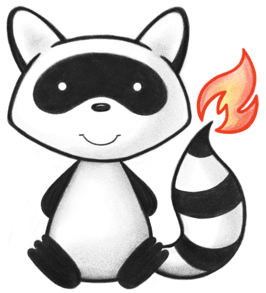
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * Defines the characteristics of a message that can be shared between systems, 052 * including the type of event that initiates the message, the content to be 053 * transmitted and what response(s), if any, are permitted. 054 */ 055@ResourceDef(name = "MessageDefinition", profile = "http://hl7.org/fhir/StructureDefinition/MessageDefinition") 056@ChildOrder(names = { "url", "identifier", "version", "name", "title", "replaces", "status", "experimental", "date", 057 "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "base", "parent", 058 "event[x]", "category", "focus", "responseRequired", "allowedResponse", "graph" }) 059public class MessageDefinition extends MetadataResource { 060 061 public enum MessageSignificanceCategory { 062 /** 063 * The message represents/requests a change that should not be processed more 064 * than once; e.g., making a booking for an appointment. 065 */ 066 CONSEQUENCE, 067 /** 068 * The message represents a response to query for current information. 069 * Retrospective processing is wrong and/or wasteful. 070 */ 071 CURRENCY, 072 /** 073 * The content is not necessarily intended to be current, and it can be 074 * reprocessed, though there may be version issues created by processing old 075 * notifications. 076 */ 077 NOTIFICATION, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("consequence".equals(codeString)) 087 return CONSEQUENCE; 088 if ("currency".equals(codeString)) 089 return CURRENCY; 090 if ("notification".equals(codeString)) 091 return NOTIFICATION; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case CONSEQUENCE: 101 return "consequence"; 102 case CURRENCY: 103 return "currency"; 104 case NOTIFICATION: 105 return "notification"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 switch (this) { 115 case CONSEQUENCE: 116 return "http://hl7.org/fhir/message-significance-category"; 117 case CURRENCY: 118 return "http://hl7.org/fhir/message-significance-category"; 119 case NOTIFICATION: 120 return "http://hl7.org/fhir/message-significance-category"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case CONSEQUENCE: 131 return "The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment."; 132 case CURRENCY: 133 return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 134 case NOTIFICATION: 135 return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case CONSEQUENCE: 146 return "Consequence"; 147 case CURRENCY: 148 return "Currency"; 149 case NOTIFICATION: 150 return "Notification"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 160 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("consequence".equals(codeString)) 165 return MessageSignificanceCategory.CONSEQUENCE; 166 if ("currency".equals(codeString)) 167 return MessageSignificanceCategory.CURRENCY; 168 if ("notification".equals(codeString)) 169 return MessageSignificanceCategory.NOTIFICATION; 170 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 171 } 172 173 public Enumeration<MessageSignificanceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 181 if ("consequence".equals(codeString)) 182 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE, code); 183 if ("currency".equals(codeString)) 184 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY, code); 185 if ("notification".equals(codeString)) 186 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION, code); 187 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 188 } 189 190 public String toCode(MessageSignificanceCategory code) { 191 if (code == MessageSignificanceCategory.NULL) 192 return null; 193 if (code == MessageSignificanceCategory.CONSEQUENCE) 194 return "consequence"; 195 if (code == MessageSignificanceCategory.CURRENCY) 196 return "currency"; 197 if (code == MessageSignificanceCategory.NOTIFICATION) 198 return "notification"; 199 return "?"; 200 } 201 202 public String toSystem(MessageSignificanceCategory code) { 203 return code.getSystem(); 204 } 205 } 206 207 public enum MessageheaderResponseRequest { 208 /** 209 * initiator expects a response for this message. 210 */ 211 ALWAYS, 212 /** 213 * initiator expects a response only if in error. 214 */ 215 ONERROR, 216 /** 217 * initiator does not expect a response. 218 */ 219 NEVER, 220 /** 221 * initiator expects a response only if successful. 222 */ 223 ONSUCCESS, 224 /** 225 * added to help the parsers with the generic types 226 */ 227 NULL; 228 229 public static MessageheaderResponseRequest fromCode(String codeString) throws FHIRException { 230 if (codeString == null || "".equals(codeString)) 231 return null; 232 if ("always".equals(codeString)) 233 return ALWAYS; 234 if ("on-error".equals(codeString)) 235 return ONERROR; 236 if ("never".equals(codeString)) 237 return NEVER; 238 if ("on-success".equals(codeString)) 239 return ONSUCCESS; 240 if (Configuration.isAcceptInvalidEnums()) 241 return null; 242 else 243 throw new FHIRException("Unknown MessageheaderResponseRequest code '" + codeString + "'"); 244 } 245 246 public String toCode() { 247 switch (this) { 248 case ALWAYS: 249 return "always"; 250 case ONERROR: 251 return "on-error"; 252 case NEVER: 253 return "never"; 254 case ONSUCCESS: 255 return "on-success"; 256 case NULL: 257 return null; 258 default: 259 return "?"; 260 } 261 } 262 263 public String getSystem() { 264 switch (this) { 265 case ALWAYS: 266 return "http://hl7.org/fhir/messageheader-response-request"; 267 case ONERROR: 268 return "http://hl7.org/fhir/messageheader-response-request"; 269 case NEVER: 270 return "http://hl7.org/fhir/messageheader-response-request"; 271 case ONSUCCESS: 272 return "http://hl7.org/fhir/messageheader-response-request"; 273 case NULL: 274 return null; 275 default: 276 return "?"; 277 } 278 } 279 280 public String getDefinition() { 281 switch (this) { 282 case ALWAYS: 283 return "initiator expects a response for this message."; 284 case ONERROR: 285 return "initiator expects a response only if in error."; 286 case NEVER: 287 return "initiator does not expect a response."; 288 case ONSUCCESS: 289 return "initiator expects a response only if successful."; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 297 public String getDisplay() { 298 switch (this) { 299 case ALWAYS: 300 return "Always"; 301 case ONERROR: 302 return "Error/reject conditions only"; 303 case NEVER: 304 return "Never"; 305 case ONSUCCESS: 306 return "Successful completion only"; 307 case NULL: 308 return null; 309 default: 310 return "?"; 311 } 312 } 313 } 314 315 public static class MessageheaderResponseRequestEnumFactory implements EnumFactory<MessageheaderResponseRequest> { 316 public MessageheaderResponseRequest fromCode(String codeString) throws IllegalArgumentException { 317 if (codeString == null || "".equals(codeString)) 318 if (codeString == null || "".equals(codeString)) 319 return null; 320 if ("always".equals(codeString)) 321 return MessageheaderResponseRequest.ALWAYS; 322 if ("on-error".equals(codeString)) 323 return MessageheaderResponseRequest.ONERROR; 324 if ("never".equals(codeString)) 325 return MessageheaderResponseRequest.NEVER; 326 if ("on-success".equals(codeString)) 327 return MessageheaderResponseRequest.ONSUCCESS; 328 throw new IllegalArgumentException("Unknown MessageheaderResponseRequest code '" + codeString + "'"); 329 } 330 331 public Enumeration<MessageheaderResponseRequest> fromType(PrimitiveType<?> code) throws FHIRException { 332 if (code == null) 333 return null; 334 if (code.isEmpty()) 335 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 336 String codeString = code.asStringValue(); 337 if (codeString == null || "".equals(codeString)) 338 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 339 if ("always".equals(codeString)) 340 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ALWAYS, code); 341 if ("on-error".equals(codeString)) 342 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONERROR, code); 343 if ("never".equals(codeString)) 344 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NEVER, code); 345 if ("on-success".equals(codeString)) 346 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONSUCCESS, code); 347 throw new FHIRException("Unknown MessageheaderResponseRequest code '" + codeString + "'"); 348 } 349 350 public String toCode(MessageheaderResponseRequest code) { 351 if (code == MessageheaderResponseRequest.NULL) 352 return null; 353 if (code == MessageheaderResponseRequest.ALWAYS) 354 return "always"; 355 if (code == MessageheaderResponseRequest.ONERROR) 356 return "on-error"; 357 if (code == MessageheaderResponseRequest.NEVER) 358 return "never"; 359 if (code == MessageheaderResponseRequest.ONSUCCESS) 360 return "on-success"; 361 return "?"; 362 } 363 364 public String toSystem(MessageheaderResponseRequest code) { 365 return code.getSystem(); 366 } 367 } 368 369 @Block() 370 public static class MessageDefinitionFocusComponent extends BackboneElement implements IBaseBackboneElement { 371 /** 372 * The kind of resource that must be the focus for this message. 373 */ 374 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 375 @Description(shortDefinition = "Type of resource", formalDefinition = "The kind of resource that must be the focus for this message.") 376 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 377 protected CodeType code; 378 379 /** 380 * A profile that reflects constraints for the focal resource (and potentially 381 * for related resources). 382 */ 383 @Child(name = "profile", type = { 384 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 385 @Description(shortDefinition = "Profile that must be adhered to by focus", formalDefinition = "A profile that reflects constraints for the focal resource (and potentially for related resources).") 386 protected CanonicalType profile; 387 388 /** 389 * Identifies the minimum number of resources of this type that must be pointed 390 * to by a message in order for it to be valid against this MessageDefinition. 391 */ 392 @Child(name = "min", type = { 393 UnsignedIntType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 394 @Description(shortDefinition = "Minimum number of focuses of this type", formalDefinition = "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.") 395 protected UnsignedIntType min; 396 397 /** 398 * Identifies the maximum number of resources of this type that must be pointed 399 * to by a message in order for it to be valid against this MessageDefinition. 400 */ 401 @Child(name = "max", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 402 @Description(shortDefinition = "Maximum number of focuses of this type", formalDefinition = "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.") 403 protected StringType max; 404 405 private static final long serialVersionUID = -68504836L; 406 407 /** 408 * Constructor 409 */ 410 public MessageDefinitionFocusComponent() { 411 super(); 412 } 413 414 /** 415 * Constructor 416 */ 417 public MessageDefinitionFocusComponent(CodeType code, UnsignedIntType min) { 418 super(); 419 this.code = code; 420 this.min = min; 421 } 422 423 /** 424 * @return {@link #code} (The kind of resource that must be the focus for this 425 * message.). This is the underlying object with id, value and 426 * extensions. The accessor "getCode" gives direct access to the value 427 */ 428 public CodeType getCodeElement() { 429 if (this.code == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.code"); 432 else if (Configuration.doAutoCreate()) 433 this.code = new CodeType(); // bb 434 return this.code; 435 } 436 437 public boolean hasCodeElement() { 438 return this.code != null && !this.code.isEmpty(); 439 } 440 441 public boolean hasCode() { 442 return this.code != null && !this.code.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #code} (The kind of resource that must be the focus for 447 * this message.). This is the underlying object with id, value and 448 * extensions. The accessor "getCode" gives direct access to the 449 * value 450 */ 451 public MessageDefinitionFocusComponent setCodeElement(CodeType value) { 452 this.code = value; 453 return this; 454 } 455 456 /** 457 * @return The kind of resource that must be the focus for this message. 458 */ 459 public String getCode() { 460 return this.code == null ? null : this.code.getValue(); 461 } 462 463 /** 464 * @param value The kind of resource that must be the focus for this message. 465 */ 466 public MessageDefinitionFocusComponent setCode(String value) { 467 if (this.code == null) 468 this.code = new CodeType(); 469 this.code.setValue(value); 470 return this; 471 } 472 473 /** 474 * @return {@link #profile} (A profile that reflects constraints for the focal 475 * resource (and potentially for related resources).). This is the 476 * underlying object with id, value and extensions. The accessor 477 * "getProfile" gives direct access to the value 478 */ 479 public CanonicalType getProfileElement() { 480 if (this.profile == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.profile"); 483 else if (Configuration.doAutoCreate()) 484 this.profile = new CanonicalType(); // bb 485 return this.profile; 486 } 487 488 public boolean hasProfileElement() { 489 return this.profile != null && !this.profile.isEmpty(); 490 } 491 492 public boolean hasProfile() { 493 return this.profile != null && !this.profile.isEmpty(); 494 } 495 496 /** 497 * @param value {@link #profile} (A profile that reflects constraints for the 498 * focal resource (and potentially for related resources).). This 499 * is the underlying object with id, value and extensions. The 500 * accessor "getProfile" gives direct access to the value 501 */ 502 public MessageDefinitionFocusComponent setProfileElement(CanonicalType value) { 503 this.profile = value; 504 return this; 505 } 506 507 /** 508 * @return A profile that reflects constraints for the focal resource (and 509 * potentially for related resources). 510 */ 511 public String getProfile() { 512 return this.profile == null ? null : this.profile.getValue(); 513 } 514 515 /** 516 * @param value A profile that reflects constraints for the focal resource (and 517 * potentially for related resources). 518 */ 519 public MessageDefinitionFocusComponent setProfile(String value) { 520 if (Utilities.noString(value)) 521 this.profile = null; 522 else { 523 if (this.profile == null) 524 this.profile = new CanonicalType(); 525 this.profile.setValue(value); 526 } 527 return this; 528 } 529 530 /** 531 * @return {@link #min} (Identifies the minimum number of resources of this type 532 * that must be pointed to by a message in order for it to be valid 533 * against this MessageDefinition.). This is the underlying object with 534 * id, value and extensions. The accessor "getMin" gives direct access 535 * to the value 536 */ 537 public UnsignedIntType getMinElement() { 538 if (this.min == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.min"); 541 else if (Configuration.doAutoCreate()) 542 this.min = new UnsignedIntType(); // bb 543 return this.min; 544 } 545 546 public boolean hasMinElement() { 547 return this.min != null && !this.min.isEmpty(); 548 } 549 550 public boolean hasMin() { 551 return this.min != null && !this.min.isEmpty(); 552 } 553 554 /** 555 * @param value {@link #min} (Identifies the minimum number of resources of this 556 * type that must be pointed to by a message in order for it to be 557 * valid against this MessageDefinition.). This is the underlying 558 * object with id, value and extensions. The accessor "getMin" 559 * gives direct access to the value 560 */ 561 public MessageDefinitionFocusComponent setMinElement(UnsignedIntType value) { 562 this.min = value; 563 return this; 564 } 565 566 /** 567 * @return Identifies the minimum number of resources of this type that must be 568 * pointed to by a message in order for it to be valid against this 569 * MessageDefinition. 570 */ 571 public int getMin() { 572 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 573 } 574 575 /** 576 * @param value Identifies the minimum number of resources of this type that 577 * must be pointed to by a message in order for it to be valid 578 * against this MessageDefinition. 579 */ 580 public MessageDefinitionFocusComponent setMin(int value) { 581 if (this.min == null) 582 this.min = new UnsignedIntType(); 583 this.min.setValue(value); 584 return this; 585 } 586 587 /** 588 * @return {@link #max} (Identifies the maximum number of resources of this type 589 * that must be pointed to by a message in order for it to be valid 590 * against this MessageDefinition.). This is the underlying object with 591 * id, value and extensions. The accessor "getMax" gives direct access 592 * to the value 593 */ 594 public StringType getMaxElement() { 595 if (this.max == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.max"); 598 else if (Configuration.doAutoCreate()) 599 this.max = new StringType(); // bb 600 return this.max; 601 } 602 603 public boolean hasMaxElement() { 604 return this.max != null && !this.max.isEmpty(); 605 } 606 607 public boolean hasMax() { 608 return this.max != null && !this.max.