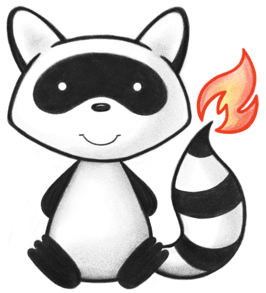
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Raw data describing a biological sequence. 050 */ 051@ResourceDef(name = "MolecularSequence", profile = "http://hl7.org/fhir/StructureDefinition/MolecularSequence") 052public class MolecularSequence extends DomainResource { 053 054 public enum SequenceType { 055 /** 056 * Amino acid sequence. 057 */ 058 AA, 059 /** 060 * DNA Sequence. 061 */ 062 DNA, 063 /** 064 * RNA Sequence. 065 */ 066 RNA, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static SequenceType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("aa".equals(codeString)) 076 return AA; 077 if ("dna".equals(codeString)) 078 return DNA; 079 if ("rna".equals(codeString)) 080 return RNA; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown SequenceType code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case AA: 090 return "aa"; 091 case DNA: 092 return "dna"; 093 case RNA: 094 return "rna"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 switch (this) { 104 case AA: 105 return "http://hl7.org/fhir/sequence-type"; 106 case DNA: 107 return "http://hl7.org/fhir/sequence-type"; 108 case RNA: 109 return "http://hl7.org/fhir/sequence-type"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case AA: 120 return "Amino acid sequence."; 121 case DNA: 122 return "DNA Sequence."; 123 case RNA: 124 return "RNA Sequence."; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case AA: 135 return "AA Sequence"; 136 case DNA: 137 return "DNA Sequence"; 138 case RNA: 139 return "RNA Sequence"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 } 147 148 public static class SequenceTypeEnumFactory implements EnumFactory<SequenceType> { 149 public SequenceType fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("aa".equals(codeString)) 154 return SequenceType.AA; 155 if ("dna".equals(codeString)) 156 return SequenceType.DNA; 157 if ("rna".equals(codeString)) 158 return SequenceType.RNA; 159 throw new IllegalArgumentException("Unknown SequenceType code '" + codeString + "'"); 160 } 161 162 public Enumeration<SequenceType> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<SequenceType>(this, SequenceType.NULL, code); 167 String codeString = code.asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<SequenceType>(this, SequenceType.NULL, code); 170 if ("aa".equals(codeString)) 171 return new Enumeration<SequenceType>(this, SequenceType.AA, code); 172 if ("dna".equals(codeString)) 173 return new Enumeration<SequenceType>(this, SequenceType.DNA, code); 174 if ("rna".equals(codeString)) 175 return new Enumeration<SequenceType>(this, SequenceType.RNA, code); 176 throw new FHIRException("Unknown SequenceType code '" + codeString + "'"); 177 } 178 179 public String toCode(SequenceType code) { 180 if (code == SequenceType.AA) 181 return "aa"; 182 if (code == SequenceType.DNA) 183 return "dna"; 184 if (code == SequenceType.RNA) 185 return "rna"; 186 return "?"; 187 } 188 189 public String toSystem(SequenceType code) { 190 return code.getSystem(); 191 } 192 } 193 194 public enum OrientationType { 195 /** 196 * Sense orientation of reference sequence. 197 */ 198 SENSE, 199 /** 200 * Antisense orientation of reference sequence. 201 */ 202 ANTISENSE, 203 /** 204 * added to help the parsers with the generic types 205 */ 206 NULL; 207 208 public static OrientationType fromCode(String codeString) throws FHIRException { 209 if (codeString == null || "".equals(codeString)) 210 return null; 211 if ("sense".equals(codeString)) 212 return SENSE; 213 if ("antisense".equals(codeString)) 214 return ANTISENSE; 215 if (Configuration.isAcceptInvalidEnums()) 216 return null; 217 else 218 throw new FHIRException("Unknown OrientationType code '" + codeString + "'"); 219 } 220 221 public String toCode() { 222 switch (this) { 223 case SENSE: 224 return "sense"; 225 case ANTISENSE: 226 return "antisense"; 227 case NULL: 228 return null; 229 default: 230 return "?"; 231 } 232 } 233 234 public String getSystem() { 235 switch (this) { 236 case SENSE: 237 return "http://hl7.org/fhir/orientation-type"; 238 case ANTISENSE: 239 return "http://hl7.org/fhir/orientation-type"; 240 case NULL: 241 return null; 242 default: 243 return "?"; 244 } 245 } 246 247 public String getDefinition() { 248 switch (this) { 249 case SENSE: 250 return "Sense orientation of reference sequence."; 251 case ANTISENSE: 252 return "Antisense orientation of reference sequence."; 253 case NULL: 254 return null; 255 default: 256 return "?"; 257 } 258 } 259 260 public String getDisplay() { 261 switch (this) { 262 case SENSE: 263 return "Sense orientation of referenceSeq"; 264 case ANTISENSE: 265 return "Antisense orientation of referenceSeq"; 266 case NULL: 267 return null; 268 default: 269 return "?"; 270 } 271 } 272 } 273 274 public static class OrientationTypeEnumFactory implements EnumFactory<OrientationType> { 275 public OrientationType fromCode(String codeString) throws IllegalArgumentException { 276 if (codeString == null || "".equals(codeString)) 277 if (codeString == null || "".equals(codeString)) 278 return null; 279 if ("sense".equals(codeString)) 280 return OrientationType.SENSE; 281 if ("antisense".equals(codeString)) 282 return OrientationType.ANTISENSE; 283 throw new IllegalArgumentException("Unknown OrientationType code '" + codeString + "'"); 284 } 285 286 public Enumeration<OrientationType> fromType(PrimitiveType<?> code) throws FHIRException { 287 if (code == null) 288 return null; 289 if (code.isEmpty()) 290 return new Enumeration<OrientationType>(this, OrientationType.NULL, code); 291 String codeString = code.asStringValue(); 292 if (codeString == null || "".equals(codeString)) 293 return new Enumeration<OrientationType>(this, OrientationType.NULL, code); 294 if ("sense".equals(codeString)) 295 return new Enumeration<OrientationType>(this, OrientationType.SENSE, code); 296 if ("antisense".equals(codeString)) 297 return new Enumeration<OrientationType>(this, OrientationType.ANTISENSE, code); 298 throw new FHIRException("Unknown OrientationType code '" + codeString + "'"); 299 } 300 301 public String toCode(OrientationType code) { 302 if (code == OrientationType.SENSE) 303 return "sense"; 304 if (code == OrientationType.ANTISENSE) 305 return "antisense"; 306 return "?"; 307 } 308 309 public String toSystem(OrientationType code) { 310 return code.getSystem(); 311 } 312 } 313 314 public enum StrandType { 315 /** 316 * Watson strand of reference sequence. 317 */ 318 WATSON, 319 /** 320 * Crick strand of reference sequence. 321 */ 322 CRICK, 323 /** 324 * added to help the parsers with the generic types 325 */ 326 NULL; 327 328 public static StrandType fromCode(String codeString) throws FHIRException { 329 if (codeString == null || "".equals(codeString)) 330 return null; 331 if ("watson".equals(codeString)) 332 return WATSON; 333 if ("crick".equals(codeString)) 334 return CRICK; 335 if (Configuration.isAcceptInvalidEnums()) 336 return null; 337 else 338 throw new FHIRException("Unknown StrandType code '" + codeString + "'"); 339 } 340 341 public String toCode() { 342 switch (this) { 343 case WATSON: 344 return "watson"; 345 case CRICK: 346 return "crick"; 347 case NULL: 348 return null; 349 default: 350 return "?"; 351 } 352 } 353 354 public String getSystem() { 355 switch (this) { 356 case WATSON: 357 return "http://hl7.org/fhir/strand-type"; 358 case CRICK: 359 return "http://hl7.org/fhir/strand-type"; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 367 public String getDefinition() { 368 switch (this) { 369 case WATSON: 370 return "Watson strand of reference sequence."; 371 case CRICK: 372 return "Crick strand of reference sequence."; 373 case NULL: 374 return null; 375 default: 376 return "?"; 377 } 378 } 379 380 public String getDisplay() { 381 switch (this) { 382 case WATSON: 383 return "Watson strand of referenceSeq"; 384 case CRICK: 385 return "Crick strand of referenceSeq"; 386 case NULL: 387 return null; 388 default: 389 return "?"; 390 } 391 } 392 } 393 394 public static class StrandTypeEnumFactory implements EnumFactory<StrandType> { 395 public StrandType fromCode(String codeString) throws IllegalArgumentException { 396 if (codeString == null || "".equals(codeString)) 397 if (codeString == null || "".equals(codeString)) 398 return null; 399 if ("watson".equals(codeString)) 400 return StrandType.WATSON; 401 if ("crick".equals(codeString)) 402 return StrandType.CRICK; 403 throw new IllegalArgumentException("Unknown StrandType code '" + codeString + "'"); 404 } 405 406 public Enumeration<StrandType> fromType(PrimitiveType<?> code) throws FHIRException { 407 if (code == null) 408 return null; 409 if (code.isEmpty()) 410 return new Enumeration<StrandType>(this, StrandType.NULL, code); 411 String codeString = code.asStringValue(); 412 if (codeString == null || "".equals(codeString)) 413 return new Enumeration<StrandType>(this, StrandType.NULL, code); 414 if ("watson".equals(codeString)) 415 return new Enumeration<StrandType>(this, StrandType.WATSON, code); 416 if ("crick".equals(codeString)) 417 return new Enumeration<StrandType>(this, StrandType.CRICK, code); 418 throw new FHIRException("Unknown StrandType code '" + codeString + "'"); 419 } 420 421 public String toCode(StrandType code) { 422 if (code == StrandType.WATSON) 423 return "watson"; 424 if (code == StrandType.CRICK) 425 return "crick"; 426 return "?"; 427 } 428 429 public String toSystem(StrandType code) { 430 return code.getSystem(); 431 } 432 } 433 434 public enum QualityType { 435 /** 436 * INDEL Comparison. 437 */ 438 INDEL, 439 /** 440 * SNP Comparison. 441 */ 442 SNP, 443 /** 444 * UNKNOWN Comparison. 445 */ 446 UNKNOWN, 447 /** 448 * added to help the parsers with the generic types 449 */ 450 NULL; 451 452 public static QualityType fromCode(String codeString) throws FHIRException { 453 if (codeString == null || "".equals(codeString)) 454 return null; 455 if ("indel".equals(codeString)) 456 return INDEL; 457 if ("snp".equals(codeString)) 458 return SNP; 459 if ("unknown".equals(codeString)) 460 return UNKNOWN; 461 if (Configuration.isAcceptInvalidEnums()) 462 return null; 463 else 464 throw new FHIRException("Unknown QualityType code '" + codeString + "'"); 465 } 466 467 public String toCode() { 468 switch (this) { 469 case INDEL: 470 return "indel"; 471 case SNP: 472 return "snp"; 473 case UNKNOWN: 474 return "unknown"; 475 case NULL: 476 return null; 477 default: 478 return "?"; 479 } 480 } 481 482 public String getSystem() { 483 switch (this) { 484 case INDEL: 485 return "http://hl7.org/fhir/quality-type"; 486 case SNP: 487 return "http://hl7.org/fhir/quality-type"; 488 case UNKNOWN: 489 return "http://hl7.org/fhir/quality-type"; 490 case NULL: 491 return null; 492 default: 493 return "?"; 494 } 495 } 496 497 public String getDefinition() { 498 switch (this) { 499 case INDEL: 500 return "INDEL Comparison."; 501 case SNP: 502 return "SNP Comparison."; 503 case UNKNOWN: 504 return "UNKNOWN Comparison."; 505 case NULL: 506 return null; 507 default: 508 return "?"; 509 } 510 } 511 512 public String getDisplay() { 513 switch (this) { 514 case INDEL: 515 return "INDEL Comparison"; 516 case SNP: 517 return "SNP Comparison"; 518 case UNKNOWN: 519 return "UNKNOWN Comparison"; 520 case NULL: 521 return null; 522 default: 523 return "?"; 524 } 525 } 526 } 527 528 public static class QualityTypeEnumFactory implements EnumFactory<QualityType> { 529 public QualityType fromCode(String codeString) throws IllegalArgumentException { 530 if (codeString == null || "".equals(codeString)) 531 if (codeString == null || "".equals(codeString)) 532 return null; 533 if ("indel".equals(codeString)) 534 return QualityType.INDEL; 535 if ("snp".equals(codeString)) 536 return QualityType.SNP; 537 if ("unknown".equals(codeString)) 538 return QualityType.UNKNOWN; 539 throw new IllegalArgumentException("Unknown QualityType code '" + codeString + "'"); 540 } 541 542 public Enumeration<QualityType> fromType(PrimitiveType<?> code) throws FHIRException { 543 if (code == null) 544 return null; 545 if (code.isEmpty()) 546 return new Enumeration<QualityType>(this, QualityType.NULL, code); 547 String codeString = code.asStringValue(); 548 if (codeString == null || "".equals(codeString)) 549 return new Enumeration<QualityType>(this, QualityType.NULL, code); 550 if ("indel".equals(codeString)) 551 return new Enumeration<QualityType>(this, QualityType.INDEL, code); 552 if ("snp".equals(codeString)) 553 return new Enumeration<QualityType>(this, QualityType.SNP, code); 554 if ("unknown".equals(codeString)) 555 return new Enumeration<QualityType>(this, QualityType.UNKNOWN, code); 556 throw new FHIRException("Unknown QualityType code '" + codeString + "'"); 557 } 558 559 public String toCode(QualityType code) { 560 if (code == QualityType.INDEL) 561 return "indel"; 562 if (code == QualityType.SNP) 563 return "snp"; 564 if (code == QualityType.UNKNOWN) 565 return "unknown"; 566 return "?"; 567 } 568 569 public String toSystem(QualityType code) { 570 return code.getSystem(); 571 } 572 } 573 574 public enum RepositoryType { 575 /** 576 * When URL is clicked, the resource can be seen directly (by webpage or by 577 * download link format). 578 */ 579 DIRECTLINK, 580 /** 581 * When the API method (e.g. [base_url]/[parameter]) related with the URL of the 582 * website is executed, the resource can be seen directly (usually in JSON or 583 * XML format). 584 */ 585 OPENAPI, 586 /** 587 * When logged into the website, the resource can be seen. 588 */ 589 LOGIN, 590 /** 591 * When logged in and follow the API in the website related with URL, the 592 * resource can be seen. 593 */ 594 OAUTH, 595 /** 596 * Some other complicated or particular way to get resource from URL. 597 */ 598 OTHER, 599 /** 600 * added to help the parsers with the generic types 601 */ 602 NULL; 603 604 public static RepositoryType fromCode(String codeString) throws FHIRException { 605 if (codeString == null || "".equals(codeString)) 606 return null; 607 if ("directlink".equals(codeString)) 608 return DIRECTLINK; 609 if ("openapi".equals(codeString)) 610 return OPENAPI; 611 if ("login".equals(codeString)) 612 return LOGIN; 613 if ("oauth".equals(codeString)) 614 return OAUTH; 615 if ("other".equals(codeString)) 616 return OTHER; 617 if (Configuration.isAcceptInvalidEnums()) 618 return null; 619 else 620 throw new FHIRException("Unknown RepositoryType code '" + codeString + "'"); 621 } 622 623 public String toCode() { 624 switch (this) { 625 case DIRECTLINK: 626 return "directlink"; 627 case OPENAPI: 628 return "openapi"; 629 case LOGIN: 630 return "login"; 631 case OAUTH: 632 return "oauth"; 633 case OTHER: 634 return "other"; 635 case NULL: 636 return null; 637 default: 638 return "?"; 639 } 640 } 641 642 public String getSystem() { 643 switch (this) { 644 case DIRECTLINK: 645 return "http://hl7.org/fhir/repository-type"; 646 case OPENAPI: 647 return "http://hl7.org/fhir/repository-type"; 648 case LOGIN: 649 return "http://hl7.org/fhir/repository-type"; 650 case OAUTH: 651 return "http://hl7.org/fhir/repository-type"; 652 case OTHER: 653 return "http://hl7.org/fhir/repository-type"; 654 case NULL: 655 return null; 656 default: 657 return "?"; 658 } 659 } 660 661 public String getDefinition() { 662 switch (this) { 663 case DIRECTLINK: 664 return "When URL is clicked, the resource can be seen directly (by webpage or by download link format)."; 665 case OPENAPI: 666 return "When the API method (e.g. [base_url]/[parameter]) related with the URL of the website is executed, the resource can be seen directly (usually in JSON or XML format)."; 667 case LOGIN: 668 return "When logged into the website, the resource can be seen."; 669 case OAUTH: 670 return "When logged in and follow the API in the website related with URL, the resource can be seen."; 671 case OTHER: 672 return "Some other complicated or particular way to get resource from URL."; 673 case NULL: 674 return null; 675 default: 676 return "?"; 677 } 678 } 679 680 public String getDisplay() { 681 switch (this) { 682 case DIRECTLINK: 683 return "Click and see"; 684 case OPENAPI: 685 return "The URL is the RESTful or other kind of API that can access to the result."; 686 case LOGIN: 687 return "Result cannot be access unless an account is logged in"; 688 case OAUTH: 689 return "Result need to be fetched with API and need LOGIN( or cookies are required when visiting the link of resource)"; 690 case OTHER: 691 return "Some other complicated or particular way to get resource from URL."; 692 case NULL: 693 return null; 694 default: 695 return "?"; 696 } 697 } 698 } 699 700 public static class RepositoryTypeEnumFactory implements EnumFactory<RepositoryType> { 701 public RepositoryType fromCode(String codeString) throws IllegalArgumentException { 702 if (codeString == null || "".equals(codeString)) 703 if (codeString == null || "".equals(codeString)) 704 return null; 705 if ("directlink".equals(codeString)) 706 return RepositoryType.DIRECTLINK; 707 if ("openapi".equals(codeString)) 708 return RepositoryType.OPENAPI; 709 if ("login".equals(codeString)) 710 return RepositoryType.LOGIN; 711 if ("oauth".equals(codeString)) 712 return RepositoryType.OAUTH; 713 if ("other".equals(codeString)) 714 return RepositoryType.OTHER; 715 throw new IllegalArgumentException("Unknown RepositoryType code '" + codeString + "'"); 716 } 717 718 public Enumeration<RepositoryType> fromType(PrimitiveType<?> code) throws FHIRException { 719 if (code == null) 720 return null; 721 if (code.isEmpty()) 722 return new Enumeration<RepositoryType>(this, RepositoryType.NULL, code); 723 String codeString = code.asStringValue(); 724 if (codeString == null || "".equals(codeString)) 725 return new Enumeration<RepositoryType>(this, RepositoryType.NULL, code); 726 if ("directlink".equals(codeString)) 727 return new Enumeration<RepositoryType>(this, RepositoryType.DIRECTLINK, code); 728 if ("openapi".equals(codeString)) 729 return new Enumeration<RepositoryType>(this, RepositoryType.OPENAPI, code); 730 if ("login".equals(codeString)) 731 return new Enumeration<RepositoryType>(this, RepositoryType.LOGIN, code); 732 if ("oauth".equals(codeString)) 733 return new Enumeration<RepositoryType>(this, RepositoryType.OAUTH, code); 734 if ("other".equals(codeString)) 735 return new Enumeration<RepositoryType>(this, RepositoryType.OTHER, code); 736 throw new FHIRException("Unknown RepositoryType code '" + codeString + "'"); 737 } 738 739 public String toCode(RepositoryType code) { 740 if (code == RepositoryType.DIRECTLINK) 741 return "directlink"; 742 if (code == RepositoryType.OPENAPI) 743 return "openapi"; 744 if (code == RepositoryType.LOGIN) 745 return "login"; 746 if (code == RepositoryType.OAUTH) 747 return "oauth"; 748 if (code == RepositoryType.OTHER) 749 return "other"; 750 return "?"; 751 } 752 753 public String toSystem(RepositoryType code) { 754 return code.getSystem(); 755 } 756 } 757 758 @Block() 759 public static class MolecularSequenceReferenceSeqComponent extends BackboneElement implements IBaseBackboneElement { 760 /** 761 * Structural unit composed of a nucleic acid molecule which controls its own 762 * replication through the interaction of specific proteins at one or more 763 * origins of replication 764 * ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)). 765 */ 766 @Child(name = "chromosome", type = { 767 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 768 @Description(shortDefinition = "Chromosome containing genetic finding", formalDefinition = "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).") 769 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chromosome-human") 770 protected CodeableConcept chromosome; 771 772 /** 773 * The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 774 * 37'. Version number must be included if a versioned release of a primary 775 * build was used. 776 */ 777 @Child(name = "genomeBuild", type = { 778 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 779 @Description(shortDefinition = "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'", formalDefinition = "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.") 780 protected StringType genomeBuild; 781 782 /** 783 * A relative reference to a DNA strand based on gene orientation. The strand 784 * that contains the open reading frame of the gene is the "sense" strand, and 785 * the opposite complementary strand is the "antisense" strand. 786 */ 787 @Child(name = "orientation", type = { 788 CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 789 @Description(shortDefinition = "sense | antisense", formalDefinition = "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.") 790 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/orientation-type") 791 protected Enumeration<OrientationType> orientation; 792 793 /** 794 * Reference identifier of reference sequence submitted to NCBI. It must match 795 * the type in the MolecularSequence.type field. For example, the prefix, ?NG_? 796 * identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, 797 * and ?NP_? for amino acid sequences. 798 */ 799 @Child(name = "referenceSeqId", type = { 800 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 801 @Description(shortDefinition = "Reference identifier", formalDefinition = "Reference identifier of reference sequence submitted to NCBI. It must match the type in the MolecularSequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.") 802 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-referenceSeq") 803 protected CodeableConcept referenceSeqId; 804 805 /** 806 * A pointer to another MolecularSequence entity as reference sequence. 807 */ 808 @Child(name = "referenceSeqPointer", type = { 809 MolecularSequence.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 810 @Description(shortDefinition = "A pointer to another MolecularSequence entity as reference sequence", formalDefinition = "A pointer to another MolecularSequence entity as reference sequence.") 811 protected Reference referenceSeqPointer; 812 813 /** 814 * The actual object that is the target of the reference (A pointer to another 815 * MolecularSequence entity as reference sequence.) 816 */ 817 protected MolecularSequence referenceSeqPointerTarget; 818 819 /** 820 * A string like "ACGT". 821 */ 822 @Child(name = "referenceSeqString", type = { 823 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 824 @Description(shortDefinition = "A string to represent reference sequence", formalDefinition = "A string like \"ACGT\".") 825 protected StringType referenceSeqString; 826 827 /** 828 * An absolute reference to a strand. The Watson strand is the strand whose 829 * 5'-end is on the short arm of the chromosome, and the Crick strand as the one 830 * whose 5'-end is on the long arm. 831 */ 832 @Child(name = "strand", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 833 @Description(shortDefinition = "watson | crick", formalDefinition = "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.") 834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/strand-type") 835 protected Enumeration<StrandType> strand; 836 837 /** 838 * Start position of the window on the reference sequence. If the coordinate 839 * system is either 0-based or 1-based, then start position is inclusive. 840 */ 841 @Child(name = "windowStart", type = { 842 IntegerType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 843 @Description(shortDefinition = "Start position of the window on the reference sequence", formalDefinition = "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 844 protected IntegerType windowStart; 845 846 /** 847 * End position of the window on the reference sequence. If the coordinate 848 * system is 0-based then end is exclusive and does not include the last 849 * position. If the coordinate system is 1-base, then end is inclusive and 850 * includes the last position. 851 */ 852 @Child(name = "windowEnd", type = { 853 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 854 @Description(shortDefinition = "End position of the window on the reference sequence", formalDefinition = "End position of the window on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 855 protected IntegerType windowEnd; 856 857 private static final long serialVersionUID = 307364267L; 858 859 /** 860 * Constructor 861 */ 862 public MolecularSequenceReferenceSeqComponent() { 863 super(); 864 } 865 866 /** 867 * @return {@link #chromosome} (Structural unit composed of a nucleic acid 868 * molecule which controls its own replication through the interaction 869 * of specific proteins at one or more origins of replication 870 * ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 871 */ 872 public CodeableConcept getChromosome() { 873 if (this.chromosome == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.chromosome"); 876 else if (Configuration.doAutoCreate()) 877 this.chromosome = new CodeableConcept(); // cc 878 return this.chromosome; 879 } 880 881 public boolean hasChromosome() { 882 return this.chromosome != null && !this.chromosome.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #chromosome} (Structural unit composed of a nucleic acid 887 * molecule which controls its own replication through the 888 * interaction of specific proteins at one or more origins of 889 * replication 890 * ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 891 */ 892 public MolecularSequenceReferenceSeqComponent setChromosome(CodeableConcept value) { 893 this.chromosome = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #genomeBuild} (The Genome Build used for reference, following 899 * GRCh build versions e.g. 'GRCh 37'. Version number must be included 900 * if a versioned release of a primary build was used.). This is the 901 * underlying object with id, value and extensions. The accessor 902 * "getGenomeBuild" gives direct access to the value 903 */ 904 public StringType getGenomeBuildElement() { 905 if (this.genomeBuild == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.genomeBuild"); 908 else if (Configuration.doAutoCreate()) 909 this.genomeBuild = new StringType(); // bb 910 return this.genomeBuild; 911 } 912 913 public boolean hasGenomeBuildElement() { 914 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 915 } 916 917 public boolean hasGenomeBuild() { 918 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #genomeBuild} (The Genome Build used for reference, 923 * following GRCh build versions e.g. 'GRCh 37'. Version number 924 * must be included if a versioned release of a primary build was 925 * used.). This is the underlying object with id, value and 926 * extensions. The accessor "getGenomeBuild" gives direct access to 927 * the value 928 */ 929 public MolecularSequenceReferenceSeqComponent setGenomeBuildElement(StringType value) { 930 this.genomeBuild = value; 931 return this; 932 } 933 934 /** 935 * @return The Genome Build used for reference, following GRCh build versions 936 * e.g. 'GRCh 37'. Version number must be included if a versioned 937 * release of a primary build was used. 938 */ 939 public String getGenomeBuild() { 940 return this.genomeBuild == null ? null : this.genomeBuild.getValue(); 941 } 942 943 /** 944 * @param value The Genome Build used for reference, following GRCh build 945 * versions e.g. 'GRCh 37'. Version number must be included if a 946 * versioned release of a primary build was used. 947 */ 948 public MolecularSequenceReferenceSeqComponent setGenomeBuild(String value) { 949 if (Utilities.noString(value)) 950 this.genomeBuild = null; 951 else { 952 if (this.genomeBuild == null) 953 this.genomeBuild = new StringType(); 954 this.genomeBuild.setValue(value); 955 } 956 return this; 957 } 958 959 /** 960 * @return {@link #orientation} (A relative reference to a DNA strand based on 961 * gene orientation. The strand that contains the open reading frame of 962 * the gene is the "sense" strand, and the opposite complementary strand 963 * is the "antisense" strand.). This is the underlying object with id, 964 * value and extensions. The accessor "getOrientation" gives direct 965 * access to the value 966 */ 967 public Enumeration<OrientationType> getOrientationElement() { 968 if (this.orientation == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.orientation"); 971 else if (Configuration.doAutoCreate()) 972 this.orientation = new Enumeration<OrientationType>(new OrientationTypeEnumFactory()); // bb 973 return this.orientation; 974 } 975 976 public boolean hasOrientationElement() { 977 return this.orientation != null && !this.orientation.isEmpty(); 978 } 979 980 public boolean hasOrientation() { 981 return this.orientation != null && !this.orientation.isEmpty(); 982 } 983 984 /** 985 * @param value {@link #orientation} (A relative reference to a DNA strand based 986 * on gene orientation. The strand that contains the open reading 987 * frame of the gene is the "sense" strand, and the opposite 988 * complementary strand is the "antisense" strand.). This is the 989 * underlying object with id, value and extensions. The accessor 990 * "getOrientation" gives direct access to the value 991 */ 992 public MolecularSequenceReferenceSeqComponent setOrientationElement(Enumeration<OrientationType> value) { 993 this.orientation = value; 994 return this; 995 } 996 997 /** 998 * @return A relative reference to a DNA strand based on gene orientation. The 999 * strand that contains the open reading frame of the gene is the 1000 * "sense" strand, and the opposite complementary strand is the 1001 * "antisense" strand. 1002 */ 1003 public OrientationType getOrientation() { 1004 return this.orientation == null ? null : this.orientation.getValue(); 1005 } 1006 1007 /** 1008 * @param value A relative reference to a DNA strand based on gene orientation. 1009 * The strand that contains the open reading frame of the gene is 1010 * the "sense" strand, and the opposite complementary strand is the 1011 * "antisense" strand. 1012 */ 1013 public MolecularSequenceReferenceSeqComponent setOrientation(OrientationType value) { 1014 if (value == null) 1015 this.orientation = null; 1016 else { 1017 if (this.orientation == null) 1018 this.orientation = new Enumeration<OrientationType>(new OrientationTypeEnumFactory()); 1019 this.orientation.setValue(value); 1020 } 1021 return this; 1022 } 1023 1024 /** 1025 * @return {@link #referenceSeqId} (Reference identifier of reference sequence 1026 * submitted to NCBI. It must match the type in the 1027 * MolecularSequence.type field. For example, the prefix, ?NG_? 1028 * identifies reference sequence for genes, ?NM_? for messenger RNA 1029 * transcripts, and ?NP_? for amino acid sequences.) 1030 */ 1031 public CodeableConcept getReferenceSeqId() { 1032 if (this.referenceSeqId == null) 1033 if (Configuration.errorOnAutoCreate()) 1034 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqId"); 1035 else if (Configuration.doAutoCreate()) 1036 this.referenceSeqId = new CodeableConcept(); // cc 1037 return this.referenceSeqId; 1038 } 1039 1040 public boolean hasReferenceSeqId() { 1041 return this.referenceSeqId != null && !this.referenceSeqId.isEmpty(); 1042 } 1043 1044 /** 1045 * @param value {@link #referenceSeqId} (Reference identifier of reference 1046 * sequence submitted to NCBI. It must match the type in the 1047 * MolecularSequence.type field. For example, the prefix, ?NG_? 1048 * identifies reference sequence for genes, ?NM_? for messenger RNA 1049 * transcripts, and ?NP_? for amino acid sequences.) 1050 */ 1051 public MolecularSequenceReferenceSeqComponent setReferenceSeqId(CodeableConcept value) { 1052 this.referenceSeqId = value; 1053 return this; 1054 } 1055 1056 /** 1057 * @return {@link #referenceSeqPointer} (A pointer to another MolecularSequence 1058 * entity as reference sequence.) 1059 */ 1060 public Reference getReferenceSeqPointer() { 1061 if (this.referenceSeqPointer == null) 1062 if (Configuration.errorOnAutoCreate()) 1063 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqPointer"); 1064 else if (Configuration.doAutoCreate()) 1065 this.referenceSeqPointer = new Reference(); // cc 1066 return this.referenceSeqPointer; 1067 } 1068 1069 public boolean hasReferenceSeqPointer() { 1070 return this.referenceSeqPointer != null && !this.referenceSeqPointer.isEmpty(); 1071 } 1072 1073 /** 1074 * @param value {@link #referenceSeqPointer} (A pointer to another 1075 * MolecularSequence entity as reference sequence.) 1076 */ 1077 public MolecularSequenceReferenceSeqComponent setReferenceSeqPointer(Reference value) { 1078 this.referenceSeqPointer = value; 1079 return this; 1080 } 1081 1082 /** 1083 * @return {@link #referenceSeqPointer} The actual object that is the target of 1084 * the reference. The reference library doesn't populate this, but you 1085 * can use it to hold the resource if you resolve it. (A pointer to 1086 * another MolecularSequence entity as reference sequence.) 1087 */ 1088 public MolecularSequence getReferenceSeqPointerTarget() { 1089 if (this.referenceSeqPointerTarget == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqPointer"); 1092 else if (Configuration.doAutoCreate()) 1093 this.referenceSeqPointerTarget = new MolecularSequence(); // aa 1094 return this.referenceSeqPointerTarget; 1095 } 1096 1097 /** 1098 * @param value {@link #referenceSeqPointer} The actual object that is the 1099 * target of the reference. The reference library doesn't use 1100 * these, but you can use it to hold the resource if you resolve 1101 * it. (A pointer to another MolecularSequence entity as reference 1102 * sequence.) 1103 */ 1104 public MolecularSequenceReferenceSeqComponent setReferenceSeqPointerTarget(MolecularSequence value) { 1105 this.referenceSeqPointerTarget = value; 1106 return this; 1107 } 1108 1109 /** 1110 * @return {@link #referenceSeqString} (A string like "ACGT".). This is the 1111 * underlying object with id, value and extensions. The accessor 1112 * "getReferenceSeqString" gives direct access to the value 1113 */ 1114 public StringType getReferenceSeqStringElement() { 1115 if (this.referenceSeqString == null) 1116 if (Configuration.errorOnAutoCreate()) 1117 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqString"); 1118 else if (Configuration.doAutoCreate()) 1119 this.referenceSeqString = new StringType(); // bb 1120 return this.referenceSeqString; 1121 } 1122 1123 public boolean hasReferenceSeqStringElement() { 1124 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 1125 } 1126 1127 public boolean hasReferenceSeqString() { 1128 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 1129 } 1130 1131 /** 1132 * @param value {@link #referenceSeqString} (A string like "ACGT".). This is the 1133 * underlying object with id, value and extensions. The accessor 1134 * "getReferenceSeqString" gives direct access to the value 1135 */ 1136 public MolecularSequenceReferenceSeqComponent setReferenceSeqStringElement(StringType value) { 1137 this.referenceSeqString = value; 1138 return this; 1139 } 1140 1141 /** 1142 * @return A string like "ACGT". 1143 */ 1144 public String getReferenceSeqString() { 1145 return this.referenceSeqString == null ? null : this.referenceSeqString.getValue(); 1146 } 1147 1148 /** 1149 * @param value A string like "ACGT". 1150 */ 1151 public MolecularSequenceReferenceSeqComponent setReferenceSeqString(String value) { 1152 if (Utilities.noString(value)) 1153 this.referenceSeqString = null; 1154 else { 1155 if (this.referenceSeqString == null) 1156 this.referenceSeqString = new StringType(); 1157 this.referenceSeqString.setValue(value); 1158 } 1159 return this; 1160 } 1161 1162 /** 1163 * @return {@link #strand} (An absolute reference to a strand. The Watson strand 1164 * is the strand whose 5'-end is on the short arm of the chromosome, and 1165 * the Crick strand as the one whose 5'-end is on the long arm.). This 1166 * is the underlying object with id, value and extensions. The accessor 1167 * "getStrand" gives direct access to the value 1168 */ 1169 public Enumeration<StrandType> getStrandElement() { 1170 if (this.strand == null) 1171 if (Configuration.errorOnAutoCreate()) 1172 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.strand"); 1173 else if (Configuration.doAutoCreate()) 1174 this.strand = new Enumeration<StrandType>(new StrandTypeEnumFactory()); // bb 1175 return this.strand; 1176 } 1177 1178 public boolean hasStrandElement() { 1179 return this.strand != null && !this.strand.isEmpty(); 1180 } 1181 1182 public boolean hasStrand() { 1183 return this.strand != null && !this.strand.isEmpty(); 1184 } 1185 1186 /** 1187 * @param value {@link #strand} (An absolute reference to a strand. The Watson 1188 * strand is the strand whose 5'-end is on the short arm of the 1189 * chromosome, and the Crick strand as the one whose 5'-end is on 1190 * the long arm.). This is the underlying object with id, value and 1191 * extensions. The accessor "getStrand" gives direct access to the 1192 * value 1193 */ 1194 public MolecularSequenceReferenceSeqComponent setStrandElement(Enumeration<StrandType> value) { 1195 this.strand = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return An absolute reference to a strand. The Watson strand is the strand 1201 * whose 5'-end is on the short arm of the chromosome, and the Crick 1202 * strand as the one whose 5'-end is on the long arm. 1203 */ 1204 public StrandType getStrand() { 1205 return this.strand == null ? null : this.strand.getValue(); 1206 } 1207 1208 /** 1209 * @param value An absolute reference to a strand. The Watson strand is the 1210 * strand whose 5'-end is on the short arm of the chromosome, and 1211 * the Crick strand as the one whose 5'-end is on the long arm. 1212 */ 1213 public MolecularSequenceReferenceSeqComponent setStrand(StrandType value) { 1214 if (value == null) 1215 this.strand = null; 1216 else { 1217 if (this.strand == null) 1218 this.strand = new Enumeration<StrandType>(new StrandTypeEnumFactory()); 1219 this.strand.setValue(value); 1220 } 1221 return this; 1222 } 1223 1224 /** 1225 * @return {@link #windowStart} (Start position of the window on the reference 1226 * sequence. If the coordinate system is either 0-based or 1-based, then 1227 * start position is inclusive.). This is the underlying object with id, 1228 * value and extensions. The accessor "getWindowStart" gives direct 1229 * access to the value 1230 */ 1231 public IntegerType getWindowStartElement() { 1232 if (this.windowStart == null) 1233 if (Configuration.errorOnAutoCreate()) 1234 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.windowStart"); 1235 else if (Configuration.doAutoCreate()) 1236 this.windowStart = new IntegerType(); // bb 1237 return this.windowStart; 1238 } 1239 1240 public boolean hasWindowStartElement() { 1241 return this.windowStart != null && !this.windowStart.isEmpty(); 1242 } 1243 1244 public boolean hasWindowStart() { 1245 return this.windowStart != null && !this.windowStart.isEmpty(); 1246 } 1247 1248 /** 1249 * @param value {@link #windowStart} (Start position of the window on the 1250 * reference sequence. If the coordinate system is either 0-based 1251 * or 1-based, then start position is inclusive.). This is the 1252 * underlying object with id, value and extensions. The accessor 1253 * "getWindowStart" gives direct access to the value 1254 */ 1255 public MolecularSequenceReferenceSeqComponent setWindowStartElement(IntegerType value) { 1256 this.windowStart = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return Start position of the window on the reference sequence. If the 1262 * coordinate system is either 0-based or 1-based, then start position 1263 * is inclusive. 