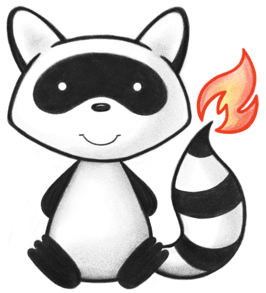
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A pharmaceutical product described in terms of its composition and dose form. 048 */ 049@ResourceDef(name = "MedicinalProductPharmaceutical", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductPharmaceutical") 050public class MedicinalProductPharmaceutical extends DomainResource { 051 052 @Block() 053 public static class MedicinalProductPharmaceuticalCharacteristicsComponent extends BackboneElement 054 implements IBaseBackboneElement { 055 /** 056 * A coded characteristic. 057 */ 058 @Child(name = "code", type = { 059 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "A coded characteristic", formalDefinition = "A coded characteristic.") 061 protected CodeableConcept code; 062 063 /** 064 * The status of characteristic e.g. assigned or pending. 065 */ 066 @Child(name = "status", type = { 067 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "The status of characteristic e.g. assigned or pending", formalDefinition = "The status of characteristic e.g. assigned or pending.") 069 protected CodeableConcept status; 070 071 private static final long serialVersionUID = 1414556635L; 072 073 /** 074 * Constructor 075 */ 076 public MedicinalProductPharmaceuticalCharacteristicsComponent() { 077 super(); 078 } 079 080 /** 081 * Constructor 082 */ 083 public MedicinalProductPharmaceuticalCharacteristicsComponent(CodeableConcept code) { 084 super(); 085 this.code = code; 086 } 087 088 /** 089 * @return {@link #code} (A coded characteristic.) 090 */ 091 public CodeableConcept getCode() { 092 if (this.code == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create MedicinalProductPharmaceuticalCharacteristicsComponent.code"); 095 else if (Configuration.doAutoCreate()) 096 this.code = new CodeableConcept(); // cc 097 return this.code; 098 } 099 100 public boolean hasCode() { 101 return this.code != null && !this.code.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #code} (A coded characteristic.) 106 */ 107 public MedicinalProductPharmaceuticalCharacteristicsComponent setCode(CodeableConcept value) { 108 this.code = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #status} (The status of characteristic e.g. assigned or 114 * pending.) 115 */ 116 public CodeableConcept getStatus() { 117 if (this.status == null) 118 if (Configuration.errorOnAutoCreate()) 119 throw new Error("Attempt to auto-create MedicinalProductPharmaceuticalCharacteristicsComponent.status"); 120 else if (Configuration.doAutoCreate()) 121 this.status = new CodeableConcept(); // cc 122 return this.status; 123 } 124 125 public boolean hasStatus() { 126 return this.status != null && !this.status.isEmpty(); 127 } 128 129 /** 130 * @param value {@link #status} (The status of characteristic e.g. assigned or 131 * pending.) 132 */ 133 public MedicinalProductPharmaceuticalCharacteristicsComponent setStatus(CodeableConcept value) { 134 this.status = value; 135 return this; 136 } 137 138 protected void listChildren(List<Property> children) { 139 super.listChildren(children); 140 children.add(new Property("code", "CodeableConcept", "A coded characteristic.", 0, 1, code)); 141 children.add(new Property("status", "CodeableConcept", "The status of characteristic e.g. assigned or pending.", 142 0, 1, status)); 143 } 144 145 @Override 146 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 147 switch (_hash) { 148 case 3059181: 149 /* code */ return new Property("code", "CodeableConcept", "A coded characteristic.", 0, 1, code); 150 case -892481550: 151 /* status */ return new Property("status", "CodeableConcept", 152 "The status of characteristic e.g. assigned or pending.", 0, 1, status); 153 default: 154 return super.getNamedProperty(_hash, _name, _checkValid); 155 } 156 157 } 158 159 @Override 160 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 161 switch (hash) { 162 case 3059181: 163 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 164 case -892481550: 165 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 166 default: 167 return super.getProperty(hash, name, checkValid); 168 } 169 170 } 171 172 @Override 173 public Base setProperty(int hash, String name, Base value) throws FHIRException { 174 switch (hash) { 175 case 3059181: // code 176 this.code = castToCodeableConcept(value); // CodeableConcept 177 return value; 178 case -892481550: // status 179 this.status = castToCodeableConcept(value); // CodeableConcept 180 return value; 181 default: 182 return super.setProperty(hash, name, value); 183 } 184 185 } 186 187 @Override 188 public Base setProperty(String name, Base value) throws FHIRException { 189 if (name.equals("code")) { 190 this.code = castToCodeableConcept(value); // CodeableConcept 191 } else if (name.equals("status")) { 192 this.status = castToCodeableConcept(value); // CodeableConcept 193 } else 194 return super.setProperty(name, value); 195 return value; 196 } 197 198 @Override 199 public void removeChild(String name, Base value) throws FHIRException { 200 if (name.equals("code")) { 201 this.code = null; 202 } else if (name.equals("status")) { 203 this.status = null; 204 } else 205 super.removeChild(name, value); 206 207 } 208 209 @Override 210 public Base makeProperty(int hash, String name) throws FHIRException { 211 switch (hash) { 212 case 3059181: 213 return getCode(); 214 case -892481550: 215 return getStatus(); 216 default: 217 return super.makeProperty(hash, name); 218 } 219 220 } 221 222 @Override 223 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 224 switch (hash) { 225 case 3059181: 226 /* code */ return new String[] { "CodeableConcept" }; 227 case -892481550: 228 /* status */ return new String[] { "CodeableConcept" }; 229 default: 230 return super.getTypesForProperty(hash, name); 231 } 232 233 } 234 235 @Override 236 public Base addChild(String name) throws FHIRException { 237 if (name.equals("code")) { 238 this.code = new CodeableConcept(); 239 return this.code; 240 } else if (name.equals("status")) { 241 this.status = new CodeableConcept(); 242 return this.status; 243 } else 244 return super.addChild(name); 245 } 246 247 public MedicinalProductPharmaceuticalCharacteristicsComponent copy() { 248 MedicinalProductPharmaceuticalCharacteristicsComponent dst = new MedicinalProductPharmaceuticalCharacteristicsComponent(); 249 copyValues(dst); 250 return dst; 251 } 252 253 public void copyValues(MedicinalProductPharmaceuticalCharacteristicsComponent dst) { 254 super.copyValues(dst); 255 dst.code = code == null ? null : code.copy(); 256 dst.status = status == null ? null : status.copy(); 257 } 258 259 @Override 260 public boolean equalsDeep(Base other_) { 261 if (!super.equalsDeep(other_)) 262 return false; 263 if (!(other_ instanceof MedicinalProductPharmaceuticalCharacteristicsComponent)) 264 return false; 265 MedicinalProductPharmaceuticalCharacteristicsComponent o = (MedicinalProductPharmaceuticalCharacteristicsComponent) other_; 266 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true); 267 } 268 269 @Override 270 public boolean equalsShallow(Base other_) { 271 if (!super.equalsShallow(other_)) 272 return false; 273 if (!(other_ instanceof MedicinalProductPharmaceuticalCharacteristicsComponent)) 274 return false; 275 MedicinalProductPharmaceuticalCharacteristicsComponent o = (MedicinalProductPharmaceuticalCharacteristicsComponent) other_; 276 return true; 277 } 278 279 public boolean isEmpty() { 280 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status); 281 } 282 283 public String fhirType() { 284 return "MedicinalProductPharmaceutical.characteristics"; 285 286 } 287 288 } 289 290 @Block() 291 public static class MedicinalProductPharmaceuticalRouteOfAdministrationComponent extends BackboneElement 292 implements IBaseBackboneElement { 293 /** 294 * Coded expression for the route. 295 */ 296 @Child(name = "code", type = { 297 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 298 @Description(shortDefinition = "Coded expression for the route", formalDefinition = "Coded expression for the route.") 299 protected CodeableConcept code; 300 301 /** 302 * The first dose (dose quantity) administered in humans can be specified, for a 303 * product under investigation, using a numerical value and its unit of 304 * measurement. 305 */ 306 @Child(name = "firstDose", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 307 @Description(shortDefinition = "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement", formalDefinition = "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement.") 308 protected Quantity firstDose; 309 310 /** 311 * The maximum single dose that can be administered as per the protocol of a 312 * clinical trial can be specified using a numerical value and its unit of 313 * measurement. 314 */ 315 @Child(name = "maxSingleDose", type = { 316 Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 317 @Description(shortDefinition = "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement", formalDefinition = "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement.") 318 protected Quantity maxSingleDose; 319 320 /** 321 * The maximum dose per day (maximum dose quantity to be administered in any one 322 * 24-h period) that can be administered as per the protocol referenced in the 323 * clinical trial authorisation. 324 */ 325 @Child(name = "maxDosePerDay", type = { 326 Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 327 @Description(shortDefinition = "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation", formalDefinition = "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation.") 328 protected Quantity maxDosePerDay; 329 330 /** 331 * The maximum dose per treatment period that can be administered as per the 332 * protocol referenced in the clinical trial authorisation. 333 */ 334 @Child(name = "maxDosePerTreatmentPeriod", type = { 335 Ratio.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 336 @Description(shortDefinition = "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation", formalDefinition = "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation.") 