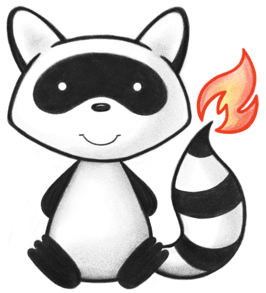
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The regulatory authorization of a medicinal product. 048 */ 049@ResourceDef(name = "MedicinalProductAuthorization", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductAuthorization") 050public class MedicinalProductAuthorization extends DomainResource { 051 052 @Block() 053 public static class MedicinalProductAuthorizationJurisdictionalAuthorizationComponent extends BackboneElement 054 implements IBaseBackboneElement { 055 /** 056 * The assigned number for the marketing authorization. 057 */ 058 @Child(name = "identifier", type = { 059 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 060 @Description(shortDefinition = "The assigned number for the marketing authorization", formalDefinition = "The assigned number for the marketing authorization.") 061 protected List<Identifier> identifier; 062 063 /** 064 * Country of authorization. 065 */ 066 @Child(name = "country", type = { 067 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "Country of authorization", formalDefinition = "Country of authorization.") 069 protected CodeableConcept country; 070 071 /** 072 * Jurisdiction within a country. 073 */ 074 @Child(name = "jurisdiction", type = { 075 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 076 @Description(shortDefinition = "Jurisdiction within a country", formalDefinition = "Jurisdiction within a country.") 077 protected List<CodeableConcept> jurisdiction; 078 079 /** 080 * The legal status of supply in a jurisdiction or region. 081 */ 082 @Child(name = "legalStatusOfSupply", type = { 083 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 084 @Description(shortDefinition = "The legal status of supply in a jurisdiction or region", formalDefinition = "The legal status of supply in a jurisdiction or region.") 085 protected CodeableConcept legalStatusOfSupply; 086 087 /** 088 * The start and expected end date of the authorization. 089 */ 090 @Child(name = "validityPeriod", type = { 091 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 092 @Description(shortDefinition = "The start and expected end date of the authorization", formalDefinition = "The start and expected end date of the authorization.") 093 protected Period validityPeriod; 094 095 private static final long serialVersionUID = -1893307291L; 096 097 /** 098 * Constructor 099 */ 100 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent() { 101 super(); 102 } 103 104 /** 105 * @return {@link #identifier} (The assigned number for the marketing 106 * authorization.) 107 */ 108 public List<Identifier> getIdentifier() { 109 if (this.identifier == null) 110 this.identifier = new ArrayList<Identifier>(); 111 return this.identifier; 112 } 113 114 /** 115 * @return Returns a reference to <code>this</code> for easy method chaining 116 */ 117 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent setIdentifier( 118 List<Identifier> theIdentifier) { 119 this.identifier = theIdentifier; 120 return this; 121 } 122 123 public boolean hasIdentifier() { 124 if (this.identifier == null) 125 return false; 126 for (Identifier item : this.identifier) 127 if (!item.isEmpty()) 128 return true; 129 return false; 130 } 131 132 public Identifier addIdentifier() { // 3 133 Identifier t = new Identifier(); 134 if (this.identifier == null) 135 this.identifier = new ArrayList<Identifier>(); 136 this.identifier.add(t); 137 return t; 138 } 139 140 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent addIdentifier(Identifier t) { // 3 141 if (t == null) 142 return this; 143 if (this.identifier == null) 144 this.identifier = new ArrayList<Identifier>(); 145 this.identifier.add(t); 146 return this; 147 } 148 149 /** 150 * @return The first repetition of repeating field {@link #identifier}, creating 151 * it if it does not already exist 152 */ 153 public Identifier getIdentifierFirstRep() { 154 if (getIdentifier().isEmpty()) { 155 addIdentifier(); 156 } 157 return getIdentifier().get(0); 158 } 159 160 /** 161 * @return {@link #country} (Country of authorization.) 162 */ 163 public CodeableConcept getCountry() { 164 if (this.country == null) 165 if (Configuration.errorOnAutoCreate()) 166 throw new Error( 167 "Attempt to auto-create MedicinalProductAuthorizationJurisdictionalAuthorizationComponent.country"); 168 else if (Configuration.doAutoCreate()) 169 this.country = new CodeableConcept(); // cc 170 return this.country; 171 } 172 173 public boolean hasCountry() { 174 return this.country != null && !this.country.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #country} (Country of authorization.) 179 */ 180 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent setCountry(CodeableConcept value) { 181 this.country = value; 182 return this; 183 } 184 185 /** 186 * @return {@link #jurisdiction} (Jurisdiction within a country.) 187 */ 188 public List<CodeableConcept> getJurisdiction() { 189 if (this.jurisdiction == null) 190 this.jurisdiction = new ArrayList<CodeableConcept>(); 191 return this.jurisdiction; 192 } 193 194 /** 195 * @return Returns a reference to <code>this</code> for easy method chaining 196 */ 197 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent setJurisdiction( 198 List<CodeableConcept> theJurisdiction) { 199 this.jurisdiction = theJurisdiction; 200 return this; 201 } 202 203 public boolean hasJurisdiction() { 204 if (this.jurisdiction == null) 205 return false; 206 for (CodeableConcept item : this.jurisdiction) 207 if (!item.isEmpty()) 208 return true; 209 return false; 210 } 211 212 public CodeableConcept addJurisdiction() { // 3 213 CodeableConcept t = new CodeableConcept(); 214 if (this.jurisdiction == null) 215 this.jurisdiction = new ArrayList<CodeableConcept>(); 216 this.jurisdiction.add(t); 217 return t; 218 } 219 220 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent addJurisdiction(CodeableConcept t) { // 3 221 if (t == null) 222 return this; 223 if (this.jurisdiction == null) 224 this.jurisdiction = new ArrayList<CodeableConcept>(); 225 this.jurisdiction.add(t); 226 return this; 227 } 228 229 /** 230 * @return The first repetition of repeating field {@link #jurisdiction}, 231 * creating it if it does not already exist 232 */ 233 public CodeableConcept getJurisdictionFirstRep() { 234 if (getJurisdiction().isEmpty()) { 235 addJurisdiction(); 236 } 237 return getJurisdiction().get(0); 238 } 239 240 /** 241 * @return {@link #legalStatusOfSupply} (The legal status of supply in a 242 * jurisdiction or region.) 243 */ 244 public CodeableConcept getLegalStatusOfSupply() { 245 if (this.legalStatusOfSupply == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error( 248 "Attempt to auto-create MedicinalProductAuthorizationJurisdictionalAuthorizationComponent.legalStatusOfSupply"); 249 else if (Configuration.doAutoCreate()) 250 this.legalStatusOfSupply = new CodeableConcept(); // cc 251 return this.legalStatusOfSupply; 252 } 253 254 public boolean hasLegalStatusOfSupply() { 255 return this.legalStatusOfSupply != null && !this.legalStatusOfSupply.isEmpty(); 256 } 257 258 /** 259 * @param value {@link #legalStatusOfSupply} (The legal status of supply in a 260 * jurisdiction or region.) 261 */ 262 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent setLegalStatusOfSupply( 263 CodeableConcept value) { 264 this.legalStatusOfSupply = value; 265 return this; 266 } 267 268 /** 269 * @return {@link #validityPeriod} (The start and expected end date of the 270 * authorization.) 271 */ 272 public Period getValidityPeriod() { 273 if (this.validityPeriod == null) 274 if (Configuration.errorOnAutoCreate()) 275 throw new Error( 276 "Attempt to auto-create MedicinalProductAuthorizationJurisdictionalAuthorizationComponent.validityPeriod"); 277 else if (Configuration.doAutoCreate()) 278 this.validityPeriod = new Period(); // cc 279 return this.validityPeriod; 280 } 281 282 public boolean hasValidityPeriod() { 283 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #validityPeriod} (The start and expected end date of the 288 * authorization.) 289 */ 290 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent setValidityPeriod(Period value) { 291 this.validityPeriod = value; 292 return this; 293 } 294 295 protected void listChildren(List<Property> children) { 296 super.listChildren(children); 297 children.add(new Property("identifier", "Identifier", "The assigned number for the marketing authorization.", 0, 298 java.lang.Integer.MAX_VALUE, identifier)); 299 children.add(new Property("country", "CodeableConcept", "Country of authorization.", 0, 1, country)); 300 children.add(new Property("jurisdiction", "CodeableConcept", "Jurisdiction within a country.", 0, 301 java.lang.Integer.MAX_VALUE, jurisdiction)); 302 children.add(new Property("legalStatusOfSupply", "CodeableConcept", 303 "The legal status of supply in a jurisdiction or region.", 0, 1, legalStatusOfSupply)); 304 children.add(new Property("validityPeriod", "Period", "The start and expected end date of the authorization.", 0, 305 1, validityPeriod)); 306 } 307 308 @Override 309 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 310 switch (_hash) { 311 case -1618432855: 312 /* identifier */ return new Property("identifier", "Identifier", 313 "The assigned number for the marketing authorization.", 0, java.lang.Integer.MAX_VALUE, identifier); 314 case 957831062: 315 /* country */ return new Property("country", "CodeableConcept", "Country of authorization.", 0, 1, country); 316 case -507075711: 317 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", "Jurisdiction within a country.", 0, 318 java.lang.Integer.MAX_VALUE, jurisdiction); 319 case -844874031: 320 /* legalStatusOfSupply */ return new Property("legalStatusOfSupply", "CodeableConcept", 321 "The legal status of supply in a jurisdiction or region.", 0, 1, legalStatusOfSupply); 322 case -1434195053: 323 /* validityPeriod */ return new Property("validityPeriod", "Period", 324 "The start and expected end date of the authorization.", 0, 1, validityPeriod); 325 default: 326 return super.getNamedProperty(_hash, _name, _checkValid); 327 } 328 329 } 330 331 @Override 332 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 333 switch (hash) { 334 case -1618432855: 335 /* identifier */ return this.identifier == null ? new Base[0] 336 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 337 case 957831062: 338 /* country */ return this.country == null ? new Base[0] : new Base[] { this.country }; // CodeableConcept 339 case -507075711: 340 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 341 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 342 case -844874031: 343 /* legalStatusOfSupply */ return this.legalStatusOfSupply == null ? new Base[0] 344 : new Base[] { this.legalStatusOfSupply }; // CodeableConcept 345 case -1434195053: 346 /* validityPeriod */ return this.validityPeriod == null ? new Base[0] : new Base[] { this.validityPeriod }; // Period 347 default: 348 return super.getProperty(hash, name, checkValid); 349 } 350 351 } 352 353 @Override 354 public Base setProperty(int hash, String name, Base value) throws FHIRException { 355 switch (hash) { 356 case -1618432855: // identifier 357 this.getIdentifier().add(castToIdentifier(value)); // Identifier 358 return value; 359 case 957831062: // country 360 this.country = castToCodeableConcept(value); // CodeableConcept 361 return value; 362 case -507075711: // jurisdiction 363 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 364 return value; 365 case -844874031: // legalStatusOfSupply 366 this.legalStatusOfSupply = castToCodeableConcept(value); // CodeableConcept 367 return value; 368 case -1434195053: // validityPeriod 369 this.validityPeriod = castToPeriod(value); // Period 370 return value; 371 default: 372 return super.setProperty(hash, name, value); 373 } 374 375 } 376 377 @Override 378 