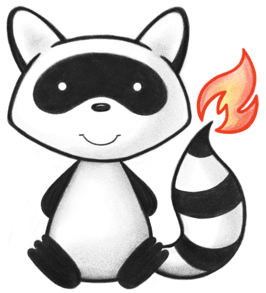
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Information about a medication that is used to support knowledge. 048 */ 049@ResourceDef(name = "MedicationKnowledge", profile = "http://hl7.org/fhir/StructureDefinition/MedicationKnowledge") 050public class MedicationKnowledge extends DomainResource { 051 052 public enum MedicationKnowledgeStatus { 053 /** 054 * The medication is available for use. 055 */ 056 ACTIVE, 057 /** 058 * The medication is not available for use. 059 */ 060 INACTIVE, 061 /** 062 * The medication was entered in error. 063 */ 064 ENTEREDINERROR, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 070 public static MedicationKnowledgeStatus fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("active".equals(codeString)) 074 return ACTIVE; 075 if ("inactive".equals(codeString)) 076 return INACTIVE; 077 if ("entered-in-error".equals(codeString)) 078 return ENTEREDINERROR; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown MedicationKnowledgeStatus code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case ACTIVE: 088 return "active"; 089 case INACTIVE: 090 return "inactive"; 091 case ENTEREDINERROR: 092 return "entered-in-error"; 093 case NULL: 094 return null; 095 default: 096 return "?"; 097 } 098 } 099 100 public String getSystem() { 101 switch (this) { 102 case ACTIVE: 103 return "http://terminology.hl7.org/CodeSystem/medicationknowledge-status"; 104 case INACTIVE: 105 return "http://terminology.hl7.org/CodeSystem/medicationknowledge-status"; 106 case ENTEREDINERROR: 107 return "http://terminology.hl7.org/CodeSystem/medicationknowledge-status"; 108 case NULL: 109 return null; 110 default: 111 return "?"; 112 } 113 } 114 115 public String getDefinition() { 116 switch (this) { 117 case ACTIVE: 118 return "The medication is available for use."; 119 case INACTIVE: 120 return "The medication is not available for use."; 121 case ENTEREDINERROR: 122 return "The medication was entered in error."; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDisplay() { 131 switch (this) { 132 case ACTIVE: 133 return "Active"; 134 case INACTIVE: 135 return "Inactive"; 136 case ENTEREDINERROR: 137 return "Entered in Error"; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 } 145 146 public static class MedicationKnowledgeStatusEnumFactory implements EnumFactory<MedicationKnowledgeStatus> { 147 public MedicationKnowledgeStatus fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("active".equals(codeString)) 152 return MedicationKnowledgeStatus.ACTIVE; 153 if ("inactive".equals(codeString)) 154 return MedicationKnowledgeStatus.INACTIVE; 155 if ("entered-in-error".equals(codeString)) 156 return MedicationKnowledgeStatus.ENTEREDINERROR; 157 throw new IllegalArgumentException("Unknown MedicationKnowledgeStatus code '" + codeString + "'"); 158 } 159 160 public Enumeration<MedicationKnowledgeStatus> fromType(PrimitiveType<?> code) throws FHIRException { 161 if (code == null) 162 return null; 163 if (code.isEmpty()) 164 return new Enumeration<MedicationKnowledgeStatus>(this, MedicationKnowledgeStatus.NULL, code); 165 String codeString = code.asStringValue(); 166 if (codeString == null || "".equals(codeString)) 167 return new Enumeration<MedicationKnowledgeStatus>(this, MedicationKnowledgeStatus.NULL, code); 168 if ("active".equals(codeString)) 169 return new Enumeration<MedicationKnowledgeStatus>(this, MedicationKnowledgeStatus.ACTIVE, code); 170 if ("inactive".equals(codeString)) 171 return new Enumeration<MedicationKnowledgeStatus>(this, MedicationKnowledgeStatus.INACTIVE, code); 172 if ("entered-in-error".equals(codeString)) 173 return new Enumeration<MedicationKnowledgeStatus>(this, MedicationKnowledgeStatus.ENTEREDINERROR, code); 174 throw new FHIRException("Unknown MedicationKnowledgeStatus code '" + codeString + "'"); 175 } 176 177 public String toCode(MedicationKnowledgeStatus code) { 178 if (code == MedicationKnowledgeStatus.NULL) 179 return null; 180 if (code == MedicationKnowledgeStatus.ACTIVE) 181 return "active"; 182 if (code == MedicationKnowledgeStatus.INACTIVE) 183 return "inactive"; 184 if (code == MedicationKnowledgeStatus.ENTEREDINERROR) 185 return "entered-in-error"; 186 return "?"; 187 } 188 189 public String toSystem(MedicationKnowledgeStatus code) { 190 return code.getSystem(); 191 } 192 } 193 194 @Block() 195 public static class MedicationKnowledgeRelatedMedicationKnowledgeComponent extends BackboneElement 196 implements IBaseBackboneElement { 197 /** 198 * The category of the associated medication knowledge reference. 199 */ 200 @Child(name = "type", type = { 201 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 202 @Description(shortDefinition = "Category of medicationKnowledge", formalDefinition = "The category of the associated medication knowledge reference.") 203 protected CodeableConcept type; 204 205 /** 206 * Associated documentation about the associated medication knowledge. 207 */ 208 @Child(name = "reference", type = { 209 MedicationKnowledge.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 210 @Description(shortDefinition = "Associated documentation about the associated medication knowledge", formalDefinition = "Associated documentation about the associated medication knowledge.") 211 protected List<Reference> reference; 212 /** 213 * The actual objects that are the target of the reference (Associated 214 * documentation about the associated medication knowledge.) 215 */ 216 protected List<MedicationKnowledge> referenceTarget; 217 218 private static final long serialVersionUID = 1285880636L; 219 220 /** 221 * Constructor 222 */ 223 public MedicationKnowledgeRelatedMedicationKnowledgeComponent() { 224 super(); 225 } 226 227 /** 228 * Constructor 229 */ 230 public MedicationKnowledgeRelatedMedicationKnowledgeComponent(CodeableConcept type) { 231 super(); 232 this.type = type; 233 } 234 235 /** 236 * @return {@link #type} (The category of the associated medication knowledge 237 * reference.) 238 */ 239 public CodeableConcept getType() { 240 if (this.type == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create MedicationKnowledgeRelatedMedicationKnowledgeComponent.type"); 243 else if (Configuration.doAutoCreate()) 244 this.type = new CodeableConcept(); // cc 245 return this.type; 246 } 247 248 public boolean hasType() { 249 return this.type != null && !this.type.isEmpty(); 250 } 251 252 /** 253 * @param value {@link #type} (The category of the associated medication 254 * knowledge reference.) 255 */ 256 public MedicationKnowledgeRelatedMedicationKnowledgeComponent setType(CodeableConcept value) { 257 this.type = value; 258 return this; 259 } 260 261 /** 262 * @return {@link #reference} (Associated documentation about the associated 263 * medication knowledge.) 264 */ 265 public List<Reference> getReference() { 266 if (this.reference == null) 267 this.reference = new ArrayList<Reference>(); 268 return this.reference; 269 } 270 271 /** 272 * @return Returns a reference to <code>this</code> for easy method chaining 273 */ 274 public MedicationKnowledgeRelatedMedicationKnowledgeComponent setReference(List<Reference> theReference) { 275 this.reference = theReference; 276 return this; 277 } 278 279 public boolean hasReference() { 280 if (this.reference == null) 281 return false; 282 for (Reference item : this.reference) 283 if (!item.isEmpty()) 284 return true; 285 return false; 286 } 287 288 public Reference addReference() { // 3 289 Reference t = new Reference(); 290 if (this.reference == null) 291 this.reference = new ArrayList<Reference>(); 292 this.reference.add(t); 293 return t; 294 } 295 296 public MedicationKnowledgeRelatedMedicationKnowledgeComponent addReference(Reference t) { // 3 297 if (t == null) 298 return this; 299 if (this.reference == null) 300 this.reference = new ArrayList<Reference>(); 301 this.reference.add(t); 302 return this; 303 } 304 305 /** 306 * @return The first repetition of repeating field {@link #reference}, creating 307 * it if it does not already exist 308 */ 309 public Reference getReferenceFirstRep() { 310 if (getReference().isEmpty()) { 311 addReference(); 312 } 313 return getReference().get(0); 314 } 315 316 /** 317 * @deprecated Use Reference#setResource(IBaseResource) instead 318 */ 319 @Deprecated 320 public List<MedicationKnowledge> getReferenceTarget() { 321 if (this.referenceTarget == null) 322 this.referenceTarget = new ArrayList<MedicationKnowledge>(); 323 return this.referenceTarget; 324 } 325 326 /** 327 * @deprecated Use Reference#setResource(IBaseResource) instead 328 */ 329 @Deprecated 330 public MedicationKnowledge addReferenceTarget() { 331 MedicationKnowledge r = new MedicationKnowledge(); 332 if (this.referenceTarget == null) 333 this.referenceTarget = new ArrayList<MedicationKnowledge>(); 334 this.referenceTarget.add(r); 335 return r; 336 } 337 338 protected void listChildren(List<Property> children) { 339 super.listChildren(children); 340 children.add(new Property("type", "CodeableConcept", 341 "The category of the associated medication knowledge reference.", 0, 1, type)); 342 children.add(new Property("reference", "Reference(MedicationKnowledge)", 343 "Associated documentation about the associated medication knowledge.", 0, java.lang.Integer.MAX_VALUE, 344 reference)); 345 } 346 347 @Override 348 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 349 switch (_hash) { 350 case 3575610: 351 /* type */ return new Property("type", "CodeableConcept", 352 "The category of the associated medication knowledge reference.", 0, 1, type); 353 case -925155509: 354 /* reference */ return new Property("reference", "Reference(MedicationKnowledge)", 355 "Associated documentation about the associated medication knowledge.", 0, java.lang.Integer.MAX_VALUE, 356 reference); 357 default: 358 return super.getNamedProperty(_hash, _name, _checkValid); 359 } 360 361 } 362 363 @Override 364 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 365 switch (hash) { 366 case 3575610: 367 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 368 case -925155509: 369 /* reference */ return this.reference == null ? new Base[0] 370 : this.reference.toArray(new Base[this.reference.size()]); // Reference 371 default: 372 return super.getProperty(hash, name, checkValid); 373 } 374 375 } 376 377 @Override 378 public Base setProperty(int hash, String name, Base value) throws FHIRException { 379 switch (hash) { 380 case 3575610: // type 381 this.type = castToCodeableConcept(value); // CodeableConcept 382 return value; 383 case -925155509: // reference 384 this.getReference().add(castToReference(value)); // Reference 385 return value; 386 default: 387 return super.setProperty(hash, name, value); 388 } 389 390 } 391 392 @Override 393 public Base setProperty(String name, Base value) throws FHIRException { 394 if (name.equals("type")) { 395 this.type = castToCodeableConcept(value); // CodeableConcept 396 } else if (name.equals("reference")) { 397 this.getReference().add(castToReference(value)); 398 } else 399 return super.setProperty(name, value); 400 return value; 401 } 402 403 @Override 404 public void removeChild(String name, Base value) throws FHIRException { 405 if (name.equals("type")) { 406 this.type = null; 407 } else if (name.equals("reference")) { 408 this.getReference().remove(castToReference(value)); 409 } else 410 super.removeChild(name, value); 411 412 } 413 414 @Override 415 public Base makeProperty(int hash, String name) throws FHIRException { 416 switch (hash) { 417 case 3575610: 418 return getType(); 419 case -925155509: 420 return addReference(); 421 default: 422 return super.makeProperty(hash, name); 423 } 424 425 } 426 427 @Override 428 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 429 switch (hash) { 430 case 3575610: 431 /* type */ return new String[] { "CodeableConcept" }; 432 case -925155509: 433 /* reference */ return new String[] { "Reference" }; 434 default: 435 return super.getTypesForProperty(hash, name); 436 } 437 438 } 439 440 @Override 441 public Base addChild(String name) throws FHIRException { 442 if (name.equals("type")) { 443 this.type = new CodeableConcept(); 444 return this.type; 445 } else if (name.equals("reference")) { 446 return addReference(); 447 } else 448 return super.addChild(name); 449 } 450 451 public MedicationKnowledgeRelatedMedicationKnowledgeComponent copy() { 452 MedicationKnowledgeRelatedMedicationKnowledgeComponent dst = new MedicationKnowledgeRelatedMedicationKnowledgeComponent(); 453 copyValues(dst); 454 return dst; 455 } 456 457 public void copyValues(MedicationKnowledgeRelatedMedicationKnowledgeComponent dst) { 458 super.copyValues(dst); 459 dst.type = type == null ? null : type.copy(); 460 if (reference != null) { 461 dst.reference = new ArrayList<Reference>(); 462 for (Reference i : reference) 463 dst.reference.add(i.copy()); 464 } 465 ; 466 } 467 468 @Override 469 public boolean equalsDeep(Base other_) { 470 if (!super.equalsDeep(other_)) 471 return false; 472 if (!(other_ instanceof MedicationKnowledgeRelatedMedicationKnowledgeComponent)) 473 return false; 474 MedicationKnowledgeRelatedMedicationKnowledgeComponent o = (MedicationKnowledgeRelatedMedicationKnowledgeComponent) other_; 475 return compareDeep(type, o.type, true) && compareDeep(reference, o.reference, true); 476 } 477 478 @Override 479 public boolean equalsShallow(Base other_) { 480 if (!super.equalsShallow(other_)) 481 return false; 482 if (!(other_ instanceof MedicationKnowledgeRelatedMedicationKnowledgeComponent)) 483 return false; 484 MedicationKnowledgeRelatedMedicationKnowledgeComponent o = (MedicationKnowledgeRelatedMedicationKnowledgeComponent) other_; 485 return true; 486 } 487 488 public boolean isEmpty() { 489 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, reference); 490 } 491 492 public String fhirType() { 493 return "MedicationKnowledge.relatedMedicationKnowledge"; 494 495 } 496 497 } 498 499 @Block() 500 public static class MedicationKnowledgeMonographComponent extends BackboneElement implements IBaseBackboneElement { 501 /** 502 * The category of documentation about the medication. (e.g. professional 503 * monograph, patient education monograph). 504 */ 505 @Child(name = "type", type = { 506 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 507 @Description(shortDefinition = "The category of medication document", formalDefinition = "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).") 508 protected CodeableConcept type; 509 510 /** 511 * Associated documentation about the medication. 512 */ 513 @Child(name = "source", type = { DocumentReference.class, 514 Media.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 515 @Description(shortDefinition = "Associated documentation about the medication", formalDefinition = "Associated documentation about the medication.") 516 protected Reference source; 517 518 /** 519 * The actual object that is the target of the reference (Associated 520 * documentation about the medication.) 521 */ 522 protected Resource sourceTarget; 523 524 private static final long serialVersionUID = 1392095381L; 525 526 /** 527 * Constructor 528 */ 529 public MedicationKnowledgeMonographComponent() { 530 super(); 531 } 532 533 /** 534 * @return {@link #type} (The category of documentation about the medication. 535 * (e.g. professional monograph, patient education monograph).) 536 */ 537 public CodeableConcept getType() { 538 if (this.type == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create MedicationKnowledgeMonographComponent.type"); 541 else if (Configuration.doAutoCreate()) 542 this.type = new CodeableConcept(); // cc 543 return this.type; 544 } 545 546 public boolean hasType() { 547 return this.type != null && !this.type.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #type} (The category of documentation about the 552 * medication. (e.g. professional monograph, patient education 553 * monograph).) 554 */ 555 public MedicationKnowledgeMonographComponent setType(CodeableConcept value) { 556 this.type = value; 557 return this; 558 } 559 560 /** 561 * @return {@link #source} (Associated documentation about the medication.) 562 */ 563 public Reference getSource() { 564 if (this.source == null) 565 if (Configuration.errorOnAutoCreate()) 566 throw new Error("Attempt to auto-create MedicationKnowledgeMonographComponent.source"); 567 else if (Configuration.doAutoCreate()) 568 this.source = new Reference(); // cc 569 return this.source; 570 } 571 572 public boolean hasSource() { 573 return this.source != null && !this.source.isEmpty(); 574 } 575 576 /** 577 * @param value {@link #source} (Associated documentation about the medication.) 578 */ 579 public MedicationKnowledgeMonographComponent setSource(Reference value) { 580 this.source = value; 581 return this; 582 } 583 584 /** 585 * @return {@link #source} The actual object that is the target of the 586 * reference. The reference library doesn't populate this, but you can 587 * use it to hold the resource if you resolve it. (Associated 588 * documentation about the medication.) 589 */ 590 public Resource getSourceTarget() { 591 return this.sourceTarget; 592 } 593 594 /** 595 * @param value {@link #source} The actual object that is the target of the 596 * reference. The reference library doesn't use these, but you can 597 * use it to hold the resource if you resolve it. (Associated 598 * documentation about the medication.) 599 */ 600 public MedicationKnowledgeMonographComponent setSourceTarget(Resource value) { 601 this.sourceTarget = value; 602 return this; 603 } 604 605 protected void listChildren(List<Property> children) { 606 super.listChildren(children); 607 children.add(new Property("type", "CodeableConcept", 608 "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).", 609 0, 1, type)); 610 children.add(new Property("source", "Reference(DocumentReference|Media)", 611 "Associated documentation about the medication.", 0, 1, source)); 612 } 613 614 @Override 615 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 616 switch (_hash) { 617 case 3575610: 618 /* type */ return new Property("type", "CodeableConcept", 619 "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).", 620 0, 1, type); 621 case -896505829: 622 /* source */ return new Property("source", "Reference(DocumentReference|Media)", 623 "Associated documentation about the medication.", 0, 1, source); 624 default: 625 return super.getNamedProperty(_hash, _name, _checkValid); 626 } 627 628 } 629 630 @Override 631 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 632 switch (hash) { 633 case 3575610: 634 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 635 case -896505829: 636 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 637 default: 638 return super.getProperty(hash, name, checkValid); 639 } 640 641 } 642 643 @Override 644 public Base setProperty(int hash, String name, Base value) throws FHIRException { 645 switch (hash) { 646 case 3575610: // type 647 this.type = castToCodeableConcept(value); // CodeableConcept 648 return value; 649 case -896505829: // source 650 this.source = castToReference(value); // Reference 651 return value; 652 default: 653 return super.setProperty(hash, name, value); 654 } 655 656 } 657 658 @Override 659 public Base setProperty(String name, Base value) throws FHIRException { 660 if (name.equals("type")) { 661 this.type = castToCodeableConcept(value); // CodeableConcept 662 } else if (name.equals("source")) { 663 this.source = castToReference(value); // Reference 664 } else 665 return super.setProperty(name, value); 666 return value; 667 } 668 669 @Override 670 public void removeChild(String name, Base value) throws FHIRException { 671 if (name.equals("type")) { 672 this.type = null; 673 } else if (name.equals("source")) { 674 this.source = null; 675 } else 676 super.removeChild(name, value); 677 678 } 679 680 @Override 681 public Base makeProperty(int hash, String name) throws FHIRException { 682 switch (hash) { 683 case 3575610: 684 return getType(); 685 case -896505829: 686 return getSource(); 687 default: 688 return super.makeProperty(hash, name); 689 } 690 691 } 692 693 @Override 694 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 695 switch (hash) { 696 case 3575610: 697 /* type */ return new String[] { "CodeableConcept" }; 698 case -896505829: 699 /* source */ return new String[] { "Reference" }; 700 default: 701 return super.getTypesForProperty(hash, name); 702 } 703 704 } 705 706 @Override 707 public Base addChild(String name) throws FHIRException { 708 if (name.equals("type")) { 709 this.type = new CodeableConcept(); 710 return this.type; 711 } else if (name.equals("source")) { 712 this.source = new Reference(); 713 return this.source; 714 } else 715 return super.addChild(name); 716 } 717 718 public MedicationKnowledgeMonographComponent copy() { 719 MedicationKnowledgeMonographComponent dst = new MedicationKnowledgeMonographComponent(); 720 copyValues(dst); 721 return dst; 722 } 723 724 public void copyValues(MedicationKnowledgeMonographComponent dst) { 725 super.copyValues(dst); 726 dst.type = type == null ? null : type.copy(); 727 dst.source = source == null ? null : source.copy(); 728 } 729 730 @Override 731 public boolean equalsDeep(Base other_) { 732 if (!super.equalsDeep(other_)) 733 return false; 734 if (!(other_ instanceof MedicationKnowledgeMonographComponent)) 735 return false; 736 MedicationKnowledgeMonographComponent o = (MedicationKnowledgeMonographComponent) other_; 737 return compareDeep(type, o.type, true) && compareDeep(source, o.source, true); 738 } 739 740 @Override 741 public boolean equalsShallow(Base other_) { 742 if (!super.equalsShallow(other_)) 743 return false; 744 if (!(other_ instanceof MedicationKnowledgeMonographComponent)) 745 return false; 746 MedicationKnowledgeMonographComponent o = (MedicationKnowledgeMonographComponent) other_; 747 return true; 748 } 749 750 public boolean isEmpty() { 751 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, source); 752 } 753 754 public String fhirType() { 755 return "MedicationKnowledge.monograph"; 756 757 } 758 759 } 760 761 @Block() 762 public static class MedicationKnowledgeIngredientComponent extends BackboneElement implements IBaseBackboneElement { 763 /** 764 * The actual ingredient - either a substance (simple ingredient) or another 765 * medication. 766 */ 767 @Child(name = "item", type = { CodeableConcept.class, 768 Substance.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 769 @Description(shortDefinition = "Medication(s) or substance(s) contained in the medication", formalDefinition = "The actual ingredient - either a substance (simple ingredient) or another medication.") 770 protected Type item; 771 772 /** 773 * Indication of whether this ingredient affects the therapeutic action of the 774 * drug. 775 */ 776 @Child(name = "isActive", type = { 777 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 778 @Description(shortDefinition = "Active ingredient indicator", formalDefinition = "Indication of whether this ingredient affects the therapeutic action of the drug.") 779 protected BooleanType isActive; 780 781 /** 782 * Specifies how many (or how much) of the items there are in this Medication. 783 * For example, 250 mg per tablet. This is expressed as a ratio where the 784 * numerator is 250mg and the denominator is 1 tablet. 785 */ 786 @Child(name = "strength", type = { Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 787 @Description(shortDefinition = "Quantity of ingredient present", formalDefinition = "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.") 788 protected Ratio strength; 789 790 private static final long serialVersionUID = 1365103497L; 791 792 /** 793 * Constructor 794 */ 795 public MedicationKnowledgeIngredientComponent() { 796 super(); 797 } 798 799 /** 800 * Constructor 801 */ 802 public MedicationKnowledgeIngredientComponent(Type item) { 803 super(); 804 this.item = item; 805 } 806 807 /** 808 * @return {@link #item} (The actual ingredient - either a substance (simple 809 * ingredient) or another medication.) 810 */ 811 public Type getItem() { 812 return this.item; 813 } 814 815 /** 816 * @return {@link #item} (The actual ingredient - either a substance (simple 817 * ingredient) or another medication.) 818 */ 819 public CodeableConcept getItemCodeableConcept() throws FHIRException { 820 if (this.item == null) 821 this.item = new CodeableConcept(); 822 if (!(this.item instanceof CodeableConcept)) 823 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 824 + this.item.getClass().getName() + " was encountered"); 825 return (CodeableConcept) this.item; 826 } 827 828 public boolean hasItemCodeableConcept() { 829 return this != null && this.item instanceof CodeableConcept; 830 } 831 832 /** 833 * @return {@link #item} (The actual ingredient - either a substance (simple 834 * ingredient) or another medication.) 835 */ 836 public Reference getItemReference() throws FHIRException { 837 if (this.item == null) 838 this.item = new Reference(); 839 if (!(this.item instanceof Reference)) 840 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 841 + " was encountered"); 842 return (Reference) this.item; 843 } 844 845 public boolean hasItemReference() { 846 return this != null && this.item instanceof Reference; 847 } 848 849 public boolean hasItem() { 850 return this.item != null && !this.item.isEmpty(); 851 } 852 853 /** 854 * @param value {@link #item} (The actual ingredient - either a substance 855 * (simple ingredient) or another medication.) 856 */ 857 public MedicationKnowledgeIngredientComponent setItem(Type value) { 858 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 859 throw new Error("Not the right type for MedicationKnowledge.ingredient.item[x]: " + value.fhirType()); 860 this.item = value; 861 return this; 862 } 863 864 /** 865 * @return {@link #isActive} (Indication of whether this ingredient affects the 866 * therapeutic action of the drug.). This is the underlying object with 867 * id, value and extensions. The accessor "getIsActive" gives direct 868 * access to the value 869 */ 870 public BooleanType getIsActiveElement() { 871 if (this.isActive == null) 872 if (Configuration.errorOnAutoCreate()) 873 throw new Error("Attempt to auto-create MedicationKnowledgeIngredientComponent.isActive"); 874 else if (Configuration.doAutoCreate()) 875 this.isActive = new BooleanType(); // bb 876 return this.isActive; 877 } 878 879 public boolean hasIsActiveElement() { 880 return this.isActive != null && !this.isActive.isEmpty(); 881 } 882 883 public boolean hasIsActive() { 884 return this.isActive != null && !this.isActive.isEmpty(); 885 } 886 887 /** 888 * @param value {@link #isActive} (Indication of whether this ingredient affects 889 * the therapeutic action of the drug.). This is the underlying 890 * object with id, value and extensions. The accessor "getIsActive" 891 * gives direct access to the value 892 */ 893 public MedicationKnowledgeIngredientComponent setIsActiveElement(BooleanType value) { 894 this.isActive = value; 895 return this; 896 } 897 898 /** 899 * @return Indication of whether this ingredient affects the therapeutic action 900 * of the drug. 901 */ 902 public boolean getIsActive() { 903 return this.isActive == null || this.isActive.isEmpty() ? false : this.isActive.getValue(); 904 } 905 906 /** 907 * @param value Indication of whether this ingredient affects the therapeutic 908 * action of the drug. 909 */ 910 public MedicationKnowledgeIngredientComponent setIsActive(boolean value) { 911 if (this.isActive == null) 912 this.isActive = new BooleanType(); 913 this.isActive.setValue(value); 914 return this; 915 } 916 917 /** 918 * @return {@link #strength} (Specifies how many (or how much) of the items 919 * there are in this Medication. For example, 250 mg per tablet. This is 920 * expressed as a ratio where the numerator is 250mg and the denominator 921 * is 1 tablet.) 922 */ 923 public Ratio getStrength() { 924 if (this.strength == null) 925 if (Configuration.errorOnAutoCreate()) 926 throw new Error("Attempt to auto-create MedicationKnowledgeIngredientComponent.strength"); 927 else if (Configuration.doAutoCreate()) 928 this.strength = new Ratio(); // cc 929 return this.strength; 930 } 931 932 public boolean hasStrength() { 933 return this.strength != null && !this.strength.isEmpty(); 934 } 935 936 /** 937 * @param value {@link #strength} (Specifies how many (or how much) of the items 938 * there are in this Medication. For example, 250 mg per tablet. 939 * This is expressed as a ratio where the numerator is 250mg and 940 * the denominator is 1 tablet.) 941 */ 942 public MedicationKnowledgeIngredientComponent setStrength(Ratio value) { 943 this.strength = value; 944 return this; 945 } 946 947 protected void listChildren(List<Property> children) { 948 super.listChildren(children); 949 children.add(new Property("item[x]", "CodeableConcept|Reference(Substance)", 950 "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item)); 951 children.add(new Property("isActive", "boolean", 952 "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive)); 953 children.add(new Property("strength", "Ratio", 954 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 955 0, 1, strength)); 956 } 957 958 @Override 959 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 960 switch (_hash) { 961 case 2116201613: 962 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Substance)", 963 "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 964 case 3242771: 965 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Substance)", 966 "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 967 case 106644494: 968 /* itemCodeableConcept */ return new Property("item[x]", "CodeableConcept|Reference(Substance)", 969 "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 970 case 1376364920: 971 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Substance)", 972 "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 973 case -748916528: 974 /* isActive */ return new Property("isActive", "boolean", 975 "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive); 976 case 1791316033: 977 /* strength */ return new Property("strength", "Ratio", 978 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 979 0, 1, strength); 980 default: 981 return super.getNamedProperty(_hash, _name, _checkValid); 982 } 983 984 } 985 986 @Override 987 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 988 switch (hash) { 989 case 3242771: 990 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 991 case -748916528: 992 /* isActive */ return this.isActive == null ? new Base[0] : new Base[] { this.isActive }; // BooleanType 993 case 1791316033: 994 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Ratio 995 default: 996 return super.getProperty(hash, name, checkValid); 997 } 998 999 } 1000 1001 @Override 1002 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1003 switch (hash) { 1004 case 3242771: // item 1005 this.item = castToType(value); // Type 1006 return value; 1007 case -748916528: // isActive 1008 this.isActive = castToBoolean(value); // BooleanType 1009 return value; 1010 case 1791316033: // strength 1011 this.strength = castToRatio(value); // Ratio 1012 return value; 1013 default: 1014 return super.setProperty(hash, name, value); 1015 } 1016 1017 } 1018 1019 @Override 1020 public Base setProperty(String name, Base value) throws FHIRException { 1021 if (name.equals("item[x]")) { 1022 this.item = castToType(value); // Type 1023 } else if (name.equals("isActive")) { 1024 this.isActive = castToBoolean(value); // BooleanType 1025 } else if (name.equals("strength")) { 1026 this.strength = castToRatio(value); // Ratio 1027 } else 1028 return super.setProperty(name, value); 1029 return value; 1030 } 1031 1032 @Override 1033 public void removeChild(String name, Base value) throws FHIRException { 1034 if (name.equals("item[x]")) { 1035 this.item = null; 1036 } else if (name.equals("isActive")) { 1037 this.isActive = null; 1038 } else if (name.equals("strength")) { 1039 this.strength = null; 1040 } else 1041 super.removeChild(name, value); 1042 1043 } 1044 1045 @Override 1046 public Base makeProperty(int hash, String name) throws FHIRException { 1047 switch (hash) { 1048 case 2116201613: 1049 return getItem(); 1050 case 3242771: 1051 return getItem(); 1052 case -748916528: 1053 return getIsActiveElement(); 1054 case 1791316033: 1055 return getStrength(); 1056 default: 1057 return super.makeProperty(hash, name); 1058 } 1059 1060 } 1061 1062 @Override 1063 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1064 switch (hash) { 1065 case 3242771: 1066 /* item */ return new String[] { "CodeableConcept", "Reference" }; 1067 case -748916528: 1068 /* isActive */ return new String[] { "boolean" }; 1069 case 1791316033: 1070 /* strength */ return new String[] { "Ratio" }; 1071 default: 1072 return super.getTypesForProperty(hash, name); 1073 } 1074 1075 } 1076 1077 @Override 1078 public Base addChild(String name) throws FHIRException { 1079 if (name.equals("itemCodeableConcept")) { 1080 this.item = new CodeableConcept(); 1081 return this.item; 1082 } else if (name.equals("itemReference")) { 1083 this.item = new Reference(); 1084 return this.item; 1085 } else if (name.equals("isActive")) { 1086 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.isActive"); 1087 } else if (name.equals("strength")) { 1088 this.strength = new Ratio(); 1089 return this.strength; 1090 } else 1091 return super.addChild(name); 1092 } 1093 1094 public MedicationKnowledgeIngredientComponent copy() { 1095 MedicationKnowledgeIngredientComponent dst = new MedicationKnowledgeIngredientComponent(); 1096 copyValues(dst); 1097 return dst; 1098 } 1099 1100 public void copyValues(MedicationKnowledgeIngredientComponent dst) { 1101 super.copyValues(dst); 1102 dst.item = item == null ? null : item.copy(); 1103 dst.isActive = isActive == null ? null : isActive.copy(); 1104 dst.strength = strength == null ? null : strength.copy(); 1105 } 1106 1107 @Override 1108 public boolean equalsDeep(Base other_) { 1109 if (!super.equalsDeep(other_)) 1110 return false; 1111 if (!