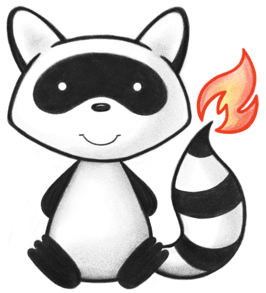
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041 042/** 043 * The manufactured item as contained in the packaged medicinal product. 044 */ 045@ResourceDef(name = "MedicinalProductManufactured", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductManufactured") 046public class MedicinalProductManufactured extends DomainResource { 047 048 /** 049 * Dose form as manufactured and before any transformation into the 050 * pharmaceutical product. 051 */ 052 @Child(name = "manufacturedDoseForm", type = { 053 CodeableConcept.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "Dose form as manufactured and before any transformation into the pharmaceutical product", formalDefinition = "Dose form as manufactured and before any transformation into the pharmaceutical product.") 055 protected CodeableConcept manufacturedDoseForm; 056 057 /** 058 * The ?real world? units in which the quantity of the manufactured item is 059 * described. 060 */ 061 @Child(name = "unitOfPresentation", type = { 062 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "The ?real world? units in which the quantity of the manufactured item is described", formalDefinition = "The ?real world? units in which the quantity of the manufactured item is described.") 064 protected CodeableConcept unitOfPresentation; 065 066 /** 067 * The quantity or "count number" of the manufactured item. 068 */ 069 @Child(name = "quantity", type = { Quantity.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 070 @Description(shortDefinition = "The quantity or \"count number\" of the manufactured item", formalDefinition = "The quantity or \"count number\" of the manufactured item.") 071 protected Quantity quantity; 072 073 /** 074 * Manufacturer of the item (Note that this should be named "manufacturer" but 075 * it currently causes technical issues). 076 */ 077 @Child(name = "manufacturer", type = { 078 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 079 @Description(shortDefinition = "Manufacturer of the item (Note that this should be named \"manufacturer\" but it currently causes technical issues)", formalDefinition = "Manufacturer of the item (Note that this should be named \"manufacturer\" but it currently causes technical issues).") 080 protected List<Reference> manufacturer; 081 /** 082 * The actual objects that are the target of the reference (Manufacturer of the 083 * item (Note that this should be named "manufacturer" but it currently causes 084 * technical issues).) 085 */ 086 protected List<Organization> manufacturerTarget; 087 088 /** 089 * Ingredient. 090 */ 091 @Child(name = "ingredient", type = { 092 MedicinalProductIngredient.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 093 @Description(shortDefinition = "Ingredient", formalDefinition = "Ingredient.") 094 protected List<Reference> ingredient; 095 /** 096 * The actual objects that are the target of the reference (Ingredient.) 097 */ 098 protected List<MedicinalProductIngredient> ingredientTarget; 099 100 /** 101 * Dimensions, color etc. 102 */ 103 @Child(name = "physicalCharacteristics", type = { 104 ProdCharacteristic.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 105 @Description(shortDefinition = "Dimensions, color etc.", formalDefinition = "Dimensions, color etc.") 106 protected ProdCharacteristic physicalCharacteristics; 107 108 /** 109 * Other codeable characteristics. 110 */ 111 @Child(name = "otherCharacteristics", type = { 112 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 113 @Description(shortDefinition = "Other codeable characteristics", formalDefinition = "Other codeable characteristics.") 