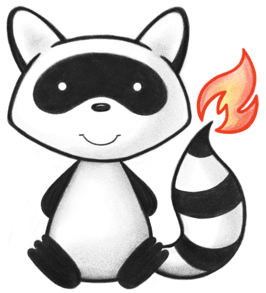
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A request to supply a diet, formula feeding (enteral) or oral nutritional 049 * supplement to a patient/resident. 050 */ 051@ResourceDef(name = "NutritionOrder", profile = "http://hl7.org/fhir/StructureDefinition/NutritionOrder") 052public class NutritionOrder extends DomainResource { 053 054 public enum NutritionOrderStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action. 057 */ 058 DRAFT, 059 /** 060 * The request is in force and ready to be acted upon. 061 */ 062 ACTIVE, 063 /** 064 * The request (and any implicit authorization to act) has been temporarily 065 * withdrawn but is expected to resume in the future. 066 */ 067 ONHOLD, 068 /** 069 * The request (and any implicit authorization to act) has been terminated prior 070 * to the known full completion of the intended actions. No further activity 071 * should occur. 072 */ 073 REVOKED, 074 /** 075 * The activity described by the request has been fully performed. No further 076 * activity will occur. 077 */ 078 COMPLETED, 079 /** 080 * This request should never have existed and should be considered 'void'. (It 081 * is possible that real-world decisions were based on it. If real-world 082 * activity has occurred, the status should be "revoked" rather than 083 * "entered-in-error".). 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring/source system does not know which of the status values 088 * currently applies for this request. Note: This concept is not to be used for 089 * "other" - one of the listed statuses is presumed to apply, but the 090 * authoring/source system does not know which. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static NutritionOrderStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("active".equals(codeString)) 104 return ACTIVE; 105 if ("on-hold".equals(codeString)) 106 return ONHOLD; 107 if ("revoked".equals(codeString)) 108 return REVOKED; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("entered-in-error".equals(codeString)) 112 return ENTEREDINERROR; 113 if ("unknown".equals(codeString)) 114 return UNKNOWN; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown NutritionOrderStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case DRAFT: 124 return "draft"; 125 case ACTIVE: 126 return "active"; 127 case ONHOLD: 128 return "on-hold"; 129 case REVOKED: 130 return "revoked"; 131 case COMPLETED: 132 return "completed"; 133 case ENTEREDINERROR: 134 return "entered-in-error"; 135 case UNKNOWN: 136 return "unknown"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case DRAFT: 147 return "http://hl7.org/fhir/request-status"; 148 case ACTIVE: 149 return "http://hl7.org/fhir/request-status"; 150 case ONHOLD: 151 return "http://hl7.org/fhir/request-status"; 152 case REVOKED: 153 return "http://hl7.org/fhir/request-status"; 154 case COMPLETED: 155 return "http://hl7.org/fhir/request-status"; 156 case ENTEREDINERROR: 157 return "http://hl7.org/fhir/request-status"; 158 case UNKNOWN: 159 return "http://hl7.org/fhir/request-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case DRAFT: 170 return "The request has been created but is not yet complete or ready for action."; 171 case ACTIVE: 172 return "The request is in force and ready to be acted upon."; 173 case ONHOLD: 174 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 175 case REVOKED: 176 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 177 case COMPLETED: 178 return "The activity described by the request has been fully performed. No further activity will occur."; 179 case ENTEREDINERROR: 180 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: 182 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case DRAFT: 193 return "Draft"; 194 case ACTIVE: 195 return "Active"; 196 case ONHOLD: 197 return "On Hold"; 198 case REVOKED: 199 return "Revoked"; 200 case COMPLETED: 201 return "Completed"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class NutritionOrderStatusEnumFactory implements EnumFactory<NutritionOrderStatus> { 215 public NutritionOrderStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return NutritionOrderStatus.DRAFT; 221 if ("active".equals(codeString)) 222 return NutritionOrderStatus.ACTIVE; 223 if ("on-hold".equals(codeString)) 224 return NutritionOrderStatus.ONHOLD; 225 if ("revoked".equals(codeString)) 226 return NutritionOrderStatus.REVOKED; 227 if ("completed".equals(codeString)) 228 return NutritionOrderStatus.COMPLETED; 229 if ("entered-in-error".equals(codeString)) 230 return NutritionOrderStatus.ENTEREDINERROR; 231 if ("unknown".equals(codeString)) 232 return NutritionOrderStatus.UNKNOWN; 233 throw new IllegalArgumentException("Unknown NutritionOrderStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<NutritionOrderStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.NULL, code); 244 if ("draft".equals(codeString)) 245 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.DRAFT, code); 246 if ("active".equals(codeString)) 247 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ACTIVE, code); 248 if ("on-hold".equals(codeString)) 249 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ONHOLD, code); 250 if ("revoked".equals(codeString)) 251 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.REVOKED, code); 252 if ("completed".equals(codeString)) 253 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.COMPLETED, code); 254 if ("entered-in-error".equals(codeString)) 255 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.ENTEREDINERROR, code); 256 if ("unknown".equals(codeString)) 257 return new Enumeration<NutritionOrderStatus>(this, NutritionOrderStatus.UNKNOWN, code); 258 throw new FHIRException("Unknown NutritionOrderStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(NutritionOrderStatus code) { 262 if (code == NutritionOrderStatus.NULL) 263 return null; 264 if (code == NutritionOrderStatus.DRAFT) 265 return "draft"; 266 if (code == NutritionOrderStatus.ACTIVE) 267 return "active"; 268 if (code == NutritionOrderStatus.ONHOLD) 269 return "on-hold"; 270 if (code == NutritionOrderStatus.REVOKED) 271 return "revoked"; 272 if (code == NutritionOrderStatus.COMPLETED) 273 return "completed"; 274 if (code == NutritionOrderStatus.ENTEREDINERROR) 275 return "entered-in-error"; 276 if (code == NutritionOrderStatus.UNKNOWN) 277 return "unknown"; 278 return "?"; 279 } 280 281 public String toSystem(NutritionOrderStatus code) { 282 return code.getSystem(); 283 } 284 } 285 286 public enum NutritiionOrderIntent { 287 /** 288 * The request is a suggestion made by someone/something that does not have an 289 * intention to ensure it occurs and without providing an authorization to act. 290 */ 291 PROPOSAL, 292 /** 293 * The request represents an intention to ensure something occurs without 294 * providing an authorization for others to act. 295 */ 296 PLAN, 297 /** 298 * The request represents a legally binding instruction authored by a Patient or 299 * RelatedPerson. 