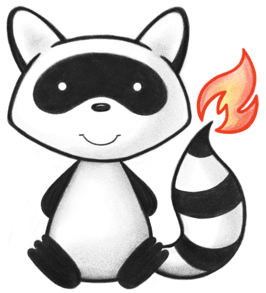
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The interactions of the medicinal product with other medicinal products, or 048 * other forms of interactions. 049 */ 050@ResourceDef(name = "MedicinalProductInteraction", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductInteraction") 051public class MedicinalProductInteraction extends DomainResource { 052 053 @Block() 054 public static class MedicinalProductInteractionInteractantComponent extends BackboneElement 055 implements IBaseBackboneElement { 056 /** 057 * The specific medication, food or laboratory test that interacts. 058 */ 059 @Child(name = "item", type = { MedicinalProduct.class, Medication.class, Substance.class, 060 ObservationDefinition.class, 061 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 062 @Description(shortDefinition = "The specific medication, food or laboratory test that interacts", formalDefinition = "The specific medication, food or laboratory test that interacts.") 063 protected Type item; 064 065 private static final long serialVersionUID = 1445276561L; 066 067 /** 068 * Constructor 069 */ 070 public MedicinalProductInteractionInteractantComponent() { 071 super(); 072 } 073 074 /** 075 * Constructor 076 */ 077 public MedicinalProductInteractionInteractantComponent(Type item) { 078 super(); 079 this.item = item; 080 } 081 082 /** 083 * @return {@link #item} (The specific medication, food or laboratory test that 084 * interacts.) 085 */ 086 public Type getItem() { 087 return this.item; 088 } 089 090 /** 091 * @return {@link #item} (The specific medication, food or laboratory test that 092 * interacts.) 093 */ 094 public Reference getItemReference() throws FHIRException { 095 if (this.item == null) 096 this.item = new Reference(); 097 if (!(this.item instanceof Reference)) 098 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 099 + " was encountered"); 100 return (Reference) this.item; 101 } 102 103 public boolean hasItemReference() { 104 return this != null && this.item instanceof Reference; 105 } 106 107 /** 108 * @return {@link #item} (The specific medication, food or laboratory test that 109 * interacts.) 110 */ 111 public CodeableConcept getItemCodeableConcept() throws FHIRException { 112 if (this.item == null) 113 this.item = new CodeableConcept(); 114 if (!(this.item instanceof CodeableConcept)) 115 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 116 + this.item.getClass().getName() + " was encountered"); 117 return (CodeableConcept) this.item; 118 } 119 120 public boolean hasItemCodeableConcept() { 121 return this != null && this.item instanceof CodeableConcept; 122 } 123 124 public boolean hasItem() { 125 return this.item != null && !this.item.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #item} (The specific medication, food or laboratory test 130 * that interacts.) 131 */ 132 public MedicinalProductInteractionInteractantComponent setItem(Type value) { 133 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 134 throw new Error("Not the right type for MedicinalProductInteraction.interactant.item[x]: " + value.fhirType()); 135 this.item = value; 136 return this; 137 } 138 139 protected void listChildren(List<Property> children) { 140 super.listChildren(children); 141 children.add(new Property("item[x]", 142 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 143 "The specific medication, food or laboratory test that interacts.", 0, 1, item)); 144 } 145 146 @Override 147 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 148 switch (_hash) { 149 case 2116201613: 150 /* item[x] */ return new Property("item[x]", 151 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 152 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 153 case 3242771: 154 /* item */ return new Property("item[x]", 155 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 156 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 157 case 1376364920: 158 /* itemReference */ return new Property("item[x]", 159 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 160 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 161 case 106644494: 162 /* itemCodeableConcept */ return new Property("item[x]", 163 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 164 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 165 default: 166 return super.getNamedProperty(_hash, _name, _checkValid); 167 } 168 169 } 170 171 @Override 