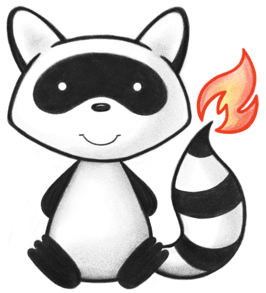
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Detailed definition of a medicinal product, typically for uses other than 048 * direct patient care (e.g. regulatory use). 049 */ 050@ResourceDef(name = "MedicinalProduct", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProduct") 051public class MedicinalProduct extends DomainResource { 052 053 @Block() 054 public static class MedicinalProductNameComponent extends BackboneElement implements IBaseBackboneElement { 055 /** 056 * The full product name. 057 */ 058 @Child(name = "productName", type = { 059 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "The full product name", formalDefinition = "The full product name.") 061 protected StringType productName; 062 063 /** 064 * Coding words or phrases of the name. 065 */ 066 @Child(name = "namePart", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 067 @Description(shortDefinition = "Coding words or phrases of the name", formalDefinition = "Coding words or phrases of the name.") 068 protected List<MedicinalProductNameNamePartComponent> namePart; 069 070 /** 071 * Country where the name applies. 072 */ 073 @Child(name = "countryLanguage", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 074 @Description(shortDefinition = "Country where the name applies", formalDefinition = "Country where the name applies.") 075 protected List<MedicinalProductNameCountryLanguageComponent> countryLanguage; 076 077 private static final long serialVersionUID = -2005005917L; 078 079 /** 080 * Constructor 081 */ 082 public MedicinalProductNameComponent() { 083 super(); 084 } 085 086 /** 087 * Constructor 088 */ 089 public MedicinalProductNameComponent(StringType productName) { 090 super(); 091 this.productName = productName; 092 } 093 094 /** 095 * @return {@link #productName} (The full product name.). This is the underlying 096 * object with id, value and extensions. The accessor "getProductName" 097 * gives direct access to the value 098 */ 099 public StringType getProductNameElement() { 100 if (this.productName == null) 101 if (Configuration.errorOnAutoCreate()) 102 throw new Error("Attempt to auto-create MedicinalProductNameComponent.productName"); 103 else if (Configuration.doAutoCreate()) 104 this.productName = new StringType(); // bb 105 return this.productName; 106 } 107 108 public boolean hasProductNameElement() { 109 return this.productName != null && !this.productName.isEmpty(); 110 } 111 112 public boolean hasProductName() { 113 return this.productName != null && !this.productName.isEmpty(); 114 } 115 116 /** 117 * @param value {@link #productName} (The full product name.). This is the 118 * underlying object with id, value and extensions. The accessor 119 * "getProductName" gives direct access to the value 120 */ 121 public MedicinalProductNameComponent setProductNameElement(StringType value) { 122 this.productName = value; 123 return this; 124 } 125 126 /** 127 * @return The full product name. 128 */ 129 public String getProductName() { 130 return this.productName == null ? null : this.productName.getValue(); 131 } 132 133 /** 134 * @param value The full product name. 135 */ 136 public MedicinalProductNameComponent setProductName(String value) { 137 if (this.productName == null) 138 this.productName = new StringType(); 139 this.productName.setValue(value); 140 return this; 141 } 142 143 /** 144 * @return {@link #namePart} (Coding words or phrases of the name.) 145 */ 146 public List<MedicinalProductNameNamePartComponent> getNamePart() { 147 if (this.namePart == null) 148 this.namePart = new ArrayList<MedicinalProductNameNamePartComponent>(); 149 return this.namePart; 150 } 151 152 /** 153 * @return Returns a reference to <code>this</code> for easy method chaining 154 */ 155 public MedicinalProductNameComponent setNamePart(List<MedicinalProductNameNamePartComponent> theNamePart) { 156 this.namePart = theNamePart; 157 return this; 158 } 159 160 public boolean hasNamePart() { 161 if (this.namePart == null) 162 return false; 163 for (MedicinalProductNameNamePartComponent item : this.namePart) 164 if (!item.isEmpty()) 165 return true; 166 return false; 167 } 168 169 public MedicinalProductNameNamePartComponent addNamePart() { // 3 170 MedicinalProductNameNamePartComponent t = new MedicinalProductNameNamePartComponent(); 171 if (this.namePart == null) 172 this.namePart = new ArrayList<MedicinalProductNameNamePartComponent>(); 173 this.namePart.add(t); 174 return t; 175 } 176 177 public MedicinalProductNameComponent addNamePart(MedicinalProductNameNamePartComponent t) { // 3 178 if (t == null) 179 return this; 180 if (this.namePart == null) 181 this.namePart = new ArrayList<MedicinalProductNameNamePartComponent>(); 182 this.namePart.add(t); 183 return this; 184 } 185 186 /** 187 * @return The first repetition of repeating field {@link #namePart}, creating 188 * it if it does not already exist 189 */ 190 public MedicinalProductNameNamePartComponent getNamePartFirstRep() { 191 if (getNamePart().isEmpty()) { 192 addNamePart(); 193 } 194 return getNamePart().get(0); 195 } 196 197 /** 198 * @return {@link #countryLanguage} (Country where the name applies.) 199 */ 200 public List<MedicinalProductNameCountryLanguageComponent> getCountryLanguage() { 201 if (this.countryLanguage == null) 202 this.countryLanguage = new ArrayList<MedicinalProductNameCountryLanguageComponent>(); 203 return this.countryLanguage; 204 } 205 206 /** 207 * @return Returns a reference to <code>this</code> for easy method chaining 208 */ 209 public MedicinalProductNameComponent setCountryLanguage( 210 List<MedicinalProductNameCountryLanguageComponent> theCountryLanguage) { 211 this.countryLanguage = theCountryLanguage; 212 return this; 213 } 214 215 public boolean hasCountryLanguage() { 216 if (this.countryLanguage == null) 217 return false; 218 for (MedicinalProductNameCountryLanguageComponent item : this.countryLanguage) 219 if (!item.isEmpty()) 220 return true; 221 return false; 222 } 223 224 public MedicinalProductNameCountryLanguageComponent addCountryLanguage() { // 3 225 MedicinalProductNameCountryLanguageComponent t = new MedicinalProductNameCountryLanguageComponent(); 226 if (this.countryLanguage == null) 227 this.countryLanguage = new ArrayList<MedicinalProductNameCountryLanguageComponent>(); 228 this.countryLanguage.add(t); 229 return t; 230 } 231 232 public MedicinalProductNameComponent addCountryLanguage(MedicinalProductNameCountryLanguageComponent t) { // 3 233 if (t == null) 234 return this; 235 if (this.countryLanguage == null) 236 this.countryLanguage = new ArrayList<MedicinalProductNameCountryLanguageComponent>(); 237 this.countryLanguage.add(t); 238 return this; 239 } 240 241 /** 242 * @return The first repetition of repeating field {@link #countryLanguage}, 243 * creating it if it does not already exist 244 */ 245 public MedicinalProductNameCountryLanguageComponent getCountryLanguageFirstRep() { 246 if (getCountryLanguage().isEmpty()) { 247 addCountryLanguage(); 248 } 249 return getCountryLanguage().get(0); 250 } 251 252 protected void listChildren(List<Property> children) { 253 super.listChildren(children); 254 children.add(new Property("productName", "string", "The full product name.", 0, 1, productName)); 255 children.add(new Property("namePart", "", "Coding words or phrases of the name.", 0, java.lang.Integer.MAX_VALUE, 256 namePart)); 257 children.add(new Property("countryLanguage", "", "Country where the name applies.", 0, 258 java.lang.Integer.MAX_VALUE, countryLanguage)); 259 } 260 261 @Override 262 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 263 switch (_hash) { 264 case -1491817446: 265 /* productName */ return new Property("productName", "string", "The full product name.", 0, 1, productName); 266 case 1840452894: 267 /* namePart */ return new Property("namePart", "", "Coding words or phrases of the name.", 0, 268 java.lang.Integer.MAX_VALUE, namePart); 269 case -141141746: 270 /* countryLanguage */ return new Property("countryLanguage", "", "Country where the name applies.", 0, 271 java.lang.Integer.MAX_VALUE, countryLanguage); 272 default: 273 return super.getNamedProperty(_hash, _name, _checkValid); 274 } 275 276 } 277 278 @Override 279 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 280 switch (hash) { 281 case -1491817446: 282 /* productName */ return this.productName == null ? new Base[0] : new Base[] { this.productName }; // StringType 283 case 1840452894: 284 /* namePart */ return this.namePart == null ? new Base[0] 285 : this.namePart.toArray(new Base[this.namePart.size()]); // MedicinalProductNameNamePartComponent 286 case -141141746: 287 /* countryLanguage */ return this.countryLanguage == null ? new Base[0] 288 : this.countryLanguage.toArray(new Base[this.countryLanguage.size()]); // MedicinalProductNameCountryLanguageComponent 289 default: 290 return super.getProperty(hash, name, checkValid); 291 } 292 293 } 294 295 @Override 296 public Base setProperty(int hash, String name, Base value) throws FHIRException { 297 switch (hash) { 298 case -1491817446: // productName 299 this.productName = castToString(value); // StringType 300 return value; 301 case 1840452894: // namePart 302 this.getNamePart().add((MedicinalProductNameNamePartComponent) value); // MedicinalProductNameNamePartComponent 303 return value; 304 case -141141746: // countryLanguage 305 this.getCountryLanguage().add((MedicinalProductNameCountryLanguageComponent) value); // MedicinalProductNameCountryLanguageComponent 306 return value; 307 default: 308 return super.setProperty(hash, name, value); 309 } 310 311 } 312 313 @Override 314 public Base setProperty(String name, Base value) throws FHIRException { 315 if (name.equals("productName")) { 316 this.productName = castToString(value); // StringType 317 } else if (name.equals("namePart")) { 318 this.getNamePart().add((MedicinalProductNameNamePartComponent) value); 319 } else if (name.equals("countryLanguage")) { 320 this.getCountryLanguage().add((MedicinalProductNameCountryLanguageComponent) value); 321 } else 322 return super.setProperty(name, value); 323 return value; 324 } 325 326 @Override 327 public Base makeProperty(int hash, String name) throws FHIRException { 328 switch (hash) { 329 case -1491817446: 330 return getProductNameElement(); 331 case 1840452894: 332 return addNamePart(); 333 case -141141746: 334 return addCountryLanguage(); 335 default: 336 return super.makeProperty(hash, name); 337 } 338 339 } 340 341 @Override 342 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 343 switch (hash) { 344 case -1491817446: 345 /* productName */ return new String[] { "string" }; 346 case 1840452894: 347 /* namePart */ return new String[] {}; 348 case -141141746: 349 /* countryLanguage */ return new String[] {}; 350 default: 351 return super.getTypesForProperty(hash, name); 352 } 353 354 } 355 356 @Override 357 public Base addChild(String name) throws FHIRException { 358 if (name.equals("productName")) { 359 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProduct.productName"); 360 } else if (name.equals("namePart")) { 361 return addNamePart(); 362 } else if (name.equals("countryLanguage")) { 363 return addCountryLanguage(); 364 } else 365 return super.addChild(name); 366 } 367 368 public MedicinalProductNameComponent copy() { 369 MedicinalProductNameComponent dst = new MedicinalProductNameComponent(); 370 copyValues(dst); 371 return dst; 372 } 373 374 public void copyValues(MedicinalProductNameComponent dst) { 375 super.copyValues(dst); 376 dst.productName = productName == null ? null : productName.copy(); 377 if (namePart != null) { 378 dst.namePart = new ArrayList<MedicinalProductNameNamePartComponent>(); 379 for (MedicinalProductNameNamePartComponent i : namePart) 380 dst.namePart.add(i.copy()); 381 } 382 ; 383 if (countryLanguage != null) { 384 dst.countryLanguage = new ArrayList<MedicinalProductNameCountryLanguageComponent>(); 385 for (MedicinalProductNameCountryLanguageComponent i : countryLanguage) 386 dst.countryLanguage.add(i.copy()); 387 } 388 ; 389 } 390 391 @Override 392 public boolean equalsDeep(Base other_) { 393 if (!super.equalsDeep(other_)) 394 return false; 395 if (!(other_ instanceof MedicinalProductNameComponent)) 396 return false; 397 MedicinalProductNameComponent o = (MedicinalProductNameComponent) other_; 398 return compareDeep(productName, o.productName, true) && compareDeep(namePart, o.namePart, true) 399 && compareDeep(countryLanguage, o.countryLanguage, true); 400 } 401 402 @Override 403 public boolean equalsShallow(Base other_) { 404 if (!super.equalsShallow(other_)) 405 return false; 406 if (!(other_ instanceof MedicinalProductNameComponent)) 407 return false; 408 MedicinalProductNameComponent o = (MedicinalProductNameComponent) other_; 409 return compareValues(productName, o.productName, true); 410 } 411 412 public boolean isEmpty() { 413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(productName, namePart, countryLanguage); 414 } 415 416 public String fhirType() { 417 return "MedicinalProduct.name"; 418 419 } 420 421 } 422 423 @Block() 424 public static class MedicinalProductNameNamePartComponent extends BackboneElement implements IBaseBackboneElement { 425 /** 426 * A fragment of a product name. 427 */ 428 @Child(name = "part", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 429 @Description(shortDefinition = "A fragment of a product name", formalDefinition = "A fragment of a product name.") 430 protected StringType part; 431 432 /** 433 * Idenifying type for this part of the name (e.g. strength part). 434 */ 435 @Child(name = "type", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 436 @Description(shortDefinition = "Idenifying type for this part of the name (e.g. strength part)", formalDefinition = "Idenifying type for this part of the name (e.g. strength part).") 437 protected Coding type; 438 439 private static final long serialVersionUID = -301533796L; 440 441 /** 442 * Constructor 443 */ 444 public MedicinalProductNameNamePartComponent() { 445 super(); 446 } 447 448 /** 449 * Constructor 450 */ 451 public MedicinalProductNameNamePartComponent(StringType part, Coding type) { 452 super(); 453 this.part = part; 454 this.type = type; 455 } 456 457 /** 458 * @return {@link #part} (A fragment of a product name.). This is the underlying 459 * object with id, value and extensions. The accessor "getPart" gives 460 * direct access to the value 461 */ 462 public StringType getPartElement() { 463 if (this.part == null) 464 if (Configuration.errorOnAutoCreate()) 465 throw new Error("Attempt to auto-create MedicinalProductNameNamePartComponent.part"); 466 else if (Configuration.doAutoCreate()) 467 this.part = new StringType(); // bb 468 return this.part; 469 } 470 471 public boolean hasPartElement() { 472 return this.part != null && !this.part.isEmpty(); 473 } 474 475 public boolean hasPart() { 476 return this.part != null && !this.part.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #part} (A fragment of a product name.). This is the 481 * underlying object with id, value and extensions. The accessor 482 * "getPart" gives direct access to the value 483 */ 484 public MedicinalProductNameNamePartComponent setPartElement(StringType value) { 485 this.part = value; 486 return this; 487 } 488 489 /** 490 * @return A fragment of a product name. 491 */ 492 public String getPart() { 493 return this.part == null ? null : this.part.getValue(); 494 } 495 496 /** 497 * @param value A fragment of a product name. 498 */ 499 public MedicinalProductNameNamePartComponent setPart(String value) { 500 if (this.part == null) 501 this.part = new StringType(); 502 this.part.setValue(value); 503 return this; 504 } 505 506 /** 507 * @return {@link #type} (Idenifying type for this part of the name (e.g. 508 * strength part).) 509 */ 510 public Coding getType() { 511 if (this.type == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create MedicinalProductNameNamePartComponent.type"); 514 else if (Configuration.doAutoCreate()) 515 this.type = new Coding(); // cc 516 return this.type; 517 } 518 519 public boolean hasType() { 520 return this.type != null && !this.type.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #type} (Idenifying type for this part of the name (e.g. 525 * strength part).) 526 */ 527 public MedicinalProductNameNamePartComponent setType(Coding value) { 528 this.type = value; 529 return this; 530 } 531 532 protected void listChildren(List<Property> children) { 533 super.listChildren(children); 534 children.add(new Property("part", "string", "A fragment of a product name.", 0, 1, part)); 535 children.add(new Property("type", "Coding", "Idenifying type for this part of the name (e.g. strength part).", 0, 536 1, type)); 537 } 538 539 @Override 540 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 541 switch (_hash) { 542 case 3433459: 543 /* part */ return new Property("part", "string", "A fragment of a product name.", 0, 1, part); 544 case 3575610: 545 /* type */ return new Property("type", "Coding", 546 "Idenifying type for this part of the name (e.g. strength part).", 0, 1, type); 547 default: 548 return super.getNamedProperty(_hash, _name, _checkValid); 549 } 550 551 } 552 553 @Override 554 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 555 switch (hash) { 556 case 3433459: 557 /* part */ return this.part == null ? new Base[0] : new Base[] { this.part }; // StringType 558 case 3575610: 559 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 560 default: 561 return super.getProperty(hash, name, checkValid); 562 } 563 564 } 565 566 @Override 567 public Base setProperty(int hash, String name, Base value) throws FHIRException { 568 switch (hash) { 569 case 3433459: // part 570 this.part = castToString(value); // StringType 571 return value; 572 case 3575610: // type 573 this.type = castToCoding(value); // Coding 574 return value; 575 default: 576 return super.setProperty(hash, name, value); 577 } 578 579 } 580 581 @Override 582 public Base setProperty(String name, Base value) throws FHIRException { 583 if (name.equals("part")) { 584 this.part = castToString(value); // StringType 585 } else if (name.equals("type")) { 586 this.type = castToCoding(value); // Coding 587 } else 588 return super.setProperty(name, value); 589 return value; 590 } 591 592 @Override 593 public Base makeProperty(int hash, String name) throws FHIRException { 594 switch (hash) { 595 case 3433459: 596 return getPartElement(); 597 case 3575610: 598 return getType(); 599 default: 600 return super.makeProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 607 switch (hash) { 608 case 3433459: 609 /* part */ return new String[] { "string" }; 610 case 3575610: 611 /* type */ return new String[] { "Coding" }; 612 default: 613 return super.getTypesForProperty(hash, name); 614 } 615 616 } 617 618 @Override 619 public Base addChild(String name) throws FHIRException { 620 if (name.equals("part")) { 621 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProduct.part"); 622 } else if (name.equals("type")) { 623 this.type = new Coding(); 624 return this.type; 625 } else 626 return super.addChild(name); 627 } 628 629 public MedicinalProductNameNamePartComponent copy() { 630 MedicinalProductNameNamePartComponent dst = new MedicinalProductNameNamePartComponent(); 631 copyValues(dst); 632 return dst; 633 } 634 635 public void copyValues(MedicinalProductNameNamePartComponent dst) { 636 super.copyValues(dst); 637 dst.part = part == null ? null : part.copy(); 638 dst.type = type == null ? null : type.copy(); 639 } 640 641 @Override 642 public boolean equalsDeep(Base other_) { 643 if (!super.equalsDeep(other_)) 644 return false; 645 if (!