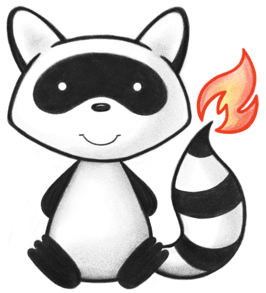
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * The clinical particulars - indications, contraindications etc. of a medicinal 047 * product, including for regulatory purposes. 048 */ 049@ResourceDef(name = "MedicinalProductContraindication", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductContraindication") 050public class MedicinalProductContraindication extends DomainResource { 051 052 @Block() 053 public static class MedicinalProductContraindicationOtherTherapyComponent extends BackboneElement 054 implements IBaseBackboneElement { 055 /** 056 * The type of relationship between the medicinal product indication or 057 * contraindication and another therapy. 058 */ 059 @Child(name = "therapyRelationshipType", type = { 060 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "The type of relationship between the medicinal product indication or contraindication and another therapy", formalDefinition = "The type of relationship between the medicinal product indication or contraindication and another therapy.") 062 protected CodeableConcept therapyRelationshipType; 063 064 /** 065 * Reference to a specific medication (active substance, medicinal product or 066 * class of products) as part of an indication or contraindication. 067 */ 068 @Child(name = "medication", type = { CodeableConcept.class, MedicinalProduct.class, Medication.class, 069 Substance.class, SubstanceSpecification.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 070 @Description(shortDefinition = "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication", formalDefinition = "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.") 071 protected Type medication; 072 073 private static final long serialVersionUID = 1438478115L; 074 075 /** 076 * Constructor 077 */ 078 public MedicinalProductContraindicationOtherTherapyComponent() { 079 super(); 080 } 081 082 /** 083 * Constructor 084 */ 085 public MedicinalProductContraindicationOtherTherapyComponent(CodeableConcept therapyRelationshipType, 086 Type medication) { 087 super(); 088 this.therapyRelationshipType = therapyRelationshipType; 089 this.medication = medication; 090 } 091 092 /** 093 * @return {@link #therapyRelationshipType} (The type of relationship between 094 * the medicinal product indication or contraindication and another 095 * therapy.) 096 */ 097 public CodeableConcept getTherapyRelationshipType() { 098 if (this.therapyRelationshipType == null) 099 if (Configuration.errorOnAutoCreate()) 100 throw new Error( 101 "Attempt to auto-create MedicinalProductContraindicationOtherTherapyComponent.therapyRelationshipType"); 102 else if (Configuration.doAutoCreate()) 103 this.therapyRelationshipType = new CodeableConcept(); // cc 104 return this.therapyRelationshipType; 105 } 106 107 public boolean hasTherapyRelationshipType() { 108 return this.therapyRelationshipType != null && !this.therapyRelationshipType.isEmpty(); 109 } 110 111 /** 112 * @param value {@link #therapyRelationshipType} (The type of relationship 113 * between the medicinal product indication or contraindication and 114 * another therapy.) 115 */ 116 public MedicinalProductContraindicationOtherTherapyComponent setTherapyRelationshipType(CodeableConcept value) { 117 this.therapyRelationshipType = value; 118 return this; 119 } 120 121 /** 122 * @return {@link #medication} (Reference to a specific medication (active 123 * substance, medicinal product or class of products) as part of an 124 * indication or contraindication.) 125 */ 126 public Type getMedication() { 127 return this.medication; 128 } 129 130 /** 131 * @return {@link #medication} (Reference to a specific medication (active 132 * substance, medicinal product or class of products) as part of an 133 * indication or contraindication.) 134 */ 135 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 136 if (this.medication == null) 137 this.medication = new CodeableConcept(); 138 if (!(this.medication instanceof CodeableConcept)) 139 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 140 + this.medication.getClass().getName() + " was encountered"); 141 return (CodeableConcept) this.medication; 142 } 143 144 public boolean hasMedicationCodeableConcept() { 145 return this != null && this.medication instanceof CodeableConcept; 146 } 147 148 /** 149 * @return {@link #medication} (Reference to a specific medication (active 150 * substance, medicinal product or class of products) as part of an 151 * indication or contraindication.) 152 */ 153 public Reference getMedicationReference() throws FHIRException { 154 if (this.medication == null) 155 this.medication = new Reference(); 156 if (!(this.medication instanceof Reference)) 157 throw new FHIRException("Type mismatch: the type Reference was expected, but " 158 + this.medication.getClass().getName() + " was encountered"); 159 return (Reference) this.medication; 160 } 161 162 public boolean hasMedicationReference() { 163 return this != null && this.medication instanceof Reference; 164 } 165 166 public boolean hasMedication() { 167 return this.medication != null && !this.medication.isEmpty(); 168 } 169 170 /** 171 * @param value {@link #medication} (Reference to a specific medication (active 172 * substance, medicinal product or class of products) as part of an 173 * indication or contraindication.) 