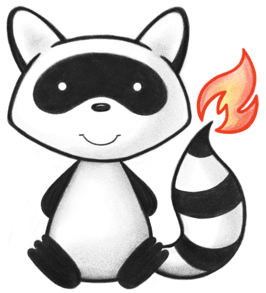
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The header for a message exchange that is either requesting or responding to 048 * an action. The reference(s) that are the subject of the action as well as 049 * other information related to the action are typically transmitted in a bundle 050 * in which the MessageHeader resource instance is the first resource in the 051 * bundle. 052 */ 053@ResourceDef(name = "MessageHeader", profile = "http://hl7.org/fhir/StructureDefinition/MessageHeader") 054public class MessageHeader extends DomainResource { 055 056 public enum ResponseType { 057 /** 058 * The message was accepted and processed without error. 059 */ 060 OK, 061 /** 062 * Some internal unexpected error occurred - wait and try again. Note - this is 063 * usually used for things like database unavailable, which may be expected to 064 * resolve, though human intervention may be required. 065 */ 066 TRANSIENTERROR, 067 /** 068 * The message was rejected because of a problem with the content. There is no 069 * point in re-sending without change. The response narrative SHALL describe the 070 * issue. 071 */ 072 FATALERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static ResponseType fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("ok".equals(codeString)) 082 return OK; 083 if ("transient-error".equals(codeString)) 084 return TRANSIENTERROR; 085 if ("fatal-error".equals(codeString)) 086 return FATALERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown ResponseType code '" + codeString + "'"); 091 } 092 093 public String toCode() { 094 switch (this) { 095 case OK: 096 return "ok"; 097 case TRANSIENTERROR: 098 return "transient-error"; 099 case FATALERROR: 100 return "fatal-error"; 101 case NULL: 102 return null; 103 default: 104 return "?"; 105 } 106 } 107 108 public String getSystem() { 109 switch (this) { 110 case OK: 111 return "http://hl7.org/fhir/response-code"; 112 case TRANSIENTERROR: 113 return "http://hl7.org/fhir/response-code"; 114 case FATALERROR: 115 return "http://hl7.org/fhir/response-code"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case OK: 126 return "The message was accepted and processed without error."; 127 case TRANSIENTERROR: 128 return "Some internal unexpected error occurred - wait and try again. Note - this is usually used for things like database unavailable, which may be expected to resolve, though human intervention may be required."; 129 case FATALERROR: 130 return "The message was rejected because of a problem with the content. There is no point in re-sending without change. The response narrative SHALL describe the issue."; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDisplay() { 139 switch (this) { 140 case OK: 141 return "OK"; 142 case TRANSIENTERROR: 143 return "Transient Error"; 144 case FATALERROR: 145 return "Fatal Error"; 146 case NULL: 147 return null; 148 default: 149 return "?"; 150 } 151 } 152 } 153 154 public static class ResponseTypeEnumFactory implements EnumFactory<ResponseType> { 155 public ResponseType fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("ok".equals(codeString)) 160 return ResponseType.OK; 161 if ("transient-error".equals(codeString)) 162 return ResponseType.TRANSIENTERROR; 163 if ("fatal-error".equals(codeString)) 164 return ResponseType.FATALERROR; 165 throw new IllegalArgumentException("Unknown ResponseType code '" + codeString + "'"); 166 } 167 168 public Enumeration<ResponseType> fromType(PrimitiveType<?> code) throws FHIRException { 169 if (code == null) 170 return null; 171 if (code.isEmpty()) 172 return new Enumeration<ResponseType>(this, ResponseType.NULL, code); 173 String codeString = code.asStringValue(); 174 if (codeString == null || "".equals(codeString)) 175 return new Enumeration<ResponseType>(this, ResponseType.NULL, code); 176 if ("ok".equals(codeString)) 177 return new Enumeration<ResponseType>(this, ResponseType.OK, code); 178 if ("transient-error".equals(codeString)) 179 return new Enumeration<ResponseType>(this, ResponseType.TRANSIENTERROR, code); 180 if ("fatal-error".equals(codeString)) 181 return new Enumeration<ResponseType>(this, ResponseType.FATALERROR, code); 182 throw new FHIRException("Unknown ResponseType code '" + codeString + "'"); 183 } 184 185 public String toCode(ResponseType code) { 186 if (code == ResponseType.NULL) 187 return null; 188 if (code == ResponseType.OK) 189 return "ok"; 190 if (code == ResponseType.TRANSIENTERROR) 191 return "transient-error"; 192 if (code == ResponseType.FATALERROR) 193 return "fatal-error"; 194 return "?"; 195 } 196 197 public String toSystem(ResponseType code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class MessageDestinationComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * Human-readable name for the target system. 206 */ 207 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 208 @Description(shortDefinition = "Name of system", formalDefinition = "Human-readable name for the target system.") 209 protected StringType name; 210 211 /** 212 * Identifies the target end system in situations where the initial message 213 * transmission is to an intermediary system. 214 */ 215 @Child(name = "target", type = { Device.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 216 @Description(shortDefinition = "Particular delivery destination within the destination", formalDefinition = "Identifies the target end system in situations where the initial message transmission is to an intermediary system.") 217 protected Reference target; 218 219 /** 220 * The actual object that is the target of the reference (Identifies the target 221 * end system in situations where the initial message transmission is to an 222 * intermediary system.) 223 */ 224 protected Device targetTarget; 225 226 /** 227 * Indicates where the message should be routed to. 228 */ 229 @Child(name = "endpoint", type = { UrlType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 230 @Description(shortDefinition = "Actual destination address or id", formalDefinition = "Indicates where the message should be routed to.") 231 protected UrlType endpoint; 232 233 /** 234 * Allows data conveyed by a message to be addressed to a particular person or 235 * department when routing to a specific application isn't sufficient. 236 */ 237 @Child(name = "receiver", type = { Practitioner.class, PractitionerRole.class, 238 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 239 @Description(shortDefinition = "Intended \"real-world\" recipient for the data", formalDefinition = "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.") 240 protected Reference receiver; 241 242 /** 243 * The actual object that is the target of the reference (Allows data conveyed 244 * by a message to be addressed to a particular person or department when 245 * routing to a specific application isn't sufficient.) 246 */ 247 protected Resource receiverTarget; 248 249 private static final long serialVersionUID = 611064500L; 250 251 /** 252 * Constructor 253 */ 254 public MessageDestinationComponent() { 255 super(); 256 } 257 258 /** 259 * Constructor 260 */ 261 public MessageDestinationComponent(UrlType endpoint) { 262 super(); 263 this.endpoint = endpoint; 264 } 265 266 /** 267 * @return {@link #name} (Human-readable name for the target system.). This is 268 * the underlying object with id, value and extensions. The accessor 269 * "getName" gives direct access to the value 270 */ 271 public StringType getNameElement() { 272 if (this.name == null) 273 if (Configuration.errorOnAutoCreate()) 274 throw new Error("Attempt to auto-create MessageDestinationComponent.name"); 275 else if (Configuration.doAutoCreate()) 276 this.name = new StringType(); // bb 277 return this.name; 278 } 279 280 public boolean hasNameElement() { 281 return this.name != null && !this.name.isEmpty(); 282 } 283 284 public boolean hasName() { 285 return this.name != null && !this.name.isEmpty(); 286 } 287 288 /** 289 * @param value {@link #name} (Human-readable name for the target system.). This 290 * is the underlying object with id, value and extensions. The 291 * accessor "getName" gives direct access to the value 292 */ 293 public MessageDestinationComponent setNameElement(StringType value) { 294 this.name = value; 295 return this; 296 } 297 298 /** 299 * @return Human-readable name for the target system. 300 */ 301 public String getName() { 302 return this.name == null ? null : this.name.getValue(); 303 } 304 305 /** 306 * @param value Human-readable name for the target system. 307 */ 308 public MessageDestinationComponent setName(String value) { 309 if (Utilities.noString(value)) 310 this.name = null; 311 else { 312 if (this.name == null) 313 this.name = new StringType(); 314 this.name.setValue(value); 315 } 316 return this; 317 } 318 319 /** 320 * @return {@link #target} (Identifies the target end system in situations where 321 * the initial message transmission is to an intermediary system.) 322 */ 323 public Reference getTarget() { 324 if (this.target == null) 325 if (Configuration.errorOnAutoCreate()) 326 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 327 else if (Configuration.doAutoCreate()) 328 this.target = new Reference(); // cc 329 return this.target; 330 } 331 332 public boolean hasTarget() { 333 return this.target != null && !this.target.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #target} (Identifies the target end system in situations 338 * where the initial message transmission is to an intermediary 339 * system.) 340 */ 341 public MessageDestinationComponent setTarget(Reference value) { 342 this.target = value; 343 return this; 344 } 345 346 /** 347 * @return {@link #target} The actual object that is the target of the 348 * reference. The reference library doesn't populate this, but you can 349 * use it to hold the resource if you resolve it. (Identifies the target 350 * end system in situations where the initial message transmission is to 351 * an intermediary system.) 352 */ 353 public Device getTargetTarget() { 354 if (this.targetTarget == null) 355 if (Configuration.errorOnAutoCreate()) 356 throw new Error("Attempt to auto-create MessageDestinationComponent.target"); 357 else if (Configuration.doAutoCreate()) 358 this.targetTarget = new Device(); // aa 359 return this.targetTarget; 360 } 361 362 /** 363 * @param value {@link #target} The actual object that is the target of the 364 * reference. The reference library doesn't use these, but you can 365 * use it to hold the resource if you resolve it. (Identifies the 366 * target end system in situations where the initial message 367 * transmission is to an intermediary system.) 368 */ 369 public MessageDestinationComponent setTargetTarget(Device value) { 370 this.targetTarget = value; 371 return this; 372 } 373 374 /** 375 * @return {@link #endpoint} (Indicates where the message should be routed to.). 376 * This is the underlying object with id, value and extensions. The 377 * accessor "getEndpoint" gives direct access to the value 378 */ 379 public UrlType getEndpointElement() { 380 if (this.endpoint == null) 381 if (Configuration.errorOnAutoCreate()) 382 throw new Error("Attempt to auto-create MessageDestinationComponent.endpoint"); 383 else if (Configuration.doAutoCreate()) 384 this.endpoint = new UrlType(); // bb 385 return this.endpoint; 386 } 387 388 public boolean hasEndpointElement() { 389 return this.endpoint != null && !this.endpoint.isEmpty(); 390 } 391 392 public boolean hasEndpoint() { 393 return this.endpoint != null && !this.endpoint.isEmpty(); 394 } 395 396 /** 397 * @param value {@link #endpoint} (Indicates where the message should be routed 398 * to.). This is the underlying object with id, value and 399 * extensions. The accessor "getEndpoint" gives direct access to 400 * the value 401 */ 402 public MessageDestinationComponent setEndpointElement(UrlType value) { 403 this.endpoint = value; 404 return this; 405 } 406 407 /** 408 * @return Indicates where the message should be routed to. 409 */ 410 public String getEndpoint() { 411 return this.endpoint == null ? null : this.endpoint.getValue(); 412 } 413 414 /** 415 * @param value Indicates where the message should be routed to. 416 */ 417 public MessageDestinationComponent setEndpoint(String value) { 418 if (this.endpoint == null) 419 this.endpoint = new UrlType(); 420 this.endpoint.setValue(value); 421 return this; 422 } 423 424 /** 425 * @return {@link #receiver} (Allows data conveyed by a message to be addressed 426 * to a particular person or department when routing to a specific 427 * application isn't sufficient.) 428 */ 429 public Reference getReceiver() { 430 if (this.receiver == null) 431 if (Configuration.errorOnAutoCreate()) 432 throw new Error("Attempt to auto-create MessageDestinationComponent.receiver"); 433 else if (Configuration.doAutoCreate()) 434 this.receiver = new Reference(); // cc 435 return this.receiver; 436 } 437 438 public boolean hasReceiver() { 439 return this.receiver != null && !this.receiver.isEmpty(); 440 } 441 442 /** 443 * @param value {@link #receiver} (Allows data conveyed by a message to be 444 * addressed to a particular person or department when routing to a 445 * specific application isn't sufficient.) 446 */ 447 public MessageDestinationComponent setReceiver(Reference value) { 448 this.receiver = value; 449 return this; 450 } 451 452 /** 453 * @return {@link #receiver} The actual object that is the target of the 454 * reference. The reference library doesn't populate this, but you can 455 * use it to hold the resource if you resolve it. (Allows data conveyed 456 * by a message to be addressed to a particular person or department 457 * when routing to a specific application isn't sufficient.) 