(other_ instanceof MedicinalProductNameNamePartComponent)) 646 return false; 647 MedicinalProductNameNamePartComponent o = (MedicinalProductNameNamePartComponent) other_; 648 return compareDeep(part, o.part, true) && compareDeep(type, o.type, true); 649 } 650 651 @Override 652 public boolean equalsShallow(Base other_) { 653 if (!super.equalsShallow(other_)) 654 return false; 655 if (!(other_ instanceof MedicinalProductNameNamePartComponent)) 656 return false; 657 MedicinalProductNameNamePartComponent o = (MedicinalProductNameNamePartComponent) other_; 658 return compareValues(part, o.part, true); 659 } 660 661 public boolean isEmpty() { 662 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(part, type); 663 } 664 665 public String fhirType() { 666 return "MedicinalProduct.name.namePart"; 667 668 } 669 670 } 671 672 @Block() 673 public static class MedicinalProductNameCountryLanguageComponent extends BackboneElement 674 implements IBaseBackboneElement { 675 /** 676 * Country code for where this name applies. 677 */ 678 @Child(name = "country", type = { 679 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 680 @Description(shortDefinition = "Country code for where this name applies", formalDefinition = "Country code for where this name applies.") 681 protected CodeableConcept country; 682 683 /** 684 * Jurisdiction code for where this name applies. 685 */ 686 @Child(name = "jurisdiction", type = { 687 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 688 @Description(shortDefinition = "Jurisdiction code for where this name applies", formalDefinition = "Jurisdiction code for where this name applies.") 689 protected CodeableConcept jurisdiction; 690 691 /** 692 * Language code for this name. 693 */ 694 @Child(name = "language", type = { 695 CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 696 @Description(shortDefinition = "Language code for this name", formalDefinition = "Language code for this name.") 697 protected CodeableConcept language; 698 699 private static final long serialVersionUID = 1627157564L; 700 701 /** 702 * Constructor 703 */ 704 public MedicinalProductNameCountryLanguageComponent() { 705 super(); 706 } 707 708 /** 709 * Constructor 710 */ 711 public MedicinalProductNameCountryLanguageComponent(CodeableConcept country, CodeableConcept language) { 712 super(); 713 this.country = country; 714 this.language = language; 715 } 716 717 /** 718 * @return {@link #country} (Country code for where this name applies.) 719 */ 720 public CodeableConcept getCountry() { 721 if (this.country == null) 722 if (Configuration.errorOnAutoCreate()) 723 throw new Error("Attempt to auto-create MedicinalProductNameCountryLanguageComponent.country"); 724 else if (Configuration.doAutoCreate()) 725 this.country = new CodeableConcept(); // cc 726 return this.country; 727 } 728 729 public boolean hasCountry() { 730 return this.country != null && !this.country.isEmpty(); 731 } 732 733 /** 734 * @param value {@link #country} (Country code for where this name applies.) 735 */ 736 public MedicinalProductNameCountryLanguageComponent setCountry(CodeableConcept value) { 737 this.country = value; 738 return this; 739 } 740 741 /** 742 * @return {@link #jurisdiction} (Jurisdiction code for where this name 743 * applies.) 744 */ 745 public CodeableConcept getJurisdiction() { 746 if (this.jurisdiction == null) 747 if (Configuration.errorOnAutoCreate()) 748 throw new Error("Attempt to auto-create MedicinalProductNameCountryLanguageComponent.jurisdiction"); 749 else if (Configuration.doAutoCreate()) 750 this.jurisdiction = new CodeableConcept(); // cc 751 return this.jurisdiction; 752 } 753 754 public boolean hasJurisdiction() { 755 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 756 } 757 758 /** 759 * @param value {@link #jurisdiction} (Jurisdiction code for where this name 760 * applies.) 761 */ 762 public MedicinalProductNameCountryLanguageComponent setJurisdiction(CodeableConcept value) { 763 this.jurisdiction = value; 764 return this; 765 } 766 767 /** 768 * @return {@link #language} (Language code for this name.) 769 */ 770 public CodeableConcept getLanguage() { 771 if (this.language == null) 772 if (Configuration.errorOnAutoCreate()) 773 throw new Error("Attempt to auto-create MedicinalProductNameCountryLanguageComponent.language"); 774 else if (Configuration.doAutoCreate()) 775 this.language = new CodeableConcept(); // cc 776 return this.language; 777 } 778 779 public boolean hasLanguage() { 780 return this.language != null && !this.language.isEmpty(); 781 } 782 783 /** 784 * @param value {@link #language} (Language code for this name.) 785 */ 786 public MedicinalProductNameCountryLanguageComponent setLanguage(CodeableConcept value) { 787 this.language = value; 788 return this; 789 } 790 791 protected void listChildren(List<Property> children) { 792 super.listChildren(children); 793 children 794 .add(new Property("country", "CodeableConcept", "Country code for where this name applies.", 0, 1, country)); 795 children.add(new Property("jurisdiction", "CodeableConcept", "Jurisdiction code for where this name applies.", 0, 796 1, jurisdiction)); 797 children.add(new Property("language", "CodeableConcept", "Language code for this name.", 0, 1, language)); 798 } 799 800 @Override 801 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 802 switch (_hash) { 803 case 957831062: 804 /* country */ return new Property("country", "CodeableConcept", "Country code for where this name applies.", 0, 805 1, country); 806 case -507075711: 807 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 808 "Jurisdiction code for where this name applies.", 0, 1, jurisdiction); 809 case -1613589672: 810 /* language */ return new Property("language", "CodeableConcept", "Language code for this name.", 0, 1, 811 language); 812 default: 813 return super.getNamedProperty(_hash, _name, _checkValid); 814 } 815 816 } 817 818 @Override 819 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 820 switch (hash) { 821 case 957831062: 822 /* country */ return this.country == null ? new Base[0] : new Base[] { this.country }; // CodeableConcept 823 case -507075711: 824 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] : new Base[] { this.jurisdiction }; // CodeableConcept 825 case -1613589672: 826 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 827 default: 828 return super.getProperty(hash, name, checkValid); 829 } 830 831 } 832 833 @Override 834 public Base setProperty(int hash, String name, Base value) throws FHIRException { 835 switch (hash) { 836 case 957831062: // country 837 this.country = castToCodeableConcept(value); // CodeableConcept 838 return value; 839 case -507075711: // jurisdiction 840 this.jurisdiction = castToCodeableConcept(value); // CodeableConcept 841 return value; 842 case -1613589672: // language 843 this.language = castToCodeableConcept(value); // CodeableConcept 844 return value; 845 default: 846 return super.setProperty(hash, name, value); 847 } 848 849 } 850 851 @Override 852 public Base setProperty(String name, Base value) throws FHIRException { 853 if (name.equals("country")) { 854 this.country = castToCodeableConcept(value); // CodeableConcept 855 } else if (name.equals("jurisdiction")) { 856 this.jurisdiction = castToCodeableConcept(value); // CodeableConcept 857 } else if (name.equals("language")) { 858 this.language = castToCodeableConcept(value); // CodeableConcept 859 } else 860 return super.setProperty(name, value); 861 return value; 862 } 863 864 @Override 865 public Base makeProperty(int hash, String name) throws FHIRException { 866 switch (hash) { 867 case 957831062: 868 return getCountry(); 869 case -507075711: 870 return getJurisdiction(); 871 case -1613589672: 872 return getLanguage(); 873 default: 874 return super.makeProperty(hash, name); 875 } 876 877 } 878 879 @Override 880 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 881 switch (hash) { 882 case 957831062: 883 /* country */ return new String[] { "CodeableConcept" }; 884 case -507075711: 885 /* jurisdiction */ return new String[] { "CodeableConcept" }; 886 case -1613589672: 887 /* language */ return new String[] { "CodeableConcept" }; 888 default: 889 return super.getTypesForProperty(hash, name); 890 } 891 892 } 893 894 @Override 895 public Base addChild(String name) throws FHIRException { 896 if (name.equals("country")) { 897 this.country = new CodeableConcept(); 898 return this.country; 899 } else if (name.equals("jurisdiction")) { 900 this.jurisdiction = new CodeableConcept(); 901 return this.jurisdiction; 902 } else if (name.equals("language")) { 903 this.language = new CodeableConcept(); 904 return this.language; 905 } else 906 return super.addChild(name); 907 } 908 909 public MedicinalProductNameCountryLanguageComponent copy() { 910 MedicinalProductNameCountryLanguageComponent dst = new MedicinalProductNameCountryLanguageComponent(); 911 copyValues(dst); 912 return dst; 913 } 914 915 public void copyValues(MedicinalProductNameCountryLanguageComponent dst) { 916 super.copyValues(dst); 917 dst.country = country == null ? null : country.copy(); 918 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 919 dst.language = language == null ? null : language.copy(); 920 } 921 922 @Override 923 public boolean equalsDeep(Base other_) { 924 if (!super.equalsDeep(other_)) 925 return false; 926 if (!(other_ instanceof MedicinalProductNameCountryLanguageComponent)) 927 return false; 928 MedicinalProductNameCountryLanguageComponent o = (MedicinalProductNameCountryLanguageComponent) other_; 929 return compareDeep(country, o.country, true) && compareDeep(jurisdiction, o.jurisdiction, true) 930 && compareDeep(language, o.language, true); 931 } 932 933 @Override 934 public boolean equalsShallow(Base other_) { 935 if (!super.equalsShallow(other_)) 936 return false; 937 if (!(other_ instanceof MedicinalProductNameCountryLanguageComponent)) 938 return false; 939 MedicinalProductNameCountryLanguageComponent o = (MedicinalProductNameCountryLanguageComponent) other_; 940 return true; 941 } 942 943 public boolean isEmpty() { 944 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(country, jurisdiction, language); 945 } 946 947 public String fhirType() { 948 return "MedicinalProduct.name.countryLanguage"; 949 950 } 951 952 } 953 954 @Block() 955 public static class MedicinalProductManufacturingBusinessOperationComponent extends BackboneElement 956 implements IBaseBackboneElement { 957 /** 958 * The type of manufacturing operation. 959 */ 960 @Child(name = "operationType", type = { 961 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 962 @Description(shortDefinition = "The type of manufacturing operation", formalDefinition = "The type of manufacturing operation.") 963 protected CodeableConcept operationType; 964 965 /** 966 * Regulatory authorization reference number. 967 */ 968 @Child(name = "authorisationReferenceNumber", type = { 969 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 970 @Description(shortDefinition = "Regulatory authorization reference number", formalDefinition = "Regulatory authorization reference number.") 971 protected Identifier authorisationReferenceNumber; 972 973 /** 974 * Regulatory authorization date. 975 */ 976 @Child(name = "effectiveDate", type = { 977 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 978 @Description(shortDefinition = "Regulatory authorization date", formalDefinition = "Regulatory authorization date.") 979 protected DateTimeType effectiveDate; 980 981 /** 982 * To indicate if this proces is commercially confidential. 983 */ 984 @Child(name = "confidentialityIndicator", type = { 985 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 986 @Description(shortDefinition = "To indicate if this proces is commercially confidential", formalDefinition = "To indicate if this proces is commercially confidential.") 987 protected CodeableConcept confidentialityIndicator; 988 989 /** 990 * The manufacturer or establishment associated with the process. 991 */ 992 @Child(name = "manufacturer", type = { 993 Organization.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 994 @Description(shortDefinition = "The manufacturer or establishment associated with the process", formalDefinition = "The manufacturer or establishment associated with the process.") 995 protected List<Reference> manufacturer; 996 /** 997 * The actual objects that are the target of the reference (The manufacturer or 998 * establishment associated with the process.) 999 */ 1000 protected List<Organization> manufacturerTarget; 1001 1002 /** 1003 * A regulator which oversees the operation. 1004 */ 1005 @Child(name = "regulator", type = { 1006 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1007 @Description(shortDefinition = "A regulator which oversees the operation", formalDefinition = "A regulator which oversees the operation.") 1008 protected Reference regulator; 1009 1010 /** 1011 * The actual object that is the target of the reference (A regulator which 1012 * oversees the operation.) 1013 */ 1014 protected Organization regulatorTarget; 1015 1016 private static final long serialVersionUID = 1259822353L; 1017 1018 /** 1019 * Constructor 1020 */ 1021 public MedicinalProductManufacturingBusinessOperationComponent() { 1022 super(); 1023 } 1024 1025 /** 1026 * @return {@link #operationType} (The type of manufacturing operation.) 1027 */ 1028 public CodeableConcept getOperationType() { 1029 if (this.operationType == null) 1030 if (Configuration.errorOnAutoCreate()) 1031 throw new Error( 1032 "Attempt to auto-create MedicinalProductManufacturingBusinessOperationComponent.operationType"); 1033 else if (Configuration.doAutoCreate()) 1034 this.operationType = new CodeableConcept(); // cc 1035 return this.operationType; 1036 } 1037 1038 public boolean hasOperationType() { 1039 return this.operationType != null && !this.operationType.isEmpty(); 1040 } 1041 1042 /** 1043 * @param value {@link #operationType} (The type of manufacturing operation.) 1044 */ 1045 public MedicinalProductManufacturingBusinessOperationComponent setOperationType(CodeableConcept value) { 1046 this.operationType = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return {@link #authorisationReferenceNumber} (Regulatory authorization 1052 * reference number.) 1053 */ 1054 public Identifier getAuthorisationReferenceNumber() { 1055 if (this.authorisationReferenceNumber == null) 1056 if (Configuration.errorOnAutoCreate()) 1057 throw new Error( 1058 "Attempt to auto-create MedicinalProductManufacturingBusinessOperationComponent.authorisationReferenceNumber"); 1059 else if (Configuration.doAutoCreate()) 1060 this.authorisationReferenceNumber = new Identifier(); // cc 1061 return this.authorisationReferenceNumber; 1062 } 1063 1064 public boolean hasAuthorisationReferenceNumber() { 1065 return this.authorisationReferenceNumber != null && !this.authorisationReferenceNumber.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #authorisationReferenceNumber} (Regulatory authorization 1070 * reference number.) 1071 */ 1072 public MedicinalProductManufacturingBusinessOperationComponent setAuthorisationReferenceNumber(Identifier value) { 1073 this.authorisationReferenceNumber = value; 1074 return this; 1075 } 1076 1077 /** 1078 * @return {@link #effectiveDate} (Regulatory authorization date.). This is the 1079 * underlying object with id, value and extensions. The accessor 1080 * "getEffectiveDate" gives direct access to the value 1081 */ 1082 public DateTimeType getEffectiveDateElement() { 1083 if (this.effectiveDate == null) 1084 if (Configuration.errorOnAutoCreate()) 1085 throw new Error( 1086 "Attempt to auto-create MedicinalProductManufacturingBusinessOperationComponent.effectiveDate"); 1087 else if (Configuration.doAutoCreate()) 1088 this.effectiveDate = new DateTimeType(); // bb 1089 return this.effectiveDate; 1090 } 1091 1092 public boolean hasEffectiveDateElement() { 1093 return this.effectiveDate != null && !this.effectiveDate.isEmpty(); 1094 } 1095 1096 public boolean hasEffectiveDate() { 1097 return this.effectiveDate != null && !this.effectiveDate.isEmpty(); 1098 } 1099 1100 /** 1101 * @param value {@link #effectiveDate} (Regulatory authorization date.). This is 1102 * the underlying object with id, value and extensions. The 1103 * accessor "getEffectiveDate" gives direct access to the value 1104 */ 1105 public MedicinalProductManufacturingBusinessOperationComponent setEffectiveDateElement(DateTimeType value) { 1106 this.effectiveDate = value; 1107 return this; 1108 } 1109 1110 /** 1111 * @return Regulatory authorization date. 1112 */ 1113 public Date getEffectiveDate() { 1114 return this.effectiveDate == null ? null : this.effectiveDate.getValue(); 1115 } 1116 1117 /** 1118 * @param value Regulatory authorization date. 1119 */ 1120 public MedicinalProductManufacturingBusinessOperationComponent setEffectiveDate(Date value) { 1121 if (value == null) 1122 this.effectiveDate = null; 1123 else { 1124 if (this.effectiveDate == null) 1125 this.effectiveDate = new DateTimeType(); 1126 this.effectiveDate.setValue(value); 1127 } 1128 return this; 1129 } 1130 1131 /** 1132 * @return {@link #confidentialityIndicator} (To indicate if this proces is 1133 * commercially confidential.) 1134 */ 1135 public CodeableConcept getConfidentialityIndicator() { 1136 if (this.confidentialityIndicator == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error( 1139 "Attempt to auto-create MedicinalProductManufacturingBusinessOperationComponent.confidentialityIndicator"); 1140 else if (Configuration.doAutoCreate()) 1141 this.confidentialityIndicator = new CodeableConcept(); // cc 1142 return this.confidentialityIndicator; 1143 } 1144 1145 public boolean hasConfidentialityIndicator() { 1146 return this.confidentialityIndicator != null && !this.confidentialityIndicator.isEmpty(); 1147 } 1148 1149 /** 1150 * @param value {@link #confidentialityIndicator} (To indicate if this proces is 1151 * commercially confidential.) 1152 */ 1153 public MedicinalProductManufacturingBusinessOperationComponent setConfidentialityIndicator(CodeableConcept value) { 1154 this.confidentialityIndicator = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return {@link #manufacturer} (The manufacturer or establishment associated 1160 * with the process.) 