300 */ 301 DIRECTIVE, 302 /** 303 * The request represents a request/demand and authorization for action by a 304 * Practitioner. 305 */ 306 ORDER, 307 /** 308 * The request represents an original authorization for action. 309 */ 310 ORIGINALORDER, 311 /** 312 * The request represents an automatically generated supplemental authorization 313 * for action based on a parent authorization together with initial results of 314 * the action taken against that parent authorization. 315 */ 316 REFLEXORDER, 317 /** 318 * The request represents the view of an authorization instantiated by a 319 * fulfilling system representing the details of the fulfiller's intention to 320 * act upon a submitted order. 321 */ 322 FILLERORDER, 323 /** 324 * An order created in fulfillment of a broader order that represents the 325 * authorization for a single activity occurrence. E.g. The administration of a 326 * single dose of a drug. 327 */ 328 INSTANCEORDER, 329 /** 330 * The request represents a component or option for a RequestGroup that 331 * establishes timing, conditionality and/or other constraints among a set of 332 * requests. Refer to [[[RequestGroup]]] for additional information on how this 333 * status is used. 334 */ 335 OPTION, 336 /** 337 * added to help the parsers with the generic types 338 */ 339 NULL; 340 341 public static NutritiionOrderIntent fromCode(String codeString) throws FHIRException { 342 if (codeString == null || "".equals(codeString)) 343 return null; 344 if ("proposal".equals(codeString)) 345 return PROPOSAL; 346 if ("plan".equals(codeString)) 347 return PLAN; 348 if ("directive".equals(codeString)) 349 return DIRECTIVE; 350 if ("order".equals(codeString)) 351 return ORDER; 352 if ("original-order".equals(codeString)) 353 return ORIGINALORDER; 354 if ("reflex-order".equals(codeString)) 355 return REFLEXORDER; 356 if ("filler-order".equals(codeString)) 357 return FILLERORDER; 358 if ("instance-order".equals(codeString)) 359 return INSTANCEORDER; 360 if ("option".equals(codeString)) 361 return OPTION; 362 if (Configuration.isAcceptInvalidEnums()) 363 return null; 364 else 365 throw new FHIRException("Unknown NutritiionOrderIntent code '" + codeString + "'"); 366 } 367 368 public String toCode() { 369 switch (this) { 370 case PROPOSAL: 371 return "proposal"; 372 case PLAN: 373 return "plan"; 374 case DIRECTIVE: 375 return "directive"; 376 case ORDER: 377 return "order"; 378 case ORIGINALORDER: 379 return "original-order"; 380 case REFLEXORDER: 381 return "reflex-order"; 382 case FILLERORDER: 383 return "filler-order"; 384 case INSTANCEORDER: 385 return "instance-order"; 386 case OPTION: 387 return "option"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getSystem() { 396 switch (this) { 397 case PROPOSAL: 398 return "http://hl7.org/fhir/request-intent"; 399 case PLAN: 400 return "http://hl7.org/fhir/request-intent"; 401 case DIRECTIVE: 402 return "http://hl7.org/fhir/request-intent"; 403 case ORDER: 404 return "http://hl7.org/fhir/request-intent"; 405 case ORIGINALORDER: 406 return "http://hl7.org/fhir/request-intent"; 407 case REFLEXORDER: 408 return "http://hl7.org/fhir/request-intent"; 409 case FILLERORDER: 410 return "http://hl7.org/fhir/request-intent"; 411 case INSTANCEORDER: 412 return "http://hl7.org/fhir/request-intent"; 413 case OPTION: 414 return "http://hl7.org/fhir/request-intent"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getDefinition() { 423 switch (this) { 424 case PROPOSAL: 425 return "The request is a suggestion made by someone/something that does not have an intention to ensure it occurs and without providing an authorization to act."; 426 case PLAN: 427 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 428 case DIRECTIVE: 429 return "The request represents a legally binding instruction authored by a Patient or RelatedPerson."; 430 case ORDER: 431 return "The request represents a request/demand and authorization for action by a Practitioner."; 432 case ORIGINALORDER: 433 return "The request represents an original authorization for action."; 434 case REFLEXORDER: 435 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization."; 436 case FILLERORDER: 437 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 438 case INSTANCEORDER: 439 return "An order created in fulfillment of a broader order that represents the authorization for a single activity occurrence. E.g. The administration of a single dose of a drug."; 440 case OPTION: 441 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests. Refer to [[[RequestGroup]]] for additional information on how this status is used."; 442 case NULL: 443 return null; 444 default: 445 return "?"; 446 } 447 } 448 449 public String getDisplay() { 450 switch (this) { 451 case PROPOSAL: 452 return "Proposal"; 453 case PLAN: 454 return "Plan"; 455 case DIRECTIVE: 456 return "Directive"; 457 case ORDER: 458 return "Order"; 459 case ORIGINALORDER: 460 return "Original Order"; 461 case REFLEXORDER: 462 return "Reflex Order"; 463 case FILLERORDER: 464 return "Filler Order"; 465 case INSTANCEORDER: 466 return "Instance Order"; 467 case OPTION: 468 return "Option"; 469 case NULL: 470 return null; 471 default: 472 return "?"; 473 } 474 } 475 } 476 477 public static class NutritiionOrderIntentEnumFactory implements EnumFactory<NutritiionOrderIntent> { 478 public NutritiionOrderIntent fromCode(String codeString) throws IllegalArgumentException { 479 if (codeString == null || "".equals(codeString)) 480 if (codeString == null || "".equals(codeString)) 481 return null; 482 if ("proposal".equals(codeString)) 483 return NutritiionOrderIntent.PROPOSAL; 484 if ("plan".equals(codeString)) 485 return NutritiionOrderIntent.PLAN; 486 if ("directive".equals(codeString)) 487 return NutritiionOrderIntent.DIRECTIVE; 488 if ("order".equals(codeString)) 489 return NutritiionOrderIntent.ORDER; 490 if ("original-order".equals(codeString)) 491 return NutritiionOrderIntent.ORIGINALORDER; 492 if ("reflex-order".equals(codeString)) 493 return NutritiionOrderIntent.REFLEXORDER; 494 if ("filler-order".equals(codeString)) 495 return NutritiionOrderIntent.FILLERORDER; 496 if ("instance-order".equals(codeString)) 497 return NutritiionOrderIntent.INSTANCEORDER; 498 if ("option".equals(codeString)) 499 return NutritiionOrderIntent.OPTION; 500 throw new IllegalArgumentException("Unknown NutritiionOrderIntent code '" + codeString + "'"); 501 } 502 503 public Enumeration<NutritiionOrderIntent> fromType(PrimitiveType<?