quantity getDaysSupply() { 2114 if (this.daysSupply == null) 2115 if (Configuration.errorOnAutoCreate()) 2116 throw new Error("Attempt to auto-create MedicationDispense.daysSupply"); 2117 else if (Configuration.doAutoCreate()) 2118 this.daysSupply = new Quantity(); // cc 2119 return this.daysSupply; 2120 } 2121 2122 public boolean hasDaysSupply() { 2123 return this.daysSupply != null && !this.daysSupply.isEmpty(); 2124 } 2125 2126 /** 2127 * @param value {@link #daysSupply} (The amount of medication expressed as a 2128 * timing amount.) 2129 */ 2130 public MedicationDispense setDaysSupply(Quantity value) { 2131 this.daysSupply = value; 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #whenPrepared} (The time when the dispensed product was 2137 * packaged and reviewed.). This is the underlying object with id, value 2138 * and extensions. The accessor "getWhenPrepared" gives direct access to 2139 * the value 2140 */ 2141 public DateTimeType getWhenPreparedElement() { 2142 if (this.whenPrepared == null) 2143 if (Configuration.errorOnAutoCreate()) 2144 throw new Error("Attempt to auto-create MedicationDispense.whenPrepared"); 2145 else if (Configuration.doAutoCreate()) 2146 this.whenPrepared = new DateTimeType(); // bb 2147 return this.whenPrepared; 2148 } 2149 2150 public boolean hasWhenPreparedElement() { 2151 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 2152 } 2153 2154 public boolean hasWhenPrepared() { 2155 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 2156 } 2157 2158 /** 2159 * @param value {@link #whenPrepared} (The time when the dispensed product was 2160 * packaged and reviewed.). This is the underlying object with id, 2161 * value and extensions. The accessor "getWhenPrepared" gives 2162 * direct access to the value 2163 */ 2164 public MedicationDispense setWhenPreparedElement(DateTimeType value) { 2165 this.whenPrepared = value; 2166 return this; 2167 } 2168 2169 /** 2170 * @return The time when the dispensed product was packaged and reviewed. 2171 */ 2172 public Date getWhenPrepared() { 2173 return this.whenPrepared == null ? null : this.whenPrepared.getValue(); 2174 } 2175 2176 /** 2177 * @param value The time when the dispensed product was packaged and reviewed. 2178 */ 2179 public MedicationDispense setWhenPrepared(Date value) { 2180 if (value == null) 2181 this.whenPrepared = null; 2182 else { 2183 if (this.whenPrepared == null) 2184 this.whenPrepared = new DateTimeType(); 2185 this.whenPrepared.setValue(value); 2186 } 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #whenHandedOver} (The time the dispensed product was provided 2192 * to the patient or their representative.). This is the underlying 2193 * object with id, value and extensions. The accessor 2194 * "getWhenHandedOver" gives direct access to the value 2195 */ 2196 public DateTimeType getWhenHandedOverElement() { 2197 if (this.whenHandedOver == null) 2198 if (Configuration.errorOnAutoCreate()) 2199 throw new Error("Attempt to auto-create MedicationDispense.whenHandedOver"); 2200 else if (Configuration.doAutoCreate()) 2201 this.whenHandedOver = new DateTimeType(); // bb 2202 return this.whenHandedOver; 2203 } 2204 2205 public boolean hasWhenHandedOverElement() { 2206 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 2207 } 2208 2209 public boolean hasWhenHandedOver() { 2210 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 2211 } 2212 2213 /** 2214 * @param value {@link #whenHandedOver} (The time the dispensed product was 2215 * provided to the patient or their representative.). This is the 2216 * underlying object with id, value and extensions. The accessor 2217 * "getWhenHandedOver" gives direct access to the value 2218 */ 2219 public MedicationDispense setWhenHandedOverElement(DateTimeType value) { 2220 this.whenHandedOver = value; 2221 return this; 2222 } 2223 2224 /** 2225 * @return The time the dispensed product was provided to the patient or their 2226 * representative. 2227 */ 2228 public Date getWhenHandedOver() { 2229 return this.whenHandedOver == null ? null : this.whenHandedOver.getValue(); 2230 } 2231 2232 /** 2233 * @param value The time the dispensed product was provided to the patient or 2234 * their representative. 2235 */ 2236 public MedicationDispense setWhenHandedOver(Date value) { 2237 if (value == null) 2238 this.whenHandedOver = null; 2239 else { 2240 if (this.whenHandedOver == null) 2241 this.whenHandedOver = new DateTimeType(); 2242 this.whenHandedOver.setValue(value); 2243 } 2244 return this; 2245 } 2246 2247 /** 2248 * @return {@link #destination} (Identification of the facility/location where 2249 * the medication was shipped to, as part of the dispense event.) 2250 */ 2251 public Reference getDestination() { 2252 if (this.destination == null) 2253 if (Configuration.errorOnAutoCreate()) 2254 throw new Error("Attempt to auto-create MedicationDispense.destination"); 2255 else if (Configuration.doAutoCreate()) 2256 this.destination = new Reference(); // cc 2257 return this.destination; 2258 } 2259 2260 public boolean hasDestination() { 2261 return this.destination != null && !this.destination.isEmpty(); 2262 } 2263 2264 /** 2265 * @param value {@link #destination} (Identification of the facility/location 2266 * where the medication was shipped to, as part of the dispense 2267 * event.) 2268 */ 2269 public MedicationDispense setDestination(Reference value) { 2270 this.destination = value; 2271 return this; 2272 } 2273 2274 /** 2275 * @return {@link #destination} The actual object that is the target of the 2276 * reference. The reference library doesn't populate this, but you can 2277 * use it to hold the resource if you resolve it. (Identification of the 2278 * facility/location where the medication was shipped to, as part of the 2279 * dispense event.) 2280 */ 2281 public Location getDestinationTarget() { 2282 if (this.destinationTarget == null) 2283 if (Configuration.errorOnAutoCreate()) 2284 throw new Error("Attempt to auto-create MedicationDispense.destination"); 2285 else if (Configuration.doAutoCreate()) 2286 this.destinationTarget = new Location(); // aa 2287 return this.destinationTarget; 2288 } 2289 2290 /** 2291 * @param value {@link #destination} The actual object that is the target of the 2292 * reference. The reference library doesn't use these, but you can 2293 * use it to hold the resource if you resolve it. (Identification 2294 * of the facility/location where the medication was shipped to, as 2295 * part of the dispense event.) 2296 */ 2297 public MedicationDispense setDestinationTarget(Location value) { 2298 this.destinationTarget = value; 2299 return this; 2300 } 2301 2302 /** 2303 * @return {@link #receiver} (Identifies the person who picked up the 2304 * medication. This will usually be a patient or their caregiver, but 2305 * some cases exist where it can be a healthcare professional.) 2306 */ 2307 public List<Reference> getReceiver() { 2308 if (this.receiver == null) 2309 this.receiver = new ArrayList<Reference>(); 2310 return this.receiver; 2311 } 2312 2313 /** 2314 * @return Returns a reference to <code>this</code> for easy method chaining 2315 */ 2316 public MedicationDispense setReceiver(List<Reference> theReceiver) { 2317 this.receiver = theReceiver; 2318 return this; 2319 } 2320 2321 public boolean hasReceiver() { 2322 if (this.receiver == null) 2323 return false; 2324 for (Reference item : this.receiver) 2325 if (!item.isEmpty()) 2326 return true; 2327 return false; 2328 } 2329 2330 public Reference addReceiver() { // 3 2331 Reference t = new Reference(); 2332 if (this.receiver == null) 2333 this.receiver = new ArrayList<Reference>(); 2334 this.receiver.add(t); 2335 return t; 2336 } 2337 2338 public MedicationDispense addReceiver(Reference t) { // 3 2339 if (t == null) 2340 return this; 2341 if (this.receiver == null) 2342 this.receiver = new ArrayList<Reference>(); 2343 this.receiver.add(t); 2344 return this; 2345 } 2346 2347 /** 2348 * @return The first repetition of repeating field {@link #receiver}, creating 2349 * it if it does not already exist 2350 */ 2351 public Reference getReceiverFirstRep() { 2352 if (getReceiver().isEmpty()) { 2353 addReceiver(); 2354 } 2355 return getReceiver().get(0); 2356 } 2357 2358 /** 2359 * @deprecated Use Reference#setResource(IBaseResource) instead 2360 */ 2361 @Deprecated 2362 public List<Resource> getReceiverTarget() { 2363 if (this.receiverTarget == null) 2364 this.receiverTarget = new ArrayList<Resource>(); 2365 return this.receiverTarget; 2366 } 2367 2368 /** 2369 * @return {@link #note} (Extra information about the dispense that could not be 2370 * conveyed in the other attributes.) 