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #max} (Identifies the maximum number of resources of this 613 * type that must be pointed to by a message in order for it to be 614 * valid against this MessageDefinition.). This is the underlying 615 * object with id, value and extensions. The accessor "getMax" 616 * gives direct access to the value 617 */ 618 public MessageDefinitionFocusComponent setMaxElement(StringType value) { 619 this.max = value; 620 return this; 621 } 622 623 /** 624 * @return Identifies the maximum number of resources of this type that must be 625 * pointed to by a message in order for it to be valid against this 626 * MessageDefinition. 627 */ 628 public String getMax() { 629 return this.max == null ? null : this.max.getValue(); 630 } 631 632 /** 633 * @param value Identifies the maximum number of resources of this type that 634 * must be pointed to by a message in order for it to be valid 635 * against this MessageDefinition. 636 */ 637 public MessageDefinitionFocusComponent setMax(String value) { 638 if (Utilities.noString(value)) 639 this.max = null; 640 else { 641 if (this.max == null) 642 this.max = new StringType(); 643 this.max.setValue(value); 644 } 645 return this; 646 } 647 648 protected void listChildren(List<Property> children) { 649 super.listChildren(children); 650 children.add( 651 new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code)); 652 children.add(new Property("profile", "canonical(StructureDefinition)", 653 "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, 654 profile)); 655 children.add(new Property("min", "unsignedInt", 656 "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 657 0, 1, min)); 658 children.add(new Property("max", "string", 659 "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 660 0, 1, max)); 661 } 662 663 @Override 664 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 665 switch (_hash) { 666 case 3059181: 667 /* code */ return new Property("code", "code", "The kind of resource that must be the focus for this message.", 668 0, 1, code); 669 case -309425751: 670 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 671 "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, 672 profile); 673 case 108114: 674 /* min */ return new Property("min", "unsignedInt", 675 "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 676 0, 1, min); 677 case 107876: 678 /* max */ return new Property("max", "string", 679 "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 680 0, 1, max); 681 default: 682 return super.getNamedProperty(_hash, _name, _checkValid); 683 } 684 685 } 686 687 @Override 688 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 689 switch (hash) { 690 case 3059181: 691 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 692 case -309425751: 693 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 694 case 108114: 695 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // UnsignedIntType 696 case 107876: 697 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 698 default: 699 return super.getProperty(hash, name, checkValid); 700 } 701 702 } 703 704 @Override 705 public Base setProperty(int hash, String name, Base value) throws FHIRException { 706 switch (hash) { 707 case 3059181: // code 708 this.code = castToCode(value); // CodeType 709 return value; 710 case -309425751: // profile 711 this.profile = castToCanonical(value); // CanonicalType 712 return value; 713 case 108114: // min 714 this.min = castToUnsignedInt(value); // UnsignedIntType 715 return value; 716 case 107876: // max 717 this.max = castToString(value); // StringType 718 return value; 719 default: 720 return super.setProperty(hash, name, value); 721 } 722 723 } 724 725 @Override 726 public Base setProperty(String name, Base value) throws FHIRException { 727 if (name.equals("code")) { 728 this.code = castToCode(value); // CodeType 729 } else if (name.equals("profile")) { 730 this.profile = castToCanonical(value); // CanonicalType 731 } else if (name.equals("min")) { 732 this.min = castToUnsignedInt(value); // UnsignedIntType 733 } else if (name.equals("max")) { 734 this.max = castToString(value); // StringType 735 } else 736 return super.setProperty(name, value); 737 return value; 738 } 739 740 @Override 741 public void removeChild(String name, Base value) throws FHIRException { 742 if (name.equals("code")) { 743 this.code = null; 744 } else if (name.equals("profile")) { 745 this.profile = null; 746 } else if (name.equals("min")) { 747 this.min = null; 748 } else if (name.equals("max")) { 749 this.max = null; 750 } else 751 super.removeChild(name, value); 752 753 } 754 755 @Override 756 public Base makeProperty(int hash, String name) throws FHIRException { 757 switch (hash) { 758 case 3059181: 759 return getCodeElement(); 760 case -309425751: 761 return getProfileElement(); 762 case 108114: 763 return getMinElement(); 764 case 107876: 765 return getMaxElement(); 766 default: 767 return super.makeProperty(hash, name); 768 } 769 770 } 771 772 @Override 773 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 774 switch (hash) { 775 case 3059181: 776 /* code */ return new String[] { "code" }; 777 case -309425751: 778 /* profile */ return new String[] { "canonical" }; 779 case 108114: 780 /* min */ return new String[] { "unsignedInt" }; 781 case 107876: 782 /* max */ return new String[] { "string" }; 783 default: 784 return super.getTypesForProperty(hash, name); 785 } 786 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("code")) { 792 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.code"); 793 } else if (name.equals("profile")) { 794 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.profile"); 795 } else if (name.equals("min")) { 796 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.min"); 797 } else if (name.equals("max")) { 798 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.max"); 799 } else 800 return super.addChild(name); 801 } 802 803 public MessageDefinitionFocusComponent copy() { 804 MessageDefinitionFocusComponent dst = new MessageDefinitionFocusComponent(); 805 copyValues(dst); 806 return dst; 807 } 808 809 public void copyValues(MessageDefinitionFocusComponent dst) { 810 super.copyValues(dst); 811 dst.code = code == null ? null : code.copy(); 812 dst.profile = profile == null ? null : profile.copy(); 813 dst.min = min == null ? null : min.copy(); 814 dst.max = max == null ? null : max.copy(); 815 } 816 817 @Override 818 public boolean equalsDeep(Base other_) { 819 if (!super.equalsDeep(other_)) 820 return false; 821 if (!(other_ instanceof MessageDefinitionFocusComponent)) 822 return false; 823 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 824 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(min, o.min, true) 825 && compareDeep(max, o.max, true); 826 } 827 828 @Override 829 public boolean equalsShallow(Base other_) { 830 if (!super.equalsShallow(other_)) 831 return false; 832 if (!(other_ instanceof MessageDefinitionFocusComponent)) 833 return false; 834 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 835 return compareValues(code, o.code, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true); 836 } 837 838 public boolean isEmpty() { 839 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, min, max); 840 } 841 842 public String fhirType() { 843 return "MessageDefinition.focus"; 844 845 } 846 847 } 848 849 @Block() 850 public static class MessageDefinitionAllowedResponseComponent extends BackboneElement 851 implements IBaseBackboneElement { 852 /** 853 * A reference to the message definition that must be adhered to by this 854 * supported response. 855 */ 856 @Child(name = "message", type = { 857 CanonicalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 858 @Description(shortDefinition = "Reference to allowed message definition response", formalDefinition = "A reference to the message definition that must be adhered to by this supported response.") 859 protected CanonicalType message; 860 861 /** 862 * Provides a description of the circumstances in which this response should be 863 * used (as opposed to one of the alternative responses). 864 */ 865 @Child(name = "situation", type = { 866 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 867 @Description(shortDefinition = "When should this response be used", formalDefinition = "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).") 868 protected MarkdownType situation; 869 870 private static final long serialVersionUID = -1943810550L; 871 872 /** 873 * Constructor 874 */ 875 public MessageDefinitionAllowedResponseComponent() { 876 super(); 877 } 878 879 /** 880 * Constructor 881 */ 882 public MessageDefinitionAllowedResponseComponent(CanonicalType message) { 883 super(); 884 this.message = message; 885 } 886 887 /** 888 * @return {@link #message} (A reference to the message definition that must be 889 * adhered to by this supported response.). This is the underlying 890 * object with id, value and extensions. The accessor "getMessage" gives 891 * direct access to the value 892 */ 893 public CanonicalType getMessageElement() { 894 if (this.message == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.message"); 897 else if (Configuration.doAutoCreate()) 898 this.message = new CanonicalType(); // bb 899 return this.message; 900 } 901 902 public boolean hasMessageElement() { 903 return this.message != null && !this.message.isEmpty(); 904 } 905 906 public boolean hasMessage() { 907 return this.message != null && !this.message.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #message} (A reference to the message definition that 912 * must be adhered to by this supported response.). This is the 913 * underlying object with id, value and extensions. The accessor 914 * "getMessage" gives direct access to the value 915 */ 916 public MessageDefinitionAllowedResponseComponent setMessageElement(CanonicalType value) { 917 this.message = value; 918 return this; 919 } 920 921 /** 922 * @return A reference to the message definition that must be adhered to by this 923 * supported response. 924 */ 925 public String getMessage() { 926 return this.message == null ? null : this.message.getValue(); 927 } 928 929 /** 930 * @param value A reference to the message definition that must be adhered to by 931 * this supported response. 932 */ 933 public MessageDefinitionAllowedResponseComponent setMessage(String value) { 934 if (this.message == null) 935 this.message = new CanonicalType(); 936 this.message.setValue(value); 937 return this; 938 } 939 940 /** 941 * @return {@link #situation} (Provides a description of the circumstances in 942 * which this response should be used (as opposed to one of the 943 * alternative responses).). This is the underlying object with id, 944 * value and extensions. The accessor "getSituation" gives direct access 945 * to the value 946 */ 947 public MarkdownType getSituationElement() { 948 if (this.situation == null) 949 if (Configuration.errorOnAutoCreate()) 950 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.situation"); 951 else if (Configuration.doAutoCreate()) 952 this.situation = new MarkdownType(); // bb 953 return this.situation; 954 } 955 956 public boolean hasSituationElement() { 957 return this.situation != null && !this.situation.isEmpty(); 958 } 959 960 public boolean hasSituation() { 961 return this.situation != null && !this.situation.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #situation} (Provides a description of the circumstances 966 * in which this response should be used (as opposed to one of the 967 * alternative responses).). This is the underlying object with id, 968 * value and extensions. The accessor "getSituation" gives direct 969 * access to the value 970 */ 971 public MessageDefinitionAllowedResponseComponent setSituationElement(MarkdownType value) { 972 this.situation = value; 973 return this; 974 } 975 976 /** 977 * @return Provides a description of the circumstances in which this response 978 * should be used (as opposed to one of the alternative responses). 979 */ 980 public String getSituation() { 981 return this.situation == null ? null : this.situation.getValue(); 982 } 983 984 /** 985 * @param value Provides a description of the circumstances in which this 986 * response should be used (as opposed to one of the alternative 987 * responses). 988 */ 989 public MessageDefinitionAllowedResponseComponent setSituation(String value) { 990 if (value == null) 991 this.situation = null; 992 else { 993 if (this.situation == null) 994 this.situation = new MarkdownType(); 995 this.situation.setValue(value); 996 } 997 return this; 998 } 999 1000 protected void listChildren(List<Property> children) { 1001 super.listChildren(children); 1002 children.add(new Property("message", "canonical(MessageDefinition)", 1003 "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message)); 1004 children.add(new Property("situation", "markdown", 1005 "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 1006 0, 1, situation)); 1007 } 1008 1009 @Override 1010 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1011 switch (_hash) { 1012 case 954925063: 1013 /* message */ return new Property("message", "canonical(MessageDefinition)", 1014 "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message); 1015 case -73377282: 1016 /* situation */ return new Property("situation", "markdown", 1017 "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 1018 0, 1, situation); 1019 default: 1020 return super.getNamedProperty(_hash, _name, _checkValid); 1021 } 1022 1023 } 1024 1025 @Override 1026 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1027 switch (hash) { 1028 case 954925063: 1029 /* message */ return this.message == null ? new Base[0] : new Base[] { this.message }; // CanonicalType 1030 case -73377282: 1031 /* situation */ return this.situation == null ? new Base[0] : new Base[] { this.situation }; // MarkdownType 1032 default: 1033 return super.getProperty(hash, name, checkValid); 1034 } 1035 1036 } 1037 1038 @Override 1039 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1040 switch (hash) { 1041 case 954925063: // message 1042 this.message = castToCanonical(value); // CanonicalType 1043 return value; 1044 case -73377282: // situation 1045 this.situation = castToMarkdown(value); // MarkdownType 1046 return value; 1047 default: 1048 return super.setProperty(hash, name, value); 1049 } 1050 1051 } 1052 1053 @Override 1054 public Base setProperty(String name, Base value) throws FHIRException { 1055 if (name.equals("message")) { 1056 this.message = castToCanonical(value); // CanonicalType 1057 } else if (name.equals("situation")) { 1058 this.situation = castToMarkdown(value); // MarkdownType 1059 } else 1060 return super.setProperty(name, value); 1061 return value; 1062 } 1063 1064 @Override 1065 public void removeChild(String name, Base value) throws FHIRException { 1066 if (name.equals("message")) { 1067 this.message = null; 1068 } else if (name.equals("situation")) { 1069 this.situation = null; 1070 } else 1071 super.removeChild(name, value); 1072 1073 } 1074 1075 @Override 1076 public Base makeProperty(int hash, String name) throws FHIRException { 1077 switch (hash) { 1078 case 954925063: 1079 return getMessageElement(); 1080 case -73377282: 1081 return getSituationElement(); 1082 default: 1083 return super.makeProperty(hash, name); 1084 } 1085 1086 } 1087 1088 @Override 1089 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1090 switch (hash) { 1091 case 954925063: 1092 /* message */ return new String[] { "canonical" }; 1093 case -73377282: 1094 /* situation */ return new String[] { "markdown" }; 1095 default: 1096 return super.getTypesForProperty(hash, name); 1097 } 1098 1099 } 1100 1101 @Override 1102 public Base addChild(String name) throws FHIRException { 1103 if (name.equals("message")) { 1104 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.message"); 1105 } else if (name.equals("situation")) { 1106 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.situation"); 1107 } else 1108 return super.addChild(name); 1109 } 1110 1111 public MessageDefinitionAllowedResponseComponent copy() { 1112 MessageDefinitionAllowedResponseComponent dst = new MessageDefinitionAllowedResponseComponent(); 1113 copyValues(dst); 1114 return dst; 1115 } 1116 1117 public void copyValues(MessageDefinitionAllowedResponseComponent dst) { 1118 super.copyValues(dst); 1119 dst.message = message == null ? null : message.copy(); 1120 dst.situation = situation == null ? null : situation.copy(); 1121 } 1122 1123 @Override 1124 public boolean equalsDeep(Base other_) { 1125 if (!super.equalsDeep(other_)) 1126 return false; 1127 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 1128 return false; 1129 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 1130 return compareDeep(message, o.message, true) && compareDeep(situation, o.situation, true); 1131 } 1132 1133 @Override 1134 public boolean equalsShallow(Base other_) { 1135 if (!super.equalsShallow(other_)) 1136 return false; 1137 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 1138 return false; 1139 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 1140 return compareValues(situation, o.situation, true); 1141 } 1142 1143 public boolean isEmpty() { 1144 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(message, situation); 1145 } 1146 1147 public String fhirType() { 1148 return "MessageDefinition.allowedResponse"; 1149 1150 } 1151 1152 } 1153 1154 /** 1155 * A formal identifier that is used to identify this message definition when it 1156 * is represented in other formats, or referenced in a specification, model, 1157 * design or an instance. 