337 protected Ratio maxDosePerTreatmentPeriod; 338 339 /** 340 * The maximum treatment period during which an Investigational Medicinal 341 * Product can be administered as per the protocol referenced in the clinical 342 * trial authorisation. 343 */ 344 @Child(name = "maxTreatmentPeriod", type = { 345 Duration.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 346 @Description(shortDefinition = "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation", formalDefinition = "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation.") 347 protected Duration maxTreatmentPeriod; 348 349 /** 350 * A species for which this route applies. 351 */ 352 @Child(name = "targetSpecies", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 353 @Description(shortDefinition = "A species for which this route applies", formalDefinition = "A species for which this route applies.") 354 protected List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent> targetSpecies; 355 356 private static final long serialVersionUID = 854394783L; 357 358 /** 359 * Constructor 360 */ 361 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent() { 362 super(); 363 } 364 365 /** 366 * Constructor 367 */ 368 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent(CodeableConcept code) { 369 super(); 370 this.code = code; 371 } 372 373 /** 374 * @return {@link #code} (Coded expression for the route.) 375 */ 376 public CodeableConcept getCode() { 377 if (this.code == null) 378 if (Configuration.errorOnAutoCreate()) 379 throw new Error("Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.code"); 380 else if (Configuration.doAutoCreate()) 381 this.code = new CodeableConcept(); // cc 382 return this.code; 383 } 384 385 public boolean hasCode() { 386 return this.code != null && !this.code.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #code} (Coded expression for the route.) 391 */ 392 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setCode(CodeableConcept value) { 393 this.code = value; 394 return this; 395 } 396 397 /** 398 * @return {@link #firstDose} (The first dose (dose quantity) administered in 399 * humans can be specified, for a product under investigation, using a 400 * numerical value and its unit of measurement.) 401 */ 402 public Quantity getFirstDose() { 403 if (this.firstDose == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error( 406 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.firstDose"); 407 else if (Configuration.doAutoCreate()) 408 this.firstDose = new Quantity(); // cc 409 return this.firstDose; 410 } 411 412 public boolean hasFirstDose() { 413 return this.firstDose != null && !this.firstDose.isEmpty(); 414 } 415 416 /** 417 * @param value {@link #firstDose} (The first dose (dose quantity) administered 418 * in humans can be specified, for a product under investigation, 419 * using a numerical value and its unit of measurement.) 420 */ 421 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setFirstDose(Quantity value) { 422 this.firstDose = value; 423 return this; 424 } 425 426 /** 427 * @return {@link #maxSingleDose} (The maximum single dose that can be 428 * administered as per the protocol of a clinical trial can be specified 429 * using a numerical value and its unit of measurement.) 430 */ 431 public Quantity getMaxSingleDose() { 432 if (this.maxSingleDose == null) 433 if (Configuration.errorOnAutoCreate()) 434 throw new Error( 435 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxSingleDose"); 436 else if (Configuration.doAutoCreate()) 437 this.maxSingleDose = new Quantity(); // cc 438 return this.maxSingleDose; 439 } 440 441 public boolean hasMaxSingleDose() { 442 return this.maxSingleDose != null && !this.maxSingleDose.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #maxSingleDose} (The maximum single dose that can be 447 * administered as per the protocol of a clinical trial can be 448 * specified using a numerical value and its unit of measurement.) 449 */ 450 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxSingleDose(Quantity value) { 451 this.maxSingleDose = value; 452 return this; 453 } 454 455 /** 456 * @return {@link #maxDosePerDay} (The maximum dose per day (maximum dose 457 * quantity to be administered in any one 24-h period) that can be 458 * administered as per the protocol referenced in the clinical trial 459 * authorisation.) 460 */ 461 public Quantity getMaxDosePerDay() { 462 if (this.maxDosePerDay == null) 463 if (Configuration.errorOnAutoCreate()) 464 throw new Error( 465 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxDosePerDay"); 466 else if (Configuration.doAutoCreate()) 467 this.maxDosePerDay = new Quantity(); // cc 468 return this.maxDosePerDay; 469 } 470 471 public boolean hasMaxDosePerDay() { 472 return this.maxDosePerDay != null && !this.maxDosePerDay.isEmpty(); 473 } 474 475 /** 476 * @param value {@link #maxDosePerDay} (The maximum dose per day (maximum dose 477 * quantity to be administered in any one 24-h period) that can be 478 * administered as per the protocol referenced in the clinical 479 * trial authorisation.) 480 */ 481 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxDosePerDay(Quantity value) { 482 this.maxDosePerDay = value; 483 return this; 484 } 485 486 /** 487 * @return {@link #maxDosePerTreatmentPeriod} (The maximum dose per treatment 488 * period that can be administered as per the protocol referenced in the 489 * clinical trial authorisation.) 490 */ 491 public Ratio getMaxDosePerTreatmentPeriod() { 492 if (this.maxDosePerTreatmentPeriod == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error( 495 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxDosePerTreatmentPeriod"); 496 else if (Configuration.doAutoCreate()) 497 this.maxDosePerTreatmentPeriod = new Ratio(); // cc 498 return this.maxDosePerTreatmentPeriod; 499 } 500 501 public boolean hasMaxDosePerTreatmentPeriod() { 502 return this.maxDosePerTreatmentPeriod != null && !this.maxDosePerTreatmentPeriod.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #maxDosePerTreatmentPeriod} (The maximum dose per 507 * treatment period that can be administered as per the protocol 508 * referenced in the clinical trial authorisation.) 509 */ 510 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxDosePerTreatmentPeriod(Ratio value) { 511 this.maxDosePerTreatmentPeriod = value; 512 return this; 513 } 514 515 /** 516 * @return {@link #maxTreatmentPeriod} (The maximum treatment period during 517 * which an Investigational Medicinal Product can be administered as per 518 * the protocol referenced in the clinical trial authorisation.) 519 */ 520 public Duration getMaxTreatmentPeriod() { 521 if (this.maxTreatmentPeriod == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error( 524 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxTreatmentPeriod"); 525 else if (Configuration.doAutoCreate()) 526 this.maxTreatmentPeriod = new Duration(); // cc 527 return this.maxTreatmentPeriod; 528 } 529 530 public boolean hasMaxTreatmentPeriod() { 531 return this.maxTreatmentPeriod != null && !this.maxTreatmentPeriod.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #maxTreatmentPeriod} (The maximum treatment period during 536 * which an Investigational Medicinal Product can be administered 537 * as per the protocol referenced in the clinical trial 538 * authorisation.) 539 */ 540 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxTreatmentPeriod(Duration value) { 541 this.maxTreatmentPeriod = value; 542 return this; 543 } 544 545 /** 546 * @return {@link #targetSpecies} (A species for which this route applies.) 547 */ 548 public List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent> getTargetSpecies() { 549 if (this.targetSpecies == null) 550 this.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 551 return this.targetSpecies; 552 } 553 554 /** 555 * @return Returns a reference to <code>this</code> for easy method chaining 556 */ 557 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setTargetSpecies( 558 List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent> theTargetSpecies) { 559 this.targetSpecies = theTargetSpecies; 560 return this; 561 } 562 563 public boolean hasTargetSpecies() { 564 if (this.targetSpecies == null) 565 return false; 566 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent item : this.targetSpecies) 567 if (!item.isEmpty()) 568 return true; 569 return false; 570 } 571 572 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent addTargetSpecies() { // 3 573 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent t = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent(); 574 if (this.targetSpecies == null) 575 this.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 576 this.targetSpecies.add(t); 577 return t; 578 } 579 580 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent addTargetSpecies( 581 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent t) { // 3 582 if (t == null) 583 return this; 584 if (this.targetSpecies == null) 585 this.