(other_ instanceof MedicationKnowledgeIngredientComponent)) 1112 return false; 1113 MedicationKnowledgeIngredientComponent o = (MedicationKnowledgeIngredientComponent) other_; 1114 return compareDeep(item, o.item, true) && compareDeep(isActive, o.isActive, true) 1115 && compareDeep(strength, o.strength, true); 1116 } 1117 1118 @Override 1119 public boolean equalsShallow(Base other_) { 1120 if (!super.equalsShallow(other_)) 1121 return false; 1122 if (!(other_ instanceof MedicationKnowledgeIngredientComponent)) 1123 return false; 1124 MedicationKnowledgeIngredientComponent o = (MedicationKnowledgeIngredientComponent) other_; 1125 return compareValues(isActive, o.isActive, true); 1126 } 1127 1128 public boolean isEmpty() { 1129 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, isActive, strength); 1130 } 1131 1132 public String fhirType() { 1133 return "MedicationKnowledge.ingredient"; 1134 1135 } 1136 1137 } 1138 1139 @Block() 1140 public static class MedicationKnowledgeCostComponent extends BackboneElement implements IBaseBackboneElement { 1141 /** 1142 * The category of the cost information. For example, manufacturers' cost, 1143 * patient cost, claim reimbursement cost, actual acquisition cost. 1144 */ 1145 @Child(name = "type", type = { 1146 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1147 @Description(shortDefinition = "The category of the cost information", formalDefinition = "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.") 1148 protected CodeableConcept type; 1149 1150 /** 1151 * The source or owner that assigns the price to the medication. 1152 */ 1153 @Child(name = "source", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1154 @Description(shortDefinition = "The source or owner for the price information", formalDefinition = "The source or owner that assigns the price to the medication.") 1155 protected StringType source; 1156 1157 /** 1158 * The price of the medication. 1159 */ 1160 @Child(name = "cost", type = { Money.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 1161 @Description(shortDefinition = "The price of the medication", formalDefinition = "The price of the medication.") 1162 protected Money cost; 1163 1164 private static final long serialVersionUID = 244671378L; 1165 1166 /** 1167 * Constructor 1168 */ 1169 public MedicationKnowledgeCostComponent() { 1170 super(); 1171 } 1172 1173 /** 1174 * Constructor 1175 */ 1176 public MedicationKnowledgeCostComponent(CodeableConcept type, Money cost) { 1177 super(); 1178 this.type = type; 1179 this.cost = cost; 1180 } 1181 1182 /** 1183 * @return {@link #type} (The category of the cost information. For example, 1184 * manufacturers' cost, patient cost, claim reimbursement cost, actual 1185 * acquisition cost.) 1186 */ 1187 public CodeableConcept getType() { 1188 if (this.type == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.type"); 1191 else if (Configuration.doAutoCreate()) 1192 this.type = new CodeableConcept(); // cc 1193 return this.type; 1194 } 1195 1196 public boolean hasType() { 1197 return this.type != null && !this.type.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #type} (The category of the cost information. For 1202 * example, manufacturers' cost, patient cost, claim reimbursement 1203 * cost, actual acquisition cost.) 1204 */ 1205 public MedicationKnowledgeCostComponent setType(CodeableConcept value) { 1206 this.type = value; 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #source} (The source or owner that assigns the price to the 1212 * medication.). This is the underlying object with id, value and 1213 * extensions. The accessor "getSource" gives direct access to the value 1214 */ 1215 public StringType getSourceElement() { 1216 if (this.source == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.source"); 1219 else if (Configuration.doAutoCreate()) 1220 this.source = new StringType(); // bb 1221 return this.source; 1222 } 1223 1224 public boolean hasSourceElement() { 1225 return this.source != null && !this.source.isEmpty(); 1226 } 1227 1228 public boolean hasSource() { 1229 return this.source != null && !this.source.isEmpty(); 1230 } 1231 1232 /** 1233 * @param value {@link #source} (The source or owner that assigns the price to 1234 * the medication.). This is the underlying object with id, value 1235 * and extensions. The accessor "getSource" gives direct access to 1236 * the value 1237 */ 1238 public MedicationKnowledgeCostComponent setSourceElement(StringType value) { 1239 this.source = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return The source or owner that assigns the price to the medication. 1245 */ 1246 public String getSource() { 1247 return this.source == null ? null : this.source.getValue(); 1248 } 1249 1250 /** 1251 * @param value The source or owner that assigns the price to the medication. 1252 */ 1253 public MedicationKnowledgeCostComponent setSource(String value) { 1254 if (Utilities.noString(value)) 1255 this.source = null; 1256 else { 1257 if (this.source == null) 1258 this.source = new StringType(); 1259 this.source.setValue(value); 1260 } 1261 return this; 1262 } 1263 1264 /** 1265 * @return {@link #cost} (The price of the medication.) 1266 */ 1267 public Money getCost() { 1268 if (this.cost == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.cost"); 1271 else if (Configuration.doAutoCreate()) 1272 this.cost = new Money(); // cc 1273 return this.cost; 1274 } 1275 1276 public boolean hasCost() { 1277 return this.cost != null && !this.cost.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #cost} (The price of the medication.) 1282 */ 1283 public MedicationKnowledgeCostComponent setCost(Money value) { 1284 this.cost = value; 1285 return this; 1286 } 1287 1288 protected void listChildren(List<Property> children) { 1289 super.listChildren(children); 1290 children.add(new Property("type", "CodeableConcept", 1291 "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.", 1292 0, 1, type)); 1293 children.add(new Property("source", "string", "The source or owner that assigns the price to the medication.", 0, 1294 1, source)); 1295 children.add(new Property("cost", "Money", "The price of the medication.", 0, 1, cost)); 1296 } 1297 1298 @Override 1299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1300 switch (_hash) { 1301 case 3575610: 1302 /* type */ return new Property("type", "CodeableConcept", 1303 "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.", 1304 0, 1, type); 1305 case -896505829: 1306 /* source */ return new Property("source", "string", 1307 "The source or owner that assigns the price to the medication.", 0, 1, source); 1308 case 3059661: 1309 /* cost */ return new Property("cost", "Money", "The price of the medication.", 0, 1, cost); 1310 default: 1311 return super.getNamedProperty(_hash, _name, _checkValid); 1312 } 1313 1314 } 1315 1316 @Override 1317 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1318 switch (hash) { 1319 case 3575610: 1320 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1321 case -896505829: 1322 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // StringType 1323 case 3059661: 1324 /* cost */ return this.cost == null ? new Base[0] : new Base[] { this.cost }; // Money 1325 default: 1326 return super.getProperty(hash, name, checkValid); 1327 } 1328 1329 } 1330 1331 @Override 1332 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1333 switch (hash) { 1334 case 3575610: // type 1335 this.type = castToCodeableConcept(value); // CodeableConcept 1336 return value; 1337 case -896505829: // source 1338 this.source = castToString(value); // StringType 1339 return value; 1340 case 3059661: // cost 1341 this.cost = castToMoney(value); // Money 1342 return value; 1343 default: 1344 return super.setProperty(hash, name, value); 1345 } 1346 1347 } 1348 1349 @Override 1350 public Base setProperty(String name, Base value) throws FHIRException { 1351 if (name.equals("type")) { 1352 this.type = castToCodeableConcept(value); // CodeableConcept 1353 } else if (name.equals("source")) { 1354 this.source = castToString(value); // StringType 1355 } else if (name.equals("cost")) { 1356 this.cost = castToMoney(value); // Money 1357 } else 1358 return super.setProperty(name, value); 1359 return value; 1360 } 1361 1362 @Override 1363 public void removeChild(String name, Base value) throws FHIRException { 1364 if (name.equals("type")) { 1365 this.type = null; 1366 } else if (name.equals("source")) { 1367 this.source = null; 1368 } else if (name.equals("cost")) { 1369 this.cost = null; 1370 } else 1371 super.removeChild(name, value); 1372 1373 } 1374 1375 @Override 1376 public Base makeProperty(int hash, String name) throws FHIRException { 1377 switch (hash) { 1378 case 3575610: 1379 return getType(); 1380 case -896505829: 1381 return getSourceElement(); 1382 case 3059661: 1383 return getCost(); 1384 default: 1385 return super.makeProperty(hash, name); 1386 } 1387 1388 } 1389 1390 @Override 1391 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1392 switch (hash) { 1393 case 3575610: 1394 /* type */ return new String[] { "CodeableConcept" }; 1395 case -896505829: 1396 /* source */ return new String[] { "string" }; 1397 case 3059661: 1398 /* cost */ return new String[] { "Money" }; 1399 default: 1400 return super.getTypesForProperty(hash, name); 1401 } 1402 1403 } 1404 1405 @Override 1406 public Base addChild(String name) throws FHIRException { 1407 if (name.equals("type")) { 1408 this.type = new CodeableConcept(); 1409 return this.type; 1410 } else if (name.equals("source")) { 1411 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.source"); 1412 } else if (name.equals("cost")) { 1413 this.cost = new Money(); 1414 return this.cost; 1415 } else 1416 return super.addChild(name); 1417 } 1418 1419 public MedicationKnowledgeCostComponent copy() { 1420 MedicationKnowledgeCostComponent dst = new MedicationKnowledgeCostComponent(); 1421 copyValues(dst); 1422 return dst; 1423 } 1424 1425 public void copyValues(MedicationKnowledgeCostComponent dst) { 1426 super.copyValues(dst); 1427 dst.type = type == null ? null : type.copy(); 1428 dst.source = source == null ? null : source.copy(); 1429 dst.cost = cost == null ? null : cost.copy(); 1430 } 1431 1432 @Override 1433 public boolean equalsDeep(Base other_) { 1434 if (!super.equalsDeep(other_)) 1435 return false; 1436 if (!(other_ instanceof MedicationKnowledgeCostComponent)) 1437 return false; 1438 MedicationKnowledgeCostComponent o = (MedicationKnowledgeCostComponent) other_; 1439 return compareDeep(type, o.type, true) && compareDeep(source, o.source, true) && compareDeep(cost, o.cost, true); 1440 } 1441 1442 @Override 1443 public boolean equalsShallow(Base other_) { 1444 if (!super.equalsShallow(other_)) 1445 return false; 1446 if (!(other_ instanceof MedicationKnowledgeCostComponent)) 1447 return false; 1448 MedicationKnowledgeCostComponent o = (MedicationKnowledgeCostComponent) other_; 1449 return compareValues(source, o.source, true); 1450 } 1451 1452 public boolean isEmpty() { 1453 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, source, cost); 1454 } 1455 1456 public String fhirType() { 1457 return "MedicationKnowledge.cost"; 1458 1459 } 1460 1461 } 1462 1463 @Block() 1464 public static class MedicationKnowledgeMonitoringProgramComponent extends BackboneElement 1465 implements IBaseBackboneElement { 1466 /** 1467 * Type of program under which the medication is monitored. 1468 */ 1469 @Child(name = "type", type = { 1470 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1471 @Description(shortDefinition = "Type of program under which the medication is monitored", formalDefinition = "Type of program under which the medication is monitored.") 1472 protected CodeableConcept type; 1473 1474 /** 1475 * Name of the reviewing program. 1476 */ 1477 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1478 @Description(shortDefinition = "Name of the reviewing program", formalDefinition = "Name of the reviewing program.") 1479 protected StringType name; 1480 1481 private static final long serialVersionUID = -280346281L; 1482 1483 /** 1484 * Constructor 1485 */ 1486 public MedicationKnowledgeMonitoringProgramComponent() { 1487 super(); 1488 } 1489 1490 /** 1491 * @return {@link #type} (Type of program under which the medication is 1492 * monitored.) 1493 */ 1494 public CodeableConcept getType() { 1495 if (this.type == null) 1496 if (Configuration.errorOnAutoCreate()) 1497 throw new Error("Attempt to auto-create MedicationKnowledgeMonitoringProgramComponent.type"); 1498 else if (Configuration.doAutoCreate()) 1499 this.type = new CodeableConcept(); // cc 1500 return this.type; 1501 } 1502 1503 public boolean hasType() { 1504 return this.type != null && !this.type.isEmpty(); 1505 } 1506 1507 /** 1508 * @param value {@link #type} (Type of program under which the medication is 1509 * monitored.) 1510 */ 1511 public MedicationKnowledgeMonitoringProgramComponent setType(CodeableConcept value) { 1512 this.type = value; 1513 return this; 1514 } 1515 1516 /** 1517 * @return {@link #name} (Name of the reviewing program.). This is the 1518 * underlying object with id, value and extensions. The accessor 1519 * "getName" gives direct access to the value 1520 */ 1521 public StringType getNameElement() { 1522 if (this.name == null) 1523 if (Configuration.errorOnAutoCreate()) 1524 throw new Error("Attempt to auto-create MedicationKnowledgeMonitoringProgramComponent.name"); 1525 else if (Configuration.doAutoCreate()) 1526 this.name = new StringType(); // bb 1527 return this.name; 1528 } 1529 1530 public boolean hasNameElement() { 1531 return this.name != null && !this.name.isEmpty(); 1532 } 1533 1534 public boolean hasName() { 1535 return this.name != null && !this.name.isEmpty(); 1536 } 1537 1538 /** 1539 * @param value {@link #name} (Name of the reviewing program.). This is the 1540 * underlying object with id, value and extensions. The accessor 1541 * "getName" gives direct access to the value 1542 */ 1543 public MedicationKnowledgeMonitoringProgramComponent setNameElement(StringType value) { 1544 this.name = value; 1545 return this; 1546 } 1547 1548 /** 1549 * @return Name of the reviewing program. 1550 */ 1551 public String getName() { 1552 return this.name == null ? null : this.name.getValue(); 1553 } 1554 1555 /** 1556 * @param value Name of the reviewing program. 1557 */ 1558 public MedicationKnowledgeMonitoringProgramComponent setName(String value) { 1559 if (Utilities.noString(value)) 1560 this.name = null; 1561 else { 1562 if (this.name == null) 1563 this.name = new StringType(); 1564 this.name.setValue(value); 1565 } 1566 return this; 1567 } 1568 1569 protected void listChildren(List<Property> children) { 1570 super.listChildren(children); 1571 children.add(new Property("type", "CodeableConcept", "Type of program under which the medication is monitored.", 1572 0, 1, type)); 1573 children.add(new Property("name", "string", "Name of the reviewing program.", 0, 1, name)); 1574 } 1575 1576 @Override 1577 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1578 switch (_hash) { 1579 case 3575610: 1580 /* type */ return new Property("type", "CodeableConcept", 1581 "Type of program under which the medication is monitored.", 0, 1, type); 1582 case 3373707: 1583 /* name */ return new Property("name", "string", "Name of the reviewing program.", 0, 1, name); 1584 default: 1585 return super.getNamedProperty(_hash, _name, _checkValid); 1586 } 1587 1588 } 1589 1590 @Override 1591 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1592 switch (hash) { 1593 case 3575610: 1594 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1595 case 3373707: 1596 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1597 default: 1598 return super.getProperty(hash, name, checkValid); 1599 } 1600 1601 } 1602 1603 @Override 1604 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1605 switch (hash) { 1606 case 3575610: // type 1607 this.type = castToCodeableConcept(value); // CodeableConcept 1608 return value; 1609 case 3373707: // name 1610 this.name = castToString(value); // StringType 1611 return value; 1612 default: 1613 return super.setProperty(hash, name, value); 1614 } 1615 1616 } 1617 1618 @Override 1619 public Base setProperty(String name, Base value) throws FHIRException { 1620 if (name.equals("type")) { 1621 this.type = castToCodeableConcept(value); // CodeableConcept 1622 } else if (name.equals("name")) { 1623 this.name = castToString(value); // StringType 1624 } else 1625 return super.setProperty(name, value); 1626 return value; 1627 } 1628 1629 @Override 1630 public void removeChild(String name, Base value) throws FHIRException { 1631 if (name.equals("type")) { 1632 this.type = null; 1633 } else if (name.equals("name")) { 1634 this.name = null; 1635 } else 1636 super.removeChild(name, value); 1637 1638 } 1639 1640 @Override 1641 public Base makeProperty(int hash, String name) throws FHIRException { 1642 switch (hash) { 1643 case 3575610: 1644 return getType(); 1645 case 3373707: 1646 return getNameElement(); 1647 default: 1648 return super.makeProperty(hash, name); 1649 } 1650 1651 } 1652 1653 @Override 1654 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1655 switch (hash) { 1656 case 3575610: 1657 /* type */ return new String[] { "CodeableConcept" }; 1658 case 3373707: 1659 /* name */ return new String[] { "string" }; 1660 default: 1661 return super.getTypesForProperty(hash, name); 1662 } 1663 1664 } 1665 1666 @Override 1667 public Base addChild(String name) throws FHIRException { 1668 if (name.equals("type")) { 1669 this.type = new CodeableConcept(); 1670 return this.type; 1671 } else if (name.equals("name")) { 1672 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.name"); 1673 } else 1674 return super.addChild(name); 1675 } 1676 1677 public MedicationKnowledgeMonitoringProgramComponent copy() { 1678 MedicationKnowledgeMonitoringProgramComponent dst = new MedicationKnowledgeMonitoringProgramComponent(); 1679 copyValues(dst); 1680 return dst; 1681 } 1682 1683 public void copyValues(MedicationKnowledgeMonitoringProgramComponent dst) { 1684 super.copyValues(dst); 1685 dst.type = type == null ? null : type.copy(); 1686 dst.name = name == null ? null : name.copy(); 1687 } 1688 1689 @Override 1690 public boolean equalsDeep(Base other_) { 1691 if (!super.equalsDeep(other_)) 1692 return false; 1693 if (!(other_ instanceof MedicationKnowledgeMonitoringProgramComponent)) 1694 return false; 1695 MedicationKnowledgeMonitoringProgramComponent o = (MedicationKnowledgeMonitoringProgramComponent) other_; 1696 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true); 1697 } 1698 1699 @Override 1700 public boolean equalsShallow(Base other_) { 1701 if (!super.equalsShallow(other_)) 1702 return false; 1703 if (!(other_ instanceof MedicationKnowledgeMonitoringProgramComponent)) 1704 return false; 1705 MedicationKnowledgeMonitoringProgramComponent o = (MedicationKnowledgeMonitoringProgramComponent) other_; 1706 return compareValues(name, o.name, true); 1707 } 1708 1709 public boolean isEmpty() { 1710 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name); 1711 } 1712 1713 public String fhirType() { 1714 return "MedicationKnowledge.monitoringProgram"; 1715 1716 } 1717 1718 } 1719 1720 @Block() 1721 public static class MedicationKnowledgeAdministrationGuidelinesComponent extends BackboneElement 1722 implements IBaseBackboneElement { 1723 /** 1724 * Dosage for the medication for the specific guidelines. 1725 */ 1726 @Child(name = "dosage", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1727 @Description(shortDefinition = "Dosage for the medication for the specific guidelines", formalDefinition = "Dosage for the medication for the specific guidelines.") 1728 protected List<MedicationKnowledgeAdministrationGuidelinesDosageComponent> dosage; 1729 1730 /** 1731 * Indication for use that apply to the specific administration guidelines. 1732 */ 1733 @Child(name = "indication", type = { CodeableConcept.class, 1734 ObservationDefinition.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1735 @Description(shortDefinition = "Indication for use that apply to the specific administration guidelines", formalDefinition = "Indication for use that apply to the specific administration guidelines.") 1736 protected Type indication; 1737 1738 /** 1739 * Characteristics of the patient that are relevant to the administration 1740 * guidelines (for example, height, weight, gender, etc.). 1741 */ 1742 @Child(name = "patientCharacteristics", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1743 @Description(shortDefinition = "Characteristics of the patient that are relevant to the administration guidelines", formalDefinition = "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).") 1744 protected List<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent> patientCharacteristics; 1745 1746 private static final long serialVersionUID = 1196999266L; 1747 1748 /** 1749 * Constructor 1750 */ 1751 public MedicationKnowledgeAdministrationGuidelinesComponent() { 1752 super(); 1753 } 1754 1755 /** 1756 * @return {@link #dosage} (Dosage for the medication for the specific 1757 * guidelines.) 1758 */ 1759 public List<MedicationKnowledgeAdministrationGuidelinesDosageComponent> getDosage() { 1760 if (this.dosage == null) 1761 this.dosage = new ArrayList<MedicationKnowledgeAdministrationGuidelinesDosageComponent>(); 1762 return this.dosage; 1763 } 1764 1765 /** 1766 * @return Returns a reference to <code>this</code> for easy method chaining 1767 */ 1768 public MedicationKnowledgeAdministrationGuidelinesComponent setDosage( 1769 List<MedicationKnowledgeAdministrationGuidelinesDosageComponent> theDosage) { 1770 this.dosage = theDosage; 1771 return this; 1772 } 1773 1774 public boolean hasDosage() { 1775 if (this.dosage == null) 1776 return false; 1777 for (MedicationKnowledgeAdministrationGuidelinesDosageComponent item : this.dosage) 1778 if (!item.isEmpty()) 1779 return true; 1780 return false; 1781 } 1782 1783 public MedicationKnowledgeAdministrationGuidelinesDosageComponent addDosage() { // 3 1784 MedicationKnowledgeAdministrationGuidelinesDosageComponent t = new MedicationKnowledgeAdministrationGuidelinesDosageComponent(); 1785 if (this.dosage == null) 1786 this.dosage = new ArrayList<MedicationKnowledgeAdministrationGuidelinesDosageComponent>(); 1787 this.dosage.add(t); 1788 return t; 1789 } 1790 1791 public MedicationKnowledgeAdministrationGuidelinesComponent addDosage( 1792 MedicationKnowledgeAdministrationGuidelinesDosageComponent t) { // 3 1793 if (t == null) 1794 return this; 1795 if (this.dosage == null) 1796 this.dosage = new ArrayList<MedicationKnowledgeAdministrationGuidelinesDosageComponent>(); 1797 this.dosage.add(t); 1798 return this; 1799 } 1800 1801 /** 1802 * @return The first repetition of repeating field {@link #dosage}, creating it 1803 * if it does not already exist 1804 */ 1805 public MedicationKnowledgeAdministrationGuidelinesDosageComponent getDosageFirstRep() { 1806 if (getDosage().isEmpty()) { 1807 addDosage(); 1808 } 1809 return getDosage().get(0); 1810 } 1811 1812 /** 1813 * @return {@link #indication} (Indication for use that apply to the specific 1814 * administration guidelines.) 1815 */ 1816 public Type getIndication() { 1817 return this.indication; 1818 } 1819 1820 /** 1821 * @return {@link #indication} (Indication for use that apply to the specific 1822 * administration guidelines.) 1823 */ 1824 public CodeableConcept getIndicationCodeableConcept() throws FHIRException { 1825 if (this.indication == null) 1826 this.indication = new CodeableConcept(); 1827 if (!(this.indication instanceof CodeableConcept)) 1828 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1829 + this.indication.getClass().getName() + " was encountered"); 1830 return (CodeableConcept) this.indication; 1831 } 1832 1833 public boolean hasIndicationCodeableConcept() { 1834 return this != null && this.indication instanceof CodeableConcept; 1835 } 1836 1837 /** 1838 * @return {@link #indication} (Indication for use that apply to the specific 1839 * administration guidelines.) 1840 */ 1841 public Reference getIndicationReference() throws FHIRException { 1842 if (this.indication == null) 1843 this.indication = new Reference(); 1844 if (!(this.indication instanceof Reference)) 1845 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1846 + this.indication.getClass().getName() + " was encountered"); 1847 return (Reference) this.indication; 1848 } 1849 1850 public boolean hasIndicationReference() { 1851 return this != null && this.indication instanceof Reference; 1852 } 1853 1854 public boolean hasIndication() { 1855 return this.indication != null && !this.indication.isEmpty(); 1856 } 1857 1858 /** 1859 * @param value {@link #indication} (Indication for use that apply to the 1860 * specific administration guidelines.) 1861 */ 1862 public MedicationKnowledgeAdministrationGuidelinesComponent setIndication(Type value) { 1863 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1864 throw new Error( 1865 "Not the right type for MedicationKnowledge.administrationGuidelines.indication[x]: " + value.fhirType()); 1866 this.indication = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return {@link #patientCharacteristics} (Characteristics of the patient that 1872 * are relevant to the administration guidelines (for example, height, 1873 * weight, gender, etc.).) 1874 */ 1875 public List<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent> getPatientCharacteristics() { 1876 if (this.patientCharacteristics == null) 1877 this.patientCharacteristics = new ArrayList<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent>(); 1878 return this.patientCharacteristics; 1879 } 1880 1881 /** 1882 * @return Returns a reference to <code>this</code> for easy method chaining 1883 */ 1884 public MedicationKnowledgeAdministrationGuidelinesComponent setPatientCharacteristics( 1885 List<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent> thePatientCharacteristics) { 1886 this.patientCharacteristics = thePatientCharacteristics; 1887 return this; 1888 } 1889 1890 public boolean hasPatientCharacteristics() { 1891 if (this.patientCharacteristics == null) 1892 return false; 1893 for (MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent item : this.patientCharacteristics) 1894 if (!item.isEmpty()) 1895 return true; 1896 return false; 1897 } 1898 1899 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent addPatientCharacteristics() { // 3 1900 MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent t = new MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent(); 1901 if (this.patientCharacteristics == null) 1902 this.patientCharacteristics = new ArrayList<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent>(); 1903 this.patientCharacteristics.add(t); 1904 return t; 1905 } 1906 1907 public MedicationKnowledgeAdministrationGuidelinesComponent addPatientCharacteristics( 1908 MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent t) { // 3 1909 if (t == null) 1910 return this; 1911 if (this.patientCharacteristics == null) 1912 this.patientCharacteristics = new ArrayList<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent>(); 1913 this.patientCharacteristics.add(t); 1914 return this; 1915 } 1916 1917 /** 1918 * @return The first repetition of repeating field 1919 * {@link #patientCharacteristics}, creating it if it does not already 1920 * exist 1921 */ 1922 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent getPatientCharacteristicsFirstRep() { 1923 if (getPatientCharacteristics().isEmpty()) { 1924 addPatientCharacteristics(); 1925 } 1926 return getPatientCharacteristics().get(0); 1927 } 1928 1929 protected void listChildren(List<Property> children) { 1930 super.listChildren(children); 1931 children.add(new Property("dosage", "", "Dosage for the medication for the specific guidelines.", 0, 1932 java.lang.Integer.MAX_VALUE, dosage)); 1933 children.add(new Property("indication[x]", "CodeableConcept|Reference(ObservationDefinition)", 1934 "Indication for use that apply to the specific administration guidelines.", 0, 1, indication)); 1935 children.add(new Property("patientCharacteristics", "", 1936 "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).", 1937 0, java.lang.Integer.MAX_VALUE, patientCharacteristics)); 1938 } 1939 1940 @Override 1941 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1942 switch (_hash) { 1943 case -1326018889: 1944 /* dosage */ return new Property("dosage", "", "Dosage for the medication for the specific guidelines.", 0, 1945 java.lang.Integer.MAX_VALUE, dosage); 1946 case -501208668: 1947 /* indication[x] */ return new Property("indication[x]", "CodeableConcept|Reference(ObservationDefinition)", 1948 "Indication for use that apply to the specific administration guidelines.", 0, 1, indication); 1949 case -597168804: 1950 /* indication */ return new Property("indication[x]", "CodeableConcept|Reference(ObservationDefinition)", 1951 "Indication for use that apply to the specific administration guidelines.", 0, 1, indication); 1952 case -1094003035: 1953 /* indicationCodeableConcept */ return new Property("indication[x]", 1954 "CodeableConcept|Reference(ObservationDefinition)", 1955 "Indication for use that apply to the specific administration guidelines.", 0, 1, indication); 1956 case 803518799: 1957 /* indicationReference */ return new Property("indication[x]", 1958 "CodeableConcept|Reference(ObservationDefinition)", 1959 "Indication for use that apply to the specific administration guidelines.", 0, 1, indication); 1960 case -960531341: 1961 /* patientCharacteristics */ return new Property("patientCharacteristics", "", 1962 "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).", 1963 0, java.lang.Integer.MAX_VALUE, patientCharacteristics); 1964 default: 1965 return super.getNamedProperty(_hash, _name, _checkValid); 1966 } 1967 1968 } 1969 1970 @Override 1971 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1972 switch (hash) { 1973 case -1326018889: 1974 /* dosage */ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // MedicationKnowledgeAdministrationGuidelinesDosageComponent 1975 case -597168804: 1976 /* indication */ return this.indication == null ? new Base[0] : new Base[] { this.indication }; // Type 1977 case -960531341: 1978 /* patientCharacteristics */ return this.patientCharacteristics == null ? new Base[0] 1979 : this.patientCharacteristics.toArray(new Base[this.patientCharacteristics.size()]); // MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent 1980 default: 1981 return super.getProperty(hash, name, checkValid); 1982 } 1983 1984 } 1985 1986 @Override 1987 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1988 switch (hash) { 1989 case -1326018889: // dosage 1990 this.getDosage().add((MedicationKnowledgeAdministrationGuidelinesDosageComponent) value); // MedicationKnowledgeAdministrationGuidelinesDosageComponent 1991 return value; 1992 case -597168804: // indication 1993 this.indication = castToType(value); // Type 1994 return value; 1995 case -960531341: // patientCharacteristics 1996 this.getPatientCharacteristics() 1997 .add((MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent) value); // MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent 1998 return value; 1999 default: 2000 return super.setProperty(hash, name, value); 2001 } 2002 2003 } 2004 2005 @Override 2006 public Base setProperty(String name, Base value) throws FHIRException { 2007 if (name.equals("dosage")) { 2008 this.getDosage().add((MedicationKnowledgeAdministrationGuidelinesDosageComponent) value); 2009 } else if (name.equals("indication[x]")) { 2010 this.indication = castToType(value); // Type 2011 } else if (name.equals("patientCharacteristics")) { 2012 this.getPatientCharacteristics() 2013 .add((MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent) value); 2014 } else 2015 return super.setProperty(name, value); 2016 return value; 2017 } 2018 2019 @Override 2020 public void removeChild(String name, Base value) throws FHIRException { 2021 if (name.equals("dosage")) { 2022 this.getDosage().remove((MedicationKnowledgeAdministrationGuidelinesDosageComponent) value); 2023 } else if (name.equals("indication[x]")) { 2024 this.indication = null; 2025 } else if (name.equals("patientCharacteristics")) { 2026 this.getPatientCharacteristics() 2027 .remove((MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent) value); 2028 } else 2029 super.removeChild(name, value); 2030 2031 } 2032 2033 @Override 2034 public Base makeProperty(int hash, String name) throws FHIRException { 2035 switch (hash) { 2036 case -1326018889: 2037 return addDosage(); 2038 case -501208668: 2039 return getIndication(); 2040 case -597168804: 2041 return getIndication(); 2042 case -960531341: 2043 return addPatientCharacteristics(); 2044 default: 2045 return super.makeProperty(hash, name); 2046 } 2047 2048 } 2049 2050 @Override 2051 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2052 switch (hash) { 2053 case -1326018889: 2054 /* dosage */ return new String[] {}; 2055 case -597168804: 2056 /* indication */ return new String[] { "CodeableConcept", "Reference" }; 2057 case -960531341: 2058 /* patientCharacteristics */ return new String[] {}; 2059 default: 2060 return super.getTypesForProperty(hash, name); 2061 } 2062 2063 } 2064 2065 @Override 2066 public Base addChild(String name) throws FHIRException { 2067 if (name.equals("dosage")) { 2068 return addDosage(); 2069 } else if (name.equals("indicationCodeableConcept")) { 2070 this.indication = new CodeableConcept(); 2071 return this.indication; 2072 } else if (name.equals("indicationReference")) { 2073 this.indication = new Reference(); 2074 return this.indication; 2075 } else if (name.equals("patientCharacteristics")) { 2076 return addPatientCharacteristics(); 2077 } else 2078 return super.addChild(name); 2079 } 2080 2081 public MedicationKnowledgeAdministrationGuidelinesComponent copy() { 2082 MedicationKnowledgeAdministrationGuidelinesComponent dst = new MedicationKnowledgeAdministrationGuidelinesComponent(); 2083 copyValues(dst); 2084 return dst; 2085 } 2086 2087 public void copyValues(MedicationKnowledgeAdministrationGuidelinesComponent dst) { 2088 super.copyValues(dst); 2089 if (dosage != null) { 2090 dst.dosage = new ArrayList<MedicationKnowledgeAdministrationGuidelinesDosageComponent>(); 2091 for (MedicationKnowledgeAdministrationGuidelinesDosageComponent i : dosage) 2092 dst.dosage.add(i.copy()); 2093 } 2094 ; 2095 dst.indication = indication == null ? null : indication.copy(); 2096 if (patientCharacteristics != null) { 2097 dst.patientCharacteristics = new ArrayList<MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent>(); 2098 for (MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent i : patientCharacteristics) 2099 dst.patientCharacteristics.add(i.copy()); 2100 } 2101 ; 2102 } 2103 2104 @Override 2105 public boolean equalsDeep(Base other_) { 2106 if (!super.equalsDeep(other_)) 2107 return false; 2108 if (!