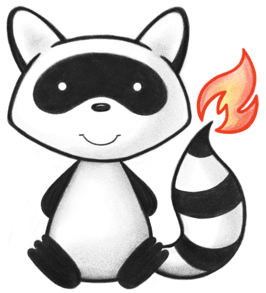
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A medicinal product in a container or package. 048 */ 049@ResourceDef(name = "MedicinalProductPackaged", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductPackaged") 050public class MedicinalProductPackaged extends DomainResource { 051 052 @Block() 053 public static class MedicinalProductPackagedBatchIdentifierComponent extends BackboneElement 054 implements IBaseBackboneElement { 055 /** 056 * A number appearing on the outer packaging of a specific batch. 057 */ 058 @Child(name = "outerPackaging", type = { 059 Identifier.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "A number appearing on the outer packaging of a specific batch", formalDefinition = "A number appearing on the outer packaging of a specific batch.") 061 protected Identifier outerPackaging; 062 063 /** 064 * A number appearing on the immediate packaging (and not the outer packaging). 065 */ 066 @Child(name = "immediatePackaging", type = { 067 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "A number appearing on the immediate packaging (and not the outer packaging)", formalDefinition = "A number appearing on the immediate packaging (and not the outer packaging).") 069 protected Identifier immediatePackaging; 070 071 private static final long serialVersionUID = 1187365068L; 072 073 /** 074 * Constructor 075 */ 076 public MedicinalProductPackagedBatchIdentifierComponent() { 077 super(); 078 } 079 080 /** 081 * Constructor 082 */ 083 public MedicinalProductPackagedBatchIdentifierComponent(Identifier outerPackaging) { 084 super(); 085 this.outerPackaging = outerPackaging; 086 } 087 088 /** 089 * @return {@link #outerPackaging} (A number appearing on the outer packaging of 090 * a specific batch.) 091 */ 092 public Identifier getOuterPackaging() { 093 if (this.outerPackaging == null) 094 if (Configuration.errorOnAutoCreate()) 095 throw new Error("Attempt to auto-create MedicinalProductPackagedBatchIdentifierComponent.outerPackaging"); 096 else if (Configuration.doAutoCreate()) 097 this.outerPackaging = new Identifier(); // cc 098 return this.outerPackaging; 099 } 100 101 public boolean hasOuterPackaging() { 102 return this.outerPackaging != null && !this.outerPackaging.isEmpty(); 103 } 104 105 /** 106 * @param value {@link #outerPackaging} (A number appearing on the outer 107 * packaging of a specific batch.) 108 */ 109 public MedicinalProductPackagedBatchIdentifierComponent setOuterPackaging(Identifier value) { 110 this.outerPackaging = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #immediatePackaging} (A number appearing on the immediate 116 * packaging (and not the outer packaging).) 117 */ 118 public Identifier getImmediatePackaging() { 119 if (this.immediatePackaging == null) 120 if (Configuration.errorOnAutoCreate()) 121 throw new Error("Attempt to auto-create MedicinalProductPackagedBatchIdentifierComponent.immediatePackaging"); 122 else if (Configuration.doAutoCreate()) 123 this.immediatePackaging = new Identifier(); // cc 124 return this.immediatePackaging; 125 } 126 127 public boolean hasImmediatePackaging() { 128 return this.immediatePackaging != null && !this.immediatePackaging.isEmpty(); 129 } 130 131 /** 132 * @param value {@link #immediatePackaging} (A number appearing on the immediate 133 * packaging (and not the outer packaging).) 134 */ 135 public MedicinalProductPackagedBatchIdentifierComponent setImmediatePackaging(Identifier value) { 136 this.immediatePackaging = value; 137 return this; 138 } 139 140 protected void listChildren(List<Property> children) { 141 super.listChildren(children); 142 children.add(new Property("outerPackaging", "Identifier", 143 "A number appearing on the outer packaging of a specific batch.", 0, 1, outerPackaging)); 144 children.add(new Property("immediatePackaging", "Identifier", 145 "A number appearing on the immediate packaging (and not the outer packaging).", 0, 1, immediatePackaging)); 146 } 147 148 @Override 149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 150 switch (_hash) { 151 case -682249912: 152 /* outerPackaging */ return new Property("outerPackaging", "Identifier", 153 "A number appearing on the outer packaging of a specific batch.", 0, 1, outerPackaging); 154 case 721147602: 155 /* immediatePackaging */ return new Property("immediatePackaging", "Identifier", 156 "A number appearing on the immediate packaging (and not the outer packaging).", 0, 1, immediatePackaging); 157 default: 158 return super.getNamedProperty(_hash, _name, _checkValid); 159 } 160 161 } 162 163 @Override 164 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 165 switch (hash) { 166 case -682249912: 167 /* outerPackaging */ return this.outerPackaging == null ? new Base[0] : new Base[] { this.outerPackaging }; // Identifier 168 case 721147602: 169 /* immediatePackaging */ return this.immediatePackaging == null ? new Base[0] 170 : new Base[] { this.immediatePackaging }; // Identifier 171 default: 172 return super.getProperty(hash, name, checkValid); 173 } 174 175 } 176 177 @Override 178 public Base setProperty(int hash, String name, Base value) throws FHIRException { 179 switch (hash) { 180 case -682249912: // outerPackaging 181 this.outerPackaging = castToIdentifier(value); // Identifier 182 return value; 183 case 721147602: // immediatePackaging 184 this.immediatePackaging = castToIdentifier(value); // Identifier 185 return value; 186 default: 187 return super.setProperty(hash, name, value); 188 } 189 190 } 191 192 @Override 193 public Base setProperty(String name, Base value) throws FHIRException { 194 if (name.equals("outerPackaging")) { 195 this.outerPackaging = castToIdentifier(value); // Identifier 196 } else if (name.equals("immediatePackaging")) { 197 this.immediatePackaging = castToIdentifier(value); // Identifier 198 } else 199 return super.setProperty(name, value); 200 return value; 201 } 202 203 @Override 204 public void removeChild(String name, Base value) throws FHIRException { 205 if (name.equals("outerPackaging")) { 206 this.outerPackaging = null; 207 } else if (name.equals("immediatePackaging")) { 208 this.immediatePackaging = null; 209 } else 210 super.removeChild(name, value); 211 212 } 213 214 @Override 215 public Base makeProperty(int hash, String name) throws FHIRException { 216 switch (hash) { 217 case -682249912: 218 return getOuterPackaging(); 219 case 721147602: 220 return getImmediatePackaging(); 221 default: 222 return super.makeProperty(hash, name); 223 } 224 225 } 226 227 @Override 228 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 229 switch (hash) { 230 case -682249912: 231 /* outerPackaging */ return new String[] { "Identifier" }; 232 case 721147602: 233 /* immediatePackaging */ return new String[] { "Identifier" }; 234 default: 235 return super.getTypesForProperty(hash, name); 236 } 237 238 } 239 240 @Override 241 public Base addChild(String name) throws FHIRException { 242 if (name.equals("outerPackaging")) { 243 this.outerPackaging = new Identifier(); 244 return this.outerPackaging; 245 } else if (name.equals("immediatePackaging")) { 246 this.immediatePackaging = new Identifier(); 247 return this.immediatePackaging; 248 } else 249 return super.addChild(name); 250 } 251 252 public MedicinalProductPackagedBatchIdentifierComponent copy() { 253 MedicinalProductPackagedBatchIdentifierComponent dst = new MedicinalProductPackagedBatchIdentifierComponent(); 254 copyValues(dst); 255 return dst; 256 } 257 258 public void copyValues(MedicinalProductPackagedBatchIdentifierComponent dst) { 259 super.copyValues(dst); 260 dst.outerPackaging = outerPackaging == null ? null : outerPackaging.copy(); 261 dst.immediatePackaging = immediatePackaging == null ? null : immediatePackaging.copy(); 262 } 263 264 @Override 265 public boolean equalsDeep(Base other_) { 266 if (!super.equalsDeep(other_)) 267 return false; 268 if (!(other_ instanceof MedicinalProductPackagedBatchIdentifierComponent)) 269 return false; 270 MedicinalProductPackagedBatchIdentifierComponent o = (MedicinalProductPackagedBatchIdentifierComponent) other_; 271 return compareDeep(outerPackaging, o.outerPackaging, true) 272 && compareDeep(immediatePackaging, o.immediatePackaging, true); 273 } 274 275 @Override 276 public boolean equalsShallow(Base other_) { 277 if (!super.equalsShallow(other_)) 278 return false; 279 if (!(other_ instanceof MedicinalProductPackagedBatchIdentifierComponent)) 280 return false; 281 MedicinalProductPackagedBatchIdentifierComponent o = (MedicinalProductPackagedBatchIdentifierComponent) other_; 282 return true; 283 } 284 285 public boolean isEmpty() { 286 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(outerPackaging, immediatePackaging); 287 } 288 289 public String fhirType() { 290 return "MedicinalProductPackaged.batchIdentifier"; 291 292 } 293 294 } 295 296 @Block() 297 public static class MedicinalProductPackagedPackageItemComponent extends BackboneElement 298 implements IBaseBackboneElement { 299 /** 300 * Including possibly Data Carrier Identifier. 301 */ 302 @Child(name = "identifier", type = { 303 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 304 @Description(shortDefinition = "Including possibly Data Carrier Identifier", formalDefinition = "Including possibly Data Carrier Identifier.") 305 protected List<Identifier> identifier; 306 307 /** 308 * The physical type of the container of the medicine. 309 */ 310 @Child(name = "type", type = { 311 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 312 @Description(shortDefinition = "The physical type of the container of the medicine", formalDefinition = "The physical type of the container of the medicine.") 313 protected CodeableConcept type; 314 315 /** 316 * The quantity of this package in the medicinal product, at the current level 317 * of packaging. The outermost is always 1. 318 */ 319 @Child(name = "quantity", type = { Quantity.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 320 @Description(shortDefinition = "The quantity of this package in the medicinal product, at the current level of packaging. The outermost is always 1", formalDefinition = "The quantity of this package in the medicinal product, at the current level of packaging. The outermost is always 1.") 321 protected Quantity quantity; 322 323 /** 324 * Material type of the package item. 325 */ 326 @Child(name = "material", type = { 327 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 328 @Description(shortDefinition = "Material type of the package item", formalDefinition = "Material type of the package item.") 329 protected List<CodeableConcept> material; 330 331 /** 332 * A possible alternate material for the packaging. 333 */ 334 @Child(name = "alternateMaterial", type = { 335 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 336 @Description(shortDefinition = "A possible alternate material for the packaging", formalDefinition = "A possible alternate material for the packaging.") 337 protected List<CodeableConcept> alternateMaterial; 338 339 /** 340 * A device accompanying a medicinal product. 341 */ 342 @Child(name = "device", type = { 343 DeviceDefinition.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 344 @Description(shortDefinition = "A device accompanying a medicinal product", formalDefinition = "A device accompanying a medicinal product.") 