174 */ 175 public MedicinalProductContraindicationOtherTherapyComponent setMedication(Type value) { 176 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 177 throw new Error( 178 "Not the right type for MedicinalProductContraindication.otherTherapy.medication[x]: " + value.fhirType()); 179 this.medication = value; 180 return this; 181 } 182 183 protected void listChildren(List<Property> children) { 184 super.listChildren(children); 185 children.add(new Property("therapyRelationshipType", "CodeableConcept", 186 "The type of relationship between the medicinal product indication or contraindication and another therapy.", 187 0, 1, therapyRelationshipType)); 188 children.add(new Property("medication[x]", 189 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 190 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 191 0, 1, medication)); 192 } 193 194 @Override 195 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 196 switch (_hash) { 197 case -551658469: 198 /* therapyRelationshipType */ return new Property("therapyRelationshipType", "CodeableConcept", 199 "The type of relationship between the medicinal product indication or contraindication and another therapy.", 200 0, 1, therapyRelationshipType); 201 case 1458402129: 202 /* medication[x] */ return new Property("medication[x]", 203 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 204 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 205 0, 1, medication); 206 case 1998965455: 207 /* medication */ return new Property("medication[x]", 208 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 209 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 210 0, 1, medication); 211 case -209845038: 212 /* medicationCodeableConcept */ return new Property("medication[x]", 213 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 214 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 215 0, 1, medication); 216 case 2104315196: 217 /* medicationReference */ return new Property("medication[x]", 218 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 219 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 220 0, 1, medication); 221 default: 222 return super.getNamedProperty(_hash, _name, _checkValid); 223 } 224 225 } 226 227 @Override 228 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 229 switch (hash) { 230 case -551658469: 231 /* therapyRelationshipType */ return this.therapyRelationshipType == null ? new Base[0] 232 : new Base[] { this.therapyRelationshipType }; // CodeableConcept 233 case 1998965455: 234 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 235 default: 236 return super.getProperty(hash, name, checkValid); 237 } 238 239 } 240 241 @Override 242 public Base setProperty(int hash, String name, Base value) throws FHIRException { 243 switch (hash) { 244 case -551658469: // therapyRelationshipType 245 this.therapyRelationshipType = castToCodeableConcept(value); // CodeableConcept 246 return value; 247 case 1998965455: // medication 248 this.medication = castToType(value); // Type 249 return value; 250 default: 251 return super.setProperty(hash, name, value); 252 } 253 254 } 255 256 @Override 257 public Base setProperty(String name, Base value) throws FHIRException { 258 if (name.equals("therapyRelationshipType")) { 259 this.therapyRelationshipType = castToCodeableConcept(value); // CodeableConcept 260 } else if (name.equals("medication[x]")) { 261 this.medication = castToType(value); // Type 262 } else 263 return super.setProperty(name, value); 264 return value; 265 } 266 267 @Override 268 public void removeChild(String name, Base value) throws FHIRException { 269 if (name.equals("therapyRelationshipType")) { 270 this.therapyRelationshipType = null; 271 } else if (name.equals("medication[x]")) { 272 this.medication = null; 273 } else 274 super.removeChild(name, value); 275 276 } 277 278 @Override 279 public Base makeProperty(int hash, String name) throws FHIRException { 280 switch (hash) { 281 case -551658469: 282 return getTherapyRelationshipType(); 283 case 1458402129: 284 return getMedication(); 285 case 1998965455: 286 return getMedication(); 287 default: 288 return super.makeProperty(hash, name); 289 } 290 291 } 292 293 @Override 294 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 295 switch (hash) { 296 case -551658469: 297 /* therapyRelationshipType */ return new String[] { "CodeableConcept" }; 298 case 1998965455: 299 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 300 default: 301 return super.getTypesForProperty(hash, name); 302 } 303 304 } 305 306 @Override 307 public Base addChild(String name) throws FHIRException { 308 if (name.equals("therapyRelationshipType")) { 309 this.therapyRelationshipType = new CodeableConcept(); 310 return this.therapyRelationshipType; 