114 protected List<CodeableConcept> otherCharacteristics; 115 116 private static final long serialVersionUID = 623073384L; 117 118 /** 119 * Constructor 120 */ 121 public MedicinalProductManufactured() { 122 super(); 123 } 124 125 /** 126 * Constructor 127 */ 128 public MedicinalProductManufactured(CodeableConcept manufacturedDoseForm, Quantity quantity) { 129 super(); 130 this.manufacturedDoseForm = manufacturedDoseForm; 131 this.quantity = quantity; 132 } 133 134 /** 135 * @return {@link #manufacturedDoseForm} (Dose form as manufactured and before 136 * any transformation into the pharmaceutical product.) 137 */ 138 public CodeableConcept getManufacturedDoseForm() { 139 if (this.manufacturedDoseForm == null) 140 if (Configuration.errorOnAutoCreate()) 141 throw new Error("Attempt to auto-create MedicinalProductManufactured.manufacturedDoseForm"); 142 else if (Configuration.doAutoCreate()) 143 this.manufacturedDoseForm = new CodeableConcept(); // cc 144 return this.manufacturedDoseForm; 145 } 146 147 public boolean hasManufacturedDoseForm() { 148 return this.manufacturedDoseForm != null && !this.manufacturedDoseForm.isEmpty(); 149 } 150 151 /** 152 * @param value {@link #manufacturedDoseForm} (Dose form as manufactured and 153 * before any transformation into the pharmaceutical product.) 154 */ 155 public MedicinalProductManufactured setManufacturedDoseForm(CodeableConcept value) { 156 this.manufacturedDoseForm = value; 157 return this; 158 } 159 160 /** 161 * @return {@link #unitOfPresentation} (The ?real world? units in which the 162 * quantity of the manufactured item is described.) 163 */ 164 public CodeableConcept getUnitOfPresentation() { 165 if (this.unitOfPresentation == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create MedicinalProductManufactured.unitOfPresentation"); 168 else if (Configuration.doAutoCreate()) 169 this.unitOfPresentation = new CodeableConcept(); // cc 170 return this.unitOfPresentation; 171 } 172 173 public boolean hasUnitOfPresentation() { 174 return this.unitOfPresentation != null && !this.unitOfPresentation.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #unitOfPresentation} (The ?real world? units in which the 179 * quantity of the manufactured item is described.) 180 */ 181 public MedicinalProductManufactured setUnitOfPresentation(CodeableConcept value) { 182 this.unitOfPresentation = value; 183 return this; 184 } 185 186 /** 187 * @return {@link #quantity} (The quantity or "count number" of the manufactured 188 * item.) 189 */ 190 public Quantity getQuantity() { 191 if (this.quantity == null) 192 if (Configuration.errorOnAutoCreate()) 193 throw new Error("Attempt to auto-create MedicinalProductManufactured.quantity"); 194 else if (Configuration.doAutoCreate()) 195 this.quantity = new Quantity(); // cc 196 return this.quantity; 197 } 198 199 public boolean hasQuantity() { 200 return this.quantity != null && !this.quantity.isEmpty(); 201 } 202 203 /** 204 * @param value {@link #quantity} (The quantity or "count number" of the 205 * manufactured item.) 206 */ 207 public MedicinalProductManufactured setQuantity(Quantity value) { 208 this.quantity = value; 209 return this; 210 } 211 212 /** 213 * @return {@link #manufacturer} (Manufacturer of the item (Note that this 214 * should be named "manufacturer" but it currently causes technical 215 * issues).) 