1161 */ 1162 public List<Reference> getManufacturer() { 1163 if (this.manufacturer == null) 1164 this.manufacturer = new ArrayList<Reference>(); 1165 return this.manufacturer; 1166 } 1167 1168 /** 1169 * @return Returns a reference to <code>this</code> for easy method chaining 1170 */ 1171 public MedicinalProductManufacturingBusinessOperationComponent setManufacturer(List<Reference> theManufacturer) { 1172 this.manufacturer = theManufacturer; 1173 return this; 1174 } 1175 1176 public boolean hasManufacturer() { 1177 if (this.manufacturer == null) 1178 return false; 1179 for (Reference item : this.manufacturer) 1180 if (!item.isEmpty()) 1181 return true; 1182 return false; 1183 } 1184 1185 public Reference addManufacturer() { // 3 1186 Reference t = new Reference(); 1187 if (this.manufacturer == null) 1188 this.manufacturer = new ArrayList<Reference>(); 1189 this.manufacturer.add(t); 1190 return t; 1191 } 1192 1193 public MedicinalProductManufacturingBusinessOperationComponent addManufacturer(Reference t) { // 3 1194 if (t == null) 1195 return this; 1196 if (this.manufacturer == null) 1197 this.manufacturer = new ArrayList<Reference>(); 1198 this.manufacturer.add(t); 1199 return this; 1200 } 1201 1202 /** 1203 * @return The first repetition of repeating field {@link #manufacturer}, 1204 * creating it if it does not already exist 1205 */ 1206 public Reference getManufacturerFirstRep() { 1207 if (getManufacturer().isEmpty()) { 1208 addManufacturer(); 1209 } 1210 return getManufacturer().get(0); 1211 } 1212 1213 /** 1214 * @deprecated Use Reference#setResource(IBaseResource) instead 1215 */ 1216 @Deprecated 1217 public List<Organization> getManufacturerTarget() { 1218 if (this.manufacturerTarget == null) 1219 this.manufacturerTarget = new ArrayList<Organization>(); 1220 return this.manufacturerTarget; 1221 } 1222 1223 /** 1224 * @deprecated Use Reference#setResource(IBaseResource) instead 1225 */ 1226 @Deprecated 1227 public Organization addManufacturerTarget() { 1228 Organization r = new Organization(); 1229 if (this.manufacturerTarget == null) 1230 this.manufacturerTarget = new ArrayList<Organization>(); 1231 this.manufacturerTarget.add(r); 1232 return r; 1233 } 1234 1235 /** 1236 * @return {@link #regulator} (A regulator which oversees the operation.) 1237 */ 1238 public Reference getRegulator() { 1239 if (this.regulator == null) 1240 if (Configuration.errorOnAutoCreate()) 1241 throw new Error("Attempt to auto-create MedicinalProductManufacturingBusinessOperationComponent.regulator"); 1242 else if (Configuration.doAutoCreate()) 1243 this.regulator = new Reference(); // cc 1244 return this.regulator; 1245 } 1246 1247 public boolean hasRegulator() { 1248 return this.regulator != null && !this.regulator.isEmpty(); 1249 } 1250 1251 /** 1252 * @param value {@link #regulator} (A regulator which oversees the operation.) 1253 */ 1254 public MedicinalProductManufacturingBusinessOperationComponent setRegulator(Reference value) { 1255 this.regulator = value; 1256 return this; 1257 } 1258 1259 /** 1260 * @return {@link #regulator} The actual object that is the target of the 1261 * reference. The reference library doesn't populate this, but you can 1262 * use it to hold the resource if you resolve it. (A regulator which 1263 * oversees the operation.) 1264 */ 1265 public Organization getRegulatorTarget() { 1266 if (this.regulatorTarget == null) 1267 if (Configuration.errorOnAutoCreate()) 1268 throw new Error("Attempt to auto-create MedicinalProductManufacturingBusinessOperationComponent.regulator"); 1269 else if (Configuration.doAutoCreate()) 1270 this.regulatorTarget = new Organization(); // aa 1271 return this.regulatorTarget; 1272 } 1273 1274 /** 1275 * @param value {@link #regulator} The actual object that is the target of the 1276 * reference. The reference library doesn't use these, but you can 1277 * use it to hold the resource if you resolve it. (A regulator 1278 * which oversees the operation.) 1279 */ 1280 public MedicinalProductManufacturingBusinessOperationComponent setRegulatorTarget(Organization value) { 1281 this.regulatorTarget = value; 1282 return this; 1283 } 1284 1285 protected void listChildren(List<Property> children) { 1286 super.listChildren(children); 1287 children.add(new Property("operationType", "CodeableConcept", "The type of manufacturing operation.", 0, 1, 1288 operationType)); 1289 children.add(new Property("authorisationReferenceNumber", "Identifier", 1290 "Regulatory authorization reference number.", 0, 1, authorisationReferenceNumber)); 1291 children.add(new Property("effectiveDate", "dateTime", "Regulatory authorization date.", 0, 1, effectiveDate)); 1292 children.add(new Property("confidentialityIndicator", "CodeableConcept", 1293 "To indicate if this proces is commercially confidential.", 0, 1, confidentialityIndicator)); 1294 children.add(new Property("manufacturer", "Reference(Organization)", 1295 "The manufacturer or establishment associated with the process.", 0, java.lang.Integer.MAX_VALUE, 1296 manufacturer)); 1297 children.add(new Property("regulator", "Reference(Organization)", "A regulator which oversees the operation.", 0, 1298 1, regulator)); 1299 } 1300 1301 @Override 1302 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1303 switch (_hash) { 1304 case 91999553: 1305 /* operationType */ return new Property("operationType", "CodeableConcept", 1306 "The type of manufacturing operation.", 0, 1, operationType); 1307 case -1940839884: 1308 /* authorisationReferenceNumber */ return new Property("authorisationReferenceNumber", "Identifier", 1309 "Regulatory authorization reference number.", 0, 1, authorisationReferenceNumber); 1310 case -930389515: 1311 /* effectiveDate */ return new Property("effectiveDate", "dateTime", "Regulatory authorization date.", 0, 1, 1312 effectiveDate); 1313 case -1449404791: 1314 /* confidentialityIndicator */ return new Property("confidentialityIndicator", "CodeableConcept", 1315 "To indicate if this proces is commercially confidential.", 0, 1, confidentialityIndicator); 1316 case -1969347631: 1317 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 1318 "The manufacturer or establishment associated with the process.", 0, java.lang.Integer.MAX_VALUE, 1319 manufacturer); 1320 case 414760449: 1321 /* regulator */ return new Property("regulator", "Reference(Organization)", 1322 "A regulator which oversees the operation.", 0, 1, regulator); 1323 default: 1324 return super.getNamedProperty(_hash, _name, _checkValid); 1325 } 1326 1327 } 1328 1329 @Override 1330 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1331 switch (hash) { 1332 case 91999553: 1333 /* operationType */ return this.operationType == null ? new Base[0] : new Base[] { this.operationType }; // CodeableConcept 1334 case -1940839884: 1335 /* authorisationReferenceNumber */ return this.authorisationReferenceNumber == null ? new Base[0] 1336 : new Base[] { this.authorisationReferenceNumber }; // Identifier 1337 case -930389515: 1338 /* effectiveDate */ return this.effectiveDate == null ? new Base[0] : new Base[] { this.effectiveDate }; // DateTimeType 1339 case -1449404791: 1340 /* confidentialityIndicator */ return this.confidentialityIndicator == null ? new Base[0] 1341 : new Base[] { this.confidentialityIndicator }; // CodeableConcept 1342 case -1969347631: 1343 /* manufacturer */ return this.manufacturer == null ? new Base[0] 1344 : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 1345 case 414760449: 1346 /* regulator */ return this.regulator == null ? new Base[0] : new Base[] { this.regulator }; // Reference 1347 default: 1348 return super.getProperty(hash, name, checkValid); 1349 } 1350 1351 } 1352 1353 @Override 1354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1355 switch (hash) { 1356 case 91999553: // operationType 1357 this.operationType = castToCodeableConcept(value); // CodeableConcept 1358 return value; 1359 case -1940839884: // authorisationReferenceNumber 1360 this.authorisationReferenceNumber = castToIdentifier(value); // Identifier 1361 return value; 1362 case -930389515: // effectiveDate 1363 this.effectiveDate = castToDateTime(value); // DateTimeType 1364 return value; 1365 case -1449404791: // confidentialityIndicator 1366 this.confidentialityIndicator = castToCodeableConcept(value); // CodeableConcept 1367 return value; 1368 case -1969347631: // manufacturer 1369 this.getManufacturer().add(castToReference(value)); // Reference 1370 return value; 1371 case 414760449: // regulator 1372 this.regulator = castToReference(value); // Reference 1373 return value; 1374 default: 1375 return super.setProperty(hash, name, value); 1376 } 1377 1378 } 1379 1380 @Override 1381 public Base setProperty(String name, Base value) throws FHIRException { 1382 if (name.equals("operationType")) { 1383 this.operationType = castToCodeableConcept(value); // CodeableConcept 1384 } else if (name.equals("authorisationReferenceNumber")) { 1385 this.authorisationReferenceNumber = castToIdentifier(value); // Identifier 1386 } else if (name.equals("effectiveDate")) { 1387 this.effectiveDate = castToDateTime(value); // DateTimeType 1388 } else if (name.equals("confidentialityIndicator")) { 1389 this.confidentialityIndicator = castToCodeableConcept(value); // CodeableConcept 1390 } else if (name.equals("manufacturer")) { 1391 this.getManufacturer().add(castToReference(value)); 1392 } else if (name.equals("regulator")) { 1393 this.regulator = castToReference(value); // Reference 1394 } else 1395 return super.setProperty(name, value); 1396 return value; 1397 } 1398 1399 @Override 1400 public Base makeProperty(int hash, String name) throws FHIRException { 1401 switch (hash) { 1402 case 91999553: 1403 return getOperationType(); 1404 case -1940839884: 1405 return getAuthorisationReferenceNumber(); 1406 case -930389515: 1407 return getEffectiveDateElement(); 1408 case -1449404791: 1409 return getConfidentialityIndicator(); 1410 case -1969347631: 1411 return addManufacturer(); 1412 case 414760449: 1413 return getRegulator(); 1414 default: 1415 return super.makeProperty(hash, name); 1416 } 1417 1418 } 1419 1420 @Override 1421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1422 switch (hash) { 1423 case 91999553: 1424 /* operationType */ return new String[] { "CodeableConcept" }; 1425 case -1940839884: 1426 /* authorisationReferenceNumber */ return new String[] { "Identifier" }; 1427 case -930389515: 1428 /* effectiveDate */ return new String[] { "dateTime" }; 1429 case -1449404791: 1430 /* confidentialityIndicator */ return new String[] { "CodeableConcept" }; 1431 case -1969347631: 1432 /* manufacturer */ return new String[] { "Reference" }; 1433 case 414760449: 1434 /* regulator */ return new String[] { "Reference" }; 1435 default: 1436 return super.getTypesForProperty(hash, name); 1437 } 1438 1439 } 1440 1441 @Override 1442 public Base addChild(String name) throws FHIRException { 1443 if (name.equals("operationType")) { 1444 this.operationType = new CodeableConcept(); 1445 return this.operationType; 1446 } else if (name.equals("authorisationReferenceNumber")) { 1447 this.authorisationReferenceNumber = new Identifier(); 1448 return this.authorisationReferenceNumber; 1449 } else if (name.equals("effectiveDate")) { 1450 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProduct.effectiveDate"); 1451 } else if (name.equals("confidentialityIndicator")) { 1452 this.confidentialityIndicator = new CodeableConcept(); 1453 return this.confidentialityIndicator; 1454 } else if (name.equals("manufacturer")) { 1455 return addManufacturer(); 1456 } else if (name.equals("regulator")) { 1457 this.regulator = new Reference(); 1458 return this.regulator; 1459 } else 1460 return super.addChild(name); 1461 } 1462 1463 public MedicinalProductManufacturingBusinessOperationComponent copy() { 1464 MedicinalProductManufacturingBusinessOperationComponent dst = new MedicinalProductManufacturingBusinessOperationComponent(); 1465 copyValues(dst); 1466 return dst; 1467 } 1468 1469 public void copyValues(MedicinalProductManufacturingBusinessOperationComponent dst) { 1470 super.copyValues(dst); 1471 dst.operationType = operationType == null ? null : operationType.copy(); 1472 dst.authorisationReferenceNumber = authorisationReferenceNumber == null ? null 1473 : authorisationReferenceNumber.copy(); 1474 dst.effectiveDate = effectiveDate == null ? null : effectiveDate.copy(); 1475 dst.confidentialityIndicator = confidentialityIndicator == null ? null : confidentialityIndicator.copy(); 1476 if (manufacturer != null) { 1477 dst.manufacturer = new ArrayList<Reference>(); 1478 for (Reference i : manufacturer) 1479 dst.manufacturer.add(i.copy()); 1480 } 1481 ; 1482 dst.regulator = regulator == null ? null : regulator.copy(); 1483 } 1484 1485 @Override 1486 public boolean equalsDeep(Base other_) { 1487 if (!super.equalsDeep(other_)) 1488 return false; 1489 if (!(other_ instanceof MedicinalProductManufacturingBusinessOperationComponent)) 1490 return false; 1491 MedicinalProductManufacturingBusinessOperationComponent o = (MedicinalProductManufacturingBusinessOperationComponent) other_; 1492 return compareDeep(operationType, o.operationType, true) 1493 && compareDeep(authorisationReferenceNumber, o.authorisationReferenceNumber, true) 1494 && compareDeep(effectiveDate, o.effectiveDate, true) 1495 && compareDeep(confidentialityIndicator, o.confidentialityIndicator, true) 1496 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(regulator, o.regulator, true); 1497 } 1498 1499 @Override 1500 public boolean equalsShallow(Base other_) { 1501 if (!super.equalsShallow(other_)) 1502 return false; 1503 if (!(other_ instanceof MedicinalProductManufacturingBusinessOperationComponent)) 1504 return false; 1505 MedicinalProductManufacturingBusinessOperationComponent o = (MedicinalProductManufacturingBusinessOperationComponent) other_; 1506 return compareValues(effectiveDate, o.effectiveDate, true); 1507 } 1508 1509 public boolean isEmpty() { 1510 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(operationType, authorisationReferenceNumber, 1511 effectiveDate, confidentialityIndicator, manufacturer, regulator); 1512 } 1513 1514 public String fhirType() { 1515 return "MedicinalProduct.manufacturingBusinessOperation"; 1516 1517 } 1518 1519 } 1520 1521 @Block() 1522 public static class MedicinalProductSpecialDesignationComponent extends BackboneElement 1523 implements IBaseBackboneElement { 1524 /** 1525 * Identifier for the designation, or procedure number. 1526 */ 1527 @Child(name = "identifier", type = { 1528 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1529 @Description(shortDefinition = "Identifier for the designation, or procedure number", formalDefinition = "Identifier for the designation, or procedure number.") 1530 protected List<Identifier> identifier; 1531 1532 /** 1533 * The type of special designation, e.g. orphan drug, minor use. 1534 */ 1535 @Child(name = "type", type = { 1536 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1537 @Description(shortDefinition = "The type of special designation, e.g. orphan drug, minor use", formalDefinition = "The type of special designation, e.g. orphan drug, minor use.") 1538 protected CodeableConcept type; 1539 1540 /** 1541 * The intended use of the product, e.g. prevention, treatment. 1542 */ 1543 @Child(name = "intendedUse", type = { 1544 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1545 @Description(shortDefinition = "The intended use of the product, e.g. prevention, treatment", formalDefinition = "The intended use of the product, e.g. prevention, treatment.") 1546 protected CodeableConcept intendedUse; 1547 1548 /** 1549 * Condition for which the medicinal use applies. 1550 */ 1551 @Child(name = "indication", type = { CodeableConcept.class, 1552 MedicinalProductIndication.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1553 @Description(shortDefinition = "Condition for which the medicinal use applies", formalDefinition = "Condition for which the medicinal use applies.") 1554 protected Type indication; 1555 1556 /** 1557 * For example granted, pending, expired or withdrawn. 1558 */ 1559 @Child(name = "status", type = { 1560 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1561 @Description(shortDefinition = "For example granted, pending, expired or withdrawn", formalDefinition = "For example granted, pending, expired or withdrawn.") 1562 protected CodeableConcept status; 1563 1564 /** 1565 * Date when the designation was granted. 1566 */ 1567 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1568 @Description(shortDefinition = "Date when the designation was granted", formalDefinition = "Date when the designation was granted.") 1569 protected DateTimeType date; 1570 1571 /** 1572 * Animal species for which this applies. 1573 */ 1574 @Child(name = "species", type = { 1575 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1576 @Description(shortDefinition = "Animal species for which this applies", formalDefinition = "Animal species for which this applies.") 1577 protected CodeableConcept species; 1578 1579 private static final long serialVersionUID = -1316809207L; 1580 1581 /** 1582 * Constructor 1583 */ 1584 public MedicinalProductSpecialDesignationComponent() { 1585 super(); 1586 } 1587 1588 /** 1589 * @return {@link #identifier} (Identifier for the designation, or procedure 1590 * number.) 1591 */ 1592 public List<Identifier> getIdentifier() { 1593 if (this.identifier == null) 1594 this.identifier = new ArrayList<Identifier>(); 1595 return this.identifier; 1596 } 1597 1598 /** 1599 * @return Returns a reference to <code>this</code> for easy method chaining 1600 */ 1601 public MedicinalProductSpecialDesignationComponent setIdentifier(List<Identifier> theIdentifier) { 1602 this.identifier = theIdentifier; 1603 return this; 1604 } 1605 1606 public boolean hasIdentifier() { 1607 if (this.identifier == null) 1608 return false; 1609 for (Identifier item : this.identifier) 1610 if (!item.isEmpty()) 1611 return true; 1612 return false; 1613 } 1614 1615 public Identifier addIdentifier() { // 3 1616 Identifier t = new Identifier(); 1617 if (this.identifier == null) 1618 this.identifier = new ArrayList<Identifier>(); 1619 this.identifier.add(t); 1620 return t; 1621 } 1622 1623 public MedicinalProductSpecialDesignationComponent addIdentifier(Identifier t) { // 3 1624 if (t == null) 1625 return this; 1626 if (this.identifier == null) 1627 this.identifier = new ArrayList<Identifier>(); 1628 this.identifier.add(t); 1629 return this; 1630 } 1631 1632 /** 1633 * @return The first repetition of repeating field {@link #identifier}, creating 1634 * it if it does not already exist 1635 */ 1636 public Identifier getIdentifierFirstRep() { 1637 if (getIdentifier().isEmpty()) { 1638 addIdentifier(); 1639 } 1640 return getIdentifier().get(0); 1641 } 1642 1643 /** 1644 * @return {@link #type} (The type of special designation, e.g. orphan drug, 1645 * minor use.) 1646 */ 1647 public CodeableConcept getType() { 1648 if (this.type == null) 1649 if (Configuration.errorOnAutoCreate()) 1650 throw new Error("Attempt to auto-create MedicinalProductSpecialDesignationComponent.type"); 1651 else if (Configuration.doAutoCreate()) 1652 this.type = new CodeableConcept(); // cc 1653 return this.type; 1654 } 1655 1656 public boolean hasType() { 1657 return this.type != null && !this.type.isEmpty(); 1658 } 1659 1660 /** 1661 * @param value {@link #type} (The type of special designation, e.g. orphan 1662 * drug, minor use.) 1663 */ 1664 public MedicinalProductSpecialDesignationComponent setType(CodeableConcept value) { 1665 this.type = value; 1666 return this; 1667 } 1668 1669 /** 1670 * @return {@link #intendedUse} (The intended use of the product, e.g. 1671 * prevention, treatment.) 