2371 */ 2372 public List<Annotation> getNote() { 2373 if (this.note == null) 2374 this.note = new ArrayList<Annotation>(); 2375 return this.note; 2376 } 2377 2378 /** 2379 * @return Returns a reference to <code>this</code> for easy method chaining 2380 */ 2381 public MedicationDispense setNote(List<Annotation> theNote) { 2382 this.note = theNote; 2383 return this; 2384 } 2385 2386 public boolean hasNote() { 2387 if (this.note == null) 2388 return false; 2389 for (Annotation item : this.note) 2390 if (!item.isEmpty()) 2391 return true; 2392 return false; 2393 } 2394 2395 public Annotation addNote() { // 3 2396 Annotation t = new Annotation(); 2397 if (this.note == null) 2398 this.note = new ArrayList<Annotation>(); 2399 this.note.add(t); 2400 return t; 2401 } 2402 2403 public MedicationDispense addNote(Annotation t) { // 3 2404 if (t == null) 2405 return this; 2406 if (this.note == null) 2407 this.note = new ArrayList<Annotation>(); 2408 this.note.add(t); 2409 return this; 2410 } 2411 2412 /** 2413 * @return The first repetition of repeating field {@link #note}, creating it if 2414 * it does not already exist 2415 */ 2416 public Annotation getNoteFirstRep() { 2417 if (getNote().isEmpty()) { 2418 addNote(); 2419 } 2420 return getNote().get(0); 2421 } 2422 2423 /** 2424 * @return {@link #dosageInstruction} (Indicates how the medication is to be 2425 * used by the patient.) 2426 */ 2427 public List<Dosage> getDosageInstruction() { 2428 if (this.dosageInstruction == null) 2429 this.dosageInstruction = new ArrayList<Dosage>(); 2430 return this.dosageInstruction; 2431 } 2432 2433 /** 2434 * @return Returns a reference to <code>this</code> for easy method chaining 2435 */ 2436 public MedicationDispense setDosageInstruction(List<Dosage> theDosageInstruction) { 2437 this.dosageInstruction = theDosageInstruction; 2438 return this; 2439 } 2440 2441 public boolean hasDosageInstruction() { 2442 if (this.dosageInstruction == null) 2443 return false; 2444 for (Dosage item : this.dosageInstruction) 2445 if (!item.isEmpty()) 2446 return true; 2447 return false; 2448 } 2449 2450 public Dosage addDosageInstruction() { // 3 2451 Dosage t = new Dosage(); 2452 if (this.dosageInstruction == null) 2453 this.dosageInstruction = new ArrayList<Dosage>(); 2454 this.dosageInstruction.add(t); 2455 return t; 2456 } 2457 2458 public MedicationDispense addDosageInstruction(Dosage t) { // 3 2459 if (t == null) 2460 return this; 2461 if (this.dosageInstruction == null) 2462 this.dosageInstruction = new ArrayList<Dosage>(); 2463 this.dosageInstruction.add(t); 2464 return this; 2465 } 2466 2467 /** 2468 * @return The first repetition of repeating field {@link #dosageInstruction}, 2469 * creating it if it does not already exist 2470 */ 2471 public Dosage getDosageInstructionFirstRep() { 2472 if (getDosageInstruction().isEmpty()) { 2473 addDosageInstruction(); 2474 } 2475 return getDosageInstruction().get(0); 2476 } 2477 2478 /** 2479 * @return {@link #substitution} (Indicates whether or not substitution was made 2480 * as part of the dispense. In some cases, substitution will be expected 2481 * but does not happen, in other cases substitution is not expected but 2482 * does happen. This block explains what substitution did or did not 2483 * happen and why. If nothing is specified, substitution was not done.) 2484 */ 2485 public MedicationDispenseSubstitutionComponent getSubstitution() { 2486 if (this.substitution == null) 2487 if (Configuration.errorOnAutoCreate()) 2488 throw new Error("Attempt to auto-create MedicationDispense.substitution"); 2489 else if (Configuration.doAutoCreate()) 2490 this.substitution = new MedicationDispenseSubstitutionComponent(); // cc 2491 return this.substitution; 2492 } 2493 2494 public boolean hasSubstitution() { 2495 return this.substitution != null && !this.substitution.isEmpty(); 2496 } 2497 2498 /** 2499 * @param value {@link #substitution} (Indicates whether or not substitution was 2500 * made as part of the dispense. In some cases, substitution will 2501 * be expected but does not happen, in other cases substitution is 2502 * not expected but does happen. This block explains what 2503 * substitution did or did not happen and why. If nothing is 2504 * specified, substitution was not done.) 2505 */ 2506 public MedicationDispense setSubstitution(MedicationDispenseSubstitutionComponent value) { 2507 this.substitution = value; 2508 return this; 2509 } 2510 2511 /** 2512 * @return {@link #detectedIssue} (Indicates an actual or potential clinical 2513 * issue with or between one or more active or proposed clinical actions 2514 * for a patient; e.g. drug-drug interaction, duplicate therapy, dosage 2515 * alert etc.) 2516 */ 2517 public List<Reference> getDetectedIssue() { 2518 if (this.detectedIssue == null) 2519 this.detectedIssue = new ArrayList<Reference>(); 2520 return this.detectedIssue; 2521 } 2522 2523 /** 2524 * @return Returns a reference to <code>this</code> for easy method chaining 2525 */ 2526 public MedicationDispense setDetectedIssue(List<Reference> theDetectedIssue) { 2527 this.detectedIssue = theDetectedIssue; 2528 return this; 2529 } 2530 2531 public boolean hasDetectedIssue() { 2532 if (this.detectedIssue == null) 2533 return false; 2534 for (Reference item : this.detectedIssue) 2535 if (!item.isEmpty()) 2536 return true; 2537 return false; 2538 } 2539 2540 public Reference addDetectedIssue() { // 3 2541 Reference t = new Reference(); 2542 if (this.detectedIssue == null) 2543 this.detectedIssue = new ArrayList<Reference>(); 2544 this.detectedIssue.add(t); 2545 return t; 2546 } 2547 2548 public MedicationDispense addDetectedIssue(Reference t) { // 3 2549 if (t == null) 2550 return this; 2551 if (this.detectedIssue == null) 2552 this.detectedIssue = new ArrayList<Reference>(); 2553 this.detectedIssue.add(t); 2554 return this; 2555 } 2556 2557 /** 2558 * @return The first repetition of repeating field {@link #detectedIssue}, 2559 * creating it if it does not already exist 2560 */ 2561 public Reference getDetectedIssueFirstRep() { 2562 if (getDetectedIssue().isEmpty()) { 2563 addDetectedIssue(); 2564 } 2565 return getDetectedIssue().get(0); 2566 } 2567 2568 /** 2569 * @deprecated Use Reference#setResource(IBaseResource) instead 2570 */ 2571 @Deprecated 2572 public List<DetectedIssue> getDetectedIssueTarget() { 2573 if (this.detectedIssueTarget == null) 2574 this.detectedIssueTarget = new ArrayList<DetectedIssue>(); 2575 return this.detectedIssueTarget; 2576 } 2577 2578 /** 2579 * @deprecated Use Reference#setResource(IBaseResource) instead 2580 */ 2581 @Deprecated 2582 public DetectedIssue addDetectedIssueTarget() { 2583 DetectedIssue r = new DetectedIssue(); 2584 if (this.detectedIssueTarget == null) 2585 this.detectedIssueTarget = new ArrayList<DetectedIssue>(); 2586 this.detectedIssueTarget.add(r); 2587 return r; 2588 } 2589 2590 /** 2591 * @return {@link #eventHistory} (A summary of the events of interest that have 2592 * occurred, such as when the dispense was verified.) 2593 */ 2594 public List<Reference> getEventHistory() { 2595 if (this.eventHistory == null) 2596 this.eventHistory = new ArrayList<Reference>(); 2597 return this.eventHistory; 2598 } 2599 2600 /** 2601 * @return Returns a reference to <code>this</code> for easy method chaining 2602 */ 2603 public MedicationDispense setEventHistory(List<Reference> theEventHistory) { 2604 this.eventHistory = theEventHistory; 2605 return this; 2606 } 2607 2608 public boolean hasEventHistory() { 2609 if (this.eventHistory == null) 2610 return false; 2611 for (Reference item : this.eventHistory) 2612 if (!item.isEmpty()) 2613 return true; 2614 return false; 2615 } 2616 2617 public Reference addEventHistory() { // 3 2618 Reference t = new Reference(); 2619 if (this.eventHistory == null) 2620 this.eventHistory = new ArrayList<Reference>(); 2621 this.eventHistory.add(t); 2622 return t; 2623 } 2624 2625 public MedicationDispense addEventHistory(Reference t) { // 3 2626 if (t == null) 2627 return this; 2628 if (this.eventHistory == null) 2629 this.eventHistory = new ArrayList<Reference>(); 2630 this.eventHistory.add(t); 2631 return this; 2632 } 2633 2634 /** 2635 * @return The first repetition of repeating field {@link #eventHistory}, 2636 * creating it if it does not already exist 2637 */ 2638 public Reference getEventHistoryFirstRep() { 2639 if (getEventHistory().isEmpty()) { 2640 addEventHistory(); 2641 } 2642 return getEventHistory().get(0); 2643 } 2644 2645 /** 2646 * @deprecated Use Reference#setResource(IBaseResource) instead 2647 */ 2648 @Deprecated 2649 public List<Provenance> getEventHistoryTarget() { 2650 if (this.eventHistoryTarget == null) 2651 this.eventHistoryTarget = new ArrayList<Provenance>(); 2652 return this.eventHistoryTarget; 2653 } 2654 2655 /** 2656 * @deprecated Use Reference#setResource(IBaseResource) instead 2657 */ 2658 @Deprecated 2659 public Provenance addEventHistoryTarget() { 2660 Provenance r = new Provenance(); 2661 if (this.eventHistoryTarget == null) 2662 this.eventHistoryTarget = new ArrayList<Provenance>(); 2663 this.eventHistoryTarget.add(r); 2664 return r; 2665 } 2666 2667 protected void listChildren(List<Property> children) { 2668 super.listChildren(children); 2669 children.add(new Property("identifier", "Identifier", 2670 "Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 2671 0, java.lang.Integer.MAX_VALUE, identifier)); 2672 children.add(new Property("partOf", "Reference(Procedure)", "The procedure that trigger the dispense.", 0, 2673 java.lang.Integer.MAX_VALUE, partOf)); 2674 children.add( 2675 new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status)); 2676 children.add(new Property("statusReason[x]", "CodeableConcept|Reference(DetectedIssue)", 2677 "Indicates the reason why a dispense was not performed.", 0, 1, statusReason)); 2678 children.add(new Property("category", "CodeableConcept", 2679 "Indicates the type of medication dispense (for example, where the medication is expected to be consumed or administered (i.e. inpatient or outpatient)).", 2680 0, 1, category)); 2681 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2682 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2683 0, 1, medication)); 2684 children.add(new Property("subject", "Reference(Patient|Group)", 2685 "A link to a resource representing the person or the group to whom the medication will be given.", 0, 1, 2686 subject)); 2687 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 2688 "The encounter or episode of care that establishes the context for this event.", 0, 1, context)); 2689 children.add(new Property("supportingInformation", "Reference(Any)", 2690 "Additional information that supports the medication being dispensed.", 0, java.lang.Integer.MAX_VALUE, 2691 supportingInformation)); 2692 children.add(new Property("performer", "", "Indicates who or what performed the event.", 0, 2693 java.lang.Integer.MAX_VALUE, performer)); 2694 children.add(new Property("location", "Reference(Location)", 2695 "The principal physical location where the dispense was performed.", 0, 1, location)); 2696 children.add(new Property("authorizingPrescription", "Reference(MedicationRequest)", 2697 "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, 2698 authorizingPrescription)); 2699 children.add(new Property("type", "CodeableConcept", 2700 "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 2701 0, 1, type)); 2702 children.add(new Property("quantity", "SimpleQuantity", 2703 "The amount of medication that has been dispensed. Includes unit of measure.", 0, 1, quantity)); 2704 children.add(new Property("daysSupply", "SimpleQuantity", "The amount of medication expressed as a timing amount.", 2705 0, 1, daysSupply)); 2706 children.add(new Property("whenPrepared", "dateTime", 2707 "The time when the dispensed product was packaged and reviewed.", 0, 1, whenPrepared)); 2708 children.add(new Property("whenHandedOver", "dateTime", 2709 "The time the dispensed product was provided to the patient or their representative.", 0, 1, whenHandedOver)); 2710 children.add(new Property("destination", "Reference(Location)", 2711 "Identification of the facility/location where the medication was shipped to, as part of the dispense event.", 2712 0, 1, destination)); 2713 children.add(new Property("receiver", "Reference(Patient|Practitioner)", 2714 "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.", 2715 0, java.lang.Integer.MAX_VALUE, receiver)); 2716 children.add(new Property("note", "Annotation", 2717 "Extra information about the dispense that could not be conveyed in the other attributes.", 0, 2718 java.lang.Integer.MAX_VALUE, note)); 2719 children 2720 .add(new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 2721 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 2722 children.add(new Property("substitution", "", 2723 "Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.", 2724 0, 1, substitution)); 2725 children.add(new Property("detectedIssue", "Reference(DetectedIssue)", 2726 "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. drug-drug interaction, duplicate therapy, dosage alert etc.", 2727 0, java.lang.Integer.MAX_VALUE, detectedIssue)); 2728 children.add(new Property("eventHistory", "Reference(Provenance)", 2729 "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, 2730 java.lang.Integer.MAX_VALUE, eventHistory)); 2731 } 2732 2733 @Override 2734 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2735 switch (_hash) { 2736 case -1618432855: 2737 /* identifier */ return new Property("identifier", "Identifier", 2738 "Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 2739 0, java.lang.Integer.MAX_VALUE, identifier); 2740 case -995410646: 2741 /* partOf */ return new Property("partOf", "Reference(Procedure)", "The procedure that trigger the dispense.", 0, 2742 java.lang.Integer.MAX_VALUE, partOf); 2743 case -892481550: 2744 /* status */ return new Property("status", "code", "A code specifying the state of the set of dispense events.", 2745 0, 1, status); 2746 case -1421632534: 2747 /* statusReason[x] */ return new Property("statusReason[x]", "CodeableConcept|Reference(DetectedIssue)", 2748 "Indicates the reason why a dispense was not performed.", 0, 1, statusReason); 2749 case 2051346646: 2750 /* statusReason */ return new Property("statusReason[x]", "CodeableConcept|Reference(DetectedIssue)", 2751 "Indicates the reason why a dispense was not performed.", 0, 1, statusReason); 2752 case 2082934763: 2753 /* statusReasonCodeableConcept */ return new Property("statusReason[x]", 2754 "CodeableConcept|Reference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, 2755 statusReason); 2756 case 1344200469: 2757 /* statusReasonReference */ return new Property("statusReason[x]", "CodeableConcept|Reference(DetectedIssue)", 2758 "Indicates the reason why a dispense was not performed.", 0, 1, statusReason); 2759 case 50511102: 2760 /* category */ return new Property("category", "CodeableConcept", 2761 "Indicates the type of medication dispense (for example, where the medication is expected to be consumed or administered (i.e. inpatient or outpatient)).", 2762 0, 1, category); 2763 case 1458402129: 2764 /* medication[x] */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2765 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2766 0, 1, medication); 2767 case 1998965455: 2768 /* medication */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2769 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2770 0, 1, medication); 2771 case -209845038: 2772 /* medicationCodeableConcept */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2773 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2774 0, 1, medication); 2775 case 2104315196: 2776 /* medicationReference */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2777 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2778 0, 1, medication); 2779 case -1867885268: 2780 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2781 "A link to a resource representing the person or the group to whom the medication will be given.", 0, 1, 2782 subject); 2783 case 951530927: 2784 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 2785 "The encounter or episode of care that establishes the context for this event.", 0, 1, context); 2786 case -1248768647: 2787 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2788 "Additional information that supports the medication being dispensed.", 0, java.lang.Integer.MAX_VALUE, 2789 supportingInformation); 2790 case 481140686: 2791 /* performer */ return new Property("performer", "", "Indicates who or what performed the event.", 0, 2792 java.lang.Integer.MAX_VALUE, performer); 2793 case 1901043637: 2794 /* location */ return new Property("location", "Reference(Location)", 2795 "The principal physical location where the dispense was performed.", 0, 1, location); 2796 case -1237557856: 2797 /* authorizingPrescription */ return new Property("authorizingPrescription", "Reference(MedicationRequest)", 2798 "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, 2799 authorizingPrescription); 2800 case 3575610: 2801 /* type */ return new Property("type", "CodeableConcept", 2802 "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 2803 0, 1, type); 2804 case -1285004149: 2805 /* quantity */ return new Property("quantity", "SimpleQuantity", 2806 "The amount of medication that has been dispensed. Includes unit of measure.", 0, 1, quantity); 2807 case 197175334: 2808 /* daysSupply */ return new Property("daysSupply", "SimpleQuantity", 2809 "The amount of medication expressed as a timing amount.", 0, 1, daysSupply); 2810 case -562837097: 2811 /* whenPrepared */ return new Property("whenPrepared", "dateTime", 2812 "The time when the dispensed product was packaged and reviewed.", 0, 1, whenPrepared); 2813 case -940241380: 2814 /* whenHandedOver */ return new Property("whenHandedOver", "dateTime", 2815 "The time the dispensed product was provided to the patient or their representative.", 0, 1, whenHandedOver); 2816 case -1429847026: 2817 /* destination */ return new Property("destination", "Reference(Location)", 2818 "Identification of the facility/location where the medication was shipped to, as part of the dispense event.", 2819 0, 1, destination); 2820 case -808719889: 2821 /* receiver */ return new Property("receiver", "Reference(Patient|Practitioner)", 2822 "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.", 2823 0, java.lang.Integer.MAX_VALUE, receiver); 2824 case 3387378: 2825 /* note */ return new Property("note", "Annotation", 2826 "Extra information about the dispense that could not be conveyed in the other attributes.", 0, 2827 java.lang.Integer.MAX_VALUE, note); 2828 case -1201373865: 2829 /* dosageInstruction */ return new Property("dosageInstruction", "Dosage", 2830 "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, 2831 dosageInstruction); 2832 case 826147581: 2833 /* substitution */ return new Property("substitution", "", 2834 "Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.", 2835 0, 1, substitution); 2836 case 51602295: 2837 /* detectedIssue */ return new Property("detectedIssue", "Reference(DetectedIssue)", 2838 "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. drug-drug interaction, duplicate therapy, dosage alert etc.", 2839 0, java.lang.Integer.MAX_VALUE, detectedIssue); 2840 case 1835190426: 2841 /* eventHistory */ return new Property("eventHistory", "Reference(Provenance)", 2842 "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, 2843 java.lang.Integer.MAX_VALUE, eventHistory); 2844 default: 2845 return super.getNamedProperty(_hash, _name, _checkValid); 2846 } 2847 2848 } 2849 2850 @Override 2851 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2852 switch (hash) { 2853 case -1618432855: 2854 /* identifier */ return this.identifier == null ? new Base[0] 2855 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2856 case -995410646: 2857 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2858 case -892481550: 2859 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationDispenseStatus> 2860 case 2051346646: 2861 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // Type 2862 case 50511102: 2863 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 2864 case 1998965455: 2865 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 2866 case -1867885268: 2867 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2868 case 951530927: 2869 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 2870 case -1248768647: 2871 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2872 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2873 case 481140686: 2874 /* performer */ return this.performer == null ? new Base[0] 2875 : this.performer.toArray(new Base[this.performer.size()]); // MedicationDispensePerformerComponent 2876 case 1901043637: 2877 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 2878 case -1237557856: 2879 /* authorizingPrescription */ return this.authorizingPrescription == null ? new Base[0] 2880 : this.authorizingPrescription.toArray(new Base[this.authorizingPrescription.size()]); // Reference 2881 case 3575610: 2882 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2883 case -1285004149: 2884 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 2885 case 197175334: 2886 /* daysSupply */ return this.daysSupply == null ? new Base[0] : new Base[] { this.daysSupply }; // Quantity 2887 case -562837097: 2888 /* whenPrepared */ return this.whenPrepared == null ? new Base[0] : new Base[] { this.whenPrepared }; // DateTimeType 2889 case -940241380: 2890 /* whenHandedOver */ return this.whenHandedOver == null ? new Base[0] : new Base[] { this.whenHandedOver }; // DateTimeType 2891 case -1429847026: 2892 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // Reference 2893 case -808719889: 2894 /* receiver */ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 2895 case 3387378: 2896 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2897 case -1201373865: 2898 /* dosageInstruction */ return this.dosageInstruction == null ? new Base[0] 2899 : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 2900 case 826147581: 2901 /* substitution */ return this.substitution == null ? new Base[0] : new Base[] { this.substitution }; // MedicationDispenseSubstitutionComponent 2902 case 51602295: 2903 /* detectedIssue */ return this.detectedIssue == null ? new Base[0] 2904 : this.detectedIssue.toArray(new Base[this.detectedIssue.size()]); // Reference 2905 case 1835190426: 2906 /* eventHistory */ return this.eventHistory == null ? new Base[0] 2907 : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2908 default: 2909 return super.getProperty(hash, name, checkValid); 2910 } 2911 2912 } 2913 2914 @Override 2915 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2916 switch (hash) { 2917 case -1618432855: // identifier 2918 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2919 return value; 2920 case -995410646: // partOf 2921 this.getPartOf().add(castToReference(value)); // Reference 2922 return value; 2923 case -892481550: // status 2924 value = new MedicationDispenseStatusEnumFactory().fromType(castToCode(value)); 2925 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatus> 2926 return value; 2927 case 2051346646: // statusReason 2928 this.statusReason = castToType(value); // Type 2929 return value; 2930 case 50511102: // category 2931 this.category = castToCodeableConcept(value); // CodeableConcept 2932 return value; 2933 case 1998965455: // medication 2934 this.medication = castToType(value); // Type 2935 return value; 2936 case -1867885268: // subject 2937 this.subject = castToReference(value); // Reference 2938 return value; 2939 case 951530927: // context 2940 this.context = castToReference(value); // Reference 2941 return value; 2942 case -1248768647: // supportingInformation 2943 this.getSupportingInformation().add(castToReference(value)); // Reference 2944 return value; 2945 case 481140686: // performer 2946 this.getPerformer().add((MedicationDispensePerformerComponent) value); // MedicationDispensePerformerComponent 2947 return value; 2948 case 1901043637: // location 2949 this.location = castToReference(value); // Reference 2950 return value; 2951 case -1237557856: // authorizingPrescription 2952 this.getAuthorizingPrescription().add(castToReference(value)); // Reference 2953 return value; 2954 case 3575610: // type 2955 this.type = castToCodeableConcept(value); // CodeableConcept 2956 return value; 2957 case -1285004149: // quantity 2958 this.quantity = castToQuantity(value); // Quantity 2959 return value; 2960 case 197175334: // daysSupply 2961 this.daysSupply = castToQuantity(value); // Quantity 2962 return value; 2963 case -562837097: // whenPrepared 2964 this.whenPrepared = castToDateTime(value); // DateTimeType 2965 return value; 2966 case -940241380: // whenHandedOver 2967 this.whenHandedOver = castToDateTime(value); // DateTimeType 2968 return value; 2969 case -1429847026: // destination 2970 this.destination = castToReference(value); // Reference 2971 return value; 2972 case -808719889: // receiver 2973 this.getReceiver().add(castToReference(value)); // Reference 2974 return value; 2975 case 3387378: // note 2976 this.getNote().add(castToAnnotation(value)); // Annotation 2977 return value; 2978 case -1201373865: // dosageInstruction 2979 