458 */ 459 public Resource getReceiverTarget() { 460 return this.receiverTarget; 461 } 462 463 /** 464 * @param value {@link #receiver} The actual object that is the target of the 465 * reference. The reference library doesn't use these, but you can 466 * use it to hold the resource if you resolve it. (Allows data 467 * conveyed by a message to be addressed to a particular person or 468 * department when routing to a specific application isn't 469 * sufficient.) 470 */ 471 public MessageDestinationComponent setReceiverTarget(Resource value) { 472 this.receiverTarget = value; 473 return this; 474 } 475 476 protected void listChildren(List<Property> children) { 477 super.listChildren(children); 478 children.add(new Property("name", "string", "Human-readable name for the target system.", 0, 1, name)); 479 children.add(new Property("target", "Reference(Device)", 480 "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 481 0, 1, target)); 482 children.add(new Property("endpoint", "url", "Indicates where the message should be routed to.", 0, 1, endpoint)); 483 children.add(new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", 484 "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 485 0, 1, receiver)); 486 } 487 488 @Override 489 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 490 switch (_hash) { 491 case 3373707: 492 /* name */ return new Property("name", "string", "Human-readable name for the target system.", 0, 1, name); 493 case -880905839: 494 /* target */ return new Property("target", "Reference(Device)", 495 "Identifies the target end system in situations where the initial message transmission is to an intermediary system.", 496 0, 1, target); 497 case 1741102485: 498 /* endpoint */ return new Property("endpoint", "url", "Indicates where the message should be routed to.", 0, 1, 499 endpoint); 500 case -808719889: 501 /* receiver */ return new Property("receiver", "Reference(Practitioner|PractitionerRole|Organization)", 502 "Allows data conveyed by a message to be addressed to a particular person or department when routing to a specific application isn't sufficient.", 503 0, 1, receiver); 504 default: 505 return super.getNamedProperty(_hash, _name, _checkValid); 506 } 507 508 } 509 510 @Override 511 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 512 switch (hash) { 513 case 3373707: 514 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 515 case -880905839: 516 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Reference 517 case 1741102485: 518 /* endpoint */ return this.endpoint == null ? new Base[0] : new Base[] { this.endpoint }; // UrlType 519 case -808719889: 520 /* receiver */ return this.receiver == null ? new Base[0] : new Base[] { this.receiver }; // Reference 521 default: 522 return super.getProperty(hash, name, checkValid); 523 } 524 525 } 526 527 @Override 528 public Base setProperty(int hash, String name, Base value) throws FHIRException { 529 switch (hash) { 530 case 3373707: // name 531 this.name = castToString(value); // StringType 532 return value; 533 case -880905839: // target 534 this.target = castToReference(value); // Reference 535 return value; 536 case 1741102485: // endpoint 537 this.endpoint = castToUrl(value); // UrlType 538 return value; 539 case -808719889: // receiver 540 this.receiver = castToReference(value); // Reference 541 return value; 542 default: 543 return super.setProperty(hash, name, value); 544 } 545 546 } 547 548 @Override 549 public Base setProperty(String name, Base value) throws FHIRException { 550 if (name.equals("name")) { 551 this.name = castToString(value); // StringType 552 } else if (name.equals("target")) { 553 this.target = castToReference(value); // Reference 554 } else if (name.equals("endpoint")) { 555 this.endpoint = castToUrl(value); // UrlType 556 } else if (name.equals("receiver")) { 557 this.receiver = castToReference(value); // Reference 558 } else 559 return super.setProperty(name, value); 560 return value; 561 } 562 563 @Override 564 public void removeChild(String name, Base value) throws FHIRException { 565 if (name.equals("name")) { 566 this.name = null; 567 } else if (name.equals("target")) { 568 this.target = null; 569 } else if (name.equals("endpoint")) { 570 this.endpoint = null; 571 } else if (name.equals("receiver")) { 572 this.receiver = null; 573 } else 574 super.removeChild(name, value); 575 576 } 577 578 @Override 579 public Base makeProperty(int hash, String name) throws FHIRException { 580 switch (hash) { 581 case 3373707: 582 return getNameElement(); 583 case -880905839: 584 return getTarget(); 585 case 1741102485: 586 return getEndpointElement(); 587 case -808719889: 588 return getReceiver(); 589 default: 590 return super.makeProperty(hash, name); 591 } 592 593 } 594 595 @Override 596 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 597 switch (hash) { 598 case 3373707: 599 /* name */ return new String[] { "string" }; 600 case -880905839: 601 /* target */ return new String[] { "Reference" }; 602 case 1741102485: 603 /* endpoint */ return new String[] { "url" }; 604 case -808719889: 605 /* receiver */ return new String[] { "Reference" }; 606 default: 607 return super.getTypesForProperty(hash, name); 608 } 609 610 } 611 612 @Override 613 public Base addChild(String name) throws FHIRException { 614 if (name.equals("name")) { 615 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.name"); 616 } else if (name.equals("target")) { 617 this.target = new Reference(); 618 return this.target; 619 } else if (name.equals("endpoint")) { 620 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.endpoint"); 621 } else if (name.equals("receiver")) { 622 this.receiver = new Reference(); 623 return this.receiver; 624 } else 625 return super.addChild(name); 626 } 627 628 public MessageDestinationComponent copy() { 629 MessageDestinationComponent dst = new MessageDestinationComponent(); 630 copyValues(dst); 631 return dst; 632 } 633 634 public void copyValues(MessageDestinationComponent dst) { 635 super.copyValues(dst); 636 dst.name = name == null ? null : name.copy(); 637 dst.target = target == null ? null : target.copy(); 638 dst.endpoint = endpoint == null ? null : endpoint.copy(); 639 dst.receiver = receiver == null ? null : receiver.copy(); 640 } 641 642 @Override 643 public boolean equalsDeep(Base other_) { 644 if (!super.equalsDeep(other_)) 645 return false; 646 if (!(other_ instanceof MessageDestinationComponent)) 647 return false; 648 MessageDestinationComponent o = (MessageDestinationComponent) other_; 649 return compareDeep(name, o.name, true) && compareDeep(target, o.target, true) 650 && compareDeep(endpoint, o.endpoint, true) && compareDeep(receiver, o.receiver, true); 651 } 652 653 @Override 654 public boolean equalsShallow(Base other_) { 655 if (!super.equalsShallow(other_)) 656 return false; 657 if (!(other_ instanceof MessageDestinationComponent)) 658 return false; 659 MessageDestinationComponent o = (MessageDestinationComponent) other_; 660 return compareValues(name, o.name, true) && compareValues(endpoint, o.endpoint, true); 661 } 662 663 public boolean isEmpty() { 664 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, target, endpoint, receiver); 665 } 666 667 public String fhirType() { 668 return "MessageHeader.destination"; 669 670 } 671 672 } 673 674 @Block() 675 public static class MessageSourceComponent extends BackboneElement implements IBaseBackboneElement { 676 /** 677 * Human-readable name for the source system. 678 */ 679 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 680 @Description(shortDefinition = "Name of system", formalDefinition = "Human-readable name for the source system.") 681 protected StringType name; 682 683 /** 684 * May include configuration or other information useful in debugging. 685 */ 686 @Child(name = "software", type = { 687 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 688 @Description(shortDefinition = "Name of software running the system", formalDefinition = "May include configuration or other information useful in debugging.") 689 protected StringType software; 690 691 /** 692 * Can convey versions of multiple systems in situations where a message passes 693 * through multiple hands. 694 */ 695 @Child(name = "version", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 696 @Description(shortDefinition = "Version of software running", formalDefinition = "Can convey versions of multiple systems in situations where a message passes through multiple hands.") 697 protected StringType version; 698 699 /** 700 * An e-mail, phone, website or other contact point to use to resolve issues 701 * with message communications. 702 */ 703 @Child(name = "contact", type = { 704 ContactPoint.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 705 @Description(shortDefinition = "Human contact for problems", formalDefinition = "An e-mail, phone, website or other contact point to use to resolve issues with message communications.") 706 protected ContactPoint contact; 707 708 /** 709 * Identifies the routing target to send acknowledgements to. 710 */ 711 @Child(name = "endpoint", type = { UrlType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 712 @Description(shortDefinition = "Actual message source address or id", formalDefinition = "Identifies the routing target to send acknowledgements to.") 713 protected UrlType endpoint; 714 715 private static final long serialVersionUID = -350916401L; 716 717 /** 718 * Constructor 719 */ 720 public MessageSourceComponent() { 721 super(); 722 } 723 724 /** 725 * Constructor 726 */ 727 public MessageSourceComponent(UrlType endpoint) { 728 super(); 729 this.endpoint = endpoint; 730 } 731 732 /** 733 * @return {@link #name} (Human-readable name for the source system.). This is 734 * the underlying object with id, value and extensions. The accessor 735 * "getName" gives direct access to the value 736 */ 737 public StringType getNameElement() { 738 if (this.name == null) 739 if (Configuration.errorOnAutoCreate()) 740 throw new Error("Attempt to auto-create MessageSourceComponent.name"); 741 else if (Configuration.doAutoCreate()) 742 this.name = new StringType(); // bb 743 return this.name; 744 } 745 746 public boolean hasNameElement() { 747 return this.name != null && !this.name.isEmpty(); 748 } 749 750 public boolean hasName() { 751 return this.name != null && !this.name.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #name} (Human-readable name for the source system.). This 756 * is the underlying object with id, value and extensions. The 757 * accessor "getName" gives direct access to the value 758 */ 759 public MessageSourceComponent setNameElement(StringType value) { 760 this.name = value; 761 return this; 762 } 763 764 /** 765 * @return Human-readable name for the source system. 766 */ 767 public String getName() { 768 return this.name == null ? null : this.name.getValue(); 769 } 770 771 /** 772 * @param value Human-readable name for the source system. 773 */ 774 public MessageSourceComponent setName(String value) { 775 if (Utilities.noString(value)) 776 this.name = null; 777 else { 778 if (this.name == null) 779 this.name = new StringType(); 780 this.name.setValue(value); 781 } 782 return this; 783 } 784 785 /** 786 * @return {@link #software} (May include configuration or other information 787 * useful in debugging.). This is the underlying object with id, value 788 * and extensions. The accessor "getSoftware" gives direct access to the 789 * value 790 */ 791 public StringType getSoftwareElement() { 792 if (this.software == null) 793 if (Configuration.errorOnAutoCreate()) 794 throw new Error("Attempt to auto-create MessageSourceComponent.software"); 795 else if (Configuration.doAutoCreate()) 796 this.software = new StringType(); // bb 797 return this.software; 798 } 799 800 public boolean hasSoftwareElement() { 801 return this.software != null && !this.software.isEmpty(); 802 } 803 804 public boolean hasSoftware() { 805 return this.software != null && !this.software.isEmpty(); 806 } 807 808 /** 809 * @param value {@link #software} (May include configuration or other 810 * information useful in debugging.). This is the underlying object 811 * with id, value and extensions. The accessor "getSoftware" gives 812 * direct access to the value 813 */ 814 public MessageSourceComponent setSoftwareElement(StringType value) { 815 this.software = value; 816 return this; 817 } 818 819 /** 820 * @return May include configuration or other information useful in debugging. 821 */ 822 public String getSoftware() { 823 return this.software == null ? null : this.software.getValue(); 824 } 825 826 /** 827 * @param value May include configuration or other information useful in 828 * debugging. 829 */ 830 public MessageSourceComponent setSoftware(String value) { 831 if (Utilities.noString(value)) 832 this.software = null; 833 else { 834 if (this.software == null) 835 this.software = new StringType(); 836 this.software.setValue(value); 837 } 838 return this; 839 } 840 841 /** 842 * @return {@link #version} (Can convey versions of multiple systems in 843 * situations where a message passes through multiple hands.). This is 844 * the underlying object with id, value and extensions. The accessor 845 * "getVersion" gives direct access to the value 846 */ 847 public StringType getVersionElement() { 848 if (this.version == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create MessageSourceComponent.version"); 851 else if (Configuration.doAutoCreate()) 852 this.version = new StringType(); // bb 853 return this.version; 854 } 855 856 public boolean hasVersionElement() { 857 return this.version != null && !this.version.isEmpty(); 858 } 859 860 public boolean hasVersion() { 861 return this.version != null && !this.version.isEmpty(); 862 } 863 864 /** 865 * @param value {@link #version} (Can convey versions of multiple systems in 866 * situations where a message passes through multiple hands.). This 867 * is the underlying object with id, value and extensions. The 868 * accessor "getVersion" gives direct access to the value 869 */ 870 public MessageSourceComponent setVersionElement(StringType value) { 871 this.version = value; 872 return this; 873 } 874 875 /** 876 * @return Can convey versions of multiple systems in situations where a message 877 * passes through multiple hands. 