345 protected List<Reference> device; 346 /** 347 * The actual objects that are the target of the reference (A device 348 * accompanying a medicinal product.) 349 */ 350 protected List<DeviceDefinition> deviceTarget; 351 352 /** 353 * The manufactured item as contained in the packaged medicinal product. 354 */ 355 @Child(name = "manufacturedItem", type = { 356 MedicinalProductManufactured.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 357 @Description(shortDefinition = "The manufactured item as contained in the packaged medicinal product", formalDefinition = "The manufactured item as contained in the packaged medicinal product.") 358 protected List<Reference> manufacturedItem; 359 /** 360 * The actual objects that are the target of the reference (The manufactured 361 * item as contained in the packaged medicinal product.) 362 */ 363 protected List<MedicinalProductManufactured> manufacturedItemTarget; 364 365 /** 366 * Allows containers within containers. 367 */ 368 @Child(name = "packageItem", type = { 369 MedicinalProductPackagedPackageItemComponent.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 370 @Description(shortDefinition = "Allows containers within containers", formalDefinition = "Allows containers within containers.") 371 protected List<MedicinalProductPackagedPackageItemComponent> packageItem; 372 373 /** 374 * Dimensions, color etc. 375 */ 376 @Child(name = "physicalCharacteristics", type = { 377 ProdCharacteristic.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 378 @Description(shortDefinition = "Dimensions, color etc.", formalDefinition = "Dimensions, color etc.") 379 protected ProdCharacteristic physicalCharacteristics; 380 381 /** 382 * Other codeable characteristics. 383 */ 384 @Child(name = "otherCharacteristics", type = { 385 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 386 @Description(shortDefinition = "Other codeable characteristics", formalDefinition = "Other codeable characteristics.") 387 protected List<CodeableConcept> otherCharacteristics; 388 389 /** 390 * Shelf Life and storage information. 391 */ 392 @Child(name = "shelfLifeStorage", type = { 393 ProductShelfLife.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 394 @Description(shortDefinition = "Shelf Life and storage information", formalDefinition = "Shelf Life and storage information.") 395 protected List<ProductShelfLife> shelfLifeStorage; 396 397 /** 398 * Manufacturer of this Package Item. 399 */ 400 @Child(name = "manufacturer", type = { 401 Organization.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 402 @Description(shortDefinition = "Manufacturer of this Package Item", formalDefinition = "Manufacturer of this Package Item.") 403 protected List<Reference> manufacturer; 404 /** 405 * The actual objects that are the target of the reference (Manufacturer of this 406 * Package Item.) 407 */ 408 protected List<Organization> manufacturerTarget; 409 410 private static final long serialVersionUID = 1454286533L; 411 412 /** 413 * Constructor 414 */ 415 public MedicinalProductPackagedPackageItemComponent() { 416 super(); 417 } 418 419 /** 420 * Constructor 421 */ 422 public MedicinalProductPackagedPackageItemComponent(CodeableConcept type, Quantity quantity) { 423 super(); 424 this.type = type; 425 this.quantity = quantity; 426 } 427 428 /** 429 * @return {@link #identifier} (Including possibly Data Carrier Identifier.) 430 */ 431 public List<Identifier> getIdentifier() { 432 if (this.identifier == null) 433 this.identifier = new ArrayList<Identifier>(); 434 return this.identifier; 435 } 436 437 /** 438 * @return Returns a reference to <code>this</code> for easy method chaining 439 */ 440 public MedicinalProductPackagedPackageItemComponent setIdentifier(List<Identifier> theIdentifier) { 441 this.identifier = theIdentifier; 442 return this; 443 } 444 445 public boolean hasIdentifier() { 446 if (this.identifier == null) 447 return false; 448 for (Identifier item : this.identifier) 449 if (!item.isEmpty()) 450 return true; 451 return false; 452 } 453 454 public Identifier addIdentifier() { // 3 455 Identifier t = new Identifier(); 456 if (this.identifier == null) 457 this.identifier = new ArrayList<Identifier>(); 458 this.identifier.add(t); 459 return t; 460 } 461 462 public MedicinalProductPackagedPackageItemComponent addIdentifier(Identifier t) { // 3 463 if (t == null) 464 return this; 465 if (this.identifier == null) 466 this.identifier = new ArrayList<Identifier>(); 467 this.identifier.add(t); 468 return this; 469 } 470 471 /** 472 * @return The first repetition of repeating field {@link #identifier}, creating 473 * it if it does not already exist 474 */ 475 public Identifier getIdentifierFirstRep() { 476 if (getIdentifier().isEmpty()) { 477 addIdentifier(); 478 } 479 return getIdentifier().get(0); 480 } 481 482 /** 483 * @return {@link #type} (The physical type of the container of the medicine.) 484 */ 485 public CodeableConcept getType() { 486 if (this.type == null) 487 if (Configuration.errorOnAutoCreate()) 488 throw new Error("Attempt to auto-create MedicinalProductPackagedPackageItemComponent.type"); 489 else if (Configuration.doAutoCreate()) 490 this.type = new CodeableConcept(); // cc 491 return this.type; 492 } 493 494 public boolean hasType() { 495 return this.type != null && !this.type.isEmpty(); 496 } 497 498 /** 499 * @param value {@link #type} (The physical type of the container of the 500 * medicine.) 501 */ 502 public MedicinalProductPackagedPackageItemComponent setType(CodeableConcept value) { 503 this.type = value; 504 return this; 505 } 506 507 /** 508 * @return {@link #quantity} (The quantity of this package in the medicinal 509 * product, at the current level of packaging. The outermost is always 510 * 1.) 511 */ 512 public Quantity getQuantity() { 513 if (this.quantity == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create MedicinalProductPackagedPackageItemComponent.quantity"); 516 else if (Configuration.doAutoCreate()) 517 this.quantity = new Quantity(); // cc 518 return this.quantity; 519 } 520 521 public boolean hasQuantity() { 522 return this.quantity != null && !this.quantity.isEmpty(); 523 } 524 525 /** 526 * @param value {@link #quantity} (The quantity of this package in the medicinal 527 * product, at the current level of packaging. The outermost is 528 * always 1.) 529 */ 530 public MedicinalProductPackagedPackageItemComponent setQuantity(Quantity value) { 531 this.quantity = value; 532 return this; 533 } 534 535 /** 536 * @return {@link #material} (Material type of the package item.) 537 */ 538 public List<CodeableConcept> getMaterial() { 539 if (this.material == null) 540 this.material = new ArrayList<CodeableConcept>(); 541 return this.material; 542 } 543 544 /** 545 * @return Returns a reference to <code>this</code> for easy method chaining 546 */ 547 public MedicinalProductPackagedPackageItemComponent setMaterial(List<CodeableConcept> theMaterial) { 548 this.material = theMaterial; 549 return this; 550 } 551 552 public boolean hasMaterial() { 553 if (this.material == null) 554 return false; 555 for (CodeableConcept item : this.material) 556 if (!item.isEmpty()) 557 return true; 558 return false; 559 } 560 561 public CodeableConcept addMaterial() { // 3 562 CodeableConcept t = new CodeableConcept(); 563 if (this.material == null) 564 this.material = new ArrayList<CodeableConcept>(); 565 this.material.add(t); 566 return t; 567 } 568 569 public MedicinalProductPackagedPackageItemComponent addMaterial(CodeableConcept t) { // 3 570 if (t == null) 571 return this; 572 if (this.material == null) 573 this.material = new ArrayList<CodeableConcept>(); 574 this.material.add(t); 575 return this; 576 } 577 578 /** 579 * @return The first repetition of repeating field {@link #material}, creating 580 * it if it does not already exist 581 */ 582 public CodeableConcept getMaterialFirstRep() { 583 if (getMaterial().isEmpty()) { 584 addMaterial(); 585 } 586 return getMaterial().get(0); 587 } 588 589 /** 590 * @return {@link #alternateMaterial} (A possible alternate material for the 591 * packaging.) 592 */ 593 public List<CodeableConcept> getAlternateMaterial() { 594 if (this.alternateMaterial == null) 595 this.alternateMaterial = new ArrayList<CodeableConcept>(); 596 return this.alternateMaterial; 597 } 598 599 /** 600 * @return Returns a reference to <code>this</code> for easy method chaining 601 */ 602 public MedicinalProductPackagedPackageItemComponent setAlternateMaterial( 603 List<CodeableConcept> theAlternateMaterial) { 604 this.alternateMaterial = theAlternateMaterial; 605 return this; 606 } 607 608 public boolean hasAlternateMaterial() { 609 if (this.alternateMaterial == null) 610 return false; 611 for (CodeableConcept item : this.alternateMaterial) 612 if (!item.isEmpty()) 613 return true; 614 return false; 615 } 616 617 public CodeableConcept addAlternateMaterial() { // 3 618 CodeableConcept t = new CodeableConcept(); 619 if (this.alternateMaterial == null) 620 this.alternateMaterial = new ArrayList<CodeableConcept>(); 621 this.alternateMaterial.add(t); 622 return t; 623 } 624 625 public MedicinalProductPackagedPackageItemComponent addAlternateMaterial(CodeableConcept t) { // 3 626 if (t == null) 627 return this; 628 if (this.alternateMaterial == null) 629 this.alternateMaterial = new ArrayList<CodeableConcept>(); 630 this.alternateMaterial.add(t); 631 return this; 632 } 633 634 /** 635 * @return The first repetition of repeating field {@link #alternateMaterial}, 636 * creating it if it does not already exist 637 */ 638 public CodeableConcept getAlternateMaterialFirstRep() { 639 if (getAlternateMaterial().isEmpty()) { 640 addAlternateMaterial(); 641 } 642 return getAlternateMaterial().get(0); 643 } 644 645 /** 646 * @return {@link #device} (A device accompanying a medicinal product.) 647 */ 648 public List<Reference> getDevice() { 649 if (this.device == null) 650 this.device = new ArrayList<Reference>(); 651 return this.device; 652 } 653 654 /** 655 * @return Returns a reference to <code>this</code> for easy method chaining 656 */ 657 public MedicinalProductPackagedPackageItemComponent setDevice(List<Reference> theDevice) { 658 this.device = theDevice; 659 return this; 660 } 661 662 public boolean hasDevice() { 663 if (this.device == null) 664 return false; 665 for (Reference item : this.device) 666 if (!item.isEmpty()) 667 return true; 668 return false; 669 } 670 671 public Reference addDevice() { // 3 672 Reference t = new Reference(); 673 if (this.device == null) 674 this.device = new ArrayList<Reference>(); 675 this.device.add(t); 676 return t; 677 } 678 679 public MedicinalProductPackagedPackageItemComponent addDevice(Reference t) { // 3 680 if (t == null) 681 return this; 682 if (this.device == null) 683 this.device = new ArrayList<Reference>(); 684 this.device.add(t); 685 return this; 686 } 687 688 /** 689 * @return The first repetition of repeating field {@link #device}, creating it 690 * if it does not already exist 691 */ 692 public Reference getDeviceFirstRep() { 693 if (getDevice().isEmpty()) { 694 addDevice(); 695 } 696 return getDevice().get(0); 697 } 698 699 /** 700 * @deprecated Use Reference#setResource(IBaseResource) instead 701 */ 702 @Deprecated 703 public List<DeviceDefinition> getDeviceTarget() { 704 if (this.deviceTarget == null) 705 this.deviceTarget = new ArrayList<DeviceDefinition>(); 706 return this.deviceTarget; 707 } 708 709 /** 710 * @deprecated Use Reference#setResource(IBaseResource) instead 711 */ 712 @Deprecated 713 public DeviceDefinition addDeviceTarget() { 714 DeviceDefinition r = new DeviceDefinition(); 715 if (this.deviceTarget == null) 716 this.deviceTarget = new ArrayList<DeviceDefinition>(); 717 this.deviceTarget.add(r); 718 return r; 719 } 720 721 /** 722 * @return {@link #manufacturedItem} (The manufactured item as contained in the 723 * packaged medicinal product.) 