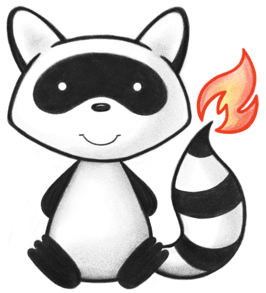
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A curated namespace that issues unique symbols within that namespace for the 052 * identification of concepts, people, devices, etc. Represents a "System" used 053 * within the Identifier and Coding data types. 054 */ 055@ResourceDef(name = "NamingSystem", profile = "http://hl7.org/fhir/StructureDefinition/NamingSystem") 056@ChildOrder(names = { "name", "status", "kind", "date", "publisher", "contact", "responsible", "type", "description", 057 "useContext", "jurisdiction", "usage", "uniqueId" }) 058public class NamingSystem extends MetadataResource { 059 060 public enum NamingSystemType { 061 /** 062 * The naming system is used to define concepts and symbols to represent those 063 * concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 064 */ 065 CODESYSTEM, 066 /** 067 * The naming system is used to manage identifiers (e.g. license numbers, order 068 * numbers, etc.). 069 */ 070 IDENTIFIER, 071 /** 072 * The naming system is used as the root for other identifiers and naming 073 * systems. 074 */ 075 ROOT, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 081 public static NamingSystemType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("codesystem".equals(codeString)) 085 return CODESYSTEM; 086 if ("identifier".equals(codeString)) 087 return IDENTIFIER; 088 if ("root".equals(codeString)) 089 return ROOT; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 094 } 095 096 public String toCode() { 097 switch (this) { 098 case CODESYSTEM: 099 return "codesystem"; 100 case IDENTIFIER: 101 return "identifier"; 102 case ROOT: 103 return "root"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 switch (this) { 113 case CODESYSTEM: 114 return "http://hl7.org/fhir/namingsystem-type"; 115 case IDENTIFIER: 116 return "http://hl7.org/fhir/namingsystem-type"; 117 case ROOT: 118 return "http://hl7.org/fhir/namingsystem-type"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case CODESYSTEM: 129 return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 130 case IDENTIFIER: 131 return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 132 case ROOT: 133 return "The naming system is used as the root for other identifiers and naming systems."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case CODESYSTEM: 144 return "Code System"; 145 case IDENTIFIER: 146 return "Identifier"; 147 case ROOT: 148 return "Root"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 } 156 157 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 158 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 159 if (codeString == null || "".equals(codeString)) 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("codesystem".equals(codeString)) 163 return NamingSystemType.CODESYSTEM; 164 if ("identifier".equals(codeString)) 165 return NamingSystemType.IDENTIFIER; 166 if ("root".equals(codeString)) 167 return NamingSystemType.ROOT; 168 throw new IllegalArgumentException("Unknown NamingSystemType code '" + codeString + "'"); 169 } 170 171 public Enumeration<NamingSystemType> fromType(PrimitiveType<?> code) throws FHIRException { 172 if (code == null) 173 return null; 174 if (code.isEmpty()) 175 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 176 String codeString = code.asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 179 if ("codesystem".equals(codeString)) 180 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM, code); 181 if ("identifier".equals(codeString)) 182 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER, code); 183 if ("root".equals(codeString)) 184 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT, code); 185 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 186 } 187 188 public String toCode(NamingSystemType code) { 189 if (code == NamingSystemType.CODESYSTEM) 190 return "codesystem"; 191 if (code == NamingSystemType.IDENTIFIER) 192 return "identifier"; 193 if (code == NamingSystemType.ROOT) 194 return "root"; 195 return "?"; 196 } 197 198 public String toSystem(NamingSystemType code) { 199 return code.getSystem(); 200 } 201 } 202 203 public enum NamingSystemIdentifierType { 204 /** 205 * An ISO object identifier; e.g. 1.2.3.4.5. 206 */ 207 OID, 208 /** 209 * A universally unique identifier of the form 210 * a5afddf4-e880-459b-876e-e4591b0acc11. 211 */ 212 UUID, 213 /** 214 * A uniform resource identifier (ideally a URL - uniform resource locator); 215 * e.g. http://unitsofmeasure.org. 216 */ 217 URI, 218 /** 219 * Some other type of unique identifier; e.g. HL7-assigned reserved string such 220 * as LN for LOINC. 221 */ 222 OTHER, 223 /** 224 * added to help the parsers with the generic types 225 */ 226 NULL; 227 228 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 229 if (codeString == null || "".equals(codeString)) 230 return null; 231 if ("oid".equals(codeString)) 232 return OID; 233 if ("uuid".equals(codeString)) 234 return UUID; 235 if ("uri".equals(codeString)) 236 return URI; 237 if ("other".equals(codeString)) 238 return OTHER; 239 if (Configuration.isAcceptInvalidEnums()) 240 return null; 241 else 242 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 243 } 244 245 public String toCode() { 246 switch (this) { 247 case OID: 248 return "oid"; 249 case UUID: 250 return "uuid"; 251 case URI: 252 return "uri"; 253 case OTHER: 254 return "other"; 255 case NULL: 256 return null; 257 default: 258 return "?"; 259 } 260 } 261 262 public String getSystem() { 263 switch (this) { 264 case OID: 265 return "http://hl7.org/fhir/namingsystem-identifier-type"; 266 case UUID: 267 return "http://hl7.org/fhir/namingsystem-identifier-type"; 268 case URI: 269 return "http://hl7.org/fhir/namingsystem-identifier-type"; 270 case OTHER: 271 return "http://hl7.org/fhir/namingsystem-identifier-type"; 272 case NULL: 273 return null; 274 default: 275 return "?"; 276 } 277 } 278 279 public String getDefinition() { 280 switch (this) { 281 case OID: 282 return "An ISO object identifier; e.g. 1.2.3.4.5."; 283 case UUID: 284 return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 285 case URI: 286 return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 287 case OTHER: 288 return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 289 case NULL: 290 return null; 291 default: 292 return "?"; 293 } 294 } 295 296 public String getDisplay() { 297 switch (this) { 298 case OID: 299 return "OID"; 300 case UUID: 301 return "UUID"; 302 case URI: 303 return "URI"; 304 case OTHER: 305 return "Other"; 306 case NULL: 307 return null; 308 default: 309 return "?"; 310 } 311 } 312 } 313 314 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 315 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 316 if (codeString == null || "".equals(codeString)) 317 if (codeString == null || "".equals(codeString)) 318 return null; 319 if ("oid".equals(codeString)) 320 return NamingSystemIdentifierType.OID; 321 if ("uuid".equals(codeString)) 322 return NamingSystemIdentifierType.UUID; 323 if ("uri".equals(codeString)) 324 return NamingSystemIdentifierType.URI; 325 if ("other".equals(codeString)) 326 return NamingSystemIdentifierType.OTHER; 327 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 328 } 329 330 public Enumeration<NamingSystemIdentifierType> fromType(PrimitiveType<?> code) throws FHIRException { 331 if (code == null) 332 return null; 333 if (code.isEmpty()) 334 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 335 String codeString = code.asStringValue(); 336 if (codeString == null || "".equals(codeString)) 337 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 338 if ("oid".equals(codeString)) 339 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID, code); 340 if ("uuid".equals(codeString)) 341 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID, code); 342 if ("uri".equals(codeString)) 343 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI, code); 344 if ("other".equals(codeString)) 345 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER, code); 346 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 347 } 348 349 public String toCode(NamingSystemIdentifierType code) { 350 if (code == NamingSystemIdentifierType.NULL) 351 return null; 352 if (code == NamingSystemIdentifierType.OID) 353 return "oid"; 354 if (code == NamingSystemIdentifierType.UUID) 355 return "uuid"; 356 if (code == NamingSystemIdentifierType.URI) 357 return "uri"; 358 if (code == NamingSystemIdentifierType.OTHER) 359 return "other"; 360 return "?"; 361 } 362 363 public String toSystem(NamingSystemIdentifierType code) { 364 return code.getSystem(); 365 } 366 } 367 368 @Block() 369 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 370 /** 371 * Identifies the unique identifier scheme used for this particular identifier. 372 */ 373 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 374 @Description(shortDefinition = "oid | uuid | uri | other", formalDefinition = "Identifies the unique identifier scheme used for this particular identifier.") 375 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/namingsystem-identifier-type") 376 protected Enumeration<NamingSystemIdentifierType> type; 377 378 /** 379 * The string that should be sent over the wire to identify the code system or 380 * identifier system. 381 */ 382 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 383 @Description(shortDefinition = "The unique identifier", formalDefinition = "The string that should be sent over the wire to identify the code system or identifier system.") 384 protected StringType value; 385 386 /** 387 * Indicates whether this identifier is the "preferred" identifier of this type. 388 */ 389 @Child(name = "preferred", type = { 390 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 391 @Description(shortDefinition = "Is this the id that should be used for this type", formalDefinition = "Indicates whether this identifier is the \"preferred\" identifier of this type.") 392 protected BooleanType preferred; 393 394 /** 395 * Notes about the past or intended usage of this identifier. 396 */ 397 @Child(name = "comment", type = { 398 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 399 @Description(shortDefinition = "Notes about identifier usage", formalDefinition = "Notes about the past or intended usage of this identifier.") 400 protected StringType comment; 401 402 /** 403 * Identifies the period of time over which this identifier is considered 404 * appropriate to refer to the naming system. Outside of this window, the 405 * identifier might be non-deterministic. 