> code) throws FHIRException { 504 if (code == null) 505 return null; 506 if (code.isEmpty()) 507 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.NULL, code); 508 String codeString = code.asStringValue(); 509 if (codeString == null || "".equals(codeString)) 510 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.NULL, code); 511 if ("proposal".equals(codeString)) 512 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.PROPOSAL, code); 513 if ("plan".equals(codeString)) 514 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.PLAN, code); 515 if ("directive".equals(codeString)) 516 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.DIRECTIVE, code); 517 if ("order".equals(codeString)) 518 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.ORDER, code); 519 if ("original-order".equals(codeString)) 520 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.ORIGINALORDER, code); 521 if ("reflex-order".equals(codeString)) 522 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.REFLEXORDER, code); 523 if ("filler-order".equals(codeString)) 524 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.FILLERORDER, code); 525 if ("instance-order".equals(codeString)) 526 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.INSTANCEORDER, code); 527 if ("option".equals(codeString)) 528 return new Enumeration<NutritiionOrderIntent>(this, NutritiionOrderIntent.OPTION, code); 529 throw new FHIRException("Unknown NutritiionOrderIntent code '" + codeString + "'"); 530 } 531 532 public String toCode(NutritiionOrderIntent code) { 533 if (code == NutritiionOrderIntent.NULL) 534 return null; 535 if (code == NutritiionOrderIntent.PROPOSAL) 536 return "proposal"; 537 if (code == NutritiionOrderIntent.PLAN) 538 return "plan"; 539 if (code == NutritiionOrderIntent.DIRECTIVE) 540 return "directive"; 541 if (code == NutritiionOrderIntent.ORDER) 542 return "order"; 543 if (code == NutritiionOrderIntent.ORIGINALORDER) 544 return "original-order"; 545 if (code == NutritiionOrderIntent.REFLEXORDER) 546 return "reflex-order"; 547 if (code == NutritiionOrderIntent.FILLERORDER) 548 return "filler-order"; 549 if (code == NutritiionOrderIntent.INSTANCEORDER) 550 return "instance-order"; 551 if (code == NutritiionOrderIntent.OPTION) 552 return "option"; 553 return "?"; 554 } 555 556 public String toSystem(NutritiionOrderIntent code) { 557 return code.getSystem(); 558 } 559 } 560 561 @Block() 562 public static class NutritionOrderOralDietComponent extends BackboneElement implements IBaseBackboneElement { 563 /** 564 * The kind of diet or dietary restriction such as fiber restricted diet or 565 * diabetic diet. 566 */ 567 @Child(name = "type", type = { 568 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 569 @Description(shortDefinition = "Type of oral diet or diet restrictions that describe what can be consumed orally", formalDefinition = "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.") 570 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diet-type") 571 protected List<CodeableConcept> type; 572 573 /** 574 * The time period and frequency at which the diet should be given. The diet 575 * should be given for the combination of all schedules if more than one 576 * schedule is present. 577 */ 578 @Child(name = "schedule", type = { 579 Timing.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 580 @Description(shortDefinition = "Scheduled frequency of diet", formalDefinition = "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.") 581 protected List<Timing> schedule; 582 583 /** 584 * Class that defines the quantity and type of nutrient modifications (for 585 * example carbohydrate, fiber or sodium) required for the oral diet. 586 */ 587 @Child(name = "nutrient", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 588 @Description(shortDefinition = "Required nutrient modifications", formalDefinition = "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.") 589 protected List<NutritionOrderOralDietNutrientComponent> nutrient; 590 591 /** 592 * Class that describes any texture modifications required for the patient to 593 * safely consume various types of solid foods. 594 */ 595 @Child(name = "texture", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 596 @Description(shortDefinition = "Required texture modifications", formalDefinition = "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.") 597 protected List<NutritionOrderOralDietTextureComponent> texture; 598 599 /** 600 * The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) 601 * of liquids or fluids served to the patient. 602 */ 603 @Child(name = "fluidConsistencyType", type = { 604 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 605 @Description(shortDefinition = "The required consistency of fluids and liquids provided to the patient", formalDefinition = "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.") 