724 */ 725 public List<Reference> getManufacturedItem() { 726 if (this.manufacturedItem == null) 727 this.manufacturedItem = new ArrayList<Reference>(); 728 return this.manufacturedItem; 729 } 730 731 /** 732 * @return Returns a reference to <code>this</code> for easy method chaining 733 */ 734 public MedicinalProductPackagedPackageItemComponent setManufacturedItem(List<Reference> theManufacturedItem) { 735 this.manufacturedItem = theManufacturedItem; 736 return this; 737 } 738 739 public boolean hasManufacturedItem() { 740 if (this.manufacturedItem == null) 741 return false; 742 for (Reference item : this.manufacturedItem) 743 if (!item.isEmpty()) 744 return true; 745 return false; 746 } 747 748 public Reference addManufacturedItem() { // 3 749 Reference t = new Reference(); 750 if (this.manufacturedItem == null) 751 this.manufacturedItem = new ArrayList<Reference>(); 752 this.manufacturedItem.add(t); 753 return t; 754 } 755 756 public MedicinalProductPackagedPackageItemComponent addManufacturedItem(Reference t) { // 3 757 if (t == null) 758 return this; 759 if (this.manufacturedItem == null) 760 this.manufacturedItem = new ArrayList<Reference>(); 761 this.manufacturedItem.add(t); 762 return this; 763 } 764 765 /** 766 * @return The first repetition of repeating field {@link #manufacturedItem}, 767 * creating it if it does not already exist 768 */ 769 public Reference getManufacturedItemFirstRep() { 770 if (getManufacturedItem().isEmpty()) { 771 addManufacturedItem(); 772 } 773 return getManufacturedItem().get(0); 774 } 775 776 /** 777 * @deprecated Use Reference#setResource(IBaseResource) instead 778 */ 779 @Deprecated 780 public List<MedicinalProductManufactured> getManufacturedItemTarget() { 781 if (this.manufacturedItemTarget == null) 782 this.manufacturedItemTarget = new ArrayList<MedicinalProductManufactured>(); 783 return this.manufacturedItemTarget; 784 } 785 786 /** 787 * @deprecated Use Reference#setResource(IBaseResource) instead 788 */ 789 @Deprecated 790 public MedicinalProductManufactured addManufacturedItemTarget() { 791 MedicinalProductManufactured r = new MedicinalProductManufactured(); 792 if (this.manufacturedItemTarget == null) 793 this.manufacturedItemTarget = new ArrayList<MedicinalProductManufactured>(); 794 this.manufacturedItemTarget.add(r); 795 return r; 796 } 797 798 /** 799 * @return {@link #packageItem} (Allows containers within containers.) 800 */ 801 public List<MedicinalProductPackagedPackageItemComponent> getPackageItem() { 802 if (this.packageItem == null) 803 this.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 804 return this.packageItem; 805 } 806 807 /** 808 * @return Returns a reference to <code>this</code> for easy method chaining 809 */ 810 public MedicinalProductPackagedPackageItemComponent setPackageItem( 811 List<MedicinalProductPackagedPackageItemComponent> thePackageItem) { 812 this.packageItem = thePackageItem; 813 return this; 814 } 815 816 public boolean hasPackageItem() { 817 if (this.packageItem == null) 818 return false; 819 for (MedicinalProductPackagedPackageItemComponent item : this.packageItem) 820 if (!item.isEmpty()) 821 return true; 822 return false; 823 } 824 825 public MedicinalProductPackagedPackageItemComponent addPackageItem() { // 3 826 MedicinalProductPackagedPackageItemComponent t = new MedicinalProductPackagedPackageItemComponent(); 827 if (this.packageItem == null) 828 this.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 829 this.packageItem.add(t); 830 return t; 831 } 832 833 public MedicinalProductPackagedPackageItemComponent addPackageItem(MedicinalProductPackagedPackageItemComponent t) { // 3 834 if (t == null) 835 return this; 836 if (this.packageItem == null) 837 this.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 838 this.packageItem.add(t); 839 return this; 840 } 841 842 /** 843 * @return The first repetition of repeating field {@link #packageItem}, 844 * creating it if it does not already exist 845 */ 846 public MedicinalProductPackagedPackageItemComponent getPackageItemFirstRep() { 847 if (getPackageItem().isEmpty()) { 848 addPackageItem(); 849 } 850 return getPackageItem().get(0); 851 } 852 853 /** 854 * @return {@link #physicalCharacteristics} (Dimensions, color etc.) 855 */ 856 public ProdCharacteristic getPhysicalCharacteristics() { 857 if (this.physicalCharacteristics == null) 858 if (Configuration.errorOnAutoCreate()) 859 throw new Error( 860 "Attempt to auto-create MedicinalProductPackagedPackageItemComponent.physicalCharacteristics"); 861 else if (Configuration.doAutoCreate()) 862 this.physicalCharacteristics = new ProdCharacteristic(); // cc 863 return this.physicalCharacteristics; 864 } 865 866 public boolean hasPhysicalCharacteristics() { 867 return this.physicalCharacteristics != null && !this.physicalCharacteristics.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #physicalCharacteristics} (Dimensions, color etc.) 872 */ 873 public MedicinalProductPackagedPackageItemComponent setPhysicalCharacteristics(ProdCharacteristic value) { 874 this.physicalCharacteristics = value; 875 return this; 876 } 877 878 /** 879 * @return {@link #otherCharacteristics} (Other codeable characteristics.) 880 */ 881 public List<CodeableConcept> getOtherCharacteristics() { 882 if (this.otherCharacteristics == null) 883 this.otherCharacteristics = new ArrayList<CodeableConcept>(); 884 return this.otherCharacteristics; 885 } 886 887 /** 888 * @return Returns a reference to <code>this</code> for easy method chaining 889 */ 890 public MedicinalProductPackagedPackageItemComponent setOtherCharacteristics( 891 List<CodeableConcept> theOtherCharacteristics) { 892 this.otherCharacteristics = theOtherCharacteristics; 893 return this; 894 } 895 896 public boolean hasOtherCharacteristics() { 897 if (this.otherCharacteristics == null) 898 return false; 899 for (CodeableConcept item : this.otherCharacteristics) 900 if (!item.isEmpty()) 901 return true; 902 return false; 903 } 904 905 public CodeableConcept addOtherCharacteristics() { // 3 906 CodeableConcept t = new CodeableConcept(); 907 if (this.otherCharacteristics == null) 908 this.otherCharacteristics = new ArrayList<CodeableConcept>(); 909 this.otherCharacteristics.add(t); 910 return t; 911 } 912 913 public MedicinalProductPackagedPackageItemComponent addOtherCharacteristics(CodeableConcept t) { // 3 914 if (t == null) 915 return this; 916 if (this.otherCharacteristics == null) 917 this.otherCharacteristics = new ArrayList<CodeableConcept>(); 918 this.otherCharacteristics.add(t); 919 return this; 920 } 921 922 /** 923 * @return The first repetition of repeating field 924 * {@link #otherCharacteristics}, creating it if it does not already 925 * exist 926 */ 927 public CodeableConcept getOtherCharacteristicsFirstRep() { 928 if (getOtherCharacteristics().isEmpty()) { 929 addOtherCharacteristics(); 930 } 931 return getOtherCharacteristics().get(0); 932 } 933 934 /** 935 * @return {@link #shelfLifeStorage} (Shelf Life and storage information.) 936 */ 937 public List<ProductShelfLife> getShelfLifeStorage() { 938 if (this.shelfLifeStorage == null) 939 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 940 return this.shelfLifeStorage; 941 } 942 943 /** 944 * @return Returns a reference to <code>this</code> for easy method chaining 945 */ 946 public MedicinalProductPackagedPackageItemComponent setShelfLifeStorage( 947 List<ProductShelfLife> theShelfLifeStorage) { 948 this.shelfLifeStorage = theShelfLifeStorage; 949 return this; 950 } 951 952 public boolean hasShelfLifeStorage() { 953 if (this.shelfLifeStorage == null) 954 return false; 955 for (ProductShelfLife item : this.shelfLifeStorage) 956 if (!item.isEmpty()) 957 return true; 958 return false; 959 } 960 961 public ProductShelfLife addShelfLifeStorage() { // 3 962 ProductShelfLife t = new ProductShelfLife(); 963 if (this.shelfLifeStorage == null) 964 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 965 this.shelfLifeStorage.add(t); 966 return t; 967 } 968 969 public MedicinalProductPackagedPackageItemComponent addShelfLifeStorage(ProductShelfLife t) { // 3 970 if (t == null) 971 return this; 972 if (this.shelfLifeStorage == null) 973 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 974 this.shelfLifeStorage.add(t); 975 return this; 976 } 977 978 /** 979 * @return The first repetition of repeating field {@link #shelfLifeStorage}, 980 * creating it if it does not already exist 981 */ 982 public ProductShelfLife getShelfLifeStorageFirstRep() { 983 if (getShelfLifeStorage().isEmpty()) { 984 addShelfLifeStorage(); 985 } 986 return getShelfLifeStorage().get(0); 987 } 988 989 /** 990 * @return {@link #manufacturer} (Manufacturer of this Package Item.) 991 */ 992 public List<Reference> getManufacturer() { 993 if (this.manufacturer == null) 994 this.manufacturer = new ArrayList<Reference>(); 995 return this.manufacturer; 996 } 997 998 /** 999 * @return Returns a reference to <code>this</code> for easy method chaining 1000 */ 1001 public MedicinalProductPackagedPackageItemComponent setManufacturer(List<Reference> theManufacturer) { 1002 this.manufacturer = theManufacturer; 1003 return this; 1004 } 1005 1006 public boolean hasManufacturer() { 1007 if (this.manufacturer == null) 1008 return false; 1009 for (Reference item : this.manufacturer) 1010 if (!item.isEmpty()) 1011 return true; 1012 return false; 1013 } 1014 1015 public Reference addManufacturer() { // 3 1016 Reference t = new Reference(); 1017 if (this.manufacturer == null) 1018 this.manufacturer = new ArrayList<Reference>(); 1019 this.manufacturer.add(t); 1020 return t; 1021 } 1022 1023 public MedicinalProductPackagedPackageItemComponent addManufacturer(Reference t) { // 3 1024 if (t == null) 1025 return this; 1026 if (this.manufacturer == null) 1027 this.manufacturer = new ArrayList<Reference>(); 1028 this.manufacturer.add(t); 1029 return this; 1030 } 1031 1032 /** 1033 * @return The first repetition of repeating field {@link #manufacturer}, 1034 * creating it if it does not already exist 1035 */ 1036 public Reference getManufacturerFirstRep() { 1037 if (getManufacturer().isEmpty()) { 1038 addManufacturer(); 1039 } 1040 return getManufacturer().get(0); 1041 } 1042 1043 /** 1044 * @deprecated Use Reference#setResource(IBaseResource) instead 1045 */ 1046 @Deprecated 1047 public List<Organization> getManufacturerTarget() { 1048 if (this.manufacturerTarget == null) 1049 this.manufacturerTarget = new ArrayList<Organization>(); 1050 return this.manufacturerTarget; 1051 } 1052 1053 /** 1054 * @deprecated Use Reference#setResource(IBaseResource) instead 