public Base setProperty(String name, Base value) throws FHIRException { 379 if (name.equals("identifier")) { 380 this.getIdentifier().add(castToIdentifier(value)); 381 } else if (name.equals("country")) { 382 this.country = castToCodeableConcept(value); // CodeableConcept 383 } else if (name.equals("jurisdiction")) { 384 this.getJurisdiction().add(castToCodeableConcept(value)); 385 } else if (name.equals("legalStatusOfSupply")) { 386 this.legalStatusOfSupply = castToCodeableConcept(value); // CodeableConcept 387 } else if (name.equals("validityPeriod")) { 388 this.validityPeriod = castToPeriod(value); // Period 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public void removeChild(String name, Base value) throws FHIRException { 396 if (name.equals("identifier")) { 397 this.getIdentifier().remove(castToIdentifier(value)); 398 } else if (name.equals("country")) { 399 this.country = null; 400 } else if (name.equals("jurisdiction")) { 401 this.getJurisdiction().remove(castToCodeableConcept(value)); 402 } else if (name.equals("legalStatusOfSupply")) { 403 this.legalStatusOfSupply = null; 404 } else if (name.equals("validityPeriod")) { 405 this.validityPeriod = null; 406 } else 407 super.removeChild(name, value); 408 409 } 410 411 @Override 412 public Base makeProperty(int hash, String name) throws FHIRException { 413 switch (hash) { 414 case -1618432855: 415 return addIdentifier(); 416 case 957831062: 417 return getCountry(); 418 case -507075711: 419 return addJurisdiction(); 420 case -844874031: 421 return getLegalStatusOfSupply(); 422 case -1434195053: 423 return getValidityPeriod(); 424 default: 425 return super.makeProperty(hash, name); 426 } 427 428 } 429 430 @Override 431 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 432 switch (hash) { 433 case -1618432855: 434 /* identifier */ return new String[] { "Identifier" }; 435 case 957831062: 436 /* country */ return new String[] { "CodeableConcept" }; 437 case -507075711: 438 /* jurisdiction */ return new String[] { "CodeableConcept" }; 439 case -844874031: 440 /* legalStatusOfSupply */ return new String[] { "CodeableConcept" }; 441 case -1434195053: 442 /* validityPeriod */ return new String[] { "Period" }; 443 default: 444 return super.getTypesForProperty(hash, name); 445 } 446 447 } 448 449 @Override 450 public Base addChild(String name) throws FHIRException { 451 if (name.equals("identifier")) { 452 return addIdentifier(); 453 } else if (name.equals("country")) { 454 this.country = new CodeableConcept(); 455 return this.country; 456 } else if (name.equals("jurisdiction")) { 457 return addJurisdiction(); 458 } else if (name.equals("legalStatusOfSupply")) { 459 this.legalStatusOfSupply = new CodeableConcept(); 460 return this.legalStatusOfSupply; 461 } else if (name.equals("validityPeriod")) { 462 this.validityPeriod = new Period(); 463 return this.validityPeriod; 464 } else 465 return super.addChild(name); 466 } 467 468 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent copy() { 469 MedicinalProductAuthorizationJurisdictionalAuthorizationComponent dst = new MedicinalProductAuthorizationJurisdictionalAuthorizationComponent(); 470 copyValues(dst); 471 return dst; 472 } 473 474 public void copyValues(MedicinalProductAuthorizationJurisdictionalAuthorizationComponent dst) { 475 super.copyValues(dst); 476 if (identifier != null) { 477 dst.identifier = new ArrayList<Identifier>(); 478 for (Identifier i : identifier) 479 dst.identifier.add(i.copy()); 480 } 481 ; 482 dst.country = country == null ? null : country.copy(); 483 if (jurisdiction != null) { 484 dst.jurisdiction = new ArrayList<CodeableConcept>(); 485 for (CodeableConcept i : jurisdiction) 486 dst.jurisdiction.add(i.copy()); 487 } 488 ; 489 dst.legalStatusOfSupply = legalStatusOfSupply == null ? null : legalStatusOfSupply.copy(); 490 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 491 } 492 493 @Override 494 public boolean equalsDeep(Base other_) { 495 if (!super.equalsDeep(other_)) 496 return false; 497 if (!(other_ instanceof MedicinalProductAuthorizationJurisdictionalAuthorizationComponent)) 498 return false; 499 MedicinalProductAuthorizationJurisdictionalAuthorizationComponent o = (MedicinalProductAuthorizationJurisdictionalAuthorizationComponent) other_; 500 return compareDeep(identifier, o.identifier, true) && compareDeep(country, o.country, true) 501 && compareDeep(jurisdiction, o.jurisdiction, true) 502 && compareDeep(legalStatusOfSupply, o.legalStatusOfSupply, true) 503 && compareDeep(validityPeriod, o.validityPeriod, true); 504 } 505 506 @Override 507 public boolean equalsShallow(Base other_) { 508 if (!super.equalsShallow(other_)) 509 return false; 510 if (!(other_ instanceof MedicinalProductAuthorizationJurisdictionalAuthorizationComponent)) 511 return false; 512 MedicinalProductAuthorizationJurisdictionalAuthorizationComponent o = (MedicinalProductAuthorizationJurisdictionalAuthorizationComponent) other_; 513 return true; 514 } 515 516 public boolean isEmpty() { 517 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, country, jurisdiction, 518 legalStatusOfSupply, validityPeriod); 519 } 520 521 public String fhirType() { 522 return "MedicinalProductAuthorization.jurisdictionalAuthorization"; 523 524 } 525 526 } 527 528 @Block() 529 public static class MedicinalProductAuthorizationProcedureComponent extends BackboneElement 530 implements IBaseBackboneElement { 531 /** 532 * Identifier for this procedure. 533 */ 534 @Child(name = "identifier", type = { 535 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 536 @Description(shortDefinition = "Identifier for this procedure", formalDefinition = "Identifier for this procedure.") 537 protected Identifier identifier; 538 539 /** 540 * Type of procedure. 541 */ 542 @Child(name = "type", type = { 543 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 544 @Description(shortDefinition = "Type of procedure", formalDefinition = "Type of procedure.") 545 protected CodeableConcept type; 546 547 /** 548 * Date of procedure. 549 */ 550 @Child(name = "date", type = { Period.class, 551 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 552 @Description(shortDefinition = "Date of procedure", formalDefinition = "Date of procedure.") 553 protected Type date; 554 555 /** 556 * Applcations submitted to obtain a marketing authorization. 557 */ 558 @Child(name = "application", type = { 559 MedicinalProductAuthorizationProcedureComponent.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 560 @Description(shortDefinition = "Applcations submitted to obtain a marketing authorization", formalDefinition = "Applcations submitted to obtain a marketing authorization.") 561 protected List<MedicinalProductAuthorizationProcedureComponent> application; 562 563 private static final long serialVersionUID = 930236001L; 564 565 /** 566 * Constructor 567 */ 568 public MedicinalProductAuthorizationProcedureComponent() { 569 super(); 570 } 571 572 /** 573 * Constructor 574 */ 575 public MedicinalProductAuthorizationProcedureComponent(CodeableConcept type) { 576 super(); 577 this.type = type; 578 } 579 580 /** 581 * @return {@link #identifier} (Identifier for this procedure.) 582 */ 583 public Identifier getIdentifier() { 584 if (this.identifier == null) 585 if (Configuration.errorOnAutoCreate()) 586 throw new Error("Attempt to auto-create MedicinalProductAuthorizationProcedureComponent.identifier"); 587 else if (Configuration.doAutoCreate()) 588 this.identifier = new Identifier(); // cc 589 return this.identifier; 590 } 591 592 public boolean hasIdentifier() { 593 return this.identifier != null && !this.identifier.isEmpty(); 594 } 595 596 /** 597 * @param value {@link #identifier} (Identifier for this procedure.) 598 */ 599 public MedicinalProductAuthorizationProcedureComponent setIdentifier(Identifier value) { 600 this.identifier = value; 601 return this; 602 } 603 604 /** 605 * @return {@link #type} (Type of procedure.) 606 */ 607 public CodeableConcept getType() { 608 if (this.type == null) 609 if (Configuration.errorOnAutoCreate()) 610 throw new Error("Attempt to auto-create MedicinalProductAuthorizationProcedureComponent.type"); 611 else if (Configuration.doAutoCreate()) 612 this.type = new CodeableConcept(); // cc 613 return this.type; 614 } 615 616 public boolean hasType() { 617 return this.type != null && !this.type.isEmpty(); 618 } 619 620 /** 621 * @param value {@link #type} (Type of procedure.) 622 */ 623 public MedicinalProductAuthorizationProcedureComponent setType(CodeableConcept value) { 624 this.type = value; 625 return this; 626 } 627 628 /** 629 * @return {@link #date} (Date of procedure.) 630 */ 631 public Type getDate() { 632 return this.date; 633 } 634 635 /** 636 * @return {@link #date} (Date of procedure.) 637 */ 638 public Period getDatePeriod() throws FHIRException { 639 if (this.date == null) 640 this.date = new Period(); 641 if (!(this.date instanceof Period)) 642 throw new FHIRException( 643 "Type mismatch: the type Period was expected, but " + this.date.getClass().getName() + " was encountered"); 644 return (Period) this.date; 645 } 646 647 public boolean hasDatePeriod() { 648 return this != null && this.date instanceof Period; 649 } 650 651 /** 652 * @return {@link #date} (Date of procedure.) 653 */ 654 public DateTimeType getDateDateTimeType() throws FHIRException { 655 if (this.date == null) 656 this.date = new DateTimeType(); 657 if (!(this.date instanceof DateTimeType)) 658 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 659 + this.date.getClass().getName() + " was encountered"); 660 return (DateTimeType) this.date; 661 } 662 663 public boolean hasDateDateTimeType() { 664 return this != null && this.date instanceof DateTimeType; 665 } 666 667 public boolean hasDate() { 668 return this.date != null && !this.date.isEmpty(); 669 } 670 671 /** 672 * @param value {@link #date} (Date of procedure.) 673 */ 674 public MedicinalProductAuthorizationProcedureComponent setDate(Type value) { 675 if (value != null && !(value instanceof Period || value instanceof DateTimeType)) 676 throw new Error("Not the right type for MedicinalProductAuthorization.procedure.date[x]: " + value.fhirType()); 677 this.date = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #application} (Applcations submitted to obtain a marketing 683 * authorization.) 