this.getDosageInstruction().add(castToDosage(value)); // Dosage 2980 return value; 2981 case 826147581: // substitution 2982 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2983 return value; 2984 case 51602295: // detectedIssue 2985 this.getDetectedIssue().add(castToReference(value)); // Reference 2986 return value; 2987 case 1835190426: // eventHistory 2988 this.getEventHistory().add(castToReference(value)); // Reference 2989 return value; 2990 default: 2991 return super.setProperty(hash, name, value); 2992 } 2993 2994 } 2995 2996 @Override 2997 public Base setProperty(String name, Base value) throws FHIRException { 2998 if (name.equals("identifier")) { 2999 this.getIdentifier().add(castToIdentifier(value)); 3000 } else if (name.equals("partOf")) { 3001 this.getPartOf().add(castToReference(value)); 3002 } else if (name.equals("status")) { 3003 value = new MedicationDispenseStatusEnumFactory().fromType(castToCode(value)); 3004 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatus> 3005 } else if (name.equals("statusReason[x]")) { 3006 this.statusReason = castToType(value); // Type 3007 } else if (name.equals("category")) { 3008 this.category = castToCodeableConcept(value); // CodeableConcept 3009 } else if (name.equals("medication[x]")) { 3010 this.medication = castToType(value); // Type 3011 } else if (name.equals("subject")) { 3012 this.subject = castToReference(value); // Reference 3013 } else if (name.equals("context")) { 3014 this.context = castToReference(value); // Reference 3015 } else if (name.equals("supportingInformation")) { 3016 this.getSupportingInformation().add(castToReference(value)); 3017 } else if (name.equals("performer")) { 3018 this.getPerformer().add((MedicationDispensePerformerComponent) value); 3019 } else if (name.equals("location")) { 3020 this.location = castToReference(value); // Reference 3021 } else if (name.equals("authorizingPrescription")) { 3022 this.getAuthorizingPrescription().add(castToReference(value)); 3023 } else if (name.equals("type")) { 3024 this.type = castToCodeableConcept(value); // CodeableConcept 3025 } else if (name.equals("quantity")) { 3026 this.quantity = castToQuantity(value); // Quantity 3027 } else if (name.equals("daysSupply")) { 3028 this.daysSupply = castToQuantity(value); // Quantity 3029 } else if (name.equals("whenPrepared")) { 3030 this.whenPrepared = castToDateTime(value); // DateTimeType 3031 } else if (name.equals("whenHandedOver")) { 3032 this.whenHandedOver = castToDateTime(value); // DateTimeType 3033 } else if (name.equals("destination")) { 3034 this.destination = castToReference(value); // Reference 3035 } else if (name.equals("receiver")) { 3036 this.getReceiver().add(castToReference(value)); 3037 } else if (name.equals("note")) { 3038 this.getNote().add(castToAnnotation(value)); 3039 } else if (name.equals("dosageInstruction")) { 3040 this.getDosageInstruction().add(castToDosage(value)); 3041 } else if (name.equals("substitution")) { 3042 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 3043 } else if (name.equals("detectedIssue")) { 3044 this.getDetectedIssue().add(castToReference(value)); 3045 } else if (name.equals("eventHistory")) { 3046 this.getEventHistory().add(castToReference(value)); 3047 } else 3048 return super.setProperty(name, value); 3049 return value; 3050 } 3051 3052 @Override 3053 public void removeChild(String name, Base value) throws FHIRException { 3054 if (name.equals("identifier")) { 3055 this.getIdentifier().remove(castToIdentifier(value)); 3056 } else if (name.equals("partOf")) { 3057 this.getPartOf().remove(castToReference(value)); 3058 } else if (name.equals("status")) { 3059 this.status = null; 3060 } else if (name.equals("statusReason[x]")) { 3061 this.statusReason = null; 3062 } else if (name.equals("category")) { 3063 this.category = null; 3064 } else if (name.equals("medication[x]")) { 3065 this.medication = null; 3066 } else if (name.equals("subject")) { 3067 this.subject = null; 3068 } else if (name.equals("context")) { 3069 this.context = null; 3070 } else if (name.equals("supportingInformation")) { 3071 this.getSupportingInformation().remove(castToReference(value)); 3072 } else if (name.equals("performer")) { 3073 this.getPerformer().remove((MedicationDispensePerformerComponent) value); 3074 } else if (name.equals("location")) { 3075 this.location = null; 3076 } else if (name.equals("authorizingPrescription")) { 3077 this.getAuthorizingPrescription().remove(castToReference(value)); 3078 } else if (name.equals("type")) { 3079 this.type = null; 3080 } else if (name.equals("quantity")) { 3081 this.quantity = null; 3082 } else if (name.equals("daysSupply")) { 3083 this.daysSupply = null; 3084 } else if (name.equals("whenPrepared")) { 3085 this.whenPrepared = null; 3086 } else if (name.equals("whenHandedOver")) { 3087 this.whenHandedOver = null; 3088 } else if (name.equals("destination")) { 3089 this.destination = null; 3090 } else if (name.equals("receiver")) { 3091 this.getReceiver().remove(castToReference(value)); 3092 } else if (name.equals("note")) { 3093 this.getNote().remove(castToAnnotation(value)); 3094 } else if (name.equals("dosageInstruction")) { 3095 this.getDosageInstruction().remove(castToDosage(value)); 3096 } else if (name.equals("substitution")) { 3097 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 3098 } else if (name.equals("detectedIssue")) { 3099 this.getDetectedIssue().remove(castToReference(value)); 3100 } else if (name.equals("eventHistory")) { 3101 this.getEventHistory().remove(castToReference(value)); 3102 } else 3103 super.removeChild(name, value); 3104 3105 } 3106 3107 @Override 3108 public Base makeProperty(int hash, String name) throws FHIRException { 3109 switch (hash) { 3110 case -1618432855: 3111 return addIdentifier(); 3112 case -995410646: 3113 return addPartOf(); 3114 case -892481550: 3115 return getStatusElement(); 3116 case -1421632534: 3117 return getStatusReason(); 3118 case 2051346646: 3119 return getStatusReason(); 3120 case 50511102: 3121 return getCategory(); 3122 case 1458402129: 3123 return getMedication(); 3124 case 1998965455: 3125 return getMedication(); 3126 case -1867885268: 3127 return getSubject(); 3128 case 951530927: 3129 return getContext(); 3130 case -1248768647: 3131 return addSupportingInformation(); 3132 case 481140686: 3133 return addPerformer(); 3134 case 1901043637: 3135 return getLocation(); 3136 case -1237557856: 3137 return addAuthorizingPrescription(); 3138 case 3575610: 3139 return getType(); 3140 case -1285004149: 3141 return getQuantity(); 3142 case 197175334: 3143 return getDaysSupply(); 3144 case -562837097: 3145 return getWhenPreparedElement(); 3146 case -940241380: 3147 return getWhenHandedOverElement(); 3148 case -1429847026: 3149 return getDestination(); 3150 case -808719889: 3151 return addReceiver(); 3152 case 3387378: 3153 return addNote(); 3154 case -1201373865: 3155 return addDosageInstruction(); 3156 case 826147581: 3157 return getSubstitution(); 3158 case 51602295: 3159 return addDetectedIssue(); 3160 case 1835190426: 3161 return addEventHistory(); 3162 default: 3163 return super.makeProperty(hash, name); 3164 } 3165 3166 } 3167 3168 @Override 3169 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3170 switch (hash) { 3171 case -1618432855: 3172 /* identifier */ return new String[] { "Identifier" }; 3173 case -995410646: 3174 /* partOf */ return new String[] { "Reference" }; 3175 case -892481550: 3176 /* status */ return new String[] { "code" }; 3177 case 2051346646: 3178 /* statusReason */ return new String[] { "CodeableConcept", "Reference" }; 3179 case 50511102: 3180 /* category */ return new String[] { "CodeableConcept" }; 3181 case 1998965455: 3182 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 3183 case -1867885268: 3184 /* subject */ return new String[] { "Reference" }; 3185 case 951530927: 3186 /* context */ return new String[] { "Reference" }; 3187 case -1248768647: 3188 /* supportingInformation */ return new String[] { "Reference" }; 3189 case 481140686: 3190 /* performer */ return new String[] {}; 3191 case 1901043637: 3192 /* location */ return new String[] { "Reference" }; 3193 case -1237557856: 3194 /* authorizingPrescription */ return new String[] { "Reference" }; 3195 case 3575610: 3196 /* type */ return new String[] { "CodeableConcept" }; 3197 case -1285004149: 3198 /* quantity */ return new String[] { "SimpleQuantity" }; 3199 case 197175334: 3200 /* daysSupply */ return new String[] { "SimpleQuantity" }; 3201 