216 */ 217 public List<Reference> getManufacturer() { 218 if (this.manufacturer == null) 219 this.manufacturer = new ArrayList<Reference>(); 220 return this.manufacturer; 221 } 222 223 /** 224 * @return Returns a reference to <code>this</code> for easy method chaining 225 */ 226 public MedicinalProductManufactured setManufacturer(List<Reference> theManufacturer) { 227 this.manufacturer = theManufacturer; 228 return this; 229 } 230 231 public boolean hasManufacturer() { 232 if (this.manufacturer == null) 233 return false; 234 for (Reference item : this.manufacturer) 235 if (!item.isEmpty()) 236 return true; 237 return false; 238 } 239 240 public Reference addManufacturer() { // 3 241 Reference t = new Reference(); 242 if (this.manufacturer == null) 243 this.manufacturer = new ArrayList<Reference>(); 244 this.manufacturer.add(t); 245 return t; 246 } 247 248 public MedicinalProductManufactured addManufacturer(Reference t) { // 3 249 if (t == null) 250 return this; 251 if (this.manufacturer == null) 252 this.manufacturer = new ArrayList<Reference>(); 253 this.manufacturer.add(t); 254 return this; 255 } 256 257 /** 258 * @return The first repetition of repeating field {@link #manufacturer}, 259 * creating it if it does not already exist 260 */ 261 public Reference getManufacturerFirstRep() { 262 if (getManufacturer().isEmpty()) { 263 addManufacturer(); 264 } 265 return getManufacturer().get(0); 266 } 267 268 /** 269 * @deprecated Use Reference#setResource(IBaseResource) instead 270 */ 271 @Deprecated 272 public List<Organization> getManufacturerTarget() { 273 if (this.manufacturerTarget == null) 274 this.manufacturerTarget = new ArrayList<Organization>(); 275 return this.manufacturerTarget; 276 } 277 278 /** 279 * @deprecated Use Reference#setResource(IBaseResource) instead 280 */ 281 @Deprecated 282 public Organization addManufacturerTarget() { 283 Organization r = new Organization(); 284 if (this.manufacturerTarget == null) 285 this.manufacturerTarget = new ArrayList<Organization>(); 286 this.manufacturerTarget.add(r); 287 return r; 288 } 289 290 /** 291 * @return {@link #ingredient} (Ingredient.) 292 */ 293 public List<Reference> getIngredient() { 294 if (this.ingredient == null) 295 this.ingredient = new ArrayList<Reference>(); 296 return this.ingredient; 297 } 298 299 /** 300 * @return Returns a reference to <code>this</code> for easy method chaining 301 */ 302 public MedicinalProductManufactured setIngredient(List<Reference> theIngredient) { 303 this.ingredient = theIngredient; 304 return this; 305 } 306 307 public boolean hasIngredient() { 308 if (this.ingredient == null) 309 return false; 310 for (Reference item : this.ingredient) 311 if (!item.isEmpty()) 312 return true; 313 return false; 314 } 315 316 public Reference addIngredient() { // 3 317 Reference t = new Reference(); 318 if (this.ingredient == null) 319 this.ingredient = new ArrayList<Reference>(); 320 this.ingredient.add(t); 321 return t; 322 } 323 324 public MedicinalProductManufactured addIngredient(Reference t) { // 3 325 if (t == null) 326 return this; 327 if (this.ingredient == null) 328 this.ingredient = new ArrayList<Reference>(); 329 this.ingredient.add(t); 330 return this; 331 } 332 333 /** 334 * @return The first repetition of repeating field {@link #ingredient}, creating 335 * it if it does not already exist 336 */ 337 public Reference getIngredientFirstRep() { 338 if (getIngredient().isEmpty()) { 339 addIngredient(); 340 } 341 return getIngredient().get(0); 342 } 343 344 /** 345 * @deprecated Use Reference#setResource(IBaseResource) instead 346 */ 347 @Deprecated 348 public List<MedicinalProductIngredient> getIngredientTarget() { 349 if (this.ingredientTarget == null) 350 this.ingredientTarget = new ArrayList<MedicinalProductIngredient>(); 351 return this.ingredientTarget; 352 } 353 354 /** 355 * @deprecated Use Reference#setResource(IBaseResource) instead 356 */ 357 @Deprecated 358 public MedicinalProductIngredient addIngredientTarget() { 359 MedicinalProductIngredient r = new MedicinalProductIngredient(); 360 if (this.ingredientTarget == null) 361 this.ingredientTarget = new ArrayList<MedicinalProductIngredient>(); 362 this.ingredientTarget.add(r); 363 return