(other_ instanceof MedicationKnowledgeAdministrationGuidelinesComponent)) 2109 return false; 2110 MedicationKnowledgeAdministrationGuidelinesComponent o = (MedicationKnowledgeAdministrationGuidelinesComponent) other_; 2111 return compareDeep(dosage, o.dosage, true) && compareDeep(indication, o.indication, true) 2112 && compareDeep(patientCharacteristics, o.patientCharacteristics, true); 2113 } 2114 2115 @Override 2116 public boolean equalsShallow(Base other_) { 2117 if (!super.equalsShallow(other_)) 2118 return false; 2119 if (!(other_ instanceof MedicationKnowledgeAdministrationGuidelinesComponent)) 2120 return false; 2121 MedicationKnowledgeAdministrationGuidelinesComponent o = (MedicationKnowledgeAdministrationGuidelinesComponent) other_; 2122 return true; 2123 } 2124 2125 public boolean isEmpty() { 2126 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(dosage, indication, patientCharacteristics); 2127 } 2128 2129 public String fhirType() { 2130 return "MedicationKnowledge.administrationGuidelines"; 2131 2132 } 2133 2134 } 2135 2136 @Block() 2137 public static class MedicationKnowledgeAdministrationGuidelinesDosageComponent extends BackboneElement 2138 implements IBaseBackboneElement { 2139 /** 2140 * The type of dosage (for example, prophylaxis, maintenance, therapeutic, 2141 * etc.). 2142 */ 2143 @Child(name = "type", type = { 2144 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2145 @Description(shortDefinition = "Type of dosage", formalDefinition = "The type of dosage (for example, prophylaxis, maintenance, therapeutic, etc.).") 2146 protected CodeableConcept type; 2147 2148 /** 2149 * Dosage for the medication for the specific guidelines. 2150 */ 2151 @Child(name = "dosage", type = { 2152 Dosage.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2153 @Description(shortDefinition = "Dosage for the medication for the specific guidelines", formalDefinition = "Dosage for the medication for the specific guidelines.") 2154 protected List<Dosage> dosage; 2155 2156 private static final long serialVersionUID = 1578257961L; 2157 2158 /** 2159 * Constructor 2160 */ 2161 public MedicationKnowledgeAdministrationGuidelinesDosageComponent() { 2162 super(); 2163 } 2164 2165 /** 2166 * Constructor 2167 */ 2168 public MedicationKnowledgeAdministrationGuidelinesDosageComponent(CodeableConcept type) { 2169 super(); 2170 this.type = type; 2171 } 2172 2173 /** 2174 * @return {@link #type} (The type of dosage (for example, prophylaxis, 2175 * maintenance, therapeutic, etc.).) 2176 */ 2177 public CodeableConcept getType() { 2178 if (this.type == null) 2179 if (Configuration.errorOnAutoCreate()) 2180 throw new Error("Attempt to auto-create MedicationKnowledgeAdministrationGuidelinesDosageComponent.type"); 2181 else if (Configuration.doAutoCreate()) 2182 this.type = new CodeableConcept(); // cc 2183 return this.type; 2184 } 2185 2186 public boolean hasType() { 2187 return this.type != null && !this.type.isEmpty(); 2188 } 2189 2190 /** 2191 * @param value {@link #type} (The type of dosage (for example, prophylaxis, 2192 * maintenance, therapeutic, etc.).) 2193 */ 2194 public MedicationKnowledgeAdministrationGuidelinesDosageComponent setType(CodeableConcept value) { 2195 this.type = value; 2196 return this; 2197 } 2198 2199 /** 2200 * @return {@link #dosage} (Dosage for the medication for the specific 2201 * guidelines.) 2202 */ 2203 public List<Dosage> getDosage() { 2204 if (this.dosage == null) 2205 this.dosage = new ArrayList<Dosage>(); 2206 return this.dosage; 2207 } 2208 2209 /** 2210 * @return Returns a reference to <code>this</code> for easy method chaining 2211 */ 2212 public MedicationKnowledgeAdministrationGuidelinesDosageComponent setDosage(List<Dosage> theDosage) { 2213 this.dosage = theDosage; 2214 return this; 2215 } 2216 2217 public boolean hasDosage() { 2218 if (this.dosage == null) 2219 return false; 2220 for (Dosage item : this.dosage) 2221 if (!item.isEmpty()) 2222 return true; 2223 return false; 2224 } 2225 2226 public Dosage addDosage() { // 3 2227 Dosage t = new Dosage(); 2228 if (this.dosage == null) 2229 this.dosage = new ArrayList<Dosage>(); 2230 this.dosage.add(t); 2231 return t; 2232 } 2233 2234 public MedicationKnowledgeAdministrationGuidelinesDosageComponent addDosage(Dosage t) { // 3 2235 if (t == null) 2236 return this; 2237 if (this.dosage == null) 2238 this.dosage = new ArrayList<Dosage>(); 2239 this.dosage.add(t); 2240 return this; 2241 } 2242 2243 /** 2244 * @return The first repetition of repeating field {@link #dosage}, creating it 2245 * if it does not already exist 2246 */ 2247 public Dosage getDosageFirstRep() { 2248 if (getDosage().isEmpty()) { 2249 addDosage(); 2250 } 2251 return getDosage().get(0); 2252 } 2253 2254 protected void listChildren(List<Property> children) { 2255 super.listChildren(children); 2256 children.add(new Property("type", "CodeableConcept", 2257 "The type of dosage (for example, prophylaxis, maintenance, therapeutic, etc.).", 0, 1, type)); 2258 children.add(new Property("dosage", "Dosage", "Dosage for the medication for the specific guidelines.", 0, 2259 java.lang.Integer.MAX_VALUE, dosage)); 2260 } 2261 2262 @Override 2263 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2264 switch (_hash) { 2265 case 3575610: 2266 /* type */ return new Property("type", "CodeableConcept", 2267 "The type of dosage (for example, prophylaxis, maintenance, therapeutic, etc.).", 0, 1, type); 2268 case -1326018889: 2269 /* dosage */ return new Property("dosage", "Dosage", "Dosage for the medication for the specific guidelines.", 2270 0, java.lang.Integer.MAX_VALUE, dosage); 2271 default: 2272 return super.getNamedProperty(_hash, _name, _checkValid); 2273 } 2274 2275 } 2276 2277 @Override 2278 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2279 switch (hash) { 2280 case 3575610: 2281 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2282 case -1326018889: 2283 /* dosage */ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 2284 default: 2285 return super.getProperty(hash, name, checkValid); 2286 } 2287 2288 } 2289 2290 @Override 2291 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2292 switch (hash) { 2293 case 3575610: // type 2294 this.type = castToCodeableConcept(value); // CodeableConcept 2295 return value; 2296 case -1326018889: // dosage 2297 this.getDosage().add(castToDosage(value)); // Dosage 2298 return value; 2299 default: 2300 return super.setProperty(hash, name, value); 2301 } 2302 2303 } 2304 2305 @Override 2306 public Base setProperty(String name, Base value) throws FHIRException { 2307 if (name.equals("type")) { 2308 this.type = castToCodeableConcept(value); // CodeableConcept 2309 } else if (name.equals("dosage")) { 2310 this.getDosage().add(castToDosage(value)); 2311 } else 2312 return super.setProperty(name, value); 2313 return value; 2314 } 2315 2316 @Override 2317 public void removeChild(String name, Base value) throws FHIRException { 2318 if (name.equals("type")) { 2319 this.type = null; 2320 } else if (name.equals("dosage")) { 2321 this.getDosage().remove(castToDosage(value)); 2322 } else 2323 super.removeChild(name, value); 2324 2325 } 2326 2327 @Override 2328 public Base makeProperty(int hash, String name) throws FHIRException { 2329 switch (hash) { 2330 case 3575610: 2331 return getType(); 2332 case -1326018889: 2333 return addDosage(); 2334 default: 2335 return super.makeProperty(hash, name); 2336 } 2337 2338 } 2339 2340 @Override 2341 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2342 switch (hash) { 2343 case 3575610: 2344 /* type */ return new String[] { "CodeableConcept" }; 2345 case -1326018889: 2346 /* dosage */ return new String[] { "Dosage" }; 2347 default: 2348 return super.getTypesForProperty(hash, name); 2349 } 2350 2351 } 2352 2353 @Override 2354 public Base addChild(String name) throws FHIRException { 2355 if (name.equals("type")) { 2356 this.type = new CodeableConcept(); 2357 return this.type; 2358 } else if (name.equals("dosage")) { 2359 return addDosage(); 2360 } else 2361 return super.addChild(name); 2362 } 2363 2364 public MedicationKnowledgeAdministrationGuidelinesDosageComponent copy() { 2365 MedicationKnowledgeAdministrationGuidelinesDosageComponent dst = new MedicationKnowledgeAdministrationGuidelinesDosageComponent(); 2366 copyValues(dst); 2367 return dst; 2368 } 2369 2370 public void copyValues(MedicationKnowledgeAdministrationGuidelinesDosageComponent dst) { 2371 super.copyValues(dst); 2372 dst.type = type == null ? null : type.copy(); 2373 if (dosage != null) { 2374 dst.dosage = new ArrayList<Dosage>(); 2375 for (Dosage i : dosage) 2376 dst.dosage.add(i.copy()); 2377 } 2378 ; 2379 } 2380 2381 @Override 2382 public boolean equalsDeep(Base other_) { 2383 if (!super.equalsDeep(other_)) 2384 return false; 2385 if (!(other_ instanceof MedicationKnowledgeAdministrationGuidelinesDosageComponent)) 2386 return false; 2387 MedicationKnowledgeAdministrationGuidelinesDosageComponent o = (MedicationKnowledgeAdministrationGuidelinesDosageComponent) other_; 2388 return compareDeep(type, o.type, true) && compareDeep(dosage, o.dosage, true); 2389 } 2390 2391 @Override 2392 public boolean equalsShallow(Base other_) { 2393 if (!super.equalsShallow(other_)) 2394 return false; 2395 if (!(other_ instanceof MedicationKnowledgeAdministrationGuidelinesDosageComponent)) 2396 return false; 2397 MedicationKnowledgeAdministrationGuidelinesDosageComponent o = (MedicationKnowledgeAdministrationGuidelinesDosageComponent) other_; 2398 return true; 2399 } 2400 2401 public boolean isEmpty() { 2402 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, dosage); 2403 } 2404 2405 public String fhirType() { 2406 return "MedicationKnowledge.administrationGuidelines.dosage"; 2407 2408 } 2409 2410 } 2411 2412 @Block() 2413 public static class MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent extends BackboneElement 2414 implements IBaseBackboneElement { 2415 /** 2416 * Specific characteristic that is relevant to the administration guideline 2417 * (e.g. height, weight, gender). 2418 */ 2419 @Child(name = "characteristic", type = { CodeableConcept.class, 2420 Quantity.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2421 @Description(shortDefinition = "Specific characteristic that is relevant to the administration guideline", formalDefinition = "Specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).") 2422 protected Type characteristic; 2423 2424 /** 2425 * The specific characteristic (e.g. height, weight, gender, etc.). 2426 */ 2427 @Child(name = "value", type = { 2428 StringType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2429 @Description(shortDefinition = "The specific characteristic", formalDefinition = "The specific characteristic (e.g. height, weight, gender, etc.).") 2430 protected List<StringType> value; 2431 2432 private static final long serialVersionUID = -133608297L; 2433 2434 /** 2435 * Constructor 2436 */ 2437 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent() { 2438 super(); 2439 } 2440 2441 /** 2442 * Constructor 2443 */ 2444 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent(Type characteristic) { 2445 super(); 2446 this.characteristic = characteristic; 2447 } 2448 2449 /** 2450 * @return {@link #characteristic} (Specific characteristic that is relevant to 2451 * the administration guideline (e.g. height, weight, gender).) 2452 */ 2453 public Type getCharacteristic() { 2454 return this.characteristic; 2455 } 2456 2457 /** 2458 * @return {@link #characteristic} (Specific characteristic that is relevant to 2459 * the administration guideline (e.g. height, weight, gender).) 2460 */ 2461 public CodeableConcept getCharacteristicCodeableConcept() throws FHIRException { 2462 if (this.characteristic == null) 2463 this.characteristic = new CodeableConcept(); 2464 if (!(this.characteristic instanceof CodeableConcept)) 2465 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2466 + this.characteristic.getClass().getName() + " was encountered"); 2467 return (CodeableConcept) this.characteristic; 2468 } 2469 2470 public boolean hasCharacteristicCodeableConcept() { 2471 return this != null && this.characteristic instanceof CodeableConcept; 2472 } 2473 2474 /** 2475 * @return {@link #characteristic} (Specific characteristic that is relevant to 2476 * the administration guideline (e.g. height, weight, gender).) 2477 */ 2478 public Quantity getCharacteristicQuantity() throws FHIRException { 2479 if (this.characteristic == null) 2480 this.characteristic = new Quantity(); 2481 if (!(this.characteristic instanceof Quantity)) 2482 throw new FHIRException("Type mismatch: the type Quantity was expected, but " 2483 + this.characteristic.getClass().getName() + " was encountered"); 2484 return (Quantity) this.characteristic; 2485 } 2486 2487 public boolean hasCharacteristicQuantity() { 2488 return this != null && this.characteristic instanceof Quantity; 2489 } 2490 2491 public boolean hasCharacteristic() { 2492 return this.characteristic != null && !this.characteristic.isEmpty(); 2493 } 2494 2495 /** 2496 * @param value {@link #characteristic} (Specific characteristic that is 2497 * relevant to the administration guideline (e.g. height, weight, 2498 * gender).) 2499 */ 2500 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent setCharacteristic(Type value) { 2501 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity)) 2502 throw new Error( 2503 "Not the right type for MedicationKnowledge.administrationGuidelines.patientCharacteristics.characteristic[x]: " 2504 + value.fhirType()); 2505 this.characteristic = value; 2506 return this; 2507 } 2508 2509 /** 2510 * @return {@link #value} (The specific characteristic (e.g. height, weight, 2511 * gender, etc.).) 2512 */ 2513 public List<StringType> getValue() { 2514 if (this.value == null) 2515 this.value = new ArrayList<StringType>(); 2516 return this.value; 2517 } 2518 2519 /** 2520 * @return Returns a reference to <code>this</code> for easy method chaining 2521 */ 2522 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent setValue( 2523 List<StringType> theValue) { 2524 this.value = theValue; 2525 return this; 2526 } 2527 2528 public boolean hasValue() { 2529 if (this.value == null) 2530 return false; 2531 for (StringType item : this.value) 2532 if (!item.isEmpty()) 2533 return true; 2534 return false; 2535 } 2536 2537 /** 2538 * @return {@link #value} (The specific characteristic (e.g. height, weight, 2539 * gender, etc.).) 2540 */ 2541 public StringType addValueElement() {// 2 2542 StringType t = new StringType(); 2543 if (this.value == null) 2544 this.value = new ArrayList<StringType>(); 2545 this.value.add(t); 2546 return t; 2547 } 2548 2549 /** 2550 * @param value {@link #value} (The specific characteristic (e.g. height, 2551 * weight, gender, etc.).) 2552 */ 2553 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent addValue(String value) { // 1 2554 StringType t = new StringType(); 2555 t.setValue(value); 2556 if (this.value == null) 2557 this.value = new ArrayList<StringType>(); 2558 this.value.add(t); 2559 return this; 2560 } 2561 2562 /** 2563 * @param value {@link #value} (The specific characteristic (e.g. height, 2564 * weight, gender, etc.).) 2565 */ 2566 public boolean hasValue(String value) { 2567 if (this.value == null) 2568 return false; 2569 for (StringType v : this.value) 2570 if (v.getValue().equals(value)) // string 2571 return true; 2572 return false; 2573 } 2574 2575 protected void listChildren(List<Property> children) { 2576 super.listChildren(children); 2577 children.add(new Property("characteristic[x]", "CodeableConcept|SimpleQuantity", 2578 "Specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 0, 2579 1, characteristic)); 2580 children.add(new Property("value", "string", "The specific characteristic (e.g. height, weight, gender, etc.).", 2581 0, java.lang.Integer.MAX_VALUE, value)); 2582 } 2583 2584 @Override 2585 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2586 switch (_hash) { 2587 case -654919419: 2588 /* characteristic[x] */ return new Property("characteristic[x]", "CodeableConcept|SimpleQuantity", 2589 "Specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 2590 0, 1, characteristic); 2591 case 366313883: 2592 /* characteristic */ return new Property("characteristic[x]", "CodeableConcept|SimpleQuantity", 2593 "Specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 2594 0, 1, characteristic); 2595 case -1259840378: 2596 /* characteristicCodeableConcept */ return new Property("characteristic[x]", "CodeableConcept|SimpleQuantity", 2597 "Specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 2598 0, 1, characteristic); 2599 case 1769373510: 2600 /* characteristicQuantity */ return new Property("characteristic[x]", "CodeableConcept|SimpleQuantity", 2601 "Specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 2602 0, 1, characteristic); 2603 case 111972721: 2604 /* value */ return new Property("value", "string", 2605 "The specific characteristic (e.g. height, weight, gender, etc.).", 0, java.lang.Integer.MAX_VALUE, value); 2606 default: 2607 return super.getNamedProperty(_hash, _name, _checkValid); 2608 } 2609 2610 } 2611 2612 @Override 2613 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2614 switch (hash) { 2615 case 366313883: 2616 /* characteristic */ return this.characteristic == null ? new Base[0] : new Base[] { this.characteristic }; // Type 2617 case 111972721: 2618 /* value */ return this.value == null ? new Base[0] : this.value.toArray(new Base[this.value.size()]); // StringType 2619 default: 2620 return super.getProperty(hash, name, checkValid); 2621 } 2622 2623 } 2624 2625 @Override 2626 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2627 switch (hash) { 2628 case 366313883: // characteristic 2629 this.characteristic = castToType(value); // Type 2630 return value; 2631 case 111972721: // value 2632 this.getValue().add(castToString(value)); // StringType 2633 return value; 2634 default: 2635 return super.setProperty(hash, name, value); 2636 } 2637 2638 } 2639 2640 @Override 2641 public Base setProperty(String name, Base value) throws FHIRException { 2642 if (name.equals("characteristic[x]")) { 2643 this.characteristic = castToType(value); // Type 2644 } else if (name.equals("value")) { 2645 this.getValue().add(castToString(value)); 2646 } else 2647 return super.setProperty(name, value); 2648 return value; 2649 } 2650 2651 @Override 2652 public void removeChild(String name, Base value) throws FHIRException { 2653 if (name.equals("characteristic[x]")) { 2654 this.characteristic = null; 2655 } else if (name.equals("value")) { 2656 this.getValue().remove(castToString(value)); 2657 } else 2658 super.removeChild(name, value); 2659 2660 } 2661 2662 @Override 2663 public Base makeProperty(int hash, String name) throws FHIRException { 2664 switch (hash) { 2665 case -654919419: 2666 return getCharacteristic(); 2667 case 366313883: 2668 return getCharacteristic(); 2669 case 111972721: 2670 return addValueElement(); 2671 default: 2672 return super.makeProperty(hash, name); 2673 } 2674 2675 } 2676 2677 @Override 2678 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2679 switch (hash) { 2680 case 366313883: 2681 /* characteristic */ return new String[] { "CodeableConcept", "SimpleQuantity" }; 2682 case 111972721: 2683 /* value */ return new String[] { "string" }; 2684 default: 2685 return super.getTypesForProperty(hash, name); 2686 } 2687 2688 } 2689 2690 @Override 2691 public Base addChild(String name) throws FHIRException { 2692 if (name.equals("characteristicCodeableConcept")) { 2693 this.characteristic = new CodeableConcept(); 2694 return this.characteristic; 2695 } else if (name.equals("characteristicQuantity")) { 2696 this.characteristic = new Quantity(); 2697 return this.characteristic; 2698 } else if (name.equals("value")) { 2699 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.value"); 2700 } else 2701 return super.addChild(name); 2702 } 2703 2704 public MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent copy() { 2705 MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent dst = new MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent(); 2706 copyValues(dst); 2707 return dst; 2708 } 2709 2710 public void copyValues(MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent dst) { 2711 super.copyValues(dst); 2712 dst.characteristic = characteristic == null ? null : characteristic.copy(); 2713 if (value != null) { 2714 dst.value = new ArrayList<StringType>(); 2715 for (StringType i : value) 2716 dst.value.add(i.copy()); 2717 } 2718 ; 2719 } 2720 2721 @Override 2722 public boolean equalsDeep(Base other_) { 2723 if (!super.equalsDeep(other_)) 2724 return false; 2725 if (!(other_ instanceof MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent)) 2726 return false; 2727 MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent o = (MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent) other_; 2728 return compareDeep(characteristic, o.characteristic, true) && compareDeep(value, o.value, true); 2729 } 2730 2731 @Override 2732 public boolean equalsShallow(Base other_) { 2733 if (!super.equalsShallow(other_)) 2734 return false; 2735 if (!(other_ instanceof MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent)) 2736 return false; 2737 MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent o = (MedicationKnowledgeAdministrationGuidelinesPatientCharacteristicsComponent) other_; 2738 return compareValues(value, o.value, true); 2739 } 2740 2741 public boolean isEmpty() { 2742 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(characteristic, value); 2743 } 2744 2745 public String fhirType() { 2746 return "MedicationKnowledge.administrationGuidelines.patientCharacteristics"; 2747 2748 } 2749 2750 } 2751 2752 @Block() 2753 public static class MedicationKnowledgeMedicineClassificationComponent extends BackboneElement 2754 implements IBaseBackboneElement { 2755 /** 2756 * The type of category for the medication (for example, therapeutic 2757 * classification, therapeutic sub-classification). 2758 */ 2759 @Child(name = "type", type = { 2760 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2761 @Description(shortDefinition = "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)", formalDefinition = "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).") 2762 protected CodeableConcept type; 2763 2764 /** 2765 * Specific category assigned to the medication (e.g. anti-infective, 2766 * anti-hypertensive, antibiotic, etc.). 2767 */ 2768 @Child(name = "classification", type = { 2769 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2770 @Description(shortDefinition = "Specific category assigned to the medication", formalDefinition = "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).") 2771 protected List<CodeableConcept> classification; 2772 2773 private static final long serialVersionUID = 1562996046L; 2774 2775 /** 2776 * Constructor 2777 */ 2778 public MedicationKnowledgeMedicineClassificationComponent() { 2779 super(); 2780 } 2781 2782 /** 2783 * Constructor 2784 */ 2785 public MedicationKnowledgeMedicineClassificationComponent(CodeableConcept type) { 2786 super(); 2787 this.type = type; 2788 } 2789 2790 /** 2791 * @return {@link #type} (The type of category for the medication (for example, 2792 * therapeutic classification, therapeutic sub-classification).) 2793 */ 2794 public CodeableConcept getType() { 2795 if (this.type == null) 2796 if (Configuration.errorOnAutoCreate()) 2797 throw new Error("Attempt to auto-create MedicationKnowledgeMedicineClassificationComponent.type"); 2798 else if (Configuration.doAutoCreate()) 2799 this.type = new CodeableConcept(); // cc 2800 return this.type; 2801 } 2802 2803 public boolean hasType() { 2804 return this.type != null && !this.type.isEmpty(); 2805 } 2806 2807 /** 2808 * @param value {@link #type} (The type of category for the medication (for 2809 * example, therapeutic classification, therapeutic 2810 * sub-classification).) 2811 */ 2812 public MedicationKnowledgeMedicineClassificationComponent setType(CodeableConcept value) { 2813 this.type = value; 2814 return this; 2815 } 2816 2817 /** 2818 * @return {@link #classification} (Specific category assigned to the medication 2819 * (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).) 2820 */ 2821 public List<CodeableConcept> getClassification() { 2822 if (this.classification == null) 2823 this.classification = new ArrayList<CodeableConcept>(); 2824 return this.classification; 2825 } 2826 2827 /** 2828 * @return Returns a reference to <code>this</code> for easy method chaining 2829 */ 2830 public MedicationKnowledgeMedicineClassificationComponent setClassification( 2831 List<CodeableConcept> theClassification) { 2832 this.classification = theClassification; 2833 return this; 2834 } 2835 2836 public boolean hasClassification() { 2837 if (this.classification == null) 2838 return false; 2839 for (CodeableConcept item : this.classification) 2840 if (!item.isEmpty()) 2841 return true; 2842 return false; 2843 } 2844 2845 public CodeableConcept addClassification() { // 3 2846 CodeableConcept t = new CodeableConcept(); 2847 if (this.classification == null) 2848 this.classification = new ArrayList<CodeableConcept>(); 2849 this.classification.add(t); 2850 return t; 2851 } 2852 2853 public MedicationKnowledgeMedicineClassificationComponent addClassification(CodeableConcept t) { // 3 2854 if (t == null) 2855 return this; 2856 if (this.classification == null) 2857 this.classification = new ArrayList<CodeableConcept>(); 2858 this.classification.add(t); 2859 return this; 2860 } 2861 2862 /** 2863 * @return The first repetition of repeating field {@link #classification}, 2864 * creating it if it does not already exist 2865 */ 2866 public CodeableConcept getClassificationFirstRep() { 2867 if (getClassification().isEmpty()) { 2868 addClassification(); 2869 } 2870 return getClassification().get(0); 2871 } 2872 2873 protected void listChildren(List<Property> children) { 2874 super.listChildren(children); 2875 children.add(new Property("type", "CodeableConcept", 2876 "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).", 2877 0, 1, type)); 2878 children.add(new Property("classification", "CodeableConcept", 2879 "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).", 0, 2880 java.lang.Integer.MAX_VALUE, classification)); 2881 } 2882 2883 @Override 2884 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2885 switch (_hash) { 2886 case 3575610: 2887 /* type */ return new Property("type", "CodeableConcept", 2888 "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).", 2889 0, 1, type); 2890 case 382350310: 2891 /* classification */ return new Property("classification", "CodeableConcept", 2892 "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).", 2893 0, java.lang.Integer.MAX_VALUE, classification); 2894 default: 2895 return super.getNamedProperty(_hash, _name, _checkValid); 2896 } 2897 2898 } 2899 2900 @Override 2901 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2902 switch (hash) { 2903 case 3575610: 2904 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2905 case 382350310: 2906 /* classification */ return this.classification == null ? new Base[0] 2907 : this.classification.toArray(new Base[this.classification.size()]); // CodeableConcept 2908 default: 2909 return super.getProperty(hash, name, checkValid); 2910 } 2911 2912 } 2913 2914 @Override 2915 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2916 switch (hash) { 2917 case 3575610: // type 2918 this.type = castToCodeableConcept(value); // CodeableConcept 2919 return value; 2920 case 382350310: // classification 2921 this.getClassification().add(castToCodeableConcept(value)); // CodeableConcept 2922 return value; 2923 default: 2924 return super.setProperty(hash, name, value); 2925 } 2926 2927 } 2928 2929 @Override 2930 public Base setProperty(String name, Base value) throws FHIRException { 2931 if (name.equals("type")) { 2932 this.type = castToCodeableConcept(value); // CodeableConcept 2933 } else if (name.equals("classification")) { 2934 this.getClassification().add(castToCodeableConcept(value)); 2935 } else 2936 return super.setProperty(name, value); 2937 return value; 2938 } 2939 2940 @Override 2941 public void removeChild(String name, Base value) throws FHIRException { 2942 if (name.equals("type")) { 2943 this.type = null; 2944 } else if (name.equals("classification")) { 2945 this.getClassification().remove(castToCodeableConcept(value)); 2946 } else 2947 super.removeChild(name, value); 2948 2949 } 2950 2951 @Override 2952 public Base makeProperty(int hash, String name) throws FHIRException { 2953 switch (hash) { 2954 case 3575610: 2955 return getType(); 2956 case 382350310: 2957 return addClassification(); 2958 default: 2959 return super.makeProperty(hash, name); 2960 } 2961 2962 } 2963 2964 @Override 2965 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2966 switch (hash) { 2967 case 3575610: 2968 /* type */ return new String[] { "CodeableConcept" }; 2969 case 382350310: 2970 /* classification */ return new String[] { "CodeableConcept" }; 2971 default: 2972 return super.getTypesForProperty(hash, name); 2973 } 2974 2975 } 2976 2977 @Override 2978 public Base addChild(String name) throws FHIRException { 2979 if (name.equals("type")) { 2980 this.type = new CodeableConcept(); 2981 return this.type; 2982 } else if (name.equals("classification")) { 2983 return addClassification(); 2984 } else 2985 return super.addChild(name); 2986 } 2987 2988 public MedicationKnowledgeMedicineClassificationComponent copy() { 2989 MedicationKnowledgeMedicineClassificationComponent dst = new MedicationKnowledgeMedicineClassificationComponent(); 2990 copyValues(dst); 2991 return dst; 2992 } 2993 2994 public void copyValues(MedicationKnowledgeMedicineClassificationComponent dst) { 2995 super.copyValues(dst); 2996 dst.type = type == null ? null : type.copy(); 2997 if (classification != null) { 2998 dst.classification = new ArrayList<CodeableConcept>(); 2999 for (CodeableConcept i : classification) 3000 dst.classification.add(i.copy()); 3001 } 3002 ; 3003 } 3004 3005 @Override 3006 public boolean equalsDeep(Base other_) { 3007 if (!super.equalsDeep(other_)) 3008 return false; 3009 if (!(other_ instanceof MedicationKnowledgeMedicineClassificationComponent)) 3010 return false; 3011 MedicationKnowledgeMedicineClassificationComponent o = (MedicationKnowledgeMedicineClassificationComponent) other_; 3012 return compareDeep(type, o.type, true) && compareDeep(classification, o.classification, true); 3013 } 3014 3015 @Override 3016 public boolean equalsShallow(Base other_) { 3017 if (!super.equalsShallow(other_)) 3018 return false; 3019 if (!(other_ instanceof MedicationKnowledgeMedicineClassificationComponent)) 3020 return false; 3021 MedicationKnowledgeMedicineClassificationComponent o = (MedicationKnowledgeMedicineClassificationComponent) other_; 3022 return true; 3023 } 3024 3025 public boolean isEmpty() { 3026 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, classification); 3027 } 3028 3029 public String fhirType() { 3030 return "MedicationKnowledge.medicineClassification"; 3031 3032 } 3033 3034 } 3035 3036 @Block() 3037 public static class MedicationKnowledgePackagingComponent extends BackboneElement implements IBaseBackboneElement { 3038 /** 3039 * A code that defines the specific type of packaging that the medication can be 3040 * found in (e.g. blister sleeve, tube, bottle). 3041 */ 3042 @Child(name = "type", type = { 3043 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3044 @Description(shortDefinition = "A code that defines the specific type of packaging that the medication can be found in", formalDefinition = "A code that defines the specific type of packaging that the medication can be found in (e.g. blister sleeve, tube, bottle).") 3045 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationknowledge-package-type") 3046 protected CodeableConcept type; 3047 3048 /** 3049 * The number of product units the package would contain if fully loaded. 3050 */ 3051 @Child(name = "quantity", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3052 @Description(shortDefinition = "The number of product units the package would contain if fully loaded", formalDefinition = "The number of product units the package would contain if fully loaded.") 3053 protected Quantity quantity; 3054 3055 private static final long serialVersionUID = -308052041L; 3056 3057 /** 3058 * Constructor 3059 */ 3060 public MedicationKnowledgePackagingComponent() { 3061 super(); 3062 } 3063 3064 /** 3065 * @return {@link #type} (A code that defines the specific type of packaging 3066 * that the medication can be found in (e.g. blister sleeve, tube, 3067 * bottle).) 3068 */ 3069 public CodeableConcept getType() { 3070 if (this.type == null) 3071 if (Configuration.errorOnAutoCreate()) 3072 throw new Error("Attempt to auto-create MedicationKnowledgePackagingComponent.type"); 3073 else if (Configuration.doAutoCreate()) 3074 this.type = new CodeableConcept(); // cc 3075 return this.type; 3076 } 3077 3078 public boolean hasType() { 3079 return this.type != null && !this.type.isEmpty(); 3080 } 3081 3082 /** 3083 * @param value {@link #type} (A code that defines the specific type of 3084 * packaging that the medication can be found in (e.g. blister 3085 * sleeve, tube, bottle).) 