case -562837097: 3202 /* whenPrepared */ return new String[] { "dateTime" }; 3203 case -940241380: 3204 /* whenHandedOver */ return new String[] { "dateTime" }; 3205 case -1429847026: 3206 /* destination */ return new String[] { "Reference" }; 3207 case -808719889: 3208 /* receiver */ return new String[] { "Reference" }; 3209 case 3387378: 3210 /* note */ return new String[] { "Annotation" }; 3211 case -1201373865: 3212 /* dosageInstruction */ return new String[] { "Dosage" }; 3213 case 826147581: 3214 /* substitution */ return new String[] {}; 3215 case 51602295: 3216 /* detectedIssue */ return new String[] { "Reference" }; 3217 case 1835190426: 3218 /* eventHistory */ return new String[] { "Reference" }; 3219 default: 3220 return super.getTypesForProperty(hash, name); 3221 } 3222 3223 } 3224 3225 @Override 3226 public Base addChild(String name) throws FHIRException { 3227 if (name.equals("identifier")) { 3228 return addIdentifier(); 3229 } else if (name.equals("partOf")) { 3230 return addPartOf(); 3231 } else if (name.equals("status")) { 3232 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.status"); 3233 } else if (name.equals("statusReasonCodeableConcept")) { 3234 this.statusReason = new CodeableConcept(); 3235 return this.statusReason; 3236 } else if (name.equals("statusReasonReference")) { 3237 this.statusReason = new Reference(); 3238 return this.statusReason; 3239 } else if (name.equals("category")) { 3240 this.category = new CodeableConcept(); 3241 return this.category; 3242 } else if (name.equals("medicationCodeableConcept")) { 3243 this.medication = new CodeableConcept(); 3244 return this.medication; 3245 } else if (name.equals("medicationReference")) { 3246 this.medication = new Reference(); 3247 return this.medication; 3248 } else if (name.equals("subject")) { 3249 this.subject = new Reference(); 3250 return this.subject; 3251 } else if (name.equals("context")) { 3252 this.context = new Reference(); 3253 return this.context; 3254 } else if (name.equals("supportingInformation")) { 3255 return addSupportingInformation(); 3256 } else if (name.equals("performer")) { 3257 return addPerformer(); 3258 } else if (name.equals("location")) { 3259 this.location = new Reference(); 3260 return this.location; 3261 } else if (name.equals("authorizingPrescription")) { 3262 return addAuthorizingPrescription(); 3263 } else if (name.equals("type")) { 3264 this.type = new CodeableConcept(); 3265 return this.type; 3266 } else if (name.equals("quantity")) { 3267 this.quantity = new Quantity(); 3268 return this.quantity; 3269 } else if (name.equals("daysSupply")) { 3270 this.daysSupply = new Quantity(); 3271 return this.daysSupply; 3272 } else if (name.equals("whenPrepared")) { 3273 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenPrepared"); 3274 } else if (name.equals("whenHandedOver")) { 3275 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenHandedOver"); 3276 } else if (name.equals("destination")) { 3277 this.destination = new Reference(); 3278 return this.destination; 3279 } else if (name.equals("receiver")) { 3280 return addReceiver(); 3281 } else if (name.equals("note")) { 3282 return addNote(); 3283 } else if (name.equals("dosageInstruction")) { 3284 return addDosageInstruction(); 3285 } else if (name.equals("substitution")) { 3286 this.substitution = new MedicationDispenseSubstitutionComponent(); 3287 return this.substitution; 3288 } else if (name.equals("detectedIssue")) { 3289 return addDetectedIssue(); 3290 } else if (name.equals("eventHistory")) { 3291 return addEventHistory(); 3292 } else 3293 return super.addChild(name); 3294 } 3295 3296 public String fhirType() { 3297 return "MedicationDispense"; 3298 3299 } 3300 3301 public MedicationDispense copy() { 3302 MedicationDispense dst = new MedicationDispense(); 3303 copyValues(dst); 3304 return dst; 3305 } 3306 3307 public void copyValues(MedicationDispense dst) { 3308 super.copyValues(dst); 3309 if (identifier != null) { 3310 dst.identifier = new ArrayList<Identifier>(); 3311 for (Identifier i : identifier) 3312 dst.identifier.add(i.copy()); 3313 } 3314 ; 3315 if (partOf != null) { 3316 dst.partOf = new ArrayList<Reference>(); 3317 for (Reference i : partOf) 3318 dst.partOf.add(i.copy()); 3319 } 3320 ; 3321 dst.status = status == null ? null : status.copy(); 3322 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3323 dst.category = category == null ? null : category.copy(); 3324 dst.medication = medication == null ? null : medication.copy(); 3325 dst.subject = subject == null ? null : subject.copy(); 3326 dst.context = context == null ? null : context.copy(); 3327 if (supportingInformation != null) { 3328 dst.supportingInformation = new ArrayList<Reference>(); 3329 for (Reference i : supportingInformation) 3330 dst.supportingInformation.add(i.copy()); 3331 } 3332 ; 3333 if (performer != null) { 3334 dst.performer = new ArrayList<MedicationDispensePerformerComponent>(); 3335 for (MedicationDispensePerformerComponent i : performer) 3336 dst.performer.add(i.copy()); 3337 } 3338 ; 3339 dst.location = location == null ? null : location.copy(); 3340 if (authorizingPrescription != null) { 3341 dst.authorizingPrescription = new ArrayList<Reference>(); 3342 for (Reference i : authorizingPrescription) 3343 dst.authorizingPrescription.add(i.copy()); 3344 } 3345 ; 3346 dst.type = type == null ? null : type.copy(); 3347 dst.quantity = quantity == null ? null : quantity.copy(); 3348 dst.daysSupply = daysSupply == null ? null : daysSupply.copy(); 3349 dst.whenPrepared = whenPrepared == null ? null : whenPrepared.copy(); 3350 dst.whenHandedOver = whenHandedOver == null ? null : whenHandedOver.copy(); 3351 dst.destination = destination == null ? null : destination.copy(); 3352 if (receiver != null) { 3353 dst.receiver = new ArrayList<Reference>(); 3354 for (Reference i : receiver) 3355 dst.receiver.add(i.copy()); 3356 } 3357 ; 3358 if (note != null) { 3359 dst.note = new ArrayList<Annotation>(); 3360 for (Annotation i : note) 3361 dst.note.add(i.copy()); 3362 } 3363 ; 3364 if (dosageInstruction != null) { 3365 dst.dosageInstruction = new ArrayList<Dosage>(); 3366 for (Dosage i : dosageInstruction) 3367 dst.dosageInstruction.add(i.copy()); 3368 } 3369 ; 3370 dst.substitution = substitution == null ? null : substitution.copy(); 3371 if (detectedIssue != null) { 3372 dst.detectedIssue = new ArrayList<Reference>(); 3373 for (Reference i : detectedIssue) 3374 dst.detectedIssue.add(i.copy()); 3375 } 3376 ; 3377 if (eventHistory != null) { 3378 dst.eventHistory = new ArrayList<Reference>(); 3379 for (Reference i : eventHistory) 3380 dst.eventHistory.add(i.copy()); 3381 } 3382 ; 3383 } 3384 3385 protected MedicationDispense typedCopy() { 3386 return copy(); 3387 } 3388 3389 @Override 3390 public boolean equalsDeep(Base other_) { 3391 if (!super.equalsDeep(other_)) 3392 return false; 3393 if (!(other_ instanceof MedicationDispense)) 3394 return false; 3395 MedicationDispense o = (MedicationDispense) other_; 3396 return compareDeep(identifier, o.identifier, true) && compareDeep(partOf, o.partOf, true) 3397 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 3398 && compareDeep(category, o.category, true) && compareDeep(medication, o.medication, true) 3399 && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 3400 && compareDeep(supportingInformation, o.supportingInformation, true) 3401 && compareDeep(performer, o.performer, true) && compareDeep(location, o.location, true) 3402 && compareDeep(authorizingPrescription, o.authorizingPrescription, true) && compareDeep(type, o.type, true) 3403 && compareDeep(quantity, o.quantity, true) && compareDeep(daysSupply, o.daysSupply, true) 3404 && compareDeep(whenPrepared, o.whenPrepared, true) && compareDeep(whenHandedOver, o.whenHandedOver, true) 3405 && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) 3406 && compareDeep(note, o.note, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 3407 && compareDeep(substitution, o.substitution, true) && compareDeep(detectedIssue, o.detectedIssue, true) 3408 && compareDeep(eventHistory, o.eventHistory, true); 3409 } 3410 3411 @Override 3412 public boolean equalsShallow(Base other_) { 3413 if (!super.equalsShallow(other_)) 3414 return false; 3415 if (!