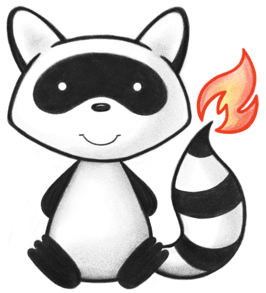
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * Indication for the Medicinal Product. 047 */ 048@ResourceDef(name = "MedicinalProductIndication", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductIndication") 049public class MedicinalProductIndication extends DomainResource { 050 051 @Block() 052 public static class MedicinalProductIndicationOtherTherapyComponent extends BackboneElement 053 implements IBaseBackboneElement { 054 /** 055 * The type of relationship between the medicinal product indication or 056 * contraindication and another therapy. 057 */ 058 @Child(name = "therapyRelationshipType", type = { 059 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "The type of relationship between the medicinal product indication or contraindication and another therapy", formalDefinition = "The type of relationship between the medicinal product indication or contraindication and another therapy.") 061 protected CodeableConcept therapyRelationshipType; 062 063 /** 064 * Reference to a specific medication (active substance, medicinal product or 065 * class of products) as part of an indication or contraindication. 066 */ 067 @Child(name = "medication", type = { CodeableConcept.class, MedicinalProduct.class, Medication.class, 068 Substance.class, SubstanceSpecification.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 069 @Description(shortDefinition = "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication", formalDefinition = "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.") 070 protected Type medication; 071 072 private static final long serialVersionUID = 1438478115L; 073 074 /** 075 * Constructor 076 */ 077 public MedicinalProductIndicationOtherTherapyComponent() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public MedicinalProductIndicationOtherTherapyComponent(CodeableConcept therapyRelationshipType, Type medication) { 085 super(); 086 this.therapyRelationshipType = therapyRelationshipType; 087 this.medication = medication; 088 } 089 090 /** 091 * @return {@link #therapyRelationshipType} (The type of relationship between 092 * the medicinal product indication or contraindication and another 093 * therapy.) 094 */ 095 public CodeableConcept getTherapyRelationshipType() { 096 if (this.therapyRelationshipType == null) 097 if (Configuration.errorOnAutoCreate()) 098 throw new Error( 099 "Attempt to auto-create MedicinalProductIndicationOtherTherapyComponent.therapyRelationshipType"); 100 else if (Configuration.doAutoCreate()) 101 this.therapyRelationshipType = new CodeableConcept(); // cc 102 return this.therapyRelationshipType; 103 } 104 105 public boolean hasTherapyRelationshipType() { 106 return this.therapyRelationshipType != null && !this.therapyRelationshipType.isEmpty(); 107 } 108 109 /** 110 * @param value {@link #therapyRelationshipType} (The type of relationship 111 * between the medicinal product indication or contraindication and 112 * another therapy.) 113 */ 114 public MedicinalProductIndicationOtherTherapyComponent setTherapyRelationshipType(CodeableConcept value) { 115 this.therapyRelationshipType = value; 116 return this; 117 } 118 119 /** 120 * @return {@link #medication} (Reference to a specific medication (active 121 * substance, medicinal product or class of products) as part of an 122 * indication or contraindication.) 123 */ 124 public Type getMedication() { 125 return this.medication; 126 } 127 128 /** 129 * @return {@link #medication} (Reference to a specific medication (active 130 * substance, medicinal product or class of products) as part of an 131 * indication or contraindication.) 132 */ 133 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 134 if (this.medication == null) 135 this.medication = new CodeableConcept(); 136 if (!(this.medication instanceof CodeableConcept)) 137 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 138 + this.medication.getClass().getName() + " was encountered"); 139 return (CodeableConcept) this.medication; 140 } 141 142 public boolean hasMedicationCodeableConcept() { 143 return this != null && this.medication instanceof CodeableConcept; 144 } 145 146 /** 147 * @return {@link #medication} (Reference to a specific medication (active 148 * substance, medicinal product or class of products) as part of an 149 * indication or contraindication.) 150 */ 151 public Reference getMedicationReference() throws FHIRException { 152 if (this.medication == null) 153 this.medication = new Reference(); 154 if (!(this.medication instanceof Reference)) 155 throw new FHIRException("Type mismatch: the type Reference was expected, but " 156 + this.medication.getClass().getName() + " was encountered"); 157 return (Reference) this.medication; 158 } 159 160 public boolean hasMedicationReference() { 161 return this != null && this.medication instanceof Reference; 162 } 163 164 public boolean hasMedication() { 165 return this.medication != null && !this.medication.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #medication} (Reference to a specific medication (active 170 * substance, medicinal product or class of products) as part of an 171 * indication or contraindication.) 