1672 */ 1673 public CodeableConcept getIntendedUse() { 1674 if (this.intendedUse == null) 1675 if (Configuration.errorOnAutoCreate()) 1676 throw new Error("Attempt to auto-create MedicinalProductSpecialDesignationComponent.intendedUse"); 1677 else if (Configuration.doAutoCreate()) 1678 this.intendedUse = new CodeableConcept(); // cc 1679 return this.intendedUse; 1680 } 1681 1682 public boolean hasIntendedUse() { 1683 return this.intendedUse != null && !this.intendedUse.isEmpty(); 1684 } 1685 1686 /** 1687 * @param value {@link #intendedUse} (The intended use of the product, e.g. 1688 * prevention, treatment.) 1689 */ 1690 public MedicinalProductSpecialDesignationComponent setIntendedUse(CodeableConcept value) { 1691 this.intendedUse = value; 1692 return this; 1693 } 1694 1695 /** 1696 * @return {@link #indication} (Condition for which the medicinal use applies.) 1697 */ 1698 public Type getIndication() { 1699 return this.indication; 1700 } 1701 1702 /** 1703 * @return {@link #indication} (Condition for which the medicinal use applies.) 1704 */ 1705 public CodeableConcept getIndicationCodeableConcept() throws FHIRException { 1706 if (this.indication == null) 1707 this.indication = new CodeableConcept(); 1708 if (!(this.indication instanceof CodeableConcept)) 1709 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1710 + this.indication.getClass().getName() + " was encountered"); 1711 return (CodeableConcept) this.indication; 1712 } 1713 1714 public boolean hasIndicationCodeableConcept() { 1715 return this != null && this.indication instanceof CodeableConcept; 1716 } 1717 1718 /** 1719 * @return {@link #indication} (Condition for which the medicinal use applies.) 1720 */ 1721 public Reference getIndicationReference() throws FHIRException { 1722 if (this.indication == null) 1723 this.indication = new Reference(); 1724 if (!(this.indication instanceof Reference)) 1725 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1726 + this.indication.getClass().getName() + " was encountered"); 1727 return (Reference) this.indication; 1728 } 1729 1730 public boolean hasIndicationReference() { 1731 return this != null && this.indication instanceof Reference; 1732 } 1733 1734 public boolean hasIndication() { 1735 return this.indication != null && !this.indication.isEmpty(); 1736 } 1737 1738 /** 1739 * @param value {@link #indication} (Condition for which the medicinal use 1740 * applies.) 1741 */ 1742 public MedicinalProductSpecialDesignationComponent setIndication(Type value) { 1743 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1744 throw new Error( 1745 "Not the right type for MedicinalProduct.specialDesignation.indication[x]: " + value.fhirType()); 1746 this.indication = value; 1747 return this; 1748 } 1749 1750 /** 1751 * @return {@link #status} (For example granted, pending, expired or withdrawn.) 1752 */ 1753 public CodeableConcept getStatus() { 1754 if (this.status == null) 1755 if (Configuration.errorOnAutoCreate()) 1756 throw new Error("Attempt to auto-create MedicinalProductSpecialDesignationComponent.status"); 1757 else if (Configuration.doAutoCreate()) 1758 this.status = new CodeableConcept(); // cc 1759 return this.status; 1760 } 1761 1762 public boolean hasStatus() { 1763 return this.status != null && !this.status.isEmpty(); 1764 } 1765 1766 /** 1767 * @param value {@link #status} (For example granted, pending, expired or 1768 * withdrawn.) 1769 */ 1770 public MedicinalProductSpecialDesignationComponent setStatus(CodeableConcept value) { 1771 this.status = value; 1772 return this; 1773 } 1774 1775 /** 1776 * @return {@link #date} (Date when the designation was granted.). This is the 1777 * underlying object with id, value and extensions. The accessor 1778 * "getDate" gives direct access to the value 1779 */ 1780 public DateTimeType getDateElement() { 1781 if (this.date == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create MedicinalProductSpecialDesignationComponent.date"); 1784 else if (Configuration.doAutoCreate()) 1785 this.date = new DateTimeType(); // bb 1786 return this.date; 1787 } 1788 1789 public boolean hasDateElement() { 1790 return this.date != null && !this.date.isEmpty(); 1791 } 1792 1793 public boolean hasDate() { 1794 return this.date != null && !this.date.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #date} (Date when the designation was granted.). This is 1799 * the underlying object with id, value and extensions. The 1800 * accessor "getDate" gives direct access to the value 1801 */ 1802 public MedicinalProductSpecialDesignationComponent setDateElement(DateTimeType value) { 1803 this.date = value; 1804 return this; 1805 } 1806 1807 /** 1808 * @return Date when the designation was granted. 1809 */ 1810 public Date getDate() { 1811 return this.date == null ? null : this.date.getValue(); 1812 } 1813 1814 /** 1815 * @param value Date when the designation was granted. 1816 */ 1817 public MedicinalProductSpecialDesignationComponent setDate(Date value) { 1818 if (value == null) 1819 this.date = null; 1820 else { 1821 if (this.date == null) 1822 this.date = new DateTimeType(); 1823 this.date.setValue(value); 1824 } 1825 return this; 1826 } 1827 1828 /** 1829 * @return {@link #species} (Animal species for which this applies.) 1830 */ 1831 public CodeableConcept getSpecies() { 1832 if (this.species == null) 1833 if (Configuration.errorOnAutoCreate()) 1834 throw new Error("Attempt to auto-create MedicinalProductSpecialDesignationComponent.species"); 1835 else if (Configuration.doAutoCreate()) 1836 this.species = new CodeableConcept(); // cc 1837 return this.species; 1838 } 1839 1840 public boolean hasSpecies() { 1841 return this.species != null && !this.species.isEmpty(); 1842 } 1843 1844 /** 1845 * @param value {@link #species} (Animal species for which this applies.) 1846 */ 1847 public MedicinalProductSpecialDesignationComponent setSpecies(CodeableConcept value) { 1848 this.species = value; 1849 return this; 1850 } 1851 1852 protected void listChildren(List<Property> children) { 1853 super.listChildren(children); 1854 children.add(new Property("identifier", "Identifier", "Identifier for the designation, or procedure number.", 0, 1855 java.lang.Integer.MAX_VALUE, identifier)); 1856 children.add(new Property("type", "CodeableConcept", 1857 "The type of special designation, e.g. orphan drug, minor use.", 0, 1, type)); 1858 children.add(new Property("intendedUse", "CodeableConcept", 1859 "The intended use of the product, e.g. prevention, treatment.", 0, 1, intendedUse)); 1860 children.add(new Property("indication[x]", "CodeableConcept|Reference(MedicinalProductIndication)", 1861 "Condition for which the medicinal use applies.", 0, 1, indication)); 1862 children.add(new Property("status", "CodeableConcept", "For example granted, pending, expired or withdrawn.", 0, 1863 1, status)); 1864 children.add(new Property("date", "dateTime", "Date when the designation was granted.", 0, 1, date)); 1865 children.add(new Property("species", "CodeableConcept", "Animal species for which this applies.", 0, 1, species)); 1866 } 1867 1868 @Override 1869 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1870 switch (_hash) { 1871 case -1618432855: 1872 /* identifier */ return new Property("identifier", "Identifier", 1873 "Identifier for the designation, or procedure number.", 0, java.lang.Integer.MAX_VALUE, identifier); 1874 case 3575610: 1875 /* type */ return new Property("type", "CodeableConcept", 1876 "The type of special designation, e.g. orphan drug, minor use.", 0, 1, type); 1877 case -1618671268: 1878 /* intendedUse */ return new Property("intendedUse", "CodeableConcept", 1879 "The intended use of the product, e.g. prevention, treatment.", 0, 1, intendedUse); 1880 case -501208668: 1881 /* indication[x] */ return new Property("indication[x]", 1882 "CodeableConcept|Reference(MedicinalProductIndication)", "Condition for which the medicinal use applies.", 1883 0, 1, indication); 1884 case -597168804: 1885 /* indication */ return new Property("indication[x]", "CodeableConcept|Reference(MedicinalProductIndication)", 1886 "Condition for which the medicinal use applies.", 0, 1, indication); 1887 case -1094003035: 1888 /* indicationCodeableConcept */ return new Property("indication[x]", 1889 "CodeableConcept|Reference(MedicinalProductIndication)", "Condition for which the medicinal use applies.", 1890 0, 1, indication); 1891 case 803518799: 1892 /* indicationReference */ return new Property("indication[x]", 1893 "CodeableConcept|Reference(MedicinalProductIndication)", "Condition for which the medicinal use applies.", 1894 0, 1, indication); 1895 case -892481550: 1896 /* status */ return new Property("status", "CodeableConcept", 1897 "For example granted, pending, expired or withdrawn.", 0, 1, status); 1898 case 3076014: 1899 /* date */ return new Property("date", "dateTime", "Date when the designation was granted.", 0, 1, date); 1900 case -2008465092: 1901 /* species */ return new Property("species", "CodeableConcept", "Animal species for which this applies.", 0, 1, 1902 species); 1903 default: 1904 return super.getNamedProperty(_hash, _name, _checkValid); 1905 } 1906 1907 } 1908 1909 @Override 1910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1911 switch (hash) { 1912 case -1618432855: 1913 /* identifier */ return this.identifier == null ? new Base[0] 1914 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1915 case 3575610: 1916 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1917 case -1618671268: 1918 /* intendedUse */ return this.intendedUse == null ? new Base[0] : new Base[] { this.intendedUse }; // CodeableConcept 1919 case -597168804: 1920 /* indication */ return this.indication == null ? new Base[0] : new Base[] { this.indication }; // Type 1921 case -892481550: 1922 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 1923 case 3076014: 1924 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1925 case -2008465092: 1926 /* species */ return this.species == null ? new Base[0] : new Base[] { this.species }; // CodeableConcept 1927 default: 1928 return super.getProperty(hash, name, checkValid); 1929 } 1930 1931 } 1932 1933 @Override 1934 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1935 switch (hash) { 1936 case -1618432855: // identifier 1937 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1938 return value; 1939 case 3575610: // type 1940 this.type = castToCodeableConcept(value); // CodeableConcept 1941 return value; 1942 case -1618671268: // intendedUse 1943 this.intendedUse = castToCodeableConcept(value); // CodeableConcept 1944 return value; 1945 case -597168804: // indication 1946 this.indication = castToType(value); // Type 1947 return value; 1948 case -892481550: // status 1949 this.status = castToCodeableConcept(value); // CodeableConcept 1950 return value; 1951 case 3076014: // date 1952 this.date = castToDateTime(value); // DateTimeType 1953 return value; 1954 case -2008465092: // species 1955 this.species = castToCodeableConcept(value); // CodeableConcept 1956 return value; 1957 default: 1958 return super.setProperty(hash, name, value); 1959 } 1960 1961 } 1962 1963 @Override 1964 public Base setProperty(String name, Base value) throws FHIRException { 1965 if (name.equals("identifier")) { 1966 this.getIdentifier().add(castToIdentifier(value)); 1967 } else if (name.equals("type")) { 1968 this.type = castToCodeableConcept(value); // CodeableConcept 1969 } else if (name.equals("intendedUse")) { 1970 this.intendedUse = castToCodeableConcept(value); // CodeableConcept 1971 } else if (name.equals("indication[x]")) { 1972 this.indication = castToType(value); // Type 1973 } else if (name.equals("status")) { 1974 this.status = castToCodeableConcept(value); // CodeableConcept 1975 } else if (name.equals("date")) { 1976 this.date = castToDateTime(value); // DateTimeType 1977 } else if (name.equals("species")) { 1978 this.species = castToCodeableConcept(value); // CodeableConcept 1979 } else 1980 return super.setProperty(name, value); 1981 return value; 1982 } 1983 1984 @Override 1985 public Base makeProperty(int hash, String name) throws FHIRException { 1986 switch (hash) { 1987 case -1618432855: 1988 return addIdentifier(); 1989 case 3575610: 1990 return getType(); 1991 case -1618671268: 1992 return getIntendedUse(); 1993 case -501208668: 1994 return getIndication(); 1995 case -597168804: 1996 return getIndication(); 1997 case -892481550: 1998 return getStatus(); 1999 case 3076014: 2000 return getDateElement(); 2001 case -2008465092: 2002 return getSpecies(); 2003 default: 2004 return super.makeProperty(hash, name); 2005 } 2006 2007 } 2008 2009 @Override 2010 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2011 switch (hash) { 2012 case -1618432855: 2013 /* identifier */ return new String[] { "Identifier" }; 2014 case 3575610: 2015 /* type */ return new String[] { "CodeableConcept" }; 2016 case -1618671268: 2017 /* intendedUse */ return new String[] { "CodeableConcept" }; 2018 case -597168804: 2019 /* indication */ return new String[] { "CodeableConcept", "Reference" }; 2020 case -892481550: 2021 /* status */ return new String[] { "CodeableConcept" }; 2022 case 3076014: 2023 /* date */ return new String[] { "dateTime" }; 2024 case -2008465092: 2025 /* species */ return new String[] { "CodeableConcept" }; 2026 default: 2027 return super.getTypesForProperty(hash, name); 2028 } 2029 2030 } 2031 2032 @Override 2033 public Base addChild(String name) throws FHIRException { 2034 if (name.equals("identifier")) { 2035 return addIdentifier(); 2036 } else if (name.equals("type")) { 2037 this.type = new CodeableConcept(); 2038 return this.type; 2039 } else if (name.equals("intendedUse")) { 2040 this.intendedUse = new CodeableConcept(); 2041 return this.intendedUse; 2042 } else if (name.equals("indicationCodeableConcept")) { 2043 this.indication = new CodeableConcept(); 2044 return this.indication; 2045 } else if (name.equals("indicationReference")) { 2046 this.indication = new Reference(); 2047 return this.indication; 2048 } else if (name.equals("status")) { 2049 this.status = new CodeableConcept(); 2050 return this.status; 2051 } else if (name.equals("date")) { 2052 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProduct.date"); 2053 } else if (name.equals("species")) { 2054 this.species = new CodeableConcept(); 2055 return this.species; 2056 } else 2057 return super.addChild(name); 2058 } 2059 2060 public MedicinalProductSpecialDesignationComponent copy() { 2061 MedicinalProductSpecialDesignationComponent dst = new MedicinalProductSpecialDesignationComponent(); 2062 copyValues(dst); 2063 return dst; 2064 } 2065 2066 public void copyValues(MedicinalProductSpecialDesignationComponent dst) { 2067 super.copyValues(dst); 2068 if (identifier != null) { 2069 dst.identifier = new ArrayList<Identifier>(); 2070 for (Identifier i : identifier) 2071 dst.identifier.add(i.copy()); 2072 } 2073 ; 2074 dst.type = type == null ? null : type.copy(); 2075 dst.intendedUse = intendedUse == null ? null : intendedUse.copy(); 2076 dst.indication = indication == null ? null : indication.copy(); 2077 dst.status = status == null ? null : status.copy(); 2078 dst.date = date == null ? null : date.copy(); 2079 dst.species = species == null ? null : species.copy(); 2080 } 2081 2082 @Override 2083 public boolean equalsDeep(Base other_) { 2084 if (!super.equalsDeep(other_)) 2085 return false; 2086 if (!(other_ instanceof MedicinalProductSpecialDesignationComponent)) 2087 return false; 2088 MedicinalProductSpecialDesignationComponent o = (MedicinalProductSpecialDesignationComponent) other_; 2089 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 2090 && compareDeep(intendedUse, o.intendedUse, true) && compareDeep(indication, o.indication, true) 2091 && compareDeep(status, o.status, true) && compareDeep(date, o.date, true) 2092 && compareDeep(species, o.species, true); 2093 } 2094 2095 @Override 2096 public boolean equalsShallow(Base other_) { 2097 if (!super.equalsShallow(other_)) 2098 return false; 2099 if (!(other_ instanceof MedicinalProductSpecialDesignationComponent)) 2100 return false; 2101 MedicinalProductSpecialDesignationComponent o = (MedicinalProductSpecialDesignationComponent) other_; 2102 return compareValues(date, o.date, true); 2103 } 2104 2105 public boolean isEmpty() { 2106 return super.isEmpty() 2107 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, intendedUse, indication, status, date, species); 2108 } 2109 2110 public String fhirType() { 2111 return "MedicinalProduct.specialDesignation"; 2112 2113 } 2114 2115 } 2116 2117 /** 2118 * Business identifier for this product. Could be an MPID. 2119 */ 2120 @Child(name = "identifier", type = { 2121 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2122 @Description(shortDefinition = "Business identifier for this product. Could be an MPID", formalDefinition = "Business identifier for this product. Could be an MPID.") 2123 protected List<Identifier> identifier; 2124 2125 /** 2126 * Regulatory type, e.g. Investigational or Authorized. 2127 */ 2128 @Child(name = "type", type = { CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2129 @Description(shortDefinition = "Regulatory type, e.g. Investigational or Authorized", formalDefinition = "Regulatory type, e.g. Investigational or Authorized.") 2130 protected CodeableConcept type; 2131 2132 /** 2133 * If this medicine applies to human or veterinary uses. 2134 */ 2135 @Child(name = "domain", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2136 @Description(shortDefinition = "If this medicine applies to human or veterinary uses", formalDefinition = "If this medicine applies to human or veterinary uses.") 2137 protected Coding domain; 2138 2139 /** 2140 * The dose form for a single part product, or combined form of a multiple part 2141 * product. 2142 */ 2143 @Child(name = "combinedPharmaceuticalDoseForm", type = { 2144 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2145 @Description(shortDefinition = "The dose form for a single part product, or combined form of a multiple part product", formalDefinition = "The dose form for a single part product, or combined form of a multiple part product.") 2146 protected CodeableConcept combinedPharmaceuticalDoseForm; 2147 2148 /** 2149 * The legal status of supply of the medicinal product as classified by the 2150 * regulator. 