878 */ 879 public String getVersion() { 880 return this.version == null ? null : this.version.getValue(); 881 } 882 883 /** 884 * @param value Can convey versions of multiple systems in situations where a 885 * message passes through multiple hands. 886 */ 887 public MessageSourceComponent setVersion(String value) { 888 if (Utilities.noString(value)) 889 this.version = null; 890 else { 891 if (this.version == null) 892 this.version = new StringType(); 893 this.version.setValue(value); 894 } 895 return this; 896 } 897 898 /** 899 * @return {@link #contact} (An e-mail, phone, website or other contact point to 900 * use to resolve issues with message communications.) 901 */ 902 public ContactPoint getContact() { 903 if (this.contact == null) 904 if (Configuration.errorOnAutoCreate()) 905 throw new Error("Attempt to auto-create MessageSourceComponent.contact"); 906 else if (Configuration.doAutoCreate()) 907 this.contact = new ContactPoint(); // cc 908 return this.contact; 909 } 910 911 public boolean hasContact() { 912 return this.contact != null && !this.contact.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #contact} (An e-mail, phone, website or other contact 917 * point to use to resolve issues with message communications.) 918 */ 919 public MessageSourceComponent setContact(ContactPoint value) { 920 this.contact = value; 921 return this; 922 } 923 924 /** 925 * @return {@link #endpoint} (Identifies the routing target to send 926 * acknowledgements to.). This is the underlying object with id, value 927 * and extensions. The accessor "getEndpoint" gives direct access to the 928 * value 929 */ 930 public UrlType getEndpointElement() { 931 if (this.endpoint == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create MessageSourceComponent.endpoint"); 934 else if (Configuration.doAutoCreate()) 935 this.endpoint = new UrlType(); // bb 936 return this.endpoint; 937 } 938 939 public boolean hasEndpointElement() { 940 return this.endpoint != null && !this.endpoint.isEmpty(); 941 } 942 943 public boolean hasEndpoint() { 944 return this.endpoint != null && !this.endpoint.isEmpty(); 945 } 946 947 /** 948 * @param value {@link #endpoint} (Identifies the routing target to send 949 * acknowledgements to.). This is the underlying object with id, 950 * value and extensions. The accessor "getEndpoint" gives direct 951 * access to the value 952 */ 953 public MessageSourceComponent setEndpointElement(UrlType value) { 954 this.endpoint = value; 955 return this; 956 } 957 958 /** 959 * @return Identifies the routing target to send acknowledgements to. 960 */ 961 public String getEndpoint() { 962 return this.endpoint == null ? null : this.endpoint.getValue(); 963 } 964 965 /** 966 * @param value Identifies the routing target to send acknowledgements to. 967 */ 968 public MessageSourceComponent setEndpoint(String value) { 969 if (this.endpoint == null) 970 this.endpoint = new UrlType(); 971 this.endpoint.setValue(value); 972 return this; 973 } 974 975 protected void listChildren(List<Property> children) { 976 super.listChildren(children); 977 children.add(new Property("name", "string", "Human-readable name for the source system.", 0, 1, name)); 978 children.add(new Property("software", "string", 979 "May include configuration or other information useful in debugging.", 0, 1, software)); 980 children.add(new Property("version", "string", 981 "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 1, 982 version)); 983 children.add(new Property("contact", "ContactPoint", 984 "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 985 1, contact)); 986 children.add(new Property("endpoint", "url", "Identifies the routing target to send acknowledgements to.", 0, 1, 987 endpoint)); 988 } 989 990 @Override 991 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 992 switch (_hash) { 993 case 3373707: 994 /* name */ return new Property("name", "string", "Human-readable name for the source system.", 0, 1, name); 995 case 1319330215: 996 /* software */ return new Property("software", "string", 997 "May include configuration or other information useful in debugging.", 0, 1, software); 998 case 351608024: 999 /* version */ return new Property("version", "string", 1000 "Can convey versions of multiple systems in situations where a message passes through multiple hands.", 0, 1001 1, version); 1002 case 951526432: 1003 /* contact */ return new Property("contact", "ContactPoint", 1004 "An e-mail, phone, website or other contact point to use to resolve issues with message communications.", 0, 1005 1, contact); 1006 case 1741102485: 1007 /* endpoint */ return new Property("endpoint", "url", 1008 "Identifies the routing target to send acknowledgements to.", 0, 1, endpoint); 1009 default: 1010 return super.getNamedProperty(_hash, _name, _checkValid); 1011 } 1012 1013 } 1014 1015 @Override 1016 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1017 switch (hash) { 1018 case 3373707: 1019 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1020 case 1319330215: 1021 /* software */ return this.software == null ? new Base[0] : new Base[] { this.software }; // StringType 1022 case 351608024: 1023 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1024 case 951526432: 1025 /* contact */ return this.contact == null ? new Base[0] : new Base[] { this.contact }; // ContactPoint 1026 case 1741102485: 1027 /* endpoint */ return this.endpoint == null ? new Base[0] : new Base[] { this.endpoint }; // UrlType 1028 default: 1029 return super.getProperty(hash, name, checkValid); 1030 } 1031 1032 } 1033 1034 @Override 1035 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1036 switch (hash) { 1037 case 3373707: // name 1038 this.name = castToString(value); // StringType 1039 return value; 1040 case 1319330215: // software 1041 this.software = castToString(value); // StringType 1042 return value; 1043 case 351608024: // version 1044 this.version = castToString(value); // StringType 1045 return value; 1046 case 951526432: // contact 1047 this.contact = castToContactPoint(value); // ContactPoint 1048 return value; 1049 case 1741102485: // endpoint 1050 this.endpoint = castToUrl(value); // UrlType 1051 return value; 1052 default: 1053 return super.setProperty(hash, name, value); 1054 } 1055 1056 } 1057 1058 @Override 1059 public Base setProperty(String name, Base value) throws FHIRException { 1060 if (name.equals("name")) { 1061 this.name = castToString(value); // StringType 1062 } else if (name.equals("software")) { 1063 this.software = castToString(value); // StringType 1064 } else if (name.equals("version")) { 1065 this.version = castToString(value); // StringType 1066 } else if (name.equals("contact")) { 1067 this.contact = castToContactPoint(value); // ContactPoint 1068 } else if (name.equals("endpoint")) { 1069 this.endpoint = castToUrl(value); // UrlType 1070 } else 1071 return super.setProperty(name, value); 1072 return value; 1073 } 1074 1075 @Override 1076 public void removeChild(String name, Base value) throws FHIRException { 1077 if (name.equals("name")) { 1078 this.name = null; 1079 } else if (name.equals("software")) { 1080 this.software = null; 1081 } else if (name.equals("version")) { 1082 this.version = null; 1083 } else if (name.equals("contact")) { 1084 this.contact = null; 1085 } else if (name.equals("endpoint")) { 1086 this.endpoint = null; 1087 } else 1088 super.removeChild(name, value); 1089 1090 } 1091 1092 @Override 1093 public Base makeProperty(int hash, String name) throws FHIRException { 1094 switch (hash) { 1095 case 3373707: 1096 return getNameElement(); 1097 case 1319330215: 1098 return getSoftwareElement(); 1099 case 351608024: 1100 return getVersionElement(); 1101 case 951526432: 1102 return getContact(); 1103 case 1741102485: 1104 return getEndpointElement(); 1105 default: 1106 return super.makeProperty(hash, name); 1107 } 1108 1109 } 1110 1111 @Override 1112 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1113 switch (hash) { 1114 case 3373707: 1115 /* name */ return new String[] { "string" }; 1116 case 1319330215: 1117 /* software */ return new String[] { "string" }; 1118 case 351608024: 1119 /* version */ return new String[] { "string" }; 1120 case 951526432: 1121 /* contact */ return new String[] { "ContactPoint" }; 1122 case 1741102485: 1123 /* endpoint */ return new String[] { "url" }; 1124 default: 1125 return super.getTypesForProperty(hash, name); 1126 } 1127 1128 } 1129 1130 @Override 1131 public Base addChild(String name) throws FHIRException { 1132 if (name.equals("name")) { 1133 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.name"); 1134 } else if (name.equals("software")) { 1135 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.software"); 1136 } else if (name.equals("version")) { 1137 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.version"); 1138 } else if (name.equals("contact")) { 1139 this.contact = new ContactPoint(); 1140 return this.contact; 1141 } else if (name.equals("endpoint")) { 1142 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.endpoint"); 1143 } else 1144 return super.addChild(name); 1145 } 1146 1147 public MessageSourceComponent copy() { 1148 MessageSourceComponent dst = new MessageSourceComponent(); 1149 copyValues(dst); 1150 return dst; 1151 } 1152 1153 public void copyValues(MessageSourceComponent dst) { 1154 super.copyValues(dst); 1155 dst.name = name == null ? null : name.copy(); 1156 dst.software = software == null ? null : software.copy(); 1157 dst.version = version == null ? null : version.copy(); 1158 dst.contact = contact == null ? null : contact.copy(); 1159 dst.endpoint = endpoint == null ? null : endpoint.copy(); 1160 } 1161 1162 @Override 1163 public boolean equalsDeep(Base other_) { 1164 if (!super.equalsDeep(other_)) 1165 return false; 1166 if (!(other_ instanceof MessageSourceComponent)) 1167 return false; 1168 MessageSourceComponent o = (MessageSourceComponent) other_; 1169 return compareDeep(name, o.name, true) && compareDeep(software, o.software, true) 1170 && compareDeep(version, o.version, true) && compareDeep(contact, o.contact, true) 1171 && compareDeep(endpoint, o.endpoint, true); 1172 } 1173 1174 @Override 1175 public boolean equalsShallow(Base other_) { 1176 if (!super.equalsShallow(other_)) 1177 return false; 1178 if (!(other_ instanceof MessageSourceComponent)) 1179 return false; 1180 MessageSourceComponent o = (MessageSourceComponent) other_; 1181 return compareValues(name, o.name, true) && compareValues(software, o.software, true) 1182 && compareValues(version, o.version, true) && compareValues(endpoint, o.endpoint, true); 1183 } 1184 1185 public boolean isEmpty() { 1186 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, software, version, contact, endpoint); 1187 } 1188 1189 public String fhirType() { 1190 return "MessageHeader.source"; 1191 1192 } 1193 1194 } 1195 1196 @Block() 1197 public static class MessageHeaderResponseComponent extends BackboneElement implements IBaseBackboneElement { 1198 /** 1199 * The MessageHeader.id of the message to which this message is a response. 1200 */ 1201 @Child(name = "identifier", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1202 @Description(shortDefinition = "Id of original message", formalDefinition = "The MessageHeader.id of the message to which this message is a response.") 1203 protected IdType identifier; 1204 1205 /** 1206 * Code that identifies the type of response to the message - whether it was 1207 * successful or not, and whether it should be resent or not. 1208 */ 1209 @Child(name = "code", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1210 @Description(shortDefinition = "ok | transient-error | fatal-error", formalDefinition = "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.") 1211 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/response-code") 1212 protected Enumeration<ResponseType> code; 1213 1214 /** 1215 * Full details of any issues found in the message. 1216 */ 1217 @Child(name = "details", type = { 1218 OperationOutcome.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1219 @Description(shortDefinition = "Specific list of hints/warnings/errors", formalDefinition = "Full details of any issues found in the message.") 1220 protected Reference details; 1221 1222 /** 1223 * The actual object that is the target of the reference (Full details of any 1224 * issues found in the message.) 