172 */ 173 public MedicinalProductIndicationOtherTherapyComponent setMedication(Type value) { 174 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 175 throw new Error( 176 "Not the right type for MedicinalProductIndication.otherTherapy.medication[x]: " + value.fhirType()); 177 this.medication = value; 178 return this; 179 } 180 181 protected void listChildren(List<Property> children) { 182 super.listChildren(children); 183 children.add(new Property("therapyRelationshipType", "CodeableConcept", 184 "The type of relationship between the medicinal product indication or contraindication and another therapy.", 185 0, 1, therapyRelationshipType)); 186 children.add(new Property("medication[x]", 187 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 188 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 189 0, 1, medication)); 190 } 191 192 @Override 193 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 194 switch (_hash) { 195 case -551658469: 196 /* therapyRelationshipType */ return new Property("therapyRelationshipType", "CodeableConcept", 197 "The type of relationship between the medicinal product indication or contraindication and another therapy.", 198 0, 1, therapyRelationshipType); 199 case 1458402129: 200 /* medication[x] */ return new Property("medication[x]", 201 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 202 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 203 0, 1, medication); 204 case 1998965455: 205 /* medication */ return new Property("medication[x]", 206 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 207 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 208 0, 1, medication); 209 case -209845038: 210 /* medicationCodeableConcept */ return new Property("medication[x]", 211 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 212 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 213 0, 1, medication); 214 case 2104315196: 215 /* medicationReference */ return new Property("medication[x]", 216 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 217 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 218 0, 1, medication); 219 default: 220 return super.getNamedProperty(_hash, _name, _checkValid); 221 } 222 223 } 224 225 @Override 226 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 227 switch (hash) { 228 case -551658469: 229 /* therapyRelationshipType */ return this.therapyRelationshipType == null ? new Base[0] 230 : new Base[] { this.therapyRelationshipType }; // CodeableConcept 231 case 1998965455: 232 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 233 default: 234 return super.getProperty(hash, name, checkValid); 235 } 236 237 } 238 239 @Override 240 public Base setProperty(int hash, String name, Base value) throws FHIRException { 241 switch (hash) { 242 case -551658469: // therapyRelationshipType 243 this.therapyRelationshipType = castToCodeableConcept(value); // CodeableConcept 244 return value; 245 case 1998965455: // medication 246 this.medication = castToType(value); // Type 247 return value; 248 default: 249 return super.setProperty(hash, name, value); 250 } 251 252 } 253 254 @Override 255 public Base setProperty(String name, Base value) throws FHIRException { 256 if (name.equals("therapyRelationshipType")) { 257 this.therapyRelationshipType = castToCodeableConcept(value); // CodeableConcept 258 } else if (name.equals("medication[x]")) { 259 this.medication = castToType(value); // Type 260 } else 261 return super.setProperty(name, value); 262 return value; 263 } 264 265 @Override 266 public void removeChild(String name, Base value) throws FHIRException { 267 if (name.equals("therapyRelationshipType")) { 268 this.therapyRelationshipType = null; 269 } else if (name.equals("medication[x]")) { 270 this.medication = null; 271 } else 272 super.removeChild(name, value); 273 274 } 275 276 @Override 277 public Base makeProperty(int hash, String name) throws FHIRException { 278 switch (hash) { 279 case -551658469: 280 return getTherapyRelationshipType(); 281 case 1458402129: 282 return getMedication(); 283 case 1998965455: 284 return getMedication(); 285 default: 286 return super.makeProperty(hash, name); 287 } 288 289 } 290 291 @Override 292 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 293 switch (hash) { 294 case -551658469: 295 /* therapyRelationshipType */ return new String[] { "CodeableConcept" }; 296 case 1998965455: 297 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 298 default: 299 return super.getTypesForProperty(hash, name); 300 } 301 302 } 303 304 @Override 305 public Base addChild(String name) throws FHIRException { 306 if (name.equals("therapyRelationshipType")) { 307 this.therapyRelationshipType = new CodeableConcept(); 308 return this.therapyRelationshipType; 309 } else if (name.equals("medicationCodeableConcept")) { 310 this.medication = new CodeableConcept(); 311 return this.medication; 312 } else if (name.equals("medicationReference")) { 313 this.medication = new Reference(); 314 return this.medication; 315 } else 316 return super.addChild(name); 317 } 318 319 public MedicinalProductIndicationOtherTherapyComponent copy() { 320 MedicinalProductIndicationOtherTherapyComponent dst = new MedicinalProductIndicationOtherTherapyComponent(); 321 copyValues(dst); 322 return dst; 323 } 324 325 public void copyValues(MedicinalProductIndicationOtherTherapyComponent dst) { 326 super.copyValues(dst); 327 dst.therapyRelationshipType = therapyRelationshipType == null ? null : therapyRelationshipType.copy(); 328 dst.medication = medication == null ? null : medication.copy(); 329 } 330 331 @Override 332 public boolean equalsDeep(Base other_) { 333 if (!super.equalsDeep(other_)) 334 return false; 335 if (!(other_ instanceof MedicinalProductIndicationOtherTherapyComponent)) 336 return false; 337 MedicinalProductIndicationOtherTherapyComponent o = (MedicinalProductIndicationOtherTherapyComponent) other_; 338 return compareDeep(therapyRelationshipType, o.therapyRelationshipType, true) 339 && compareDeep(medication, o.medication, true); 340 } 341 342 @Override 343 public boolean equalsShallow(Base other_) { 344 if (!super.equalsShallow(other_)) 345 return false; 346 if (!