684 */ 685 public List<MedicinalProductAuthorizationProcedureComponent> getApplication() { 686 if (this.application == null) 687 this.application = new ArrayList<MedicinalProductAuthorizationProcedureComponent>(); 688 return this.application; 689 } 690 691 /** 692 * @return Returns a reference to <code>this</code> for easy method chaining 693 */ 694 public MedicinalProductAuthorizationProcedureComponent setApplication( 695 List<MedicinalProductAuthorizationProcedureComponent> theApplication) { 696 this.application = theApplication; 697 return this; 698 } 699 700 public boolean hasApplication() { 701 if (this.application == null) 702 return false; 703 for (MedicinalProductAuthorizationProcedureComponent item : this.application) 704 if (!item.isEmpty()) 705 return true; 706 return false; 707 } 708 709 public MedicinalProductAuthorizationProcedureComponent addApplication() { // 3 710 MedicinalProductAuthorizationProcedureComponent t = new MedicinalProductAuthorizationProcedureComponent(); 711 if (this.application == null) 712 this.application = new ArrayList<MedicinalProductAuthorizationProcedureComponent>(); 713 this.application.add(t); 714 return t; 715 } 716 717 public MedicinalProductAuthorizationProcedureComponent addApplication( 718 MedicinalProductAuthorizationProcedureComponent t) { // 3 719 if (t == null) 720 return this; 721 if (this.application == null) 722 this.application = new ArrayList<MedicinalProductAuthorizationProcedureComponent>(); 723 this.application.add(t); 724 return this; 725 } 726 727 /** 728 * @return The first repetition of repeating field {@link #application}, 729 * creating it if it does not already exist 730 */ 731 public MedicinalProductAuthorizationProcedureComponent getApplicationFirstRep() { 732 if (getApplication().isEmpty()) { 733 addApplication(); 734 } 735 return getApplication().get(0); 736 } 737 738 protected void listChildren(List<Property> children) { 739 super.listChildren(children); 740 children.add(new Property("identifier", "Identifier", "Identifier for this procedure.", 0, 1, identifier)); 741 children.add(new Property("type", "CodeableConcept", "Type of procedure.", 0, 1, type)); 742 children.add(new Property("date[x]", "Period|dateTime", "Date of procedure.", 0, 1, date)); 743 children.add(new Property("application", "@MedicinalProductAuthorization.procedure", 744 "Applcations submitted to obtain a marketing authorization.", 0, java.lang.Integer.MAX_VALUE, application)); 745 } 746 747 @Override 748 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 749 switch (_hash) { 750 case -1618432855: 751 /* identifier */ return new Property("identifier", "Identifier", "Identifier for this procedure.", 0, 1, 752 identifier); 753 case 3575610: 754 /* type */ return new Property("type", "CodeableConcept", "Type of procedure.", 0, 1, type); 755 case 1443311122: 756 /* date[x] */ return new Property("date[x]", "Period|dateTime", "Date of procedure.", 0, 1, date); 757 case 3076014: 758 /* date */ return new Property("date[x]", "Period|dateTime", "Date of procedure.", 0, 1, date); 759 case 432297743: 760 /* datePeriod */ return new Property("date[x]", "Period|dateTime", "Date of procedure.", 0, 1, date); 761 case 185136489: 762 /* dateDateTime */ return new Property("date[x]", "Period|dateTime", "Date of procedure.", 0, 1, date); 763 case 1554253136: 764 /* application */ return new Property("application", "@MedicinalProductAuthorization.procedure", 765 "Applcations submitted to obtain a marketing authorization.", 0, java.lang.Integer.MAX_VALUE, application); 766 default: 767 return super.getNamedProperty(_hash, _name, _checkValid); 768 } 769 770 } 771 772 @Override 773 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 774 switch (hash) { 775 case -1618432855: 776 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 777 case 3575610: 778 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 779 case 3076014: 780 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // Type 781 case 1554253136: 782 /* application */ return this.application == null ? new Base[0] 783 : this.application.toArray(new Base[this.application.size()]); // MedicinalProductAuthorizationProcedureComponent 784 default: 785 return super.getProperty(hash, name, checkValid); 786 } 787 788 } 789 790 @Override 791 public Base setProperty(int hash, String name, Base value) throws FHIRException { 792 switch (hash) { 793 case -1618432855: // identifier 794 this.identifier = castToIdentifier(value); // Identifier 795 return value; 796 case 3575610: // type 797 this.type = castToCodeableConcept(value); // CodeableConcept 798 return value; 799 case 3076014: // date 800 this.date = castToType(value); // Type 801 return value; 802 case 1554253136: // application 803 this.getApplication().add((MedicinalProductAuthorizationProcedureComponent) value); // MedicinalProductAuthorizationProcedureComponent 804 return value; 805 default: 806 return super.setProperty(hash, name, value); 807 } 808 809 } 810 811 @Override 812 public Base setProperty(String name, Base value) throws FHIRException { 813 if (name.equals("identifier")) { 814 this.identifier = castToIdentifier(value); // Identifier 815 } else if (name.equals("type")) { 816 this.type = castToCodeableConcept(value); // CodeableConcept 817 } else if (name.equals("date[x]")) { 818 this.date = castToType(value); // Type 819 } else if (name.equals("application")) { 820 this.getApplication().add((MedicinalProductAuthorizationProcedureComponent) value); 821 } else 822 return super.setProperty(name, value); 823 return value; 824 } 825 826 @Override 827 public void removeChild(String name, Base value) throws FHIRException { 828 if (name.equals("identifier")) { 829 this.identifier = null; 830 } else if (name.equals("type")) { 831 this.type = null; 832 } else if (name.equals("date[x]")) { 833 this.date = null; 834 } else if (name.equals("application")) { 835 this.getApplication().remove((MedicinalProductAuthorizationProcedureComponent) value); 836 } else 837 super.removeChild(name, value); 838 839 } 840 841 @Override 842 public Base makeProperty(int hash, String name) throws FHIRException { 843 switch (hash) { 844 case -1618432855: 845 return getIdentifier(); 846 case 3575610: 847 return getType(); 848 case 1443311122: 849 return getDate(); 850 case 3076014: 851 return getDate(); 852 case 1554253136: 853 return addApplication(); 854 default: 855 return super.makeProperty(hash, name); 856 } 857 858 } 859 860 @Override 861 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 862 switch (hash) { 863 case -1618432855: 864 /* identifier */ return new String[] { "Identifier" }; 865 case 3575610: 866 /* type */ return new String[] { "CodeableConcept" }; 867 case 3076014: 868 /* date */ return new String[] { "Period", "dateTime" }; 869 case 1554253136: 870 /* application */ return new String[] { "@MedicinalProductAuthorization.procedure" }; 871 default: 872 return super.getTypesForProperty(hash, name); 873 } 874 875 } 876 877 @Override 878 public Base addChild(String name) throws FHIRException { 879 if (name.equals("identifier")) { 880 this.identifier = new Identifier(); 881 return this.identifier; 882 } else if (name.equals("type")) { 883 this.type = new CodeableConcept(); 884 return this.type; 885 } else if (name.equals("datePeriod")) { 886 this.date = new Period(); 887 return this.date; 888 } else if (name.equals("dateDateTime")) { 889 this.date = new DateTimeType(); 890 return this.date; 891 } else if (name.equals("application")) { 892 return addApplication(); 893 } else 894 return super.addChild(name); 895 } 896 897 public MedicinalProductAuthorizationProcedureComponent copy() { 898 MedicinalProductAuthorizationProcedureComponent dst = new MedicinalProductAuthorizationProcedureComponent(); 899 copyValues(dst); 900 return dst; 901 } 902 903 public void copyValues(MedicinalProductAuthorizationProcedureComponent dst) { 904 super.copyValues(dst); 905 dst.identifier = identifier == null ? null : identifier.copy(); 906 dst.type = type == null ? null : type.copy(); 907 dst.date = date == null ? null : date.copy(); 908 if (application != null) { 909 dst.application = new ArrayList<MedicinalProductAuthorizationProcedureComponent>(); 910 for (MedicinalProductAuthorizationProcedureComponent i : application) 911 dst.application.add(i.copy()); 912 } 913 ; 914 } 915 916 @Override 917 public boolean equalsDeep(Base other_) { 918 if (!super.equalsDeep(other_)) 919 return false; 920 if (!(other_ instanceof MedicinalProductAuthorizationProcedureComponent)) 921 return false; 922 MedicinalProductAuthorizationProcedureComponent o = (MedicinalProductAuthorizationProcedureComponent) other_; 923 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 924 && compareDeep(date, o.date, true) && compareDeep(application, o.application, true); 925 } 926 927 @Override 928 public boolean equalsShallow(Base other_) { 929 if (!super.equalsShallow(other_)) 930 return false; 931 if (!(other_ instanceof MedicinalProductAuthorizationProcedureComponent)) 932 return false; 933 MedicinalProductAuthorizationProcedureComponent o = (MedicinalProductAuthorizationProcedureComponent) other_; 934 return true; 935 } 936 937 public boolean isEmpty() { 938 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, date, application); 939 } 940 941 public String fhirType() { 942 return "MedicinalProductAuthorization.procedure"; 943 944 } 945 946 } 947 948 /** 949 * Business identifier for the marketing authorization, as assigned by a 950 * regulator. 951 */ 952 @Child(name = "identifier", type = { 953 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 954 @Description(shortDefinition = "Business identifier for the marketing authorization, as assigned by a regulator", formalDefinition = "Business identifier for the marketing authorization, as assigned by a regulator.") 955 protected List<Identifier> identifier; 956 957 /** 958 * The medicinal product that is being authorized. 959 */ 960 @Child(name = "subject", type = { MedicinalProduct.class, 961 MedicinalProductPackaged.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 962 @Description(shortDefinition = "The medicinal product that is being authorized", formalDefinition = "The medicinal product that is being authorized.") 963 protected Reference subject; 964 965 /** 966 * The actual object that is the target of the reference (The medicinal product 967 * that is being authorized.) 968 */ 969 protected Resource subjectTarget; 970 971 /** 972 * The country in which the marketing authorization has been granted. 973 */ 974 @Child(name = "country", type = { 975 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 976 @Description(shortDefinition = "The country in which the marketing authorization has been granted", formalDefinition = "The country in which the marketing authorization has been granted.") 977 protected List<CodeableConcept> country; 978 979 /** 980 * Jurisdiction within a country. 981 */ 982 @Child(name = "jurisdiction", type = { 983 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 984 @Description(shortDefinition = "Jurisdiction within a country", formalDefinition = "Jurisdiction within a country.") 985 protected List<CodeableConcept> jurisdiction; 986 987 /** 988 * The status of the marketing authorization. 989 */ 990 @Child(name = "status", type = { 991 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 992 @Description(shortDefinition = "The status of the marketing authorization", formalDefinition = "The status of the marketing authorization.") 993 protected CodeableConcept status; 994 995 /** 996 * The date at which the given status has become applicable. 997 */ 998 @Child(name = "statusDate", type = { 999 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1000 @Description(shortDefinition = "The date at which the given status has become applicable", formalDefinition = "The date at which the given status has become applicable.") 1001 protected DateTimeType statusDate; 1002 1003 /** 1004 * The date when a suspended the marketing or the marketing authorization of the 1005 * product is anticipated to be restored. 1006 */ 1007 @Child(name = "restoreDate", type = { 1008 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1009 @Description(shortDefinition = "The date when a suspended the marketing or the marketing authorization of the product is anticipated to be restored", formalDefinition = "The date when a suspended the marketing or the marketing authorization of the product is anticipated to be restored.") 1010 protected DateTimeType restoreDate; 1011 1012 /** 1013 * The beginning of the time period in which the marketing authorization is in 1014 * the specific status shall be specified A complete date consisting of day, 1015 * month and year shall be specified using the ISO 8601 date format. 1016 */ 1017 @Child(name = "validityPeriod", type = { 1018 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1019 @Description(shortDefinition = "The beginning of the time period in which the marketing authorization is in the specific status shall be specified A complete date consisting of day, month and year shall be specified using the ISO 8601 date format", formalDefinition = "The beginning of the time period in which the marketing authorization is in the specific status shall be specified A complete date consisting of day, month and year shall be specified using the ISO 8601 date format.") 