1055 */ 1056 @Deprecated 1057 public Organization addManufacturerTarget() { 1058 Organization r = new Organization(); 1059 if (this.manufacturerTarget == null) 1060 this.manufacturerTarget = new ArrayList<Organization>(); 1061 this.manufacturerTarget.add(r); 1062 return r; 1063 } 1064 1065 protected void listChildren(List<Property> children) { 1066 super.listChildren(children); 1067 children.add(new Property("identifier", "Identifier", "Including possibly Data Carrier Identifier.", 0, 1068 java.lang.Integer.MAX_VALUE, identifier)); 1069 children.add( 1070 new Property("type", "CodeableConcept", "The physical type of the container of the medicine.", 0, 1, type)); 1071 children.add(new Property("quantity", "Quantity", 1072 "The quantity of this package in the medicinal product, at the current level of packaging. The outermost is always 1.", 1073 0, 1, quantity)); 1074 children.add(new Property("material", "CodeableConcept", "Material type of the package item.", 0, 1075 java.lang.Integer.MAX_VALUE, material)); 1076 children.add(new Property("alternateMaterial", "CodeableConcept", 1077 "A possible alternate material for the packaging.", 0, java.lang.Integer.MAX_VALUE, alternateMaterial)); 1078 children.add(new Property("device", "Reference(DeviceDefinition)", "A device accompanying a medicinal product.", 1079 0, java.lang.Integer.MAX_VALUE, device)); 1080 children.add(new Property("manufacturedItem", "Reference(MedicinalProductManufactured)", 1081 "The manufactured item as contained in the packaged medicinal product.", 0, java.lang.Integer.MAX_VALUE, 1082 manufacturedItem)); 1083 children.add(new Property("packageItem", "@MedicinalProductPackaged.packageItem", 1084 "Allows containers within containers.", 0, java.lang.Integer.MAX_VALUE, packageItem)); 1085 children.add(new Property("physicalCharacteristics", "ProdCharacteristic", "Dimensions, color etc.", 0, 1, 1086 physicalCharacteristics)); 1087 children.add(new Property("otherCharacteristics", "CodeableConcept", "Other codeable characteristics.", 0, 1088 java.lang.Integer.MAX_VALUE, otherCharacteristics)); 1089 children.add(new Property("shelfLifeStorage", "ProductShelfLife", "Shelf Life and storage information.", 0, 1090 java.lang.Integer.MAX_VALUE, shelfLifeStorage)); 1091 children.add(new Property("manufacturer", "Reference(Organization)", "Manufacturer of this Package Item.", 0, 1092 java.lang.Integer.MAX_VALUE, manufacturer)); 1093 } 1094 1095 @Override 1096 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1097 switch (_hash) { 1098 case -1618432855: 1099 /* identifier */ return new Property("identifier", "Identifier", "Including possibly Data Carrier Identifier.", 1100 0, java.lang.Integer.MAX_VALUE, identifier); 1101 case 3575610: 1102 /* type */ return new Property("type", "CodeableConcept", "The physical type of the container of the medicine.", 1103 0, 1, type); 1104 case -1285004149: 1105 /* quantity */ return new Property("quantity", "Quantity", 1106 "The quantity of this package in the medicinal product, at the current level of packaging. The outermost is always 1.", 1107 0, 1, quantity); 1108 case 299066663: 1109 /* material */ return new Property("material", "CodeableConcept", "Material type of the package item.", 0, 1110 java.lang.Integer.MAX_VALUE, material); 1111 case -1021448255: 1112 /* alternateMaterial */ return new Property("alternateMaterial", "CodeableConcept", 1113 "A possible alternate material for the packaging.", 0, java.lang.Integer.MAX_VALUE, alternateMaterial); 1114 case -1335157162: 1115 /* device */ return new Property("device", "Reference(DeviceDefinition)", 1116 "A device accompanying a medicinal product.", 0, java.lang.Integer.MAX_VALUE, device); 1117 case 62093686: 1118 /* manufacturedItem */ return new Property("manufacturedItem", "Reference(MedicinalProductManufactured)", 1119 "The manufactured item as contained in the packaged medicinal product.", 0, java.lang.Integer.MAX_VALUE, 1120 manufacturedItem); 1121 case 908628089: 1122 /* packageItem */ return new Property("packageItem", "@MedicinalProductPackaged.packageItem", 1123 "Allows containers within containers.", 0, java.lang.Integer.MAX_VALUE, packageItem); 1124 case -1599676319: 1125 /* physicalCharacteristics */ return new Property("physicalCharacteristics", "ProdCharacteristic", 1126 "Dimensions, color etc.", 0, 1, physicalCharacteristics); 1127 case 722135304: 1128 /* otherCharacteristics */ return new Property("otherCharacteristics", "CodeableConcept", 1129 "Other codeable characteristics.", 0, java.lang.Integer.MAX_VALUE, otherCharacteristics); 1130 case 172049237: 1131 /* shelfLifeStorage */ return new Property("shelfLifeStorage", "ProductShelfLife", 1132 "Shelf Life and storage information.", 0, java.lang.Integer.MAX_VALUE, shelfLifeStorage); 1133 case -1969347631: 1134 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 1135 "Manufacturer of this Package Item.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 1136 default: 1137 return super.getNamedProperty(_hash, _name, _checkValid); 1138 } 1139 1140 } 1141 1142 @Override 1143 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1144 switch (hash) { 1145 case -1618432855: 1146 /* identifier */ return this.identifier == null ? new Base[0] 1147 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1148 case 3575610: 1149 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1150 case -1285004149: 1151 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 1152 case 299066663: 1153 /* material */ return this.material == null ? new Base[0] 1154 : this.material.toArray(new Base[this.material.size()]); // CodeableConcept 1155 case -1021448255: 1156 /* alternateMaterial */ return this.alternateMaterial == null ? new Base[0] 1157 : this.alternateMaterial.toArray(new Base[this.alternateMaterial.size()]); // CodeableConcept 1158 case -1335157162: 1159 /* device */ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 1160 case 62093686: 1161 /* manufacturedItem */ return this.manufacturedItem == null ? new Base[0] 1162 : this.manufacturedItem.toArray(new Base[this.manufacturedItem.size()]); // Reference 1163 case 908628089: 1164 /* packageItem */ return this.packageItem == null ? new Base[0] 1165 : this.packageItem.toArray(new Base[this.packageItem.size()]); // MedicinalProductPackagedPackageItemComponent 1166 case -1599676319: 1167 /* physicalCharacteristics */ return this.physicalCharacteristics == null ? new Base[0] 1168 : new Base[] { this.physicalCharacteristics }; // ProdCharacteristic 1169 case 722135304: 1170 /* otherCharacteristics */ return this.otherCharacteristics == null ? new Base[0] 1171 : this.otherCharacteristics.toArray(new Base[this.otherCharacteristics.size()]); // CodeableConcept 1172 case 172049237: 1173 /* shelfLifeStorage */ return this.shelfLifeStorage == null ? new Base[0] 1174 : this.shelfLifeStorage.toArray(new Base[this.shelfLifeStorage.size()]); // ProductShelfLife 1175 case -1969347631: 1176 /* manufacturer */ return this.manufacturer == null ? new Base[0] 1177 : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 1178 default: 1179 return super.getProperty(hash, name, checkValid); 1180 } 1181 1182 } 1183 1184 @Override 1185 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1186 switch (hash) { 1187 case -1618432855: // identifier 1188 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1189 return value; 1190 case 3575610: // type 1191 this.type = castToCodeableConcept(value); // CodeableConcept 1192 return value; 1193 case -1285004149: // quantity 1194 this.quantity = castToQuantity(value); // Quantity 1195 return value; 1196 case 299066663: // material 1197 this.getMaterial().add(castToCodeableConcept(value)); // CodeableConcept 1198 return value; 1199 case -1021448255: // alternateMaterial 1200 this.getAlternateMaterial().add(castToCodeableConcept(value)); // CodeableConcept 1201 return value; 1202 case -1335157162: // device 1203 this.getDevice().add(castToReference(value)); // Reference 1204 return value; 1205 case 62093686: // manufacturedItem 1206 this.getManufacturedItem().add(castToReference(value)); // Reference 1207 return value; 1208 case 908628089: // packageItem 1209 this.getPackageItem().add((MedicinalProductPackagedPackageItemComponent) value); // MedicinalProductPackagedPackageItemComponent 1210 return value; 1211 case -1599676319: // physicalCharacteristics 1212 this.physicalCharacteristics = castToProdCharacteristic(value); // ProdCharacteristic 1213 return value; 1214 case 722135304: // otherCharacteristics 1215 this.getOtherCharacteristics().add(castToCodeableConcept(value)); // CodeableConcept 1216 return value; 1217 case 172049237: // shelfLifeStorage 1218 this.getShelfLifeStorage().add(castToProductShelfLife(value)); // ProductShelfLife 1219 return value; 1220 case -1969347631: // manufacturer 1221 this.getManufacturer().add(castToReference(value)); // Reference 1222 return value; 1223 default: 1224 return super.setProperty(hash, name, value); 1225 } 1226 1227 } 1228 1229 @Override 1230 public Base setProperty(String name, Base value) throws FHIRException { 1231 if (name.equals("identifier")) { 1232 this.getIdentifier().add(castToIdentifier(value)); 1233 } else if (name.equals("type")) { 1234 this.type = castToCodeableConcept(value); // CodeableConcept 1235 } else if (name.equals("quantity")) { 1236 this.quantity = castToQuantity(value); // Quantity 1237 } else if (name.equals("material")) { 1238 this.getMaterial().add(castToCodeableConcept(value)); 1239 } else if (name.equals("alternateMaterial")) { 1240 this.getAlternateMaterial().add(castToCodeableConcept(value)); 1241 } else if (name.equals("device")) { 1242 this.getDevice().add(castToReference(value)); 1243 } else if (name.equals("manufacturedItem")) { 1244 this.getManufacturedItem().add(castToReference(value)); 1245 } else if (name.equals("packageItem")) { 1246 this.getPackageItem().add((MedicinalProductPackagedPackageItemComponent) value); 1247 } else if (name.equals("physicalCharacteristics")) { 1248 this.physicalCharacteristics = castToProdCharacteristic(value); // ProdCharacteristic 1249 } else if (name.equals("otherCharacteristics")) { 1250 this.getOtherCharacteristics().add(castToCodeableConcept(value)); 1251 } else if (name.equals("shelfLifeStorage")) { 1252 this.getShelfLifeStorage().add(castToProductShelfLife(value)); 1253 } else if (name.equals("manufacturer")) { 1254 this.getManufacturer().add(castToReference(value)); 1255 } else 1256 return super.setProperty(name, value); 1257 return value; 1258 } 1259 1260 @Override 1261 public void removeChild(String name, Base value) throws FHIRException { 1262 if (name.equals("identifier")) { 1263 this.getIdentifier().remove(castToIdentifier(value)); 1264 } else if (name.equals("type")) { 1265 this.type = null; 1266 } else if (name.equals("quantity")) { 1267 this.quantity = null; 1268 } else if (name.equals("material")) { 1269 this.getMaterial().remove(castToCodeableConcept(value)); 1270 } else if (name.equals("alternateMaterial")) { 1271 this.getAlternateMaterial().remove(castToCodeableConcept(value)); 1272 } else if (name.equals("device")) { 1273 this.getDevice().remove(castToReference(value)); 1274 } else if (name.equals("manufacturedItem")) { 1275 