(other_ instanceof MedicinalProductIndicationOtherTherapyComponent)) 347 return false; 348 MedicinalProductIndicationOtherTherapyComponent o = (MedicinalProductIndicationOtherTherapyComponent) other_; 349 return true; 350 } 351 352 public boolean isEmpty() { 353 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(therapyRelationshipType, medication); 354 } 355 356 public String fhirType() { 357 return "MedicinalProductIndication.otherTherapy"; 358 359 } 360 361 } 362 363 /** 364 * The medication for which this is an indication. 365 */ 366 @Child(name = "subject", type = { MedicinalProduct.class, 367 Medication.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 368 @Description(shortDefinition = "The medication for which this is an indication", formalDefinition = "The medication for which this is an indication.") 369 protected List<Reference> subject; 370 /** 371 * The actual objects that are the target of the reference (The medication for 372 * which this is an indication.) 373 */ 374 protected List<Resource> subjectTarget; 375 376 /** 377 * The disease, symptom or procedure that is the indication for treatment. 378 */ 379 @Child(name = "diseaseSymptomProcedure", type = { 380 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 381 @Description(shortDefinition = "The disease, symptom or procedure that is the indication for treatment", formalDefinition = "The disease, symptom or procedure that is the indication for treatment.") 382 protected CodeableConcept diseaseSymptomProcedure; 383 384 /** 385 * The status of the disease or symptom for which the indication applies. 386 */ 387 @Child(name = "diseaseStatus", type = { 388 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 389 @Description(shortDefinition = "The status of the disease or symptom for which the indication applies", formalDefinition = "The status of the disease or symptom for which the indication applies.") 390 protected CodeableConcept diseaseStatus; 391 392 /** 393 * Comorbidity (concurrent condition) or co-infection as part of the indication. 394 */ 395 @Child(name = "comorbidity", type = { 396 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 397 @Description(shortDefinition = "Comorbidity (concurrent condition) or co-infection as part of the indication", formalDefinition = "Comorbidity (concurrent condition) or co-infection as part of the indication.") 398 protected List<CodeableConcept> comorbidity; 399 400 /** 401 * The intended effect, aim or strategy to be achieved by the indication. 402 */ 403 @Child(name = "intendedEffect", type = { 404 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 405 @Description(shortDefinition = "The intended effect, aim or strategy to be achieved by the indication", formalDefinition = "The intended effect, aim or strategy to be achieved by the indication.") 406 protected CodeableConcept intendedEffect; 407 408 /** 409 * Timing or duration information as part of the indication. 410 */ 411 @Child(name = "duration", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 412 @Description(shortDefinition = "Timing or duration information as part of the indication", formalDefinition = "Timing or duration information as part of the indication.") 413 protected Quantity duration; 414 415 /** 416 * Information about the use of the medicinal product in relation to other 417 * therapies described as part of the indication. 418 */ 419 @Child(name = "otherTherapy", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 420 @Description(shortDefinition = "Information about the use of the medicinal product in relation to other therapies described as part of the indication", formalDefinition = "Information about the use of the medicinal product in relation to other therapies described as part of the indication.") 421 protected List<MedicinalProductIndicationOtherTherapyComponent> otherTherapy; 422 423 /** 424 * Describe the undesirable effects of the medicinal product. 425 */ 426 @Child(name = "undesirableEffect", type = { 427 MedicinalProductUndesirableEffect.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 428 @Description(shortDefinition = "Describe the undesirable effects of the medicinal product", formalDefinition = "Describe the undesirable effects of the medicinal product.") 429 protected List<Reference> undesirableEffect; 430 /** 431 * The actual objects that are the target of the reference (Describe the 432 * undesirable effects of the medicinal product.) 433 */ 434 protected List<MedicinalProductUndesirableEffect> undesirableEffectTarget; 435 436 /** 437 * The population group to which this applies. 438 */ 439 @Child(name = "population", type = { 440 Population.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 441 @Description(shortDefinition = "The population group to which this applies", formalDefinition = "The population group to which this applies.") 442 protected List<Population> population; 443 444 private static final long serialVersionUID = 1205519664L; 445 446 /** 447 * Constructor 448 */ 449 public MedicinalProductIndication() { 450 super(); 451 } 452 453 /** 454 * @return {@link #subject} (The medication for which this is an indication.) 