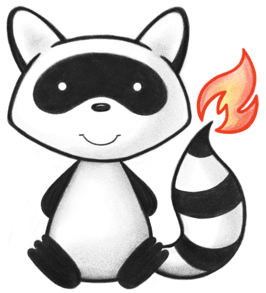
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A record of a medication that is being consumed by a patient. A 046 * MedicationStatement may indicate that the patient may be taking the 047 * medication now or has taken the medication in the past or will be taking the 048 * medication in the future. The source of this information can be the patient, 049 * significant other (such as a family member or spouse), or a clinician. A 050 * common scenario where this information is captured is during the history 051 * taking process during a patient visit or stay. The medication information may 052 * come from sources such as the patient's memory, from a prescription bottle, 053 * or from a list of medications the patient, clinician or other party 054 * maintains. 055 * 056 * The primary difference between a medication statement and a medication 057 * administration is that the medication administration has complete 058 * administration information and is based on actual administration information 059 * from the person who administered the medication. A medication statement is 060 * often, if not always, less specific. There is no required date/time when the 061 * medication was administered, in fact we only know that a source has reported 062 * the patient is taking this medication, where details such as time, quantity, 063 * or rate or even medication product may be incomplete or missing or less 064 * precise. As stated earlier, the medication statement information may come 065 * from the patient's memory, from a prescription bottle or from a list of 066 * medications the patient, clinician or other party maintains. Medication 067 * administration is more formal and is not missing detailed information. 068 */ 069@ResourceDef(name = "MedicationStatement", profile = "http://hl7.org/fhir/StructureDefinition/MedicationStatement") 070public class MedicationStatement extends DomainResource { 071 072 public enum MedicationStatementStatus { 073 /** 074 * The medication is still being taken. 075 */ 076 ACTIVE, 077 /** 078 * The medication is no longer being taken. 079 */ 080 COMPLETED, 081 /** 082 * Some of the actions that are implied by the medication statement may have 083 * occurred. For example, the patient may have taken some of the medication. 084 * Clinical decision support systems should take this status into account. 085 */ 086 ENTEREDINERROR, 087 /** 088 * The medication may be taken at some time in the future. 089 */ 090 INTENDED, 091 /** 092 * Actions implied by the statement have been permanently halted, before all of 093 * them occurred. This should not be used if the statement was entered in error. 094 */ 095 STOPPED, 096 /** 097 * Actions implied by the statement have been temporarily halted, but are 098 * expected to continue later. May also be called 'suspended'. 099 */ 100 ONHOLD, 101 /** 102 * The state of the medication use is not currently known. 103 */ 104 UNKNOWN, 105 /** 106 * The medication was not consumed by the patient 107 */ 108 NOTTAKEN, 109 /** 110 * added to help the parsers with the generic types 111 */ 112 NULL; 113 114 public static MedicationStatementStatus fromCode(String codeString) throws FHIRException { 115 if (codeString == null || "".equals(codeString)) 116 return null; 117 if ("active".equals(codeString)) 118 return ACTIVE; 119 if ("completed".equals(codeString)) 120 return COMPLETED; 121 if ("entered-in-error".equals(codeString)) 122 return ENTEREDINERROR; 123 if ("intended".equals(codeString)) 124 return INTENDED; 125 if ("stopped".equals(codeString)) 126 return STOPPED; 127 if ("on-hold".equals(codeString)) 128 return ONHOLD; 129 if ("unknown".equals(codeString)) 130 return UNKNOWN; 131 if ("not-taken".equals(codeString)) 132 return NOTTAKEN; 133 if (Configuration.isAcceptInvalidEnums()) 134 return null; 135 else 136 throw new FHIRException("Unknown MedicationStatementStatus code '" + codeString + "'"); 137 } 138 139 public String toCode() { 140 switch (this) { 141 case ACTIVE: 142 return "active"; 143 case COMPLETED: 144 return "completed"; 145 case ENTEREDINERROR: 146 return "entered-in-error"; 147 case INTENDED: 148 return "intended"; 149 case STOPPED: 150 return "stopped"; 151 case ONHOLD: 152 return "on-hold"; 153 case UNKNOWN: 154 return "unknown"; 155 case NOTTAKEN: 156 return "not-taken"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getSystem() { 165 switch (this) { 166 case ACTIVE: 167 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 168 case COMPLETED: 169 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 170 case ENTEREDINERROR: 171 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 172 case INTENDED: 173 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 174 case STOPPED: 175 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 176 case ONHOLD: 177 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 178 case UNKNOWN: 179 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 180 case NOTTAKEN: 181 return "http://hl7.org/fhir/CodeSystem/medication-statement-status"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 189 public String getDefinition() { 190 switch (this) { 191 case ACTIVE: 192 return "The medication is still being taken."; 193 case COMPLETED: 194 return "The medication is no longer being taken."; 195 case ENTEREDINERROR: 196 return "Some of the actions that are implied by the medication statement may have occurred. For example, the patient may have taken some of the medication. Clinical decision support systems should take this status into account."; 197 case INTENDED: 198 return "The medication may be taken at some time in the future."; 199 case STOPPED: 200 return "Actions implied by the statement have been permanently halted, before all of them occurred. This should not be used if the statement was entered in error."; 201 case ONHOLD: 202 return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called 'suspended'."; 203 case UNKNOWN: 204 return "The state of the medication use is not currently known."; 205 case NOTTAKEN: 206 return "The medication was not consumed by the patient"; 207 case NULL: 208 return null; 209 default: 210 return "?"; 211 } 212 } 213 214 public String getDisplay() { 215 switch (this) { 216 case ACTIVE: 217 return "Active"; 218 case COMPLETED: 219 return "Completed"; 220 case ENTEREDINERROR: 221 return "Entered in Error"; 222 case INTENDED: 223 return "Intended"; 224 case STOPPED: 225 return "Stopped"; 226 case ONHOLD: 227 return "On Hold"; 228 case UNKNOWN: 229 return "Unknown"; 230 case NOTTAKEN: 231 return "Not Taken"; 232 case NULL: 233 return null; 234 default: 235 return "?"; 236 } 237 } 238 } 239 240 public static class MedicationStatementStatusEnumFactory implements EnumFactory<MedicationStatementStatus> { 241 public MedicationStatementStatus fromCode(String codeString) throws IllegalArgumentException { 242 if (codeString == null || "".equals(codeString)) 243 if (codeString == null || "".equals(codeString)) 244 return null; 245 if ("active".equals(codeString)) 246 return MedicationStatementStatus.ACTIVE; 247 if ("completed".equals(codeString)) 248 return MedicationStatementStatus.COMPLETED; 249 if ("entered-in-error".equals(codeString)) 250 return MedicationStatementStatus.ENTEREDINERROR; 251 if ("intended".equals(codeString)) 252 return MedicationStatementStatus.INTENDED; 253 if ("stopped".equals(codeString)) 254 return MedicationStatementStatus.STOPPED; 255 if ("on-hold".equals(codeString)) 256 return MedicationStatementStatus.ONHOLD; 257 if ("unknown".equals(codeString)) 258 return MedicationStatementStatus.UNKNOWN; 259 if ("not-taken".equals(codeString)) 260 return MedicationStatementStatus.NOTTAKEN; 261 throw new IllegalArgumentException("Unknown MedicationStatementStatus code '" + codeString + "'"); 262 } 263 264 public Enumeration<MedicationStatementStatus> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.NULL, code); 269 String codeString = code.asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.NULL, code); 272 if ("active".equals(codeString)) 273 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ACTIVE, code); 274 if ("completed".equals(codeString)) 275 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.COMPLETED, code); 276 if ("entered-in-error".equals(codeString)) 277 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ENTEREDINERROR, code); 278 if ("intended".equals(codeString)) 279 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.INTENDED, code); 280 if ("stopped".equals(codeString)) 281 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.STOPPED, code); 282 if ("on-hold".equals(codeString)) 283 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ONHOLD, code); 284 if ("unknown".equals(codeString)) 285 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.UNKNOWN, code); 286 if ("not-taken".equals(codeString)) 287 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.NOTTAKEN, code); 288 throw new FHIRException("Unknown MedicationStatementStatus code '" + codeString + "'"); 289 } 290 291 public String toCode(MedicationStatementStatus code) { 292 if (code == MedicationStatementStatus.NULL) 293 return null; 294 if (code == MedicationStatementStatus.ACTIVE) 295 return "active"; 296 if (code == MedicationStatementStatus.COMPLETED) 297 return "completed"; 298 if (code == MedicationStatementStatus.ENTEREDINERROR) 299 return "entered-in-error"; 300 if (code == MedicationStatementStatus.INTENDED) 301 return "intended"; 302 if (code == MedicationStatementStatus.STOPPED) 303 return "stopped"; 304 if (code == MedicationStatementStatus.ONHOLD) 305 return "on-hold"; 306 if (code == MedicationStatementStatus.UNKNOWN) 307 return "unknown"; 308 if (code == MedicationStatementStatus.NOTTAKEN) 309 return "not-taken"; 310 return "?"; 311 } 312 313 public String toSystem(MedicationStatementStatus code) { 314 return code.getSystem(); 315 } 316 } 317 318 /** 319 * Identifiers associated with this Medication Statement that are defined by 320 * business processes and/or used to refer to it when a direct URL reference to 321 * the resource itself is not appropriate. They are business identifiers 322 * assigned to this resource by the performer or other systems and remain 323 * constant as the resource is updated and propagates from server to server. 324 */ 325 @Child(name = "identifier", type = { 326 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 327 @Description(shortDefinition = "External identifier", formalDefinition = "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.") 328 protected List<Identifier> identifier; 329 330 /** 331 * A plan, proposal or order that is fulfilled in whole or in part by this 332 * event. 333 */ 334 @Child(name = "basedOn", type = { MedicationRequest.class, CarePlan.class, 335 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 336 @Description(shortDefinition = "Fulfils plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this event.") 