406 */ 407 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 408 @Description(shortDefinition = "When is identifier valid?", formalDefinition = "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.") 409 protected Period period; 410 411 private static final long serialVersionUID = -1458889328L; 412 413 /** 414 * Constructor 415 */ 416 public NamingSystemUniqueIdComponent() { 417 super(); 418 } 419 420 /** 421 * Constructor 422 */ 423 public NamingSystemUniqueIdComponent(Enumeration<NamingSystemIdentifierType> type, StringType value) { 424 super(); 425 this.type = type; 426 this.value = value; 427 } 428 429 /** 430 * @return {@link #type} (Identifies the unique identifier scheme used for this 431 * particular identifier.). This is the underlying object with id, value 432 * and extensions. The accessor "getType" gives direct access to the 433 * value 434 */ 435 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 436 if (this.type == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 439 else if (Configuration.doAutoCreate()) 440 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 441 return this.type; 442 } 443 444 public boolean hasTypeElement() { 445 return this.type != null && !this.type.isEmpty(); 446 } 447 448 public boolean hasType() { 449 return this.type != null && !this.type.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #type} (Identifies the unique identifier scheme used for 454 * this particular identifier.). This is the underlying object with 455 * id, value and extensions. The accessor "getType" gives direct 456 * access to the value 457 */ 458 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 459 this.type = value; 460 return this; 461 } 462 463 /** 464 * @return Identifies the unique identifier scheme used for this particular 465 * identifier. 466 */ 467 public NamingSystemIdentifierType getType() { 468 return this.type == null ? null : this.type.getValue(); 469 } 470 471 /** 472 * @param value Identifies the unique identifier scheme used for this particular 473 * identifier. 474 */ 475 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 476 if (this.type == null) 477 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 478 this.type.setValue(value); 479 return this; 480 } 481 482 /** 483 * @return {@link #value} (The string that should be sent over the wire to 484 * identify the code system or identifier system.). This is the 485 * underlying object with id, value and extensions. The accessor 486 * "getValue" gives direct access to the value 487 */ 488 public StringType getValueElement() { 489 if (this.value == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 492 else if (Configuration.doAutoCreate()) 493 this.value = new StringType(); // bb 494 return this.value; 495 } 496 497 public boolean hasValueElement() { 498 return this.value != null && !this.value.isEmpty(); 499 } 500 501 public boolean hasValue() { 502 return this.value != null && !this.value.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #value} (The string that should be sent over the wire to 507 * identify the code system or identifier system.). This is the 508 * underlying object with id, value and extensions. The accessor 509 * "getValue" gives direct access to the value 510 */ 511 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 512 this.value = value; 513 return this; 514 } 515 516 /** 517 * @return The string that should be sent over the wire to identify the code 518 * system or identifier system. 519 */ 520 public String getValue() { 521 return this.value == null ? null : this.value.getValue(); 522 } 523 524 /** 525 * @param value The string that should be sent over the wire to identify the 526 * code system or identifier system. 527 */ 528 public NamingSystemUniqueIdComponent setValue(String value) { 529 if (this.value == null) 530 this.value = new StringType(); 531 this.value.setValue(value); 532 return this; 533 } 534 535 /** 536 * @return {@link #preferred} (Indicates whether this identifier is the 537 * "preferred" identifier of this type.). This is the underlying object 538 * with id, value and extensions. The accessor "getPreferred" gives 539 * direct access to the value 540 */ 541 public BooleanType getPreferredElement() { 542 if (this.preferred == null) 543 if (Configuration.errorOnAutoCreate()) 544 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 545 else if (Configuration.doAutoCreate()) 546 this.preferred = new BooleanType(); // bb 547 return this.preferred; 548 } 549 550 public boolean hasPreferredElement() { 551 return this.preferred != null && !this.preferred.isEmpty(); 552 } 553 554 public boolean hasPreferred() { 555 return this.preferred != null && !this.preferred.isEmpty(); 556 } 557 558 /** 559 * @param value {@link #preferred} (Indicates whether this identifier is the 560 * "preferred" identifier of this type.). This is the underlying 561 * object with id, value and extensions. The accessor 562 * "getPreferred" gives direct access to the value 563 */ 564 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 565 this.preferred = value; 566 return this; 567 } 568 569 /** 570 * @return Indicates whether this identifier is the "preferred" identifier of 571 * this type. 572 */ 573 public boolean getPreferred() { 574 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 575 } 576 577 /** 578 * @param value Indicates whether this identifier is the "preferred" identifier 579 * of this type. 580 */ 581 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 582 if (this.preferred == null) 583 this.preferred = new BooleanType(); 584 this.preferred.setValue(value); 585 return this; 586 } 587 588 /** 589 * @return {@link #comment} (Notes about the past or intended usage of this 590 * identifier.). This is the underlying object with id, value and 591 * extensions. The accessor "getComment" gives direct access to the 592 * value 593 */ 594 public StringType getCommentElement() { 595 if (this.comment == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.comment"); 598 else if (Configuration.doAutoCreate()) 599 this.comment = new StringType(); // bb 600 return this.comment; 601 } 602 603 public boolean hasCommentElement() { 604 return this.comment != null && !this.comment.isEmpty(); 605 } 606 607 public boolean hasComment() { 608 return this.comment != null && !this.comment.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #comment} (Notes about the past or intended usage of this 613 * identifier.). This is the underlying object with id, value and 614 * extensions. The accessor "getComment" gives direct access to the 615 * value 616 */ 617 public NamingSystemUniqueIdComponent setCommentElement(StringType value) { 618 this.comment = value; 619 return this; 620 } 621 622 /** 623 * @return Notes about the past or intended usage of this identifier. 624 */ 625 public String getComment() { 626 return this.comment == null ? null : this.comment.getValue(); 627 } 628 629 /** 630 * @param value Notes about the past or intended usage of this identifier. 631 */ 632 public NamingSystemUniqueIdComponent setComment(String value) { 633 if (Utilities.noString(value)) 634 this.comment = null; 635 else { 636 if (this.comment == null) 637 this.comment = new StringType(); 638 this.comment.setValue(value); 639 } 640 return this; 641 } 642 643 /** 644 * @return {@link #period} (Identifies the period of time over which this 645 * identifier is considered appropriate to refer to the naming system. 646 * Outside of this window, the identifier might be non-deterministic.) 647 */ 648 public Period getPeriod() { 649 if (this.period == null) 650 if (Configuration.errorOnAutoCreate()) 651 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 652 else if (Configuration.doAutoCreate()) 653 this.period = new Period(); // cc 654 return this.period; 655 } 656 657 public boolean hasPeriod() { 658 return this.period != null && !this.period.isEmpty(); 659 } 660 661 /** 662 * @param value {@link #period} (Identifies the period of time over which this 663 * identifier is considered appropriate to refer to the naming 664 * system. Outside of this window, the identifier might be 665 * non-deterministic.) 666 */ 667 public NamingSystemUniqueIdComponent setPeriod(Period value) { 668 this.period = value; 669 return this; 670 } 671 672 protected void listChildren(List<Property> children) { 673 super.listChildren(children); 674 children.add(new Property("type", "code", 675 "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type)); 676 children.add(new Property("value", "string", 677 "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, 678 value)); 679 children.add(new Property("preferred", "boolean", 680 "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred)); 681 children.add(new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, 682 comment)); 683 children.add(new Property("period", "Period", 684 "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 685 0, 1, period)); 686 } 687 688 @Override 689 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 690 switch (_hash) { 691 case 3575610: 692 /* type */ return new Property("type", "code", 693 "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type); 694 case 111972721: 695 /* value */ return new Property("value", "string", 696 "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, 697 value); 698 case -1294005119: 699 /* preferred */ return new Property("preferred", "boolean", 700 "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred); 701 case 950398559: 702 /* comment */ return new Property("comment", "string", 703 "Notes about the past or intended usage of this identifier.", 0, 1, comment); 704 case -991726143: 705 /* period */ return new Property("period", "Period", 706 "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 707 0, 1, period); 708 default: 709 return super.getNamedProperty(_hash, _name, _checkValid); 710 } 711 712 } 713 714 @Override 715 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 716 switch (hash) { 717 case 3575610: 718 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<NamingSystemIdentifierType> 719 case 111972721: 720 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 721 case -1294005119: 722 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 723 case 950398559: 724 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 725 case -991726143: 726 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 727 default: 728 return super.getProperty(hash, name, checkValid); 729 } 730 731 } 732 733 @Override 734 public Base setProperty(int hash, String name, Base value) throws FHIRException { 735 switch (hash) { 736 case 3575610: // type 737 value = new