455 */ 456 public List<Reference> getSubject() { 457 if (this.subject == null) 458 this.subject = new ArrayList<Reference>(); 459 return this.subject; 460 } 461 462 /** 463 * @return Returns a reference to <code>this</code> for easy method chaining 464 */ 465 public MedicinalProductIndication setSubject(List<Reference> theSubject) { 466 this.subject = theSubject; 467 return this; 468 } 469 470 public boolean hasSubject() { 471 if (this.subject == null) 472 return false; 473 for (Reference item : this.subject) 474 if (!item.isEmpty()) 475 return true; 476 return false; 477 } 478 479 public Reference addSubject() { // 3 480 Reference t = new Reference(); 481 if (this.subject == null) 482 this.subject = new ArrayList<Reference>(); 483 this.subject.add(t); 484 return t; 485 } 486 487 public MedicinalProductIndication addSubject(Reference t) { // 3 488 if (t == null) 489 return this; 490 if (this.subject == null) 491 this.subject = new ArrayList<Reference>(); 492 this.subject.add(t); 493 return this; 494 } 495 496 /** 497 * @return The first repetition of repeating field {@link #subject}, creating it 498 * if it does not already exist 499 */ 500 public Reference getSubjectFirstRep() { 501 if (getSubject().isEmpty()) { 502 addSubject(); 503 } 504 return getSubject().get(0); 505 } 506 507 /** 508 * @deprecated Use Reference#setResource(IBaseResource) instead 509 */ 510 @Deprecated 511 public List<Resource> getSubjectTarget() { 512 if (this.subjectTarget == null) 513 this.subjectTarget = new ArrayList<Resource>(); 514 return this.subjectTarget; 515 } 516 517 /** 518 * @return {@link #diseaseSymptomProcedure} (The disease, symptom or procedure 519 * that is the indication for treatment.) 520 */ 521 public CodeableConcept getDiseaseSymptomProcedure() { 522 if (this.diseaseSymptomProcedure == null) 523 if (Configuration.errorOnAutoCreate()) 524 throw new Error("Attempt to auto-create MedicinalProductIndication.diseaseSymptomProcedure"); 525 else if (Configuration.doAutoCreate()) 526 this.diseaseSymptomProcedure = new CodeableConcept(); // cc 527 return this.diseaseSymptomProcedure; 528 } 529 530 public boolean hasDiseaseSymptomProcedure() { 531 return this.diseaseSymptomProcedure != null && !this.diseaseSymptomProcedure.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #diseaseSymptomProcedure} (The disease, symptom or 536 * procedure that is the indication for treatment.) 537 */ 538 public MedicinalProductIndication setDiseaseSymptomProcedure(CodeableConcept value) { 539 this.diseaseSymptomProcedure = value; 540 return this; 541 } 542 543 /** 544 * @return {@link #diseaseStatus} (The status of the disease or symptom for 545 * which the indication applies.) 546 */ 547 public CodeableConcept getDiseaseStatus() { 548 if (this.diseaseStatus == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create MedicinalProductIndication.diseaseStatus"); 551 else if (Configuration.doAutoCreate()) 552 this.diseaseStatus = new CodeableConcept(); // cc 553 return this.diseaseStatus; 554 } 555 556 public boolean hasDiseaseStatus() { 557 return this.diseaseStatus != null && !this.diseaseStatus.isEmpty(); 558 } 559 560 /** 561 * @param value {@link #diseaseStatus} (The status of the disease or symptom for 562 * which the indication applies.) 563 */ 564 public MedicinalProductIndication setDiseaseStatus(CodeableConcept value) { 565 this.diseaseStatus = value; 566 return this; 567 } 568 569 /** 570 * @return {@link #comorbidity} (Comorbidity (concurrent condition) or 571 * co-infection as part of the indication.) 572 */ 573 public List<CodeableConcept> getComorbidity() { 574 if (this.comorbidity == null) 575 this.comorbidity = new ArrayList<CodeableConcept>(); 576 return this.comorbidity; 577 } 578 579 /** 580 * @return Returns a reference to <code>this</code> for easy method chaining 581 */ 582 public MedicinalProductIndication setComorbidity(List<CodeableConcept> theComorbidity) { 583 this.comorbidity = theComorbidity; 584 return this; 585 } 586 587 public boolean hasComorbidity() { 588 if (this.comorbidity == null) 589 return false; 590 for (CodeableConcept item : this.comorbidity) 591 if (!item.isEmpty()) 592 return true; 593 return false; 594 } 595 596 public CodeableConcept addComorbidity() { // 3 597 CodeableConcept t = new CodeableConcept(); 598 if (this.comorbidity == null) 599 this.comorbidity = new ArrayList<CodeableConcept>(); 600 this.comorbidity.add(t); 601 return t; 602 } 603 604 public MedicinalProductIndication addComorbidity(CodeableConcept t) { // 3 605 if (t == null) 606 return this; 607 if (this.comorbidity == null) 608 this.comorbidity = new ArrayList<CodeableConcept>(); 609 this.comorbidity.add(t); 610 return this; 611 } 612 613 /** 614 * @return The first repetition of repeating field {@link #comorbidity}, 615 * creating it if it does not already exist 616 */ 617 public CodeableConcept getComorbidityFirstRep() { 618 if (getComorbidity().isEmpty()) { 619 addComorbidity(); 620 } 621 return getComorbidity().get(0); 622 } 623 624 /** 625 * @return {@link #intendedEffect} (The intended effect, aim or strategy to be 626 * achieved by the indication.) 627 */ 628 public CodeableConcept getIntendedEffect() { 629 if (this.intendedEffect == null) 630 if (Configuration.errorOnAutoCreate()) 631 throw new Error("Attempt to auto-create MedicinalProductIndication.intendedEffect"); 632 else if (Configuration.doAutoCreate()) 633 this.intendedEffect = new CodeableConcept(); // cc 634 return this.intendedEffect; 635 } 636 637 public boolean hasIntendedEffect() { 638 return this.intendedEffect != null && !this.intendedEffect.isEmpty(); 639 } 640 641 /** 642 * @param value {@link #intendedEffect} (The intended effect, aim or strategy to 643 * be achieved by the indication.) 644 */ 645 public MedicinalProductIndication setIntendedEffect(CodeableConcept value) { 646 this.intendedEffect = value; 647 return this; 648 } 649 650 /** 651 * @return {@link #duration} (Timing or duration information as part of the 652 * indication.) 