337 protected List<Reference> basedOn; 338 /** 339 * The actual objects that are the target of the reference (A plan, proposal or 340 * order that is fulfilled in whole or in part by this event.) 341 */ 342 protected List<Resource> basedOnTarget; 343 344 /** 345 * A larger event of which this particular event is a component or step. 346 */ 347 @Child(name = "partOf", type = { MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, 348 Procedure.class, 349 Observation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 350 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 351 protected List<Reference> partOf; 352 /** 353 * The actual objects that are the target of the reference (A larger event of 354 * which this particular event is a component or step.) 355 */ 356 protected List<Resource> partOfTarget; 357 358 /** 359 * A code representing the patient or other source's judgment about the state of 360 * the medication used that this statement is about. Generally, this will be 361 * active or completed. 362 */ 363 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 364 @Description(shortDefinition = "active | completed | entered-in-error | intended | stopped | on-hold | unknown | not-taken", formalDefinition = "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally, this will be active or completed.") 365 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-statement-status") 366 protected Enumeration<MedicationStatementStatus> status; 367 368 /** 369 * Captures the reason for the current state of the MedicationStatement. 370 */ 371 @Child(name = "statusReason", type = { 372 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 373 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the MedicationStatement.") 374 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reason-medication-status-codes") 375 protected List<CodeableConcept> statusReason; 376 377 /** 378 * Indicates where the medication is expected to be consumed or administered. 379 */ 380 @Child(name = "category", type = { 381 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 382 @Description(shortDefinition = "Type of medication usage", formalDefinition = "Indicates where the medication is expected to be consumed or administered.") 383 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-statement-category") 384 protected CodeableConcept category; 385 386 /** 387 * Identifies the medication being administered. This is either a link to a 388 * resource representing the details of the medication or a simple attribute 389 * carrying a code that identifies the medication from a known list of 390 * medications. 391 */ 392 @Child(name = "medication", type = { CodeableConcept.class, 393 Medication.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 394 @Description(shortDefinition = "What medication was taken", formalDefinition = "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 395 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 396 protected Type medication; 397 398 /** 399 * The person, animal or group who is/was taking the medication. 400 */ 401 @Child(name = "subject", type = { Patient.class, 402 Group.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 403 @Description(shortDefinition = "Who is/was taking the medication", formalDefinition = "The person, animal or group who is/was taking the medication.") 404 protected Reference subject; 405 406 /** 407 * The actual object that is the target of the reference (The person, animal or 408 * group who is/was taking the medication.) 409 */ 410 protected Resource subjectTarget; 411 412 /** 413 * The encounter or episode of care that establishes the context for this 414 * MedicationStatement. 415 */ 416 @Child(name = "context", type = { Encounter.class, 417 EpisodeOfCare.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 418 @Description(shortDefinition = "Encounter / Episode associated with MedicationStatement", formalDefinition = "The encounter or episode of care that establishes the context for this MedicationStatement.") 419 protected Reference context; 420 421 /** 422 * The actual object that is the target of the reference (The encounter or 423 * episode of care that establishes the context for this MedicationStatement.) 424 */ 425 protected Resource contextTarget; 426 427 /** 428 * The interval of time during which it is being asserted that the patient 429 * is/was/will be taking the medication (or was not taking, when the 430 * MedicationStatement.taken element is No). 431 */ 432 @Child(name = "effective", type = { DateTimeType.class, 433 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 434 @Description(shortDefinition = "The date/time or interval when the medication is/was/will be taken", formalDefinition = "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.taken element is No).") 435 protected Type effective; 436 437 /** 438 * The date when the medication statement was asserted by the information 439 * source. 440 */ 441 @Child(name = "dateAsserted", type = { 442 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 443 @Description(shortDefinition = "When the statement was asserted?", formalDefinition = "The date when the medication statement was asserted by the information source.") 444 protected DateTimeType dateAsserted; 445 446 /** 447 * The person or organization that provided the information about the taking of 448 * this medication. Note: Use derivedFrom when a MedicationStatement is derived 449 * from other resources, e.g. Claim or MedicationRequest. 450 */ 451 @Child(name = "informationSource", type = { Patient.class, Practitioner.class, PractitionerRole.class, 452 RelatedPerson.class, Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 453 @Description(shortDefinition = "Person or organization that provided the information about the taking of this medication", formalDefinition = "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.") 454 protected Reference informationSource; 455 456 /** 457 * The actual object that is the target of the reference (The person or 458 * organization that provided the information about the taking of this 459 * medication. Note: Use derivedFrom when a MedicationStatement is derived from 460 * other resources, e.g. Claim or MedicationRequest.) 461 */ 462 protected Resource informationSourceTarget; 463 464 /** 465 * Allows linking the MedicationStatement to the underlying MedicationRequest, 466 * or to other information that supports or is used to derive the 467 * MedicationStatement. 468 */ 469 @Child(name = "derivedFrom", type = { 470 Reference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 471 @Description(shortDefinition = "Additional supporting information", formalDefinition = "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.") 472 protected List<Reference> derivedFrom; 473 /** 474 * The actual objects that are the target of the reference (Allows linking the 475 * MedicationStatement to the underlying MedicationRequest, or to other 476 * information that supports or is used to derive the MedicationStatement.) 477 */ 478 protected List<Resource> derivedFromTarget; 479 480 /** 481 * A reason for why the medication is being/was taken. 482 */ 483 @Child(name = "reasonCode", type = { 484 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 485 @Description(shortDefinition = "Reason for why the medication is being/was taken", formalDefinition = "A reason for why the medication is being/was taken.") 486 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 487 protected List<CodeableConcept> reasonCode; 488 489 /** 490 * Condition or observation that supports why the medication is being/was taken. 491 */ 492 @Child(name = "reasonReference", type = { Condition.class, Observation.class, 493 DiagnosticReport.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 494 @Description(shortDefinition = "Condition or observation that supports why the medication is being/was taken", formalDefinition = "Condition or observation that supports why the medication is being/was taken.") 495 protected List<Reference> reasonReference; 496 /** 497 * The actual objects that are the target of the reference (Condition or 498 * observation that supports why the medication is being/was taken.) 499 */ 500 protected List<Resource> reasonReferenceTarget; 501 502 /** 503 * Provides extra information about the medication statement that is not 504 * conveyed by the other attributes. 505 */ 506 @Child(name = "note", type = { 507 Annotation.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 508 @Description(shortDefinition = "Further information about the statement", formalDefinition = "Provides extra information about the medication statement that is not conveyed by the other attributes.") 509 protected List<Annotation> note; 510 511 /** 512 * Indicates how the medication is/was or should be taken by the patient. 513 */ 514 @Child(name = "dosage", type = { 515 Dosage.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 516 @Description(shortDefinition = "Details of how medication is/was taken or should be taken", formalDefinition = "Indicates how the medication is/was or should be taken by the patient.") 517 protected List<Dosage> dosage; 518 519 private static final long serialVersionUID = 1912813418L; 520 521 /** 522 * Constructor 523 */ 524 public MedicationStatement() { 525 super(); 526 } 527 528 /** 529 * Constructor 530 */ 531 public MedicationStatement(Enumeration<MedicationStatementStatus> status, Type medication, Reference subject) { 532 super(); 533 this.status = status; 534 this.medication = medication; 535 this.subject = subject; 536 } 537 538 /** 539 * @return {@link #identifier} (Identifiers associated with this Medication 540 * Statement that are defined by business processes and/or used to refer 541 * to it when a direct URL reference to the resource itself is not 542 * appropriate. They are business identifiers assigned to this resource 543 * by the performer or other systems and remain constant as the resource 544 * is updated and propagates from server to server.) 545 */ 546 public List<Identifier> getIdentifier() { 547 if (this.identifier == null) 548 this.identifier = new ArrayList<Identifier>(); 549 return this.identifier; 550 } 551 552 /** 553 * @return Returns a reference to <code>this</code> for easy method chaining 554 */ 555 public MedicationStatement setIdentifier(List<Identifier> theIdentifier) { 556 this.identifier = theIdentifier; 557 return this; 558 } 559 560 public boolean hasIdentifier() { 561 if (this.identifier == null) 562 return false; 563 for (Identifier item : this.identifier) 564 if (!item.isEmpty()) 565 return true; 566 return false; 567 } 568 569 public Identifier addIdentifier() { // 3 570 Identifier t = new Identifier(); 571 if (this.identifier == null) 572 this.identifier = new ArrayList<Identifier>(); 573 this.identifier.add(t); 574 return t; 575 } 576 577 public MedicationStatement addIdentifier(Identifier t) { // 3 578 if (t == null) 579 return this; 580 if (this.identifier == null) 581 this.identifier = new ArrayList<Identifier>(); 582 this.identifier.add(t); 583 return this; 584 } 585 586 /** 587 * @return The first repetition of repeating field {@link #identifier}, creating 588 * it if it does not already exist 589 */ 590 public Identifier getIdentifierFirstRep() { 591 if (getIdentifier().isEmpty()) { 592 addIdentifier(); 593 } 594 return getIdentifier().get(0); 595 } 596 597 /** 598 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 599 * whole or in part by this event.) 