606 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consistency-type") 607 protected List<CodeableConcept> fluidConsistencyType; 608 609 /** 610 * Free text or additional instructions or information pertaining to the oral 611 * diet. 612 */ 613 @Child(name = "instruction", type = { 614 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 615 @Description(shortDefinition = "Instructions or additional information about the oral diet", formalDefinition = "Free text or additional instructions or information pertaining to the oral diet.") 616 protected StringType instruction; 617 618 private static final long serialVersionUID = 973058412L; 619 620 /** 621 * Constructor 622 */ 623 public NutritionOrderOralDietComponent() { 624 super(); 625 } 626 627 /** 628 * @return {@link #type} (The kind of diet or dietary restriction such as fiber 629 * restricted diet or diabetic diet.) 630 */ 631 public List<CodeableConcept> getType() { 632 if (this.type == null) 633 this.type = new ArrayList<CodeableConcept>(); 634 return this.type; 635 } 636 637 /** 638 * @return Returns a reference to <code>this</code> for easy method chaining 639 */ 640 public NutritionOrderOralDietComponent setType(List<CodeableConcept> theType) { 641 this.type = theType; 642 return this; 643 } 644 645 public boolean hasType() { 646 if (this.type == null) 647 return false; 648 for (CodeableConcept item : this.type) 649 if (!item.isEmpty()) 650 return true; 651 return false; 652 } 653 654 public CodeableConcept addType() { // 3 655 CodeableConcept t = new CodeableConcept(); 656 if (this.type == null) 657 this.type = new ArrayList<CodeableConcept>(); 658 this.type.add(t); 659 return t; 660 } 661 662 public NutritionOrderOralDietComponent addType(CodeableConcept t) { // 3 663 if (t == null) 664 return this; 665 if (this.type == null) 666 this.type = new ArrayList<CodeableConcept>(); 667 this.type.add(t); 668 return this; 669 } 670 671 /** 672 * @return The first repetition of repeating field {@link #type}, creating it if 673 * it does not already exist 674 */ 675 public CodeableConcept getTypeFirstRep() { 676 if (getType().isEmpty()) { 677 addType(); 678 } 679 return getType().get(0); 680 } 681 682 /** 683 * @return {@link #schedule} (The time period and frequency at which the diet 684 * should be given. The diet should be given for the combination of all 685 * schedules if more than one schedule is present.) 686 */ 687 public List<Timing> getSchedule() { 688 if (this.schedule == null) 689 this.schedule = new ArrayList<Timing>(); 690 return this.schedule; 691 } 692 693 /** 694 * @return Returns a reference to <code>this</code> for easy method chaining 695 */ 696 public NutritionOrderOralDietComponent setSchedule(List<Timing> theSchedule) { 697 this.schedule = theSchedule; 698 return this; 699 } 700 701 public boolean hasSchedule() { 702 if (this.schedule == null) 703 return false; 704 for (Timing item : this.schedule) 705 if (!item.isEmpty()) 706 return true; 707 return false; 708 } 709 710 public Timing addSchedule() { // 3 711 Timing t = new Timing(); 712 if (this.schedule == null) 713 this.schedule = new ArrayList<Timing>(); 714 this.schedule.add(t); 715 return t; 716 } 717 718 public NutritionOrderOralDietComponent addSchedule(Timing t) { // 3 719 if (t == null) 720 return this; 721 if (this.schedule == null) 722 this.schedule = new ArrayList<Timing>(); 723 this.schedule.add(t); 724 return this; 725 } 726 727 /** 728 * @return The first repetition of repeating field {@link #schedule}, creating 729 * it if it does not already exist 730 */ 731 public Timing getScheduleFirstRep() { 732 if (getSchedule().isEmpty()) { 733 addSchedule(); 734 } 735 return getSchedule().get(0); 736 } 737 738 /** 739 * @return {@link #nutrient} (Class that defines the quantity and type of 740 * nutrient modifications (for example carbohydrate, fiber or sodium) 741 * required for the oral diet.) 742 */ 743 public List<NutritionOrderOralDietNutrientComponent> getNutrient() { 744 if (this.nutrient == null) 745 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 746 return this.nutrient; 747 } 748 749 /** 750 * @return Returns a reference to <code>this</code> for easy method chaining 751 */ 752 public NutritionOrderOralDietComponent setNutrient(List<NutritionOrderOralDietNutrientComponent> theNutrient) { 753 this.nutrient = theNutrient; 754 return this; 755 } 756 757 public boolean hasNutrient() { 758 if (this.nutrient == null) 759 return false; 760 for (NutritionOrderOralDietNutrientComponent item : this.nutrient) 761 if (!item.isEmpty()) 762 return true; 763 return false; 764 } 765 766 public NutritionOrderOralDietNutrientComponent addNutrient() { // 3 767 NutritionOrderOralDietNutrientComponent t = new NutritionOrderOralDietNutrientComponent(); 768 if (this.nutrient == null) 769 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 770 this.nutrient.add(t); 771 return t; 772 } 773 774 public NutritionOrderOralDietComponent addNutrient(NutritionOrderOralDietNutrientComponent t) { // 3 775 if (t == null) 776 return this; 777 if (this.nutrient == null) 778 this.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 779 this.nutrient.add(t); 780 return this; 781 } 782 783 /** 784 * @return The first repetition of repeating field {@link #nutrient}, creating 785 * it if it does not already exist 786 */ 787 public NutritionOrderOralDietNutrientComponent getNutrientFirstRep() { 788 if (getNutrient().isEmpty()) { 789 addNutrient(); 790 } 791 return getNutrient().get(0); 792 } 793 794 /** 795 * @return {@link #texture} (Class that describes any texture modifications 796 * required for the patient to safely consume various types of solid 797 * foods.) 798 */ 799 public List<NutritionOrderOralDietTextureComponent> getTexture() { 800 if (this.texture == null) 801 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 802 return this.texture; 803 } 804 805 /** 806 * @return Returns a reference to <code>this</code> for easy method chaining 807 */ 808 public NutritionOrderOralDietComponent setTexture(List<NutritionOrderOralDietTextureComponent> theTexture) { 809 this.texture = theTexture; 810 return this; 811 } 812 813 public boolean hasTexture() { 814 if (this.texture == null) 815 return false; 816 for (NutritionOrderOralDietTextureComponent item : this.texture) 817 if (!item.isEmpty()) 818 return true; 819 return false; 820 } 821 822 public NutritionOrderOralDietTextureComponent addTexture() { // 3 823 NutritionOrderOralDietTextureComponent t = new NutritionOrderOralDietTextureComponent(); 824 if (this.texture == null) 825 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 826 this.texture.add(t); 827 return t; 828 } 829 830 public NutritionOrderOralDietComponent addTexture(NutritionOrderOralDietTextureComponent t) { // 3 831 if (t == null) 832 return this; 833 if (this.texture == null) 834 this.