1158 */ 1159 @Child(name = "identifier", type = { 1160 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1161 @Description(shortDefinition = "Primary key for the message definition on a given server", formalDefinition = "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1162 protected List<Identifier> identifier; 1163 1164 /** 1165 * A MessageDefinition that is superseded by this definition. 1166 */ 1167 @Child(name = "replaces", type = { 1168 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1169 @Description(shortDefinition = "Takes the place of", formalDefinition = "A MessageDefinition that is superseded by this definition.") 1170 protected List<CanonicalType> replaces; 1171 1172 /** 1173 * Explanation of why this message definition is needed and why it has been 1174 * designed as it has. 1175 */ 1176 @Child(name = "purpose", type = { MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1177 @Description(shortDefinition = "Why this message definition is defined", formalDefinition = "Explanation of why this message definition is needed and why it has been designed as it has.") 1178 protected MarkdownType purpose; 1179 1180 /** 1181 * A copyright statement relating to the message definition and/or its contents. 1182 * Copyright statements are generally legal restrictions on the use and 1183 * publishing of the message definition. 1184 */ 1185 @Child(name = "copyright", type = { 1186 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1187 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.") 1188 protected MarkdownType copyright; 1189 1190 /** 1191 * The MessageDefinition that is the basis for the contents of this resource. 1192 */ 1193 @Child(name = "base", type = { CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1194 @Description(shortDefinition = "Definition this one is based on", formalDefinition = "The MessageDefinition that is the basis for the contents of this resource.") 1195 protected CanonicalType base; 1196 1197 /** 1198 * Identifies a protocol or workflow that this MessageDefinition represents a 1199 * step in. 1200 */ 1201 @Child(name = "parent", type = { 1202 CanonicalType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1203 @Description(shortDefinition = "Protocol/workflow this is part of", formalDefinition = "Identifies a protocol or workflow that this MessageDefinition represents a step in.") 1204 protected List<CanonicalType> parent; 1205 1206 /** 1207 * Event code or link to the EventDefinition. 1208 */ 1209 @Child(name = "event", type = { Coding.class, 1210 UriType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1211 @Description(shortDefinition = "Event code or link to the EventDefinition", formalDefinition = "Event code or link to the EventDefinition.") 1212 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-events") 1213 protected Type event; 1214 1215 /** 1216 * The impact of the content of the message. 1217 */ 1218 @Child(name = "category", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1219 @Description(shortDefinition = "consequence | currency | notification", formalDefinition = "The impact of the content of the message.") 1220 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-significance-category") 1221 protected Enumeration<MessageSignificanceCategory> category; 1222 1223 /** 1224 * Identifies the resource (or resources) that are being addressed by the event. 1225 * For example, the Encounter for an admit message or two Account records for a 1226 * merge. 1227 */ 1228 @Child(name = "focus", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1229 @Description(shortDefinition = "Resource(s) that are the subject of the event", formalDefinition = "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.") 1230 protected List<MessageDefinitionFocusComponent> focus; 1231 1232 /** 1233 * Declare at a message definition level whether a response is required or only 1234 * upon error or success, or never. 1235 */ 1236 @Child(name = "responseRequired", type = { 1237 CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1238 @Description(shortDefinition = "always | on-error | never | on-success", formalDefinition = "Declare at a message definition level whether a response is required or only upon error or success, or never.") 1239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/messageheader-response-request") 1240 protected Enumeration<MessageheaderResponseRequest> responseRequired; 1241 1242 /** 1243 * Indicates what types of messages may be sent as an application-level response 1244 * to this message. 1245 */ 1246 @Child(name = "allowedResponse", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1247 @Description(shortDefinition = "Responses to this message", formalDefinition = "Indicates what types of messages may be sent as an application-level response to this message.") 1248 protected List<MessageDefinitionAllowedResponseComponent> allowedResponse; 1249 1250 /** 1251 * Canonical reference to a GraphDefinition. If a URL is provided, it is the 1252 * canonical reference to a [[[GraphDefinition]]] that it controls what 1253 * resources are to be added to the bundle when building the document. The 1254 * GraphDefinition can also specify profiles that apply to the various 1255 * resources. 1256 */ 1257 @Child(name = "graph", type = { 1258 CanonicalType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1259 @Description(shortDefinition = "Canonical reference to a GraphDefinition", formalDefinition = "Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a [[[GraphDefinition]]] that it controls what resources are to be added to the bundle when building the document. The GraphDefinition can also specify profiles that apply to the various resources.") 1260 protected List<CanonicalType> graph; 1261 1262 private static final long serialVersionUID = 927775347L; 1263 1264 /** 1265 * Constructor 1266 */ 1267 public MessageDefinition() { 1268 super(); 1269 } 1270 1271 /** 1272 * Constructor 1273 */ 1274 public MessageDefinition(Enumeration<PublicationStatus> status, DateTimeType date, Type event) { 1275 super(); 1276 this.status = status; 1277 this.date = date; 1278 this.event = event; 1279 } 1280 1281 /** 1282 * @return {@link #url} (The business identifier that is used to reference the 1283 * MessageDefinition and *is* expected to be consistent from server to 1284 * server.). This is the underlying object with id, value and 1285 * extensions. The accessor "getUrl" gives direct access to the value 1286 */ 1287 public UriType getUrlElement() { 1288 if (this.url == null) 1289 if (Configuration.errorOnAutoCreate()) 1290 throw new Error("Attempt to auto-create MessageDefinition.url"); 1291 else if (Configuration.doAutoCreate()) 1292 this.url = new UriType(); // bb 1293 return this.url; 1294 } 1295 1296 public boolean hasUrlElement() { 1297 return this.url != null && !this.url.isEmpty(); 1298 } 1299 1300 public boolean hasUrl() { 1301 return this.url != null && !this.url.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #url} (The business identifier that is used to reference 1306 * the MessageDefinition and *is* expected to be consistent from 1307 * server to server.). This is the underlying object with id, value 1308 * and extensions. The accessor "getUrl" gives direct access to the 1309 * value 1310 */ 1311 public MessageDefinition setUrlElement(UriType value) { 1312 this.url = value; 1313 return this; 1314 } 1315 1316 /** 1317 * @return The business identifier that is used to reference the 1318 * MessageDefinition and *is* expected to be consistent from server to 1319 * server. 1320 */ 1321 public String getUrl() { 1322 return this.url == null ? null : this.url.getValue(); 1323 } 1324 1325 /** 1326 * @param value The business identifier that is used to reference the 1327 * MessageDefinition and *is* expected to be consistent from server 1328 * to server. 1329 */ 1330 public MessageDefinition setUrl(String value) { 1331 if (Utilities.noString(value)) 1332 this.url = null; 1333 else { 1334 if (this.url == null) 1335 this.url = new UriType(); 1336 this.url.setValue(value); 1337 } 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #identifier} (A formal identifier that is used to identify 1343 * this message definition when it is represented in other formats, or 1344 * referenced in a specification, model, design or an instance.) 1345 */ 1346 public List<Identifier> getIdentifier() { 1347 if (this.identifier == null) 1348 this.identifier = new ArrayList<Identifier>(); 1349 return this.identifier; 1350 } 1351 1352 /** 1353 * @return Returns a reference to <code>this</code> for easy method chaining 1354 */ 1355 public MessageDefinition setIdentifier(List<Identifier> theIdentifier) { 1356 this.identifier = theIdentifier; 1357 return this; 1358 } 1359 1360 public boolean hasIdentifier() { 1361 if (this.identifier == null) 1362 return false; 1363 for (Identifier item : this.identifier) 1364 if (!item.isEmpty()) 1365 return true; 1366 return false; 1367 } 1368 1369 public Identifier addIdentifier() { // 3 1370 Identifier t = new Identifier(); 1371 if (this.identifier == null) 1372 this.identifier = new ArrayList<Identifier>(); 1373 this.identifier.add(t); 1374 return t; 1375 } 1376 1377 public MessageDefinition addIdentifier(Identifier t) { // 3 1378 if (t == null) 1379 return this; 1380 if (this.identifier == null) 1381 this.identifier = new ArrayList<Identifier>(); 1382 this.identifier.add(t); 1383 return this; 1384 } 1385 1386 /** 1387 * @return The first repetition of repeating field {@link #identifier}, creating 1388 * it if it does not already exist 1389 */ 1390 public Identifier getIdentifierFirstRep() { 1391 if (getIdentifier().isEmpty()) { 1392 addIdentifier(); 1393 } 1394 return getIdentifier().get(0); 1395 } 1396 1397 /** 1398 * @return {@link #version} (The identifier that is used to identify this 1399 * version of the message definition when it is referenced in a 1400 * specification, model, design or instance. This is an arbitrary value 1401 * managed by the message definition author and is not expected to be 1402 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 1403 * if a managed version is not available. There is also no expectation 1404 * that versions can be placed in a lexicographical sequence.). This is 1405 * the underlying object with id, value and extensions. The accessor 1406 * "getVersion" gives direct access to the value 1407 */ 1408 public StringType getVersionElement() { 1409 if (this.version == null) 1410 if (Configuration.errorOnAutoCreate()) 1411 throw new Error("Attempt to auto-create MessageDefinition.version"); 1412 else if (Configuration.doAutoCreate()) 1413 this.version = new StringType(); // bb 1414 return this.version; 1415 } 1416 1417 public boolean hasVersionElement() { 1418 return this.version != null && !this.version.isEmpty(); 1419 } 1420 1421 public boolean hasVersion() { 1422 return this.version != null && !this.version.isEmpty(); 1423 } 1424 1425 /** 1426 * @param value {@link #version} (The identifier that is used to identify this 1427 * version of the message definition when it is referenced in a 1428 * specification, model, design or instance. This is an arbitrary 1429 * value managed by the message definition author and is not 1430 * expected to be globally unique. For example, it might be a 1431 * timestamp (e.g. yyyymmdd) if a managed version is not available. 1432 * There is also no expectation that versions can be placed in a 1433 * lexicographical sequence.). This is the underlying object with 1434 * id, value and extensions. The accessor "getVersion" gives direct 1435 * access to the value 1436 */ 1437 public MessageDefinition setVersionElement(StringType value) { 1438 this.version = value; 1439 return this; 1440 } 1441 1442 /** 1443 * @return The identifier that is used to identify this version of the message 1444 * definition when it is referenced in a specification, model, design or 1445 * instance. This is an arbitrary value managed by the message 1446 * definition author and is not expected to be globally unique. For 1447 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 1448 * is not available. There is also no expectation that versions can be 1449 * placed in a lexicographical sequence. 1450 */ 1451 public String getVersion() { 1452 return this.version == null ? null : this.version.getValue(); 1453 } 1454 1455 /** 1456 * @param value The identifier that is used to identify this version of the 1457 * message definition when it is referenced in a specification, 1458 * model, design or instance. This is an arbitrary value managed by 1459 * the message definition author and is not expected to be globally 1460 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 1461 * a managed version is not available. There is also no expectation 1462 * that versions can be placed in a lexicographical sequence. 1463 */ 1464 public MessageDefinition setVersion(String value) { 1465 if (Utilities.noString(value)) 1466 this.version = null; 1467 else { 1468 if (this.version == null) 1469 this.version = new StringType(); 1470 this.version.setValue(value); 1471 } 1472 return this; 1473 } 1474 1475 /** 1476 * @return {@link #name} (A natural language name identifying the message 1477 * definition. This name should be usable as an identifier for the 1478 * module by machine processing applications such as code generation.). 1479 * This is the underlying object with id, value and extensions. The 1480 * accessor "getName" gives direct access to the value 1481 */ 1482 public StringType getNameElement() { 1483 if (this.name == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create MessageDefinition.name"); 1486 else if (Configuration.doAutoCreate()) 1487 this.name = new StringType(); // bb 1488 return this.name; 1489 } 1490 1491 public boolean hasNameElement() { 1492 return this.name != null && !this.name.isEmpty(); 1493 } 1494 1495 public boolean hasName() { 1496 return this.name != null && !this.name.isEmpty(); 1497 } 1498 1499 /** 1500 * @param value {@link #name} (A natural language name identifying the message 1501 * definition. This name should be usable as an identifier for the 1502 * module by machine processing applications such as code 1503 * generation.). This is the underlying object with id, value and 1504 * extensions. The accessor "getName" gives direct access to the 1505 * value 1506 */ 1507 public MessageDefinition setNameElement(StringType value) { 1508 this.name = value; 1509 return this; 1510 } 1511 1512 /** 1513 * @return A natural language name identifying the message definition. This name 1514 * should be usable as an identifier for the module by machine 1515 * processing applications such as code generation. 1516 */ 1517 public String getName() { 1518 return this.name == null ? null : this.name.getValue(); 1519 } 1520 1521 /** 1522 * @param value A natural language name identifying the message definition. This 1523 * name should be usable as an identifier for the module by machine 1524 * processing applications such as code generation. 1525 */ 1526 public MessageDefinition setName(String value) { 1527 if (Utilities.noString(value)) 1528 this.name = null; 1529 else { 1530 if (this.name == null) 1531 this.name = new StringType(); 1532 this.name.setValue(value); 1533 } 1534 return this; 1535 } 1536 1537 /** 1538 * @return {@link #title} (A short, descriptive, user-friendly title for the 1539 * message definition.). This is the underlying object with id, value 1540 * and extensions. The accessor "getTitle" gives direct access to the 1541 * value 1542 */ 1543 public StringType getTitleElement() { 1544 if (this.title == null) 1545 if (Configuration.errorOnAutoCreate()) 1546 throw new Error("Attempt to auto-create MessageDefinition.title"); 1547 else if (Configuration.doAutoCreate()) 1548 this.title = new StringType(); // bb 1549 return this.title; 1550 } 1551 1552 public boolean hasTitleElement() { 1553 return this.title != null && !this.title.isEmpty(); 1554 } 1555 1556 public boolean hasTitle() { 1557 return this.title != null && !this.title.isEmpty(); 1558 } 1559 1560 /** 1561 * @param value {@link #title} (A short, descriptive, user-friendly title for 1562 * the message definition.). This is the underlying object with id, 1563 * value and extensions. The accessor "getTitle" gives direct 1564 * access to the value 1565 */ 1566 public MessageDefinition setTitleElement(StringType value) { 1567 this.title = value; 1568 return this; 1569 } 1570 1571 /** 1572 * @return A short, descriptive, user-friendly title for the message definition. 