653 */ 654 public Quantity getDuration() { 655 if (this.duration == null) 656 if (Configuration.errorOnAutoCreate()) 657 throw new Error("Attempt to auto-create MedicinalProductIndication.duration"); 658 else if (Configuration.doAutoCreate()) 659 this.duration = new Quantity(); // cc 660 return this.duration; 661 } 662 663 public boolean hasDuration() { 664 return this.duration != null && !this.duration.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #duration} (Timing or duration information as part of the 669 * indication.) 670 */ 671 public MedicinalProductIndication setDuration(Quantity value) { 672 this.duration = value; 673 return this; 674 } 675 676 /** 677 * @return {@link #otherTherapy} (Information about the use of the medicinal 678 * product in relation to other therapies described as part of the 679 * indication.) 680 */ 681 public List<MedicinalProductIndicationOtherTherapyComponent> getOtherTherapy() { 682 if (this.otherTherapy == null) 683 this.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 684 return this.otherTherapy; 685 } 686 687 /** 688 * @return Returns a reference to <code>this</code> for easy method chaining 689 */ 690 public MedicinalProductIndication setOtherTherapy( 691 List<MedicinalProductIndicationOtherTherapyComponent> theOtherTherapy) { 692 this.otherTherapy = theOtherTherapy; 693 return this; 694 } 695 696 public boolean hasOtherTherapy() { 697 if (this.otherTherapy == null) 698 return false; 699 for (MedicinalProductIndicationOtherTherapyComponent item : this.otherTherapy) 700 if (!item.isEmpty()) 701 return true; 702 return false; 703 } 704 705 public MedicinalProductIndicationOtherTherapyComponent addOtherTherapy() { // 3 706 MedicinalProductIndicationOtherTherapyComponent t = new MedicinalProductIndicationOtherTherapyComponent(); 707 if (this.otherTherapy == null) 708 this.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 709 this.otherTherapy.add(t); 710 return t; 711 } 712 713 public MedicinalProductIndication addOtherTherapy(MedicinalProductIndicationOtherTherapyComponent t) { // 3 714 if (t == null) 715 return this; 716 if (this.otherTherapy == null) 717 this.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 718 this.otherTherapy.add(t); 719 return this; 720 } 721 722 /** 723 * @return The first repetition of repeating field {@link #otherTherapy}, 724 * creating it if it does not already exist 725 */ 726 public MedicinalProductIndicationOtherTherapyComponent getOtherTherapyFirstRep() { 727 if (getOtherTherapy().isEmpty()) { 728 addOtherTherapy(); 729 } 730 return getOtherTherapy().get(0); 731 } 732 733 /** 734 * @return {@link #undesirableEffect} (Describe the undesirable effects of the 735 * medicinal product.) 736 */ 737 public List<Reference> getUndesirableEffect() { 738 if (this.undesirableEffect == null) 739 this.undesirableEffect = new ArrayList<Reference>(); 740 return this.undesirableEffect; 741 } 742 743 /** 744 * @return Returns a reference to <code>this</code> for easy method chaining 745 */ 746 public MedicinalProductIndication setUndesirableEffect(List<Reference> theUndesirableEffect) { 747 this.undesirableEffect = theUndesirableEffect; 748 return this; 749 } 750 751 public boolean hasUndesirableEffect() { 752 if (this.undesirableEffect == null) 753 return false; 754 for (Reference item : this.undesirableEffect) 755 if (!item.isEmpty()) 756 return true; 757 return false; 758 } 759 760 public Reference addUndesirableEffect() { // 3 761 Reference t = new Reference(); 762 if (this.undesirableEffect == null) 763 this.undesirableEffect = new ArrayList<Reference>(); 764 this.undesirableEffect.add(t); 765 return t; 766 } 767 768 public MedicinalProductIndication addUndesirableEffect(Reference t) { // 3 769 if (t == null) 770 return this; 771 if (this.undesirableEffect == null) 772 this.undesirableEffect = new ArrayList<Reference>(); 773 this.undesirableEffect.add(t); 774 return this; 775 } 776 777 /** 778 * @return The first repetition of repeating field {@link #undesirableEffect}, 779 * creating it if it does not already exist 780 */ 781 public Reference getUndesirableEffectFirstRep() { 782 if (getUndesirableEffect().isEmpty()) { 783 addUndesirableEffect(); 784 } 785 return getUndesirableEffect().get(0); 786 } 787 788 /** 789 * @deprecated Use Reference#setResource(IBaseResource) instead 790 */ 791 @Deprecated 792 public List<MedicinalProductUndesirableEffect> getUndesirableEffectTarget() { 793 if (this.undesirableEffectTarget == null) 794 this.undesirableEffectTarget = new ArrayList<MedicinalProductUndesirableEffect>(); 795 return this.undesirableEffectTarget; 796 } 797 798 /** 799 * @deprecated Use Reference#setResource(IBaseResource) instead 800 */ 801 @Deprecated 802 public MedicinalProductUndesirableEffect addUndesirableEffectTarget() { 803 MedicinalProductUndesirableEffect r = new MedicinalProductUndesirableEffect(); 804 if (this.undesirableEffectTarget == null) 805 this.undesirableEffectTarget = new ArrayList<MedicinalProductUndesirableEffect>(); 806 this.undesirableEffectTarget.add(r); 807 return r; 808 } 809 810 /** 811 * @return {@link #population} (The population group to which this applies.) 