172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 173 switch (hash) { 174 case 3242771: 175 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 176 default: 177 return super.getProperty(hash, name, checkValid); 178 } 179 180 } 181 182 @Override 183 public Base setProperty(int hash, String name, Base value) throws FHIRException { 184 switch (hash) { 185 case 3242771: // item 186 this.item = castToType(value); // Type 187 return value; 188 default: 189 return super.setProperty(hash, name, value); 190 } 191 192 } 193 194 @Override 195 public Base setProperty(String name, Base value) throws FHIRException { 196 if (name.equals("item[x]")) { 197 this.item = castToType(value); // Type 198 } else 199 return super.setProperty(name, value); 200 return value; 201 } 202 203 @Override 204 public void removeChild(String name, Base value) throws FHIRException { 205 if (name.equals("item[x]")) { 206 this.item = null; 207 } else 208 super.removeChild(name, value); 209 210 } 211 212 @Override 213 public Base makeProperty(int hash, String name) throws FHIRException { 214 switch (hash) { 215 case 2116201613: 216 return getItem(); 217 case 3242771: 218 return getItem(); 219 default: 220 return super.makeProperty(hash, name); 221 } 222 223 } 224 225 @Override 226 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 227 switch (hash) { 228 case 3242771: 229 /* item */ return new String[] { "Reference", "CodeableConcept" }; 230 default: 231 return super.getTypesForProperty(hash, name); 232 } 233 234 } 235 236 @Override 237 public Base addChild(String name) throws FHIRException { 238 if (name.equals("itemReference")) { 239 this.item = new Reference(); 240 return this.item; 241 } else if (name.equals("itemCodeableConcept")) { 242 this.item = new CodeableConcept(); 243 return this.item; 244 } else 245 return super.addChild(name); 246 } 247 248 public MedicinalProductInteractionInteractantComponent copy() { 249 MedicinalProductInteractionInteractantComponent dst = new MedicinalProductInteractionInteractantComponent(); 250 copyValues(dst); 251 return dst; 252 } 253 254 public void copyValues(MedicinalProductInteractionInteractantComponent dst) { 255 super.copyValues(dst); 256 dst.item = item == null ? null : item.copy(); 257 } 258 259 @Override 260 public boolean equalsDeep(Base other_) { 261 if (!super.equalsDeep(other_)) 262 return false; 263 if (!(other_ instanceof MedicinalProductInteractionInteractantComponent)) 264 return false; 265 MedicinalProductInteractionInteractantComponent o = (MedicinalProductInteractionInteractantComponent) other_; 266 return compareDeep(item, o.item, true); 267 } 268 269 @Override 270 public boolean equalsShallow(Base other_) { 271 if (!super.equalsShallow(other_)) 272 return false; 273 if (!(other_ instanceof MedicinalProductInteractionInteractantComponent)) 274 return false; 275 MedicinalProductInteractionInteractantComponent o = (MedicinalProductInteractionInteractantComponent) other_; 276 return true; 277 } 278 279 public boolean isEmpty() { 280 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 281 } 282 283 public String fhirType() { 284 return "MedicinalProductInteraction.interactant"; 285 286 } 287 288 } 289 290 /** 291 * The medication for which this is a described interaction. 292 */ 293 @Child(name = "subject", type = { MedicinalProduct.class, Medication.class, 294 Substance.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 295 @Description(shortDefinition = "The medication for which this is a described interaction", formalDefinition = "The medication for which this is a described interaction.") 296 protected List<Reference> subject; 297 /** 298 * The actual objects that are the target of the reference (The medication for 299 * which this is a described interaction.) 300 */ 301 protected List<Resource> subjectTarget; 302 303 /** 304 * The interaction described. 305 */ 306 @Child(name = "description", type = { 307 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 308 @Description(shortDefinition = "The interaction described", formalDefinition = "The interaction described.") 309 protected StringType description; 310 311 /** 312 * The specific medication, food or laboratory test that interacts. 313 */ 314 @Child(name = "interactant", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 315 @Description(shortDefinition = "The specific medication, food or laboratory test that interacts", formalDefinition = "The specific medication, food or laboratory test that interacts.") 