311 } else if (name.equals("medicationCodeableConcept")) { 312 this.medication = new CodeableConcept(); 313 return this.medication; 314 } else if (name.equals("medicationReference")) { 315 this.medication = new Reference(); 316 return this.medication; 317 } else 318 return super.addChild(name); 319 } 320 321 public MedicinalProductContraindicationOtherTherapyComponent copy() { 322 MedicinalProductContraindicationOtherTherapyComponent dst = new MedicinalProductContraindicationOtherTherapyComponent(); 323 copyValues(dst); 324 return dst; 325 } 326 327 public void copyValues(MedicinalProductContraindicationOtherTherapyComponent dst) { 328 super.copyValues(dst); 329 dst.therapyRelationshipType = therapyRelationshipType == null ? null : therapyRelationshipType.copy(); 330 dst.medication = medication == null ? null : medication.copy(); 331 } 332 333 @Override 334 public boolean equalsDeep(Base other_) { 335 if (!super.equalsDeep(other_)) 336 return false; 337 if (!(other_ instanceof MedicinalProductContraindicationOtherTherapyComponent)) 338 return false; 339 MedicinalProductContraindicationOtherTherapyComponent o = (MedicinalProductContraindicationOtherTherapyComponent) other_; 340 return compareDeep(therapyRelationshipType, o.therapyRelationshipType, true) 341 && compareDeep(medication, o.medication, true); 342 } 343 344 @Override 345 public boolean equalsShallow(Base other_) { 346 if (!super.equalsShallow(other_)) 347 return false; 348 if (!(other_ instanceof MedicinalProductContraindicationOtherTherapyComponent)) 349 return false; 350 MedicinalProductContraindicationOtherTherapyComponent o = (MedicinalProductContraindicationOtherTherapyComponent) other_; 351 return true; 352 } 353 354 public boolean isEmpty() { 355 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(therapyRelationshipType, medication); 356 } 357 358 public String fhirType() { 359 return "MedicinalProductContraindication.otherTherapy"; 360 361 } 362 363 } 364 365 /** 366 * The medication for which this is an indication. 367 */ 368 @Child(name = "subject", type = { MedicinalProduct.class, 369 Medication.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 370 @Description(shortDefinition = "The medication for which this is an indication", formalDefinition = "The medication for which this is an indication.") 371 protected List<Reference> subject; 372 /** 373 * The actual objects that are the target of the reference (The medication for 374 * which this is an indication.) 375 */ 376 protected List<Resource> subjectTarget; 377 378 /** 379 * The disease, symptom or procedure for the contraindication. 380 */ 381 @Child(name = "disease", type = { 382 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 383 @Description(shortDefinition = "The disease, symptom or procedure for the contraindication", formalDefinition = "The disease, symptom or procedure for the contraindication.") 384 protected CodeableConcept disease; 385 386 /** 387 * The status of the disease or symptom for the contraindication. 388 */ 389 @Child(name = "diseaseStatus", type = { 390 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 391 @Description(shortDefinition = "The status of the disease or symptom for the contraindication", formalDefinition = "The status of the disease or symptom for the contraindication.") 392 protected CodeableConcept diseaseStatus; 393 394 /** 395 * A comorbidity (concurrent condition) or coinfection. 396 */ 397 @Child(name = "comorbidity", type = { 398 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 399 @Description(shortDefinition = "A comorbidity (concurrent condition) or coinfection", formalDefinition = "A comorbidity (concurrent condition) or coinfection.") 400 protected List<CodeableConcept> comorbidity; 401 402 /** 403 * Information about the use of the medicinal product in relation to other 404 * therapies as part of the indication. 405 */ 406 @Child(name = "therapeuticIndication", type = { 407 MedicinalProductIndication.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 408 @Description(shortDefinition = "Information about the use of the medicinal product in relation to other therapies as part of the indication", formalDefinition = "Information about the use of the medicinal product in relation to other therapies as part of the indication.") 409 protected List<Reference> therapeuticIndication; 410 /** 411 * The actual objects that are the target of the reference (Information about 412 * the use of the medicinal product in relation to other therapies as part of 413 * the indication.) 414 */ 415 protected List<MedicinalProductIndication> therapeuticIndicationTarget; 416 417 /** 418 * Information about the use of the medicinal product in relation to other 419 * therapies described as part of the indication. 420 */ 421 @Child(name = "otherTherapy", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 422 @Description(shortDefinition = "Information about the use of the medicinal product in relation to other therapies described as part of the indication", formalDefinition = "Information about the use of the medicinal product in relation to other therapies described as part of the indication.") 