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 835 this.texture.add(t); 836 return this; 837 } 838 839 /** 840 * @return The first repetition of repeating field {@link #texture}, creating it 841 * if it does not already exist 842 */ 843 public NutritionOrderOralDietTextureComponent getTextureFirstRep() { 844 if (getTexture().isEmpty()) { 845 addTexture(); 846 } 847 return getTexture().get(0); 848 } 849 850 /** 851 * @return {@link #fluidConsistencyType} (The required consistency (e.g. 852 * honey-thick, nectar-thick, thin, thickened.) of liquids or fluids 853 * served to the patient.) 854 */ 855 public List<CodeableConcept> getFluidConsistencyType() { 856 if (this.fluidConsistencyType == null) 857 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 858 return this.fluidConsistencyType; 859 } 860 861 /** 862 * @return Returns a reference to <code>this</code> for easy method chaining 863 */ 864 public NutritionOrderOralDietComponent setFluidConsistencyType(List<CodeableConcept> theFluidConsistencyType) { 865 this.fluidConsistencyType = theFluidConsistencyType; 866 return this; 867 } 868 869 public boolean hasFluidConsistencyType() { 870 if (this.fluidConsistencyType == null) 871 return false; 872 for (CodeableConcept item : this.fluidConsistencyType) 873 if (!item.isEmpty()) 874 return true; 875 return false; 876 } 877 878 public CodeableConcept addFluidConsistencyType() { // 3 879 CodeableConcept t = new CodeableConcept(); 880 if (this.fluidConsistencyType == null) 881 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 882 this.fluidConsistencyType.add(t); 883 return t; 884 } 885 886 public NutritionOrderOralDietComponent addFluidConsistencyType(CodeableConcept t) { // 3 887 if (t == null) 888 return this; 889 if (this.fluidConsistencyType == null) 890 this.fluidConsistencyType = new ArrayList<CodeableConcept>(); 891 this.fluidConsistencyType.add(t); 892 return this; 893 } 894 895 /** 896 * @return The first repetition of repeating field 897 * {@link #fluidConsistencyType}, creating it if it does not already 898 * exist 899 */ 900 public CodeableConcept getFluidConsistencyTypeFirstRep() { 901 if (getFluidConsistencyType().isEmpty()) { 902 addFluidConsistencyType(); 903 } 904 return getFluidConsistencyType().get(0); 905 } 906 907 /** 908 * @return {@link #instruction} (Free text or additional instructions or 909 * information pertaining to the oral diet.). This is the underlying 910 * object with id, value and extensions. The accessor "getInstruction" 911 * gives direct access to the value 912 */ 913 public StringType getInstructionElement() { 914 if (this.instruction == null) 915 if (Configuration.errorOnAutoCreate()) 916 throw new Error("Attempt to auto-create NutritionOrderOralDietComponent.instruction"); 917 else if (Configuration.doAutoCreate()) 918 this.instruction = new StringType(); // bb 919 return this.instruction; 920 } 921 922 public boolean hasInstructionElement() { 923 return this.instruction != null && !this.instruction.isEmpty(); 924 } 925 926 public boolean hasInstruction() { 927 return this.instruction != null && !this.instruction.isEmpty(); 928 } 929 930 /** 931 * @param value {@link #instruction} (Free text or additional instructions or 932 * information pertaining to the oral diet.). This is the 933 * underlying object with id, value and extensions. The accessor 934 * "getInstruction" gives direct access to the value 935 */ 936 public NutritionOrderOralDietComponent setInstructionElement(StringType value) { 937 this.instruction = value; 938 return this; 939 } 940 941 /** 942 * @return Free text or additional instructions or information pertaining to the 943 * oral diet. 944 */ 945 public String getInstruction() { 946 return this.instruction == null ? null : this.instruction.getValue(); 947 } 948 949 /** 950 * @param value Free text or additional instructions or information pertaining 951 * to the oral diet. 952 */ 953 public NutritionOrderOralDietComponent setInstruction(String value) { 954 if (Utilities.noString(value)) 955 this.instruction = null; 956 else { 957 if (this.instruction == null) 958 this.instruction = new StringType(); 959 this.instruction.setValue(value); 960 } 961 return this; 962 } 963 964 protected void listChildren(List<Property> children) { 965 super.listChildren(children); 966 children.add(new Property("type", "CodeableConcept", 967 "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, 968 java.lang.Integer.MAX_VALUE, type)); 969 children.add(new Property("schedule", "Timing", 970 "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.", 971 0, java.lang.Integer.MAX_VALUE, schedule)); 972 children.add(new Property("nutrient", "", 973 "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.", 974 0, java.lang.Integer.MAX_VALUE, nutrient)); 975 children.add(new Property("texture", "", 976 "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 977 0, java.lang.Integer.MAX_VALUE, texture)); 978 children.add(new Property("fluidConsistencyType", "CodeableConcept", 979 "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 980 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType)); 981 children.add(new Property("instruction", "string", 982 "Free text or additional instructions or information pertaining to the oral diet.", 0, 1, instruction)); 983 } 984 985 @Override 986 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 987 switch (_hash) { 988 case 3575610: 989 /* type */ return new Property("type", "CodeableConcept", 990 "The kind of diet or dietary restriction such as fiber restricted diet or diabetic diet.", 0, 991 java.lang.Integer.MAX_VALUE, type); 992 case -697920873: 993 /* schedule */ return new Property("schedule", "Timing", 994 "The time period and frequency at which the diet should be given. The diet should be given for the combination of all schedules if more than one schedule is present.", 995 0, java.lang.Integer.MAX_VALUE, schedule); 996 case -1671151641: 997 /* nutrient */ return new Property("nutrient", "", 998 "Class that defines the quantity and type of nutrient modifications (for example carbohydrate, fiber or sodium) required for the oral diet.", 999 0, java.lang.Integer.MAX_VALUE, nutrient); 1000 case -1417816805: 1001 /* texture */ return new Property("texture", "", 1002 "Class that describes any texture modifications required for the patient to safely consume various types of solid foods.", 1003 0, java.lang.Integer.MAX_VALUE, texture); 