this.getManufacturedItem().remove(castToReference(value)); 1276 } else if (name.equals("packageItem")) { 1277 this.getPackageItem().remove((MedicinalProductPackagedPackageItemComponent) value); 1278 } else if (name.equals("physicalCharacteristics")) { 1279 this.physicalCharacteristics = null; 1280 } else if (name.equals("otherCharacteristics")) { 1281 this.getOtherCharacteristics().remove(castToCodeableConcept(value)); 1282 } else if (name.equals("shelfLifeStorage")) { 1283 this.getShelfLifeStorage().remove(castToProductShelfLife(value)); 1284 } else if (name.equals("manufacturer")) { 1285 this.getManufacturer().remove(castToReference(value)); 1286 } else 1287 super.removeChild(name, value); 1288 1289 } 1290 1291 @Override 1292 public Base makeProperty(int hash, String name) throws FHIRException { 1293 switch (hash) { 1294 case -1618432855: 1295 return addIdentifier(); 1296 case 3575610: 1297 return getType(); 1298 case -1285004149: 1299 return getQuantity(); 1300 case 299066663: 1301 return addMaterial(); 1302 case -1021448255: 1303 return addAlternateMaterial(); 1304 case -1335157162: 1305 return addDevice(); 1306 case 62093686: 1307 return addManufacturedItem(); 1308 case 908628089: 1309 return addPackageItem(); 1310 case -1599676319: 1311 return getPhysicalCharacteristics(); 1312 case 722135304: 1313 return addOtherCharacteristics(); 1314 case 172049237: 1315 return addShelfLifeStorage(); 1316 case -1969347631: 1317 return addManufacturer(); 1318 default: 1319 return super.makeProperty(hash, name); 1320 } 1321 1322 } 1323 1324 @Override 1325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1326 switch (hash) { 1327 case -1618432855: 1328 /* identifier */ return new String[] { "Identifier" }; 1329 case 3575610: 1330 /* type */ return new String[] { "CodeableConcept" }; 1331 case -1285004149: 1332 /* quantity */ return new String[] { "Quantity" }; 1333 case 299066663: 1334 /* material */ return new String[] { "CodeableConcept" }; 1335 case -1021448255: 1336 /* alternateMaterial */ return new String[] { "CodeableConcept" }; 1337 case -1335157162: 1338 /* device */ return new String[] { "Reference" }; 1339 case 62093686: 1340 /* manufacturedItem */ return new String[] { "Reference" }; 1341 case 908628089: 1342 /* packageItem */ return new String[] { "@MedicinalProductPackaged.packageItem" }; 1343 case -1599676319: 1344 /* physicalCharacteristics */ return new String[] { "ProdCharacteristic" }; 1345 case 722135304: 1346 /* otherCharacteristics */ return new String[] { "CodeableConcept" }; 1347 case 172049237: 1348 /* shelfLifeStorage */ return new String[] { "ProductShelfLife" }; 1349 case -1969347631: 1350 /* manufacturer */ return new String[] { "Reference" }; 1351 default: 1352 return super.getTypesForProperty(hash, name); 1353 } 1354 1355 } 1356 1357 @Override 1358 public Base addChild(String name) throws FHIRException { 1359 if (name.equals("identifier")) { 1360 return addIdentifier(); 1361 } else if (name.equals("type")) { 1362 this.type = new CodeableConcept(); 1363 return this.type; 1364 } else if (name.equals("quantity")) { 1365 this.quantity = new Quantity(); 1366 return this.quantity; 1367 } else if (name.equals("material")) { 1368 return addMaterial(); 1369 } else if (name.equals("alternateMaterial")) { 1370 return addAlternateMaterial(); 1371 } else if (name.equals("device")) { 1372 return addDevice(); 1373 } else if (name.equals("manufacturedItem")) { 1374 return addManufacturedItem(); 1375 } else if (name.equals("packageItem")) { 1376 return addPackageItem(); 1377 } else if (name.equals("physicalCharacteristics")) { 1378 this.physicalCharacteristics = new ProdCharacteristic(); 1379 return this.physicalCharacteristics; 1380 } else if (name.equals("otherCharacteristics")) { 1381 return addOtherCharacteristics(); 1382 } else if (name.equals("shelfLifeStorage")) { 1383 return addShelfLifeStorage(); 1384 } else if (name.equals("manufacturer")) { 1385 return addManufacturer(); 1386 } else 1387 return super.addChild(name); 1388 } 1389 1390 public MedicinalProductPackagedPackageItemComponent copy() { 1391 MedicinalProductPackagedPackageItemComponent dst = new MedicinalProductPackagedPackageItemComponent(); 1392 copyValues(dst); 1393 return dst; 1394 } 1395 1396 public void copyValues(MedicinalProductPackagedPackageItemComponent dst) { 1397 super.copyValues(dst); 1398 if (identifier != null) { 1399 dst.identifier = new ArrayList<Identifier>(); 1400 for (Identifier i : identifier) 1401 dst.identifier.add(i.copy()); 1402 } 1403 ; 1404 dst.type = type == null ? null : type.copy(); 1405 dst.quantity = quantity == null ? null : quantity.copy(); 1406 if (material != null) { 1407 dst.material = new ArrayList<CodeableConcept>(); 1408 for (CodeableConcept i : material) 1409 dst.material.add(i.copy()); 1410 } 1411 ; 1412 if (alternateMaterial != null) { 1413 dst.alternateMaterial = new ArrayList<CodeableConcept>(); 1414 for (CodeableConcept i : alternateMaterial) 1415 dst.alternateMaterial.add(i.copy()); 1416 } 1417 ; 1418 if (device != null) { 1419 dst.device = new ArrayList<Reference>(); 1420 for (Reference i : device) 1421 dst.device.add(i.copy()); 1422 } 1423 ; 1424 if (manufacturedItem != null) { 1425 dst.manufacturedItem = new ArrayList<Reference>(); 1426 for (Reference i : manufacturedItem) 1427 dst.manufacturedItem.add(i.copy()); 1428 } 1429 ; 1430 if (packageItem != null) { 1431 dst.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 1432 for (MedicinalProductPackagedPackageItemComponent i : packageItem) 1433 dst.packageItem.add(i.copy()); 1434 } 1435 ; 1436 dst.physicalCharacteristics = physicalCharacteristics == null ? null : physicalCharacteristics.copy(); 1437 if (otherCharacteristics != null) { 1438 dst.otherCharacteristics = new ArrayList<CodeableConcept>(); 1439 for (CodeableConcept i : otherCharacteristics) 1440 dst.otherCharacteristics.add(i.copy()); 1441 } 1442 ; 1443 if (shelfLifeStorage != null) { 1444 dst.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 1445 for (ProductShelfLife i : shelfLifeStorage) 1446 dst.shelfLifeStorage.add(i.copy()); 1447 } 1448 ; 1449 if (manufacturer != null) { 1450 dst.manufacturer = new ArrayList<Reference>(); 1451 for (Reference i : manufacturer) 1452 dst.manufacturer.add(i.copy()); 1453 } 1454 ; 1455 } 1456 1457 @Override 1458 public boolean equalsDeep(Base other_) { 1459 if (!super.equalsDeep(other_)) 1460 return false; 1461 if (!(other_ instanceof MedicinalProductPackagedPackageItemComponent)) 1462 return false; 1463 MedicinalProductPackagedPackageItemComponent o = (MedicinalProductPackagedPackageItemComponent) other_; 1464 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 1465 && compareDeep(quantity, o.quantity, true) && compareDeep(material, o.material, true) 1466 && compareDeep(alternateMaterial, o.alternateMaterial, true) && compareDeep(device, o.device, true) 1467 && compareDeep(manufacturedItem, o.manufacturedItem, true) && compareDeep(packageItem, o.packageItem, true) 1468 && compareDeep(physicalCharacteristics, o.physicalCharacteristics, true) 1469 && compareDeep(otherCharacteristics, o.otherCharacteristics, true) 1470 && compareDeep(shelfLifeStorage, o.shelfLifeStorage, true) && compareDeep(manufacturer, o.manufacturer, true); 1471 } 1472 1473 @Override 1474 public boolean equalsShallow(Base other_) { 1475 if (!super.equalsShallow(other_)) 1476 return false; 1477 if (!(other_ instanceof MedicinalProductPackagedPackageItemComponent)) 1478 return false; 1479 MedicinalProductPackagedPackageItemComponent o = (MedicinalProductPackagedPackageItemComponent) other_; 1480 return true; 1481 } 1482 1483 public boolean isEmpty() { 1484 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, quantity, material, 1485 alternateMaterial, device, manufacturedItem, packageItem, physicalCharacteristics, otherCharacteristics, 1486 shelfLifeStorage, manufacturer); 1487 } 1488 1489 public String fhirType() { 1490 return "MedicinalProductPackaged.packageItem"; 1491 1492 } 1493 1494 } 1495 1496 /** 1497 * Unique identifier. 1498 */ 1499 @Child(name = "identifier", type = { 1500 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1501 @Description(shortDefinition = "Unique identifier", formalDefinition = "Unique identifier.") 1502 protected List<Identifier> identifier; 1503 1504 /** 1505 * The product with this is a pack for. 1506 */ 1507 @Child(name = "subject", type = { 1508 MedicinalProduct.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1509 @Description(shortDefinition = "The product with this is a pack for", formalDefinition = "The product with this is a pack for.") 1510 protected List<Reference> subject; 1511 /** 1512 * The actual objects that are the target of the reference (The product with 1513 * this is a pack for.) 1514 */ 1515 protected List<MedicinalProduct> subjectTarget; 1516 1517 /** 1518 * Textual description. 1519 */ 1520 @Child(name = "description", type = { 1521 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1522 @Description(shortDefinition = "Textual description", formalDefinition = "Textual description.") 1523 protected StringType description; 1524 1525 /** 1526 * The legal status of supply of the medicinal product as classified by the 1527 * regulator. 1528 */ 1529 @Child(name = "legalStatusOfSupply", type = { 1530 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1531 @Description(shortDefinition = "The legal status of supply of the medicinal product as classified by the regulator", formalDefinition = "The legal status of supply of the medicinal product as classified by the regulator.") 1532 protected CodeableConcept legalStatusOfSupply; 1533 1534 /** 1535 * Marketing information. 1536 */ 1537 @Child(name = "marketingStatus", type = { 1538 MarketingStatus.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1539 @Description(shortDefinition = "Marketing information", formalDefinition = "Marketing information.") 1540 protected List<MarketingStatus> marketingStatus; 1541 1542 /** 1543 * Manufacturer of this Package Item. 1544 */ 1545 @Child(name = "marketingAuthorization", type = { 1546 MedicinalProductAuthorization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1547 @Description(shortDefinition = "Manufacturer of this Package Item", formalDefinition = "Manufacturer of this Package Item.") 1548 protected Reference marketingAuthorization; 1549 1550 /** 1551 * The actual object that is the target of the reference (Manufacturer of this 1552 * Package Item.) 1553 */ 1554 protected MedicinalProductAuthorization marketingAuthorizationTarget; 1555 1556 /** 1557 * Manufacturer of this Package Item. 1558 */ 1559 @Child(name = "manufacturer", type = { 1560 Organization.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1561 @Description(shortDefinition = "Manufacturer of this Package Item", formalDefinition = "Manufacturer of this Package Item.") 1562 protected List<Reference> manufacturer; 1563 /** 1564 * The actual objects that are the target of the reference (Manufacturer of this 1565 * Package Item.) 1566 */ 1567 protected List<Organization> manufacturerTarget; 1568 1569 /** 1570 * Batch numbering. 1571 */ 1572 @Child(name = "batchIdentifier", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1573 @Description(shortDefinition = "Batch numbering", formalDefinition = "Batch numbering.") 