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 586 this.targetSpecies.add(t); 587 return this; 588 } 589 590 /** 591 * @return The first repetition of repeating field {@link #targetSpecies}, 592 * creating it if it does not already exist 593 */ 594 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent getTargetSpeciesFirstRep() { 595 if (getTargetSpecies().isEmpty()) { 596 addTargetSpecies(); 597 } 598 return getTargetSpecies().get(0); 599 } 600 601 protected void listChildren(List<Property> children) { 602 super.listChildren(children); 603 children.add(new Property("code", "CodeableConcept", "Coded expression for the route.", 0, 1, code)); 604 children.add(new Property("firstDose", "Quantity", 605 "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement.", 606 0, 1, firstDose)); 607 children.add(new Property("maxSingleDose", "Quantity", 608 "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement.", 609 0, 1, maxSingleDose)); 610 children.add(new Property("maxDosePerDay", "Quantity", 611 "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation.", 612 0, 1, maxDosePerDay)); 613 children.add(new Property("maxDosePerTreatmentPeriod", "Ratio", 614 "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation.", 615 0, 1, maxDosePerTreatmentPeriod)); 616 children.add(new Property("maxTreatmentPeriod", "Duration", 617 "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation.", 618 0, 1, maxTreatmentPeriod)); 619 children.add(new Property("targetSpecies", "", "A species for which this route applies.", 0, 620 java.lang.Integer.MAX_VALUE, targetSpecies)); 621 } 622 623 @Override 624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 625 switch (_hash) { 626 case 3059181: 627 /* code */ return new Property("code", "CodeableConcept", "Coded expression for the route.", 0, 1, code); 628 case 132551405: 629 /* firstDose */ return new Property("firstDose", "Quantity", 630 "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement.", 631 0, 1, firstDose); 632 case -259207927: 633 /* maxSingleDose */ return new Property("maxSingleDose", "Quantity", 634 "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement.", 635 0, 1, maxSingleDose); 636 case -2017475520: 637 /* maxDosePerDay */ return new Property("maxDosePerDay", "Quantity", 638 "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation.", 639 0, 1, maxDosePerDay); 640 case -608040195: 641 /* maxDosePerTreatmentPeriod */ return new Property("maxDosePerTreatmentPeriod", "Ratio", 642 "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation.", 643 0, 1, maxDosePerTreatmentPeriod); 644 case 920698453: 645 /* maxTreatmentPeriod */ return new Property("maxTreatmentPeriod", "Duration", 646 "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation.", 647 0, 1, maxTreatmentPeriod); 648 case 295481963: 649 /* targetSpecies */ return new Property("targetSpecies", "", "A species for which this route applies.", 0, 650 java.lang.Integer.MAX_VALUE, targetSpecies); 651 default: 652 return super.getNamedProperty(_hash, _name, _checkValid); 653 } 654 655 } 656 657 @Override 658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 659 switch (hash) { 660 case 3059181: 661 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 662 case 132551405: 663 /* firstDose */ return this.firstDose == null ? new Base[0] : new Base[] { this.firstDose }; // Quantity 664 case -259207927: 665 /* maxSingleDose */ return this.maxSingleDose == null ? new Base[0] : new Base[] { this.maxSingleDose }; // Quantity 666 case -2017475520: 667 /* maxDosePerDay */ return this.maxDosePerDay == null ? new Base[0] : new Base[] { this.maxDosePerDay }; // Quantity 668 case -608040195: 669 /* maxDosePerTreatmentPeriod */ return this.maxDosePerTreatmentPeriod == null ? new Base[0] 670 : new Base[] { this.maxDosePerTreatmentPeriod }; // Ratio 671 case 920698453: 672 /* maxTreatmentPeriod */ return this.maxTreatmentPeriod == null ? new Base[0] 673 : new Base[] { this.maxTreatmentPeriod }; // Duration 674 case 295481963: 675 /* targetSpecies */ return this.targetSpecies == null ? new Base[0] 676 : this.targetSpecies.toArray(new Base[this.targetSpecies.size()]); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent 677 default: 678 return super.getProperty(hash, name, checkValid); 679 } 680 681 } 682 683 @Override 684 public Base setProperty(int hash, String name, Base value) throws FHIRException { 685 switch (hash) { 686 case 3059181: // code 687 this.code = castToCodeableConcept(value); // CodeableConcept 688 return value; 689 case 132551405: // firstDose 690 this.firstDose = castToQuantity(value); // Quantity 691 return value; 692 case -259207927: // maxSingleDose 693 this.maxSingleDose = castToQuantity(value); // Quantity 694 return value; 695 case -2017475520: // maxDosePerDay 696 this.maxDosePerDay = castToQuantity(value); // Quantity 697 return value; 698 case -608040195: // maxDosePerTreatmentPeriod 699 this.maxDosePerTreatmentPeriod = castToRatio(value); // Ratio 700 return value; 701 case 920698453: // maxTreatmentPeriod 702 this.maxTreatmentPeriod = castToDuration(value); // Duration 703 return value; 704 case 295481963: // targetSpecies 705 this.getTargetSpecies().add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) value); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent 706 return value; 707 default: 708 return super.setProperty(hash, name, value); 709 } 710 711 } 712 713 @Override 714 public Base setProperty(String name, Base value) throws FHIRException { 715 if (name.equals("code")) { 716 this.code = castToCodeableConcept(value); // CodeableConcept 717 } else if (name.equals("firstDose")) { 718 this.firstDose = castToQuantity(value); // Quantity 719 } else if (name.equals("maxSingleDose")) { 720 this.maxSingleDose = castToQuantity(value); // Quantity 721 } else if (name.equals("maxDosePerDay")) { 722 this.maxDosePerDay = castToQuantity(value); // Quantity 723 } else if (name.equals("maxDosePerTreatmentPeriod")) { 724 this.maxDosePerTreatmentPeriod = castToRatio(value); // Ratio 725 } else if (name.equals("maxTreatmentPeriod")) { 726 this.maxTreatmentPeriod = castToDuration(value); // Duration 727 } else if (name.equals("targetSpecies")) { 728 this.getTargetSpecies().add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) value); 729 } else 730 return super.setProperty(name, value); 731 return value; 732 } 733 734 @Override 735 public void removeChild(String name, Base value) throws FHIRException { 736 if (name.equals("code")) { 737 this.code = null; 738 } else if (name.equals("firstDose")) { 739 this.firstDose = null; 740 } else if (name.equals("maxSingleDose")) { 741 this.maxSingleDose = null; 742 } else if (name.equals("maxDosePerDay")) { 743 this.maxDosePerDay = null; 744 } else if (name.equals("maxDosePerTreatmentPeriod")) { 745 this.maxDosePerTreatmentPeriod = null; 746 } else if (name.equals("maxTreatmentPeriod")) { 747 this.maxTreatmentPeriod = null; 748 } else if (name.equals("targetSpecies")) { 749 this.getTargetSpecies().remove((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) value); 750 } else 751 super.removeChild(name, value); 752 753 } 754 755 @Override 756 public Base makeProperty(int hash, String name) throws FHIRException { 757 switch (hash) { 758 case 3059181: 759 return getCode(); 760 case 132551405: 761 return getFirstDose(); 762 case -259207927: 763 return getMaxSingleDose(); 764 case -2017475520: 765 return getMaxDosePerDay(); 766 case -608040195: 767 return getMaxDosePerTreatmentPeriod(); 768 case 920698453: 769 return getMaxTreatmentPeriod(); 770 case 295481963: 771 return addTargetSpecies(); 772 default: 773 return super.makeProperty(hash, name); 774 } 775 776 } 777 778 @Override 779 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 780 switch (hash) { 781 case 3059181: 782 /* code */ return new String[] { "CodeableConcept" }; 783 case 132551405: 784 /* firstDose */ return new String[] { "Quantity" }; 785 case -259207927: 786 /* maxSingleDose */ return new String[] { "Quantity" }; 787 case -2017475520: 788 /* maxDosePerDay */ return new String[] { "Quantity" }; 789 case -608040195: 790 /* maxDosePerTreatmentPeriod */ return new String[] { "Ratio" }; 791 case 920698453: 792 /* maxTreatmentPeriod */ return new String[] { "Duration" }; 793 case 295481963: 794 /* targetSpecies */ return new String[] {}; 795 default: 796 return super.getTypesForProperty(hash, name); 797 } 798 799 } 800 801 @Override 802 public Base addChild(String name) throws FHIRException { 803 if (name.equals("code")) { 804 this.code = new CodeableConcept(); 805 return this.code; 806 } else if (name.equals("firstDose")) { 807 this.firstDose = new Quantity(); 808 return this.firstDose; 809 } else if (name.equals("maxSingleDose")) { 810 this.maxSingleDose = new Quantity(); 811 return this.maxSingleDose; 812 } else if (name.equals("maxDosePerDay")) { 813 this.maxDosePerDay = new Quantity(); 814 return this.maxDosePerDay; 815 } else if (name.equals("maxDosePerTreatmentPeriod")) { 816 this.maxDosePerTreatmentPeriod = new Ratio(); 817 return this.maxDosePerTreatmentPeriod; 818 } else if (name.equals("maxTreatmentPeriod")) { 819 this.maxTreatmentPeriod = new Duration(); 820 return this.maxTreatmentPeriod; 821 } else if (name.equals("targetSpecies")) { 822 return addTargetSpecies(); 823 } else 824 return super.addChild(name); 825 } 826 827 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent copy() { 828 MedicinalProductPharmaceuticalRouteOfAdministrationComponent dst = new MedicinalProductPharmaceuticalRouteOfAdministrationComponent(); 829 copyValues(dst); 830 return dst; 831 } 832 833 public void copyValues(MedicinalProductPharmaceuticalRouteOfAdministrationComponent dst) { 834 super.copyValues(dst); 835 dst.code = code == null ? null : code.copy(); 836 dst.firstDose = firstDose == null ? null : firstDose.copy(); 837 dst.maxSingleDose = maxSingleDose == null ? null : maxSingleDose.copy(); 838 dst.maxDosePerDay = maxDosePerDay == null ? null : maxDosePerDay.copy(); 839 dst.maxDosePerTreatmentPeriod = maxDosePerTreatmentPeriod == null ? null : maxDosePerTreatmentPeriod.copy(); 840 dst.maxTreatmentPeriod = maxTreatmentPeriod == null ? null : maxTreatmentPeriod.copy(); 841 if (targetSpecies != null) { 842 dst.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 843 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent i : targetSpecies) 844 dst.targetSpecies.add(i.copy()); 845 } 846 ; 847 } 848 849 @Override 850 public boolean equalsDeep(Base other_) { 851 if (!super.equalsDeep(other_)) 852 return false; 853 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationComponent)) 854 return false; 855 MedicinalProductPharmaceuticalRouteOfAdministrationComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationComponent) other_; 856 return compareDeep(code, o.code, true) && compareDeep(firstDose, o.firstDose, true) 857 && compareDeep(maxSingleDose, o.maxSingleDose, true) && compareDeep(maxDosePerDay, o.maxDosePerDay, true) 858 && compareDeep(maxDosePerTreatmentPeriod, o.maxDosePerTreatmentPeriod, true) 859 && compareDeep(maxTreatmentPeriod, o.maxTreatmentPeriod, true) 860 && compareDeep(targetSpecies, o.targetSpecies, true); 861 } 862 863 @Override 864 public boolean equalsShallow(Base other_) { 865 if (!super.equalsShallow(other_)) 866 return false; 867 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationComponent)) 868 return false; 869 MedicinalProductPharmaceuticalRouteOfAdministrationComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationComponent) other_; 870 return true; 871 } 872 873 public boolean isEmpty() { 874 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, firstDose, maxSingleDose, maxDosePerDay, 875 maxDosePerTreatmentPeriod, maxTreatmentPeriod, targetSpecies); 876 } 877 878 public String fhirType() { 879 return "MedicinalProductPharmaceutical.routeOfAdministration"; 880 881 } 882 883 } 884 885 @Block() 886 public static class MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent extends BackboneElement 887 implements IBaseBackboneElement { 888 /** 889 * Coded expression for the species. 