1004 case -525105592: 1005 /* fluidConsistencyType */ return new Property("fluidConsistencyType", "CodeableConcept", 1006 "The required consistency (e.g. honey-thick, nectar-thick, thin, thickened.) of liquids or fluids served to the patient.", 1007 0, java.lang.Integer.MAX_VALUE, fluidConsistencyType); 1008 case 301526158: 1009 /* instruction */ return new Property("instruction", "string", 1010 "Free text or additional instructions or information pertaining to the oral diet.", 0, 1, instruction); 1011 default: 1012 return super.getNamedProperty(_hash, _name, _checkValid); 1013 } 1014 1015 } 1016 1017 @Override 1018 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1019 switch (hash) { 1020 case 3575610: 1021 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1022 case -697920873: 1023 /* schedule */ return this.schedule == null ? new Base[0] 1024 : this.schedule.toArray(new Base[this.schedule.size()]); // Timing 1025 case -1671151641: 1026 /* nutrient */ return this.nutrient == null ? new Base[0] 1027 : this.nutrient.toArray(new Base[this.nutrient.size()]); // NutritionOrderOralDietNutrientComponent 1028 case -1417816805: 1029 /* texture */ return this.texture == null ? new Base[0] : this.texture.toArray(new Base[this.texture.size()]); // NutritionOrderOralDietTextureComponent 1030 case -525105592: 1031 /* fluidConsistencyType */ return this.fluidConsistencyType == null ? new Base[0] 1032 : this.fluidConsistencyType.toArray(new Base[this.fluidConsistencyType.size()]); // CodeableConcept 1033 case 301526158: 1034 /* instruction */ return this.instruction == null ? new Base[0] : new Base[] { this.instruction }; // StringType 1035 default: 1036 return super.getProperty(hash, name, checkValid); 1037 } 1038 1039 } 1040 1041 @Override 1042 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1043 switch (hash) { 1044 case 3575610: // type 1045 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1046 return value; 1047 case -697920873: // schedule 1048 this.getSchedule().add(castToTiming(value)); // Timing 1049 return value; 1050 case -1671151641: // nutrient 1051 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); // NutritionOrderOralDietNutrientComponent 1052 return value; 1053 case -1417816805: // texture 1054 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); // NutritionOrderOralDietTextureComponent 1055 return value; 1056 case -525105592: // fluidConsistencyType 1057 this.getFluidConsistencyType().add(castToCodeableConcept(value)); // CodeableConcept 1058 return value; 1059 case 301526158: // instruction 1060 this.instruction = castToString(value); // StringType 1061 return value; 1062 default: 1063 return super.setProperty(hash, name, value); 1064 } 1065 1066 } 1067 1068 @Override 1069 public Base setProperty(String name, Base value) throws FHIRException { 1070 if (name.equals("type")) { 1071 this.getType().add(castToCodeableConcept(value)); 1072 } else if (name.equals("schedule")) { 1073 this.getSchedule().add(castToTiming(value)); 1074 } else if (name.equals("nutrient")) { 1075 this.getNutrient().add((NutritionOrderOralDietNutrientComponent) value); 1076 } else if (name.equals("texture")) { 1077 this.getTexture().add((NutritionOrderOralDietTextureComponent) value); 1078 } else if (name.equals("fluidConsistencyType")) { 1079 this.getFluidConsistencyType().add(castToCodeableConcept(value)); 1080 } else if (name.equals("instruction")) { 1081 this.instruction = castToString(value); // StringType 1082 } else 1083 return super.setProperty(name, value); 1084 return value; 1085 } 1086 1087 @Override 1088 public void removeChild(String name, Base value) throws FHIRException { 1089 if (name.equals("type")) { 1090 this.getType().remove(castToCodeableConcept(value)); 1091 } else if (name.equals("schedule")) { 1092 this.getSchedule().remove(castToTiming(value)); 1093 } else if (name.equals("nutrient")) { 1094 this.getNutrient().remove((NutritionOrderOralDietNutrientComponent) value); 1095 } else if (name.equals("texture")) { 1096 this.getTexture().remove((NutritionOrderOralDietTextureComponent) value); 1097 } else if (name.equals("fluidConsistencyType")) { 1098 this.getFluidConsistencyType().remove(castToCodeableConcept(value)); 1099 } else if (name.equals("instruction")) { 1100 this.instruction = null; 1101 } else 1102 super.removeChild(name, value); 1103 1104 } 1105 1106 @Override 1107 public Base makeProperty(int hash, String name) throws FHIRException { 1108 switch (hash) { 1109 case 3575610: 1110 return addType(); 1111 case -697920873: 1112 return addSchedule(); 1113 case -1671151641: 1114 return addNutrient(); 1115 case -1417816805: 1116 return addTexture(); 1117 case -525105592: 1118 return addFluidConsistencyType(); 1119 case 301526158: 1120 return getInstructionElement(); 1121 default: 1122 return super.makeProperty(hash, name); 1123 } 1124 1125 } 1126 1127 @Override 1128 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1129 switch (hash) { 1130 case 3575610: 1131 /* type */ return new String[] { "CodeableConcept" }; 1132 case -697920873: 1133 /* schedule */ return new String[] { "Timing" }; 1134 case -1671151641: 1135 /* nutrient */ return new String[] {}; 1136 case -1417816805: 1137 /* texture */ return new String[] {}; 1138 case -525105592: 1139 /* fluidConsistencyType */ return new String[] { "CodeableConcept" }; 1140 case 301526158: 1141 /* instruction */ return new String[] { "string" }; 1142 default: 1143 return super.getTypesForProperty(hash, name); 1144 } 1145 1146 } 1147 1148 @Override 1149 public Base addChild(String name) throws FHIRException { 1150 if (name.equals("type")) { 1151 return addType(); 1152 } else if (name.equals("schedule")) { 1153 return addSchedule(); 1154 } else if (name.equals("nutrient")) { 1155 return addNutrient(); 1156 } else if (name.equals("texture")) { 1157 return addTexture(); 1158 } else if (name.equals("fluidConsistencyType")) { 1159 return addFluidConsistencyType(); 1160 } else if (name.equals("instruction")) { 1161 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 1162 } else 1163 return super.addChild(name); 1164 } 1165 1166 public NutritionOrderOralDietComponent copy() { 1167 NutritionOrderOralDietComponent dst = new NutritionOrderOralDietComponent(); 1168 copyValues(dst); 1169 return dst; 1170 } 1171 1172 public void