423 protected List<MedicinalProductContraindicationOtherTherapyComponent> otherTherapy; 424 425 /** 426 * The population group to which this applies. 427 */ 428 @Child(name = "population", type = { 429 Population.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 430 @Description(shortDefinition = "The population group to which this applies", formalDefinition = "The population group to which this applies.") 431 protected List<Population> population; 432 433 private static final long serialVersionUID = -1746103034L; 434 435 /** 436 * Constructor 437 */ 438 public MedicinalProductContraindication() { 439 super(); 440 } 441 442 /** 443 * @return {@link #subject} (The medication for which this is an indication.) 444 */ 445 public List<Reference> getSubject() { 446 if (this.subject == null) 447 this.subject = new ArrayList<Reference>(); 448 return this.subject; 449 } 450 451 /** 452 * @return Returns a reference to <code>this</code> for easy method chaining 453 */ 454 public MedicinalProductContraindication setSubject(List<Reference> theSubject) { 455 this.subject = theSubject; 456 return this; 457 } 458 459 public boolean hasSubject() { 460 if (this.subject == null) 461 return false; 462 for (Reference item : this.subject) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public Reference addSubject() { // 3 469 Reference t = new Reference(); 470 if (this.subject == null) 471 this.subject = new ArrayList<Reference>(); 472 this.subject.add(t); 473 return t; 474 } 475 476 public MedicinalProductContraindication addSubject(Reference t) { // 3 477 if (t == null) 478 return this; 479 if (this.subject == null) 480 this.subject = new ArrayList<Reference>(); 481 this.subject.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #subject}, creating it 487 * if it does not already exist 488 */ 489 public Reference getSubjectFirstRep() { 490 if (getSubject().isEmpty()) { 491 addSubject(); 492 } 493 return getSubject().get(0); 494 } 495 496 /** 497 * @deprecated Use Reference#setResource(IBaseResource) instead 498 */ 499 @Deprecated 500 public List<Resource> getSubjectTarget() { 501 if (this.subjectTarget == null) 502 this.subjectTarget = new ArrayList<Resource>(); 503 return this.subjectTarget; 504 } 505 506 /** 507 * @return {@link #disease} (The disease, symptom or procedure for the 508 * contraindication.) 509 */ 510 public CodeableConcept getDisease() { 511 if (this.disease == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create MedicinalProductContraindication.disease"); 514 else if (Configuration.doAutoCreate()) 515 this.disease = new CodeableConcept(); // cc 516 return this.disease; 517 } 518 519 public boolean hasDisease() { 520 return this.disease != null && !this.disease.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #disease} (The disease, symptom or procedure for the 525 * contraindication.) 526 */ 527 public MedicinalProductContraindication setDisease(CodeableConcept value) { 528 this.disease = value; 529 return this; 530 } 531 532 /** 533 * @return {@link #diseaseStatus} (The status of the disease or symptom for the 534 * contraindication.) 535 */ 536 public CodeableConcept getDiseaseStatus() { 537 if (this.diseaseStatus == null) 538 if (Configuration.errorOnAutoCreate()) 539 throw new Error("Attempt to auto-create MedicinalProductContraindication.diseaseStatus"); 540 else if (Configuration.doAutoCreate()) 541 this.diseaseStatus = new CodeableConcept(); // cc 542 return this.diseaseStatus; 543 } 544 545 public boolean hasDiseaseStatus() { 546 return this.diseaseStatus != null && !this.diseaseStatus.isEmpty(); 547 } 548 549 /** 550 * @param value {@link #diseaseStatus} (The status of the disease or symptom for 551 * the contraindication.) 552 */ 553 public MedicinalProductContraindication setDiseaseStatus(CodeableConcept value) { 554 this.diseaseStatus = value; 555 return this; 556 } 557 558 /** 559 * @return {@link #comorbidity} (A comorbidity (concurrent condition) or 560 * coinfection.) 561 */ 562 public List<CodeableConcept> getComorbidity() { 563 if (this.comorbidity == null) 564 this.comorbidity = new ArrayList<CodeableConcept>(); 565 return this.comorbidity; 566 } 567 568 /** 569 * @return Returns a reference to <code>this</code> for easy method chaining 570 */ 571 public MedicinalProductContraindication setComorbidity(List<CodeableConcept> theComorbidity) { 572 this.comorbidity = theComorbidity; 573 return this; 574 } 575 576 public boolean hasComorbidity() { 577 if (this.comorbidity == null) 578 return false; 579 for (CodeableConcept item : this.comorbidity) 580 if (!item.isEmpty()) 581 return true; 582 return false; 583 } 584 585 public CodeableConcept addComorbidity() { // 3 586 CodeableConcept t = new CodeableConcept(); 587 if (this.comorbidity == null) 588 this.comorbidity = new ArrayList<CodeableConcept>(); 589 this.comorbidity.add(t); 590 return t; 591 } 592 593 public MedicinalProductContraindication addComorbidity(CodeableConcept t) { // 3 594 if (t == null) 595 return this; 596 if (this.comorbidity == null) 597 this.comorbidity = new ArrayList<CodeableConcept>(); 598 this.comorbidity.add(t); 599 return this; 600 } 601 602 /** 603 * @return The first repetition of repeating field {@link #comorbidity}, 604 * creating it if it does not already exist 605 */ 606 public CodeableConcept getComorbidityFirstRep() { 607 if (getComorbidity().isEmpty()) { 608 addComorbidity(); 609 } 610 return getComorbidity().get(0); 611 } 612 613 /** 614 * @return {@link #therapeuticIndication} (Information about the use of the 615 * medicinal product in relation to other therapies as part of the 616 * indication.) 