(other_ instanceof MedicationDispense)) 3416 return false; 3417 MedicationDispense o = (MedicationDispense) other_; 3418 return compareValues(status, o.status, true) && compareValues(whenPrepared, o.whenPrepared, true) 3419 && compareValues(whenHandedOver, o.whenHandedOver, true); 3420 } 3421 3422 public boolean isEmpty() { 3423 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, partOf, status, statusReason, category, 3424 medication, subject, context, supportingInformation, performer, location, authorizingPrescription, type, 3425 quantity, daysSupply, whenPrepared, whenHandedOver, destination, receiver, note, dosageInstruction, 3426 substitution, detectedIssue, eventHistory); 3427 } 3428 3429 @Override 3430 public ResourceType getResourceType() { 3431 return ResourceType.MedicationDispense; 3432 } 3433 3434 /** 3435 * Search parameter: <b>identifier</b> 3436 * <p> 3437 * Description: <b>Returns dispenses with this external identifier</b><br> 3438 * Type: <b>token</b><br> 3439 * Path: <b>MedicationDispense.identifier</b><br> 3440 * </p> 3441 */ 3442 @SearchParamDefinition(name = "identifier", path = "MedicationDispense.identifier", description = "Returns dispenses with this external identifier", type = "token") 3443 public static final String SP_IDENTIFIER = "identifier"; 3444 /** 3445 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3446 * <p> 3447 * Description: <b>Returns dispenses with this external identifier</b><br> 3448 * Type: <b>token</b><br> 3449 * Path: <b>MedicationDispense.identifier</b><br> 3450 * </p> 3451 */ 3452 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3453 SP_IDENTIFIER); 3454 3455 /** 3456 * Search parameter: <b>performer</b> 3457 * <p> 3458 * Description: <b>Returns dispenses performed by a specific individual</b><br> 3459 * Type: <b>reference</b><br> 3460 * Path: <b>MedicationDispense.performer.actor</b><br> 3461 * </p> 3462 */ 3463 @SearchParamDefinition(name = "performer", path = "MedicationDispense.performer.actor", description = "Returns dispenses performed by a specific individual", type = "reference", providesMembershipIn = { 3464 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 3465 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3466 public static final String SP_PERFORMER = "performer"; 3467 /** 3468 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3469 * <p> 3470 * Description: <b>Returns dispenses performed by a specific individual</b><br> 3471 * Type: <b>reference</b><br> 3472 * Path: <b>MedicationDispense.performer.actor</b><br> 3473 * </p> 3474 */ 3475 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3476 SP_PERFORMER); 3477 3478 /** 3479 * Constant for fluent queries to be used to add include statements. Specifies 3480 * the path value of "<b>MedicationDispense:performer</b>". 3481 */ 3482 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 3483 "MedicationDispense:performer").toLocked(); 3484 3485 /** 3486 * Search parameter: <b>code</b> 3487 * <p> 3488 * Description: <b>Returns dispenses of this medicine code</b><br> 3489 * Type: <b>token</b><br> 3490 * Path: <b>MedicationDispense.medicationCodeableConcept</b><br> 3491 * </p> 3492 */ 3493 @SearchParamDefinition(name = "code", path = "(MedicationDispense.medication as CodeableConcept)", description = "Returns dispenses of this medicine code", type = "token") 3494 public static final String SP_CODE = "code"; 3495 /** 3496 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3497 * <p> 3498 * Description: <b>Returns dispenses of this medicine code</b><br> 3499 * Type: <b>token</b><br> 3500 * Path: <b>MedicationDispense.medicationCodeableConcept</b><br> 3501 * </p> 3502 */ 3503 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3504 SP_CODE); 3505 3506 /** 3507 * Search parameter: <b>receiver</b> 3508 * <p> 3509 * Description: <b>The identity of a receiver to list dispenses for</b><br> 3510 * Type: <b>reference</b><br> 3511 * Path: <b>MedicationDispense.receiver</b><br> 3512 * </p> 3513 */ 3514 @SearchParamDefinition(name = "receiver", path = "MedicationDispense.receiver", description = "The identity of a receiver to list dispenses for", type = "reference", providesMembershipIn = { 3515 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3516 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Patient.class, 3517 Practitioner.class }) 3518 public static final String SP_RECEIVER = "receiver"; 3519 /** 3520 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 3521 * <p> 3522 * Description: <b>The identity of a receiver to list dispenses for</b><br> 3523 * Type: <b>reference</b><br> 3524 * Path: <b>MedicationDispense.receiver</b><br> 3525 * </p> 3526 */ 3527 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3528 SP_RECEIVER); 3529 3530 /** 3531 * Constant for fluent queries to be used to add include statements. Specifies 3532 * the path value of "<b>MedicationDispense:receiver</b>". 3533 */ 3534 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include( 3535 "MedicationDispense:receiver").toLocked(); 3536 3537 /** 3538 * Search parameter: <b>subject</b> 3539 * <p> 3540 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 3541 * Type: <b>reference</b><br> 3542 * Path: <b>MedicationDispense.subject</b><br> 3543 * </p> 3544 */ 3545 @SearchParamDefinition(name = "subject", path = "MedicationDispense.subject", description = "The identity of a patient for whom to list dispenses", type = "reference", providesMembershipIn = { 3546 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3547 public static final String SP_SUBJECT = "subject"; 3548 /** 3549 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3550 * <p> 3551 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 3552 * Type: <b>reference</b><br> 3553 * Path: <b>MedicationDispense.subject</b><br> 3554 * </p> 3555 */ 3556 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3557 SP_SUBJECT); 3558 3559 /** 3560 * Constant for fluent queries to be used to add include statements. Specifies 3561 * the path value of "<b>MedicationDispense:subject</b>". 3562 */ 3563 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3564 "MedicationDispense:subject").toLocked(); 3565 3566 /** 3567 * Search parameter: <b>destination</b> 3568 * <p> 3569 * Description: <b>Returns dispenses that should be sent to a specific 3570 * destination</b><br> 3571 * Type: <b>reference</b><br> 3572 * Path: <b>MedicationDispense.destination</b><br> 3573 * </p> 3574 */ 3575 @SearchParamDefinition(name = "destination", path = "MedicationDispense.destination", description = "Returns dispenses that should be sent to a specific destination", type = "reference", target = { 3576 Location.class }) 3577 public static final String SP_DESTINATION = "destination"; 3578 /** 3579 * <b>Fluent Client</b> search parameter constant for <b>destination</b> 3580 * <p> 3581 * Description: <b>Returns dispenses that should be sent to a specific 3582 * destination</b><br> 3583 * Type: <b>reference</b><br> 3584 * Path: <b>MedicationDispense.destination</b><br> 3585 * </p> 3586 */ 3587 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DESTINATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3588 SP_DESTINATION); 3589 3590 /** 3591 * Constant for fluent queries to be used to add include statements. Specifies 3592 * the path value of "<b>MedicationDispense:destination</b>". 3593 */ 3594 public static final ca.uhn.fhir.model.api.Include INCLUDE_DESTINATION = new ca.uhn.fhir.model.api.Include( 3595 "MedicationDispense:destination").toLocked(); 3596 3597 /** 3598 * Search parameter: <b>medication</b> 3599 * <p> 3600 * Description: <b>Returns dispenses of this medicine resource</b><br> 3601 * Type: <b>reference</b><br> 3602 * Path: <b>MedicationDispense.medicationReference</b><br> 3603 * </p> 3604 */ 3605 @SearchParamDefinition(name = "medication", path = "(MedicationDispense.medication as Reference)", description = "Returns dispenses of this medicine resource", type = "reference", target = { 3606 Medication.class }) 3607 public static final String SP_MEDICATION = "medication"; 3608 /** 3609 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3610 * <p> 3611 * Description: <b>Returns dispenses of this medicine resource</b><br> 3612 * Type: <b>reference</b><br> 3613 * Path: <b>MedicationDispense.medicationReference</b><br> 3614 * </p> 3615 */ 3616 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3617 SP_MEDICATION); 3618 3619 /** 3620 * Constant for fluent queries to be used to add include statements. Specifies 3621 * the path value of "<b>MedicationDispense:medication</b>". 