copyValues(NutritionOrderOralDietComponent dst) { 1173 super.copyValues(dst); 1174 if (type != null) { 1175 dst.type = new ArrayList<CodeableConcept>(); 1176 for (CodeableConcept i : type) 1177 dst.type.add(i.copy()); 1178 } 1179 ; 1180 if (schedule != null) { 1181 dst.schedule = new ArrayList<Timing>(); 1182 for (Timing i : schedule) 1183 dst.schedule.add(i.copy()); 1184 } 1185 ; 1186 if (nutrient != null) { 1187 dst.nutrient = new ArrayList<NutritionOrderOralDietNutrientComponent>(); 1188 for (NutritionOrderOralDietNutrientComponent i : nutrient) 1189 dst.nutrient.add(i.copy()); 1190 } 1191 ; 1192 if (texture != null) { 1193 dst.texture = new ArrayList<NutritionOrderOralDietTextureComponent>(); 1194 for (NutritionOrderOralDietTextureComponent i : texture) 1195 dst.texture.add(i.copy()); 1196 } 1197 ; 1198 if (fluidConsistencyType != null) { 1199 dst.fluidConsistencyType = new ArrayList<CodeableConcept>(); 1200 for (CodeableConcept i : fluidConsistencyType) 1201 dst.fluidConsistencyType.add(i.copy()); 1202 } 1203 ; 1204 dst.instruction = instruction == null ? null : instruction.copy(); 1205 } 1206 1207 @Override 1208 public boolean equalsDeep(Base other_) { 1209 if (!super.equalsDeep(other_)) 1210 return false; 1211 if (!(other_ instanceof NutritionOrderOralDietComponent)) 1212 return false; 1213 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other_; 1214 return compareDeep(type, o.type, true) && compareDeep(schedule, o.schedule, true) 1215 && compareDeep(nutrient, o.nutrient, true) && compareDeep(texture, o.texture, true) 1216 && compareDeep(fluidConsistencyType, o.fluidConsistencyType, true) 1217 && compareDeep(instruction, o.instruction, true); 1218 } 1219 1220 @Override 1221 public boolean equalsShallow(Base other_) { 1222 if (!super.equalsShallow(other_)) 1223 return false; 1224 if (!(other_ instanceof NutritionOrderOralDietComponent)) 1225 return false; 1226 NutritionOrderOralDietComponent o = (NutritionOrderOralDietComponent) other_; 1227 return compareValues(instruction, o.instruction, true); 1228 } 1229 1230 public boolean isEmpty() { 1231 return super.isEmpty() 1232 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, schedule, nutrient, texture, fluidConsistencyType, instruction); 1233 } 1234 1235 public String fhirType() { 1236 return "NutritionOrder.oralDiet"; 1237 1238 } 1239 1240 } 1241 1242 @Block() 1243 public static class NutritionOrderOralDietNutrientComponent extends BackboneElement implements IBaseBackboneElement { 1244 /** 1245 * The nutrient that is being modified such as carbohydrate or sodium. 1246 */ 1247 @Child(name = "modifier", type = { 1248 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1249 @Description(shortDefinition = "Type of nutrient that is being modified", formalDefinition = "The nutrient that is being modified such as carbohydrate or sodium.") 1250 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/nutrient-code") 1251 protected CodeableConcept modifier; 1252 1253 /** 1254 * The quantity of the specified nutrient to include in diet. 1255 */ 1256 @Child(name = "amount", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1257 @Description(shortDefinition = "Quantity of the specified nutrient", formalDefinition = "The quantity of the specified nutrient to include in diet.") 1258 protected Quantity amount; 1259 1260 private static final long serialVersionUID = 1042462093L; 1261 1262 /** 1263 * Constructor 1264 */ 1265 public NutritionOrderOralDietNutrientComponent() { 1266 super(); 1267 } 1268 1269 /** 1270 * @return {@link #modifier} (The nutrient that is being modified such as 1271 * carbohydrate or sodium.) 1272 */ 1273 public CodeableConcept getModifier() { 1274 if (this.modifier == null) 1275 if (Configuration.errorOnAutoCreate()) 1276 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.modifier"); 1277 else if (Configuration.doAutoCreate()) 1278 this.modifier = new CodeableConcept(); // cc 1279 return this.modifier; 1280 } 1281 1282 public boolean hasModifier() { 1283 return this.modifier != null && !this.modifier.isEmpty(); 1284 } 1285 1286 /** 1287 * @param value {@link #modifier} (The nutrient that is being modified such as 1288 * carbohydrate or sodium.) 1289 */ 1290 public NutritionOrderOralDietNutrientComponent setModifier(CodeableConcept value) { 1291 this.modifier = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return {@link #amount} (The quantity of the specified nutrient to include in 1297 * diet.) 1298 */ 1299 public Quantity getAmount() { 1300 if (this.amount == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create NutritionOrderOralDietNutrientComponent.amount"); 1303 else if (Configuration.doAutoCreate()) 1304 this.amount = new Quantity(); // cc 1305 return this.amount; 1306 } 1307 1308 public boolean hasAmount() { 1309 return this.amount != null && !this.amount.isEmpty(); 1310 } 1311 1312 /** 1313 * @param value {@link #amount} (The quantity of the specified nutrient to 1314 * include in diet.) 1315 */ 1316 public NutritionOrderOralDietNutrientComponent setAmount(Quantity value) { 1317 this.amount = value; 1318 return this; 1319 } 1320 1321 protected void listChildren(List<Property> children) { 1322 super.listChildren(children); 1323 children.add(new Property("modifier", "CodeableConcept", 1324 "The nutrient that is being modified such as carbohydrate or sodium.", 0, 1, modifier)); 1325 children.add(new Property("amount", "SimpleQuantity", 1326 "The quantity of the specified nutrient to include in diet.", 0, 1, amount)); 1327 } 1328 1329 @Override 1330 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1331 switch (_hash) { 1332 case -615513385: 1333 /* modifier */ return new Property("modifier", "CodeableConcept", 1334 "The nutrient that is being modified such as carbohydrate or sodium.", 0, 1, modifier); 1335 case -1413853096: 1336 /* amount */ return new Property("amount", "SimpleQuantity", 1337 "The quantity of the specified nutrient to include in diet.", 0, 1, amount); 1338 default: 1339 return super.getNamedProperty(_hash, _name, _checkValid); 1340 } 1341 1342 } 1343 1344 @Override 1345 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1346 switch (hash) { 1347 case -615513385: 1348 /* modifier */ return this.modifier == null ? new Base[0] : new Base[] { this.modifier }; // CodeableConcept 1349 case -1413853096: 1350 