1264 */ 1265 public int getWindowStart() { 1266 return this.windowStart == null || this.windowStart.isEmpty() ? 0 : this.windowStart.getValue(); 1267 } 1268 1269 /** 1270 * @param value Start position of the window on the reference sequence. If the 1271 * coordinate system is either 0-based or 1-based, then start 1272 * position is inclusive. 1273 */ 1274 public MolecularSequenceReferenceSeqComponent setWindowStart(int value) { 1275 if (this.windowStart == null) 1276 this.windowStart = new IntegerType(); 1277 this.windowStart.setValue(value); 1278 return this; 1279 } 1280 1281 /** 1282 * @return {@link #windowEnd} (End position of the window on the reference 1283 * sequence. If the coordinate system is 0-based then end is exclusive 1284 * and does not include the last position. If the coordinate system is 1285 * 1-base, then end is inclusive and includes the last position.). This 1286 * is the underlying object with id, value and extensions. The accessor 1287 * "getWindowEnd" gives direct access to the value 1288 */ 1289 public IntegerType getWindowEndElement() { 1290 if (this.windowEnd == null) 1291 if (Configuration.errorOnAutoCreate()) 1292 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.windowEnd"); 1293 else if (Configuration.doAutoCreate()) 1294 this.windowEnd = new IntegerType(); // bb 1295 return this.windowEnd; 1296 } 1297 1298 public boolean hasWindowEndElement() { 1299 return this.windowEnd != null && !this.windowEnd.isEmpty(); 1300 } 1301 1302 public boolean hasWindowEnd() { 1303 return this.windowEnd != null && !this.windowEnd.isEmpty(); 1304 } 1305 1306 /** 1307 * @param value {@link #windowEnd} (End position of the window on the reference 1308 * sequence. If the coordinate system is 0-based then end is 1309 * exclusive and does not include the last position. If the 1310 * coordinate system is 1-base, then end is inclusive and includes 1311 * the last position.). This is the underlying object with id, 1312 * value and extensions. The accessor "getWindowEnd" gives direct 1313 * access to the value 1314 */ 1315 public MolecularSequenceReferenceSeqComponent setWindowEndElement(IntegerType value) { 1316 this.windowEnd = value; 1317 return this; 1318 } 1319 1320 /** 1321 * @return End position of the window on the reference sequence. If the 1322 * coordinate system is 0-based then end is exclusive and does not 1323 * include the last position. If the coordinate system is 1-base, then 1324 * end is inclusive and includes the last position. 1325 */ 1326 public int getWindowEnd() { 1327 return this.windowEnd == null || this.windowEnd.isEmpty() ? 0 : this.windowEnd.getValue(); 1328 } 1329 1330 /** 1331 * @param value End position of the window on the reference sequence. If the 1332 * coordinate system is 0-based then end is exclusive and does not 1333 * include the last position. If the coordinate system is 1-base, 1334 * then end is inclusive and includes the last position. 1335 */ 1336 public MolecularSequenceReferenceSeqComponent setWindowEnd(int value) { 1337 if (this.windowEnd == null) 1338 this.windowEnd = new IntegerType(); 1339 this.windowEnd.setValue(value); 1340 return this; 1341 } 1342 1343 protected void listChildren(List<Property> children) { 1344 super.listChildren(children); 1345 children.add(new Property("chromosome", "CodeableConcept", 1346 "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 1347 0, 1, chromosome)); 1348 children.add(new Property("genomeBuild", "string", 1349 "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 1350 0, 1, genomeBuild)); 1351 children.add(new Property("orientation", "code", 1352 "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.", 1353 0, 1, orientation)); 1354 children.add(new Property("referenceSeqId", "CodeableConcept", 1355 "Reference identifier of reference sequence submitted to NCBI. It must match the type in the MolecularSequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 1356 0, 1, referenceSeqId)); 1357 children.add(new Property("referenceSeqPointer", "Reference(MolecularSequence)", 1358 "A pointer to another MolecularSequence entity as reference sequence.", 0, 1, referenceSeqPointer)); 1359 children.add(new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, referenceSeqString)); 1360 children.add(new Property("strand", "code", 1361 "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.", 1362 0, 1, strand)); 1363 children.add(new Property("windowStart", "integer", 1364 "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 1365 0, 1, windowStart)); 1366 children.add(new Property("windowEnd", "integer", 1367 "End position of the window on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 1368 0, 1, windowEnd)); 1369 } 1370 1371 @Override 1372 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1373 switch (_hash) { 1374 case -1499470472: 1375 /* chromosome */ return new Property("chromosome", "CodeableConcept", 1376 "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 1377 0, 1, chromosome); 1378 case 1061239735: 1379 /* genomeBuild */ return new Property("genomeBuild", "string", 1380 "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 1381 0, 1, genomeBuild); 1382 case -1439500848: 1383 /* orientation */ return new Property("orientation", "code", 1384 "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.", 1385 0, 1, orientation); 1386 case -1911500465: 1387 /* referenceSeqId */ return new Property("referenceSeqId", "CodeableConcept", 1388 "Reference identifier of reference sequence submitted to NCBI. It must match the type in the MolecularSequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 1389 0, 1, referenceSeqId); 1390 case 1923414665: 1391 /* referenceSeqPointer */ return new Property("referenceSeqPointer", "Reference(MolecularSequence)", 1392 "A pointer to another MolecularSequence entity as reference sequence.", 0, 1, referenceSeqPointer); 1393 case -1648301499: 1394 /* referenceSeqString */ return new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, 1395 referenceSeqString); 1396 case -891993594: 1397 /* strand */ return new Property("strand", "code", 1398 "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.", 1399 0, 1, strand); 1400 case 1903685202: 1401 /* windowStart */ return new Property("windowStart", "integer", 1402 "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 1403 0, 1, windowStart); 1404 case -217026869: 1405 /* windowEnd */ return new Property("windowEnd", "integer", 1406 "End position of the window on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 1407 0, 1, windowEnd); 1408 default: 1409 return super.getNamedProperty(_hash, _name, _checkValid); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1416 switch (hash) { 1417 case -1499470472: 1418 /* chromosome */ return this.chromosome == null ? new Base[0] : new Base[] { this.chromosome }; // CodeableConcept 1419 case 1061239735: 1420 /* genomeBuild */ return this.genomeBuild == null ? new Base[0] : new Base[] { this.genomeBuild }; // StringType 1421 case -1439500848: 1422 /* orientation */ return this.orientation == null ? new Base[0] : new Base[] { this.orientation }; // Enumeration<OrientationType> 1423 case -1911500465: 1424 /* referenceSeqId */ return this.referenceSeqId == null ? new Base[0] : new Base[] { this.referenceSeqId }; // CodeableConcept 1425 case 1923414665: 1426 /* referenceSeqPointer */ return this.referenceSeqPointer == null ? new Base[0] 1427 : new Base[] { this.referenceSeqPointer }; // Reference 1428 case -1648301499: 1429 /* referenceSeqString */ return this.referenceSeqString == null ? new Base[0] 1430 : new Base[] { this.referenceSeqString }; // StringType 1431 case -891993594: 1432 /* strand */ return this.strand == null ? new Base[0] : new Base[] { this.strand }; // Enumeration<StrandType> 1433 case 1903685202: 1434 /* windowStart */ return this.windowStart == null ? new Base[0] : new Base[] { this.windowStart }; // IntegerType 1435 case -217026869: 1436 /* windowEnd */ return this.windowEnd == null ? new Base[0] : new Base[] { this.windowEnd }; // IntegerType 1437 default: 1438 return super.getProperty(hash, name, checkValid); 1439 } 1440 1441 } 1442 1443 @Override 1444 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1445 switch (hash) { 1446 case -1499470472: // chromosome 1447 this.chromosome = castToCodeableConcept(value); // CodeableConcept 1448 return value; 1449 case 1061239735: // genomeBuild 1450 this.genomeBuild = castToString(value); // StringType 1451 return value; 1452 case -1439500848: // orientation 1453 value = new OrientationTypeEnumFactory().fromType(castToCode(value)); 1454 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1455 return value; 1456 case -1911500465: // referenceSeqId 1457 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 1458 return value; 1459 case 1923414665: // referenceSeqPointer 1460 this.referenceSeqPointer = castToReference(value); // Reference 1461 return value; 1462 case -1648301499: // referenceSeqString 1463 this.referenceSeqString = castToString(value); // StringType 1464 return value; 1465 case -891993594: // strand 1466 value = new StrandTypeEnumFactory().fromType(castToCode(value)); 1467 this.strand = (Enumeration) value; // Enumeration<StrandType> 1468 return value; 1469 case 1903685202: // windowStart 1470 this.windowStart = castToInteger(value); // IntegerType 1471 return value; 1472 case -217026869: // windowEnd 1473 this.windowEnd = castToInteger(value); // IntegerType 1474 return value; 1475 default: 1476 return super.setProperty(hash, name, value); 1477 } 1478 1479 } 1480 1481 @Override 1482 public Base setProperty(String name, Base value) throws FHIRException { 1483 if (name.equals("chromosome")) { 1484 this.chromosome = castToCodeableConcept(value); // CodeableConcept 1485 } else if (name.equals("genomeBuild")) { 1486 this.genomeBuild = castToString(value); // StringType 1487 } else if (name.equals("orientation")) { 1488 value = new OrientationTypeEnumFactory().fromType(castToCode(value)); 1489 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1490 } else if (name.equals("referenceSeqId")) { 1491 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 1492 } else if (name.equals("referenceSeqPointer")) { 1493 this.referenceSeqPointer = castToReference(value); // Reference 1494 } else if (name.equals("referenceSeqString")) { 1495 this.referenceSeqString = castToString(value); // StringType 1496 } else if (name.equals("strand")) { 1497 value = new StrandTypeEnumFactory().fromType(castToCode(value)); 1498 this.strand = (Enumeration) value; // Enumeration<StrandType> 1499 } else if (name.equals("windowStart")) { 1500 this.windowStart = castToInteger(value); // IntegerType 1501 } else if (name.equals("windowEnd")) { 1502 this.windowEnd = castToInteger(value); // IntegerType 1503 } else 1504 return super.setProperty(name, value); 1505 return value; 1506 } 1507 1508 @Override 1509 public Base makeProperty(int hash, String name) throws FHIRException { 1510 switch (hash) { 1511 case -1499470472: 1512 return getChromosome(); 1513 case 1061239735: 1514 return getGenomeBuildElement(); 1515 case -1439500848: 1516 return getOrientationElement(); 1517 case -1911500465: 1518 return getReferenceSeqId(); 1519 case 1923414665: 1520 return getReferenceSeqPointer(); 1521 case -1648301499: 1522 return getReferenceSeqStringElement(); 1523 case -891993594: 1524 return getStrandElement(); 1525 case 1903685202: 1526 return getWindowStartElement(); 1527 case -217026869: 1528 return getWindowEndElement(); 1529 default: 1530 return super.makeProperty(hash, name); 1531 } 1532 1533 } 1534 1535 @Override 1536 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1537 switch (hash) { 1538 case -1499470472: 1539 /* chromosome */ return new String[] { "CodeableConcept" }; 1540 case 1061239735: 1541 /* genomeBuild */ return new String[] { "string" }; 1542 case -1439500848: 1543 /* orientation */ return new String[] { "code" }; 1544 case -1911500465: 1545 /* referenceSeqId */ return new String[] { "CodeableConcept" }; 1546 case 1923414665: 1547 /* referenceSeqPointer */ return new String[] { "Reference" }; 1548 case -1648301499: 1549 /* referenceSeqString */ return new String[] { "string" }; 1550 case -891993594: 1551 /* strand */ return new String[] { "code" }; 1552 case 1903685202: 1553 /* windowStart */ return new String[] { "integer" }; 1554 case -217026869: 1555 /* windowEnd */ return new String[] { "integer" }; 1556 default: 1557 return super.getTypesForProperty(hash, name); 1558 } 1559 1560 } 1561 1562 @Override 1563 public Base addChild(String name) throws FHIRException { 1564 if (name.equals("chromosome")) { 1565 this.chromosome = new CodeableConcept(); 1566 return this.chromosome; 1567 } else if (name.equals("genomeBuild")) { 1568 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.genomeBuild"); 1569 } else if (name.equals("orientation")) { 1570 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.orientation"); 1571 } else if (name.equals("referenceSeqId")) { 1572 this.referenceSeqId = new CodeableConcept(); 1573 return this.referenceSeqId; 1574 } else if (name.equals("referenceSeqPointer")) { 1575 this.referenceSeqPointer = new Reference(); 1576 return this.referenceSeqPointer; 1577 } else if (name.equals("referenceSeqString")) { 1578 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.referenceSeqString"); 1579 } else if (name.equals("strand")) { 1580 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.strand"); 1581 } else if (name.equals("windowStart")) { 1582 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.windowStart"); 1583 } else if (name.equals("windowEnd")) { 1584 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.windowEnd"); 1585 } else 1586 return super.addChild(name); 1587 } 1588 1589 public MolecularSequenceReferenceSeqComponent copy() { 1590 MolecularSequenceReferenceSeqComponent dst = new MolecularSequenceReferenceSeqComponent(); 1591 copyValues(dst); 1592 return dst; 1593 } 1594 1595 public void copyValues(MolecularSequenceReferenceSeqComponent dst) { 1596 super.copyValues(dst); 1597 dst.chromosome = chromosome == null ? null : chromosome.copy(); 1598 dst.genomeBuild = genomeBuild == null ? null : genomeBuild.copy(); 1599 dst.orientation = orientation == null ? null : orientation.copy(); 1600 dst.referenceSeqId = referenceSeqId == null ? null : referenceSeqId.copy(); 1601 dst.referenceSeqPointer = referenceSeqPointer == null ? null : referenceSeqPointer.copy(); 1602 dst.referenceSeqString = referenceSeqString == null ? null : referenceSeqString.copy(); 1603 dst.strand = strand == null ? null : strand.copy(); 1604 dst.windowStart = windowStart == null ? null : windowStart.copy(); 1605 dst.windowEnd = windowEnd == null ? null : windowEnd.copy(); 1606 } 1607 1608 @Override 1609 public boolean equalsDeep(Base other_) { 1610 if (!super.equalsDeep(other_)) 1611 return false; 1612 if (!(other_ instanceof MolecularSequenceReferenceSeqComponent)) 1613 return false; 1614 MolecularSequenceReferenceSeqComponent o = (MolecularSequenceReferenceSeqComponent) other_; 1615 return compareDeep(chromosome, o.chromosome, true) && compareDeep(genomeBuild, o.genomeBuild, true) 1616 && compareDeep(orientation, o.orientation, true) && compareDeep(referenceSeqId, o.referenceSeqId, true) 1617 && compareDeep(referenceSeqPointer, o.referenceSeqPointer, true) 1618 && compareDeep(referenceSeqString, o.referenceSeqString, true) && compareDeep(strand, o.strand, true) 1619 && compareDeep(windowStart, o.windowStart, true) && compareDeep(windowEnd, o.windowEnd, true); 1620 } 1621 1622 @Override 1623 public boolean equalsShallow(Base other_) { 1624 if (!super.equalsShallow(other_)) 1625 return false; 1626 if (!(other_ instanceof MolecularSequenceReferenceSeqComponent)) 1627 return false; 1628 MolecularSequenceReferenceSeqComponent o = (MolecularSequenceReferenceSeqComponent) other_; 1629 return compareValues(genomeBuild, o.genomeBuild, true) && compareValues(orientation, o.orientation, true) 1630 && compareValues(referenceSeqString, o.referenceSeqString, true) && compareValues(strand, o.strand, true) 1631 && compareValues(windowStart, o.windowStart, true) && compareValues(windowEnd, o.windowEnd, true); 1632 } 1633 1634 public boolean isEmpty() { 1635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(chromosome, genomeBuild, orientation, 1636 referenceSeqId, referenceSeqPointer, referenceSeqString, strand, windowStart, windowEnd); 1637 } 1638 1639 public String fhirType() { 1640 return "MolecularSequence.referenceSeq"; 1641 1642 } 1643 1644 } 1645 1646 @Block() 1647 public static class MolecularSequenceVariantComponent extends BackboneElement implements IBaseBackboneElement { 1648 /** 1649 * Start position of the variant on the reference sequence. If the coordinate 1650 * system is either 0-based or 1-based, then start position is inclusive. 1651 */ 1652 @Child(name = "start", type = { IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1653 @Description(shortDefinition = "Start position of the variant on the reference sequence", formalDefinition = "Start position of the variant on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 1654 protected IntegerType start; 1655 1656 /** 1657 * End position of the variant on the reference sequence. If the coordinate 1658 * system is 0-based then end is exclusive and does not include the last 1659 * position. If the coordinate system is 1-base, then end is inclusive and 1660 * includes the last position. 1661 */ 1662 @Child(name = "end", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1663 @Description(shortDefinition = "End position of the variant on the reference sequence", formalDefinition = "End position of the variant on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 1664 protected IntegerType end; 1665 1666 /** 1667 * An allele is one of a set of coexisting sequence variants of a gene 1668 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1669 * Nucleotide(s)/amino acids from start position of sequence to stop position of 1670 * sequence on the positive (+) strand of the observed sequence. When the 1671 * sequence type is DNA, it should be the sequence on the positive (+) strand. 1672 * This will lay in the range between variant.start and variant.end. 1673 */ 1674 @Child(name = "observedAllele", type = { 1675 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1676 @Description(shortDefinition = "Allele that was observed", formalDefinition = "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.") 1677 protected StringType observedAllele; 1678 1679 /** 1680 * An allele is one of a set of coexisting sequence variants of a gene 1681 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1682 * Nucleotide(s)/amino acids from start position of sequence to stop position of 1683 * sequence on the positive (+) strand of the reference sequence. When the 1684 * sequence type is DNA, it should be the sequence on the positive (+) strand. 1685 * This will lay in the range between variant.start and variant.end. 1686 */ 1687 @Child(name = "referenceAllele", type = { 1688 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1689 @Description(shortDefinition = "Allele in the reference sequence", formalDefinition = "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.") 1690 protected StringType referenceAllele; 1691 1692 /** 1693 * Extended CIGAR string for aligning the sequence with reference bases. See 1694 * detailed documentation 1695 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1696 */ 1697 @Child(name = "cigar", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1698 @Description(shortDefinition = "Extended CIGAR string for aligning the sequence with reference bases", formalDefinition = "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).") 1699 protected StringType cigar; 1700 1701 /** 1702 * A pointer to an Observation containing variant information. 1703 */ 1704 @Child(name = "variantPointer", type = { 1705 Observation.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1706 @Description(shortDefinition = "Pointer to observed variant information", formalDefinition = "A pointer to an Observation containing variant information.") 1707 protected Reference variantPointer; 1708 1709 /** 1710 * The actual object that is the target of the reference (A pointer to an 1711 * Observation containing variant information.) 1712 */ 1713 protected Observation variantPointerTarget; 1714 1715 private static final long serialVersionUID = 105611837L; 1716 1717 /** 1718 * Constructor 1719 */ 1720 public MolecularSequenceVariantComponent() { 1721 super(); 1722 } 1723 1724 /** 1725 * @return {@link #start} (Start position of the variant on the reference 1726 * sequence. If the coordinate system is either 0-based or 1-based, then 1727 * start position is inclusive.). This is the underlying object with id, 1728 * value and extensions. The accessor "getStart" gives direct access to 1729 * the value 1730 */ 1731 public IntegerType getStartElement() { 1732 if (this.start == null) 1733 if (Configuration.errorOnAutoCreate()) 1734 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.start"); 1735 else if (Configuration.doAutoCreate()) 1736 this.start = new IntegerType(); // bb 1737 return this.start; 1738 } 1739 1740 public boolean hasStartElement() { 1741 return this.start != null && !this.start.isEmpty(); 1742 } 1743 1744 public boolean hasStart() { 1745 return this.start != null && !this.start.isEmpty(); 1746 } 1747 1748 /** 1749 * @param value {@link #start} (Start position of the variant on the reference 1750 * sequence. If the coordinate system is either 0-based or 1-based, 1751 * then start position is inclusive.). This is the underlying 1752 * object with id, value and extensions. The accessor "getStart" 1753 * gives direct access to the value 1754 */ 1755 public MolecularSequenceVariantComponent setStartElement(IntegerType value) { 1756 this.start = value; 1757 return this; 1758 } 1759 1760 /** 1761 * @return Start position of the variant on the reference sequence. If the 1762 * coordinate system is either 0-based or 1-based, then start position 1763 * is inclusive. 1764 */ 1765 public int getStart() { 1766 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1767 } 1768 1769 /** 1770 * @param value Start position of the variant on the reference sequence. If the 1771 * coordinate system is either 0-based or 1-based, then start 1772 * position is inclusive. 1773 */ 1774 public MolecularSequenceVariantComponent setStart(int value) { 1775 if (this.start == null) 1776 this.start = new IntegerType(); 1777 this.start.setValue(value); 1778 return this; 1779 } 1780 1781 /** 1782 * @return {@link #end} (End position of the variant on the reference sequence. 1783 * If the coordinate system is 0-based then end is exclusive and does 1784 * not include the last position. If the coordinate system is 1-base, 1785 * then end is inclusive and includes the last position.). This is the 1786 * underlying object with id, value and extensions. The accessor 1787 * "getEnd" gives direct access to the value 1788 */ 1789 public IntegerType getEndElement() { 1790 if (this.end == null) 1791 if (Configuration.errorOnAutoCreate()) 1792 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.end"); 1793 else if (Configuration.doAutoCreate()) 1794 this.end = new IntegerType(); // bb 1795 return this.end; 1796 } 1797 1798 public boolean hasEndElement() { 1799 return this.end != null && !this.end.isEmpty(); 1800 } 1801 1802 public boolean hasEnd() { 1803 return this.end != null && !this.end.isEmpty(); 1804 } 1805 1806 /** 1807 * @param value {@link #end} (End position of the variant on the reference 1808 * sequence. If the coordinate system is 0-based then end is 1809 * exclusive and does not include the last position. If the 1810 * coordinate system is 1-base, then end is inclusive and includes 1811 * the last position.). This is the underlying object with id, 1812 * value and extensions. The accessor "getEnd" gives direct access 1813 * to the value 1814 */ 1815 public MolecularSequenceVariantComponent setEndElement(IntegerType value) { 1816 this.end = value; 1817 return this; 1818 } 1819 1820 /** 1821 * @return End position of the variant on the reference sequence. If the 1822 * coordinate system is 0-based then end is exclusive and does not 1823 * include the last position. If the coordinate system is 1-base, then 1824 * end is inclusive and includes the last position. 1825 */ 1826 public int getEnd() { 1827 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1828 } 1829 1830 /** 1831 * @param value End position of the variant on the reference sequence. If the 1832 * coordinate system is 0-based then end is exclusive and does not 1833 * include the last position. If the coordinate system is 1-base, 1834 * then end is inclusive and includes the last position. 1835 */ 1836 public MolecularSequenceVariantComponent setEnd(int value) { 1837 if (this.end == null) 1838 this.end = new IntegerType(); 1839 this.end.setValue(value); 1840 return this; 1841 } 1842 1843 /** 1844 * @return {@link #observedAllele} (An allele is one of a set of coexisting 1845 * sequence variants of a gene 1846 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1847 * Nucleotide(s)/amino acids from start position of sequence to stop 1848 * position of sequence on the positive (+) strand of the observed 1849 * sequence. When the sequence type is DNA, it should be the sequence on 1850 * the positive (+) strand. This will lay in the range between 1851 * variant.start and variant.end.). This is the underlying object with 1852 * id, value and extensions. The accessor "getObservedAllele" gives 1853 * direct access to the value 1854 */ 1855 public StringType getObservedAlleleElement() { 1856 if (this.observedAllele == null) 1857 if (Configuration.errorOnAutoCreate()) 1858 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.observedAllele"); 1859 else if (Configuration.doAutoCreate()) 1860 this.observedAllele = new StringType(); // bb 1861 return this.observedAllele; 1862 } 1863 1864 public boolean hasObservedAlleleElement() { 1865 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1866 } 1867 1868 public boolean hasObservedAllele() { 1869 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1870 } 1871 1872 /** 1873 * @param value {@link #observedAllele} (An allele is one of a set of coexisting 1874 * sequence variants of a gene 1875 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1876 * Nucleotide(s)/amino acids from start position of sequence to 1877 * stop position of sequence on the positive (+) strand of the 1878 * observed sequence. When the sequence type is DNA, it should be 1879 * the sequence on the positive (+) strand. This will lay in the 1880 * range between variant.start and variant.end.). This is the 1881 * underlying object with id, value and extensions. The accessor 1882 * "getObservedAllele" gives direct access to the value 1883 */ 1884 public MolecularSequenceVariantComponent setObservedAlleleElement(StringType value) { 1885 this.observedAllele = value; 1886 return this; 1887 } 1888 1889 /** 1890 * @return An allele is one of a set of coexisting sequence variants of a gene 1891 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1892 * Nucleotide(s)/amino acids from start position of sequence to stop 1893 * position of sequence on the positive (+) strand of the observed 1894 * sequence. When the sequence type is DNA, it should be the sequence on 1895 * the positive (+) strand. This will lay in the range between 1896 * variant.start and variant.end. 1897 */ 1898 public String getObservedAllele() { 1899 return this.observedAllele == null ? null : this.observedAllele.getValue(); 1900 } 1901 1902 /** 1903 * @param value An allele is one of a set of coexisting sequence variants of a 1904 * gene 1905 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1906 * Nucleotide(s)/amino acids from start position of sequence to 1907 * stop position of sequence on the positive (+) strand of the 1908 * observed sequence. When the sequence type is DNA, it should be 1909 * the sequence on the positive (+) strand. This will lay in the 1910 * range between variant.start and variant.end. 1911 */ 1912 public MolecularSequenceVariantComponent setObservedAllele(String value) { 1913 if (Utilities.noString(value)) 1914 this.observedAllele = null; 1915 else { 1916 if (this.observedAllele == null) 1917 this.observedAllele = new StringType(); 1918 this.observedAllele.setValue(value); 1919 } 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #referenceAllele} (An allele is one of a set of coexisting 1925 * sequence variants of a gene 1926 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1927 * Nucleotide(s)/amino acids from start position of sequence to stop 1928 * position of sequence on the positive (+) strand of the reference 1929 * sequence. When the sequence type is DNA, it should be the sequence on 1930 * the positive (+) strand. This will lay in the range between 1931 * variant.start and variant.end.). This is the underlying object with 1932 * id, value and extensions. The accessor "getReferenceAllele" gives 1933 * direct access to the value 1934 */ 1935 public StringType getReferenceAlleleElement() { 1936 if (this.referenceAllele == null) 1937 if (Configuration.errorOnAutoCreate()) 1938 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.referenceAllele"); 1939 else if (Configuration.doAutoCreate()) 1940 this.referenceAllele = new StringType(); // bb 1941 return this.referenceAllele; 1942 } 1943 1944 public boolean hasReferenceAlleleElement() { 1945 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1946 } 1947 1948 public boolean hasReferenceAllele() { 1949 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1950 } 1951 1952 /** 1953 * @param value {@link #referenceAllele} (An allele is one of a set of 1954 * coexisting sequence variants of a gene 1955 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1956 * Nucleotide(s)/amino acids from start position of sequence to 1957 * stop position of sequence on the positive (+) strand of the 1958 * reference sequence. When the sequence type is DNA, it should be 1959 * the sequence on the positive (+) strand. This will lay in the 1960 * range between variant.start and variant.end.). This is the 1961 * underlying object with id, value and extensions. The accessor 1962 * "getReferenceAllele" gives direct access to the value 1963 */ 1964 public MolecularSequenceVariantComponent setReferenceAlleleElement(StringType value) { 1965 this.referenceAllele = value; 1966 return this; 1967 } 1968 1969 /** 1970 * @return An allele is one of a set of coexisting sequence variants of a gene 1971 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1972 * Nucleotide(s)/amino acids from start position of sequence to stop 1973 * position of sequence on the positive (+) strand of the reference 1974 * sequence. When the sequence type is DNA, it should be the sequence on 1975 * the positive (+) strand. This will lay in the range between 1976 * variant.start and variant.end. 1977 */ 1978 public String getReferenceAllele() { 1979 return this.referenceAllele == null ? null : this.referenceAllele.getValue(); 1980 } 1981 1982 /** 1983 * @param value An allele is one of a set of coexisting sequence variants of a 1984 * gene 1985 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1986 * Nucleotide(s)/amino acids from start position of sequence to 1987 * stop position of sequence on the positive (+) strand of the 1988 * reference sequence. When the sequence type is DNA, it should be 1989 * the sequence on the positive (+) strand. This will lay in the 1990 * range between variant.start and variant.end. 1991 */ 1992 public MolecularSequenceVariantComponent setReferenceAllele(String value) { 1993 if (Utilities.noString(value)) 1994 this.referenceAllele = null; 1995 else { 1996 if (this.referenceAllele == null) 1997 this.referenceAllele = new StringType(); 1998 this.referenceAllele.setValue(value); 1999 } 2000 return this; 2001 } 2002 2003 /** 2004 * @return {@link #cigar} (Extended CIGAR string for aligning the sequence with 2005 * reference bases. See detailed documentation 2006 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). 2007 * This is the underlying object with id, value and extensions. The 2008 * accessor "getCigar" gives direct access to the value 2009 */ 2010 public StringType getCigarElement() { 2011 if (this.cigar == null) 2012 if (Configuration.errorOnAutoCreate()) 2013 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.cigar"); 2014 else if (Configuration.doAutoCreate()) 2015 this.cigar = new StringType(); // bb 2016 return this.cigar; 2017 } 2018 2019 public boolean hasCigarElement() { 2020 return this.cigar != null && !this.cigar.isEmpty(); 2021 } 2022 2023 public boolean hasCigar() { 2024 return this.cigar != null && !this.cigar.isEmpty(); 2025 } 2026 2027 /** 2028 * @param value {@link #cigar} (Extended CIGAR string for aligning the sequence 2029 * with reference bases. See detailed documentation 2030 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). 2031 * This is the underlying object with id, value and extensions. The 2032 * accessor "getCigar" gives direct access to the value 2033 */ 2034 public MolecularSequenceVariantComponent setCigarElement(StringType value) { 2035 this.cigar = value; 2036 return this; 2037 } 2038 2039 /** 2040 * @return Extended CIGAR string for aligning the sequence with reference bases. 2041 * See detailed documentation 2042 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 2043 */ 2044 public String getCigar() { 2045 return this.cigar == null ? null : this.cigar.getValue(); 2046 } 2047 2048 /** 2049 * @param value Extended CIGAR string for aligning the sequence with reference 2050 * bases. See detailed documentation 2051 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 2052 */ 2053 public MolecularSequenceVariantComponent setCigar(String value) { 2054 if (Utilities.noString(value)) 2055 this.cigar = null; 2056 else { 2057 if (this.cigar == null) 2058 this.cigar = new StringType(); 2059 this.cigar.setValue(value); 2060 } 2061 return this; 2062 } 2063 2064 /** 2065 * @return {@link #variantPointer} (A pointer to an Observation containing 2066 * variant information.) 2067 */ 2068 public Reference getVariantPointer() { 2069 if (this.variantPointer == null) 2070 if (Configuration.errorOnAutoCreate()) 2071 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.variantPointer"); 2072 else if (Configuration.doAutoCreate()) 2073 this.variantPointer = new Reference(); // cc 2074 return this.variantPointer; 2075 } 2076 2077 public boolean hasVariantPointer() { 2078 return this.variantPointer != null && !this.variantPointer.isEmpty(); 2079 } 2080 2081 /** 2082 * @param value {@link #variantPointer} (A pointer to an Observation containing 2083 * variant information.) 2084 */ 2085 public MolecularSequenceVariantComponent setVariantPointer(Reference value) { 2086 this.variantPointer = value; 2087 return this; 2088 } 2089 2090 /** 2091 * @return {@link #variantPointer} The actual object that is the target of the 2092 * reference. The reference library doesn't populate this, but you can 2093 * use it to hold the resource if you resolve it. (A pointer to an 2094 * Observation containing variant information.) 2095 */ 2096 public Observation getVariantPointerTarget() { 2097 if (this.variantPointerTarget == null) 2098 if (Configuration.errorOnAutoCreate()) 2099 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.variantPointer"); 2100 else if (Configuration.doAutoCreate()) 2101 this.variantPointerTarget = new Observation(); // aa 2102 return this.variantPointerTarget; 2103 } 2104 2105 /** 2106 * @param value {@link #variantPointer} The actual object that is the target of 2107 * the reference. The reference library doesn't use these, but you 2108 * can use it to hold the resource if you resolve it. (A pointer to 2109 * an Observation containing variant information.) 