890 */ 891 @Child(name = "code", type = { 892 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 893 @Description(shortDefinition = "Coded expression for the species", formalDefinition = "Coded expression for the species.") 894 protected CodeableConcept code; 895 896 /** 897 * A species specific time during which consumption of animal product is not 898 * appropriate. 899 */ 900 @Child(name = "withdrawalPeriod", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 901 @Description(shortDefinition = "A species specific time during which consumption of animal product is not appropriate", formalDefinition = "A species specific time during which consumption of animal product is not appropriate.") 902 protected List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> withdrawalPeriod; 903 904 private static final long serialVersionUID = -664052812L; 905 906 /** 907 * Constructor 908 */ 909 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent() { 910 super(); 911 } 912 913 /** 914 * Constructor 915 */ 916 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent(CodeableConcept code) { 917 super(); 918 this.code = code; 919 } 920 921 /** 922 * @return {@link #code} (Coded expression for the species.) 923 */ 924 public CodeableConcept getCode() { 925 if (this.code == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error( 928 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent.code"); 929 else if (Configuration.doAutoCreate()) 930 this.code = new CodeableConcept(); // cc 931 return this.code; 932 } 933 934 public boolean hasCode() { 935 return this.code != null && !this.code.isEmpty(); 936 } 937 938 /** 939 * @param value {@link #code} (Coded expression for the species.) 940 */ 941 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent setCode(CodeableConcept value) { 942 this.code = value; 943 return this; 944 } 945 946 /** 947 * @return {@link #withdrawalPeriod} (A species specific time during which 948 * consumption of animal product is not appropriate.) 949 */ 950 public List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> getWithdrawalPeriod() { 951 if (this.withdrawalPeriod == null) 952 this.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 953 return this.withdrawalPeriod; 954 } 955 956 /** 957 * @return Returns a reference to <code>this</code> for easy method chaining 958 */ 959 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent setWithdrawalPeriod( 960 List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> theWithdrawalPeriod) { 961 this.withdrawalPeriod = theWithdrawalPeriod; 962 return this; 963 } 964 965 public boolean hasWithdrawalPeriod() { 966 if (this.withdrawalPeriod == null) 967 return false; 968 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent item : this.withdrawalPeriod) 969 if (!item.isEmpty()) 970 return true; 971 return false; 972 } 973 974 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent addWithdrawalPeriod() { // 3 975 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent t = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(); 976 if (this.withdrawalPeriod == null) 977 this.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 978 this.withdrawalPeriod.add(t); 979 return t; 980 } 981 982 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent addWithdrawalPeriod( 983 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent t) { // 3 984 if (t == null) 985 return this; 986 if (this.withdrawalPeriod == null) 987 this.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 988 this.withdrawalPeriod.add(t); 989 return this; 990 } 991 992 /** 993 * @return The first repetition of repeating field {@link #withdrawalPeriod}, 994 * creating it if it does not already exist 995 */ 996 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent getWithdrawalPeriodFirstRep() { 997 if (getWithdrawalPeriod().isEmpty()) { 998 addWithdrawalPeriod(); 999 } 1000 return getWithdrawalPeriod().get(0); 1001 } 1002 1003 protected void listChildren(List<Property> children) { 1004 super.listChildren(children); 1005 children.add(new Property("code", "CodeableConcept", "Coded expression for the species.", 0, 1, code)); 1006 children.add(new Property("withdrawalPeriod", "", 1007 "A species specific time during which consumption of animal product is not appropriate.", 0, 1008 java.lang.Integer.MAX_VALUE, withdrawalPeriod)); 1009 } 1010 1011 @Override 1012 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1013 switch (_hash) { 1014 case 3059181: 1015 /* code */ return new Property("code", "CodeableConcept", "Coded expression for the species.", 0, 1, code); 1016 case -98450730: 1017 /* withdrawalPeriod */ return new Property("withdrawalPeriod", "", 1018 "A species specific time during which consumption of animal product is not appropriate.", 0, 1019 java.lang.Integer.MAX_VALUE, withdrawalPeriod); 1020 default: 1021 return super.getNamedProperty(_hash, _name, _checkValid); 1022 } 1023 1024 } 1025 1026 @Override 1027 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1028 switch (hash) { 1029 case 3059181: 1030 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1031 case -98450730: 1032 /* withdrawalPeriod */ return this.withdrawalPeriod == null ? new Base[0] 1033 : this.withdrawalPeriod.toArray(new Base[this.withdrawalPeriod.size()]); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1034 default: 1035 return super.getProperty(hash, name, checkValid); 1036 } 1037 1038 } 1039 1040 @Override 1041 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1042 switch (hash) { 1043 case 3059181: // code 1044 this.code = castToCodeableConcept(value); // CodeableConcept 1045 return value; 1046 case -98450730: // withdrawalPeriod 1047 this.getWithdrawalPeriod() 1048 .add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1049 return value; 1050 default: 1051 return super.setProperty(hash, name, value); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base setProperty(String name, Base value) throws FHIRException { 1058 if (name.equals("code")) { 1059 this.code = castToCodeableConcept(value); // CodeableConcept 1060 } else if (name.equals("withdrawalPeriod")) { 1061 this.getWithdrawalPeriod() 1062 .add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); 1063 } else 1064 return super.setProperty(name, value); 1065 return value; 1066 } 1067 1068 @Override 1069 public void removeChild(String name, Base value) throws FHIRException { 1070 if (name.equals("code")) { 1071 this.code = null; 1072 } else if (name.equals("withdrawalPeriod")) { 1073 this.getWithdrawalPeriod() 1074 .remove((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); 1075 } else 1076 super.removeChild(name, value); 1077 1078 } 1079 1080 @Override 1081 public Base makeProperty(int hash, String name) throws FHIRException { 1082 switch (hash) { 1083 case 3059181: 1084 return getCode(); 1085 case -98450730: 1086 return addWithdrawalPeriod(); 1087 default: 1088 return super.makeProperty(hash, name); 1089 } 1090 1091 } 1092 1093 @Override 1094 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1095 switch (hash) { 1096 case 3059181: 1097 /* code */ return new String[] { "CodeableConcept" }; 1098 case -98450730: 1099 /* withdrawalPeriod */ return new String[] {}; 1100 default: 1101 return super.getTypesForProperty(hash, name); 1102 } 1103 1104 } 1105 1106 @Override 1107 public Base addChild(String name) throws FHIRException { 1108 if (name.equals("code")) { 1109 this.code = new CodeableConcept(); 1110 return this.code; 1111 } else if (name.equals("withdrawalPeriod")) { 1112 return addWithdrawalPeriod(); 1113 } else 1114 return super.addChild(name); 1115 } 1116 1117 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent copy() { 1118 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent dst = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent(); 1119 copyValues(dst); 1120 return dst; 1121 } 1122 1123 public void copyValues(MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent dst) { 1124 super.copyValues(dst); 1125 dst.code = code == null ? null : code.copy(); 1126 if (withdrawalPeriod != null) { 1127 dst.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 1128 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent i : withdrawalPeriod) 1129 dst.withdrawalPeriod.add(i.copy()); 1130 } 1131 ; 1132 } 1133 1134 @Override 1135 public boolean equalsDeep(Base other_) { 1136 if (!super.equalsDeep(other_)) 1137 return false; 1138 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent)) 1139 return false; 1140 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) other_; 1141 return compareDeep(code, o.code, true) && compareDeep(withdrawalPeriod, o.withdrawalPeriod, true); 1142 } 1143 1144 @Override 1145 public boolean equalsShallow(Base other_) { 1146 if (!super.equalsShallow(other_)) 1147 return false; 1148 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent)) 1149 return false; 1150 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) other_; 1151 return true; 1152 } 1153 1154 public boolean isEmpty() { 1155 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, withdrawalPeriod); 1156 } 1157 1158 public String fhirType() { 1159 return "MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies"; 1160 1161 } 1162 1163 } 1164 1165 @Block() 1166 public static class MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1167 extends BackboneElement implements IBaseBackboneElement { 1168 /** 1169 * Coded expression for the type of tissue for which the withdrawal period 1170 * applues, e.g. meat, milk. 1171 */ 1172 @Child(name = "tissue", type = { 1173 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1174 @Description(shortDefinition = "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk", formalDefinition = "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk.") 1175 protected CodeableConcept tissue; 1176 1177 /** 1178 * A value for the time. 1179 */ 1180 @Child(name = "value", type = { Quantity.