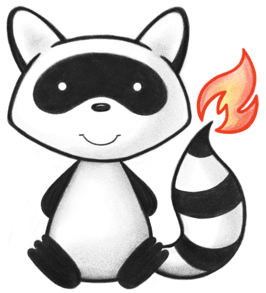
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * An amount of economic utility in some recognized currency. 047 */ 048@DatatypeDef(name = "Money") 049public class Money extends Type implements ICompositeType { 050 051 /** 052 * Numerical value (with implicit precision). 053 */ 054 @Child(name = "value", type = { DecimalType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 055 @Description(shortDefinition = "Numerical value (with implicit precision)", formalDefinition = "Numerical value (with implicit precision).") 056 protected DecimalType value; 057 058 /** 059 * ISO 4217 Currency Code. 060 */ 061 @Child(name = "currency", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 062 @Description(shortDefinition = "ISO 4217 Currency Code", formalDefinition = "ISO 4217 Currency Code.") 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/currencies") 064 protected CodeType currency; 065 066 private static final long serialVersionUID = -484637938L; 067 068 /** 069 * Constructor 070 */ 071 public Money() { 072 super(); 073 } 074 075 /** 076 * @return {@link #value} (Numerical value (with implicit precision).). This is 077 * the underlying object with id, value and extensions. The accessor 078 * "getValue" gives direct access to the value 079 */ 080 public DecimalType getValueElement() { 081 if (this.value == null) 082 if (Configuration.errorOnAutoCreate()) 083 throw new Error("Attempt to auto-create Money.value"); 084 else if (Configuration.doAutoCreate()) 085 this.value = new DecimalType(); // bb 086 return this.value; 087 } 088 089 public boolean hasValueElement() { 090 return this.value != null && !this.value.isEmpty(); 091 } 092 093 public boolean hasValue() { 094 return this.value != null && !this.value.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #value} (Numerical value (with implicit precision).). 099 * This is the underlying object with id, value and extensions. The 100 * accessor "getValue" gives direct access to the value 101 */ 102 public Money setValueElement(DecimalType value) { 103 this.value = value; 104 return this; 105 } 106 107 /** 108 * @return Numerical value (with implicit precision). 109 */ 110 public BigDecimal getValue() { 111 return this.value == null ? null : this.value.getValue(); 112 } 113 114 /** 115 * @param value Numerical value (with implicit precision). 116 */ 117 public Money setValue(BigDecimal value) { 118 if (value == null) 119 this.value = null; 120 else { 121 if (this.value == null) 122 this.value = new DecimalType(); 123 this.value.setValue(value); 124 } 125 return this; 126 } 127 128 /** 129 * @param value Numerical value (with implicit precision). 130 */ 131 public Money setValue(long value) { 132 this.value = new DecimalType(); 133 this.value.setValue(value); 134 return this; 135 } 136 137 /** 138 * @param value Numerical value (with implicit precision). 139 */ 140 public Money setValue(double value) { 141 this.value = new DecimalType(); 142 this.value.setValue(value); 143 return this; 144 } 145 146 /** 147 * @return {@link #currency} (ISO 4217 Currency Code.). This is the underlying 148 * object with id, value and extensions. The accessor "getCurrency" 149 * gives direct access to the value 150 */ 151 public CodeType getCurrencyElement() { 152 if (this.currency == null) 153 if (Configuration.errorOnAutoCreate()) 154 throw new Error("Attempt to auto-create Money.currency"); 155 else if (Configuration.doAutoCreate()) 156 this.currency = new CodeType(); // bb 157 return this.currency; 158 } 159 160 public boolean hasCurrencyElement() { 161 return this.currency != null && !this.currency.isEmpty(); 162 } 163 164 public boolean hasCurrency() { 165 return this.currency != null && !this.currency.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #currency} (ISO 4217 Currency Code.). This is the 170 * underlying object with id, value and extensions. The accessor 171 * "getCurrency" gives direct access to the value 172 */ 173 public Money setCurrencyElement(CodeType value) { 174 this.currency = value; 175 return this; 176 } 177 178 /** 179 * @return ISO 4217 Currency Code. 180 */ 181 public String getCurrency() { 182 return this.currency == null ? null : this.currency.getValue(); 183 } 184 185 /** 186 * @param value ISO 4217 Currency Code. 187 */ 188 public Money setCurrency(String value) { 189 if (Utilities.noString(value)) 190 this.currency = null; 191 else { 192 