1020 protected Period validityPeriod; 1021 1022 /** 1023 * A period of time after authorization before generic product applicatiosn can 1024 * be submitted. 1025 */ 1026 @Child(name = "dataExclusivityPeriod", type = { 1027 Period.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1028 @Description(shortDefinition = "A period of time after authorization before generic product applicatiosn can be submitted", formalDefinition = "A period of time after authorization before generic product applicatiosn can be submitted.") 1029 protected Period dataExclusivityPeriod; 1030 1031 /** 1032 * The date when the first authorization was granted by a Medicines Regulatory 1033 * Agency. 1034 */ 1035 @Child(name = "dateOfFirstAuthorization", type = { 1036 DateTimeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1037 @Description(shortDefinition = "The date when the first authorization was granted by a Medicines Regulatory Agency", formalDefinition = "The date when the first authorization was granted by a Medicines Regulatory Agency.") 1038 protected DateTimeType dateOfFirstAuthorization; 1039 1040 /** 1041 * Date of first marketing authorization for a company's new medicinal product 1042 * in any country in the World. 1043 */ 1044 @Child(name = "internationalBirthDate", type = { 1045 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1046 @Description(shortDefinition = "Date of first marketing authorization for a company's new medicinal product in any country in the World", formalDefinition = "Date of first marketing authorization for a company's new medicinal product in any country in the World.") 1047 protected DateTimeType internationalBirthDate; 1048 1049 /** 1050 * The legal framework against which this authorization is granted. 1051 */ 1052 @Child(name = "legalBasis", type = { 1053 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1054 @Description(shortDefinition = "The legal framework against which this authorization is granted", formalDefinition = "The legal framework against which this authorization is granted.") 1055 protected CodeableConcept legalBasis; 1056 1057 /** 1058 * Authorization in areas within a country. 1059 */ 1060 @Child(name = "jurisdictionalAuthorization", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1061 @Description(shortDefinition = "Authorization in areas within a country", formalDefinition = "Authorization in areas within a country.") 1062 protected List<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent> jurisdictionalAuthorization; 1063 1064 /** 1065 * Marketing Authorization Holder. 1066 */ 1067 @Child(name = "holder", type = { Organization.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1068 @Description(shortDefinition = "Marketing Authorization Holder", formalDefinition = "Marketing Authorization Holder.") 1069 protected Reference holder; 1070 1071 /** 1072 * The actual object that is the target of the reference (Marketing 1073 * Authorization Holder.) 1074 */ 1075 protected Organization holderTarget; 1076 1077 /** 1078 * Medicines Regulatory Agency. 1079 */ 1080 @Child(name = "regulator", type = { 1081 Organization.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1082 @Description(shortDefinition = "Medicines Regulatory Agency", formalDefinition = "Medicines Regulatory Agency.") 1083 protected Reference regulator; 1084 1085 /** 1086 * The actual object that is the target of the reference (Medicines Regulatory 1087 * Agency.) 1088 */ 1089 protected Organization regulatorTarget; 1090 1091 /** 1092 * The regulatory procedure for granting or amending a marketing authorization. 1093 */ 1094 @Child(name = "procedure", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 1095 @Description(shortDefinition = "The regulatory procedure for granting or amending a marketing authorization", formalDefinition = "The regulatory procedure for granting or amending a marketing authorization.") 1096 protected MedicinalProductAuthorizationProcedureComponent procedure; 1097 1098 private static final long serialVersionUID = 16249078L; 1099 1100 /** 1101 * Constructor 1102 */ 1103 public MedicinalProductAuthorization() { 1104 super(); 1105 } 1106 1107 /** 1108 * @return {@link #identifier} (Business identifier for the marketing 1109 * authorization, as assigned by a regulator.) 1110 */ 1111 public List<Identifier> getIdentifier() { 1112 if (this.identifier == null) 1113 this.identifier = new ArrayList<Identifier>(); 1114 return this.identifier; 1115 } 1116 1117 /** 1118 * @return Returns a reference to <code>this</code> for easy method chaining 1119 */ 1120 public MedicinalProductAuthorization setIdentifier(List<Identifier> theIdentifier) { 1121 this.identifier = theIdentifier; 1122 return this; 1123 } 1124 1125 public boolean hasIdentifier() { 1126 if (this.identifier == null) 1127 return false; 1128 for (Identifier item : this.identifier) 1129 if (!item.isEmpty()) 1130 return true; 1131 return false; 1132 } 1133 1134 public Identifier addIdentifier() { // 3 1135 Identifier t = new Identifier(); 1136 if (this.identifier == null) 1137 this.identifier = new ArrayList<Identifier>(); 1138 this.identifier.add(t); 1139 return t; 1140 } 1141 1142 public MedicinalProductAuthorization addIdentifier(Identifier t) { // 3 1143 if (t == null) 1144 return this; 1145 if (this.identifier == null) 1146 this.identifier = new ArrayList<Identifier>(); 1147 this.identifier.add(t); 1148 return this; 1149 } 1150 1151 /** 1152 * @return The first repetition of repeating field {@link #identifier}, creating 1153 * it if it does not already exist 1154 */ 1155 public Identifier getIdentifierFirstRep() { 1156 if (getIdentifier().isEmpty()) { 1157 addIdentifier(); 1158 } 1159 return getIdentifier().get(0); 1160 } 1161 1162 /** 1163 * @return {@link #subject} (The medicinal product that is being authorized.) 1164 */ 1165 public Reference getSubject() { 1166 if (this.subject == null) 1167 if (Configuration.errorOnAutoCreate()) 1168 throw new Error("Attempt to auto-create MedicinalProductAuthorization.subject"); 1169 else if (Configuration.doAutoCreate()) 1170 this.subject = new Reference(); // cc 1171 return this.subject; 1172 } 1173 1174 public boolean hasSubject() { 1175 return this.subject != null && !this.subject.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #subject} (The medicinal product that is being 1180 * authorized.) 1181 */ 1182 public MedicinalProductAuthorization setSubject(Reference value) { 1183 this.subject = value; 1184 return this; 1185 } 1186 1187 /** 1188 * @return {@link #subject} The actual object that is the target of the 1189 * reference. The reference library doesn't populate this, but you can 1190 * use it to hold the resource if you resolve it. (The medicinal product 1191 * that is being authorized.) 1192 */ 1193 public Resource getSubjectTarget() { 1194 return this.subjectTarget; 1195 } 1196 1197 /** 1198 * @param value {@link #subject} The actual object that is the target of the 1199 * reference. The reference library doesn't use these, but you can 1200 * use it to hold the resource if you resolve it. (The medicinal 1201 * product that is being authorized.) 1202 */ 1203 public MedicinalProductAuthorization setSubjectTarget(Resource value) { 1204 this.subjectTarget = value; 1205 return this; 1206 } 1207 1208 /** 1209 * @return {@link #country} (The country in which the marketing authorization 1210 * has been granted.) 1211 */ 1212 public List<CodeableConcept> getCountry() { 1213 if (this.country == null) 1214 this.country = new ArrayList<CodeableConcept>(); 1215 return this.country; 1216 } 1217 1218 /** 1219 * @return Returns a reference to <code>this</code> for easy method chaining 1220 */ 1221 public MedicinalProductAuthorization setCountry(List<CodeableConcept> theCountry) { 1222 this.country = theCountry; 1223 return this; 1224 } 1225 1226 public boolean hasCountry() { 1227 if (this.country == null) 1228 return false; 1229 for (CodeableConcept item : this.country) 1230 if (!item.isEmpty()) 1231 return true; 1232 return false; 1233 } 1234 1235 public CodeableConcept addCountry() { // 3 1236 CodeableConcept t = new CodeableConcept(); 1237 if (this.country == null) 1238 this.country = new ArrayList<CodeableConcept>(); 1239 this.country.add(t); 1240 return t; 1241 } 1242 1243 public MedicinalProductAuthorization addCountry(CodeableConcept t) { // 3 1244 if (t == null) 1245 return this; 1246 if (this.country == null) 1247 this.country = new ArrayList<CodeableConcept>(); 1248 this.country.add(t); 1249 return this; 1250 } 1251 1252 /** 1253 * @return The first repetition of repeating field {@link #country}, creating it 1254 * if it does not already exist 1255 */ 1256 public CodeableConcept getCountryFirstRep() { 1257 if (getCountry().isEmpty()) { 1258 addCountry(); 1259 } 1260 return getCountry().get(0); 1261 } 1262 1263 /** 1264 * @return {@link #jurisdiction} (Jurisdiction within a country.) 1265 */ 1266 public List<CodeableConcept> getJurisdiction() { 1267 if (this.jurisdiction == null) 1268 this.jurisdiction = new ArrayList<CodeableConcept>(); 1269 return this.jurisdiction; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public MedicinalProductAuthorization setJurisdiction(List<CodeableConcept> theJurisdiction) { 1276 this.jurisdiction = theJurisdiction; 1277 return this; 1278 } 1279 1280 public boolean hasJurisdiction() { 1281 if (this.jurisdiction == null) 1282 return false; 1283 for (CodeableConcept item : this.jurisdiction) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public CodeableConcept addJurisdiction() { // 3 1290 CodeableConcept t = new CodeableConcept(); 1291 if (this.jurisdiction == null) 1292 this.jurisdiction = new ArrayList<CodeableConcept>(); 1293 this.jurisdiction.add(t); 1294 return t; 1295 } 1296 1297 public MedicinalProductAuthorization addJurisdiction(CodeableConcept t) { // 3 1298 if (t == null) 1299 return this; 1300 if (this.jurisdiction == null) 1301 this.jurisdiction = new ArrayList<CodeableConcept>(); 1302 this.jurisdiction.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #jurisdiction}, 1308 * creating it if it does not already exist 1309 */ 1310 public CodeableConcept getJurisdictionFirstRep() { 1311 if (getJurisdiction().isEmpty()) { 1312 addJurisdiction(); 1313 } 1314 return getJurisdiction().get(0); 1315 } 1316 1317 /** 1318 * @return {@link #status} (The status of the marketing authorization.) 1319 */ 1320 public CodeableConcept getStatus() { 1321 if (this.status == null) 1322 if (Configuration.errorOnAutoCreate()) 1323 throw new Error("Attempt to auto-create MedicinalProductAuthorization.status"); 1324 else if (Configuration.doAutoCreate()) 1325 this.status = new CodeableConcept(); // cc 1326 return this.status; 1327 } 1328 1329 public boolean hasStatus() { 1330 return this.status != null && !this.status.isEmpty(); 1331 } 1332 1333 /** 1334 * @param value {@link #status} (The status of the marketing authorization.) 1335 */ 1336 public MedicinalProductAuthorization setStatus(CodeableConcept value) { 1337 this.status = value; 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #statusDate} (The date at which the given status has become 1343 * applicable.). This is the underlying object with id, value and 1344 * extensions. The accessor "getStatusDate" gives direct access to the 1345 * value 1346 */ 1347 public DateTimeType getStatusDateElement() { 1348 if (this.statusDate == null) 1349 if (Configuration.errorOnAutoCreate()) 1350 throw new Error("Attempt to auto-create MedicinalProductAuthorization.statusDate"); 1351 else if (Configuration.doAutoCreate()) 1352 this.statusDate = new DateTimeType(); // bb 1353 return this.statusDate; 1354 } 1355 1356 public boolean hasStatusDateElement() { 1357 return this.statusDate != null && !this.statusDate.isEmpty(); 1358 } 1359 1360 public boolean hasStatusDate() { 1361 return this.statusDate != null && !this.statusDate.isEmpty(); 1362 } 1363 1364 /** 1365 * @param value {@link #statusDate} (The date at which the given status has 1366 * become applicable.). This is the underlying object with id, 1367 * value and extensions. The accessor "getStatusDate" gives direct 1368 * access to the value 1369 */ 1370 public MedicinalProductAuthorization setStatusDateElement(DateTimeType value) { 1371 this.statusDate = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return The date at which the given status has become applicable. 