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1181 @Description(shortDefinition = "A value for the time", formalDefinition = "A value for the time.") 1182 protected Quantity value; 1183 1184 /** 1185 * Extra information about the withdrawal period. 1186 */ 1187 @Child(name = "supportingInformation", type = { 1188 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1189 @Description(shortDefinition = "Extra information about the withdrawal period", formalDefinition = "Extra information about the withdrawal period.") 1190 protected StringType supportingInformation; 1191 1192 private static final long serialVersionUID = -1113691238L; 1193 1194 /** 1195 * Constructor 1196 */ 1197 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent() { 1198 super(); 1199 } 1200 1201 /** 1202 * Constructor 1203 */ 1204 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent( 1205 CodeableConcept tissue, Quantity value) { 1206 super(); 1207 this.tissue = tissue; 1208 this.value = value; 1209 } 1210 1211 /** 1212 * @return {@link #tissue} (Coded expression for the type of tissue for which 1213 * the withdrawal period applues, e.g. meat, milk.) 1214 */ 1215 public CodeableConcept getTissue() { 1216 if (this.tissue == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error( 1219 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.tissue"); 1220 else if (Configuration.doAutoCreate()) 1221 this.tissue = new CodeableConcept(); // cc 1222 return this.tissue; 1223 } 1224 1225 public boolean hasTissue() { 1226 return this.tissue != null && !this.tissue.isEmpty(); 1227 } 1228 1229 /** 1230 * @param value {@link #tissue} (Coded expression for the type of tissue for 1231 * which the withdrawal period applues, e.g. meat, milk.) 1232 */ 1233 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setTissue( 1234 CodeableConcept value) { 1235 this.tissue = value; 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #value} (A value for the time.) 1241 */ 1242 public Quantity getValue() { 1243 if (this.value == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error( 1246 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.value"); 1247 else if (Configuration.doAutoCreate()) 1248 this.value = new Quantity(); // cc 1249 return this.value; 1250 } 1251 1252 public boolean hasValue() { 1253 return this.value != null && !this.value.isEmpty(); 1254 } 1255 1256 /** 1257 * @param value {@link #value} (A value for the time.) 1258 */ 1259 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setValue( 1260 Quantity value) { 1261 this.value = value; 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #supportingInformation} (Extra information about the 1267 * withdrawal period.). This is the underlying object with id, value and 1268 * extensions. The accessor "getSupportingInformation" gives direct 1269 * access to the value 1270 */ 1271 public StringType getSupportingInformationElement() { 1272 if (this.supportingInformation == null) 1273 if (Configuration.errorOnAutoCreate()) 1274 throw new Error( 1275 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.supportingInformation"); 1276 else if (Configuration.doAutoCreate()) 1277 this.supportingInformation = new StringType(); // bb 1278 return this.supportingInformation; 1279 } 1280 1281 public boolean hasSupportingInformationElement() { 1282 return this.supportingInformation != null && !this.supportingInformation.isEmpty(); 1283 } 1284 1285 public boolean hasSupportingInformation() { 1286 return this.supportingInformation != null && !this.supportingInformation.isEmpty(); 1287 } 1288 1289 /** 1290 * @param value {@link #supportingInformation} (Extra information about the 1291 * withdrawal period.). This is the underlying object with id, 1292 * value and extensions. The accessor "getSupportingInformation" 1293 * gives direct access to the value 1294 */ 1295 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setSupportingInformationElement( 1296 StringType value) { 1297 this.supportingInformation = value; 1298 return this; 1299 } 1300 1301 /** 1302 * @return Extra information about the withdrawal period. 1303 */ 1304 public String getSupportingInformation() { 1305 return this.supportingInformation == null ? null : this.supportingInformation.getValue(); 1306 } 1307 1308 /** 1309 * @param value Extra information about the withdrawal period. 1310 */ 1311 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setSupportingInformation( 1312 String value) { 1313 if (Utilities.noString(value)) 1314 this.supportingInformation = null; 1315 else { 1316 if (this.supportingInformation == null) 1317 this.supportingInformation = new StringType(); 1318 this.supportingInformation.setValue(value); 1319 } 1320 return this; 1321 } 1322 1323 protected void listChildren(List<Property> children) { 1324 super.listChildren(children); 1325 children.add(new Property("tissue", "CodeableConcept", 1326 "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk.", 0, 1, 1327 tissue)); 1328 children.add(new Property("value", "Quantity", "A value for the time.", 0, 1, value)); 1329 children.add(new Property("supportingInformation", "string", "Extra information about the withdrawal period.", 0, 1330 1, supportingInformation)); 1331 } 1332 1333 @Override 1334 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1335 switch (_hash) { 1336 case -873475867: 1337 /* tissue */ return new Property("tissue", "CodeableConcept", 1338 "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk.", 0, 1, 1339 tissue); 1340 case 111972721: 1341 /* value */ return new Property("value", "Quantity", "A value for the time.", 0, 1, value); 1342 case -1248768647: 1343 /* supportingInformation */ return new Property("supportingInformation", "string", 1344 "Extra information about the withdrawal period.", 0, 1, supportingInformation); 1345 default: 1346 return super.getNamedProperty(_hash, _name, _checkValid); 1347 } 1348 1349 } 1350 1351 @Override 1352 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1353 switch (hash) { 1354 case -873475867: 1355 /* tissue */ return this.tissue == null ? new Base[0] : new Base[] { this.tissue }; // CodeableConcept 1356 case 111972721: 1357 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Quantity 1358 case -1248768647: 1359 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 1360 : new Base[] { this.supportingInformation }; // StringType 1361 default: 1362 return super.getProperty(hash, name, checkValid); 1363 } 1364 1365 } 1366 1367 @Override 1368 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1369 switch (hash) { 1370 case -873475867: // tissue 1371 this.tissue = castToCodeableConcept(value); // CodeableConcept 1372 return value; 1373 case 111972721: // value 1374 this.value = castToQuantity(value); // Quantity 1375 return value; 1376 case -1248768647: // supportingInformation 1377 this.supportingInformation = castToString(value); // StringType 1378 return value; 1379 default: 1380 return super.setProperty(hash, name, value); 1381 } 1382 1383 } 1384 1385 @Override 1386 public Base setProperty(String name, Base value) throws FHIRException { 1387 if (name.equals("tissue")) { 1388 this.tissue = castToCodeableConcept(value); // CodeableConcept 1389 } else if (name.equals("value")) { 1390 this.value = castToQuantity(value); // Quantity 1391 } else if (name.equals("supportingInformation")) { 1392 this.supportingInformation = castToString(value); // StringType 1393 } else 1394 return super.setProperty(name, value); 1395 return value; 1396 } 1397 1398 @Override 1399 public void removeChild(String name, Base value) throws FHIRException { 1400 if (name.equals("tissue")) { 1401 this.tissue = null; 1402 } else if (name.equals("value")) { 1403 this.value = null; 1404 } else if (name.equals("supportingInformation")) { 1405 this.supportingInformation = null; 1406 } else 1407 super.removeChild(name, value); 1408 1409 } 1410 1411 @Override 1412 public Base makeProperty(int hash, String name) throws FHIRException { 1413 switch (hash) { 1414 case -873475867: 1415 return getTissue(); 1416 case 111972721: 1417 return getValue(); 1418 case -1248768647: 1419 return getSupportingInformationElement(); 1420 default: 1421 return super.makeProperty(hash, name); 1422 } 1423 1424 } 1425 1426 @Override 1427 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1428 switch (hash) { 1429 case -873475867: 1430 /* tissue */ return new String[] { "CodeableConcept" }; 1431 case 111972721: 1432 /* value */ return new String[] { "Quantity" }; 1433 case -1248768647: 1434 /* supportingInformation */ return new String[] { "string" }; 1435 default: 1436 return super.getTypesForProperty(hash, name); 1437 } 1438 1439 } 1440 1441 @Override 1442 public Base addChild(String name) throws FHIRException { 1443 if (name.equals("tissue")) { 1444 this.tissue = new CodeableConcept(); 1445 return this.tissue; 1446 } else if (name.equals("value")) { 1447 this.value = new Quantity(); 1448 return this.value; 1449 } else if (name.equals("supportingInformation")) { 1450 throw new FHIRException( 1451 "Cannot call addChild on a singleton property MedicinalProductPharmaceutical.supportingInformation"); 1452 } else 1453 return super.addChild(name); 1454 } 1455 1456 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent copy() { 1457 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent dst = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(); 1458 copyValues(dst); 1459 return dst; 1460 } 1461 1462 public void copyValues( 1463 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent dst) { 1464 super.copyValues(dst); 1465 dst.tissue = tissue == null ? null : tissue.copy(); 1466 dst.value = value == null ? null : value.copy(); 1467 dst.supportingInformation = supportingInformation == null ? null : supportingInformation.copy(); 1468 } 1469 1470 @Override 1471 public boolean equalsDeep(Base other_) { 1472 if (!super.equalsDeep(other_)) 1473 return false; 1474 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent)) 1475 return false; 1476 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) other_; 1477 return compareDeep(tissue, o.tissue, true) && compareDeep(value, o.value, true) 1478 && compareDeep(supportingInformation, o.supportingInformation, true); 1479 } 1480 1481 @Override 1482 public boolean equalsShallow(Base other_) { 1483 if (!super.equalsShallow(other_)) 1484 return false; 1485 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent)) 1486 return false; 1487 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) other_; 1488 return compareValues(supportingInformation, o.supportingInformation, true); 1489 } 1490 1491 public boolean isEmpty() { 1492 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(tissue, value, supportingInformation); 1493 } 1494 1495 public String fhirType() { 1496 return "MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.withdrawalPeriod"; 1497 1498 } 1499 1500 } 1501 1502 /** 1503 * An identifier for the pharmaceutical medicinal product. 