1573 */ 1574 public String getTitle() { 1575 return this.title == null ? null : this.title.getValue(); 1576 } 1577 1578 /** 1579 * @param value A short, descriptive, user-friendly title for the message 1580 * definition. 1581 */ 1582 public MessageDefinition setTitle(String value) { 1583 if (Utilities.noString(value)) 1584 this.title = null; 1585 else { 1586 if (this.title == null) 1587 this.title = new StringType(); 1588 this.title.setValue(value); 1589 } 1590 return this; 1591 } 1592 1593 /** 1594 * @return {@link #replaces} (A MessageDefinition that is superseded by this 1595 * definition.) 1596 */ 1597 public List<CanonicalType> getReplaces() { 1598 if (this.replaces == null) 1599 this.replaces = new ArrayList<CanonicalType>(); 1600 return this.replaces; 1601 } 1602 1603 /** 1604 * @return Returns a reference to <code>this</code> for easy method chaining 1605 */ 1606 public MessageDefinition setReplaces(List<CanonicalType> theReplaces) { 1607 this.replaces = theReplaces; 1608 return this; 1609 } 1610 1611 public boolean hasReplaces() { 1612 if (this.replaces == null) 1613 return false; 1614 for (CanonicalType item : this.replaces) 1615 if (!item.isEmpty()) 1616 return true; 1617 return false; 1618 } 1619 1620 /** 1621 * @return {@link #replaces} (A MessageDefinition that is superseded by this 1622 * definition.) 1623 */ 1624 public CanonicalType addReplacesElement() {// 2 1625 CanonicalType t = new CanonicalType(); 1626 if (this.replaces == null) 1627 this.replaces = new ArrayList<CanonicalType>(); 1628 this.replaces.add(t); 1629 return t; 1630 } 1631 1632 /** 1633 * @param value {@link #replaces} (A MessageDefinition that is superseded by 1634 * this definition.) 1635 */ 1636 public MessageDefinition addReplaces(String value) { // 1 1637 CanonicalType t = new CanonicalType(); 1638 t.setValue(value); 1639 if (this.replaces == null) 1640 this.replaces = new ArrayList<CanonicalType>(); 1641 this.replaces.add(t); 1642 return this; 1643 } 1644 1645 /** 1646 * @param value {@link #replaces} (A MessageDefinition that is superseded by 1647 * this definition.) 1648 */ 1649 public boolean hasReplaces(String value) { 1650 if (this.replaces == null) 1651 return false; 1652 for (CanonicalType v : this.replaces) 1653 if (v.getValue().equals(value)) // canonical(MessageDefinition) 1654 return true; 1655 return false; 1656 } 1657 1658 /** 1659 * @return {@link #status} (The status of this message definition. Enables 1660 * tracking the life-cycle of the content.). This is the underlying 1661 * object with id, value and extensions. The accessor "getStatus" gives 1662 * direct access to the value 1663 */ 1664 public Enumeration<PublicationStatus> getStatusElement() { 1665 if (this.status == null) 1666 if (Configuration.errorOnAutoCreate()) 1667 throw new Error("Attempt to auto-create MessageDefinition.status"); 1668 else if (Configuration.doAutoCreate()) 1669 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1670 return this.status; 1671 } 1672 1673 public boolean hasStatusElement() { 1674 return this.status != null && !this.status.isEmpty(); 1675 } 1676 1677 public boolean hasStatus() { 1678 return this.status != null && !this.status.isEmpty(); 1679 } 1680 1681 /** 1682 * @param value {@link #status} (The status of this message definition. Enables 1683 * tracking the life-cycle of the content.). This is the underlying 1684 * object with id, value and extensions. The accessor "getStatus" 1685 * gives direct access to the value 1686 */ 1687 public MessageDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1688 this.status = value; 1689 return this; 1690 } 1691 1692 /** 1693 * @return The status of this message definition. Enables tracking the 1694 * life-cycle of the content. 1695 */ 1696 public PublicationStatus getStatus() { 1697 return this.status == null ? null : this.status.getValue(); 1698 } 1699 1700 /** 1701 * @param value The status of this message definition. Enables tracking the 1702 * life-cycle of the content. 1703 */ 1704 public MessageDefinition setStatus(PublicationStatus value) { 1705 if (this.status == null) 1706 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1707 this.status.setValue(value); 1708 return this; 1709 } 1710 1711 /** 1712 * @return {@link #experimental} (A Boolean value to indicate that this message 1713 * definition is authored for testing purposes (or 1714 * education/evaluation/marketing) and is not intended to be used for 1715 * genuine usage.). This is the underlying object with id, value and 1716 * extensions. The accessor "getExperimental" gives direct access to the 1717 * value 1718 */ 1719 public BooleanType getExperimentalElement() { 1720 if (this.experimental == null) 1721 if (Configuration.errorOnAutoCreate()) 1722 throw new Error("Attempt to auto-create MessageDefinition.experimental"); 1723 else if (Configuration.doAutoCreate()) 1724 this.experimental = new BooleanType(); // bb 1725 return this.experimental; 1726 } 1727 1728 public boolean hasExperimentalElement() { 1729 return this.experimental != null && !this.experimental.isEmpty(); 1730 } 1731 1732 public boolean hasExperimental() { 1733 return this.experimental != null && !this.experimental.isEmpty(); 1734 } 1735 1736 /** 1737 * @param value {@link #experimental} (A Boolean value to indicate that this 1738 * message definition is authored for testing purposes (or 1739 * education/evaluation/marketing) and is not intended to be used 1740 * for genuine usage.). This is the underlying object with id, 1741 * value and extensions. The accessor "getExperimental" gives 1742 * direct access to the value 1743 */ 1744 public MessageDefinition setExperimentalElement(BooleanType value) { 1745 this.experimental = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return A Boolean value to indicate that this message definition is authored 1751 * for testing purposes (or education/evaluation/marketing) and is not 1752 * intended to be used for genuine usage. 1753 */ 1754 public boolean getExperimental() { 1755 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1756 } 1757 1758 /** 1759 * @param value A Boolean value to indicate that this message definition is 1760 * authored for testing purposes (or 1761 * education/evaluation/marketing) and is not intended to be used 1762 * for genuine usage. 1763 */ 1764 public MessageDefinition setExperimental(boolean value) { 1765 if (this.experimental == null) 1766 this.experimental = new BooleanType(); 1767 this.experimental.setValue(value); 1768 return this; 1769 } 1770 1771 /** 1772 * @return {@link #date} (The date (and optionally time) when the message 1773 * definition was published. The date must change when the business 1774 * version changes and it must change if the status code changes. In 1775 * addition, it should change when the substantive content of the 1776 * message definition changes.). This is the underlying object with id, 1777 * value and extensions. The accessor "getDate" gives direct access to 1778 * the value 1779 */ 1780 public DateTimeType getDateElement() { 1781 if (this.date == null) 1782 if (Configuration.errorOnAutoCreate()) 1783 throw new Error("Attempt to auto-create MessageDefinition.date"); 1784 else if (Configuration.doAutoCreate()) 1785 this.date = new DateTimeType(); // bb 1786 return this.date; 1787 } 1788 1789 public boolean hasDateElement() { 1790 return this.date != null && !this.date.isEmpty(); 1791 } 1792 1793 public boolean hasDate() { 1794 return this.date != null && !this.date.isEmpty(); 1795 } 1796 1797 /** 1798 * @param value {@link #date} (The date (and optionally time) when the message 1799 * definition was published. The date must change when the business 1800 * version changes and it must change if the status code changes. 1801 * In addition, it should change when the substantive content of 1802 * the message definition changes.). This is the underlying object 1803 * with id, value and extensions. The accessor "getDate" gives 1804 * direct access to the value 1805 */ 1806 public MessageDefinition setDateElement(DateTimeType value) { 1807 this.date = value; 1808 return this; 1809 } 1810 1811 /** 1812 * @return The date (and optionally time) when the message definition was 1813 * published. The date must change when the business version changes and 1814 * it must change if the status code changes. In addition, it should 1815 * change when the substantive content of the message definition 1816 * changes. 1817 */ 1818 public Date getDate() { 1819 return this.date == null ? null : this.date.getValue(); 1820 } 1821 1822 /** 1823 * @param value The date (and optionally time) when the message definition was 1824 * published. The date must change when the business version 1825 * changes and it must change if the status code changes. In 1826 * addition, it should change when the substantive content of the 1827 * message definition changes. 1828 */ 1829 public MessageDefinition setDate(Date value) { 1830 if (this.date == null) 1831 this.date = new DateTimeType(); 1832 this.date.setValue(value); 1833 return this; 1834 } 1835 1836 /** 1837 * @return {@link #publisher} (The name of the organization or individual that 1838 * published the message definition.). This is the underlying object 1839 * with id, value and extensions. The accessor "getPublisher" gives 1840 * direct access to the value 1841 */ 1842 public StringType getPublisherElement() { 1843 if (this.publisher == null) 1844 if (Configuration.errorOnAutoCreate()) 1845 throw new Error("Attempt to auto-create MessageDefinition.publisher"); 1846 else if (Configuration.doAutoCreate()) 1847 this.publisher = new StringType(); // bb 1848 return this.publisher; 1849 } 1850 1851 public boolean hasPublisherElement() { 1852 return this.publisher != null && !this.publisher.isEmpty(); 1853 } 1854 1855 public boolean hasPublisher() { 1856 return this.publisher != null && !this.publisher.isEmpty(); 1857 } 1858 1859 /** 1860 * @param value {@link #publisher} (The name of the organization or individual 1861 * that published the message definition.). This is the underlying 1862 * object with id, value and extensions. The accessor 1863 * "getPublisher" gives direct access to the value 1864 */ 1865 public MessageDefinition setPublisherElement(StringType value) { 1866 this.publisher = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return The name of the organization or individual that published the message 1872 * definition. 1873 */ 1874 public String getPublisher() { 1875 return this.publisher == null ? null : this.publisher.getValue(); 1876 } 1877 1878 /** 1879 * @param value The name of the organization or individual that published the 1880 * message definition. 1881 */ 1882 public MessageDefinition setPublisher(String value) { 1883 if (Utilities.noString(value)) 1884 this.publisher = null; 1885 else { 1886 if (this.publisher == null) 1887 this.publisher = new StringType(); 1888 this.publisher.setValue(value); 1889 } 1890 return this; 1891 } 1892 1893 /** 1894 * @return {@link #contact} (Contact details to assist a user in finding and 1895 * communicating with the publisher.) 1896 */ 1897 public List<ContactDetail> getContact() { 1898 if (this.contact == null) 1899 this.contact = new ArrayList<ContactDetail>(); 1900 return this.contact; 1901 } 1902 1903 /** 1904 * @return Returns a reference to <code>this</code> for easy method chaining 1905 */ 1906 public MessageDefinition setContact(List<ContactDetail> theContact) { 1907 this.contact = theContact; 1908 return this; 1909 } 1910 1911 public boolean hasContact() { 1912 if (this.contact == null) 1913 return false; 1914 for (ContactDetail item : this.contact) 1915 if (!item.isEmpty()) 1916 return true; 1917 return false; 1918 } 1919 1920 public ContactDetail addContact() { // 3 1921 ContactDetail t = new ContactDetail(); 1922 if (this.contact == null) 1923 this.contact = new ArrayList<ContactDetail>(); 1924 this.contact.add(t); 1925 return t; 1926 } 1927 1928 public MessageDefinition addContact(ContactDetail t) { // 3 1929 if (t == null) 1930 return this; 1931 if (this.contact == null) 1932 this.contact = new ArrayList<ContactDetail>(); 1933 this.contact.add(t); 1934 return this; 1935 } 1936 1937 /** 1938 * @return The first repetition of repeating field {@link #contact}, creating it 1939 * if it does not already exist 1940 */ 1941 public ContactDetail getContactFirstRep() { 1942 if (getContact().isEmpty()) { 1943 addContact(); 1944 } 1945 return getContact().get(0); 1946 } 1947 1948 /** 1949 * @return {@link #description} (A free text natural language description of the 1950 * message definition from a consumer's perspective.). This is the 1951 * underlying object with id, value and extensions. The accessor 1952 * "getDescription" gives direct access to the value 1953 */ 1954 public MarkdownType getDescriptionElement() { 1955 if (this.description == null) 1956 if (Configuration.errorOnAutoCreate()) 1957 throw new Error("Attempt to auto-create MessageDefinition.description"); 1958 else if (Configuration.doAutoCreate()) 1959 this.description = new MarkdownType(); // bb 1960 return this.description; 1961 } 1962 1963 public boolean hasDescriptionElement() { 1964 return this.description != null && !this.description.isEmpty(); 1965 } 1966 1967 public boolean hasDescription() { 1968 return this.description != null && !this.description.isEmpty(); 1969 } 1970 1971 /** 1972 * @param value {@link #description} (A free text natural language description 1973 * of the message definition from a consumer's perspective.). This 1974 * is the underlying object with id, value and extensions. The 1975 * accessor "getDescription" gives direct access to the value 1976 */ 1977 public MessageDefinition setDescriptionElement(MarkdownType value) { 1978 this.description = value; 1979 return this; 1980 } 1981 1982 /** 1983 * @return A free text natural language description of the message definition 1984 * from a consumer's perspective. 1985 */ 1986 public String getDescription() { 1987 return this.description == null ? null : this.description.getValue(); 1988 } 1989 1990 /** 1991 * @param value A free text natural language description of the message 1992 * definition from a consumer's perspective. 1993 */ 1994 public MessageDefinition setDescription(String value) { 1995 if (value == null) 1996 this.description = null; 1997 else { 1998 if (this.description == null) 1999 this.description = new MarkdownType(); 2000 this.description.setValue(value); 2001 } 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #useContext} (The content was developed with a focus and 2007 * intent of supporting the contexts that are listed. These contexts may 2008 * be general categories (gender, age, ...) or may be references to 2009 * specific programs (insurance plans, studies, ...) and may be used to 2010 * assist with indexing and searching for appropriate message definition 2011 * instances.) 