600 */ 601 public List<Reference> getBasedOn() { 602 if (this.basedOn == null) 603 this.basedOn = new ArrayList<Reference>(); 604 return this.basedOn; 605 } 606 607 /** 608 * @return Returns a reference to <code>this</code> for easy method chaining 609 */ 610 public MedicationStatement setBasedOn(List<Reference> theBasedOn) { 611 this.basedOn = theBasedOn; 612 return this; 613 } 614 615 public boolean hasBasedOn() { 616 if (this.basedOn == null) 617 return false; 618 for (Reference item : this.basedOn) 619 if (!item.isEmpty()) 620 return true; 621 return false; 622 } 623 624 public Reference addBasedOn() { // 3 625 Reference t = new Reference(); 626 if (this.basedOn == null) 627 this.basedOn = new ArrayList<Reference>(); 628 this.basedOn.add(t); 629 return t; 630 } 631 632 public MedicationStatement addBasedOn(Reference t) { // 3 633 if (t == null) 634 return this; 635 if (this.basedOn == null) 636 this.basedOn = new ArrayList<Reference>(); 637 this.basedOn.add(t); 638 return this; 639 } 640 641 /** 642 * @return The first repetition of repeating field {@link #basedOn}, creating it 643 * if it does not already exist 644 */ 645 public Reference getBasedOnFirstRep() { 646 if (getBasedOn().isEmpty()) { 647 addBasedOn(); 648 } 649 return getBasedOn().get(0); 650 } 651 652 /** 653 * @deprecated Use Reference#setResource(IBaseResource) instead 654 */ 655 @Deprecated 656 public List<Resource> getBasedOnTarget() { 657 if (this.basedOnTarget == null) 658 this.basedOnTarget = new ArrayList<Resource>(); 659 return this.basedOnTarget; 660 } 661 662 /** 663 * @return {@link #partOf} (A larger event of which this particular event is a 664 * component or step.) 665 */ 666 public List<Reference> getPartOf() { 667 if (this.partOf == null) 668 this.partOf = new ArrayList<Reference>(); 669 return this.partOf; 670 } 671 672 /** 673 * @return Returns a reference to <code>this</code> for easy method chaining 674 */ 675 public MedicationStatement setPartOf(List<Reference> thePartOf) { 676 this.partOf = thePartOf; 677 return this; 678 } 679 680 public boolean hasPartOf() { 681 if (this.partOf == null) 682 return false; 683 for (Reference item : this.partOf) 684 if (!item.isEmpty()) 685 return true; 686 return false; 687 } 688 689 public Reference addPartOf() { // 3 690 Reference t = new Reference(); 691 if (this.partOf == null) 692 this.partOf = new ArrayList<Reference>(); 693 this.partOf.add(t); 694 return t; 695 } 696 697 public MedicationStatement addPartOf(Reference t) { // 3 698 if (t == null) 699 return this; 700 if (this.partOf == null) 701 this.partOf = new ArrayList<Reference>(); 702 this.partOf.add(t); 703 return this; 704 } 705 706 /** 707 * @return The first repetition of repeating field {@link #partOf}, creating it 708 * if it does not already exist 709 */ 710 public Reference getPartOfFirstRep() { 711 if (getPartOf().isEmpty()) { 712 addPartOf(); 713 } 714 return getPartOf().get(0); 715 } 716 717 /** 718 * @deprecated Use Reference#setResource(IBaseResource) instead 719 */ 720 @Deprecated 721 public List<Resource> getPartOfTarget() { 722 if (this.partOfTarget == null) 723 this.partOfTarget = new ArrayList<Resource>(); 724 return this.partOfTarget; 725 } 726 727 /** 728 * @return {@link #status} (A code representing the patient or other source's 729 * judgment about the state of the medication used that this statement 730 * is about. Generally, this will be active or completed.). This is the 731 * underlying object with id, value and extensions. The accessor 732 * "getStatus" gives direct access to the value 733 */ 734 public Enumeration<MedicationStatementStatus> getStatusElement() { 735 if (this.status == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create MedicationStatement.status"); 738 else if (Configuration.doAutoCreate()) 739 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); // bb 740 return this.status; 741 } 742 743 public boolean hasStatusElement() { 744 return this.status != null && !this.status.isEmpty(); 745 } 746 747 public boolean hasStatus() { 748 return this.status != null && !this.status.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #status} (A code representing the patient or other 753 * source's judgment about the state of the medication used that 754 * this statement is about. Generally, this will be active or 755 * completed.). This is the underlying object with id, value and 756 * extensions. The accessor "getStatus" gives direct access to the 757 * value 758 */ 759 public MedicationStatement setStatusElement(Enumeration<MedicationStatementStatus> value) { 760 this.status = value; 761 return this; 762 } 763 764 /** 765 * @return A code representing the patient or other source's judgment about the 766 * state of the medication used that this statement is about. Generally, 767 * this will be active or completed. 768 */ 769 public MedicationStatementStatus getStatus() { 770 return this.status == null ? null : this.status.getValue(); 771 } 772 773 /** 774 * @param value A code representing the patient or other source's judgment about 775 * the state of the medication used that this statement is about. 776 * Generally, this will be active or completed. 777 */ 778 public MedicationStatement setStatus(MedicationStatementStatus value) { 779 if (this.status == null) 780 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); 781 this.status.setValue(value); 782 return this; 783 } 784 785 /** 786 * @return {@link #statusReason} (Captures the reason for the current state of 787 * the MedicationStatement.) 788 */ 789 public List<CodeableConcept> getStatusReason() { 790 if (this.statusReason == null) 791 this.statusReason = new ArrayList<CodeableConcept>(); 792 return this.statusReason; 793 } 794 795 /** 796 * @return Returns a reference to <code>this</code> for easy method chaining 797 */ 798 public MedicationStatement setStatusReason(List<CodeableConcept> theStatusReason) { 799 this.statusReason = theStatusReason; 800 return this; 801 } 802 803 public boolean hasStatusReason() { 804 if (this.statusReason == null) 805 return false; 806 for (CodeableConcept item : this.statusReason) 807 if (!item.isEmpty()) 808 return true; 809 return false; 810 } 811 812 public CodeableConcept addStatusReason() { // 3 813 CodeableConcept t = new CodeableConcept(); 814 if (this.statusReason == null) 815 this.statusReason = new ArrayList<CodeableConcept>(); 816 this.statusReason.add(t); 817 return t; 818 } 819 820 public MedicationStatement addStatusReason(CodeableConcept t) { // 3 821 if (t == null) 822 return this; 823 if (this.statusReason == null) 824 this.statusReason = new ArrayList<CodeableConcept>(); 825 this.statusReason.add(t); 826 return this; 827 } 828 829 /** 830 * @return The first repetition of repeating field {@link #statusReason}, 831 * creating it if it does not already exist 832 */ 833 public CodeableConcept getStatusReasonFirstRep() { 834 if (getStatusReason().isEmpty()) { 835 addStatusReason(); 836 } 837 return getStatusReason().get(0); 838 } 839 840 /** 841 * @return {@link #category} (Indicates where the medication is expected to be 842 * consumed or administered.) 843 */ 844 public CodeableConcept getCategory() { 845 if (this.category == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create MedicationStatement.category"); 848 else if (Configuration.doAutoCreate()) 849 this.category = new CodeableConcept(); // cc 850 return this.category; 851 } 852 853 public boolean hasCategory() { 854 return this.category != null && !this.category.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #category} (Indicates where the medication is expected to 859 * be consumed or administered.) 860 */ 861 public MedicationStatement setCategory(CodeableConcept value) { 862 this.category = value; 863 return this; 864 } 865 866 /** 867 * @return {@link #medication} (Identifies the medication being administered. 868 * This is either a link to a resource representing the details of the 869 * medication or a simple attribute carrying a code that identifies the 870 * medication from a known list of medications.) 871 */ 872 public Type getMedication() { 873 return this.medication; 874 } 875 876 /** 877 * @return {@link #medication} (Identifies the medication being administered. 878 * This is either a link to a resource representing the details of the 879 * medication or a simple attribute carrying a code that identifies the 880 * medication from a known list of medications.) 881 */ 882 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 883 if (this.medication == null) 884 this.medication = new CodeableConcept(); 885 if (!(this.medication instanceof CodeableConcept)) 886 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 887 + this.medication.getClass().getName() + " was encountered"); 888 return (CodeableConcept) this.medication; 889 } 890 891 public boolean hasMedicationCodeableConcept() { 892 return this != null && this.medication instanceof CodeableConcept; 893 } 894 895 /** 896 * @return {@link #medication} (Identifies the medication being administered. 897 * This is either a link to a resource representing the details of the 898 * medication or a simple attribute carrying a code that identifies the 899 * medication from a known list of medications.) 900 */ 901 public Reference getMedicationReference() throws FHIRException { 902 if (this.medication == null) 903 this.medication = new Reference(); 904 if (!(this.medication instanceof Reference)) 905 throw new FHIRException("Type mismatch: the type Reference was expected, but " 906 + this.medication.getClass().getName() + " was encountered"); 907 return (Reference) this.medication; 908 } 909 910 public boolean hasMedicationReference() { 911 return this != null && this.medication instanceof Reference; 912 } 913 914 public boolean hasMedication() { 915 return this.medication != null && !this.medication.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #medication} (Identifies the medication being 920 * administered. This is either a link to a resource representing 921 * the details of the medication or a simple attribute carrying a 922 * code that identifies the medication from a known list of 923 * medications.) 