316 protected List<MedicinalProductInteractionInteractantComponent> interactant; 317 318 /** 319 * The type of the interaction e.g. drug-drug interaction, drug-food 320 * interaction, drug-lab test interaction. 321 */ 322 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 323 @Description(shortDefinition = "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction", formalDefinition = "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.") 324 protected CodeableConcept type; 325 326 /** 327 * The effect of the interaction, for example "reduced gastric absorption of 328 * primary medication". 329 */ 330 @Child(name = "effect", type = { 331 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 332 @Description(shortDefinition = "The effect of the interaction, for example \"reduced gastric absorption of primary medication\"", formalDefinition = "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".") 333 protected CodeableConcept effect; 334 335 /** 336 * The incidence of the interaction, e.g. theoretical, observed. 337 */ 338 @Child(name = "incidence", type = { 339 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 340 @Description(shortDefinition = "The incidence of the interaction, e.g. theoretical, observed", formalDefinition = "The incidence of the interaction, e.g. theoretical, observed.") 341 protected CodeableConcept incidence; 342 343 /** 344 * Actions for managing the interaction. 345 */ 346 @Child(name = "management", type = { 347 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 348 @Description(shortDefinition = "Actions for managing the interaction", formalDefinition = "Actions for managing the interaction.") 349 protected CodeableConcept management; 350 351 private static final long serialVersionUID = -1872687169L; 352 353 /** 354 * Constructor 355 */ 356 public MedicinalProductInteraction() { 357 super(); 358 } 359 360 /** 361 * @return {@link #subject} (The medication for which this is a described 362 * interaction.) 363 */ 364 public List<Reference> getSubject() { 365 if (this.subject == null) 366 this.subject = new ArrayList<Reference>(); 367 return this.subject; 368 } 369 370 /** 371 * @return Returns a reference to <code>this</code> for easy method chaining 372 */ 373 public MedicinalProductInteraction setSubject(List<Reference> theSubject) { 374 this.subject = theSubject; 375 return this; 376 } 377 378 public boolean hasSubject() { 379 if (this.subject == null) 380 return false; 381 for (Reference item : this.subject) 382 if (!item.isEmpty()) 383 return true; 384 return false; 385 } 386 387 public Reference addSubject() { // 3 388 Reference t = new Reference(); 389 if (this.subject == null) 390 this.subject = new ArrayList<Reference>(); 391 this.subject.add(t); 392 return t; 393 } 394 395 public MedicinalProductInteraction addSubject(Reference t) { // 3 396 if (t == null) 397 return this; 398 if (this.subject == null) 399 this.subject = new ArrayList<Reference>(); 400 this.subject.add(t); 401 return this; 402 } 403 404 /** 405 * @return The first repetition of repeating field {@link #subject}, creating it 406 * if it does not already exist 407 */ 408 public Reference getSubjectFirstRep() { 409 if (getSubject().isEmpty()) { 410 addSubject(); 411 } 412 return getSubject().get(0); 413 } 414 415 /** 416 * @deprecated Use Reference#setResource(IBaseResource) instead 417 */ 418 @Deprecated 419 public List<Resource> getSubjectTarget() { 420 if (this.subjectTarget == null) 421 this.subjectTarget = new ArrayList<Resource>(); 422 return this.subjectTarget; 423 } 424 425 /** 426 * @return {@link #description} (The interaction described.). This is the 427 * underlying object with id, value and extensions. The accessor 428 * "getDescription" gives direct access to the value 429 */ 430 public StringType getDescriptionElement() { 431 if (this.description == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create MedicinalProductInteraction.description"); 434 else if (Configuration.doAutoCreate()) 435 this.description = new StringType(); // bb 436 return this.description; 437 } 438 439 public boolean hasDescriptionElement() { 440 return this.description != null && !this.description.isEmpty(); 441 } 442 443 public boolean hasDescription() { 444 return this.description != null && !this.description.isEmpty(); 445 } 446 447 /** 448 * @param value {@link #description} (The interaction described.). This is the 449 * underlying object with id, value and extensions. The accessor 450 * "getDescription" gives direct access to the value 451 */ 452 public MedicinalProductInteraction setDescriptionElement(StringType value) { 453 this.description = value; 454 return this; 455 } 456 457 /** 458 * @return The interaction described. 