2110 */ 2111 public MolecularSequenceVariantComponent setVariantPointerTarget(Observation value) { 2112 this.variantPointerTarget = value; 2113 return this; 2114 } 2115 2116 protected void listChildren(List<Property> children) { 2117 super.listChildren(children); 2118 children.add(new Property("start", "integer", 2119 "Start position of the variant on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 2120 0, 1, start)); 2121 children.add(new Property("end", "integer", 2122 "End position of the variant on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 2123 0, 1, end)); 2124 children.add(new Property("observedAllele", "string", 2125 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2126 0, 1, observedAllele)); 2127 children.add(new Property("referenceAllele", "string", 2128 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2129 0, 1, referenceAllele)); 2130 children.add(new Property("cigar", "string", 2131 "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 2132 0, 1, cigar)); 2133 children.add(new Property("variantPointer", "Reference(Observation)", 2134 "A pointer to an Observation containing variant information.", 0, 1, variantPointer)); 2135 } 2136 2137 @Override 2138 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2139 switch (_hash) { 2140 case 109757538: 2141 /* start */ return new Property("start", "integer", 2142 "Start position of the variant on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 2143 0, 1, start); 2144 case 100571: 2145 /* end */ return new Property("end", "integer", 2146 "End position of the variant on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 2147 0, 1, end); 2148 case -1418745787: 2149 /* observedAllele */ return new Property("observedAllele", "string", 2150 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2151 0, 1, observedAllele); 2152 case 364045960: 2153 /* referenceAllele */ return new Property("referenceAllele", "string", 2154 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2155 0, 1, referenceAllele); 2156 case 94658738: 2157 /* cigar */ return new Property("cigar", "string", 2158 "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 2159 0, 1, cigar); 2160 case -1654319624: 2161 /* variantPointer */ return new Property("variantPointer", "Reference(Observation)", 2162 "A pointer to an Observation containing variant information.", 0, 1, variantPointer); 2163 default: 2164 return super.getNamedProperty(_hash, _name, _checkValid); 2165 } 2166 2167 } 2168 2169 @Override 2170 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2171 switch (hash) { 2172 case 109757538: 2173 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 2174 case 100571: 2175 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 2176 case -1418745787: 2177 /* observedAllele */ return this.observedAllele == null ? new Base[0] : new Base[] { this.observedAllele }; // StringType 2178 case 364045960: 2179 /* referenceAllele */ return this.referenceAllele == null ? new Base[0] : new Base[] { this.referenceAllele }; // StringType 2180 case 94658738: 2181 /* cigar */ return this.cigar == null ? new Base[0] : new Base[] { this.cigar }; // StringType 2182 case -1654319624: 2183 /* variantPointer */ return this.variantPointer == null ? new Base[0] : new Base[] { this.variantPointer }; // Reference 2184 default: 2185 return super.getProperty(hash, name, checkValid); 2186 } 2187 2188 } 2189 2190 @Override 2191 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2192 switch (hash) { 2193 case 109757538: // start 2194 this.start = castToInteger(value); // IntegerType 2195 return value; 2196 case 100571: // end 2197 this.end = castToInteger(value); // IntegerType 2198 return value; 2199 case -1418745787: // observedAllele 2200 this.observedAllele = castToString(value); // StringType 2201 return value; 2202 case 364045960: // referenceAllele 2203 this.referenceAllele = castToString(value); // StringType 2204 return value; 2205 case 94658738: // cigar 2206 this.cigar = castToString(value); // StringType 2207 return value; 2208 case -1654319624: // variantPointer 2209 this.variantPointer = castToReference(value); // Reference 2210 return value; 2211 default: 2212 return super.setProperty(hash, name, value); 2213 } 2214 2215 } 2216 2217 @Override 2218 public Base setProperty(String name, Base value) throws FHIRException { 2219 if (name.equals("start")) { 2220 this.start = castToInteger(value); // IntegerType 2221 } else if (name.equals("end")) { 2222 this.end = castToInteger(value); // IntegerType 2223 } else if (name.equals("observedAllele")) { 2224 this.observedAllele = castToString(value); // StringType 2225 } else if (name.equals("referenceAllele")) { 2226 this.referenceAllele = castToString(value); // StringType 2227 } else if (name.equals("cigar")) { 2228 this.cigar = castToString(value); // StringType 2229 } else if (name.equals("variantPointer")) { 2230 this.variantPointer = castToReference(value); // Reference 2231 } else 2232 return super.setProperty(name, value); 2233 return value; 2234 } 2235 2236 @Override 2237 public Base makeProperty(int hash, String name) throws FHIRException { 2238 switch (hash) { 2239 case 109757538: 2240 return getStartElement(); 2241 case 100571: 2242 return getEndElement(); 2243 case -1418745787: 2244 return getObservedAlleleElement(); 2245 case 364045960: 2246 return getReferenceAlleleElement(); 2247 case 94658738: 2248 return getCigarElement(); 2249 case -1654319624: 2250 return getVariantPointer(); 2251 default: 2252 return super.makeProperty(hash, name); 2253 } 2254 2255 } 2256 2257 @Override 2258 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2259 switch (hash) { 2260 case 109757538: 2261 /* start */ return new String[] { "integer" }; 2262 case 100571: 2263 /* end */ return new String[] { "integer" }; 2264 case -1418745787: 2265 /* observedAllele */ return new String[] { "string" }; 2266 case 364045960: 2267 /* referenceAllele */ return new String[] { "string" }; 2268 case 94658738: 2269 /* cigar */ return new String[] { "string" }; 2270 case -1654319624: 2271 /* variantPointer */ return new String[] { "Reference" }; 2272 default: 2273 return super.getTypesForProperty(hash, name); 2274 } 2275 2276 } 2277 2278 @Override 2279 public Base addChild(String name) throws FHIRException { 2280 if (name.equals("start")) { 2281 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 2282 } else if (name.equals("end")) { 2283 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 2284 } else if (name.equals("observedAllele")) { 2285 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.observedAllele"); 2286 } else if (name.equals("referenceAllele")) { 2287 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.referenceAllele"); 2288 } else if (name.equals("cigar")) { 2289 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.cigar"); 2290 } else if (name.equals("variantPointer")) { 2291 this.variantPointer = new Reference(); 2292 return this.variantPointer; 2293 } else 2294 return super.addChild(name); 2295 } 2296 2297 public MolecularSequenceVariantComponent copy() { 2298 MolecularSequenceVariantComponent dst = new MolecularSequenceVariantComponent(); 2299 copyValues(dst); 2300 return dst; 2301 } 2302 2303 public void copyValues(MolecularSequenceVariantComponent dst) { 2304 super.copyValues(dst); 2305 dst.start = start == null ? null : start.copy(); 2306 dst.end = end == null ? null : end.copy(); 2307 dst.observedAllele = observedAllele == null ? null : observedAllele.copy(); 2308 dst.referenceAllele = referenceAllele == null ? null : referenceAllele.copy(); 2309 dst.cigar = cigar == null ? null : cigar.copy(); 2310 dst.variantPointer = variantPointer == null ? null : variantPointer.copy(); 2311 } 2312 2313 @Override 2314 public boolean equalsDeep(Base other_) { 2315 if (!super.equalsDeep(other_)) 2316 return false; 2317 if (!(other_ instanceof MolecularSequenceVariantComponent)) 2318 return false; 2319 MolecularSequenceVariantComponent o = (MolecularSequenceVariantComponent) other_; 2320 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 2321 && compareDeep(observedAllele, o.observedAllele, true) 2322 && compareDeep(referenceAllele, o.referenceAllele, true) && compareDeep(cigar, o.cigar, true) 2323 && compareDeep(variantPointer, o.variantPointer, true); 2324 } 2325 2326 @Override 2327 public boolean equalsShallow(Base other_) { 2328 if (!super.equalsShallow(other_)) 2329 return false; 2330 if (!(other_ instanceof MolecularSequenceVariantComponent)) 2331 return false; 2332 MolecularSequenceVariantComponent o = (MolecularSequenceVariantComponent) other_; 2333 return compareValues(start, o.start, true) && compareValues(end, o.end, true) 2334 && compareValues(observedAllele, o.observedAllele, true) 2335 && compareValues(referenceAllele, o.referenceAllele, true) && compareValues(cigar, o.cigar, true); 2336 } 2337 2338 public boolean isEmpty() { 2339 return super.isEmpty() 2340 && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end, observedAllele, referenceAllele, cigar, variantPointer); 2341 } 2342 2343 public String fhirType() { 2344 return "MolecularSequence.variant"; 2345 2346 } 2347 2348 } 2349 2350 @Block() 2351 public static class MolecularSequenceQualityComponent extends BackboneElement implements IBaseBackboneElement { 2352 /** 2353 * INDEL / SNP / Undefined variant. 2354 */ 2355 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2356 @Description(shortDefinition = "indel | snp | unknown", formalDefinition = "INDEL / SNP / Undefined variant.") 2357 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/quality-type") 2358 protected Enumeration<QualityType> type; 2359 2360 /** 2361 * Gold standard sequence used for comparing against. 2362 */ 2363 @Child(name = "standardSequence", type = { 2364 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2365 @Description(shortDefinition = "Standard sequence for comparison", formalDefinition = "Gold standard sequence used for comparing against.") 2366 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-quality-standardSequence") 2367 protected CodeableConcept standardSequence; 2368 2369 /** 2370 * Start position of the sequence. If the coordinate system is either 0-based or 2371 * 1-based, then start position is inclusive. 2372 */ 2373 @Child(name = "start", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2374 @Description(shortDefinition = "Start position of the sequence", formalDefinition = "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 2375 protected IntegerType start; 2376 2377 /** 2378 * End position of the sequence. If the coordinate system is 0-based then end is 2379 * exclusive and does not include the last position. If the coordinate system is 2380 * 1-base, then end is inclusive and includes the last position. 2381 */ 2382 @Child(name = "end", type = { IntegerType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2383 @Description(shortDefinition = "End position of the sequence", formalDefinition = "End position of the sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 2384 protected IntegerType end; 2385 2386 /** 2387 * The score of an experimentally derived feature such as a p-value 2388 * ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)). 2389 */ 2390 @Child(name = "score", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2391 @Description(shortDefinition = "Quality score for the comparison", formalDefinition = "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).") 2392 protected Quantity score; 2393 2394 /** 2395 * Which method is used to get sequence quality. 2396 */ 2397 @Child(name = "method", type = { 2398 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2399 @Description(shortDefinition = "Method to get quality", formalDefinition = "Which method is used to get sequence quality.") 2400 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-quality-method") 2401 protected CodeableConcept method; 2402 2403 /** 2404 * True positives, from the perspective of the truth data, i.e. the number of 2405 * sites in the Truth Call Set for which there are paths through the Query Call 2406 * Set that are consistent with all of the alleles at this site, and for which 2407 * there is an accurate genotype call for the event. 2408 */ 2409 @Child(name = "truthTP", type = { 2410 DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2411 @Description(shortDefinition = "True positives from the perspective of the truth data", formalDefinition = "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.") 2412 protected DecimalType truthTP; 2413 2414 /** 2415 * True positives, from the perspective of the query data, i.e. the number of 2416 * sites in the Query Call Set for which there are paths through the Truth Call 2417 * Set that are consistent with all of the alleles at this site, and for which 2418 * there is an accurate genotype call for the event. 2419 */ 2420 @Child(name = "queryTP", type = { 2421 DecimalType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2422 @Description(shortDefinition = "True positives from the perspective of the query data", formalDefinition = "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.") 2423 protected DecimalType queryTP; 2424 2425 /** 2426 * False negatives, i.e. the number of sites in the Truth Call Set for which 2427 * there is no path through the Query Call Set that is consistent with all of 2428 * the alleles at this site, or sites for which there is an inaccurate genotype 2429 * call for the event. Sites with correct variant but incorrect genotype are 2430 * counted here. 2431 */ 2432 @Child(name = "truthFN", type = { 2433 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2434 @Description(shortDefinition = "False negatives", formalDefinition = "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.") 2435 protected DecimalType truthFN; 2436 2437 /** 2438 * False positives, i.e. the number of sites in the Query Call Set for which 2439 * there is no path through the Truth Call Set that is consistent with this 2440 * site. Sites with correct variant but incorrect genotype are counted here. 2441 */ 2442 @Child(name = "queryFP", type = { 2443 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2444 @Description(shortDefinition = "False positives", formalDefinition = "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.") 2445 protected DecimalType queryFP; 2446 2447 /** 2448 * The number of false positives where the non-REF alleles in the Truth and 2449 * Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 2450 * or similar). 2451 */ 2452 @Child(name = "gtFP", type = { DecimalType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2453 @Description(shortDefinition = "False positives where the non-REF alleles in the Truth and Query Call Sets match", formalDefinition = "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).") 2454 protected DecimalType gtFP; 2455 2456 /** 2457 * QUERY.TP / (QUERY.TP + QUERY.FP). 2458 */ 2459 @Child(name = "precision", type = { 2460 DecimalType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2461 @Description(shortDefinition = "Precision of comparison", formalDefinition = "QUERY.TP / (QUERY.TP + QUERY.FP).") 2462 protected DecimalType precision; 2463 2464 /** 2465 * TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2466 */ 2467 @Child(name = "recall", type = { 2468 DecimalType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 2469 @Description(shortDefinition = "Recall of comparison", formalDefinition = "TRUTH.TP / (TRUTH.TP + TRUTH.FN).") 2470 protected DecimalType recall; 2471 2472 /** 2473 * Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / 2474 * (precision + recall). 2475 */ 2476 @Child(name = "fScore", type = { 2477 DecimalType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 2478 @Description(shortDefinition = "F-score", formalDefinition = "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).") 2479 protected DecimalType fScore; 2480 2481 /** 2482 * Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity 2483 * tradeoff. 2484 */ 2485 @Child(name = "roc", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 2486 @Description(shortDefinition = "Receiver Operator Characteristic (ROC) Curve", formalDefinition = "Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity tradeoff.") 2487 protected MolecularSequenceQualityRocComponent roc; 2488 2489 private static final long serialVersionUID = -811933526L; 2490 2491 /** 2492 * Constructor 2493 */ 2494 public MolecularSequenceQualityComponent() { 2495 super(); 2496 } 2497 2498 /** 2499 * Constructor 2500 */ 2501 public MolecularSequenceQualityComponent(Enumeration<QualityType> type) { 2502 super(); 2503 this.type = type; 2504 } 2505 2506 /** 2507 * @return {@link #type} (INDEL / SNP / Undefined variant.). This is the 2508 * underlying object with id, value and extensions. The accessor 2509 * "getType" gives direct access to the value 2510 */ 2511 public Enumeration<QualityType> getTypeElement() { 2512 if (this.type == null) 2513 if (Configuration.errorOnAutoCreate()) 2514 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.type"); 2515 else if (Configuration.doAutoCreate()) 2516 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); // bb 2517 return this.type; 2518 } 2519 2520 public boolean hasTypeElement() { 2521 return this.type != null && !this.type.isEmpty(); 2522 } 2523 2524 public boolean hasType() { 2525 return this.type != null && !this.type.isEmpty(); 2526 } 2527 2528 /** 2529 * @param value {@link #type} (INDEL / SNP / Undefined variant.). This is the 2530 * underlying object with id, value and extensions. The accessor 2531 * "getType" gives direct access to the value 2532 */ 2533 public MolecularSequenceQualityComponent setTypeElement(Enumeration<QualityType> value) { 2534 this.type = value; 2535 return this; 2536 } 2537 2538 /** 2539 * @return INDEL / SNP / Undefined variant. 2540 */ 2541 public QualityType getType() { 2542 return this.type == null ? null : this.type.getValue(); 2543 } 2544 2545 /** 2546 * @param value INDEL / SNP / Undefined variant. 2547 */ 2548 public MolecularSequenceQualityComponent setType(QualityType value) { 2549 if (this.type == null) 2550 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); 2551 this.type.setValue(value); 2552 return this; 2553 } 2554 2555 /** 2556 * @return {@link #standardSequence} (Gold standard sequence used for comparing 2557 * against.) 2558 */ 2559 public CodeableConcept getStandardSequence() { 2560 if (this.standardSequence == null) 2561 if (Configuration.errorOnAutoCreate()) 2562 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.standardSequence"); 2563 else if (Configuration.doAutoCreate()) 2564 this.standardSequence = new CodeableConcept(); // cc 2565 return this.standardSequence; 2566 } 2567 2568 public boolean hasStandardSequence() { 2569 return this.standardSequence != null && !this.standardSequence.isEmpty(); 2570 } 2571 2572 /** 2573 * @param value {@link #standardSequence} (Gold standard sequence used for 2574 * comparing against.) 2575 */ 2576 public MolecularSequenceQualityComponent setStandardSequence(CodeableConcept value) { 2577 this.standardSequence = value; 2578 return this; 2579 } 2580 2581 /** 2582 * @return {@link #start} (Start position of the sequence. If the coordinate 2583 * system is either 0-based or 1-based, then start position is 2584 * inclusive.). This is the underlying object with id, value and 2585 * extensions. The accessor "getStart" gives direct access to the value 2586 */ 2587 public IntegerType getStartElement() { 2588 if (this.start == null) 2589 if (Configuration.errorOnAutoCreate()) 2590 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.start"); 2591 else if (Configuration.doAutoCreate()) 2592 this.start = new IntegerType(); // bb 2593 return this.start; 2594 } 2595 2596 public boolean hasStartElement() { 2597 return this.start != null && !this.start.isEmpty(); 2598 } 2599 2600 public boolean hasStart() { 2601 return this.start != null && !this.start.isEmpty(); 2602 } 2603 2604 /** 2605 * @param value {@link #start} (Start position of the sequence. If the 2606 * coordinate system is either 0-based or 1-based, then start 2607 * position is inclusive.). This is the underlying object with id, 2608 * value and extensions. The accessor "getStart" gives direct 2609 * access to the value 2610 */ 2611 public MolecularSequenceQualityComponent setStartElement(IntegerType value) { 2612 this.start = value; 2613 return this; 2614 } 2615 2616 /** 2617 * @return Start position of the sequence. If the coordinate system is either 2618 * 0-based or 1-based, then start position is inclusive. 2619 */ 2620 public int getStart() { 2621 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 2622 } 2623 2624 /** 2625 * @param value Start position of the sequence. If the coordinate system is 2626 * either 0-based or 1-based, then start position is inclusive. 2627 */ 2628 public MolecularSequenceQualityComponent setStart(int value) { 2629 if (this.start == null) 2630 this.start = new IntegerType(); 2631 this.start.setValue(value); 2632 return this; 2633 } 2634 2635 /** 2636 * @return {@link #end} (End position of the sequence. If the coordinate system 2637 * is 0-based then end is exclusive and does not include the last 2638 * position. If the coordinate system is 1-base, then end is inclusive 2639 * and includes the last position.). This is the underlying object with 2640 * id, value and extensions. The accessor "getEnd" gives direct access 2641 * to the value 2642 */ 2643 public IntegerType getEndElement() { 2644 if (this.end == null) 2645 if (Configuration.errorOnAutoCreate()) 2646 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.end"); 2647 else if (Configuration.doAutoCreate()) 2648 this.end = new IntegerType(); // bb 2649 return this.end; 2650 } 2651 2652 public boolean hasEndElement() { 2653 return this.end != null && !this.end.isEmpty(); 2654 } 2655 2656 public boolean hasEnd() { 2657 return this.end != null && !this.end.isEmpty(); 2658 } 2659 2660 /** 2661 * @param value {@link #end} (End position of the sequence. If the coordinate 2662 * system is 0-based then end is exclusive and does not include the 2663 * last position. If the coordinate system is 1-base, then end is 2664 * inclusive and includes the last position.). This is the 2665 * underlying object with id, value and extensions. The accessor 2666 * "getEnd" gives direct access to the value 2667 */ 2668 public MolecularSequenceQualityComponent setEndElement(IntegerType value) { 2669 this.end = value; 2670 return this; 2671 } 2672 2673 /** 2674 * @return End position of the sequence. If the coordinate system is 0-based 2675 * then end is exclusive and does not include the last position. If the 2676 * coordinate system is 1-base, then end is inclusive and includes the 2677 * last position. 2678 */ 2679 public int getEnd() { 2680 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 2681 } 2682 2683 /** 2684 * @param value End position of the sequence. If the coordinate system is 2685 * 0-based then end is exclusive and does not include the last 2686 * position. If the coordinate system is 1-base, then end is 2687 * inclusive and includes the last position. 2688 */ 2689 public MolecularSequenceQualityComponent setEnd(int value) { 2690 if (this.end == null) 2691 this.end = new IntegerType(); 2692 this.end.setValue(value); 2693 return this; 2694 } 2695 2696 /** 2697 * @return {@link #score} (The score of an experimentally derived feature such 2698 * as a p-value 2699 * ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 2700 */ 2701 public Quantity getScore() { 2702 if (this.score == null) 2703 if (Configuration.errorOnAutoCreate()) 2704 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.score"); 2705 else if (Configuration.doAutoCreate()) 2706 this.score = new Quantity(); // cc 2707 return this.score; 2708 } 2709 2710 public boolean hasScore() { 2711 return this.score != null && !this.score.isEmpty(); 2712 } 2713 2714 /** 2715 * @param value {@link #score} (The score of an experimentally derived feature 2716 * such as a p-value 2717 * ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 2718 */ 2719 public MolecularSequenceQualityComponent setScore(Quantity value) { 2720 this.score = value; 2721 return this; 2722 } 2723 2724 /** 2725 * @return {@link #method} (Which method is used to get sequence quality.) 2726 */ 2727 public CodeableConcept getMethod() { 2728 if (this.method == null) 2729 if (Configuration.errorOnAutoCreate()) 2730 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.method"); 2731 else if (Configuration.doAutoCreate()) 2732 this.method = new CodeableConcept(); // cc 2733 return this.method; 2734 } 2735 2736 public boolean hasMethod() { 2737 return this.method != null && !this.method.isEmpty(); 2738 } 2739 2740 /** 2741 * @param value {@link #method} (Which method is used to get sequence quality.) 2742 */ 2743 public MolecularSequenceQualityComponent setMethod(CodeableConcept value) { 2744 this.method = value; 2745 return this; 2746 } 2747 2748 /** 2749 * @return {@link #truthTP} (True positives, from the perspective of the truth 2750 * data, i.e. the number of sites in the Truth Call Set for which there 2751 * are paths through the Query Call Set that are consistent with all of 2752 * the alleles at this site, and for which there is an accurate genotype 2753 * call for the event.). This is the underlying object with id, value 2754 * and extensions. The accessor "getTruthTP" gives direct access to the 2755 * value 2756 */ 2757 public DecimalType getTruthTPElement() { 2758 if (this.truthTP == null) 2759 if (Configuration.errorOnAutoCreate()) 2760 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.truthTP"); 2761 else if (Configuration.doAutoCreate()) 2762 this.truthTP = new DecimalType(); // bb 2763 return this.truthTP; 2764 } 2765 2766 public boolean hasTruthTPElement() { 2767 return this.truthTP != null && !this.truthTP.isEmpty(); 2768 } 2769 2770 public boolean hasTruthTP() { 2771 return this.truthTP != null && !this.truthTP.isEmpty(); 2772 } 2773 2774 /** 2775 * @param value {@link #truthTP} (True positives, from the perspective of the 2776 * truth data, i.e. the number of sites in the Truth Call Set for 2777 * which there are paths through the Query Call Set that are 2778 * consistent with all of the alleles at this site, and for which 2779 * there is an accurate genotype call for the event.). This is the 2780 * underlying object with id, value and extensions. The accessor 2781 * "getTruthTP" gives direct access to the value 2782 */ 2783 public MolecularSequenceQualityComponent setTruthTPElement(DecimalType value) { 2784 this.truthTP = value; 2785 return this; 2786 } 2787 2788 /** 2789 * @return True positives, from the perspective of the truth data, i.e. the 2790 * number of sites in the Truth Call Set for which there are paths 2791 * through the Query Call Set that are consistent with all of the 2792 * alleles at this site, and for which there is an accurate genotype 2793 * call for the event. 2794 */ 2795 public BigDecimal getTruthTP() { 2796 return this.truthTP == null ? null : this.truthTP.getValue(); 2797 } 2798 2799 /** 2800 * @param value True positives, from the perspective of the truth data, i.e. the 2801 * number of sites in the Truth Call Set for which there are paths 2802 * through the Query Call Set that are consistent with all of the 2803 * alleles at this site, and for which there is an accurate 2804 * genotype call for the event. 2805 */ 2806 public MolecularSequenceQualityComponent setTruthTP(BigDecimal value) { 2807 if (value == null) 2808 this.truthTP = null; 2809 else { 2810 if (this.truthTP == null) 2811 this.truthTP = new DecimalType(); 2812 this.truthTP.setValue(value); 2813 } 2814 return this; 2815 } 2816 2817 /** 2818 * @param value True positives, from the perspective of the truth data, i.e. the 2819 * number of sites in the Truth Call Set for which there are paths 2820 * through the Query Call Set that are consistent with all of the 2821 * alleles at this site, and for which there is an accurate 2822 * genotype call for the event. 2823 */ 2824 public MolecularSequenceQualityComponent setTruthTP(long value) { 2825 this.truthTP = new DecimalType(); 2826 this.truthTP.setValue(value); 2827 return this; 2828 } 2829 2830 /** 2831 * @param value True positives, from the perspective of the truth data, i.e. the 2832 * number of sites in the Truth Call Set for which there are paths 2833 * through the Query Call Set that are consistent with all of the 2834 * alleles at this site, and for which there is an accurate 2835 * genotype call for the event. 2836 */ 2837 public MolecularSequenceQualityComponent setTruthTP(double value) { 2838 this.truthTP = new DecimalType(); 2839 this.truthTP.setValue(value); 2840 return this; 2841 } 2842 2843 /** 2844 * @return {@link #queryTP} (True positives, from the perspective of the query 2845 * data, i.e. the number of sites in the Query Call Set for which there 2846 * are paths through the Truth Call Set that are consistent with all of 2847 * the alleles at this site, and for which there is an accurate genotype 2848 * call for the event.). This is the underlying object with id, value 2849 * and extensions. The accessor "getQueryTP" gives direct access to the 2850 * value 2851 */ 2852 public DecimalType getQueryTPElement() { 2853 if (this.queryTP == null) 2854 if (Configuration.errorOnAutoCreate()) 2855 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.queryTP"); 2856 else if (Configuration.doAutoCreate()) 2857 this.queryTP = new DecimalType(); // bb 2858 return this.queryTP; 2859 } 2860 2861 public boolean hasQueryTPElement() { 2862 return this.queryTP != null && !this.queryTP.isEmpty(); 2863 } 2864 2865 public boolean hasQueryTP() { 2866 return this.queryTP != null && !this.queryTP.isEmpty(); 2867 } 2868 2869 /** 2870 * @param value {@link #queryTP} (True positives, from the perspective of the 2871 * query data, i.e. the number of sites in the Query Call Set for 2872 * which there are paths through the Truth Call Set that are 2873 * consistent with all of the alleles at this site, and for which 2874 * there is an accurate genotype call for the event.). This is the 2875 * underlying object with id, value and extensions. The accessor 2876 * "getQueryTP" gives direct access to the value 2877 */ 2878 public MolecularSequenceQualityComponent setQueryTPElement(DecimalType value) { 2879 this.queryTP = value; 2880 return this; 2881 } 2882 2883 /** 2884 * @return True positives, from the perspective of the query data, i.e. the 2885 * number of sites in the Query Call Set for which there are paths 2886 * through the Truth Call Set that are consistent with all of the 2887 * alleles at this site, and for which there is an accurate genotype 2888 * call for the event. 2889 */ 2890 public BigDecimal getQueryTP() { 2891 return this.queryTP == null ? null : this.queryTP.getValue(); 2892 } 2893 2894 /** 2895 * @param value True positives, from the perspective of the query data, i.e. the 2896 * number of sites in the Query Call Set for which there are paths 2897 * through the Truth Call Set that are consistent with all of the 2898 * alleles at this site, and for which there is an accurate 2899 * genotype call for the event. 2900 */ 2901 public MolecularSequenceQualityComponent setQueryTP(BigDecimal value) { 2902 if (value == null) 2903 this.queryTP = null; 2904 else { 2905 if (this.queryTP == null) 2906 this.queryTP = new DecimalType(); 2907 this.queryTP.setValue(value); 2908 } 2909 return this; 2910 } 2911 2912 /** 2913 * @param value True positives, from the perspective of the query data, i.e. the 2914 * number of sites in the Query Call Set for which there are paths 2915 * through the Truth Call Set that are consistent with all of the 2916 * alleles at this site, and for which there is an accurate 2917 * genotype call for the event. 2918 */ 2919 public MolecularSequenceQualityComponent setQueryTP(long value) { 2920 this.queryTP = new DecimalType(); 2921 this.queryTP.setValue(value); 2922 return this; 2923 } 2924 2925 /** 2926 * @param value True positives, from the perspective of the query data, i.e. the 2927 * number of sites in the Query Call Set for which there are paths 2928 * through the Truth Call Set that are consistent with all of the 2929 * alleles at this site, and for which there is an accurate 2930 * genotype call for the event. 2931 */ 2932 public MolecularSequenceQualityComponent setQueryTP(double value) { 2933 this.queryTP = new DecimalType(); 2934 this.queryTP.setValue(value); 2935 return this; 2936 } 2937 2938 /** 2939 * @return {@link #truthFN} (False negatives, i.e. the number of sites in the 2940 * Truth Call Set for which there is no path through the Query Call Set 2941 * that is consistent with all of the alleles at this site, or sites for 2942 * which there is an inaccurate genotype call for the event. Sites with 2943 * correct variant but incorrect genotype are counted here.). This is 2944 * the underlying object with id, value and extensions. The accessor 2945 * "getTruthFN" gives direct access to the value 2946 */ 2947 public DecimalType getTruthFNElement() { 2948 if (this.truthFN == null) 2949 if (Configuration.errorOnAutoCreate()) 2950 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.truthFN"); 2951 else if (Configuration.doAutoCreate()) 2952 this.truthFN = new DecimalType(); // bb 2953 return this.truthFN; 2954 } 2955 2956 public boolean hasTruthFNElement() { 2957 return this.truthFN != null && !this.truthFN.isEmpty(); 2958 } 2959 2960 public boolean hasTruthFN() { 2961 return this.truthFN != null && !this.truthFN.isEmpty(); 2962 } 2963 2964 /** 2965 * @param value {@link #truthFN} (False negatives, i.e. the number of sites in 2966 * the Truth Call Set for which there is no path through the Query 2967 * Call Set that is consistent with all of the alleles at this 2968 * site, or sites for which there is an inaccurate genotype call 2969 * for the event. Sites with correct variant but incorrect genotype 2970 * are counted here.). This is the underlying object with id, value 2971 * and extensions. The accessor "getTruthFN" gives direct access to 2972 * the value 2973 */ 2974 public MolecularSequenceQualityComponent setTruthFNElement(DecimalType value) { 2975 this.truthFN = value; 2976 return this; 2977 } 2978 2979 /** 2980 * @return False negatives, i.e. the number of sites in the Truth Call Set for 2981 * which there is no path through the Query Call Set that is consistent 2982 * with all of the alleles at this site, or sites for which there is an 2983 * inaccurate genotype call for the event. Sites with correct variant 2984 * but incorrect genotype are counted here. 2985 */ 2986 public BigDecimal getTruthFN() { 2987 return this.truthFN == null ? null : this.truthFN.getValue(); 2988 } 2989 2990 /** 2991 * @param value False negatives, i.e. the number of sites in the Truth Call Set 2992 * for which there is no path through the Query Call Set that is 2993 * consistent with all of the alleles at this site, or sites for 2994 * which there is an inaccurate genotype call for the event. Sites 2995 * with correct variant but incorrect genotype are counted here. 2996 */ 2997 public MolecularSequenceQualityComponent setTruthFN(BigDecimal value) { 2998 if (value == null) 2999 this.truthFN = null; 3000 else { 3001 if (this.truthFN == null) 3002 this.truthFN = new DecimalType(); 3003 this.truthFN.setValue(value); 3004 } 3005 return this; 3006 } 3007 3008 /** 3009 * @param value False negatives, i.e. the number of sites in the Truth Call Set 3010 * for which there is no path through the Query Call Set that is 3011 * consistent with all of the alleles at this site, or sites for 3012 * which there is an inaccurate genotype call for the event. Sites 3013 * with correct variant but incorrect genotype are counted here. 3014 */ 3015 public MolecularSequenceQualityComponent setTruthFN(long value) { 3016 this.truthFN = new DecimalType(); 3017 this.truthFN.setValue(value); 3018 return this; 3019 } 3020 3021 /** 3022 * @param value False negatives, i.e. the number of sites in the Truth Call Set 3023 * for which there is no path through the Query Call Set that is 3024 * consistent with all of the alleles at this site, or sites for 3025 * which there is an inaccurate genotype call for the event. Sites 3026 * with correct variant but incorrect genotype are counted here. 3027 */ 3028 public MolecularSequenceQualityComponent setTruthFN(double value) { 3029 this.truthFN = new DecimalType(); 3030 this.truthFN.setValue(value); 3031 return this; 3032 } 3033 3034 /** 3035 * @return {@link #queryFP} (False positives, i.e. the number of sites in the 3036 * Query Call Set for which there is no path through the Truth Call Set 3037 * that is consistent with this site. Sites with correct variant but 3038 * incorrect genotype are counted here.). This is the underlying object 3039 * with id, value and extensions. The accessor "getQueryFP" gives direct 3040 * access to the value 3041 */ 3042 public DecimalType getQueryFPElement() { 3043 if (this.queryFP == null) 3044 if (Configuration.errorOnAutoCreate()) 3045 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.queryFP"); 3046 else if (Configuration.doAutoCreate()) 3047 this.queryFP = new DecimalType(); // bb 3048 return this.queryFP; 3049 } 3050 3051 public boolean hasQueryFPElement() { 3052 return this.queryFP != null && !this.queryFP.isEmpty(); 3053 } 3054 3055 public boolean hasQueryFP() { 3056 return this.queryFP != null && !this.queryFP.isEmpty(); 3057 } 3058 3059 /** 3060 * @param value {@link #queryFP} (False positives, i.e. the number of sites in 3061 * the Query Call Set for which there is no path through the Truth 3062 * Call Set that is consistent with this site. Sites with correct 3063 * variant but incorrect genotype are counted here.). This is the 3064 * underlying object with id, value and extensions. The accessor 3065 * "getQueryFP" gives direct access to the value 3066 */ 3067 public MolecularSequenceQualityComponent setQueryFPElement(DecimalType value) { 3068 this.queryFP = value; 3069 return this; 3070 } 3071 3072 /** 3073 * @return False positives, i.e. the number of sites in the Query Call Set for 3074 * which there is no path through the Truth Call Set that is consistent 3075 * with this site. Sites with correct variant but incorrect genotype are 3076 * counted here. 3077 */ 3078 public BigDecimal getQueryFP() { 3079 return this.queryFP == null ? null : this.queryFP.getValue(); 3080 } 3081 3082 /** 3083 * @param value False positives, i.e. the number of sites in the Query Call Set 3084 * for which there is no path through the Truth Call Set that is 3085 * consistent with this site. Sites with correct variant but 3086 * incorrect genotype are counted here. 3087 */ 3088 public MolecularSequenceQualityComponent setQueryFP(BigDecimal value) { 3089 if (value == null) 3090 this.queryFP = null; 3091 else { 3092 if (this.queryFP == null) 3093 this.queryFP = new DecimalType(); 3094 this.queryFP.setValue(value); 3095 } 3096 return this; 3097 } 3098 3099 /** 3100 * @param value False positives, i.e. the number of sites in the Query Call Set 3101 * for which there is no path through the Truth Call Set that is 3102 * consistent with this site. Sites with correct variant but 3103 * incorrect genotype are counted here. 3104 */ 3105 public MolecularSequenceQualityComponent setQueryFP(long value) { 3106 this.queryFP = new DecimalType(); 3107 this.queryFP.setValue(value); 3108 return this; 3109 } 3110 3111 /** 3112 * @param value False positives, i.e. the number of sites in the Query Call Set 3113 * for which there is no path through the Truth Call Set that is 3114 * consistent with this site. Sites with correct variant but 3115 * incorrect genotype are counted here. 3116 */ 3117 public MolecularSequenceQualityComponent setQueryFP(double value) { 3118 this.queryFP = new DecimalType(); 3119 this.queryFP.setValue(value); 3120 return this; 3121 } 3122 3123 /** 3124 * @return {@link #gtFP} (The number of false positives where the non-REF 3125 * alleles in the Truth and Query Call Sets match (i.e. cases where the 3126 * truth is 1/1 and the query is 0/1 or similar).). This is the 3127 * underlying object with id, value and extensions. The accessor 3128 * "getGtFP" gives direct access to the value 3129 */ 3130 public DecimalType getGtFPElement() { 3131 if (this.gtFP == null) 3132 if (Configuration.errorOnAutoCreate()) 3133 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.gtFP"); 3134 else if (Configuration.doAutoCreate()) 3135 this.gtFP = new DecimalType(); // bb 3136 return this.gtFP; 3137 } 3138 3139 public boolean hasGtFPElement() { 3140 return this.gtFP != null && !this.gtFP.isEmpty(); 3141 } 3142 3143 public boolean hasGtFP() { 3144 return this.gtFP != null && !this.gtFP.isEmpty(); 3145 } 3146 3147 /** 3148 * @param value {@link #gtFP} (The number of false positives where the non-REF 3149 * alleles in the Truth and Query Call Sets match (i.e. cases where 3150 * the truth is 1/1 and the query is 0/1 or similar).). This is the 3151 * underlying object with id, value and extensions. The accessor 3152 * "getGtFP" gives direct access to the value 3153 */ 3154 public MolecularSequenceQualityComponent setGtFPElement(DecimalType value) { 3155 this.gtFP = value; 3156 return this; 3157 } 3158 3159 /** 3160 * @return The number of false positives where the non-REF alleles in the Truth 3161 * and Query Call Sets match (i.e. cases where the truth is 1/1 and the 3162 * query is 0/1 or similar). 