2151 */ 2152 @Child(name = "legalStatusOfSupply", type = { 2153 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2154 @Description(shortDefinition = "The legal status of supply of the medicinal product as classified by the regulator", formalDefinition = "The legal status of supply of the medicinal product as classified by the regulator.") 2155 protected CodeableConcept legalStatusOfSupply; 2156 2157 /** 2158 * Whether the Medicinal Product is subject to additional monitoring for 2159 * regulatory reasons. 2160 */ 2161 @Child(name = "additionalMonitoringIndicator", type = { 2162 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2163 @Description(shortDefinition = "Whether the Medicinal Product is subject to additional monitoring for regulatory reasons", formalDefinition = "Whether the Medicinal Product is subject to additional monitoring for regulatory reasons.") 2164 protected CodeableConcept additionalMonitoringIndicator; 2165 2166 /** 2167 * Whether the Medicinal Product is subject to special measures for regulatory 2168 * reasons. 2169 */ 2170 @Child(name = "specialMeasures", type = { 2171 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2172 @Description(shortDefinition = "Whether the Medicinal Product is subject to special measures for regulatory reasons", formalDefinition = "Whether the Medicinal Product is subject to special measures for regulatory reasons.") 2173 protected List<StringType> specialMeasures; 2174 2175 /** 2176 * If authorised for use in children. 2177 */ 2178 @Child(name = "paediatricUseIndicator", type = { 2179 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2180 @Description(shortDefinition = "If authorised for use in children", formalDefinition = "If authorised for use in children.") 2181 protected CodeableConcept paediatricUseIndicator; 2182 2183 /** 2184 * Allows the product to be classified by various systems. 2185 */ 2186 @Child(name = "productClassification", type = { 2187 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2188 @Description(shortDefinition = "Allows the product to be classified by various systems", formalDefinition = "Allows the product to be classified by various systems.") 2189 protected List<CodeableConcept> productClassification; 2190 2191 /** 2192 * Marketing status of the medicinal product, in contrast to marketing 2193 * authorizaton. 2194 */ 2195 @Child(name = "marketingStatus", type = { 2196 MarketingStatus.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2197 @Description(shortDefinition = "Marketing status of the medicinal product, in contrast to marketing authorizaton", formalDefinition = "Marketing status of the medicinal product, in contrast to marketing authorizaton.") 2198 protected List<MarketingStatus> marketingStatus; 2199 2200 /** 2201 * Pharmaceutical aspects of product. 2202 */ 2203 @Child(name = "pharmaceuticalProduct", type = { 2204 MedicinalProductPharmaceutical.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2205 @Description(shortDefinition = "Pharmaceutical aspects of product", formalDefinition = "Pharmaceutical aspects of product.") 2206 protected List<Reference> pharmaceuticalProduct; 2207 /** 2208 * The actual objects that are the target of the reference (Pharmaceutical 2209 * aspects of product.) 2210 */ 2211 protected List<MedicinalProductPharmaceutical> pharmaceuticalProductTarget; 2212 2213 /** 2214 * Package representation for the product. 2215 */ 2216 @Child(name = "packagedMedicinalProduct", type = { 2217 MedicinalProductPackaged.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2218 @Description(shortDefinition = "Package representation for the product", formalDefinition = "Package representation for the product.") 2219 protected List<Reference> packagedMedicinalProduct; 2220 /** 2221 * The actual objects that are the target of the reference (Package 2222 * representation for the product.) 2223 */ 2224 protected List<MedicinalProductPackaged> packagedMedicinalProductTarget; 2225 2226 /** 2227 * Supporting documentation, typically for regulatory submission. 2228 */ 2229 @Child(name = "attachedDocument", type = { 2230 DocumentReference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2231 @Description(shortDefinition = "Supporting documentation, typically for regulatory submission", formalDefinition = "Supporting documentation, typically for regulatory submission.") 2232 protected List<Reference> attachedDocument; 2233 /** 2234 * The actual objects that are the target of the reference (Supporting 2235 * documentation, typically for regulatory submission.) 2236 */ 2237 protected List<DocumentReference> attachedDocumentTarget; 2238 2239 /** 2240 * A master file for to the medicinal product (e.g. Pharmacovigilance System 2241 * Master File). 2242 */ 2243 @Child(name = "masterFile", type = { 2244 DocumentReference.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2245 @Description(shortDefinition = "A master file for to the medicinal product (e.g. Pharmacovigilance System Master File)", formalDefinition = "A master file for to the medicinal product (e.g. Pharmacovigilance System Master File).") 2246 protected List<Reference> masterFile; 2247 /** 2248 * The actual objects that are the target of the reference (A master file for to 2249 * the medicinal product (e.g. Pharmacovigilance System Master File).) 2250 */ 2251 protected List<DocumentReference> masterFileTarget; 2252 2253 /** 2254 * A product specific contact, person (in a role), or an organization. 2255 */ 2256 @Child(name = "contact", type = { Organization.class, 2257 PractitionerRole.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2258 @Description(shortDefinition = "A product specific contact, person (in a role), or an organization", formalDefinition = "A product specific contact, person (in a role), or an organization.") 2259 protected List<Reference> contact; 2260 /** 2261 * The actual objects that are the target of the reference (A product specific 2262 * contact, person (in a role), or an organization.) 2263 */ 2264 protected List<Resource> contactTarget; 2265 2266 /** 2267 * Clinical trials or studies that this product is involved in. 2268 */ 2269 @Child(name = "clinicalTrial", type = { 2270 ResearchStudy.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2271 @Description(shortDefinition = "Clinical trials or studies that this product is involved in", formalDefinition = "Clinical trials or studies that this product is involved in.") 2272 protected List<Reference> clinicalTrial; 2273 /** 2274 * The actual objects that are the target of the reference (Clinical trials or 2275 * studies that this product is involved in.) 2276 */ 2277 protected List<ResearchStudy> clinicalTrialTarget; 2278 2279 /** 2280 * The product's name, including full name and possibly coded parts. 2281 */ 2282 @Child(name = "name", type = {}, order = 16, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2283 @Description(shortDefinition = "The product's name, including full name and possibly coded parts", formalDefinition = "The product's name, including full name and possibly coded parts.") 2284 protected List<MedicinalProductNameComponent> name; 2285 2286 /** 2287 * Reference to another product, e.g. for linking authorised to investigational 2288 * product. 2289 */ 2290 @Child(name = "crossReference", type = { 2291 Identifier.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2292 @Description(shortDefinition = "Reference to another product, e.g. for linking authorised to investigational product", formalDefinition = "Reference to another product, e.g. for linking authorised to investigational product.") 2293 protected List<Identifier> crossReference; 2294 2295 /** 2296 * An operation applied to the product, for manufacturing or adminsitrative 2297 * purpose. 2298 */ 2299 @Child(name = "manufacturingBusinessOperation", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2300 @Description(shortDefinition = "An operation applied to the product, for manufacturing or adminsitrative purpose", formalDefinition = "An operation applied to the product, for manufacturing or adminsitrative purpose.") 2301 protected List<MedicinalProductManufacturingBusinessOperationComponent> manufacturingBusinessOperation; 2302 2303 /** 2304 * Indicates if the medicinal product has an orphan designation for the 2305 * treatment of a rare disease. 2306 */ 2307 @Child(name = "specialDesignation", type = {}, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2308 @Description(shortDefinition = "Indicates if the medicinal product has an orphan designation for the treatment of a rare disease", formalDefinition = "Indicates if the medicinal product has an orphan designation for the treatment of a rare disease.") 2309 protected List<MedicinalProductSpecialDesignationComponent> specialDesignation; 2310 2311 private static final long serialVersionUID = -899196111L; 2312 2313 /** 2314 * Constructor 2315 */ 2316 public MedicinalProduct() { 2317 super(); 2318 } 2319 2320 /** 2321 * @return {@link #identifier} (Business identifier for this product. Could be 2322 * an MPID.) 2323 */ 2324 public List<Identifier> getIdentifier() { 2325 if (this.identifier == null) 2326 this.identifier = new ArrayList<Identifier>(); 2327 return this.identifier; 2328 } 2329 2330 /** 2331 * @return Returns a reference to <code>this</code> for easy method chaining 2332 */ 2333 public MedicinalProduct setIdentifier(List<Identifier> theIdentifier) { 2334 this.identifier = theIdentifier; 2335 return this; 2336 } 2337 2338 public boolean hasIdentifier() { 2339 if (this.identifier == null) 2340 return false; 2341 for (Identifier item : this.identifier) 2342 if (!item.isEmpty()) 2343 return true; 2344 return false; 2345 } 2346 2347 public Identifier addIdentifier() { // 3 2348 Identifier t = new Identifier(); 2349 if (this.identifier == null) 2350 this.identifier = new ArrayList<Identifier>(); 2351 this.identifier.add(t); 2352 return t; 2353 } 2354 2355 public MedicinalProduct addIdentifier(Identifier t) { // 3 2356 if (t == null) 2357 return this; 2358 if (this.identifier == null) 2359 this.identifier = new ArrayList<Identifier>(); 2360 this.identifier.add(t); 2361 return this; 2362 } 2363 2364 /** 2365 * @return The first repetition of repeating field {@link #identifier}, creating 2366 * it if it does not already exist 2367 */ 2368 public Identifier getIdentifierFirstRep() { 2369 if (getIdentifier().isEmpty()) { 2370 addIdentifier(); 2371 } 2372 return getIdentifier().get(0); 2373 } 2374 2375 /** 2376 * @return {@link #type} (Regulatory type, e.g. Investigational or Authorized.) 2377 */ 2378 public CodeableConcept getType() { 2379 if (this.type == null) 2380 if (Configuration.errorOnAutoCreate()) 2381 throw new Error("Attempt to auto-create MedicinalProduct.type"); 2382 else if (Configuration.doAutoCreate()) 2383 this.type = new CodeableConcept(); // cc 2384 return this.type; 2385 } 2386 2387 public boolean hasType() { 2388 return this.type != null && !this.type.isEmpty(); 2389 } 2390 2391 /** 2392 * @param value {@link #type} (Regulatory type, e.g. Investigational or 2393 * Authorized.) 2394 */ 2395 public MedicinalProduct setType(CodeableConcept value) { 2396 this.type = value; 2397 return this; 2398 } 2399 2400 /** 2401 * @return {@link #domain} (If this medicine applies to human or veterinary 2402 * uses.) 2403 */ 2404 public Coding getDomain() { 2405 if (this.domain == null) 2406 if (Configuration.errorOnAutoCreate()) 2407 throw new Error("Attempt to auto-create MedicinalProduct.domain"); 2408 else if (Configuration.doAutoCreate()) 2409 this.domain = new Coding(); // cc 2410 return this.domain; 2411 } 2412 2413 public boolean hasDomain() { 2414 return this.domain != null && !this.domain.isEmpty(); 2415 } 2416 2417 /** 2418 * @param value {@link #domain} (If this medicine applies to human or veterinary 2419 * uses.) 2420 */ 2421 public MedicinalProduct setDomain(Coding value) { 2422 this.domain = value; 2423 return this; 2424 } 2425 2426 /** 2427 * @return {@link #combinedPharmaceuticalDoseForm} (The dose form for a single 2428 * part product, or combined form of a multiple part product.) 2429 */ 2430 public CodeableConcept getCombinedPharmaceuticalDoseForm() { 2431 if (this.combinedPharmaceuticalDoseForm == null) 2432 if (Configuration.errorOnAutoCreate()) 2433 throw new Error("Attempt to auto-create MedicinalProduct.combinedPharmaceuticalDoseForm"); 2434 else if (Configuration.doAutoCreate()) 2435 this.combinedPharmaceuticalDoseForm = new CodeableConcept(); // cc 2436 return this.combinedPharmaceuticalDoseForm; 2437 } 2438 2439 public boolean hasCombinedPharmaceuticalDoseForm() { 2440 return this.combinedPharmaceuticalDoseForm != null && !this.combinedPharmaceuticalDoseForm.isEmpty(); 2441 } 2442 2443 /** 2444 * @param value {@link #combinedPharmaceuticalDoseForm} (The dose form for a 2445 * single part product, or combined form of a multiple part 2446 * product.) 2447 */ 2448 public MedicinalProduct setCombinedPharmaceuticalDoseForm(CodeableConcept value) { 2449 this.combinedPharmaceuticalDoseForm = value; 2450 return this; 2451 } 2452 2453 /** 2454 * @return {@link #legalStatusOfSupply} (The legal status of supply of the 2455 * medicinal product as classified by the regulator.) 2456 */ 2457 public CodeableConcept getLegalStatusOfSupply() { 2458 if (this.legalStatusOfSupply == null) 2459 if (Configuration.errorOnAutoCreate()) 2460 throw new Error("Attempt to auto-create MedicinalProduct.legalStatusOfSupply"); 2461 else if (Configuration.doAutoCreate()) 2462 this.legalStatusOfSupply = new CodeableConcept(); // cc 2463 return this.legalStatusOfSupply; 2464 } 2465 2466 public boolean hasLegalStatusOfSupply() { 2467 return this.legalStatusOfSupply != null && !this.legalStatusOfSupply.isEmpty(); 2468 } 2469 2470 /** 2471 * @param value {@link #legalStatusOfSupply} (The legal status of supply of the 2472 * medicinal product as classified by the regulator.) 2473 */ 2474 public MedicinalProduct setLegalStatusOfSupply(CodeableConcept value) { 2475 this.legalStatusOfSupply = value; 2476 return this; 2477 } 2478 2479 /** 2480 * @return {@link #additionalMonitoringIndicator} (Whether the Medicinal Product 2481 * is subject to additional monitoring for regulatory reasons.) 2482 */ 2483 public CodeableConcept getAdditionalMonitoringIndicator() { 2484 if (this.additionalMonitoringIndicator == null) 2485 if (Configuration.errorOnAutoCreate()) 2486 throw new Error("Attempt to auto-create MedicinalProduct.additionalMonitoringIndicator"); 2487 else if (Configuration.doAutoCreate()) 2488 this.additionalMonitoringIndicator = new CodeableConcept(); // cc 2489 return this.additionalMonitoringIndicator; 2490 } 2491 2492 public boolean hasAdditionalMonitoringIndicator() { 2493 return this.additionalMonitoringIndicator != null && !this.additionalMonitoringIndicator.isEmpty(); 2494 } 2495 2496 /** 2497 * @param value {@link #additionalMonitoringIndicator} (Whether the Medicinal 2498 * Product is subject to additional monitoring for regulatory 2499 * reasons.) 2500 */ 2501 public MedicinalProduct setAdditionalMonitoringIndicator(CodeableConcept value) { 2502 this.additionalMonitoringIndicator = value; 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #specialMeasures} (Whether the Medicinal Product is subject to 2508 * special measures for regulatory reasons.) 2509 */ 2510 public List<StringType> getSpecialMeasures() { 2511 if (this.specialMeasures == null) 2512 this.specialMeasures = new ArrayList<StringType>(); 2513 return this.specialMeasures; 2514 } 2515 2516 /** 2517 * @return Returns a reference to <code>this</code> for easy method chaining 2518 */ 2519 public MedicinalProduct setSpecialMeasures(List<StringType> theSpecialMeasures) { 2520 this.specialMeasures = theSpecialMeasures; 2521 return this; 2522 } 2523 2524 public boolean hasSpecialMeasures() { 2525 if (this.specialMeasures == null) 2526 return false; 2527 for (StringType item : this.specialMeasures) 2528 if (!item.isEmpty()) 2529 return true; 2530 return false; 2531 } 2532 2533 /** 2534 * @return {@link #specialMeasures} (Whether the Medicinal Product is subject to 2535 * special measures for regulatory reasons.) 2536 */ 2537 public StringType addSpecialMeasuresElement() {// 2 2538 StringType t = new StringType(); 2539 if (this.specialMeasures == null) 2540 this.specialMeasures = new ArrayList<StringType>(); 2541 this.specialMeasures.add(t); 2542 return t; 2543 } 2544 2545 /** 2546 * @param value {@link #specialMeasures} (Whether the Medicinal Product is 2547 * subject to special measures for regulatory reasons.) 2548 */ 2549 public MedicinalProduct addSpecialMeasures(String value) { // 1 2550 StringType t = new StringType(); 2551 t.setValue(value); 2552 if (this.specialMeasures == null) 2553 this.specialMeasures = new ArrayList<StringType>(); 2554 this.specialMeasures.add(t); 2555 return this; 2556 } 2557 2558 /** 2559 * @param value {@link #specialMeasures} (Whether the Medicinal Product is 2560 * subject to special measures for regulatory reasons.) 2561 */ 2562 public boolean hasSpecialMeasures(String value) { 2563 if (this.specialMeasures == null) 2564 return false; 2565 for (StringType v : this.specialMeasures) 2566 if (v.getValue().equals(value)) // string 2567 return true; 2568 return false; 2569 } 2570 2571 /** 2572 * @return {@link #paediatricUseIndicator} (If authorised for use in children.) 2573 */ 2574 public CodeableConcept getPaediatricUseIndicator() { 2575 if (this.paediatricUseIndicator == null) 2576 if (Configuration.errorOnAutoCreate()) 2577 throw new Error("Attempt to auto-create MedicinalProduct.paediatricUseIndicator"); 2578 else if (Configuration.doAutoCreate()) 2579 this.paediatricUseIndicator = new CodeableConcept(); // cc 2580 return this.paediatricUseIndicator; 2581 } 2582 2583 public boolean hasPaediatricUseIndicator() { 2584 return this.paediatricUseIndicator != null && !this.paediatricUseIndicator.isEmpty(); 2585 } 2586 2587 /** 2588 * @param value {@link #paediatricUseIndicator} (If authorised for use in 2589 * children.) 2590 */ 2591 public MedicinalProduct setPaediatricUseIndicator(CodeableConcept value) { 2592 this.paediatricUseIndicator = value; 2593 return this; 2594 } 2595 2596 /** 2597 * @return {@link #productClassification} (Allows the product to be classified 2598 * by various systems.) 