1504 */ 1505 @Child(name = "identifier", type = { 1506 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1507 @Description(shortDefinition = "An identifier for the pharmaceutical medicinal product", formalDefinition = "An identifier for the pharmaceutical medicinal product.") 1508 protected List<Identifier> identifier; 1509 1510 /** 1511 * The administrable dose form, after necessary reconstitution. 1512 */ 1513 @Child(name = "administrableDoseForm", type = { 1514 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1515 @Description(shortDefinition = "The administrable dose form, after necessary reconstitution", formalDefinition = "The administrable dose form, after necessary reconstitution.") 1516 protected CodeableConcept administrableDoseForm; 1517 1518 /** 1519 * Todo. 1520 */ 1521 @Child(name = "unitOfPresentation", type = { 1522 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1523 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1524 protected CodeableConcept unitOfPresentation; 1525 1526 /** 1527 * Ingredient. 1528 */ 1529 @Child(name = "ingredient", type = { 1530 MedicinalProductIngredient.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1531 @Description(shortDefinition = "Ingredient", formalDefinition = "Ingredient.") 1532 protected List<Reference> ingredient; 1533 /** 1534 * The actual objects that are the target of the reference (Ingredient.) 1535 */ 1536 protected List<MedicinalProductIngredient> ingredientTarget; 1537 1538 /** 1539 * Accompanying device. 1540 */ 1541 @Child(name = "device", type = { 1542 DeviceDefinition.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1543 @Description(shortDefinition = "Accompanying device", formalDefinition = "Accompanying device.") 1544 protected List<Reference> device; 1545 /** 1546 * The actual objects that are the target of the reference (Accompanying 1547 * device.) 1548 */ 1549 protected List<DeviceDefinition> deviceTarget; 1550 1551 /** 1552 * Characteristics e.g. a products onset of action. 1553 */ 1554 @Child(name = "characteristics", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1555 @Description(shortDefinition = "Characteristics e.g. a products onset of action", formalDefinition = "Characteristics e.g. a products onset of action.") 1556 protected List<MedicinalProductPharmaceuticalCharacteristicsComponent> characteristics; 1557 1558 /** 1559 * The path by which the pharmaceutical product is taken into or makes contact 1560 * with the body. 1561 */ 1562 @Child(name = "routeOfAdministration", type = {}, order = 6, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1563 @Description(shortDefinition = "The path by which the pharmaceutical product is taken into or makes contact with the body", formalDefinition = "The path by which the pharmaceutical product is taken into or makes contact with the body.") 1564 protected List<MedicinalProductPharmaceuticalRouteOfAdministrationComponent> routeOfAdministration; 1565 1566 private static final long serialVersionUID = -1201548050L; 1567 1568 /** 1569 * Constructor 1570 */ 1571 public MedicinalProductPharmaceutical() { 1572 super(); 1573 } 1574 1575 /** 1576 * Constructor 1577 */ 1578 public MedicinalProductPharmaceutical(CodeableConcept administrableDoseForm) { 1579 super(); 1580 this.administrableDoseForm = administrableDoseForm; 1581 } 1582 1583 /** 1584 * @return {@link #identifier} (An identifier for the pharmaceutical medicinal 1585 * product.) 1586 */ 1587 public List<Identifier> getIdentifier() { 1588 if (this.identifier == null) 1589 this.identifier = new ArrayList<Identifier>(); 1590 return this.identifier; 1591 } 1592 1593 /** 1594 * @return Returns a reference to <code>this</code> for easy method chaining 1595 */ 1596 public MedicinalProductPharmaceutical setIdentifier(List<Identifier> theIdentifier) { 1597 this.identifier = theIdentifier; 1598 return this; 1599 } 1600 1601 public boolean hasIdentifier() { 1602 if (this.identifier == null) 1603 return false; 1604 for (Identifier item : this.identifier) 1605 if (!item.isEmpty()) 1606 return true; 1607 return false; 1608 } 1609 1610 public Identifier addIdentifier() { // 3 1611 Identifier t = new Identifier(); 1612 if (this.identifier == null) 1613 this.identifier = new ArrayList<Identifier>(); 1614 this.identifier.add(t); 1615 return t; 1616 } 1617 1618 public MedicinalProductPharmaceutical addIdentifier(Identifier t) { // 3 1619 if (t == null) 1620 return this; 1621 if (this.identifier == null) 1622 this.identifier = new ArrayList<Identifier>(); 1623 this.identifier.add(t); 1624 return this; 1625 } 1626 1627 /** 1628 * @return The first repetition of repeating field {@link #identifier}, creating 1629 * it if it does not already exist 1630 */ 1631 public Identifier getIdentifierFirstRep() { 1632 if (getIdentifier().isEmpty()) { 1633 addIdentifier(); 1634 } 1635 return getIdentifier().get(0); 1636 } 1637 1638 /** 1639 * @return {@link #administrableDoseForm} (The administrable dose form, after 1640 * necessary reconstitution.) 1641 */ 1642 public CodeableConcept getAdministrableDoseForm() { 1643 if (this.administrableDoseForm == null) 1644 if (Configuration.errorOnAutoCreate()) 1645 throw new Error("Attempt to auto-create MedicinalProductPharmaceutical.administrableDoseForm"); 1646 else if (Configuration.doAutoCreate()) 1647 this.administrableDoseForm = new CodeableConcept(); // cc 1648 return this.administrableDoseForm; 1649 } 1650 1651 public boolean hasAdministrableDoseForm() { 1652 return this.administrableDoseForm != null && !this.administrableDoseForm.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #administrableDoseForm} (The administrable dose form, 1657 * after necessary reconstitution.) 1658 */ 1659 public MedicinalProductPharmaceutical setAdministrableDoseForm(CodeableConcept value) { 1660 this.administrableDoseForm = value; 1661 return this; 1662 } 1663 1664 /** 1665 * @return {@link #unitOfPresentation} (Todo.) 1666 */ 1667 public CodeableConcept getUnitOfPresentation() { 1668 if (this.unitOfPresentation == null) 1669 if (Configuration.errorOnAutoCreate()) 1670 throw new Error("Attempt to auto-create MedicinalProductPharmaceutical.unitOfPresentation"); 1671 else if (Configuration.doAutoCreate()) 1672 this.unitOfPresentation = new CodeableConcept(); // cc 1673 return this.unitOfPresentation; 1674 } 1675 1676 public boolean hasUnitOfPresentation() { 1677 return this.unitOfPresentation != null && !this.unitOfPresentation.isEmpty(); 1678 } 1679 1680 /** 1681 * @param value {@link #unitOfPresentation} (Todo.) 1682 */ 1683 public MedicinalProductPharmaceutical setUnitOfPresentation(CodeableConcept value) { 1684 this.unitOfPresentation = value; 1685 return this; 1686 } 1687 1688 /** 1689 * @return {@link #ingredient} (Ingredient.) 1690 */ 1691 public List<Reference> getIngredient() { 1692 if (this.ingredient == null) 1693 this.ingredient = new ArrayList<Reference>(); 1694 return this.ingredient; 1695 } 1696 1697 /** 1698 * @return Returns a reference to <code>this</code> for easy method chaining 1699 */ 1700 public MedicinalProductPharmaceutical setIngredient(List<Reference> theIngredient) { 1701 this.ingredient = theIngredient; 1702 return this; 1703 } 1704 1705 public boolean hasIngredient() { 1706 if (this.ingredient == null) 1707 return false; 1708 for (Reference item : this.ingredient) 1709 if (!item.isEmpty()) 1710 return true; 1711 return false; 1712 } 1713 1714 public Reference addIngredient() { // 3 1715 Reference t = new Reference(); 1716 if (this.ingredient == null) 1717 this.ingredient = new ArrayList<Reference>(); 1718 this.ingredient.add(t); 1719 return t; 1720 } 1721 1722 public MedicinalProductPharmaceutical addIngredient(Reference t) { // 3 1723 if (t == null) 1724 return this; 1725 if (this.ingredient == null) 1726 this.ingredient = new ArrayList<Reference>(); 1727 this.ingredient.add(t); 1728 return this; 1729 } 1730 1731 /** 1732 * @return The first repetition of repeating field {@link #ingredient}, creating 1733 * it if it does not already exist 1734 */ 1735 public Reference getIngredientFirstRep() { 1736 if (getIngredient().isEmpty()) { 1737 addIngredient(); 1738 } 1739 return getIngredient().get(0); 1740 } 1741 1742 /** 1743 * @return {@link #device} (Accompanying device.) 1744 */ 1745 public List<Reference> getDevice() { 1746 if (this.device == null) 1747 this.device = new ArrayList<Reference>(); 1748 return this.device; 1749 } 1750 1751 /** 1752 * @return Returns a reference to <code>this</code> for easy method chaining 1753 */ 1754 public MedicinalProductPharmaceutical setDevice(List<Reference> theDevice) { 1755 this.device = theDevice; 1756 return this; 1757 } 1758 1759 public boolean hasDevice() { 1760 if (this.device == null) 1761 return false; 1762 for (Reference item : this.device) 1763 if (!item.isEmpty()) 1764 return true; 1765 return false; 1766 } 1767 1768 public Reference addDevice() { // 3 1769 Reference t = new Reference(); 1770 if (this.device == null) 1771 this.device = new ArrayList<Reference>(); 1772 this.device.add(t); 1773 return t; 1774 } 1775 1776 public MedicinalProductPharmaceutical addDevice(Reference t) { // 3 1777 if (t == null) 1778 return this; 1779 if (this.device == null) 1780 this.device = new ArrayList<Reference>(); 1781 this.device.add(t); 1782 return this; 1783 } 1784 1785 /** 1786 * @return The first repetition of repeating field {@link #device}, creating it 1787 * if it does not already exist 1788 */ 1789 public Reference getDeviceFirstRep() { 1790 if (getDevice().isEmpty()) { 1791 addDevice(); 1792 } 1793 return getDevice().get(0); 1794 } 1795 1796 /** 1797 * @return {@link #characteristics} (Characteristics e.g. a products onset of 1798 * action.) 