617 */ 618 public List<Reference> getTherapeuticIndication() { 619 if (this.therapeuticIndication == null) 620 this.therapeuticIndication = new ArrayList<Reference>(); 621 return this.therapeuticIndication; 622 } 623 624 /** 625 * @return Returns a reference to <code>this</code> for easy method chaining 626 */ 627 public MedicinalProductContraindication setTherapeuticIndication(List<Reference> theTherapeuticIndication) { 628 this.therapeuticIndication = theTherapeuticIndication; 629 return this; 630 } 631 632 public boolean hasTherapeuticIndication() { 633 if (this.therapeuticIndication == null) 634 return false; 635 for (Reference item : this.therapeuticIndication) 636 if (!item.isEmpty()) 637 return true; 638 return false; 639 } 640 641 public Reference addTherapeuticIndication() { // 3 642 Reference t = new Reference(); 643 if (this.therapeuticIndication == null) 644 this.therapeuticIndication = new ArrayList<Reference>(); 645 this.therapeuticIndication.add(t); 646 return t; 647 } 648 649 public MedicinalProductContraindication addTherapeuticIndication(Reference t) { // 3 650 if (t == null) 651 return this; 652 if (this.therapeuticIndication == null) 653 this.therapeuticIndication = new ArrayList<Reference>(); 654 this.therapeuticIndication.add(t); 655 return this; 656 } 657 658 /** 659 * @return The first repetition of repeating field 660 * {@link #therapeuticIndication}, creating it if it does not already 661 * exist 662 */ 663 public Reference getTherapeuticIndicationFirstRep() { 664 if (getTherapeuticIndication().isEmpty()) { 665 addTherapeuticIndication(); 666 } 667 return getTherapeuticIndication().get(0); 668 } 669 670 /** 671 * @deprecated Use Reference#setResource(IBaseResource) instead 672 */ 673 @Deprecated 674 public List<MedicinalProductIndication> getTherapeuticIndicationTarget() { 675 if (this.therapeuticIndicationTarget == null) 676 this.therapeuticIndicationTarget = new ArrayList<MedicinalProductIndication>(); 677 return this.therapeuticIndicationTarget; 678 } 679 680 /** 681 * @deprecated Use Reference#setResource(IBaseResource) instead 682 */ 683 @Deprecated 684 public MedicinalProductIndication addTherapeuticIndicationTarget() { 685 MedicinalProductIndication r = new MedicinalProductIndication(); 686 if (this.therapeuticIndicationTarget == null) 687 this.therapeuticIndicationTarget = new ArrayList<MedicinalProductIndication>(); 688 this.therapeuticIndicationTarget.add(r); 689 return r; 690 } 691 692 /** 693 * @return {@link #otherTherapy} (Information about the use of the medicinal 694 * product in relation to other therapies described as part of the 695 * indication.) 696 */ 697 public List<MedicinalProductContraindicationOtherTherapyComponent> getOtherTherapy() { 698 if (this.otherTherapy == null) 699 this.otherTherapy = new ArrayList<MedicinalProductContraindicationOtherTherapyComponent>(); 700 return this.otherTherapy; 701 } 702 703 /** 704 * @return Returns a reference to <code>this</code> for easy method chaining 705 */ 706 public MedicinalProductContraindication setOtherTherapy( 707 List<MedicinalProductContraindicationOtherTherapyComponent> theOtherTherapy) { 708 this.otherTherapy = theOtherTherapy; 709 return this; 710 } 711 712 public boolean hasOtherTherapy() { 713 if (this.otherTherapy == null) 714 return false; 715 for (MedicinalProductContraindicationOtherTherapyComponent item : this.otherTherapy) 716 if (!item.isEmpty()) 717 return true; 718 return false; 719 } 720 721 public MedicinalProductContraindicationOtherTherapyComponent addOtherTherapy() { // 3 722 MedicinalProductContraindicationOtherTherapyComponent t = new MedicinalProductContraindicationOtherTherapyComponent(); 723 if (this.otherTherapy == null) 724 this.otherTherapy = new ArrayList<MedicinalProductContraindicationOtherTherapyComponent>(); 725 this.otherTherapy.add(t); 726 return t; 727 } 728 729 public MedicinalProductContraindication addOtherTherapy(MedicinalProductContraindicationOtherTherapyComponent t) { // 3 730 if (t == null) 731 return this; 732 if (this.otherTherapy == null) 733 this.otherTherapy = new ArrayList<MedicinalProductContraindicationOtherTherapyComponent>(); 734 this.otherTherapy.add(t); 735 return this; 736 } 737 738 /** 739 * @return The first repetition of repeating field {@link #otherTherapy}, 740 * creating it if it does not already exist 741 */ 742 public MedicinalProductContraindicationOtherTherapyComponent getOtherTherapyFirstRep() { 743 if (getOtherTherapy().isEmpty()) { 744 addOtherTherapy(); 745 } 746 return getOtherTherapy().get(0); 747 } 748 749 /** 750 * @return {@link #population} (The population group to which this applies.) 