1225 */ 1226 protected OperationOutcome detailsTarget; 1227 1228 private static final long serialVersionUID = -1008716838L; 1229 1230 /** 1231 * Constructor 1232 */ 1233 public MessageHeaderResponseComponent() { 1234 super(); 1235 } 1236 1237 /** 1238 * Constructor 1239 */ 1240 public MessageHeaderResponseComponent(IdType identifier, Enumeration<ResponseType> code) { 1241 super(); 1242 this.identifier = identifier; 1243 this.code = code; 1244 } 1245 1246 /** 1247 * @return {@link #identifier} (The MessageHeader.id of the message to which 1248 * this message is a response.). This is the underlying object with id, 1249 * value and extensions. The accessor "getIdentifier" gives direct 1250 * access to the value 1251 */ 1252 public IdType getIdentifierElement() { 1253 if (this.identifier == null) 1254 if (Configuration.errorOnAutoCreate()) 1255 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.identifier"); 1256 else if (Configuration.doAutoCreate()) 1257 this.identifier = new IdType(); // bb 1258 return this.identifier; 1259 } 1260 1261 public boolean hasIdentifierElement() { 1262 return this.identifier != null && !this.identifier.isEmpty(); 1263 } 1264 1265 public boolean hasIdentifier() { 1266 return this.identifier != null && !this.identifier.isEmpty(); 1267 } 1268 1269 /** 1270 * @param value {@link #identifier} (The MessageHeader.id of the message to 1271 * which this message is a response.). This is the underlying 1272 * object with id, value and extensions. The accessor 1273 * "getIdentifier" gives direct access to the value 1274 */ 1275 public MessageHeaderResponseComponent setIdentifierElement(IdType value) { 1276 this.identifier = value; 1277 return this; 1278 } 1279 1280 /** 1281 * @return The MessageHeader.id of the message to which this message is a 1282 * response. 1283 */ 1284 public String getIdentifier() { 1285 return this.identifier == null ? null : this.identifier.getValue(); 1286 } 1287 1288 /** 1289 * @param value The MessageHeader.id of the message to which this message is a 1290 * response. 1291 */ 1292 public MessageHeaderResponseComponent setIdentifier(String value) { 1293 if (this.identifier == null) 1294 this.identifier = new IdType(); 1295 this.identifier.setValue(value); 1296 return this; 1297 } 1298 1299 /** 1300 * @return {@link #code} (Code that identifies the type of response to the 1301 * message - whether it was successful or not, and whether it should be 1302 * resent or not.). This is the underlying object with id, value and 1303 * extensions. The accessor "getCode" gives direct access to the value 1304 */ 1305 public Enumeration<ResponseType> getCodeElement() { 1306 if (this.code == null) 1307 if (Configuration.errorOnAutoCreate()) 1308 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.code"); 1309 else if (Configuration.doAutoCreate()) 1310 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); // bb 1311 return this.code; 1312 } 1313 1314 public boolean hasCodeElement() { 1315 return this.code != null && !this.code.isEmpty(); 1316 } 1317 1318 public boolean hasCode() { 1319 return this.code != null && !this.code.isEmpty(); 1320 } 1321 1322 /** 1323 * @param value {@link #code} (Code that identifies the type of response to the 1324 * message - whether it was successful or not, and whether it 1325 * should be resent or not.). This is the underlying object with 1326 * id, value and extensions. The accessor "getCode" gives direct 1327 * access to the value 1328 */ 1329 public MessageHeaderResponseComponent setCodeElement(Enumeration<ResponseType> value) { 1330 this.code = value; 1331 return this; 1332 } 1333 1334 /** 1335 * @return Code that identifies the type of response to the message - whether it 1336 * was successful or not, and whether it should be resent or not. 1337 */ 1338 public ResponseType getCode() { 1339 return this.code == null ? null : this.code.getValue(); 1340 } 1341 1342 /** 1343 * @param value Code that identifies the type of response to the message - 1344 * whether it was successful or not, and whether it should be 1345 * resent or not. 1346 */ 1347 public MessageHeaderResponseComponent setCode(ResponseType value) { 1348 if (this.code == null) 1349 this.code = new Enumeration<ResponseType>(new ResponseTypeEnumFactory()); 1350 this.code.setValue(value); 1351 return this; 1352 } 1353 1354 /** 1355 * @return {@link #details} (Full details of any issues found in the message.) 1356 */ 1357 public Reference getDetails() { 1358 if (this.details == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 1361 else if (Configuration.doAutoCreate()) 1362 this.details = new Reference(); // cc 1363 return this.details; 1364 } 1365 1366 public boolean hasDetails() { 1367 return this.details != null && !this.details.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #details} (Full details of any issues found in the 1372 * message.) 1373 */ 1374 public MessageHeaderResponseComponent setDetails(Reference value) { 1375 this.details = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #details} The actual object that is the target of the 1381 * reference. The reference library doesn't populate this, but you can 1382 * use it to hold the resource if you resolve it. (Full details of any 1383 * issues found in the message.) 1384 */ 1385 public OperationOutcome getDetailsTarget() { 1386 if (this.detailsTarget == null) 1387 if (Configuration.errorOnAutoCreate()) 1388 throw new Error("Attempt to auto-create MessageHeaderResponseComponent.details"); 1389 else if (Configuration.doAutoCreate()) 1390 this.detailsTarget = new OperationOutcome(); // aa 1391 return this.detailsTarget; 1392 } 1393 1394 /** 1395 * @param value {@link #details} The actual object that is the target of the 1396 * reference. The reference library doesn't use these, but you can 1397 * use it to hold the resource if you resolve it. (Full details of 1398 * any issues found in the message.) 1399 */ 1400 public MessageHeaderResponseComponent setDetailsTarget(OperationOutcome value) { 1401 this.detailsTarget = value; 1402 return this; 1403 } 1404 1405 protected void listChildren(List<Property> children) { 1406 super.listChildren(children); 1407 children.add(new Property("identifier", "id", 1408 "The MessageHeader.id of the message to which this message is a response.", 0, 1, identifier)); 1409 children.add(new Property("code", "code", 1410 "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 1411 0, 1, code)); 1412 children.add(new Property("details", "Reference(OperationOutcome)", 1413 "Full details of any issues found in the message.", 0, 1, details)); 1414 } 1415 1416 @Override 1417 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1418 switch (_hash) { 1419 case -1618432855: 1420 /* identifier */ return new Property("identifier", "id", 1421 "The MessageHeader.id of the message to which this message is a response.", 0, 1, identifier); 1422 case 3059181: 1423 /* code */ return new Property("code", "code", 1424 "Code that identifies the type of response to the message - whether it was successful or not, and whether it should be resent or not.", 1425 0, 1, code); 1426 case 1557721666: 1427 /* details */ return new Property("details", "Reference(OperationOutcome)", 1428 "Full details of any issues found in the message.", 0, 1, details); 1429 default: 1430 return super.getNamedProperty(_hash, _name, _checkValid); 1431 } 1432 1433 } 1434 1435 @Override 1436 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1437 switch (hash) { 1438 case -1618432855: 1439 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // IdType 1440 case 3059181: 1441 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<ResponseType> 1442 case 1557721666: 1443 /* details */ return this.details == null ? new Base[0] : new Base[] { this.details }; // Reference 1444 default: 1445 return super.getProperty(hash, name, checkValid); 1446 } 1447 1448 } 1449 1450 @Override 1451 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1452 switch (hash) { 1453 case -1618432855: // identifier 1454 this.identifier = castToId(value); // IdType 1455 return value; 1456 case 3059181: // code 1457 value = new ResponseTypeEnumFactory().fromType(castToCode(value)); 1458 this.code = (Enumeration) value; // Enumeration<ResponseType> 1459 return value; 1460 case 1557721666: // details 1461 this.details = castToReference(value); // Reference 1462 return value; 1463 default: 1464 return super.setProperty(hash, name, value); 1465 } 1466 1467 } 1468 1469 @Override 1470 public Base setProperty(String name, Base value) throws FHIRException { 1471 if (name.equals("identifier")) { 1472 this.identifier = castToId(value); // IdType 1473 } else if (name.equals("code")) { 1474 value = new ResponseTypeEnumFactory().fromType(castToCode(value)); 1475 this.code = (Enumeration) value; // Enumeration<ResponseType> 1476 } else if (name.equals("details")) { 1477 this.details = castToReference(value); // Reference 1478 } else 1479 return super.setProperty(name, value); 1480 return value; 1481 } 1482 1483 @Override 1484 public void removeChild(String name, Base value) throws FHIRException { 1485 if (name.equals("identifier")) { 1486 this.identifier = null; 1487 } else if (name.equals("code")) { 1488 this.code = null; 1489 } else if (name.equals("details")) { 1490 this.details = null; 1491 } else 1492 super.removeChild(name, value); 1493 1494 } 1495 1496 @Override 1497 public Base makeProperty(int hash, String name) throws FHIRException { 1498 switch (hash) { 1499 case -1618432855: 1500 return getIdentifierElement(); 1501 case 3059181: 1502 return getCodeElement(); 1503 case 1557721666: 1504 return getDetails(); 1505 default: 1506 return super.makeProperty(hash, name); 1507 } 1508 1509 } 1510 1511 @Override 1512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1513 switch (hash) { 1514 case -1618432855: 1515 /* identifier */ return new String[] { "id" }; 1516 case 3059181: 1517 /* code */ return new String[] { "code" }; 1518 case 1557721666: 1519 /* details */ return new String[] { "Reference" }; 1520 default: 1521 return super.getTypesForProperty(hash, name); 1522 } 1523 1524 } 1525 1526 @Override 1527 public Base addChild(String name) throws FHIRException { 1528 if (name.equals("identifier")) { 1529 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.identifier"); 1530 } else if (name.equals("code")) { 1531 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.code"); 1532 } else if (name.equals("details")) { 1533 this.details = new Reference(); 1534 return this.details; 1535 } else 1536 return super.addChild(name); 1537 } 1538 1539 public MessageHeaderResponseComponent copy() { 1540 MessageHeaderResponseComponent dst = new MessageHeaderResponseComponent(); 1541 copyValues(dst); 1542 return dst; 1543 } 1544 1545 public void copyValues(MessageHeaderResponseComponent dst) { 1546 super.copyValues(dst); 1547 dst.identifier = identifier == null ? null : identifier.copy(); 1548 dst.code = code == null ? null : code.copy(); 1549 dst.details = details == null ? null : details.copy(); 1550 } 1551 1552 @Override 1553 public boolean equalsDeep(Base other_) { 1554 if (!super.equalsDeep(other_)) 1555 return false; 1556 if (!(other_ instanceof MessageHeaderResponseComponent)) 1557 return false; 1558 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other_; 1559 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 1560 && compareDeep(details, o.details, true); 1561 } 1562 1563 @Override 1564 public boolean equalsShallow(Base other_) { 1565 if (!super.equalsShallow(other_)) 1566 return false; 1567 if (!(other_ instanceof MessageHeaderResponseComponent)) 1568 return false; 1569 MessageHeaderResponseComponent o = (MessageHeaderResponseComponent) other_; 1570 return compareValues(identifier, o.identifier, true) && compareValues(code, o.code, true); 1571 } 1572 1573 public boolean isEmpty() { 1574 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, details); 1575 } 1576 1577 public String fhirType() { 1578 return "MessageHeader.response"; 1579 1580 } 1581 1582 } 1583 1584 /** 1585 * Code that identifies the event this message represents and connects it with 1586 * its definition. Events defined as part of the FHIR specification have the 1587 * system value "http://terminology.hl7.org/CodeSystem/message-events". 1588 * Alternatively uri to the EventDefinition. 1589 */ 1590 @Child(name = "event", type = { Coding.class, 1591 UriType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1592 @Description(shortDefinition = "Code for the event this message represents or link to event definition", formalDefinition = "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://terminology.hl7.org/CodeSystem/message-events\". Alternatively uri to the EventDefinition.") 1593 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-events") 1594 protected Type event; 1595 1596 /** 1597 * The destination application which the message is intended for. 1598 */ 1599 @Child(name = "destination", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1600 @Description(shortDefinition = "Message destination application(s)", formalDefinition = "The destination application which the message is intended for.") 1601 protected List<MessageDestinationComponent> destination; 1602 1603 /** 1604 * Identifies the sending system to allow the use of a trust relationship. 1605 */ 1606 @Child(name = "sender", type = { Practitioner.class, PractitionerRole.class, 1607 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1608 @Description(shortDefinition = "Real world sender of the message", formalDefinition = "Identifies the sending system to allow the use of a trust relationship.") 1609 protected Reference sender; 1610 1611 /** 1612 * The actual object that is the target of the reference (Identifies the sending 1613 * system to allow the use of a trust relationship.) 1614 */ 1615 protected Resource senderTarget; 1616 1617 /** 1618 * The person or device that performed the data entry leading to this message. 1619 * When there is more than one candidate, pick the most proximal to the message. 1620 * Can provide other enterers in extensions. 1621 */ 1622 @Child(name = "enterer", type = { Practitioner.class, 1623 PractitionerRole.