3163 */ 3164 public BigDecimal getGtFP() { 3165 return this.gtFP == null ? null : this.gtFP.getValue(); 3166 } 3167 3168 /** 3169 * @param value The number of false positives where the non-REF alleles in the 3170 * Truth and Query Call Sets match (i.e. cases where the truth is 3171 * 1/1 and the query is 0/1 or similar). 3172 */ 3173 public MolecularSequenceQualityComponent setGtFP(BigDecimal value) { 3174 if (value == null) 3175 this.gtFP = null; 3176 else { 3177 if (this.gtFP == null) 3178 this.gtFP = new DecimalType(); 3179 this.gtFP.setValue(value); 3180 } 3181 return this; 3182 } 3183 3184 /** 3185 * @param value The number of false positives where the non-REF alleles in the 3186 * Truth and Query Call Sets match (i.e. cases where the truth is 3187 * 1/1 and the query is 0/1 or similar). 3188 */ 3189 public MolecularSequenceQualityComponent setGtFP(long value) { 3190 this.gtFP = new DecimalType(); 3191 this.gtFP.setValue(value); 3192 return this; 3193 } 3194 3195 /** 3196 * @param value The number of false positives where the non-REF alleles in the 3197 * Truth and Query Call Sets match (i.e. cases where the truth is 3198 * 1/1 and the query is 0/1 or similar). 3199 */ 3200 public MolecularSequenceQualityComponent setGtFP(double value) { 3201 this.gtFP = new DecimalType(); 3202 this.gtFP.setValue(value); 3203 return this; 3204 } 3205 3206 /** 3207 * @return {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is the 3208 * underlying object with id, value and extensions. The accessor 3209 * "getPrecision" gives direct access to the value 3210 */ 3211 public DecimalType getPrecisionElement() { 3212 if (this.precision == null) 3213 if (Configuration.errorOnAutoCreate()) 3214 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.precision"); 3215 else if (Configuration.doAutoCreate()) 3216 this.precision = new DecimalType(); // bb 3217 return this.precision; 3218 } 3219 3220 public boolean hasPrecisionElement() { 3221 return this.precision != null && !this.precision.isEmpty(); 3222 } 3223 3224 public boolean hasPrecision() { 3225 return this.precision != null && !this.precision.isEmpty(); 3226 } 3227 3228 /** 3229 * @param value {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is 3230 * the underlying object with id, value and extensions. The 3231 * accessor "getPrecision" gives direct access to the value 3232 */ 3233 public MolecularSequenceQualityComponent setPrecisionElement(DecimalType value) { 3234 this.precision = value; 3235 return this; 3236 } 3237 3238 /** 3239 * @return QUERY.TP / (QUERY.TP + QUERY.FP). 3240 */ 3241 public BigDecimal getPrecision() { 3242 return this.precision == null ? null : this.precision.getValue(); 3243 } 3244 3245 /** 3246 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 3247 */ 3248 public MolecularSequenceQualityComponent setPrecision(BigDecimal value) { 3249 if (value == null) 3250 this.precision = null; 3251 else { 3252 if (this.precision == null) 3253 this.precision = new DecimalType(); 3254 this.precision.setValue(value); 3255 } 3256 return this; 3257 } 3258 3259 /** 3260 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 3261 */ 3262 public MolecularSequenceQualityComponent setPrecision(long value) { 3263 this.precision = new DecimalType(); 3264 this.precision.setValue(value); 3265 return this; 3266 } 3267 3268 /** 3269 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 3270 */ 3271 public MolecularSequenceQualityComponent setPrecision(double value) { 3272 this.precision = new DecimalType(); 3273 this.precision.setValue(value); 3274 return this; 3275 } 3276 3277 /** 3278 * @return {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the 3279 * underlying object with id, value and extensions. The accessor 3280 * "getRecall" gives direct access to the value 3281 */ 3282 public DecimalType getRecallElement() { 3283 if (this.recall == null) 3284 if (Configuration.errorOnAutoCreate()) 3285 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.recall"); 3286 else if (Configuration.doAutoCreate()) 3287 this.recall = new DecimalType(); // bb 3288 return this.recall; 3289 } 3290 3291 public boolean hasRecallElement() { 3292 return this.recall != null && !this.recall.isEmpty(); 3293 } 3294 3295 public boolean hasRecall() { 3296 return this.recall != null && !this.recall.isEmpty(); 3297 } 3298 3299 /** 3300 * @param value {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the 3301 * underlying object with id, value and extensions. The accessor 3302 * "getRecall" gives direct access to the value 3303 */ 3304 public MolecularSequenceQualityComponent setRecallElement(DecimalType value) { 3305 this.recall = value; 3306 return this; 3307 } 3308 3309 /** 3310 * @return TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3311 */ 3312 public BigDecimal getRecall() { 3313 return this.recall == null ? null : this.recall.getValue(); 3314 } 3315 3316 /** 3317 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3318 */ 3319 public MolecularSequenceQualityComponent setRecall(BigDecimal value) { 3320 if (value == null) 3321 this.recall = null; 3322 else { 3323 if (this.recall == null) 3324 this.recall = new DecimalType(); 3325 this.recall.setValue(value); 3326 } 3327 return this; 3328 } 3329 3330 /** 3331 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3332 */ 3333 public MolecularSequenceQualityComponent setRecall(long value) { 3334 this.recall = new DecimalType(); 3335 this.recall.setValue(value); 3336 return this; 3337 } 3338 3339 /** 3340 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3341 */ 3342 public MolecularSequenceQualityComponent setRecall(double value) { 3343 this.recall = new DecimalType(); 3344 this.recall.setValue(value); 3345 return this; 3346 } 3347 3348 /** 3349 * @return {@link #fScore} (Harmonic mean of Recall and Precision, computed as: 3350 * 2 * precision * recall / (precision + recall).). This is the 3351 * underlying object with id, value and extensions. The accessor 3352 * "getFScore" gives direct access to the value 3353 */ 3354 public DecimalType getFScoreElement() { 3355 if (this.fScore == null) 3356 if (Configuration.errorOnAutoCreate()) 3357 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.fScore"); 3358 else if (Configuration.doAutoCreate()) 3359 this.fScore = new DecimalType(); // bb 3360 return this.fScore; 3361 } 3362 3363 public boolean hasFScoreElement() { 3364 return this.fScore != null && !this.fScore.isEmpty(); 3365 } 3366 3367 public boolean hasFScore() { 3368 return this.fScore != null && !this.fScore.isEmpty(); 3369 } 3370 3371 /** 3372 * @param value {@link #fScore} (Harmonic mean of Recall and Precision, computed 3373 * as: 2 * precision * recall / (precision + recall).). This is the 3374 * underlying object with id, value and extensions. The accessor 3375 * "getFScore" gives direct access to the value 3376 */ 3377 public MolecularSequenceQualityComponent setFScoreElement(DecimalType value) { 3378 this.fScore = value; 3379 return this; 3380 } 3381 3382 /** 3383 * @return Harmonic mean of Recall and Precision, computed as: 2 * precision * 3384 * recall / (precision + recall). 3385 */ 3386 public BigDecimal getFScore() { 3387 return this.fScore == null ? null : this.fScore.getValue(); 3388 } 3389 3390 /** 3391 * @param value Harmonic mean of Recall and Precision, computed as: 2 * 3392 * precision * recall / (precision + recall). 3393 */ 3394 public MolecularSequenceQualityComponent setFScore(BigDecimal value) { 3395 if (value == null) 3396 this.fScore = null; 3397 else { 3398 if (this.fScore == null) 3399 this.fScore = new DecimalType(); 3400 this.fScore.setValue(value); 3401 } 3402 return this; 3403 } 3404 3405 /** 3406 * @param value Harmonic mean of Recall and Precision, computed as: 2 * 3407 * precision * recall / (precision + recall). 3408 */ 3409 public MolecularSequenceQualityComponent setFScore(long value) { 3410 this.fScore = new DecimalType(); 3411 this.fScore.setValue(value); 3412 return this; 3413 } 3414 3415 /** 3416 * @param value Harmonic mean of Recall and Precision, computed as: 2 * 3417 * precision * recall / (precision + recall). 3418 */ 3419 public MolecularSequenceQualityComponent setFScore(double value) { 3420 this.fScore = new DecimalType(); 3421 this.fScore.setValue(value); 3422 return this; 3423 } 3424 3425 /** 3426 * @return {@link #roc} (Receiver Operator Characteristic (ROC) Curve to give 3427 * sensitivity/specificity tradeoff.) 3428 */ 3429 public MolecularSequenceQualityRocComponent getRoc() { 3430 if (this.roc == null) 3431 if (Configuration.errorOnAutoCreate()) 3432 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.roc"); 3433 else if (Configuration.doAutoCreate()) 3434 this.roc = new MolecularSequenceQualityRocComponent(); // cc 3435 return this.roc; 3436 } 3437 3438 public boolean hasRoc() { 3439 return this.roc != null && !this.roc.isEmpty(); 3440 } 3441 3442 /** 3443 * @param value {@link #roc} (Receiver Operator Characteristic (ROC) Curve to 3444 * give sensitivity/specificity tradeoff.) 3445 */ 3446 public MolecularSequenceQualityComponent setRoc(MolecularSequenceQualityRocComponent value) { 3447 this.roc = value; 3448 return this; 3449 } 3450 3451 protected void listChildren(List<Property> children) { 3452 super.listChildren(children); 3453 children.add(new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type)); 3454 children.add(new Property("standardSequence", "CodeableConcept", 3455 "Gold standard sequence used for comparing against.", 0, 1, standardSequence)); 3456 children.add(new Property("start", "integer", 3457 "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 3458 0, 1, start)); 3459 children.add(new Property("end", "integer", 3460 "End position of the sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 3461 0, 1, end)); 3462 children.add(new Property("score", "Quantity", 3463 "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 3464 0, 1, score)); 3465 children.add( 3466 new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 0, 1, method)); 3467 children.add(new Property("truthTP", "decimal", 3468 "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3469 0, 1, truthTP)); 3470 children.add(new Property("queryTP", "decimal", 3471 "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3472 0, 1, queryTP)); 3473 children.add(new Property("truthFN", "decimal", 3474 "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 3475 0, 1, truthFN)); 3476 children.add(new Property("queryFP", "decimal", 3477 "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 3478 0, 1, queryFP)); 3479 children.add(new Property("gtFP", "decimal", 3480 "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 3481 0, 1, gtFP)); 3482 children.add(new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, precision)); 3483 children.add(new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall)); 3484 children.add(new Property("fScore", "decimal", 3485 "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, 3486 fScore)); 3487 children.add(new Property("roc", "", 3488 "Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity tradeoff.", 0, 1, roc)); 3489 } 3490 3491 @Override 3492 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3493 switch (_hash) { 3494 case 3575610: 3495 /* type */ return new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type); 3496 case -1861227106: 3497 /* standardSequence */ return new Property("standardSequence", "CodeableConcept", 3498 "Gold standard sequence used for comparing against.", 0, 1, standardSequence); 3499 case 109757538: 3500 /* start */ return new Property("start", "integer", 3501 "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 3502 0, 1, start); 3503 case 100571: 3504 /* end */ return new Property("end", "integer", 3505 "End position of the sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 3506 0, 1, end); 3507 case 109264530: 3508 /* score */ return new Property("score", "Quantity", 3509 "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 3510 0, 1, score); 3511 case -1077554975: 3512 /* method */ return new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 3513 0, 1, method); 3514 case -1048421849: 3515 /* truthTP */ return new Property("truthTP", "decimal", 3516 "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3517 0, 1, truthTP); 3518 case 655102276: 3519 /* queryTP */ return new Property("queryTP", "decimal", 3520 "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3521 0, 1, queryTP); 3522 case -1048422285: 3523 /* truthFN */ return new Property("truthFN", "decimal", 3524 "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 3525 0, 1, truthFN); 3526 case 655101842: 3527 /* queryFP */ return new Property("queryFP", "decimal", 3528 "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 3529 0, 1, queryFP); 3530 case 3182199: 3531 /* gtFP */ return new Property("gtFP", "decimal", 3532 "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 3533 0, 1, gtFP); 3534 case -1376177026: 3535 /* precision */ return new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, 3536 precision); 3537 case -934922479: 3538 /* recall */ return new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall); 3539 case -1295082036: 3540 /* fScore */ return new Property("fScore", "decimal", 3541 "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, 3542 fScore); 3543 case 113094: 3544 /* roc */ return new Property("roc", "", 3545 "Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity tradeoff.", 0, 1, roc); 3546 default: 3547 return super.getNamedProperty(_hash, _name, _checkValid); 3548 } 3549 3550 } 3551 3552 @Override 3553 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3554 switch (hash) { 3555 case 3575610: 3556 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<QualityType> 3557 case -1861227106: 3558 /* standardSequence */ return this.standardSequence == null ? new Base[0] 3559 : new Base[] { this.standardSequence }; // CodeableConcept 3560 case 109757538: 3561 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 3562 case 100571: 3563 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 3564 case 109264530: 3565 /* score */ return this.score == null ? new Base[0] : new Base[] { this.score }; // Quantity 3566 case -1077554975: 3567 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 3568 case -1048421849: 3569 /* truthTP */ return this.truthTP == null ? new Base[0] : new Base[] { this.truthTP }; // DecimalType 3570 case 655102276: 3571 /* queryTP */ return this.queryTP == null ? new Base[0] : new Base[] { this.queryTP }; // DecimalType 3572 case -1048422285: 3573 /* truthFN */ return this.truthFN == null ? new Base[0] : new Base[] { this.truthFN }; // DecimalType 3574 case 655101842: 3575 /* queryFP */ return this.queryFP == null ? new Base[0] : new Base[] { this.queryFP }; // DecimalType 3576 case 3182199: 3577 /* gtFP */ return this.gtFP == null ? new Base[0] : new Base[] { this.gtFP }; // DecimalType 3578 case -1376177026: 3579 /* precision */ return this.precision == null ? new Base[0] : new Base[] { this.precision }; // DecimalType 3580 case -934922479: 3581 /* recall */ return this.recall == null ? new Base[0] : new Base[] { this.recall }; // DecimalType 3582 case -1295082036: 3583 /* fScore */ return this.fScore == null ? new Base[0] : new Base[] { this.fScore }; // DecimalType 3584 case 113094: 3585 /* roc */ return this.roc == null ? new Base[0] : new Base[] { this.roc }; // MolecularSequenceQualityRocComponent 3586 default: 3587 return super.getProperty(hash, name, checkValid); 3588 } 3589 3590 } 3591 3592 @Override 3593 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3594 switch (hash) { 3595 case 3575610: // type 3596 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 3597 this.type = (Enumeration) value; // Enumeration<QualityType> 3598 return value; 3599 case -1861227106: // standardSequence 3600 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 3601 return value; 3602 case 109757538: // start 3603 this.start = castToInteger(value); // IntegerType 3604 return value; 3605 case 100571: // end 3606 this.end = castToInteger(value); // IntegerType 3607 return value; 3608 case 109264530: // score 3609 this.score = castToQuantity(value); // Quantity 3610 return value; 3611 case -1077554975: // method 3612 this.method = castToCodeableConcept(value); // CodeableConcept 3613 return value; 3614 case -1048421849: // truthTP 3615 this.truthTP = castToDecimal(value); // DecimalType 3616 return value; 3617 case 655102276: // queryTP 3618 this.queryTP = castToDecimal(value); // DecimalType 3619 return value; 3620 case -1048422285: // truthFN 3621 this.truthFN = castToDecimal(value); // DecimalType 3622 return value; 3623 case 655101842: // queryFP 3624 this.queryFP = castToDecimal(value); // DecimalType 3625 return value; 3626 case 3182199: // gtFP 3627 this.gtFP = castToDecimal(value); // DecimalType 3628 return value; 3629 case -1376177026: // precision 3630 this.precision = castToDecimal(value); // DecimalType 3631 return value; 3632 case -934922479: // recall 3633 this.recall = castToDecimal(value); // DecimalType 3634 return value; 3635 case -1295082036: // fScore 3636 this.fScore = castToDecimal(value); // DecimalType 3637 return value; 3638 case 113094: // roc 3639 this.roc = (MolecularSequenceQualityRocComponent) value; // MolecularSequenceQualityRocComponent 3640 return value; 3641 default: 3642 return super.setProperty(hash, name, value); 3643 } 3644 3645 } 3646 3647 @Override 3648 public Base setProperty(String name, Base value) throws FHIRException { 3649 if (name.equals("type")) { 3650 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 3651 this.type = (Enumeration) value; // Enumeration<QualityType> 3652 } else if (name.equals("standardSequence")) { 3653 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 3654 } else if (name.equals("start")) { 3655 this.start = castToInteger(value); // IntegerType 3656 } else if (name.equals("end")) { 3657 this.end = castToInteger(value); // IntegerType 3658 } else if (name.equals("score")) { 3659 this.score = castToQuantity(value); // Quantity 3660 } else if (name.equals("method")) { 3661 this.method = castToCodeableConcept(value); // CodeableConcept 3662 } else if (name.equals("truthTP")) { 3663 this.truthTP = castToDecimal(value); // DecimalType 3664 } else if (name.equals("queryTP")) { 3665 this.queryTP = castToDecimal(value); // DecimalType 3666 } else if (name.equals("truthFN")) { 3667 this.truthFN = castToDecimal(value); // DecimalType 3668 } else if (name.equals("queryFP")) { 3669 this.queryFP = castToDecimal(value); // DecimalType 3670 } else if (name.equals("gtFP")) { 3671 this.gtFP = castToDecimal(value); // DecimalType 3672 } else if (name.equals("precision")) { 3673 this.precision = castToDecimal(value); // DecimalType 3674 } else if (name.equals("recall")) { 3675 this.recall = castToDecimal(value); // DecimalType 3676 } else if (name.equals("fScore")) { 3677 this.fScore = castToDecimal(value); // DecimalType 3678 } else if (name.equals("roc")) { 3679 this.roc = (MolecularSequenceQualityRocComponent) value; // MolecularSequenceQualityRocComponent 3680 } else 3681 return super.setProperty(name, value); 3682 return value; 3683 } 3684 3685 @Override 3686 public Base makeProperty(int hash, String name) throws FHIRException { 3687 switch (hash) { 3688 case 3575610: 3689 return getTypeElement(); 3690 case -1861227106: 3691 return getStandardSequence(); 3692 case 109757538: 3693 return getStartElement(); 3694 case 100571: 3695 return getEndElement(); 3696 case 109264530: 3697 return getScore(); 3698 case -1077554975: 3699 return getMethod(); 3700 case -1048421849: 3701 return getTruthTPElement(); 3702 case 655102276: 3703 return getQueryTPElement(); 3704 case -1048422285: 3705 return getTruthFNElement(); 3706 case 655101842: 3707 return getQueryFPElement(); 3708 case 3182199: 3709 return getGtFPElement(); 3710 case -1376177026: 3711 return getPrecisionElement(); 3712 case -934922479: 3713 return getRecallElement(); 3714 case -1295082036: 3715 return getFScoreElement(); 3716 case 113094: 3717 return getRoc(); 3718 default: 3719 return super.makeProperty(hash, name); 3720 } 3721 3722 } 3723 3724 @Override 3725 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3726 switch (hash) { 3727 case 3575610: 3728 /* type */ return new String[] { "code" }; 3729 case -1861227106: 3730 /* standardSequence */ return new String[] { "CodeableConcept" }; 3731 case 109757538: 3732 /* start */ return new String[] { "integer" }; 3733 case 100571: 3734 /* end */ return new String[] { "integer" }; 3735 case 109264530: 3736 /* score */ return new String[] { "Quantity" }; 3737 case -1077554975: 3738 /* method */ return new String[] { "CodeableConcept" }; 3739 case -1048421849: 3740 /* truthTP */ return new String[] { "decimal" }; 3741 case 655102276: 3742 /* queryTP */ return new String[] { "decimal" }; 3743 case -1048422285: 3744 /* truthFN */ return new String[] { "decimal" }; 3745 case 655101842: 3746 /* queryFP */ return new String[] { "decimal" }; 3747 case 3182199: 3748 /* gtFP */ return new String[] { "decimal" }; 3749 case -1376177026: 3750 /* precision */ return new String[] { "decimal" }; 3751 case -934922479: 3752 /* recall */ return new String[] { "decimal" }; 3753 case -1295082036: 3754 /* fScore */ return new String[] { "decimal" }; 3755 case 113094: 3756 /* roc */ return new String[] {}; 3757 default: 3758 return super.getTypesForProperty(hash, name); 3759 } 3760 3761 } 3762 3763 @Override 3764 public Base addChild(String name) throws FHIRException { 3765 if (name.equals("type")) { 3766 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 3767 } else if (name.equals("standardSequence")) { 3768 this.standardSequence = new CodeableConcept(); 3769 return this.standardSequence; 3770 } else if (name.equals("start")) { 3771 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 3772 } else if (name.equals("end")) { 3773 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 3774 } else if (name.equals("score")) { 3775 this.score = new Quantity(); 3776 return this.score; 3777 } else if (name.equals("method")) { 3778 this.method = new CodeableConcept(); 3779 return this.method; 3780 } else if (name.equals("truthTP")) { 3781 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.truthTP"); 3782 } else if (name.equals("queryTP")) { 3783 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.queryTP"); 3784 } else if (name.equals("truthFN")) { 3785 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.truthFN"); 3786 } else if (name.equals("queryFP")) { 3787 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.queryFP"); 3788 } else if (name.equals("gtFP")) { 3789 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.gtFP"); 3790 } else if (name.equals("precision")) { 3791 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.precision"); 3792 } else if (name.equals("recall")) { 3793 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.recall"); 3794 } else if (name.equals("fScore")) { 3795 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.fScore"); 3796 } else if (name.equals("roc")) { 3797 this.roc = new MolecularSequenceQualityRocComponent(); 3798 return this.roc; 3799 } else 3800 return super.addChild(name); 3801 } 3802 3803 public MolecularSequenceQualityComponent copy() { 3804 MolecularSequenceQualityComponent dst = new MolecularSequenceQualityComponent(); 3805 copyValues(dst); 3806 return dst; 3807 } 3808 3809 public void copyValues(MolecularSequenceQualityComponent dst) { 3810 super.copyValues(dst); 3811 dst.type = type == null ? null : type.copy(); 3812 dst.standardSequence = standardSequence == null ? null : standardSequence.copy(); 3813 dst.start = start == null ? null : start.copy(); 3814 dst.end = end == null ? null : end.copy(); 3815 dst.score = score == null ? null : score.copy(); 3816 dst.method = method == null ? null : method.copy(); 3817 dst.truthTP = truthTP == null ? null : truthTP.copy(); 3818 dst.queryTP = queryTP == null ? null : queryTP.copy(); 3819 dst.truthFN = truthFN == null ? null : truthFN.copy(); 3820 dst.queryFP = queryFP == null ? null : queryFP.copy(); 3821 dst.gtFP = gtFP == null ? null : gtFP.copy(); 3822 dst.precision = precision == null ? null : precision.copy(); 3823 dst.recall = recall == null ? null : recall.copy(); 3824 dst.fScore = fScore == null ? null : fScore.copy(); 3825 dst.roc = roc == null ? null : roc.copy(); 3826 } 3827 3828 @Override 3829 public boolean equalsDeep(Base other_) { 3830 if (!super.equalsDeep(other_)) 3831 return false; 3832 if (!(other_ instanceof MolecularSequenceQualityComponent)) 3833 return false; 3834 MolecularSequenceQualityComponent o = (MolecularSequenceQualityComponent) other_; 3835 return compareDeep(type, o.type, true) && compareDeep(standardSequence, o.standardSequence, true) 3836 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(score, o.score, true) 3837 && compareDeep(method, o.method, true) && compareDeep(truthTP, o.truthTP, true) 3838 && compareDeep(queryTP, o.queryTP, true) && compareDeep(truthFN, o.truthFN, true) 3839 && compareDeep(queryFP, o.queryFP, true) && compareDeep(gtFP, o.gtFP, true) 3840 && compareDeep(precision, o.precision, true) && compareDeep(recall, o.recall, true) 3841 && compareDeep(fScore, o.fScore, true) && compareDeep(roc, o.roc, true); 3842 } 3843 3844 @Override 3845 public boolean equalsShallow(Base other_) { 3846 if (!super.equalsShallow(other_)) 3847 return false; 3848 if (!(other_ instanceof MolecularSequenceQualityComponent)) 3849 return false; 3850 MolecularSequenceQualityComponent o = (MolecularSequenceQualityComponent) other_; 3851 return compareValues(type, o.type, true) && compareValues(start, o.start, true) && compareValues(end, o.end, true) 3852 && compareValues(truthTP, o.truthTP, true) && compareValues(queryTP, o.queryTP, true) 3853 && compareValues(truthFN, o.truthFN, true) && compareValues(queryFP, o.queryFP, true) 3854 && compareValues(gtFP, o.gtFP, true) && compareValues(precision, o.precision, true) 3855 && compareValues(recall, o.recall, true) && compareValues(fScore, o.fScore, true); 3856 } 3857 3858 public boolean isEmpty() { 3859 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, standardSequence, start, end, score, method, 3860 truthTP, queryTP, truthFN, queryFP, gtFP, precision, recall, fScore, roc); 3861 } 3862 3863 public String fhirType() { 3864 return "MolecularSequence.quality"; 3865 3866 } 3867 3868 } 3869 3870 @Block() 3871 public static class MolecularSequenceQualityRocComponent extends BackboneElement implements IBaseBackboneElement { 3872 /** 3873 * Invidual data point representing the GQ (genotype quality) score threshold. 3874 */ 3875 @Child(name = "score", type = { 3876 IntegerType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3877 @Description(shortDefinition = "Genotype quality score", formalDefinition = "Invidual data point representing the GQ (genotype quality) score threshold.") 3878 protected List<IntegerType> score; 3879 3880 /** 3881 * The number of true positives if the GQ score threshold was set to "score" 3882 * field value. 3883 */ 3884 @Child(name = "numTP", type = { 3885 IntegerType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3886 @Description(shortDefinition = "Roc score true positive numbers", formalDefinition = "The number of true positives if the GQ score threshold was set to \"score\" field value.") 3887 protected List<IntegerType> numTP; 3888 3889 /** 3890 * The number of false positives if the GQ score threshold was set to "score" 3891 * field value. 3892 */ 3893 @Child(name = "numFP", type = { 3894 IntegerType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3895 @Description(shortDefinition = "Roc score false positive numbers", formalDefinition = "The number of false positives if the GQ score threshold was set to \"score\" field value.") 3896 protected List<IntegerType> numFP; 3897 3898 /** 3899 * The number of false negatives if the GQ score threshold was set to "score" 3900 * field value. 3901 */ 3902 @Child(name = "numFN", type = { 3903 IntegerType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3904 @Description(shortDefinition = "Roc score false negative numbers", formalDefinition = "The number of false negatives if the GQ score threshold was set to \"score\" field value.") 3905 protected List<IntegerType> numFN; 3906 3907 /** 3908 * Calculated precision if the GQ score threshold was set to "score" field 3909 * value. 3910 */ 3911 @Child(name = "precision", type = { 3912 DecimalType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3913 @Description(shortDefinition = "Precision of the GQ score", formalDefinition = "Calculated precision if the GQ score threshold was set to \"score\" field value.") 3914 protected List<DecimalType> precision; 3915 3916 /** 3917 * Calculated sensitivity if the GQ score threshold was set to "score" field 3918 * value. 3919 */ 3920 @Child(name = "sensitivity", type = { 3921 DecimalType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3922 @Description(shortDefinition = "Sensitivity of the GQ score", formalDefinition = "Calculated sensitivity if the GQ score threshold was set to \"score\" field value.") 3923 protected List<DecimalType> sensitivity; 3924 3925 /** 3926 * Calculated fScore if the GQ score threshold was set to "score" field value. 3927 */ 3928 @Child(name = "fMeasure", type = { 3929 DecimalType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3930 @Description(shortDefinition = "FScore of the GQ score", formalDefinition = "Calculated fScore if the GQ score threshold was set to \"score\" field value.") 3931 protected List<DecimalType> fMeasure; 3932 3933 private static final long serialVersionUID = 1923392132L; 3934 3935 /** 3936 * Constructor 3937 */ 3938 public MolecularSequenceQualityRocComponent() { 3939 super(); 3940 } 3941 3942 /** 3943 * @return {@link #score} (Invidual data point representing the GQ (genotype 3944 * quality) score threshold.) 3945 */ 3946 public List<IntegerType> getScore() { 3947 if (this.score == null) 3948 this.score = new ArrayList<IntegerType>(); 3949 return this.score; 3950 } 3951 3952 /** 3953 * @return Returns a reference to <code>this</code> for easy method chaining 3954 */ 3955 public MolecularSequenceQualityRocComponent setScore(List<IntegerType> theScore) { 3956 this.score = theScore; 3957 return this; 3958 } 3959 3960 public boolean hasScore() { 3961 if (this.score == null) 3962 return false; 3963 for (IntegerType item : this.score) 3964 if (!item.isEmpty()) 3965 return true; 3966 return false; 3967 } 3968 3969 /** 3970 * @return {@link #score} (Invidual data point representing the GQ (genotype 3971 * quality) score threshold.) 3972 */ 3973 public IntegerType addScoreElement() {// 2 3974 IntegerType t = new IntegerType(); 3975 if (this.score == null) 3976 this.score = new ArrayList<IntegerType>(); 3977 this.score.add(t); 3978 return t; 3979 } 3980 3981 /** 3982 * @param value {@link #score} (Invidual data point representing the GQ 3983 * (genotype quality) score threshold.) 3984 */ 3985 public MolecularSequenceQualityRocComponent addScore(int value) { // 1 3986 IntegerType t = new IntegerType(); 3987 t.setValue(value); 3988 if (this.score == null) 3989 this.score = new ArrayList<IntegerType>(); 3990 this.score.add(t); 3991 return this; 3992 } 3993 3994 /** 3995 * @param value {@link #score} (Invidual data point representing the GQ 3996 * (genotype quality) score threshold.) 3997 */ 3998 public boolean hasScore(int value) { 3999 if (this.score == null) 4000 return false; 4001 for (IntegerType v : this.score) 4002 if (v.getValue().equals(value)) // integer 4003 return true; 4004 return false; 4005 } 4006 4007 /** 4008 * @return {@link #numTP} (The number of true positives if the GQ score 4009 * threshold was set to "score" field value.) 4010 */ 4011 public List<IntegerType> getNumTP() { 4012 if (this.numTP == null) 4013 this.numTP = new ArrayList<IntegerType>(); 4014 return this.numTP; 4015 } 4016 4017 /** 4018 * @return Returns a reference to <code>this</code> for easy method chaining 4019 */ 4020 public MolecularSequenceQualityRocComponent setNumTP(List<IntegerType> theNumTP) { 4021 this.numTP = theNumTP; 4022 return this; 4023 } 4024 4025 public boolean hasNumTP() { 4026 if (this.numTP == null) 4027 return false; 4028 for (IntegerType item : this.numTP) 4029 if (!item.isEmpty()) 4030 return true; 4031 return false; 4032 } 4033 4034 /** 4035 * @return {@link #numTP} (The number of true positives if the GQ score 4036 * threshold was set to "score" field value.) 4037 */ 4038 public IntegerType addNumTPElement() {// 2 4039 IntegerType t = new IntegerType(); 4040 if (this.numTP == null) 4041 this.numTP = new ArrayList<IntegerType>(); 4042 this.numTP.add(t); 4043 return t; 4044 } 4045 4046 /** 4047 * @param value {@link #numTP} (The number of true positives if the GQ score 4048 * threshold was set to "score" field value.) 4049 */ 4050 public MolecularSequenceQualityRocComponent addNumTP(int value) { // 1 4051 IntegerType t = new IntegerType(); 4052 t.setValue(value); 4053 if (this.numTP == null) 4054 this.numTP = new ArrayList<IntegerType>(); 4055 this.numTP.add(t); 4056 return this; 4057 } 4058 4059 /** 4060 * @param value {@link #numTP} (The number of true positives if the GQ score 4061 * threshold was set to "score" field value.) 4062 */ 4063 public boolean hasNumTP(int value) { 4064 if (this.numTP == null) 4065 return false; 4066 for (IntegerType v : this.numTP) 4067 if (v.getValue().equals(value)) // integer 4068 return true; 4069 return false; 4070 } 4071 4072 /** 4073 * @return {@link #numFP} (The number of false positives if the GQ score 4074 * threshold was set to "score" field value.) 4075 */ 4076 public List<IntegerType> getNumFP() { 4077 if (this.numFP == null) 4078 this.numFP = new ArrayList<IntegerType>(); 4079 return this.numFP; 4080 } 4081 4082 /** 4083 * @return Returns a reference to <code>this</code> for easy method chaining 4084 */ 4085 public MolecularSequenceQualityRocComponent setNumFP(List<IntegerType> theNumFP) { 4086 this.numFP = theNumFP; 4087 return this; 4088 } 4089 4090 public boolean hasNumFP() { 4091 if (this.numFP == null) 4092 return false; 4093 for (IntegerType item : this.numFP) 4094 if (!item.isEmpty()) 4095 return true; 4096 return false; 4097 } 4098 4099 /** 4100 * @return {@link #numFP} (The number of false positives if the GQ score 4101 * threshold was set to "score" field value.) 4102 */ 4103 public IntegerType addNumFPElement() {// 2 4104 IntegerType t = new IntegerType(); 4105 if (this.numFP == null) 4106 this.numFP = new ArrayList<IntegerType>(); 4107 this.numFP.add(t); 4108 return t; 4109 } 4110 4111 /** 4112 * @param value {@link #numFP} (The number of false positives if the GQ score 4113 * threshold was set to "score" field value.) 4114 */ 4115 public MolecularSequenceQualityRocComponent addNumFP(int value) { // 1 4116 IntegerType t = new IntegerType(); 4117 t.setValue(value); 4118 if (this.numFP == null) 4119 this.numFP = new ArrayList<IntegerType>(); 4120 this.numFP.add(t); 4121 return this; 4122 } 4123 4124 /** 4125 * @param value {@link #numFP} (The number of false positives if the GQ score 4126 * threshold was set to "score" field value.) 4127 */ 4128 public boolean hasNumFP(int value) { 4129 if (this.numFP == null) 4130 return false; 4131 for (IntegerType v : this.numFP) 4132 if (v.getValue().equals(value)) // integer 4133 return true; 4134 return false; 4135 } 4136 4137 /** 4138 * @return {@link #numFN} (The number of false negatives if the GQ score 4139 * threshold was set to "score" field value.) 4140 */ 4141 public List<IntegerType> getNumFN() { 4142 if (this.numFN == null) 4143 this.numFN = new ArrayList<IntegerType>(); 4144 return this.numFN; 4145 } 4146 4147 /** 4148 * @return Returns a reference to <code>this</code> for easy method chaining 4149 */ 4150 public MolecularSequenceQualityRocComponent setNumFN(List<IntegerType> theNumFN) { 4151 this.numFN = theNumFN; 4152 return this; 4153 } 4154 4155 public boolean hasNumFN() { 4156 if (this.numFN == null) 4157 return false; 4158 for (IntegerType item : this.numFN) 4159 if (!item.isEmpty()) 4160 return true; 4161 return false; 4162 } 4163 4164 /** 4165 * @return {@link #numFN} (The number of false negatives if the GQ score 4166 * threshold was set to "score" field value.) 4167 */ 4168 public IntegerType addNumFNElement() {// 2 4169 IntegerType t = new IntegerType(); 4170 if (this.numFN == null) 4171 this.numFN = new ArrayList<IntegerType>(); 4172 this.numFN.add(t); 4173 return t; 4174 } 4175 4176 /** 4177 * @param value {@link #numFN} (The number of false negatives if the GQ score 4178 * threshold was set to "score" field value.) 4179 */ 4180 public MolecularSequenceQualityRocComponent addNumFN(int value) { // 1 4181 IntegerType t = new IntegerType(); 4182 t.setValue(value); 4183 if (this.numFN == null) 4184 this.numFN = new ArrayList<IntegerType>(); 4185 this.numFN.add(t); 4186 return this; 4187 } 4188 4189 /** 4190 * @param value {@link #numFN} (The number of false negatives if the GQ score 4191 * threshold was set to "score" field value.) 4192 */ 4193 public boolean hasNumFN(int value) { 4194 if (this.numFN == null) 4195 return false; 4196 for (IntegerType v : this.numFN) 4197 if (v.getValue().equals(value)) // integer 4198 return true; 4199 return false; 4200 } 4201 4202 /** 4203 * @return {@link #precision} (Calculated precision if the GQ score threshold 4204 * was set to "score" field value.) 4205 */ 4206 public List<DecimalType> getPrecision() { 4207 if (this.precision == null) 4208 this.precision = new ArrayList<DecimalType>(); 4209 return this.precision; 4210 } 4211 4212 /** 4213 * @return Returns a reference to <code>this</code> for easy method chaining 4214 */ 4215 public MolecularSequenceQualityRocComponent setPrecision(List<DecimalType> thePrecision) { 4216 this.precision = thePrecision; 4217 return this; 4218 } 4219 4220 public boolean hasPrecision() { 4221 if (this.precision == null) 4222 return false; 4223 for (DecimalType item : this.precision) 4224 if (!item.isEmpty()) 4225 return true; 4226 return false; 4227 } 4228 4229 /** 4230 * @return {@link #precision} (Calculated precision if the GQ score threshold 4231 * was set to "score" field value.) 4232 */ 4233 public DecimalType addPrecisionElement() {// 2 4234 DecimalType t = new DecimalType(); 4235 if (this.precision == null) 4236 this.precision = new ArrayList<DecimalType>(); 4237 this.precision.add(t); 4238 return t; 4239 } 4240 4241 /** 4242 * @param value {@link #precision} (Calculated precision if the GQ score 4243 * threshold was set to "score" field value.) 