r; 364 } 365 366 /** 367 * @return {@link #physicalCharacteristics} (Dimensions, color etc.) 368 */ 369 public ProdCharacteristic getPhysicalCharacteristics() { 370 if (this.physicalCharacteristics == null) 371 if (Configuration.errorOnAutoCreate()) 372 throw new Error("Attempt to auto-create MedicinalProductManufactured.physicalCharacteristics"); 373 else if (Configuration.doAutoCreate()) 374 this.physicalCharacteristics = new ProdCharacteristic(); // cc 375 return this.physicalCharacteristics; 376 } 377 378 public boolean hasPhysicalCharacteristics() { 379 return this.physicalCharacteristics != null && !this.physicalCharacteristics.isEmpty(); 380 } 381 382 /** 383 * @param value {@link #physicalCharacteristics} (Dimensions, color etc.) 384 */ 385 public MedicinalProductManufactured setPhysicalCharacteristics(ProdCharacteristic value) { 386 this.physicalCharacteristics = value; 387 return this; 388 } 389 390 /** 391 * @return {@link #otherCharacteristics} (Other codeable characteristics.) 392 */ 393 public List<CodeableConcept> getOtherCharacteristics() { 394 if (this.otherCharacteristics == null) 395 this.otherCharacteristics = new ArrayList<CodeableConcept>(); 396 return this.otherCharacteristics; 397 } 398 399 /** 400 * @return Returns a reference to <code>this</code> for easy method chaining 401 */ 402 public MedicinalProductManufactured setOtherCharacteristics(List<CodeableConcept> theOtherCharacteristics) { 403 this.otherCharacteristics = theOtherCharacteristics; 404 return this; 405 } 406 407 public boolean hasOtherCharacteristics() { 408 if (this.otherCharacteristics == null) 409 return false; 410 for (CodeableConcept item : this.otherCharacteristics) 411 if (!item.isEmpty()) 412 return true; 413 return false; 414 } 415 416 public CodeableConcept addOtherCharacteristics() { // 3 417 CodeableConcept t = new CodeableConcept(); 418 if (this.otherCharacteristics == null) 419 this.otherCharacteristics = new ArrayList<CodeableConcept>(); 420 this.otherCharacteristics.add(t); 421 return t; 422 } 423 424 public MedicinalProductManufactured addOtherCharacteristics(CodeableConcept t) { // 3 425 if (t == null) 426 return this; 427 if (this.otherCharacteristics == null) 428 this.otherCharacteristics = new ArrayList<CodeableConcept>(); 429 this.otherCharacteristics.add(t); 430 return this; 431 } 432 433 /** 434 * @return The first repetition of repeating field 435 * {@link #otherCharacteristics}, creating it if it does not already 436 * exist 437 */ 438 public CodeableConcept getOtherCharacteristicsFirstRep() { 439 if (getOtherCharacteristics().isEmpty()) { 440 addOtherCharacteristics(); 441 } 442 return getOtherCharacteristics().get(0); 443 } 444 445 protected void listChildren(List<Property> children) { 446 super.listChildren(children); 447 children.add(new Property("manufacturedDoseForm", "CodeableConcept", 448 "Dose form as manufactured and before any transformation into the pharmaceutical product.", 0, 1, 449 manufacturedDoseForm)); 450 children.add(new Property("unitOfPresentation", "CodeableConcept", 451 "The ?real world? units in which the quantity of the manufactured item is described.", 0, 1, 452 unitOfPresentation)); 453 children.add(new Property("quantity", "Quantity", "The quantity or \"count number\" of the manufactured item.", 0, 454 1, quantity)); 455 children.add(new Property("manufacturer", "Reference(Organization)", 456 "Manufacturer of the item (Note that this should be named \"manufacturer\" but it currently causes technical issues).", 457 0, java.lang.Integer.MAX_VALUE, manufacturer)); 458 children.add(new Property("ingredient", "Reference(MedicinalProductIngredient)", "Ingredient.", 0, 459 java.lang.Integer.MAX_VALUE, ingredient)); 460 children.add(new