3086 */ 3087 public MedicationKnowledgePackagingComponent setType(CodeableConcept value) { 3088 this.type = value; 3089 return this; 3090 } 3091 3092 /** 3093 * @return {@link #quantity} (The number of product units the package would 3094 * contain if fully loaded.) 3095 */ 3096 public Quantity getQuantity() { 3097 if (this.quantity == null) 3098 if (Configuration.errorOnAutoCreate()) 3099 throw new Error("Attempt to auto-create MedicationKnowledgePackagingComponent.quantity"); 3100 else if (Configuration.doAutoCreate()) 3101 this.quantity = new Quantity(); // cc 3102 return this.quantity; 3103 } 3104 3105 public boolean hasQuantity() { 3106 return this.quantity != null && !this.quantity.isEmpty(); 3107 } 3108 3109 /** 3110 * @param value {@link #quantity} (The number of product units the package would 3111 * contain if fully loaded.) 3112 */ 3113 public MedicationKnowledgePackagingComponent setQuantity(Quantity value) { 3114 this.quantity = value; 3115 return this; 3116 } 3117 3118 protected void listChildren(List<Property> children) { 3119 super.listChildren(children); 3120 children.add(new Property("type", "CodeableConcept", 3121 "A code that defines the specific type of packaging that the medication can be found in (e.g. blister sleeve, tube, bottle).", 3122 0, 1, type)); 3123 children.add(new Property("quantity", "SimpleQuantity", 3124 "The number of product units the package would contain if fully loaded.", 0, 1, quantity)); 3125 } 3126 3127 @Override 3128 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3129 switch (_hash) { 3130 case 3575610: 3131 /* type */ return new Property("type", "CodeableConcept", 3132 "A code that defines the specific type of packaging that the medication can be found in (e.g. blister sleeve, tube, bottle).", 3133 0, 1, type); 3134 case -1285004149: 3135 /* quantity */ return new Property("quantity", "SimpleQuantity", 3136 "The number of product units the package would contain if fully loaded.", 0, 1, quantity); 3137 default: 3138 return super.getNamedProperty(_hash, _name, _checkValid); 3139 } 3140 3141 } 3142 3143 @Override 3144 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3145 switch (hash) { 3146 case 3575610: 3147 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3148 case -1285004149: 3149 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 3150 default: 3151 return super.getProperty(hash, name, checkValid); 3152 } 3153 3154 } 3155 3156 @Override 3157 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3158 switch (hash) { 3159 case 3575610: // type 3160 this.type = castToCodeableConcept(value); // CodeableConcept 3161 return value; 3162 case -1285004149: // quantity 3163 this.quantity = castToQuantity(value); // Quantity 3164 return value; 3165 default: 3166 return super.setProperty(hash, name, value); 3167 } 3168 3169 } 3170 3171 @Override 3172 public Base setProperty(String name, Base value) throws FHIRException { 3173 if (name.equals("type")) { 3174 this.type = castToCodeableConcept(value); // CodeableConcept 3175 } else if (name.equals("quantity")) { 3176 this.quantity = castToQuantity(value); // Quantity 3177 } else 3178 return super.setProperty(name, value); 3179 return value; 3180 } 3181 3182 @Override 3183 public void removeChild(String name, Base value) throws FHIRException { 3184 if (name.equals("type")) { 3185 this.type = null; 3186 } else if (name.equals("quantity")) { 3187 this.quantity = null; 3188 } else 3189 super.removeChild(name, value); 3190 3191 } 3192 3193 @Override 3194 public Base makeProperty(int hash, String name) throws FHIRException { 3195 switch (hash) { 3196 case 3575610: 3197 return getType(); 3198 case -1285004149: 3199 return getQuantity(); 3200 default: 3201 return super.makeProperty(hash, name); 3202 } 3203 3204 } 3205 3206 @Override 3207 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3208 switch (hash) { 3209 case 3575610: 3210 /* type */ return new String[] { "CodeableConcept" }; 3211 case -1285004149: 3212 /* quantity */ return new String[] { "SimpleQuantity" }; 3213 default: 3214 return super.getTypesForProperty(hash, name); 3215 } 3216 3217 } 3218 3219 @Override 3220 public Base addChild(String name) throws FHIRException { 3221 if (name.equals("type")) { 3222 this.type = new CodeableConcept(); 3223 return this.type; 3224 } else if (name.equals("quantity")) { 3225 this.quantity = new Quantity(); 3226 return this.quantity; 3227 } else 3228 return super.addChild(name); 3229 } 3230 3231 public MedicationKnowledgePackagingComponent copy() { 3232 MedicationKnowledgePackagingComponent dst = new MedicationKnowledgePackagingComponent(); 3233 copyValues(dst); 3234 return dst; 3235 } 3236 3237 public void copyValues(MedicationKnowledgePackagingComponent dst) { 3238 super.copyValues(dst); 3239 dst.type = type == null ? null : type.copy(); 3240 dst.quantity = quantity == null ? null : quantity.copy(); 3241 } 3242 3243 @Override 3244 public boolean equalsDeep(Base other_) { 3245 if (!super.equalsDeep(other_)) 3246 return false; 3247 if (!(other_ instanceof MedicationKnowledgePackagingComponent)) 3248 return false; 3249 MedicationKnowledgePackagingComponent o = (MedicationKnowledgePackagingComponent) other_; 3250 return compareDeep(type, o.type, true) && compareDeep(quantity, o.quantity, true); 3251 } 3252 3253 @Override 3254 public boolean equalsShallow(Base other_) { 3255 if (!super.equalsShallow(other_)) 3256 return false; 3257 if (!(other_ instanceof MedicationKnowledgePackagingComponent)) 3258 return false; 3259 MedicationKnowledgePackagingComponent o = (MedicationKnowledgePackagingComponent) other_; 3260 return true; 3261 } 3262 3263 public boolean isEmpty() { 3264 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, quantity); 3265 } 3266 3267 public String fhirType() { 3268 return "MedicationKnowledge.packaging"; 3269 3270 } 3271 3272 } 3273 3274 @Block() 3275 public static class MedicationKnowledgeDrugCharacteristicComponent extends BackboneElement 3276 implements IBaseBackboneElement { 3277 /** 3278 * A code specifying which characteristic of the medicine is being described 3279 * (for example, colour, shape, imprint). 3280 */ 3281 @Child(name = "type", type = { 3282 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3283 @Description(shortDefinition = "Code specifying the type of characteristic of medication", formalDefinition = "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).") 3284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationknowledge-characteristic") 3285 protected CodeableConcept type; 3286 3287 /** 3288 * Description of the characteristic. 3289 */ 3290 @Child(name = "value", type = { CodeableConcept.class, StringType.class, Quantity.class, 3291 Base64BinaryType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3292 @Description(shortDefinition = "Description of the characteristic", formalDefinition = "Description of the characteristic.") 3293 protected Type value; 3294 3295 private static final long serialVersionUID = -491121170L; 3296 3297 /** 3298 * Constructor 3299 */ 3300 public MedicationKnowledgeDrugCharacteristicComponent() { 3301 super(); 3302 } 3303 3304 /** 3305 * @return {@link #type} (A code specifying which characteristic of the medicine 3306 * is being described (for example, colour, shape, imprint).) 3307 */ 3308 public CodeableConcept getType() { 3309 if (this.type == null) 3310 if (Configuration.errorOnAutoCreate()) 3311 throw new Error("Attempt to auto-create MedicationKnowledgeDrugCharacteristicComponent.type"); 3312 else if (Configuration.doAutoCreate()) 3313 this.type = new CodeableConcept(); // cc 3314 return this.type; 3315 } 3316 3317 public boolean hasType() { 3318 return this.type != null && !this.type.isEmpty(); 3319 } 3320 3321 /** 3322 * @param value {@link #type} (A code specifying which characteristic of the 3323 * medicine is being described (for example, colour, shape, 3324 * imprint).) 3325 */ 3326 public MedicationKnowledgeDrugCharacteristicComponent setType(CodeableConcept value) { 3327 this.type = value; 3328 return this; 3329 } 3330 3331 /** 3332 * @return {@link #value} (Description of the characteristic.) 3333 */ 3334 public Type getValue() { 3335 return this.value; 3336 } 3337 3338 /** 3339 * @return {@link #value} (Description of the characteristic.) 3340 */ 3341 public CodeableConcept getValueCodeableConcept() throws FHIRException { 3342 if (this.value == null) 3343 this.value = new CodeableConcept(); 3344 if (!(this.value instanceof CodeableConcept)) 3345 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 3346 + this.value.getClass().getName() + " was encountered"); 3347 return (CodeableConcept) this.value; 3348 } 3349 3350 public boolean hasValueCodeableConcept() { 3351 return this != null && this.value instanceof CodeableConcept; 3352 } 3353 3354 /** 3355 * @return {@link #value} (Description of the characteristic.) 3356 */ 3357 public StringType getValueStringType() throws FHIRException { 3358 if (this.value == null) 3359 this.value = new StringType(); 3360 if (!(this.value instanceof StringType)) 3361 throw new FHIRException("Type mismatch: the type StringType was expected, but " 3362 + this.value.getClass().getName() + " was encountered"); 3363 return (StringType) this.value; 3364 } 3365 3366 public boolean hasValueStringType() { 3367 return this != null && this.value instanceof StringType; 3368 } 3369 3370 /** 3371 * @return {@link #value} (Description of the characteristic.) 3372 */ 3373 public Quantity getValueQuantity() throws FHIRException { 3374 if (this.value == null) 3375 this.value = new Quantity(); 3376 if (!(this.value instanceof Quantity)) 3377 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 3378 + " was encountered"); 3379 return (Quantity) this.value; 3380 } 3381 3382 public boolean hasValueQuantity() { 3383 return this != null && this.value instanceof Quantity; 3384 } 3385 3386 /** 3387 * @return {@link #value} (Description of the characteristic.) 3388 */ 3389 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 3390 if (this.value == null) 3391 this.value = new Base64BinaryType(); 3392 if (!(this.value instanceof Base64BinaryType)) 3393 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but " 3394 + this.value.getClass().getName() + " was encountered"); 3395 return (Base64BinaryType) this.value; 3396 } 3397 3398 public boolean hasValueBase64BinaryType() { 3399 return this != null && this.value instanceof Base64BinaryType; 3400 } 3401 3402 public boolean hasValue() { 3403 return this.value != null && !this.value.isEmpty(); 3404 } 3405 3406 /** 3407 * @param value {@link #value} (Description of the characteristic.) 3408 */ 3409 public MedicationKnowledgeDrugCharacteristicComponent setValue(Type value) { 3410 if (value != null && !(value instanceof CodeableConcept || value instanceof StringType 3411 || value instanceof Quantity || value instanceof Base64BinaryType)) 3412 throw new Error("Not the right type for MedicationKnowledge.drugCharacteristic.value[x]: " + value.fhirType()); 3413 this.value = value; 3414 return this; 3415 } 3416 3417 protected void listChildren(List<Property> children) { 3418 super.listChildren(children); 3419 children.add(new Property("type", "CodeableConcept", 3420 "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).", 3421 0, 1, type)); 3422 children.add(new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3423 "Description of the characteristic.", 0, 1, value)); 3424 } 3425 3426 @Override 3427 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3428 switch (_hash) { 3429 case 3575610: 3430 /* type */ return new Property("type", "CodeableConcept", 3431 "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).", 3432 0, 1, type); 3433 case -1410166417: 3434 /* value[x] */ return new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3435 "Description of the characteristic.", 0, 1, value); 3436 case 111972721: 3437 /* value */ return new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3438 "Description of the characteristic.", 0, 1, value); 3439 case 924902896: 3440 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3441 "Description of the characteristic.", 0, 1, value); 3442 case -1424603934: 3443 /* valueString */ return new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3444 "Description of the characteristic.", 0, 1, value); 3445 case -2029823716: 3446 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3447 "Description of the characteristic.", 0, 1, value); 3448 case -1535024575: 3449 /* valueBase64Binary */ return new Property("value[x]", "CodeableConcept|string|SimpleQuantity|base64Binary", 3450 "Description of the characteristic.", 0, 1, value); 3451 default: 3452 return super.getNamedProperty(_hash, _name, _checkValid); 3453 } 3454 3455 } 3456 3457 @Override 3458 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3459 switch (hash) { 3460 case 3575610: 3461 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3462 case 111972721: 3463 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3464 default: 3465 return super.getProperty(hash, name, checkValid); 3466 } 3467 3468 } 3469 3470 @Override 3471 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3472 switch (hash) { 3473 case 3575610: // type 3474 this.type = castToCodeableConcept(value); // CodeableConcept 3475 return value; 3476 case 111972721: // value 3477 this.value = castToType(value); // Type 3478 return value; 3479 default: 3480 return super.setProperty(hash, name, value); 3481 } 3482 3483 } 3484 3485 @Override 3486 public Base setProperty(String name, Base value) throws FHIRException { 3487 if (name.equals("type")) { 3488 this.type = castToCodeableConcept(value); // CodeableConcept 3489 } else if (name.equals("value[x]")) { 3490 this.value = castToType(value); // Type 3491 } else 3492 return super.setProperty(name, value); 3493 return value; 3494 } 3495 3496 @Override 3497 public void removeChild(String name, Base value) throws FHIRException { 3498 if (name.equals("type")) { 3499 this.type = null; 3500 } else if (name.equals("value[x]")) { 3501 this.value = null; 3502 } else 3503 super.removeChild(name, value); 3504 3505 } 3506 3507 @Override 3508 public Base makeProperty(int hash, String name) throws FHIRException { 3509 switch (hash) { 3510 case 3575610: 3511 return getType(); 3512 case -1410166417: 3513 return getValue(); 3514 case 111972721: 3515 return getValue(); 3516 default: 3517 return super.makeProperty(hash, name); 3518 } 3519 3520 } 3521 3522 @Override 3523 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3524 switch (hash) { 3525 case 3575610: 3526 /* type */ return new String[] { "CodeableConcept" }; 3527 case 111972721: 3528 /* value */ return new String[] { "CodeableConcept", "string", "SimpleQuantity", "base64Binary" }; 3529 default: 3530 return super.getTypesForProperty(hash, name); 3531 } 3532 3533 } 3534 3535 @Override 3536 public Base addChild(String name) throws FHIRException { 3537 if (name.equals("type")) { 3538 this.type = new CodeableConcept(); 3539 return this.type; 3540 } else if (name.equals("valueCodeableConcept")) { 3541 this.value = new CodeableConcept(); 3542 return this.value; 3543 } else if (name.equals("valueString")) { 3544 this.value = new StringType(); 3545 return this.value; 3546 } else if (name.equals("valueQuantity")) { 3547 this.value = new Quantity(); 3548 return this.value; 3549 } else if (name.equals("valueBase64Binary")) { 3550 this.value = new Base64BinaryType(); 3551 return this.value; 3552 } else 3553 return super.addChild(name); 3554 } 3555 3556 public MedicationKnowledgeDrugCharacteristicComponent copy() { 3557 MedicationKnowledgeDrugCharacteristicComponent dst = new MedicationKnowledgeDrugCharacteristicComponent(); 3558 copyValues(dst); 3559 return dst; 3560 } 3561 3562 public void copyValues(MedicationKnowledgeDrugCharacteristicComponent dst) { 3563 super.copyValues(dst); 3564 dst.type = type == null ? null : type.copy(); 3565 dst.value = value == null ? null : value.copy(); 3566 } 3567 3568 @Override 3569 public boolean equalsDeep(Base other_) { 3570 if (!super.equalsDeep(other_)) 3571 return false; 3572 if (!(other_ instanceof MedicationKnowledgeDrugCharacteristicComponent)) 3573 return false; 3574 MedicationKnowledgeDrugCharacteristicComponent o = (MedicationKnowledgeDrugCharacteristicComponent) other_; 3575 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3576 } 3577 3578 @Override 3579 public boolean equalsShallow(Base other_) { 3580 if (!super.equalsShallow(other_)) 3581 return false; 3582 if (!(other_ instanceof MedicationKnowledgeDrugCharacteristicComponent)) 3583 return false; 3584 MedicationKnowledgeDrugCharacteristicComponent o = (MedicationKnowledgeDrugCharacteristicComponent) other_; 3585 return true; 3586 } 3587 3588 public boolean isEmpty() { 3589 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3590 } 3591 3592 public String fhirType() { 3593 return "MedicationKnowledge.drugCharacteristic"; 3594 3595 } 3596 3597 } 3598 3599 @Block() 3600 public static class MedicationKnowledgeRegulatoryComponent extends BackboneElement implements IBaseBackboneElement { 3601 /** 3602 * The authority that is specifying the regulations. 3603 */ 3604 @Child(name = "regulatoryAuthority", type = { 3605 Organization.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3606 @Description(shortDefinition = "Specifies the authority of the regulation", formalDefinition = "The authority that is specifying the regulations.") 3607 protected Reference regulatoryAuthority; 3608 3609 /** 3610 * The actual object that is the target of the reference (The authority that is 3611 * specifying the regulations.) 3612 */ 3613 protected Organization regulatoryAuthorityTarget; 3614 3615 /** 3616 * Specifies if changes are allowed when dispensing a medication from a 3617 * regulatory perspective. 3618 */ 3619 @Child(name = "substitution", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3620 @Description(shortDefinition = "Specifies if changes are allowed when dispensing a medication from a regulatory perspective", formalDefinition = "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.") 3621 protected List<MedicationKnowledgeRegulatorySubstitutionComponent> substitution; 3622 3623 /** 3624 * Specifies the schedule of a medication in jurisdiction. 3625 */ 3626 @Child(name = "schedule", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3627 @Description(shortDefinition = "Specifies the schedule of a medication in jurisdiction", formalDefinition = "Specifies the schedule of a medication in jurisdiction.") 3628 protected List<MedicationKnowledgeRegulatoryScheduleComponent> schedule; 3629 3630 /** 3631 * The maximum number of units of the medication that can be dispensed in a 3632 * period. 3633 */ 3634 @Child(name = "maxDispense", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = false) 3635 @Description(shortDefinition = "The maximum number of units of the medication that can be dispensed in a period", formalDefinition = "The maximum number of units of the medication that can be dispensed in a period.") 3636 protected MedicationKnowledgeRegulatoryMaxDispenseComponent maxDispense; 3637 3638 private static final long serialVersionUID = -1252605487L; 3639 3640 /** 3641 * Constructor 3642 */ 3643 public MedicationKnowledgeRegulatoryComponent() { 3644 super(); 3645 } 3646 3647 /** 3648 * Constructor 3649 */ 3650 public MedicationKnowledgeRegulatoryComponent(Reference regulatoryAuthority) { 3651 super(); 3652 this.regulatoryAuthority = regulatoryAuthority; 3653 } 3654 3655 /** 3656 * @return {@link #regulatoryAuthority} (The authority that is specifying the 3657 * regulations.) 3658 */ 3659 public Reference getRegulatoryAuthority() { 3660 if (this.regulatoryAuthority == null) 3661 if (Configuration.errorOnAutoCreate()) 3662 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.regulatoryAuthority"); 3663 else if (Configuration.doAutoCreate()) 3664 this.regulatoryAuthority = new Reference(); // cc 3665 return this.regulatoryAuthority; 3666 } 3667 3668 public boolean hasRegulatoryAuthority() { 3669 return this.regulatoryAuthority != null && !this.regulatoryAuthority.isEmpty(); 3670 } 3671 3672 /** 3673 * @param value {@link #regulatoryAuthority} (The authority that is specifying 3674 * the regulations.) 3675 */ 3676 public MedicationKnowledgeRegulatoryComponent setRegulatoryAuthority(Reference value) { 3677 this.regulatoryAuthority = value; 3678 return this; 3679 } 3680 3681 /** 3682 * @return {@link #regulatoryAuthority} The actual object that is the target of 3683 * the reference. The reference library doesn't populate this, but you 3684 * can use it to hold the resource if you resolve it. (The authority 3685 * that is specifying the regulations.) 3686 */ 3687 public Organization getRegulatoryAuthorityTarget() { 3688 if (this.regulatoryAuthorityTarget == null) 3689 if (Configuration.errorOnAutoCreate()) 3690 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.regulatoryAuthority"); 3691 else if (Configuration.doAutoCreate()) 3692 this.regulatoryAuthorityTarget = new Organization(); // aa 3693 return this.regulatoryAuthorityTarget; 3694 } 3695 3696 /** 3697 * @param value {@link #regulatoryAuthority} The actual object that is the 3698 * target of the reference. The reference library doesn't use 3699 * these, but you can use it to hold the resource if you resolve 3700 * it. (The authority that is specifying the regulations.) 3701 */ 3702 public MedicationKnowledgeRegulatoryComponent setRegulatoryAuthorityTarget(Organization value) { 3703 this.regulatoryAuthorityTarget = value; 3704 return this; 3705 } 3706 3707 /** 3708 * @return {@link #substitution} (Specifies if changes are allowed when 3709 * dispensing a medication from a regulatory perspective.) 3710 */ 3711 public List<MedicationKnowledgeRegulatorySubstitutionComponent> getSubstitution() { 3712 if (this.substitution == null) 3713 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3714 return this.substitution; 3715 } 3716 3717 /** 3718 * @return Returns a reference to <code>this</code> for easy method chaining 3719 */ 3720 public MedicationKnowledgeRegulatoryComponent setSubstitution( 3721 List<MedicationKnowledgeRegulatorySubstitutionComponent> theSubstitution) { 3722 this.substitution = theSubstitution; 3723 return this; 3724 } 3725 3726 public boolean hasSubstitution() { 3727 if (this.substitution == null) 3728 return false; 3729 for (MedicationKnowledgeRegulatorySubstitutionComponent item : this.substitution) 3730 if (!item.isEmpty()) 3731 return true; 3732 return false; 3733 } 3734 3735 public MedicationKnowledgeRegulatorySubstitutionComponent addSubstitution() { // 3 3736 MedicationKnowledgeRegulatorySubstitutionComponent t = new MedicationKnowledgeRegulatorySubstitutionComponent(); 3737 if (this.substitution == null) 3738 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3739 this.substitution.add(t); 3740 return t; 3741 } 3742 3743 public MedicationKnowledgeRegulatoryComponent addSubstitution( 3744 MedicationKnowledgeRegulatorySubstitutionComponent t) { // 3 3745 if (t == null) 3746 return this; 3747 if (this.substitution == null) 3748 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3749 this.substitution.add(t); 3750 return this; 3751 } 3752 3753 /** 3754 * @return The first repetition of repeating field {@link #substitution}, 3755 * creating it if it does not already exist 3756 */ 3757 public MedicationKnowledgeRegulatorySubstitutionComponent getSubstitutionFirstRep() { 3758 if (getSubstitution().isEmpty()) { 3759 addSubstitution(); 3760 } 3761 return getSubstitution().get(0); 3762 } 3763 3764 /** 3765 * @return {@link #schedule} (Specifies the schedule of a medication in 3766 * jurisdiction.) 3767 */ 3768 public List<MedicationKnowledgeRegulatoryScheduleComponent> getSchedule() { 3769 if (this.schedule == null) 3770 this.schedule = new ArrayList<MedicationKnowledgeRegulatoryScheduleComponent>(); 3771 return this.schedule; 3772 } 3773 3774 /** 3775 * @return Returns a reference to <code>this</code> for easy method chaining 3776 */ 3777 public MedicationKnowledgeRegulatoryComponent setSchedule( 3778 List<MedicationKnowledgeRegulatoryScheduleComponent> theSchedule) { 3779 this.schedule = theSchedule; 3780 return this; 3781 } 3782 3783 public boolean hasSchedule() { 3784 if (this.schedule == null) 3785 return false; 3786 for (MedicationKnowledgeRegulatoryScheduleComponent item : this.schedule) 3787 if (!item.isEmpty()) 3788 return true; 3789 return false; 3790 } 3791 3792 public MedicationKnowledgeRegulatoryScheduleComponent addSchedule() { // 3 3793 MedicationKnowledgeRegulatoryScheduleComponent t = new MedicationKnowledgeRegulatoryScheduleComponent(); 3794 if (this.schedule == null) 3795 this.schedule = new ArrayList<MedicationKnowledgeRegulatoryScheduleComponent>(); 3796 this.schedule.add(t); 3797 return t; 3798 } 3799 3800 public MedicationKnowledgeRegulatoryComponent addSchedule(MedicationKnowledgeRegulatoryScheduleComponent t) { // 3 3801 if (t == null) 3802 return this; 3803 if (this.schedule == null) 3804 this.schedule = new ArrayList<MedicationKnowledgeRegulatoryScheduleComponent>(); 3805 this.schedule.add(t); 3806 return this; 3807 } 3808 3809 /** 3810 * @return The first repetition of repeating field {@link #schedule}, creating 3811 * it if it does not already exist 3812 */ 3813 public MedicationKnowledgeRegulatoryScheduleComponent getScheduleFirstRep() { 3814 if (getSchedule().isEmpty()) { 3815 addSchedule(); 3816 } 3817 return getSchedule().get(0); 3818 } 3819 3820 /** 3821 * @return {@link #maxDispense} (The maximum number of units of the medication 3822 * that can be dispensed in a period.) 3823 */ 3824 public MedicationKnowledgeRegulatoryMaxDispenseComponent getMaxDispense() { 3825 if (this.maxDispense == null) 3826 if (Configuration.errorOnAutoCreate()) 3827 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.maxDispense"); 3828 else if (Configuration.doAutoCreate()) 3829 this.maxDispense = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); // cc 3830 return this.maxDispense; 3831 } 3832 3833 public boolean hasMaxDispense() { 3834 return this.maxDispense != null && !this.maxDispense.isEmpty(); 3835 } 3836 3837 /** 3838 * @param value {@link #maxDispense} (The maximum number of units of the 3839 * medication that can be dispensed in a period.) 3840 */ 3841 public MedicationKnowledgeRegulatoryComponent setMaxDispense( 3842 MedicationKnowledgeRegulatoryMaxDispenseComponent value) { 3843 this.maxDispense = value; 3844 return this; 3845 } 3846 3847 protected void listChildren(List<Property> children) { 3848 super.listChildren(children); 3849 children.add(new Property("regulatoryAuthority", "Reference(Organization)", 3850 "The authority that is specifying the regulations.", 0, 1, regulatoryAuthority)); 3851 children.add(new Property("substitution", "", 3852 "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.", 0, 3853 java.lang.Integer.MAX_VALUE, substitution)); 3854 children.add(new Property("schedule", "", "Specifies the schedule of a medication in jurisdiction.", 0, 3855 java.lang.Integer.MAX_VALUE, schedule)); 3856 children.add(new Property("maxDispense", "", 3857 "The maximum number of units of the medication that can be dispensed in a period.", 0, 1, maxDispense)); 3858 } 3859 3860 @Override 3861 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3862 switch (_hash) { 3863 case 711233419: 3864 /* regulatoryAuthority */ return new Property("regulatoryAuthority", "Reference(Organization)", 3865 "The authority that is specifying the regulations.", 0, 1, regulatoryAuthority); 3866 case 826147581: 3867 /* substitution */ return new Property("substitution", "", 3868 "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.", 0, 3869 java.lang.Integer.MAX_VALUE, substitution); 3870 case -697920873: 3871 /* schedule */ return new Property("schedule", "", "Specifies the schedule of a medication in jurisdiction.", 0, 3872 java.lang.Integer.MAX_VALUE, schedule); 3873 case -1977784607: 3874 /* maxDispense */ return new Property("maxDispense", "", 3875 "The maximum number of units of the medication that can be dispensed in a period.", 0, 1, maxDispense); 3876 default: 3877 return super.getNamedProperty(_hash, _name, _checkValid); 3878 } 3879 3880 } 3881 3882 @Override 3883 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3884 switch (hash) { 3885 case 711233419: 3886 /* regulatoryAuthority */ return this.regulatoryAuthority == null ? new Base[0] 3887 : new Base[] { this.regulatoryAuthority }; // Reference 3888 case 826147581: 3889 /* substitution */ return this.substitution == null ? new Base[0] 3890 : this.substitution.toArray(new Base[this.substitution.size()]); // MedicationKnowledgeRegulatorySubstitutionComponent 3891 case -697920873: 3892 /* schedule */ return this.schedule == null ? new Base[0] 3893 : this.schedule.toArray(new Base[this.schedule.size()]); // MedicationKnowledgeRegulatoryScheduleComponent 3894 case -1977784607: 3895 /* maxDispense */ return this.maxDispense == null ? new Base[0] : new Base[] { this.maxDispense }; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3896 default: 3897 return super.getProperty(hash, name, checkValid); 3898 } 3899 3900 } 3901 3902 @Override 3903 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3904 switch (hash) { 3905 case 711233419: // regulatoryAuthority 3906 this.regulatoryAuthority = castToReference(value); // Reference 3907 return value; 3908 case 826147581: // substitution 3909 this.getSubstitution().add((MedicationKnowledgeRegulatorySubstitutionComponent) value); // MedicationKnowledgeRegulatorySubstitutionComponent 3910 return value; 3911 case -697920873: // schedule 3912 this.getSchedule().add((MedicationKnowledgeRegulatoryScheduleComponent) value); // MedicationKnowledgeRegulatoryScheduleComponent 3913 return value; 3914 case -1977784607: // maxDispense 3915 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3916 return value; 3917 default: 3918 return super.setProperty(hash, name, value); 3919 } 3920 3921 } 3922 3923 @Override 3924 public Base setProperty(String name, Base value) throws FHIRException { 3925 if (name.equals("regulatoryAuthority")) { 3926 this.regulatoryAuthority = castToReference(value); // Reference 3927 } else if (name.equals("substitution")) { 3928 this.getSubstitution().add((MedicationKnowledgeRegulatorySubstitutionComponent) value); 3929 } else if (name.equals("schedule")) { 3930 this.getSchedule().add((MedicationKnowledgeRegulatoryScheduleComponent) value); 3931 } else if (name.equals("maxDispense")) { 3932 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3933 } else 3934 return super.setProperty(name, value); 3935 return value; 3936 } 3937 3938 @Override 3939 public void removeChild(String name, Base value) throws FHIRException { 3940 if (name.equals("regulatoryAuthority")) { 3941 this.regulatoryAuthority = null; 3942 } else if (name.equals("substitution")) { 3943 this.getSubstitution().remove((MedicationKnowledgeRegulatorySubstitutionComponent) value); 3944 } else if (name.equals("schedule")) { 3945 this.getSchedule().remove((MedicationKnowledgeRegulatoryScheduleComponent) value); 3946 } else if (name.equals("maxDispense")) { 3947 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3948 } else 3949 super.removeChild(name, value); 3950 3951 } 3952 3953 @Override 3954 public Base makeProperty(int hash, String name) throws FHIRException { 3955 switch (hash) { 3956 case 711233419: 3957 return getRegulatoryAuthority(); 3958 case 826147581: 3959 return addSubstitution(); 3960 case -697920873: 3961 return addSchedule(); 3962 case -1977784607: 3963 return getMaxDispense(); 3964 default: 3965 return super.makeProperty(hash, name); 3966 } 3967 3968 } 3969 3970 @Override 3971 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3972 switch (hash) { 3973 case 711233419: 3974 /* regulatoryAuthority */ return new String[] { "Reference" }; 3975 case 826147581: 3976 /* substitution */ return new String[] {}; 3977 case -697920873: 3978 /* schedule */ return new String[] {}; 3979 case -1977784607: 3980 /* maxDispense */ return new String[] {}; 3981 default: 3982 return super.getTypesForProperty(hash, name); 3983 } 3984 3985 } 3986 3987 @Override 3988 public Base addChild(String name) throws FHIRException { 3989 if (name.equals("regulatoryAuthority")) { 3990 this.regulatoryAuthority = new Reference(); 3991 return this.regulatoryAuthority; 3992 } else if (name.equals("substitution")) { 3993 return addSubstitution(); 3994 } else if (name.equals("schedule")) { 3995 return addSchedule(); 3996 } else if (name.equals("maxDispense")) { 3997 this.maxDispense = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); 3998 return this.maxDispense; 3999 } else 4000 return super.addChild(name); 4001 } 4002 4003 public MedicationKnowledgeRegulatoryComponent copy() { 4004 MedicationKnowledgeRegulatoryComponent dst = new MedicationKnowledgeRegulatoryComponent(); 4005 copyValues(dst); 4006 return dst; 4007 } 4008 4009 public void copyValues(MedicationKnowledgeRegulatoryComponent dst) { 4010 super.copyValues(dst); 4011 dst.regulatoryAuthority = regulatoryAuthority == null ? null : regulatoryAuthority.copy(); 4012 if (substitution != null) { 4013 dst.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 4014 for (MedicationKnowledgeRegulatorySubstitutionComponent i : substitution) 4015 dst.substitution.add(i.copy()); 4016 } 4017 ; 4018 if (schedule != null) { 4019 dst.schedule = new ArrayList<MedicationKnowledgeRegulatoryScheduleComponent>(); 4020 for (MedicationKnowledgeRegulatoryScheduleComponent i : schedule) 4021 dst.schedule.add(i.copy()); 4022 } 4023 ; 4024 dst.maxDispense = maxDispense == null ? null : maxDispense.copy(); 4025 } 4026 4027 @Override 4028 public boolean equalsDeep(Base other_) { 4029 if (!super.equalsDeep(other_)) 4030 return false; 4031 if (!