4244 */ 4245 public MolecularSequenceQualityRocComponent addPrecision(BigDecimal value) { // 1 4246 DecimalType t = new DecimalType(); 4247 t.setValue(value); 4248 if (this.precision == null) 4249 this.precision = new ArrayList<DecimalType>(); 4250 this.precision.add(t); 4251 return this; 4252 } 4253 4254 /** 4255 * @param value {@link #precision} (Calculated precision if the GQ score 4256 * threshold was set to "score" field value.) 4257 */ 4258 public boolean hasPrecision(BigDecimal value) { 4259 if (this.precision == null) 4260 return false; 4261 for (DecimalType v : this.precision) 4262 if (v.getValue().equals(value)) // decimal 4263 return true; 4264 return false; 4265 } 4266 4267 /** 4268 * @return {@link #sensitivity} (Calculated sensitivity if the GQ score 4269 * threshold was set to "score" field value.) 4270 */ 4271 public List<DecimalType> getSensitivity() { 4272 if (this.sensitivity == null) 4273 this.sensitivity = new ArrayList<DecimalType>(); 4274 return this.sensitivity; 4275 } 4276 4277 /** 4278 * @return Returns a reference to <code>this</code> for easy method chaining 4279 */ 4280 public MolecularSequenceQualityRocComponent setSensitivity(List<DecimalType> theSensitivity) { 4281 this.sensitivity = theSensitivity; 4282 return this; 4283 } 4284 4285 public boolean hasSensitivity() { 4286 if (this.sensitivity == null) 4287 return false; 4288 for (DecimalType item : this.sensitivity) 4289 if (!item.isEmpty()) 4290 return true; 4291 return false; 4292 } 4293 4294 /** 4295 * @return {@link #sensitivity} (Calculated sensitivity if the GQ score 4296 * threshold was set to "score" field value.) 4297 */ 4298 public DecimalType addSensitivityElement() {// 2 4299 DecimalType t = new DecimalType(); 4300 if (this.sensitivity == null) 4301 this.sensitivity = new ArrayList<DecimalType>(); 4302 this.sensitivity.add(t); 4303 return t; 4304 } 4305 4306 /** 4307 * @param value {@link #sensitivity} (Calculated sensitivity if the GQ score 4308 * threshold was set to "score" field value.) 4309 */ 4310 public MolecularSequenceQualityRocComponent addSensitivity(BigDecimal value) { // 1 4311 DecimalType t = new DecimalType(); 4312 t.setValue(value); 4313 if (this.sensitivity == null) 4314 this.sensitivity = new ArrayList<DecimalType>(); 4315 this.sensitivity.add(t); 4316 return this; 4317 } 4318 4319 /** 4320 * @param value {@link #sensitivity} (Calculated sensitivity if the GQ score 4321 * threshold was set to "score" field value.) 4322 */ 4323 public boolean hasSensitivity(BigDecimal value) { 4324 if (this.sensitivity == null) 4325 return false; 4326 for (DecimalType v : this.sensitivity) 4327 if (v.getValue().equals(value)) // decimal 4328 return true; 4329 return false; 4330 } 4331 4332 /** 4333 * @return {@link #fMeasure} (Calculated fScore if the GQ score threshold was 4334 * set to "score" field value.) 4335 */ 4336 public List<DecimalType> getFMeasure() { 4337 if (this.fMeasure == null) 4338 this.fMeasure = new ArrayList<DecimalType>(); 4339 return this.fMeasure; 4340 } 4341 4342 /** 4343 * @return Returns a reference to <code>this</code> for easy method chaining 4344 */ 4345 public MolecularSequenceQualityRocComponent setFMeasure(List<DecimalType> theFMeasure) { 4346 this.fMeasure = theFMeasure; 4347 return this; 4348 } 4349 4350 public boolean hasFMeasure() { 4351 if (this.fMeasure == null) 4352 return false; 4353 for (DecimalType item : this.fMeasure) 4354 if (!item.isEmpty()) 4355 return true; 4356 return false; 4357 } 4358 4359 /** 4360 * @return {@link #fMeasure} (Calculated fScore if the GQ score threshold was 4361 * set to "score" field value.) 4362 */ 4363 public DecimalType addFMeasureElement() {// 2 4364 DecimalType t = new DecimalType(); 4365 if (this.fMeasure == null) 4366 this.fMeasure = new ArrayList<DecimalType>(); 4367 this.fMeasure.add(t); 4368 return t; 4369 } 4370 4371 /** 4372 * @param value {@link #fMeasure} (Calculated fScore if the GQ score threshold 4373 * was set to "score" field value.) 4374 */ 4375 public MolecularSequenceQualityRocComponent addFMeasure(BigDecimal value) { // 1 4376 DecimalType t = new DecimalType(); 4377 t.setValue(value); 4378 if (this.fMeasure == null) 4379 this.fMeasure = new ArrayList<DecimalType>(); 4380 this.fMeasure.add(t); 4381 return this; 4382 } 4383 4384 /** 4385 * @param value {@link #fMeasure} (Calculated fScore if the GQ score threshold 4386 * was set to "score" field value.) 4387 */ 4388 public boolean hasFMeasure(BigDecimal value) { 4389 if (this.fMeasure == null) 4390 return false; 4391 for (DecimalType v : this.fMeasure) 4392 if (v.getValue().equals(value)) // decimal 4393 return true; 4394 return false; 4395 } 4396 4397 protected void listChildren(List<Property> children) { 4398 super.listChildren(children); 4399 children.add(new Property("score", "integer", 4400 "Invidual data point representing the GQ (genotype quality) score threshold.", 0, java.lang.Integer.MAX_VALUE, 4401 score)); 4402 children.add(new Property("numTP", "integer", 4403 "The number of true positives if the GQ score threshold was set to \"score\" field value.", 0, 4404 java.lang.Integer.MAX_VALUE, numTP)); 4405 children.add(new Property("numFP", "integer", 4406 "The number of false positives if the GQ score threshold was set to \"score\" field value.", 0, 4407 java.lang.Integer.MAX_VALUE, numFP)); 4408 children.add(new Property("numFN", "integer", 4409 "The number of false negatives if the GQ score threshold was set to \"score\" field value.", 0, 4410 java.lang.Integer.MAX_VALUE, numFN)); 4411 children.add(new Property("precision", "decimal", 4412 "Calculated precision if the GQ score threshold was set to \"score\" field value.", 0, 4413 java.lang.Integer.MAX_VALUE, precision)); 4414 children.add(new Property("sensitivity", "decimal", 4415 "Calculated sensitivity if the GQ score threshold was set to \"score\" field value.", 0, 4416 java.lang.Integer.MAX_VALUE, sensitivity)); 4417 children.add(new Property("fMeasure", "decimal", 4418 "Calculated fScore if the GQ score threshold was set to \"score\" field value.", 0, 4419 java.lang.Integer.MAX_VALUE, fMeasure)); 4420 } 4421 4422 @Override 4423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4424 switch (_hash) { 4425 case 109264530: 4426 /* score */ return new Property("score", "integer", 4427 "Invidual data point representing the GQ (genotype quality) score threshold.", 0, 4428 java.lang.Integer.MAX_VALUE, score); 4429 case 105180290: 4430 /* numTP */ return new Property("numTP", "integer", 4431 "The number of true positives if the GQ score threshold was set to \"score\" field value.", 0, 4432 java.lang.Integer.MAX_VALUE, numTP); 4433 case 105179856: 4434 /* numFP */ return new Property("numFP", "integer", 4435 "The number of false positives if the GQ score threshold was set to \"score\" field value.", 0, 4436 java.lang.Integer.MAX_VALUE, numFP); 4437 case 105179854: 4438 /* numFN */ return new Property("numFN", "integer", 4439 "The number of false negatives if the GQ score threshold was set to \"score\" field value.", 0, 4440 java.lang.Integer.MAX_VALUE, numFN); 4441 case -1376177026: 4442 /* precision */ return new Property("precision", "decimal", 4443 "Calculated precision if the GQ score threshold was set to \"score\" field value.", 0, 4444 java.lang.Integer.MAX_VALUE, precision); 4445 case 564403871: 4446 /* sensitivity */ return new Property("sensitivity", "decimal", 4447 "Calculated sensitivity if the GQ score threshold was set to \"score\" field value.", 0, 4448 java.lang.Integer.MAX_VALUE, sensitivity); 4449 case -18997736: 4450 /* fMeasure */ return new Property("fMeasure", "decimal", 4451 "Calculated fScore if the GQ score threshold was set to \"score\" field value.", 0, 4452 java.lang.Integer.MAX_VALUE, fMeasure); 4453 default: 4454 return super.getNamedProperty(_hash, _name, _checkValid); 4455 } 4456 4457 } 4458 4459 @Override 4460 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4461 switch (hash) { 4462 case 109264530: 4463 /* score */ return this.score == null ? new Base[0] : this.score.toArray(new Base[this.score.size()]); // IntegerType 4464 case 105180290: 4465 /* numTP */ return this.numTP == null ? new Base[0] : this.numTP.toArray(new Base[this.numTP.size()]); // IntegerType 4466 case 105179856: 4467 /* numFP */ return this.numFP == null ? new Base[0] : this.numFP.toArray(new Base[this.numFP.size()]); // IntegerType 4468 case 105179854: 4469 /* numFN */ return this.numFN == null ? new Base[0] : this.numFN.toArray(new Base[this.numFN.size()]); // IntegerType 4470 case -1376177026: 4471 /* precision */ return this.precision == null ? new Base[0] 4472 : this.precision.toArray(new Base[this.precision.size()]); // DecimalType 4473 case 564403871: 4474 /* sensitivity */ return this.sensitivity == null ? new Base[0] 4475 : this.sensitivity.toArray(new Base[this.sensitivity.size()]); // DecimalType 4476 case -18997736: 4477 /* fMeasure */ return this.fMeasure == null ? new Base[0] 4478 : this.fMeasure.toArray(new Base[this.fMeasure.size()]); // DecimalType 4479 default: 4480 return super.getProperty(hash, name, checkValid); 4481 } 4482 4483 } 4484 4485 @Override 4486 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4487 switch (hash) { 4488 case 109264530: // score 4489 this.getScore().add(castToInteger(value)); // IntegerType 4490 return value; 4491 case 105180290: // numTP 4492 this.getNumTP().add(castToInteger(value)); // IntegerType 4493 return value; 4494 case 105179856: // numFP 4495 this.getNumFP().add(castToInteger(value)); // IntegerType 4496 return value; 4497 case 105179854: // numFN 4498 this.getNumFN().add(castToInteger(value)); // IntegerType 4499 return value; 4500 case -1376177026: // precision 4501 this.getPrecision().add(castToDecimal(value)); // DecimalType 4502 return value; 4503 case 564403871: // sensitivity 4504 this.getSensitivity().add(castToDecimal(value)); // DecimalType 4505 return value; 4506 case -18997736: // fMeasure 4507 this.getFMeasure().add(castToDecimal(value)); // DecimalType 4508 return value; 4509 default: 4510 return super.setProperty(hash, name, value); 4511 } 4512 4513 } 4514 4515 @Override 4516 public Base setProperty(String name, Base value) throws FHIRException { 4517 if (name.equals("score")) { 4518 this.getScore().add(castToInteger(value)); 4519 } else if (name.equals("numTP")) { 4520 this.getNumTP().add(castToInteger(value)); 4521 } else if (name.equals("numFP")) { 4522 this.getNumFP().add(castToInteger(value)); 4523 } else if (name.equals("numFN")) { 4524 this.getNumFN().add(castToInteger(value)); 4525 } else if (name.equals("precision")) { 4526 this.getPrecision().add(castToDecimal(value)); 4527 } else if (name.equals("sensitivity")) { 4528 this.getSensitivity().add(castToDecimal(value)); 4529 } else if (name.equals("fMeasure")) { 4530 this.getFMeasure().add(castToDecimal(value)); 4531 } else 4532 return super.setProperty(name, value); 4533 return value; 4534 } 4535 4536 @Override 4537 public Base makeProperty(int hash, String name) throws FHIRException { 4538 switch (hash) { 4539 case 109264530: 4540 return addScoreElement(); 4541 case 105180290: 4542 return addNumTPElement(); 4543 case 105179856: 4544 return addNumFPElement(); 4545 case 105179854: 4546 return addNumFNElement(); 4547 case -1376177026: 4548 return addPrecisionElement(); 4549 case 564403871: 4550 return addSensitivityElement(); 4551 case -18997736: 4552 return addFMeasureElement(); 4553 default: 4554 return super.makeProperty(hash, name); 4555 } 4556 4557 } 4558 4559 @Override 4560 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4561 switch (hash) { 4562 case 109264530: 4563 /* score */ return new String[] { "integer" }; 4564 case 105180290: 4565 /* numTP */ return new String[] { "integer" }; 4566 case 105179856: 4567 /* numFP */ return new String[] { "integer" }; 4568 case 105179854: 4569 /* numFN */ return new String[] { "integer" }; 4570 case -1376177026: 4571 /* precision */ return new String[] { "decimal" }; 4572 case 564403871: 4573 /* sensitivity */ return new String[] { "decimal" }; 4574 case -18997736: 4575 /* fMeasure */ return new String[] { "decimal" }; 4576 default: 4577 return super.getTypesForProperty(hash, name); 4578 } 4579 4580 } 4581 4582 @Override 4583 public Base addChild(String name) throws FHIRException { 4584 if (name.equals("score")) { 4585 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.score"); 4586 } else if (name.equals("numTP")) { 4587 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.numTP"); 4588 } else if (name.equals("numFP")) { 4589 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.numFP"); 4590 } else if (name.equals("numFN")) { 4591 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.numFN"); 4592 } else if (name.equals("precision")) { 4593 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.precision"); 4594 } else if (name.equals("sensitivity")) { 4595 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.sensitivity"); 4596 } else if (name.equals("fMeasure")) { 4597 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.fMeasure"); 4598 } else 4599 return super.addChild(name); 4600 } 4601 4602 public MolecularSequenceQualityRocComponent copy() { 4603 MolecularSequenceQualityRocComponent dst = new MolecularSequenceQualityRocComponent(); 4604 copyValues(dst); 4605 return dst; 4606 } 4607 4608 public void copyValues(MolecularSequenceQualityRocComponent dst) { 4609 super.copyValues(dst); 4610 if (score != null) { 4611 dst.score = new ArrayList<IntegerType>(); 4612 for (IntegerType i : score) 4613 dst.score.add(i.copy()); 4614 } 4615 ; 4616 if (numTP != null) { 4617 dst.numTP = new ArrayList<IntegerType>(); 4618 for (IntegerType i : numTP) 4619 dst.numTP.add(i.copy()); 4620 } 4621 ; 4622 if (numFP != null) { 4623 dst.numFP = new ArrayList<IntegerType>(); 4624 for (IntegerType i : numFP) 4625 dst.numFP.add(i.copy()); 4626 } 4627 ; 4628 if (numFN != null) { 4629 dst.numFN = new ArrayList<IntegerType>(); 4630 for (IntegerType i : numFN) 4631 dst.numFN.add(i.copy()); 4632 } 4633 ; 4634 if (precision != null) { 4635 dst.precision = new ArrayList<DecimalType>(); 4636 for (DecimalType i : precision) 4637 dst.precision.add(i.copy()); 4638 } 4639 ; 4640 if (sensitivity != null) { 4641 dst.sensitivity = new ArrayList<DecimalType>(); 4642 for (DecimalType i : sensitivity) 4643 dst.sensitivity.add(i.copy()); 4644 } 4645 ; 4646 if (fMeasure != null) { 4647 dst.fMeasure = new ArrayList<DecimalType>(); 4648 for (DecimalType i : fMeasure) 4649 dst.fMeasure.add(i.copy()); 4650 } 4651 ; 4652 } 4653 4654 @Override 4655 public boolean equalsDeep(Base other_) { 4656 if (!super.equalsDeep(other_)) 4657 return false; 4658 if (!(other_ instanceof MolecularSequenceQualityRocComponent)) 4659 return false; 4660 MolecularSequenceQualityRocComponent o = (MolecularSequenceQualityRocComponent) other_; 4661 return compareDeep(score, o.score, true) && compareDeep(numTP, o.numTP, true) && compareDeep(numFP, o.numFP, true) 4662 && compareDeep(numFN, o.numFN, true) && compareDeep(precision, o.precision, true) 4663 && compareDeep(sensitivity, o.sensitivity, true) && compareDeep(fMeasure, o.fMeasure, true); 4664 } 4665 4666 @Override 4667 public boolean equalsShallow(Base other_) { 4668 if (!super.equalsShallow(other_)) 4669 return false; 4670 if (!(other_ instanceof MolecularSequenceQualityRocComponent)) 4671 return false; 4672 MolecularSequenceQualityRocComponent o = (MolecularSequenceQualityRocComponent) other_; 4673 return compareValues(score, o.score, true) && compareValues(numTP, o.numTP, true) 4674 && compareValues(numFP, o.numFP, true) && compareValues(numFN, o.numFN, true) 4675 && compareValues(precision, o.precision, true) && compareValues(sensitivity, o.sensitivity, true) 4676 && compareValues(fMeasure, o.fMeasure, true); 4677 } 4678 4679 public boolean isEmpty() { 4680 return super.isEmpty() 4681 && ca.uhn.fhir.util.ElementUtil.isEmpty(score, numTP, numFP, numFN, precision, sensitivity, fMeasure); 4682 } 4683 4684 public String fhirType() { 4685 return "MolecularSequence.quality.roc"; 4686 4687 } 4688 4689 } 4690 4691 @Block() 4692 public static class MolecularSequenceRepositoryComponent extends BackboneElement implements IBaseBackboneElement { 4693 /** 4694 * Click and see / RESTful API / Need login to see / RESTful API with 4695 * authentication / Other ways to see resource. 4696 */ 4697 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4698 @Description(shortDefinition = "directlink | openapi | login | oauth | other", formalDefinition = "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.") 4699 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/repository-type") 4700 protected Enumeration<RepositoryType> type; 4701 4702 /** 4703 * URI of an external repository which contains further details about the 4704 * genetics data. 4705 */ 4706 @Child(name = "url", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4707 @Description(shortDefinition = "URI of the repository", formalDefinition = "URI of an external repository which contains further details about the genetics data.") 4708 protected UriType url; 4709 4710 /** 4711 * URI of an external repository which contains further details about the 4712 * genetics data. 4713 */ 4714 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4715 @Description(shortDefinition = "Repository's name", formalDefinition = "URI of an external repository which contains further details about the genetics data.") 4716 protected StringType name; 4717 4718 /** 4719 * Id of the variant in this external repository. The server will understand how 4720 * to use this id to call for more info about datasets in external repository. 4721 */ 4722 @Child(name = "datasetId", type = { 4723 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4724 @Description(shortDefinition = "Id of the dataset that used to call for dataset in repository", formalDefinition = "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.") 4725 protected StringType datasetId; 4726 4727 /** 4728 * Id of the variantset in this external repository. The server will understand 4729 * how to use this id to call for more info about variantsets in external 4730 * repository. 4731 */ 4732 @Child(name = "variantsetId", type = { 4733 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4734 @Description(shortDefinition = "Id of the variantset that used to call for variantset in repository", formalDefinition = "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.") 4735 protected StringType variantsetId; 4736 4737 /** 4738 * Id of the read in this external repository. 4739 */ 4740 @Child(name = "readsetId", type = { 4741 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 4742 @Description(shortDefinition = "Id of the read", formalDefinition = "Id of the read in this external repository.") 4743 protected StringType readsetId; 4744 4745 private static final long serialVersionUID = -899243265L; 4746 4747 /** 4748 * Constructor 4749 */ 4750 public MolecularSequenceRepositoryComponent() { 4751 super(); 4752 } 4753 4754 /** 4755 * Constructor 4756 */ 4757 public MolecularSequenceRepositoryComponent(Enumeration<RepositoryType> type) { 4758 super(); 4759 this.type = type; 4760 } 4761 4762 /** 4763 * @return {@link #type} (Click and see / RESTful API / Need login to see / 4764 * RESTful API with authentication / Other ways to see resource.). This 4765 * is the underlying object with id, value and extensions. The accessor 4766 * "getType" gives direct access to the value 4767 */ 4768 public Enumeration<RepositoryType> getTypeElement() { 4769 if (this.type == null) 4770 if (Configuration.errorOnAutoCreate()) 4771 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.type"); 4772 else if (Configuration.doAutoCreate()) 4773 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); // bb 4774 return this.type; 4775 } 4776 4777 public boolean hasTypeElement() { 4778 return this.type != null && !this.type.isEmpty(); 4779 } 4780 4781 public boolean hasType() { 4782 return this.type != null && !this.type.isEmpty(); 4783 } 4784 4785 /** 4786 * @param value {@link #type} (Click and see / RESTful API / Need login to see / 4787 * RESTful API with authentication / Other ways to see resource.). 4788 * This is the underlying object with id, value and extensions. The 4789 * accessor "getType" gives direct access to the value 4790 */ 4791 public MolecularSequenceRepositoryComponent setTypeElement(Enumeration<RepositoryType> value) { 4792 this.type = value; 4793 return this; 4794 } 4795 4796 /** 4797 * @return Click and see / RESTful API / Need login to see / RESTful API with 4798 * authentication / Other ways to see resource. 4799 */ 4800 public RepositoryType getType() { 4801 return this.type == null ? null : this.type.getValue(); 4802 } 4803 4804 /** 4805 * @param value Click and see / RESTful API / Need login to see / RESTful API 4806 * with authentication / Other ways to see resource. 4807 */ 4808 public MolecularSequenceRepositoryComponent setType(RepositoryType value) { 4809 if (this.type == null) 4810 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); 4811 this.type.setValue(value); 4812 return this; 4813 } 4814 4815 /** 4816 * @return {@link #url} (URI of an external repository which contains further 4817 * details about the genetics data.). This is the underlying object with 4818 * id, value and extensions. The accessor "getUrl" gives direct access 4819 * to the value 4820 */ 4821 public UriType getUrlElement() { 4822 if (this.url == null) 4823 if (Configuration.errorOnAutoCreate()) 4824 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.url"); 4825 else if (Configuration.doAutoCreate()) 4826 this.url = new UriType(); // bb 4827 return this.url; 4828 } 4829 4830 public boolean hasUrlElement() { 4831 return this.url != null && !this.url.isEmpty(); 4832 } 4833 4834 public boolean hasUrl() { 4835 return this.url != null && !this.url.isEmpty(); 4836 } 4837 4838 /** 4839 * @param value {@link #url} (URI of an external repository which contains 4840 * further details about the genetics data.). This is the 4841 * underlying object with id, value and extensions. The accessor 4842 * "getUrl" gives direct access to the value 4843 */ 4844 public MolecularSequenceRepositoryComponent setUrlElement(UriType value) { 4845 this.url = value; 4846 return this; 4847 } 4848 4849 /** 4850 * @return URI of an external repository which contains further details about 4851 * the genetics data. 4852 */ 4853 public String getUrl() { 4854 return this.url == null ? null : this.url.getValue(); 4855 } 4856 4857 /** 4858 * @param value URI of an external repository which contains further details 4859 * about the genetics data. 4860 */ 4861 public MolecularSequenceRepositoryComponent setUrl(String value) { 4862 if (Utilities.noString(value)) 4863 this.url = null; 4864 else { 4865 if (this.url == null) 4866 this.url = new UriType(); 4867 this.url.setValue(value); 4868 } 4869 return this; 4870 } 4871 4872 /** 4873 * @return {@link #name} (URI of an external repository which contains further 4874 * details about the genetics data.). This is the underlying object with 4875 * id, value and extensions. The accessor "getName" gives direct access 4876 * to the value 4877 */ 4878 public StringType getNameElement() { 4879 if (this.name == null) 4880 if (Configuration.errorOnAutoCreate()) 4881 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.name"); 4882 else if (Configuration.doAutoCreate()) 4883 this.name = new StringType(); // bb 4884 return this.name; 4885 } 4886 4887 public boolean hasNameElement() { 4888 return this.name != null && !this.name.isEmpty(); 4889 } 4890 4891 public boolean hasName() { 4892 return this.name != null && !this.name.isEmpty(); 4893 } 4894 4895 /** 4896 * @param value {@link #name} (URI of an external repository which contains 4897 * further details about the genetics data.). This is the 4898 * underlying object with id, value and extensions. The accessor 4899 * "getName" gives direct access to the value 4900 */ 4901 public MolecularSequenceRepositoryComponent setNameElement(StringType value) { 4902 this.name = value; 4903 return this; 4904 } 4905 4906 /** 4907 * @return URI of an external repository which contains further details about 4908 * the genetics data. 4909 */ 4910 public String getName() { 4911 return this.name == null ? null : this.name.getValue(); 4912 } 4913 4914 /** 4915 * @param value URI of an external repository which contains further details 4916 * about the genetics data. 4917 */ 4918 public MolecularSequenceRepositoryComponent setName(String value) { 4919 if (Utilities.noString(value)) 4920 this.name = null; 4921 else { 4922 if (this.name == null) 4923 this.name = new StringType(); 4924 this.name.setValue(value); 4925 } 4926 return this; 4927 } 4928 4929 /** 4930 * @return {@link #datasetId} (Id of the variant in this external repository. 4931 * The server will understand how to use this id to call for more info 4932 * about datasets in external repository.). This is the underlying 4933 * object with id, value and extensions. The accessor "getDatasetId" 4934 * gives direct access to the value 4935 */ 4936 public StringType getDatasetIdElement() { 4937 if (this.datasetId == null) 4938 if (Configuration.errorOnAutoCreate()) 4939 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.datasetId"); 4940 else if (Configuration.doAutoCreate()) 4941 this.datasetId = new StringType(); // bb 4942 return this.datasetId; 4943 } 4944 4945 public boolean hasDatasetIdElement() { 4946 return this.datasetId != null && !this.datasetId.isEmpty(); 4947 } 4948 4949 public boolean hasDatasetId() { 4950 return this.datasetId != null && !this.datasetId.isEmpty(); 4951 } 4952 4953 /** 4954 * @param value {@link #datasetId} (Id of the variant in this external 4955 * repository. The server will understand how to use this id to 4956 * call for more info about datasets in external repository.). This 4957 * is the underlying object with id, value and extensions. The 4958 * accessor "getDatasetId" gives direct access to the value 4959 */ 4960 public MolecularSequenceRepositoryComponent setDatasetIdElement(StringType value) { 4961 this.datasetId = value; 4962 return this; 4963 } 4964 4965 /** 4966 * @return Id of the variant in this external repository. The server will 4967 * understand how to use this id to call for more info about datasets in 4968 * external repository. 4969 */ 4970 public String getDatasetId() { 4971 return this.datasetId == null ? null : this.datasetId.getValue(); 4972 } 4973 4974 /** 4975 * @param value Id of the variant in this external repository. The server will 4976 * understand how to use this id to call for more info about 4977 * datasets in external repository. 4978 */ 4979 public MolecularSequenceRepositoryComponent setDatasetId(String value) { 4980 if (Utilities.noString(value)) 4981 this.datasetId = null; 4982 else { 4983 if (this.datasetId == null) 4984 this.datasetId = new StringType(); 4985 this.datasetId.setValue(value); 4986 } 4987 return this; 4988 } 4989 4990 /** 4991 * @return {@link #variantsetId} (Id of the variantset in this external 4992 * repository. The server will understand how to use this id to call for 4993 * more info about variantsets in external repository.). This is the 4994 * underlying object with id, value and extensions. The accessor 4995 * "getVariantsetId" gives direct access to the value 4996 */ 4997 public StringType getVariantsetIdElement() { 4998 if (this.variantsetId == null) 4999 if (Configuration.errorOnAutoCreate()) 5000 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.variantsetId"); 5001 else if (Configuration.doAutoCreate()) 5002 this.variantsetId = new StringType(); // bb 5003 return this.variantsetId; 5004 } 5005 5006 public boolean hasVariantsetIdElement() { 5007 return this.variantsetId != null && !this.variantsetId.isEmpty(); 5008 } 5009 5010 public boolean hasVariantsetId() { 5011 return this.variantsetId != null && !this.variantsetId.isEmpty(); 5012 } 5013 5014 /** 5015 * @param value {@link #variantsetId} (Id of the variantset in this external 5016 * repository. The server will understand how to use this id to 5017 * call for more info about variantsets in external repository.). 5018 * This is the underlying object with id, value and extensions. The 5019 * accessor "getVariantsetId" gives direct access to the value 5020 */ 5021 public MolecularSequenceRepositoryComponent setVariantsetIdElement(StringType value) { 5022 this.variantsetId = value; 5023 return this; 5024 } 5025 5026 /** 5027 * @return Id of the variantset in this external repository. The server will 5028 * understand how to use this id to call for more info about variantsets 5029 * in external repository. 5030 */ 5031 public String getVariantsetId() { 5032 return this.variantsetId == null ? null : this.variantsetId.getValue(); 5033 } 5034 5035 /** 5036 * @param value Id of the variantset in this external repository. The server 5037 * will understand how to use this id to call for more info about 5038 * variantsets in external repository. 5039 */ 5040 public MolecularSequenceRepositoryComponent setVariantsetId(String value) { 5041 if (Utilities.noString(value)) 5042 this.variantsetId = null; 5043 else { 5044 if (this.variantsetId == null) 5045 this.variantsetId = new StringType(); 5046 this.variantsetId.setValue(value); 5047 } 5048 return this; 5049 } 5050 5051 /** 5052 * @return {@link #readsetId} (Id of the read in this external repository.). 5053 * This is the underlying object with id, value and extensions. The 5054 * accessor "getReadsetId" gives direct access to the value 5055 */ 5056 public StringType getReadsetIdElement() { 5057 if (this.readsetId == null) 5058 if (Configuration.errorOnAutoCreate()) 5059 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.readsetId"); 5060 else if (Configuration.doAutoCreate()) 5061 this.readsetId = new StringType(); // bb 5062 return this.readsetId; 5063 } 5064 5065 public boolean hasReadsetIdElement() { 5066 return this.readsetId != null && !this.readsetId.isEmpty(); 5067 } 5068 5069 public boolean hasReadsetId() { 5070 return this.readsetId != null && !this.readsetId.isEmpty(); 5071 } 5072 5073 /** 5074 * @param value {@link #readsetId} (Id of the read in this external 5075 * repository.). This is the underlying object with id, value and 5076 * extensions. The accessor "getReadsetId" gives direct access to 5077 * the value 5078 */ 5079 public MolecularSequenceRepositoryComponent setReadsetIdElement(StringType value) { 5080 this.readsetId = value; 5081 return this; 5082 } 5083 5084 /** 5085 * @return Id of the read in this external repository. 5086 */ 5087 public String getReadsetId() { 5088 return this.readsetId == null ? null : this.readsetId.getValue(); 5089 } 5090 5091 /** 5092 * @param value Id of the read in this external repository. 5093 */ 5094 public MolecularSequenceRepositoryComponent setReadsetId(String value) { 5095 if (Utilities.noString(value)) 5096 this.readsetId = null; 5097 else { 5098 if (this.readsetId == null) 5099 this.readsetId = new StringType(); 5100 this.readsetId.setValue(value); 5101 } 5102 return this; 5103 } 5104 5105 protected void listChildren(List<Property> children) { 5106 super.listChildren(children); 5107 children.add(new Property("type", "code", 5108 "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 5109 0, 1, type)); 5110 children.add(new Property("url", "uri", 5111 "URI of an external repository which contains further details about the genetics data.", 0, 1, url)); 5112 children.add(new Property("name", "string", 5113 "URI of an external repository which contains further details about the genetics data.", 0, 1, name)); 5114 children.add(new Property("datasetId", "string", 5115 "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 5116 0, 1, datasetId)); 5117 children.add(new Property("variantsetId", "string", 5118 "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 5119 0, 1, variantsetId)); 5120 children.add(new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, readsetId)); 5121 } 5122 5123 @Override 5124 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5125 switch (_hash) { 5126 case 3575610: 5127 /* type */ return new Property("type", "code", 5128 "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 5129 0, 1, type); 5130 case 116079: 5131 /* url */ return new Property("url", "uri", 5132 "URI of an external repository which contains further details about the genetics data.", 0, 1, url); 5133 case 3373707: 5134 /* name */ return new Property("name", "string", 5135 "URI of an external repository which contains further details about the genetics data.", 0, 1, name); 5136 case -345342029: 5137 /* datasetId */ return new Property("datasetId", "string", 5138 "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 5139 0, 1, datasetId); 5140 case 1929752504: 5141 /* variantsetId */ return new Property("variantsetId", "string", 5142 "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 5143 0, 1, variantsetId); 5144 case -1095407289: 5145 /* readsetId */ return new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, 5146 readsetId); 5147 default: 5148 return super.getNamedProperty(_hash, _name, _checkValid); 5149 } 5150 5151 } 5152 5153 @Override 5154 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5155 switch (hash) { 5156 case 3575610: 5157 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<RepositoryType> 5158 case 116079: 5159 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5160 case 3373707: 5161 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5162 case -345342029: 5163 /* datasetId */ return this.datasetId == null ? new Base[0] : new Base[] { this.datasetId }; // StringType 5164 case 1929752504: 5165 /* variantsetId */ return this.variantsetId == null ? new Base[0] : new Base[] { this.variantsetId }; // StringType 5166 case -1095407289: 5167 /* readsetId */ return this.readsetId == null ? new Base[0] : new Base[] { this.readsetId }; // StringType 5168 default: 5169 return super.getProperty(hash, name, checkValid); 5170 } 5171 5172 } 5173 5174 @Override 5175 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5176 switch (hash) { 5177 case 3575610: // type 5178 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 5179 this.type = (Enumeration) value; // Enumeration<RepositoryType> 5180 return value; 5181 case 116079: // url 5182 this.url = castToUri(value); // UriType 5183 return value; 5184 case 3373707: // name 5185 this.name = castToString(value); // StringType 5186 return value; 5187 case -345342029: // datasetId 5188 this.datasetId = castToString(value); // StringType 5189 return value; 5190 case 1929752504: // variantsetId 5191 this.variantsetId = castToString(value); // StringType 5192 return value; 5193 case -1095407289: // readsetId 5194 this.readsetId = castToString(value); // StringType 5195 return value; 5196 default: 5197 return super.setProperty(hash, name, value); 5198 } 5199 5200 } 5201 5202 @Override 5203 public Base setProperty(String name, Base value) throws FHIRException { 5204 if (name.equals("type")) { 5205 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 5206 this.type = (Enumeration) value; // Enumeration<RepositoryType> 5207 } else if (name.equals("url")) { 5208 this.url = castToUri(value); // UriType 5209 } else if (name.equals("name")) { 5210 this.name = castToString(value); // StringType 5211 } else if (name.equals("datasetId")) { 5212 this.datasetId = castToString(value); // StringType 5213 } else if (name.equals("variantsetId")) { 5214 this.variantsetId = castToString(value); // StringType 5215 } else if (name.equals("readsetId")) { 5216 this.readsetId = castToString(value); // StringType 5217 } else 5218 return super.setProperty(name, value); 5219 return value; 5220 } 5221 5222 @Override 5223 public Base makeProperty(int hash, String name) throws FHIRException { 5224 switch (hash) { 5225 case 3575610: 5226 return getTypeElement(); 5227 case 116079: 5228 return getUrlElement(); 5229 case 3373707: 5230 return getNameElement(); 5231 case -345342029: 5232 return getDatasetIdElement(); 5233 case 1929752504: 5234 return getVariantsetIdElement(); 5235 case -1095407289: 5236 return getReadsetIdElement(); 5237 default: 5238 return super.makeProperty(hash, name); 5239 } 5240 5241 } 5242 5243 @Override 5244 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5245 switch (hash) { 5246 case 3575610: 5247 /* type */ return new String[] { "code" }; 5248 case 116079: 5249 /* url */ return new String[] { "uri" }; 5250 case 3373707: 5251 /* name */ return new String[] { "string" }; 5252 case -345342029: 5253 /* datasetId */ return new String[] { "string" }; 5254 case 1929752504: 5255 /* variantsetId */ return new String[] { "string" }; 5256 case -1095407289: 5257 /* readsetId */ return new String[] { "string" }; 5258 default: 5259 return super.getTypesForProperty(hash, name); 5260 } 5261 5262 } 5263 5264 @Override 5265 public Base addChild(String name) throws FHIRException { 5266 if (name.equals("type")) { 5267 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 5268 } else if (name.equals("url")) { 5269 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.url"); 5270 } else if (name.equals("name")) { 5271 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.name"); 5272 } else if (name.equals("datasetId")) { 5273 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.datasetId"); 5274 } else if (name.equals("variantsetId")) { 5275 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.variantsetId"); 5276 } else if (name.equals("readsetId")) { 5277 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.readsetId"); 5278 } else 5279 return super.addChild(name); 5280 } 5281 5282 public MolecularSequenceRepositoryComponent copy() { 5283 MolecularSequenceRepositoryComponent dst = new MolecularSequenceRepositoryComponent(); 5284 copyValues(dst); 5285 return dst; 5286 } 5287 5288 public void copyValues(MolecularSequenceRepositoryComponent dst) { 5289 super.copyValues(dst); 5290 dst.type = type == null ? null : type.copy(); 5291 dst.url = url == null ? null : url.copy(); 5292 dst.name = name == null ? null : name.copy(); 5293 dst.datasetId = datasetId == null ? null : datasetId.copy(); 5294 dst.variantsetId = variantsetId == null ? null : variantsetId.copy(); 5295 dst.readsetId = readsetId == null ? null : readsetId.copy(); 5296 } 5297 5298 @Override 5299 public boolean equalsDeep(Base other_) { 5300 if (!super.equalsDeep(other_)) 5301 return false; 5302 if (!(other_ instanceof MolecularSequenceRepositoryComponent)) 5303 return false; 5304 MolecularSequenceRepositoryComponent o = (MolecularSequenceRepositoryComponent) other_; 5305 return compareDeep(type, o.type, true) && compareDeep(url, o.url, true) && compareDeep(name, o.name, true) 5306 && compareDeep(datasetId, o.datasetId, true) && compareDeep(variantsetId, o.variantsetId, true) 5307 && compareDeep(readsetId, o.readsetId, true); 5308 } 5309 5310 @Override 5311 public boolean equalsShallow(Base other_) { 5312 if (!super.equalsShallow(other_)) 5313 return false; 5314 if (!