1574 protected List<MedicinalProductPackagedBatchIdentifierComponent> batchIdentifier; 1575 1576 /** 1577 * A packaging item, as a contained for medicine, possibly with other packaging 1578 * items within. 1579 */ 1580 @Child(name = "packageItem", type = {}, order = 8, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1581 @Description(shortDefinition = "A packaging item, as a contained for medicine, possibly with other packaging items within", formalDefinition = "A packaging item, as a contained for medicine, possibly with other packaging items within.") 1582 protected List<MedicinalProductPackagedPackageItemComponent> packageItem; 1583 1584 private static final long serialVersionUID = -1530863773L; 1585 1586 /** 1587 * Constructor 1588 */ 1589 public MedicinalProductPackaged() { 1590 super(); 1591 } 1592 1593 /** 1594 * @return {@link #identifier} (Unique identifier.) 1595 */ 1596 public List<Identifier> getIdentifier() { 1597 if (this.identifier == null) 1598 this.identifier = new ArrayList<Identifier>(); 1599 return this.identifier; 1600 } 1601 1602 /** 1603 * @return Returns a reference to <code>this</code> for easy method chaining 1604 */ 1605 public MedicinalProductPackaged setIdentifier(List<Identifier> theIdentifier) { 1606 this.identifier = theIdentifier; 1607 return this; 1608 } 1609 1610 public boolean hasIdentifier() { 1611 if (this.identifier == null) 1612 return false; 1613 for (Identifier item : this.identifier) 1614 if (!item.isEmpty()) 1615 return true; 1616 return false; 1617 } 1618 1619 public Identifier addIdentifier() { // 3 1620 Identifier t = new Identifier(); 1621 if (this.identifier == null) 1622 this.identifier = new ArrayList<Identifier>(); 1623 this.identifier.add(t); 1624 return t; 1625 } 1626 1627 public MedicinalProductPackaged addIdentifier(Identifier t) { // 3 1628 if (t == null) 1629 return this; 1630 if (this.identifier == null) 1631 this.identifier = new ArrayList<Identifier>(); 1632 this.identifier.add(t); 1633 return this; 1634 } 1635 1636 /** 1637 * @return The first repetition of repeating field {@link #identifier}, creating 1638 * it if it does not already exist 1639 */ 1640 public Identifier getIdentifierFirstRep() { 1641 if (getIdentifier().isEmpty()) { 1642 addIdentifier(); 1643 } 1644 return getIdentifier().get(0); 1645 } 1646 1647 /** 1648 * @return {@link #subject} (The product with this is a pack for.) 1649 */ 1650 public List<Reference> getSubject() { 1651 if (this.subject == null) 1652 this.subject = new ArrayList<Reference>(); 1653 return this.subject; 1654 } 1655 1656 /** 1657 * @return Returns a reference to <code>this</code> for easy method chaining 1658 */ 1659 public MedicinalProductPackaged setSubject(List<Reference> theSubject) { 1660 this.subject = theSubject; 1661 return this; 1662 } 1663 1664 public boolean hasSubject() { 1665 if (this.subject == null) 1666 return false; 1667 for (Reference item : this.subject) 1668 if (!item.isEmpty()) 1669 return true; 1670 return false; 1671 } 1672 1673 public Reference addSubject() { // 3 1674 Reference t = new Reference(); 1675 if (this.subject == null) 1676 this.subject = new ArrayList<Reference>(); 1677 this.subject.add(t); 1678 return t; 1679 } 1680 1681 public MedicinalProductPackaged addSubject(Reference t) { // 3 1682 if (t == null) 1683 return this; 1684 if (this.subject == null) 1685 this.subject = new ArrayList<Reference>(); 1686 this.subject.add(t); 1687 return this; 1688 } 1689 1690 /** 1691 * @return The first repetition of repeating field {@link #subject}, creating it 1692 * if it does not already exist 1693 */ 1694 public Reference getSubjectFirstRep() { 1695 if (getSubject().isEmpty()) { 1696 addSubject(); 1697 } 1698 return getSubject().get(0); 1699 } 1700 1701 /** 1702 * @deprecated Use Reference#setResource(IBaseResource) instead 1703 */ 1704 @Deprecated 1705 public List<MedicinalProduct> getSubjectTarget() { 1706 if (this.subjectTarget == null) 1707 this.subjectTarget = new ArrayList<MedicinalProduct>(); 1708 return this.subjectTarget; 1709 } 1710 1711 /** 1712 * @deprecated Use Reference#setResource(IBaseResource) instead 1713 */ 1714 @Deprecated 1715 public MedicinalProduct addSubjectTarget() { 1716 MedicinalProduct r = new MedicinalProduct(); 1717 if (this.subjectTarget == null) 1718 this.subjectTarget = new ArrayList<MedicinalProduct>(); 1719 this.subjectTarget.add(r); 1720 return r; 1721 } 1722 1723 /** 1724 * @return {@link #description} (Textual description.). This is the underlying 1725 * object with id, value and extensions. The accessor "getDescription" 1726 * gives direct access to the value 1727 */ 1728 public StringType getDescriptionElement() { 1729 if (this.description == null) 1730 if (Configuration.errorOnAutoCreate()) 1731 throw new Error("Attempt to auto-create MedicinalProductPackaged.description"); 1732 else if (Configuration.doAutoCreate()) 1733 this.description = new StringType(); // bb 1734 return this.description; 1735 } 1736 1737 public boolean hasDescriptionElement() { 1738 return this.description != null && !this.description.isEmpty(); 1739 } 1740 1741 public boolean hasDescription() { 1742 return this.description != null && !this.description.isEmpty(); 1743 } 1744 1745 /** 1746 * @param value {@link #description} (Textual description.). This is the 1747 * underlying object with id, value and extensions. The accessor 1748 * "getDescription" gives direct access to the value 1749 */ 1750 public MedicinalProductPackaged setDescriptionElement(StringType value) { 1751 this.description = value; 1752 return this; 1753 } 1754 1755 /** 1756 * @return Textual description. 1757 */ 1758 public String getDescription() { 1759 return this.description == null ? null : this.description.getValue(); 1760 } 1761 1762 /** 1763 * @param value Textual description. 1764 */ 1765 public MedicinalProductPackaged setDescription(String value) { 1766 if (Utilities.noString(value)) 1767 this.description = null; 1768 else { 1769 if (this.description == null) 1770 this.description = new StringType(); 1771 this.description.setValue(value); 1772 } 1773 return this; 1774 } 1775 1776 /** 1777 * @return {@link #legalStatusOfSupply} (The legal status of supply of the 1778 * medicinal product as classified by the regulator.) 1779 */ 1780 public CodeableConcept getLegalStatusOfSupply() { 1781 if (this.legalStatusOfSupply == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create MedicinalProductPackaged.legalStatusOfSupply"); 1784 else if (Configuration.doAutoCreate()) 1785 this.legalStatusOfSupply = new CodeableConcept(); // cc 1786 return this.legalStatusOfSupply; 1787 } 1788 1789 public boolean hasLegalStatusOfSupply() { 1790 return this.legalStatusOfSupply != null && !this.legalStatusOfSupply.isEmpty(); 1791 } 1792 1793 /** 1794 * @param value {@link #legalStatusOfSupply} (The legal status of supply of the 1795 * medicinal product as classified by the regulator.) 1796 */ 1797 public MedicinalProductPackaged setLegalStatusOfSupply(CodeableConcept value) { 1798 this.legalStatusOfSupply = value; 1799 return this; 1800 } 1801 1802 /** 1803 * @return {@link #marketingStatus} (Marketing information.) 1804 */ 1805 public List<MarketingStatus> getMarketingStatus() { 1806 if (this.marketingStatus == null) 1807 this.marketingStatus = new ArrayList<MarketingStatus>(); 1808 return this.marketingStatus; 1809 } 1810 1811 /** 1812 * @return Returns a reference to <code>this</code> for easy method chaining 1813 */ 1814 public MedicinalProductPackaged setMarketingStatus(List<MarketingStatus> theMarketingStatus) { 1815 this.marketingStatus = theMarketingStatus; 1816 return this; 1817 } 1818 1819 public boolean hasMarketingStatus() { 1820 if (this.marketingStatus == null) 1821 return false; 1822 for (MarketingStatus item : this.marketingStatus) 1823 if (!item.isEmpty()) 1824 return true; 1825 return false; 1826 } 1827 1828 public MarketingStatus addMarketingStatus() { // 3 1829 MarketingStatus t = new MarketingStatus(); 1830 if (this.marketingStatus == null) 1831 this.marketingStatus = new ArrayList<MarketingStatus>(); 1832 this.marketingStatus.add(t); 1833 return t; 1834 } 1835 1836 public MedicinalProductPackaged addMarketingStatus(MarketingStatus t) { // 3 1837 if (t == null) 1838 return this; 1839 if (this.marketingStatus == null) 1840 this.marketingStatus = new ArrayList<MarketingStatus>(); 1841 this.marketingStatus.add(t); 1842 return this; 1843 } 1844 1845 /** 1846 * @return The first repetition of repeating field {@link #marketingStatus}, 1847 * creating it if it does not already exist 1848 */ 1849 public MarketingStatus getMarketingStatusFirstRep() { 1850 if (getMarketingStatus().isEmpty()) { 1851 addMarketingStatus(); 1852 } 1853 return getMarketingStatus().get(0); 1854 } 1855 1856 /** 1857 * @return {@link #marketingAuthorization} (Manufacturer of this Package Item.) 1858 */ 1859 public Reference getMarketingAuthorization() { 1860 if (this.marketingAuthorization == null) 1861 if (Configuration.errorOnAutoCreate()) 1862 throw new Error("Attempt to auto-create MedicinalProductPackaged.marketingAuthorization"); 1863 else if (Configuration.doAutoCreate()) 1864 this.marketingAuthorization = new Reference(); // cc 1865 return this.marketingAuthorization; 1866 } 1867 1868 public boolean hasMarketingAuthorization() { 1869 return this.marketingAuthorization != null && !this.marketingAuthorization.isEmpty(); 1870 } 1871 1872 /** 1873 * @param value {@link #marketingAuthorization} (Manufacturer of this Package 1874 * Item.) 1875 */ 1876 public MedicinalProductPackaged setMarketingAuthorization(Reference value) { 1877 this.marketingAuthorization = value; 1878 return this; 1879 } 1880 1881 /** 1882 * @return {@link #marketingAuthorization} The actual object that is the target 1883 * of the reference. The reference library doesn't populate this, but 1884 * you can use it to hold the resource if you resolve it. (Manufacturer 1885 * of this Package Item.) 1886 */ 1887 public MedicinalProductAuthorization getMarketingAuthorizationTarget() { 1888 if (this.marketingAuthorizationTarget == null) 1889 if (Configuration.errorOnAutoCreate()) 1890 throw new Error("Attempt to auto-create MedicinalProductPackaged.marketingAuthorization"); 1891 else if (Configuration.doAutoCreate()) 1892 this.marketingAuthorizationTarget = new MedicinalProductAuthorization(); // aa 1893 return this.marketingAuthorizationTarget; 1894 } 1895 1896 /** 1897 * @param value {@link #marketingAuthorization} The actual object that is the 1898 * target of the reference. The reference library doesn't use 1899 * these, but you can use it to hold the resource if you resolve 1900 * it. (Manufacturer of this Package Item.) 1901 */ 1902 public MedicinalProductPackaged setMarketingAuthorizationTarget(MedicinalProductAuthorization value) { 1903 this.marketingAuthorizationTarget = value; 1904 return this; 1905 } 1906 1907 /** 1908 * @return {@link #manufacturer} (Manufacturer of this Package Item.) 1909 */ 1910 public List<Reference> getManufacturer() { 1911 if (this.manufacturer == null) 1912 this.manufacturer = new ArrayList<Reference>(); 1913 return this.manufacturer; 1914 } 1915 1916 /** 1917 * @return Returns a reference to <code>this</code> for easy method chaining 1918 */ 1919 public MedicinalProductPackaged