2012 */ 2013 public List<UsageContext> getUseContext() { 2014 if (this.useContext == null) 2015 this.useContext = new ArrayList<UsageContext>(); 2016 return this.useContext; 2017 } 2018 2019 /** 2020 * @return Returns a reference to <code>this</code> for easy method chaining 2021 */ 2022 public MessageDefinition setUseContext(List<UsageContext> theUseContext) { 2023 this.useContext = theUseContext; 2024 return this; 2025 } 2026 2027 public boolean hasUseContext() { 2028 if (this.useContext == null) 2029 return false; 2030 for (UsageContext item : this.useContext) 2031 if (!item.isEmpty()) 2032 return true; 2033 return false; 2034 } 2035 2036 public UsageContext addUseContext() { // 3 2037 UsageContext t = new UsageContext(); 2038 if (this.useContext == null) 2039 this.useContext = new ArrayList<UsageContext>(); 2040 this.useContext.add(t); 2041 return t; 2042 } 2043 2044 public MessageDefinition addUseContext(UsageContext t) { // 3 2045 if (t == null) 2046 return this; 2047 if (this.useContext == null) 2048 this.useContext = new ArrayList<UsageContext>(); 2049 this.useContext.add(t); 2050 return this; 2051 } 2052 2053 /** 2054 * @return The first repetition of repeating field {@link #useContext}, creating 2055 * it if it does not already exist 2056 */ 2057 public UsageContext getUseContextFirstRep() { 2058 if (getUseContext().isEmpty()) { 2059 addUseContext(); 2060 } 2061 return getUseContext().get(0); 2062 } 2063 2064 /** 2065 * @return {@link #jurisdiction} (A legal or geographic region in which the 2066 * message definition is intended to be used.) 2067 */ 2068 public List<CodeableConcept> getJurisdiction() { 2069 if (this.jurisdiction == null) 2070 this.jurisdiction = new ArrayList<CodeableConcept>(); 2071 return this.jurisdiction; 2072 } 2073 2074 /** 2075 * @return Returns a reference to <code>this</code> for easy method chaining 2076 */ 2077 public MessageDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2078 this.jurisdiction = theJurisdiction; 2079 return this; 2080 } 2081 2082 public boolean hasJurisdiction() { 2083 if (this.jurisdiction == null) 2084 return false; 2085 for (CodeableConcept item : this.jurisdiction) 2086 if (!item.isEmpty()) 2087 return true; 2088 return false; 2089 } 2090 2091 public CodeableConcept addJurisdiction() { // 3 2092 CodeableConcept t = new CodeableConcept(); 2093 if (this.jurisdiction == null) 2094 this.jurisdiction = new ArrayList<CodeableConcept>(); 2095 this.jurisdiction.add(t); 2096 return t; 2097 } 2098 2099 public MessageDefinition addJurisdiction(CodeableConcept t) { // 3 2100 if (t == null) 2101 return this; 2102 if (this.jurisdiction == null) 2103 this.jurisdiction = new ArrayList<CodeableConcept>(); 2104 this.jurisdiction.add(t); 2105 return this; 2106 } 2107 2108 /** 2109 * @return The first repetition of repeating field {@link #jurisdiction}, 2110 * creating it if it does not already exist 2111 */ 2112 public CodeableConcept getJurisdictionFirstRep() { 2113 if (getJurisdiction().isEmpty()) { 2114 addJurisdiction(); 2115 } 2116 return getJurisdiction().get(0); 2117 } 2118 2119 /** 2120 * @return {@link #purpose} (Explanation of why this message definition is 2121 * needed and why it has been designed as it has.). This is the 2122 * underlying object with id, value and extensions. The accessor 2123 * "getPurpose" gives direct access to the value 2124 */ 2125 public MarkdownType getPurposeElement() { 2126 if (this.purpose == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create MessageDefinition.purpose"); 2129 else if (Configuration.doAutoCreate()) 2130 this.purpose = new MarkdownType(); // bb 2131 return this.purpose; 2132 } 2133 2134 public boolean hasPurposeElement() { 2135 return this.purpose != null && !this.purpose.isEmpty(); 2136 } 2137 2138 public boolean hasPurpose() { 2139 return this.purpose != null && !this.purpose.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #purpose} (Explanation of why this message definition is 2144 * needed and why it has been designed as it has.). This is the 2145 * underlying object with id, value and extensions. The accessor 2146 * "getPurpose" gives direct access to the value 2147 */ 2148 public MessageDefinition setPurposeElement(MarkdownType value) { 2149 this.purpose = value; 2150 return this; 2151 } 2152 2153 /** 2154 * @return Explanation of why this message definition is needed and why it has 2155 * been designed as it has. 2156 */ 2157 public String getPurpose() { 2158 return this.purpose == null ? null : this.purpose.getValue(); 2159 } 2160 2161 /** 2162 * @param value Explanation of why this message definition is needed and why it 2163 * has been designed as it has. 2164 */ 2165 public MessageDefinition setPurpose(String value) { 2166 if (value == null) 2167 this.purpose = null; 2168 else { 2169 if (this.purpose == null) 2170 this.purpose = new MarkdownType(); 2171 this.purpose.setValue(value); 2172 } 2173 return this; 2174 } 2175 2176 /** 2177 * @return {@link #copyright} (A copyright statement relating to the message 2178 * definition and/or its contents. Copyright statements are generally 2179 * legal restrictions on the use and publishing of the message 2180 * definition.). This is the underlying object with id, value and 2181 * extensions. The accessor "getCopyright" gives direct access to the 2182 * value 2183 */ 2184 public MarkdownType getCopyrightElement() { 2185 if (this.copyright == null) 2186 if (Configuration.errorOnAutoCreate()) 2187 throw new Error("Attempt to auto-create MessageDefinition.copyright"); 2188 else if (Configuration.doAutoCreate()) 2189 this.copyright = new MarkdownType(); // bb 2190 return this.copyright; 2191 } 2192 2193 public boolean hasCopyrightElement() { 2194 return this.copyright != null && !this.copyright.isEmpty(); 2195 } 2196 2197 public boolean hasCopyright() { 2198 return this.copyright != null && !this.copyright.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #copyright} (A copyright statement relating to the 2203 * message definition and/or its contents. Copyright statements are 2204 * generally legal restrictions on the use and publishing of the 2205 * message definition.). This is the underlying object with id, 2206 * value and extensions. The accessor "getCopyright" gives direct 2207 * access to the value 2208 */ 2209 public MessageDefinition setCopyrightElement(MarkdownType value) { 2210 this.copyright = value; 2211 return this; 2212 } 2213 2214 /** 2215 * @return A copyright statement relating to the message definition and/or its 2216 * contents. Copyright statements are generally legal restrictions on 2217 * the use and publishing of the message definition. 2218 */ 2219 public String getCopyright() { 2220 return this.copyright == null ? null : this.copyright.getValue(); 2221 } 2222 2223 /** 2224 * @param value A copyright statement relating to the message definition and/or 2225 * its contents. Copyright statements are generally legal 2226 * restrictions on the use and publishing of the message 2227 * definition. 2228 */ 2229 public MessageDefinition setCopyright(String value) { 2230 if (value == null) 2231 this.copyright = null; 2232 else { 2233 if (this.copyright == null) 2234 this.copyright = new MarkdownType(); 2235 this.copyright.setValue(value); 2236 } 2237 return this; 2238 } 2239 2240 /** 2241 * @return {@link #base} (The MessageDefinition that is the basis for the 2242 * contents of this resource.). This is the underlying object with id, 2243 * value and extensions. The accessor "getBase" gives direct access to 2244 * the value 2245 */ 2246 public CanonicalType getBaseElement() { 2247 if (this.base == null) 2248 if (Configuration.errorOnAutoCreate()) 2249 throw new Error("Attempt to auto-create MessageDefinition.base"); 2250 else if (Configuration.doAutoCreate()) 2251 this.base = new CanonicalType(); // bb 2252 return this.base; 2253 } 2254 2255 public boolean hasBaseElement() { 2256 return this.base != null && !this.base.isEmpty(); 2257 } 2258 2259 public boolean hasBase() { 2260 return this.base != null && !this.base.isEmpty(); 2261 } 2262 2263 /** 2264 * @param value {@link #base} (The MessageDefinition that is the basis for the 2265 * contents of this resource.). This is the underlying object with 2266 * id, value and extensions. The accessor "getBase" gives direct 2267 * access to the value 2268 */ 2269 public MessageDefinition setBaseElement(CanonicalType value) { 2270 this.base = value; 2271 return this; 2272 } 2273 2274 /** 2275 * @return The MessageDefinition that is the basis for the contents of this 2276 * resource. 2277 */ 2278 public String getBase() { 2279 return this.base == null ? null : this.base.getValue(); 2280 } 2281 2282 /** 2283 * @param value The MessageDefinition that is the basis for the contents of this 2284 * resource. 2285 */ 2286 public MessageDefinition setBase(String value) { 2287 if (Utilities.noString(value)) 2288 this.base = null; 2289 else { 2290 if (this.base == null) 2291 this.base = new CanonicalType(); 2292 this.base.setValue(value); 2293 } 2294 return this; 2295 } 2296 2297 /** 2298 * @return {@link #parent} (Identifies a protocol or workflow that this 2299 * MessageDefinition represents a step in.) 2300 */ 2301 public List<CanonicalType> getParent() { 2302 if (this.parent == null) 2303 this.parent = new ArrayList<CanonicalType>(); 2304 return this.parent; 2305 } 2306 2307 /** 2308 * @return Returns a reference to <code>this</code> for easy method chaining 2309 */ 2310 public MessageDefinition setParent(List<CanonicalType> theParent) { 2311 this.parent = theParent; 2312 return this; 2313 } 2314 2315 public boolean hasParent() { 2316 if (this.parent == null) 2317 return false; 2318 for (CanonicalType item : this.parent) 2319 if (!item.isEmpty()) 2320 return true; 2321 return false; 2322 } 2323 2324 /** 2325 * @return {@link #parent} (Identifies a protocol or workflow that this 2326 * MessageDefinition represents a step in.) 2327 */ 2328 public CanonicalType addParentElement() {// 2 2329 CanonicalType t = new CanonicalType(); 2330 if (this.parent == null) 2331 this.parent = new ArrayList<CanonicalType>(); 2332 this.parent.add(t); 2333 return t; 2334 } 2335 2336 /** 2337 * @param value {@link #parent} (Identifies a protocol or workflow that this 2338 * MessageDefinition represents a step in.) 2339 */ 2340 public MessageDefinition addParent(String value) { // 1 2341 CanonicalType t = new CanonicalType(); 2342 t.setValue(value); 2343 if (this.parent == null) 2344 this.parent = new ArrayList<CanonicalType>(); 2345 this.parent.add(t); 2346 return this; 2347 } 2348 2349 /** 2350 * @param value {@link #parent} (Identifies a protocol or workflow that this 2351 * MessageDefinition represents a step in.) 2352 */ 2353 public boolean hasParent(String value) { 2354 if (this.parent == null) 2355 return false; 2356 for (CanonicalType v : this.parent) 2357 if (v.getValue().equals(value)) // canonical(ActivityDefinition|PlanDefinition) 2358 return true; 2359 return false; 2360 } 2361 2362 /** 2363 * @return {@link #event} (Event code or link to the EventDefinition.) 2364 */ 2365 public Type getEvent() { 2366 return this.event; 2367 } 2368 2369 /** 2370 * @return {@link #event} (Event code or link to the EventDefinition.) 2371 */ 2372 public Coding getEventCoding() throws FHIRException { 2373 if (this.event == null) 2374 this.event = new Coding(); 2375 if (!(this.event instanceof Coding)) 2376 throw new FHIRException( 2377 "Type mismatch: the type Coding was expected, but " + this.event.getClass().getName() + " was encountered"); 2378 return (Coding) this.event; 2379 } 2380 2381 public boolean hasEventCoding() { 2382 return this != null && this.event instanceof Coding; 2383 } 2384 2385 /** 2386 * @return {@link #event} (Event code or link to the EventDefinition.) 2387 */ 2388 public UriType getEventUriType() throws FHIRException { 2389 if (this.event == null) 2390 this.event = new UriType(); 2391 if (!(this.event instanceof UriType)) 2392 throw new FHIRException( 2393 "Type mismatch: the type UriType was expected, but " + this.event.getClass().getName() + " was encountered"); 2394 return (UriType) this.event; 2395 } 2396 2397 public boolean hasEventUriType() { 2398 return this != null && this.event instanceof UriType; 2399 } 2400 2401 public boolean hasEvent() { 2402 return this.event != null && !this.event.isEmpty(); 2403 } 2404 2405 /** 2406 * @param value {@link #event} (Event code or link to the EventDefinition.) 2407 */ 2408 public MessageDefinition setEvent(Type value) { 2409 if (value != null && !(value instanceof Coding || value instanceof UriType)) 2410 throw new Error("Not the right type for MessageDefinition.event[x]: " + value.fhirType()); 2411 this.event = value; 2412 return this; 2413 } 2414 2415 /** 2416 * @return {@link #category} (The impact of the content of the message.). This 2417 * is the underlying object with id, value and extensions. The accessor 2418 * "getCategory" gives direct access to the value 2419 */ 2420 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 2421 if (this.category == null) 2422 if (Configuration.errorOnAutoCreate()) 2423 throw new Error("Attempt to auto-create MessageDefinition.category"); 2424 else if (Configuration.doAutoCreate()) 2425 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 2426 return this.category; 2427 } 2428 2429 public boolean hasCategoryElement() { 2430 return this.category != null && !this.category.isEmpty(); 2431 } 2432 2433 public boolean hasCategory() { 2434 return this.category != null && !this.category.isEmpty(); 2435 } 2436 2437 /** 2438 * @param value {@link #category} (The impact of the content of the message.). 2439 * This is the underlying object with id, value and extensions. The 2440 * accessor "getCategory" gives direct access to the value 2441 */ 2442 public MessageDefinition setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 2443 this.category = value; 2444 return this; 2445 } 2446 2447 /** 2448 * @return The impact of the content of the message. 2449 */ 2450 public MessageSignificanceCategory getCategory() { 2451 return this.category == null ? null : this.category.getValue(); 2452 } 2453 2454 /** 2455 * @param value The impact of the content of the message. 2456 */ 2457 public MessageDefinition setCategory(MessageSignificanceCategory value) { 2458 if (value == null) 2459 this.category = null; 2460 else { 2461 if (this.category == null) 2462 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 2463 this.category.setValue(value); 2464 } 2465 return this; 2466 } 2467 2468 /** 2469 * @return {@link #focus} (Identifies the resource (or resources) that are being 2470 * addressed by the event. For example, the Encounter for an admit 2471 * message or two Account records for a merge.) 2472 */ 2473 public List<MessageDefinitionFocusComponent> getFocus() { 2474 if (this.focus == null) 2475 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2476 return this.focus; 2477 } 2478 2479 /** 2480 * @return Returns a reference to <code>this</code> for easy method chaining 2481 */ 2482 public MessageDefinition setFocus(List<MessageDefinitionFocusComponent> theFocus) { 2483 this.focus = theFocus; 2484 return this; 2485 } 2486 2487 public boolean hasFocus() { 2488 if (this.focus == null) 2489 return false; 2490 for (MessageDefinitionFocusComponent item : this.focus) 2491 if (!item.isEmpty()) 2492 return true; 2493 return false; 2494 } 2495 2496 public MessageDefinitionFocusComponent addFocus() { // 3 2497 MessageDefinitionFocusComponent t = new MessageDefinitionFocusComponent(); 2498 if (this.focus == null) 2499 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2500 this.focus.add(t); 2501 return t; 2502 } 2503 2504 public MessageDefinition addFocus(MessageDefinitionFocusComponent t) { // 3 2505 if (t == null) 2506 return this; 2507 if (this.focus == null) 2508 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2509 this.focus.add(t); 2510 return this; 2511 } 2512 2513 /** 2514 * @return The first repetition of repeating field {@link #focus}, creating it 2515 * if it does not already exist 2516 */ 2517 public MessageDefinitionFocusComponent getFocusFirstRep() { 2518 if (getFocus().isEmpty()) { 2519 addFocus(); 2520 } 2521 return getFocus().get(0); 2522 } 2523 2524 /** 2525 * @return {@link #responseRequired} (Declare at a message definition level 2526 * whether a response is required or only upon error or success, or 2527 * never.). This is the underlying object with id, value and extensions. 2528 * The accessor "getResponseRequired" gives direct access to the value 2529 */ 2530 public Enumeration<MessageheaderResponseRequest> getResponseRequiredElement() { 2531 if (this.responseRequired == null) 2532 if (Configuration.errorOnAutoCreate()) 2533 throw new Error("Attempt to auto-create MessageDefinition.responseRequired"); 2534 else if (Configuration.doAutoCreate()) 2535 this.responseRequired = new Enumeration<MessageheaderResponseRequest>( 2536 new MessageheaderResponseRequestEnumFactory()); // bb 2537 return this.responseRequired; 2538 } 2539 2540 public boolean hasResponseRequiredElement() { 2541 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2542 } 2543 2544 public boolean hasResponseRequired() { 2545 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2546 } 2547 2548 /** 2549 * @param value {@link #responseRequired} (Declare at a message definition level 2550 * whether a response is required or only upon error or success, or 2551 * never.). This is the underlying object with id, value and 2552 * extensions. The accessor "getResponseRequired" gives direct 2553 * access to the value 2554 */ 2555 public MessageDefinition setResponseRequiredElement(Enumeration<MessageheaderResponseRequest> value) { 2556 this.responseRequired = value; 2557 return this; 2558 } 2559 2560 /** 2561 * @return Declare at a message definition level whether a response is required 2562 * or only upon error or success, or never. 2563 */ 2564 public MessageheaderResponseRequest getResponseRequired() { 2565 return this.responseRequired == null ? null : this.responseRequired.getValue(); 2566 } 2567 2568 /** 2569 * @param value Declare at a message definition level whether a response is 2570 * required or only upon error or success, or never. 2571 */ 2572 public MessageDefinition setResponseRequired(MessageheaderResponseRequest value) { 2573 if (value == null) 2574 this.responseRequired = null; 2575 else { 2576 if (this.responseRequired == null) 2577 this.responseRequired = new Enumeration<MessageheaderResponseRequest>( 2578 new MessageheaderResponseRequestEnumFactory()); 2579 this.responseRequired.setValue(value); 2580 } 2581 return this; 2582 } 2583 2584 /** 2585 * @return {@link #allowedResponse} (Indicates what types of messages may be 2586 * sent as an application-level response to this message.) 