NamingSystemIdentifierTypeEnumFactory().fromType(castToCode(value)); 738 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 739 return value; 740 case 111972721: // value 741 this.value = castToString(value); // StringType 742 return value; 743 case -1294005119: // preferred 744 this.preferred = castToBoolean(value); // BooleanType 745 return value; 746 case 950398559: // comment 747 this.comment = castToString(value); // StringType 748 return value; 749 case -991726143: // period 750 this.period = castToPeriod(value); // Period 751 return value; 752 default: 753 return super.setProperty(hash, name, value); 754 } 755 756 } 757 758 @Override 759 public Base setProperty(String name, Base value) throws FHIRException { 760 if (name.equals("type")) { 761 value = new NamingSystemIdentifierTypeEnumFactory().fromType(castToCode(value)); 762 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 763 } else if (name.equals("value")) { 764 this.value = castToString(value); // StringType 765 } else if (name.equals("preferred")) { 766 this.preferred = castToBoolean(value); // BooleanType 767 } else if (name.equals("comment")) { 768 this.comment = castToString(value); // StringType 769 } else if (name.equals("period")) { 770 this.period = castToPeriod(value); // Period 771 } else 772 return super.setProperty(name, value); 773 return value; 774 } 775 776 @Override 777 public void removeChild(String name, Base value) throws FHIRException { 778 if (name.equals("type")) { 779 this.type = null; 780 } else if (name.equals("value")) { 781 this.value = null; 782 } else if (name.equals("preferred")) { 783 this.preferred = null; 784 } else if (name.equals("comment")) { 785 this.comment = null; 786 } else if (name.equals("period")) { 787 this.period = null; 788 } else 789 super.removeChild(name, value); 790 791 } 792 793 @Override 794 public Base makeProperty(int hash, String name) throws FHIRException { 795 switch (hash) { 796 case 3575610: 797 return getTypeElement(); 798 case 111972721: 799 return getValueElement(); 800 case -1294005119: 801 return getPreferredElement(); 802 case 950398559: 803 return getCommentElement(); 804 case -991726143: 805 return getPeriod(); 806 default: 807 return super.makeProperty(hash, name); 808 } 809 810 } 811 812 @Override 813 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 814 switch (hash) { 815 case 3575610: 816 /* type */ return new String[] { "code" }; 817 case 111972721: 818 /* value */ return new String[] { "string" }; 819 case -1294005119: 820 /* preferred */ return new String[] { "boolean" }; 821 case 950398559: 822 /* comment */ return new String[] { "string" }; 823 case -991726143: 824 /* period */ return new String[] { "Period" }; 825 default: 826 return super.getTypesForProperty(hash, name); 827 } 828 829 } 830 831 @Override 832 public Base addChild(String name) throws FHIRException { 833 if (name.equals("type")) { 834 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.type"); 835 } else if (name.equals("value")) { 836 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.value"); 837 } else if (name.equals("preferred")) { 838 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.preferred"); 839 } else if (name.equals("comment")) { 840 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.comment"); 841 } else if (name.equals("period")) { 842 this.period = new Period(); 843 return this.period; 844 } else 845 return super.addChild(name); 846 } 847 848 public NamingSystemUniqueIdComponent copy() { 849 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 850 copyValues(dst); 851 return dst; 852 } 853 854 public void copyValues(NamingSystemUniqueIdComponent dst) { 855 super.copyValues(dst); 856 dst.type = type == null ? null : type.copy(); 857 dst.value = value == null ? null : value.copy(); 858 dst.preferred = preferred == null ? null : preferred.copy(); 859 dst.comment = comment == null ? null : comment.copy(); 860 dst.period = period == null ? null : period.copy(); 861 } 862 863 @Override 864 public boolean equalsDeep(Base other_) { 865 if (!super.equalsDeep(other_)) 866 return false; 867 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 868 return false; 869 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 870 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) 871 && compareDeep(preferred, o.preferred, true) && compareDeep(comment, o.comment, true) 872 && compareDeep(period, o.period, true); 873 } 874 875 @Override 876 public boolean equalsShallow(Base other_) { 877 if (!super.equalsShallow(other_)) 878 return false; 879 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 880 return false; 881 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 882 return compareValues(type, o.type, true) && compareValues(value, o.value, true) 883 && compareValues(preferred, o.preferred, true) && compareValues(comment, o.comment, true); 884 } 885 886 public boolean isEmpty() { 887 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, preferred, comment, period); 888 } 889 890 public String fhirType() { 891 return "NamingSystem.uniqueId"; 892 893 } 894 895 } 896 897 /** 898 * Indicates the purpose for the naming system - what kinds of things does it 899 * make unique? 900 */ 901 @Child(name = "kind", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 902 @Description(shortDefinition = "codesystem | identifier | root", formalDefinition = "Indicates the purpose for the naming system - what kinds of things does it make unique?") 903 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/namingsystem-type") 904 protected Enumeration<NamingSystemType> kind; 905 906 /** 907 * The name of the organization that is responsible for issuing identifiers or 908 * codes for this namespace and ensuring their non-collision. 909 */ 910 @Child(name = "responsible", type = { 911 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 912 @Description(shortDefinition = "Who maintains system namespace?", formalDefinition = "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.") 913 protected StringType responsible; 914 915 /** 916 * Categorizes a naming system for easier search by grouping related naming 917 * systems. 918 */ 919 @Child(name = "type", type = { 920 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 921 @Description(shortDefinition = "e.g. driver, provider, patient, bank etc.", formalDefinition = "Categorizes a naming system for easier search by grouping related naming systems.") 922 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/identifier-type") 923 protected CodeableConcept type; 924 925 /** 926 * Provides guidance on the use of the namespace, including the handling of 927 * formatting characters, use of upper vs. lower case, etc. 928 */ 929 @Child(name = "usage", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 930 @Description(shortDefinition = "How/where is it used", formalDefinition = "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.") 931 protected StringType usage; 932 933 /** 934 * Indicates how the system may be identified when referenced in electronic 935 * exchange. 936 */ 937 @Child(name = "uniqueId", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 938 @Description(shortDefinition = "Unique identifiers used for system", formalDefinition = "Indicates how the system may be identified when referenced in electronic exchange.") 939 protected List<NamingSystemUniqueIdComponent> uniqueId; 940 941 private static final long serialVersionUID = 1686086580L; 942 943 /** 944 * Constructor 945 */ 946 public NamingSystem() { 947 super(); 948 } 949 950 /** 951 * Constructor 952 */ 953 public NamingSystem(StringType name, Enumeration<PublicationStatus> status, Enumeration<NamingSystemType> kind, 954 DateTimeType date) { 955 super(); 956 this.name = name; 957 this.status = status; 958 this.kind = kind; 959 this.date = date; 960 } 961 962 /** 963 * @return {@link #name} (A natural language name identifying the naming system. 964 * This name should be usable as an identifier for the module by machine 965 * processing applications such as code generation.). This is the 966 * underlying object with id, value and extensions. The accessor 967 * "getName" gives direct access to the value 968 */ 969 public StringType getNameElement() { 970 if (this.name == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create NamingSystem.name"); 973 else if (Configuration.doAutoCreate()) 974 this.name = new StringType(); // bb 975 return this.name; 976 } 977 978 public boolean hasNameElement() { 979 return this.name != null && !this.name.isEmpty(); 980 } 981 982 public boolean hasName() { 983 return this.name != null && !this.name.isEmpty(); 984 } 985 986 /** 987 * @param value {@link #name} (A natural language name identifying the naming 988 * system. This name should be usable as an identifier for the 989 * module by machine processing applications such as code 990 * generation.). This is the underlying object with id, value and 991 * extensions. The accessor "getName" gives direct access to the 992 * value 993 */ 994 public NamingSystem setNameElement(StringType value) { 995 this.name = value; 996 return this; 997 } 998 999 /** 1000 * @return A natural language name identifying the naming system. This name 1001 * should be usable as an identifier for the module by machine 1002 * processing applications such as code generation. 1003 */ 1004 public String getName() { 1005 return this.name == null ? null : this.name.getValue(); 1006 } 1007 1008 /** 1009 * @param value A natural language name identifying the naming system. This name 1010 * should be usable as an identifier for the module by machine 1011 * processing applications such as code generation. 1012 */ 1013 public NamingSystem setName(String value) { 1014 if (this.name == null) 1015 this.name = new StringType(); 1016 this.name.setValue(value); 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #status} (The status of this naming system. Enables tracking 1022 * the life-cycle of the content.). This is the underlying object with 1023 * id, value and extensions. The accessor "getStatus" gives direct 1024 * access to the value 1025 */ 1026 public Enumeration<PublicationStatus> getStatusElement() { 1027 if (this.status == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create NamingSystem.status"); 1030 else if (Configuration.doAutoCreate()) 1031 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1032 return this.status; 1033 } 1034 1035 public boolean hasStatusElement() { 1036 return this.status != null && !this.status.isEmpty(); 1037 } 1038 1039 public boolean hasStatus() { 1040 return this.status != null && !this.status.isEmpty(); 1041 } 1042 1043 /** 1044 * @param value {@link #status} (The status of this naming system. Enables 1045 * tracking the life-cycle of the content.). This is the underlying 1046 * object with id, value and extensions. The accessor "getStatus" 1047 * gives direct access to the value 1048 */ 1049 public NamingSystem setStatusElement(Enumeration<PublicationStatus> value) { 1050 this.status = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return The status of this naming system. Enables tracking the life-cycle of 1056 * the content. 