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1624 @Description(shortDefinition = "The source of the data entry", formalDefinition = "The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.") 1625 protected Reference enterer; 1626 1627 /** 1628 * The actual object that is the target of the reference (The person or device 1629 * that performed the data entry leading to this message. When there is more 1630 * than one candidate, pick the most proximal to the message. Can provide other 1631 * enterers in extensions.) 1632 */ 1633 protected Resource entererTarget; 1634 1635 /** 1636 * The logical author of the message - the person or device that decided the 1637 * described event should happen. When there is more than one candidate, pick 1638 * the most proximal to the MessageHeader. Can provide other authors in 1639 * extensions. 1640 */ 1641 @Child(name = "author", type = { Practitioner.class, 1642 PractitionerRole.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1643 @Description(shortDefinition = "The source of the decision", formalDefinition = "The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.") 1644 protected Reference author; 1645 1646 /** 1647 * The actual object that is the target of the reference (The logical author of 1648 * the message - the person or device that decided the described event should 1649 * happen. When there is more than one candidate, pick the most proximal to the 1650 * MessageHeader. Can provide other authors in extensions.) 1651 */ 1652 protected Resource authorTarget; 1653 1654 /** 1655 * The source application from which this message originated. 1656 */ 1657 @Child(name = "source", type = {}, order = 5, min = 1, max = 1, modifier = false, summary = true) 1658 @Description(shortDefinition = "Message source application", formalDefinition = "The source application from which this message originated.") 1659 protected MessageSourceComponent source; 1660 1661 /** 1662 * The person or organization that accepts overall responsibility for the 1663 * contents of the message. The implication is that the message event happened 1664 * under the policies of the responsible party. 1665 */ 1666 @Child(name = "responsible", type = { Practitioner.class, PractitionerRole.class, 1667 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1668 @Description(shortDefinition = "Final responsibility for event", formalDefinition = "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.") 1669 protected Reference responsible; 1670 1671 /** 1672 * The actual object that is the target of the reference (The person or 1673 * organization that accepts overall responsibility for the contents of the 1674 * message. The implication is that the message event happened under the 1675 * policies of the responsible party.) 1676 */ 1677 protected Resource responsibleTarget; 1678 1679 /** 1680 * Coded indication of the cause for the event - indicates a reason for the 1681 * occurrence of the event that is a focus of this message. 1682 */ 1683 @Child(name = "reason", type = { 1684 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1685 @Description(shortDefinition = "Cause of event", formalDefinition = "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.") 1686 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-reason-encounter") 1687 protected CodeableConcept reason; 1688 1689 /** 1690 * Information about the message that this message is a response to. Only 1691 * present if this message is a response. 1692 */ 1693 @Child(name = "response", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = true) 1694 @Description(shortDefinition = "If this is a reply to prior message", formalDefinition = "Information about the message that this message is a response to. Only present if this message is a response.") 1695 protected MessageHeaderResponseComponent response; 1696 1697 /** 1698 * The actual data of the message - a reference to the root/focus class of the 1699 * event. 1700 */ 1701 @Child(name = "focus", type = { 1702 Reference.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1703 @Description(shortDefinition = "The actual content of the message", formalDefinition = "The actual data of the message - a reference to the root/focus class of the event.") 1704 protected List<Reference> focus; 1705 /** 1706 * The actual objects that are the target of the reference (The actual data of 1707 * the message - a reference to the root/focus class of the event.) 1708 */ 1709 protected List<Resource> focusTarget; 1710 1711 /** 1712 * Permanent link to the MessageDefinition for this message. 1713 */ 1714 @Child(name = "definition", type = { 1715 CanonicalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1716 @Description(shortDefinition = "Link to the definition for this message", formalDefinition = "Permanent link to the MessageDefinition for this message.") 1717 protected CanonicalType definition; 1718 1719 private static final long serialVersionUID = -1039408819L; 1720 1721 /** 1722 * Constructor 1723 */ 1724 public MessageHeader() { 1725 super(); 1726 } 1727 1728 /** 1729 * Constructor 1730 */ 1731 public MessageHeader(Type event, MessageSourceComponent source) { 1732 super(); 1733 this.event = event; 1734 this.source = source; 1735 } 1736 1737 /** 1738 * @return {@link #event} (Code that identifies the event this message 1739 * represents and connects it with its definition. Events defined as 1740 * part of the FHIR specification have the system value 1741 * "http://terminology.hl7.org/CodeSystem/message-events". Alternatively 1742 * uri to the EventDefinition.) 1743 */ 1744 public Type getEvent() { 1745 return this.event; 1746 } 1747 1748 /** 1749 * @return {@link #event} (Code that identifies the event this message 1750 * represents and connects it with its definition. Events defined as 1751 * part of the FHIR specification have the system value 1752 * "http://terminology.hl7.org/CodeSystem/message-events". Alternatively 1753 * uri to the EventDefinition.) 1754 */ 1755 public Coding getEventCoding() throws FHIRException { 1756 if (this.event == null) 1757 this.event = new Coding(); 1758 if (!(this.event instanceof Coding)) 1759 throw new FHIRException( 1760 "Type mismatch: the type Coding was expected, but " + this.event.getClass().getName() + " was encountered"); 1761 return (Coding) this.event; 1762 } 1763 1764 public boolean hasEventCoding() { 1765 return this.event instanceof Coding; 1766 } 1767 1768 /** 1769 * @return {@link #event} (Code that identifies the event this message 1770 * represents and connects it with its definition. Events defined as 1771 * part of the FHIR specification have the system value 1772 * "http://terminology.hl7.org/CodeSystem/message-events". Alternatively 1773 * uri to the EventDefinition.) 1774 */ 1775 public UriType getEventUriType() throws FHIRException { 1776 if (this.event == null) 1777 this.event = new UriType(); 1778 if (!(this.event instanceof UriType)) 1779 throw new FHIRException( 1780 "Type mismatch: the type UriType was expected, but " + this.event.getClass().getName() + " was encountered"); 1781 return (UriType) this.event; 1782 } 1783 1784 public boolean hasEventUriType() { 1785 return this.event instanceof UriType; 1786 } 1787 1788 public boolean hasEvent() { 1789 return this.event != null && !this.event.isEmpty(); 1790 } 1791 1792 /** 1793 * @param value {@link #event} (Code that identifies the event this message 1794 * represents and connects it with its definition. Events defined 1795 * as part of the FHIR specification have the system value 1796 * "http://terminology.hl7.org/CodeSystem/message-events". 1797 * Alternatively uri to the EventDefinition.) 1798 */ 1799 public MessageHeader setEvent(Type value) { 1800 if (value != null && !(value instanceof Coding || value instanceof UriType)) 1801 throw new Error("Not the right type for MessageHeader.event[x]: " + value.fhirType()); 1802 this.event = value; 1803 return this; 1804 } 1805 1806 /** 1807 * @return {@link #destination} (The destination application which the message 1808 * is intended for.) 1809 */ 1810 public List<MessageDestinationComponent> getDestination() { 1811 if (this.destination == null) 1812 this.destination = new ArrayList<MessageDestinationComponent>(); 1813 return this.destination; 1814 } 1815 1816 /** 1817 * @return Returns a reference to <code>this</code> for easy method chaining 1818 */ 1819 public MessageHeader setDestination(List<MessageDestinationComponent> theDestination) { 1820 this.destination = theDestination; 1821 return this; 1822 } 1823 1824 public boolean hasDestination() { 1825 if (this.destination == null) 1826 return false; 1827 for (MessageDestinationComponent item : this.destination) 1828 if (!item.isEmpty()) 1829 return true; 1830 return false; 1831 } 1832 1833 public MessageDestinationComponent addDestination() { // 3 1834 MessageDestinationComponent t = new MessageDestinationComponent(); 1835 if (this.destination == null) 1836 this.destination = new ArrayList<MessageDestinationComponent>(); 1837 this.destination.add(t); 1838 return t; 1839 } 1840 1841 public MessageHeader addDestination(MessageDestinationComponent t) { // 3 1842 if (t == null) 1843 return this; 1844 if (this.destination == null) 1845 this.destination = new ArrayList<MessageDestinationComponent>(); 1846 this.destination.add(t); 1847 return this; 1848 } 1849 1850 /** 1851 * @return The first repetition of repeating field {@link #destination}, 1852 * creating it if it does not already exist 1853 */ 1854 public MessageDestinationComponent getDestinationFirstRep() { 1855 if (getDestination().isEmpty()) { 1856 addDestination(); 1857 } 1858 return getDestination().get(0); 1859 } 1860 1861 /** 1862 * @return {@link #sender} (Identifies the sending system to allow the use of a 1863 * trust relationship.) 1864 */ 1865 public Reference getSender() { 1866 if (this.sender == null) 1867 if (Configuration.errorOnAutoCreate()) 1868 throw new Error("Attempt to auto-create MessageHeader.sender"); 1869 else if (Configuration.doAutoCreate()) 1870 this.sender = new Reference(); // cc 1871 return this.sender; 1872 } 1873 1874 public boolean hasSender() { 1875 return this.sender != null && !this.sender.isEmpty(); 1876 } 1877 1878 /** 1879 * @param value {@link #sender} (Identifies the sending system to allow the use 1880 * of a trust relationship.) 1881 */ 1882 public MessageHeader setSender(Reference value) { 1883 this.sender = value; 1884 return this; 1885 } 1886 1887 /** 1888 * @return {@link #sender} The actual object that is the target of the 1889 * reference. The reference library doesn't populate this, but you can 1890 * use it to hold the resource if you resolve it. (Identifies the 1891 * sending system to allow the use of a trust relationship.) 1892 */ 1893 public Resource getSenderTarget() { 1894 return this.senderTarget; 1895 } 1896 1897 /** 1898 * @param value {@link #sender} The actual object that is the target of the 1899 * reference. The reference library doesn't use these, but you can 1900 * use it to hold the resource if you resolve it. (Identifies the 1901 * sending system to allow the use of a trust relationship.) 1902 */ 1903 public MessageHeader setSenderTarget(Resource value) { 1904 this.senderTarget = value; 1905 return this; 1906 } 1907 1908 /** 1909 * @return {@link #enterer} (The person or device that performed the data entry 1910 * leading to this message. When there is more than one candidate, pick 1911 * the most proximal to the message. Can provide other enterers in 1912 * extensions.) 1913 */ 1914 public Reference getEnterer() { 1915 if (this.enterer == null) 1916 if (Configuration.errorOnAutoCreate()) 1917 throw new Error("Attempt to auto-create MessageHeader.enterer"); 1918 else if (Configuration.doAutoCreate()) 1919 this.enterer = new Reference(); // cc 1920 return this.enterer; 1921 } 1922 1923 public boolean hasEnterer() { 1924 return this.enterer != null && !this.enterer.isEmpty(); 1925 } 1926 1927 /** 1928 * @param value {@link #enterer} (The person or device that performed the data 1929 * entry leading to this message. When there is more than one 1930 * candidate, pick the most proximal to the message. Can provide 1931 * other enterers in extensions.) 1932 */ 1933 public MessageHeader setEnterer(Reference value) { 1934 this.enterer = value; 1935 return this; 1936 } 1937 1938 /** 1939 * @return {@link #enterer} The actual object that is the target of the 1940 * reference. The reference library doesn't populate this, but you can 1941 * use it to hold the resource if you resolve it. (The person or device 1942 * that performed the data entry leading to this message. When there is 1943 * more than one candidate, pick the most proximal to the message. Can 1944 * provide other enterers in extensions.) 1945 */ 1946 public Resource getEntererTarget() { 1947 return this.entererTarget; 1948 } 1949 1950 /** 1951 * @param value {@link #enterer} The actual object that is the target of the 1952 * reference. The reference library doesn't use these, but you can 1953 * use it to hold the resource if you resolve it. (The person or 1954 * device that performed the data entry leading to this message. 1955 * When there is more than one candidate, pick the most proximal to 1956 * the message. Can provide other enterers in extensions.) 1957 */ 1958 public MessageHeader setEntererTarget(Resource value) { 1959 this.entererTarget = value; 1960 return this; 1961 } 1962 1963 /** 1964 * @return {@link #author} (The logical author of the message - the person or 1965 * device that decided the described event should happen. When there is 1966 * more than one candidate, pick the most proximal to the MessageHeader. 