1377 */ 1378 public Date getStatusDate() { 1379 return this.statusDate == null ? null : this.statusDate.getValue(); 1380 } 1381 1382 /** 1383 * @param value The date at which the given status has become applicable. 1384 */ 1385 public MedicinalProductAuthorization setStatusDate(Date value) { 1386 if (value == null) 1387 this.statusDate = null; 1388 else { 1389 if (this.statusDate == null) 1390 this.statusDate = new DateTimeType(); 1391 this.statusDate.setValue(value); 1392 } 1393 return this; 1394 } 1395 1396 /** 1397 * @return {@link #restoreDate} (The date when a suspended the marketing or the 1398 * marketing authorization of the product is anticipated to be 1399 * restored.). This is the underlying object with id, value and 1400 * extensions. The accessor "getRestoreDate" gives direct access to the 1401 * value 1402 */ 1403 public DateTimeType getRestoreDateElement() { 1404 if (this.restoreDate == null) 1405 if (Configuration.errorOnAutoCreate()) 1406 throw new Error("Attempt to auto-create MedicinalProductAuthorization.restoreDate"); 1407 else if (Configuration.doAutoCreate()) 1408 this.restoreDate = new DateTimeType(); // bb 1409 return this.restoreDate; 1410 } 1411 1412 public boolean hasRestoreDateElement() { 1413 return this.restoreDate != null && !this.restoreDate.isEmpty(); 1414 } 1415 1416 public boolean hasRestoreDate() { 1417 return this.restoreDate != null && !this.restoreDate.isEmpty(); 1418 } 1419 1420 /** 1421 * @param value {@link #restoreDate} (The date when a suspended the marketing or 1422 * the marketing authorization of the product is anticipated to be 1423 * restored.). This is the underlying object with id, value and 1424 * extensions. The accessor "getRestoreDate" gives direct access to 1425 * the value 1426 */ 1427 public MedicinalProductAuthorization setRestoreDateElement(DateTimeType value) { 1428 this.restoreDate = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return The date when a suspended the marketing or the marketing 1434 * authorization of the product is anticipated to be restored. 1435 */ 1436 public Date getRestoreDate() { 1437 return this.restoreDate == null ? null : this.restoreDate.getValue(); 1438 } 1439 1440 /** 1441 * @param value The date when a suspended the marketing or the marketing 1442 * authorization of the product is anticipated to be restored. 1443 */ 1444 public MedicinalProductAuthorization setRestoreDate(Date value) { 1445 if (value == null) 1446 this.restoreDate = null; 1447 else { 1448 if (this.restoreDate == null) 1449 this.restoreDate = new DateTimeType(); 1450 this.restoreDate.setValue(value); 1451 } 1452 return this; 1453 } 1454 1455 /** 1456 * @return {@link #validityPeriod} (The beginning of the time period in which 1457 * the marketing authorization is in the specific status shall be 1458 * specified A complete date consisting of day, month and year shall be 1459 * specified using the ISO 8601 date format.) 1460 */ 1461 public Period getValidityPeriod() { 1462 if (this.validityPeriod == null) 1463 if (Configuration.errorOnAutoCreate()) 1464 throw new Error("Attempt to auto-create MedicinalProductAuthorization.validityPeriod"); 1465 else if (Configuration.doAutoCreate()) 1466 this.validityPeriod = new Period(); // cc 1467 return this.validityPeriod; 1468 } 1469 1470 public boolean hasValidityPeriod() { 1471 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 1472 } 1473 1474 /** 1475 * @param value {@link #validityPeriod} (The beginning of the time period in 1476 * which the marketing authorization is in the specific status 1477 * shall be specified A complete date consisting of day, month and 1478 * year shall be specified using the ISO 8601 date format.) 1479 */ 1480 public MedicinalProductAuthorization setValidityPeriod(Period value) { 1481 this.validityPeriod = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #dataExclusivityPeriod} (A period of time after authorization 1487 * before generic product applicatiosn can be submitted.) 1488 */ 1489 public Period getDataExclusivityPeriod() { 1490 if (this.dataExclusivityPeriod == null) 1491 if (Configuration.errorOnAutoCreate()) 1492 throw new Error("Attempt to auto-create MedicinalProductAuthorization.dataExclusivityPeriod"); 1493 else if (Configuration.doAutoCreate()) 1494 this.dataExclusivityPeriod = new Period(); // cc 1495 return this.dataExclusivityPeriod; 1496 } 1497 1498 public boolean hasDataExclusivityPeriod() { 1499 return this.dataExclusivityPeriod != null && !this.dataExclusivityPeriod.isEmpty(); 1500 } 1501 1502 /** 1503 * @param value {@link #dataExclusivityPeriod} (A period of time after 1504 * authorization before generic product applicatiosn can be 1505 * submitted.) 1506 */ 1507 public MedicinalProductAuthorization setDataExclusivityPeriod(Period value) { 1508 this.dataExclusivityPeriod = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return {@link #dateOfFirstAuthorization} (The date when the first 1514 * authorization was granted by a Medicines Regulatory Agency.). This is 1515 * the underlying object with id, value and extensions. The accessor 1516 * "getDateOfFirstAuthorization" gives direct access to the value 1517 */ 1518 public DateTimeType getDateOfFirstAuthorizationElement() { 1519 if (this.dateOfFirstAuthorization == null) 1520 if (Configuration.errorOnAutoCreate()) 1521 throw new Error("Attempt to auto-create MedicinalProductAuthorization.dateOfFirstAuthorization"); 1522 else if (Configuration.doAutoCreate()) 1523 this.dateOfFirstAuthorization = new DateTimeType(); // bb 1524 return this.dateOfFirstAuthorization; 1525 } 1526 1527 public boolean hasDateOfFirstAuthorizationElement() { 1528 return this.dateOfFirstAuthorization != null && !this.dateOfFirstAuthorization.isEmpty(); 1529 } 1530 1531 public boolean hasDateOfFirstAuthorization() { 1532 return this.dateOfFirstAuthorization != null && !this.dateOfFirstAuthorization.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #dateOfFirstAuthorization} (The date when the first 1537 * authorization was granted by a Medicines Regulatory Agency.). 1538 * This is the underlying object with id, value and extensions. The 1539 * accessor "getDateOfFirstAuthorization" gives direct access to 1540 * the value 1541 */ 1542 public MedicinalProductAuthorization setDateOfFirstAuthorizationElement(DateTimeType value) { 1543 this.dateOfFirstAuthorization = value; 1544 return this; 1545 } 1546 1547 /** 1548 * @return The date when the first authorization was granted by a Medicines 1549 * Regulatory Agency. 1550 */ 1551 public Date getDateOfFirstAuthorization() { 1552 return this.dateOfFirstAuthorization == null ? null : this.dateOfFirstAuthorization.getValue(); 1553 } 1554 1555 /** 1556 * @param value The date when the first authorization was granted by a Medicines 1557 * Regulatory Agency. 1558 */ 1559 public MedicinalProductAuthorization setDateOfFirstAuthorization(Date value) { 1560 if (value == null) 1561 this.dateOfFirstAuthorization = null; 1562 else { 1563 if (this.dateOfFirstAuthorization == null) 1564 this.dateOfFirstAuthorization = new DateTimeType(); 1565 this.dateOfFirstAuthorization.setValue(value); 1566 } 1567 return this; 1568 } 1569 1570 /** 1571 * @return {@link #internationalBirthDate} (Date of first marketing 1572 * authorization for a company's new medicinal product in any country in 1573 * the World.). This is the underlying object with id, value and 1574 * extensions. The accessor "getInternationalBirthDate" gives direct 1575 * access to the value 1576 */ 1577 public DateTimeType getInternationalBirthDateElement() { 1578 if (this.internationalBirthDate == null) 1579 if (Configuration.errorOnAutoCreate()) 1580 throw new Error("Attempt to auto-create MedicinalProductAuthorization.internationalBirthDate"); 1581 else if (Configuration.doAutoCreate()) 1582 this.internationalBirthDate = new DateTimeType(); // bb 1583 return this.internationalBirthDate; 1584 } 1585 1586 public boolean hasInternationalBirthDateElement() { 1587 return this.internationalBirthDate != null && !this.internationalBirthDate.isEmpty(); 1588 } 1589 1590 public boolean hasInternationalBirthDate() { 1591 return this.internationalBirthDate != null && !this.internationalBirthDate.isEmpty(); 1592 } 1593 1594 /** 1595 * @param value {@link #internationalBirthDate} (Date of first marketing 1596 * authorization for a company's new medicinal product in any 1597 * country in the World.). This is the underlying object with id, 1598 * value and extensions. The accessor "getInternationalBirthDate" 1599 * gives direct access to the value 1600 */ 1601 public MedicinalProductAuthorization setInternationalBirthDateElement(DateTimeType value) { 1602 this.internationalBirthDate = value; 1603 return this; 1604 } 1605 1606 /** 1607 * @return Date of first marketing authorization for a company's new medicinal 1608 * product in any country in the World. 1609 */ 1610 public Date getInternationalBirthDate() { 1611 return this.internationalBirthDate == null ? null : this.internationalBirthDate.getValue(); 1612 } 1613 1614 /** 1615 * @param value Date of first marketing authorization for a company's new 1616 * medicinal product in any country in the World. 1617 */ 1618 public MedicinalProductAuthorization setInternationalBirthDate(Date value) { 1619 if (value == null) 1620 this.internationalBirthDate = null; 1621 else { 1622 if (this.internationalBirthDate == null) 1623 this.internationalBirthDate = new DateTimeType(); 1624 this.internationalBirthDate.setValue(value); 1625 } 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #legalBasis} (The legal framework against which this 1631 * authorization is granted.) 1632 */ 1633 public CodeableConcept getLegalBasis() { 1634 if (this.legalBasis == null) 1635 if (Configuration.errorOnAutoCreate()) 1636 throw new Error("Attempt to auto-create MedicinalProductAuthorization.legalBasis"); 1637 else if (Configuration.doAutoCreate()) 1638 this.legalBasis = new CodeableConcept(); // cc 1639 return this.legalBasis; 1640 } 1641 1642 public boolean hasLegalBasis() { 1643 return this.legalBasis != null && !this.legalBasis.isEmpty(); 1644 } 1645 1646 /** 1647 * @param value {@link #legalBasis} (The legal framework against which this 1648 * authorization is granted.) 1649 */ 1650 public MedicinalProductAuthorization setLegalBasis(CodeableConcept value) { 1651 this.legalBasis = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return {@link #jurisdictionalAuthorization} (Authorization in areas within a 1657 * country.) 