1057 */ 1058 public PublicationStatus getStatus() { 1059 return this.status == null ? null : this.status.getValue(); 1060 } 1061 1062 /** 1063 * @param value The status of this naming system. Enables tracking the 1064 * life-cycle of the content. 1065 */ 1066 public NamingSystem setStatus(PublicationStatus value) { 1067 if (this.status == null) 1068 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1069 this.status.setValue(value); 1070 return this; 1071 } 1072 1073 /** 1074 * @return {@link #kind} (Indicates the purpose for the naming system - what 1075 * kinds of things does it make unique?). This is the underlying object 1076 * with id, value and extensions. The accessor "getKind" gives direct 1077 * access to the value 1078 */ 1079 public Enumeration<NamingSystemType> getKindElement() { 1080 if (this.kind == null) 1081 if (Configuration.errorOnAutoCreate()) 1082 throw new Error("Attempt to auto-create NamingSystem.kind"); 1083 else if (Configuration.doAutoCreate()) 1084 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 1085 return this.kind; 1086 } 1087 1088 public boolean hasKindElement() { 1089 return this.kind != null && !this.kind.isEmpty(); 1090 } 1091 1092 public boolean hasKind() { 1093 return this.kind != null && !this.kind.isEmpty(); 1094 } 1095 1096 /** 1097 * @param value {@link #kind} (Indicates the purpose for the naming system - 1098 * what kinds of things does it make unique?). This is the 1099 * underlying object with id, value and extensions. The accessor 1100 * "getKind" gives direct access to the value 1101 */ 1102 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 1103 this.kind = value; 1104 return this; 1105 } 1106 1107 /** 1108 * @return Indicates the purpose for the naming system - what kinds of things 1109 * does it make unique? 1110 */ 1111 public NamingSystemType getKind() { 1112 return this.kind == null ? null : this.kind.getValue(); 1113 } 1114 1115 /** 1116 * @param value Indicates the purpose for the naming system - what kinds of 1117 * things does it make unique? 1118 */ 1119 public NamingSystem setKind(NamingSystemType value) { 1120 if (this.kind == null) 1121 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 1122 this.kind.setValue(value); 1123 return this; 1124 } 1125 1126 /** 1127 * @return {@link #date} (The date (and optionally time) when the naming system 1128 * was published. The date must change when the business version changes 1129 * and it must change if the status code changes. In addition, it should 1130 * change when the substantive content of the naming system changes.). 1131 * This is the underlying object with id, value and extensions. The 1132 * accessor "getDate" gives direct access to the value 1133 */ 1134 public DateTimeType getDateElement() { 1135 if (this.date == null) 1136 if (Configuration.errorOnAutoCreate()) 1137 throw new Error("Attempt to auto-create NamingSystem.date"); 1138 else if (Configuration.doAutoCreate()) 1139 this.date = new DateTimeType(); // bb 1140 return this.date; 1141 } 1142 1143 public boolean hasDateElement() { 1144 return this.date != null && !this.date.isEmpty(); 1145 } 1146 1147 public boolean hasDate() { 1148 return this.date != null && !this.date.isEmpty(); 1149 } 1150 1151 /** 1152 * @param value {@link #date} (The date (and optionally time) when the naming 1153 * system was published. The date must change when the business 1154 * version changes and it must change if the status code changes. 1155 * In addition, it should change when the substantive content of 1156 * the naming system changes.). This is the underlying object with 1157 * id, value and extensions. The accessor "getDate" gives direct 1158 * access to the value 1159 */ 1160 public NamingSystem setDateElement(DateTimeType value) { 1161 this.date = value; 1162 return this; 1163 } 1164 1165 /** 1166 * @return The date (and optionally time) when the naming system was published. 1167 * The date must change when the business version changes and it must 1168 * change if the status code changes. In addition, it should change when 1169 * the substantive content of the naming system changes. 1170 */ 1171 public Date getDate() { 1172 return this.date == null ? null : this.date.getValue(); 1173 } 1174 1175 /** 1176 * @param value The date (and optionally time) when the naming system was 1177 * published. The date must change when the business version 1178 * changes and it must change if the status code changes. In 1179 * addition, it should change when the substantive content of the 1180 * naming system changes. 1181 */ 1182 public NamingSystem setDate(Date value) { 1183 if (this.date == null) 1184 this.date = new DateTimeType(); 1185 this.date.setValue(value); 1186 return this; 1187 } 1188 1189 /** 1190 * @return {@link #publisher} (The name of the organization or individual that 1191 * published the naming system.). This is the underlying object with id, 1192 * value and extensions. The accessor "getPublisher" gives direct access 1193 * to the value 1194 */ 1195 public StringType getPublisherElement() { 1196 if (this.publisher == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create NamingSystem.publisher"); 1199 else if (Configuration.doAutoCreate()) 1200 this.publisher = new StringType(); // bb 1201 return this.publisher; 1202 } 1203 1204 public boolean hasPublisherElement() { 1205 return this.publisher != null && !this.publisher.isEmpty(); 1206 } 1207 1208 public boolean hasPublisher() { 1209 return this.publisher != null && !this.publisher.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #publisher} (The name of the organization or individual 1214 * that published the naming system.). This is the underlying 1215 * object with id, value and extensions. The accessor 1216 * "getPublisher" gives direct access to the value 1217 */ 1218 public NamingSystem setPublisherElement(StringType value) { 1219 this.publisher = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return The name of the organization or individual that published the naming 1225 * system. 1226 */ 1227 public String getPublisher() { 1228 return this.publisher == null ? null : this.publisher.getValue(); 1229 } 1230 1231 /** 1232 * @param value The name of the organization or individual that published the 1233 * naming system. 1234 */ 1235 public NamingSystem setPublisher(String value) { 1236 if (Utilities.noString(value)) 1237 this.publisher = null; 1238 else { 1239 if (this.publisher == null) 1240 this.publisher = new StringType(); 1241 this.publisher.setValue(value); 1242 } 1243 return this; 1244 } 1245 1246 /** 1247 * @return {@link #contact} (Contact details to assist a user in finding and 1248 * communicating with the publisher.) 1249 */ 1250 public List<ContactDetail> getContact() { 1251 if (this.contact == null) 1252 this.contact = new ArrayList<ContactDetail>(); 1253 return this.contact; 1254 } 1255 1256 /** 1257 * @return Returns a reference to <code>this</code> for easy method chaining 1258 */ 1259 public NamingSystem setContact(List<ContactDetail> theContact) { 1260 this.contact = theContact; 1261 return this; 1262 } 1263 1264 public boolean hasContact() { 1265 if (this.contact == null) 1266 return false; 1267 for (ContactDetail item : this.contact) 1268 if (!item.isEmpty()) 1269 return true; 1270 return false; 1271 } 1272 1273 public ContactDetail addContact() { // 3 1274 ContactDetail t = new ContactDetail(); 1275 if (this.contact == null) 1276 this.contact = new ArrayList<ContactDetail>(); 1277 this.contact.add(t); 1278 return t; 1279 } 1280 1281 public NamingSystem addContact(ContactDetail t) { // 3 1282 if (t == null) 1283 return this; 1284 if (this.contact == null) 1285 this.contact = new ArrayList<ContactDetail>(); 1286 this.contact.add(t); 1287 return this; 1288 } 1289 1290 /** 1291 * @return The first repetition of repeating field {@link #contact}, creating it 1292 * if it does not already exist 1293 */ 1294 public ContactDetail getContactFirstRep() { 1295 if (getContact().isEmpty()) { 1296 addContact(); 1297 } 1298 return getContact().get(0); 1299 } 1300 1301 /** 1302 * @return {@link #responsible} (The name of the organization that is 1303 * responsible for issuing identifiers or codes for this namespace and 1304 * ensuring their non-collision.). This is the underlying object with 1305 * id, value and extensions. The accessor "getResponsible" gives direct 1306 * access to the value 1307 */ 1308 public StringType getResponsibleElement() { 1309 if (this.responsible == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1312 else if (Configuration.doAutoCreate()) 1313 this.responsible = new StringType(); // bb 1314 return this.responsible; 1315 } 1316 1317 public boolean hasResponsibleElement() { 1318 return this.responsible != null && !this.responsible.isEmpty(); 1319 } 1320 1321 public boolean hasResponsible() { 1322 return this.responsible != null && !this.responsible.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #responsible} (The name of the organization that is 1327 * responsible for issuing identifiers or codes for this namespace 1328 * and ensuring their non-collision.). This is the underlying 1329 * object with id, value and extensions. The accessor 1330 * "getResponsible" gives direct access to the value 1331 */ 1332 public NamingSystem setResponsibleElement(StringType value) { 1333 this.responsible = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return The name of the organization that is responsible for issuing 1339 * identifiers or codes for this namespace and ensuring their 1340 * non-collision. 1341 */ 1342 public String getResponsible() { 1343 return this.responsible == null ? null : this.responsible.getValue(); 1344 } 1345 1346 /** 1347 * @param value The name of the organization that is responsible for issuing 1348 * identifiers or codes for this namespace and ensuring their 1349 * non-collision. 