924 */ 925 public MedicationStatement setMedication(Type value) { 926 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 927 throw new Error("Not the right type for MedicationStatement.medication[x]: " + value.fhirType()); 928 this.medication = value; 929 return this; 930 } 931 932 /** 933 * @return {@link #subject} (The person, animal or group who is/was taking the 934 * medication.) 935 */ 936 public Reference getSubject() { 937 if (this.subject == null) 938 if (Configuration.errorOnAutoCreate()) 939 throw new Error("Attempt to auto-create MedicationStatement.subject"); 940 else if (Configuration.doAutoCreate()) 941 this.subject = new Reference(); // cc 942 return this.subject; 943 } 944 945 public boolean hasSubject() { 946 return this.subject != null && !this.subject.isEmpty(); 947 } 948 949 /** 950 * @param value {@link #subject} (The person, animal or group who is/was taking 951 * the medication.) 952 */ 953 public MedicationStatement setSubject(Reference value) { 954 this.subject = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #subject} The actual object that is the target of the 960 * reference. The reference library doesn't populate this, but you can 961 * use it to hold the resource if you resolve it. (The person, animal or 962 * group who is/was taking the medication.) 963 */ 964 public Resource getSubjectTarget() { 965 return this.subjectTarget; 966 } 967 968 /** 969 * @param value {@link #subject} The actual object that is the target of the 970 * reference. The reference library doesn't use these, but you can 971 * use it to hold the resource if you resolve it. (The person, 972 * animal or group who is/was taking the medication.) 973 */ 974 public MedicationStatement setSubjectTarget(Resource value) { 975 this.subjectTarget = value; 976 return this; 977 } 978 979 /** 980 * @return {@link #context} (The encounter or episode of care that establishes 981 * the context for this MedicationStatement.) 982 */ 983 public Reference getContext() { 984 if (this.context == null) 985 if (Configuration.errorOnAutoCreate()) 986 throw new Error("Attempt to auto-create MedicationStatement.context"); 987 else if (Configuration.doAutoCreate()) 988 this.context = new Reference(); // cc 989 return this.context; 990 } 991 992 public boolean hasContext() { 993 return this.context != null && !this.context.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #context} (The encounter or episode of care that 998 * establishes the context for this MedicationStatement.) 999 */ 1000 public MedicationStatement setContext(Reference value) { 1001 this.context = value; 1002 return this; 1003 } 1004 1005 /** 1006 * @return {@link #context} The actual object that is the target of the 1007 * reference. The reference library doesn't populate this, but you can 1008 * use it to hold the resource if you resolve it. (The encounter or 1009 * episode of care that establishes the context for this 1010 * MedicationStatement.) 1011 */ 1012 public Resource getContextTarget() { 1013 return this.contextTarget; 1014 } 1015 1016 /** 1017 * @param value {@link #context} The actual object that is the target of the 1018 * reference. The reference library doesn't use these, but you can 1019 * use it to hold the resource if you resolve it. (The encounter or 1020 * episode of care that establishes the context for this 1021 * MedicationStatement.) 1022 */ 1023 public MedicationStatement setContextTarget(Resource value) { 1024 this.contextTarget = value; 1025 return this; 1026 } 1027 1028 /** 1029 * @return {@link #effective} (The interval of time during which it is being 1030 * asserted that the patient is/was/will be taking the medication (or 1031 * was not taking, when the MedicationStatement.taken element is No).) 1032 */ 1033 public Type getEffective() { 1034 return this.effective; 1035 } 1036 1037 /** 1038 * @return {@link #effective} (The interval of time during which it is being 1039 * asserted that the patient is/was/will be taking the medication (or 1040 * was not taking, when the MedicationStatement.taken element is No).) 1041 */ 1042 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1043 if (this.effective == null) 1044 this.effective = new DateTimeType(); 1045 if (!(this.effective instanceof DateTimeType)) 1046 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1047 + this.effective.getClass().getName() + " was encountered"); 1048 return (DateTimeType) this.effective; 1049 } 1050 1051 public boolean hasEffectiveDateTimeType() { 1052 return this != null && this.effective instanceof DateTimeType; 1053 } 1054 1055 /** 1056 * @return {@link #effective} (The interval of time during which it is being 1057 * asserted that the patient is/was/will be taking the medication (or 1058 * was not taking, when the MedicationStatement.taken element is No).) 1059 */ 1060 public Period getEffectivePeriod() throws FHIRException { 1061 if (this.effective == null) 1062 this.effective = new Period(); 1063 if (!(this.effective instanceof Period)) 1064 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1065 + " was encountered"); 1066 return (Period) this.effective; 1067 } 1068 1069 public boolean hasEffectivePeriod() { 1070 return this != null && this.effective instanceof Period; 1071 } 1072 1073 public boolean hasEffective() { 1074 return this.effective != null && !this.effective.isEmpty(); 1075 } 1076 1077 /** 1078 * @param value {@link #effective} (The interval of time during which it is 1079 * being asserted that the patient is/was/will be taking the 1080 * medication (or was not taking, when the 1081 * MedicationStatement.taken element is No).) 1082 */ 1083 public MedicationStatement setEffective(Type value) { 1084 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1085 throw new Error("Not the right type for MedicationStatement.effective[x]: " + value.fhirType()); 1086 this.effective = value; 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #dateAsserted} (The date when the medication statement was 1092 * asserted by the information source.). This is the underlying object 1093 * with id, value and extensions. The accessor "getDateAsserted" gives 1094 * direct access to the value 1095 */ 1096 public DateTimeType getDateAssertedElement() { 1097 if (this.dateAsserted == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create MedicationStatement.dateAsserted"); 1100 else if (Configuration.doAutoCreate()) 1101 this.dateAsserted = new DateTimeType(); // bb 1102 return this.dateAsserted; 1103 } 1104 1105 public boolean hasDateAssertedElement() { 1106 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 1107 } 1108 1109 public boolean hasDateAsserted() { 1110 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 1111 } 1112 1113 /** 1114 * @param value {@link #dateAsserted} (The date when the medication statement 1115 * was asserted by the information source.). This is the underlying 1116 * object with id, value and extensions. The accessor 1117 * "getDateAsserted" gives direct access to the value 1118 */ 1119 public MedicationStatement setDateAssertedElement(DateTimeType value) { 1120 this.dateAsserted = value; 1121 return this; 1122 } 1123 1124 /** 1125 * @return The date when the medication statement was asserted by the 1126 * information source. 1127 */ 1128 public Date getDateAsserted() { 1129 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 1130 } 1131 1132 /** 1133 * @param value The date when the medication statement was asserted by the 1134 * information source. 1135 */ 1136 public MedicationStatement setDateAsserted(Date value) { 1137 if (value == null) 1138 this.dateAsserted = null; 1139 else { 1140 if (this.dateAsserted == null) 1141 this.dateAsserted = new DateTimeType(); 1142 this.dateAsserted.setValue(value); 1143 } 1144 return this; 1145 } 1146 1147 /** 1148 * @return {@link #informationSource} (The person or organization that provided 1149 * the information about the taking of this medication. Note: Use 1150 * derivedFrom when a MedicationStatement is derived from other 1151 * resources, e.g. Claim or MedicationRequest.) 1152 */ 1153 public Reference getInformationSource() { 1154 if (this.informationSource == null) 1155 if (Configuration.errorOnAutoCreate()) 1156 throw new Error("Attempt to auto-create MedicationStatement.informationSource"); 1157 else if (Configuration.doAutoCreate()) 1158 this.informationSource = new Reference(); // cc 1159 return this.informationSource; 1160 } 1161 1162 public boolean hasInformationSource() { 1163 return this.informationSource != null && !this.informationSource.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #informationSource} (The person or organization that 1168 * provided the information about the taking of this medication. 1169 * Note: Use derivedFrom when a MedicationStatement is derived from 1170 * other resources, e.g. Claim or MedicationRequest.) 1171 */ 1172 public MedicationStatement setInformationSource(Reference value) { 1173 this.informationSource = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #informationSource} The actual object that is the target of 1179 * the reference. The reference library doesn't populate this, but you 1180 * can use it to hold the resource if you resolve it. (The person or 1181 * organization that provided the information about the taking of this 1182 * medication. Note: Use derivedFrom when a MedicationStatement is 1183 * derived from other resources, e.g. Claim or MedicationRequest.) 1184 */ 1185 public Resource getInformationSourceTarget() { 1186 return this.informationSourceTarget; 1187 } 1188 1189 /** 1190 * @param value {@link #informationSource} The actual object that is the target 1191 * of the reference. The reference library doesn't use these, but 1192 * you can use it to hold the resource if you resolve it. (The 1193 * person or organization that provided the information about the 1194 * taking of this medication. Note: Use derivedFrom when a 1195 * MedicationStatement is derived from other resources, e.g. Claim 1196 * or MedicationRequest.) 1197 */ 1198 public MedicationStatement setInformationSourceTarget(Resource value) { 1199 this.informationSourceTarget = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return {@link #derivedFrom} (Allows linking the MedicationStatement to the 1205 * underlying MedicationRequest, or to other information that supports 1206 * or is used to derive the MedicationStatement.) 