812 */ 813 public List<Population> getPopulation() { 814 if (this.population == null) 815 this.population = new ArrayList<Population>(); 816 return this.population; 817 } 818 819 /** 820 * @return Returns a reference to <code>this</code> for easy method chaining 821 */ 822 public MedicinalProductIndication setPopulation(List<Population> thePopulation) { 823 this.population = thePopulation; 824 return this; 825 } 826 827 public boolean hasPopulation() { 828 if (this.population == null) 829 return false; 830 for (Population item : this.population) 831 if (!item.isEmpty()) 832 return true; 833 return false; 834 } 835 836 public Population addPopulation() { // 3 837 Population t = new Population(); 838 if (this.population == null) 839 this.population = new ArrayList<Population>(); 840 this.population.add(t); 841 return t; 842 } 843 844 public MedicinalProductIndication addPopulation(Population t) { // 3 845 if (t == null) 846 return this; 847 if (this.population == null) 848 this.population = new ArrayList<Population>(); 849 this.population.add(t); 850 return this; 851 } 852 853 /** 854 * @return The first repetition of repeating field {@link #population}, creating 855 * it if it does not already exist 856 */ 857 public Population getPopulationFirstRep() { 858 if (getPopulation().isEmpty()) { 859 addPopulation(); 860 } 861 return getPopulation().get(0); 862 } 863 864 protected void listChildren(List<Property> children) { 865 super.listChildren(children); 866 children.add(new Property("subject", "Reference(MedicinalProduct|Medication)", 867 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject)); 868 children.add(new Property("diseaseSymptomProcedure", "CodeableConcept", 869 "The disease, symptom or procedure that is the indication for treatment.", 0, 1, diseaseSymptomProcedure)); 870 children.add(new Property("diseaseStatus", "CodeableConcept", 871 "The status of the disease or symptom for which the indication applies.", 0, 1, diseaseStatus)); 872 children.add(new Property("comorbidity", "CodeableConcept", 873 "Comorbidity (concurrent condition) or co-infection as part of the indication.", 0, java.lang.Integer.MAX_VALUE, 874 comorbidity)); 875 children.add(new Property("intendedEffect", "CodeableConcept", 876 "The intended effect, aim or strategy to be achieved by the indication.", 0, 1, intendedEffect)); 877 children.add(new Property("duration", "Quantity", "Timing or duration information as part of the indication.", 0, 1, 878 duration)); 879 children.add(new Property("otherTherapy", "", 880 "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 881 0, java.lang.Integer.MAX_VALUE, otherTherapy)); 882 children.add(new Property("undesirableEffect", "Reference(MedicinalProductUndesirableEffect)", 883 "Describe the undesirable effects of the medicinal product.", 0, java.lang.Integer.MAX_VALUE, 884 undesirableEffect)); 885 children.add(new Property("population", "Population", "The population group to which this applies.", 0, 886 java.lang.Integer.MAX_VALUE, population)); 887 } 888 889 @Override 890 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 891 switch (_hash) { 892 case -1867885268: 893 /* subject */ return new Property("subject", "Reference(MedicinalProduct|Medication)", 894 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject); 895 case -1497395130: 896 /* diseaseSymptomProcedure */ return new Property("diseaseSymptomProcedure", "CodeableConcept", 897 "The disease, symptom or procedure that is the indication for treatment.", 0, 1, diseaseSymptomProcedure); 898 case -505503602: 899 /* diseaseStatus */ return new Property("diseaseStatus", "CodeableConcept", 900 "The status of the disease or symptom for which the indication applies.", 0, 1, diseaseStatus); 901 case -406395211: 902 /* comorbidity */ return new Property("comorbidity", "CodeableConcept", 903 "Comorbidity (concurrent condition) or co-infection as part of the indication.", 0, 904 java.lang.Integer.MAX_VALUE, comorbidity); 905 case 1587112348: 906 /* intendedEffect */ return new Property("intendedEffect", "CodeableConcept", 907 "The intended effect, aim or strategy to be achieved by the indication.", 0, 1, intendedEffect); 908 case -1992012396: 909 /* duration */ return new Property("duration", "Quantity", 910 "Timing or duration information as part of the indication.", 0, 1, duration); 911 case -544509127: 912 /* otherTherapy */ return new Property("otherTherapy", "", 913 "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 914 0, java.lang.Integer.MAX_VALUE, otherTherapy); 915 case 444367565: 916 /* undesirableEffect */ return new Property("undesirableEffect", "Reference(MedicinalProductUndesirableEffect)", 917 "Describe the undesirable effects of the medicinal product.", 0, java.lang.Integer.MAX_VALUE, 918 undesirableEffect); 919 case -2023558323: 920 /* population */ return new Property("population", "Population", "The population group to which this applies.", 0, 921 java.lang.Integer.MAX_VALUE, population); 922 default: 923 return super.getNamedProperty(_hash, _name, _checkValid); 924 } 925 926 } 927 928 @Override 929 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 930 switch (hash) { 931 case -1867885268: 932 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 933 case -1497395130: 934 /* diseaseSymptomProcedure */ return this.diseaseSymptomProcedure == null ? new Base[0] 935 : new Base[] { this.diseaseSymptomProcedure }; // CodeableConcept 936 case -505503602: 937 /* diseaseStatus */ return this.diseaseStatus == null ? new Base[0] : new Base[] { this.diseaseStatus }; // CodeableConcept 938 case -406395211: 939 /* comorbidity */ return this.comorbidity == null ? new Base[0] 940 : this.comorbidity.toArray(new Base[this.comorbidity.size()]); // CodeableConcept 941 case 1587112348: 942 /* intendedEffect */ return this.intendedEffect == null ? new Base[0] : new Base[] { this.intendedEffect }; // CodeableConcept 943 case -1992012396: 944 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Quantity 945 case -544509127: 946 /* otherTherapy */ return this.otherTherapy == null ? new Base[0] 947 : this.otherTherapy.toArray(new Base[this.otherTherapy.size()]); // MedicinalProductIndicationOtherTherapyComponent 948 case 444367565: 949 /* undesirableEffect */ return this.undesirableEffect == null ? new Base[0] 950 : this.undesirableEffect.toArray(new Base[this.undesirableEffect.size()]); // Reference 951 case -2023558323: 952 /* population */ return this.population == null ? new Base[0] 953 : this.population.toArray(new Base[this.population.size()]); // Population 954 default: 955 return super.getProperty(hash, name, checkValid); 956 } 957 958 } 959 960 @Override 961 public Base setProperty(int hash, String name, Base value) throws FHIRException { 962 switch (hash) { 963 case -1867885268: // subject 964 this.getSubject().add(castToReference(value)); // Reference 965 return value; 966 case -1497395130: // diseaseSymptomProcedure 967 this.diseaseSymptomProcedure = castToCodeableConcept(value); // CodeableConcept 968 return value; 969 case -505503602: // diseaseStatus 970 this.diseaseStatus = castToCodeableConcept(value); // CodeableConcept 971 return value; 972 case -406395211: // comorbidity 973 this.getComorbidity().add(castToCodeableConcept(value)); // CodeableConcept 974 return value; 975 case 1587112348: // intendedEffect 976 this.intendedEffect = castToCodeableConcept(value); // CodeableConcept 977 return value; 978 case -1992012396: // duration 979 this.duration = castToQuantity(value); // Quantity 980 return value; 981 case -544509127: // otherTherapy 982 this.getOtherTherapy().add((MedicinalProductIndicationOtherTherapyComponent) value); // MedicinalProductIndicationOtherTherapyComponent 983 return value; 984 case 444367565: // undesirableEffect 985 this.getUndesirableEffect().add(castToReference(value)); // Reference 986 return value; 987 case -2023558323: // population 988 this.getPopulation().add(castToPopulation(value)); // Population 989 return value; 990 default: 991 return super.setProperty(hash, name, value); 992 } 993 994 } 995 996 @Override 997 public Base setProperty(String name, Base value) throws FHIRException { 998 if (name.equals("subject")) { 999 this.getSubject().add(castToReference(value)); 1000 } else if (name.equals("diseaseSymptomProcedure")) { 1001 this.diseaseSymptomProcedure = castToCodeableConcept(value); // CodeableConcept 1002 } else if (name.equals("diseaseStatus")) { 1003 this.diseaseStatus = castToCodeableConcept(value); // CodeableConcept 1004 } else if (name.equals("comorbidity")) { 1005 this.getComorbidity().add(castToCodeableConcept(value)); 1006 } else if (name.equals("intendedEffect")) { 1007 this.intendedEffect = castToCodeableConcept(value); // CodeableConcept 1008 } else if (name.equals("duration")) { 1009 this.duration = castToQuantity(value); // Quantity 1010 } else if (name.equals("otherTherapy")) { 1011 this.getOtherTherapy().add((MedicinalProductIndicationOtherTherapyComponent) value); 1012 } else if (name.equals("undesirableEffect")) { 1013 this.getUndesirableEffect().add(castToReference(value)); 1014 } else if (name.equals("population")) { 1015 this.getPopulation().add(castToPopulation(value)); 1016 } else 1017 return super.setProperty(name, value); 1018 return value; 1019 } 1020 1021 @Override 1022 public void removeChild(String name, Base value) throws FHIRException { 1023 if (name.equals("subject")) { 1024 this.getSubject().remove(castToReference(value)); 1025 } else if (name.equals("diseaseSymptomProcedure")) { 1026 this.diseaseSymptomProcedure = null; 1027 } else if (name.equals("diseaseStatus")) { 1028 this.diseaseStatus = null; 1029 } else if (name.equals("comorbidity")) { 1030 this.getComorbidity().remove(castToCodeableConcept(value)); 1031 } else if (name.equals("intendedEffect")) { 1032 this.intendedEffect = null; 1033 } else if (name.equals("duration")) { 1034 this.duration = null; 1035 } else if (name.equals("otherTherapy")) { 1036 this.getOtherTherapy().remove((MedicinalProductIndicationOtherTherapyComponent) value); 1037 } else if (name.equals("undesirableEffect")) { 1038 this.getUndesirableEffect().remove(castToReference(value)); 1039 } else if (name.equals("population")) { 1040 this.getPopulation().remove(castToPopulation(value)); 1041 } else 1042 super.removeChild(name, value); 1043 1044 } 1045 1046 @Override 1047 public Base makeProperty(int hash, String name) throws FHIRException { 1048 switch (hash) { 1049 case -1867885268: 1050 return addSubject(); 1051 case -1497395130: 1052 return getDiseaseSymptomProcedure(); 1053 case -505503602: 1054 return getDiseaseStatus(); 1055 case -406395211: 1056 return addComorbidity(); 1057 case 1587112348: 1058 return getIntendedEffect(); 1059 case -1992012396: 1060 return getDuration(); 1061 case -544509127: 1062 return addOtherTherapy(); 1063 case 444367565: 1064 return addUndesirableEffect(); 1065 case -2023558323: 1066 return addPopulation(); 1067 default: 1068 return super.makeProperty(hash, name); 1069 } 1070 1071 } 1072 1073 @Override 1074 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1075 switch (hash) { 1076 case -1867885268: 1077 /* subject */ return new String[] { "Reference" }; 1078 case -1497395130: 1079 /* diseaseSymptomProcedure */ return new String[] { "CodeableConcept" }; 1080 case -505503602: 1081 /* diseaseStatus */ return new String[] { "CodeableConcept" }; 1082 case -406395211: 1083 /* comorbidity */ return new String[] { "CodeableConcept" }; 1084 case 1587112348: 1085 /* intendedEffect */ return new String[] { "CodeableConcept" }; 1086 case -1992012396: 1087 /* duration */ return new String[] { "Quantity" }; 1088 case -544509127: 1089 /* otherTherapy */ return new String[] {}; 1090 case 