1350 */ 1351 public NamingSystem setResponsible(String value) { 1352 if (Utilities.noString(value)) 1353 this.responsible = null; 1354 else { 1355 if (this.responsible == null) 1356 this.responsible = new StringType(); 1357 this.responsible.setValue(value); 1358 } 1359 return this; 1360 } 1361 1362 /** 1363 * @return {@link #type} (Categorizes a naming system for easier search by 1364 * grouping related naming systems.) 1365 */ 1366 public CodeableConcept getType() { 1367 if (this.type == null) 1368 if (Configuration.errorOnAutoCreate()) 1369 throw new Error("Attempt to auto-create NamingSystem.type"); 1370 else if (Configuration.doAutoCreate()) 1371 this.type = new CodeableConcept(); // cc 1372 return this.type; 1373 } 1374 1375 public boolean hasType() { 1376 return this.type != null && !this.type.isEmpty(); 1377 } 1378 1379 /** 1380 * @param value {@link #type} (Categorizes a naming system for easier search by 1381 * grouping related naming systems.) 1382 */ 1383 public NamingSystem setType(CodeableConcept value) { 1384 this.type = value; 1385 return this; 1386 } 1387 1388 /** 1389 * @return {@link #description} (A free text natural language description of the 1390 * naming system from a consumer's perspective. Details about what the 1391 * namespace identifies including scope, granularity, version labeling, 1392 * etc.). This is the underlying object with id, value and extensions. 1393 * The accessor "getDescription" gives direct access to the value 1394 */ 1395 public MarkdownType getDescriptionElement() { 1396 if (this.description == null) 1397 if (Configuration.errorOnAutoCreate()) 1398 throw new Error("Attempt to auto-create NamingSystem.description"); 1399 else if (Configuration.doAutoCreate()) 1400 this.description = new MarkdownType(); // bb 1401 return this.description; 1402 } 1403 1404 public boolean hasDescriptionElement() { 1405 return this.description != null && !this.description.isEmpty(); 1406 } 1407 1408 public boolean hasDescription() { 1409 return this.description != null && !this.description.isEmpty(); 1410 } 1411 1412 /** 1413 * @param value {@link #description} (A free text natural language description 1414 * of the naming system from a consumer's perspective. Details 1415 * about what the namespace identifies including scope, 1416 * granularity, version labeling, etc.). This is the underlying 1417 * object with id, value and extensions. The accessor 1418 * "getDescription" gives direct access to the value 1419 */ 1420 public NamingSystem setDescriptionElement(MarkdownType value) { 1421 this.description = value; 1422 return this; 1423 } 1424 1425 /** 1426 * @return A free text natural language description of the naming system from a 1427 * consumer's perspective. Details about what the namespace identifies 1428 * including scope, granularity, version labeling, etc. 1429 */ 1430 public String getDescription() { 1431 return this.description == null ? null : this.description.getValue(); 1432 } 1433 1434 /** 1435 * @param value A free text natural language description of the naming system 1436 * from a consumer's perspective. Details about what the namespace 1437 * identifies including scope, granularity, version labeling, etc. 1438 */ 1439 public NamingSystem setDescription(String value) { 1440 if (value == null) 1441 this.description = null; 1442 else { 1443 if (this.description == null) 1444 this.description = new MarkdownType(); 1445 this.description.setValue(value); 1446 } 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #useContext} (The content was developed with a focus and 1452 * intent of supporting the contexts that are listed. These contexts may 1453 * be general categories (gender, age, ...) or may be references to 1454 * specific programs (insurance plans, studies, ...) and may be used to 1455 * assist with indexing and searching for appropriate naming system 1456 * instances.) 1457 */ 1458 public List<UsageContext> getUseContext() { 1459 if (this.useContext == null) 1460 this.useContext = new ArrayList<UsageContext>(); 1461 return this.useContext; 1462 } 1463 1464 /** 1465 * @return Returns a reference to <code>this</code> for easy method chaining 1466 */ 1467 public NamingSystem setUseContext(List<UsageContext> theUseContext) { 1468 this.useContext = theUseContext; 1469 return this; 1470 } 1471 1472 public boolean hasUseContext() { 1473 if (this.useContext == null) 1474 return false; 1475 for (UsageContext item : this.useContext) 1476 if (!item.isEmpty()) 1477 return true; 1478 return false; 1479 } 1480 1481 public UsageContext addUseContext() { // 3 1482 UsageContext t = new UsageContext(); 1483 if (this.useContext == null) 1484 this.useContext = new ArrayList<UsageContext>(); 1485 this.useContext.add(t); 1486 return t; 1487 } 1488 1489 public NamingSystem addUseContext(UsageContext t) { // 3 1490 if (t == null) 1491 return this; 1492 if (this.useContext == null) 1493 this.useContext = new ArrayList<UsageContext>(); 1494 this.useContext.add(t); 1495 return this; 1496 } 1497 1498 /** 1499 * @return The first repetition of repeating field {@link #useContext}, creating 1500 * it if it does not already exist 1501 */ 1502 public UsageContext getUseContextFirstRep() { 1503 if (getUseContext().isEmpty()) { 1504 addUseContext(); 1505 } 1506 return getUseContext().get(0); 1507 } 1508 1509 /** 1510 * @return {@link #jurisdiction} (A legal or geographic region in which the 1511 * naming system is intended to be used.) 1512 */ 1513 public List<CodeableConcept> getJurisdiction() { 1514 if (this.jurisdiction == null) 1515 this.jurisdiction = new ArrayList<CodeableConcept>(); 1516 return this.jurisdiction; 1517 } 1518 1519 /** 1520 * @return Returns a reference to <code>this</code> for easy method chaining 1521 */ 1522 public NamingSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 1523 this.jurisdiction = theJurisdiction; 1524 return this; 1525 } 1526 1527 public boolean hasJurisdiction() { 1528 if (this.jurisdiction == null) 1529 return false; 1530 for (CodeableConcept item : this.jurisdiction) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 public CodeableConcept addJurisdiction() { // 3 1537 CodeableConcept t = new CodeableConcept(); 1538 if (this.jurisdiction == null) 1539 this.jurisdiction = new ArrayList<CodeableConcept>(); 1540 this.jurisdiction.add(t); 1541 return t; 1542 } 1543 1544 public NamingSystem addJurisdiction(CodeableConcept t) { // 3 1545 if (t == null) 1546 return this; 1547 if (this.jurisdiction == null) 1548 this.jurisdiction = new ArrayList<CodeableConcept>(); 1549 this.jurisdiction.add(t); 1550 return this; 1551 } 1552 1553 /** 1554 * @return The first repetition of repeating field {@link #jurisdiction}, 1555 * creating it if it does not already exist 1556 */ 1557 public CodeableConcept getJurisdictionFirstRep() { 1558 if (getJurisdiction().isEmpty()) { 1559 addJurisdiction(); 1560 } 1561 return getJurisdiction().get(0); 1562 } 1563 1564 /** 1565 * @return {@link #usage} (Provides guidance on the use of the namespace, 1566 * including the handling of formatting characters, use of upper vs. 1567 * lower case, etc.). This is the underlying object with id, value and 1568 * extensions. The accessor "getUsage" gives direct access to the value 1569 */ 1570 public StringType getUsageElement() { 1571 if (this.usage == null) 1572 if (Configuration.errorOnAutoCreate()) 1573 throw new Error("Attempt to auto-create NamingSystem.usage"); 1574 else if (Configuration.doAutoCreate()) 1575 this.usage = new StringType(); // bb 1576 return this.usage; 1577 } 1578 1579 public boolean hasUsageElement() { 1580 return this.usage != null && !this.usage.isEmpty(); 1581 } 1582 1583 public boolean hasUsage() { 1584 return this.usage != null && !this.usage.isEmpty(); 1585 } 1586 1587 /** 1588 * @param value {@link #usage} (Provides guidance on the use of the namespace, 1589 * including the handling of formatting characters, use of upper 1590 * vs. lower case, etc.). This is the underlying object with id, 1591 * value and extensions. The accessor "getUsage" gives direct 1592 * access to the value 1593 */ 1594 public NamingSystem setUsageElement(StringType value) { 1595 this.usage = value; 1596 return this; 1597 } 1598 1599 /** 1600 * @return Provides guidance on the use of the namespace, including the handling 1601 * of formatting characters, use of upper vs. lower case, etc. 1602 */ 1603 public String getUsage() { 1604 return this.usage == null ? null : this.usage.getValue(); 1605 } 1606 1607 /** 1608 * @param value Provides guidance on the use of the namespace, including the 1609 * handling of formatting characters, use of upper vs. lower case, 1610 * etc. 1611 */ 1612 public NamingSystem setUsage(String value) { 1613 if (Utilities.noString(value)) 1614 this.usage = null; 1615 else { 1616 if (this.usage == null) 1617 this.usage = new StringType(); 1618 this.usage.setValue(value); 1619 } 1620 return this; 1621 } 1622 1623 /** 1624 * @return {@link #uniqueId} (Indicates how the system may be identified when 1625 * referenced in electronic exchange.) 1626 */ 1627 public List<NamingSystemUniqueIdComponent> getUniqueId() { 1628 if (this.uniqueId == null) 1629 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1630 return this.uniqueId; 1631 } 1632 1633 /** 1634 * @return Returns a reference to <code>this</code> for easy method chaining 1635 */ 1636 public NamingSystem setUniqueId(List<NamingSystemUniqueIdComponent> theUniqueId) { 1637 this.uniqueId = theUniqueId; 1638 return this; 1639 } 1640 1641 public boolean hasUniqueId() { 1642 if (this.uniqueId == null) 1643 return false; 1644 for (NamingSystemUniqueIdComponent item : this.uniqueId) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 public NamingSystemUniqueIdComponent addUniqueId() { // 3 1651 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 1652 if (this.uniqueId == null) 1653 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1654 this.uniqueId.add(t); 1655 return t; 1656 } 1657 1658 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { // 3 1659 if (t == null) 1660 return this; 1661 if (this.uniqueId == null) 1662 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1663 this.uniqueId.add(t); 1664 return this; 1665 } 1666 1667 /** 1668 * @return The first repetition of repeating field {@link #uniqueId}, creating 1669 * it if it does not already exist 1670 */ 1671 public NamingSystemUniqueIdComponent getUniqueIdFirstRep() { 1672 if (getUniqueId().isEmpty()) { 1673 addUniqueId(); 1674 } 1675 return getUniqueId().get(0); 1676 } 1677 1678 protected void listChildren(List<Property> children) { 1679 super.listChildren(children); 1680 children.add(new Property("name", "string", 1681 "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1682 0, 1, name)); 1683 children.add(new Property("status", "code", 1684 "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status)); 1685 children.add(new Property("kind", "code", 1686 "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind)); 1687 children.add(new Property("date", "dateTime", 1688 "The date (and optionally time) when the naming system was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 1689 0, 1, date)); 1690 children.add(new Property("publisher", "string", 1691 "The name of the organization or individual that published the naming system.", 0, 1, publisher)); 1692 children.add(new Property("contact", "ContactDetail", 1693 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1694 java.lang.Integer.MAX_VALUE, contact)); 1695 children.add(new Property("responsible", "string", 1696 "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 1697 0, 1, responsible)); 1698 children.add(new Property("type", "CodeableConcept", 1699 "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type)); 1700 children.add(new Property("description", "markdown", 1701 "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 1702 0, 1, description)); 1703 children.add(new Property("useContext", "UsageContext", 1704 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 1705 0, java.lang.Integer.MAX_VALUE, useContext)); 1706 children.add(new Property("jurisdiction", "CodeableConcept", 1707 "A legal or geographic region in which the naming system is intended to be used.", 0, 1708 java.lang.Integer.MAX_VALUE, jurisdiction)); 1709 children.add(new Property("usage", "string", 1710 "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 1711 0, 1, usage)); 1712 children.add(new Property("uniqueId", "", 1713 "Indicates how the system may be identified when referenced in electronic exchange.", 0, 1714 java.lang.Integer.MAX_VALUE, uniqueId)); 1715 } 1716 1717 @Override 1718 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1719 switch (_hash) { 1720 case 3373707: 1721 /* name */ return new Property("name", "string", 1722 "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1723 0, 1, name); 1724 case -892481550: 1725 /* status */ return new Property("status", "code", 1726 "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status); 1727 case 3292052: 1728 /* kind */ return new Property("kind", "code", 1729 "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind); 1730 case 3076014: 1731 /* date */ return new Property("date", "dateTime", 1732 "The date (and optionally time) when the naming system was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 1733 0, 1, date); 1734 case 1447404028: 1735 /* publisher */ return new Property("publisher", "string", 1736 "The name of the organization or individual that published the naming system.", 0, 1, publisher); 1737 case 951526432: 1738 /* contact */ return new Property("contact", "ContactDetail", 1739 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1740 java.lang.Integer.MAX_VALUE, contact); 1741 case 1847674614: 1742 /* responsible */ return new Property("responsible", "string", 1743 "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 1744 0, 1, responsible); 1745 case 3575610: 1746 /* type */ return new Property("type", "CodeableConcept", 1747 "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type); 1748 case -1724546052: 1749 /* description */ return new Property("description", "markdown", 1750 "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 1751 0, 1, description); 1752 case -669707736: 1753 /* useContext */ return new Property("useContext", "UsageContext", 1754 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 1755 0, java.lang.Integer.MAX_VALUE, useContext); 1756 case -507075711: 1757 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 1758 "A legal or geographic region in which the naming system is intended to be used.", 0, 1759 java.lang.Integer.MAX_VALUE, jurisdiction); 1760 case 111574433: 1761 /* usage */ return new Property("usage", "string", 1762 "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 1763 0, 1, usage); 1764 case -294460212: 1765 /* uniqueId */ return new Property("uniqueId", "", 1766 "Indicates how the system may be identified when referenced in electronic exchange.", 0, 1767 java.lang.Integer.MAX_VALUE, uniqueId); 1768 default: 1769 return super.getNamedProperty(_hash, _name, _checkValid); 1770 } 1771 1772 } 1773 1774 @Override 1775 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1776 switch (hash) { 1777 case 3373707: 1778 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1779 case -892481550: 1780 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 1781 case 3292052: 1782 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<NamingSystemType> 1783 case 3076014: 1784 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1785 case 1447404028: 1786 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 1787 case 951526432: 1788 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1789 case 1847674614: 1790 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // StringType 1791 case 3575610: 1792 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1793 case -1724546052: 1794 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 1795 case -669707736: 1796 /* useContext */ return this.useContext == null ? new Base[0] 1797 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1798 case -507075711: 1799 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 1800 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1801 case 111574433: 1802 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 1803 case -294460212: 1804 /* uniqueId */ return this.uniqueId == null ? new Base[0] : this.uniqueId.toArray(new Base[this.uniqueId.size()]); // NamingSystemUniqueIdComponent 1805 default: 1806 return super.getProperty(hash, name, checkValid); 1807 } 1808 1809 } 1810 1811 @Override 1812 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1813 switch (hash) { 1814 case 3373707: // name 1815 this.name = castToString(value); // StringType 1816 return value; 1817 case -892481550: // status 1818 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1819 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1820 return value; 1821 case 3292052: // kind 1822 value = new NamingSystemTypeEnumFactory().fromType(castToCode(value)); 1823 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 1824 return value; 1825 case 3076014: // date 1826 this.date = castToDateTime(value); // DateTimeType 1827 return value; 1828 case 1447404028: // publisher 1829 this.publisher = castToString(value); // StringType 1830 return value; 1831 case 951526432: // contact 1832 this.getContact().add(castToContactDetail(value)); // ContactDetail 1833 return value; 1834 case 1847674614: // responsible 1835 this.responsible = castToString(value); // StringType 1836 return value; 1837 case 3575610: // type 1838 this.type = castToCodeableConcept(value); // CodeableConcept 1839 return value; 1840 case -1724546052: // description 1841 this.description = castToMarkdown(value); // MarkdownType 1842 return value; 1843 case -669707736: // useContext 1844 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1845 return value; 1846 case -507075711: // jurisdiction 1847 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1848 return value; 1849 case 111574433: // usage 1850 this.usage = castToString(value); // StringType 1851 return value; 1852 case -294460212: // uniqueId 1853 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); // NamingSystemUniqueIdComponent 1854 return value; 1855 default: 1856 return super.setProperty(hash, name, value); 1857 } 1858 1859 } 1860 1861 @Override 1862 public Base setProperty(String name, Base value) throws FHIRException { 1863 if (name.equals("name")) { 1864 this.name = castToString(value); // StringType 1865 } else if (name.equals("status")) { 1866 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1867 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1868 } else if (name.equals("kind")) { 1869 value = new NamingSystemTypeEnumFactory().fromType(castToCode(value)); 1870 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 1871 } else if (name.equals("date")) { 1872 this.date = castToDateTime(value); // DateTimeType 1873 } else if (name.equals("publisher")) { 1874 this.publisher = castToString(value); // StringType 1875 } else if (name.equals("contact")) { 1876 this.getContact().add(castToContactDetail(value)); 1877 } else if (name.equals("responsible")) { 1878 this.responsible = castToString(value); // StringType 1879 } else if (name.equals("type")) { 1880 this.type = castToCodeableConcept(value); // CodeableConcept 1881 } else if (name.equals("description")) { 1882 this.description = castToMarkdown(value); // MarkdownType 1883 } else if (name.equals("useContext")) { 1884 this.getUseContext().add(castToUsageContext(value)); 1885 } else if (name.equals("jurisdiction")) { 1886 this.getJurisdiction().add(castToCodeableConcept(value)); 1887 } else if (name.equals("usage")) { 1888 this.usage = castToString(value); // StringType 1889 } else if (name.equals("uniqueId")) { 1890 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 1891 } else 1892 return super.setProperty(name, value); 1893 return value; 1894 } 1895 1896 @Override 1897 public void removeChild(String name, Base value) throws FHIRException { 1898 if (name.equals("name")) { 1899 this.name = null; 1900 } else if (name.equals("status")) { 1901 this.status = null; 1902 } else if (name.equals("kind")) { 1903 this.kind = null; 1904 } else if (name.equals("date")) { 1905 this.date = null; 1906 } else if (name.equals("publisher")) { 1907 this.publisher = null; 1908 } else if (name.equals("contact")) { 1909 this.getContact().remove(castToContactDetail(value)); 1910 } else if (name.equals("responsible")) { 1911 this.responsible = null; 1912 } else if (name.equals("type")) { 1913 this.type = null; 1914 } else if (name.equals("description")) { 1915 this.description = null; 1916 } else if (name.equals("useContext")) { 1917 this.getUseContext().remove(castToUsageContext(value)); 1918 } else if (name.equals("jurisdiction")) { 1919 this.getJurisdiction().remove(castToCodeableConcept(value)); 1920 } else if (name.equals("usage")) { 1921 this.usage = null; 1922 } else if (name.equals("uniqueId")) { 1923 this.getUniqueId().remove((NamingSystemUniqueIdComponent) value); 1924 } else 1925 super.removeChild(name, value); 1926 1927 } 1928 1929 @Override 1930 public Base makeProperty(int hash, String name) throws FHIRException { 1931 switch (hash) { 1932 case 3373707: 1933 return getNameElement(); 1934 case -892481550: 1935 return getStatusElement(); 1936 case 