setManufacturer(List<Reference> theManufacturer) { 1920 this.manufacturer = theManufacturer; 1921 return this; 1922 } 1923 1924 public boolean hasManufacturer() { 1925 if (this.manufacturer == null) 1926 return false; 1927 for (Reference item : this.manufacturer) 1928 if (!item.isEmpty()) 1929 return true; 1930 return false; 1931 } 1932 1933 public Reference addManufacturer() { // 3 1934 Reference t = new Reference(); 1935 if (this.manufacturer == null) 1936 this.manufacturer = new ArrayList<Reference>(); 1937 this.manufacturer.add(t); 1938 return t; 1939 } 1940 1941 public MedicinalProductPackaged addManufacturer(Reference t) { // 3 1942 if (t == null) 1943 return this; 1944 if (this.manufacturer == null) 1945 this.manufacturer = new ArrayList<Reference>(); 1946 this.manufacturer.add(t); 1947 return this; 1948 } 1949 1950 /** 1951 * @return The first repetition of repeating field {@link #manufacturer}, 1952 * creating it if it does not already exist 1953 */ 1954 public Reference getManufacturerFirstRep() { 1955 if (getManufacturer().isEmpty()) { 1956 addManufacturer(); 1957 } 1958 return getManufacturer().get(0); 1959 } 1960 1961 /** 1962 * @deprecated Use Reference#setResource(IBaseResource) instead 1963 */ 1964 @Deprecated 1965 public List<Organization> getManufacturerTarget() { 1966 if (this.manufacturerTarget == null) 1967 this.manufacturerTarget = new ArrayList<Organization>(); 1968 return this.manufacturerTarget; 1969 } 1970 1971 /** 1972 * @deprecated Use Reference#setResource(IBaseResource) instead 1973 */ 1974 @Deprecated 1975 public Organization addManufacturerTarget() { 1976 Organization r = new Organization(); 1977 if (this.manufacturerTarget == null) 1978 this.manufacturerTarget = new ArrayList<Organization>(); 1979 this.manufacturerTarget.add(r); 1980 return r; 1981 } 1982 1983 /** 1984 * @return {@link #batchIdentifier} (Batch numbering.) 1985 */ 1986 public List<MedicinalProductPackagedBatchIdentifierComponent> getBatchIdentifier() { 1987 if (this.batchIdentifier == null) 1988 this.batchIdentifier = new ArrayList<MedicinalProductPackagedBatchIdentifierComponent>(); 1989 return this.batchIdentifier; 1990 } 1991 1992 /** 1993 * @return Returns a reference to <code>this</code> for easy method chaining 1994 */ 1995 public MedicinalProductPackaged setBatchIdentifier( 1996 List<MedicinalProductPackagedBatchIdentifierComponent> theBatchIdentifier) { 1997 this.batchIdentifier = theBatchIdentifier; 1998 return this; 1999 } 2000 2001 public boolean hasBatchIdentifier() { 2002 if (this.batchIdentifier == null) 2003 return false; 2004 for (MedicinalProductPackagedBatchIdentifierComponent item : this.batchIdentifier) 2005 if (!item.isEmpty()) 2006 return true; 2007 return false; 2008 } 2009 2010 public MedicinalProductPackagedBatchIdentifierComponent addBatchIdentifier() { // 3 2011 MedicinalProductPackagedBatchIdentifierComponent t = new MedicinalProductPackagedBatchIdentifierComponent(); 2012 if (this.batchIdentifier == null) 2013 this.batchIdentifier = new ArrayList<MedicinalProductPackagedBatchIdentifierComponent>(); 2014 this.batchIdentifier.add(t); 2015 return t; 2016 } 2017 2018 public MedicinalProductPackaged addBatchIdentifier(MedicinalProductPackagedBatchIdentifierComponent t) { // 3 2019 if (t == null) 2020 return this; 2021 if (this.batchIdentifier == null) 2022 this.batchIdentifier = new ArrayList<MedicinalProductPackagedBatchIdentifierComponent>(); 2023 this.batchIdentifier.add(t); 2024 return this; 2025 } 2026 2027 /** 2028 * @return The first repetition of repeating field {@link #batchIdentifier}, 2029 * creating it if it does not already exist 2030 */ 2031 public MedicinalProductPackagedBatchIdentifierComponent getBatchIdentifierFirstRep() { 2032 if (getBatchIdentifier().isEmpty()) { 2033 addBatchIdentifier(); 2034 } 2035 return getBatchIdentifier().get(0); 2036 } 2037 2038 /** 2039 * @return {@link #packageItem} (A packaging item, as a contained for medicine, 2040 * possibly with other packaging items within.) 2041 */ 2042 public List<MedicinalProductPackagedPackageItemComponent> getPackageItem() { 2043 if (this.packageItem == null) 2044 this.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 2045 return this.packageItem; 2046 } 2047 2048 /** 2049 * @return Returns a reference to <code>this</code> for easy method chaining 2050 */ 2051 public MedicinalProductPackaged setPackageItem(List<MedicinalProductPackagedPackageItemComponent> thePackageItem) { 2052 this.packageItem = thePackageItem; 2053 return this; 2054 } 2055 2056 public boolean hasPackageItem() { 2057 if (this.packageItem == null) 2058 return false; 2059 for (MedicinalProductPackagedPackageItemComponent item : this.packageItem) 2060 if (!item.isEmpty()) 2061 return true; 2062 return false; 2063 } 2064 2065 public MedicinalProductPackagedPackageItemComponent addPackageItem() { // 3 2066 MedicinalProductPackagedPackageItemComponent t = new MedicinalProductPackagedPackageItemComponent(); 2067 if (this.packageItem == null) 2068 this.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 2069 this.packageItem.add(t); 2070 return t; 2071 } 2072 2073 public MedicinalProductPackaged addPackageItem(MedicinalProductPackagedPackageItemComponent t) { // 3 2074 if (t == null) 2075 return this; 2076 if (this.packageItem == null) 2077 this.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 2078 this.packageItem.add(t); 2079 return this; 2080 } 2081 2082 /** 2083 * @return The first repetition of repeating field {@link #packageItem}, 2084 * creating it if it does not already exist 2085 */ 2086 public MedicinalProductPackagedPackageItemComponent getPackageItemFirstRep() { 2087 if (getPackageItem().isEmpty()) { 2088 addPackageItem(); 2089 } 2090 return getPackageItem().get(0); 2091 } 2092 2093 protected void listChildren(List<Property> children) { 2094 super.listChildren(children); 2095 children.add( 2096 new Property("identifier", "Identifier", "Unique identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2097 children.add(new Property("subject", "Reference(MedicinalProduct)", "The product with this is a pack for.", 0, 2098 java.lang.Integer.MAX_VALUE, subject)); 2099 children.add(new Property("description", "string", "Textual description.", 0, 1, description)); 2100 children.add(new Property("legalStatusOfSupply", "CodeableConcept", 2101 "The legal status of supply of the medicinal product as classified by the regulator.", 0, 1, 2102 legalStatusOfSupply)); 2103 children.add(new Property("marketingStatus", "MarketingStatus", "Marketing information.", 0, 2104 java.lang.Integer.MAX_VALUE, marketingStatus)); 2105 children.add(new Property("marketingAuthorization", "Reference(MedicinalProductAuthorization)", 2106 "Manufacturer of this Package Item.", 0, 1, marketingAuthorization)); 2107 children.add(new Property("manufacturer", "Reference(Organization)", "Manufacturer of this Package Item.", 0, 2108 java.lang.Integer.MAX_VALUE, manufacturer)); 2109 children 2110 .add(new Property("batchIdentifier", "", "Batch numbering.", 0, java.lang.Integer.MAX_VALUE, batchIdentifier)); 2111 children.add(new Property("packageItem", "", 2112 "A packaging item, as a contained for medicine, possibly with other packaging items within.", 0, 2113 java.lang.Integer.MAX_VALUE, packageItem)); 2114 } 2115 2116 @Override 2117 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2118 switch (_hash) { 2119 case -1618432855: 2120 /* identifier */ return new Property("identifier", "Identifier", "Unique identifier.", 0, 2121 java.lang.Integer.MAX_VALUE, identifier); 2122 case -1867885268: 2123 /* subject */ return new Property("subject", "Reference(MedicinalProduct)", 2124 "The product with this is a pack for.", 0, java.lang.Integer.MAX_VALUE, subject); 2125 case -1724546052: 2126 /* description */ return new Property("description", "string", "Textual description.", 0, 1, description); 2127 case -844874031: 2128 /* legalStatusOfSupply */ return new Property("legalStatusOfSupply", "CodeableConcept", 2129 "The legal status of supply of the medicinal product as classified by the regulator.", 0, 1, 2130 legalStatusOfSupply); 2131 case 70767032: 2132 /* marketingStatus */ return new Property("marketingStatus", "MarketingStatus", "Marketing information.", 0, 2133 java.lang.Integer.MAX_VALUE, marketingStatus); 2134 case 571831283: 2135 /* marketingAuthorization */ return new Property("marketingAuthorization", 2136 "Reference(MedicinalProductAuthorization)", "Manufacturer of this Package Item.", 0, 1, 2137 marketingAuthorization); 2138 case -1969347631: 2139 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 2140 "Manufacturer of this Package Item.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 2141 case -1688395901: 2142 /* batchIdentifier */ return new Property("batchIdentifier", "", "Batch numbering.", 0, 2143 java.lang.Integer.MAX_VALUE, batchIdentifier); 2144 case 908628089: 2145 /* packageItem */ return new Property("packageItem", "", 2146 "A packaging item, as a contained for medicine, possibly with other packaging items within.", 0, 2147 java.lang.Integer.MAX_VALUE, packageItem); 2148 default: 2149 return super.getNamedProperty(_hash, _name, _checkValid); 2150 } 2151 2152 } 2153 2154 @Override 2155 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2156 switch (hash) { 2157 case -1618432855: 2158 /* identifier */ return this.identifier == null ? new Base[0] 2159 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2160 case -1867885268: 2161 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 2162 case -1724546052: 2163 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2164 case -844874031: 2165 /* legalStatusOfSupply */ return this.legalStatusOfSupply == null ? new Base[0] 2166 : new Base[] { this.legalStatusOfSupply }; // CodeableConcept 2167 case 70767032: 2168 /* marketingStatus */ return this.marketingStatus == null ? new Base[0] 2169 : this.marketingStatus.toArray(new Base[this.marketingStatus.size()]); // MarketingStatus 2170 case 571831283: 2171 /* marketingAuthorization */ return this.marketingAuthorization == null ? new Base[0] 2172 : new Base[] { this.marketingAuthorization }; // Reference 2173 case -1969347631: 2174 /* manufacturer */ return this.manufacturer == null ? new Base[0] 2175 : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 2176 case -1688395901: 2177 /* batchIdentifier */ return this.batchIdentifier == null ? new Base[0] 2178 : this.batchIdentifier.toArray(new Base[this.batchIdentifier.size()]); // MedicinalProductPackagedBatchIdentifierComponent 2179 case 908628089: 2180 /* packageItem */ return this.packageItem == null ? new Base[0] 2181 : this.packageItem.toArray(new Base[this.packageItem.size()]); // MedicinalProductPackagedPackageItemComponent 2182 default: 2183 return super.getProperty(hash, name, checkValid); 2184 } 2185 2186 } 2187 2188 @Override 2189 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2190 switch (hash) { 2191 case -1618432855: // identifier 2192 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2193 return value; 2194 case -1867885268: // subject 2195 this.getSubject().add(castToReference(value)); // Reference 2196 return value; 2197 case -1724546052: // description 2198 this.description = castToString(value); // StringType 2199 return value; 