2587 */ 2588 public List<MessageDefinitionAllowedResponseComponent> getAllowedResponse() { 2589 if (this.allowedResponse == null) 2590 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2591 return this.allowedResponse; 2592 } 2593 2594 /** 2595 * @return Returns a reference to <code>this</code> for easy method chaining 2596 */ 2597 public MessageDefinition setAllowedResponse(List<MessageDefinitionAllowedResponseComponent> theAllowedResponse) { 2598 this.allowedResponse = theAllowedResponse; 2599 return this; 2600 } 2601 2602 public boolean hasAllowedResponse() { 2603 if (this.allowedResponse == null) 2604 return false; 2605 for (MessageDefinitionAllowedResponseComponent item : this.allowedResponse) 2606 if (!item.isEmpty()) 2607 return true; 2608 return false; 2609 } 2610 2611 public MessageDefinitionAllowedResponseComponent addAllowedResponse() { // 3 2612 MessageDefinitionAllowedResponseComponent t = new MessageDefinitionAllowedResponseComponent(); 2613 if (this.allowedResponse == null) 2614 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2615 this.allowedResponse.add(t); 2616 return t; 2617 } 2618 2619 public MessageDefinition addAllowedResponse(MessageDefinitionAllowedResponseComponent t) { // 3 2620 if (t == null) 2621 return this; 2622 if (this.allowedResponse == null) 2623 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2624 this.allowedResponse.add(t); 2625 return this; 2626 } 2627 2628 /** 2629 * @return The first repetition of repeating field {@link #allowedResponse}, 2630 * creating it if it does not already exist 2631 */ 2632 public MessageDefinitionAllowedResponseComponent getAllowedResponseFirstRep() { 2633 if (getAllowedResponse().isEmpty()) { 2634 addAllowedResponse(); 2635 } 2636 return getAllowedResponse().get(0); 2637 } 2638 2639 /** 2640 * @return {@link #graph} (Canonical reference to a GraphDefinition. If a URL is 2641 * provided, it is the canonical reference to a [[[GraphDefinition]]] 2642 * that it controls what resources are to be added to the bundle when 2643 * building the document. The GraphDefinition can also specify profiles 2644 * that apply to the various resources.) 2645 */ 2646 public List<CanonicalType> getGraph() { 2647 if (this.graph == null) 2648 this.graph = new ArrayList<CanonicalType>(); 2649 return this.graph; 2650 } 2651 2652 /** 2653 * @return Returns a reference to <code>this</code> for easy method chaining 2654 */ 2655 public MessageDefinition setGraph(List<CanonicalType> theGraph) { 2656 this.graph = theGraph; 2657 return this; 2658 } 2659 2660 public boolean hasGraph() { 2661 if (this.graph == null) 2662 return false; 2663 for (CanonicalType item : this.graph) 2664 if (!item.isEmpty()) 2665 return true; 2666 return false; 2667 } 2668 2669 /** 2670 * @return {@link #graph} (Canonical reference to a GraphDefinition. If a URL is 2671 * provided, it is the canonical reference to a [[[GraphDefinition]]] 2672 * that it controls what resources are to be added to the bundle when 2673 * building the document. The GraphDefinition can also specify profiles 2674 * that apply to the various resources.) 2675 */ 2676 public CanonicalType addGraphElement() {// 2 2677 CanonicalType t = new CanonicalType(); 2678 if (this.graph == null) 2679 this.graph = new ArrayList<CanonicalType>(); 2680 this.graph.add(t); 2681 return t; 2682 } 2683 2684 /** 2685 * @param value {@link #graph} (Canonical reference to a GraphDefinition. If a 2686 * URL is provided, it is the canonical reference to a 2687 * [[[GraphDefinition]]] that it controls what resources are to be 2688 * added to the bundle when building the document. The 2689 * GraphDefinition can also specify profiles that apply to the 2690 * various resources.) 2691 */ 2692 public MessageDefinition addGraph(String value) { // 1 2693 CanonicalType t = new CanonicalType(); 2694 t.setValue(value); 2695 if (this.graph == null) 2696 this.graph = new ArrayList<CanonicalType>(); 2697 this.graph.add(t); 2698 return this; 2699 } 2700 2701 /** 2702 * @param value {@link #graph} (Canonical reference to a GraphDefinition. If a 2703 * URL is provided, it is the canonical reference to a 2704 * [[[GraphDefinition]]] that it controls what resources are to be 2705 * added to the bundle when building the document. The 2706 * GraphDefinition can also specify profiles that apply to the 2707 * various resources.) 2708 */ 2709 public boolean hasGraph(String value) { 2710 if (this.graph == null) 2711 return false; 2712 for (CanonicalType v : this.graph) 2713 if (v.getValue().equals(value)) // canonical(GraphDefinition) 2714 return true; 2715 return false; 2716 } 2717 2718 protected void listChildren(List<Property> children) { 2719 super.listChildren(children); 2720 children.add(new Property("url", "uri", 2721 "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 2722 0, 1, url)); 2723 children.add(new Property("identifier", "Identifier", 2724 "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2725 0, java.lang.Integer.MAX_VALUE, identifier)); 2726 children.add(new Property("version", "string", 2727 "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2728 0, 1, version)); 2729 children.add(new Property("name", "string", 2730 "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2731 0, 1, name)); 2732 children.add(new Property("title", "string", 2733 "A short, descriptive, user-friendly title for the message definition.", 0, 1, title)); 2734 children.add(new Property("replaces", "canonical(MessageDefinition)", 2735 "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2736 children.add(new Property("status", "code", 2737 "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2738 children.add(new Property("experimental", "boolean", 2739 "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2740 0, 1, experimental)); 2741 children.add(new Property("date", "dateTime", 2742 "The date (and optionally time) when the message definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 2743 0, 1, date)); 2744 children.add(new Property("publisher", "string", 2745 "The name of the organization or individual that published the message definition.", 0, 1, publisher)); 2746 children.add(new Property("contact", "ContactDetail", 2747 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2748 java.lang.Integer.MAX_VALUE, contact)); 2749 children.add(new Property("description", "markdown", 2750 "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, 2751 description)); 2752 children.add(new Property("useContext", "UsageContext", 2753 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 2754 0, java.lang.Integer.MAX_VALUE, useContext)); 2755 children.add(new Property("jurisdiction", "CodeableConcept", 2756 "A legal or geographic region in which the message definition is intended to be used.", 0, 2757 java.lang.Integer.MAX_VALUE, jurisdiction)); 2758 children.add(new Property("purpose", "markdown", 2759 "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2760 children.add(new Property("copyright", "markdown", 2761 "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 2762 0, 1, copyright)); 2763 children.add(new Property("base", "canonical(MessageDefinition)", 2764 "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base)); 2765 children.add(new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", 2766 "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, 2767 java.lang.Integer.MAX_VALUE, parent)); 2768 children.add(new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event)); 2769 children.add(new Property("category", "code", "The impact of the content of the message.", 0, 1, category)); 2770 children.add(new Property("focus", "", 2771 "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 2772 0, java.lang.Integer.MAX_VALUE, focus)); 2773 children.add(new Property("responseRequired", "code", 2774 "Declare at a message definition level whether a response is required or only upon error or success, or never.", 2775 0, 1, responseRequired)); 2776 children.add(new Property("allowedResponse", "", 2777 "Indicates what types of messages may be sent as an application-level response to this message.", 0, 2778 java.lang.Integer.MAX_VALUE, allowedResponse)); 2779 children.add(new Property("graph", "canonical(GraphDefinition)", 2780 "Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a [[[GraphDefinition]]] that it controls what resources are to be added to the bundle when building the document. The GraphDefinition can also specify profiles that apply to the various resources.", 2781 0, java.lang.Integer.MAX_VALUE, graph)); 2782 } 2783 2784 @Override 2785 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2786 switch (_hash) { 2787 case 116079: 2788 /* url */ return new Property("url", "uri", 2789 "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 2790 0, 1, url); 2791 case -1618432855: 2792 /* identifier */ return new Property("identifier", "Identifier", 2793 "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2794 0, java.lang.Integer.MAX_VALUE, identifier); 2795 case 351608024: 2796 /* version */ return new Property("version", "string", 2797 "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2798 0, 1, version); 2799 case 3373707: 2800 /* name */ return new Property("name", "string", 2801 "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2802 0, 1, name); 2803 case 110371416: 2804 /* title */ return new Property("title", "string", 2805 "A short, descriptive, user-friendly title for the message definition.", 0, 1, title); 2806 case -430332865: 2807 /* replaces */ return new Property("replaces", "canonical(MessageDefinition)", 2808 "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces); 2809 case -892481550: 2810 /* status */ return new Property("status", "code", 2811 "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2812 case -404562712: 2813 /* experimental */ return new Property("experimental", "boolean", 2814 "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2815 0, 1, experimental); 2816 case 3076014: 2817 /* date */ return new Property("date", "dateTime", 2818 "The date (and optionally time) when the message definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 2819 0, 1, date); 2820 case 1447404028: 2821 /* publisher */ return new Property("publisher", "string", 2822 "The name of the organization or individual that published the message definition.", 0, 1, publisher); 2823 case 951526432: 2824 /* contact */ return new Property("contact", "ContactDetail", 2825 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2826 java.lang.Integer.MAX_VALUE, contact); 2827 case -1724546052: 2828 /* description */ return new Property("description", "markdown", 2829 "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, 2830 description); 2831 case -669707736: 2832 /* useContext */ return new Property("useContext", "UsageContext", 2833 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 2834 0, java.lang.Integer.MAX_VALUE, useContext); 2835 case -507075711: 2836 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 2837 "A legal or geographic region in which the message definition is intended to be used.", 0, 2838 java.lang.Integer.MAX_VALUE, jurisdiction); 2839 case -220463842: 2840 /* purpose */ return new Property("purpose", "markdown", 2841 "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, 2842 purpose); 2843 case 1522889671: 2844 /* copyright */ return new Property("copyright", "markdown", 2845 "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 2846 0, 1, copyright); 2847 case 3016401: 2848 /* base */ return new Property("base", "canonical(MessageDefinition)", 2849 "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base); 2850 case -995424086: 2851 /* parent */ return new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", 2852 "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, 2853 java.lang.Integer.MAX_VALUE, parent); 2854 case 278115238: 2855 /* event[x] */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, 2856 event); 2857 case 96891546: 2858 /* event */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, 2859 event); 2860 case -355957084: 2861 /* eventCoding */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 2862 1, event); 2863 case 278109298: 2864 /* eventUri */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, 2865 event); 2866 case 50511102: 2867 /* category */ return new Property("category", "code", "The impact of the content of the message.", 0, 1, 2868 category); 2869 case 97604824: 2870 /* focus */ return new Property("focus", "", 2871 "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 2872 0, java.lang.Integer.MAX_VALUE, focus); 2873 case 791597824: 2874 /* responseRequired */ return new Property("responseRequired", "code", 2875 "Declare at a message definition level whether a response is required or only upon error or success, or never.", 2876 0, 1, responseRequired); 2877 case -1130933751: 2878 /* allowedResponse */ return new Property("allowedResponse", "", 2879 "Indicates what types of messages may be sent as an application-level response to this message.", 0, 2880 java.lang.Integer.MAX_VALUE, allowedResponse); 2881 case 98615630: 2882 /* graph */ return new Property("graph", "canonical(GraphDefinition)", 2883 "Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a [[[GraphDefinition]]] that it controls what resources are to be added to the bundle when building the document. The GraphDefinition can also specify profiles that apply to the various resources.", 2884 0, java.lang.Integer.MAX_VALUE, graph); 2885 default: 2886 return super.getNamedProperty(_hash, _name, _checkValid); 2887 } 2888 2889 } 2890 2891 @Override 2892 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2893 switch (hash) { 2894 case 116079: 2895 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2896 case -1618432855: 2897 /* identifier */ return this.identifier == null ? new Base[0] 2898 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2899 case 351608024: 2900 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 2901 case 3373707: 2902 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2903 case 110371416: 2904 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2905 case -430332865: 2906 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 2907 case -892481550: 2908 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 2909 case -404562712: 2910 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 2911 case 3076014: 2912 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2913 case 1447404028: 2914 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 2915 case 951526432: 2916 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2917 case -1724546052: 2918 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2919 case -669707736: 2920 /* useContext */ return this.useContext == null ? new Base[0] 2921 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2922 case -507075711: 2923 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 2924 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2925 case -220463842: 2926 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 2927 case 1522889671: 2928 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 2929 case 3016401: 2930 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // CanonicalType 2931 case -995424086: 2932 /* parent */ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // CanonicalType 2933 case 96891546: 2934 /* event */ return this.event == null ? new Base[0] : new Base[] { this.event }; // Type 2935 case 50511102: 2936 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // Enumeration<MessageSignificanceCategory> 2937 case 97604824: 2938 /* focus */ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // MessageDefinitionFocusComponent 2939 case 791597824: 2940 /* responseRequired */ return this.responseRequired == null ? new Base[0] : new Base[] { this.responseRequired }; // Enumeration<MessageheaderResponseRequest> 2941 case -1130933751: 2942 /* allowedResponse */ return this.allowedResponse == null ? new Base[0] 2943 : this.allowedResponse.toArray(new Base[this.allowedResponse.size()]); // MessageDefinitionAllowedResponseComponent 2944 case 98615630: 2945 /* graph */ return this.graph == null ? new Base[0] : this.graph.toArray(new Base[this.graph.size()]); // CanonicalType 2946 default: 2947 return super.getProperty(hash, name, checkValid); 2948 } 2949 2950 } 2951 2952 @Override 2953 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2954 switch (hash) { 2955 case 116079: // url 2956 this.url = castToUri(value); // UriType 2957 return value; 2958 case -1618432855: // identifier 2959 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2960 return value; 2961 case 351608024: // version 2962 this.version = castToString(value); // StringType 2963 return value; 2964 case 3373707: // name 2965 this.name = castToString(value); // StringType 2966 return value; 2967 case 110371416: // title 2968 this.title = castToString(value); // StringType 2969 return value; 2970 case -430332865: // replaces 2971 this.getReplaces().add(castToCanonical(value)); // CanonicalType 2972 return value; 2973 case -892481550: // status 2974 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2975 