1799 */ 1800 public List<MedicinalProductPharmaceuticalCharacteristicsComponent> getCharacteristics() { 1801 if (this.characteristics == null) 1802 this.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 1803 return this.characteristics; 1804 } 1805 1806 /** 1807 * @return Returns a reference to <code>this</code> for easy method chaining 1808 */ 1809 public MedicinalProductPharmaceutical setCharacteristics( 1810 List<MedicinalProductPharmaceuticalCharacteristicsComponent> theCharacteristics) { 1811 this.characteristics = theCharacteristics; 1812 return this; 1813 } 1814 1815 public boolean hasCharacteristics() { 1816 if (this.characteristics == null) 1817 return false; 1818 for (MedicinalProductPharmaceuticalCharacteristicsComponent item : this.characteristics) 1819 if (!item.isEmpty()) 1820 return true; 1821 return false; 1822 } 1823 1824 public MedicinalProductPharmaceuticalCharacteristicsComponent addCharacteristics() { // 3 1825 MedicinalProductPharmaceuticalCharacteristicsComponent t = new MedicinalProductPharmaceuticalCharacteristicsComponent(); 1826 if (this.characteristics == null) 1827 this.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 1828 this.characteristics.add(t); 1829 return t; 1830 } 1831 1832 public MedicinalProductPharmaceutical addCharacteristics(MedicinalProductPharmaceuticalCharacteristicsComponent t) { // 3 1833 if (t == null) 1834 return this; 1835 if (this.characteristics == null) 1836 this.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 1837 this.characteristics.add(t); 1838 return this; 1839 } 1840 1841 /** 1842 * @return The first repetition of repeating field {@link #characteristics}, 1843 * creating it if it does not already exist 1844 */ 1845 public MedicinalProductPharmaceuticalCharacteristicsComponent getCharacteristicsFirstRep() { 1846 if (getCharacteristics().isEmpty()) { 1847 addCharacteristics(); 1848 } 1849 return getCharacteristics().get(0); 1850 } 1851 1852 /** 1853 * @return {@link #routeOfAdministration} (The path by which the pharmaceutical 1854 * product is taken into or makes contact with the body.) 1855 */ 1856 public List<MedicinalProductPharmaceuticalRouteOfAdministrationComponent> getRouteOfAdministration() { 1857 if (this.routeOfAdministration == null) 1858 this.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 1859 return this.routeOfAdministration; 1860 } 1861 1862 /** 1863 * @return Returns a reference to <code>this</code> for easy method chaining 1864 */ 1865 public MedicinalProductPharmaceutical setRouteOfAdministration( 1866 List<MedicinalProductPharmaceuticalRouteOfAdministrationComponent> theRouteOfAdministration) { 1867 this.routeOfAdministration = theRouteOfAdministration; 1868 return this; 1869 } 1870 1871 public boolean hasRouteOfAdministration() { 1872 if (this.routeOfAdministration == null) 1873 return false; 1874 for (MedicinalProductPharmaceuticalRouteOfAdministrationComponent item : this.routeOfAdministration) 1875 if (!item.isEmpty()) 1876 return true; 1877 return false; 1878 } 1879 1880 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent addRouteOfAdministration() { // 3 1881 MedicinalProductPharmaceuticalRouteOfAdministrationComponent t = new MedicinalProductPharmaceuticalRouteOfAdministrationComponent(); 1882 if (this.routeOfAdministration == null) 1883 this.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 1884 this.routeOfAdministration.add(t); 1885 return t; 1886 } 1887 1888 public MedicinalProductPharmaceutical addRouteOfAdministration( 1889 MedicinalProductPharmaceuticalRouteOfAdministrationComponent t) { // 3 1890 if (t == null) 1891 return this; 1892 if (this.routeOfAdministration == null) 1893 this.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 1894 this.routeOfAdministration.add(t); 1895 return this; 1896 } 1897 1898 /** 1899 * @return The first repetition of repeating field 1900 * {@link #routeOfAdministration}, creating it if it does not already 1901 * exist 1902 */ 1903 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent getRouteOfAdministrationFirstRep() { 1904 if (getRouteOfAdministration().isEmpty()) { 1905 addRouteOfAdministration(); 1906 } 1907 return getRouteOfAdministration().get(0); 1908 } 1909 1910 protected void listChildren(List<Property> children) { 1911 super.listChildren(children); 1912 children.add(new Property("identifier", "Identifier", "An identifier for the pharmaceutical medicinal product.", 0, 1913 java.lang.Integer.MAX_VALUE, identifier)); 1914 children.add(new Property("administrableDoseForm", "CodeableConcept", 1915 "The administrable dose form, after necessary reconstitution.", 0, 1, administrableDoseForm)); 1916 children.add(new Property("unitOfPresentation", "CodeableConcept", "Todo.", 0, 1, unitOfPresentation)); 1917 children.add(new Property("ingredient", "Reference(MedicinalProductIngredient)", "Ingredient.", 0, 1918 java.lang.Integer.MAX_VALUE, ingredient)); 1919 children.add(new Property("device", "Reference(DeviceDefinition)", "Accompanying device.", 0, 1920 java.lang.Integer.MAX_VALUE, device)); 1921 children.add(new Property("characteristics", "", "Characteristics e.g. a products onset of action.", 0, 1922 java.lang.Integer.MAX_VALUE, characteristics)); 1923 children.add(new Property("routeOfAdministration", "", 1924 "The path by which the pharmaceutical product is taken into or makes contact with the body.", 0, 1925 java.lang.Integer.MAX_VALUE, routeOfAdministration)); 1926 } 1927 1928 @Override 1929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1930 switch (_hash) { 1931 case -1618432855: 1932 /* identifier */ return new Property("identifier", "Identifier", 1933 "An identifier for the pharmaceutical medicinal product.", 0, java.lang.Integer.MAX_VALUE, identifier); 1934 case 1446105202: 1935 /* administrableDoseForm */ return new Property("administrableDoseForm", "CodeableConcept", 1936 "The administrable dose form, after necessary reconstitution.", 0, 1, administrableDoseForm); 1937 case -1427765963: 1938 /* unitOfPresentation */ return new Property("unitOfPresentation", "CodeableConcept", "Todo.", 0, 1, 1939 unitOfPresentation); 1940 case -206409263: 1941 /* ingredient */ return new Property("ingredient", "Reference(MedicinalProductIngredient)", "Ingredient.", 0, 1942 java.lang.Integer.MAX_VALUE, ingredient); 1943 case -1335157162: 1944 /* device */ return new Property("device", "Reference(DeviceDefinition)", "Accompanying device.", 0, 1945 java.lang.Integer.MAX_VALUE, device); 1946 case -1529171400: 1947 /* characteristics */ return new Property("characteristics", "", 1948 "Characteristics e.g. a products onset of action.", 0, java.lang.Integer.MAX_VALUE, characteristics); 1949 case 1742084734: 1950 /* routeOfAdministration */ return new Property("routeOfAdministration", "", 1951 "The path by which the pharmaceutical product is taken into or makes contact with the body.", 0, 1952 java.lang.Integer.MAX_VALUE, routeOfAdministration); 1953 default: 1954 return super.getNamedProperty(_hash, _name, _checkValid); 1955 } 1956 1957 } 1958 1959 @Override 1960 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1961 switch (hash) { 1962 case -1618432855: 1963 /* identifier */ return this.identifier == null ? new Base[0] 1964 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1965 case 1446105202: 1966 /* administrableDoseForm */ return this.administrableDoseForm == null ? new Base[0] 1967 : new Base[] { this.administrableDoseForm }; // CodeableConcept 1968 case -1427765963: 1969 /* unitOfPresentation */ return this.unitOfPresentation == null ? new Base[0] 1970 : new Base[] { this.unitOfPresentation }; // CodeableConcept 1971 case -206409263: 1972 /* ingredient */ return this.ingredient == null ? new Base[0] 1973 : this.ingredient.toArray(new Base[this.ingredient.size()]); // Reference 1974 case -1335157162: 1975 /* device */ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 1976 case -1529171400: 1977 /* characteristics */ return this.characteristics == null ? new Base[0] 1978 : this.characteristics.toArray(new Base[this.characteristics.size()]); // MedicinalProductPharmaceuticalCharacteristicsComponent 1979 case 1742084734: 1980 /* routeOfAdministration */ return this.routeOfAdministration == null ? new Base[0] 1981 : this.routeOfAdministration.toArray(new Base[this.routeOfAdministration.size()]); // MedicinalProductPharmaceuticalRouteOfAdministrationComponent 1982 default: 1983 return super.getProperty(hash, name, checkValid); 1984 } 1985 1986 } 1987 1988 @Override 1989 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1990 switch (hash) { 1991 case -1618432855: // identifier 1992 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1993 return value; 1994 case 1446105202: // administrableDoseForm 1995 this.administrableDoseForm = castToCodeableConcept(value); // CodeableConcept 1996 return value; 1997 case -1427765963: // unitOfPresentation 1998 this.unitOfPresentation = castToCodeableConcept(value); // CodeableConcept 1999 return value; 2000 case -206409263: // ingredient 2001 this.getIngredient().add(castToReference(value)); // Reference 2002 return value; 2003 case -1335157162: // device 2004 this.getDevice().add(castToReference(value)); // Reference 2005 return value; 2006 case -1529171400: // characteristics 2007 this.getCharacteristics().add((MedicinalProductPharmaceuticalCharacteristicsComponent) value); // MedicinalProductPharmaceuticalCharacteristicsComponent 2008 return value; 2009 case 1742084734: // routeOfAdministration 2010 