459 */ 460 public String getDescription() { 461 return this.description == null ? null : this.description.getValue(); 462 } 463 464 /** 465 * @param value The interaction described. 466 */ 467 public MedicinalProductInteraction setDescription(String value) { 468 if (Utilities.noString(value)) 469 this.description = null; 470 else { 471 if (this.description == null) 472 this.description = new StringType(); 473 this.description.setValue(value); 474 } 475 return this; 476 } 477 478 /** 479 * @return {@link #interactant} (The specific medication, food or laboratory 480 * test that interacts.) 481 */ 482 public List<MedicinalProductInteractionInteractantComponent> getInteractant() { 483 if (this.interactant == null) 484 this.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 485 return this.interactant; 486 } 487 488 /** 489 * @return Returns a reference to <code>this</code> for easy method chaining 490 */ 491 public MedicinalProductInteraction setInteractant( 492 List<MedicinalProductInteractionInteractantComponent> theInteractant) { 493 this.interactant = theInteractant; 494 return this; 495 } 496 497 public boolean hasInteractant() { 498 if (this.interactant == null) 499 return false; 500 for (MedicinalProductInteractionInteractantComponent item : this.interactant) 501 if (!item.isEmpty()) 502 return true; 503 return false; 504 } 505 506 public MedicinalProductInteractionInteractantComponent addInteractant() { // 3 507 MedicinalProductInteractionInteractantComponent t = new MedicinalProductInteractionInteractantComponent(); 508 if (this.interactant == null) 509 this.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 510 this.interactant.add(t); 511 return t; 512 } 513 514 public MedicinalProductInteraction addInteractant(MedicinalProductInteractionInteractantComponent t) { // 3 515 if (t == null) 516 return this; 517 if (this.interactant == null) 518 this.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 519 this.interactant.add(t); 520 return this; 521 } 522 523 /** 524 * @return The first repetition of repeating field {@link #interactant}, 525 * creating it if it does not already exist 526 */ 527 public MedicinalProductInteractionInteractantComponent getInteractantFirstRep() { 528 if (getInteractant().isEmpty()) { 529 addInteractant(); 530 } 531 return getInteractant().get(0); 532 } 533 534 /** 535 * @return {@link #type} (The type of the interaction e.g. drug-drug 536 * interaction, drug-food interaction, drug-lab test interaction.) 537 */ 538 public CodeableConcept getType() { 539 if (this.type == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create MedicinalProductInteraction.type"); 542 else if (Configuration.doAutoCreate()) 543 this.type = new CodeableConcept(); // cc 544 return this.type; 545 } 546 547 public boolean hasType() { 548 return this.type != null && !this.type.isEmpty(); 549 } 550 551 /** 552 * @param value {@link #type} (The type of the interaction e.g. drug-drug 553 * interaction, drug-food interaction, drug-lab test interaction.) 554 */ 555 public MedicinalProductInteraction setType(CodeableConcept value) { 556 this.type = value; 557 return this; 558 } 559 560 /** 561 * @return {@link #effect} (The effect of the interaction, for example "reduced 562 * gastric absorption of primary medication".) 563 */ 564 public CodeableConcept getEffect() { 565 if (this.effect == null) 566 if (Configuration.errorOnAutoCreate()) 567 throw new Error("Attempt to auto-create MedicinalProductInteraction.effect"); 568 else if (Configuration.doAutoCreate()) 569 this.effect = new CodeableConcept(); // cc 570 return this.effect; 571 } 572 573 public boolean hasEffect() { 574 return this.effect != null && !this.effect.isEmpty(); 575 } 576 577 /** 578 * @param value {@link #effect} (The effect of the interaction, for example 579 * "reduced gastric absorption of primary medication".) 580 */ 581 public MedicinalProductInteraction setEffect(CodeableConcept value) { 582 this.effect = value; 583 return this; 584 } 585 586 /** 587 * @return {@link #incidence} (The incidence of the interaction, e.g. 588 * theoretical, observed.) 