751 */ 752 public List<Population> getPopulation() { 753 if (this.population == null) 754 this.population = new ArrayList<Population>(); 755 return this.population; 756 } 757 758 /** 759 * @return Returns a reference to <code>this</code> for easy method chaining 760 */ 761 public MedicinalProductContraindication setPopulation(List<Population> thePopulation) { 762 this.population = thePopulation; 763 return this; 764 } 765 766 public boolean hasPopulation() { 767 if (this.population == null) 768 return false; 769 for (Population item : this.population) 770 if (!item.isEmpty()) 771 return true; 772 return false; 773 } 774 775 public Population addPopulation() { // 3 776 Population t = new Population(); 777 if (this.population == null) 778 this.population = new ArrayList<Population>(); 779 this.population.add(t); 780 return t; 781 } 782 783 public MedicinalProductContraindication addPopulation(Population t) { // 3 784 if (t == null) 785 return this; 786 if (this.population == null) 787 this.population = new ArrayList<Population>(); 788 this.population.add(t); 789 return this; 790 } 791 792 /** 793 * @return The first repetition of repeating field {@link #population}, creating 794 * it if it does not already exist 795 */ 796 public Population getPopulationFirstRep() { 797 if (getPopulation().isEmpty()) { 798 addPopulation(); 799 } 800 return getPopulation().get(0); 801 } 802 803 protected void listChildren(List<Property> children) { 804 super.listChildren(children); 805 children.add(new Property("subject", "Reference(MedicinalProduct|Medication)", 806 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject)); 807 children.add(new Property("disease", "CodeableConcept", 808 "The disease, symptom or procedure for the contraindication.", 0, 1, disease)); 809 children.add(new Property("diseaseStatus", "CodeableConcept", 810 "The status of the disease or symptom for the contraindication.", 0, 1, diseaseStatus)); 811 children.add(new Property("comorbidity", "CodeableConcept", "A comorbidity (concurrent condition) or coinfection.", 812 0, java.lang.Integer.MAX_VALUE, comorbidity)); 813 children.add(new Property("therapeuticIndication", "Reference(MedicinalProductIndication)", 814 "Information about the use of the medicinal product in relation to other therapies as part of the indication.", 815 0, java.lang.Integer.MAX_VALUE, therapeuticIndication)); 816 children.add(new Property("otherTherapy", "", 817 "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 818 0, java.lang.Integer.MAX_VALUE, otherTherapy)); 819 children.add(new Property("population", "Population", "The population group to which this applies.", 0, 820 java.lang.Integer.MAX_VALUE, population)); 821 } 822 823 @Override 824 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 825 switch (_hash) { 826 case -1867885268: 827 /* subject */ return new Property("subject", "Reference(MedicinalProduct|Medication)", 828 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject); 829 case 1671426428: 830 /* disease */ return new Property("disease", "CodeableConcept", 831 "The disease, symptom or procedure for the contraindication.", 0, 1, disease); 832 case -505503602: 833 /* diseaseStatus */ return new Property("diseaseStatus", "CodeableConcept", 834 "The status of the disease or symptom for the contraindication.", 0, 1, diseaseStatus); 835 case -406395211: 836 /* comorbidity */ return new Property("comorbidity", "CodeableConcept", 837 "A comorbidity (concurrent condition) or coinfection.", 0, java.lang.Integer.MAX_VALUE, comorbidity); 838 case -1925150262: 839 /* therapeuticIndication */ return new Property("therapeuticIndication", "Reference(MedicinalProductIndication)", 840 "Information about the use of the medicinal product in relation to other therapies as part of the indication.", 841 0, java.lang.Integer.MAX_VALUE, therapeuticIndication); 842 case -544509127: 843 /* otherTherapy */ return new Property("otherTherapy", "", 844 "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 845 0, java.lang.Integer.MAX_VALUE, otherTherapy); 846 case -2023558323: 847 /* population */ return new Property("population", "Population", "The population group to which this applies.", 0, 848 java.lang.Integer.MAX_VALUE, population); 849 default: 850 return super.getNamedProperty(_hash, _name, _checkValid); 851 } 852 853 } 854 855 @Override 856 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 857 switch (hash) { 858 case -1867885268: 859 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 860 case 1671426428: 861 /* disease */ return this.disease == null ? new Base[0] : new Base[] { this.disease }; // CodeableConcept 862 case -505503602: 863 /* diseaseStatus */ return this.diseaseStatus == null ? new Base[0] : new