(other_ instanceof MedicationKnowledgeRegulatoryComponent)) 4032 return false; 4033 MedicationKnowledgeRegulatoryComponent o = (MedicationKnowledgeRegulatoryComponent) other_; 4034 return compareDeep(regulatoryAuthority, o.regulatoryAuthority, true) 4035 && compareDeep(substitution, o.substitution, true) && compareDeep(schedule, o.schedule, true) 4036 && compareDeep(maxDispense, o.maxDispense, true); 4037 } 4038 4039 @Override 4040 public boolean equalsShallow(Base other_) { 4041 if (!super.equalsShallow(other_)) 4042 return false; 4043 if (!(other_ instanceof MedicationKnowledgeRegulatoryComponent)) 4044 return false; 4045 MedicationKnowledgeRegulatoryComponent o = (MedicationKnowledgeRegulatoryComponent) other_; 4046 return true; 4047 } 4048 4049 public boolean isEmpty() { 4050 return super.isEmpty() 4051 && ca.uhn.fhir.util.ElementUtil.isEmpty(regulatoryAuthority, substitution, schedule, maxDispense); 4052 } 4053 4054 public String fhirType() { 4055 return "MedicationKnowledge.regulatory"; 4056 4057 } 4058 4059 } 4060 4061 @Block() 4062 public static class MedicationKnowledgeRegulatorySubstitutionComponent extends BackboneElement 4063 implements IBaseBackboneElement { 4064 /** 4065 * Specifies the type of substitution allowed. 4066 */ 4067 @Child(name = "type", type = { 4068 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4069 @Description(shortDefinition = "Specifies the type of substitution allowed", formalDefinition = "Specifies the type of substitution allowed.") 4070 protected CodeableConcept type; 4071 4072 /** 4073 * Specifies if regulation allows for changes in the medication when dispensing. 4074 */ 4075 @Child(name = "allowed", type = { 4076 BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4077 @Description(shortDefinition = "Specifies if regulation allows for changes in the medication when dispensing", formalDefinition = "Specifies if regulation allows for changes in the medication when dispensing.") 4078 protected BooleanType allowed; 4079 4080 private static final long serialVersionUID = 396354861L; 4081 4082 /** 4083 * Constructor 4084 */ 4085 public MedicationKnowledgeRegulatorySubstitutionComponent() { 4086 super(); 4087 } 4088 4089 /** 4090 * Constructor 4091 */ 4092 public MedicationKnowledgeRegulatorySubstitutionComponent(CodeableConcept type, BooleanType allowed) { 4093 super(); 4094 this.type = type; 4095 this.allowed = allowed; 4096 } 4097 4098 /** 4099 * @return {@link #type} (Specifies the type of substitution allowed.) 4100 */ 4101 public CodeableConcept getType() { 4102 if (this.type == null) 4103 if (Configuration.errorOnAutoCreate()) 4104 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatorySubstitutionComponent.type"); 4105 else if (Configuration.doAutoCreate()) 4106 this.type = new CodeableConcept(); // cc 4107 return this.type; 4108 } 4109 4110 public boolean hasType() { 4111 return this.type != null && !this.type.isEmpty(); 4112 } 4113 4114 /** 4115 * @param value {@link #type} (Specifies the type of substitution allowed.) 4116 */ 4117 public MedicationKnowledgeRegulatorySubstitutionComponent setType(CodeableConcept value) { 4118 this.type = value; 4119 return this; 4120 } 4121 4122 /** 4123 * @return {@link #allowed} (Specifies if regulation allows for changes in the 4124 * medication when dispensing.). This is the underlying object with id, 4125 * value and extensions. The accessor "getAllowed" gives direct access 4126 * to the value 4127 */ 4128 public BooleanType getAllowedElement() { 4129 if (this.allowed == null) 4130 if (Configuration.errorOnAutoCreate()) 4131 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatorySubstitutionComponent.allowed"); 4132 else if (Configuration.doAutoCreate()) 4133 this.allowed = new BooleanType(); // bb 4134 return this.allowed; 4135 } 4136 4137 public boolean hasAllowedElement() { 4138 return this.allowed != null && !this.allowed.isEmpty(); 4139 } 4140 4141 public boolean hasAllowed() { 4142 return this.allowed != null && !this.allowed.isEmpty(); 4143 } 4144 4145 /** 4146 * @param value {@link #allowed} (Specifies if regulation allows for changes in 4147 * the medication when dispensing.). This is the underlying object 4148 * with id, value and extensions. The accessor "getAllowed" gives 4149 * direct access to the value 4150 */ 4151 public MedicationKnowledgeRegulatorySubstitutionComponent setAllowedElement(BooleanType value) { 4152 this.allowed = value; 4153 return this; 4154 } 4155 4156 /** 4157 * @return Specifies if regulation allows for changes in the medication when 4158 * dispensing. 4159 */ 4160 public boolean getAllowed() { 4161 return this.allowed == null || this.allowed.isEmpty() ? false : this.allowed.getValue(); 4162 } 4163 4164 /** 4165 * @param value Specifies if regulation allows for changes in the medication 4166 * when dispensing. 4167 */ 4168 public MedicationKnowledgeRegulatorySubstitutionComponent setAllowed(boolean value) { 4169 if (this.allowed == null) 4170 this.allowed = new BooleanType(); 4171 this.allowed.setValue(value); 4172 return this; 4173 } 4174 4175 protected void listChildren(List<Property> children) { 4176 super.listChildren(children); 4177 children.add(new Property("type", "CodeableConcept", "Specifies the type of substitution allowed.", 0, 1, type)); 4178 children.add(new Property("allowed", "boolean", 4179 "Specifies if regulation allows for changes in the medication when dispensing.", 0, 1, allowed)); 4180 } 4181 4182 @Override 4183 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4184 switch (_hash) { 4185 case 3575610: 4186 /* type */ return new Property("type", "CodeableConcept", "Specifies the type of substitution allowed.", 0, 1, 4187 type); 4188 case -911343192: 4189 /* allowed */ return new Property("allowed", "boolean", 4190 "Specifies if regulation allows for changes in the medication when dispensing.", 0, 1, allowed); 4191 default: 4192 return super.getNamedProperty(_hash, _name, _checkValid); 4193 } 4194 4195 } 4196 4197 @Override 4198 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4199 switch (hash) { 4200 case 3575610: 4201 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4202 case -911343192: 4203 /* allowed */ return this.allowed == null ? new Base[0] : new Base[] { this.allowed }; // BooleanType 4204 default: 4205 return super.getProperty(hash, name, checkValid); 4206 } 4207 4208 } 4209 4210 @Override 4211 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4212 switch (hash) { 4213 case 3575610: // type 4214 this.type = castToCodeableConcept(value); // CodeableConcept 4215 return value; 4216 case -911343192: // allowed 4217 this.allowed = castToBoolean(value); // BooleanType 4218 return value; 4219 default: 4220 return super.setProperty(hash, name, value); 4221 } 4222 4223 } 4224 4225 @Override 4226 public Base setProperty(String name, Base value) throws FHIRException { 4227 if (name.equals("type")) { 4228 this.type = castToCodeableConcept(value); // CodeableConcept 4229 } else if (name.equals("allowed")) { 4230 this.allowed = castToBoolean(value); // BooleanType 4231 } else 4232 return super.setProperty(name, value); 4233 return value; 4234 } 4235 4236 @Override 4237 public void removeChild(String name, Base value) throws FHIRException { 4238 if (name.equals("type")) { 4239 this.type = null; 4240 } else if (name.equals("allowed")) { 4241 this.allowed = null; 4242 } else 4243 super.removeChild(name, value); 4244 4245 } 4246 4247 @Override 4248 public Base makeProperty(int hash, String name) throws FHIRException { 4249 switch (hash) { 4250 case 3575610: 4251 return getType(); 4252 case -911343192: 4253 return getAllowedElement(); 4254 default: 4255 return super.makeProperty(hash, name); 4256 } 4257 4258 } 4259 4260 @Override 4261 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4262 switch (hash) { 4263 case 3575610: 4264 /* type */ return new String[] { "CodeableConcept" }; 4265 case -911343192: 4266 /* allowed */ return new String[] { "boolean" }; 4267 default: 4268 return super.getTypesForProperty(hash, name); 4269 } 4270 4271 } 4272 4273 @Override 4274 public Base addChild(String name) throws FHIRException { 4275 if (name.equals("type")) { 4276 this.type = new CodeableConcept(); 4277 return this.type; 4278 } else if (name.equals("allowed")) { 4279 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.allowed"); 4280 } else 4281 return super.addChild(name); 4282 } 4283 4284 public MedicationKnowledgeRegulatorySubstitutionComponent copy() { 4285 MedicationKnowledgeRegulatorySubstitutionComponent dst = new MedicationKnowledgeRegulatorySubstitutionComponent(); 4286 copyValues(dst); 4287 return dst; 4288 } 4289 4290 public void copyValues(MedicationKnowledgeRegulatorySubstitutionComponent dst) { 4291 super.copyValues(dst); 4292 dst.type = type == null ? null : type.copy(); 4293 dst.allowed = allowed == null ? null : allowed.copy(); 4294 } 4295 4296 @Override 4297 public boolean equalsDeep(Base other_) { 4298 if (!super.equalsDeep(other_)) 4299 return false; 4300 if (!(other_ instanceof MedicationKnowledgeRegulatorySubstitutionComponent)) 4301 return false; 4302 MedicationKnowledgeRegulatorySubstitutionComponent o = (MedicationKnowledgeRegulatorySubstitutionComponent) other_; 4303 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true); 4304 } 4305 4306 @Override 4307 public boolean equalsShallow(Base other_) { 4308 if (!super.equalsShallow(other_)) 4309 return false; 4310 if (!(other_ instanceof MedicationKnowledgeRegulatorySubstitutionComponent)) 4311 return false; 4312 MedicationKnowledgeRegulatorySubstitutionComponent o = (MedicationKnowledgeRegulatorySubstitutionComponent) other_; 4313 return compareValues(allowed, o.allowed, true); 4314 } 4315 4316 public boolean isEmpty() { 4317 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed); 4318 } 4319 4320 public String fhirType() { 4321 return "MedicationKnowledge.regulatory.substitution"; 4322 4323 } 4324 4325 } 4326 4327 @Block() 4328 public static class MedicationKnowledgeRegulatoryScheduleComponent extends BackboneElement 4329 implements IBaseBackboneElement { 4330 /** 4331 * Specifies the specific drug schedule. 4332 */ 4333 @Child(name = "schedule", type = { 4334 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4335 @Description(shortDefinition = "Specifies the specific drug schedule", formalDefinition = "Specifies the specific drug schedule.") 4336 protected CodeableConcept schedule; 4337 4338 private static final long serialVersionUID = 1955520912L; 4339 4340 /** 4341 * Constructor 4342 */ 4343 public MedicationKnowledgeRegulatoryScheduleComponent() { 4344 super(); 4345 } 4346 4347 /** 4348 * Constructor 4349 */ 4350 public MedicationKnowledgeRegulatoryScheduleComponent(CodeableConcept schedule) { 4351 super(); 4352 this.schedule = schedule; 4353 } 4354 4355 /** 4356 * @return {@link #schedule} (Specifies the specific drug schedule.) 4357 */ 4358 public CodeableConcept getSchedule() { 4359 if (this.schedule == null) 4360 if (Configuration.errorOnAutoCreate()) 4361 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryScheduleComponent.schedule"); 4362 else if (Configuration.doAutoCreate()) 4363 this.schedule = new CodeableConcept(); // cc 4364 return this.schedule; 4365 } 4366 4367 public boolean hasSchedule() { 4368 return this.schedule != null && !this.schedule.isEmpty(); 4369 } 4370 4371 /** 4372 * @param value {@link #schedule} (Specifies the specific drug schedule.) 4373 */ 4374 public MedicationKnowledgeRegulatoryScheduleComponent setSchedule(CodeableConcept value) { 4375 this.schedule = value; 4376 return this; 4377 } 4378 4379 protected void listChildren(List<Property> children) { 4380 super.listChildren(children); 4381 children 4382 .add(new Property("schedule", "CodeableConcept", "Specifies the specific drug schedule.", 0, 1, schedule)); 4383 } 4384 4385 @Override 4386 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4387 switch (_hash) { 4388 case -697920873: 4389 /* schedule */ return new Property("schedule", "CodeableConcept", "Specifies the specific drug schedule.", 0, 1, 4390 schedule); 4391 default: 4392 return super.getNamedProperty(_hash, _name, _checkValid); 4393 } 4394 4395 } 4396 4397 @Override 4398 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4399 switch (hash) { 4400 case -697920873: 4401 /* schedule */ return this.schedule == null ? new Base[0] : new Base[] { this.schedule }; // CodeableConcept 4402 default: 4403 return super.getProperty(hash, name, checkValid); 4404 } 4405 4406 } 4407 4408 @Override 4409 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4410 switch (hash) { 4411 case -697920873: // schedule 4412 this.schedule = castToCodeableConcept(value); // CodeableConcept 4413 return value; 4414 default: 4415 return super.setProperty(hash, name, value); 4416 } 4417 4418 } 4419 4420 @Override 4421 public Base setProperty(String name, Base value) throws FHIRException { 4422 if (name.equals("schedule")) { 4423 this.schedule = castToCodeableConcept(value); // CodeableConcept 4424 } else 4425 return super.setProperty(name, value); 4426 return value; 4427 } 4428 4429 @Override 4430 public void removeChild(String name, Base value) throws FHIRException { 4431 if (name.equals("schedule")) { 4432 this.schedule = null; 4433 } else 4434 super.removeChild(name, value); 4435 4436 } 4437 4438 @Override 4439 public Base makeProperty(int hash, String name) throws FHIRException { 4440 switch (hash) { 4441 case -697920873: 4442 return getSchedule(); 4443 default: 4444 return super.makeProperty(hash, name); 4445 } 4446 4447 } 4448 4449 @Override 4450 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4451 switch (hash) { 4452 case -697920873: 4453 /* schedule */ return new String[] { "CodeableConcept" }; 4454 default: 4455 return super.getTypesForProperty(hash, name); 4456 } 4457 4458 } 4459 4460 @Override 4461 public Base addChild(String name) throws FHIRException { 4462 if (name.equals("schedule")) { 4463 this.schedule = new CodeableConcept(); 4464 return this.schedule; 4465 } else 4466 return super.addChild(name); 4467 } 4468 4469 public MedicationKnowledgeRegulatoryScheduleComponent copy() { 4470 MedicationKnowledgeRegulatoryScheduleComponent dst = new MedicationKnowledgeRegulatoryScheduleComponent(); 4471 copyValues(dst); 4472 return dst; 4473 } 4474 4475 public void copyValues(MedicationKnowledgeRegulatoryScheduleComponent dst) { 4476 super.copyValues(dst); 4477 dst.schedule = schedule == null ? null : schedule.copy(); 4478 } 4479 4480 @Override 4481 public boolean equalsDeep(Base other_) { 4482 if (!super.equalsDeep(other_)) 4483 return false; 4484 if (!(other_ instanceof MedicationKnowledgeRegulatoryScheduleComponent)) 4485 return false; 4486 MedicationKnowledgeRegulatoryScheduleComponent o = (MedicationKnowledgeRegulatoryScheduleComponent) other_; 4487 return compareDeep(schedule, o.schedule, true); 4488 } 4489 4490 @Override 4491 public boolean equalsShallow(Base other_) { 4492 if (!super.equalsShallow(other_)) 4493 return false; 4494 if (!(other_ instanceof MedicationKnowledgeRegulatoryScheduleComponent)) 4495 return false; 4496 MedicationKnowledgeRegulatoryScheduleComponent o = (MedicationKnowledgeRegulatoryScheduleComponent) other_; 4497 return true; 4498 } 4499 4500 public boolean isEmpty() { 4501 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(schedule); 4502 } 4503 4504 public String fhirType() { 4505 return "MedicationKnowledge.regulatory.schedule"; 4506 4507 } 4508 4509 } 4510 4511 @Block() 4512 public static class MedicationKnowledgeRegulatoryMaxDispenseComponent extends BackboneElement 4513 implements IBaseBackboneElement { 4514 /** 4515 * The maximum number of units of the medication that can be dispensed. 4516 */ 4517 @Child(name = "quantity", type = { Quantity.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4518 @Description(shortDefinition = "The maximum number of units of the medication that can be dispensed", formalDefinition = "The maximum number of units of the medication that can be dispensed.") 4519 protected Quantity quantity; 4520 4521 /** 4522 * The period that applies to the maximum number of units. 4523 */ 4524 @Child(name = "period", type = { Duration.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4525 @Description(shortDefinition = "The period that applies to the maximum number of units", formalDefinition = "The period that applies to the maximum number of units.") 4526 protected Duration period; 4527 4528 private static final long serialVersionUID = -441724185L; 4529 4530 /** 4531 * Constructor 4532 */ 4533 public MedicationKnowledgeRegulatoryMaxDispenseComponent() { 4534 super(); 4535 } 4536 4537 /** 4538 * Constructor 4539 */ 4540 public MedicationKnowledgeRegulatoryMaxDispenseComponent(Quantity quantity) { 4541 super(); 4542 this.quantity = quantity; 4543 } 4544 4545 /** 4546 * @return {@link #quantity} (The maximum number of units of the medication that 4547 * can be dispensed.) 4548 */ 4549 public Quantity getQuantity() { 4550 if (this.quantity == null) 4551 if (Configuration.errorOnAutoCreate()) 4552 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryMaxDispenseComponent.quantity"); 4553 else if (Configuration.doAutoCreate()) 4554 this.quantity = new Quantity(); // cc 4555 return this.quantity; 4556 } 4557 4558 public boolean hasQuantity() { 4559 return this.quantity != null && !this.quantity.isEmpty(); 4560 } 4561 4562 /** 4563 * @param value {@link #quantity} (The maximum number of units of the medication 4564 * that can be dispensed.) 4565 */ 4566 public MedicationKnowledgeRegulatoryMaxDispenseComponent setQuantity(Quantity value) { 4567 this.quantity = value; 4568 return this; 4569 } 4570 4571 /** 4572 * @return {@link #period} (The period that applies to the maximum number of 4573 * units.) 4574 */ 4575 public Duration getPeriod() { 4576 if (this.period == null) 4577 if (Configuration.errorOnAutoCreate()) 4578 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryMaxDispenseComponent.period"); 4579 else if (Configuration.doAutoCreate()) 4580 this.period = new Duration(); // cc 4581 return this.period; 4582 } 4583 4584 public boolean hasPeriod() { 4585 return this.period != null && !this.period.isEmpty(); 4586 } 4587 4588 /** 4589 * @param value {@link #period} (The period that applies to the maximum number 4590 * of units.) 4591 */ 4592 public MedicationKnowledgeRegulatoryMaxDispenseComponent setPeriod(Duration value) { 4593 this.period = value; 4594 return this; 4595 } 4596 4597 protected void listChildren(List<Property> children) { 4598 super.listChildren(children); 4599 children.add(new Property("quantity", "SimpleQuantity", 4600 "The maximum number of units of the medication that can be dispensed.", 0, 1, quantity)); 4601 children.add( 4602 new Property("period", "Duration", "The period that applies to the maximum number of units.", 0, 1, period)); 4603 } 4604 4605 @Override 4606 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4607 switch (_hash) { 4608 case -1285004149: 4609 /* quantity */ return new Property("quantity", "SimpleQuantity", 4610 "The maximum number of units of the medication that can be dispensed.", 0, 1, quantity); 4611 case -991726143: 4612 /* period */ return new Property("period", "Duration", 4613 "The period that applies to the maximum number of units.", 0, 1, period); 4614 default: 4615 return super.getNamedProperty(_hash, _name, _checkValid); 4616 } 4617 4618 } 4619 4620 @Override 4621 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4622 switch (hash) { 4623 case -1285004149: 4624 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 4625 case -991726143: 4626 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Duration 4627 default: 4628 return super.getProperty(hash, name, checkValid); 4629 } 4630 4631 } 4632 4633 @Override 4634 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4635 switch (hash) { 4636 case -1285004149: // quantity 4637 this.quantity = castToQuantity(value); // Quantity 4638 return value; 4639 case -991726143: // period 4640 this.period = castToDuration(value); // Duration 4641 return value; 4642 default: 4643 return super.setProperty(hash, name, value); 4644 } 4645 4646 } 4647 4648 @Override 4649 public Base setProperty(String name, Base value) throws FHIRException { 4650 if (name.equals("quantity")) { 4651 this.quantity = castToQuantity(value); // Quantity 4652 } else if (name.equals("period")) { 4653 this.period = castToDuration(value); // Duration 4654 } else 4655 return super.setProperty(name, value); 4656 return value; 4657 } 4658 4659 @Override 4660 public void removeChild(String name, Base value) throws FHIRException { 4661 if (name.equals("quantity")) { 4662 this.quantity = null; 4663 } else if (name.equals("period")) { 4664 this.period = null; 4665 } else 4666 super.removeChild(name, value); 4667 4668 } 4669 4670 @Override 4671 public Base makeProperty(int hash, String name) throws FHIRException { 4672 switch (hash) { 4673 case -1285004149: 4674 return getQuantity(); 4675 case -991726143: 4676 return getPeriod(); 4677 default: 4678 return super.makeProperty(hash, name); 4679 } 4680 4681 } 4682 4683 @Override 4684 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4685 switch (hash) { 4686 case -1285004149: 4687 /* quantity */ return new String[] { "SimpleQuantity" }; 4688 case -991726143: 4689 /* period */ return new String[] { "Duration" }; 4690 default: 4691 return super.getTypesForProperty(hash, name); 4692 } 4693 4694 } 4695 4696 @Override 4697 public Base addChild(String name) throws FHIRException { 4698 if (name.equals("quantity")) { 4699 this.quantity = new Quantity(); 4700 return this.quantity; 4701 } else if (name.equals("period")) { 4702 this.period = new Duration(); 4703 return this.period; 4704 } else 4705 return super.addChild(name); 4706 } 4707 4708 public MedicationKnowledgeRegulatoryMaxDispenseComponent copy() { 4709 MedicationKnowledgeRegulatoryMaxDispenseComponent dst = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); 4710 copyValues(dst); 4711 return dst; 4712 } 4713 4714 public void copyValues(MedicationKnowledgeRegulatoryMaxDispenseComponent dst) { 4715 super.copyValues(dst); 4716 dst.quantity = quantity == null ? null : quantity.copy(); 4717 dst.period = period == null ? null : period.copy(); 4718 } 4719 4720 @Override 4721 public boolean equalsDeep(Base other_) { 4722 if (!super.equalsDeep(other_)) 4723 return false; 4724 if (!(other_ instanceof MedicationKnowledgeRegulatoryMaxDispenseComponent)) 4725 return false; 4726 MedicationKnowledgeRegulatoryMaxDispenseComponent o = (MedicationKnowledgeRegulatoryMaxDispenseComponent) other_; 4727 return compareDeep(quantity, o.quantity, true) && compareDeep(period, o.period, true); 4728 } 4729 4730 @Override 4731 public boolean equalsShallow(Base other_) { 4732 if (!super.equalsShallow(other_)) 4733 return false; 4734 if (!(other_ instanceof MedicationKnowledgeRegulatoryMaxDispenseComponent)) 4735 return false; 4736 MedicationKnowledgeRegulatoryMaxDispenseComponent o = (MedicationKnowledgeRegulatoryMaxDispenseComponent) other_; 4737 return true; 4738 } 4739 4740 public boolean isEmpty() { 4741 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, period); 4742 } 4743 4744 public String fhirType() { 4745 return "MedicationKnowledge.regulatory.maxDispense"; 4746 4747 } 4748 4749 } 4750 4751 @Block() 4752 public static class MedicationKnowledgeKineticsComponent extends BackboneElement implements IBaseBackboneElement { 4753 /** 4754 * The drug concentration measured at certain discrete points in time. 4755 */ 4756 @Child(name = "areaUnderCurve", type = { 4757 Quantity.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4758 @Description(shortDefinition = "The drug concentration measured at certain discrete points in time", formalDefinition = "The drug concentration measured at certain discrete points in time.") 4759 protected List<Quantity> areaUnderCurve; 4760 4761 /** 4762 * The median lethal dose of a drug. 4763 */ 4764 @Child(name = "lethalDose50", type = { 4765 Quantity.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4766 @Description(shortDefinition = "The median lethal dose of a drug", formalDefinition = "The median lethal dose of a drug.") 4767 protected List<Quantity> lethalDose50; 4768 4769 /** 4770 * The time required for any specified property (e.g., the concentration of a 4771 * substance in the body) to decrease by half. 4772 */ 4773 @Child(name = "halfLifePeriod", type = { 4774 Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 4775 @Description(shortDefinition = "Time required for concentration in the body to decrease by half", formalDefinition = "The time required for any specified property (e.g., the concentration of a substance in the body) to decrease by half.") 4776 protected Duration halfLifePeriod; 4777 4778 private static final long serialVersionUID = -206244264L; 4779 4780 /** 4781 * Constructor 4782 */ 4783 public MedicationKnowledgeKineticsComponent() { 4784 super(); 4785 } 4786 4787 /** 4788 * @return {@link #areaUnderCurve} (The drug concentration measured at certain 4789 * discrete points in time.) 4790 */ 4791 public List<Quantity> getAreaUnderCurve() { 4792 if (this.areaUnderCurve == null) 4793 this.areaUnderCurve = new ArrayList<Quantity>(); 4794 return this.areaUnderCurve; 4795 } 4796 4797 /** 4798 * @return Returns a reference to <code>this</code> for easy method chaining 4799 */ 4800 public MedicationKnowledgeKineticsComponent setAreaUnderCurve(List<Quantity> theAreaUnderCurve) { 4801 this.areaUnderCurve = theAreaUnderCurve; 4802 return this; 4803 } 4804 4805 public boolean hasAreaUnderCurve() { 4806 if (this.areaUnderCurve == null) 4807 return false; 4808 for (Quantity item : this.areaUnderCurve) 4809 if (!item.isEmpty()) 4810 return true; 4811 return false; 4812 } 4813 4814 public Quantity addAreaUnderCurve() { // 3 4815 Quantity t = new Quantity(); 4816 if (this.areaUnderCurve == null) 4817 this.areaUnderCurve = new ArrayList<Quantity>(); 4818 this.areaUnderCurve.add(t); 4819 return t; 4820 } 4821 4822 public MedicationKnowledgeKineticsComponent addAreaUnderCurve(Quantity t) { // 3 4823 if (t == null) 4824 return this; 4825 if (this.areaUnderCurve == null) 4826 this.areaUnderCurve = new ArrayList<Quantity>(); 4827 this.areaUnderCurve.add(t); 4828 return this; 4829 } 4830 4831 /** 4832 * @return The first repetition of repeating field {@link #areaUnderCurve}, 4833 * creating it if it does not already exist 4834 */ 4835 public Quantity getAreaUnderCurveFirstRep() { 4836 if (getAreaUnderCurve().isEmpty()) { 4837 addAreaUnderCurve(); 4838 } 4839 return getAreaUnderCurve().get(0); 4840 } 4841 4842 /** 4843 * @return {@link #lethalDose50} (The median lethal dose of a drug.) 4844 */ 4845 public List<Quantity> getLethalDose50() { 4846 if (this.lethalDose50 == null) 4847 this.lethalDose50 = new ArrayList<Quantity>(); 4848 return this.lethalDose50; 4849 } 4850 4851 /** 4852 * @return Returns a reference to <code>this</code> for easy method chaining 4853 */ 4854 public MedicationKnowledgeKineticsComponent setLethalDose50(List<Quantity> theLethalDose50) { 4855 this.lethalDose50 = theLethalDose50; 4856 return this; 4857 } 4858 4859 public boolean hasLethalDose50() { 4860 if (this.lethalDose50 == null) 4861 return false; 4862 for (Quantity item : this.lethalDose50) 4863 if (!item.isEmpty()) 4864 return true; 4865 return false; 4866 } 4867 4868 public Quantity addLethalDose50() { // 3 4869 Quantity t = new Quantity(); 4870 if (this.lethalDose50 == null) 4871 this.lethalDose50 = new ArrayList<Quantity>(); 4872 this.lethalDose50.add(t); 4873 return t; 4874 } 4875 4876 public MedicationKnowledgeKineticsComponent addLethalDose50(Quantity t) { // 3 4877 if (t == null) 4878 return this; 4879 if (this.lethalDose50 == null) 4880 this.lethalDose50 = new ArrayList<Quantity>(); 4881 this.lethalDose50.add(t); 4882 return this; 4883 } 4884 4885 /** 4886 * @return The first repetition of repeating field {@link #lethalDose50}, 4887 * creating it if it does not already exist 4888 */ 4889 public Quantity getLethalDose50FirstRep() { 4890 if (getLethalDose50().isEmpty()) { 4891 addLethalDose50(); 4892 } 4893 return getLethalDose50().get(0); 4894 } 4895 4896 /** 4897 * @return {@link #halfLifePeriod} (The time required for any specified property 4898 * (e.g., the concentration of a substance in the body) to decrease by 4899 * half.) 4900 */ 4901 public Duration getHalfLifePeriod() { 4902 if (this.halfLifePeriod == null) 4903 if (Configuration.errorOnAutoCreate()) 4904 throw new Error("Attempt to auto-create MedicationKnowledgeKineticsComponent.halfLifePeriod"); 4905 else if (Configuration.doAutoCreate()) 4906 this.halfLifePeriod = new Duration(); // cc 4907 return this.halfLifePeriod; 4908 } 4909 4910 public boolean hasHalfLifePeriod() { 4911 return this.halfLifePeriod != null && !this.halfLifePeriod.isEmpty(); 4912 } 4913 4914 /** 4915 * @param value {@link #halfLifePeriod} (The time required for any specified 4916 * property (e.g., the concentration of a substance in the body) to 4917 * decrease by half.) 4918 */ 4919 public MedicationKnowledgeKineticsComponent setHalfLifePeriod(Duration value) { 4920 this.halfLifePeriod = value; 4921 return this; 4922 } 4923 4924 protected void listChildren(List<Property> children) { 4925 super.listChildren(children); 4926 children.add(new Property("areaUnderCurve", "SimpleQuantity", 4927 "The drug concentration measured at certain discrete points in time.", 0, java.lang.Integer.MAX_VALUE, 4928 areaUnderCurve)); 4929 children.add(new Property("lethalDose50", "SimpleQuantity", "The median lethal dose of a drug.", 0, 4930 java.lang.Integer.MAX_VALUE, lethalDose50)); 4931 children.add(new Property("halfLifePeriod", "Duration", 4932 "The time required for any specified property (e.g., the concentration of a substance in the body) to decrease by half.", 4933 0, 1, halfLifePeriod)); 4934 } 4935 4936 @Override 4937 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4938 switch (_hash) { 4939 case 1243936100: 4940 /* areaUnderCurve */ return new Property("areaUnderCurve", "SimpleQuantity", 4941 "The drug concentration measured at certain discrete points in time.", 0, java.lang.Integer.MAX_VALUE, 4942 areaUnderCurve); 4943 case 302983216: 4944 /* lethalDose50 */ return new Property("lethalDose50", "SimpleQuantity", "The median lethal dose of a drug.", 0, 4945 java.lang.Integer.MAX_VALUE, lethalDose50); 4946 case -628810640: 4947 /* halfLifePeriod */ return new Property("halfLifePeriod", "Duration", 4948 "The time required for any specified property (e.g., the concentration of a substance in the body) to decrease by half.", 4949 0, 1, halfLifePeriod); 4950 default: 4951 return super.getNamedProperty(_hash, _name, _checkValid); 4952 } 4953 4954 } 4955 4956 @Override 4957 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4958 switch (hash) { 4959 case 1243936100: 4960 /* areaUnderCurve */ return this.areaUnderCurve == null ? new Base[0] 4961 : this.areaUnderCurve.toArray(new Base[this.areaUnderCurve.size()]); // Quantity 4962 case 302983216: 4963 /* lethalDose50 */ return this.lethalDose50 == null ? new Base[0] 4964 : this.lethalDose50.toArray(new Base[this.lethalDose50.size()]); // Quantity 4965 case -628810640: 4966 /* halfLifePeriod */ return this.halfLifePeriod == null ? new Base[0] : new Base[] { this.halfLifePeriod }; // Duration 4967 default: 4968 return super.getProperty(hash, name, checkValid); 4969 } 4970 4971 } 4972 4973 @Override 4974 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4975 switch (hash) { 4976 case 1243936100: // areaUnderCurve 4977 this.getAreaUnderCurve().add(castToQuantity(value)); // Quantity 4978 return value; 4979 case 302983216: // lethalDose50 4980 this.getLethalDose50().add(castToQuantity(value)); // Quantity 4981 return value; 4982 case -628810640: // halfLifePeriod 4983 this.halfLifePeriod = castToDuration(value); // Duration 4984 return value; 4985 default: 4986 return super.setProperty(hash, name, value); 4987 } 4988 4989 } 4990 4991 @Override 4992 public Base setProperty(String name, Base value) throws FHIRException { 4993 if (name.equals("areaUnderCurve")) { 4994 this.getAreaUnderCurve().add(castToQuantity(value)); 4995 } else if (name.equals("lethalDose50")) { 4996 this.getLethalDose50().add(castToQuantity(value)); 4997 } else if (name.equals("halfLifePeriod")) { 4998 this.halfLifePeriod = castToDuration(value); // Duration 4999 } else 5000 return super.setProperty(name, value); 5001 return value; 5002 } 5003 5004 @Override 5005 public void removeChild(String name, Base value) throws FHIRException { 5006 if (name.equals("areaUnderCurve")) { 5007 this.getAreaUnderCurve().remove(castToQuantity(value)); 5008 } else if (name.equals("lethalDose50")) { 5009 this.getLethalDose50().remove(castToQuantity(value)); 5010 } else if (name.equals("halfLifePeriod")) { 5011 this.halfLifePeriod = null; 5012 } else 5013 super.removeChild(name, value); 5014 5015 } 5016 5017 @Override 5018 public Base makeProperty(int hash, String name) throws FHIRException { 5019 switch (hash) { 5020 case 1243936100: 5021 return addAreaUnderCurve(); 5022 case 302983216: 5023 return addLethalDose50(); 5024 case -628810640: 5025 return getHalfLifePeriod(); 5026 default: 5027 return super.makeProperty(hash, name); 5028 } 5029 5030 } 5031 5032 @Override 5033 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5034 switch (hash) { 5035 case 1243936100: 5036 /* areaUnderCurve */ return new String[] { "SimpleQuantity" }; 5037 case 302983216: 5038 /* lethalDose50 */ return new String[] { "SimpleQuantity" }; 5039 case -628810640: 5040 /* halfLifePeriod */ return new String[] { "Duration" }; 5041 default: 5042 return super.getTypesForProperty(hash, name); 5043 } 5044 5045 } 5046 5047 @Override 5048 public Base addChild(String name) throws FHIRException { 5049 if (name.equals("areaUnderCurve")) { 5050 return addAreaUnderCurve(); 5051 } else if (name.equals("lethalDose50")) { 5052 return addLethalDose50(); 5053 } else if (name.equals("halfLifePeriod")) { 5054 this.halfLifePeriod = new Duration(); 5055 return this.halfLifePeriod; 5056 } else 5057 return super.addChild(name); 5058 } 5059 5060 public MedicationKnowledgeKineticsComponent copy() { 5061 MedicationKnowledgeKineticsComponent dst = new MedicationKnowledgeKineticsComponent(); 5062 copyValues(dst); 5063 return dst; 5064 } 5065 5066 public void copyValues(MedicationKnowledgeKineticsComponent dst) { 5067 super.copyValues(dst); 5068 if (areaUnderCurve != null) { 5069 dst.areaUnderCurve = new ArrayList<Quantity>(); 5070 for (Quantity i : areaUnderCurve) 5071 dst.areaUnderCurve.add(i.copy()); 5072 } 5073 ; 5074 if (lethalDose50 != null) { 5075 dst.lethalDose50 = new ArrayList<Quantity>(); 5076 for (Quantity i : lethalDose50) 5077 dst.lethalDose50.add(i.copy()); 5078 } 5079 ; 5080 dst.halfLifePeriod = halfLifePeriod == null ? null : halfLifePeriod.copy(); 5081 } 5082 5083 @Override 5084 public boolean equalsDeep(Base other_) { 5085 if (!super.equalsDeep(other_)) 5086 return false; 5087 if (!