1967 * Can provide other authors in extensions.) 1968 */ 1969 public Reference getAuthor() { 1970 if (this.author == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create MessageHeader.author"); 1973 else if (Configuration.doAutoCreate()) 1974 this.author = new Reference(); // cc 1975 return this.author; 1976 } 1977 1978 public boolean hasAuthor() { 1979 return this.author != null && !this.author.isEmpty(); 1980 } 1981 1982 /** 1983 * @param value {@link #author} (The logical author of the message - the person 1984 * or device that decided the described event should happen. When 1985 * there is more than one candidate, pick the most proximal to the 1986 * MessageHeader. Can provide other authors in extensions.) 1987 */ 1988 public MessageHeader setAuthor(Reference value) { 1989 this.author = value; 1990 return this; 1991 } 1992 1993 /** 1994 * @return {@link #author} The actual object that is the target of the 1995 * reference. The reference library doesn't populate this, but you can 1996 * use it to hold the resource if you resolve it. (The logical author of 1997 * the message - the person or device that decided the described event 1998 * should happen. When there is more than one candidate, pick the most 1999 * proximal to the MessageHeader. Can provide other authors in 2000 * extensions.) 2001 */ 2002 public Resource getAuthorTarget() { 2003 return this.authorTarget; 2004 } 2005 2006 /** 2007 * @param value {@link #author} The actual object that is the target of the 2008 * reference. The reference library doesn't use these, but you can 2009 * use it to hold the resource if you resolve it. (The logical 2010 * author of the message - the person or device that decided the 2011 * described event should happen. When there is more than one 2012 * candidate, pick the most proximal to the MessageHeader. Can 2013 * provide other authors in extensions.) 2014 */ 2015 public MessageHeader setAuthorTarget(Resource value) { 2016 this.authorTarget = value; 2017 return this; 2018 } 2019 2020 /** 2021 * @return {@link #source} (The source application from which this message 2022 * originated.) 2023 */ 2024 public MessageSourceComponent getSource() { 2025 if (this.source == null) 2026 if (Configuration.errorOnAutoCreate()) 2027 throw new Error("Attempt to auto-create MessageHeader.source"); 2028 else if (Configuration.doAutoCreate()) 2029 this.source = new MessageSourceComponent(); // cc 2030 return this.source; 2031 } 2032 2033 public boolean hasSource() { 2034 return this.source != null && !this.source.isEmpty(); 2035 } 2036 2037 /** 2038 * @param value {@link #source} (The source application from which this message 2039 * originated.) 2040 */ 2041 public MessageHeader setSource(MessageSourceComponent value) { 2042 this.source = value; 2043 return this; 2044 } 2045 2046 /** 2047 * @return {@link #responsible} (The person or organization that accepts overall 2048 * responsibility for the contents of the message. The implication is 2049 * that the message event happened under the policies of the responsible 2050 * party.) 2051 */ 2052 public Reference getResponsible() { 2053 if (this.responsible == null) 2054 if (Configuration.errorOnAutoCreate()) 2055 throw new Error("Attempt to auto-create MessageHeader.responsible"); 2056 else if (Configuration.doAutoCreate()) 2057 this.responsible = new Reference(); // cc 2058 return this.responsible; 2059 } 2060 2061 public boolean hasResponsible() { 2062 return this.responsible != null && !this.responsible.isEmpty(); 2063 } 2064 2065 /** 2066 * @param value {@link #responsible} (The person or organization that accepts 2067 * overall responsibility for the contents of the message. The 2068 * implication is that the message event happened under the 2069 * policies of the responsible party.) 2070 */ 2071 public MessageHeader setResponsible(Reference value) { 2072 this.responsible = value; 2073 return this; 2074 } 2075 2076 /** 2077 * @return {@link #responsible} The actual object that is the target of the 2078 * reference. The reference library doesn't populate this, but you can 2079 * use it to hold the resource if you resolve it. (The person or 2080 * organization that accepts overall responsibility for the contents of 2081 * the message. The implication is that the message event happened under 2082 * the policies of the responsible party.) 2083 */ 2084 public Resource getResponsibleTarget() { 2085 return this.responsibleTarget; 2086 } 2087 2088 /** 2089 * @param value {@link #responsible} The actual object that is the target of the 2090 * reference. The reference library doesn't use these, but you can 2091 * use it to hold the resource if you resolve it. (The person or 2092 * organization that accepts overall responsibility for the 2093 * contents of the message. The implication is that the message 2094 * event happened under the policies of the responsible party.) 2095 */ 2096 public MessageHeader setResponsibleTarget(Resource value) { 2097 this.responsibleTarget = value; 2098 return this; 2099 } 2100 2101 /** 2102 * @return {@link #reason} (Coded indication of the cause for the event - 2103 * indicates a reason for the occurrence of the event that is a focus of 2104 * this message.) 2105 */ 2106 public CodeableConcept getReason() { 2107 if (this.reason == null) 2108 if (Configuration.errorOnAutoCreate()) 2109 throw new Error("Attempt to auto-create MessageHeader.reason"); 2110 else if (Configuration.doAutoCreate()) 2111 this.reason = new CodeableConcept(); // cc 2112 return this.reason; 2113 } 2114 2115 public boolean hasReason() { 2116 return this.reason != null && !this.reason.isEmpty(); 2117 } 2118 2119 /** 2120 * @param value {@link #reason} (Coded indication of the cause for the event - 2121 * indicates a reason for the occurrence of the event that is a 2122 * focus of this message.) 2123 */ 2124 public MessageHeader setReason(CodeableConcept value) { 2125 this.reason = value; 2126 return this; 2127 } 2128 2129 /** 2130 * @return {@link #response} (Information about the message that this message is 2131 * a response to. Only present if this message is a response.) 2132 */ 2133 public MessageHeaderResponseComponent getResponse() { 2134 if (this.response == null) 2135 if (Configuration.errorOnAutoCreate()) 2136 throw new Error("Attempt to auto-create MessageHeader.response"); 2137 else if (Configuration.doAutoCreate()) 2138 this.response = new MessageHeaderResponseComponent(); // cc 2139 return this.response; 2140 } 2141 2142 public boolean hasResponse() { 2143 return this.response != null && !this.response.isEmpty(); 2144 } 2145 2146 /** 2147 * @param value {@link #response} (Information about the message that this 2148 * message is a response to. Only present if this message is a 2149 * response.) 2150 */ 2151 public MessageHeader setResponse(MessageHeaderResponseComponent value) { 2152 this.response = value; 2153 return this; 2154 } 2155 2156 /** 2157 * @return {@link #focus} (The actual data of the message - a reference to the 2158 * root/focus class of the event.) 2159 */ 2160 public List<Reference> getFocus() { 2161 if (this.focus == null) 2162 this.focus = new ArrayList<Reference>(); 2163 return this.focus; 2164 } 2165 2166 /** 2167 * @return Returns a reference to <code>this</code> for easy method chaining 2168 */ 2169 public MessageHeader setFocus(List<Reference> theFocus) { 2170 this.focus = theFocus; 2171 return this; 2172 } 2173 2174 public boolean hasFocus() { 2175 if (this.focus == null) 2176 return false; 2177 for (Reference item : this.focus) 2178 if (!item.isEmpty()) 2179 return true; 2180 return false; 2181 } 2182 2183 public Reference addFocus() { // 3 2184 Reference t = new Reference(); 2185 if (this.focus == null) 2186 this.focus = new ArrayList<Reference>(); 2187 this.focus.add(t); 2188 return t; 2189 } 2190 2191 public MessageHeader addFocus(Reference t) { // 3 2192 if (t == null) 2193 return this; 2194 if (this.focus == null) 2195 this.focus = new ArrayList<Reference>(); 2196 this.focus.add(t); 2197 return this; 2198 } 2199 2200 /** 2201 * @return The first repetition of repeating field {@link #focus}, creating it 2202 * if it does not already exist 2203 */ 2204 public Reference getFocusFirstRep() { 2205 if (getFocus().isEmpty()) { 2206 addFocus(); 2207 } 2208 return getFocus().get(0); 2209 } 2210 2211 /** 2212 * @return {@link #definition} (Permanent link to the MessageDefinition for this 2213 * message.). This is the underlying object with id, value and 2214 * extensions. The accessor "getDefinition" gives direct access to the 2215 * value 2216 */ 2217 public CanonicalType getDefinitionElement() { 2218 if (this.definition == null) 2219 if (Configuration.errorOnAutoCreate()) 2220 throw new Error("Attempt to auto-create MessageHeader.definition"); 2221 else if (Configuration.doAutoCreate()) 2222 this.definition = new CanonicalType(); // bb 2223 return this.definition; 2224 } 2225 2226 public boolean hasDefinitionElement() { 2227 return this.definition != null && !this.definition.isEmpty(); 2228 } 2229 2230 public boolean hasDefinition() { 2231 return this.definition != null && !this.definition.isEmpty(); 2232 } 2233 2234 /** 2235 * @param value {@link #definition} (Permanent link to the MessageDefinition for 2236 * this message.). This is the underlying object with id, value and 2237 * extensions. The accessor "getDefinition" gives direct access to 2238 * the value 2239 */ 2240 public MessageHeader setDefinitionElement(CanonicalType value) { 2241 this.definition = value; 2242 return this; 2243 } 2244 2245 /** 2246 * @return Permanent link to the MessageDefinition for this message. 2247 */ 2248 public String getDefinition() { 2249 return this.definition == null ? null : this.definition.getValue(); 2250 } 2251 2252 /** 2253 * @param value Permanent link to the MessageDefinition for this message. 2254 */ 2255 public MessageHeader setDefinition(String value) { 2256 if (Utilities.noString(value)) 2257 this.definition = null; 2258 else { 2259 if (this.definition == null) 2260 this.definition = new CanonicalType(); 2261 this.definition.setValue(value); 2262 } 2263 return this; 2264 } 2265 2266 protected void listChildren(List<Property> children) { 2267 super.listChildren(children); 2268 children.add(new Property("event[x]", "Coding|uri", 2269 "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://terminology.hl7.org/CodeSystem/message-events\". Alternatively uri to the EventDefinition.", 2270 0, 1, event)); 2271 children.add(new Property("destination", "", "The destination application which the message is intended for.", 0, 2272 java.lang.Integer.MAX_VALUE, destination)); 2273 children.add(new Property("sender", "Reference(Practitioner|PractitionerRole|Organization)", 2274 "Identifies the sending system to allow the use of a trust relationship.", 0, 1, sender)); 2275 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole)", 2276 "The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.", 2277 0, 1, enterer)); 2278 children.add(new Property("author", "Reference(Practitioner|PractitionerRole)", 2279 "The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 2280 0, 1, author)); 2281 children 2282 .add(new Property("source", "", "The source application from which this message originated.", 0, 1, source)); 2283 children.add(new Property("responsible", "Reference(Practitioner|PractitionerRole|Organization)", 2284 "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 2285 0, 1, responsible)); 2286 children.add(new Property("reason", "CodeableConcept", 2287 "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 2288 0, 1, reason)); 2289 children.add(new Property("response", "", 2290 "Information about the message that this message is a response to. Only present if this message is a response.", 2291 0, 1, response)); 2292 children.add(new Property("focus", "Reference(Any)", 2293 "The actual data of the message - a reference to the root/focus class of the event.", 0, 2294 java.lang.Integer.MAX_VALUE, focus)); 2295 children.add(new Property("definition", "canonical(MessageDefinition)", 2296 "Permanent link to the MessageDefinition for this message.", 0, 1, definition)); 2297 } 2298 2299 @Override 2300 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2301 switch (_hash) { 2302 case 278115238: 2303 /* event[x] */ return new Property("event[x]", "Coding|uri", 2304 "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://terminology.hl7.org/CodeSystem/message-events\". Alternatively uri to the EventDefinition.", 2305 0, 1, event); 2306 case 96891546: 2307 /* event */ return new Property("event[x]", "Coding|uri", 2308 "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://terminology.hl7.org/CodeSystem/message-events\". Alternatively uri to the EventDefinition.", 2309 0, 1, event); 2310 case -355957084: 2311 /* eventCoding */ return new Property("event[x]", "Coding|uri", 2312 "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://terminology.hl7.org/CodeSystem/message-events\". Alternatively uri to the EventDefinition.", 2313 0, 1, event); 2314 case 278109298: 2315 /* eventUri */ return new Property("event[x]", "Coding|uri", 2316 "Code that identifies the event this message represents and connects it with its definition. Events defined as part of the FHIR specification have the system value \"http://terminology.hl7.org/CodeSystem/message-events\". Alternatively uri to the EventDefinition.", 2317 0, 1, event); 2318 case -1429847026: 2319 /* destination */ return new Property("destination", "", 2320 "The destination application which the message is intended for.", 0, java.lang.Integer.MAX_VALUE, 2321 