2200 case -844874031: // legalStatusOfSupply 2201 this.legalStatusOfSupply = castToCodeableConcept(value); // CodeableConcept 2202 return value; 2203 case 70767032: // marketingStatus 2204 this.getMarketingStatus().add(castToMarketingStatus(value)); // MarketingStatus 2205 return value; 2206 case 571831283: // marketingAuthorization 2207 this.marketingAuthorization = castToReference(value); // Reference 2208 return value; 2209 case -1969347631: // manufacturer 2210 this.getManufacturer().add(castToReference(value)); // Reference 2211 return value; 2212 case -1688395901: // batchIdentifier 2213 this.getBatchIdentifier().add((MedicinalProductPackagedBatchIdentifierComponent) value); // MedicinalProductPackagedBatchIdentifierComponent 2214 return value; 2215 case 908628089: // packageItem 2216 this.getPackageItem().add((MedicinalProductPackagedPackageItemComponent) value); // MedicinalProductPackagedPackageItemComponent 2217 return value; 2218 default: 2219 return super.setProperty(hash, name, value); 2220 } 2221 2222 } 2223 2224 @Override 2225 public Base setProperty(String name, Base value) throws FHIRException { 2226 if (name.equals("identifier")) { 2227 this.getIdentifier().add(castToIdentifier(value)); 2228 } else if (name.equals("subject")) { 2229 this.getSubject().add(castToReference(value)); 2230 } else if (name.equals("description")) { 2231 this.description = castToString(value); // StringType 2232 } else if (name.equals("legalStatusOfSupply")) { 2233 this.legalStatusOfSupply = castToCodeableConcept(value); // CodeableConcept 2234 } else if (name.equals("marketingStatus")) { 2235 this.getMarketingStatus().add(castToMarketingStatus(value)); 2236 } else if (name.equals("marketingAuthorization")) { 2237 this.marketingAuthorization = castToReference(value); // Reference 2238 } else if (name.equals("manufacturer")) { 2239 this.getManufacturer().add(castToReference(value)); 2240 } else if (name.equals("batchIdentifier")) { 2241 this.getBatchIdentifier().add((MedicinalProductPackagedBatchIdentifierComponent) value); 2242 } else if (name.equals("packageItem")) { 2243 this.getPackageItem().add((MedicinalProductPackagedPackageItemComponent) value); 2244 } else 2245 return super.setProperty(name, value); 2246 return value; 2247 } 2248 2249 @Override 2250 public void removeChild(String name, Base value) throws FHIRException { 2251 if (name.equals("identifier")) { 2252 this.getIdentifier().remove(castToIdentifier(value)); 2253 } else if (name.equals("subject")) { 2254 this.getSubject().remove(castToReference(value)); 2255 } else if (name.equals("description")) { 2256 this.description = null; 2257 } else if (name.equals("legalStatusOfSupply")) { 2258 this.legalStatusOfSupply = null; 2259 } else if (name.equals("marketingStatus")) { 2260 this.getMarketingStatus().remove(castToMarketingStatus(value)); 2261 } else if (name.equals("marketingAuthorization")) { 2262 this.marketingAuthorization = null; 2263 } else if (name.equals("manufacturer")) { 2264 this.getManufacturer().remove(castToReference(value)); 2265 } else if (name.equals("batchIdentifier")) { 2266 this.getBatchIdentifier().remove((MedicinalProductPackagedBatchIdentifierComponent) value); 2267 } else if (name.equals("packageItem")) { 2268 this.getPackageItem().remove((MedicinalProductPackagedPackageItemComponent) value); 2269 } else 2270 super.removeChild(name, value); 2271 2272 } 2273 2274 @Override 2275 public Base makeProperty(int hash, String name) throws FHIRException { 2276 switch (hash) { 2277 case -1618432855: 2278 return addIdentifier(); 2279 case -1867885268: 2280 return addSubject(); 2281 case -1724546052: 2282 return getDescriptionElement(); 2283 case -844874031: 2284 return getLegalStatusOfSupply(); 2285 case 70767032: 2286 return addMarketingStatus(); 2287 case 571831283: 2288 return getMarketingAuthorization(); 2289 case -1969347631: 2290 return addManufacturer(); 2291 case -1688395901: 2292 return addBatchIdentifier(); 2293 case 908628089: 2294 return addPackageItem(); 2295 default: 2296 return super.makeProperty(hash, name); 2297 } 2298 2299 } 2300 2301 @Override 2302 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2303 switch (hash) { 2304 case -1618432855: 2305 /* identifier */ return new String[] { "Identifier" }; 2306 case -1867885268: 2307 /* subject */ return new String[] { "Reference" }; 2308 case -1724546052: 2309 /* description */ return new String[] { "string" }; 2310 case -844874031: 2311 /* legalStatusOfSupply */ return new String[] { "CodeableConcept" }; 2312 case 70767032: 2313 /* marketingStatus */ return new String[] { "MarketingStatus" }; 2314 case 571831283: 2315 /* marketingAuthorization */ return new String[] { "Reference" }; 2316 case -1969347631: 2317 /* manufacturer */ return new String[] { "Reference" }; 2318 case -1688395901: 2319 /* batchIdentifier */ return new String[] {}; 2320 case 908628089: 2321 /* packageItem */ return new String[] {}; 2322 default: 2323 return super.getTypesForProperty(hash, name); 2324 } 2325 2326 } 2327 2328 @Override 2329 public Base addChild(String name) throws FHIRException { 2330 if (name.equals("identifier")) { 2331 return addIdentifier(); 2332 } else if (name.equals("subject")) { 2333 return addSubject(); 2334 } else if (name.equals("description")) { 2335 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductPackaged.description"); 2336 } else if (name.equals("legalStatusOfSupply")) { 2337 this.legalStatusOfSupply = new CodeableConcept(); 2338 return this.legalStatusOfSupply; 2339 } else if (name.equals("marketingStatus")) { 2340 return addMarketingStatus(); 2341 } else if (name.equals("marketingAuthorization")) { 2342 this.marketingAuthorization = new Reference(); 2343 return this.marketingAuthorization; 2344 } else if (name.equals("manufacturer")) { 2345 return addManufacturer(); 2346 } else if (name.equals("batchIdentifier")) { 2347 return addBatchIdentifier(); 2348 } else if (name.equals("packageItem")) { 2349 return addPackageItem(); 2350 } else 2351 return super.addChild(name); 2352 } 2353 2354 public String fhirType() { 2355 return "MedicinalProductPackaged"; 2356 2357 } 2358 2359 public MedicinalProductPackaged copy() { 2360 MedicinalProductPackaged dst = new MedicinalProductPackaged(); 2361 copyValues(dst); 2362 return dst; 2363 } 2364 2365 public void copyValues(MedicinalProductPackaged dst) { 2366 super.copyValues(dst); 2367 if (identifier != null) { 2368 dst.identifier = new ArrayList<Identifier>(); 2369 for (Identifier i : identifier) 2370 dst.identifier.add(i.copy()); 2371 } 2372 ; 2373 if (subject != null) { 2374 dst.subject = new ArrayList<Reference>(); 2375 for (Reference i : subject) 2376 dst.subject.add(i.copy()); 2377 } 2378 ; 2379 dst.description = description == null ? null : description.copy(); 2380 dst.legalStatusOfSupply = legalStatusOfSupply == null ? null : legalStatusOfSupply.copy(); 2381 if (marketingStatus != null) { 2382 dst.marketingStatus = new ArrayList<MarketingStatus>(); 2383 for (MarketingStatus i : marketingStatus) 2384 dst.marketingStatus.add(i.copy()); 2385 } 2386 ; 2387 dst.marketingAuthorization = marketingAuthorization == null ? null : marketingAuthorization.copy(); 2388 if (manufacturer != null) { 2389 dst.manufacturer = new ArrayList<Reference>(); 2390 for (Reference i : manufacturer) 2391 dst.manufacturer.add(i.copy()); 2392 } 2393 ; 2394 if (batchIdentifier != null) { 2395 dst.batchIdentifier = new ArrayList<MedicinalProductPackagedBatchIdentifierComponent>(); 2396 for (MedicinalProductPackagedBatchIdentifierComponent i : batchIdentifier) 2397 dst.batchIdentifier.add(i.copy()); 2398 } 2399 ; 2400 if (packageItem != null) { 2401 dst.packageItem = new ArrayList<MedicinalProductPackagedPackageItemComponent>(); 2402 for (MedicinalProductPackagedPackageItemComponent i : packageItem) 2403 dst.packageItem.add(i.copy()); 2404 } 2405 ; 2406 } 2407 2408 protected MedicinalProductPackaged typedCopy() { 2409 return copy(); 2410 } 2411 2412 @Override 2413 public boolean equalsDeep(Base other_) { 2414 if (!super.equalsDeep(other_)) 2415 return false; 2416 if (!(other_ instanceof MedicinalProductPackaged)) 2417 return false; 2418 MedicinalProductPackaged o = (MedicinalProductPackaged) other_; 2419 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 2420 && compareDeep(description, o.description, true) 2421 && compareDeep(legalStatusOfSupply, o.legalStatusOfSupply, true) 2422 && compareDeep(marketingStatus, o.marketingStatus, true) 2423 && compareDeep(marketingAuthorization, o.marketingAuthorization, true) 2424 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(batchIdentifier, o.batchIdentifier, true) 2425 && compareDeep(packageItem, o.packageItem, true); 2426 } 2427 2428 @Override 2429 public boolean equalsShallow(Base other_) { 2430 if (!super.equalsShallow(other_)) 2431 return false; 2432 if (!(other_ instanceof MedicinalProductPackaged)) 2433 return false; 2434 MedicinalProductPackaged o = (MedicinalProductPackaged) other_; 2435 return compareValues(description, o.description, true); 2436 } 2437 2438 public boolean isEmpty() { 2439 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subject, description, 2440 legalStatusOfSupply, marketingStatus, marketingAuthorization, manufacturer, batchIdentifier, packageItem); 2441 } 2442 2443 @Override 2444 public ResourceType getResourceType() { 2445 return ResourceType.MedicinalProductPackaged; 2446 } 2447 2448 /** 2449 * Search parameter: <b>identifier</b> 2450 * <p> 2451 * Description: <b>Unique identifier</b><br> 2452 * Type: <b>token</b><br> 2453 * Path: <b>MedicinalProductPackaged.identifier</b><br> 2454 * </p> 2455 */ 2456 @SearchParamDefinition(name = "identifier", path = "MedicinalProductPackaged.identifier", description = "Unique identifier", type = "token") 2457 public static final String SP_IDENTIFIER = "identifier"; 2458 /** 2459 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2460 * <p> 2461 * Description: <b>Unique identifier</b><br> 2462 * Type: <b>token</b><br> 2463 * Path: <b>MedicinalProductPackaged.identifier</b><br> 2464 * </p> 2465 */ 2466 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2467 SP_IDENTIFIER); 2468 2469 /** 2470 * Search parameter: <b>subject</b> 2471 * <p> 2472 * Description: <b>The product with this is a pack for</b><br> 2473 * Type: <b>reference</b><br> 2474 * Path: <b>MedicinalProductPackaged.subject</b><br> 2475 * </p> 2476 */ 2477 @SearchParamDefinition(name = "subject", path = "MedicinalProductPackaged.subject", description = "The product with this is a pack for", type = "reference", target = { 2478 MedicinalProduct.class }) 2479 public static final String SP_SUBJECT = "subject"; 2480 /** 2481 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2482 * <p> 2483 * Description: <b>The product with this is a pack for</b><br> 2484 * Type: <b>reference</b><br> 2485 * Path: <b>MedicinalProductPackaged.subject</b><br> 2486 * </p> 2487 */ 2488 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2489 SP_SUBJECT); 2490 2491 /** 2492 * Constant for fluent queries to be used to add include statements. Specifies 2493 * the path value of "<b>MedicinalProductPackaged:subject</b>". 2494 */ 2495 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2496 "MedicinalProductPackaged:subject").toLocked(); 2497 2498}