(other_ instanceof MolecularSequenceRepositoryComponent)) 5315 return false; 5316 MolecularSequenceRepositoryComponent o = (MolecularSequenceRepositoryComponent) other_; 5317 return compareValues(type, o.type, true) && compareValues(url, o.url, true) && compareValues(name, o.name, true) 5318 && compareValues(datasetId, o.datasetId, true) && compareValues(variantsetId, o.variantsetId, true) 5319 && compareValues(readsetId, o.readsetId, true); 5320 } 5321 5322 public boolean isEmpty() { 5323 return super.isEmpty() 5324 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, url, name, datasetId, variantsetId, readsetId); 5325 } 5326 5327 public String fhirType() { 5328 return "MolecularSequence.repository"; 5329 5330 } 5331 5332 } 5333 5334 @Block() 5335 public static class MolecularSequenceStructureVariantComponent extends BackboneElement 5336 implements IBaseBackboneElement { 5337 /** 5338 * Information about chromosome structure variation DNA change type. 5339 */ 5340 @Child(name = "variantType", type = { 5341 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 5342 @Description(shortDefinition = "Structural variant change type", formalDefinition = "Information about chromosome structure variation DNA change type.") 5343 protected CodeableConcept variantType; 5344 5345 /** 5346 * Used to indicate if the outer and inner start-end values have the same 5347 * meaning. 5348 */ 5349 @Child(name = "exact", type = { BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5350 @Description(shortDefinition = "Does the structural variant have base pair resolution breakpoints?", formalDefinition = "Used to indicate if the outer and inner start-end values have the same meaning.") 5351 protected BooleanType exact; 5352 5353 /** 5354 * Length of the variant chromosome. 5355 */ 5356 @Child(name = "length", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 5357 @Description(shortDefinition = "Structural variant length", formalDefinition = "Length of the variant chromosome.") 5358 protected IntegerType length; 5359 5360 /** 5361 * Structural variant outer. 5362 */ 5363 @Child(name = "outer", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = true) 5364 @Description(shortDefinition = "Structural variant outer", formalDefinition = "Structural variant outer.") 5365 protected MolecularSequenceStructureVariantOuterComponent outer; 5366 5367 /** 5368 * Structural variant inner. 5369 */ 5370 @Child(name = "inner", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 5371 @Description(shortDefinition = "Structural variant inner", formalDefinition = "Structural variant inner.") 5372 protected MolecularSequenceStructureVariantInnerComponent inner; 5373 5374 private static final long serialVersionUID = -1943515207L; 5375 5376 /** 5377 * Constructor 5378 */ 5379 public MolecularSequenceStructureVariantComponent() { 5380 super(); 5381 } 5382 5383 /** 5384 * @return {@link #variantType} (Information about chromosome structure 5385 * variation DNA change type.) 5386 */ 5387 public CodeableConcept getVariantType() { 5388 if (this.variantType == null) 5389 if (Configuration.errorOnAutoCreate()) 5390 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.variantType"); 5391 else if (Configuration.doAutoCreate()) 5392 this.variantType = new CodeableConcept(); // cc 5393 return this.variantType; 5394 } 5395 5396 public boolean hasVariantType() { 5397 return this.variantType != null && !this.variantType.isEmpty(); 5398 } 5399 5400 /** 5401 * @param value {@link #variantType} (Information about chromosome structure 5402 * variation DNA change type.) 5403 */ 5404 public MolecularSequenceStructureVariantComponent setVariantType(CodeableConcept value) { 5405 this.variantType = value; 5406 return this; 5407 } 5408 5409 /** 5410 * @return {@link #exact} (Used to indicate if the outer and inner start-end 5411 * values have the same meaning.). This is the underlying object with 5412 * id, value and extensions. The accessor "getExact" gives direct access 5413 * to the value 5414 */ 5415 public BooleanType getExactElement() { 5416 if (this.exact == null) 5417 if (Configuration.errorOnAutoCreate()) 5418 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.exact"); 5419 else if (Configuration.doAutoCreate()) 5420 this.exact = new BooleanType(); // bb 5421 return this.exact; 5422 } 5423 5424 public boolean hasExactElement() { 5425 return this.exact != null && !this.exact.isEmpty(); 5426 } 5427 5428 public boolean hasExact() { 5429 return this.exact != null && !this.exact.isEmpty(); 5430 } 5431 5432 /** 5433 * @param value {@link #exact} (Used to indicate if the outer and inner 5434 * start-end values have the same meaning.). This is the underlying 5435 * object with id, value and extensions. The accessor "getExact" 5436 * gives direct access to the value 5437 */ 5438 public MolecularSequenceStructureVariantComponent setExactElement(BooleanType value) { 5439 this.exact = value; 5440 return this; 5441 } 5442 5443 /** 5444 * @return Used to indicate if the outer and inner start-end values have the 5445 * same meaning. 5446 */ 5447 public boolean getExact() { 5448 return this.exact == null || this.exact.isEmpty() ? false : this.exact.getValue(); 5449 } 5450 5451 /** 5452 * @param value Used to indicate if the outer and inner start-end values have 5453 * the same meaning. 5454 */ 5455 public MolecularSequenceStructureVariantComponent setExact(boolean value) { 5456 if (this.exact == null) 5457 this.exact = new BooleanType(); 5458 this.exact.setValue(value); 5459 return this; 5460 } 5461 5462 /** 5463 * @return {@link #length} (Length of the variant chromosome.). This is the 5464 * underlying object with id, value and extensions. The accessor 5465 * "getLength" gives direct access to the value 5466 */ 5467 public IntegerType getLengthElement() { 5468 if (this.length == null) 5469 if (Configuration.errorOnAutoCreate()) 5470 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.length"); 5471 else if (Configuration.doAutoCreate()) 5472 this.length = new IntegerType(); // bb 5473 return this.length; 5474 } 5475 5476 public boolean hasLengthElement() { 5477 return this.length != null && !this.length.isEmpty(); 5478 } 5479 5480 public boolean hasLength() { 5481 return this.length != null && !this.length.isEmpty(); 5482 } 5483 5484 /** 5485 * @param value {@link #length} (Length of the variant chromosome.). This is the 5486 * underlying object with id, value and extensions. The accessor 5487 * "getLength" gives direct access to the value 5488 */ 5489 public MolecularSequenceStructureVariantComponent setLengthElement(IntegerType value) { 5490 this.length = value; 5491 return this; 5492 } 5493 5494 /** 5495 * @return Length of the variant chromosome. 5496 */ 5497 public int getLength() { 5498 return this.length == null || this.length.isEmpty() ? 0 : this.length.getValue(); 5499 } 5500 5501 /** 5502 * @param value Length of the variant chromosome. 5503 */ 5504 public MolecularSequenceStructureVariantComponent setLength(int value) { 5505 if (this.length == null) 5506 this.length = new IntegerType(); 5507 this.length.setValue(value); 5508 return this; 5509 } 5510 5511 /** 5512 * @return {@link #outer} (Structural variant outer.) 5513 */ 5514 public MolecularSequenceStructureVariantOuterComponent getOuter() { 5515 if (this.outer == null) 5516 if (Configuration.errorOnAutoCreate()) 5517 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.outer"); 5518 else if (Configuration.doAutoCreate()) 5519 this.outer = new MolecularSequenceStructureVariantOuterComponent(); // cc 5520 return this.outer; 5521 } 5522 5523 public boolean hasOuter() { 5524 return this.outer != null && !this.outer.isEmpty(); 5525 } 5526 5527 /** 5528 * @param value {@link #outer} (Structural variant outer.) 5529 */ 5530 public MolecularSequenceStructureVariantComponent setOuter(MolecularSequenceStructureVariantOuterComponent value) { 5531 this.outer = value; 5532 return this; 5533 } 5534 5535 /** 5536 * @return {@link #inner} (Structural variant inner.) 5537 */ 5538 public MolecularSequenceStructureVariantInnerComponent getInner() { 5539 if (this.inner == null) 5540 if (Configuration.errorOnAutoCreate()) 5541 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.inner"); 5542 else if (Configuration.doAutoCreate()) 5543 this.inner = new MolecularSequenceStructureVariantInnerComponent(); // cc 5544 return this.inner; 5545 } 5546 5547 public boolean hasInner() { 5548 return this.inner != null && !this.inner.isEmpty(); 5549 } 5550 5551 /** 5552 * @param value {@link #inner} (Structural variant inner.) 5553 */ 5554 public MolecularSequenceStructureVariantComponent setInner(MolecularSequenceStructureVariantInnerComponent value) { 5555 this.inner = value; 5556 return this; 5557 } 5558 5559 protected void listChildren(List<Property> children) { 5560 super.listChildren(children); 5561 children.add(new Property("variantType", "CodeableConcept", 5562 "Information about chromosome structure variation DNA change type.", 0, 1, variantType)); 5563 children.add(new Property("exact", "boolean", 5564 "Used to indicate if the outer and inner start-end values have the same meaning.", 0, 1, exact)); 5565 children.add(new Property("length", "integer", "Length of the variant chromosome.", 0, 1, length)); 5566 children.add(new Property("outer", "", "Structural variant outer.", 0, 1, outer)); 5567 children.add(new Property("inner", "", "Structural variant inner.", 0, 1, inner)); 5568 } 5569 5570 @Override 5571 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5572 switch (_hash) { 5573 case -1601222305: 5574 /* variantType */ return new Property("variantType", "CodeableConcept", 5575 "Information about chromosome structure variation DNA change type.", 0, 1, variantType); 5576 case 96946943: 5577 /* exact */ return new Property("exact", "boolean", 5578 "Used to indicate if the outer and inner start-end values have the same meaning.", 0, 1, exact); 5579 case -1106363674: 5580 /* length */ return new Property("length", "integer", "Length of the variant chromosome.", 0, 1, length); 5581 case 106111099: 5582 /* outer */ return new Property("outer", "", "Structural variant outer.", 0, 1, outer); 5583 case 100355670: 5584 /* inner */ return new Property("inner", "", "Structural variant inner.", 0, 1, inner); 5585 default: 5586 return super.getNamedProperty(_hash, _name, _checkValid); 5587 } 5588 5589 } 5590 5591 @Override 5592 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5593 switch (hash) { 5594 case -1601222305: 5595 /* variantType */ return this.variantType == null ? new Base[0] : new Base[] { this.variantType }; // CodeableConcept 5596 case 96946943: 5597 /* exact */ return this.exact == null ? new Base[0] : new Base[] { this.exact }; // BooleanType 5598 case -1106363674: 5599 /* length */ return this.length == null ? new Base[0] : new Base[] { this.length }; // IntegerType 5600 case 106111099: 5601 /* outer */ return this.outer == null ? new Base[0] : new Base[] { this.outer }; // MolecularSequenceStructureVariantOuterComponent 5602 case 100355670: 5603 /* inner */ return this.inner == null ? new Base[0] : new Base[] { this.inner }; // MolecularSequenceStructureVariantInnerComponent 5604 default: 5605 return super.getProperty(hash, name, checkValid); 5606 } 5607 5608 } 5609 5610 @Override 5611 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5612 switch (hash) { 5613 case -1601222305: // variantType 5614 this.variantType = castToCodeableConcept(value); // CodeableConcept 5615 return value; 5616 case 96946943: // exact 5617 this.exact = castToBoolean(value); // BooleanType 5618 return value; 5619 case -1106363674: // length 5620 this.length = castToInteger(value); // IntegerType 5621 return value; 5622 case 106111099: // outer 5623 this.outer = (MolecularSequenceStructureVariantOuterComponent) value; // MolecularSequenceStructureVariantOuterComponent 5624 return value; 5625 case 100355670: // inner 5626 this.inner = (MolecularSequenceStructureVariantInnerComponent) value; // MolecularSequenceStructureVariantInnerComponent 5627 return value; 5628 default: 5629 return super.setProperty(hash, name, value); 5630 } 5631 5632 } 5633 5634 @Override 5635 public Base setProperty(String name, Base value) throws FHIRException { 5636 if (name.equals("variantType")) { 5637 this.variantType = castToCodeableConcept(value); // CodeableConcept 5638 } else if (name.equals("exact")) { 5639 this.exact = castToBoolean(value); // BooleanType 5640 } else if (name.equals("length")) { 5641 this.length = castToInteger(value); // IntegerType 5642 } else if (name.equals("outer")) { 5643 this.outer = (MolecularSequenceStructureVariantOuterComponent) value; // MolecularSequenceStructureVariantOuterComponent 5644 } else if (name.equals("inner")) { 5645 this.inner = (MolecularSequenceStructureVariantInnerComponent) value; // MolecularSequenceStructureVariantInnerComponent 5646 } else 5647 return super.setProperty(name, value); 5648 return value; 5649 } 5650 5651 @Override 5652 public Base makeProperty(int hash, String name) throws FHIRException { 5653 switch (hash) { 5654 case -1601222305: 5655 return getVariantType(); 5656 case 96946943: 5657 return getExactElement(); 5658 case -1106363674: 5659 return getLengthElement(); 5660 case 106111099: 5661 return getOuter(); 5662 case 100355670: 5663 return getInner(); 5664 default: 5665 return super.makeProperty(hash, name); 5666 } 5667 5668 } 5669 5670 @Override 5671 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5672 switch (hash) { 5673 case -1601222305: 5674 /* variantType */ return new String[] { "CodeableConcept" }; 5675 case 96946943: 5676 /* exact */ return new String[] { "boolean" }; 5677 case -1106363674: 5678 /* length */ return new String[] { "integer" }; 5679 case 106111099: 5680 /* outer */ return new String[] {}; 5681 case 100355670: 5682 /* inner */ return new String[] {}; 5683 default: 5684 return super.getTypesForProperty(hash, name); 5685 } 5686 5687 } 5688 5689 @Override 5690 public Base addChild(String name) throws FHIRException { 5691 if (name.equals("variantType")) { 5692 this.variantType = new CodeableConcept(); 5693 return this.variantType; 5694 } else if (name.equals("exact")) { 5695 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.exact"); 5696 } else if (name.equals("length")) { 5697 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.length"); 5698 } else if (name.equals("outer")) { 5699 this.outer = new MolecularSequenceStructureVariantOuterComponent(); 5700 return this.outer; 5701 } else if (name.equals("inner")) { 5702 this.inner = new MolecularSequenceStructureVariantInnerComponent(); 5703 return this.inner; 5704 } else 5705 return super.addChild(name); 5706 } 5707 5708 public MolecularSequenceStructureVariantComponent copy() { 5709 MolecularSequenceStructureVariantComponent dst = new MolecularSequenceStructureVariantComponent(); 5710 copyValues(dst); 5711 return dst; 5712 } 5713 5714 public void copyValues(MolecularSequenceStructureVariantComponent dst) { 5715 super.copyValues(dst); 5716 dst.variantType = variantType == null ? null : variantType.copy(); 5717 dst.exact = exact == null ? null : exact.copy(); 5718 dst.length = length == null ? null : length.copy(); 5719 dst.outer = outer == null ? null : outer.copy(); 5720 dst.inner = inner == null ? null : inner.copy(); 5721 } 5722 5723 @Override 5724 public boolean equalsDeep(Base other_) { 5725 if (!super.equalsDeep(other_)) 5726 return false; 5727 if (!(other_ instanceof MolecularSequenceStructureVariantComponent)) 5728 return false; 5729 MolecularSequenceStructureVariantComponent o = (MolecularSequenceStructureVariantComponent) other_; 5730 return compareDeep(variantType, o.variantType, true) && compareDeep(exact, o.exact, true) 5731 && compareDeep(length, o.length, true) && compareDeep(outer, o.outer, true) 5732 && compareDeep(inner, o.inner, true); 5733 } 5734 5735 @Override 5736 public boolean equalsShallow(Base other_) { 5737 if (!super.equalsShallow(other_)) 5738 return false; 5739 if (!(other_ instanceof MolecularSequenceStructureVariantComponent)) 5740 return false; 5741 MolecularSequenceStructureVariantComponent o = (MolecularSequenceStructureVariantComponent) other_; 5742 return compareValues(exact, o.exact, true) && compareValues(length, o.length, true); 5743 } 5744 5745 public boolean isEmpty() { 5746 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(variantType, exact, length, outer, inner); 5747 } 5748 5749 public String fhirType() { 5750 return "MolecularSequence.structureVariant"; 5751 5752 } 5753 5754 } 5755 5756 @Block() 5757 public static class MolecularSequenceStructureVariantOuterComponent extends BackboneElement 5758 implements IBaseBackboneElement { 5759 /** 5760 * Structural variant outer start. If the coordinate system is either 0-based or 5761 * 1-based, then start position is inclusive. 5762 */ 5763 @Child(name = "start", type = { IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 5764 @Description(shortDefinition = "Structural variant outer start", formalDefinition = "Structural variant outer start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 5765 protected IntegerType start; 5766 5767 /** 5768 * Structural variant outer end. If the coordinate system is 0-based then end is 5769 * exclusive and does not include the last position. If the coordinate system is 5770 * 1-base, then end is inclusive and includes the last position. 5771 */ 5772 @Child(name = "end", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5773 @Description(shortDefinition = "Structural variant outer end", formalDefinition = "Structural variant outer end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 5774 protected IntegerType end; 5775 5776 private static final long serialVersionUID = -1798864889L; 5777 5778 /** 5779 * Constructor 5780 */ 5781 public MolecularSequenceStructureVariantOuterComponent() { 5782 super(); 5783 } 5784 5785 /** 5786 * @return {@link #start} (Structural variant outer start. If the coordinate 5787 * system is either 0-based or 1-based, then start position is 5788 * inclusive.). This is the underlying object with id, value and 5789 * extensions. The accessor "getStart" gives direct access to the value 5790 */ 5791 public IntegerType getStartElement() { 5792 if (this.start == null) 5793 if (Configuration.errorOnAutoCreate()) 5794 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantOuterComponent.start"); 5795 else if (Configuration.doAutoCreate()) 5796 this.start = new IntegerType(); // bb 5797 return this.start; 5798 } 5799 5800 public boolean hasStartElement() { 5801 return this.start != null && !this.start.isEmpty(); 5802 } 5803 5804 public boolean hasStart() { 5805 return this.start != null && !this.start.isEmpty(); 5806 } 5807 5808 /** 5809 * @param value {@link #start} (Structural variant outer start. If the 5810 * coordinate system is either 0-based or 1-based, then start 5811 * position is inclusive.). This is the underlying object with id, 5812 * value and extensions. The accessor "getStart" gives direct 5813 * access to the value 5814 */ 5815 public MolecularSequenceStructureVariantOuterComponent setStartElement(IntegerType value) { 5816 this.start = value; 5817 return this; 5818 } 5819 5820 /** 5821 * @return Structural variant outer start. If the coordinate system is either 5822 * 0-based or 1-based, then start position is inclusive. 5823 */ 5824 public int getStart() { 5825 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 5826 } 5827 5828 /** 5829 * @param value Structural variant outer start. If the coordinate system is 5830 * either 0-based or 1-based, then start position is inclusive. 5831 */ 5832 public MolecularSequenceStructureVariantOuterComponent setStart(int value) { 5833 if (this.start == null) 5834 this.start = new IntegerType(); 5835 this.start.setValue(value); 5836 return this; 5837 } 5838 5839 /** 5840 * @return {@link #end} (Structural variant outer end. If the coordinate system 5841 * is 0-based then end is exclusive and does not include the last 5842 * position. If the coordinate system is 1-base, then end is inclusive 5843 * and includes the last position.). This is the underlying object with 5844 * id, value and extensions. The accessor "getEnd" gives direct access 5845 * to the value 5846 */ 5847 public IntegerType getEndElement() { 5848 if (this.end == null) 5849 if (Configuration.errorOnAutoCreate()) 5850 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantOuterComponent.end"); 5851 else if (Configuration.doAutoCreate()) 5852 this.end = new IntegerType(); // bb 5853 return this.end; 5854 } 5855 5856 public boolean hasEndElement() { 5857 return this.end != null && !this.end.isEmpty(); 5858 } 5859 5860 public boolean hasEnd() { 5861 return this.end != null && !this.end.isEmpty(); 5862 } 5863 5864 /** 5865 * @param value {@link #end} (Structural variant outer end. If the coordinate 5866 * system is 0-based then end is exclusive and does not include the 5867 * last position. If the coordinate system is 1-base, then end is 5868 * inclusive and includes the last position.). This is the 5869 * underlying object with id, value and extensions. The accessor 5870 * "getEnd" gives direct access to the value 5871 */ 5872 public MolecularSequenceStructureVariantOuterComponent setEndElement(IntegerType value) { 5873 this.end = value; 5874 return this; 5875 } 5876 5877 /** 5878 * @return Structural variant outer end. If the coordinate system is 0-based 5879 * then end is exclusive and does not include the last position. If the 5880 * coordinate system is 1-base, then end is inclusive and includes the 5881 * last position. 5882 */ 5883 public int getEnd() { 5884 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 5885 } 5886 5887 /** 5888 * @param value Structural variant outer end. If the coordinate system is 5889 * 0-based then end is exclusive and does not include the last 5890 * position. If the coordinate system is 1-base, then end is 5891 * inclusive and includes the last position. 5892 */ 5893 public MolecularSequenceStructureVariantOuterComponent setEnd(int value) { 5894 if (this.end == null) 5895 this.end = new IntegerType(); 5896 this.end.setValue(value); 5897 return this; 5898 } 5899 5900 protected void listChildren(List<Property> children) { 5901 super.listChildren(children); 5902 children.add(new Property("start", "integer", 5903 "Structural variant outer start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 5904 0, 1, start)); 5905 children.add(new Property("end", "integer", 5906 "Structural variant outer end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 5907 0, 1, end)); 5908 } 5909 5910 @Override 5911 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5912 switch (_hash) { 5913 case 109757538: 5914 /* start */ return new Property("start", "integer", 5915 "Structural variant outer start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 5916 0, 1, start); 5917 case 100571: 5918 /* end */ return new Property("end", "integer", 5919 "Structural variant outer end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 5920 0, 1, end); 5921 default: 5922 return super.getNamedProperty(_hash, _name, _checkValid); 5923 } 5924 5925 } 5926 5927 @Override 5928 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5929 switch (hash) { 5930 case 109757538: 5931 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 5932 case 100571: 5933 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 5934 default: 5935 return super.getProperty(hash, name, checkValid); 5936 } 5937 5938 } 5939 5940 @Override 5941 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5942 switch (hash) { 5943 case 109757538: // start 5944 this.start = castToInteger(value); // IntegerType 5945 return value; 5946 case 100571: // end 5947 this.end = castToInteger(value); // IntegerType 5948 return value; 5949 default: 5950 return super.setProperty(hash, name, value); 5951 } 5952 5953 } 5954 5955 @Override 5956 public Base setProperty(String name, Base value) throws FHIRException { 5957 if (name.equals("start")) { 5958 this.start = castToInteger(value); // IntegerType 5959 } else if (name.equals("end")) { 5960 this.end = castToInteger(value); // IntegerType 5961 } else 5962 return super.setProperty(name, value); 5963 return value; 5964 } 5965 5966 @Override 5967 public Base makeProperty(int hash, String name) throws FHIRException { 5968 switch (hash) { 5969 case 109757538: 5970 return getStartElement(); 5971 case 100571: 5972 return getEndElement(); 5973 default: 5974 return super.makeProperty(hash, name); 5975 } 5976 5977 } 5978 5979 @Override 5980 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5981 switch (hash) { 5982 case 109757538: 5983 /* start */ return new String[] { "integer" }; 5984 case 100571: 5985 /* end */ return new String[] { "integer" }; 5986 default: 5987 return super.getTypesForProperty(hash, name); 5988 } 5989 5990 } 5991 5992 @Override 5993 public Base addChild(String name) throws FHIRException { 5994 if (name.equals("start")) { 5995 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 5996 } else if (name.equals("end")) { 5997 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 5998 } else 5999 return super.addChild(name); 6000 } 6001 6002 public MolecularSequenceStructureVariantOuterComponent copy() { 6003 MolecularSequenceStructureVariantOuterComponent dst = new MolecularSequenceStructureVariantOuterComponent(); 6004 copyValues(dst); 6005 return dst; 6006 } 6007 6008 public void copyValues(MolecularSequenceStructureVariantOuterComponent dst) { 6009 super.copyValues(dst); 6010 dst.start = start == null ? null : start.copy(); 6011 dst.end = end == null ? null : end.copy(); 6012 } 6013 6014 @Override 6015 public boolean equalsDeep(Base other_) { 6016 if (!super.equalsDeep(other_)) 6017 return false; 6018 if (!(other_ instanceof MolecularSequenceStructureVariantOuterComponent)) 6019 return false; 6020 MolecularSequenceStructureVariantOuterComponent o = (MolecularSequenceStructureVariantOuterComponent) other_; 6021 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 6022 } 6023 6024 @Override 6025 public boolean equalsShallow(Base other_) { 6026 if (!super.equalsShallow(other_)) 6027 return false; 6028 if (!(other_ instanceof MolecularSequenceStructureVariantOuterComponent)) 6029 return false; 6030 MolecularSequenceStructureVariantOuterComponent o = (MolecularSequenceStructureVariantOuterComponent) other_; 6031 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 6032 } 6033 6034 public boolean isEmpty() { 6035 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 6036 } 6037 6038 public String fhirType() { 6039 return "MolecularSequence.structureVariant.outer"; 6040 6041 } 6042 6043 } 6044 6045 @Block() 6046 public static class MolecularSequenceStructureVariantInnerComponent extends BackboneElement 6047 implements IBaseBackboneElement { 6048 /** 6049 * Structural variant inner start. If the coordinate system is either 0-based or 6050 * 1-based, then start position is inclusive. 6051 */ 6052 @Child(name = "start", type = { IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 6053 @Description(shortDefinition = "Structural variant inner start", formalDefinition = "Structural variant inner start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 6054 protected IntegerType start; 6055 6056 /** 6057 * Structural variant inner end. If the coordinate system is 0-based then end is 6058 * exclusive and does not include the last position. If the coordinate system is 6059 * 1-base, then end is inclusive and includes the last position. 6060 */ 6061 @Child(name = "end", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 6062 @Description(shortDefinition = "Structural variant inner end", formalDefinition = "Structural variant inner end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 6063 protected IntegerType end; 6064 6065 private static final long serialVersionUID = -1798864889L; 6066 6067 /** 6068 * Constructor 6069 */ 6070 public MolecularSequenceStructureVariantInnerComponent() { 6071 super(); 6072 } 6073 6074 /** 6075 * @return {@link #start} (Structural variant inner start. If the coordinate 6076 * system is either 0-based or 1-based, then start position is 6077 * inclusive.). This is the underlying object with id, value and 6078 * extensions. The accessor "getStart" gives direct access to the value 6079 */ 6080 public IntegerType getStartElement() { 6081 if (this.start == null) 6082 if (Configuration.errorOnAutoCreate()) 6083 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantInnerComponent.start"); 6084 else if (Configuration.doAutoCreate()) 6085 this.start = new IntegerType(); // bb 6086 return this.start; 6087 } 6088 6089 public boolean hasStartElement() { 6090 return this.start != null && !this.start.isEmpty(); 6091 } 6092 6093 public boolean hasStart() { 6094 return this.start != null && !this.start.isEmpty(); 6095 } 6096 6097 /** 6098 * @param value {@link #start} (Structural variant inner start. If the 6099 * coordinate system is either 0-based or 1-based, then start 6100 * position is inclusive.). This is the underlying object with id, 6101 * value and extensions. The accessor "getStart" gives direct 6102 * access to the value 6103 */ 6104 public MolecularSequenceStructureVariantInnerComponent setStartElement(IntegerType value) { 6105 this.start = value; 6106 return this; 6107 } 6108 6109 /** 6110 * @return Structural variant inner start. If the coordinate system is either 6111 * 0-based or 1-based, then start position is inclusive. 6112 */ 6113 public int getStart() { 6114 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 6115 } 6116 6117 /** 6118 * @param value Structural variant inner start. If the coordinate system is 6119 * either 0-based or 1-based, then start position is inclusive. 6120 */ 6121 public MolecularSequenceStructureVariantInnerComponent setStart(int value) { 6122 if (this.start == null) 6123 this.start = new IntegerType(); 6124 this.start.setValue(value); 6125 return this; 6126 } 6127 6128 /** 6129 * @return {@link #end} (Structural variant inner end. If the coordinate system 6130 * is 0-based then end is exclusive and does not include the last 6131 * position. If the coordinate system is 1-base, then end is inclusive 6132 * and includes the last position.). This is the underlying object with 6133 * id, value and extensions. The accessor "getEnd" gives direct access 6134 * to the value 6135 */ 6136 public IntegerType getEndElement() { 6137 if (this.end == null) 6138 if (Configuration.errorOnAutoCreate()) 6139 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantInnerComponent.end"); 6140 else if (Configuration.doAutoCreate()) 6141 this.end = new IntegerType(); // bb 6142 return this.end; 6143 } 6144 6145 public boolean hasEndElement() { 6146 return this.end != null && !this.end.isEmpty(); 6147 } 6148 6149 public boolean hasEnd() { 6150 return this.end != null && !this.end.isEmpty(); 6151 } 6152 6153 /** 6154 * @param value {@link #end} (Structural variant inner end. If the coordinate 6155 * system is 0-based then end is exclusive and does not include the 6156 * last position. If the coordinate system is 1-base, then end is 6157 * inclusive and includes the last position.). This is the 6158 * underlying object with id, value and extensions. The accessor 6159 * "getEnd" gives direct access to the value 6160 */ 6161 public MolecularSequenceStructureVariantInnerComponent setEndElement(IntegerType value) { 6162 this.end = value; 6163 return this; 6164 } 6165 6166 /** 6167 * @return Structural variant inner end. If the coordinate system is 0-based 6168 * then end is exclusive and does not include the last position. If the 6169 * coordinate system is 1-base, then end is inclusive and includes the 6170 * last position. 6171 */ 6172 public int getEnd() { 6173 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 6174 } 6175 6176 /** 6177 * @param value Structural variant inner end. If the coordinate system is 6178 * 0-based then end is exclusive and does not include the last 6179 * position. If the coordinate system is 1-base, then end is 6180 * inclusive and includes the last position. 6181 */ 6182 public MolecularSequenceStructureVariantInnerComponent setEnd(int value) { 6183 if (this.end == null) 6184 this.end = new IntegerType(); 6185 this.end.setValue(value); 6186 return this; 6187 } 6188 6189 protected void listChildren(List<Property> children) { 6190 super.listChildren(children); 6191 children.add(new Property("start", "integer", 6192 "Structural variant inner start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 6193 0, 1, start)); 6194 children.add(new Property("end", "integer", 6195 "Structural variant inner end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 6196 0, 1, end)); 6197 } 6198 6199 @Override 6200 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6201 switch (_hash) { 6202 case 109757538: 6203 /* start */ return new Property("start", "integer", 6204 "Structural variant inner start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 6205 0, 1, start); 6206 case 100571: 6207 /* end */ return new Property("end", "integer", 6208 "Structural variant inner end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 6209 0, 1, end); 6210 default: 6211 return super.getNamedProperty(_hash, _name, _checkValid); 6212 } 6213 6214 } 6215 6216 @Override 6217 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6218 switch (hash) { 6219 case 109757538: 6220 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 6221 case 100571: 6222 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 6223 default: 6224 return super.getProperty(hash, name, checkValid); 6225 } 6226 6227 } 6228 6229 @Override 6230 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6231 switch (hash) { 6232 case 109757538: // start 6233 this.start = castToInteger(value); // IntegerType 6234 return value; 6235 case 100571: // end 6236 this.end = castToInteger(value); // IntegerType 6237 return value; 6238 default: 6239 return super.setProperty(hash, name, value); 6240 } 6241 6242 } 6243 6244 @Override 6245 public Base setProperty(String name, Base value) throws FHIRException { 6246 if (name.equals("start")) { 6247 this.start = castToInteger(value); // IntegerType 6248 } else if (name.equals("end")) { 6249 this.end = castToInteger(value); // IntegerType 6250 } else 6251 return super.setProperty(name, value); 6252 return value; 6253 } 6254 6255 @Override 6256 public Base makeProperty(int hash, String name) throws FHIRException { 6257 switch (hash) { 6258 case 109757538: 6259 return getStartElement(); 6260 case 100571: 6261 return getEndElement(); 6262 default: 6263 return super.makeProperty(hash, name); 6264 } 6265 6266 } 6267 6268 @Override 6269 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6270 switch (hash) { 6271 case 109757538: 6272 /* start */ return new String[] { "integer" }; 6273 case 100571: 6274 /* end */ return new String[] { "integer" }; 6275 default: 6276 return super.getTypesForProperty(hash, name); 6277 } 6278 6279 } 6280 6281 @Override 6282 public Base addChild(String name) throws FHIRException { 6283 if (name.equals("start")) { 6284 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 6285 } else if (name.equals("end")) { 6286 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 6287 } else 6288 return super.addChild(name); 6289 } 6290 6291 public MolecularSequenceStructureVariantInnerComponent copy() { 6292 MolecularSequenceStructureVariantInnerComponent dst = new MolecularSequenceStructureVariantInnerComponent(); 6293 copyValues(dst); 6294 return dst; 6295 } 6296 6297 public void copyValues(MolecularSequenceStructureVariantInnerComponent dst) { 6298 super.copyValues(dst); 6299 dst.start = start == null ? null : start.copy(); 6300 dst.end = end == null ? null : end.copy(); 6301 } 6302 6303 @Override 6304 public boolean equalsDeep(Base other_) { 6305 if (!super.equalsDeep(other_)) 6306 return false; 6307 if (!(other_ instanceof MolecularSequenceStructureVariantInnerComponent)) 6308 return false; 6309 MolecularSequenceStructureVariantInnerComponent o = (MolecularSequenceStructureVariantInnerComponent) other_; 6310 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 6311 } 6312 6313 @Override 6314 public boolean equalsShallow(Base other_) { 6315 if (!super.equalsShallow(other_)) 6316 return false; 6317 if (!(other_ instanceof MolecularSequenceStructureVariantInnerComponent)) 6318 return false; 6319 MolecularSequenceStructureVariantInnerComponent o = (MolecularSequenceStructureVariantInnerComponent) other_; 6320 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 6321 } 6322 6323 public boolean isEmpty() { 6324 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 6325 } 6326 6327 public String fhirType() { 6328 return "MolecularSequence.structureVariant.inner"; 6329 6330 } 6331 6332 } 6333 6334 /** 6335 * A unique identifier for this particular sequence instance. This is a 6336 * FHIR-defined id. 6337 */ 6338 @Child(name = "identifier", type = { 6339 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6340 @Description(shortDefinition = "Unique ID for this particular sequence. This is a FHIR-defined id", formalDefinition = "A unique identifier for this particular sequence instance. This is a FHIR-defined id.") 6341 protected List<Identifier> identifier; 6342 6343 /** 6344 * Amino Acid Sequence/ DNA Sequence / RNA Sequence. 6345 */ 6346 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 6347 @Description(shortDefinition = "aa | dna | rna", formalDefinition = "Amino Acid Sequence/ DNA Sequence / RNA Sequence.") 6348 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-type") 6349 protected Enumeration<SequenceType> type; 6350 6351 /** 6352 * Whether the sequence is numbered starting at 0 (0-based numbering or 6353 * coordinates, inclusive start, exclusive end) or starting at 1 (1-based 6354 * numbering, inclusive start and inclusive end). 6355 */ 6356 @Child(name = "coordinateSystem", type = { 6357 IntegerType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 6358 @Description(shortDefinition = "Base number of coordinate system (0 for 0-based numbering or coordinates, inclusive start, exclusive end, 1 for 1-based numbering, inclusive start, inclusive end)", formalDefinition = "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).") 6359 protected IntegerType coordinateSystem; 6360 6361 /** 6362 * The patient whose sequencing results are described by this resource. 6363 */ 6364 @Child(name = "patient", type = { Patient.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 6365 @Description(shortDefinition = "Who and/or what this is about", formalDefinition = "The patient whose sequencing results are described by this resource.") 6366 protected Reference patient; 6367 6368 /** 6369 * The actual object that is the target of the reference (The patient whose 6370 * sequencing results are described by this resource.) 6371 */ 6372 protected Patient patientTarget; 6373 6374 /** 6375 * Specimen used for sequencing. 6376 */ 6377 @Child(name = "specimen", type = { Specimen.