1658 */ 1659 public List<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent> getJurisdictionalAuthorization() { 1660 if (this.jurisdictionalAuthorization == null) 1661 this.jurisdictionalAuthorization = new ArrayList<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent>(); 1662 return this.jurisdictionalAuthorization; 1663 } 1664 1665 /** 1666 * @return Returns a reference to <code>this</code> for easy method chaining 1667 */ 1668 public MedicinalProductAuthorization setJurisdictionalAuthorization( 1669 List<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent> theJurisdictionalAuthorization) { 1670 this.jurisdictionalAuthorization = theJurisdictionalAuthorization; 1671 return this; 1672 } 1673 1674 public boolean hasJurisdictionalAuthorization() { 1675 if (this.jurisdictionalAuthorization == null) 1676 return false; 1677 for (MedicinalProductAuthorizationJurisdictionalAuthorizationComponent item : this.jurisdictionalAuthorization) 1678 if (!item.isEmpty()) 1679 return true; 1680 return false; 1681 } 1682 1683 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent addJurisdictionalAuthorization() { // 3 1684 MedicinalProductAuthorizationJurisdictionalAuthorizationComponent t = new MedicinalProductAuthorizationJurisdictionalAuthorizationComponent(); 1685 if (this.jurisdictionalAuthorization == null) 1686 this.jurisdictionalAuthorization = new ArrayList<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent>(); 1687 this.jurisdictionalAuthorization.add(t); 1688 return t; 1689 } 1690 1691 public MedicinalProductAuthorization addJurisdictionalAuthorization( 1692 MedicinalProductAuthorizationJurisdictionalAuthorizationComponent t) { // 3 1693 if (t == null) 1694 return this; 1695 if (this.jurisdictionalAuthorization == null) 1696 this.jurisdictionalAuthorization = new ArrayList<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent>(); 1697 this.jurisdictionalAuthorization.add(t); 1698 return this; 1699 } 1700 1701 /** 1702 * @return The first repetition of repeating field 1703 * {@link #jurisdictionalAuthorization}, creating it if it does not 1704 * already exist 1705 */ 1706 public MedicinalProductAuthorizationJurisdictionalAuthorizationComponent getJurisdictionalAuthorizationFirstRep() { 1707 if (getJurisdictionalAuthorization().isEmpty()) { 1708 addJurisdictionalAuthorization(); 1709 } 1710 return getJurisdictionalAuthorization().get(0); 1711 } 1712 1713 /** 1714 * @return {@link #holder} (Marketing Authorization Holder.) 1715 */ 1716 public Reference getHolder() { 1717 if (this.holder == null) 1718 if (Configuration.errorOnAutoCreate()) 1719 throw new Error("Attempt to auto-create MedicinalProductAuthorization.holder"); 1720 else if (Configuration.doAutoCreate()) 1721 this.holder = new Reference(); // cc 1722 return this.holder; 1723 } 1724 1725 public boolean hasHolder() { 1726 return this.holder != null && !this.holder.isEmpty(); 1727 } 1728 1729 /** 1730 * @param value {@link #holder} (Marketing Authorization Holder.) 1731 */ 1732 public MedicinalProductAuthorization setHolder(Reference value) { 1733 this.holder = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return {@link #holder} The actual object that is the target of the 1739 * reference. The reference library doesn't populate this, but you can 1740 * use it to hold the resource if you resolve it. (Marketing 1741 * Authorization Holder.) 1742 */ 1743 public Organization getHolderTarget() { 1744 if (this.holderTarget == null) 1745 if (Configuration.errorOnAutoCreate()) 1746 throw new Error("Attempt to auto-create MedicinalProductAuthorization.holder"); 1747 else if (Configuration.doAutoCreate()) 1748 this.holderTarget = new Organization(); // aa 1749 return this.holderTarget; 1750 } 1751 1752 /** 1753 * @param value {@link #holder} The actual object that is the target of the 1754 * reference. The reference library doesn't use these, but you can 1755 * use it to hold the resource if you resolve it. (Marketing 1756 * Authorization Holder.) 1757 */ 1758 public MedicinalProductAuthorization setHolderTarget(Organization value) { 1759 this.holderTarget = value; 1760 return this; 1761 } 1762 1763 /** 1764 * @return {@link #regulator} (Medicines Regulatory Agency.) 1765 */ 1766 public Reference getRegulator() { 1767 if (this.regulator == null) 1768 if (Configuration.errorOnAutoCreate()) 1769 throw new Error("Attempt to auto-create MedicinalProductAuthorization.regulator"); 1770 else if (Configuration.doAutoCreate()) 1771 this.regulator = new Reference(); // cc 1772 return this.regulator; 1773 } 1774 1775 public boolean hasRegulator() { 1776 return this.regulator != null && !this.regulator.isEmpty(); 1777 } 1778 1779 /** 1780 * @param value {@link #regulator} (Medicines Regulatory Agency.) 1781 */ 1782 public MedicinalProductAuthorization setRegulator(Reference value) { 1783 this.regulator = value; 1784 return this; 1785 } 1786 1787 /** 1788 * @return {@link #regulator} The actual object that is the target of the 1789 * reference. The reference library doesn't populate this, but you can 1790 * use it to hold the resource if you resolve it. (Medicines Regulatory 1791 * Agency.) 1792 */ 1793 public Organization getRegulatorTarget() { 1794 if (this.regulatorTarget == null) 1795 if (Configuration.errorOnAutoCreate()) 1796 throw new Error("Attempt to auto-create MedicinalProductAuthorization.regulator"); 1797 else if (Configuration.doAutoCreate()) 1798 this.regulatorTarget = new Organization(); // aa 1799 return this.regulatorTarget; 1800 } 1801 1802 /** 1803 * @param value {@link #regulator} The actual object that is the target of the 1804 * reference. The reference library doesn't use these, but you can 1805 * use it to hold the resource if you resolve it. (Medicines 1806 * Regulatory Agency.) 1807 */ 1808 public MedicinalProductAuthorization setRegulatorTarget(Organization value) { 1809 this.regulatorTarget = value; 1810 return this; 1811 } 1812 1813 /** 1814 * @return {@link #procedure} (The regulatory procedure for granting or amending 1815 * a marketing authorization.) 1816 */ 1817 public MedicinalProductAuthorizationProcedureComponent getProcedure() { 1818 if (this.procedure == null) 1819 if (Configuration.errorOnAutoCreate()) 1820 throw new Error("Attempt to auto-create MedicinalProductAuthorization.procedure"); 1821 else if (Configuration.doAutoCreate()) 1822 this.procedure = new MedicinalProductAuthorizationProcedureComponent(); // cc 1823 return this.procedure; 1824 } 1825 1826 public boolean hasProcedure() { 1827 return this.procedure != null && !this.procedure.isEmpty(); 1828 } 1829 1830 /** 1831 * @param value {@link #procedure} (The regulatory procedure for granting or 1832 * amending a marketing authorization.) 1833 */ 1834 public MedicinalProductAuthorization setProcedure(MedicinalProductAuthorizationProcedureComponent value) { 1835 this.procedure = value; 1836 return this; 1837 } 1838 1839 protected void listChildren(List<Property> children) { 1840 super.listChildren(children); 1841 children.add(new Property("identifier", "Identifier", 1842 "Business identifier for the marketing authorization, as assigned by a regulator.", 0, 1843 java.lang.Integer.MAX_VALUE, identifier)); 1844 children.add(new Property("subject", "Reference(MedicinalProduct|MedicinalProductPackaged)", 1845 "The medicinal product that is being authorized.", 0, 1, subject)); 1846 children.add(new Property("country", "CodeableConcept", 1847 "The country in which the marketing authorization has been granted.", 0, java.lang.Integer.MAX_VALUE, country)); 1848 children.add(new Property("jurisdiction", "CodeableConcept", "Jurisdiction within a country.", 0, 1849 java.lang.Integer.MAX_VALUE, jurisdiction)); 1850 children.add(new Property("status", "CodeableConcept", "The status of the marketing authorization.", 0, 1, status)); 1851 children.add(new Property("statusDate", "dateTime", "The date at which the given status has become applicable.", 0, 1852 1, statusDate)); 1853 children.add(new Property("restoreDate", "dateTime", 1854 "The date when a suspended the marketing or the marketing authorization of the product is anticipated to be restored.", 1855 0, 1, restoreDate)); 1856 children.add(new Property("validityPeriod", "Period", 1857 "The beginning of the time period in which the marketing authorization is in the specific status shall be specified A complete date consisting of day, month and year shall be specified using the ISO 8601 date format.", 1858 0, 1, validityPeriod)); 1859 children.add(new Property("dataExclusivityPeriod", "Period", 1860 "A period of time after authorization before generic product applicatiosn can be submitted.", 0, 1, 1861 dataExclusivityPeriod)); 1862 children.add(new Property("dateOfFirstAuthorization", "dateTime", 1863 "The date when the first authorization was granted by a Medicines Regulatory Agency.", 0, 1, 1864 dateOfFirstAuthorization)); 1865 children.add(new Property("internationalBirthDate", "dateTime", 1866 "Date of first marketing authorization for a company's new medicinal product in any country in the World.", 0, 1867 1, internationalBirthDate)); 1868 children.add(new Property("legalBasis", "CodeableConcept", 1869 "The legal framework against which this authorization is granted.", 0, 1, legalBasis)); 1870 children.add(new Property("jurisdictionalAuthorization", "", "Authorization in areas within a country.", 0, 1871 java.lang.Integer.MAX_VALUE, jurisdictionalAuthorization)); 1872 children.add(new Property("holder", "Reference(Organization)", "Marketing Authorization Holder.", 0, 1, holder)); 1873 children.add(new Property("regulator", "Reference(Organization)", "Medicines Regulatory Agency.", 0, 1, regulator)); 1874 children.add(new Property("procedure", "", 1875 "The regulatory procedure for granting or amending a marketing authorization.", 0, 1, procedure)); 1876 } 1877 1878 @Override 1879 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1880 switch (_hash) { 1881 case -1618432855: 1882 /* identifier */ return new Property("identifier", "Identifier", 1883 "Business identifier for the marketing authorization, as assigned by a regulator.", 0, 1884 java.lang.Integer.MAX_VALUE, identifier); 1885 case -1867885268: 1886 /* subject */ return new Property("subject", "Reference(MedicinalProduct|MedicinalProductPackaged)", 1887 "The medicinal product that is being authorized.", 0, 1, subject); 1888 case 957831062: 1889 /* country */ return new Property("country", "CodeableConcept", 1890 "The country in which the marketing authorization has been granted.", 0, java.lang.Integer.MAX_VALUE, 1891 country); 1892 case -507075711: 1893 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", "Jurisdiction within a country.", 0, 1894 java.lang.Integer.MAX_VALUE, jurisdiction); 1895 case -892481550: 1896 /* status */ return new Property("status", "CodeableConcept", "The status of the marketing authorization.", 0, 1, 1897 status); 1898 case 247524032: 1899 /* statusDate */ return new Property("statusDate", "dateTime", 1900 "The date at which the given status has become applicable.", 0, 1, statusDate); 1901 case 329465692: 1902 /* restoreDate */ return new Property("restoreDate", "dateTime", 1903 "The date when a suspended the marketing or the marketing authorization of the product is anticipated to be restored.", 1904 0, 1, restoreDate); 1905 case -1434195053: 1906 /* validityPeriod */ return new Property("validityPeriod", "Period", 1907 "The beginning of the time period in which the marketing authorization is in the specific status shall be specified A complete date consisting of day, month and year shall be specified using the ISO 8601 date format.", 1908 0, 1, validityPeriod); 1909 case 1940655806: 1910 /* dataExclusivityPeriod */ return new Property("dataExclusivityPeriod", "Period", 1911 "A period of time after authorization before generic product applicatiosn can be submitted.", 0, 1, 1912 dataExclusivityPeriod); 1913 case -1026933074: 1914 /* dateOfFirstAuthorization */ return new Property("dateOfFirstAuthorization", "dateTime", 1915 "The date when the first authorization was granted by a Medicines Regulatory Agency.", 0, 1, 1916 dateOfFirstAuthorization); 1917 case 400069151: 1918 /* internationalBirthDate */ return new Property("internationalBirthDate", "dateTime", 1919 "Date of first marketing authorization for a company's new medicinal product in any country in the World.", 0, 1920 1, internationalBirthDate); 1921 case 552357125: 1922 /* legalBasis */ return new Property("legalBasis", "CodeableConcept", 1923 "The legal framework against which this authorization is granted.", 0, 1, legalBasis); 1924 case 1459432557: 1925 /* jurisdictionalAuthorization */ return new