Base[] { this.diseaseStatus }; // CodeableConcept 864 case -406395211: 865 /* comorbidity */ return this.comorbidity == null ? new Base[0] 866 : this.comorbidity.toArray(new Base[this.comorbidity.size()]); // CodeableConcept 867 case -1925150262: 868 /* therapeuticIndication */ return this.therapeuticIndication == null ? new Base[0] 869 : this.therapeuticIndication.toArray(new Base[this.therapeuticIndication.size()]); // Reference 870 case -544509127: 871 /* otherTherapy */ return this.otherTherapy == null ? new Base[0] 872 : this.otherTherapy.toArray(new Base[this.otherTherapy.size()]); // MedicinalProductContraindicationOtherTherapyComponent 873 case -2023558323: 874 /* population */ return this.population == null ? new Base[0] 875 : this.population.toArray(new Base[this.population.size()]); // Population 876 default: 877 return super.getProperty(hash, name, checkValid); 878 } 879 880 } 881 882 @Override 883 public Base setProperty(int hash, String name, Base value) throws FHIRException { 884 switch (hash) { 885 case -1867885268: // subject 886 this.getSubject().add(castToReference(value)); // Reference 887 return value; 888 case 1671426428: // disease 889 this.disease = castToCodeableConcept(value); // CodeableConcept 890 return value; 891 case -505503602: // diseaseStatus 892 this.diseaseStatus = castToCodeableConcept(value); // CodeableConcept 893 return value; 894 case -406395211: // comorbidity 895 this.getComorbidity().add(castToCodeableConcept(value)); // CodeableConcept 896 return value; 897 case -1925150262: // therapeuticIndication 898 this.getTherapeuticIndication().add(castToReference(value)); // Reference 899 return value; 900 case -544509127: // otherTherapy 901 this.getOtherTherapy().add((MedicinalProductContraindicationOtherTherapyComponent) value); // MedicinalProductContraindicationOtherTherapyComponent 902 return value; 903 case -2023558323: // population 904 this.getPopulation().add(castToPopulation(value)); // Population 905 return value; 906 default: 907 return super.setProperty(hash, name, value); 908 } 909 910 } 911 912 @Override 913 public Base setProperty(String name, Base value) throws FHIRException { 914 if (name.equals("subject")) { 915 this.getSubject().add(castToReference(value)); 916 } else if (name.equals("disease")) { 917 this.disease = castToCodeableConcept(value); // CodeableConcept 918 } else if (name.equals("diseaseStatus")) { 919 this.diseaseStatus = castToCodeableConcept(value); // CodeableConcept 920 } else if (name.equals("comorbidity")) { 921 this.getComorbidity().add(castToCodeableConcept(value)); 922 } else if (name.equals("therapeuticIndication")) { 923 this.getTherapeuticIndication().add(castToReference(value)); 924 } else if (name.equals("otherTherapy")) { 925 this.getOtherTherapy().add((MedicinalProductContraindicationOtherTherapyComponent) value); 926 } else if (name.equals("population")) { 927 this.getPopulation().add(castToPopulation(value)); 928 } else 929 return super.setProperty(name, value); 930 return value; 931 } 932 933 @Override 934 public void removeChild(String name, Base value) throws FHIRException { 935 if (name.equals("subject")) { 936 this.getSubject().remove(castToReference(value)); 937 } else if (name.equals("disease")) { 938 this.disease = null; 939 } else if (name.equals("diseaseStatus")) { 940 this.diseaseStatus = null; 941 } else if (name.equals("comorbidity")) { 942 this.getComorbidity().remove(castToCodeableConcept(value)); 943 } else if (name.equals("therapeuticIndication")) { 944 this.getTherapeuticIndication().remove(castToReference(value)); 945 } else if (name.equals("otherTherapy")) { 946 this.getOtherTherapy().remove((MedicinalProductContraindicationOtherTherapyComponent) value); 947 } else if (name.equals("population")) { 948 this.getPopulation().remove(castToPopulation(value)); 949 } else 950 super.removeChild(name, value); 951 952 } 953 954 @Override 955 public Base makeProperty(int hash, String name) throws FHIRException { 956 switch (hash) { 957 case -1867885268: 958 return addSubject(); 959 case 1671426428: 960 return getDisease(); 961 case -505503602: 962 return getDiseaseStatus(); 963 case -406395211: 964 return addComorbidity(); 965 case -1925150262: 966 return addTherapeuticIndication(); 967 case -544509127: 968 return addOtherTherapy(); 969 case -2023558323: 970 return addPopulation(); 971 default: 972 return super.makeProperty(hash, name); 973 } 974 975 } 976 977 @Override 978 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 979 switch (hash) { 980 case -1867885268: 981 /* subject */ return new String[] { "Reference" }; 982 case 1671426428: 983 /* disease */ return new String[] { "CodeableConcept" }; 984 case -505503602: 985 /* diseaseStatus */ return new String[] { "CodeableConcept" }; 986 case -406395211: 987 /* comorbidity */ return new String[] { "CodeableConcept" }; 988 case -1925150262: 989 /* therapeuticIndication */ return new String[] { "Reference" }; 990 case -544509127: 991 /* otherTherapy */ return new String[] {}; 992 case -2023558323: 993 /* population */ return new String[] { "Population" }; 994 default: 995 return super.getTypesForProperty(hash, name); 996 } 997 998 } 999 1000 @Override 1001 public Base addChild(String name) throws FHIRException { 1002 if (name.equals("subject")) { 1003 return addSubject(); 1004 } else if (name.equals("disease")) { 1005 this.disease = new CodeableConcept(); 1006 return this.disease; 1007 } else if (name.equals("diseaseStatus")) { 1008 this.diseaseStatus = new CodeableConcept(); 1009 return this.diseaseStatus; 1010 } else if (name.equals("comorbidity")) { 1011 return addComorbidity(); 1012 } else if (name.equals("therapeuticIndication")) { 1013 return addTherapeuticIndication(); 1014 } else if (name.equals("otherTherapy")) { 1015 return addOtherTherapy(); 1016 } else if (name.equals("population")) { 1017 return addPopulation(); 1018 } else 1019 return super.addChild(name); 1020 } 1021 1022 public String fhirType() { 1023 return "MedicinalProductContraindication"; 1024 1025 } 1026 1027 public MedicinalProductContraindication copy() { 1028 MedicinalProductContraindication dst = new MedicinalProductContraindication(); 1029 copyValues(dst); 1030 return dst; 1031 } 1032 1033 public void copyValues(MedicinalProductContraindication dst) { 1034 super.copyValues(dst); 1035 if (subject != null) { 1036 dst.subject = new ArrayList<Reference>(); 1037 for (Reference i : subject) 1038 dst.subject.add(i.copy()); 1039 } 1040 ; 1041 dst.disease = disease == null ? null : disease.copy(); 1042 dst.diseaseStatus = diseaseStatus == null ? null : diseaseStatus.copy(); 1043 if (comorbidity != null) { 1044 dst.comorbidity = new ArrayList<CodeableConcept>(); 1045 for (CodeableConcept i : comorbidity) 1046 dst.comorbidity.add(i.copy()); 1047 } 1048 ; 1049 if (therapeuticIndication != null) { 1050 dst.therapeuticIndication = new ArrayList<Reference>(); 1051 for (Reference i : therapeuticIndication) 1052 dst.therapeuticIndication.add(i.copy()); 1053 } 1054 ; 1055 if (otherTherapy != null) { 1056 dst.otherTherapy = new ArrayList<MedicinalProductContraindicationOtherTherapyComponent>(); 1057 for (MedicinalProductContraindicationOtherTherapyComponent i : otherTherapy) 1058 dst.otherTherapy.add(i.copy()); 1059 } 1060 ; 1061 if (population != null) { 1062 dst.population = new ArrayList<Population>(); 1063 for (Population i : population) 1064 dst.population.add(i.copy()); 1065 } 1066 ; 1067 } 1068 1069 protected MedicinalProductContraindication typedCopy() { 1070 return copy(); 1071 } 1072 1073 @Override 1074 public boolean equalsDeep(Base other_) { 1075 if (!super.equalsDeep(other_)) 1076 return false; 1077 if (!(other_ instanceof MedicinalProductContraindication)) 1078 return false; 1079 MedicinalProductContraindication o = (MedicinalProductContraindication) other_; 1080 return compareDeep(subject, o.subject, true) && compareDeep(disease, o.disease, true) 1081 && compareDeep(diseaseStatus, o.diseaseStatus, true) && compareDeep(comorbidity, o.comorbidity, true) 1082 && compareDeep(therapeuticIndication, o.therapeuticIndication, true) 1083 && compareDeep(otherTherapy, o.otherTherapy, true) && compareDeep(population, o.population, true); 1084 } 1085 1086 @Override 1087 public boolean equalsShallow(Base other_) { 1088 if (!super.equalsShallow(other_)) 1089 return false; 1090 if (!(other_ instanceof MedicinalProductContraindication)) 1091 return false; 1092 MedicinalProductContraindication o = (MedicinalProductContraindication) other_; 1093 return true; 1094 } 1095 1096 public boolean isEmpty() { 1097 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subject, disease, diseaseStatus, comorbidity, 1098 therapeuticIndication, otherTherapy, population); 1099 } 1100 1101 @Override 1102 public ResourceType getResourceType() { 1103 return ResourceType.MedicinalProductContraindication; 1104 } 1105 1106 /** 1107 * Search parameter: <b>subject</b> 1108 * <p> 1109 * Description: <b>The medication for which this is an contraindication</b><br> 1110 * Type: <b>reference</b><br> 1111 * Path: <b>MedicinalProductContraindication.subject</b><br> 1112 * </p> 1113 */ 1114 @SearchParamDefinition(name = "subject", path = "MedicinalProductContraindication.subject", description = "The medication for which this is an contraindication", type = "reference", target = { 1115 Medication.class, MedicinalProduct.class }) 1116 public static final String SP_SUBJECT = "subject"; 1117 /** 1118 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1119 * <p> 1120 * Description: <b>The medication for which this is an contraindication</b><br> 1121 * Type: <b>reference</b><br> 1122 * Path: <b>MedicinalProductContraindication.subject</b><br> 1123 * </p> 1124 */ 1125 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1126 SP_SUBJECT); 1127 1128 /** 1129 * Constant for fluent queries to be used to add include statements. Specifies 1130 * the path value of "<b>MedicinalProductContraindication:subject</b>". 1131 */ 1132 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1133 "MedicinalProductContraindication:subject").toLocked(); 1134 1135}