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 6378 @Description(shortDefinition = "Specimen used for sequencing", formalDefinition = "Specimen used for sequencing.") 6379 protected Reference specimen; 6380 6381 /** 6382 * The actual object that is the target of the reference (Specimen used for 6383 * sequencing.) 6384 */ 6385 protected Specimen specimenTarget; 6386 6387 /** 6388 * The method for sequencing, for example, chip information. 6389 */ 6390 @Child(name = "device", type = { Device.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 6391 @Description(shortDefinition = "The method for sequencing", formalDefinition = "The method for sequencing, for example, chip information.") 6392 protected Reference device; 6393 6394 /** 6395 * The actual object that is the target of the reference (The method for 6396 * sequencing, for example, chip information.) 6397 */ 6398 protected Device deviceTarget; 6399 6400 /** 6401 * The organization or lab that should be responsible for this result. 6402 */ 6403 @Child(name = "performer", type = { 6404 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 6405 @Description(shortDefinition = "Who should be responsible for test result", formalDefinition = "The organization or lab that should be responsible for this result.") 6406 protected Reference performer; 6407 6408 /** 6409 * The actual object that is the target of the reference (The organization or 6410 * lab that should be responsible for this result.) 6411 */ 6412 protected Organization performerTarget; 6413 6414 /** 6415 * The number of copies of the sequence of interest. (RNASeq). 6416 */ 6417 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 6418 @Description(shortDefinition = "The number of copies of the sequence of interest. (RNASeq)", formalDefinition = "The number of copies of the sequence of interest. (RNASeq).") 6419 protected Quantity quantity; 6420 6421 /** 6422 * A sequence that is used as a reference to describe variants that are present 6423 * in a sequence analyzed. 6424 */ 6425 @Child(name = "referenceSeq", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = true) 6426 @Description(shortDefinition = "A sequence used as reference", formalDefinition = "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.") 6427 protected MolecularSequenceReferenceSeqComponent referenceSeq; 6428 6429 /** 6430 * The definition of variant here originates from Sequence ontology 6431 * ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). 6432 * This element can represent amino acid or nucleic sequence change(including 6433 * insertion,deletion,SNP,etc.) It can represent some complex mutation or 6434 * segment variation with the assist of CIGAR string. 6435 */ 6436 @Child(name = "variant", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6437 @Description(shortDefinition = "Variant in sequence", formalDefinition = "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.") 6438 protected List<MolecularSequenceVariantComponent> variant; 6439 6440 /** 6441 * Sequence that was observed. It is the result marked by referenceSeq along 6442 * with variant records on referenceSeq. This shall start from 6443 * referenceSeq.windowStart and end by referenceSeq.windowEnd. 6444 */ 6445 @Child(name = "observedSeq", type = { 6446 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 6447 @Description(shortDefinition = "Sequence that was observed", formalDefinition = "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall start from referenceSeq.windowStart and end by referenceSeq.windowEnd.") 6448 protected StringType observedSeq; 6449 6450 /** 6451 * An experimental feature attribute that defines the quality of the feature in 6452 * a quantitative way, such as a phred quality score 6453 * ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)). 6454 */ 6455 @Child(name = "quality", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6456 @Description(shortDefinition = "An set of value as quality of sequence", formalDefinition = "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).") 6457 protected List<MolecularSequenceQualityComponent> quality; 6458 6459 /** 6460 * Coverage (read depth or depth) is the average number of reads representing a 6461 * given nucleotide in the reconstructed sequence. 6462 */ 6463 @Child(name = "readCoverage", type = { 6464 IntegerType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 6465 @Description(shortDefinition = "Average number of reads representing a given nucleotide in the reconstructed sequence", formalDefinition = "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.") 6466 protected IntegerType readCoverage; 6467 6468 /** 6469 * Configurations of the external repository. The repository shall store 6470 * target's observedSeq or records related with target's observedSeq. 6471 */ 6472 @Child(name = "repository", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6473 @Description(shortDefinition = "External repository which contains detailed report related with observedSeq in this resource", formalDefinition = "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.") 6474 protected List<MolecularSequenceRepositoryComponent> repository; 6475 6476 /** 6477 * Pointer to next atomic sequence which at most contains one variant. 6478 */ 6479 @Child(name = "pointer", type = { 6480 MolecularSequence.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6481 @Description(shortDefinition = "Pointer to next atomic sequence", formalDefinition = "Pointer to next atomic sequence which at most contains one variant.") 6482 protected List<Reference> pointer; 6483 /** 6484 * The actual objects that are the target of the reference (Pointer to next 6485 * atomic sequence which at most contains one variant.) 6486 */ 6487 protected List<MolecularSequence> pointerTarget; 6488 6489 /** 6490 * Information about chromosome structure variation. 6491 */ 6492 @Child(name = "structureVariant", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6493 @Description(shortDefinition = "Structural variant", formalDefinition = "Information about chromosome structure variation.") 6494 protected List<MolecularSequenceStructureVariantComponent> structureVariant; 6495 6496 private static final long serialVersionUID = -1541133500L; 6497 6498 /** 6499 * Constructor 6500 */ 6501 public MolecularSequence() { 6502 super(); 6503 } 6504 6505 /** 6506 * Constructor 6507 */ 6508 public MolecularSequence(IntegerType coordinateSystem) { 6509 super(); 6510 this.coordinateSystem = coordinateSystem; 6511 } 6512 6513 /** 6514 * @return {@link #identifier} (A unique identifier for this particular sequence 6515 * instance. This is a FHIR-defined id.) 6516 */ 6517 public List<Identifier> getIdentifier() { 6518 if (this.identifier == null) 6519 this.identifier = new ArrayList<Identifier>(); 6520 return this.identifier; 6521 } 6522 6523 /** 6524 * @return Returns a reference to <code>this</code> for easy method chaining 6525 */ 6526 public MolecularSequence setIdentifier(List<Identifier> theIdentifier) { 6527 this.identifier = theIdentifier; 6528 return this; 6529 } 6530 6531 public boolean hasIdentifier() { 6532 if (this.identifier == null) 6533 return false; 6534 for (Identifier item : this.identifier) 6535 if (!item.isEmpty()) 6536 return true; 6537 return false; 6538 } 6539 6540 public Identifier addIdentifier() { // 3 6541 Identifier t = new Identifier(); 6542 if (this.identifier == null) 6543 this.identifier = new ArrayList<Identifier>(); 6544 this.identifier.add(t); 6545 return t; 6546 } 6547 6548 public MolecularSequence addIdentifier(Identifier t) { // 3 6549 if (t == null) 6550 return this; 6551 if (this.identifier == null) 6552 this.identifier = new ArrayList<Identifier>(); 6553 this.identifier.add(t); 6554 return this; 6555 } 6556 6557 /** 6558 * @return The first repetition of repeating field {@link #identifier}, creating 6559 * it if it does not already exist 6560 */ 6561 public Identifier getIdentifierFirstRep() { 6562 if (getIdentifier().isEmpty()) { 6563 addIdentifier(); 6564 } 6565 return getIdentifier().get(0); 6566 } 6567 6568 /** 6569 * @return {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). 6570 * This is the underlying object with id, value and extensions. The 6571 * accessor "getType" gives direct access to the value 6572 */ 6573 public Enumeration<SequenceType> getTypeElement() { 6574 if (this.type == null) 6575 if (Configuration.errorOnAutoCreate()) 6576 throw new Error("Attempt to auto-create MolecularSequence.type"); 6577 else if (Configuration.doAutoCreate()) 6578 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); // bb 6579 return this.type; 6580 } 6581 6582 public boolean hasTypeElement() { 6583 return this.type != null && !this.type.isEmpty(); 6584 } 6585 6586 public boolean hasType() { 6587 return this.type != null && !this.type.isEmpty(); 6588 } 6589 6590 /** 6591 * @param value {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA 6592 * Sequence.). This is the underlying object with id, value and 6593 * extensions. The accessor "getType" gives direct access to the 6594 * value 6595 */ 6596 public MolecularSequence setTypeElement(Enumeration<SequenceType> value) { 6597 this.type = value; 6598 return this; 6599 } 6600 6601 /** 6602 * @return Amino Acid Sequence/ DNA Sequence / RNA Sequence. 6603 */ 6604 public SequenceType getType() { 6605 return this.type == null ? null : this.type.getValue(); 6606 } 6607 6608 /** 6609 * @param value Amino Acid Sequence/ DNA Sequence / RNA Sequence. 6610 */ 6611 public MolecularSequence setType(SequenceType value) { 6612 if (value == null) 6613 this.type = null; 6614 else { 6615 if (this.type == null) 6616 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); 6617 this.type.setValue(value); 6618 } 6619 return this; 6620 } 6621 6622 /** 6623 * @return {@link #coordinateSystem} (Whether the sequence is numbered starting 6624 * at 0 (0-based numbering or coordinates, inclusive start, exclusive 6625 * end) or starting at 1 (1-based numbering, inclusive start and 6626 * inclusive end).). This is the underlying object with id, value and 6627 * extensions. The accessor "getCoordinateSystem" gives direct access to 6628 * the value 6629 */ 6630 public IntegerType getCoordinateSystemElement() { 6631 if (this.coordinateSystem == null) 6632 if (Configuration.errorOnAutoCreate()) 6633 throw new Error("Attempt to auto-create MolecularSequence.coordinateSystem"); 6634 else if (Configuration.doAutoCreate()) 6635 this.coordinateSystem = new IntegerType(); // bb 6636 return this.coordinateSystem; 6637 } 6638 6639 public boolean hasCoordinateSystemElement() { 6640 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 6641 } 6642 6643 public boolean hasCoordinateSystem() { 6644 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 6645 } 6646 6647 /** 6648 * @param value {@link #coordinateSystem} (Whether the sequence is numbered 6649 * starting at 0 (0-based numbering or coordinates, inclusive 6650 * start, exclusive end) or starting at 1 (1-based numbering, 6651 * inclusive start and inclusive end).). This is the underlying 6652 * object with id, value and extensions. The accessor 6653 * "getCoordinateSystem" gives direct access to the value 6654 */ 6655 public MolecularSequence setCoordinateSystemElement(IntegerType value) { 6656 this.coordinateSystem = value; 6657 return this; 6658 } 6659 6660 /** 6661 * @return Whether the sequence is numbered starting at 0 (0-based numbering or 6662 * coordinates, inclusive start, exclusive end) or starting at 1 6663 * (1-based numbering, inclusive start and inclusive end). 6664 */ 6665 public int getCoordinateSystem() { 6666 return this.coordinateSystem == null || this.coordinateSystem.isEmpty() ? 0 : this.coordinateSystem.getValue(); 6667 } 6668 6669 /** 6670 * @param value Whether the sequence is numbered starting at 0 (0-based 6671 * numbering or coordinates, inclusive start, exclusive end) or 6672 * starting at 1 (1-based numbering, inclusive start and inclusive 6673 * end). 6674 */ 6675 public MolecularSequence setCoordinateSystem(int value) { 6676 if (this.coordinateSystem == null) 6677 this.coordinateSystem = new IntegerType(); 6678 this.coordinateSystem.setValue(value); 6679 return this; 6680 } 6681 6682 /** 6683 * @return {@link #patient} (The patient whose sequencing results are described 6684 * by this resource.) 6685 */ 6686 public Reference getPatient() { 6687 if (this.patient == null) 6688 if (Configuration.errorOnAutoCreate()) 6689 throw new Error("Attempt to auto-create MolecularSequence.patient"); 6690 else if (Configuration.doAutoCreate()) 6691 this.patient = new Reference(); // cc 6692 return this.patient; 6693 } 6694 6695 public boolean hasPatient() { 6696 return this.patient != null && !this.patient.isEmpty(); 6697 } 6698 6699 /** 6700 * @param value {@link #patient} (The patient whose sequencing results are 6701 * described by this resource.) 6702 */ 6703 public MolecularSequence setPatient(Reference value) { 6704 this.patient = value; 6705 return this; 6706 } 6707 6708 /** 6709 * @return {@link #patient} The actual object that is the target of the 6710 * reference. The reference library doesn't populate this, but you can 6711 * use it to hold the resource if you resolve it. (The patient whose 6712 * sequencing results are described by this resource.) 6713 */ 6714 public Patient getPatientTarget() { 6715 if (this.patientTarget == null) 6716 if (Configuration.errorOnAutoCreate()) 6717 throw new Error("Attempt to auto-create MolecularSequence.patient"); 6718 else if (Configuration.doAutoCreate()) 6719 this.patientTarget = new Patient(); // aa 6720 return this.patientTarget; 6721 } 6722 6723 /** 6724 * @param value {@link #patient} The actual object that is the target of the 6725 * reference. The reference library doesn't use these, but you can 6726 * use it to hold the resource if you resolve it. (The patient 6727 * whose sequencing results are described by this resource.) 6728 */ 6729 public MolecularSequence setPatientTarget(Patient value) { 6730 this.patientTarget = value; 6731 return this; 6732 } 6733 6734 /** 6735 * @return {@link #specimen} (Specimen used for sequencing.) 6736 */ 6737 public Reference getSpecimen() { 6738 if (this.specimen == null) 6739 if (Configuration.errorOnAutoCreate()) 6740 throw new Error("Attempt to auto-create MolecularSequence.specimen"); 6741 else if (Configuration.doAutoCreate()) 6742 this.specimen = new Reference(); // cc 6743 return this.specimen; 6744 } 6745 6746 public boolean hasSpecimen() { 6747 return this.specimen != null && !this.specimen.isEmpty(); 6748 } 6749 6750 /** 6751 * @param value {@link #specimen} (Specimen used for sequencing.) 6752 */ 6753 public MolecularSequence setSpecimen(Reference value) { 6754 this.specimen = value; 6755 return this; 6756 } 6757 6758 /** 6759 * @return {@link #specimen} The actual object that is the target of the 6760 * reference. The reference library doesn't populate this, but you can 6761 * use it to hold the resource if you resolve it. (Specimen used for 6762 * sequencing.) 6763 */ 6764 public Specimen getSpecimenTarget() { 6765 if (this.specimenTarget == null) 6766 if (Configuration.errorOnAutoCreate()) 6767 throw new Error("Attempt to auto-create MolecularSequence.specimen"); 6768 else if (Configuration.doAutoCreate()) 6769 this.specimenTarget = new Specimen(); // aa 6770 return this.specimenTarget; 6771 } 6772 6773 /** 6774 * @param value {@link #specimen} The actual object that is the target of the 6775 * reference. The reference library doesn't use these, but you can 6776 * use it to hold the resource if you resolve it. (Specimen used 6777 * for sequencing.) 6778 */ 6779 public MolecularSequence setSpecimenTarget(Specimen value) { 6780 this.specimenTarget = value; 6781 return this; 6782 } 6783 6784 /** 6785 * @return {@link #device} (The method for sequencing, for example, chip 6786 * information.) 6787 */ 6788 public Reference getDevice() { 6789 if (this.device == null) 6790 if (Configuration.errorOnAutoCreate()) 6791 throw new Error("Attempt to auto-create MolecularSequence.device"); 6792 else if (Configuration.doAutoCreate()) 6793 this.device = new Reference(); // cc 6794 return this.device; 6795 } 6796 6797 public boolean hasDevice() { 6798 return this.device != null && !this.device.isEmpty(); 6799 } 6800 6801 /** 6802 * @param value {@link #device} (The method for sequencing, for example, chip 6803 * information.) 6804 */ 6805 public MolecularSequence setDevice(Reference value) { 6806 this.device = value; 6807 return this; 6808 } 6809 6810 /** 6811 * @return {@link #device} The actual object that is the target of the 6812 * reference. The reference library doesn't populate this, but you can 6813 * use it to hold the resource if you resolve it. (The method for 6814 * sequencing, for example, chip information.) 6815 */ 6816 public Device getDeviceTarget() { 6817 if (this.deviceTarget == null) 6818 if (Configuration.errorOnAutoCreate()) 6819 throw new Error("Attempt to auto-create MolecularSequence.device"); 6820 else if (Configuration.doAutoCreate()) 6821 this.deviceTarget = new Device(); // aa 6822 return this.deviceTarget; 6823 } 6824 6825 /** 6826 * @param value {@link #device} The actual object that is the target of the 6827 * reference. The reference library doesn't use these, but you can 6828 * use it to hold the resource if you resolve it. (The method for 6829 * sequencing, for example, chip information.) 6830 */ 6831 public MolecularSequence setDeviceTarget(Device value) { 6832 this.deviceTarget = value; 6833 return this; 6834 } 6835 6836 /** 6837 * @return {@link #performer} (The organization or lab that should be 6838 * responsible for this result.) 6839 */ 6840 public Reference getPerformer() { 6841 if (this.performer == null) 6842 if (Configuration.errorOnAutoCreate()) 6843 throw new Error("Attempt to auto-create MolecularSequence.performer"); 6844 else if (Configuration.doAutoCreate()) 6845 this.performer = new Reference(); // cc 6846 return this.performer; 6847 } 6848 6849 public boolean hasPerformer() { 6850 return this.performer != null && !this.performer.isEmpty(); 6851 } 6852 6853 /** 6854 * @param value {@link #performer} (The organization or lab that should be 6855 * responsible for this result.) 6856 */ 6857 public MolecularSequence setPerformer(Reference value) { 6858 this.performer = value; 6859 return this; 6860 } 6861 6862 /** 6863 * @return {@link #performer} The actual object that is the target of the 6864 * reference. The reference library doesn't populate this, but you can 6865 * use it to hold the resource if you resolve it. (The organization or 6866 * lab that should be responsible for this result.) 6867 */ 6868 public Organization getPerformerTarget() { 6869 if (this.performerTarget == null) 6870 if (Configuration.errorOnAutoCreate()) 6871 throw new Error("Attempt to auto-create MolecularSequence.performer"); 6872 else if (Configuration.doAutoCreate()) 6873 this.performerTarget = new Organization(); // aa 6874 return this.performerTarget; 6875 } 6876 6877 /** 6878 * @param value {@link #performer} The actual object that is the target of the 6879 * reference. The reference library doesn't use these, but you can 6880 * use it to hold the resource if you resolve it. (The organization 6881 * or lab that should be responsible for this result.) 6882 */ 6883 public MolecularSequence setPerformerTarget(Organization value) { 6884 this.performerTarget = value; 6885 return this; 6886 } 6887 6888 /** 6889 * @return {@link #quantity} (The number of copies of the sequence of interest. 6890 * (RNASeq).) 6891 */ 6892 public Quantity getQuantity() { 6893 if (this.quantity == null) 6894 if (Configuration.errorOnAutoCreate()) 6895 throw new Error("Attempt to auto-create MolecularSequence.quantity"); 6896 else if (Configuration.doAutoCreate()) 6897 this.quantity = new Quantity(); // cc 6898 return this.quantity; 6899 } 6900 6901 public boolean hasQuantity() { 6902 return this.quantity != null && !this.quantity.isEmpty(); 6903 } 6904 6905 /** 6906 * @param value {@link #quantity} (The number of copies of the sequence of 6907 * interest. (RNASeq).) 6908 */ 6909 public MolecularSequence setQuantity(Quantity value) { 6910 this.quantity = value; 6911 return this; 6912 } 6913 6914 /** 6915 * @return {@link #referenceSeq} (A sequence that is used as a reference to 6916 * describe variants that are present in a sequence analyzed.) 6917 */ 6918 public MolecularSequenceReferenceSeqComponent getReferenceSeq() { 6919 if (this.referenceSeq == null) 6920 if (Configuration.errorOnAutoCreate()) 6921 throw new Error("Attempt to auto-create MolecularSequence.referenceSeq"); 6922 else if (Configuration.doAutoCreate()) 6923 this.referenceSeq = new MolecularSequenceReferenceSeqComponent(); // cc 6924 return this.referenceSeq; 6925 } 6926 6927 public boolean hasReferenceSeq() { 6928 return this.referenceSeq != null && !this.referenceSeq.isEmpty(); 6929 } 6930 6931 /** 6932 * @param value {@link #referenceSeq} (A sequence that is used as a reference to 6933 * describe variants that are present in a sequence analyzed.) 6934 */ 6935 public MolecularSequence setReferenceSeq(MolecularSequenceReferenceSeqComponent value) { 6936 this.referenceSeq = value; 6937 return this; 6938 } 6939 6940 /** 6941 * @return {@link #variant} (The definition of variant here originates from 6942 * Sequence ontology 6943 * ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). 6944 * This element can represent amino acid or nucleic sequence 6945 * change(including insertion,deletion,SNP,etc.) It can represent some 6946 * complex mutation or segment variation with the assist of CIGAR 6947 * string.) 6948 */ 6949 public List<MolecularSequenceVariantComponent> getVariant() { 6950 if (this.variant == null) 6951 this.variant = new ArrayList<MolecularSequenceVariantComponent>(); 6952 return this.variant; 6953 } 6954 6955 /** 6956 * @return Returns a reference to <code>this</code> for easy method chaining 6957 */ 6958 public MolecularSequence setVariant(List<MolecularSequenceVariantComponent> theVariant) { 6959 this.variant = theVariant; 6960 return this; 6961 } 6962 6963 public boolean hasVariant() { 6964 if (this.variant == null) 6965 return false; 6966 for (MolecularSequenceVariantComponent item : this.variant) 6967 if (!item.isEmpty()) 6968 return true; 6969 return false; 6970 } 6971 6972 public MolecularSequenceVariantComponent addVariant() { // 3 6973 MolecularSequenceVariantComponent t = new MolecularSequenceVariantComponent(); 6974 if (this.variant == null) 6975 this.variant = new ArrayList<MolecularSequenceVariantComponent>(); 6976 this.variant.add(t); 6977 return t; 6978 } 6979 6980 public MolecularSequence addVariant(MolecularSequenceVariantComponent t) { // 3 6981 if (t == null) 6982 return this; 6983 if (this.variant == null) 6984 this.variant = new ArrayList<MolecularSequenceVariantComponent>(); 6985 this.variant.add(t); 6986 return this; 6987 } 6988 6989 /** 6990 * @return The first repetition of repeating field {@link #variant}, creating it 6991 * if it does not already exist 6992 */ 6993 public MolecularSequenceVariantComponent getVariantFirstRep() { 6994 if (getVariant().isEmpty()) { 6995 addVariant(); 6996 } 6997 return getVariant().get(0); 6998 } 6999 7000 /** 7001 * @return {@link #observedSeq} (Sequence that was observed. It is the result 7002 * marked by referenceSeq along with variant records on referenceSeq. 7003 * This shall start from referenceSeq.windowStart and end by 7004 * referenceSeq.windowEnd.). This is the underlying object with id, 7005 * value and extensions. The accessor "getObservedSeq" gives direct 7006 * access to the value 7007 */ 7008 public StringType getObservedSeqElement() { 7009 if (this.observedSeq == null) 7010 if (Configuration.errorOnAutoCreate()) 7011 throw new Error("Attempt to auto-create MolecularSequence.observedSeq"); 7012 else if (Configuration.doAutoCreate()) 7013 this.observedSeq = new StringType(); // bb 7014 return this.observedSeq; 7015 } 7016 7017 public boolean hasObservedSeqElement() { 7018 return this.observedSeq != null && !this.observedSeq.isEmpty(); 7019 } 7020 7021 public boolean hasObservedSeq() { 7022 return this.observedSeq != null && !this.observedSeq.isEmpty(); 7023 } 7024 7025 /** 7026 * @param value {@link #observedSeq} (Sequence that was observed. It is the 7027 * result marked by referenceSeq along with variant records on 7028 * referenceSeq. This shall start from referenceSeq.windowStart and 7029 * end by referenceSeq.windowEnd.). This is the underlying object 7030 * with id, value and extensions. The accessor "getObservedSeq" 7031 * gives direct access to the value 7032 */ 7033 public MolecularSequence setObservedSeqElement(StringType value) { 7034 this.observedSeq = value; 7035 return this; 7036 } 7037 7038 /** 7039 * @return Sequence that was observed. It is the result marked by referenceSeq 7040 * along with variant records on referenceSeq. This shall start from 7041 * referenceSeq.windowStart and end by referenceSeq.windowEnd. 7042 */ 7043 public String getObservedSeq() { 7044 return this.observedSeq == null ? null : this.observedSeq.getValue(); 7045 } 7046 7047 /** 7048 * @param value Sequence that was observed. It is the result marked by 7049 * referenceSeq along with variant records on referenceSeq. This 7050 * shall start from referenceSeq.windowStart and end by 7051 * referenceSeq.windowEnd. 7052 */ 7053 public MolecularSequence setObservedSeq(String value) { 7054 if (Utilities.noString(value)) 7055 this.observedSeq = null; 7056 else { 7057 if (this.observedSeq == null) 7058 this.observedSeq = new StringType(); 7059 this.observedSeq.setValue(value); 7060 } 7061 return this; 7062 } 7063 7064 /** 7065 * @return {@link #quality} (An experimental feature attribute that defines the 7066 * quality of the feature in a quantitative way, such as a phred quality 7067 * score 7068 * ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).) 7069 */ 7070 public List<MolecularSequenceQualityComponent> getQuality() { 7071 if (this.quality == null) 7072 this.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7073 return this.quality; 7074 } 7075 7076 /** 7077 * @return Returns a reference to <code>this</code> for easy method chaining 7078 */ 7079 public MolecularSequence setQuality(List<MolecularSequenceQualityComponent> theQuality) { 7080 this.quality = theQuality; 7081 return this; 7082 } 7083 7084 public boolean hasQuality() { 7085 if (this.quality == null) 7086 return false; 7087 for (MolecularSequenceQualityComponent item : this.quality) 7088 if (!item.isEmpty()) 7089 return true; 7090 return false; 7091 } 7092 7093 public MolecularSequenceQualityComponent addQuality() { // 3 7094 MolecularSequenceQualityComponent t = new MolecularSequenceQualityComponent(); 7095 if (this.quality == null) 7096 this.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7097 this.quality.add(t); 7098 return t; 7099 } 7100 7101 public MolecularSequence addQuality(MolecularSequenceQualityComponent t) { // 3 7102 if (t == null) 7103 return this; 7104 if (this.quality == null) 7105 this.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7106 this.quality.add(t); 7107 return this; 7108 } 7109 7110 /** 7111 * @return The first repetition of repeating field {@link #quality}, creating it 7112 * if it does not already exist 7113 */ 7114 public MolecularSequenceQualityComponent getQualityFirstRep() { 7115 if (getQuality().isEmpty()) { 7116 addQuality(); 7117 } 7118 return getQuality().get(0); 7119 } 7120 7121 /** 7122 * @return {@link #readCoverage} (Coverage (read depth or depth) is the average 7123 * number of reads representing a given nucleotide in the reconstructed 7124 * sequence.). This is the underlying object with id, value and 7125 * extensions. The accessor "getReadCoverage" gives direct access to the 7126 * value 7127 */ 7128 public IntegerType getReadCoverageElement() { 7129 if (this.readCoverage == null) 7130 if (Configuration.errorOnAutoCreate()) 7131 throw new Error("Attempt to auto-create MolecularSequence.readCoverage"); 7132 else if (Configuration.doAutoCreate()) 7133 this.readCoverage = new IntegerType(); // bb 7134 return this.readCoverage; 7135 } 7136 7137 public boolean hasReadCoverageElement() { 7138 return this.readCoverage != null && !this.readCoverage.isEmpty(); 7139 } 7140 7141 public boolean hasReadCoverage() { 7142 return this.readCoverage != null && !this.readCoverage.isEmpty(); 7143 } 7144 7145 /** 7146 * @param value {@link #readCoverage} (Coverage (read depth or depth) is the 7147 * average number of reads representing a given nucleotide in the 7148 * reconstructed sequence.). This is the underlying object with id, 7149 * value and extensions. The accessor "getReadCoverage" gives 7150 * direct access to the value 7151 */ 7152 public MolecularSequence setReadCoverageElement(IntegerType value) { 7153 this.readCoverage = value; 7154 return this; 7155 } 7156 7157 /** 7158 * @return Coverage (read depth or depth) is the average number of reads 7159 * representing a given nucleotide in the reconstructed sequence. 7160 */ 7161 public int getReadCoverage() { 7162 return this.readCoverage == null || this.readCoverage.isEmpty() ? 0 : this.readCoverage.getValue(); 7163 } 7164 7165 /** 7166 * @param value Coverage (read depth or depth) is the average number of reads 7167 * representing a given nucleotide in the reconstructed sequence. 7168 */ 7169 public MolecularSequence setReadCoverage(int value) { 7170 if (this.readCoverage == null) 7171 this.readCoverage = new IntegerType(); 7172 this.readCoverage.setValue(value); 7173 return this; 7174 } 7175 7176 /** 7177 * @return {@link #repository} (Configurations of the external repository. The 7178 * repository shall store target's observedSeq or records related with 7179 * target's observedSeq.) 7180 */ 7181 public List<MolecularSequenceRepositoryComponent> getRepository() { 7182 if (this.repository == null) 7183 this.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7184 return this.repository; 7185 } 7186 7187 /** 7188 * @return Returns a reference to <code>this</code> for easy method chaining 7189 */ 7190 public MolecularSequence setRepository(List<MolecularSequenceRepositoryComponent> theRepository) { 7191 this.repository = theRepository; 7192 return this; 7193 } 7194 7195 public boolean hasRepository() { 7196 if (this.repository == null) 7197 return false; 7198 for (MolecularSequenceRepositoryComponent item : this.repository) 7199 if (!item.isEmpty()) 7200 return true; 7201 return false; 7202 } 7203 7204 public MolecularSequenceRepositoryComponent addRepository() { // 3 7205 MolecularSequenceRepositoryComponent t = new MolecularSequenceRepositoryComponent(); 7206 if (this.repository == null) 7207 this.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7208 this.repository.add(t); 7209 return t; 7210 } 7211 7212 public MolecularSequence addRepository(MolecularSequenceRepositoryComponent t) { // 3 7213 if (t == null) 7214 return this; 7215 if (this.repository == null) 7216 this.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7217 this.repository.add(t); 7218 return this; 7219 } 7220 7221 /** 7222 * @return The first repetition of repeating field {@link #repository}, creating 7223 * it if it does not already exist 7224 */ 7225 public MolecularSequenceRepositoryComponent getRepositoryFirstRep() { 7226 if (getRepository().isEmpty()) { 7227 addRepository(); 7228 } 7229 return getRepository().get(0); 7230 } 7231 7232 /** 7233 * @return {@link #pointer} (Pointer to next atomic sequence which at most 7234 * contains one variant.) 7235 */ 7236 public List<Reference> getPointer() { 7237 if (this.pointer == null) 7238 this.pointer = new ArrayList<Reference>(); 7239 return this.pointer; 7240 } 7241 7242 /** 7243 * @return Returns a reference to <code>this</code> for easy method chaining 7244 */ 7245 public MolecularSequence setPointer(List<Reference> thePointer) { 7246 this.pointer = thePointer; 7247 return this; 7248 } 7249 7250 public boolean hasPointer() { 7251 if (this.pointer == null) 7252 return false; 7253 for (Reference item : this.pointer) 7254 if (!item.isEmpty()) 7255 return true; 7256 return false; 7257 } 7258 7259 public Reference addPointer() { // 3 7260 Reference t = new Reference(); 7261 if (this.pointer == null) 7262 this.pointer = new ArrayList<Reference>(); 7263 this.pointer.add(t); 7264 return t; 7265 } 7266 7267 public MolecularSequence addPointer(Reference t) { // 3 7268 if (t == null) 7269 return this; 7270 if (this.pointer == null) 7271 this.pointer = new ArrayList<Reference>(); 7272 this.pointer.add(t); 7273 return this; 7274 } 7275 7276 /** 7277 * @return The first repetition of repeating field {@link #pointer}, creating it 7278 * if it does not already exist 7279 */ 7280 public Reference getPointerFirstRep() { 7281 if (getPointer().isEmpty()) { 7282 addPointer(); 7283 } 7284 return getPointer().get(0); 7285 } 7286 7287 /** 7288 * @deprecated Use Reference#setResource(IBaseResource) instead 7289 */ 7290 @Deprecated 7291 public List<MolecularSequence> getPointerTarget() { 7292 if (this.pointerTarget == null) 7293 this.pointerTarget = new ArrayList<MolecularSequence>(); 7294 return this.pointerTarget; 7295 } 7296 7297 /** 7298 * @deprecated Use Reference#setResource(IBaseResource) instead 7299 */ 7300 @Deprecated 7301 public MolecularSequence addPointerTarget() { 7302 MolecularSequence r = new MolecularSequence(); 7303 if (this.pointerTarget == null) 7304 this.pointerTarget = new ArrayList<MolecularSequence>(); 7305 this.pointerTarget.add(r); 7306 return r; 7307 } 7308 7309 /** 7310 * @return {@link #structureVariant} (Information about chromosome structure 7311 * variation.) 7312 */ 7313 public List<MolecularSequenceStructureVariantComponent> getStructureVariant() { 7314 if (this.structureVariant == null) 7315 this.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7316 return this.structureVariant; 7317 } 7318 7319 /** 7320 * @return Returns a reference to <code>this</code> for easy method chaining 7321 */ 7322 public MolecularSequence setStructureVariant(List<MolecularSequenceStructureVariantComponent> theStructureVariant) { 7323 this.structureVariant = theStructureVariant; 7324 return this; 7325 } 7326 7327 public boolean hasStructureVariant() { 7328 if (this.structureVariant == null) 7329 return false; 7330 for (MolecularSequenceStructureVariantComponent item : this.structureVariant) 7331 if (!item.isEmpty()) 7332 return true; 7333 return false; 7334 } 7335 7336 public MolecularSequenceStructureVariantComponent addStructureVariant() { // 3 7337 MolecularSequenceStructureVariantComponent t = new MolecularSequenceStructureVariantComponent(); 7338 if (this.structureVariant == null) 7339 this.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7340 this.structureVariant.add(t); 7341 return t; 7342 } 7343 7344 public MolecularSequence addStructureVariant(MolecularSequenceStructureVariantComponent t) { // 3 7345 if (t == null) 7346 return this; 7347 if (this.structureVariant == null) 7348 this.