444367565: 1091 /* undesirableEffect */ return new String[] { "Reference" }; 1092 case -2023558323: 1093 /* population */ return new String[] { "Population" }; 1094 default: 1095 return super.getTypesForProperty(hash, name); 1096 } 1097 1098 } 1099 1100 @Override 1101 public Base addChild(String name) throws FHIRException { 1102 if (name.equals("subject")) { 1103 return addSubject(); 1104 } else if (name.equals("diseaseSymptomProcedure")) { 1105 this.diseaseSymptomProcedure = new CodeableConcept(); 1106 return this.diseaseSymptomProcedure; 1107 } else if (name.equals("diseaseStatus")) { 1108 this.diseaseStatus = new CodeableConcept(); 1109 return this.diseaseStatus; 1110 } else if (name.equals("comorbidity")) { 1111 return addComorbidity(); 1112 } else if (name.equals("intendedEffect")) { 1113 this.intendedEffect = new CodeableConcept(); 1114 return this.intendedEffect; 1115 } else if (name.equals("duration")) { 1116 this.duration = new Quantity(); 1117 return this.duration; 1118 } else if (name.equals("otherTherapy")) { 1119 return addOtherTherapy(); 1120 } else if (name.equals("undesirableEffect")) { 1121 return addUndesirableEffect(); 1122 } else if (name.equals("population")) { 1123 return addPopulation(); 1124 } else 1125 return super.addChild(name); 1126 } 1127 1128 public String fhirType() { 1129 return "MedicinalProductIndication"; 1130 1131 } 1132 1133 public MedicinalProductIndication copy() { 1134 MedicinalProductIndication dst = new MedicinalProductIndication(); 1135 copyValues(dst); 1136 return dst; 1137 } 1138 1139 public void copyValues(MedicinalProductIndication dst) { 1140 super.copyValues(dst); 1141 if (subject != null) { 1142 dst.subject = new ArrayList<Reference>(); 1143 for (Reference i : subject) 1144 dst.subject.add(i.copy()); 1145 } 1146 ; 1147 dst.diseaseSymptomProcedure = diseaseSymptomProcedure == null ? null : diseaseSymptomProcedure.copy(); 1148 dst.diseaseStatus = diseaseStatus == null ? null : diseaseStatus.copy(); 1149 if (comorbidity != null) { 1150 dst.comorbidity = new ArrayList<CodeableConcept>(); 1151 for (CodeableConcept i : comorbidity) 1152 dst.comorbidity.add(i.copy()); 1153 } 1154 ; 1155 dst.intendedEffect = intendedEffect == null ? null : intendedEffect.copy(); 1156 dst.duration = duration == null ? null : duration.copy(); 1157 if (otherTherapy != null) { 1158 dst.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 1159 for (MedicinalProductIndicationOtherTherapyComponent i : otherTherapy) 1160 dst.otherTherapy.add(i.copy()); 1161 } 1162 ; 1163 if (undesirableEffect != null) { 1164 dst.undesirableEffect = new ArrayList<Reference>(); 1165 for (Reference i : undesirableEffect) 1166 dst.undesirableEffect.add(i.copy()); 1167 } 1168 ; 1169 if (population != null) { 1170 dst.population = new ArrayList<Population>(); 1171 for (Population i : population) 1172 dst.population.add(i.copy()); 1173 } 1174 ; 1175 } 1176 1177 protected MedicinalProductIndication typedCopy() { 1178 return copy(); 1179 } 1180 1181 @Override 1182 public boolean equalsDeep(Base other_) { 1183 if (!super.equalsDeep(other_)) 1184 return false; 1185 if (!(other_ instanceof MedicinalProductIndication)) 1186 return false; 1187 MedicinalProductIndication o = (MedicinalProductIndication) other_; 1188 return compareDeep(subject, o.subject, true) 1189 && compareDeep(diseaseSymptomProcedure, o.diseaseSymptomProcedure, true) 1190 && compareDeep(diseaseStatus, o.diseaseStatus, true) && compareDeep(comorbidity, o.comorbidity, true) 1191 && compareDeep(intendedEffect, o.intendedEffect, true) && compareDeep(duration, o.duration, true) 1192 && compareDeep(otherTherapy, o.otherTherapy, true) && compareDeep(undesirableEffect, o.undesirableEffect, true) 1193 && compareDeep(population, o.population, true); 1194 } 1195 1196 @Override 1197 public boolean equalsShallow(Base other_) { 1198 if (!super.equalsShallow(other_)) 1199 return false; 1200 if (!(other_ instanceof MedicinalProductIndication)) 1201 return false; 1202 MedicinalProductIndication o = (MedicinalProductIndication) other_; 1203 return true; 1204 } 1205 1206 public boolean isEmpty() { 1207 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subject, diseaseSymptomProcedure, diseaseStatus, 1208 comorbidity, intendedEffect, duration, otherTherapy, undesirableEffect, population); 1209 } 1210 1211 @Override 1212 public ResourceType getResourceType() { 1213 return ResourceType.MedicinalProductIndication; 1214 } 1215 1216 /** 1217 * Search parameter: <b>subject</b> 1218 * <p> 1219 * Description: <b>The medication for which this is an indication</b><br> 1220 * Type: <b>reference</b><br> 1221 * Path: <b>MedicinalProductIndication.subject</b><br> 1222 * </p> 1223 */ 1224 @SearchParamDefinition(name = "subject", path = "MedicinalProductIndication.subject", description = "The medication for which this is an indication", type = "reference", target = { 1225 Medication.class, MedicinalProduct.class }) 1226 public static final String SP_SUBJECT = "subject"; 1227 /** 1228 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1229 * <p> 1230 * Description: <b>The medication for which this is an indication</b><br> 1231 * Type: <b>reference</b><br> 1232 * Path: <b>MedicinalProductIndication.subject</b><br> 1233 * </p> 1234 */ 1235 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1236 SP_SUBJECT); 1237 1238 /** 1239 * Constant for fluent queries to be used to add include statements. Specifies 1240 * the path value of "<b>MedicinalProductIndication:subject</b>". 1241 */ 1242 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1243 "MedicinalProductIndication:subject").toLocked(); 1244 1245}