1207 */ 1208 public List<Reference> getDerivedFrom() { 1209 if (this.derivedFrom == null) 1210 this.derivedFrom = new ArrayList<Reference>(); 1211 return this.derivedFrom; 1212 } 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public MedicationStatement setDerivedFrom(List<Reference> theDerivedFrom) { 1218 this.derivedFrom = theDerivedFrom; 1219 return this; 1220 } 1221 1222 public boolean hasDerivedFrom() { 1223 if (this.derivedFrom == null) 1224 return false; 1225 for (Reference item : this.derivedFrom) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 public Reference addDerivedFrom() { // 3 1232 Reference t = new Reference(); 1233 if (this.derivedFrom == null) 1234 this.derivedFrom = new ArrayList<Reference>(); 1235 this.derivedFrom.add(t); 1236 return t; 1237 } 1238 1239 public MedicationStatement addDerivedFrom(Reference t) { // 3 1240 if (t == null) 1241 return this; 1242 if (this.derivedFrom == null) 1243 this.derivedFrom = new ArrayList<Reference>(); 1244 this.derivedFrom.add(t); 1245 return this; 1246 } 1247 1248 /** 1249 * @return The first repetition of repeating field {@link #derivedFrom}, 1250 * creating it if it does not already exist 1251 */ 1252 public Reference getDerivedFromFirstRep() { 1253 if (getDerivedFrom().isEmpty()) { 1254 addDerivedFrom(); 1255 } 1256 return getDerivedFrom().get(0); 1257 } 1258 1259 /** 1260 * @deprecated Use Reference#setResource(IBaseResource) instead 1261 */ 1262 @Deprecated 1263 public List<Resource> getDerivedFromTarget() { 1264 if (this.derivedFromTarget == null) 1265 this.derivedFromTarget = new ArrayList<Resource>(); 1266 return this.derivedFromTarget; 1267 } 1268 1269 /** 1270 * @return {@link #reasonCode} (A reason for why the medication is being/was 1271 * taken.) 1272 */ 1273 public List<CodeableConcept> getReasonCode() { 1274 if (this.reasonCode == null) 1275 this.reasonCode = new ArrayList<CodeableConcept>(); 1276 return this.reasonCode; 1277 } 1278 1279 /** 1280 * @return Returns a reference to <code>this</code> for easy method chaining 1281 */ 1282 public MedicationStatement setReasonCode(List<CodeableConcept> theReasonCode) { 1283 this.reasonCode = theReasonCode; 1284 return this; 1285 } 1286 1287 public boolean hasReasonCode() { 1288 if (this.reasonCode == null) 1289 return false; 1290 for (CodeableConcept item : this.reasonCode) 1291 if (!item.isEmpty()) 1292 return true; 1293 return false; 1294 } 1295 1296 public CodeableConcept addReasonCode() { // 3 1297 CodeableConcept t = new CodeableConcept(); 1298 if (this.reasonCode == null) 1299 this.reasonCode = new ArrayList<CodeableConcept>(); 1300 this.reasonCode.add(t); 1301 return t; 1302 } 1303 1304 public MedicationStatement addReasonCode(CodeableConcept t) { // 3 1305 if (t == null) 1306 return this; 1307 if (this.reasonCode == null) 1308 this.reasonCode = new ArrayList<CodeableConcept>(); 1309 this.reasonCode.add(t); 1310 return this; 1311 } 1312 1313 /** 1314 * @return The first repetition of repeating field {@link #reasonCode}, creating 1315 * it if it does not already exist 1316 */ 1317 public CodeableConcept getReasonCodeFirstRep() { 1318 if (getReasonCode().isEmpty()) { 1319 addReasonCode(); 1320 } 1321 return getReasonCode().get(0); 1322 } 1323 1324 /** 1325 * @return {@link #reasonReference} (Condition or observation that supports why 1326 * the medication is being/was taken.) 1327 */ 1328 public List<Reference> getReasonReference() { 1329 if (this.reasonReference == null) 1330 this.reasonReference = new ArrayList<Reference>(); 1331 return this.reasonReference; 1332 } 1333 1334 /** 1335 * @return Returns a reference to <code>this</code> for easy method chaining 1336 */ 1337 public MedicationStatement setReasonReference(List<Reference> theReasonReference) { 1338 this.reasonReference = theReasonReference; 1339 return this; 1340 } 1341 1342 public boolean hasReasonReference() { 1343 if (this.reasonReference == null) 1344 return false; 1345 for (Reference item : this.reasonReference) 1346 if (!item.isEmpty()) 1347 return true; 1348 return false; 1349 } 1350 1351 public Reference addReasonReference() { // 3 1352 Reference t = new Reference(); 1353 if (this.reasonReference == null) 1354 this.reasonReference = new ArrayList<Reference>(); 1355 this.reasonReference.add(t); 1356 return t; 1357 } 1358 1359 public MedicationStatement addReasonReference(Reference t) { // 3 1360 if (t == null) 1361 return this; 1362 if (this.reasonReference == null) 1363 this.reasonReference = new ArrayList<Reference>(); 1364 this.reasonReference.add(t); 1365 return this; 1366 } 1367 1368 /** 1369 * @return The first repetition of repeating field {@link #reasonReference}, 1370 * creating it if it does not already exist 1371 */ 1372 public Reference getReasonReferenceFirstRep() { 1373 if (getReasonReference().isEmpty()) { 1374 addReasonReference(); 1375 } 1376 return getReasonReference().get(0); 1377 } 1378 1379 /** 1380 * @deprecated Use Reference#setResource(IBaseResource) instead 1381 */ 1382 @Deprecated 1383 public List<Resource> getReasonReferenceTarget() { 1384 if (this.reasonReferenceTarget == null) 1385 this.reasonReferenceTarget = new ArrayList<Resource>(); 1386 return this.reasonReferenceTarget; 1387 } 1388 1389 /** 1390 * @return {@link #note} (Provides extra information about the medication 1391 * statement that is not conveyed by the other attributes.) 1392 */ 1393 public List<Annotation> getNote() { 1394 if (this.note == null) 1395 this.note = new ArrayList<Annotation>(); 1396 return this.note; 1397 } 1398 1399 /** 1400 * @return Returns a reference to <code>this</code> for easy method chaining 1401 */ 1402 public MedicationStatement setNote(List<Annotation> theNote) { 1403 this.note = theNote; 1404 return this; 1405 } 1406 1407 public boolean hasNote() { 1408 if (this.note == null) 1409 return false; 1410 for (Annotation item : this.note) 1411 if (!item.isEmpty()) 1412 return true; 1413 return false; 1414 } 1415 1416 public Annotation addNote() { // 3 1417 Annotation t = new Annotation(); 1418 if (this.note == null) 1419 this.note = new ArrayList<Annotation>(); 1420 this.note.add(t); 1421 return t; 1422 } 1423 1424 public MedicationStatement addNote(Annotation t) { // 3 1425 if (t == null) 1426 return this; 1427 if (this.note == null) 1428 this.note = new ArrayList<Annotation>(); 1429 this.note.add(t); 1430 return this; 1431 } 1432 1433 /** 1434 * @return The first repetition of repeating field {@link #note}, creating it if 1435 * it does not already exist 1436 */ 1437 public Annotation getNoteFirstRep() { 1438 if (getNote().isEmpty()) { 1439 addNote(); 1440 } 1441 return getNote().get(0); 1442 } 1443 1444 /** 1445 * @return {@link #dosage} (Indicates how the medication is/was or should be 1446 * taken by the patient.) 1447 */ 1448 public List<Dosage> getDosage() { 1449 if (this.dosage == null) 1450 this.dosage = new ArrayList<Dosage>(); 1451 return this.dosage; 1452 } 1453 1454 /** 1455 * @return Returns a reference to <code>this</code> for easy method chaining 1456 */ 1457 public MedicationStatement setDosage(List<Dosage> theDosage) { 1458 this.dosage = theDosage; 1459 return this; 1460 } 1461 1462 public boolean hasDosage() { 1463 if (this.dosage == null) 1464 return false; 1465 for (Dosage item : this.dosage) 1466 if (!item.isEmpty()) 1467 return true; 1468 return false; 1469 } 1470 1471 public Dosage addDosage() { // 3 1472 Dosage t = new Dosage(); 1473 if (this.dosage == null) 1474 this.dosage = new ArrayList<Dosage>(); 1475 this.dosage.add(t); 1476 return t; 1477 } 1478 1479 public MedicationStatement addDosage(Dosage t) { // 3 1480 if (t == null) 1481 return this; 1482 if (this.dosage == null) 1483 this.dosage = new ArrayList<Dosage>(); 1484 this.dosage.add(t); 1485 return this; 1486 } 1487 1488 /** 1489 * @return The first repetition of repeating field {@link #dosage}, creating it 1490 * if it does not already exist 1491 */ 1492 public Dosage getDosageFirstRep() { 1493 if (getDosage().isEmpty()) { 1494 addDosage(); 1495 } 1496 return getDosage().get(0); 1497 } 1498 1499 protected void listChildren(List<Property> children) { 1500 super.listChildren(children); 1501 children.add(new Property("identifier", "Identifier", 1502 "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 1503 0, java.lang.Integer.MAX_VALUE, identifier)); 1504 children.add(new Property("basedOn", "Reference(MedicationRequest|CarePlan|ServiceRequest)", 1505 "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, 1506 java.lang.Integer.MAX_VALUE, basedOn)); 1507 children.add(new Property("partOf", 1508 "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Observation)", 1509 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1510 partOf)); 1511 children.add(new Property("status", "code", 1512 "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally, this will be active or completed.", 1513 0, 1, status)); 1514 children.add(new Property("statusReason", "CodeableConcept", 1515 "Captures the reason for the current state of the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, 1516 statusReason)); 1517 children.add(new Property("category", "CodeableConcept", 1518 "Indicates where the medication is expected to be consumed or administered.", 0, 1, category)); 1519 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1520 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1521 0, 1, medication)); 1522 children.add(new Property("subject", "Reference(Patient|Group)", 1523 "The person, animal or group who is/was taking the medication.", 0, 1, subject)); 1524 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 1525 "The encounter or episode of care that establishes the context for this MedicationStatement.", 0, 1, context)); 1526 children.add(new Property("effective[x]", "dateTime|Period", 1527 "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.taken element is No).", 1528 0, 1, effective)); 1529 children.add(new Property("dateAsserted", "dateTime", 1530 "The date when the medication statement was asserted by the information source.", 0, 1, dateAsserted)); 1531 children.add(new Property("informationSource", 1532 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 1533 "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.", 1534 0, 1, informationSource)); 1535 children.add(new Property("derivedFrom", "Reference(Any)", 1536 "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 1537 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1538 children.add(new Property("reasonCode", "CodeableConcept", "A reason for why the medication is being/was taken.", 0, 1539 java.lang.Integer.MAX_VALUE, reasonCode)); 1540 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 1541 "Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, 1542 reasonReference)); 1543 children.add(new Property("note", "Annotation", 1544 "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, 1545 java.lang.Integer.MAX_VALUE, note)); 1546 children 1547 .add(new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 1548 0, java.lang.Integer.MAX_VALUE, dosage)); 1549 } 1550 1551 @Override 1552 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1553 switch (_hash) { 1554 case -1618432855: 1555 /* identifier */ return new Property("identifier", "Identifier", 1556 "Identifiers associated with this Medication Statement that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 1557 0, java.lang.Integer.MAX_VALUE, identifier); 1558 case -332612366: 1559 /* basedOn */ return new Property("basedOn", "Reference(MedicationRequest|CarePlan|ServiceRequest)", 