2599 */ 2600 public List<CodeableConcept> getProductClassification() { 2601 if (this.productClassification == null) 2602 this.productClassification = new ArrayList<CodeableConcept>(); 2603 return this.productClassification; 2604 } 2605 2606 /** 2607 * @return Returns a reference to <code>this</code> for easy method chaining 2608 */ 2609 public MedicinalProduct setProductClassification(List<CodeableConcept> theProductClassification) { 2610 this.productClassification = theProductClassification; 2611 return this; 2612 } 2613 2614 public boolean hasProductClassification() { 2615 if (this.productClassification == null) 2616 return false; 2617 for (CodeableConcept item : this.productClassification) 2618 if (!item.isEmpty()) 2619 return true; 2620 return false; 2621 } 2622 2623 public CodeableConcept addProductClassification() { // 3 2624 CodeableConcept t = new CodeableConcept(); 2625 if (this.productClassification == null) 2626 this.productClassification = new ArrayList<CodeableConcept>(); 2627 this.productClassification.add(t); 2628 return t; 2629 } 2630 2631 public MedicinalProduct addProductClassification(CodeableConcept t) { // 3 2632 if (t == null) 2633 return this; 2634 if (this.productClassification == null) 2635 this.productClassification = new ArrayList<CodeableConcept>(); 2636 this.productClassification.add(t); 2637 return this; 2638 } 2639 2640 /** 2641 * @return The first repetition of repeating field 2642 * {@link #productClassification}, creating it if it does not already 2643 * exist 2644 */ 2645 public CodeableConcept getProductClassificationFirstRep() { 2646 if (getProductClassification().isEmpty()) { 2647 addProductClassification(); 2648 } 2649 return getProductClassification().get(0); 2650 } 2651 2652 /** 2653 * @return {@link #marketingStatus} (Marketing status of the medicinal product, 2654 * in contrast to marketing authorizaton.) 2655 */ 2656 public List<MarketingStatus> getMarketingStatus() { 2657 if (this.marketingStatus == null) 2658 this.marketingStatus = new ArrayList<MarketingStatus>(); 2659 return this.marketingStatus; 2660 } 2661 2662 /** 2663 * @return Returns a reference to <code>this</code> for easy method chaining 2664 */ 2665 public MedicinalProduct setMarketingStatus(List<MarketingStatus> theMarketingStatus) { 2666 this.marketingStatus = theMarketingStatus; 2667 return this; 2668 } 2669 2670 public boolean hasMarketingStatus() { 2671 if (this.marketingStatus == null) 2672 return false; 2673 for (MarketingStatus item : this.marketingStatus) 2674 if (!item.isEmpty()) 2675 return true; 2676 return false; 2677 } 2678 2679 public MarketingStatus addMarketingStatus() { // 3 2680 MarketingStatus t = new MarketingStatus(); 2681 if (this.marketingStatus == null) 2682 this.marketingStatus = new ArrayList<MarketingStatus>(); 2683 this.marketingStatus.add(t); 2684 return t; 2685 } 2686 2687 public MedicinalProduct addMarketingStatus(MarketingStatus t) { // 3 2688 if (t == null) 2689 return this; 2690 if (this.marketingStatus == null) 2691 this.marketingStatus = new ArrayList<MarketingStatus>(); 2692 this.marketingStatus.add(t); 2693 return this; 2694 } 2695 2696 /** 2697 * @return The first repetition of repeating field {@link #marketingStatus}, 2698 * creating it if it does not already exist 2699 */ 2700 public MarketingStatus getMarketingStatusFirstRep() { 2701 if (getMarketingStatus().isEmpty()) { 2702 addMarketingStatus(); 2703 } 2704 return getMarketingStatus().get(0); 2705 } 2706 2707 /** 2708 * @return {@link #pharmaceuticalProduct} (Pharmaceutical aspects of product.) 2709 */ 2710 public List<Reference> getPharmaceuticalProduct() { 2711 if (this.pharmaceuticalProduct == null) 2712 this.pharmaceuticalProduct = new ArrayList<Reference>(); 2713 return this.pharmaceuticalProduct; 2714 } 2715 2716 /** 2717 * @return Returns a reference to <code>this</code> for easy method chaining 2718 */ 2719 public MedicinalProduct setPharmaceuticalProduct(List<Reference> thePharmaceuticalProduct) { 2720 this.pharmaceuticalProduct = thePharmaceuticalProduct; 2721 return this; 2722 } 2723 2724 public boolean hasPharmaceuticalProduct() { 2725 if (this.pharmaceuticalProduct == null) 2726 return false; 2727 for (Reference item : this.pharmaceuticalProduct) 2728 if (!item.isEmpty()) 2729 return true; 2730 return false; 2731 } 2732 2733 public Reference addPharmaceuticalProduct() { // 3 2734 Reference t = new Reference(); 2735 if (this.pharmaceuticalProduct == null) 2736 this.pharmaceuticalProduct = new ArrayList<Reference>(); 2737 this.pharmaceuticalProduct.add(t); 2738 return t; 2739 } 2740 2741 public MedicinalProduct addPharmaceuticalProduct(Reference t) { // 3 2742 if (t == null) 2743 return this; 2744 if (this.pharmaceuticalProduct == null) 2745 this.pharmaceuticalProduct = new ArrayList<Reference>(); 2746 this.pharmaceuticalProduct.add(t); 2747 return this; 2748 } 2749 2750 /** 2751 * @return The first repetition of repeating field 2752 * {@link #pharmaceuticalProduct}, creating it if it does not already 2753 * exist 2754 */ 2755 public Reference getPharmaceuticalProductFirstRep() { 2756 if (getPharmaceuticalProduct().isEmpty()) { 2757 addPharmaceuticalProduct(); 2758 } 2759 return getPharmaceuticalProduct().get(0); 2760 } 2761 2762 /** 2763 * @deprecated Use Reference#setResource(IBaseResource) instead 2764 */ 2765 @Deprecated 2766 public List<MedicinalProductPharmaceutical> getPharmaceuticalProductTarget() { 2767 if (this.pharmaceuticalProductTarget == null) 2768 this.pharmaceuticalProductTarget = new ArrayList<MedicinalProductPharmaceutical>(); 2769 return this.pharmaceuticalProductTarget; 2770 } 2771 2772 /** 2773 * @deprecated Use Reference#setResource(IBaseResource) instead 2774 */ 2775 @Deprecated 2776 public MedicinalProductPharmaceutical addPharmaceuticalProductTarget() { 2777 MedicinalProductPharmaceutical r = new MedicinalProductPharmaceutical(); 2778 if (this.pharmaceuticalProductTarget == null) 2779 this.pharmaceuticalProductTarget = new ArrayList<MedicinalProductPharmaceutical>(); 2780 this.pharmaceuticalProductTarget.add(r); 2781 return r; 2782 } 2783 2784 /** 2785 * @return {@link #packagedMedicinalProduct} (Package representation for the 2786 * product.) 2787 */ 2788 public List<Reference> getPackagedMedicinalProduct() { 2789 if (this.packagedMedicinalProduct == null) 2790 this.packagedMedicinalProduct = new ArrayList<Reference>(); 2791 return this.packagedMedicinalProduct; 2792 } 2793 2794 /** 2795 * @return Returns a reference to <code>this</code> for easy method chaining 2796 */ 2797 public MedicinalProduct setPackagedMedicinalProduct(List<Reference> thePackagedMedicinalProduct) { 2798 this.packagedMedicinalProduct = thePackagedMedicinalProduct; 2799 return this; 2800 } 2801 2802 public boolean hasPackagedMedicinalProduct() { 2803 if (this.packagedMedicinalProduct == null) 2804 return false; 2805 for (Reference item : this.packagedMedicinalProduct) 2806 if (!item.isEmpty()) 2807 return true; 2808 return false; 2809 } 2810 2811 public Reference addPackagedMedicinalProduct() { // 3 2812 Reference t = new Reference(); 2813 if (this.packagedMedicinalProduct == null) 2814 this.packagedMedicinalProduct = new ArrayList<Reference>(); 2815 this.packagedMedicinalProduct.add(t); 2816 return t; 2817 } 2818 2819 public MedicinalProduct addPackagedMedicinalProduct(Reference t) { // 3 2820 if (t == null) 2821 return this; 2822 if (this.packagedMedicinalProduct == null) 2823 this.packagedMedicinalProduct = new ArrayList<Reference>(); 2824 this.packagedMedicinalProduct.add(t); 2825 return this; 2826 } 2827 2828 /** 2829 * @return The first repetition of repeating field 2830 * {@link #packagedMedicinalProduct}, creating it if it does not already 2831 * exist 2832 */ 2833 public Reference getPackagedMedicinalProductFirstRep() { 2834 if (getPackagedMedicinalProduct().isEmpty()) { 2835 addPackagedMedicinalProduct(); 2836 } 2837 return getPackagedMedicinalProduct().get(0); 2838 } 2839 2840 /** 2841 * @deprecated Use Reference#setResource(IBaseResource) instead 2842 */ 2843 @Deprecated 2844 public List<MedicinalProductPackaged> getPackagedMedicinalProductTarget() { 2845 if (this.packagedMedicinalProductTarget == null) 2846 this.packagedMedicinalProductTarget = new ArrayList<MedicinalProductPackaged>(); 2847 return this.packagedMedicinalProductTarget; 2848 } 2849 2850 /** 2851 * @deprecated Use Reference#setResource(IBaseResource) instead 2852 */ 2853 @Deprecated 2854 public MedicinalProductPackaged addPackagedMedicinalProductTarget() { 2855 MedicinalProductPackaged r = new MedicinalProductPackaged(); 2856 if (this.packagedMedicinalProductTarget == null) 2857 this.packagedMedicinalProductTarget = new ArrayList<MedicinalProductPackaged>(); 2858 this.packagedMedicinalProductTarget.add(r); 2859 return r; 2860 } 2861 2862 /** 2863 * @return {@link #attachedDocument} (Supporting documentation, typically for 2864 * regulatory submission.) 2865 */ 2866 public List<Reference> getAttachedDocument() { 2867 if (this.attachedDocument == null) 2868 this.attachedDocument = new ArrayList<Reference>(); 2869 return this.attachedDocument; 2870 } 2871 2872 /** 2873 * @return Returns a reference to <code>this</code> for easy method chaining 2874 */ 2875 public MedicinalProduct setAttachedDocument(List<Reference> theAttachedDocument) { 2876 this.attachedDocument = theAttachedDocument; 2877 return this; 2878 } 2879 2880 public boolean hasAttachedDocument() { 2881 if (this.attachedDocument == null) 2882 return false; 2883 for (Reference item : this.attachedDocument) 2884 if (!item.isEmpty()) 2885 return true; 2886 return false; 2887 } 2888 2889 public Reference addAttachedDocument() { // 3 2890 Reference t = new Reference(); 2891 if (this.attachedDocument == null) 2892 this.attachedDocument = new ArrayList<Reference>(); 2893 this.attachedDocument.add(t); 2894 return t; 2895 } 2896 2897 public MedicinalProduct addAttachedDocument(Reference t) { // 3 2898 if (t == null) 2899 return this; 2900 if (this.attachedDocument == null) 2901 this.attachedDocument = new ArrayList<Reference>(); 2902 this.attachedDocument.add(t); 2903 return this; 2904 } 2905 2906 /** 2907 * @return The first repetition of repeating field {@link #attachedDocument}, 2908 * creating it if it does not already exist 2909 */ 2910 public Reference getAttachedDocumentFirstRep() { 2911 if (getAttachedDocument().isEmpty()) { 2912 addAttachedDocument(); 2913 } 2914 return getAttachedDocument().get(0); 2915 } 2916 2917 /** 2918 * @deprecated Use Reference#setResource(IBaseResource) instead 2919 */ 2920 @Deprecated 2921 public List<DocumentReference> getAttachedDocumentTarget() { 2922 if (this.attachedDocumentTarget == null) 2923 this.attachedDocumentTarget = new ArrayList<DocumentReference>(); 2924 return this.attachedDocumentTarget; 2925 } 2926 2927 /** 2928 * @deprecated Use Reference#setResource(IBaseResource) instead 2929 */ 2930 @Deprecated 2931 public DocumentReference addAttachedDocumentTarget() { 2932 DocumentReference r = new DocumentReference(); 2933 if (this.attachedDocumentTarget == null) 2934 this.attachedDocumentTarget = new ArrayList<DocumentReference>(); 2935 this.attachedDocumentTarget.add(r); 2936 return r; 2937 } 2938 2939 /** 2940 * @return {@link #masterFile} (A master file for to the medicinal product (e.g. 2941 * Pharmacovigilance System Master File).) 2942 */ 2943 public List<Reference> getMasterFile() { 2944 if (this.masterFile == null) 2945 this.masterFile = new ArrayList<Reference>(); 2946 return this.masterFile; 2947 } 2948 2949 /** 2950 * @return Returns a reference to <code>this</code> for easy method chaining 2951 */ 2952 public MedicinalProduct setMasterFile(List<Reference> theMasterFile) { 2953 this.masterFile = theMasterFile; 2954 return this; 2955 } 2956 2957 public boolean hasMasterFile() { 2958 if (this.masterFile == null) 2959 return false; 2960 for (Reference item : this.masterFile) 2961 if (!item.isEmpty()) 2962 return true; 2963 return false; 2964 } 2965 2966 public Reference addMasterFile() { // 3 2967 Reference t = new Reference(); 2968 if (this.masterFile == null) 2969 this.masterFile = new ArrayList<Reference>(); 2970 this.masterFile.add(t); 2971 return t; 2972 } 2973 2974 public MedicinalProduct addMasterFile(Reference t) { // 3 2975 if (t == null) 2976 return this; 2977 if (this.masterFile == null) 2978 this.masterFile = new ArrayList<Reference>(); 2979 this.masterFile.add(t); 2980 return this; 2981 } 2982 2983 /** 2984 * @return The first repetition of repeating field {@link #masterFile}, creating 2985 * it if it does not already exist 2986 */ 2987 public Reference getMasterFileFirstRep() { 2988 if (getMasterFile().isEmpty()) { 2989 addMasterFile(); 2990 } 2991 return getMasterFile().get(0); 2992 } 2993 2994 /** 2995 * @deprecated Use Reference#setResource(IBaseResource) instead 2996 */ 2997 @Deprecated 2998 public List<DocumentReference> getMasterFileTarget() { 2999 if (this.masterFileTarget == null) 3000 this.masterFileTarget = new ArrayList<DocumentReference>(); 3001 return this.masterFileTarget; 3002 } 3003 3004 /** 3005 * @deprecated Use Reference#setResource(IBaseResource) instead 3006 */ 3007 @Deprecated 3008 public DocumentReference addMasterFileTarget() { 3009 DocumentReference r = new DocumentReference(); 3010 if (this.masterFileTarget == null) 3011 this.masterFileTarget = new ArrayList<DocumentReference>(); 3012 this.masterFileTarget.add(r); 3013 return r; 3014 } 3015 3016 /** 3017 * @return {@link #contact} (A product specific contact, person (in a role), or 3018 * an organization.) 3019 */ 3020 public List<Reference> getContact() { 3021 if (this.contact == null) 3022 this.contact = new ArrayList<Reference>(); 3023 return this.contact; 3024 } 3025 3026 /** 3027 * @return Returns a reference to <code>this</code> for easy method chaining 3028 */ 3029 public MedicinalProduct setContact(List<Reference> theContact) { 3030 this.contact = theContact; 3031 return this; 3032 } 3033 3034 public boolean hasContact() { 3035 if (this.contact == null) 3036 return false; 3037 for (Reference item : this.contact) 3038 if (!item.isEmpty()) 3039 return true; 3040 return false; 3041 } 3042 3043 public Reference addContact() { // 3 3044 Reference t = new Reference(); 3045 if (this.contact == null) 3046 this.contact = new ArrayList<Reference>(); 3047 this.contact.add(t); 3048 return t; 3049 } 3050 3051 public MedicinalProduct addContact(Reference t) { // 3 3052 if (t == null) 3053 return this; 3054 if (this.contact == null) 3055 this.contact = new ArrayList<Reference>(); 3056 this.contact.add(t); 3057 return this; 3058 } 3059 3060 /** 3061 * @return The first repetition of repeating field {@link #contact}, creating it 3062 * if it does not already exist 3063 */ 3064 public Reference getContactFirstRep() { 3065 if (getContact().isEmpty()) { 3066 addContact(); 3067 } 3068 return getContact().get(0); 3069 } 3070 3071 /** 3072 * @deprecated Use Reference#setResource(IBaseResource) instead 3073 */ 3074 @Deprecated 3075 public List<Resource> getContactTarget() { 3076 if (this.contactTarget == null) 3077 this.contactTarget = new ArrayList<Resource>(); 3078 return this.contactTarget; 3079 } 3080 3081 /** 3082 * @return {@link #clinicalTrial} (Clinical trials or studies that this product 3083 * is involved in.) 3084 */ 3085 public List<Reference> getClinicalTrial() { 3086 if (this.clinicalTrial == null) 3087 this.clinicalTrial = new ArrayList<Reference>(); 3088 return this.clinicalTrial; 3089 } 3090 3091 /** 3092 * @return Returns a reference to <code>this</code> for easy method chaining 3093 */ 3094 public MedicinalProduct setClinicalTrial(List<Reference> theClinicalTrial) { 3095 this.clinicalTrial = theClinicalTrial; 3096 return this; 3097 } 3098 3099 public boolean hasClinicalTrial() { 3100 if (this.clinicalTrial == null) 3101 return false; 3102 for (Reference item : this.clinicalTrial) 3103 if (!item.isEmpty()) 3104 return true; 3105 return false; 3106 } 3107 3108 public Reference addClinicalTrial() { // 3 3109 Reference t = new Reference(); 3110 if (this.clinicalTrial == null) 3111 this.clinicalTrial = new ArrayList<Reference>(); 3112 this.clinicalTrial.add(t); 3113 return t; 3114 } 3115 3116 public MedicinalProduct addClinicalTrial(Reference t) { // 3 3117 if (t == null) 3118 return this; 3119 if (this.clinicalTrial == null) 3120 this.clinicalTrial = new ArrayList<Reference>(); 3121 this.clinicalTrial.add(t); 3122 return this; 3123 } 3124 3125 /** 3126 * @return The first repetition of repeating field {@link #clinicalTrial}, 3127 * creating it if it does not already exist 3128 */ 3129 public Reference getClinicalTrialFirstRep() { 3130 if (getClinicalTrial().isEmpty()) { 3131 addClinicalTrial(); 3132 } 3133 return getClinicalTrial().get(0); 3134 } 3135 3136 /** 3137 * @deprecated Use Reference#setResource(IBaseResource) instead 3138 */ 3139 @Deprecated 3140 public List<ResearchStudy> getClinicalTrialTarget() { 3141 if (this.clinicalTrialTarget == null) 3142 this.clinicalTrialTarget = new ArrayList<ResearchStudy>(); 3143 return this.clinicalTrialTarget; 3144 } 3145 3146 /** 3147 * @deprecated Use Reference#setResource(IBaseResource) instead 3148 */ 3149 @Deprecated 3150 public ResearchStudy addClinicalTrialTarget() { 3151 ResearchStudy r = new ResearchStudy(); 3152 if (this.clinicalTrialTarget == null) 3153 this.clinicalTrialTarget = new ArrayList<ResearchStudy>(); 3154 this.clinicalTrialTarget.add(r); 3155 return r; 3156 } 3157 3158 /** 3159 * @return {@link #name} (The product's name, including full name and possibly 3160 * coded parts.) 3161 */ 3162 public List<MedicinalProductNameComponent> getName() { 3163 if (this.name == null) 3164 this.name = new ArrayList<MedicinalProductNameComponent>(); 3165 return this.name; 3166 } 3167 3168 /** 3169 * @return Returns a reference to <code>this</code> for easy method chaining 3170 */ 3171 public MedicinalProduct setName(List<MedicinalProductNameComponent> theName) { 3172 this.name = theName; 3173 return this; 3174 } 3175 3176 public boolean hasName() { 3177 if (this.name == null) 3178 return false; 3179 for (MedicinalProductNameComponent item : this.name) 3180 if (!item.isEmpty()) 3181 return true; 3182 return false; 3183 } 3184 3185 public MedicinalProductNameComponent addName() { // 3 3186 MedicinalProductNameComponent t = new MedicinalProductNameComponent(); 3187 if (this.name == null) 3188 this.name = new ArrayList<MedicinalProductNameComponent>(); 3189 this.name.add(t); 3190 return t; 3191 } 3192 3193 public MedicinalProduct addName(MedicinalProductNameComponent t) { // 3 3194 if (t == null) 3195 return this; 3196 if (this.name == null) 3197 this.name = new ArrayList<MedicinalProductNameComponent>(); 3198 this.name.add(t); 3199 return this; 3200 } 3201 3202 /** 3203 * @return The first repetition of repeating field {@link #name}, creating it if 3204 * it does not already exist 3205 */ 3206 public MedicinalProductNameComponent getNameFirstRep() { 3207 if (getName().isEmpty()) { 3208 addName(); 3209 } 3210 return getName().get(0); 3211 } 3212 3213 /** 3214 * @return {@link #crossReference} (Reference to another product, e.g. for 3215 * linking authorised to investigational product.) 