589 */ 590 public CodeableConcept getIncidence() { 591 if (this.incidence == null) 592 if (Configuration.errorOnAutoCreate()) 593 throw new Error("Attempt to auto-create MedicinalProductInteraction.incidence"); 594 else if (Configuration.doAutoCreate()) 595 this.incidence = new CodeableConcept(); // cc 596 return this.incidence; 597 } 598 599 public boolean hasIncidence() { 600 return this.incidence != null && !this.incidence.isEmpty(); 601 } 602 603 /** 604 * @param value {@link #incidence} (The incidence of the interaction, e.g. 605 * theoretical, observed.) 606 */ 607 public MedicinalProductInteraction setIncidence(CodeableConcept value) { 608 this.incidence = value; 609 return this; 610 } 611 612 /** 613 * @return {@link #management} (Actions for managing the interaction.) 614 */ 615 public CodeableConcept getManagement() { 616 if (this.management == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create MedicinalProductInteraction.management"); 619 else if (Configuration.doAutoCreate()) 620 this.management = new CodeableConcept(); // cc 621 return this.management; 622 } 623 624 public boolean hasManagement() { 625 return this.management != null && !this.management.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #management} (Actions for managing the interaction.) 630 */ 631 public MedicinalProductInteraction setManagement(CodeableConcept value) { 632 this.management = value; 633 return this; 634 } 635 636 protected void listChildren(List<Property> children) { 637 super.listChildren(children); 638 children.add(new Property("subject", "Reference(MedicinalProduct|Medication|Substance)", 639 "The medication for which this is a described interaction.", 0, java.lang.Integer.MAX_VALUE, subject)); 640 children.add(new Property("description", "string", "The interaction described.", 0, 1, description)); 641 children.add(new Property("interactant", "", "The specific medication, food or laboratory test that interacts.", 0, 642 java.lang.Integer.MAX_VALUE, interactant)); 643 children.add(new Property("type", "CodeableConcept", 644 "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.", 0, 645 1, type)); 646 children.add(new Property("effect", "CodeableConcept", 647 "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".", 0, 1, 648 effect)); 649 children.add(new Property("incidence", "CodeableConcept", 650 "The incidence of the interaction, e.g. theoretical, observed.", 0, 1, incidence)); 651 children 652 .add(new Property("management", "CodeableConcept", "Actions for managing the interaction.", 0, 1, management)); 653 } 654 655 @Override 656 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 657 switch (_hash) { 658 case -1867885268: 659 /* subject */ return new Property("subject", "Reference(MedicinalProduct|Medication|Substance)", 660 "The medication for which this is a described interaction.", 0, java.lang.Integer.MAX_VALUE, subject); 661 case -1724546052: 662 /* description */ return new Property("description", "string", "The interaction described.", 0, 1, description); 663 case 1844097009: 664 /* interactant */ return new Property("interactant", "", 665 "The specific medication, food or laboratory test that interacts.", 0, java.lang.Integer.MAX_VALUE, 666 interactant); 667 case 3575610: 668 /* type */ return new Property("type", "CodeableConcept", 669 "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.", 670 0, 1, type); 671 case -1306084975: 672 /* effect */ return new Property("effect", "CodeableConcept", 673 "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".", 0, 1, 674 effect); 675 case -1598467132: 676 /* incidence */ return new Property("incidence", "CodeableConcept", 677 "The incidence of the interaction, e.g. theoretical, observed.", 0, 1, incidence); 678 case -1799980989: 679 /* management */ return new Property("management", "CodeableConcept", "Actions for managing the interaction.", 0, 680 1, management); 681 default: 682 return super.getNamedProperty(_hash, _name, _checkValid); 683 } 684 685 } 686 687 @Override 688 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 689 switch (hash) { 690 case -1867885268: 691 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 692 case -1724546052: 693 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 694 case 1844097009: 695 /* interactant */ return this.interactant == null ? new Base[0] 696 : this.interactant.toArray(new Base[this.interactant.size()]); // MedicinalProductInteractionInteractantComponent 697 case 3575610: 698 