(other_ instanceof MedicationKnowledgeKineticsComponent)) 5088 return false; 5089 MedicationKnowledgeKineticsComponent o = (MedicationKnowledgeKineticsComponent) other_; 5090 return compareDeep(areaUnderCurve, o.areaUnderCurve, true) && compareDeep(lethalDose50, o.lethalDose50, true) 5091 && compareDeep(halfLifePeriod, o.halfLifePeriod, true); 5092 } 5093 5094 @Override 5095 public boolean equalsShallow(Base other_) { 5096 if (!super.equalsShallow(other_)) 5097 return false; 5098 if (!(other_ instanceof MedicationKnowledgeKineticsComponent)) 5099 return false; 5100 MedicationKnowledgeKineticsComponent o = (MedicationKnowledgeKineticsComponent) other_; 5101 return true; 5102 } 5103 5104 public boolean isEmpty() { 5105 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(areaUnderCurve, lethalDose50, halfLifePeriod); 5106 } 5107 5108 public String fhirType() { 5109 return "MedicationKnowledge.kinetics"; 5110 5111 } 5112 5113 } 5114 5115 /** 5116 * A code that specifies this medication, or a textual description if no code is 5117 * available. Usage note: This could be a standard medication code such as a 5118 * code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local 5119 * formulary code, optionally with translations to other code systems. 5120 */ 5121 @Child(name = "code", type = { CodeableConcept.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 5122 @Description(shortDefinition = "Code that identifies this medication", formalDefinition = "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.") 5123 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 5124 protected CodeableConcept code; 5125 5126 /** 5127 * A code to indicate if the medication is in active use. The status refers to 5128 * the validity about the information of the medication and not to its medicinal 5129 * properties. 5130 */ 5131 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 5132 @Description(shortDefinition = "active | inactive | entered-in-error", formalDefinition = "A code to indicate if the medication is in active use. The status refers to the validity about the information of the medication and not to its medicinal properties.") 5133 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationknowledge-status") 5134 protected Enumeration<MedicationKnowledgeStatus> status; 5135 5136 /** 5137 * Describes the details of the manufacturer of the medication product. This is 5138 * not intended to represent the distributor of a medication product. 5139 */ 5140 @Child(name = "manufacturer", type = { 5141 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5142 @Description(shortDefinition = "Manufacturer of the item", formalDefinition = "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.") 5143 protected Reference manufacturer; 5144 5145 /** 5146 * The actual object that is the target of the reference (Describes the details 5147 * of the manufacturer of the medication product. This is not intended to 5148 * represent the distributor of a medication product.) 5149 */ 5150 protected Organization manufacturerTarget; 5151 5152 /** 5153 * Describes the form of the item. Powder; tablets; capsule. 5154 */ 5155 @Child(name = "doseForm", type = { 5156 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 5157 @Description(shortDefinition = "powder | tablets | capsule +", formalDefinition = "Describes the form of the item. Powder; tablets; capsule.") 5158 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-form-codes") 5159 protected CodeableConcept doseForm; 5160 5161 /** 5162 * Specific amount of the drug in the packaged product. For example, when 5163 * specifying a product that has the same strength (For example, Insulin 5164 * glargine 100 unit per mL solution for injection), this attribute provides 5165 * additional clarification of the package amount (For example, 3 mL, 10mL, 5166 * etc.). 5167 */ 5168 @Child(name = "amount", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 5169 @Description(shortDefinition = "Amount of drug in package", formalDefinition = "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).") 5170 protected Quantity amount; 5171 5172 /** 5173 * Additional names for a medication, for example, the name(s) given to a 5174 * medication in different countries. For example, acetaminophen and paracetamol 5175 * or salbutamol and albuterol. 5176 */ 5177 @Child(name = "synonym", type = { 5178 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 5179 @Description(shortDefinition = "Additional names for a medication", formalDefinition = "Additional names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.") 5180 protected List<StringType> synonym; 5181 5182 /** 5183 * Associated or related knowledge about a medication. 5184 */ 5185 @Child(name = "relatedMedicationKnowledge", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5186 @Description(shortDefinition = "Associated or related medication information", formalDefinition = "Associated or related knowledge about a medication.") 5187 protected List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> relatedMedicationKnowledge; 5188 5189 /** 5190 * Associated or related medications. For example, if the medication is a 5191 * branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. 5192 * Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this 5193 * would link to a branded product (e.g. Crestor). 5194 */ 5195 @Child(name = "associatedMedication", type = { 5196 Medication.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5197 @Description(shortDefinition = "A medication resource that is associated with this medication", formalDefinition = "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor).") 5198 protected List<Reference> associatedMedication; 5199 /** 5200 * The actual objects that are the target of the reference (Associated or 5201 * related medications. For example, if the medication is a branded product 5202 * (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this 5203 * is a generic medication (e.g. Rosuvastatin), this would link to a branded 5204 * product (e.g. Crestor).) 5205 */ 5206 protected List<Medication> associatedMedicationTarget; 5207 5208 /** 5209 * Category of the medication or product (e.g. branded product, therapeutic 5210 * moeity, generic product, innovator product, etc.). 5211 */ 5212 @Child(name = "productType", type = { 5213 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5214 @Description(shortDefinition = "Category of the medication or product", formalDefinition = "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).") 5215 protected List<CodeableConcept> productType; 5216 5217 /** 5218 * Associated documentation about the medication. 5219 */ 5220 @Child(name = "monograph", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5221 @Description(shortDefinition = "Associated documentation about the medication", formalDefinition = "Associated documentation about the medication.") 5222 protected List<MedicationKnowledgeMonographComponent> monograph; 5223 5224 /** 5225 * Identifies a particular constituent of interest in the product. 5226 */ 5227 @Child(name = "ingredient", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5228 @Description(shortDefinition = "Active or inactive ingredient", formalDefinition = "Identifies a particular constituent of interest in the product.") 5229 protected List<MedicationKnowledgeIngredientComponent> ingredient; 5230 5231 /** 5232 * The instructions for preparing the medication. 5233 */ 5234 @Child(name = "preparationInstruction", type = { 5235 MarkdownType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 5236 @Description(shortDefinition = "The instructions for preparing the medication", formalDefinition = "The instructions for preparing the medication.") 5237 protected MarkdownType preparationInstruction; 5238 5239 /** 5240 * The intended or approved route of administration. 5241 */ 5242 @Child(name = "intendedRoute", type = { 5243 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5244 @Description(shortDefinition = "The intended or approved route of administration", formalDefinition = "The intended or approved route of administration.") 5245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/route-codes") 5246 protected List<CodeableConcept> intendedRoute; 5247 5248 /** 5249 * The price of the medication. 5250 */ 5251 @Child(name = "cost", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5252 @Description(shortDefinition = "The pricing of the medication", formalDefinition = "The price of the medication.") 5253 protected List<MedicationKnowledgeCostComponent> cost; 5254 5255 /** 5256 * The program under which the medication is reviewed. 5257 */ 5258 @Child(name = "monitoringProgram", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5259 @Description(shortDefinition = "Program under which a medication is reviewed", formalDefinition = "The program under which the medication is reviewed.") 5260 protected List<MedicationKnowledgeMonitoringProgramComponent> monitoringProgram; 5261 5262 /** 5263 * Guidelines for the administration of the medication. 5264 */ 5265 @Child(name = "administrationGuidelines", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5266 @Description(shortDefinition = "Guidelines for administration of the medication", formalDefinition = "Guidelines for the administration of the medication.") 5267 protected List<MedicationKnowledgeAdministrationGuidelinesComponent> administrationGuidelines; 5268 5269 /** 5270 * Categorization of the medication within a formulary or classification system. 5271 */ 5272 @Child(name = "medicineClassification", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5273 @Description(shortDefinition = "Categorization of the medication within a formulary or classification system", formalDefinition = "Categorization of the medication within a formulary or classification system.") 5274 protected List<MedicationKnowledgeMedicineClassificationComponent> medicineClassification; 5275 5276 /** 5277 * Information that only applies to packages (not products). 5278 */ 5279 @Child(name = "packaging", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = false) 5280 @Description(shortDefinition = "Details about packaged medications", formalDefinition = "Information that only applies to packages (not products).") 5281 protected MedicationKnowledgePackagingComponent packaging; 5282 5283 /** 5284 * Specifies descriptive properties of the medicine, such as color, shape, 5285 * imprints, etc. 5286 */ 5287 @Child(name = "drugCharacteristic", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5288 @Description(shortDefinition = "Specifies descriptive properties of the medicine", formalDefinition = "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.") 5289 protected List<MedicationKnowledgeDrugCharacteristicComponent> drugCharacteristic; 5290 5291 /** 5292 * Potential clinical issue with or between medication(s) (for example, 5293 * drug-drug interaction, drug-disease contraindication, drug-allergy 5294 * interaction, etc.). 5295 */ 5296 @Child(name = "contraindication", type = { 5297 DetectedIssue.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5298 @Description(shortDefinition = "Potential clinical issue with or between medication(s)", formalDefinition = "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).") 5299 protected List<Reference> contraindication; 5300 /** 5301 * The actual objects that are the target of the reference (Potential clinical 5302 * issue with or between medication(s) (for example, drug-drug interaction, 5303 * drug-disease contraindication, drug-allergy interaction, etc.).) 5304 */ 5305 protected List<DetectedIssue> contraindicationTarget; 5306 5307 /** 5308 * Regulatory information about a medication. 5309 */ 5310 @Child(name = "regulatory", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5311 @Description(shortDefinition = "Regulatory information about a medication", formalDefinition = "Regulatory information about a medication.") 5312 protected List<MedicationKnowledgeRegulatoryComponent> regulatory; 5313 5314 /** 5315 * The time course of drug absorption, distribution, metabolism and excretion of 5316 * a medication from the body. 5317 */ 5318 @Child(name = "kinetics", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5319 @Description(shortDefinition = "The time course of drug absorption, distribution, metabolism and excretion of a medication from the body", formalDefinition = "The time course of drug absorption, distribution, metabolism and excretion of a medication from the body.") 5320 protected List<MedicationKnowledgeKineticsComponent> kinetics; 5321 5322 private static final long serialVersionUID = -1230067857L; 5323 5324 /** 5325 * Constructor 5326 */ 5327 public MedicationKnowledge() { 5328 super(); 5329 } 5330 5331 /** 5332 * @return {@link #code} (A code that specifies this medication, or a textual 5333 * description if no code is available. Usage note: This could be a 5334 * standard medication code such as a code from RxNorm, SNOMED CT, IDMP 5335 * etc. It could also be a national or local formulary code, optionally 5336 * with translations to other code systems.) 5337 */ 5338 public CodeableConcept getCode() { 5339 if (this.code == null) 5340 if (Configuration.errorOnAutoCreate()) 5341 throw new Error("Attempt to auto-create MedicationKnowledge.code"); 5342 else if (Configuration.doAutoCreate()) 5343 this.code = new CodeableConcept(); // cc 5344 return this.code; 5345 } 5346 5347 public boolean hasCode() { 5348 return this.code != null && !this.code.isEmpty(); 5349 } 5350 5351 /** 5352 * @param value {@link #code} (A code that specifies this medication, or a 5353 * textual description if no code is available. Usage note: This 5354 * could be a standard medication code such as a code from RxNorm, 5355 * SNOMED CT, IDMP etc. It could also be a national or local 5356 * formulary code, optionally with translations to other code 5357 * systems.) 5358 */ 5359 public MedicationKnowledge setCode(CodeableConcept value) { 5360 this.code = value; 5361 return this; 5362 } 5363 5364 /** 5365 * @return {@link #status} (A code to indicate if the medication is in active 5366 * use. The status refers to the validity about the information of the 5367 * medication and not to its medicinal properties.). This is the 5368 * underlying object with id, value and extensions. The accessor 5369 * "getStatus" gives direct access to the value 5370 */ 5371 public Enumeration<MedicationKnowledgeStatus> getStatusElement() { 5372 if (this.status == null) 5373 if (Configuration.errorOnAutoCreate()) 5374 throw new Error("Attempt to auto-create MedicationKnowledge.status"); 5375 else if (Configuration.doAutoCreate()) 5376 this.status = new Enumeration<MedicationKnowledgeStatus>(new MedicationKnowledgeStatusEnumFactory()); // bb 5377 return this.status; 5378 } 5379 5380 public boolean hasStatusElement() { 5381 return this.status != null && !this.status.isEmpty(); 5382 } 5383 5384 public boolean hasStatus() { 5385 return this.status != null && !this.status.isEmpty(); 5386 } 5387 5388 /** 5389 * @param value {@link #status} (A code to indicate if the medication is in 5390 * active use. The status refers to the validity about the 5391 * information of the medication and not to its medicinal 5392 * properties.). This is the underlying object with id, value and 5393 * extensions. The accessor "getStatus" gives direct access to the 5394 * value 5395 */ 5396 public MedicationKnowledge setStatusElement(Enumeration<MedicationKnowledgeStatus> value) { 5397 this.status = value; 5398 return this; 5399 } 5400 5401 /** 5402 * @return A code to indicate if the medication is in active use. The status 5403 * refers to the validity about the information of the medication and 5404 * not to its medicinal properties. 5405 */ 5406 public MedicationKnowledgeStatus getStatus() { 5407 return this.status == null ? null : this.status.getValue(); 5408 } 5409 5410 /** 5411 * @param value A code to indicate if the medication is in active use. The 5412 * status refers to the validity about the information of the 5413 * medication and not to its medicinal properties. 5414 */ 5415 public MedicationKnowledge setStatus(MedicationKnowledgeStatus value) { 5416 if (value == null) 5417 this.status = null; 5418 else { 5419 if (this.status == null) 5420 this.status = new Enumeration<MedicationKnowledgeStatus>(new MedicationKnowledgeStatusEnumFactory()); 5421 this.status.setValue(value); 5422 } 5423 return this; 5424 } 5425 5426 /** 5427 * @return {@link #manufacturer} (Describes the details of the manufacturer of 5428 * the medication product. This is not intended to represent the 5429 * distributor of a medication product.) 5430 */ 5431 public Reference getManufacturer() { 5432 if (this.manufacturer == null) 5433 if (Configuration.errorOnAutoCreate()) 5434 throw new Error("Attempt to auto-create MedicationKnowledge.manufacturer"); 5435 else if (Configuration.doAutoCreate()) 5436 this.manufacturer = new Reference(); // cc 5437 return this.manufacturer; 5438 } 5439 5440 public boolean hasManufacturer() { 5441 return this.manufacturer != null && !this.manufacturer.isEmpty(); 5442 } 5443 5444 /** 5445 * @param value {@link #manufacturer} (Describes the details of the manufacturer 5446 * of the medication product. This is not intended to represent the 5447 * distributor of a medication product.) 5448 */ 5449 public MedicationKnowledge setManufacturer(Reference value) { 5450 this.manufacturer = value; 5451 return this; 5452 } 5453 5454 /** 5455 * @return {@link #manufacturer} The actual object that is the target of the 5456 * reference. The reference library doesn't populate this, but you can 5457 * use it to hold the resource if you resolve it. (Describes the details 5458 * of the manufacturer of the medication product. This is not intended 5459 * to represent the distributor of a medication product.) 5460 */ 5461 public Organization getManufacturerTarget() { 5462 if (this.manufacturerTarget == null) 5463 if (Configuration.errorOnAutoCreate()) 5464 throw new Error("Attempt to auto-create MedicationKnowledge.manufacturer"); 5465 else if (Configuration.doAutoCreate()) 5466 this.manufacturerTarget = new Organization(); // aa 5467 return this.manufacturerTarget; 5468 } 5469 5470 /** 5471 * @param value {@link #manufacturer} The actual object that is the target of 5472 * the reference. The reference library doesn't use these, but you 5473 * can use it to hold the resource if you resolve it. (Describes 5474 * the details of the manufacturer of the medication product. This 5475 * is not intended to represent the distributor of a medication 5476 * product.) 5477 */ 5478 public MedicationKnowledge setManufacturerTarget(Organization value) { 5479 this.manufacturerTarget = value; 5480 return this; 5481 } 5482 5483 /** 5484 * @return {@link #doseForm} (Describes the form of the item. Powder; tablets; 5485 * capsule.) 5486 */ 5487 public CodeableConcept getDoseForm() { 5488 if (this.doseForm == null) 5489 if (Configuration.errorOnAutoCreate()) 5490 throw new Error("Attempt to auto-create MedicationKnowledge.doseForm"); 5491 else if (Configuration.doAutoCreate()) 5492 this.doseForm = new CodeableConcept(); // cc 5493 return this.doseForm; 5494 } 5495 5496 public boolean hasDoseForm() { 5497 return this.doseForm != null && !this.doseForm.isEmpty(); 5498 } 5499 5500 /** 5501 * @param value {@link #doseForm} (Describes the form of the item. Powder; 5502 * tablets; capsule.) 5503 */ 5504 public MedicationKnowledge setDoseForm(CodeableConcept value) { 5505 this.doseForm = value; 5506 return this; 5507 } 5508 5509 /** 5510 * @return {@link #amount} (Specific amount of the drug in the packaged product. 5511 * For example, when specifying a product that has the same strength 5512 * (For example, Insulin glargine 100 unit per mL solution for 5513 * injection), this attribute provides additional clarification of the 5514 * package amount (For example, 3 mL, 10mL, etc.).) 5515 */ 5516 public Quantity getAmount() { 5517 if (this.amount == null) 5518 if (Configuration.errorOnAutoCreate()) 5519 throw new Error("Attempt to auto-create MedicationKnowledge.amount"); 5520 else if (Configuration.doAutoCreate()) 5521 this.amount = new Quantity(); // cc 5522 return this.amount; 5523 } 5524 5525 public boolean hasAmount() { 5526 return this.amount != null && !this.amount.isEmpty(); 5527 } 5528 5529 /** 5530 * @param value {@link #amount} (Specific amount of the drug in the packaged 5531 * product. For example, when specifying a product that has the 5532 * same strength (For example, Insulin glargine 100 unit per mL 5533 * solution for injection), this attribute provides additional 5534 * clarification of the package amount (For example, 3 mL, 10mL, 5535 * etc.).) 5536 */ 5537 public MedicationKnowledge setAmount(Quantity value) { 5538 this.amount = value; 5539 return this; 5540 } 5541 5542 /** 5543 * @return {@link #synonym} (Additional names for a medication, for example, the 5544 * name(s) given to a medication in different countries. For example, 5545 * acetaminophen and paracetamol or salbutamol and albuterol.) 5546 */ 5547 public List<StringType> getSynonym() { 5548 if (this.synonym == null) 5549 this.synonym = new ArrayList<StringType>(); 5550 return this.synonym; 5551 } 5552 5553 /** 5554 * @return Returns a reference to <code>this</code> for easy method chaining 5555 */ 5556 public MedicationKnowledge setSynonym(List<StringType> theSynonym) { 5557 this.synonym = theSynonym; 5558 return this; 5559 } 5560 5561 public boolean hasSynonym() { 5562 if (this.synonym == null) 5563 return false; 5564 for (StringType item : this.synonym) 5565 if (!item.isEmpty()) 5566 return true; 5567 return false; 5568 } 5569 5570 /** 5571 * @return {@link #synonym} (Additional names for a medication, for example, the 5572 * name(s) given to a medication in different countries. For example, 5573 * acetaminophen and paracetamol or salbutamol and albuterol.) 5574 */ 5575 public StringType addSynonymElement() {// 2 5576 StringType t = new StringType(); 5577 if (this.synonym == null) 5578 this.synonym = new ArrayList<StringType>(); 5579 this.synonym.add(t); 5580 return t; 5581 } 5582 5583 /** 5584 * @param value {@link #synonym} (Additional names for a medication, for 5585 * example, the name(s) given to a medication in different 5586 * countries. For example, acetaminophen and paracetamol or 5587 * salbutamol and albuterol.) 5588 */ 5589 public MedicationKnowledge addSynonym(String value) { // 1 5590 StringType t = new StringType(); 5591 t.setValue(value); 5592 if (this.synonym == null) 5593 this.synonym = new ArrayList<StringType>(); 5594 this.synonym.add(t); 5595 return this; 5596 } 5597 5598 /** 5599 * @param value {@link #synonym} (Additional names for a medication, for 5600 * example, the name(s) given to a medication in different 5601 * countries. For example, acetaminophen and paracetamol or 5602 * salbutamol and albuterol.) 5603 */ 5604 public boolean hasSynonym(String value) { 5605 if (this.synonym == null) 5606 return false; 5607 for (StringType v : this.synonym) 5608 if (v.getValue().equals(value)) // string 5609 return true; 5610 return false; 5611 } 5612 5613 /** 5614 * @return {@link #relatedMedicationKnowledge} (Associated or related knowledge 5615 * about a medication.) 5616 */ 5617 public List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> getRelatedMedicationKnowledge() { 5618 if (this.relatedMedicationKnowledge == null) 5619 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 5620 return this.relatedMedicationKnowledge; 5621 } 5622 5623 /** 5624 * @return Returns a reference to <code>this</code> for easy method chaining 5625 */ 5626 public MedicationKnowledge setRelatedMedicationKnowledge( 5627 List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> theRelatedMedicationKnowledge) { 5628 this.relatedMedicationKnowledge = theRelatedMedicationKnowledge; 5629 return this; 5630 } 5631 5632 public boolean hasRelatedMedicationKnowledge() { 5633 if (this.relatedMedicationKnowledge == null) 5634 return false; 5635 for (MedicationKnowledgeRelatedMedicationKnowledgeComponent item : this.relatedMedicationKnowledge) 5636 if (!item.isEmpty()) 5637 return true; 5638 return false; 5639 } 5640 5641 public MedicationKnowledgeRelatedMedicationKnowledgeComponent addRelatedMedicationKnowledge() { // 3 5642 MedicationKnowledgeRelatedMedicationKnowledgeComponent t = new MedicationKnowledgeRelatedMedicationKnowledgeComponent(); 5643 if (this.relatedMedicationKnowledge == null) 5644 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 5645 this.relatedMedicationKnowledge.add(t); 5646 return t; 5647 } 5648 5649 public MedicationKnowledge addRelatedMedicationKnowledge(MedicationKnowledgeRelatedMedicationKnowledgeComponent t) { // 3 5650 if (t == null) 5651 return this; 5652 if (this.relatedMedicationKnowledge == null) 5653 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 5654 this.relatedMedicationKnowledge.add(t); 5655 return this; 5656 } 5657 5658 /** 5659 * @return The first repetition of repeating field 5660 * {@link #relatedMedicationKnowledge}, creating it if it does not 5661 * already exist 5662 */ 5663 public MedicationKnowledgeRelatedMedicationKnowledgeComponent getRelatedMedicationKnowledgeFirstRep() { 5664 if (getRelatedMedicationKnowledge().isEmpty()) { 5665 addRelatedMedicationKnowledge(); 5666 } 5667 return getRelatedMedicationKnowledge().get(0); 5668 } 5669 5670 /** 5671 * @return {@link #associatedMedication} (Associated or related medications. For 5672 * example, if the medication is a branded product (e.g. Crestor), this 5673 * is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic 5674 * medication (e.g. Rosuvastatin), this would link to a branded product 5675 * (e.g. Crestor).) 5676 */ 5677 public List<Reference> getAssociatedMedication() { 5678 if (this.associatedMedication == null) 5679 this.associatedMedication = new ArrayList<Reference>(); 5680 return this.associatedMedication; 5681 } 5682 5683 /** 5684 * @return Returns a reference to <code>this</code> for easy method chaining 5685 */ 5686 public MedicationKnowledge setAssociatedMedication(List<Reference> theAssociatedMedication) { 5687 this.associatedMedication = theAssociatedMedication; 5688 return this; 5689 } 5690 5691 public boolean hasAssociatedMedication() { 5692 if (this.associatedMedication == null) 5693 return false; 5694 for (Reference item : this.associatedMedication) 5695 if (!item.isEmpty()) 5696 return true; 5697 return false; 5698 } 5699 5700 public Reference addAssociatedMedication() { // 3 5701 Reference t = new Reference(); 5702 if (this.associatedMedication == null) 5703 this.associatedMedication = new ArrayList<Reference>(); 5704 this.associatedMedication.add(t); 5705 return t; 5706 } 5707 5708 public MedicationKnowledge addAssociatedMedication(Reference t) { // 3 5709 if (t == null) 5710 return this; 5711 if (this.associatedMedication == null) 5712 this.associatedMedication = new ArrayList<Reference>(); 5713 this.associatedMedication.add(t); 5714 return this; 5715 } 5716 5717 /** 5718 * @return The first repetition of repeating field 5719 * {@link #associatedMedication}, creating it if it does not already 5720 * exist 5721 */ 5722 public Reference getAssociatedMedicationFirstRep() { 5723 if (getAssociatedMedication().isEmpty()) { 5724 addAssociatedMedication(); 5725 } 5726 return getAssociatedMedication().get(0); 5727 } 5728 5729 /** 5730 * @deprecated Use Reference#setResource(IBaseResource) instead 5731 */ 5732 @Deprecated 5733 public List<Medication> getAssociatedMedicationTarget() { 5734 if (this.associatedMedicationTarget == null) 5735 this.associatedMedicationTarget = new ArrayList<Medication>(); 5736 return this.associatedMedicationTarget; 5737 } 5738 5739 /** 5740 * @deprecated Use Reference#setResource(IBaseResource) instead 5741 */ 5742 @Deprecated 5743 public Medication addAssociatedMedicationTarget() { 5744 Medication r = new Medication(); 5745 if (this.associatedMedicationTarget == null) 5746 this.associatedMedicationTarget = new ArrayList<Medication>(); 5747 this.associatedMedicationTarget.add(r); 5748 return r; 5749 } 5750 5751 /** 5752 * @return {@link #productType} (Category of the medication or product (e.g. 5753 * branded product, therapeutic moeity, generic product, innovator 5754 * product, etc.).) 5755 */ 5756 public List<CodeableConcept> getProductType() { 5757 if (this.productType == null) 5758 this.productType = new ArrayList<CodeableConcept>(); 5759 return this.productType; 5760 } 5761 5762 /** 5763 * @return Returns a reference to <code>this</code> for easy method chaining 5764 */ 5765 public MedicationKnowledge setProductType(List<CodeableConcept> theProductType) { 5766 this.productType = theProductType; 5767 return this; 5768 } 5769 5770 public boolean hasProductType() { 5771 if (this.productType == null) 5772 return false; 5773 for (CodeableConcept item : this.productType) 5774 if (!item.isEmpty()) 5775 return true; 5776 return false; 5777 } 5778 5779 public CodeableConcept addProductType() { // 3 5780 CodeableConcept t = new CodeableConcept(); 5781 if (this.productType == null) 5782 this.productType = new ArrayList<CodeableConcept>(); 5783 this.productType.add(t); 5784 return t; 5785 } 5786 5787 public MedicationKnowledge addProductType(CodeableConcept t) { // 3 5788 if (t == null) 5789 return this; 5790 if (this.productType == null) 5791 this.productType = new ArrayList<CodeableConcept>(); 5792 this.productType.add(t); 5793 return this; 5794 } 5795 5796 /** 5797 * @return The first repetition of repeating field {@link #productType}, 5798 * creating it if it does not already exist 5799 */ 5800 public CodeableConcept getProductTypeFirstRep() { 5801 if (getProductType().isEmpty()) { 5802 addProductType(); 5803 } 5804 return getProductType().get(0); 5805 } 5806 5807 /** 5808 * @return {@link #monograph} (Associated documentation about the medication.) 5809 */ 5810 public List<MedicationKnowledgeMonographComponent> getMonograph() { 5811 if (this.monograph == null) 5812 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 5813 return this.monograph; 5814 } 5815 5816 /** 5817 * @return Returns a reference to <code>this</code> for easy method chaining 5818 */ 5819 public MedicationKnowledge setMonograph(List<MedicationKnowledgeMonographComponent> theMonograph) { 5820 this.monograph = theMonograph; 5821 return this; 5822 } 5823 5824 public boolean hasMonograph() { 5825 if (this.monograph == null) 5826 return false; 5827 for (MedicationKnowledgeMonographComponent item : this.monograph) 5828 if (!item.isEmpty()) 5829 return true; 5830 return false; 5831 } 5832 5833 public MedicationKnowledgeMonographComponent addMonograph() { // 3 5834 MedicationKnowledgeMonographComponent t = new MedicationKnowledgeMonographComponent(); 5835 if (this.monograph == null) 5836 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 5837 this.monograph.add(t); 5838 return t; 5839 } 5840 5841 public MedicationKnowledge addMonograph(MedicationKnowledgeMonographComponent t) { // 3 5842 if (t == null) 5843 return this; 5844 if (this.monograph == null) 5845 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 5846 this.monograph.add(t); 5847 return this; 5848 } 5849 5850 /** 5851 * @return The first repetition of repeating field {@link #monograph}, creating 5852 * it if it does not already exist 5853 */ 5854 public MedicationKnowledgeMonographComponent getMonographFirstRep() { 5855 if (getMonograph().isEmpty()) { 5856 addMonograph(); 5857 } 5858 return getMonograph().get(0); 5859 } 5860 5861 /** 5862 * @return {@link #ingredient} (Identifies a particular constituent of interest 5863 * in the product.) 