1560 "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, 1561 java.lang.Integer.MAX_VALUE, basedOn); 1562 case -995410646: 1563 /* partOf */ return new Property("partOf", 1564 "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Observation)", 1565 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1566 partOf); 1567 case -892481550: 1568 /* status */ return new Property("status", "code", 1569 "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally, this will be active or completed.", 1570 0, 1, status); 1571 case 2051346646: 1572 /* statusReason */ return new Property("statusReason", "CodeableConcept", 1573 "Captures the reason for the current state of the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, 1574 statusReason); 1575 case 50511102: 1576 /* category */ return new Property("category", "CodeableConcept", 1577 "Indicates where the medication is expected to be consumed or administered.", 0, 1, category); 1578 case 1458402129: 1579 /* medication[x] */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1580 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1581 0, 1, medication); 1582 case 1998965455: 1583 /* medication */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1584 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1585 0, 1, medication); 1586 case -209845038: 1587 /* medicationCodeableConcept */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1588 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1589 0, 1, medication); 1590 case 2104315196: 1591 /* medicationReference */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 1592 "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 1593 0, 1, medication); 1594 case -1867885268: 1595 /* subject */ return new Property("subject", "Reference(Patient|Group)", 1596 "The person, animal or group who is/was taking the medication.", 0, 1, subject); 1597 case 951530927: 1598 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 1599 "The encounter or episode of care that establishes the context for this MedicationStatement.", 0, 1, context); 1600 case 247104889: 1601 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 1602 "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.taken element is No).", 1603 0, 1, effective); 1604 case -1468651097: 1605 /* effective */ return new Property("effective[x]", "dateTime|Period", 1606 "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.taken element is No).", 1607 0, 1, effective); 1608 case -275306910: 1609 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 1610 "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.taken element is No).", 1611 0, 1, effective); 1612 case -403934648: 1613 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 1614 "The interval of time during which it is being asserted that the patient is/was/will be taking the medication (or was not taking, when the MedicationStatement.taken element is No).", 1615 0, 1, effective); 1616 case -1980855245: 1617 /* dateAsserted */ return new Property("dateAsserted", "dateTime", 1618 "The date when the medication statement was asserted by the information source.", 0, 1, dateAsserted); 1619 case -2123220889: 1620 /* informationSource */ return new Property("informationSource", 1621 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 1622 "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g. Claim or MedicationRequest.", 1623 0, 1, informationSource); 1624 case 1077922663: 1625 /* derivedFrom */ return new Property("derivedFrom", "Reference(Any)", 1626 "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 1627 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1628 case 722137681: 1629 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1630 "A reason for why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1631 case -1146218137: 1632 /* reasonReference */ return new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 1633 "Condition or observation that supports why the medication is being/was taken.", 0, 1634 java.lang.Integer.MAX_VALUE, reasonReference); 1635 case 3387378: 1636 /* note */ return new Property("note", "Annotation", 1637 "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, 1638 java.lang.Integer.MAX_VALUE, note); 1639 case -1326018889: 1640 /* dosage */ return new Property("dosage", "Dosage", 1641 "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, 1642 dosage); 1643 default: 1644 return super.getNamedProperty(_hash, _name, _checkValid); 1645 } 1646 1647 } 1648 1649 @Override 1650 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1651 switch (hash) { 1652 case -1618432855: 1653 /* identifier */ return this.identifier == null ? new Base[0] 1654 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1655 case -332612366: 1656 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1657 case -995410646: 1658 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1659 case -892481550: 1660 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationStatementStatus> 1661 case 2051346646: 1662 /* statusReason */ return this.statusReason == null ? new Base[0] 1663 : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 1664 case 50511102: 1665 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1666 case 1998965455: 1667 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 1668 case -1867885268: 1669 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1670 case 951530927: 1671 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 1672 case -1468651097: 1673 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 1674 case -1980855245: 1675 /* dateAsserted */ return this.dateAsserted == null ? new Base[0] : new Base[] { this.dateAsserted }; // DateTimeType 1676 case -2123220889: 1677 /* informationSource */ return this.informationSource == null ? new Base[0] 1678 : new Base[] { this.informationSource }; // Reference 1679 case 1077922663: 1680 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] 1681 : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1682 case 722137681: 1683 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1684 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1685 case -1146218137: 1686 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1687 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1688 case 3387378: 1689 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1690 case -1326018889: 1691 /* dosage */ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 1692 default: 1693 return super.getProperty(hash, name, checkValid); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1700 switch (hash) { 1701 case -1618432855: // identifier 1702 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1703 return value; 1704 case -332612366: // basedOn 1705 this.getBasedOn().add(castToReference(value)); // Reference 1706 return value; 1707 case -995410646: // partOf 1708 this.getPartOf().add(castToReference(value)); // Reference 1709 return value; 1710 case -892481550: // status 1711 value = new MedicationStatementStatusEnumFactory().fromType(castToCode(value)); 1712 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatus> 1713 return value; 1714 case 2051346646: // statusReason 1715 this.getStatusReason().add(castToCodeableConcept(value)); // CodeableConcept 1716 return value; 1717 case 50511102: // category 1718 this.category = castToCodeableConcept(value); // CodeableConcept 1719 return value; 1720 case 1998965455: // medication 1721 this.medication = castToType(value); // Type 1722 return value; 1723 case -1867885268: // subject 1724 this.subject = castToReference(value); // Reference 1725 return value; 1726 case 951530927: // context 1727 this.context = castToReference(value); // Reference 1728 return value; 1729 case -1468651097: // effective 1730 this.effective = castToType(value); // Type 1731 return value; 1732 case -1980855245: // dateAsserted 1733 this.dateAsserted = castToDateTime(value); // DateTimeType 1734 return value; 1735 case -2123220889: // informationSource 1736 this.informationSource = castToReference(value); // Reference 1737 return value; 1738 case 1077922663: // derivedFrom 1739 this.getDerivedFrom().add(castToReference(value)); // Reference 1740 return value; 1741 case 722137681: // reasonCode 1742 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1743 return value; 1744 case -1146218137: // reasonReference 1745 this.getReasonReference().add(castToReference(value)); // Reference 1746 return value; 1747 case 3387378: // note 1748 this.getNote().add(castToAnnotation(value)); // Annotation 1749 return value; 1750 case -1326018889: // dosage 1751 this.getDosage().add(castToDosage(value)); // Dosage 1752 return value; 1753 default: 1754 return super.setProperty(hash, name, value); 1755 } 1756 1757 } 1758 1759 @Override 1760 public Base setProperty(String name, Base value) throws FHIRException { 1761 if (name.equals("identifier")) { 1762 this.getIdentifier().add(castToIdentifier(value)); 1763 } else if (name.equals("basedOn")) { 1764 this.getBasedOn().add(castToReference(value)); 1765 } else if (name.equals("partOf")) { 1766 this.getPartOf().add(castToReference(value)); 1767 } else if (name.equals("status")) { 1768 value = new MedicationStatementStatusEnumFactory().fromType(castToCode(value)); 1769 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatus> 1770 } else if (name.equals("statusReason")) { 1771 this.getStatusReason().add(castToCodeableConcept(value)); 1772 } else if (name.equals("category")) { 1773 this.category = castToCodeableConcept(value); // CodeableConcept 1774 } else if (name.equals("medication[x]")) { 1775 this.medication = castToType(value); // Type 1776 } else if (name.equals("subject")) { 1777 this.subject = castToReference(value); // Reference 1778 } else if (name.equals("context")) { 1779 this.context = castToReference(value); // Reference 1780 } else if (name.equals("effective[x]")) { 1781 this.effective = castToType(value); // Type 1782 } else if (name.equals("dateAsserted")) { 1783 this.dateAsserted = castToDateTime(value); // DateTimeType 1784 } else if (name.equals("informationSource")) { 1785 this.informationSource = castToReference(value); // Reference 1786 } else if (name.equals("derivedFrom")) { 1787 this.getDerivedFrom().add(castToReference(value)); 1788 } else if (name.equals("reasonCode")) { 1789 this.getReasonCode().add(castToCodeableConcept(value)); 1790 } else if (name.equals("reasonReference")) { 1791 this.getReasonReference().add(castToReference(value)); 1792 } else if (name.equals("note")) { 1793 this.getNote().add(castToAnnotation(value)); 1794 } else if (name.equals("dosage")) { 1795 this.getDosage().add(castToDosage(value)); 1796 } else 1797 return super.setProperty(name, value); 1798 return value; 1799 } 1800 1801 @Override 1802 public void removeChild(String name, Base value) throws FHIRException { 1803 if (name.equals("identifier")) { 1804 