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 699 case -1306084975: 700 /* effect */ return this.effect == null ? new Base[0] : new Base[] { this.effect }; // CodeableConcept 701 case -1598467132: 702 /* incidence */ return this.incidence == null ? new Base[0] : new Base[] { this.incidence }; // CodeableConcept 703 case -1799980989: 704 /* management */ return this.management == null ? new Base[0] : new Base[] { this.management }; // CodeableConcept 705 default: 706 return super.getProperty(hash, name, checkValid); 707 } 708 709 } 710 711 @Override 712 public Base setProperty(int hash, String name, Base value) throws FHIRException { 713 switch (hash) { 714 case -1867885268: // subject 715 this.getSubject().add(castToReference(value)); // Reference 716 return value; 717 case -1724546052: // description 718 this.description = castToString(value); // StringType 719 return value; 720 case 1844097009: // interactant 721 this.getInteractant().add((MedicinalProductInteractionInteractantComponent) value); // MedicinalProductInteractionInteractantComponent 722 return value; 723 case 3575610: // type 724 this.type = castToCodeableConcept(value); // CodeableConcept 725 return value; 726 case -1306084975: // effect 727 this.effect = castToCodeableConcept(value); // CodeableConcept 728 return value; 729 case -1598467132: // incidence 730 this.incidence = castToCodeableConcept(value); // CodeableConcept 731 return value; 732 case -1799980989: // management 733 this.management = castToCodeableConcept(value); // CodeableConcept 734 return value; 735 default: 736 return super.setProperty(hash, name, value); 737 } 738 739 } 740 741 @Override 742 public Base setProperty(String name, Base value) throws FHIRException { 743 if (name.equals("subject")) { 744 this.getSubject().add(castToReference(value)); 745 } else if (name.equals("description")) { 746 this.description = castToString(value); // StringType 747 } else if (name.equals("interactant")) { 748 this.getInteractant().add((MedicinalProductInteractionInteractantComponent) value); 749 } else if (name.equals("type")) { 750 this.type = castToCodeableConcept(value); // CodeableConcept 751 } else if (name.equals("effect")) { 752 this.effect = castToCodeableConcept(value); // CodeableConcept 753 } else if (name.equals("incidence")) { 754 this.incidence = castToCodeableConcept(value); // CodeableConcept 755 } else if (name.equals("management")) { 756 this.management = castToCodeableConcept(value); // CodeableConcept 757 } else 758 return super.setProperty(name, value); 759 return value; 760 } 761 762 @Override 763 public void removeChild(String name, Base value) throws FHIRException { 764 if (name.equals("subject")) { 765 this.getSubject().remove(castToReference(value)); 766 } else if (name.equals("description")) { 767 this.description = null; 768 } else if (name.equals("interactant")) { 769 this.getInteractant().remove((MedicinalProductInteractionInteractantComponent) value); 770 } else if (name.equals("type")) { 771 this.type = null; 772 } else if (name.equals("effect")) { 773 this.effect = null; 774 } else if (name.equals("incidence")) { 775 this.incidence = null; 776 } else if (name.equals("management")) { 777 this.management = null; 778 } else 779 super.removeChild(name, value); 780 781 } 782 783 @Override 784 public Base makeProperty(int hash, String name) throws FHIRException { 785 switch (hash) { 786 case -1867885268: 787 return addSubject(); 788 case -1724546052: 789 return getDescriptionElement(); 790 case 1844097009: 791 return addInteractant(); 792 case 3575610: 793 return getType(); 794 case -1306084975: 795 return getEffect(); 796 case -1598467132: 797 return getIncidence(); 798 case -1799980989: 799 return getManagement(); 800 default: 801 return super.makeProperty(hash, name); 802 } 803 804 } 805 806 @Override 807 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 808 switch (hash) { 809 case -1867885268: 810 /* subject */ return new String[] { "Reference" }; 811 case -1724546052: 812 /* description */ return new String[] { "string" }; 813 case 1844097009: 814 /* interactant */ return new String[] {}; 815 case 3575610: 816 /* type */ return new String[] { "CodeableConcept" }; 817 case -1306084975: 818 /* effect */ return new String[] { "CodeableConcept" }; 819 case -1598467132: 820 /* incidence */ return new String[] { "CodeableConcept" }; 821 case -1799980989: 822 /* management */ return new String[] { "CodeableConcept" }; 