5864 */ 5865 public List<MedicationKnowledgeIngredientComponent> getIngredient() { 5866 if (this.ingredient == null) 5867 this.ingredient = new ArrayList<MedicationKnowledgeIngredientComponent>(); 5868 return this.ingredient; 5869 } 5870 5871 /** 5872 * @return Returns a reference to <code>this</code> for easy method chaining 5873 */ 5874 public MedicationKnowledge setIngredient(List<MedicationKnowledgeIngredientComponent> theIngredient) { 5875 this.ingredient = theIngredient; 5876 return this; 5877 } 5878 5879 public boolean hasIngredient() { 5880 if (this.ingredient == null) 5881 return false; 5882 for (MedicationKnowledgeIngredientComponent item : this.ingredient) 5883 if (!item.isEmpty()) 5884 return true; 5885 return false; 5886 } 5887 5888 public MedicationKnowledgeIngredientComponent addIngredient() { // 3 5889 MedicationKnowledgeIngredientComponent t = new MedicationKnowledgeIngredientComponent(); 5890 if (this.ingredient == null) 5891 this.ingredient = new ArrayList<MedicationKnowledgeIngredientComponent>(); 5892 this.ingredient.add(t); 5893 return t; 5894 } 5895 5896 public MedicationKnowledge addIngredient(MedicationKnowledgeIngredientComponent t) { // 3 5897 if (t == null) 5898 return this; 5899 if (this.ingredient == null) 5900 this.ingredient = new ArrayList<MedicationKnowledgeIngredientComponent>(); 5901 this.ingredient.add(t); 5902 return this; 5903 } 5904 5905 /** 5906 * @return The first repetition of repeating field {@link #ingredient}, creating 5907 * it if it does not already exist 5908 */ 5909 public MedicationKnowledgeIngredientComponent getIngredientFirstRep() { 5910 if (getIngredient().isEmpty()) { 5911 addIngredient(); 5912 } 5913 return getIngredient().get(0); 5914 } 5915 5916 /** 5917 * @return {@link #preparationInstruction} (The instructions for preparing the 5918 * medication.). This is the underlying object with id, value and 5919 * extensions. The accessor "getPreparationInstruction" gives direct 5920 * access to the value 5921 */ 5922 public MarkdownType getPreparationInstructionElement() { 5923 if (this.preparationInstruction == null) 5924 if (Configuration.errorOnAutoCreate()) 5925 throw new Error("Attempt to auto-create MedicationKnowledge.preparationInstruction"); 5926 else if (Configuration.doAutoCreate()) 5927 this.preparationInstruction = new MarkdownType(); // bb 5928 return this.preparationInstruction; 5929 } 5930 5931 public boolean hasPreparationInstructionElement() { 5932 return this.preparationInstruction != null && !this.preparationInstruction.isEmpty(); 5933 } 5934 5935 public boolean hasPreparationInstruction() { 5936 return this.preparationInstruction != null && !this.preparationInstruction.isEmpty(); 5937 } 5938 5939 /** 5940 * @param value {@link #preparationInstruction} (The instructions for preparing 5941 * the medication.). This is the underlying object with id, value 5942 * and extensions. The accessor "getPreparationInstruction" gives 5943 * direct access to the value 5944 */ 5945 public MedicationKnowledge setPreparationInstructionElement(MarkdownType value) { 5946 this.preparationInstruction = value; 5947 return this; 5948 } 5949 5950 /** 5951 * @return The instructions for preparing the medication. 5952 */ 5953 public String getPreparationInstruction() { 5954 return this.preparationInstruction == null ? null : this.preparationInstruction.getValue(); 5955 } 5956 5957 /** 5958 * @param value The instructions for preparing the medication. 5959 */ 5960 public MedicationKnowledge setPreparationInstruction(String value) { 5961 if (value == null) 5962 this.preparationInstruction = null; 5963 else { 5964 if (this.preparationInstruction == null) 5965 this.preparationInstruction = new MarkdownType(); 5966 this.preparationInstruction.setValue(value); 5967 } 5968 return this; 5969 } 5970 5971 /** 5972 * @return {@link #intendedRoute} (The intended or approved route of 5973 * administration.) 5974 */ 5975 public List<CodeableConcept> getIntendedRoute() { 5976 if (this.intendedRoute == null) 5977 this.intendedRoute = new ArrayList<CodeableConcept>(); 5978 return this.intendedRoute; 5979 } 5980 5981 /** 5982 * @return Returns a reference to <code>this</code> for easy method chaining 5983 */ 5984 public MedicationKnowledge setIntendedRoute(List<CodeableConcept> theIntendedRoute) { 5985 this.intendedRoute = theIntendedRoute; 5986 return this; 5987 } 5988 5989 public boolean hasIntendedRoute() { 5990 if (this.intendedRoute == null) 5991 return false; 5992 for (CodeableConcept item : this.intendedRoute) 5993 if (!item.isEmpty()) 5994 return true; 5995 return false; 5996 } 5997 5998 public CodeableConcept addIntendedRoute() { // 3 5999 CodeableConcept t = new CodeableConcept(); 6000 if (this.intendedRoute == null) 6001 this.intendedRoute = new ArrayList<CodeableConcept>(); 6002 this.intendedRoute.add(t); 6003 return t; 6004 } 6005 6006 public MedicationKnowledge addIntendedRoute(CodeableConcept t) { // 3 6007 if (t == null) 6008 return this; 6009 if (this.intendedRoute == null) 6010 this.intendedRoute = new ArrayList<CodeableConcept>(); 6011 this.intendedRoute.add(t); 6012 return this; 6013 } 6014 6015 /** 6016 * @return The first repetition of repeating field {@link #intendedRoute}, 6017 * creating it if it does not already exist 6018 */ 6019 public CodeableConcept getIntendedRouteFirstRep() { 6020 if (getIntendedRoute().isEmpty()) { 6021 addIntendedRoute(); 6022 } 6023 return getIntendedRoute().get(0); 6024 } 6025 6026 /** 6027 * @return {@link #cost} (The price of the medication.) 6028 */ 6029 public List<MedicationKnowledgeCostComponent> getCost() { 6030 if (this.cost == null) 6031 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6032 return this.cost; 6033 } 6034 6035 /** 6036 * @return Returns a reference to <code>this</code> for easy method chaining 6037 */ 6038 public MedicationKnowledge setCost(List<MedicationKnowledgeCostComponent> theCost) { 6039 this.cost = theCost; 6040 return this; 6041 } 6042 6043 public boolean hasCost() { 6044 if (this.cost == null) 6045 return false; 6046 for (MedicationKnowledgeCostComponent item : this.cost) 6047 if (!item.isEmpty()) 6048 return true; 6049 return false; 6050 } 6051 6052 public MedicationKnowledgeCostComponent addCost() { // 3 6053 MedicationKnowledgeCostComponent t = new MedicationKnowledgeCostComponent(); 6054 if (this.cost == null) 6055 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6056 this.cost.add(t); 6057 return t; 6058 } 6059 6060 public MedicationKnowledge addCost(MedicationKnowledgeCostComponent t) { // 3 6061 if (t == null) 6062 return this; 6063 if (this.cost == null) 6064 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6065 this.cost.add(t); 6066 return this; 6067 } 6068 6069 /** 6070 * @return The first repetition of repeating field {@link #cost}, creating it if 6071 * it does not already exist 6072 */ 6073 public MedicationKnowledgeCostComponent getCostFirstRep() { 6074 if (getCost().isEmpty()) { 6075 addCost(); 6076 } 6077 return getCost().get(0); 6078 } 6079 6080 /** 6081 * @return {@link #monitoringProgram} (The program under which the medication is 6082 * reviewed.) 6083 */ 6084 public List<MedicationKnowledgeMonitoringProgramComponent> getMonitoringProgram() { 6085 if (this.monitoringProgram == null) 6086 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6087 return this.monitoringProgram; 6088 } 6089 6090 /** 6091 * @return Returns a reference to <code>this</code> for easy method chaining 6092 */ 6093 public MedicationKnowledge setMonitoringProgram( 6094 List<MedicationKnowledgeMonitoringProgramComponent> theMonitoringProgram) { 6095 this.monitoringProgram = theMonitoringProgram; 6096 return this; 6097 } 6098 6099 public boolean hasMonitoringProgram() { 6100 if (this.monitoringProgram == null) 6101 return false; 6102 for (MedicationKnowledgeMonitoringProgramComponent item : this.monitoringProgram) 6103 if (!item.isEmpty()) 6104 return true; 6105 return false; 6106 } 6107 6108 public MedicationKnowledgeMonitoringProgramComponent addMonitoringProgram() { // 3 6109 MedicationKnowledgeMonitoringProgramComponent t = new MedicationKnowledgeMonitoringProgramComponent(); 6110 if (this.monitoringProgram == null) 6111 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6112 this.monitoringProgram.add(t); 6113 return t; 6114 } 6115 6116 public MedicationKnowledge addMonitoringProgram(MedicationKnowledgeMonitoringProgramComponent t) { // 3 6117 if (t == null) 6118 return this; 6119 if (this.monitoringProgram == null) 6120 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6121 this.monitoringProgram.add(t); 6122 return this; 6123 } 6124 6125 /** 6126 * @return The first repetition of repeating field {@link #monitoringProgram}, 6127 * creating it if it does not already exist 6128 */ 6129 public MedicationKnowledgeMonitoringProgramComponent getMonitoringProgramFirstRep() { 6130 if (getMonitoringProgram().isEmpty()) { 6131 addMonitoringProgram(); 6132 } 6133 return getMonitoringProgram().get(0); 6134 } 6135 6136 /** 6137 * @return {@link #administrationGuidelines} (Guidelines for the administration 6138 * of the medication.) 6139 */ 6140 public List<MedicationKnowledgeAdministrationGuidelinesComponent> getAdministrationGuidelines() { 6141 if (this.administrationGuidelines == null) 6142 this.administrationGuidelines = new ArrayList<MedicationKnowledgeAdministrationGuidelinesComponent>(); 6143 return this.administrationGuidelines; 6144 } 6145 6146 /** 6147 * @return Returns a reference to <code>this</code> for easy method chaining 6148 */ 6149 public MedicationKnowledge setAdministrationGuidelines( 6150 List<MedicationKnowledgeAdministrationGuidelinesComponent> theAdministrationGuidelines) { 6151 this.administrationGuidelines = theAdministrationGuidelines; 6152 return this; 6153 } 6154 6155 public boolean hasAdministrationGuidelines() { 6156 if (this.administrationGuidelines == null) 6157 return false; 6158 for (MedicationKnowledgeAdministrationGuidelinesComponent item : this.administrationGuidelines) 6159 if (!item.isEmpty()) 6160 return true; 6161 return false; 6162 } 6163 6164 public MedicationKnowledgeAdministrationGuidelinesComponent addAdministrationGuidelines() { // 3 6165 MedicationKnowledgeAdministrationGuidelinesComponent t = new MedicationKnowledgeAdministrationGuidelinesComponent(); 6166 if (this.administrationGuidelines == null) 6167 this.administrationGuidelines = new ArrayList<MedicationKnowledgeAdministrationGuidelinesComponent>(); 6168 this.administrationGuidelines.add(t); 6169 return t; 6170 } 6171 6172 public MedicationKnowledge addAdministrationGuidelines(MedicationKnowledgeAdministrationGuidelinesComponent t) { // 3 6173 if (t == null) 6174 return this; 6175 if (this.administrationGuidelines == null) 6176 this.administrationGuidelines = new ArrayList<MedicationKnowledgeAdministrationGuidelinesComponent>(); 6177 this.administrationGuidelines.add(t); 6178 return this; 6179 } 6180 6181 /** 6182 * @return The first repetition of repeating field 6183 * {@link #administrationGuidelines}, creating it if it does not already 6184 * exist 6185 */ 6186 public MedicationKnowledgeAdministrationGuidelinesComponent getAdministrationGuidelinesFirstRep() { 6187 if (getAdministrationGuidelines().isEmpty()) { 6188 addAdministrationGuidelines(); 6189 } 6190 return getAdministrationGuidelines().get(0); 6191 } 6192 6193 /** 6194 * @return {@link #medicineClassification} (Categorization of the medication 6195 * within a formulary or classification system.) 6196 */ 6197 public List<MedicationKnowledgeMedicineClassificationComponent> getMedicineClassification() { 6198 if (this.medicineClassification == null) 6199 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6200 return this.medicineClassification; 6201 } 6202 6203 /** 6204 * @return Returns a reference to <code>this</code> for easy method chaining 6205 */ 6206 public MedicationKnowledge setMedicineClassification( 6207 List<MedicationKnowledgeMedicineClassificationComponent> theMedicineClassification) { 6208 this.medicineClassification = theMedicineClassification; 6209 return this; 6210 } 6211 6212 public boolean hasMedicineClassification() { 6213 if (this.medicineClassification == null) 6214 return false; 6215 for (MedicationKnowledgeMedicineClassificationComponent item : this.medicineClassification) 6216 if (!item.isEmpty()) 6217 return true; 6218 return false; 6219 } 6220 6221 public MedicationKnowledgeMedicineClassificationComponent addMedicineClassification() { // 3 6222 MedicationKnowledgeMedicineClassificationComponent t = new MedicationKnowledgeMedicineClassificationComponent(); 6223 if (this.medicineClassification == null) 6224 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6225 this.medicineClassification.add(t); 6226 return t; 6227 } 6228 6229 public MedicationKnowledge addMedicineClassification(MedicationKnowledgeMedicineClassificationComponent t) { // 3 6230 if (t == null) 6231 return this; 6232 if (this.medicineClassification == null) 6233 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6234 this.medicineClassification.add(t); 6235 return this; 6236 } 6237 6238 /** 6239 * @return The first repetition of repeating field 6240 * {@link #medicineClassification}, creating it if it does not already 6241 * exist 6242 */ 6243 public MedicationKnowledgeMedicineClassificationComponent getMedicineClassificationFirstRep() { 6244 if (getMedicineClassification().isEmpty()) { 6245 addMedicineClassification(); 6246 } 6247 return getMedicineClassification().get(0); 6248 } 6249 6250 /** 6251 * @return {@link #packaging} (Information that only applies to packages (not 6252 * products).) 6253 */ 6254 public MedicationKnowledgePackagingComponent getPackaging() { 6255 if (this.packaging == null) 6256 if (Configuration.errorOnAutoCreate()) 6257 throw new Error("Attempt to auto-create MedicationKnowledge.packaging"); 6258 else if (Configuration.doAutoCreate()) 6259 this.packaging = new MedicationKnowledgePackagingComponent(); // cc 6260 return this.packaging; 6261 } 6262 6263 public boolean hasPackaging() { 6264 return this.packaging != null && !this.packaging.isEmpty(); 6265 } 6266 6267 /** 6268 * @param value {@link #packaging} (Information that only applies to packages 6269 * (not products).) 6270 */ 6271 public MedicationKnowledge setPackaging(MedicationKnowledgePackagingComponent value) { 6272 this.packaging = value; 6273 return this; 6274 } 6275 6276 /** 6277 * @return {@link #drugCharacteristic} (Specifies descriptive properties of the 6278 * medicine, such as color, shape, imprints, etc.) 6279 */ 6280 public List<MedicationKnowledgeDrugCharacteristicComponent> getDrugCharacteristic() { 6281 if (this.drugCharacteristic == null) 6282 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDrugCharacteristicComponent>(); 6283 return this.drugCharacteristic; 6284 } 6285 6286 /** 6287 * @return Returns a reference to <code>this</code> for easy method chaining 6288 */ 6289 public MedicationKnowledge setDrugCharacteristic( 6290 List<MedicationKnowledgeDrugCharacteristicComponent> theDrugCharacteristic) { 6291 this.drugCharacteristic = theDrugCharacteristic; 6292 return this; 6293 } 6294 6295 public boolean hasDrugCharacteristic() { 6296 if (this.drugCharacteristic == null) 6297 return false; 6298 for (MedicationKnowledgeDrugCharacteristicComponent item : this.drugCharacteristic) 6299 if (!item.isEmpty()) 6300 return true; 6301 return false; 6302 } 6303 6304 public MedicationKnowledgeDrugCharacteristicComponent addDrugCharacteristic() { // 3 6305 MedicationKnowledgeDrugCharacteristicComponent t = new MedicationKnowledgeDrugCharacteristicComponent(); 6306 if (this.drugCharacteristic == null) 6307 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDrugCharacteristicComponent>(); 6308 this.drugCharacteristic.add(t); 6309 return t; 6310 } 6311 6312 public MedicationKnowledge addDrugCharacteristic(MedicationKnowledgeDrugCharacteristicComponent t) { // 3 6313 if (t == null) 6314 return this; 6315 if (this.drugCharacteristic == null) 6316 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDrugCharacteristicComponent>(); 6317 this.drugCharacteristic.add(t); 6318 return this; 6319 } 6320 6321 /** 6322 * @return The first repetition of repeating field {@link #drugCharacteristic}, 6323 * creating it if it does not already exist 6324 */ 6325 public MedicationKnowledgeDrugCharacteristicComponent getDrugCharacteristicFirstRep() { 6326 if (getDrugCharacteristic().isEmpty()) { 6327 addDrugCharacteristic(); 6328 } 6329 return getDrugCharacteristic().get(0); 6330 } 6331 6332 /** 6333 * @return {@link #contraindication} (Potential clinical issue with or between 6334 * medication(s) (for example, drug-drug interaction, drug-disease 6335 * contraindication, drug-allergy interaction, etc.).) 6336 */ 6337 public List<Reference> getContraindication() { 6338 if (this.contraindication == null) 6339 this.contraindication = new ArrayList<Reference>(); 6340 return this.contraindication; 6341 } 6342 6343 /** 6344 * @return Returns a reference to <code>this</code> for easy method chaining 6345 */ 6346 public MedicationKnowledge setContraindication(List<Reference> theContraindication) { 6347 this.contraindication = theContraindication; 6348 return this; 6349 } 6350 6351 public boolean hasContraindication() { 6352 if (this.contraindication == null) 6353 return false; 6354 for (Reference item : this.contraindication) 6355 if (!item.isEmpty()) 6356 return true; 6357 return false; 6358 } 6359 6360 public Reference addContraindication() { // 3 6361 Reference t = new Reference(); 6362 if (this.contraindication == null) 6363 this.contraindication = new ArrayList<Reference>(); 6364 this.contraindication.add(t); 6365 return t; 6366 } 6367 6368 public MedicationKnowledge addContraindication(Reference t) { // 3 6369 if (t == null) 6370 return this; 6371 if (this.contraindication == null) 6372 this.contraindication = new ArrayList<Reference>(); 6373 this.contraindication.add(t); 6374 return this; 6375 } 6376 6377 /** 6378 * @return The first repetition of repeating field {@link #contraindication}, 6379 * creating it if it does not already exist 6380 */ 6381 public Reference getContraindicationFirstRep() { 6382 if (getContraindication().isEmpty()) { 6383 addContraindication(); 6384 } 6385 return getContraindication().get(0); 6386 } 6387 6388 /** 6389 * @deprecated Use Reference#setResource(IBaseResource) instead 6390 */ 6391 @Deprecated 6392 public List<DetectedIssue> getContraindicationTarget() { 6393 if (this.contraindicationTarget == null) 6394 this.contraindicationTarget = new ArrayList<DetectedIssue>(); 6395 return this.contraindicationTarget; 6396 } 6397 6398 /** 6399 * @deprecated Use Reference#setResource(IBaseResource) instead 6400 */ 6401 @Deprecated 6402 public DetectedIssue addContraindicationTarget() { 6403 DetectedIssue r = new DetectedIssue(); 6404 if (this.contraindicationTarget == null) 6405 this.contraindicationTarget = new ArrayList<DetectedIssue>(); 6406 this.contraindicationTarget.add(r); 6407 return r; 6408 } 6409 6410 /** 6411 * @return {@link #regulatory} (Regulatory information about a medication.) 6412 */ 6413 public List<MedicationKnowledgeRegulatoryComponent> getRegulatory() { 6414 if (this.regulatory == null) 6415 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6416 return this.regulatory; 6417 } 6418 6419 /** 6420 * @return Returns a reference to <code>this</code> for easy method chaining 6421 */ 6422 public MedicationKnowledge setRegulatory(List<MedicationKnowledgeRegulatoryComponent> theRegulatory) { 6423 this.regulatory = theRegulatory; 6424 return this; 6425 } 6426 6427 public boolean hasRegulatory() { 6428 if (this.regulatory == null) 6429 return false; 6430 for (MedicationKnowledgeRegulatoryComponent item : this.regulatory) 6431 if (!item.isEmpty()) 6432 return true; 6433 return false; 6434 } 6435 6436 public MedicationKnowledgeRegulatoryComponent addRegulatory() { // 3 6437 MedicationKnowledgeRegulatoryComponent t = new MedicationKnowledgeRegulatoryComponent(); 6438 if (this.regulatory == null) 6439 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6440 this.regulatory.add(t); 6441 return t; 6442 } 6443 6444 public MedicationKnowledge addRegulatory(MedicationKnowledgeRegulatoryComponent t) { // 3 6445 if (t == null) 6446 return this; 6447 if (this.regulatory == null) 6448 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6449 this.regulatory.add(t); 6450 return this; 6451 } 6452 6453 /** 6454 * @return The first repetition of repeating field {@link #regulatory}, creating 6455 * it if it does not already exist 6456 */ 6457 public MedicationKnowledgeRegulatoryComponent getRegulatoryFirstRep() { 6458 if (getRegulatory().isEmpty()) { 6459 addRegulatory(); 6460 } 6461 return getRegulatory().get(0); 6462 } 6463 6464 /** 6465 * @return {@link #kinetics} (The time course of drug absorption, distribution, 6466 * metabolism and excretion of a medication from the body.) 6467 */ 6468 public List<MedicationKnowledgeKineticsComponent> getKinetics() { 6469 if (this.kinetics == null) 6470 this.kinetics = new ArrayList<MedicationKnowledgeKineticsComponent>(); 6471 return this.kinetics; 6472 } 6473 6474 /** 6475 * @return Returns a reference to <code>this</code> for easy method chaining 6476 */ 6477 public MedicationKnowledge setKinetics(List<MedicationKnowledgeKineticsComponent> theKinetics) { 6478 this.kinetics = theKinetics; 6479 return this; 6480 } 6481 6482 public boolean hasKinetics() { 6483 if (this.kinetics == null) 6484 return false; 6485 for (MedicationKnowledgeKineticsComponent item : this.kinetics) 6486 if (!item.isEmpty()) 6487 return true; 6488 return false; 6489 } 6490 6491 public MedicationKnowledgeKineticsComponent addKinetics() { // 3 6492 MedicationKnowledgeKineticsComponent t = new MedicationKnowledgeKineticsComponent(); 6493 if (this.kinetics == null) 6494 this.kinetics = new ArrayList<MedicationKnowledgeKineticsComponent>(); 6495 this.kinetics.add(t); 6496 return t; 6497 } 6498 6499 public MedicationKnowledge addKinetics(MedicationKnowledgeKineticsComponent t) { // 3 6500 if (t == null) 6501 return this; 6502 if (this.kinetics == null) 6503 this.kinetics = new ArrayList<MedicationKnowledgeKineticsComponent>(); 6504 this.kinetics.add(t); 6505 return this; 6506 } 6507 6508 /** 6509 * @return The first repetition of repeating field {@link #kinetics}, creating 6510 * it if it does not already exist 6511 */ 6512 public MedicationKnowledgeKineticsComponent getKineticsFirstRep() { 6513 if (getKinetics().isEmpty()) { 6514 addKinetics(); 6515 } 6516 return getKinetics().get(0); 6517 } 6518 6519 protected void listChildren(List<Property> children) { 6520 super.listChildren(children); 6521 children.add(new Property("code", "CodeableConcept", 6522 "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 6523 0, 1, code)); 6524 children.add(new Property("status", "code", 6525 "A code to indicate if the medication is in active use. The status refers to the validity about the information of the medication and not to its medicinal properties.", 6526 0, 1, status)); 6527 children.add(new Property("manufacturer", "Reference(Organization)", 6528 "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 6529 0, 1, manufacturer)); 6530 children.add(new Property("doseForm", "CodeableConcept", 6531 "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm)); 6532 children.add(new Property("amount", "SimpleQuantity", 6533 "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 6534 0, 1, amount)); 6535 children.add(new Property("synonym", "string", 6536 "Additional names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.", 6537 0, java.lang.Integer.MAX_VALUE, synonym)); 6538 children.add(new Property("relatedMedicationKnowledge", "", "Associated or related knowledge about a medication.", 6539 0, java.lang.Integer.MAX_VALUE, relatedMedicationKnowledge)); 6540 children.add(new Property("associatedMedication", "Reference(Medication)", 6541 "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor).", 6542 0, java.lang.Integer.MAX_VALUE, associatedMedication)); 6543 children.add(new Property("productType", "CodeableConcept", 6544 "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).", 6545 0, java.lang.Integer.MAX_VALUE, productType)); 6546 children.add(new Property("monograph", "", "Associated documentation about the medication.", 0, 6547 java.lang.Integer.MAX_VALUE, monograph)); 6548 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, 6549 java.lang.Integer.MAX_VALUE, ingredient)); 6550 children.add(new Property("preparationInstruction", "markdown", "The instructions for preparing the medication.", 0, 6551 1, preparationInstruction)); 6552 children.add(new Property("intendedRoute", "CodeableConcept", "The intended or approved route of administration.", 6553 0, java.lang.Integer.MAX_VALUE, intendedRoute)); 6554 children.add(new Property("cost", "", "The price of the medication.", 0, java.lang.Integer.MAX_VALUE, cost)); 6555 children.add(new Property("monitoringProgram", "", "The program under which the medication is reviewed.", 0, 6556 java.lang.Integer.MAX_VALUE, monitoringProgram)); 6557 children.add(new Property("administrationGuidelines", "", "Guidelines for the administration of the medication.", 0, 6558 java.lang.Integer.MAX_VALUE, administrationGuidelines)); 6559 children.add(new Property("medicineClassification", "", 6560 "Categorization of the medication within a formulary or classification system.", 0, java.lang.Integer.MAX_VALUE, 6561 medicineClassification)); 6562 children.add( 6563 new Property("packaging", "", "Information that only applies to packages (not products).", 0, 1, packaging)); 6564 children.add(new Property("drugCharacteristic", "", 6565 "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.", 0, 6566 java.lang.Integer.MAX_VALUE, drugCharacteristic)); 6567 children.add(new Property("contraindication", "Reference(DetectedIssue)", 6568 "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).", 6569 0, java.lang.Integer.MAX_VALUE, contraindication)); 6570 children.add(new Property("regulatory", "", "Regulatory information about a medication.", 0, 6571 java.lang.Integer.MAX_VALUE, regulatory)); 6572 children.add(new Property("kinetics", "", 6573 "The time course of drug absorption, distribution, metabolism and excretion of a medication from the body.", 0, 6574 java.lang.Integer.MAX_VALUE, kinetics)); 6575 } 6576 6577 @Override 6578 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6579 switch (_hash) { 6580 case 3059181: 6581 /* code */ return new Property("code", "CodeableConcept", 6582 "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 6583 0, 1, code); 6584 case -892481550: 6585 /* status */ return new Property("status", "code", 6586 "A code to indicate if the medication is in active use. The status refers to the validity about the information of the medication and not to its medicinal properties.", 6587 0, 1, status); 6588 case -1969347631: 6589 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 6590 "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 6591 0, 1, manufacturer); 6592 case 1303858817: 6593 /* doseForm */ return new Property("doseForm", "CodeableConcept", 6594 "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm); 6595 case -1413853096: 6596 /* amount */ return new Property("amount", "SimpleQuantity", 6597 "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 6598 0, 1, amount); 6599 case -1742128133: 6600 /* synonym */ return new Property("synonym", "string", 6601 "Additional names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.", 6602 0, java.lang.Integer.MAX_VALUE, synonym); 6603 case 723067972: 6604 /* relatedMedicationKnowledge */ return new Property("relatedMedicationKnowledge", "", 6605 "Associated or related knowledge about a medication.", 0, java.lang.Integer.MAX_VALUE, 6606 relatedMedicationKnowledge); 6607 case 1312779381: 6608 /* associatedMedication */ return new Property("associatedMedication", "Reference(Medication)", 6609 "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor).", 6610 0, java.lang.Integer.MAX_VALUE, associatedMedication); 6611 case -1491615543: 6612 /* productType */ return new Property("productType", "CodeableConcept", 6613 "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).", 6614 0, java.lang.Integer.MAX_VALUE, productType); 6615 case -1442980789: 6616 /* monograph */ return new Property("monograph", "", "Associated documentation about the medication.", 0, 6617 java.lang.Integer.MAX_VALUE, monograph); 6618 case -206409263: 6619 /* ingredient */ return new Property("ingredient", "", 6620 "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, 6621 ingredient); 6622 case 1025456503: 6623 /* preparationInstruction */ return new Property("preparationInstruction", "markdown", 6624 "The instructions for preparing the medication.", 0, 1, preparationInstruction); 6625 case -767798050: 6626 /* intendedRoute */ return new Property("intendedRoute", "CodeableConcept", 6627 "The intended or approved route of administration.", 0, java.lang.Integer.MAX_VALUE, intendedRoute); 6628 case 3059661: 6629 /* cost */ return new Property("cost", "", "The price of the medication.", 0, java.lang.Integer.MAX_VALUE, cost); 6630 case 569848092: 6631 /* monitoringProgram */ return new Property("monitoringProgram", "", 6632 "The program under which the medication is reviewed.", 0, java.lang.Integer.MAX_VALUE, monitoringProgram); 6633 case 496930945: 6634 /* administrationGuidelines */ return new Property("administrationGuidelines", "", 6635 "Guidelines for the administration of the medication.", 0, java.lang.Integer.MAX_VALUE, 6636 administrationGuidelines); 6637 case 1791551680: 6638 /* medicineClassification */ return new Property("medicineClassification", "", 6639 "Categorization of the medication within a formulary or classification system.", 0, 6640 java.lang.Integer.MAX_VALUE, medicineClassification); 6641 case 1802065795: 6642 /* packaging */ return new Property("packaging", "", "Information that only applies to packages (not products).", 6643 0, 1, packaging); 6644 case -844126885: 6645 /* drugCharacteristic */ return new Property("drugCharacteristic", "", 6646 "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.", 0, 6647 java.lang.Integer.MAX_VALUE, drugCharacteristic); 6648 case 107135229: 6649 /* contraindication */ return new Property("contraindication", "Reference(DetectedIssue)", 6650 "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).", 6651 0, java.lang.Integer.MAX_VALUE, contraindication); 6652 case -27327848: 6653 /* regulatory */ return new Property("regulatory", "", "Regulatory information about a medication.", 0, 6654 java.lang.Integer.MAX_VALUE, regulatory); 6655 case -553207110: 6656 /* kinetics */ return new Property("kinetics", "", 6657 "The time course of drug absorption, distribution, metabolism and excretion of a medication from the body.", 6658 0, java.lang.Integer.MAX_VALUE, kinetics); 6659 default: 6660 return super.getNamedProperty(_hash, _name, _checkValid); 6661 } 6662 6663 } 6664 6665 @Override 6666 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6667 switch (hash) { 6668 case 3059181: 6669 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 6670 case -892481550: 6671 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationKnowledgeStatus> 6672 case -1969347631: 6673 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // Reference 6674 case 1303858817: 6675 /* doseForm */ return this.doseForm == null ? new Base[0] : new Base[] { this.doseForm }; // CodeableConcept 6676 case -1413853096: 6677 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Quantity 6678 case -1742128133: 6679 /* synonym */ return this.synonym == null ? new Base[0] : this.synonym.toArray(new Base[this.synonym.size()]); // StringType 6680 case 723067972: 6681 /* relatedMedicationKnowledge */ return this.relatedMedicationKnowledge == null ? new Base[0] 6682 : this.relatedMedicationKnowledge.toArray(new Base[this.relatedMedicationKnowledge.size()]); // MedicationKnowledgeRelatedMedicationKnowledgeComponent 6683 case 1312779381: 6684 /* associatedMedication */ return this.associatedMedication == null ? new Base[0] 6685 : this.associatedMedication.toArray(new Base[this.associatedMedication.size()]); // Reference 6686 case -1491615543: 6687 /* productType */ return this.productType == null ? new Base[0] 6688 : this.productType.toArray(new Base[this.productType.size()]); // CodeableConcept 6689 case -1442980789: 6690 /* monograph */ return this.monograph == null ? new Base[0] 6691 : this.monograph.toArray(new Base[this.monograph.size()]); // MedicationKnowledgeMonographComponent 6692 case -206409263: 6693 /* ingredient */ return this.ingredient == null ? new Base[0] 6694 : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationKnowledgeIngredientComponent 6695 case 1025456503: 6696 /* preparationInstruction */ return this.preparationInstruction == null ? new Base[0] 6697 : new Base[] { this.preparationInstruction }; // MarkdownType 6698 case -767798050: 6699 /* intendedRoute */ return this.intendedRoute == null ? new Base[0] 6700 : this.intendedRoute.toArray(new Base[this.intendedRoute.size()]); // CodeableConcept 6701 case 3059661: 6702 /* cost */ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // MedicationKnowledgeCostComponent 6703 case 569848092: 6704 /* monitoringProgram */ return this.monitoringProgram == null ? new Base[0] 6705 : this.monitoringProgram.toArray(new Base[this.monitoringProgram.size()]); // MedicationKnowledgeMonitoringProgramComponent 6706 case 496930945: 6707 /* administrationGuidelines */ return this.administrationGuidelines == null ? new Base[0] 6708 : this.administrationGuidelines.toArray(new Base[this.administrationGuidelines.size()]); // MedicationKnowledgeAdministrationGuidelinesComponent 6709 case 1791551680: 6710 /* medicineClassification */ return this.medicineClassification == null ? new Base[0] 6711 : this.medicineClassification.toArray(new Base[this.medicineClassification.size()]); // MedicationKnowledgeMedicineClassificationComponent 6712 case 1802065795: 6713 /* packaging */ return this.packaging == null ? new Base[0] : new Base[] { this.packaging }; // MedicationKnowledgePackagingComponent 6714 case -844126885: 6715 /* drugCharacteristic */ return this.drugCharacteristic == null ? new Base[0] 6716 : this.drugCharacteristic.toArray(new Base[this.drugCharacteristic.size()]); // MedicationKnowledgeDrugCharacteristicComponent 6717 case 107135229: 6718 /* contraindication */ return this.contraindication == null ? new Base[0] 6719 : this.contraindication.toArray(new Base[this.contraindication.size()]); // Reference 6720 case -27327848: 6721 /* regulatory */ return this.regulatory == null ? new Base[0] 6722 : this.regulatory.toArray(new Base[this.regulatory.size()]); // MedicationKnowledgeRegulatoryComponent 6723 case -553207110: 6724 /* kinetics */ return this.kinetics == null ? new Base[0] : this.kinetics.toArray(new Base[this.kinetics.size()]); // MedicationKnowledgeKineticsComponent 6725 default: 6726 return super.getProperty(hash, name, checkValid); 6727 } 6728 6729 } 6730 6731 @Override 6732 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6733 switch (hash) { 6734 case 3059181: // code 6735 this.code = castToCodeableConcept(value); // CodeableConcept 6736 return value; 6737 case -892481550: // status 6738 value = new MedicationKnowledgeStatusEnumFactory().fromType(castToCode(value)); 6739 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatus> 6740 return value; 