Property("jurisdictionalAuthorization", "", 1926 "Authorization in areas within a country.", 0, java.lang.Integer.MAX_VALUE, jurisdictionalAuthorization); 1927 case -1211707988: 1928 /* holder */ return new Property("holder", "Reference(Organization)", "Marketing Authorization Holder.", 0, 1, 1929 holder); 1930 case 414760449: 1931 /* regulator */ return new Property("regulator", "Reference(Organization)", "Medicines Regulatory Agency.", 0, 1, 1932 regulator); 1933 case -1095204141: 1934 /* procedure */ return new Property("procedure", "", 1935 "The regulatory procedure for granting or amending a marketing authorization.", 0, 1, procedure); 1936 default: 1937 return super.getNamedProperty(_hash, _name, _checkValid); 1938 } 1939 1940 } 1941 1942 @Override 1943 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1944 switch (hash) { 1945 case -1618432855: 1946 /* identifier */ return this.identifier == null ? new Base[0] 1947 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1948 case -1867885268: 1949 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1950 case 957831062: 1951 /* country */ return this.country == null ? new Base[0] : this.country.toArray(new Base[this.country.size()]); // CodeableConcept 1952 case -507075711: 1953 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 1954 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1955 case -892481550: 1956 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 1957 case 247524032: 1958 /* statusDate */ return this.statusDate == null ? new Base[0] : new Base[] { this.statusDate }; // DateTimeType 1959 case 329465692: 1960 /* restoreDate */ return this.restoreDate == null ? new Base[0] : new Base[] { this.restoreDate }; // DateTimeType 1961 case -1434195053: 1962 /* validityPeriod */ return this.validityPeriod == null ? new Base[0] : new Base[] { this.validityPeriod }; // Period 1963 case 1940655806: 1964 /* dataExclusivityPeriod */ return this.dataExclusivityPeriod == null ? new Base[0] 1965 : new Base[] { this.dataExclusivityPeriod }; // Period 1966 case -1026933074: 1967 /* dateOfFirstAuthorization */ return this.dateOfFirstAuthorization == null ? new Base[0] 1968 : new Base[] { this.dateOfFirstAuthorization }; // DateTimeType 1969 case 400069151: 1970 /* internationalBirthDate */ return this.internationalBirthDate == null ? new Base[0] 1971 : new Base[] { this.internationalBirthDate }; // DateTimeType 1972 case 552357125: 1973 /* legalBasis */ return this.legalBasis == null ? new Base[0] : new Base[] { this.legalBasis }; // CodeableConcept 1974 case 1459432557: 1975 /* jurisdictionalAuthorization */ return this.jurisdictionalAuthorization == null ? new Base[0] 1976 : this.jurisdictionalAuthorization.toArray(new Base[this.jurisdictionalAuthorization.size()]); // MedicinalProductAuthorizationJurisdictionalAuthorizationComponent 1977 case -1211707988: 1978 /* holder */ return this.holder == null ? new Base[0] : new Base[] { this.holder }; // Reference 1979 case 414760449: 1980 /* regulator */ return this.regulator == null ? new Base[0] : new Base[] { this.regulator }; // Reference 1981 case -1095204141: 1982 /* procedure */ return this.procedure == null ? new Base[0] : new Base[] { this.procedure }; // MedicinalProductAuthorizationProcedureComponent 1983 default: 1984 return super.getProperty(hash, name, checkValid); 1985 } 1986 1987 } 1988 1989 @Override 1990 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1991 switch (hash) { 1992 case -1618432855: // identifier 1993 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1994 return value; 1995 case -1867885268: // subject 1996 this.subject = castToReference(value); // Reference 1997 return value; 1998 case 957831062: // country 1999 this.getCountry().add(castToCodeableConcept(value)); // CodeableConcept 2000 return value; 2001 case -507075711: // jurisdiction 2002 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2003 return value; 2004 case -892481550: // status 2005 this.status = castToCodeableConcept(value); // CodeableConcept 2006 return value; 2007 case 247524032: // statusDate 2008 this.statusDate = castToDateTime(value); // DateTimeType 2009 return value; 2010 case 329465692: // restoreDate 2011 this.restoreDate = castToDateTime(value); // DateTimeType 2012 return value; 2013 case -1434195053: // validityPeriod 2014 this.validityPeriod = castToPeriod(value); // Period 2015 return value; 2016 case 1940655806: // dataExclusivityPeriod 2017 this.dataExclusivityPeriod = castToPeriod(value); // Period 2018 return value; 2019 case -1026933074: // dateOfFirstAuthorization 2020 this.dateOfFirstAuthorization = castToDateTime(value); // DateTimeType 2021 return value; 2022 case 400069151: // internationalBirthDate 2023 this.internationalBirthDate = castToDateTime(value); // DateTimeType 2024 return value; 2025 case 552357125: // legalBasis 2026 this.legalBasis = castToCodeableConcept(value); // CodeableConcept 2027 return value; 2028 case 1459432557: // jurisdictionalAuthorization 2029 this.getJurisdictionalAuthorization() 2030 .add((MedicinalProductAuthorizationJurisdictionalAuthorizationComponent) value); // MedicinalProductAuthorizationJurisdictionalAuthorizationComponent 2031 return value; 2032 case -1211707988: // holder 2033 this.holder = castToReference(value); // Reference 2034 return value; 2035 case 414760449: // regulator 2036 this.regulator = castToReference(value); // Reference 2037 return value; 2038 case -1095204141: // procedure 2039 this.procedure = (MedicinalProductAuthorizationProcedureComponent) value; // MedicinalProductAuthorizationProcedureComponent 2040 return value; 2041 default: 2042 return super.setProperty(hash, name, value); 2043 } 2044 2045 } 2046 2047 @Override 2048 public Base setProperty(String name, Base value) throws FHIRException { 2049 if (name.equals("identifier")) { 2050 this.getIdentifier().add(castToIdentifier(value)); 2051 } else if (name.equals("subject")) { 2052 this.subject = castToReference(value); // Reference 2053 } else if (name.equals("country")) { 2054 this.getCountry().add(castToCodeableConcept(value)); 2055 } else if (name.equals("jurisdiction")) { 2056 this.getJurisdiction().add(castToCodeableConcept(value)); 2057 } else if (name.equals("status")) { 2058 this.status = castToCodeableConcept(value); // CodeableConcept 2059 } else if (name.equals("statusDate")) { 2060 this.statusDate = castToDateTime(value); // DateTimeType 2061 } else if (name.equals("restoreDate")) { 2062 this.restoreDate = castToDateTime(value); // DateTimeType 2063 } else if (name.equals("validityPeriod")) { 2064 this.validityPeriod = castToPeriod(value); // Period 2065 } else if (name.equals("dataExclusivityPeriod")) { 2066 this.dataExclusivityPeriod = castToPeriod(value); // Period 2067 } else if (name.equals("dateOfFirstAuthorization")) { 2068 this.dateOfFirstAuthorization = castToDateTime(value); // DateTimeType 2069 } else if (name.equals("internationalBirthDate")) { 2070 this.internationalBirthDate = castToDateTime(value); // DateTimeType 2071 } else if (name.equals("legalBasis")) { 2072 this.legalBasis = castToCodeableConcept(value); // CodeableConcept 2073 } else if (name.equals("jurisdictionalAuthorization")) { 2074 this.getJurisdictionalAuthorization() 2075 .add((MedicinalProductAuthorizationJurisdictionalAuthorizationComponent) value); 2076 } else if (name.equals("holder")) { 2077 this.holder = castToReference(value); // Reference 2078 } else if (name.equals("regulator")) { 2079 this.regulator = castToReference(value); // Reference 2080 } else if (name.equals("procedure")) { 2081 this.procedure = (MedicinalProductAuthorizationProcedureComponent) value; // MedicinalProductAuthorizationProcedureComponent 2082 } else 2083 return super.setProperty(name, value); 2084 return value; 2085 } 2086 2087 @Override 2088 public void removeChild(String name, Base value) throws FHIRException { 2089 if (name.equals("identifier")) { 2090 this.getIdentifier().remove(castToIdentifier(value)); 2091 } else if (name.equals("subject")) { 2092 this.subject = null; 2093 } else if (name.equals("country")) { 2094 this.getCountry().remove(castToCodeableConcept(value)); 2095 } else if (name.equals("jurisdiction")) { 2096 this.getJurisdiction().remove(castToCodeableConcept(value)); 2097 } else if (name.equals("status")) { 2098 this.status = null; 2099 } else if (name.equals("statusDate")) { 2100 this.statusDate = null; 2101 } else if (name.equals("restoreDate")) { 2102 this.restoreDate = null; 2103 } else if (name.equals("validityPeriod")) { 2104 this.validityPeriod = null; 2105 } else if (name.equals("dataExclusivityPeriod")) { 2106 this.dataExclusivityPeriod = null; 2107 } else if (name.equals("dateOfFirstAuthorization")) { 2108 this.dateOfFirstAuthorization = null; 2109 } else if (name.equals("internationalBirthDate")) { 2110 this.internationalBirthDate = null; 2111 } else if (name.equals("legalBasis")) { 2112 this.legalBasis = null; 2113 } else if (name.equals("jurisdictionalAuthorization")) { 2114 this.getJurisdictionalAuthorization() 2115 .remove((MedicinalProductAuthorizationJurisdictionalAuthorizationComponent) value); 2116 } else if (name.equals("holder")) { 2117 this.holder = null; 2118 } else if (name.equals("regulator")) { 2119 this.regulator = null; 2120 } else if (name.equals("procedure")) { 2121 this.procedure = (MedicinalProductAuthorizationProcedureComponent) value; // MedicinalProductAuthorizationProcedureComponent 2122 } else 2123 super.removeChild(name, value); 2124 2125 } 2126 2127 @Override 2128 public Base makeProperty(int hash, String name) throws FHIRException { 2129 switch (hash) { 2130 case -1618432855: 2131 return addIdentifier(); 2132 case -1867885268: 2133 return getSubject(); 2134 case 957831062: 2135 return addCountry(); 2136 case -507075711: 2137 return addJurisdiction(); 2138 case -892481550: 2139 return getStatus(); 2140 case 247524032: 2141 return getStatusDateElement(); 2142 case 329465692: 2143 return getRestoreDateElement(); 2144 case -1434195053: 2145 return getValidityPeriod(); 2146 case 1940655806: 2147 return getDataExclusivityPeriod(); 2148 case -1026933074: 2149 return getDateOfFirstAuthorizationElement(); 2150 case 400069151: 2151 return getInternationalBirthDateElement(); 2152 case 552357125: 2153 return getLegalBasis(); 2154 case 1459432557: 2155 return addJurisdictionalAuthorization(); 2156 case -1211707988: 2157 return getHolder(); 2158 case 414760449: 2159 return getRegulator(); 2160 case -1095204141: 2161 return getProcedure(); 2162 default: 2163 return super.makeProperty(hash, name); 2164 } 2165 2166 } 2167 2168 @Override 2169 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2170 switch (hash) { 2171 case -1618432855: 2172 /* identifier */ return new String[] { "Identifier" }; 2173 case -1867885268: 2174 /* subject */ return new String[] { "Reference" }; 2175 case 957831062: 2176 /* country */ return new String[] { "CodeableConcept" }; 2177 case -507075711: 2178 /* jurisdiction */ return new String[] { "CodeableConcept" }; 2179 case -892481550: 2180 /* status */ return new String[] { "CodeableConcept" }; 2181 case 247524032: 2182 /* statusDate */ return new String[] { "dateTime" }; 2183 case 329465692: 2184 /* restoreDate */ return new String[] { "dateTime" }; 2185 case -1434195053: 2186 /* validityPeriod */ return new String[] { "Period" }; 2187 case 1940655806: 2188 /* dataExclusivityPeriod */ return new String[] { "Period" }; 2189 case -1026933074: 2190 /* dateOfFirstAuthorization */ return new String[] { "dateTime" }; 2191 case 400069151: 2192 /* internationalBirthDate */ return new String[] { "dateTime" }; 2193 case 552357125: 2194 /* legalBasis */ return new String[] { "CodeableConcept" }; 2195 case 1459432557: 2196 /* jurisdictionalAuthorization */ return new String[] {}; 2197 case -1211707988: 2198 /* holder */ return new String[] { "Reference" }; 2199 