823 default: 824 return super.getTypesForProperty(hash, name); 825 } 826 827 } 828 829 @Override 830 public Base addChild(String name) throws FHIRException { 831 if (name.equals("subject")) { 832 return addSubject(); 833 } else if (name.equals("description")) { 834 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductInteraction.description"); 835 } else if (name.equals("interactant")) { 836 return addInteractant(); 837 } else if (name.equals("type")) { 838 this.type = new CodeableConcept(); 839 return this.type; 840 } else if (name.equals("effect")) { 841 this.effect = new CodeableConcept(); 842 return this.effect; 843 } else if (name.equals("incidence")) { 844 this.incidence = new CodeableConcept(); 845 return this.incidence; 846 } else if (name.equals("management")) { 847 this.management = new CodeableConcept(); 848 return this.management; 849 } else 850 return super.addChild(name); 851 } 852 853 public String fhirType() { 854 return "MedicinalProductInteraction"; 855 856 } 857 858 public MedicinalProductInteraction copy() { 859 MedicinalProductInteraction dst = new MedicinalProductInteraction(); 860 copyValues(dst); 861 return dst; 862 } 863 864 public void copyValues(MedicinalProductInteraction dst) { 865 super.copyValues(dst); 866 if (subject != null) { 867 dst.subject = new ArrayList<Reference>(); 868 for (Reference i : subject) 869 dst.subject.add(i.copy()); 870 } 871 ; 872 dst.description = description == null ? null : description.copy(); 873 if (interactant != null) { 874 dst.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 875 for (MedicinalProductInteractionInteractantComponent i : interactant) 876 dst.interactant.add(i.copy()); 877 } 878 ; 879 dst.type = type == null ? null : type.copy(); 880 dst.effect = effect == null ? null : effect.copy(); 881 dst.incidence = incidence == null ? null : incidence.copy(); 882 dst.management = management == null ? null : management.copy(); 883 } 884 885 protected MedicinalProductInteraction typedCopy() { 886 return copy(); 887 } 888 889 @Override 890 public boolean equalsDeep(Base other_) { 891 if (!super.equalsDeep(other_)) 892 return false; 893 if (!(other_ instanceof MedicinalProductInteraction)) 894 return false; 895 MedicinalProductInteraction o = (MedicinalProductInteraction) other_; 896 return compareDeep(subject, o.subject, true) && compareDeep(description, o.description, true) 897 && compareDeep(interactant, o.interactant, true) && compareDeep(type, o.type, true) 898 && compareDeep(effect, o.effect, true) && compareDeep(incidence, o.incidence, true) 899 && compareDeep(management, o.management, true); 900 } 901 902 @Override 903 public boolean equalsShallow(Base other_) { 904 if (!super.equalsShallow(other_)) 905 return false; 906 if (!(other_ instanceof MedicinalProductInteraction)) 907 return false; 908 MedicinalProductInteraction o = (MedicinalProductInteraction) other_; 909 return compareValues(description, o.description, true); 910 } 911 912 public boolean isEmpty() { 913 return super.isEmpty() 914 && ca.uhn.fhir.util.ElementUtil.isEmpty(subject, description, interactant, type, effect, incidence, management); 915 } 916 917 @Override 918 public ResourceType getResourceType() { 919 return ResourceType.MedicinalProductInteraction; 920 } 921 922 /** 923 * Search parameter: <b>subject</b> 924 * <p> 925 * Description: <b>The medication for which this is an interaction</b><br> 926 * Type: <b>reference</b><br> 927 * Path: <b>MedicinalProductInteraction.subject</b><br> 928 * </p> 929 */ 930 @SearchParamDefinition(name = "subject", path = "MedicinalProductInteraction.subject", description = "The medication for which this is an interaction", type = "reference", target = { 931 Medication.class, MedicinalProduct.class, Substance.class }) 932 public static final String SP_SUBJECT = "subject"; 933 /** 934 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 935 * <p> 936 * Description: <b>The medication for which this is an interaction</b><br> 937 * Type: <b>reference</b><br> 938 * Path: <b>MedicinalProductInteraction.subject</b><br> 939 * </p> 940 */ 941 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 942 SP_SUBJECT); 943 944 /** 945 * Constant for fluent queries to be used to add include statements. Specifies 946 * the path value of "<b>MedicinalProductInteraction:subject</b>". 947 */ 948 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 949 "MedicinalProductInteraction:subject").toLocked(); 950 951}