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2976 return value; 2977 case -404562712: // experimental 2978 this.experimental = castToBoolean(value); // BooleanType 2979 return value; 2980 case 3076014: // date 2981 this.date = castToDateTime(value); // DateTimeType 2982 return value; 2983 case 1447404028: // publisher 2984 this.publisher = castToString(value); // StringType 2985 return value; 2986 case 951526432: // contact 2987 this.getContact().add(castToContactDetail(value)); // ContactDetail 2988 return value; 2989 case -1724546052: // description 2990 this.description = castToMarkdown(value); // MarkdownType 2991 return value; 2992 case -669707736: // useContext 2993 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2994 return value; 2995 case -507075711: // jurisdiction 2996 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2997 return value; 2998 case -220463842: // purpose 2999 this.purpose = castToMarkdown(value); // MarkdownType 3000 return value; 3001 case 1522889671: // copyright 3002 this.copyright = castToMarkdown(value); // MarkdownType 3003 return value; 3004 case 3016401: // base 3005 this.base = castToCanonical(value); // CanonicalType 3006 return value; 3007 case -995424086: // parent 3008 this.getParent().add(castToCanonical(value)); // CanonicalType 3009 return value; 3010 case 96891546: // event 3011 this.event = castToType(value); // Type 3012 return value; 3013 case 50511102: // category 3014 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 3015 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 3016 return value; 3017 case 97604824: // focus 3018 this.getFocus().add((MessageDefinitionFocusComponent) value); // MessageDefinitionFocusComponent 3019 return value; 3020 case 791597824: // responseRequired 3021 value = new MessageheaderResponseRequestEnumFactory().fromType(castToCode(value)); 3022 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 3023 return value; 3024 case -1130933751: // allowedResponse 3025 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); // MessageDefinitionAllowedResponseComponent 3026 return value; 3027 case 98615630: // graph 3028 this.getGraph().add(castToCanonical(value)); // CanonicalType 3029 return value; 3030 default: 3031 return super.setProperty(hash, name, value); 3032 } 3033 3034 } 3035 3036 @Override 3037 public Base setProperty(String name, Base value) throws FHIRException { 3038 if (name.equals("url")) { 3039 this.url = castToUri(value); // UriType 3040 } else if (name.equals("identifier")) { 3041 this.getIdentifier().add(castToIdentifier(value)); 3042 } else if (name.equals("version")) { 3043 this.version = castToString(value); // StringType 3044 } else if (name.equals("name")) { 3045 this.name = castToString(value); // StringType 3046 } else if (name.equals("title")) { 3047 this.title = castToString(value); // StringType 3048 } else if (name.equals("replaces")) { 3049 this.getReplaces().add(castToCanonical(value)); 3050 } else if (name.equals("status")) { 3051 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3052 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3053 } else if (name.equals("experimental")) { 3054 this.experimental = castToBoolean(value); // BooleanType 3055 } else if (name.equals("date")) { 3056 this.date = castToDateTime(value); // DateTimeType 3057 } else if (name.equals("publisher")) { 3058 this.publisher = castToString(value); // StringType 3059 } else if (name.equals("contact")) { 3060 this.getContact().add(castToContactDetail(value)); 3061 } else if (name.equals("description")) { 3062 this.description = castToMarkdown(value); // MarkdownType 3063 } else if (name.equals("useContext")) { 3064 this.getUseContext().add(castToUsageContext(value)); 3065 } else if (name.equals("jurisdiction")) { 3066 this.getJurisdiction().add(castToCodeableConcept(value)); 3067 } else if (name.equals("purpose")) { 3068 this.purpose = castToMarkdown(value); // MarkdownType 3069 } else if (name.equals("copyright")) { 3070 this.copyright = castToMarkdown(value); // MarkdownType 3071 } else if (name.equals("base")) { 3072 this.base = castToCanonical(value); // CanonicalType 3073 } else if (name.equals("parent")) { 3074 this.getParent().add(castToCanonical(value)); 3075 } else if (name.equals("event[x]")) { 3076 this.event = castToType(value); // Type 3077 } else if (name.equals("category")) { 3078 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 3079 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 3080 } else if (name.equals("focus")) { 3081 this.getFocus().add((MessageDefinitionFocusComponent) value); 3082 } else if (name.equals("responseRequired")) { 3083 value = new MessageheaderResponseRequestEnumFactory().fromType(castToCode(value)); 3084 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 3085 } else if (name.equals("allowedResponse")) { 3086 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); 3087 } else if (name.equals("graph")) { 3088 this.getGraph().add(castToCanonical(value)); 3089 } else 3090 return super.setProperty(name, value); 3091 return value; 3092 } 3093 3094 @Override 3095 public void removeChild(String name, Base value) throws FHIRException { 3096 if (name.equals("url")) { 3097 this.url = null; 3098 } else if (name.equals("identifier")) { 3099 this.getIdentifier().remove(castToIdentifier(value)); 3100 } else if (name.equals("version")) { 3101 this.version = null; 3102 } else if (name.equals("name")) { 3103 this.name = null; 3104 } else if (name.equals("title")) { 3105 this.title = null; 3106 } else if (name.equals("replaces")) { 3107 this.getReplaces().remove(castToCanonical(value)); 3108 } else if (name.equals("status")) { 3109 this.status = null; 3110 } else if (name.equals("experimental")) { 3111 this.experimental = null; 3112 } else if (name.equals("date")) { 3113 this.date = null; 3114 } else if (name.equals("publisher")) { 3115 this.publisher = null; 3116 } else if (name.equals("contact")) { 3117 this.getContact().remove(castToContactDetail(value)); 3118 } else if (name.equals("description")) { 3119 this.description = null; 3120 } else if (name.equals("useContext")) { 3121 this.getUseContext().remove(castToUsageContext(value)); 3122 } else if (name.equals("jurisdiction")) { 3123 this.getJurisdiction().remove(castToCodeableConcept(value)); 3124 } else if (name.equals("purpose")) { 3125 this.purpose = null; 3126 } else if (name.equals("copyright")) { 3127 this.copyright = null; 3128 } else if (name.equals("base")) { 3129 this.base = null; 3130 } else if (name.equals("parent")) { 3131 this.getParent().remove(castToCanonical(value)); 3132 } else if (name.equals("event[x]")) { 3133 this.event = null; 3134 } else if (name.equals("category")) { 3135 this.category = null; 3136 } else if (name.equals("focus")) { 3137 this.getFocus().remove((MessageDefinitionFocusComponent) value); 3138 } else if (name.equals("responseRequired")) { 3139 this.responseRequired = null; 3140 } else if (name.equals("allowedResponse")) { 3141 this.getAllowedResponse().remove((MessageDefinitionAllowedResponseComponent) value); 3142 } else if (name.equals("graph")) { 3143 this.getGraph().remove(castToCanonical(value)); 3144 } else 3145 super.removeChild(name, value); 3146 3147 } 3148 3149 @Override 3150 public Base makeProperty(int hash, String name) throws FHIRException { 3151 switch (hash) { 3152 case 116079: 3153 return getUrlElement(); 3154 case -1618432855: 3155 return addIdentifier(); 3156 case 351608024: 3157 return getVersionElement(); 3158 case 3373707: 3159 return getNameElement(); 3160 case 110371416: 3161 return getTitleElement(); 3162 case -430332865: 3163 return addReplacesElement(); 3164 case -892481550: 3165 return getStatusElement(); 3166 case -404562712: 3167 return getExperimentalElement(); 3168 case 3076014: 3169 return getDateElement(); 3170 case 1447404028: 3171 return getPublisherElement(); 3172 case 951526432: 3173 return addContact(); 3174 case -1724546052: 3175 return getDescriptionElement(); 3176 case -669707736: 3177 return addUseContext(); 3178 case -507075711: 3179 return addJurisdiction(); 3180 case -220463842: 3181 return getPurposeElement(); 3182 case 1522889671: 3183 return getCopyrightElement(); 3184 case 3016401: 3185 return getBaseElement(); 3186 case -995424086: 3187 return addParentElement(); 3188 case 278115238: 3189 return getEvent(); 3190 case 96891546: 3191 return getEvent(); 3192 case 50511102: 3193 return getCategoryElement(); 3194 case 97604824: 3195 return addFocus(); 3196 case 791597824: 3197 return getResponseRequiredElement(); 3198 case -1130933751: 3199 return addAllowedResponse(); 3200 case 98615630: 3201 return addGraphElement(); 3202 default: 3203 return super.makeProperty(hash, name); 3204 } 3205 3206 } 3207 3208 @Override 3209 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3210 switch (hash) { 3211 case 116079: 3212 /* url */ return new String[] { "uri" }; 3213 case -1618432855: 3214 /* identifier */ return new String[] { "Identifier" }; 3215 case 351608024: 3216 /* version */ return new String[] { "string" }; 3217 case 3373707: 3218 /* name */ return new String[] { "string" }; 3219 case 110371416: 3220 /* title */ return new String[] { "string" }; 3221 case -430332865: 3222 /* replaces */ return new String[] { "canonical" }; 3223 case -892481550: 3224 /* status */ return new String[] { "code" }; 3225 case -404562712: 3226 /* experimental */ return new String[] { "boolean" }; 3227 case 3076014: 3228 /* date */ return new String[] { "dateTime" }; 3229 case 1447404028: 3230 /* publisher */ return new String[] { "string" }; 3231 case 951526432: 3232 /* contact */ return new String[] { "ContactDetail" }; 3233 case -1724546052: 3234 /* description */ return new String[] { "markdown" }; 3235 case -669707736: 3236 /* useContext */ return new String[] { "UsageContext" }; 3237 case -507075711: 3238 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3239 case -220463842: 3240 /* purpose */ return new String[] { "markdown" }; 3241 case 1522889671: 3242 /* copyright */ return new String[] { "markdown" }; 3243 case 3016401: 3244 /* base */ return new String[] { "canonical" }; 3245 case -995424086: 3246 /* parent */ return new String[] { "canonical" }; 3247 case 96891546: 3248 /* event */ return new String[] { "Coding", "uri" }; 3249 case 50511102: 3250 /* category */ return new String[] { "code" }; 3251 case 97604824: 3252 /* focus */ return new String[] {}; 3253 case 791597824: 3254 /* responseRequired */ return new String[] { "code" }; 3255 case -1130933751: 3256 /* allowedResponse */ return new String[] {}; 3257 case 98615630: 3258 /* graph */ return new String[] { "canonical" }; 3259 default: 3260 return super.getTypesForProperty(hash, name); 3261 } 3262 3263 } 3264 3265 @Override 3266 public Base addChild(String name) throws FHIRException { 3267 if (name.equals("url")) { 3268 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.url"); 3269 } else if (name.equals("identifier")) { 3270 return addIdentifier(); 3271 } else if (name.equals("version")) { 3272 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.version"); 3273 } else if (name.equals("name")) { 3274 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.name"); 3275 } else if (name.equals("title")) { 3276 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.title"); 3277 } else if (name.equals("replaces")) { 3278 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.replaces"); 3279 } else if (name.equals("status")) { 3280 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.status"); 3281 } else if (name.equals("experimental")) { 3282 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.experimental"); 3283 } else if (name.equals("date")) { 3284 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.date"); 3285 } else if (name.equals("publisher")) { 3286 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.publisher"); 3287 } else if (name.equals("contact")) { 3288 return addContact(); 3289 } else if (name.equals("description")) { 3290 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.description"); 3291 } else if (name.equals("useContext")) { 3292 return addUseContext(); 3293 } else if (name.equals("jurisdiction")) { 3294 return addJurisdiction(); 3295 } else if (name.equals("purpose")) { 3296 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.purpose"); 3297 } else if (name.equals("copyright")) { 3298 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyright"); 3299 } else if (name.equals("base")) { 3300 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.base"); 3301 } else if (name.equals("parent")) { 3302 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.parent"); 3303 } else if (name.equals("eventCoding")) { 3304 this.event = new Coding(); 3305 return this.event; 3306 } else if (name.equals("eventUri")) { 3307 this.event = new UriType(); 3308 return this.event; 3309 } else if (name.equals("category")) { 3310 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.category"); 3311 } else if (name.equals("focus")) { 3312 return addFocus(); 3313 } else if (name.equals("responseRequired")) { 3314 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.responseRequired"); 3315 } else if (name.equals("allowedResponse")) { 3316 return addAllowedResponse(); 3317 } else if (name.equals("graph")) { 3318 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.graph"); 3319 } else 3320 return super.addChild(name); 3321 } 3322 3323 public String fhirType() { 3324 return "MessageDefinition"; 3325 3326 } 3327 3328 public MessageDefinition copy() { 3329 MessageDefinition dst = new MessageDefinition(); 3330 copyValues(dst); 3331 return dst; 3332 } 3333 3334 public void copyValues(MessageDefinition dst) { 3335 super.copyValues(dst); 3336 dst.url = url == null ? null : url.copy(); 3337 if (identifier != null) { 3338 dst.identifier = new ArrayList<Identifier>(); 3339 for (Identifier i : identifier) 3340 dst.identifier.add(i.copy()); 3341 } 3342 ; 3343 dst.version = version == null ? null : version.copy(); 3344 dst.name = name == null ? null : name.copy(); 3345 dst.title = title == null ? null : title.copy(); 3346 if (replaces != null) { 3347 dst.replaces = new ArrayList<CanonicalType>(); 3348 for (CanonicalType i : replaces) 3349 dst.replaces.add(i.copy()); 3350 } 3351 ; 3352 dst.status = status == null ? null : status.copy(); 3353 dst.experimental = experimental == null ? null : experimental.copy(); 3354 dst.date = date == null ? null : date.copy(); 3355 dst.publisher = publisher == null ? null : publisher.copy(); 3356 if (contact != null) { 3357 dst.contact = new ArrayList<ContactDetail>(); 3358 for (ContactDetail i : contact) 3359 dst.contact.add(i.copy()); 3360 } 3361 ; 3362 dst.description = description == null ? null : description.copy(); 3363 if (useContext != null) { 3364 dst.useContext = new ArrayList<UsageContext>(); 3365 for (UsageContext i : useContext) 3366 dst.useContext.add(i.copy()); 3367 } 3368 ; 3369 if (jurisdiction != null) { 3370 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3371 for (CodeableConcept i : jurisdiction) 3372 dst.jurisdiction.add(i.copy()); 3373 } 3374 ; 3375 dst.purpose = purpose == null ? null : purpose.copy(); 3376 dst.copyright = copyright == null ? null : copyright.copy(); 3377 dst.base = base == null ? null : base.copy(); 3378 if (parent != null) { 3379 dst.parent = new ArrayList<CanonicalType>(); 3380 for (CanonicalType i : parent) 3381 dst.parent.add(i.copy()); 3382 } 3383 ; 3384 dst.event = event == null ? null : event.copy(); 3385 dst.category = category == null ? null : category.copy(); 3386 if (focus != null) { 3387 dst.focus = new ArrayList<MessageDefinitionFocusComponent>(); 3388 for (MessageDefinitionFocusComponent i : focus) 3389 dst.focus.add(i.copy()); 3390 } 3391 ; 3392 dst.responseRequired = responseRequired == null ? null : responseRequired.copy(); 3393 if (allowedResponse != null) { 3394 dst.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 3395 for (MessageDefinitionAllowedResponseComponent i : allowedResponse) 3396 dst.allowedResponse.add(i.copy()); 3397 } 3398 ; 3399 if (graph != null) { 3400 dst.graph = new ArrayList<CanonicalType>(); 3401 for (CanonicalType i : graph) 3402 dst.graph.add(i.copy()); 3403 } 3404 ; 3405 } 3406 3407 protected MessageDefinition typedCopy() { 3408 return copy(); 3409 } 3410 3411 @Override 3412 public boolean equalsDeep(Base other_) { 3413 if (!super.equalsDeep(other_)) 3414 return false; 3415 if (!(other_ instanceof MessageDefinition)) 3416 return false; 3417 MessageDefinition o = (MessageDefinition) other_; 3418 return compareDeep(identifier, o.identifier, true) && compareDeep(replaces, o.replaces, true) 3419 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 3420 && compareDeep(base, o.base, true) && compareDeep(parent, o.parent, true) && compareDeep(event, o.event, true) 3421 && compareDeep(category, o.category, true) && compareDeep(focus, o.focus, true) 3422 && compareDeep(responseRequired, o.responseRequired, true) 3423 && compareDeep(allowedResponse, o.allowedResponse, true) && compareDeep(graph, o.graph, true); 3424 } 3425 3426 @Override 3427 public boolean equalsShallow(Base other_) { 3428 if (!super.equalsShallow(other_)) 3429 return false; 3430 if (!