destination); 2322 case -905962955: 2323 /* sender */ return new Property("sender", "Reference(Practitioner|PractitionerRole|Organization)", 2324 "Identifies the sending system to allow the use of a trust relationship.", 0, 1, sender); 2325 case -1591951995: 2326 /* enterer */ return new Property("enterer", "Reference(Practitioner|PractitionerRole)", 2327 "The person or device that performed the data entry leading to this message. When there is more than one candidate, pick the most proximal to the message. Can provide other enterers in extensions.", 2328 0, 1, enterer); 2329 case -1406328437: 2330 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole)", 2331 "The logical author of the message - the person or device that decided the described event should happen. When there is more than one candidate, pick the most proximal to the MessageHeader. Can provide other authors in extensions.", 2332 0, 1, author); 2333 case -896505829: 2334 /* source */ return new Property("source", "", "The source application from which this message originated.", 0, 1, 2335 source); 2336 case 1847674614: 2337 /* responsible */ return new Property("responsible", "Reference(Practitioner|PractitionerRole|Organization)", 2338 "The person or organization that accepts overall responsibility for the contents of the message. The implication is that the message event happened under the policies of the responsible party.", 2339 0, 1, responsible); 2340 case -934964668: 2341 /* reason */ return new Property("reason", "CodeableConcept", 2342 "Coded indication of the cause for the event - indicates a reason for the occurrence of the event that is a focus of this message.", 2343 0, 1, reason); 2344 case -340323263: 2345 /* response */ return new Property("response", "", 2346 "Information about the message that this message is a response to. Only present if this message is a response.", 2347 0, 1, response); 2348 case 97604824: 2349 /* focus */ return new Property("focus", "Reference(Any)", 2350 "The actual data of the message - a reference to the root/focus class of the event.", 0, 2351 java.lang.Integer.MAX_VALUE, focus); 2352 case -1014418093: 2353 /* definition */ return new Property("definition", "canonical(MessageDefinition)", 2354 "Permanent link to the MessageDefinition for this message.", 0, 1, definition); 2355 default: 2356 return super.getNamedProperty(_hash, _name, _checkValid); 2357 } 2358 2359 } 2360 2361 @Override 2362 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2363 switch (hash) { 2364 case 96891546: 2365 /* event */ return this.event == null ? new Base[0] : new Base[] { this.event }; // Type 2366 case -1429847026: 2367 /* destination */ return this.destination == null ? new Base[0] 2368 : this.destination.toArray(new Base[this.destination.size()]); // MessageDestinationComponent 2369 case -905962955: 2370 /* sender */ return this.sender == null ? new Base[0] : new Base[] { this.sender }; // Reference 2371 case -1591951995: 2372 /* enterer */ return this.enterer == null ? new Base[0] : new Base[] { this.enterer }; // Reference 2373 case -1406328437: 2374 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 2375 case -896505829: 2376 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // MessageSourceComponent 2377 case 1847674614: 2378 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // Reference 2379 case -934964668: 2380 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 2381 case -340323263: 2382 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // MessageHeaderResponseComponent 2383 case 97604824: 2384 /* focus */ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 2385 case -1014418093: 2386 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // CanonicalType 2387 default: 2388 return super.getProperty(hash, name, checkValid); 2389 } 2390 2391 } 2392 2393 @Override 2394 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2395 switch (hash) { 2396 case 96891546: // event 2397 this.event = castToType(value); // Type 2398 return value; 2399 case -1429847026: // destination 2400 this.getDestination().add((MessageDestinationComponent) value); // MessageDestinationComponent 2401 return value; 2402 case -905962955: // sender 2403 this.sender = castToReference(value); // Reference 2404 return value; 2405 case -1591951995: // enterer 2406 this.enterer = castToReference(value); // Reference 2407 return value; 2408 case -1406328437: // author 2409 this.author = castToReference(value); // Reference 2410 return value; 2411 case -896505829: // source 2412 this.source = (MessageSourceComponent) value; // MessageSourceComponent 2413 return value; 2414 case 1847674614: // responsible 2415 this.responsible = castToReference(value); // Reference 2416 return value; 2417 case -934964668: // reason 2418 this.reason = castToCodeableConcept(value); // CodeableConcept 2419 return value; 2420 case -340323263: // response 2421 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 2422 return value; 2423 case 97604824: // focus 2424 this.getFocus().add(castToReference(value)); // Reference 2425 return value; 2426 case -1014418093: // definition 2427 this.definition = castToCanonical(value); // CanonicalType 2428 return value; 2429 default: 2430 return super.setProperty(hash, name, value); 2431 } 2432 2433 } 2434 2435 @Override 2436 public Base setProperty(String name, Base value) throws FHIRException { 2437 if (name.equals("event[x]")) { 2438 this.event = castToType(value); // Type 2439 } else if (name.equals("destination")) { 2440 this.getDestination().add((MessageDestinationComponent) value); 2441 } else if (name.equals("sender")) { 2442 this.sender = castToReference(value); // Reference 2443 } else if (name.equals("enterer")) { 2444 this.enterer = castToReference(value); // Reference 2445 } else if (name.equals("author")) { 2446 this.author = castToReference(value); // Reference 2447 } else if (name.equals("source")) { 2448 this.source = (MessageSourceComponent) value; // MessageSourceComponent 2449 } else if (name.equals("responsible")) { 2450 this.responsible = castToReference(value); // Reference 2451 } else if (name.equals("reason")) { 2452 this.reason = castToCodeableConcept(value); // CodeableConcept 2453 } else if (name.equals("response")) { 2454 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 2455 } else if (name.equals("focus")) { 2456 this.getFocus().add(castToReference(value)); 2457 } else if (name.equals("definition")) { 2458 this.definition = castToCanonical(value); // CanonicalType 2459 } else 2460 return super.setProperty(name, value); 2461 return value; 2462 } 2463 2464 @Override 2465 public void removeChild(String name, Base value) throws FHIRException { 2466 if (name.equals("event[x]")) { 2467 this.event = null; 2468 } else if (name.equals("destination")) { 2469 this.getDestination().remove((MessageDestinationComponent) value); 2470 } else if (name.equals("sender")) { 2471 this.sender = null; 2472 } else if (name.equals("enterer")) { 2473 this.enterer = null; 2474 } else if (name.equals("author")) { 2475 this.author = null; 2476 } else if (name.equals("source")) { 2477 this.source = (MessageSourceComponent) value; // MessageSourceComponent 2478 } else if (name.equals("responsible")) { 2479 this.responsible = null; 2480 } else if (name.equals("reason")) { 2481 this.reason = null; 2482 } else if (name.equals("response")) { 2483 this.response = (MessageHeaderResponseComponent) value; // MessageHeaderResponseComponent 2484 } else if (name.equals("focus")) { 2485 this.getFocus().remove(castToReference(value)); 2486 } else if (name.equals("definition")) { 2487 this.definition = null; 2488 } else 2489 super.removeChild(name, value); 2490 2491 } 2492 2493 @Override 2494 public Base makeProperty(int hash, String name) throws FHIRException { 2495 switch (hash) { 2496 case 278115238: 2497 return getEvent(); 2498 case 96891546: 2499 return getEvent(); 2500 case -1429847026: 2501 return addDestination(); 2502 case -905962955: 2503 return getSender(); 2504 case -1591951995: 2505 return getEnterer(); 2506 case -1406328437: 2507 return getAuthor(); 2508 case -896505829: 2509 return getSource(); 2510 case 1847674614: 2511 return getResponsible(); 2512 case -934964668: 2513 return getReason(); 2514 case -340323263: 2515 return getResponse(); 2516 case 97604824: 2517 return addFocus(); 2518 case -1014418093: 2519 return getDefinitionElement(); 2520 default: 2521 return super.makeProperty(hash, name); 2522 } 2523 2524 } 2525 2526 @Override 2527 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2528 switch (hash) { 2529 case 96891546: 2530 /* event */ return new String[] { "Coding", "uri" }; 2531 case -1429847026: 2532 /* destination */ return new String[] {}; 2533 case -905962955: 2534 /* sender */ return new String[] { "Reference" }; 2535 case -1591951995: 2536 /* enterer */ return new String[] { "Reference" }; 2537 case -1406328437: 2538 /* author */ return new String[] { "Reference" }; 2539 case -896505829: 2540 /* source */ return new String[] {}; 2541 case 1847674614: 2542 /* responsible */ return new String[] { "Reference" }; 2543 case -934964668: 2544 /* reason */ return new String[] { "CodeableConcept" }; 2545 case -340323263: 2546 /* response */ return new String[] {}; 2547 case 97604824: 2548 /* focus */ return new String[] { "Reference" }; 2549 case -1014418093: 2550 /* definition */ return new String[] { "canonical" }; 2551 default: 2552 return super.getTypesForProperty(hash, name); 2553 } 2554 2555 } 2556 2557 @Override 2558 public Base addChild(String name) throws FHIRException { 2559 if (name.equals("eventCoding")) { 2560 this.event = new Coding(); 2561 return this.event; 2562 } else if (name.equals("eventUri")) { 2563 this.event = new UriType(); 2564 return this.event; 2565 } else if (name.equals("destination")) { 2566 return addDestination(); 2567 } else if (name.equals("sender")) { 2568 this.sender = new Reference(); 2569 return this.sender; 2570 } else if (name.equals("enterer")) { 2571 this.enterer = new Reference(); 2572 return this.enterer; 2573 } else if (name.equals("author")) { 2574 this.author = new Reference(); 2575 return this.author; 2576 } else if (name.equals("source")) { 2577 this.source = new MessageSourceComponent(); 2578 return this.source; 2579 } else if (name.equals("responsible")) { 2580 this.responsible = new Reference(); 2581 return this.responsible; 2582 } else if (name.equals("reason")) { 2583 this.reason = new CodeableConcept(); 2584 return this.reason; 2585 } else if (name.equals("response")) { 2586 this.response = new MessageHeaderResponseComponent(); 2587 return this.response; 2588 } else if (name.equals("focus")) { 2589 return addFocus(); 2590 } else if (name.equals("definition")) { 2591 throw new FHIRException("Cannot call addChild on a singleton property MessageHeader.definition"); 2592 } else 2593 return super.addChild(name); 2594 } 2595 2596 public String fhirType() { 2597 return "MessageHeader"; 2598 2599 } 2600 2601 public MessageHeader copy() { 2602 MessageHeader dst = new MessageHeader(); 2603 copyValues(dst); 2604 return dst; 2605 } 2606 2607 public void copyValues(MessageHeader dst) { 2608 super.copyValues(dst); 2609 dst.event = event == null ? null : event.copy(); 2610 if (destination != null) { 2611 dst.destination = new ArrayList<MessageDestinationComponent>(); 2612 for (MessageDestinationComponent i : destination) 2613 dst.destination.add(i.copy()); 2614 } 2615 ; 2616 dst.sender = sender == null ? null : sender.copy(); 2617 dst.enterer = enterer == null ? null : enterer.copy(); 2618 dst.author = author == null ? null : author.copy(); 2619 dst.source = source == null ? null : source.copy(); 2620 dst.responsible = responsible == null ? null : responsible.copy(); 2621 dst.reason = reason == null ? null : reason.copy(); 2622 dst.response = response == null ? null : response.copy(); 2623 if (focus != null) { 2624 dst.focus = new ArrayList<Reference>(); 2625 for (Reference i : focus) 2626 dst.focus.add(i.copy()); 2627 } 2628 ; 2629 dst.definition = definition == null ? null : definition.copy(); 2630 } 2631 2632 protected MessageHeader typedCopy() { 2633 return copy(); 2634 } 2635 2636 @Override 2637 public boolean equalsDeep(Base other_) { 2638 if (!super.equalsDeep(other_)) 2639 return false; 2640 if (!(other_ instanceof MessageHeader)) 2641 return false; 2642 MessageHeader o = (MessageHeader) other_; 2643 return compareDeep(event, o.event, true) && compareDeep(destination, o.destination, true) 2644 && compareDeep(sender, o.sender, true) && compareDeep(enterer, o.enterer, true) 2645 && compareDeep(author, o.author, true) && compareDeep(source, o.source, true) 2646 && compareDeep(responsible, o.responsible, true) && compareDeep(reason, o.reason, true) 2647 && compareDeep(response, o.response, true) && compareDeep(focus, o.focus, true) 2648 && compareDeep(definition, o.definition, true); 2649 } 2650 2651 @Override 2652 public boolean equalsShallow(Base other_) { 2653 if (!super.equalsShallow(other_)) 2654 return false; 2655 if (!