case 414760449: 2200 /* regulator */ return new String[] { "Reference" }; 2201 case -1095204141: 2202 /* procedure */ return new String[] {}; 2203 default: 2204 return super.getTypesForProperty(hash, name); 2205 } 2206 2207 } 2208 2209 @Override 2210 public Base addChild(String name) throws FHIRException { 2211 if (name.equals("identifier")) { 2212 return addIdentifier(); 2213 } else if (name.equals("subject")) { 2214 this.subject = new Reference(); 2215 return this.subject; 2216 } else if (name.equals("country")) { 2217 return addCountry(); 2218 } else if (name.equals("jurisdiction")) { 2219 return addJurisdiction(); 2220 } else if (name.equals("status")) { 2221 this.status = new CodeableConcept(); 2222 return this.status; 2223 } else if (name.equals("statusDate")) { 2224 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductAuthorization.statusDate"); 2225 } else if (name.equals("restoreDate")) { 2226 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductAuthorization.restoreDate"); 2227 } else if (name.equals("validityPeriod")) { 2228 this.validityPeriod = new Period(); 2229 return this.validityPeriod; 2230 } else if (name.equals("dataExclusivityPeriod")) { 2231 this.dataExclusivityPeriod = new Period(); 2232 return this.dataExclusivityPeriod; 2233 } else if (name.equals("dateOfFirstAuthorization")) { 2234 throw new FHIRException( 2235 "Cannot call addChild on a singleton property MedicinalProductAuthorization.dateOfFirstAuthorization"); 2236 } else if (name.equals("internationalBirthDate")) { 2237 throw new FHIRException( 2238 "Cannot call addChild on a singleton property MedicinalProductAuthorization.internationalBirthDate"); 2239 } else if (name.equals("legalBasis")) { 2240 this.legalBasis = new CodeableConcept(); 2241 return this.legalBasis; 2242 } else if (name.equals("jurisdictionalAuthorization")) { 2243 return addJurisdictionalAuthorization(); 2244 } else if (name.equals("holder")) { 2245 this.holder = new Reference(); 2246 return this.holder; 2247 } else if (name.equals("regulator")) { 2248 this.regulator = new Reference(); 2249 return this.regulator; 2250 } else if (name.equals("procedure")) { 2251 this.procedure = new MedicinalProductAuthorizationProcedureComponent(); 2252 return this.procedure; 2253 } else 2254 return super.addChild(name); 2255 } 2256 2257 public String fhirType() { 2258 return "MedicinalProductAuthorization"; 2259 2260 } 2261 2262 public MedicinalProductAuthorization copy() { 2263 MedicinalProductAuthorization dst = new MedicinalProductAuthorization(); 2264 copyValues(dst); 2265 return dst; 2266 } 2267 2268 public void copyValues(MedicinalProductAuthorization dst) { 2269 super.copyValues(dst); 2270 if (identifier != null) { 2271 dst.identifier = new ArrayList<Identifier>(); 2272 for (Identifier i : identifier) 2273 dst.identifier.add(i.copy()); 2274 } 2275 ; 2276 dst.subject = subject == null ? null : subject.copy(); 2277 if (country != null) { 2278 dst.country = new ArrayList<CodeableConcept>(); 2279 for (CodeableConcept i : country) 2280 dst.country.add(i.copy()); 2281 } 2282 ; 2283 if (jurisdiction != null) { 2284 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2285 for (CodeableConcept i : jurisdiction) 2286 dst.jurisdiction.add(i.copy()); 2287 } 2288 ; 2289 dst.status = status == null ? null : status.copy(); 2290 dst.statusDate = statusDate == null ? null : statusDate.copy(); 2291 dst.restoreDate = restoreDate == null ? null : restoreDate.copy(); 2292 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 2293 dst.dataExclusivityPeriod = dataExclusivityPeriod == null ? null : dataExclusivityPeriod.copy(); 2294 dst.dateOfFirstAuthorization = dateOfFirstAuthorization == null ? null : dateOfFirstAuthorization.copy(); 2295 dst.internationalBirthDate = internationalBirthDate == null ? null : internationalBirthDate.copy(); 2296 dst.legalBasis = legalBasis == null ? null : legalBasis.copy(); 2297 if (jurisdictionalAuthorization != null) { 2298 dst.jurisdictionalAuthorization = new ArrayList<MedicinalProductAuthorizationJurisdictionalAuthorizationComponent>(); 2299 for (MedicinalProductAuthorizationJurisdictionalAuthorizationComponent i : jurisdictionalAuthorization) 2300 dst.jurisdictionalAuthorization.add(i.copy()); 2301 } 2302 ; 2303 dst.holder = holder == null ? null : holder.copy(); 2304 dst.regulator = regulator == null ? null : regulator.copy(); 2305 dst.procedure = procedure == null ? null : procedure.copy(); 2306 } 2307 2308 protected MedicinalProductAuthorization typedCopy() { 2309 return copy(); 2310 } 2311 2312 @Override 2313 public boolean equalsDeep(Base other_) { 2314 if (!super.equalsDeep(other_)) 2315 return false; 2316 if (!(other_ instanceof MedicinalProductAuthorization)) 2317 return false; 2318 MedicinalProductAuthorization o = (MedicinalProductAuthorization) other_; 2319 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 2320 && compareDeep(country, o.country, true) && compareDeep(jurisdiction, o.jurisdiction, true) 2321 && compareDeep(status, o.status, true) && compareDeep(statusDate, o.statusDate, true) 2322 && compareDeep(restoreDate, o.restoreDate, true) && compareDeep(validityPeriod, o.validityPeriod, true) 2323 && compareDeep(dataExclusivityPeriod, o.dataExclusivityPeriod, true) 2324 && compareDeep(dateOfFirstAuthorization, o.dateOfFirstAuthorization, true) 2325 && compareDeep(internationalBirthDate, o.internationalBirthDate, true) 2326 && compareDeep(legalBasis, o.legalBasis, true) 2327 && compareDeep(jurisdictionalAuthorization, o.jurisdictionalAuthorization, true) 2328 && compareDeep(holder, o.holder, true) && compareDeep(regulator, o.regulator, true) 2329 && compareDeep(procedure, o.procedure, true); 2330 } 2331 2332 @Override 2333 public boolean equalsShallow(Base other_) { 2334 if (!super.equalsShallow(other_)) 2335 return false; 2336 if (!(other_ instanceof MedicinalProductAuthorization)) 2337 return false; 2338 MedicinalProductAuthorization o = (MedicinalProductAuthorization) other_; 2339 return compareValues(statusDate, o.statusDate, true) && compareValues(restoreDate, o.restoreDate, true) 2340 && compareValues(dateOfFirstAuthorization, o.dateOfFirstAuthorization, true) 2341 && compareValues(internationalBirthDate, o.internationalBirthDate, true); 2342 } 2343 2344 public boolean isEmpty() { 2345 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subject, country, jurisdiction, status, 2346 statusDate, restoreDate, validityPeriod, dataExclusivityPeriod, dateOfFirstAuthorization, 2347 internationalBirthDate, legalBasis, jurisdictionalAuthorization, holder, regulator, procedure); 2348 } 2349 2350 @Override 2351 public ResourceType getResourceType() { 2352 return ResourceType.MedicinalProductAuthorization; 2353 } 2354 2355 /** 2356 * Search parameter: <b>identifier</b> 2357 * <p> 2358 * Description: <b>Business identifier for the marketing authorization, as 2359 * assigned by a regulator</b><br> 2360 * Type: <b>token</b><br> 2361 * Path: <b>MedicinalProductAuthorization.identifier</b><br> 2362 * </p> 2363 */ 2364 @SearchParamDefinition(name = "identifier", path = "MedicinalProductAuthorization.identifier", description = "Business identifier for the marketing authorization, as assigned by a regulator", type = "token") 2365 public static final String SP_IDENTIFIER = "identifier"; 2366 /** 2367 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2368 * <p> 2369 * Description: <b>Business identifier for the marketing authorization, as 2370 * assigned by a regulator</b><br> 2371 * Type: <b>token</b><br> 2372 * Path: <b>MedicinalProductAuthorization.identifier</b><br> 2373 * </p> 2374 */ 2375 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2376 SP_IDENTIFIER); 2377 2378 /** 2379 * Search parameter: <b>country</b> 2380 * <p> 2381 * Description: <b>The country in which the marketing authorization has been 2382 * granted</b><br> 2383 * Type: <b>token</b><br> 2384 * Path: <b>MedicinalProductAuthorization.country</b><br> 2385 * </p> 2386 */ 2387 @SearchParamDefinition(name = "country", path = "MedicinalProductAuthorization.country", description = "The country in which the marketing authorization has been granted", type = "token") 2388 public static final String SP_COUNTRY = "country"; 2389 /** 2390 * <b>Fluent Client</b> search parameter constant for <b>country</b> 2391 * <p> 2392 * Description: <b>The country in which the marketing authorization has been 2393 * granted</b><br> 2394 * Type: <b>token</b><br> 2395 * Path: <b>MedicinalProductAuthorization.country</b><br> 2396 * </p> 2397 */ 2398 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COUNTRY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2399 SP_COUNTRY); 2400 2401 /** 2402 * Search parameter: <b>subject</b> 2403 * <p> 2404 * Description: <b>The medicinal product that is being authorized</b><br> 2405 * Type: <b>reference</b><br> 2406 * Path: <b>MedicinalProductAuthorization.subject</b><br> 2407 * </p> 2408 */ 2409 @SearchParamDefinition(name = "subject", path = "MedicinalProductAuthorization.subject", description = "The medicinal product that is being authorized", type = "reference", target = { 2410 MedicinalProduct.class, MedicinalProductPackaged.class }) 2411 public static final String SP_SUBJECT = "subject"; 2412 /** 2413 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2414 * <p> 2415 * Description: <b>The medicinal product that is being authorized</b><br> 2416 * Type: <b>reference</b><br> 2417 * Path: <b>MedicinalProductAuthorization.subject</b><br> 2418 * </p> 2419 */ 2420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2421 SP_SUBJECT); 2422 2423 /** 2424 * Constant for fluent queries to be used to add include statements. Specifies 2425 * the path value of "<b>MedicinalProductAuthorization:subject</b>". 2426 */ 2427 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2428 "MedicinalProductAuthorization:subject").toLocked(); 2429 2430 /** 2431 * Search parameter: <b>holder</b> 2432 * <p> 2433 * Description: <b>Marketing Authorization Holder</b><br> 2434 * Type: <b>reference</b><br> 2435 * Path: <b>MedicinalProductAuthorization.holder</b><br> 2436 * </p> 2437 */ 2438 @SearchParamDefinition(name = "holder", path = "MedicinalProductAuthorization.holder", description = "Marketing Authorization Holder", type = "reference", target = { 2439 Organization.class }) 2440 public static final String SP_HOLDER = "holder"; 2441 /** 2442 * <b>Fluent Client</b> search parameter constant for <b>holder</b> 2443 * <p> 2444 * Description: <b>Marketing Authorization Holder</b><br> 2445 * Type: <b>reference</b><br> 2446 * Path: <b>MedicinalProductAuthorization.holder</b><br> 2447 * </p> 2448 */ 2449 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2450 SP_HOLDER); 2451 2452 /** 2453 * Constant for fluent queries to be used to add include statements. Specifies 2454 * the path value of "<b>MedicinalProductAuthorization:holder</b>". 2455 */ 2456 public static final ca.uhn.fhir.model.api.Include INCLUDE_HOLDER = new ca.uhn.fhir.model.api.Include( 2457 "MedicinalProductAuthorization:holder").toLocked(); 2458 2459 /** 2460 * Search parameter: <b>status</b> 2461 * <p> 2462 * Description: <b>The status of the marketing authorization</b><br> 2463 * Type: <b>token</b><br> 2464 * Path: <b>MedicinalProductAuthorization.status</b><br> 2465 * </p> 2466 */ 2467 @SearchParamDefinition(name = "status", path = "MedicinalProductAuthorization.status", description = "The status of the marketing authorization", type = "token") 2468 public static final String SP_STATUS = "status"; 2469 /** 2470 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2471 * <p> 2472 * Description: <b>The status of the marketing authorization</b><br> 2473 * Type: <b>token</b><br> 2474 * Path: <b>MedicinalProductAuthorization.status</b><br> 2475 * </p> 2476 */ 2477 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2478 SP_STATUS); 2479 2480}