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7349 this.structureVariant.add(t); 7350 return this; 7351 } 7352 7353 /** 7354 * @return The first repetition of repeating field {@link #structureVariant}, 7355 * creating it if it does not already exist 7356 */ 7357 public MolecularSequenceStructureVariantComponent getStructureVariantFirstRep() { 7358 if (getStructureVariant().isEmpty()) { 7359 addStructureVariant(); 7360 } 7361 return getStructureVariant().get(0); 7362 } 7363 7364 protected void listChildren(List<Property> children) { 7365 super.listChildren(children); 7366 children.add(new Property("identifier", "Identifier", 7367 "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, 7368 java.lang.Integer.MAX_VALUE, identifier)); 7369 children.add(new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type)); 7370 children.add(new Property("coordinateSystem", "integer", 7371 "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 7372 0, 1, coordinateSystem)); 7373 children.add(new Property("patient", "Reference(Patient)", 7374 "The patient whose sequencing results are described by this resource.", 0, 1, patient)); 7375 children.add(new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen)); 7376 children.add(new Property("device", "Reference(Device)", 7377 "The method for sequencing, for example, chip information.", 0, 1, device)); 7378 children.add(new Property("performer", "Reference(Organization)", 7379 "The organization or lab that should be responsible for this result.", 0, 1, performer)); 7380 children.add(new Property("quantity", "Quantity", "The number of copies of the sequence of interest. (RNASeq).", 0, 7381 1, quantity)); 7382 children.add(new Property("referenceSeq", "", 7383 "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, 7384 referenceSeq)); 7385 children.add(new Property("variant", "", 7386 "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 7387 0, java.lang.Integer.MAX_VALUE, variant)); 7388 children.add(new Property("observedSeq", "string", 7389 "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall start from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 7390 0, 1, observedSeq)); 7391 children.add(new Property("quality", "", 7392 "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 7393 0, java.lang.Integer.MAX_VALUE, quality)); 7394 children.add(new Property("readCoverage", "integer", 7395 "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 7396 0, 1, readCoverage)); 7397 children.add(new Property("repository", "", 7398 "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 7399 0, java.lang.Integer.MAX_VALUE, repository)); 7400 children.add(new Property("pointer", "Reference(MolecularSequence)", 7401 "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, 7402 pointer)); 7403 children.add(new Property("structureVariant", "", "Information about chromosome structure variation.", 0, 7404 java.lang.Integer.MAX_VALUE, structureVariant)); 7405 } 7406 7407 @Override 7408 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7409 switch (_hash) { 7410 case -1618432855: 7411 /* identifier */ return new Property("identifier", "Identifier", 7412 "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, 7413 java.lang.Integer.MAX_VALUE, identifier); 7414 case 3575610: 7415 /* type */ return new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type); 7416 case 354212295: 7417 /* coordinateSystem */ return new Property("coordinateSystem", "integer", 7418 "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 7419 0, 1, coordinateSystem); 7420 case -791418107: 7421 /* patient */ return new Property("patient", "Reference(Patient)", 7422 "The patient whose sequencing results are described by this resource.", 0, 1, patient); 7423 case -2132868344: 7424 /* specimen */ return new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, 7425 specimen); 7426 case -1335157162: 7427 /* device */ return new Property("device", "Reference(Device)", 7428 "The method for sequencing, for example, chip information.", 0, 1, device); 7429 case 481140686: 7430 /* performer */ return new Property("performer", "Reference(Organization)", 7431 "The organization or lab that should be responsible for this result.", 0, 1, performer); 7432 case -1285004149: 7433 /* quantity */ return new Property("quantity", "Quantity", 7434 "The number of copies of the sequence of interest. (RNASeq).", 0, 1, quantity); 7435 case -502547180: 7436 /* referenceSeq */ return new Property("referenceSeq", "", 7437 "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, 7438 referenceSeq); 7439 case 236785797: 7440 /* variant */ return new Property("variant", "", 7441 "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 7442 0, java.lang.Integer.MAX_VALUE, variant); 7443 case 125541495: 7444 /* observedSeq */ return new Property("observedSeq", "string", 7445 "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall start from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 7446 0, 1, observedSeq); 7447 case 651215103: 7448 /* quality */ return new Property("quality", "", 7449 "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 7450 0, java.lang.Integer.MAX_VALUE, quality); 7451 case -1798816354: 7452 /* readCoverage */ return new Property("readCoverage", "integer", 7453 "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 7454 0, 1, readCoverage); 7455 case 1950800714: 7456 /* repository */ return new Property("repository", "", 7457 "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 7458 0, java.lang.Integer.MAX_VALUE, repository); 7459 case -400605635: 7460 /* pointer */ return new Property("pointer", "Reference(MolecularSequence)", 7461 "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, 7462 pointer); 7463 case 757269394: 7464 /* structureVariant */ return new Property("structureVariant", "", 7465 "Information about chromosome structure variation.", 0, java.lang.Integer.MAX_VALUE, structureVariant); 7466 default: 7467 return super.getNamedProperty(_hash, _name, _checkValid); 7468 } 7469 7470 } 7471 7472 @Override 7473 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7474 switch (hash) { 7475 case -1618432855: 7476 /* identifier */ return this.identifier == null ? new Base[0] 7477 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 7478 case 3575610: 7479 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SequenceType> 7480 case 354212295: 7481 /* coordinateSystem */ return this.coordinateSystem == null ? new Base[0] : new Base[] { this.coordinateSystem }; // IntegerType 7482 case -791418107: 7483 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 7484 case -2132868344: 7485 /* specimen */ return this.specimen == null ? new Base[0] : new Base[] { this.specimen }; // Reference 7486 case -1335157162: 7487 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 7488 case 481140686: 7489 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 7490 case -1285004149: 7491 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7492 case -502547180: 7493 /* referenceSeq */ return this.referenceSeq == null ? new Base[0] : new Base[] { this.referenceSeq }; // MolecularSequenceReferenceSeqComponent 7494 case 236785797: 7495 /* variant */ return this.variant == null ? new Base[0] : this.variant.toArray(new Base[this.variant.size()]); // MolecularSequenceVariantComponent 7496 case 125541495: 7497 /* observedSeq */ return this.observedSeq == null ? new Base[0] : new Base[] { this.observedSeq }; // StringType 7498 case 651215103: 7499 /* quality */ return this.quality == null ? new Base[0] : this.quality.toArray(new Base[this.quality.size()]); // MolecularSequenceQualityComponent 7500 case -1798816354: 7501 /* readCoverage */ return this.readCoverage == null ? new Base[0] : new Base[] { this.readCoverage }; // IntegerType 7502 case 1950800714: 7503 /* repository */ return this.repository == null ? new Base[0] 7504 : this.repository.toArray(new Base[this.repository.size()]); // MolecularSequenceRepositoryComponent 7505 case -400605635: 7506 /* pointer */ return this.pointer == null ? new Base[0] : this.pointer.toArray(new Base[this.pointer.size()]); // Reference 7507 case 757269394: 7508 /* structureVariant */ return this.structureVariant == null ? new Base[0] 7509 : this.structureVariant.toArray(new Base[this.structureVariant.size()]); // MolecularSequenceStructureVariantComponent 7510 default: 7511 return super.getProperty(hash, name, checkValid); 7512 } 7513 7514 } 7515 7516 @Override 7517 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7518 switch (hash) { 7519 case -1618432855: // identifier 7520 this.getIdentifier().add(castToIdentifier(value)); // Identifier 7521 return value; 7522 case 3575610: // type 7523 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 7524 this.type = (Enumeration) value; // Enumeration<SequenceType> 7525 return value; 7526 case 354212295: // coordinateSystem 7527 this.coordinateSystem = castToInteger(value); // IntegerType 7528 return value; 7529 case -791418107: // patient 7530 this.patient = castToReference(value); // Reference 7531 return value; 7532 case -2132868344: // specimen 7533 this.specimen = castToReference(value); // Reference 7534 return value; 7535 case -1335157162: // device 7536 this.device = castToReference(value); // Reference 7537 return value; 7538 case 481140686: // performer 7539 this.performer = castToReference(value); // Reference 7540 return value; 7541 case -1285004149: // quantity 7542 this.quantity = castToQuantity(value); // Quantity 7543 return value; 7544 case -502547180: // referenceSeq 7545 this.referenceSeq = (MolecularSequenceReferenceSeqComponent) value; // MolecularSequenceReferenceSeqComponent 7546 return value; 7547 case 236785797: // variant 7548 this.getVariant().add((MolecularSequenceVariantComponent) value); // MolecularSequenceVariantComponent 7549 return value; 7550 case 125541495: // observedSeq 7551 this.observedSeq = castToString(value); // StringType 7552 return value; 7553 case 651215103: // quality 7554 this.getQuality().add((MolecularSequenceQualityComponent) value); // MolecularSequenceQualityComponent 7555 return value; 7556 case -1798816354: // readCoverage 7557 this.readCoverage = castToInteger(value); // IntegerType 7558 return value; 7559 case 1950800714: // repository 7560 this.getRepository().add((MolecularSequenceRepositoryComponent) value); // MolecularSequenceRepositoryComponent 7561 return value; 7562 case -400605635: // pointer 7563 this.getPointer().add(castToReference(value)); // Reference 7564 return value; 7565 case 757269394: // structureVariant 7566 this.getStructureVariant().add((MolecularSequenceStructureVariantComponent) value); // MolecularSequenceStructureVariantComponent 7567 return value; 7568 default: 7569 return super.setProperty(hash, name, value); 7570 } 7571 7572 } 7573 7574 @Override 7575 public Base setProperty(String name, Base value) throws FHIRException { 7576 if (name.equals("identifier")) { 7577 this.getIdentifier().add(castToIdentifier(value)); 7578 } else if (name.equals("type")) { 7579 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 7580 this.type = (Enumeration) value; // Enumeration<SequenceType> 7581 } else if (name.equals("coordinateSystem")) { 7582 this.coordinateSystem = castToInteger(value); // IntegerType 7583 } else if (name.equals("patient")) { 7584 this.patient = castToReference(value); // Reference 7585 } else if (name.equals("specimen")) { 7586 this.specimen = castToReference(value); // Reference 7587 } else if (name.equals("device")) { 7588 this.device = castToReference(value); // Reference 7589 } else if (name.equals("performer")) { 7590 this.performer = castToReference(value); // Reference 7591 } else if (name.equals("quantity")) { 7592 this.quantity = castToQuantity(value); // Quantity 7593 } else if (name.equals("referenceSeq")) { 7594 this.referenceSeq = (MolecularSequenceReferenceSeqComponent) value; // MolecularSequenceReferenceSeqComponent 7595 } else if (name.equals("variant")) { 7596 this.getVariant().add((MolecularSequenceVariantComponent) value); 7597 } else if (name.equals("observedSeq")) { 7598 this.observedSeq = castToString(value); // StringType 7599 } else if (name.equals("quality")) { 7600 this.getQuality().add((MolecularSequenceQualityComponent) value); 7601 } else if (name.equals("readCoverage")) { 7602 this.readCoverage = castToInteger(value); // IntegerType 7603 } else if (name.equals("repository")) { 7604 this.getRepository().add((MolecularSequenceRepositoryComponent) value); 7605 } else if (name.equals("pointer")) { 7606 this.getPointer().add(castToReference(value)); 7607 } else if (name.equals("structureVariant")) { 7608 this.getStructureVariant().add((MolecularSequenceStructureVariantComponent) value); 7609 } else 7610 return super.setProperty(name, value); 7611 return value; 7612 } 7613 7614 @Override 7615 public Base makeProperty(int hash, String name) throws FHIRException { 7616 switch (hash) { 7617 case -1618432855: 7618 return addIdentifier(); 7619 case 3575610: 7620 return getTypeElement(); 7621 case 354212295: 7622 return getCoordinateSystemElement(); 7623 case -791418107: 7624 return getPatient(); 7625 case -2132868344: 7626 return getSpecimen(); 7627 case -1335157162: 7628 return getDevice(); 7629 case 481140686: 7630 return getPerformer(); 7631 case -1285004149: 7632 return getQuantity(); 7633 case -502547180: 7634 return getReferenceSeq(); 7635 case 236785797: 7636 return addVariant(); 7637 case 125541495: 7638 return getObservedSeqElement(); 7639 case 651215103: 7640 return addQuality(); 7641 case -1798816354: 7642 return getReadCoverageElement(); 7643 case 1950800714: 7644 return addRepository(); 7645 case -400605635: 7646 return addPointer(); 7647 case 757269394: 7648 return addStructureVariant(); 7649 default: 7650 return super.makeProperty(hash, name); 7651 } 7652 7653 } 7654 7655 @Override 7656 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7657 switch (hash) { 7658 case -1618432855: 7659 /* identifier */ return new String[] { "Identifier" }; 7660 case 3575610: 7661 /* type */ return new String[] { "code" }; 7662 case 354212295: 7663 /* coordinateSystem */ return new String[] { "integer" }; 7664 case -791418107: 7665 /* patient */ return new String[] { "Reference" }; 7666 case -2132868344: 7667 /* specimen */ return new String[] { "Reference" }; 7668 case -1335157162: 7669 /* device */ return new String[] { "Reference" }; 7670 case 481140686: 7671 /* performer */ return new String[] { "Reference" }; 7672 case -1285004149: 7673 /* quantity */ return new String[] { "Quantity" }; 7674 case -502547180: 7675 /* referenceSeq */ return new String[] {}; 7676 case 236785797: 7677 /* variant */ return new String[] {}; 7678 case 125541495: 7679 /* observedSeq */ return new String[] { "string" }; 7680 case 651215103: 7681 /* quality */ return new String[] {}; 7682 case -1798816354: 7683 /* readCoverage */ return new String[] { "integer" }; 7684 case 1950800714: 7685 /* repository */ return new String[] {}; 7686 case -400605635: 7687 /* pointer */ return new String[] { "Reference" }; 7688 case 757269394: 7689 /* structureVariant */ return new String[] {}; 7690 default: 7691 return super.getTypesForProperty(hash, name); 7692 } 7693 7694 } 7695 7696 @Override 7697 public Base addChild(String name) throws FHIRException { 7698 if (name.equals("identifier")) { 7699 return addIdentifier(); 7700 } else if (name.equals("type")) { 7701 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 7702 } else if (name.equals("coordinateSystem")) { 7703 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.coordinateSystem"); 7704 } else if (name.equals("patient")) { 7705 this.patient = new Reference(); 7706 return this.patient; 7707 } else if (name.equals("specimen")) { 7708 this.specimen = new Reference(); 7709 return this.specimen; 7710 } else if (name.equals("device")) { 7711 this.device = new Reference(); 7712 return this.device; 7713 } else if (name.equals("performer")) { 7714 this.performer = new Reference(); 7715 return this.performer; 7716 } else if (name.equals("quantity")) { 7717 this.quantity = new Quantity(); 7718 return this.quantity; 7719 } else if (name.equals("referenceSeq")) { 7720 this.referenceSeq = new MolecularSequenceReferenceSeqComponent(); 7721 return this.referenceSeq; 7722 } else if (name.equals("variant")) { 7723 return addVariant(); 7724 } else if (name.equals("observedSeq")) { 7725 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.observedSeq"); 7726 } else if (name.equals("quality")) { 7727 return addQuality(); 7728 } else if (name.equals("readCoverage")) { 7729 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.readCoverage"); 7730 } else if (name.equals("repository")) { 7731 return addRepository(); 7732 } else if (name.equals("pointer")) { 7733 return addPointer(); 7734 } else if (name.equals("structureVariant")) { 7735 return addStructureVariant(); 7736 } else 7737 return super.addChild(name); 7738 } 7739 7740 public String fhirType() { 7741 return "MolecularSequence"; 7742 7743 } 7744 7745 public MolecularSequence copy() { 7746 MolecularSequence dst = new MolecularSequence(); 7747 copyValues(dst); 7748 return dst; 7749 } 7750 7751 public void copyValues(MolecularSequence dst) { 7752 super.copyValues(dst); 7753 if (identifier != null) { 7754 dst.identifier = new ArrayList<Identifier>(); 7755 for (Identifier i : identifier) 7756 dst.identifier.add(i.copy()); 7757 } 7758 ; 7759 dst.type = type == null ? null : type.copy(); 7760 dst.coordinateSystem = coordinateSystem == null ? null : coordinateSystem.copy(); 7761 dst.patient = patient == null ? null : patient.copy(); 7762 dst.specimen = specimen == null ? null : specimen.copy(); 7763 dst.device = device == null ? null : device.copy(); 7764 dst.performer = performer == null ? null : performer.copy(); 7765 dst.quantity = quantity == null ? null : quantity.copy(); 7766 dst.referenceSeq = referenceSeq == null ? null : referenceSeq.copy(); 7767 if (variant != null) { 7768 dst.variant = new ArrayList<MolecularSequenceVariantComponent>(); 7769 for (MolecularSequenceVariantComponent i : variant) 7770 dst.variant.add(i.copy()); 7771 } 7772 ; 7773 dst.observedSeq = observedSeq == null ? null : observedSeq.copy(); 7774 if (quality != null) { 7775 dst.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7776 for (MolecularSequenceQualityComponent i : quality) 7777 dst.quality.add(i.copy()); 7778 } 7779 ; 7780 dst.readCoverage = readCoverage == null ? null : readCoverage.copy(); 7781 if (repository != null) { 7782 dst.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7783 for (MolecularSequenceRepositoryComponent i : repository) 7784 dst.repository.add(i.copy()); 7785 } 7786 ; 7787 if (pointer != null) { 7788 dst.pointer = new ArrayList<Reference>(); 7789 for (Reference i : pointer) 7790 dst.pointer.add(i.copy()); 7791 } 7792 ; 7793 if (structureVariant != null) { 7794 dst.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7795 for (MolecularSequenceStructureVariantComponent i : structureVariant) 7796 dst.structureVariant.add(i.copy()); 7797 } 7798 ; 7799 } 7800 7801 protected MolecularSequence typedCopy() { 7802 return copy(); 7803 } 7804 7805 @Override 7806 public boolean equalsDeep(Base other_) { 7807 if (!super.equalsDeep(other_)) 7808 return false; 7809 if (!(other_ instanceof MolecularSequence)) 7810 return false; 7811 MolecularSequence o = (MolecularSequence) other_; 7812 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 7813 && compareDeep(coordinateSystem, o.coordinateSystem, true) && compareDeep(patient, o.patient, true) 7814 && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 7815 && compareDeep(performer, o.performer, true) && compareDeep(quantity, o.quantity, true) 7816 && compareDeep(referenceSeq, o.referenceSeq, true) && compareDeep(variant, o.variant, true) 7817 && compareDeep(observedSeq, o.observedSeq, true) && compareDeep(quality, o.quality, true) 7818 && compareDeep(readCoverage, o.readCoverage, true) && compareDeep(repository, o.repository, true) 7819 && compareDeep(pointer, o.pointer, true) && compareDeep(structureVariant, o.structureVariant, true); 7820 } 7821 7822 @Override 7823 public boolean equalsShallow(Base other_) { 7824 if (!super.equalsShallow(other_)) 7825 return false; 7826 if (!(other_ instanceof MolecularSequence)) 7827 return false; 7828 MolecularSequence o = (MolecularSequence) other_; 7829 return compareValues(type, o.type, true) && compareValues(coordinateSystem, o.coordinateSystem, true) 7830 && compareValues(observedSeq, o.observedSeq, true) && compareValues(readCoverage, o.readCoverage, true); 7831 } 7832 7833 public boolean isEmpty() { 7834 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coordinateSystem, patient, 7835 specimen, device, performer, quantity, referenceSeq, variant, observedSeq, quality, readCoverage, repository, 7836 pointer, structureVariant); 7837 } 7838 7839 @Override 7840 public ResourceType getResourceType() { 7841 return ResourceType.MolecularSequence; 7842 } 7843 7844 /** 7845 * Search parameter: <b>identifier</b> 7846 * <p> 7847 * Description: <b>The unique identity for a particular sequence</b><br> 7848 * Type: <b>token</b><br> 7849 * Path: <b>MolecularSequence.identifier</b><br> 7850 * </p> 7851 */ 7852 @SearchParamDefinition(name = "identifier", path = "MolecularSequence.identifier", description = "The unique identity for a particular sequence", type = "token") 7853 public static final String SP_IDENTIFIER = "identifier"; 7854 /** 7855 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7856 * <p> 7857 * Description: <b>The unique identity for a particular sequence</b><br> 7858 * Type: <b>token</b><br> 7859 * Path: <b>MolecularSequence.identifier</b><br> 7860 * </p> 7861 */ 7862 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7863 SP_IDENTIFIER); 7864 7865 /** 7866 * Search parameter: <b>referenceseqid-variant-coordinate</b> 7867 * <p> 7868 * Description: <b>Search parameter by reference sequence and variant 7869 * coordinate. This will refer to part of a locus or part of a gene where search 7870 * region will be represented in 1-based system. Since the coordinateSystem can 7871 * either be 0-based or 1-based, this search query will include the result of 7872 * both coordinateSystem that contains the equivalent segment of the gene or 7873 * whole genome sequence. For example, a search for sequence can be represented 7874 * as `referenceSeqId-variant-coordinate=NC_000001.11$lt345$gt123`, this means 7875 * it will search for the MolecularSequence resource with variants on 7876 * NC_000001.11 and with position >123 and <345, where in 1-based system 7877 * resource, all strings within region NC_000001.11:124-344 will be revealed, 7878 * while in 0-based system resource, all strings within region 7879 * NC_000001.11:123-344 will be revealed. You may want to check detail about 7880 * 0-based v.s. 1-based above.</b><br> 7881 * Type: <b>composite</b><br> 7882 * Path: <b></b><br> 7883 * </p> 7884 */ 7885 @SearchParamDefinition(name = "referenceseqid-variant-coordinate", path = "MolecularSequence.variant", description = "Search parameter by reference sequence and variant coordinate. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `referenceSeqId-variant-coordinate=NC_000001.11$lt345$gt123`, this means it will search for the MolecularSequence resource with variants on NC_000001.11 and with position >123 and <345, where in 1-based system resource, all strings within region NC_000001.11:124-344 will be revealed, while in 0-based system resource, all strings within region NC_000001.11:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 7886 "referenceseqid", "variant-start" }) 7887 public static final String SP_REFERENCESEQID_VARIANT_COORDINATE = "referenceseqid-variant-coordinate"; 7888 /** 7889 * <b>Fluent Client</b> search parameter constant for 7890 * <b>referenceseqid-variant-coordinate</b> 7891 * <p> 7892 * Description: <b>Search parameter by reference sequence and variant 7893 * coordinate. This will refer to part of a locus or part of a gene where search 7894 * region will be represented in 1-based system. Since the coordinateSystem can 7895 * either be 0-based or 1-based, this search query will include the result of 7896 * both coordinateSystem that contains the equivalent segment of the gene or 7897 * whole genome sequence. For example, a search for sequence can be represented 7898 * as `referenceSeqId-variant-coordinate=NC_000001.11$lt345$gt123`, this means 7899 * it will search for the MolecularSequence resource with variants on 7900 * NC_000001.11 and with position >123 and <345, where in 1-based system 7901 * resource, all strings within region NC_000001.11:124-344 will be revealed, 7902 * while in 0-based system resource, all strings within region 7903 * NC_000001.11:123-344 will be revealed. You may want to check detail about 7904 * 0-based v.s. 1-based above.</b><br> 7905 * Type: <b>composite</b><br> 7906 * Path: <b></b><br> 7907 * </p> 7908 */ 7909 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> REFERENCESEQID_VARIANT_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 7910 SP_REFERENCESEQID_VARIANT_COORDINATE); 7911 7912 /** 7913 * Search parameter: <b>chromosome</b> 7914 * <p> 7915 * Description: <b>Chromosome number of the reference sequence</b><br> 7916 * Type: <b>token</b><br> 7917 * Path: <b>MolecularSequence.referenceSeq.chromosome</b><br> 7918 * </p> 7919 */ 7920 @SearchParamDefinition(name = "chromosome", path = "MolecularSequence.referenceSeq.chromosome", description = "Chromosome number of the reference sequence", type = "token") 7921 public static final String SP_CHROMOSOME = "chromosome"; 7922 /** 7923 * <b>Fluent Client</b> search parameter constant for <b>chromosome</b> 7924 * <p> 7925 * Description: <b>Chromosome number of the reference sequence</b><br> 7926 * Type: <b>token</b><br> 7927 * Path: <b>MolecularSequence.referenceSeq.chromosome</b><br> 7928 * </p> 7929 */ 7930 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHROMOSOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7931 SP_CHROMOSOME); 7932 7933 /** 7934 * Search parameter: <b>window-end</b> 7935 * <p> 7936 * Description: <b>End position (0-based exclusive, which menas the acid at this 7937 * position will not be included, 1-based inclusive, which means the acid at 7938 * this position will be included) of the reference sequence.</b><br> 7939 * Type: <b>number</b><br> 7940 * Path: <b>MolecularSequence.referenceSeq.windowEnd</b><br> 7941 * </p> 7942 */ 7943 @SearchParamDefinition(name = "window-end", path = "MolecularSequence.referenceSeq.windowEnd", description = "End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.", type = "number") 7944 public static final String SP_WINDOW_END = "window-end"; 7945 /** 7946 * <b>Fluent Client</b> search parameter constant for <b>window-end</b> 7947 * <p> 7948 * Description: <b>End position (0-based exclusive, which menas the acid at this 7949 * position will not be included, 1-based inclusive, which means the acid at 7950 * this position will be included) of the reference sequence.</b><br> 7951 * Type: <b>number</b><br> 7952 * Path: <b>MolecularSequence.referenceSeq.windowEnd</b><br> 7953 * </p> 7954 */ 7955 public static final ca.uhn.fhir.rest.gclient.NumberClientParam WINDOW_END = new ca.uhn.fhir.rest.gclient.NumberClientParam( 7956 SP_WINDOW_END); 7957 7958 /** 7959 * Search parameter: <b>type</b> 7960 * <p> 7961 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 7962 * Type: <b>token</b><br> 7963 * Path: <b>MolecularSequence.type</b><br> 7964 * </p> 7965 */ 7966 @SearchParamDefinition(name = "type", path = "MolecularSequence.type", description = "Amino Acid Sequence/ DNA Sequence / RNA Sequence", type = "token") 7967 public static final String SP_TYPE = "type"; 7968 /** 7969 * <b>Fluent Client</b> search parameter constant for <b>type</b> 7970 * <p> 7971 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 7972 * Type: <b>token</b><br> 7973 * Path: <b>MolecularSequence.type</b><br> 7974 * </p> 7975 */ 7976 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 7977 SP_TYPE); 7978 7979 /** 7980 * Search parameter: <b>window-start</b> 7981 * <p> 7982 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 7983 * means the nucleic acid or amino acid at this position will be included) of 7984 * the reference sequence.</b><br> 7985 * Type: <b>number</b><br> 7986 * Path: <b>MolecularSequence.referenceSeq.windowStart</b><br> 7987 * </p> 7988 */ 7989 @SearchParamDefinition(name = "window-start", path = "MolecularSequence.referenceSeq.windowStart", description = "Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.", type = "number") 7990 public static final String SP_WINDOW_START = "window-start"; 7991 /** 7992 * <b>Fluent Client</b> search parameter constant for <b>window-start</b> 7993 * <p> 7994 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 7995 * means the nucleic acid or amino acid at this position will be included) of 7996 * the reference sequence.</b><br> 7997 * Type: <b>number</b><br> 7998 * Path: <b>MolecularSequence.referenceSeq.windowStart</b><br> 7999 * </p> 8000 */ 8001 public static final ca.uhn.fhir.rest.gclient.NumberClientParam WINDOW_START = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8002 SP_WINDOW_START); 8003 8004 /** 8005 * Search parameter: <b>variant-end</b> 8006 * <p> 8007 * Description: <b>End position (0-based exclusive, which menas the acid at this 8008 * position will not be included, 1-based inclusive, which means the acid at 8009 * this position will be included) of the variant.</b><br> 8010 * Type: <b>number</b><br> 8011 * Path: <b>MolecularSequence.variant.end</b><br> 8012 * </p> 8013 */ 8014 @SearchParamDefinition(name = "variant-end", path = "MolecularSequence.variant.end", description = "End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the variant.", type = "number") 8015 public static final String SP_VARIANT_END = "variant-end"; 8016 /** 8017 * <b>Fluent Client</b> search parameter constant for <b>variant-end</b> 8018 * <p> 8019 * Description: <b>End position (0-based exclusive, which menas the acid at this 8020 * position will not be included, 1-based inclusive, which means the acid at 8021 * this position will be included) of the variant.</b><br> 8022 * Type: <b>number</b><br> 8023 * Path: <b>MolecularSequence.variant.end</b><br> 8024 * </p> 8025 */ 8026 public static final ca.uhn.fhir.rest.gclient.NumberClientParam VARIANT_END = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8027 SP_VARIANT_END); 8028 8029 /** 8030 * Search parameter: <b>chromosome-variant-coordinate</b> 8031 * <p> 8032 * Description: <b>Search parameter by chromosome and variant coordinate. This 8033 * will refer to part of a locus or part of a gene where search region will be 8034 * represented in 1-based system. Since the coordinateSystem can either be 8035 * 0-based or 1-based, this search query will include the result of both 8036 * coordinateSystem that contains the equivalent segment of the gene or whole 8037 * genome sequence. For example, a search for sequence can be represented as 8038 * `chromosome-variant-coordinate=1$lt345$gt123`, this means it will search for 8039 * the MolecularSequence resource with variants on chromosome 1 and with 8040 * position >123 and <345, where in 1-based system resource, all strings within 8041 * region 1:124-344 will be revealed, while in 0-based system resource, all 8042 * strings within region 1:123-344 will be revealed. You may want to check 8043 * detail about 0-based v.s. 1-based above.</b><br> 8044 * Type: <b>composite</b><br> 8045 * Path: <b></b><br> 8046 * </p> 8047 */ 8048 @SearchParamDefinition(name = "chromosome-variant-coordinate", path = "MolecularSequence.variant", description = "Search parameter by chromosome and variant coordinate. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `chromosome-variant-coordinate=1$lt345$gt123`, this means it will search for the MolecularSequence resource with variants on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8049 "chromosome", "variant-start" }) 8050 public static final String SP_CHROMOSOME_VARIANT_COORDINATE = "chromosome-variant-coordinate"; 8051 /** 8052 * <b>Fluent Client</b> search parameter constant for 8053 * <b>chromosome-variant-coordinate</b> 8054 * <p> 8055 * Description: <b>Search parameter by chromosome and variant coordinate. This 8056 * will refer to part of a locus or part of a gene where search region will be 8057 * represented in 1-based system. Since the coordinateSystem can either be 8058 * 0-based or 1-based, this search query will include the result of both 8059 * coordinateSystem that contains the equivalent segment of the gene or whole 8060 * genome sequence. For example, a search for sequence can be represented as 8061 * `chromosome-variant-coordinate=1$lt345$gt123`, this means it will search for 8062 * the MolecularSequence resource with variants on chromosome 1 and with 8063 * position >123 and <345, where in 1-based system resource, all strings within 8064 * region 1:124-344 will be revealed, while in 0-based system resource, all 8065 * strings within region 1:123-344 will be revealed. You may want to check 8066 * detail about 0-based v.s. 1-based above.</b><br> 8067 * Type: <b>composite</b><br> 8068 * Path: <b></b><br> 8069 * </p> 8070 */ 8071 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> CHROMOSOME_VARIANT_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8072 SP_CHROMOSOME_VARIANT_COORDINATE); 8073 8074 /** 8075 * Search parameter: <b>patient</b> 8076 * <p> 8077 * Description: <b>The subject that the observation is about</b><br> 8078 * Type: <b>reference</b><br> 8079 * Path: <b>MolecularSequence.patient</b><br> 8080 * </p> 8081 */ 8082 @SearchParamDefinition(name = "patient", path = "MolecularSequence.patient", description = "The subject that the observation is about", type = "reference", providesMembershipIn = { 8083 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 8084 public static final String SP_PATIENT = "patient"; 8085 /** 8086 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 8087 * <p> 8088 * Description: <b>The subject that the observation is about</b><br> 8089 * Type: <b>reference</b><br> 8090 * Path: <b>MolecularSequence.patient</b><br> 8091 * </p> 8092 */ 8093 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 8094 SP_PATIENT); 8095 8096 /** 8097 * Constant for fluent queries to be used to add include statements. Specifies 8098 * the path value of "<b>MolecularSequence:patient</b>". 8099 */ 8100 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 8101 "MolecularSequence:patient").toLocked(); 8102 8103 /** 8104 * Search parameter: <b>variant-start</b> 8105 * <p> 8106 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 8107 * means the nucleic acid or amino acid at this position will be included) of 8108 * the variant.</b><br> 8109 * Type: <b>number</b><br> 8110 * Path: <b>MolecularSequence.variant.start</b><br> 8111 * </p> 8112 */ 8113 @SearchParamDefinition(name = "variant-start", path = "MolecularSequence.variant.start", description = "Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the variant.", type = "number") 8114 public static final String SP_VARIANT_START = "variant-start"; 8115 /** 8116 * <b>Fluent Client</b> search parameter constant for <b>variant-start</b> 8117 * <p> 8118 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 8119 * means the nucleic acid or amino acid at this position will be included) of 8120 * the variant.</b><br> 8121 * Type: <b>number</b><br> 8122 * Path: <b>MolecularSequence.variant.start</b><br> 8123 * </p> 8124 */ 8125 public static final ca.uhn.fhir.rest.gclient.NumberClientParam VARIANT_START = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8126 SP_VARIANT_START); 8127 8128 /** 8129 * Search parameter: <b>chromosome-window-coordinate</b> 8130 * <p> 8131 * Description: <b>Search parameter by chromosome and window. This will refer to 8132 * part of a locus or part of a gene where search region will be represented in 8133 * 1-based system. Since the coordinateSystem can either be 0-based or 1-based, 8134 * this search query will include the result of both coordinateSystem that 8135 * contains the equivalent segment of the gene or whole genome sequence. For 8136 * example, a search for sequence can be represented as 8137 * `chromosome-window-coordinate=1$lt345$gt123`, this means it will search for 8138 * the MolecularSequence resource with a window on chromosome 1 and with 8139 * position >123 and <345, where in 1-based system resource, all strings within 8140 * region 1:124-344 will be revealed, while in 0-based system resource, all 8141 * strings within region 1:123-344 will be revealed. You may want to check 8142 * detail about 0-based v.s. 1-based above.</b><br> 8143 * Type: <b>composite</b><br> 8144 * Path: <b></b><br> 8145 * </p> 8146 */ 8147 @SearchParamDefinition(name = "chromosome-window-coordinate", path = "MolecularSequence.referenceSeq", description = "Search parameter by chromosome and window. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `chromosome-window-coordinate=1$lt345$gt123`, this means it will search for the MolecularSequence resource with a window on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8148 "chromosome", "window-start" }) 8149 public static final String SP_CHROMOSOME_WINDOW_COORDINATE = "chromosome-window-coordinate"; 8150 /** 8151 * <b>Fluent Client</b> search parameter constant for 8152 * <b>chromosome-window-coordinate</b> 8153 * <p> 8154 * Description: <b>Search parameter by chromosome and window. This will refer to 8155 * part of a locus or part of a gene where search region will be represented in 8156 * 1-based system. Since the coordinateSystem can either be 0-based or 1-based, 8157 * this search query will include the result of both coordinateSystem that 8158 * contains the equivalent segment of the gene or whole genome sequence. For 8159 * example, a search for sequence can be represented as 8160 * `chromosome-window-coordinate=1$lt345$gt123`, this means it will search for 8161 * the MolecularSequence resource with a window on chromosome 1 and with 8162 * position >123 and <345, where in 1-based system resource, all strings within 8163 * region 1:124-344 will be revealed, while in 0-based system resource, all 8164 * strings within region 1:123-344 will be revealed. You may want to check 8165 * detail about 0-based v.s. 1-based above.</b><br> 8166 * Type: <b>composite</b><br> 8167 * Path: <b></b><br> 8168 * </p> 8169 */ 8170 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> CHROMOSOME_WINDOW_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8171 SP_CHROMOSOME_WINDOW_COORDINATE); 8172 8173 /** 8174 * Search parameter: <b>referenceseqid-window-coordinate</b> 8175 * <p> 8176 * Description: <b>Search parameter by reference sequence and window. This will 8177 * refer to part of a locus or part of a gene where search region will be 8178 * represented in 1-based system. Since the coordinateSystem can either be 8179 * 0-based or 1-based, this search query will include the result of both 8180 * coordinateSystem that contains the equivalent segment of the gene or whole 8181 * genome sequence. For example, a search for sequence can be represented as 8182 * `referenceSeqId-window-coordinate=NC_000001.11$lt345$gt123`, this means it 8183 * will search for the MolecularSequence resource with a window on NC_000001.11 8184 * and with position >123 and <345, where in 1-based system resource, all 8185 * strings within region NC_000001.11:124-344 will be revealed, while in 0-based 8186 * system resource, all strings within region NC_000001.11:123-344 will be 8187 * revealed. You may want to check detail about 0-based v.s. 1-based 8188 * above.</b><br> 8189 * Type: <b>composite</b><br> 8190 * Path: <b></b><br> 8191 * </p> 8192 */ 8193 @SearchParamDefinition(name = "referenceseqid-window-coordinate", path = "MolecularSequence.referenceSeq", description = "Search parameter by reference sequence and window. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `referenceSeqId-window-coordinate=NC_000001.11$lt345$gt123`, this means it will search for the MolecularSequence resource with a window on NC_000001.11 and with position >123 and <345, where in 1-based system resource, all strings within region NC_000001.11:124-344 will be revealed, while in 0-based system resource, all strings within region NC_000001.11:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8194 "referenceseqid", "window-start" }) 8195 public static final String SP_REFERENCESEQID_WINDOW_COORDINATE = "referenceseqid-window-coordinate"; 8196 /** 8197 * <b>Fluent Client</b> search parameter constant for 8198 * <b>referenceseqid-window-coordinate</b> 8199 * <p> 8200 * Description: <b>Search parameter by reference sequence and window. This will 8201 * refer to part of a locus or part of a gene where search region will be 8202 * represented in 1-based system. Since the coordinateSystem can either be 8203 * 0-based or 1-based, this search query will include the result of both 8204 * coordinateSystem that contains the equivalent segment of the gene or whole 8205 * genome sequence. For example, a search for sequence can be represented as 8206 * `referenceSeqId-window-coordinate=NC_000001.11$lt345$gt123`, this means it 8207 * will search for the MolecularSequence resource with a window on NC_000001.11 8208 * and with position >123 and <345, where in 1-based system resource, all 8209 * strings within region NC_000001.11:124-344 will be revealed, while in 0-based 8210 * system resource, all strings within region NC_000001.11:123-344 will be 8211 * revealed. You may want to check detail about 0-based v.s. 1-based 8212 * above.</b><br> 8213 * Type: <b>composite</b><br> 8214 * Path: <b></b><br> 8215 * </p> 8216 */ 8217 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> REFERENCESEQID_WINDOW_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8218 SP_REFERENCESEQID_WINDOW_COORDINATE); 8219 8220 /** 8221 * Search parameter: <b>referenceseqid</b> 8222 * <p> 8223 * Description: <b>Reference Sequence of the sequence</b><br> 8224 * Type: <b>token</b><br> 8225 * Path: <b>MolecularSequence.referenceSeq.referenceSeqId</b><br> 8226 * </p> 8227 */ 8228 @SearchParamDefinition(name = "referenceseqid", path = "MolecularSequence.referenceSeq.referenceSeqId", description = "Reference Sequence of the sequence", type = "token") 8229 public static final String SP_REFERENCESEQID = "referenceseqid"; 8230 /** 8231 * <b>Fluent Client</b> search parameter constant for <b>referenceseqid</b> 8232 * <p> 8233 * Description: <b>Reference Sequence of the sequence</b><br> 8234 * Type: <b>token</b><br> 8235 * Path: <b>MolecularSequence.referenceSeq.referenceSeqId</b><br> 8236 * </p> 8237 */ 8238 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REFERENCESEQID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8239 SP_REFERENCESEQID); 8240 8241}