this.getIdentifier().remove(castToIdentifier(value)); 1805 } else if (name.equals("basedOn")) { 1806 this.getBasedOn().remove(castToReference(value)); 1807 } else if (name.equals("partOf")) { 1808 this.getPartOf().remove(castToReference(value)); 1809 } else if (name.equals("status")) { 1810 this.status = null; 1811 } else if (name.equals("statusReason")) { 1812 this.getStatusReason().remove(castToCodeableConcept(value)); 1813 } else if (name.equals("category")) { 1814 this.category = null; 1815 } else if (name.equals("medication[x]")) { 1816 this.medication = null; 1817 } else if (name.equals("subject")) { 1818 this.subject = null; 1819 } else if (name.equals("context")) { 1820 this.context = null; 1821 } else if (name.equals("effective[x]")) { 1822 this.effective = null; 1823 } else if (name.equals("dateAsserted")) { 1824 this.dateAsserted = null; 1825 } else if (name.equals("informationSource")) { 1826 this.informationSource = null; 1827 } else if (name.equals("derivedFrom")) { 1828 this.getDerivedFrom().remove(castToReference(value)); 1829 } else if (name.equals("reasonCode")) { 1830 this.getReasonCode().remove(castToCodeableConcept(value)); 1831 } else if (name.equals("reasonReference")) { 1832 this.getReasonReference().remove(castToReference(value)); 1833 } else if (name.equals("note")) { 1834 this.getNote().remove(castToAnnotation(value)); 1835 } else if (name.equals("dosage")) { 1836 this.getDosage().remove(castToDosage(value)); 1837 } else 1838 super.removeChild(name, value); 1839 1840 } 1841 1842 @Override 1843 public Base makeProperty(int hash, String name) throws FHIRException { 1844 switch (hash) { 1845 case -1618432855: 1846 return addIdentifier(); 1847 case -332612366: 1848 return addBasedOn(); 1849 case -995410646: 1850 return addPartOf(); 1851 case -892481550: 1852 return getStatusElement(); 1853 case 2051346646: 1854 return addStatusReason(); 1855 case 50511102: 1856 return getCategory(); 1857 case 1458402129: 1858 return getMedication(); 1859 case 1998965455: 1860 return getMedication(); 1861 case -1867885268: 1862 return getSubject(); 1863 case 951530927: 1864 return getContext(); 1865 case 247104889: 1866 return getEffective(); 1867 case -1468651097: 1868 return getEffective(); 1869 case -1980855245: 1870 return getDateAssertedElement(); 1871 case -2123220889: 1872 return getInformationSource(); 1873 case 1077922663: 1874 return addDerivedFrom(); 1875 case 722137681: 1876 return addReasonCode(); 1877 case -1146218137: 1878 return addReasonReference(); 1879 case 3387378: 1880 return addNote(); 1881 case -1326018889: 1882 return addDosage(); 1883 default: 1884 return super.makeProperty(hash, name); 1885 } 1886 1887 } 1888 1889 @Override 1890 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1891 switch (hash) { 1892 case -1618432855: 1893 /* identifier */ return new String[] { "Identifier" }; 1894 case -332612366: 1895 /* basedOn */ return new String[] { "Reference" }; 1896 case -995410646: 1897 /* partOf */ return new String[] { "Reference" }; 1898 case -892481550: 1899 /* status */ return new String[] { "code" }; 1900 case 2051346646: 1901 /* statusReason */ return new String[] { "CodeableConcept" }; 1902 case 50511102: 1903 /* category */ return new String[] { "CodeableConcept" }; 1904 case 1998965455: 1905 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 1906 case -1867885268: 1907 /* subject */ return new String[] { "Reference" }; 1908 case 951530927: 1909 /* context */ return new String[] { "Reference" }; 1910 case -1468651097: 1911 /* effective */ return new String[] { "dateTime", "Period" }; 1912 case -1980855245: 1913 /* dateAsserted */ return new String[] { "dateTime" }; 1914 case -2123220889: 1915 /* informationSource */ return new String[] { "Reference" }; 1916 case 1077922663: 1917 /* derivedFrom */ return new String[] { "Reference" }; 1918 case 722137681: 1919 /* reasonCode */ return new String[] { "CodeableConcept" }; 1920 case -1146218137: 1921 /* reasonReference */ return new String[] { "Reference" }; 1922 case 3387378: 1923 /* note */ return new String[] { "Annotation" }; 1924 case -1326018889: 1925 /* dosage */ return new String[] { "Dosage" }; 1926 default: 1927 return super.getTypesForProperty(hash, name); 1928 } 1929 1930 } 1931 1932 @Override 1933 public Base addChild(String name) throws FHIRException { 1934 if (name.equals("identifier")) { 1935 return addIdentifier(); 1936 } else if (name.equals("basedOn")) { 1937 return addBasedOn(); 1938 } else if (name.equals("partOf")) { 1939 return addPartOf(); 1940 } else if (name.equals("status")) { 1941 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.status"); 1942 } else if (name.equals("statusReason")) { 1943 return addStatusReason(); 1944 } else if (name.equals("category")) { 1945 this.category = new CodeableConcept(); 1946 return this.category; 1947 } else if (name.equals("medicationCodeableConcept")) { 1948 this.medication = new CodeableConcept(); 1949 return this.medication; 1950 } else if (name.equals("medicationReference")) { 1951 this.medication = new Reference(); 1952 return this.medication; 1953 } else if (name.equals("subject")) { 1954 this.subject = new Reference(); 1955 return this.subject; 1956 } else if (name.equals("context")) { 1957 this.context = new Reference(); 1958 return this.context; 1959 } else if (name.equals("effectiveDateTime")) { 1960 this.effective = new DateTimeType(); 1961 return this.effective; 1962 } else if (name.equals("effectivePeriod")) { 1963 this.effective = new Period(); 1964 return this.effective; 1965 } else if (name.equals("dateAsserted")) { 1966 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.dateAsserted"); 1967 } else if (name.equals("informationSource")) { 1968 this.informationSource = new Reference(); 1969 return this.informationSource; 1970 } else if (name.equals("derivedFrom")) { 1971 return addDerivedFrom(); 1972 } else if (name.equals("reasonCode")) { 1973 return addReasonCode(); 1974 } else if (name.equals("reasonReference")) { 1975 return addReasonReference(); 1976 } else if (name.equals("note")) { 1977 return addNote(); 1978 } else if (name.equals("dosage")) { 1979 return addDosage(); 1980 } else 1981 return super.addChild(name); 1982 } 1983 1984 public String fhirType() { 1985 return "MedicationStatement"; 1986 1987 } 1988 1989 public MedicationStatement copy() { 1990 MedicationStatement dst = new MedicationStatement(); 1991 copyValues(dst); 1992 return dst; 1993 } 1994 1995 public void copyValues(MedicationStatement dst) { 1996 super.copyValues(dst); 1997 if (identifier != null) { 1998 dst.identifier = new ArrayList<Identifier>(); 1999 for (Identifier i : identifier) 2000 dst.identifier.add(i.copy()); 2001 } 2002 ; 2003 if (basedOn != null) { 2004 dst.basedOn = new ArrayList<Reference>(); 2005 for (Reference i : basedOn) 2006 dst.basedOn.add(i.copy()); 2007 } 2008 ; 2009 if (partOf != null) { 2010 dst.partOf = new ArrayList<Reference>(); 2011 for (Reference i : partOf) 2012 dst.partOf.add(i.copy()); 2013 } 2014 ; 2015 dst.status = status == null ? null : status.copy(); 2016 if (statusReason != null) { 2017 dst.statusReason = new ArrayList<CodeableConcept>(); 2018 for (CodeableConcept i : statusReason) 2019 dst.statusReason.add(i.copy()); 2020 } 2021 ; 2022 dst.category = category == null ? null : category.copy(); 2023 dst.medication = medication == null ? null : medication.copy(); 2024 dst.subject = subject == null ? null : subject.copy(); 2025 dst.context = context == null ? null : context.copy(); 2026 dst.effective = effective == null ? null : effective.copy(); 2027 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 2028 dst.informationSource = informationSource == null ? null : informationSource.copy(); 2029 if (derivedFrom != null) { 2030 dst.derivedFrom = new ArrayList<Reference>(); 2031 for (Reference i : derivedFrom) 2032 dst.derivedFrom.add(i.copy()); 2033 } 2034 ; 2035 if (reasonCode != null) { 2036 dst.reasonCode = new ArrayList<CodeableConcept>(); 2037 for (CodeableConcept i : reasonCode) 2038 dst.reasonCode.add(i.copy()); 2039 } 2040 ; 2041 if (reasonReference != null) { 2042 dst.reasonReference = new ArrayList<Reference>(); 2043 for (Reference i : reasonReference) 2044 dst.reasonReference.add(i.copy()); 2045 } 2046 ; 2047 if (note != null) { 2048 dst.note = new ArrayList<Annotation>(); 2049 for (Annotation i : note) 2050 dst.note.add(i.copy()); 2051 } 2052 ; 2053 if (dosage != null) { 2054 dst.dosage = new ArrayList<Dosage>(); 2055 for (Dosage i : dosage) 2056 dst.dosage.add(i.copy()); 2057 } 2058 ; 2059 } 2060 2061 protected MedicationStatement typedCopy() { 2062 return copy(); 2063 } 2064 2065 @Override 2066 public boolean equalsDeep(Base other_) { 2067 if (!super.equalsDeep(other_)) 2068 return false; 2069 if (!(other_ instanceof MedicationStatement)) 2070 return false; 2071 MedicationStatement o = (MedicationStatement) other_; 2072 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2073 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 2074 && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) 2075 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 2076 && compareDeep(context, o.context, true) && compareDeep(effective, o.effective, true) 2077 && compareDeep(dateAsserted, o.dateAsserted, true) && compareDeep(informationSource, o.informationSource, true) 2078 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(reasonCode, o.reasonCode, true) 2079 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(note, o.note, true) 2080 && compareDeep(dosage, o.dosage, true); 2081 } 2082 2083 @Override 2084 public boolean equalsShallow(Base other_) { 2085 if (!super.equalsShallow(other_)) 2086 return false; 2087 if (!