(other_ instanceof MessageDefinition)) 3431 return false; 3432 MessageDefinition o = (MessageDefinition) other_; 3433 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 3434 && compareValues(category, o.category, true) && compareValues(responseRequired, o.responseRequired, true); 3435 } 3436 3437 public boolean isEmpty() { 3438 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, replaces, purpose, copyright, base, 3439 parent, event, category, focus, responseRequired, allowedResponse, graph); 3440 } 3441 3442 @Override 3443 public ResourceType getResourceType() { 3444 return ResourceType.MessageDefinition; 3445 } 3446 3447 /** 3448 * Search parameter: <b>date</b> 3449 * <p> 3450 * Description: <b>The message definition publication date</b><br> 3451 * Type: <b>date</b><br> 3452 * Path: <b>MessageDefinition.date</b><br> 3453 * </p> 3454 */ 3455 @SearchParamDefinition(name = "date", path = "MessageDefinition.date", description = "The message definition publication date", type = "date") 3456 public static final String SP_DATE = "date"; 3457 /** 3458 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3459 * <p> 3460 * Description: <b>The message definition publication date</b><br> 3461 * Type: <b>date</b><br> 3462 * Path: <b>MessageDefinition.date</b><br> 3463 * </p> 3464 */ 3465 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3466 SP_DATE); 3467 3468 /** 3469 * Search parameter: <b>identifier</b> 3470 * <p> 3471 * Description: <b>External identifier for the message definition</b><br> 3472 * Type: <b>token</b><br> 3473 * Path: <b>MessageDefinition.identifier</b><br> 3474 * </p> 3475 */ 3476 @SearchParamDefinition(name = "identifier", path = "MessageDefinition.identifier", description = "External identifier for the message definition", type = "token") 3477 public static final String SP_IDENTIFIER = "identifier"; 3478 /** 3479 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3480 * <p> 3481 * Description: <b>External identifier for the message definition</b><br> 3482 * Type: <b>token</b><br> 3483 * Path: <b>MessageDefinition.identifier</b><br> 3484 * </p> 3485 */ 3486 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3487 SP_IDENTIFIER); 3488 3489 /** 3490 * Search parameter: <b>parent</b> 3491 * <p> 3492 * Description: <b>A resource that is the parent of the definition</b><br> 3493 * Type: <b>reference</b><br> 3494 * Path: <b>MessageDefinition.parent</b><br> 3495 * </p> 3496 */ 3497 @SearchParamDefinition(name = "parent", path = "MessageDefinition.parent", description = "A resource that is the parent of the definition", type = "reference", target = { 3498 ActivityDefinition.class, PlanDefinition.class }) 3499 public static final String SP_PARENT = "parent"; 3500 /** 3501 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 3502 * <p> 3503 * Description: <b>A resource that is the parent of the definition</b><br> 3504 * Type: <b>reference</b><br> 3505 * Path: <b>MessageDefinition.parent</b><br> 3506 * </p> 3507 */ 3508 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3509 SP_PARENT); 3510 3511 /** 3512 * Constant for fluent queries to be used to add include statements. Specifies 3513 * the path value of "<b>MessageDefinition:parent</b>". 3514 */ 3515 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 3516 "MessageDefinition:parent").toLocked(); 3517 3518 /** 3519 * Search parameter: <b>context-type-value</b> 3520 * <p> 3521 * Description: <b>A use context type and value assigned to the message 3522 * definition</b><br> 3523 * Type: <b>composite</b><br> 3524 * Path: <b></b><br> 3525 * </p> 3526 */ 3527 @SearchParamDefinition(name = "context-type-value", path = "MessageDefinition.useContext", description = "A use context type and value assigned to the message definition", type = "composite", compositeOf = { 3528 "context-type", "context" }) 3529 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3530 /** 3531 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3532 * <p> 3533 * Description: <b>A use context type and value assigned to the message 3534 * definition</b><br> 3535 * Type: <b>composite</b><br> 3536 * Path: <b></b><br> 3537 * </p> 3538 */ 3539 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3540 SP_CONTEXT_TYPE_VALUE); 3541 3542 /** 3543 * Search parameter: <b>jurisdiction</b> 3544 * <p> 3545 * Description: <b>Intended jurisdiction for the message definition</b><br> 3546 * Type: <b>token</b><br> 3547 * Path: <b>MessageDefinition.jurisdiction</b><br> 3548 * </p> 3549 */ 3550 @SearchParamDefinition(name = "jurisdiction", path = "MessageDefinition.jurisdiction", description = "Intended jurisdiction for the message definition", type = "token") 3551 public static final String SP_JURISDICTION = "jurisdiction"; 3552 /** 3553 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3554 * <p> 3555 * Description: <b>Intended jurisdiction for the message definition</b><br> 3556 * Type: <b>token</b><br> 3557 * Path: <b>MessageDefinition.jurisdiction</b><br> 3558 * </p> 3559 */ 3560 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3561 SP_JURISDICTION); 3562 3563 /** 3564 * Search parameter: <b>description</b> 3565 * <p> 3566 * Description: <b>The description of the message definition</b><br> 3567 * Type: <b>string</b><br> 3568 * Path: <b>MessageDefinition.description</b><br> 3569 * </p> 3570 */ 3571 @SearchParamDefinition(name = "description", path = "MessageDefinition.description", description = "The description of the message definition", type = "string") 3572 public static final String SP_DESCRIPTION = "description"; 3573 /** 3574 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3575 * <p> 3576 * Description: <b>The description of the message definition</b><br> 3577 * Type: <b>string</b><br> 3578 * Path: <b>MessageDefinition.description</b><br> 3579 * </p> 3580 */ 3581 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3582 SP_DESCRIPTION); 3583 3584 /** 3585 * Search parameter: <b>focus</b> 3586 * <p> 3587 * Description: <b>A resource that is a permitted focus of the message</b><br> 3588 * Type: <b>token</b><br> 3589 * Path: <b>MessageDefinition.focus.code</b><br> 3590 * </p> 3591 */ 3592 @SearchParamDefinition(name = "focus", path = "MessageDefinition.focus.code", description = "A resource that is a permitted focus of the message", type = "token") 3593 public static final String SP_FOCUS = "focus"; 3594 /** 3595 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 3596 * <p> 3597 * Description: <b>A resource that is a permitted focus of the message</b><br> 3598 * Type: <b>token</b><br> 3599 * Path: <b>MessageDefinition.focus.code</b><br> 3600 * </p> 3601 */ 3602 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3603 SP_FOCUS); 3604 3605 /** 3606 * Search parameter: <b>context-type</b> 3607 * <p> 3608 * Description: <b>A type of use context assigned to the message 3609 * definition</b><br> 3610 * Type: <b>token</b><br> 3611 * Path: <b>MessageDefinition.useContext.code</b><br> 3612 * </p> 3613 */ 3614 @SearchParamDefinition(name = "context-type", path = "MessageDefinition.useContext.code", description = "A type of use context assigned to the message definition", type = "token") 3615 public static final String SP_CONTEXT_TYPE = "context-type"; 3616 /** 3617 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3618 * <p> 3619 * Description: <b>A type of use context assigned to the message 3620 * definition</b><br> 3621 * Type: <b>token</b><br> 3622 * Path: <b>MessageDefinition.useContext.code</b><br> 3623 * </p> 3624 */ 3625 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3626 SP_CONTEXT_TYPE); 3627 3628 /** 3629 * Search parameter: <b>title</b> 3630 * <p> 3631 * Description: <b>The human-friendly name of the message definition</b><br> 3632 * Type: <b>string</b><br> 3633 * Path: <b>MessageDefinition.title</b><br> 3634 * </p> 3635 */ 3636 @SearchParamDefinition(name = "title", path = "MessageDefinition.title", description = "The human-friendly name of the message definition", type = "string") 3637 public static final String SP_TITLE = "title"; 3638 /** 3639 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3640 * <p> 3641 * Description: <b>The human-friendly name of the message definition</b><br> 3642 * Type: <b>string</b><br> 3643 * Path: <b>MessageDefinition.title</b><br> 3644 * </p> 3645 */ 3646 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3647 SP_TITLE); 3648 3649 /** 3650 * Search parameter: <b>version</b> 3651 * <p> 3652 * Description: <b>The business version of the message definition</b><br> 3653 * Type: <b>token</b><br> 3654 * Path: <b>MessageDefinition.version</b><br> 3655 * </p> 3656 */ 3657 @SearchParamDefinition(name = "version", path = "MessageDefinition.version", description = "The business version of the message definition", type = "token") 3658 public static final String SP_VERSION = "version"; 3659 /** 3660 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3661 * <p> 3662 * Description: <b>The business version of the message definition</b><br> 3663 * Type: <b>token</b><br> 3664 * Path: <b>MessageDefinition.version</b><br> 3665 * </p> 3666 */ 3667 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3668 SP_VERSION); 3669 3670 /** 3671 * Search parameter: <b>url</b> 3672 * <p> 3673 * Description: <b>The uri that identifies the message definition</b><br> 3674 * Type: <b>uri</b><br> 3675 * Path: <b>MessageDefinition.url</b><br> 3676 * </p> 3677 */ 3678 @SearchParamDefinition(name = "url", path = "MessageDefinition.url", description = "The uri that identifies the message definition", type = "uri") 3679 public static final String SP_URL = "url"; 3680 /** 3681 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3682 * <p> 3683 * Description: <b>The uri that identifies the message definition</b><br> 3684 * Type: <b>uri</b><br> 3685 * Path: <b>MessageDefinition.url</b><br> 3686 * </p> 3687 */ 3688 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3689 3690 /** 3691 * Search parameter: <b>context-quantity</b> 3692 * <p> 3693 * Description: <b>A quantity- or range-valued use context assigned to the 3694 * message definition</b><br> 3695 * Type: <b>quantity</b><br> 3696 * Path: <b>MessageDefinition.useContext.valueQuantity, 3697 * MessageDefinition.useContext.valueRange</b><br> 3698 * </p> 3699 */ 3700 @SearchParamDefinition(name = "context-quantity", path = "(MessageDefinition.useContext.value as Quantity) | (MessageDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the message definition", type = "quantity") 3701 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3702 /** 3703 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3704 * <p> 3705 * Description: <b>A quantity- or range-valued use context assigned to the 3706 * message definition</b><br> 3707 * Type: <b>quantity</b><br> 3708 * Path: <b>MessageDefinition.useContext.valueQuantity, 3709 * MessageDefinition.useContext.valueRange</b><br> 3710 * </p> 3711 */ 3712 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3713 SP_CONTEXT_QUANTITY); 3714 3715 /** 3716 * Search parameter: <b>name</b> 3717 * <p> 3718 * Description: <b>Computationally friendly name of the message 3719 * definition</b><br> 3720 * Type: <b>string</b><br> 3721 * Path: <b>MessageDefinition.name</b><br> 3722 * </p> 3723 */ 3724 @SearchParamDefinition(name = "name", path = "MessageDefinition.name", description = "Computationally friendly name of the message definition", type = "string") 3725 public static final String SP_NAME = "name"; 3726 /** 3727 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3728 * <p> 3729 * Description: <b>Computationally friendly name of the message 3730 * definition</b><br> 3731 * Type: <b>string</b><br> 3732 * Path: <b>MessageDefinition.name</b><br> 3733 * </p> 3734 */ 3735 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3736 SP_NAME); 3737 3738 /** 3739 * Search parameter: <b>context</b> 3740 * <p> 3741 * Description: <b>A use context assigned to the message definition</b><br> 3742 * Type: <b>token</b><br> 3743 * Path: <b>MessageDefinition.useContext.valueCodeableConcept</b><br> 3744 * </p> 3745 */ 3746 @SearchParamDefinition(name = "context", path = "(MessageDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the message definition", type = "token") 3747 public static final String SP_CONTEXT = "context"; 3748 /** 3749 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3750 * <p> 3751 * Description: <b>A use context assigned to the message definition</b><br> 3752 * Type: <b>token</b><br> 3753 * Path: <b>MessageDefinition.useContext.valueCodeableConcept</b><br> 3754 * </p> 3755 */ 3756 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3757 SP_CONTEXT); 3758 3759 /** 3760 * Search parameter: <b>publisher</b> 3761 * <p> 3762 * Description: <b>Name of the publisher of the message definition</b><br> 3763 * Type: <b>string</b><br> 3764 * Path: <b>MessageDefinition.publisher</b><br> 3765 * </p> 3766 */ 3767 @SearchParamDefinition(name = "publisher", path = "MessageDefinition.publisher", description = "Name of the publisher of the message definition", type = "string") 3768 public static final String SP_PUBLISHER = "publisher"; 3769 /** 3770 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3771 * <p> 3772 * Description: <b>Name of the publisher of the message definition</b><br> 3773 * Type: <b>string</b><br> 3774 * Path: <b>MessageDefinition.publisher</b><br> 3775 * </p> 3776 */ 3777 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3778 SP_PUBLISHER); 3779 3780 /** 3781 * Search parameter: <b>event</b> 3782 * <p> 3783 * Description: <b>The event that triggers the message or link to the event 3784 * definition.</b><br> 3785 * Type: <b>token</b><br> 3786 * Path: <b>MessageDefinition.event[x]</b><br> 3787 * </p> 3788 */ 3789 @SearchParamDefinition(name = "event", path = "MessageDefinition.event", description = "The event that triggers the message or link to the event definition.", type = "token") 3790 public static final String SP_EVENT = "event"; 3791 /** 3792 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3793 * <p> 3794 * Description: <b>The event that triggers the message or link to the event 3795 * definition.</b><br> 3796 * Type: <b>token</b><br> 3797 * Path: <b>MessageDefinition.event[x]</b><br> 3798 * </p> 3799 */ 3800 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3801 SP_EVENT); 3802 3803 /** 3804 * Search parameter: <b>category</b> 3805 * <p> 3806 * Description: <b>The behavior associated with the message</b><br> 3807 * Type: <b>token</b><br> 3808 * Path: <b>MessageDefinition.category</b><br> 3809 * </p> 3810 */ 3811 @SearchParamDefinition(name = "category", path = "MessageDefinition.category", description = "The behavior associated with the message", type = "token") 3812 public static final String SP_CATEGORY = "category"; 3813 /** 3814 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3815 * <p> 3816 * Description: <b>The behavior associated with the message</b><br> 3817 * Type: <b>token</b><br> 3818 * Path: <b>MessageDefinition.category</b><br> 3819 * </p> 3820 */ 3821 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3822 SP_CATEGORY); 3823 3824 /** 3825 * Search parameter: <b>context-type-quantity</b> 3826 * <p> 3827 * Description: <b>A use context type and quantity- or range-based value 3828 * assigned to the message definition</b><br> 3829 * Type: <b>composite</b><br> 3830 * Path: <b></b><br> 3831 * </p> 3832 */ 3833 @SearchParamDefinition(name = "context-type-quantity", path = "MessageDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the message definition", type = "composite", compositeOf = { 3834 "context-type", "context-quantity" }) 3835 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3836 /** 3837 * <b>Fluent Client</b> search parameter constant for 3838 * <b>context-type-quantity</b> 3839 * <p> 3840 * Description: <b>A use context type and quantity- or range-based value 3841 * assigned to the message definition</b><br> 3842 * Type: <b>composite</b><br> 3843 * Path: <b></b><br> 3844 * </p> 3845 */ 3846 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3847 SP_CONTEXT_TYPE_QUANTITY); 3848 3849 /** 3850 * Search parameter: <b>status</b> 3851 * <p> 3852 * Description: <b>The current status of the message definition</b><br> 3853 * Type: <b>token</b><br> 3854 * Path: <b>MessageDefinition.status</b><br> 3855 * </p> 3856 */ 3857 @SearchParamDefinition(name = "status", path = "MessageDefinition.status", description = "The current status of the message definition", type = "token") 3858 public static final String SP_STATUS = "status"; 3859 /** 3860 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3861 * <p> 3862 * Description: <b>The current status of the message definition</b><br> 3863 * Type: <b>token</b><br> 3864 * Path: <b>MessageDefinition.status</b><br> 3865 * </p> 3866 */ 3867 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3868 SP_STATUS); 3869 3870}