this.getRouteOfAdministration().add((MedicinalProductPharmaceuticalRouteOfAdministrationComponent) value); // MedicinalProductPharmaceuticalRouteOfAdministrationComponent 2011 return value; 2012 default: 2013 return super.setProperty(hash, name, value); 2014 } 2015 2016 } 2017 2018 @Override 2019 public Base setProperty(String name, Base value) throws FHIRException { 2020 if (name.equals("identifier")) { 2021 this.getIdentifier().add(castToIdentifier(value)); 2022 } else if (name.equals("administrableDoseForm")) { 2023 this.administrableDoseForm = castToCodeableConcept(value); // CodeableConcept 2024 } else if (name.equals("unitOfPresentation")) { 2025 this.unitOfPresentation = castToCodeableConcept(value); // CodeableConcept 2026 } else if (name.equals("ingredient")) { 2027 this.getIngredient().add(castToReference(value)); 2028 } else if (name.equals("device")) { 2029 this.getDevice().add(castToReference(value)); 2030 } else if (name.equals("characteristics")) { 2031 this.getCharacteristics().add((MedicinalProductPharmaceuticalCharacteristicsComponent) value); 2032 } else if (name.equals("routeOfAdministration")) { 2033 this.getRouteOfAdministration().add((MedicinalProductPharmaceuticalRouteOfAdministrationComponent) value); 2034 } else 2035 return super.setProperty(name, value); 2036 return value; 2037 } 2038 2039 @Override 2040 public void removeChild(String name, Base value) throws FHIRException { 2041 if (name.equals("identifier")) { 2042 this.getIdentifier().remove(castToIdentifier(value)); 2043 } else if (name.equals("administrableDoseForm")) { 2044 this.administrableDoseForm = null; 2045 } else if (name.equals("unitOfPresentation")) { 2046 this.unitOfPresentation = null; 2047 } else if (name.equals("ingredient")) { 2048 this.getIngredient().remove(castToReference(value)); 2049 } else if (name.equals("device")) { 2050 this.getDevice().remove(castToReference(value)); 2051 } else if (name.equals("characteristics")) { 2052 this.getCharacteristics().remove((MedicinalProductPharmaceuticalCharacteristicsComponent) value); 2053 } else if (name.equals("routeOfAdministration")) { 2054 this.getRouteOfAdministration().remove((MedicinalProductPharmaceuticalRouteOfAdministrationComponent) value); 2055 } else 2056 super.removeChild(name, value); 2057 2058 } 2059 2060 @Override 2061 public Base makeProperty(int hash, String name) throws FHIRException { 2062 switch (hash) { 2063 case -1618432855: 2064 return addIdentifier(); 2065 case 1446105202: 2066 return getAdministrableDoseForm(); 2067 case -1427765963: 2068 return getUnitOfPresentation(); 2069 case -206409263: 2070 return addIngredient(); 2071 case -1335157162: 2072 return addDevice(); 2073 case -1529171400: 2074 return addCharacteristics(); 2075 case 1742084734: 2076 return addRouteOfAdministration(); 2077 default: 2078 return super.makeProperty(hash, name); 2079 } 2080 2081 } 2082 2083 @Override 2084 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2085 switch (hash) { 2086 case -1618432855: 2087 /* identifier */ return new String[] { "Identifier" }; 2088 case 1446105202: 2089 /* administrableDoseForm */ return new String[] { "CodeableConcept" }; 2090 case -1427765963: 2091 /* unitOfPresentation */ return new String[] { "CodeableConcept" }; 2092 case -206409263: 2093 /* ingredient */ return new String[] { "Reference" }; 2094 case -1335157162: 2095 /* device */ return new String[] { "Reference" }; 2096 case -1529171400: 2097 /* characteristics */ return new String[] {}; 2098 case 1742084734: 2099 /* routeOfAdministration */ return new String[] {}; 2100 default: 2101 return super.getTypesForProperty(hash, name); 2102 } 2103 2104 } 2105 2106 @Override 2107 public Base addChild(String name) throws FHIRException { 2108 if (name.equals("identifier")) { 2109 return addIdentifier(); 2110 } else if (name.equals("administrableDoseForm")) { 2111 this.administrableDoseForm = new CodeableConcept(); 2112 return this.administrableDoseForm; 2113 } else if (name.equals("unitOfPresentation")) { 2114 this.unitOfPresentation = new CodeableConcept(); 2115 return this.unitOfPresentation; 2116 } else if (name.equals("ingredient")) { 2117 return addIngredient(); 2118 } else if (name.equals("device")) { 2119 return addDevice(); 2120 } else if (name.equals("characteristics")) { 2121 return addCharacteristics(); 2122 } else if (name.equals("routeOfAdministration")) { 2123 return addRouteOfAdministration(); 2124 } else 2125 return super.addChild(name); 2126 } 2127 2128 public String fhirType() { 2129 return "MedicinalProductPharmaceutical"; 2130 2131 } 2132 2133 public MedicinalProductPharmaceutical copy() { 2134 MedicinalProductPharmaceutical dst = new MedicinalProductPharmaceutical(); 2135 copyValues(dst); 2136 return dst; 2137 } 2138 2139 public void copyValues(MedicinalProductPharmaceutical dst) { 2140 super.copyValues(dst); 2141 if (identifier != null) { 2142 dst.identifier = new ArrayList<Identifier>(); 2143 for (Identifier i : identifier) 2144 dst.identifier.add(i.copy()); 2145 } 2146 ; 2147 dst.administrableDoseForm = administrableDoseForm == null ? null : administrableDoseForm.copy(); 2148 dst.unitOfPresentation = unitOfPresentation == null ? null : unitOfPresentation.copy(); 2149 if (ingredient != null) { 2150 dst.ingredient = new ArrayList<Reference>(); 2151 for (Reference i : ingredient) 2152 dst.ingredient.add(i.copy()); 2153 } 2154 ; 2155 if (device != null) { 2156 dst.device = new ArrayList<Reference>(); 2157 for (Reference i : device) 2158 dst.device.add(i.copy()); 2159 } 2160 ; 2161 if (characteristics != null) { 2162 dst.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 2163 for (MedicinalProductPharmaceuticalCharacteristicsComponent i : characteristics) 2164 dst.characteristics.add(i.copy()); 2165 } 2166 ; 2167 if (routeOfAdministration != null) { 2168 dst.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 2169 for (MedicinalProductPharmaceuticalRouteOfAdministrationComponent i : routeOfAdministration) 2170 dst.routeOfAdministration.add(i.copy()); 2171 } 2172 ; 2173 } 2174 2175 protected MedicinalProductPharmaceutical typedCopy() { 2176 return copy(); 2177 } 2178 2179 @Override 2180 public boolean equalsDeep(Base other_) { 2181 if (!super.equalsDeep(other_)) 2182 return false; 2183 if (!(other_ instanceof MedicinalProductPharmaceutical)) 2184 return false; 2185 MedicinalProductPharmaceutical o = (MedicinalProductPharmaceutical) other_; 2186 return compareDeep(identifier, o.identifier, true) 2187 && compareDeep(administrableDoseForm, o.administrableDoseForm, true) 2188 && compareDeep(unitOfPresentation, o.unitOfPresentation, true) && compareDeep(ingredient, o.ingredient, true) 2189 && compareDeep(device, o.device, true) && compareDeep(characteristics, o.characteristics, true) 2190 && compareDeep(routeOfAdministration, o.routeOfAdministration, true); 2191 } 2192 2193 @Override 2194 public boolean equalsShallow(Base other_) { 2195 if (!super.equalsShallow(other_)) 2196 return false; 2197 if (!(other_ instanceof MedicinalProductPharmaceutical)) 2198 return false; 2199 MedicinalProductPharmaceutical o = (MedicinalProductPharmaceutical) other_; 2200 return true; 2201 } 2202 2203 public boolean isEmpty() { 2204 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, administrableDoseForm, 2205 unitOfPresentation, ingredient, device, characteristics, routeOfAdministration); 2206 } 2207 2208 @Override 2209 public ResourceType getResourceType() { 2210 return ResourceType.MedicinalProductPharmaceutical; 2211 } 2212 2213 /** 2214 * Search parameter: <b>identifier</b> 2215 * <p> 2216 * Description: <b>An identifier for the pharmaceutical medicinal 2217 * product</b><br> 2218 * Type: <b>token</b><br> 2219 * Path: <b>MedicinalProductPharmaceutical.identifier</b><br> 2220 * </p> 2221 */ 2222 @SearchParamDefinition(name = "identifier", path = "MedicinalProductPharmaceutical.identifier", description = "An identifier for the pharmaceutical medicinal product", type = "token") 2223 public static final String SP_IDENTIFIER = "identifier"; 2224 /** 2225 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2226 * <p> 2227 * Description: <b>An identifier for the pharmaceutical medicinal 2228 * product</b><br> 2229 * Type: <b>token</b><br> 2230 * Path: <b>MedicinalProductPharmaceutical.identifier</b><br> 2231 * </p> 2232 */ 2233 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2234 SP_IDENTIFIER); 2235 2236 /** 2237 * Search parameter: <b>route</b> 2238 * <p> 2239 * Description: <b>Coded expression for the route</b><br> 2240 * Type: <b>token</b><br> 2241 * Path: <b>MedicinalProductPharmaceutical.routeOfAdministration.code</b><br> 2242 * </p> 2243 */ 2244 @SearchParamDefinition(name = "route", path = "MedicinalProductPharmaceutical.routeOfAdministration.code", description = "Coded expression for the route", type = "token") 2245 public static final String SP_ROUTE = "route"; 2246 /** 2247 * <b>Fluent Client</b> search parameter constant for <b>route</b> 2248 * <p> 2249 * Description: <b>Coded expression for the route</b><br> 2250 * Type: <b>token</b><br> 2251 * Path: <b>MedicinalProductPharmaceutical.routeOfAdministration.code</b><br> 2252 * </p> 2253 */ 2254 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2255 SP_ROUTE); 2256 2257 /** 2258 * Search parameter: <b>target-species</b> 2259 * <p> 2260 * Description: <b>Coded expression for the species</b><br> 2261 * Type: <b>token</b><br> 2262 * Path: 2263 * <b>MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.code</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name = "target-species", path = "MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.code", description = "Coded expression for the species", type = "token") 2267 public static final String SP_TARGET_SPECIES = "target-species"; 2268 /** 2269 * <b>Fluent Client</b> search parameter constant for <b>target-species</b> 2270 * <p> 2271 * Description: <b>Coded expression for the species</b><br> 2272 * Type: <b>token</b><br> 2273 * Path: 2274 * <b>MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.code</b><br> 2275 * </p> 2276 */ 2277 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_SPECIES = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2278 SP_TARGET_SPECIES); 2279 2280}