(other_ instanceof MessageHeader)) 2656 return false; 2657 MessageHeader o = (MessageHeader) other_; 2658 return true; 2659 } 2660 2661 public boolean isEmpty() { 2662 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(event, destination, sender, enterer, author, source, 2663 responsible, reason, response, focus, definition); 2664 } 2665 2666 @Override 2667 public ResourceType getResourceType() { 2668 return ResourceType.MessageHeader; 2669 } 2670 2671 /** 2672 * Search parameter: <b>code</b> 2673 * <p> 2674 * Description: <b>ok | transient-error | fatal-error</b><br> 2675 * Type: <b>token</b><br> 2676 * Path: <b>MessageHeader.response.code</b><br> 2677 * </p> 2678 */ 2679 @SearchParamDefinition(name = "code", path = "MessageHeader.response.code", description = "ok | transient-error | fatal-error", type = "token") 2680 public static final String SP_CODE = "code"; 2681 /** 2682 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2683 * <p> 2684 * Description: <b>ok | transient-error | fatal-error</b><br> 2685 * Type: <b>token</b><br> 2686 * Path: <b>MessageHeader.response.code</b><br> 2687 * </p> 2688 */ 2689 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2690 SP_CODE); 2691 2692 /** 2693 * Search parameter: <b>receiver</b> 2694 * <p> 2695 * Description: <b>Intended "real-world" recipient for the data</b><br> 2696 * Type: <b>reference</b><br> 2697 * Path: <b>MessageHeader.destination.receiver</b><br> 2698 * </p> 2699 */ 2700 @SearchParamDefinition(name = "receiver", path = "MessageHeader.destination.receiver", description = "Intended \"real-world\" recipient for the data", type = "reference", providesMembershipIn = { 2701 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 2702 Practitioner.class, PractitionerRole.class }) 2703 public static final String SP_RECEIVER = "receiver"; 2704 /** 2705 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 2706 * <p> 2707 * Description: <b>Intended "real-world" recipient for the data</b><br> 2708 * Type: <b>reference</b><br> 2709 * Path: <b>MessageHeader.destination.receiver</b><br> 2710 * </p> 2711 */ 2712 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2713 SP_RECEIVER); 2714 2715 /** 2716 * Constant for fluent queries to be used to add include statements. Specifies 2717 * the path value of "<b>MessageHeader:receiver</b>". 2718 */ 2719 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include( 2720 "MessageHeader:receiver").toLocked(); 2721 2722 /** 2723 * Search parameter: <b>author</b> 2724 * <p> 2725 * Description: <b>The source of the decision</b><br> 2726 * Type: <b>reference</b><br> 2727 * Path: <b>MessageHeader.author</b><br> 2728 * </p> 2729 */ 2730 @SearchParamDefinition(name = "author", path = "MessageHeader.author", description = "The source of the decision", type = "reference", providesMembershipIn = { 2731 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 2732 PractitionerRole.class }) 2733 public static final String SP_AUTHOR = "author"; 2734 /** 2735 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2736 * <p> 2737 * Description: <b>The source of the decision</b><br> 2738 * Type: <b>reference</b><br> 2739 * Path: <b>MessageHeader.author</b><br> 2740 * </p> 2741 */ 2742 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2743 SP_AUTHOR); 2744 2745 /** 2746 * Constant for fluent queries to be used to add include statements. Specifies 2747 * the path value of "<b>MessageHeader:author</b>". 2748 */ 2749 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 2750 "MessageHeader:author").toLocked(); 2751 2752 /** 2753 * Search parameter: <b>destination</b> 2754 * <p> 2755 * Description: <b>Name of system</b><br> 2756 * Type: <b>string</b><br> 2757 * Path: <b>MessageHeader.destination.name</b><br> 2758 * </p> 2759 */ 2760 @SearchParamDefinition(name = "destination", path = "MessageHeader.destination.name", description = "Name of system", type = "string") 2761 public static final String SP_DESTINATION = "destination"; 2762 /** 2763 * <b>Fluent Client</b> search parameter constant for <b>destination</b> 2764 * <p> 2765 * Description: <b>Name of system</b><br> 2766 * Type: <b>string</b><br> 2767 * Path: <b>MessageHeader.destination.name</b><br> 2768 * </p> 2769 */ 2770 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESTINATION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2771 SP_DESTINATION); 2772 2773 /** 2774 * Search parameter: <b>focus</b> 2775 * <p> 2776 * Description: <b>The actual content of the message</b><br> 2777 * Type: <b>reference</b><br> 2778 * Path: <b>MessageHeader.focus</b><br> 2779 * </p> 2780 */ 2781 @SearchParamDefinition(name = "focus", path = "MessageHeader.focus", description = "The actual content of the message", type = "reference") 2782 public static final String SP_FOCUS = "focus"; 2783 /** 2784 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 2785 * <p> 2786 * Description: <b>The actual content of the message</b><br> 2787 * Type: <b>reference</b><br> 2788 * Path: <b>MessageHeader.focus</b><br> 2789 * </p> 2790 */ 2791 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2792 SP_FOCUS); 2793 2794 /** 2795 * Constant for fluent queries to be used to add include statements. Specifies 2796 * the path value of "<b>MessageHeader:focus</b>". 2797 */ 2798 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include( 2799 "MessageHeader:focus").toLocked(); 2800 2801 /** 2802 * Search parameter: <b>source</b> 2803 * <p> 2804 * Description: <b>Name of system</b><br> 2805 * Type: <b>string</b><br> 2806 * Path: <b>MessageHeader.source.name</b><br> 2807 * </p> 2808 */ 2809 @SearchParamDefinition(name = "source", path = "MessageHeader.source.name", description = "Name of system", type = "string") 2810 public static final String SP_SOURCE = "source"; 2811 /** 2812 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2813 * <p> 2814 * Description: <b>Name of system</b><br> 2815 * Type: <b>string</b><br> 2816 * Path: <b>MessageHeader.source.name</b><br> 2817 * </p> 2818 */ 2819 public static final ca.uhn.fhir.rest.gclient.StringClientParam SOURCE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2820 SP_SOURCE); 2821 2822 /** 2823 * Search parameter: <b>target</b> 2824 * <p> 2825 * Description: <b>Particular delivery destination within the 2826 * destination</b><br> 2827 * Type: <b>reference</b><br> 2828 * Path: <b>MessageHeader.destination.target</b><br> 2829 * </p> 2830 */ 2831 @SearchParamDefinition(name = "target", path = "MessageHeader.destination.target", description = "Particular delivery destination within the destination", type = "reference", providesMembershipIn = { 2832 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 2833 public static final String SP_TARGET = "target"; 2834 /** 2835 * <b>Fluent Client</b> search parameter constant for <b>target</b> 2836 * <p> 2837 * Description: <b>Particular delivery destination within the 2838 * destination</b><br> 2839 * Type: <b>reference</b><br> 2840 * Path: <b>MessageHeader.destination.target</b><br> 2841 * </p> 2842 */ 2843 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam TARGET = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2844 SP_TARGET); 2845 2846 /** 2847 * Constant for fluent queries to be used to add include statements. Specifies 2848 * the path value of "<b>MessageHeader:target</b>". 2849 */ 2850 public static final ca.uhn.fhir.model.api.Include INCLUDE_TARGET = new ca.uhn.fhir.model.api.Include( 2851 "MessageHeader:target").toLocked(); 2852 2853 /** 2854 * Search parameter: <b>destination-uri</b> 2855 * <p> 2856 * Description: <b>Actual destination address or id</b><br> 2857 * Type: <b>uri</b><br> 2858 * Path: <b>MessageHeader.destination.endpoint</b><br> 2859 * </p> 2860 */ 2861 @SearchParamDefinition(name = "destination-uri", path = "MessageHeader.destination.endpoint", description = "Actual destination address or id", type = "uri") 2862 public static final String SP_DESTINATION_URI = "destination-uri"; 2863 /** 2864 * <b>Fluent Client</b> search parameter constant for <b>destination-uri</b> 2865 * <p> 2866 * Description: <b>Actual destination address or id</b><br> 2867 * Type: <b>uri</b><br> 2868 * Path: <b>MessageHeader.destination.endpoint</b><br> 2869 * </p> 2870 */ 2871 public static final ca.uhn.fhir.rest.gclient.UriClientParam DESTINATION_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 2872 SP_DESTINATION_URI); 2873 2874 /** 2875 * Search parameter: <b>source-uri</b> 2876 * <p> 2877 * Description: <b>Actual message source address or id</b><br> 2878 * Type: <b>uri</b><br> 2879 * Path: <b>MessageHeader.source.endpoint</b><br> 2880 * </p> 2881 */ 2882 @SearchParamDefinition(name = "source-uri", path = "MessageHeader.source.endpoint", description = "Actual message source address or id", type = "uri") 2883 public static final String SP_SOURCE_URI = "source-uri"; 2884 /** 2885 * <b>Fluent Client</b> search parameter constant for <b>source-uri</b> 2886 * <p> 2887 * Description: <b>Actual message source address or id</b><br> 2888 * Type: <b>uri</b><br> 2889 * Path: <b>MessageHeader.source.endpoint</b><br> 2890 * </p> 2891 */ 2892 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 2893 SP_SOURCE_URI); 2894 2895 /** 2896 * Search parameter: <b>sender</b> 2897 * <p> 2898 * Description: <b>Real world sender of the message</b><br> 2899 * Type: <b>reference</b><br> 2900 * Path: <b>MessageHeader.sender</b><br> 2901 * </p> 2902 */ 2903 @SearchParamDefinition(name = "sender", path = "MessageHeader.sender", description = "Real world sender of the message", type = "reference", target = { 2904 Organization.class, Practitioner.class, PractitionerRole.class }) 2905 public static final String SP_SENDER = "sender"; 2906 /** 2907 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 2908 * <p> 2909 * Description: <b>Real world sender of the message</b><br> 2910 * Type: <b>reference</b><br> 2911 * Path: <b>MessageHeader.sender</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2915 SP_SENDER); 2916 2917 /** 2918 * Constant for fluent queries to be used to add include statements. Specifies 2919 * the path value of "<b>MessageHeader:sender</b>". 2920 */ 2921 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include( 2922 "MessageHeader:sender").toLocked(); 2923 2924 /** 2925 * Search parameter: <b>responsible</b> 2926 * <p> 2927 * Description: <b>Final responsibility for event</b><br> 2928 * Type: <b>reference</b><br> 2929 * Path: <b>MessageHeader.responsible</b><br> 2930 * </p> 2931 */ 2932 @SearchParamDefinition(name = "responsible", path = "MessageHeader.responsible", description = "Final responsibility for event", type = "reference", providesMembershipIn = { 2933 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 2934 Practitioner.class, PractitionerRole.class }) 2935 public static final String SP_RESPONSIBLE = "responsible"; 2936 /** 2937 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 2938 * <p> 2939 * Description: <b>Final responsibility for event</b><br> 2940 * Type: <b>reference</b><br> 2941 * Path: <b>MessageHeader.responsible</b><br> 2942 * </p> 2943 */ 2944 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2945 SP_RESPONSIBLE); 2946 2947 /** 2948 * Constant for fluent queries to be used to add include statements. Specifies 2949 * the path value of "<b>MessageHeader:responsible</b>". 2950 */ 2951 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSIBLE = new ca.uhn.fhir.model.api.Include( 2952 "MessageHeader:responsible").toLocked(); 2953 2954 /** 2955 * Search parameter: <b>enterer</b> 2956 * <p> 2957 * Description: <b>The source of the data entry</b><br> 2958 * Type: <b>reference</b><br> 2959 * Path: <b>MessageHeader.enterer</b><br> 2960 * </p> 2961 */ 2962 @SearchParamDefinition(name = "enterer", path = "MessageHeader.enterer", description = "The source of the data entry", type = "reference", providesMembershipIn = { 2963 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 2964 PractitionerRole.class }) 2965 public static final String SP_ENTERER = "enterer"; 2966 /** 2967 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 2968 * <p> 2969 * Description: <b>The source of the data entry</b><br> 2970 * Type: <b>reference</b><br> 2971 * Path: <b>MessageHeader.enterer</b><br> 2972 * </p> 2973 */ 2974 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2975 SP_ENTERER); 2976 2977 /** 2978 * Constant for fluent queries to be used to add include statements. Specifies 2979 * the path value of "<b>MessageHeader:enterer</b>". 2980 */ 2981 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include( 2982 "MessageHeader:enterer").toLocked(); 2983 2984 /** 2985 * Search parameter: <b>response-id</b> 2986 * <p> 2987 * Description: <b>Id of original message</b><br> 2988 * Type: <b>token</b><br> 2989 * Path: <b>MessageHeader.response.identifier</b><br> 2990 * </p> 2991 */ 2992 @SearchParamDefinition(name = "response-id", path = "MessageHeader.response.identifier", description = "Id of original message", type = "token") 2993 public static final String SP_RESPONSE_ID = "response-id"; 2994 /** 2995 * <b>Fluent Client</b> search parameter constant for <b>response-id</b> 2996 * <p> 2997 * Description: <b>Id of original message</b><br> 2998 * Type: <b>token</b><br> 2999 * Path: <b>MessageHeader.response.identifier</b><br> 3000 * </p> 3001 */ 3002 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESPONSE_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3003 SP_RESPONSE_ID); 3004 3005 /** 3006 * Search parameter: <b>event</b> 3007 * <p> 3008 * Description: <b>Code for the event this message represents or link to event 3009 * definition</b><br> 3010 * Type: <b>token</b><br> 3011 * Path: <b>MessageHeader.event[x]</b><br> 3012 * </p> 3013 */ 3014 @SearchParamDefinition(name = "event", path = "MessageHeader.event", description = "Code for the event this message represents or link to event definition", type = "token") 3015 public static final String SP_EVENT = "event"; 3016 /** 3017 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3018 * <p> 3019 * Description: <b>Code for the event this message represents or link to event 3020 * definition</b><br> 3021 * Type: <b>token</b><br> 3022 * Path: <b>MessageHeader.event[x]</b><br> 3023 * </p> 3024 */ 3025 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3026 SP_EVENT); 3027 3028}