if (this.currency == null) 193 this.currency = new CodeType(); 194 this.currency.setValue(value); 195 } 196 return this; 197 } 198 199 protected void listChildren(List<Property> children) { 200 super.listChildren(children); 201 children.add(new Property("value", "decimal", "Numerical value (with implicit precision).", 0, 1, value)); 202 children.add(new Property("currency", "code", "ISO 4217 Currency Code.", 0, 1, currency)); 203 } 204 205 @Override 206 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 207 switch (_hash) { 208 case 111972721: 209 /* value */ return new Property("value", "decimal", "Numerical value (with implicit precision).", 0, 1, value); 210 case 575402001: 211 /* currency */ return new Property("currency", "code", "ISO 4217 Currency Code.", 0, 1, currency); 212 default: 213 return super.getNamedProperty(_hash, _name, _checkValid); 214 } 215 216 } 217 218 @Override 219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 220 switch (hash) { 221 case 111972721: 222 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DecimalType 223 case 575402001: 224 /* currency */ return this.currency == null ? new Base[0] : new Base[] { this.currency }; // CodeType 225 default: 226 return super.getProperty(hash, name, checkValid); 227 } 228 229 } 230 231 @Override 232 public Base setProperty(int hash, String name, Base value) throws FHIRException { 233 switch (hash) { 234 case 111972721: // value 235 this.value = castToDecimal(value); // DecimalType 236 return value; 237 case 575402001: // currency 238 this.currency = castToCode(value); // CodeType 239 return value; 240 default: 241 return super.setProperty(hash, name, value); 242 } 243 244 } 245 246 @Override 247 public Base setProperty(String name, Base value) throws FHIRException { 248 if (name.equals("value")) { 249 this.value = castToDecimal(value); // DecimalType 250 } else if (name.equals("currency")) { 251 this.currency = castToCode(value); // CodeType 252 } else 253 return super.setProperty(name, value); 254 return value; 255 } 256 257 @Override 258 public void removeChild(String name, Base value) throws FHIRException { 259 if (name.equals("value")) { 260 this.value = null; 261 } else if (name.equals("currency")) { 262 this.currency = null; 263 } else 264 super.removeChild(name, value); 265 266 } 267 268 @Override 269 public Base makeProperty(int hash, String name) throws FHIRException { 270 switch (hash) { 271 case 111972721: 272 return getValueElement(); 273 case 575402001: 274 return getCurrencyElement(); 275 default: 276 return super.makeProperty(hash, name); 277 } 278 279 } 280 281 @Override 282 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 283 switch (hash) { 284 case 111972721: 285 /* value */ return new String[] { "decimal" }; 286 case 575402001: 287 /* currency */ return new String[] { "code" }; 288 default: 289 return super.getTypesForProperty(hash, name); 290 } 291 292 } 293 294 @Override 295 public Base addChild(String name) throws FHIRException { 296 if (name.equals("value")) { 297 throw new FHIRException("Cannot call addChild on a singleton property Money.value"); 298 } else if (name.equals("currency")) { 299 throw new FHIRException("Cannot call addChild on a singleton property Money.currency"); 300 } else 301 return super.addChild(name); 302 } 303 304 public String fhirType() { 305 return "Money"; 306 307 } 308 309 public Money copy() { 310 Money dst = new Money(); 311 copyValues(dst); 312 return dst; 313 } 314 315 public void copyValues(Money dst) { 316 super.copyValues(dst); 317 dst.value = value == null ? null : value.copy(); 318 dst.currency = currency == null ? null : currency.copy(); 319 } 320 321 protected Money typedCopy() { 322 return copy(); 323 } 324 325 @Override 326 public boolean equalsDeep(Base other_) { 327 if (!super.equalsDeep(other_)) 328 return false; 329 if (!(other_ instanceof Money)) 330 return false; 331 Money o = (Money) other_; 332 return compareDeep(value, o.value, true) && compareDeep(currency, o.currency, true); 333 } 334 335 @Override 336 public boolean equalsShallow(Base other_) { 337 if (!super.equalsShallow(other_)) 338 return false; 339 if (!(other_ instanceof Money)) 340 return false; 341 Money o = (Money) other_; 342 return compareValues(value, o.value, true) && compareValues(currency, o.currency, true); 343 } 344 345 public boolean isEmpty() { 346 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, currency); 347 } 348 349}