6741 case -1969347631: // manufacturer 6742 this.manufacturer = castToReference(value); // Reference 6743 return value; 6744 case 1303858817: // doseForm 6745 this.doseForm = castToCodeableConcept(value); // CodeableConcept 6746 return value; 6747 case -1413853096: // amount 6748 this.amount = castToQuantity(value); // Quantity 6749 return value; 6750 case -1742128133: // synonym 6751 this.getSynonym().add(castToString(value)); // StringType 6752 return value; 6753 case 723067972: // relatedMedicationKnowledge 6754 this.getRelatedMedicationKnowledge().add((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); // MedicationKnowledgeRelatedMedicationKnowledgeComponent 6755 return value; 6756 case 1312779381: // associatedMedication 6757 this.getAssociatedMedication().add(castToReference(value)); // Reference 6758 return value; 6759 case -1491615543: // productType 6760 this.getProductType().add(castToCodeableConcept(value)); // CodeableConcept 6761 return value; 6762 case -1442980789: // monograph 6763 this.getMonograph().add((MedicationKnowledgeMonographComponent) value); // MedicationKnowledgeMonographComponent 6764 return value; 6765 case -206409263: // ingredient 6766 this.getIngredient().add((MedicationKnowledgeIngredientComponent) value); // MedicationKnowledgeIngredientComponent 6767 return value; 6768 case 1025456503: // preparationInstruction 6769 this.preparationInstruction = castToMarkdown(value); // MarkdownType 6770 return value; 6771 case -767798050: // intendedRoute 6772 this.getIntendedRoute().add(castToCodeableConcept(value)); // CodeableConcept 6773 return value; 6774 case 3059661: // cost 6775 this.getCost().add((MedicationKnowledgeCostComponent) value); // MedicationKnowledgeCostComponent 6776 return value; 6777 case 569848092: // monitoringProgram 6778 this.getMonitoringProgram().add((MedicationKnowledgeMonitoringProgramComponent) value); // MedicationKnowledgeMonitoringProgramComponent 6779 return value; 6780 case 496930945: // administrationGuidelines 6781 this.getAdministrationGuidelines().add((MedicationKnowledgeAdministrationGuidelinesComponent) value); // MedicationKnowledgeAdministrationGuidelinesComponent 6782 return value; 6783 case 1791551680: // medicineClassification 6784 this.getMedicineClassification().add((MedicationKnowledgeMedicineClassificationComponent) value); // MedicationKnowledgeMedicineClassificationComponent 6785 return value; 6786 case 1802065795: // packaging 6787 this.packaging = (MedicationKnowledgePackagingComponent) value; // MedicationKnowledgePackagingComponent 6788 return value; 6789 case -844126885: // drugCharacteristic 6790 this.getDrugCharacteristic().add((MedicationKnowledgeDrugCharacteristicComponent) value); // MedicationKnowledgeDrugCharacteristicComponent 6791 return value; 6792 case 107135229: // contraindication 6793 this.getContraindication().add(castToReference(value)); // Reference 6794 return value; 6795 case -27327848: // regulatory 6796 this.getRegulatory().add((MedicationKnowledgeRegulatoryComponent) value); // MedicationKnowledgeRegulatoryComponent 6797 return value; 6798 case -553207110: // kinetics 6799 this.getKinetics().add((MedicationKnowledgeKineticsComponent) value); // MedicationKnowledgeKineticsComponent 6800 return value; 6801 default: 6802 return super.setProperty(hash, name, value); 6803 } 6804 6805 } 6806 6807 @Override 6808 public Base setProperty(String name, Base value) throws FHIRException { 6809 if (name.equals("code")) { 6810 this.code = castToCodeableConcept(value); // CodeableConcept 6811 } else if (name.equals("status")) { 6812 value = new MedicationKnowledgeStatusEnumFactory().fromType(castToCode(value)); 6813 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatus> 6814 } else if (name.equals("manufacturer")) { 6815 this.manufacturer = castToReference(value); // Reference 6816 } else if (name.equals("doseForm")) { 6817 this.doseForm = castToCodeableConcept(value); // CodeableConcept 6818 } else if (name.equals("amount")) { 6819 this.amount = castToQuantity(value); // Quantity 6820 } else if (name.equals("synonym")) { 6821 this.getSynonym().add(castToString(value)); 6822 } else if (name.equals("relatedMedicationKnowledge")) { 6823 this.getRelatedMedicationKnowledge().add((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); 6824 } else if (name.equals("associatedMedication")) { 6825 this.getAssociatedMedication().add(castToReference(value)); 6826 } else if (name.equals("productType")) { 6827 this.getProductType().add(castToCodeableConcept(value)); 6828 } else if (name.equals("monograph")) { 6829 this.getMonograph().add((MedicationKnowledgeMonographComponent) value); 6830 } else if (name.equals("ingredient")) { 6831 this.getIngredient().add((MedicationKnowledgeIngredientComponent) value); 6832 } else if (name.equals("preparationInstruction")) { 6833 this.preparationInstruction = castToMarkdown(value); // MarkdownType 6834 } else if (name.equals("intendedRoute")) { 6835 this.getIntendedRoute().add(castToCodeableConcept(value)); 6836 } else if (name.equals("cost")) { 6837 this.getCost().add((MedicationKnowledgeCostComponent) value); 6838 } else if (name.equals("monitoringProgram")) { 6839 this.getMonitoringProgram().add((MedicationKnowledgeMonitoringProgramComponent) value); 6840 } else if (name.equals("administrationGuidelines")) { 6841 this.getAdministrationGuidelines().add((MedicationKnowledgeAdministrationGuidelinesComponent) value); 6842 } else if (name.equals("medicineClassification")) { 6843 this.getMedicineClassification().add((MedicationKnowledgeMedicineClassificationComponent) value); 6844 } else if (name.equals("packaging")) { 6845 this.packaging = (MedicationKnowledgePackagingComponent) value; // MedicationKnowledgePackagingComponent 6846 } else if (name.equals("drugCharacteristic")) { 6847 this.getDrugCharacteristic().add((MedicationKnowledgeDrugCharacteristicComponent) value); 6848 } else if (name.equals("contraindication")) { 6849 this.getContraindication().add(castToReference(value)); 6850 } else if (name.equals("regulatory")) { 6851 this.getRegulatory().add((MedicationKnowledgeRegulatoryComponent) value); 6852 } else if (name.equals("kinetics")) { 6853 this.getKinetics().add((MedicationKnowledgeKineticsComponent) value); 6854 } else 6855 return super.setProperty(name, value); 6856 return value; 6857 } 6858 6859 @Override 6860 public void removeChild(String name, Base value) throws FHIRException { 6861 if (name.equals("code")) { 6862 this.code = null; 6863 } else if (name.equals("status")) { 6864 this.status = null; 6865 } else if (name.equals("manufacturer")) { 6866 this.manufacturer = null; 6867 } else if (name.equals("doseForm")) { 6868 this.doseForm = null; 6869 } else if (name.equals("amount")) { 6870 this.amount = null; 6871 } else if (name.equals("synonym")) { 6872 this.getSynonym().remove(castToString(value)); 6873 } else if (name.equals("relatedMedicationKnowledge")) { 6874 this.getRelatedMedicationKnowledge().remove((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); 6875 } else if (name.equals("associatedMedication")) { 6876 this.getAssociatedMedication().remove(castToReference(value)); 6877 } else if (name.equals("productType")) { 6878 this.getProductType().remove(castToCodeableConcept(value)); 6879 } else if (name.equals("monograph")) { 6880 this.getMonograph().remove((MedicationKnowledgeMonographComponent) value); 6881 } else if (name.equals("ingredient")) { 6882 this.getIngredient().remove((MedicationKnowledgeIngredientComponent) value); 6883 } else if (name.equals("preparationInstruction")) { 6884 this.preparationInstruction = null; 6885 } else if (name.equals("intendedRoute")) { 6886 this.getIntendedRoute().remove(castToCodeableConcept(value)); 6887 } else if (name.equals("cost")) { 6888 this.getCost().remove((MedicationKnowledgeCostComponent) value); 6889 } else if (name.equals("monitoringProgram")) { 6890 this.getMonitoringProgram().remove((MedicationKnowledgeMonitoringProgramComponent) value); 6891 } else if (name.equals("administrationGuidelines")) { 6892 this.getAdministrationGuidelines().remove((MedicationKnowledgeAdministrationGuidelinesComponent) value); 6893 } else if (name.equals("medicineClassification")) { 6894 this.getMedicineClassification().remove((MedicationKnowledgeMedicineClassificationComponent) value); 6895 } else if (name.equals("packaging")) { 6896 this.packaging = (MedicationKnowledgePackagingComponent) value; // MedicationKnowledgePackagingComponent 6897 } else if (name.equals("drugCharacteristic")) { 6898 this.getDrugCharacteristic().remove((MedicationKnowledgeDrugCharacteristicComponent) value); 6899 } else if (name.equals("contraindication")) { 6900 this.getContraindication().remove(castToReference(value)); 6901 } else if (name.equals("regulatory")) { 6902 this.getRegulatory().remove((MedicationKnowledgeRegulatoryComponent) value); 6903 } else if (name.equals("kinetics")) { 6904 this.getKinetics().remove((MedicationKnowledgeKineticsComponent) value); 6905 } else 6906 super.removeChild(name, value); 6907 6908 } 6909 6910 @Override 6911 public Base makeProperty(int hash, String name) throws FHIRException { 6912 switch (hash) { 6913 case 3059181: 6914 return getCode(); 6915 case -892481550: 6916 return getStatusElement(); 6917 case -1969347631: 6918 return getManufacturer(); 6919 case 1303858817: 6920 return getDoseForm(); 6921 case -1413853096: 6922 return getAmount(); 6923 case -1742128133: 6924 return addSynonymElement(); 6925 case 723067972: 6926 return addRelatedMedicationKnowledge(); 6927 case 1312779381: 6928 return addAssociatedMedication(); 6929 case -1491615543: 6930 return addProductType(); 6931 case -1442980789: 6932 return addMonograph(); 6933 case -206409263: 6934 return addIngredient(); 6935 case 1025456503: 6936 return getPreparationInstructionElement(); 6937 case -767798050: 6938 return addIntendedRoute(); 6939 case 3059661: 6940 return addCost(); 6941 case 569848092: 6942 return addMonitoringProgram(); 6943 case 496930945: 6944 return addAdministrationGuidelines(); 6945 case 1791551680: 6946 return addMedicineClassification(); 6947 case 1802065795: 6948 return getPackaging(); 6949 case -844126885: 6950 return addDrugCharacteristic(); 6951 case 107135229: 6952 return addContraindication(); 6953 case -27327848: 6954 return addRegulatory(); 6955 case -553207110: 6956 return addKinetics(); 6957 default: 6958 return super.makeProperty(hash, name); 6959 } 6960 6961 } 6962 6963 @Override 6964 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6965 switch (hash) { 6966 case 3059181: 6967 /* code */ return new String[] { "CodeableConcept" }; 6968 case -892481550: 6969 /* status */ return new String[] { "code" }; 6970 case -1969347631: 6971 /* manufacturer */ return new String[] { "Reference" }; 6972 case 1303858817: 6973 /* doseForm */ return new String[] { "CodeableConcept" }; 6974 case -1413853096: 6975 /* amount */ return new String[] { "SimpleQuantity" }; 6976 case -1742128133: 6977 /* synonym */ return new String[] { "string" }; 6978 case 723067972: 6979 /* relatedMedicationKnowledge */ return new String[] {}; 6980 case 1312779381: 6981 /* associatedMedication */ return new String[] { "Reference" }; 6982 case -1491615543: 6983 /* productType */ return new String[] { "CodeableConcept" }; 6984 case -1442980789: 6985 /* monograph */ return new String[] {}; 6986 case -206409263: 6987 /* ingredient */ return new String[] {}; 6988 case 1025456503: 6989 /* preparationInstruction */ return new String[] { "markdown" }; 6990 case -767798050: 6991 /* intendedRoute */ return new String[] { "CodeableConcept" }; 6992 case 3059661: 6993 /* cost */ return new String[] {}; 6994 case 569848092: 6995 /* monitoringProgram */ return new String[] {}; 6996 case 496930945: 6997 /* administrationGuidelines */ return new String[] {}; 6998 case 1791551680: 6999 /* medicineClassification */ return new String[] {}; 7000 case 1802065795: 7001 /* packaging */ return new String[] {}; 7002 case -844126885: 7003 /* drugCharacteristic */ return new String[] {}; 7004 case 107135229: 7005 /* contraindication */ return new String[] { "Reference" }; 7006 case -27327848: 7007 /* regulatory */ return new String[] {}; 7008 case -553207110: 7009 /* kinetics */ return new String[] {}; 7010 default: 7011 return super.getTypesForProperty(hash, name); 7012 } 7013 7014 } 7015 7016 @Override 7017 public Base addChild(String name) throws FHIRException { 7018 if (name.equals("code")) { 7019 this.code = new CodeableConcept(); 7020 return this.code; 7021 } else if (name.equals("status")) { 7022 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.status"); 7023 } else if (name.equals("manufacturer")) { 7024 this.manufacturer = new Reference(); 7025 return this.manufacturer; 7026 } else if (name.equals("doseForm")) { 7027 this.doseForm = new CodeableConcept(); 7028 return this.doseForm; 7029 } else if (name.equals("amount")) { 7030 this.amount = new Quantity(); 7031 return this.amount; 7032 } else if (name.equals("synonym")) { 7033 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.synonym"); 7034 } else if (name.equals("relatedMedicationKnowledge")) { 7035 return addRelatedMedicationKnowledge(); 7036 } else if (name.equals("associatedMedication")) { 7037 return addAssociatedMedication(); 7038 } else if (name.equals("productType")) { 7039 return addProductType(); 7040 } else if (name.equals("monograph")) { 7041 return addMonograph(); 7042 } else if (name.equals("ingredient")) { 7043 return addIngredient(); 7044 } else if (name.equals("preparationInstruction")) { 7045 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.preparationInstruction"); 7046 } else if (name.equals("intendedRoute")) { 7047 return addIntendedRoute(); 7048 } else if (name.equals("cost")) { 7049 return addCost(); 7050 } else if (name.equals("monitoringProgram")) { 7051 return addMonitoringProgram(); 7052 } else if (name.equals("administrationGuidelines")) { 7053 return addAdministrationGuidelines(); 7054 } else if (name.equals("medicineClassification")) { 7055 return addMedicineClassification(); 7056 } else if (name.equals("packaging")) { 7057 this.packaging = new MedicationKnowledgePackagingComponent(); 7058 return this.packaging; 7059 } else if (name.equals("drugCharacteristic")) { 7060 return addDrugCharacteristic(); 7061 } else if (name.equals("contraindication")) { 7062 return addContraindication(); 7063 } else if (name.equals("regulatory")) { 7064 return addRegulatory(); 7065 } else if (name.equals("kinetics")) { 7066 return addKinetics(); 7067 } else 7068 return super.addChild(name); 7069 } 7070 7071 public String fhirType() { 7072 return "MedicationKnowledge"; 7073 7074 } 7075 7076 public MedicationKnowledge copy() { 7077 MedicationKnowledge dst = new MedicationKnowledge(); 7078 copyValues(dst); 7079 return dst; 7080 } 7081 7082 public void copyValues(MedicationKnowledge dst) { 7083 super.copyValues(dst); 7084 dst.code = code == null ? null : code.copy(); 7085 dst.status = status == null ? null : status.copy(); 7086 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 7087 dst.doseForm = doseForm == null ? null : doseForm.copy(); 7088 dst.amount = amount == null ? null : amount.copy(); 7089 if (synonym != null) { 7090 dst.synonym = new ArrayList<StringType>(); 7091 for (StringType i : synonym) 7092 dst.synonym.add(i.copy()); 7093 } 7094 ; 7095 if (relatedMedicationKnowledge != null) { 7096 dst.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 7097 for (MedicationKnowledgeRelatedMedicationKnowledgeComponent i : relatedMedicationKnowledge) 7098 dst.relatedMedicationKnowledge.add(i.copy()); 7099 } 7100 ; 7101 if (associatedMedication != null) { 7102 dst.associatedMedication = new ArrayList<Reference>(); 7103 for (Reference i : associatedMedication) 7104 dst.associatedMedication.add(i.copy()); 7105 } 7106 ; 7107 if (productType != null) { 7108 dst.productType = new ArrayList<CodeableConcept>(); 7109 for (CodeableConcept i : productType) 7110 dst.productType.add(i.copy()); 7111 } 7112 ; 7113 if (monograph != null) { 7114 dst.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 7115 for (MedicationKnowledgeMonographComponent i : monograph) 7116 dst.monograph.add(i.copy()); 7117 } 7118 ; 7119 if (ingredient != null) { 7120 dst.ingredient = new ArrayList<MedicationKnowledgeIngredientComponent>(); 7121 for (MedicationKnowledgeIngredientComponent i : ingredient) 7122 dst.ingredient.add(i.copy()); 7123 } 7124 ; 7125 dst.preparationInstruction = preparationInstruction == null ? null : preparationInstruction.copy(); 7126 if (intendedRoute != null) { 7127 dst.intendedRoute = new ArrayList<CodeableConcept>(); 7128 for (CodeableConcept i : intendedRoute) 7129 dst.intendedRoute.add(i.copy()); 7130 } 7131 ; 7132 if (cost != null) { 7133 dst.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 7134 for (MedicationKnowledgeCostComponent i : cost) 7135 dst.cost.add(i.copy()); 7136 } 7137 ; 7138 if (monitoringProgram != null) { 7139 dst.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 7140 for (MedicationKnowledgeMonitoringProgramComponent i : monitoringProgram) 7141 dst.monitoringProgram.add(i.copy()); 7142 } 7143 ; 7144 if (administrationGuidelines != null) { 7145 dst.administrationGuidelines = new ArrayList<MedicationKnowledgeAdministrationGuidelinesComponent>(); 7146 for (MedicationKnowledgeAdministrationGuidelinesComponent i : administrationGuidelines) 7147 dst.administrationGuidelines.add(i.copy()); 7148 } 7149 ; 7150 if (medicineClassification != null) { 7151 dst.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 7152 for (MedicationKnowledgeMedicineClassificationComponent i : medicineClassification) 7153 dst.medicineClassification.add(i.copy()); 7154 } 7155 ; 7156 dst.packaging = packaging == null ? null : packaging.copy(); 7157 if (drugCharacteristic != null) { 7158 dst.drugCharacteristic = new ArrayList<MedicationKnowledgeDrugCharacteristicComponent>(); 7159 for (MedicationKnowledgeDrugCharacteristicComponent i : drugCharacteristic) 7160 dst.drugCharacteristic.add(i.copy()); 7161 } 7162 ; 7163 if (contraindication != null) { 7164 dst.contraindication = new ArrayList<Reference>(); 7165 for (Reference i : contraindication) 7166 dst.contraindication.add(i.copy()); 7167 } 7168 ; 7169 if (regulatory != null) { 7170 dst.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 7171 for (MedicationKnowledgeRegulatoryComponent i : regulatory) 7172 dst.regulatory.add(i.copy()); 7173 } 7174 ; 7175 if (kinetics != null) { 7176 dst.kinetics = new ArrayList<MedicationKnowledgeKineticsComponent>(); 7177 for (MedicationKnowledgeKineticsComponent i : kinetics) 7178 dst.kinetics.add(i.copy()); 7179 } 7180 ; 7181 } 7182 7183 protected MedicationKnowledge typedCopy() { 7184 return copy(); 7185 } 7186 7187 @Override 7188 public boolean equalsDeep(Base other_) { 7189 if (!super.equalsDeep(other_)) 7190 return false; 7191 if (!(other_ instanceof MedicationKnowledge)) 7192 return false; 7193 MedicationKnowledge o = (MedicationKnowledge) other_; 7194 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true) 7195 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(doseForm, o.doseForm, true) 7196 && compareDeep(amount, o.amount, true) && compareDeep(synonym, o.synonym, true) 7197 && compareDeep(relatedMedicationKnowledge, o.relatedMedicationKnowledge, true) 7198 && compareDeep(associatedMedication, o.associatedMedication, true) 7199 && compareDeep(productType, o.productType, true) && compareDeep(monograph, o.monograph, true) 7200 && compareDeep(ingredient, o.ingredient, true) 7201 && compareDeep(preparationInstruction, o.preparationInstruction, true) 7202 && compareDeep(intendedRoute, o.intendedRoute, true) && compareDeep(cost, o.cost, true) 7203 && compareDeep(monitoringProgram, o.monitoringProgram, true) 7204 && compareDeep(administrationGuidelines, o.administrationGuidelines, true) 7205 && compareDeep(medicineClassification, o.medicineClassification, true) 7206 && compareDeep(packaging, o.packaging, true) && compareDeep(drugCharacteristic, o.drugCharacteristic, true) 7207 && compareDeep(contraindication, o.contraindication, true) && compareDeep(regulatory, o.regulatory, true) 7208 && compareDeep(kinetics, o.kinetics, true); 7209 } 7210 7211 @Override 7212 public boolean equalsShallow(Base other_) { 7213 if (!super.equalsShallow(other_)) 7214 return false; 7215 if (!(other_ instanceof MedicationKnowledge)) 7216 return false; 7217 MedicationKnowledge o = (MedicationKnowledge) other_; 7218 return compareValues(status, o.status, true) && compareValues(synonym, o.synonym, true) 7219 && compareValues(preparationInstruction, o.preparationInstruction, true); 7220 } 7221 7222 public boolean isEmpty() { 7223 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status, manufacturer, doseForm, amount, 7224 synonym, relatedMedicationKnowledge, associatedMedication, productType, monograph, ingredient, 7225 preparationInstruction, intendedRoute, cost, monitoringProgram, administrationGuidelines, 7226 medicineClassification, packaging, drugCharacteristic, contraindication, regulatory, kinetics); 7227 } 7228 7229 @Override 7230 public ResourceType getResourceType() { 7231 return ResourceType.MedicationKnowledge; 7232 } 7233 7234 /** 7235 * Search parameter: <b>code</b> 7236 * <p> 7237 * Description: <b>Code that identifies this medication</b><br> 7238 * Type: <b>token</b><br> 7239 * Path: <b>MedicationKnowledge.code</b><br> 7240 * </p> 7241 */ 7242 @SearchParamDefinition(name = "code", path = "MedicationKnowledge.code", description = "Code that identifies this medication", type = "token") 7243 public static final String SP_CODE = "code"; 7244 /** 7245 * <b>Fluent Client</b> search parameter constant for <b>code</b> 7246 * <p> 7247 * Description: <b>Code that identifies this medication</b><br> 7248 * Type: <b>token</b><br> 7249 * Path: <b>MedicationKnowledge.code</b><br> 7250 * </p> 7251 */ 7252 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7253 SP_CODE); 7254 7255 /** 7256 * Search parameter: <b>ingredient</b> 7257 * <p> 7258 * Description: <b>Medication(s) or substance(s) contained in the 7259 * medication</b><br> 7260 * Type: <b>reference</b><br> 7261 * Path: <b>MedicationKnowledge.ingredient.itemReference</b><br> 7262 * </p> 7263 */ 7264 @SearchParamDefinition(name = "ingredient", path = "(MedicationKnowledge.ingredient.item as Reference)", description = "Medication(s) or substance(s) contained in the medication", type = "reference", target = { 7265 Substance.class }) 7266 public static final String SP_INGREDIENT = "ingredient"; 7267 /** 7268 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 7269 * <p> 7270 * Description: <b>Medication(s) or substance(s) contained in the 7271 * medication</b><br> 7272 * Type: <b>reference</b><br> 7273 * Path: <b>MedicationKnowledge.ingredient.itemReference</b><br> 7274 * </p> 7275 */ 7276 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 7277 SP_INGREDIENT); 7278 7279 /** 7280 * Constant for fluent queries to be used to add include statements. Specifies 7281 * the path value of "<b>MedicationKnowledge:ingredient</b>". 7282 */ 7283 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include( 7284 "MedicationKnowledge:ingredient").toLocked(); 7285 7286 /** 7287 * Search parameter: <b>doseform</b> 7288 * <p> 7289 * Description: <b>powder | tablets | capsule +</b><br> 7290 * Type: <b>token</b><br> 7291 * Path: <b>MedicationKnowledge.doseForm</b><br> 7292 * </p> 7293 */ 7294 @SearchParamDefinition(name = "doseform", path = "MedicationKnowledge.doseForm", description = "powder | tablets | capsule +", type = "token") 7295 public static final String SP_DOSEFORM = "doseform"; 7296 /** 7297 * <b>Fluent Client</b> search parameter constant for <b>doseform</b> 7298 * <p> 7299 * Description: <b>powder | tablets | capsule +</b><br> 7300 * Type: <b>token</b><br> 7301 * Path: <b>MedicationKnowledge.doseForm</b><br> 7302 * </p> 7303 */ 7304 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSEFORM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7305 SP_DOSEFORM); 7306 7307 /** 7308 * Search parameter: <b>classification-type</b> 7309 * <p> 7310 * Description: <b>The type of category for the medication (for example, 7311 * therapeutic classification, therapeutic sub-classification)</b><br> 7312 * Type: <b>token</b><br> 7313 * Path: <b>MedicationKnowledge.medicineClassification.type</b><br> 7314 * </p> 7315 */ 7316 @SearchParamDefinition(name = "classification-type", path = "MedicationKnowledge.medicineClassification.type", description = "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)", type = "token") 7317 public static final String SP_CLASSIFICATION_TYPE = "classification-type"; 7318 /** 7319 * <b>Fluent Client</b> search parameter constant for <b>classification-type</b> 7320 * <p> 7321 * Description: <b>The type of category for the medication (for example, 7322 * therapeutic classification, therapeutic sub-classification)</b><br> 7323 * Type: <b>token</b><br> 7324 * Path: <b>MedicationKnowledge.medicineClassification.type</b><br> 7325 * </p> 7326 */ 7327 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7328 SP_CLASSIFICATION_TYPE); 7329 7330 /** 7331 * Search parameter: <b>monograph-type</b> 7332 * <p> 7333 * Description: <b>The category of medication document</b><br> 7334 * Type: <b>token</b><br> 7335 * Path: <b>MedicationKnowledge.monograph.type</b><br> 7336 * </p> 7337 */ 7338 @SearchParamDefinition(name = "monograph-type", path = "MedicationKnowledge.monograph.type", description = "The category of medication document", type = "token") 7339 public static final String SP_MONOGRAPH_TYPE = "monograph-type"; 7340 /** 7341 * <b>Fluent Client</b> search parameter constant for <b>monograph-type</b> 7342 * <p> 7343 * Description: <b>The category of medication document</b><br> 7344 * Type: <b>token</b><br> 7345 * Path: <b>MedicationKnowledge.monograph.type</b><br> 7346 * </p> 7347 */ 7348 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONOGRAPH_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7349 SP_MONOGRAPH_TYPE); 7350 7351 /** 7352 * Search parameter: <b>classification</b> 7353 * <p> 7354 * Description: <b>Specific category assigned to the medication</b><br> 7355 * Type: <b>token</b><br> 7356 * Path: <b>MedicationKnowledge.medicineClassification.classification</b><br> 7357 * </p> 7358 */ 7359 @SearchParamDefinition(name = "classification", path = "MedicationKnowledge.medicineClassification.classification", description = "Specific category assigned to the medication", type = "token") 7360 public static final String SP_CLASSIFICATION = "classification"; 7361 /** 7362 * <b>Fluent Client</b> search parameter constant for <b>classification</b> 7363 * <p> 7364 * Description: <b>Specific category assigned to the medication</b><br> 7365 * Type: <b>token</b><br> 7366 * Path: <b>MedicationKnowledge.medicineClassification.classification</b><br> 7367 * </p> 7368 */ 7369 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7370 SP_CLASSIFICATION); 7371 7372 /** 7373 * Search parameter: <b>manufacturer</b> 7374 * <p> 7375 * Description: <b>Manufacturer of the item</b><br> 7376 * Type: <b>reference</b><br> 7377 * Path: <b>MedicationKnowledge.manufacturer</b><br> 7378 * </p> 7379 */ 7380 @SearchParamDefinition(name = "manufacturer", path = "MedicationKnowledge.manufacturer", description = "Manufacturer of the item", type = "reference", target = { 7381 Organization.class }) 7382 public static final String SP_MANUFACTURER = "manufacturer"; 7383 /** 7384 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 7385 * <p> 7386 * Description: <b>Manufacturer of the item</b><br> 7387 * Type: <b>reference</b><br> 7388 * Path: <b>MedicationKnowledge.manufacturer</b><br> 7389 * </p> 7390 */ 7391 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 7392 SP_MANUFACTURER); 7393 7394 /** 7395 * Constant for fluent queries to be used to add include statements. Specifies 7396 * the path value of "<b>MedicationKnowledge:manufacturer</b>". 7397 */ 7398 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include( 7399 "MedicationKnowledge:manufacturer").toLocked(); 7400 7401 /** 7402 * Search parameter: <b>ingredient-code</b> 7403 * <p> 7404 * Description: <b>Medication(s) or substance(s) contained in the 7405 * medication</b><br> 7406 * Type: <b>token</b><br> 7407 * Path: <b>MedicationKnowledge.ingredient.itemCodeableConcept</b><br> 7408 * </p> 7409 */ 7410 @SearchParamDefinition(name = "ingredient-code", path = "(MedicationKnowledge.ingredient.item as CodeableConcept)", description = "Medication(s) or substance(s) contained in the medication", type = "token") 7411 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 7412 /** 7413 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 7414 * <p> 7415 * Description: <b>Medication(s) or substance(s) contained in the 7416 * medication</b><br> 7417 * Type: <b>token</b><br> 7418 * Path: <b>MedicationKnowledge.ingredient.itemCodeableConcept</b><br> 7419 * </p> 7420 */ 7421 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7422 SP_INGREDIENT_CODE); 7423 7424 /** 7425 * Search parameter: <b>source-cost</b> 7426 * <p> 7427 * Description: <b>The source or owner for the price information</b><br> 7428 * Type: <b>token</b><br> 7429 * Path: <b>MedicationKnowledge.cost.source</b><br> 7430 * </p> 7431 */ 7432 @SearchParamDefinition(name = "source-cost", path = "MedicationKnowledge.cost.source", description = "The source or owner for the price information", type = "token") 7433 public static final String SP_SOURCE_COST = "source-cost"; 7434 /** 7435 * <b>Fluent Client</b> search parameter constant for <b>source-cost</b> 7436 * <p> 7437 * Description: <b>The source or owner for the price information</b><br> 7438 * Type: <b>token</b><br> 7439 * Path: <b>MedicationKnowledge.cost.source</b><br> 7440 * </p> 7441 */ 7442 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_COST = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7443 SP_SOURCE_COST); 7444 7445 /** 7446 * Search parameter: <b>monograph</b> 7447 * <p> 7448 * Description: <b>Associated documentation about the medication</b><br> 7449 * Type: <b>reference</b><br> 7450 * Path: <b>MedicationKnowledge.monograph.source</b><br> 7451 * </p> 7452 */ 7453 @SearchParamDefinition(name = "monograph", path = "MedicationKnowledge.monograph.source", description = "Associated documentation about the medication", type = "reference", target = { 7454 DocumentReference.class, Media.class }) 7455 public static final String SP_MONOGRAPH = "monograph"; 7456 /** 7457 * <b>Fluent Client</b> search parameter constant for <b>monograph</b> 7458 * <p> 7459 * Description: <b>Associated documentation about the medication</b><br> 7460 * Type: <b>reference</b><br> 7461 * Path: <b>MedicationKnowledge.monograph.source</b><br> 7462 * </p> 7463 */ 7464 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MONOGRAPH = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 7465 SP_MONOGRAPH); 7466 7467 /** 7468 * Constant for fluent queries to be used to add include statements. Specifies 7469 * the path value of "<b>MedicationKnowledge:monograph</b>". 7470 */ 7471 public static final ca.uhn.fhir.model.api.Include INCLUDE_MONOGRAPH = new ca.uhn.fhir.model.api.Include( 7472 "MedicationKnowledge:monograph").toLocked(); 7473 7474 /** 7475 * Search parameter: <b>monitoring-program-name</b> 7476 * <p> 7477 * Description: <b>Name of the reviewing program</b><br> 7478 * Type: <b>token</b><br> 7479 * Path: <b>MedicationKnowledge.monitoringProgram.name</b><br> 7480 * </p> 7481 */ 7482 @SearchParamDefinition(name = "monitoring-program-name", path = "MedicationKnowledge.monitoringProgram.name", description = "Name of the reviewing program", type = "token") 7483 public static final String SP_MONITORING_PROGRAM_NAME = "monitoring-program-name"; 7484 /** 7485 * <b>Fluent Client</b> search parameter constant for 7486 * <b>monitoring-program-name</b> 7487 * <p> 7488 * Description: <b>Name of the reviewing program</b><br> 7489 * Type: <b>token</b><br> 7490 * Path: <b>MedicationKnowledge.monitoringProgram.name</b><br> 7491 * </p> 7492 */ 7493 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONITORING_PROGRAM_NAME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7494 SP_MONITORING_PROGRAM_NAME); 7495 7496 /** 7497 * Search parameter: <b>monitoring-program-type</b> 7498 * <p> 7499 * Description: <b>Type of program under which the medication is 7500 * monitored</b><br> 7501 * Type: <b>token</b><br> 7502 * Path: <b>MedicationKnowledge.monitoringProgram.type</b><br> 7503 * </p> 7504 */ 7505 @SearchParamDefinition(name = "monitoring-program-type", path = "MedicationKnowledge.monitoringProgram.type", description = "Type of program under which the medication is monitored", type = "token") 7506 public static final String SP_MONITORING_PROGRAM_TYPE = "monitoring-program-type"; 7507 /** 7508 * <b>Fluent Client</b> search parameter constant for 7509 * <b>monitoring-program-type</b> 7510 * <p> 7511 * Description: <b>Type of program under which the medication is 7512 * monitored</b><br> 7513 * Type: <b>token</b><br> 7514 * Path: <b>MedicationKnowledge.monitoringProgram.type</b><br> 7515 * </p> 7516 */ 7517 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONITORING_PROGRAM_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7518 SP_MONITORING_PROGRAM_TYPE); 7519 7520 /** 7521 * Search parameter: <b>status</b> 7522 * <p> 7523 * Description: <b>active | inactive | entered-in-error</b><br> 7524 * Type: <b>token</b><br> 7525 * Path: <b>MedicationKnowledge.status</b><br> 7526 * </p> 7527 */ 7528 @SearchParamDefinition(name = "status", path = "MedicationKnowledge.status", description = "active | inactive | entered-in-error", type = "token") 7529 public static final String SP_STATUS = "status"; 7530 /** 7531 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7532 * <p> 7533 * Description: <b>active | inactive | entered-in-error</b><br> 7534 * Type: <b>token</b><br> 7535 * Path: <b>MedicationKnowledge.status</b><br> 7536 * </p> 7537 */ 7538 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7539 SP_STATUS); 7540 7541}