3622 */ 3623 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include( 3624 "MedicationDispense:medication").toLocked(); 3625 3626 /** 3627 * Search parameter: <b>responsibleparty</b> 3628 * <p> 3629 * Description: <b>Returns dispenses with the specified responsible 3630 * party</b><br> 3631 * Type: <b>reference</b><br> 3632 * Path: <b>MedicationDispense.substitution.responsibleParty</b><br> 3633 * </p> 3634 */ 3635 @SearchParamDefinition(name = "responsibleparty", path = "MedicationDispense.substitution.responsibleParty", description = "Returns dispenses with the specified responsible party", type = "reference", target = { 3636 Practitioner.class, PractitionerRole.class }) 3637 public static final String SP_RESPONSIBLEPARTY = "responsibleparty"; 3638 /** 3639 * <b>Fluent Client</b> search parameter constant for <b>responsibleparty</b> 3640 * <p> 3641 * Description: <b>Returns dispenses with the specified responsible 3642 * party</b><br> 3643 * Type: <b>reference</b><br> 3644 * Path: <b>MedicationDispense.substitution.responsibleParty</b><br> 3645 * </p> 3646 */ 3647 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSIBLEPARTY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3648 SP_RESPONSIBLEPARTY); 3649 3650 /** 3651 * Constant for fluent queries to be used to add include statements. Specifies 3652 * the path value of "<b>MedicationDispense:responsibleparty</b>". 3653 */ 3654 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSIBLEPARTY = new ca.uhn.fhir.model.api.Include( 3655 "MedicationDispense:responsibleparty").toLocked(); 3656 3657 /** 3658 * Search parameter: <b>type</b> 3659 * <p> 3660 * Description: <b>Returns dispenses of a specific type</b><br> 3661 * Type: <b>token</b><br> 3662 * Path: <b>MedicationDispense.type</b><br> 3663 * </p> 3664 */ 3665 @SearchParamDefinition(name = "type", path = "MedicationDispense.type", description = "Returns dispenses of a specific type", type = "token") 3666 public static final String SP_TYPE = "type"; 3667 /** 3668 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3669 * <p> 3670 * Description: <b>Returns dispenses of a specific type</b><br> 3671 * Type: <b>token</b><br> 3672 * Path: <b>MedicationDispense.type</b><br> 3673 * </p> 3674 */ 3675 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3676 SP_TYPE); 3677 3678 /** 3679 * Search parameter: <b>whenhandedover</b> 3680 * <p> 3681 * Description: <b>Returns dispenses handed over on this date</b><br> 3682 * Type: <b>date</b><br> 3683 * Path: <b>MedicationDispense.whenHandedOver</b><br> 3684 * </p> 3685 */ 3686 @SearchParamDefinition(name = "whenhandedover", path = "MedicationDispense.whenHandedOver", description = "Returns dispenses handed over on this date", type = "date") 3687 public static final String SP_WHENHANDEDOVER = "whenhandedover"; 3688 /** 3689 * <b>Fluent Client</b> search parameter constant for <b>whenhandedover</b> 3690 * <p> 3691 * Description: <b>Returns dispenses handed over on this date</b><br> 3692 * Type: <b>date</b><br> 3693 * Path: <b>MedicationDispense.whenHandedOver</b><br> 3694 * </p> 3695 */ 3696 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHENHANDEDOVER = new ca.uhn.fhir.rest.gclient.DateClientParam( 3697 SP_WHENHANDEDOVER); 3698 3699 /** 3700 * Search parameter: <b>whenprepared</b> 3701 * <p> 3702 * Description: <b>Returns dispenses prepared on this date</b><br> 3703 * Type: <b>date</b><br> 3704 * Path: <b>MedicationDispense.whenPrepared</b><br> 3705 * </p> 3706 */ 3707 @SearchParamDefinition(name = "whenprepared", path = "MedicationDispense.whenPrepared", description = "Returns dispenses prepared on this date", type = "date") 3708 public static final String SP_WHENPREPARED = "whenprepared"; 3709 /** 3710 * <b>Fluent Client</b> search parameter constant for <b>whenprepared</b> 3711 * <p> 3712 * Description: <b>Returns dispenses prepared on this date</b><br> 3713 * Type: <b>date</b><br> 3714 * Path: <b>MedicationDispense.whenPrepared</b><br> 3715 * </p> 3716 */ 3717 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHENPREPARED = new ca.uhn.fhir.rest.gclient.DateClientParam( 3718 SP_WHENPREPARED); 3719 3720 /** 3721 * Search parameter: <b>prescription</b> 3722 * <p> 3723 * Description: <b>The identity of a prescription to list dispenses from</b><br> 3724 * Type: <b>reference</b><br> 3725 * Path: <b>MedicationDispense.authorizingPrescription</b><br> 3726 * </p> 3727 */ 3728 @SearchParamDefinition(name = "prescription", path = "MedicationDispense.authorizingPrescription", description = "The identity of a prescription to list dispenses from", type = "reference", target = { 3729 MedicationRequest.class }) 3730 public static final String SP_PRESCRIPTION = "prescription"; 3731 /** 3732 * <b>Fluent Client</b> search parameter constant for <b>prescription</b> 3733 * <p> 3734 * Description: <b>The identity of a prescription to list dispenses from</b><br> 3735 * Type: <b>reference</b><br> 3736 * Path: <b>MedicationDispense.authorizingPrescription</b><br> 3737 * </p> 3738 */ 3739 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIPTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3740 SP_PRESCRIPTION); 3741 3742 /** 3743 * Constant for fluent queries to be used to add include statements. Specifies 3744 * the path value of "<b>MedicationDispense:prescription</b>". 3745 */ 3746 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIPTION = new ca.uhn.fhir.model.api.Include( 3747 "MedicationDispense:prescription").toLocked(); 3748 3749 /** 3750 * Search parameter: <b>patient</b> 3751 * <p> 3752 * Description: <b>The identity of a patient to list dispenses for</b><br> 3753 * Type: <b>reference</b><br> 3754 * Path: <b>MedicationDispense.subject</b><br> 3755 * </p> 3756 */ 3757 @SearchParamDefinition(name = "patient", path = "MedicationDispense.subject.where(resolve() is Patient)", description = "The identity of a patient to list dispenses for", type = "reference", providesMembershipIn = { 3758 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3759 public static final String SP_PATIENT = "patient"; 3760 /** 3761 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3762 * <p> 3763 * Description: <b>The identity of a patient to list dispenses for</b><br> 3764 * Type: <b>reference</b><br> 3765 * Path: <b>MedicationDispense.subject</b><br> 3766 * </p> 3767 */ 3768 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3769 SP_PATIENT); 3770 3771 /** 3772 * Constant for fluent queries to be used to add include statements. Specifies 3773 * the path value of "<b>MedicationDispense:patient</b>". 3774 */ 3775 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3776 "MedicationDispense:patient").toLocked(); 3777 3778 /** 3779 * Search parameter: <b>context</b> 3780 * <p> 3781 * Description: <b>Returns dispenses with a specific context (episode or episode 3782 * of care)</b><br> 3783 * Type: <b>reference</b><br> 3784 * Path: <b>MedicationDispense.context</b><br> 3785 * </p> 3786 */ 3787 @SearchParamDefinition(name = "context", path = "MedicationDispense.context", description = "Returns dispenses with a specific context (episode or episode of care)", type = "reference", target = { 3788 Encounter.class, EpisodeOfCare.class }) 3789 public static final String SP_CONTEXT = "context"; 3790 /** 3791 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3792 * <p> 3793 * Description: <b>Returns dispenses with a specific context (episode or episode 3794 * of care)</b><br> 3795 * Type: <b>reference</b><br> 3796 * Path: <b>MedicationDispense.context</b><br> 3797 * </p> 3798 */ 3799 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3800 SP_CONTEXT); 3801 3802 /** 3803 * Constant for fluent queries to be used to add include statements. Specifies 3804 * the path value of "<b>MedicationDispense:context</b>". 3805 */ 3806 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include( 3807 "MedicationDispense:context").toLocked(); 3808 3809 /** 3810 * Search parameter: <b>status</b> 3811 * <p> 3812 * Description: <b>Returns dispenses with a specified dispense status</b><br> 3813 * Type: <b>token</b><br> 3814 * Path: <b>MedicationDispense.status</b><br> 3815 * </p> 3816 */ 3817 @SearchParamDefinition(name = "status", path = "MedicationDispense.status", description = "Returns dispenses with a specified dispense status", type = "token") 3818 public static final String SP_STATUS = "status"; 3819 /** 3820 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3821 * <p> 3822 * Description: <b>Returns dispenses with a specified dispense status</b><br> 3823 * Type: <b>token</b><br> 3824 * Path: <b>MedicationDispense.status</b><br> 3825 * </p> 3826 */ 3827 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3828 SP_STATUS); 3829 3830}