3292052: 1937 return getKindElement(); 1938 case 3076014: 1939 return getDateElement(); 1940 case 1447404028: 1941 return getPublisherElement(); 1942 case 951526432: 1943 return addContact(); 1944 case 1847674614: 1945 return getResponsibleElement(); 1946 case 3575610: 1947 return getType(); 1948 case -1724546052: 1949 return getDescriptionElement(); 1950 case -669707736: 1951 return addUseContext(); 1952 case -507075711: 1953 return addJurisdiction(); 1954 case 111574433: 1955 return getUsageElement(); 1956 case -294460212: 1957 return addUniqueId(); 1958 default: 1959 return super.makeProperty(hash, name); 1960 } 1961 1962 } 1963 1964 @Override 1965 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1966 switch (hash) { 1967 case 3373707: 1968 /* name */ return new String[] { "string" }; 1969 case -892481550: 1970 /* status */ return new String[] { "code" }; 1971 case 3292052: 1972 /* kind */ return new String[] { "code" }; 1973 case 3076014: 1974 /* date */ return new String[] { "dateTime" }; 1975 case 1447404028: 1976 /* publisher */ return new String[] { "string" }; 1977 case 951526432: 1978 /* contact */ return new String[] { "ContactDetail" }; 1979 case 1847674614: 1980 /* responsible */ return new String[] { "string" }; 1981 case 3575610: 1982 /* type */ return new String[] { "CodeableConcept" }; 1983 case -1724546052: 1984 /* description */ return new String[] { "markdown" }; 1985 case -669707736: 1986 /* useContext */ return new String[] { "UsageContext" }; 1987 case -507075711: 1988 /* jurisdiction */ return new String[] { "CodeableConcept" }; 1989 case 111574433: 1990 /* usage */ return new String[] { "string" }; 1991 case -294460212: 1992 /* uniqueId */ return new String[] {}; 1993 default: 1994 return super.getTypesForProperty(hash, name); 1995 } 1996 1997 } 1998 1999 @Override 2000 public Base addChild(String name) throws FHIRException { 2001 if (name.equals("name")) { 2002 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 2003 } else if (name.equals("status")) { 2004 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 2005 } else if (name.equals("kind")) { 2006 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 2007 } else if (name.equals("date")) { 2008 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 2009 } else if (name.equals("publisher")) { 2010 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 2011 } else if (name.equals("contact")) { 2012 return addContact(); 2013 } else if (name.equals("responsible")) { 2014 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 2015 } else if (name.equals("type")) { 2016 this.type = new CodeableConcept(); 2017 return this.type; 2018 } else if (name.equals("description")) { 2019 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 2020 } else if (name.equals("useContext")) { 2021 return addUseContext(); 2022 } else if (name.equals("jurisdiction")) { 2023 return addJurisdiction(); 2024 } else if (name.equals("usage")) { 2025 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 2026 } else if (name.equals("uniqueId")) { 2027 return addUniqueId(); 2028 } else 2029 return super.addChild(name); 2030 } 2031 2032 public String fhirType() { 2033 return "NamingSystem"; 2034 2035 } 2036 2037 public NamingSystem copy() { 2038 NamingSystem dst = new NamingSystem(); 2039 copyValues(dst); 2040 return dst; 2041 } 2042 2043 public void copyValues(NamingSystem dst) { 2044 super.copyValues(dst); 2045 dst.name = name == null ? null : name.copy(); 2046 dst.status = status == null ? null : status.copy(); 2047 dst.kind = kind == null ? null : kind.copy(); 2048 dst.date = date == null ? null : date.copy(); 2049 dst.publisher = publisher == null ? null : publisher.copy(); 2050 if (contact != null) { 2051 dst.contact = new ArrayList<ContactDetail>(); 2052 for (ContactDetail i : contact) 2053 dst.contact.add(i.copy()); 2054 } 2055 ; 2056 dst.responsible = responsible == null ? null : responsible.copy(); 2057 dst.type = type == null ? null : type.copy(); 2058 dst.description = description == null ? null : description.copy(); 2059 if (useContext != null) { 2060 dst.useContext = new ArrayList<UsageContext>(); 2061 for (UsageContext i : useContext) 2062 dst.useContext.add(i.copy()); 2063 } 2064 ; 2065 if (jurisdiction != null) { 2066 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2067 for (CodeableConcept i : jurisdiction) 2068 dst.jurisdiction.add(i.copy()); 2069 } 2070 ; 2071 dst.usage = usage == null ? null : usage.copy(); 2072 if (uniqueId != null) { 2073 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2074 for (NamingSystemUniqueIdComponent i : uniqueId) 2075 dst.uniqueId.add(i.copy()); 2076 } 2077 ; 2078 } 2079 2080 protected NamingSystem typedCopy() { 2081 return copy(); 2082 } 2083 2084 @Override 2085 public boolean equalsDeep(Base other_) { 2086 if (!super.equalsDeep(other_)) 2087 return false; 2088 if (!(other_ instanceof NamingSystem)) 2089 return false; 2090 NamingSystem o = (NamingSystem) other_; 2091 return compareDeep(kind, o.kind, true) && compareDeep(responsible, o.responsible, true) 2092 && compareDeep(type, o.type, true) && compareDeep(usage, o.usage, true) 2093 && compareDeep(uniqueId, o.uniqueId, true); 2094 } 2095 2096 @Override 2097 public boolean equalsShallow(Base other_) { 2098 if (!super.equalsShallow(other_)) 2099 return false; 2100 if (!(other_ instanceof NamingSystem)) 2101 return false; 2102 NamingSystem o = (NamingSystem) other_; 2103 return compareValues(kind, o.kind, true) && compareValues(responsible, o.responsible, true) 2104 && compareValues(usage, o.usage, true); 2105 } 2106 2107 public boolean isEmpty() { 2108 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, responsible, type, usage, uniqueId); 2109 } 2110 2111 @Override 2112 public ResourceType getResourceType() { 2113 return ResourceType.NamingSystem; 2114 } 2115 2116 /** 2117 * Search parameter: <b>date</b> 2118 * <p> 2119 * Description: <b>The naming system publication date</b><br> 2120 * Type: <b>date</b><br> 2121 * Path: <b>NamingSystem.date</b><br> 2122 * </p> 2123 */ 2124 @SearchParamDefinition(name = "date", path = "NamingSystem.date", description = "The naming system publication date", type = "date") 2125 public static final String SP_DATE = "date"; 2126 /** 2127 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2128 * <p> 2129 * Description: <b>The naming system publication date</b><br> 2130 * Type: <b>date</b><br> 2131 * Path: <b>NamingSystem.date</b><br> 2132 * </p> 2133 */ 2134 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2135 SP_DATE); 2136 2137 /** 2138 * Search parameter: <b>period</b> 2139 * <p> 2140 * Description: <b>When is identifier valid?</b><br> 2141 * Type: <b>date</b><br> 2142 * Path: <b>NamingSystem.uniqueId.period</b><br> 2143 * </p> 2144 */ 2145 @SearchParamDefinition(name = "period", path = "NamingSystem.uniqueId.period", description = "When is identifier valid?", type = "date") 2146 public static final String SP_PERIOD = "period"; 2147 /** 2148 * <b>Fluent Client</b> search parameter constant for <b>period</b> 2149 * <p> 2150 * Description: <b>When is identifier valid?</b><br> 2151 * Type: <b>date</b><br> 2152 * Path: <b>NamingSystem.uniqueId.period</b><br> 2153 * </p> 2154 */ 2155 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 2156 SP_PERIOD); 2157 2158 /** 2159 * Search parameter: <b>context-type-value</b> 2160 * <p> 2161 * Description: <b>A use context type and value assigned to the naming 2162 * system</b><br> 2163 * Type: <b>composite</b><br> 2164 * Path: <b></b><br> 2165 * </p> 2166 */ 2167 @SearchParamDefinition(name = "context-type-value", path = "NamingSystem.useContext", description = "A use context type and value assigned to the naming system", type = "composite", compositeOf = { 2168 "context-type", "context" }) 2169 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2170 /** 2171 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2172 * <p> 2173 * Description: <b>A use context type and value assigned to the naming 2174 * system</b><br> 2175 * Type: <b>composite</b><br> 2176 * Path: <b></b><br> 2177 * </p> 2178 */ 2179 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2180 SP_CONTEXT_TYPE_VALUE); 2181 2182 /** 2183 * Search parameter: <b>kind</b> 2184 * <p> 2185 * Description: <b>codesystem | identifier | root</b><br> 2186 * Type: <b>token</b><br> 2187 * Path: <b>NamingSystem.kind</b><br> 2188 * </p> 2189 */ 2190 @SearchParamDefinition(name = "kind", path = "NamingSystem.kind", description = "codesystem | identifier | root", type = "token") 2191 public static final String SP_KIND = "kind"; 2192 /** 2193 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 2194 * <p> 2195 * Description: <b>codesystem | identifier | root</b><br> 2196 * Type: <b>token</b><br> 2197 * Path: <b>NamingSystem.kind</b><br> 2198 * </p> 2199 */ 2200 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2201 SP_KIND); 2202 2203 /** 2204 * Search parameter: <b>jurisdiction</b> 2205 * <p> 2206 * Description: <b>Intended jurisdiction for the naming system</b><br> 2207 * Type: <b>token</b><br> 2208 * Path: <b>NamingSystem.jurisdiction</b><br> 2209 * </p> 2210 */ 2211 @SearchParamDefinition(name = "jurisdiction", path = "NamingSystem.jurisdiction", description = "Intended jurisdiction for the naming system", type = "token") 2212 public static final String SP_JURISDICTION = "jurisdiction"; 2213 /** 2214 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2215 * <p> 2216 * Description: <b>Intended jurisdiction for the naming system</b><br> 2217 * Type: <b>token</b><br> 2218 * Path: <b>NamingSystem.jurisdiction</b><br> 2219 * </p> 2220 */ 2221 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2222 SP_JURISDICTION); 2223 2224 /** 2225 * Search parameter: <b>description</b> 2226 * <p> 2227 * Description: <b>The description of the naming system</b><br> 2228 * Type: <b>string</b><br> 2229 * Path: <b>NamingSystem.description</b><br> 2230 * </p> 2231 */ 2232 @SearchParamDefinition(name = "description", path = "NamingSystem.description", description = "The description of the naming system", type = "string") 2233 public static final String SP_DESCRIPTION = "description"; 2234 /** 2235 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2236 * <p> 2237 * Description: <b>The description of the naming system</b><br> 2238 * Type: <b>string</b><br> 2239 * Path: <b>NamingSystem.description</b><br> 2240 * </p> 2241 */ 2242 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2243 SP_DESCRIPTION); 2244 2245 /** 2246 * Search parameter: <b>context-type</b> 2247 * <p> 2248 * Description: <b>A type of use context assigned to the naming