3216 */ 3217 public List<Identifier> getCrossReference() { 3218 if (this.crossReference == null) 3219 this.crossReference = new ArrayList<Identifier>(); 3220 return this.crossReference; 3221 } 3222 3223 /** 3224 * @return Returns a reference to <code>this</code> for easy method chaining 3225 */ 3226 public MedicinalProduct setCrossReference(List<Identifier> theCrossReference) { 3227 this.crossReference = theCrossReference; 3228 return this; 3229 } 3230 3231 public boolean hasCrossReference() { 3232 if (this.crossReference == null) 3233 return false; 3234 for (Identifier item : this.crossReference) 3235 if (!item.isEmpty()) 3236 return true; 3237 return false; 3238 } 3239 3240 public Identifier addCrossReference() { // 3 3241 Identifier t = new Identifier(); 3242 if (this.crossReference == null) 3243 this.crossReference = new ArrayList<Identifier>(); 3244 this.crossReference.add(t); 3245 return t; 3246 } 3247 3248 public MedicinalProduct addCrossReference(Identifier t) { // 3 3249 if (t == null) 3250 return this; 3251 if (this.crossReference == null) 3252 this.crossReference = new ArrayList<Identifier>(); 3253 this.crossReference.add(t); 3254 return this; 3255 } 3256 3257 /** 3258 * @return The first repetition of repeating field {@link #crossReference}, 3259 * creating it if it does not already exist 3260 */ 3261 public Identifier getCrossReferenceFirstRep() { 3262 if (getCrossReference().isEmpty()) { 3263 addCrossReference(); 3264 } 3265 return getCrossReference().get(0); 3266 } 3267 3268 /** 3269 * @return {@link #manufacturingBusinessOperation} (An operation applied to the 3270 * product, for manufacturing or adminsitrative purpose.) 3271 */ 3272 public List<MedicinalProductManufacturingBusinessOperationComponent> getManufacturingBusinessOperation() { 3273 if (this.manufacturingBusinessOperation == null) 3274 this.manufacturingBusinessOperation = new ArrayList<MedicinalProductManufacturingBusinessOperationComponent>(); 3275 return this.manufacturingBusinessOperation; 3276 } 3277 3278 /** 3279 * @return Returns a reference to <code>this</code> for easy method chaining 3280 */ 3281 public MedicinalProduct setManufacturingBusinessOperation( 3282 List<MedicinalProductManufacturingBusinessOperationComponent> theManufacturingBusinessOperation) { 3283 this.manufacturingBusinessOperation = theManufacturingBusinessOperation; 3284 return this; 3285 } 3286 3287 public boolean hasManufacturingBusinessOperation() { 3288 if (this.manufacturingBusinessOperation == null) 3289 return false; 3290 for (MedicinalProductManufacturingBusinessOperationComponent item : this.manufacturingBusinessOperation) 3291 if (!item.isEmpty()) 3292 return true; 3293 return false; 3294 } 3295 3296 public MedicinalProductManufacturingBusinessOperationComponent addManufacturingBusinessOperation() { // 3 3297 MedicinalProductManufacturingBusinessOperationComponent t = new MedicinalProductManufacturingBusinessOperationComponent(); 3298 if (this.manufacturingBusinessOperation == null) 3299 this.manufacturingBusinessOperation = new ArrayList<MedicinalProductManufacturingBusinessOperationComponent>(); 3300 this.manufacturingBusinessOperation.add(t); 3301 return t; 3302 } 3303 3304 public MedicinalProduct addManufacturingBusinessOperation(MedicinalProductManufacturingBusinessOperationComponent t) { // 3 3305 if (t == null) 3306 return this; 3307 if (this.manufacturingBusinessOperation == null) 3308 this.manufacturingBusinessOperation = new ArrayList<MedicinalProductManufacturingBusinessOperationComponent>(); 3309 this.manufacturingBusinessOperation.add(t); 3310 return this; 3311 } 3312 3313 /** 3314 * @return The first repetition of repeating field 3315 * {@link #manufacturingBusinessOperation}, creating it if it does not 3316 * already exist 3317 */ 3318 public MedicinalProductManufacturingBusinessOperationComponent getManufacturingBusinessOperationFirstRep() { 3319 if (getManufacturingBusinessOperation().isEmpty()) { 3320 addManufacturingBusinessOperation(); 3321 } 3322 return getManufacturingBusinessOperation().get(0); 3323 } 3324 3325 /** 3326 * @return {@link #specialDesignation} (Indicates if the medicinal product has 3327 * an orphan designation for the treatment of a rare disease.) 3328 */ 3329 public List<MedicinalProductSpecialDesignationComponent> getSpecialDesignation() { 3330 if (this.specialDesignation == null) 3331 this.specialDesignation = new ArrayList<MedicinalProductSpecialDesignationComponent>(); 3332 return this.specialDesignation; 3333 } 3334 3335 /** 3336 * @return Returns a reference to <code>this</code> for easy method chaining 3337 */ 3338 public MedicinalProduct setSpecialDesignation( 3339 List<MedicinalProductSpecialDesignationComponent> theSpecialDesignation) { 3340 this.specialDesignation = theSpecialDesignation; 3341 return this; 3342 } 3343 3344 public boolean hasSpecialDesignation() { 3345 if (this.specialDesignation == null) 3346 return false; 3347 for (MedicinalProductSpecialDesignationComponent item : this.specialDesignation) 3348 if (!item.isEmpty()) 3349 return true; 3350 return false; 3351 } 3352 3353 public MedicinalProductSpecialDesignationComponent addSpecialDesignation() { // 3 3354 MedicinalProductSpecialDesignationComponent t = new MedicinalProductSpecialDesignationComponent(); 3355 if (this.specialDesignation == null) 3356 this.specialDesignation = new ArrayList<MedicinalProductSpecialDesignationComponent>(); 3357 this.specialDesignation.add(t); 3358 return t; 3359 } 3360 3361 public MedicinalProduct addSpecialDesignation(MedicinalProductSpecialDesignationComponent t) { // 3 3362 if (t == null) 3363 return this; 3364 if (this.specialDesignation == null) 3365 this.specialDesignation = new ArrayList<MedicinalProductSpecialDesignationComponent>(); 3366 this.specialDesignation.add(t); 3367 return this; 3368 } 3369 3370 /** 3371 * @return The first repetition of repeating field {@link #specialDesignation}, 3372 * creating it if it does not already exist 3373 */ 3374 public MedicinalProductSpecialDesignationComponent getSpecialDesignationFirstRep() { 3375 if (getSpecialDesignation().isEmpty()) { 3376 addSpecialDesignation(); 3377 } 3378 return getSpecialDesignation().get(0); 3379 } 3380 3381 protected void listChildren(List<Property> children) { 3382 super.listChildren(children); 3383 children.add(new Property("identifier", "Identifier", "Business identifier for this product. Could be an MPID.", 0, 3384 java.lang.Integer.MAX_VALUE, identifier)); 3385 children.add( 3386 new Property("type", "CodeableConcept", "Regulatory type, e.g. Investigational or Authorized.", 0, 1, type)); 3387 children 3388 .add(new Property("domain", "Coding", "If this medicine applies to human or veterinary uses.", 0, 1, domain)); 3389 children.add(new Property("combinedPharmaceuticalDoseForm", "CodeableConcept", 3390 "The dose form for a single part product, or combined form of a multiple part product.", 0, 1, 3391 combinedPharmaceuticalDoseForm)); 3392 children.add(new Property("legalStatusOfSupply", "CodeableConcept", 3393 "The legal status of supply of the medicinal product as classified by the regulator.", 0, 1, 3394 legalStatusOfSupply)); 3395 children.add(new Property("additionalMonitoringIndicator", "CodeableConcept", 3396 "Whether the Medicinal Product is subject to additional monitoring for regulatory reasons.", 0, 1, 3397 additionalMonitoringIndicator)); 3398 children.add(new Property("specialMeasures", "string", 3399 "Whether the Medicinal Product is subject to special measures for regulatory reasons.", 0, 3400 java.lang.Integer.MAX_VALUE, specialMeasures)); 3401 children.add(new Property("paediatricUseIndicator", "CodeableConcept", "If authorised for use in children.", 0, 1, 3402 paediatricUseIndicator)); 3403 children.add(new Property("productClassification", "CodeableConcept", 3404 "Allows the product to be classified by various systems.", 0, java.lang.Integer.MAX_VALUE, 3405 productClassification)); 3406 children.add(new Property("marketingStatus", "MarketingStatus", 3407 "Marketing status of the medicinal product, in contrast to marketing authorizaton.", 0, 3408 java.lang.Integer.MAX_VALUE, marketingStatus)); 3409 children.add(new Property("pharmaceuticalProduct", "Reference(MedicinalProductPharmaceutical)", 3410 "Pharmaceutical aspects of product.", 0, java.lang.Integer.MAX_VALUE, pharmaceuticalProduct)); 3411 children.add(new Property("packagedMedicinalProduct", "Reference(MedicinalProductPackaged)", 3412 "Package representation for the product.", 0, java.lang.Integer.MAX_VALUE, packagedMedicinalProduct)); 3413 children.add(new Property("attachedDocument", "Reference(DocumentReference)", 3414 "Supporting documentation, typically for regulatory submission.", 0, java.lang.Integer.MAX_VALUE, 3415 attachedDocument)); 3416 children.add(new Property("masterFile", "Reference(DocumentReference)", 3417 "A master file for to the medicinal product (e.g. Pharmacovigilance System Master File).", 0, 3418 java.lang.Integer.MAX_VALUE, masterFile)); 3419 children.add(new Property("contact", "Reference(Organization|PractitionerRole)", 3420 "A product specific contact, person (in a role), or an organization.", 0, java.lang.Integer.MAX_VALUE, 3421 contact)); 3422 children.add(new Property("clinicalTrial", "Reference(ResearchStudy)", 3423 "Clinical trials or studies that this product is involved in.", 0, java.lang.Integer.MAX_VALUE, clinicalTrial)); 3424 children.add(new Property("name", "", "The product's name, including full name and possibly coded parts.", 0, 3425 java.lang.Integer.MAX_VALUE, name)); 3426 children.add(new Property("crossReference", "Identifier", 3427 "Reference to another product, e.g. for linking authorised to investigational product.", 0, 3428 java.lang.Integer.MAX_VALUE, crossReference)); 3429 children.add(new Property("manufacturingBusinessOperation", "", 3430 "An operation applied to the product, for manufacturing or adminsitrative purpose.", 0, 3431 java.lang.Integer.MAX_VALUE, manufacturingBusinessOperation)); 3432 children.add(new Property("specialDesignation", "", 3433 "Indicates if the medicinal product has an orphan designation for the treatment of a rare disease.", 0, 3434 java.lang.Integer.MAX_VALUE, specialDesignation)); 3435 } 3436 3437 @Override 3438 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3439 switch (_hash) { 3440 case -1618432855: 3441 /* identifier */ return new Property("identifier", "Identifier", 3442 "Business identifier for this product. Could be an MPID.", 0, java.lang.Integer.MAX_VALUE, identifier); 3443 case 3575610: 3444 /* type */ return new Property("type", "CodeableConcept", "Regulatory type, e.g. Investigational or Authorized.", 3445 0, 1, type); 3446 case -1326197564: 3447 /* domain */ return new Property("domain", "Coding", "If this medicine applies to human or veterinary uses.", 0, 3448 1, domain); 3449 case -1992898487: 3450 /* combinedPharmaceuticalDoseForm */ return new Property("combinedPharmaceuticalDoseForm", "CodeableConcept", 3451 "The dose form for a single part product, or combined form of a multiple part product.", 0, 1, 3452 combinedPharmaceuticalDoseForm); 3453 case -844874031: 3454 /* legalStatusOfSupply */ return new Property("legalStatusOfSupply", "CodeableConcept", 3455 "The legal status of supply of the medicinal product as classified by the regulator.", 0, 1, 3456 legalStatusOfSupply); 3457 case 1935999744: 3458 /* additionalMonitoringIndicator */ return new Property("additionalMonitoringIndicator", "CodeableConcept", 3459 "Whether the Medicinal Product is subject to additional monitoring for regulatory reasons.", 0, 1, 3460 additionalMonitoringIndicator); 3461 case 975102638: 3462 /* specialMeasures */ return new Property("specialMeasures", "string", 3463 "Whether the Medicinal Product is subject to special measures for regulatory reasons.", 0, 3464 java.lang.Integer.MAX_VALUE, specialMeasures); 3465 case -1019867160: 3466 /* paediatricUseIndicator */ return new Property("paediatricUseIndicator", "CodeableConcept", 3467 "If authorised for use in children.", 0, 1, paediatricUseIndicator); 3468 case 1247936181: 3469 /* productClassification */ return new Property("productClassification", "CodeableConcept", 3470 "Allows the product to be classified by various systems.", 0, java.lang.Integer.MAX_VALUE, 3471 productClassification); 3472 case 70767032: 3473 /* marketingStatus */ return new Property("marketingStatus", "MarketingStatus", 3474 "Marketing status of the medicinal product, in contrast to marketing authorizaton.", 0, 3475 java.lang.Integer.MAX_VALUE, marketingStatus); 3476 case 443273260: 3477 /* pharmaceuticalProduct */ return new Property("pharmaceuticalProduct", 3478 "Reference(MedicinalProductPharmaceutical)", "Pharmaceutical aspects of product.", 0, 3479 java.lang.Integer.MAX_VALUE, pharmaceuticalProduct); 3480 case -361025513: 3481 /* packagedMedicinalProduct */ return new Property("packagedMedicinalProduct", 3482 "Reference(MedicinalProductPackaged)", "Package representation for the product.", 0, 3483 java.lang.Integer.MAX_VALUE, packagedMedicinalProduct); 3484 case -513945889: 3485 /* attachedDocument */ return new Property("attachedDocument", "Reference(DocumentReference)", 3486 "Supporting documentation, typically for regulatory submission.", 0, java.lang.Integer.MAX_VALUE, 3487 attachedDocument); 3488 case -2039573762: 3489 /* masterFile */ return new Property("masterFile", "Reference(DocumentReference)", 3490 "A master file for to the medicinal product (e.g. Pharmacovigilance System Master File).", 0, 3491 java.lang.Integer.MAX_VALUE, masterFile); 3492 case 951526432: 3493 /* contact */ return new Property("contact", "Reference(Organization|PractitionerRole)", 3494 "A product specific contact, person (in a role), or an organization.", 0, java.lang.Integer.MAX_VALUE, 3495 contact); 3496 case 1232866243: 3497 /* clinicalTrial */ return new Property("clinicalTrial", "Reference(ResearchStudy)", 3498 "Clinical trials or studies that this product is involved in.", 0, java.lang.Integer.MAX_VALUE, 3499 clinicalTrial); 3500 case 3373707: 3501 /* name */ return new Property("name", "", "The product's name, including full name and possibly coded parts.", 0, 3502 java.lang.Integer.MAX_VALUE, name); 3503 case -986968341: 3504 /* crossReference */ return new Property("crossReference", "Identifier", 3505 "Reference to another product, e.g. for linking authorised to investigational product.", 0, 3506 java.lang.Integer.MAX_VALUE, crossReference); 3507 case -171103255: 3508 /* manufacturingBusinessOperation */ return new Property("manufacturingBusinessOperation", "", 3509 "An operation applied to the product, for manufacturing or adminsitrative purpose.", 0, 3510 java.lang.Integer.MAX_VALUE, manufacturingBusinessOperation); 3511 case -964310658: 3512 /* specialDesignation */ return new Property("specialDesignation", "", 3513 "Indicates if the medicinal product has an orphan designation for the treatment of a rare disease.", 0, 3514 java.lang.Integer.MAX_VALUE, specialDesignation); 3515 default: 3516 return super.getNamedProperty(_hash, _name, _checkValid); 3517 } 3518 3519 } 3520 3521 @Override 3522 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3523 switch (hash) { 3524 case -1618432855: 3525 /* identifier */ return this.identifier == null ? new Base[0] 3526 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3527 case 3575610: 3528 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3529 case -1326197564: 3530 /* domain */ return this.domain == null ? new Base[0] : new Base[] { this.domain }; // Coding 3531 case -1992898487: 3532 /* combinedPharmaceuticalDoseForm */ return this.combinedPharmaceuticalDoseForm == null ? new Base[0] 3533 : new Base[] { this.combinedPharmaceuticalDoseForm }; // CodeableConcept 3534 case -844874031: 3535 /* legalStatusOfSupply */ return this.legalStatusOfSupply == null ? new Base[0] 3536 : new Base[] { this.legalStatusOfSupply }; // CodeableConcept 3537 case 1935999744: 3538 /* additionalMonitoringIndicator */ return this.additionalMonitoringIndicator == null ? new Base[0] 3539 : new Base[] { this.additionalMonitoringIndicator }; // CodeableConcept 3540 case 975102638: 3541 /* specialMeasures */ return this.specialMeasures == null ? new Base[0] 3542 : this.specialMeasures.toArray(new Base[this.specialMeasures.size()]); // StringType 3543 case -1019867160: 3544 /* paediatricUseIndicator */ return this.paediatricUseIndicator == null ? new Base[0] 3545 : new Base[] { this.paediatricUseIndicator }; // CodeableConcept 3546 case 1247936181: 3547 /* productClassification */ return this.productClassification == null ? new Base[0] 3548 : this.productClassification.toArray(new Base[this.productClassification.size()]); // CodeableConcept 3549 case 70767032: 3550 /* marketingStatus */ return this.marketingStatus == null ? new Base[0] 3551 : this.marketingStatus.toArray(new Base[this.marketingStatus.size()]); // MarketingStatus 3552 case 443273260: 3553 /* pharmaceuticalProduct */ return this.pharmaceuticalProduct == null ? new Base[0] 3554 : this.pharmaceuticalProduct.toArray(new Base[this.pharmaceuticalProduct.size()]); // Reference 3555 case -361025513: 3556 /* packagedMedicinalProduct */ return this.packagedMedicinalProduct == null ? new Base[0] 3557 : this.packagedMedicinalProduct.toArray(new Base[this.packagedMedicinalProduct.size()]); // Reference 3558 case -513945889: 3559 /* attachedDocument */ return this.attachedDocument == null ? new Base[0] 3560 : this.attachedDocument.toArray(new Base[this.attachedDocument.size()]); // Reference 3561 case -2039573762: 3562 /* masterFile */ return this.masterFile == null ? new Base[0] 3563 : this.masterFile.toArray(new Base[this.masterFile.size()]); // Reference 3564 case 951526432: 3565 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // Reference 3566 case 1232866243: 3567 /* clinicalTrial */ return this.clinicalTrial == null ? new Base[0] 3568 : this.clinicalTrial.toArray(new Base[this.clinicalTrial.size()]); // Reference 3569 case 3373707: 3570 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // MedicinalProductNameComponent 3571 case -986968341: 3572 /* crossReference */ return this.crossReference == null ? new Base[0] 3573 : this.crossReference.toArray(new Base[this.crossReference.size()]); // Identifier 3574 case -171103255: 3575 /* manufacturingBusinessOperation */ return this.manufacturingBusinessOperation == null ? new Base[0] 3576 : this.manufacturingBusinessOperation.toArray(new Base[this.manufacturingBusinessOperation.size()]); // MedicinalProductManufacturingBusinessOperationComponent 3577 case -964310658: 3578 /* specialDesignation */ return this.specialDesignation == null ? new Base[0] 3579 : this.specialDesignation.toArray(new Base[this.specialDesignation.size()]); // MedicinalProductSpecialDesignationComponent 3580 default: 3581 return super.getProperty(hash, name, checkValid); 3582 } 3583 3584 } 3585 3586 @Override 3587 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3588 switch (hash) { 3589 case -1618432855: // identifier 3590 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3591 return value; 3592 case 3575610: // type 3593 this.type = castToCodeableConcept(value); // CodeableConcept 3594 return value; 3595 case -1326197564: // domain 3596 this.domain = castToCoding(value); // Coding 3597 return value; 3598 case -1992898487: // combinedPharmaceuticalDoseForm 3599 this.combinedPharmaceuticalDoseForm = castToCodeableConcept(value); // CodeableConcept 3600 return value; 3601 case -844874031: // legalStatusOfSupply 3602 this.legalStatusOfSupply = castToCodeableConcept(value); // CodeableConcept 3603 return value; 3604 case 1935999744: // additionalMonitoringIndicator 3605 this.additionalMonitoringIndicator = castToCodeableConcept(value); // CodeableConcept 