Property("physicalCharacteristics", "ProdCharacteristic", "Dimensions, color etc.", 0, 1, 461 physicalCharacteristics)); 462 children.add(new Property("otherCharacteristics", "CodeableConcept", "Other codeable characteristics.", 0, 463 java.lang.Integer.MAX_VALUE, otherCharacteristics)); 464 } 465 466 @Override 467 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 468 switch (_hash) { 469 case -1451400348: 470 /* manufacturedDoseForm */ return new Property("manufacturedDoseForm", "CodeableConcept", 471 "Dose form as manufactured and before any transformation into the pharmaceutical product.", 0, 1, 472 manufacturedDoseForm); 473 case -1427765963: 474 /* unitOfPresentation */ return new Property("unitOfPresentation", "CodeableConcept", 475 "The ?real world? units in which the quantity of the manufactured item is described.", 0, 1, 476 unitOfPresentation); 477 case -1285004149: 478 /* quantity */ return new Property("quantity", "Quantity", 479 "The quantity or \"count number\" of the manufactured item.", 0, 1, quantity); 480 case -1969347631: 481 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 482 "Manufacturer of the item (Note that this should be named \"manufacturer\" but it currently causes technical issues).", 483 0, java.lang.Integer.MAX_VALUE, manufacturer); 484 case -206409263: 485 /* ingredient */ return new Property("ingredient", "Reference(MedicinalProductIngredient)", "Ingredient.", 0, 486 java.lang.Integer.MAX_VALUE, ingredient); 487 case -1599676319: 488 /* physicalCharacteristics */ return new Property("physicalCharacteristics", "ProdCharacteristic", 489 "Dimensions, color etc.", 0, 1, physicalCharacteristics); 490 case 722135304: 491 /* otherCharacteristics */ return new Property("otherCharacteristics", "CodeableConcept", 492 "Other codeable characteristics.", 0, java.lang.Integer.MAX_VALUE, otherCharacteristics); 493 default: 494 return super.getNamedProperty(_hash, _name, _checkValid); 495 } 496 497 } 498 499 @Override 500 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 501 switch (hash) { 502 case -1451400348: 503 /* manufacturedDoseForm */ return this.manufacturedDoseForm == null ? new Base[0] 504 : new Base[] { this.manufacturedDoseForm }; // CodeableConcept 505 case -1427765963: 506 /* unitOfPresentation */ return this.unitOfPresentation == null ? new Base[0] 507 : new Base[] { this.unitOfPresentation }; // CodeableConcept 508 case -1285004149: 509 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 510 case -1969347631: 511 /* manufacturer */ return this.manufacturer == null ? new Base[0] 512 : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 513 case -206409263: 514 /* ingredient */ return this.ingredient == null ? new Base[0] 515 : this.ingredient.toArray(new Base[this.ingredient.size()]); // Reference 516 case -1599676319: 517 /* physicalCharacteristics */ return this.physicalCharacteristics == null ? new Base[0] 518 : new Base[] { this.physicalCharacteristics }; // ProdCharacteristic 519 case 722135304: 520 /* otherCharacteristics */ return this.otherCharacteristics == null ? new Base[0] 521 : this.otherCharacteristics.toArray(new Base[this.otherCharacteristics.size()]); // CodeableConcept 522 default: 523 return super.getProperty(hash, name, checkValid); 524 } 525 526 } 527 528 @Override 529 public Base setProperty(int hash, String name, Base value) throws FHIRException { 530 switch (hash) { 531 case -1451400348: // manufacturedDoseForm 532 this.manufacturedDoseForm = castToCodeableConcept(value); // CodeableConcept 533 return value; 534 case -1427765963: // unitOfPresentation 535 this.unitOfPresentation = castToCodeableConcept(value); // CodeableConcept 536 return value; 537 case -1285004149: // quantity 538 this.quantity = castToQuantity(value); // Quantity 539 return value; 540 case -1969347631: // manufacturer 541 this.getManufacturer().add(castToReference(value)); // Reference 542 return value; 543 case -206409263: // ingredient 544 this.getIngredient().add(castToReference(value)); // Reference 545 return value; 546 case -1599676319: // physicalCharacteristics 547 this.physicalCharacteristics = castToProdCharacteristic(value); // ProdCharacteristic 548 return value; 549 case 722135304: // otherCharacteristics 550 this.getOtherCharacteristics().add(castToCodeableConcept(value)); // CodeableConcept 551 return value; 552 default: 553 return super.setProperty(hash, name, value); 554 } 555 556 } 557 558 @Override 559 public Base setProperty(String name, Base value) throws FHIRException { 560 if (name.equals("manufacturedDoseForm")) { 561 this.manufacturedDoseForm = castToCodeableConcept(value); // CodeableConcept 562 } else if (name.equals("unitOfPresentation")) { 563 this.unitOfPresentation = castToCodeableConcept(value); // CodeableConcept 564 } else if (name.equals("quantity")) { 565 this.quantity = castToQuantity(value); // Quantity 566 } else if (name.equals("manufacturer")) { 567 this.getManufacturer().add(castToReference(value)); 568 } else if (name.equals("ingredient")) { 569 this.getIngredient().add(castToReference(value)); 570 } else if (name.equals("physicalCharacteristics")) { 571 this.physicalCharacteristics = castToProdCharacteristic(value); // ProdCharacteristic 572 } else if (name.equals("otherCharacteristics")) { 573 this.getOtherCharacteristics().add(castToCodeableConcept(value)); 574 } else 575 return super.setProperty(name, value); 576 return value; 577 } 578 579 @Override 580 public void removeChild(String name, Base value) throws FHIRException { 581 if (name.equals("manufacturedDoseForm")) { 582 this.manufacturedDoseForm = null; 583 } else if (name.equals("unitOfPresentation")) { 584 this.unitOfPresentation = null; 585 } else if (name.equals("quantity")) { 586 this.quantity = null; 587 } else if (name.equals("manufacturer")) { 588 this.getManufacturer().remove(castToReference(value)); 589 } else if (name.equals("ingredient")) { 590 this.getIngredient().remove(castToReference(value)); 591 } else if (name.equals("physicalCharacteristics")) { 592 this.physicalCharacteristics = null; 593 } else if (name.equals("otherCharacteristics")) { 594 this.getOtherCharacteristics().remove(castToCodeableConcept(value)); 595 } else 596 super.removeChild(name, value); 597 598 } 599 600 @Override 601 public Base makeProperty(int hash, String name) throws FHIRException { 602 switch (hash) { 603 case -1451400348: 604 return getManufacturedDoseForm(); 605 case -1427765963: 606 return getUnitOfPresentation(); 607 case -1285004149: 608 return getQuantity(); 609 case -1969347631: 610 return addManufacturer(); 611 case -206409263: 612 return addIngredient(); 613 case -1599676319: 614 return getPhysicalCharacteristics(); 615 case 722135304: 616 return addOtherCharacteristics(); 617 default: 618 return super.makeProperty(hash, name); 619 } 620 621 } 622 623 @Override 624 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 625 switch (hash) { 626 case -1451400348: 627 /* manufacturedDoseForm */ return new String[] { "CodeableConcept" }; 628 case -1427765963: 629 /* unitOfPresentation */ return new String[] { "CodeableConcept" }; 630 case -1285004149: 631 /* quantity */ return new String[] { "Quantity" }; 632 case -1969347631: 633 /* manufacturer */ return new String[] { "Reference" }; 634 case -206409263: 635 /* ingredient */ return new String[] { "Reference" }; 636 case -1599676319: 637 /* physicalCharacteristics */ return new String[] { "ProdCharacteristic" }; 638 case 722135304: 639 /* otherCharacteristics */ return new String[] { "CodeableConcept" }; 640 default: 641 return super.getTypesForProperty(hash, name); 642 } 643 644 } 645 646 @Override 647 public Base addChild(String name) throws FHIRException { 648 if (name.equals("manufacturedDoseForm")) { 649 this.manufacturedDoseForm = new CodeableConcept(); 650 return this.manufacturedDoseForm; 651 } else if (name.equals("unitOfPresentation")) { 652 this.unitOfPresentation = new CodeableConcept(); 653 return this.unitOfPresentation; 654 } else if (name.equals("quantity")) { 655 this.quantity = new Quantity(); 656 return this.quantity; 657 } else if (name.equals("manufacturer")) { 658 return addManufacturer(); 659 } else if (name.equals("ingredient")) { 660 return addIngredient(); 661 } else if (name.equals("physicalCharacteristics")) { 662 this.physicalCharacteristics = new ProdCharacteristic(); 663 return this.physicalCharacteristics; 664 } else if (name.equals("otherCharacteristics")) { 665 return addOtherCharacteristics(); 666 } else 667 return super.addChild(name); 668 } 669 670 public String fhirType() { 671 return "MedicinalProductManufactured"; 672 673 } 674 675 public MedicinalProductManufactured copy() { 676 MedicinalProductManufactured dst = new MedicinalProductManufactured(); 677 copyValues(dst); 678 return dst; 679 } 680 681 public void copyValues(MedicinalProductManufactured dst) { 682 super.copyValues(dst); 683 dst.manufacturedDoseForm = manufacturedDoseForm == null ? null : manufacturedDoseForm.copy(); 684 dst.unitOfPresentation = unitOfPresentation == null ? null : unitOfPresentation.copy(); 685 dst.quantity = quantity == null ? null : quantity.copy(); 686 if (manufacturer != null) { 687 dst.manufacturer = new ArrayList<Reference>(); 688 for (Reference i : manufacturer) 689 dst.manufacturer.add(i.copy()); 690 } 691 ; 692 if (ingredient != null) { 693 dst.ingredient = new ArrayList<Reference>(); 694 for (Reference i : ingredient) 695 dst.ingredient.add(i.copy()); 696 } 697 ; 698 dst.physicalCharacteristics = physicalCharacteristics == null ? null : physicalCharacteristics.copy(); 699 if (otherCharacteristics != null) { 700 dst.otherCharacteristics = new ArrayList<CodeableConcept>(); 701 for (CodeableConcept i : otherCharacteristics) 702 dst.otherCharacteristics.add(i.copy()); 703 } 704 ; 705 } 706 707 protected MedicinalProductManufactured typedCopy() { 708 return copy(); 709 } 710 711 @Override 712 public boolean equalsDeep(Base other_) { 713 if (!super.equalsDeep(other_)) 714 return false; 715 if (!(other_ instanceof MedicinalProductManufactured)) 716 return false; 717 MedicinalProductManufactured o = (MedicinalProductManufactured) other_; 718 return compareDeep(manufacturedDoseForm, o.manufacturedDoseForm, true) 719 && compareDeep(unitOfPresentation, o.unitOfPresentation, true) && compareDeep(quantity, o.quantity, true) 720 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(ingredient, o.ingredient, true) 721 && compareDeep(physicalCharacteristics, o.physicalCharacteristics, true) 722 && compareDeep(otherCharacteristics, o.otherCharacteristics, true); 723 } 724 725 @Override 726 public boolean equalsShallow(Base other_) { 727 if (!super.equalsShallow(other_)) 728 return false; 729 if (!(other_ instanceof MedicinalProductManufactured)) 730 return false; 731 MedicinalProductManufactured o = (MedicinalProductManufactured) other_; 732 return true; 733 } 734 735 public boolean isEmpty() { 736 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(manufacturedDoseForm, unitOfPresentation, quantity, 737 manufacturer, ingredient, physicalCharacteristics, otherCharacteristics); 738 } 739 740 @Override 741 public ResourceType getResourceType() { 742 return ResourceType.MedicinalProductManufactured; 743 } 744 745}