system</b><br> 2249 * Type: <b>token</b><br> 2250 * Path: <b>NamingSystem.useContext.code</b><br> 2251 * </p> 2252 */ 2253 @SearchParamDefinition(name = "context-type", path = "NamingSystem.useContext.code", description = "A type of use context assigned to the naming system", type = "token") 2254 public static final String SP_CONTEXT_TYPE = "context-type"; 2255 /** 2256 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2257 * <p> 2258 * Description: <b>A type of use context assigned to the naming system</b><br> 2259 * Type: <b>token</b><br> 2260 * Path: <b>NamingSystem.useContext.code</b><br> 2261 * </p> 2262 */ 2263 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2264 SP_CONTEXT_TYPE); 2265 2266 /** 2267 * Search parameter: <b>type</b> 2268 * <p> 2269 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 2270 * Type: <b>token</b><br> 2271 * Path: <b>NamingSystem.type</b><br> 2272 * </p> 2273 */ 2274 @SearchParamDefinition(name = "type", path = "NamingSystem.type", description = "e.g. driver, provider, patient, bank etc.", type = "token") 2275 public static final String SP_TYPE = "type"; 2276 /** 2277 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2278 * <p> 2279 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 2280 * Type: <b>token</b><br> 2281 * Path: <b>NamingSystem.type</b><br> 2282 * </p> 2283 */ 2284 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2285 SP_TYPE); 2286 2287 /** 2288 * Search parameter: <b>id-type</b> 2289 * <p> 2290 * Description: <b>oid | uuid | uri | other</b><br> 2291 * Type: <b>token</b><br> 2292 * Path: <b>NamingSystem.uniqueId.type</b><br> 2293 * </p> 2294 */ 2295 @SearchParamDefinition(name = "id-type", path = "NamingSystem.uniqueId.type", description = "oid | uuid | uri | other", type = "token") 2296 public static final String SP_ID_TYPE = "id-type"; 2297 /** 2298 * <b>Fluent Client</b> search parameter constant for <b>id-type</b> 2299 * <p> 2300 * Description: <b>oid | uuid | uri | other</b><br> 2301 * Type: <b>token</b><br> 2302 * Path: <b>NamingSystem.uniqueId.type</b><br> 2303 * </p> 2304 */ 2305 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ID_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2306 SP_ID_TYPE); 2307 2308 /** 2309 * Search parameter: <b>context-quantity</b> 2310 * <p> 2311 * Description: <b>A quantity- or range-valued use context assigned to the 2312 * naming system</b><br> 2313 * Type: <b>quantity</b><br> 2314 * Path: <b>NamingSystem.useContext.valueQuantity, 2315 * NamingSystem.useContext.valueRange</b><br> 2316 * </p> 2317 */ 2318 @SearchParamDefinition(name = "context-quantity", path = "(NamingSystem.useContext.value as Quantity) | (NamingSystem.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the naming system", type = "quantity") 2319 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2320 /** 2321 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2322 * <p> 2323 * Description: <b>A quantity- or range-valued use context assigned to the 2324 * naming system</b><br> 2325 * Type: <b>quantity</b><br> 2326 * Path: <b>NamingSystem.useContext.valueQuantity, 2327 * NamingSystem.useContext.valueRange</b><br> 2328 * </p> 2329 */ 2330 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 2331 SP_CONTEXT_QUANTITY); 2332 2333 /** 2334 * Search parameter: <b>responsible</b> 2335 * <p> 2336 * Description: <b>Who maintains system namespace?</b><br> 2337 * Type: <b>string</b><br> 2338 * Path: <b>NamingSystem.responsible</b><br> 2339 * </p> 2340 */ 2341 @SearchParamDefinition(name = "responsible", path = "NamingSystem.responsible", description = "Who maintains system namespace?", type = "string") 2342 public static final String SP_RESPONSIBLE = "responsible"; 2343 /** 2344 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 2345 * <p> 2346 * Description: <b>Who maintains system namespace?</b><br> 2347 * Type: <b>string</b><br> 2348 * Path: <b>NamingSystem.responsible</b><br> 2349 * </p> 2350 */ 2351 public static final ca.uhn.fhir.rest.gclient.StringClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2352 SP_RESPONSIBLE); 2353 2354 /** 2355 * Search parameter: <b>contact</b> 2356 * <p> 2357 * Description: <b>Name of an individual to contact</b><br> 2358 * Type: <b>string</b><br> 2359 * Path: <b>NamingSystem.contact.name</b><br> 2360 * </p> 2361 */ 2362 @SearchParamDefinition(name = "contact", path = "NamingSystem.contact.name", description = "Name of an individual to contact", type = "string") 2363 public static final String SP_CONTACT = "contact"; 2364 /** 2365 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 2366 * <p> 2367 * Description: <b>Name of an individual to contact</b><br> 2368 * Type: <b>string</b><br> 2369 * Path: <b>NamingSystem.contact.name</b><br> 2370 * </p> 2371 */ 2372 public static final ca.uhn.fhir.rest.gclient.StringClientParam CONTACT = new ca.uhn.fhir.rest.gclient.StringClientParam( 2373 SP_CONTACT); 2374 2375 /** 2376 * Search parameter: <b>name</b> 2377 * <p> 2378 * Description: <b>Computationally friendly name of the naming system</b><br> 2379 * Type: <b>string</b><br> 2380 * Path: <b>NamingSystem.name</b><br> 2381 * </p> 2382 */ 2383 @SearchParamDefinition(name = "name", path = "NamingSystem.name", description = "Computationally friendly name of the naming system", type = "string") 2384 public static final String SP_NAME = "name"; 2385 /** 2386 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2387 * <p> 2388 * Description: <b>Computationally friendly name of the naming system</b><br> 2389 * Type: <b>string</b><br> 2390 * Path: <b>NamingSystem.name</b><br> 2391 * </p> 2392 */ 2393 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 2394 SP_NAME); 2395 2396 /** 2397 * Search parameter: <b>context</b> 2398 * <p> 2399 * Description: <b>A use context assigned to the naming system</b><br> 2400 * Type: <b>token</b><br> 2401 * Path: <b>NamingSystem.useContext.valueCodeableConcept</b><br> 2402 * </p> 2403 */ 2404 @SearchParamDefinition(name = "context", path = "(NamingSystem.useContext.value as CodeableConcept)", description = "A use context assigned to the naming system", type = "token") 2405 public static final String SP_CONTEXT = "context"; 2406 /** 2407 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2408 * <p> 2409 * Description: <b>A use context assigned to the naming system</b><br> 2410 * Type: <b>token</b><br> 2411 * Path: <b>NamingSystem.useContext.valueCodeableConcept</b><br> 2412 * </p> 2413 */ 2414 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2415 SP_CONTEXT); 2416 2417 /** 2418 * Search parameter: <b>publisher</b> 2419 * <p> 2420 * Description: <b>Name of the publisher of the naming system</b><br> 2421 * Type: <b>string</b><br> 2422 * Path: <b>NamingSystem.publisher</b><br> 2423 * </p> 2424 */ 2425 @SearchParamDefinition(name = "publisher", path = "NamingSystem.publisher", description = "Name of the publisher of the naming system", type = "string") 2426 public static final String SP_PUBLISHER = "publisher"; 2427 /** 2428 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2429 * <p> 2430 * Description: <b>Name of the publisher of the naming system</b><br> 2431 * Type: <b>string</b><br> 2432 * Path: <b>NamingSystem.publisher</b><br> 2433 * </p> 2434 */ 2435 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 2436 SP_PUBLISHER); 2437 2438 /** 2439 * Search parameter: <b>telecom</b> 2440 * <p> 2441 * Description: <b>Contact details for individual or organization</b><br> 2442 * Type: <b>token</b><br> 2443 * Path: <b>NamingSystem.contact.telecom</b><br> 2444 * </p> 2445 */ 2446 @SearchParamDefinition(name = "telecom", path = "NamingSystem.contact.telecom", description = "Contact details for individual or organization", type = "token") 2447 public static final String SP_TELECOM = "telecom"; 2448 /** 2449 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2450 * <p> 2451 * Description: <b>Contact details for individual or organization</b><br> 2452 * Type: <b>token</b><br> 2453 * Path: <b>NamingSystem.contact.telecom</b><br> 2454 * </p> 2455 */ 2456 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2457 SP_TELECOM); 2458 2459 /** 2460 * Search parameter: <b>value</b> 2461 * <p> 2462 * Description: <b>The unique identifier</b><br> 2463 * Type: <b>string</b><br> 2464 * Path: <b>NamingSystem.uniqueId.value</b><br> 2465 * </p> 2466 */ 2467 @SearchParamDefinition(name = "value", path = "NamingSystem.uniqueId.value", description = "The unique identifier", type = "string") 2468 public static final String SP_VALUE = "value"; 2469 /** 2470 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2471 * <p> 2472 * Description: <b>The unique identifier</b><br> 2473 * Type: <b>string</b><br> 2474 * Path: <b>NamingSystem.uniqueId.value</b><br> 2475 * </p> 2476 */ 2477 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2478 SP_VALUE); 2479 2480 /** 2481 * Search parameter: <b>context-type-quantity</b> 2482 * <p> 2483 * Description: <b>A use context type and quantity- or range-based value 2484 * assigned to the naming system</b><br> 2485 * Type: <b>composite</b><br> 2486 * Path: <b></b><br> 2487 * </p> 2488 */ 2489 @SearchParamDefinition(name = "context-type-quantity", path = "NamingSystem.useContext", description = "A use context type and quantity- or range-based value assigned to the naming system", type = "composite", compositeOf = { 2490 "context-type", "context-quantity" }) 2491 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2492 /** 2493 * <b>Fluent Client</b> search parameter constant for 2494 * <b>context-type-quantity</b> 2495 * <p> 2496 * Description: <b>A use context type and quantity- or range-based value 2497 * assigned to the naming system</b><br> 2498 * Type: <b>composite</b><br> 2499 * Path: <b></b><br> 2500 * </p> 2501 */ 2502 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 2503 SP_CONTEXT_TYPE_QUANTITY); 2504 2505 /** 2506 * Search parameter: <b>status</b> 2507 * <p> 2508 * Description: <b>The current status of the naming system</b><br> 2509 * Type: <b>token</b><br> 2510 * Path: <b>NamingSystem.status</b><br> 2511 * </p> 2512 */ 2513 @SearchParamDefinition(name = "status", path = "NamingSystem.status", description = "The current status of the naming system", type = "token") 2514 public static final String SP_STATUS = "status"; 2515 /** 2516 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2517 * <p> 2518 * Description: <b>The current status of the naming system</b><br> 2519 * Type: <b>token</b><br> 2520 * Path: <b>NamingSystem.status</b><br> 2521 * </p> 2522 */ 2523 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2524 SP_STATUS); 2525 2526}