3606 return value; 3607 case 975102638: // specialMeasures 3608 this.getSpecialMeasures().add(castToString(value)); // StringType 3609 return value; 3610 case -1019867160: // paediatricUseIndicator 3611 this.paediatricUseIndicator = castToCodeableConcept(value); // CodeableConcept 3612 return value; 3613 case 1247936181: // productClassification 3614 this.getProductClassification().add(castToCodeableConcept(value)); // CodeableConcept 3615 return value; 3616 case 70767032: // marketingStatus 3617 this.getMarketingStatus().add(castToMarketingStatus(value)); // MarketingStatus 3618 return value; 3619 case 443273260: // pharmaceuticalProduct 3620 this.getPharmaceuticalProduct().add(castToReference(value)); // Reference 3621 return value; 3622 case -361025513: // packagedMedicinalProduct 3623 this.getPackagedMedicinalProduct().add(castToReference(value)); // Reference 3624 return value; 3625 case -513945889: // attachedDocument 3626 this.getAttachedDocument().add(castToReference(value)); // Reference 3627 return value; 3628 case -2039573762: // masterFile 3629 this.getMasterFile().add(castToReference(value)); // Reference 3630 return value; 3631 case 951526432: // contact 3632 this.getContact().add(castToReference(value)); // Reference 3633 return value; 3634 case 1232866243: // clinicalTrial 3635 this.getClinicalTrial().add(castToReference(value)); // Reference 3636 return value; 3637 case 3373707: // name 3638 this.getName().add((MedicinalProductNameComponent) value); // MedicinalProductNameComponent 3639 return value; 3640 case -986968341: // crossReference 3641 this.getCrossReference().add(castToIdentifier(value)); // Identifier 3642 return value; 3643 case -171103255: // manufacturingBusinessOperation 3644 this.getManufacturingBusinessOperation().add((MedicinalProductManufacturingBusinessOperationComponent) value); // MedicinalProductManufacturingBusinessOperationComponent 3645 return value; 3646 case -964310658: // specialDesignation 3647 this.getSpecialDesignation().add((MedicinalProductSpecialDesignationComponent) value); // MedicinalProductSpecialDesignationComponent 3648 return value; 3649 default: 3650 return super.setProperty(hash, name, value); 3651 } 3652 3653 } 3654 3655 @Override 3656 public Base setProperty(String name, Base value) throws FHIRException { 3657 if (name.equals("identifier")) { 3658 this.getIdentifier().add(castToIdentifier(value)); 3659 } else if (name.equals("type")) { 3660 this.type = castToCodeableConcept(value); // CodeableConcept 3661 } else if (name.equals("domain")) { 3662 this.domain = castToCoding(value); // Coding 3663 } else if (name.equals("combinedPharmaceuticalDoseForm")) { 3664 this.combinedPharmaceuticalDoseForm = castToCodeableConcept(value); // CodeableConcept 3665 } else if (name.equals("legalStatusOfSupply")) { 3666 this.legalStatusOfSupply = castToCodeableConcept(value); // CodeableConcept 3667 } else if (name.equals("additionalMonitoringIndicator")) { 3668 this.additionalMonitoringIndicator = castToCodeableConcept(value); // CodeableConcept 3669 } else if (name.equals("specialMeasures")) { 3670 this.getSpecialMeasures().add(castToString(value)); 3671 } else if (name.equals("paediatricUseIndicator")) { 3672 this.paediatricUseIndicator = castToCodeableConcept(value); // CodeableConcept 3673 } else if (name.equals("productClassification")) { 3674 this.getProductClassification().add(castToCodeableConcept(value)); 3675 } else if (name.equals("marketingStatus")) { 3676 this.getMarketingStatus().add(castToMarketingStatus(value)); 3677 } else if (name.equals("pharmaceuticalProduct")) { 3678 this.getPharmaceuticalProduct().add(castToReference(value)); 3679 } else if (name.equals("packagedMedicinalProduct")) { 3680 this.getPackagedMedicinalProduct().add(castToReference(value)); 3681 } else if (name.equals("attachedDocument")) { 3682 this.getAttachedDocument().add(castToReference(value)); 3683 } else if (name.equals("masterFile")) { 3684 this.getMasterFile().add(castToReference(value)); 3685 } else if (name.equals("contact")) { 3686 this.getContact().add(castToReference(value)); 3687 } else if (name.equals("clinicalTrial")) { 3688 this.getClinicalTrial().add(castToReference(value)); 3689 } else if (name.equals("name")) { 3690 this.getName().add((MedicinalProductNameComponent) value); 3691 } else if (name.equals("crossReference")) { 3692 this.getCrossReference().add(castToIdentifier(value)); 3693 } else if (name.equals("manufacturingBusinessOperation")) { 3694 this.getManufacturingBusinessOperation().add((MedicinalProductManufacturingBusinessOperationComponent) value); 3695 } else if (name.equals("specialDesignation")) { 3696 this.getSpecialDesignation().add((MedicinalProductSpecialDesignationComponent) value); 3697 } else 3698 return super.setProperty(name, value); 3699 return value; 3700 } 3701 3702 @Override 3703 public Base makeProperty(int hash, String name) throws FHIRException { 3704 switch (hash) { 3705 case -1618432855: 3706 return addIdentifier(); 3707 case 3575610: 3708 return getType(); 3709 case -1326197564: 3710 return getDomain(); 3711 case -1992898487: 3712 return getCombinedPharmaceuticalDoseForm(); 3713 case -844874031: 3714 return getLegalStatusOfSupply(); 3715 case 1935999744: 3716 return getAdditionalMonitoringIndicator(); 3717 case 975102638: 3718 return addSpecialMeasuresElement(); 3719 case -1019867160: 3720 return getPaediatricUseIndicator(); 3721 case 1247936181: 3722 return addProductClassification(); 3723 case 70767032: 3724 return addMarketingStatus(); 3725 case 443273260: 3726 return addPharmaceuticalProduct(); 3727 case -361025513: 3728 return addPackagedMedicinalProduct(); 3729 case -513945889: 3730 return addAttachedDocument(); 3731 case -2039573762: 3732 return addMasterFile(); 3733 case 951526432: 3734 return addContact(); 3735 case 1232866243: 3736 return addClinicalTrial(); 3737 case 3373707: 3738 return addName(); 3739 case -986968341: 3740 return addCrossReference(); 3741 case -171103255: 3742 return addManufacturingBusinessOperation(); 3743 case -964310658: 3744 return addSpecialDesignation(); 3745 default: 3746 return super.makeProperty(hash, name); 3747 } 3748 3749 } 3750 3751 @Override 3752 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3753 switch (hash) { 3754 case -1618432855: 3755 /* identifier */ return new String[] { "Identifier" }; 3756 case 3575610: 3757 /* type */ return new String[] { "CodeableConcept" }; 3758 case -1326197564: 3759 /* domain */ return new String[] { "Coding" }; 3760 case -1992898487: 3761 /* combinedPharmaceuticalDoseForm */ return new String[] { "CodeableConcept" }; 3762 case -844874031: 3763 /* legalStatusOfSupply */ return new String[] { "CodeableConcept" }; 3764 case 1935999744: 3765 /* additionalMonitoringIndicator */ return new String[] { "CodeableConcept" }; 3766 case 975102638: 3767 /* specialMeasures */ return new String[] { "string" }; 3768 case -1019867160: 3769 /* paediatricUseIndicator */ return new String[] { "CodeableConcept" }; 3770 case 1247936181: 3771 /* productClassification */ return new String[] { "CodeableConcept" }; 3772 case 70767032: 3773 /* marketingStatus */ return new String[] { "MarketingStatus" }; 3774 case 443273260: 3775 /* pharmaceuticalProduct */ return new String[] { "Reference" }; 3776 case -361025513: 3777 /* packagedMedicinalProduct */ return new String[] { "Reference" }; 3778 case -513945889: 3779 /* attachedDocument */ return new String[] { "Reference" }; 3780 case -2039573762: 3781 /* masterFile */ return new String[] { "Reference" }; 3782 case 951526432: 3783 /* contact */ return new String[] { "Reference" }; 3784 case 1232866243: 3785 /* clinicalTrial */ return new String[] { "Reference" }; 3786 case 3373707: 3787 /* name */ return new String[] {}; 3788 case -986968341: 3789 /* crossReference */ return new String[] { "Identifier" }; 3790 case -171103255: 3791 /* manufacturingBusinessOperation */ return new String[] {}; 3792 case -964310658: 3793 /* specialDesignation */ return new String[] {}; 3794 default: 3795 return super.getTypesForProperty(hash, name); 3796 } 3797 3798 } 3799 3800 @Override 3801 public Base addChild(String name) throws FHIRException { 3802 if (name.equals("identifier")) { 3803 return addIdentifier(); 3804 } else if (name.equals("type")) { 3805 this.type = new CodeableConcept(); 3806 return this.type; 3807 } else if (name.equals("domain")) { 3808 this.domain = new Coding(); 3809 return this.domain; 3810 } else if (name.equals("combinedPharmaceuticalDoseForm")) { 3811 this.combinedPharmaceuticalDoseForm = new CodeableConcept(); 3812 return this.combinedPharmaceuticalDoseForm; 3813 } else if (name.equals("legalStatusOfSupply")) { 3814 this.legalStatusOfSupply = new CodeableConcept(); 3815 return this.legalStatusOfSupply; 3816 } else if (name.equals("additionalMonitoringIndicator")) { 3817 this.additionalMonitoringIndicator = new CodeableConcept(); 3818 return this.additionalMonitoringIndicator; 3819 } else if (name.equals("specialMeasures")) { 3820 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProduct.specialMeasures"); 3821 } else if (name.equals("paediatricUseIndicator")) { 3822 this.paediatricUseIndicator = new CodeableConcept(); 3823 return this.paediatricUseIndicator; 3824 } else if (name.equals("productClassification")) { 3825 return addProductClassification(); 3826 } else if (name.equals("marketingStatus")) { 3827 return addMarketingStatus(); 3828 } else if (name.equals("pharmaceuticalProduct")) { 3829 return addPharmaceuticalProduct(); 3830 } else if (name.equals("packagedMedicinalProduct")) { 3831 return addPackagedMedicinalProduct(); 3832 } else if (name.equals("attachedDocument")) { 3833 return addAttachedDocument(); 3834 } else if (name.equals("masterFile")) { 3835 return addMasterFile(); 3836 } else if (name.equals("contact")) { 3837 return addContact(); 3838 } else if (name.equals("clinicalTrial")) { 3839 return addClinicalTrial(); 3840 } else if (name.equals("name")) { 3841 return addName(); 3842 } else if (name.equals("crossReference")) { 3843 return addCrossReference(); 3844 } else if (name.equals("manufacturingBusinessOperation")) { 3845 return addManufacturingBusinessOperation(); 3846 } else if (name.equals("specialDesignation")) { 3847 return addSpecialDesignation(); 3848 } else 3849 return super.addChild(name); 3850 } 3851 3852 public String fhirType() { 3853 return "MedicinalProduct"; 3854 3855 } 3856 3857 public MedicinalProduct copy() { 3858 MedicinalProduct dst = new MedicinalProduct(); 3859 copyValues(dst); 3860 return dst; 3861 } 3862 3863 public void copyValues(MedicinalProduct dst) { 3864 super.copyValues(dst); 3865 if (identifier != null) { 3866 dst.identifier = new ArrayList<Identifier>(); 3867 for (Identifier i : identifier) 3868 dst.identifier.add(i.copy()); 3869 } 3870 ; 3871 dst.type = type == null ? null : type.copy(); 3872 dst.domain = domain == null ? null : domain.copy(); 3873 dst.combinedPharmaceuticalDoseForm = combinedPharmaceuticalDoseForm == null ? null 3874 : combinedPharmaceuticalDoseForm.copy(); 3875 dst.legalStatusOfSupply = legalStatusOfSupply == null ? null : legalStatusOfSupply.copy(); 3876 dst.additionalMonitoringIndicator = additionalMonitoringIndicator == null ? null 3877 : additionalMonitoringIndicator.copy(); 3878 if (specialMeasures != null) { 3879 dst.specialMeasures = new ArrayList<StringType>(); 3880 for (StringType i : specialMeasures) 3881 dst.specialMeasures.add(i.copy()); 3882 } 3883 ; 3884 dst.paediatricUseIndicator = paediatricUseIndicator == null ? null : paediatricUseIndicator.copy(); 3885 if (productClassification != null) { 3886 dst.productClassification = new ArrayList<CodeableConcept>(); 3887 for (CodeableConcept i : productClassification) 3888 dst.productClassification.add(i.copy()); 3889 } 3890 ; 3891 if (marketingStatus != null) { 3892 dst.marketingStatus = new ArrayList<MarketingStatus>(); 3893 for (MarketingStatus i : marketingStatus) 3894 dst.marketingStatus.add(i.copy()); 3895 } 3896 ; 3897 if (pharmaceuticalProduct != null) { 3898 dst.pharmaceuticalProduct = new ArrayList<Reference>(); 3899 for (Reference i : pharmaceuticalProduct) 3900 dst.pharmaceuticalProduct.add(i.copy()); 3901 } 3902 ; 3903 if (packagedMedicinalProduct != null) { 3904 dst.packagedMedicinalProduct = new ArrayList<Reference>(); 3905 for (Reference i : packagedMedicinalProduct) 3906 dst.packagedMedicinalProduct.add(i.copy()); 3907 } 3908 ; 3909 if (attachedDocument != null) { 3910 dst.attachedDocument = new ArrayList<Reference>(); 3911 for (Reference i : attachedDocument) 3912 dst.attachedDocument.add(i.copy()); 3913 } 3914 ; 3915 if (masterFile != null) { 3916 dst.masterFile = new ArrayList<Reference>(); 3917 for (Reference i : masterFile) 3918 dst.masterFile.add(i.copy()); 3919 } 3920 ; 3921 if (contact != null) { 3922 dst.contact = new ArrayList<Reference>(); 3923 for (Reference i : contact) 3924 dst.contact.add(i.copy()); 3925 } 3926 ; 3927 if (clinicalTrial != null) { 3928 dst.clinicalTrial = new ArrayList<Reference>(); 3929 for (Reference i : clinicalTrial) 3930 dst.clinicalTrial.add(i.copy()); 3931 } 3932 ; 3933 if (name != null) { 3934 dst.name = new ArrayList<MedicinalProductNameComponent>(); 3935 for (MedicinalProductNameComponent i : name) 3936 dst.name.add(i.copy()); 3937 } 3938 ; 3939 if (crossReference != null) { 3940 dst.crossReference = new ArrayList<Identifier>(); 3941 for (Identifier i : crossReference) 3942 dst.crossReference.add(i.copy()); 3943 } 3944 ; 3945 if (manufacturingBusinessOperation != null) { 3946 dst.manufacturingBusinessOperation = new ArrayList<MedicinalProductManufacturingBusinessOperationComponent>(); 3947 for (MedicinalProductManufacturingBusinessOperationComponent i : manufacturingBusinessOperation) 3948 dst.manufacturingBusinessOperation.add(i.copy()); 3949 } 3950 ; 3951 if (specialDesignation != null) { 3952 dst.specialDesignation = new ArrayList<MedicinalProductSpecialDesignationComponent>(); 3953 for (MedicinalProductSpecialDesignationComponent i : specialDesignation) 3954 dst.specialDesignation.add(i.copy()); 3955 } 3956 ; 3957 } 3958 3959 protected MedicinalProduct typedCopy() { 3960 return copy(); 3961 } 3962 3963 @Override 3964 public boolean equalsDeep(Base other_) { 3965 if (!super.equalsDeep(other_)) 3966 return false; 3967 if (!(other_ instanceof MedicinalProduct)) 3968 return false; 3969 MedicinalProduct o = (MedicinalProduct) other_; 3970 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 3971 && compareDeep(domain, o.domain, true) 3972 && compareDeep(combinedPharmaceuticalDoseForm, o.combinedPharmaceuticalDoseForm, true) 3973 && compareDeep(legalStatusOfSupply, o.legalStatusOfSupply, true) 3974 && compareDeep(additionalMonitoringIndicator, o.additionalMonitoringIndicator, true) 3975 && compareDeep(specialMeasures, o.specialMeasures, true) 3976 && compareDeep(paediatricUseIndicator, o.paediatricUseIndicator, true) 3977 && compareDeep(productClassification, o.productClassification, true) 3978 && compareDeep(marketingStatus, o.marketingStatus, true) 3979 && compareDeep(pharmaceuticalProduct, o.pharmaceuticalProduct, true) 3980 && compareDeep(packagedMedicinalProduct, o.packagedMedicinalProduct, true) 3981 && compareDeep(attachedDocument, o.attachedDocument, true) && compareDeep(masterFile, o.masterFile, true) 3982 && compareDeep(contact, o.contact, true) && compareDeep(clinicalTrial, o.clinicalTrial, true) 3983 && compareDeep(name, o.name, true) && compareDeep(crossReference, o.crossReference, true) 3984 && compareDeep(manufacturingBusinessOperation, o.manufacturingBusinessOperation, true) 3985 && compareDeep(specialDesignation, o.specialDesignation, true); 3986 } 3987 3988 @Override 3989 public boolean equalsShallow(Base other_) { 3990 if (!super.equalsShallow(other_)) 3991 return false; 3992 if (!(other_ instanceof MedicinalProduct)) 3993 return false; 3994 MedicinalProduct o = (MedicinalProduct) other_; 3995 return compareValues(specialMeasures, o.specialMeasures, true); 3996 } 3997 3998 public boolean isEmpty() { 3999 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, domain, 4000 combinedPharmaceuticalDoseForm, legalStatusOfSupply, additionalMonitoringIndicator, specialMeasures, 4001 paediatricUseIndicator, productClassification, marketingStatus, pharmaceuticalProduct, packagedMedicinalProduct, 4002 attachedDocument, masterFile, contact, clinicalTrial, name, crossReference, manufacturingBusinessOperation, 4003 specialDesignation); 4004 } 4005 4006 @Override 4007 public ResourceType getResourceType() { 4008 return ResourceType.MedicinalProduct; 4009 } 4010 4011 /** 4012 * Search parameter: <b>identifier</b> 4013 * <p> 4014 * Description: <b>Business identifier for this product. Could be an 4015 * MPID</b><br> 4016 * Type: <b>token</b><br> 4017 * Path: <b>MedicinalProduct.identifier</b><br> 4018 * </p> 4019 */ 4020 @SearchParamDefinition(name = "identifier", path = "MedicinalProduct.identifier", description = "Business identifier for this product. Could be an MPID", type = "token") 4021 public static final String SP_IDENTIFIER = "identifier"; 4022 /** 4023 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4024 * <p> 4025 * Description: <b>Business identifier for this product. Could be an 4026 * MPID</b><br> 4027 * Type: <b>token</b><br> 4028 * Path: <b>MedicinalProduct.identifier</b><br> 4029 * </p> 4030 */ 4031 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4032 SP_IDENTIFIER); 4033 4034 /** 4035 * Search parameter: <b>name</b> 4036 * <p> 4037 * Description: <b>The full product name</b><br> 4038 * Type: <b>string</b><br> 4039 * Path: <b>MedicinalProduct.name.productName</b><br> 4040 * </p> 4041 */ 4042 @SearchParamDefinition(name = "name", path = "MedicinalProduct.name.productName", description = "The full product name", type = "string") 4043 public static final String SP_NAME = "name"; 4044 /** 4045 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4046 * <p> 4047 * Description: <b>The full product name</b><br> 4048 * Type: <b>string</b><br> 4049 * Path: <b>MedicinalProduct.name.productName</b><br> 4050 * </p> 4051 */ 4052 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4053 SP_NAME); 4054 4055 /** 4056 * Search parameter: <b>name-language</b> 4057 * <p> 4058 * Description: <b>Language code for this name</b><br> 4059 * Type: <b>token</b><br> 4060 * Path: <b>MedicinalProduct.name.countryLanguage.language</b><br> 4061 * </p> 4062 */ 4063 @SearchParamDefinition(name = "name-language", path = "MedicinalProduct.name.countryLanguage.language", description = "Language code for this name", type = "token") 4064 public static final String SP_NAME_LANGUAGE = "name-language"; 4065 /** 4066 * <b>Fluent Client</b> search parameter constant for <b>name-language</b> 4067 * <p> 4068 * Description: <b>Language code for this name</b><br> 4069 * Type: <b>token</b><br> 4070 * Path: <b>MedicinalProduct.name.countryLanguage.language</b><br> 4071 * </p> 4072 */ 4073 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NAME_LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4074 SP_NAME_LANGUAGE); 4075 4076}