(other_ instanceof MedicationStatement)) 2088 return false; 2089 MedicationStatement o = (MedicationStatement) other_; 2090 return compareValues(status, o.status, true) && compareValues(dateAsserted, o.dateAsserted, true); 2091 } 2092 2093 public boolean isEmpty() { 2094 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, statusReason, 2095 category, medication, subject, context, effective, dateAsserted, informationSource, derivedFrom, reasonCode, 2096 reasonReference, note, dosage); 2097 } 2098 2099 @Override 2100 public ResourceType getResourceType() { 2101 return ResourceType.MedicationStatement; 2102 } 2103 2104 /** 2105 * Search parameter: <b>identifier</b> 2106 * <p> 2107 * Description: <b>Return statements with this external identifier</b><br> 2108 * Type: <b>token</b><br> 2109 * Path: <b>MedicationStatement.identifier</b><br> 2110 * </p> 2111 */ 2112 @SearchParamDefinition(name = "identifier", path = "MedicationStatement.identifier", description = "Return statements with this external identifier", type = "token") 2113 public static final String SP_IDENTIFIER = "identifier"; 2114 /** 2115 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2116 * <p> 2117 * Description: <b>Return statements with this external identifier</b><br> 2118 * Type: <b>token</b><br> 2119 * Path: <b>MedicationStatement.identifier</b><br> 2120 * </p> 2121 */ 2122 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2123 SP_IDENTIFIER); 2124 2125 /** 2126 * Search parameter: <b>effective</b> 2127 * <p> 2128 * Description: <b>Date when patient was taking (or not taking) the 2129 * medication</b><br> 2130 * Type: <b>date</b><br> 2131 * Path: <b>MedicationStatement.effective[x]</b><br> 2132 * </p> 2133 */ 2134 @SearchParamDefinition(name = "effective", path = "MedicationStatement.effective", description = "Date when patient was taking (or not taking) the medication", type = "date") 2135 public static final String SP_EFFECTIVE = "effective"; 2136 /** 2137 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 2138 * <p> 2139 * Description: <b>Date when patient was taking (or not taking) the 2140 * medication</b><br> 2141 * Type: <b>date</b><br> 2142 * Path: <b>MedicationStatement.effective[x]</b><br> 2143 * </p> 2144 */ 2145 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2146 SP_EFFECTIVE); 2147 2148 /** 2149 * Search parameter: <b>code</b> 2150 * <p> 2151 * Description: <b>Return statements of this medication code</b><br> 2152 * Type: <b>token</b><br> 2153 * Path: <b>MedicationStatement.medicationCodeableConcept</b><br> 2154 * </p> 2155 */ 2156 @SearchParamDefinition(name = "code", path = "(MedicationStatement.medication as CodeableConcept)", description = "Return statements of this medication code", type = "token") 2157 public static final String SP_CODE = "code"; 2158 /** 2159 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2160 * <p> 2161 * Description: <b>Return statements of this medication code</b><br> 2162 * Type: <b>token</b><br> 2163 * Path: <b>MedicationStatement.medicationCodeableConcept</b><br> 2164 * </p> 2165 */ 2166 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2167 SP_CODE); 2168 2169 /** 2170 * Search parameter: <b>subject</b> 2171 * <p> 2172 * Description: <b>The identity of a patient, animal or group to list statements 2173 * for</b><br> 2174 * Type: <b>reference</b><br> 2175 * Path: <b>MedicationStatement.subject</b><br> 2176 * </p> 2177 */ 2178 @SearchParamDefinition(name = "subject", path = "MedicationStatement.subject", description = "The identity of a patient, animal or group to list statements for", type = "reference", providesMembershipIn = { 2179 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2180 public static final String SP_SUBJECT = "subject"; 2181 /** 2182 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2183 * <p> 2184 * Description: <b>The identity of a patient, animal or group to list statements 2185 * for</b><br> 2186 * Type: <b>reference</b><br> 2187 * Path: <b>MedicationStatement.subject</b><br> 2188 * </p> 2189 */ 2190 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2191 SP_SUBJECT); 2192 2193 /** 2194 * Constant for fluent queries to be used to add include statements. Specifies 2195 * the path value of "<b>MedicationStatement:subject</b>". 2196 */ 2197 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2198 "MedicationStatement:subject").toLocked(); 2199 2200 /** 2201 * Search parameter: <b>patient</b> 2202 * <p> 2203 * Description: <b>Returns statements for a specific patient.</b><br> 2204 * Type: <b>reference</b><br> 2205 * Path: <b>MedicationStatement.subject</b><br> 2206 * </p> 2207 */ 2208 @SearchParamDefinition(name = "patient", path = "MedicationStatement.subject.where(resolve() is Patient)", description = "Returns statements for a specific patient.", type = "reference", target = { 2209 Patient.class }) 2210 public static final String SP_PATIENT = "patient"; 2211 /** 2212 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2213 * <p> 2214 * Description: <b>Returns statements for a specific patient.</b><br> 2215 * Type: <b>reference</b><br> 2216 * Path: <b>MedicationStatement.subject</b><br> 2217 * </p> 2218 */ 2219 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2220 SP_PATIENT); 2221 2222 /** 2223 * Constant for fluent queries to be used to add include statements. Specifies 2224 * the path value of "<b>MedicationStatement:patient</b>". 2225 */ 2226 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2227 "MedicationStatement:patient").toLocked(); 2228 2229 /** 2230 * Search parameter: <b>context</b> 2231 * <p> 2232 * Description: <b>Returns statements for a specific context (episode or episode 2233 * of Care).</b><br> 2234 * Type: <b>reference</b><br> 2235 * Path: <b>MedicationStatement.context</b><br> 2236 * </p> 2237 */ 2238 @SearchParamDefinition(name = "context", path = "MedicationStatement.context", description = "Returns statements for a specific context (episode or episode of Care).", type = "reference", target = { 2239 Encounter.class, EpisodeOfCare.class }) 2240 public static final String SP_CONTEXT = "context"; 2241 /** 2242 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2243 * <p> 2244 * Description: <b>Returns statements for a specific context (episode or episode 2245 * of Care).</b><br> 2246 * Type: <b>reference</b><br> 2247 * Path: <b>MedicationStatement.context</b><br> 2248 * </p> 2249 */ 2250 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2251 SP_CONTEXT); 2252 2253 /** 2254 * Constant for fluent queries to be used to add include statements. Specifies 2255 * the path value of "<b>MedicationStatement:context</b>". 2256 */ 2257 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include( 2258 "MedicationStatement:context").toLocked(); 2259 2260 /** 2261 * Search parameter: <b>medication</b> 2262 * <p> 2263 * Description: <b>Return statements of this medication reference</b><br> 2264 * Type: <b>reference</b><br> 2265 * Path: <b>MedicationStatement.medicationReference</b><br> 2266 * </p> 2267 */ 2268 @SearchParamDefinition(name = "medication", path = "(MedicationStatement.medication as Reference)", description = "Return statements of this medication reference", type = "reference", target = { 2269 Medication.class }) 2270 public static final String SP_MEDICATION = "medication"; 2271 /** 2272 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2273 * <p> 2274 * Description: <b>Return statements of this medication reference</b><br> 2275 * Type: <b>reference</b><br> 2276 * Path: <b>MedicationStatement.medicationReference</b><br> 2277 * </p> 2278 */ 2279 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2280 SP_MEDICATION); 2281 2282 /** 2283 * Constant for fluent queries to be used to add include statements. Specifies 2284 * the path value of "<b>MedicationStatement:medication</b>". 2285 */ 2286 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include( 2287 "MedicationStatement:medication").toLocked(); 2288 2289 /** 2290 * Search parameter: <b>part-of</b> 2291 * <p> 2292 * Description: <b>Returns statements that are part of another event.</b><br> 2293 * Type: <b>reference</b><br> 2294 * Path: <b>MedicationStatement.partOf</b><br> 2295 * </p> 2296 */ 2297 @SearchParamDefinition(name = "part-of", path = "MedicationStatement.partOf", description = "Returns statements that are part of another event.", type = "reference", target = { 2298 MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Observation.class, 2299 Procedure.class }) 2300 public static final String SP_PART_OF = "part-of"; 2301 /** 2302 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2303 * <p> 2304 * Description: <b>Returns statements that are part of another event.</b><br> 2305 * Type: <b>reference</b><br> 2306 * Path: <b>MedicationStatement.partOf</b><br> 2307 * </p> 2308 */ 2309 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2310 SP_PART_OF); 2311 2312 /** 2313 * Constant for fluent queries to be used to add include statements. Specifies 2314 * the path value of "<b>MedicationStatement:part-of</b>". 2315 */ 2316 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 2317 "MedicationStatement:part-of").toLocked(); 2318 2319 /** 2320 * Search parameter: <b>source</b> 2321 * <p> 2322 * Description: <b>Who or where the information in the statement came 2323 * from</b><br> 2324 * Type: <b>reference</b><br> 2325 * Path: <b>MedicationStatement.informationSource</b><br> 2326 * </p> 2327 */ 2328 @SearchParamDefinition(name = "source", path = "MedicationStatement.informationSource", description = "Who or where the information in the statement came from", type = "reference", providesMembershipIn = { 2329 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2330 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 2331 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2332 public static final String SP_SOURCE = "source"; 2333 /** 2334 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2335 * <p> 2336 * Description: <b>Who or where the information in the statement came 2337 * from</b><br> 2338 * Type: <b>reference</b><br> 2339 * Path: <b>MedicationStatement.informationSource</b><br> 2340 * </p> 2341 */ 2342 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2343 SP_SOURCE); 2344 2345 /** 2346 * Constant for fluent queries to be used to add include statements. Specifies 2347 * the path value of "<b>MedicationStatement:source</b>". 2348 */ 2349 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include( 2350 "MedicationStatement:source").toLocked(); 2351 2352 /** 2353 * Search parameter: <b>category</b> 2354 * <p> 2355 * Description: <b>Returns statements of this category of 2356 * medicationstatement</b><br> 2357 * Type: <b>token</b><br> 2358 * Path: <b>MedicationStatement.category</b><br> 2359 * </p> 2360 */ 2361 @SearchParamDefinition(name = "category", path = "MedicationStatement.category", description = "Returns statements of this category of medicationstatement", type = "token") 2362 public static final String SP_CATEGORY = "category"; 2363 /** 2364 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2365 * <p> 2366 * Description: <b>Returns statements of this category of 2367 * medicationstatement</b><br> 2368 * Type: <b>token</b><br> 2369 * Path: <b>MedicationStatement.category</b><br> 2370 * </p> 2371 */ 2372 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2373 SP_CATEGORY); 2374 2375 /** 2376 * Search parameter: <b>status</b> 2377 * <p> 2378 * Description: <b>Return statements that match the given status</b><br> 2379 * Type: <b>token</b><br> 2380 * Path: <b>MedicationStatement.status</b><br> 2381 * </p> 2382 */ 2383 @SearchParamDefinition(name = "status", path = "MedicationStatement.status", description = "Return statements that match the given status", type = "token") 2384 public static final String SP_STATUS = "status"; 2385 /** 2386 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2387 * <p> 2388 * Description: <b>Return statements that match the given status</b><br> 2389 * Type: <b>token</b><br> 2390 * Path: <b>MedicationStatement.status</b><br> 2391 * </p> 2392 */ 2393 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2394 SP_STATUS); 2395 2396}