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Quantity 1351 default: 1352 return super.getProperty(hash, name, checkValid); 1353 } 1354 1355 } 1356 1357 @Override 1358 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1359 switch (hash) { 1360 case -615513385: // modifier 1361 this.modifier = castToCodeableConcept(value); // CodeableConcept 1362 return value; 1363 case -1413853096: // amount 1364 this.amount = castToQuantity(value); // Quantity 1365 return value; 1366 default: 1367 return super.setProperty(hash, name, value); 1368 } 1369 1370 } 1371 1372 @Override 1373 public Base setProperty(String name, Base value) throws FHIRException { 1374 if (name.equals("modifier")) { 1375 this.modifier = castToCodeableConcept(value); // CodeableConcept 1376 } else if (name.equals("amount")) { 1377 this.amount = castToQuantity(value); // Quantity 1378 } else 1379 return super.setProperty(name, value); 1380 return value; 1381 } 1382 1383 @Override 1384 public void removeChild(String name, Base value) throws FHIRException { 1385 if (name.equals("modifier")) { 1386 this.modifier = null; 1387 } else if (name.equals("amount")) { 1388 this.amount = null; 1389 } else 1390 super.removeChild(name, value); 1391 1392 } 1393 1394 @Override 1395 public Base makeProperty(int hash, String name) throws FHIRException { 1396 switch (hash) { 1397 case -615513385: 1398 return getModifier(); 1399 case -1413853096: 1400 return getAmount(); 1401 default: 1402 return super.makeProperty(hash, name); 1403 } 1404 1405 } 1406 1407 @Override 1408 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1409 switch (hash) { 1410 case -615513385: 1411 /* modifier */ return new String[] { "CodeableConcept" }; 1412 case -1413853096: 1413 /* amount */ return new String[] { "SimpleQuantity" }; 1414 default: 1415 return super.getTypesForProperty(hash, name); 1416 } 1417 1418 } 1419 1420 @Override 1421 public Base addChild(String name) throws FHIRException { 1422 if (name.equals("modifier")) { 1423 this.modifier = new CodeableConcept(); 1424 return this.modifier; 1425 } else if (name.equals("amount")) { 1426 this.amount = new Quantity(); 1427 return this.amount; 1428 } else 1429 return super.addChild(name); 1430 } 1431 1432 public NutritionOrderOralDietNutrientComponent copy() { 1433 NutritionOrderOralDietNutrientComponent dst = new NutritionOrderOralDietNutrientComponent(); 1434 copyValues(dst); 1435 return dst; 1436 } 1437 1438 public void copyValues(NutritionOrderOralDietNutrientComponent dst) { 1439 super.copyValues(dst); 1440 dst.modifier = modifier == null ? null : modifier.copy(); 1441 dst.amount = amount == null ? null : amount.copy(); 1442 } 1443 1444 @Override 1445 public boolean equalsDeep(Base other_) { 1446 if (!super.equalsDeep(other_)) 1447 return false; 1448 if (!(other_ instanceof NutritionOrderOralDietNutrientComponent)) 1449 return false; 1450 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other_; 1451 return compareDeep(modifier, o.modifier, true) && compareDeep(amount, o.amount, true); 1452 } 1453 1454 @Override 1455 public boolean equalsShallow(Base other_) { 1456 if (!super.equalsShallow(other_)) 1457 return false; 1458 if (!(other_ instanceof NutritionOrderOralDietNutrientComponent)) 1459 return false; 1460 NutritionOrderOralDietNutrientComponent o = (NutritionOrderOralDietNutrientComponent) other_; 1461 return true; 1462 } 1463 1464 public boolean isEmpty() { 1465 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifier, amount); 1466 } 1467 1468 public String fhirType() { 1469 return "NutritionOrder.oralDiet.nutrient"; 1470 1471 } 1472 1473 } 1474 1475 @Block() 1476 public static class NutritionOrderOralDietTextureComponent extends BackboneElement implements IBaseBackboneElement { 1477 /** 1478 * Any texture modifications (for solid foods) that should be made, e.g. easy to 1479 * chew, chopped, ground, and pureed. 1480 */ 1481 @Child(name = "modifier", type = { 1482 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1483 @Description(shortDefinition = "Code to indicate how to alter the texture of the foods, e.g. pureed", formalDefinition = "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.") 1484 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/texture-code") 1485 protected CodeableConcept modifier; 1486 1487 /** 1488 * The food type(s) (e.g. meats, all foods) that the texture modification 1489 * applies to. This could be all foods types. 1490 */ 1491 @Child(name = "foodType", type = { 1492 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1493 @Description(shortDefinition = "Concepts that are used to identify an entity that is ingested for nutritional purposes", formalDefinition = "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.") 1494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/modified-foodtype") 1495 protected CodeableConcept foodType; 1496 1497 private static final long serialVersionUID = -56402817L; 1498 1499 /** 1500 * Constructor 1501 */ 1502 public NutritionOrderOralDietTextureComponent() { 1503 super(); 1504 } 1505 1506 /** 1507 * @return {@link #modifier} (Any texture modifications (for solid foods) that 1508 * should be made, e.g. easy to chew, chopped, ground, and pureed.) 1509 */ 1510 public CodeableConcept getModifier() { 1511 if (this.modifier == null) 1512 if (Configuration.errorOnAutoCreate()) 1513 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.modifier"); 1514 else if (Configuration.doAutoCreate()) 1515 this.modifier = new CodeableConcept(); // cc 1516 return this.modifier; 1517 } 1518 1519 public boolean hasModifier() { 1520 return this.modifier != null && !this.modifier.isEmpty(); 1521 } 1522 1523 /** 1524 * @param value {@link #modifier} (Any texture modifications (for solid foods) 1525 * that should be made, e.g. easy to chew, chopped, ground, and 1526 * pureed.) 1527 */ 1528 public NutritionOrderOralDietTextureComponent setModifier(CodeableConcept value) { 1529 this.modifier = value; 1530 return this; 1531 } 1532 1533 /** 1534 * @return {@link #foodType} (The food type(s) (e.g. meats, all foods) that the 1535 * texture modification applies to. This could be all foods types.) 1536 */ 1537 public CodeableConcept getFoodType() { 1538 if (this.foodType == null) 1539 if (Configuration.errorOnAutoCreate()) 1540 throw new Error("Attempt to auto-create NutritionOrderOralDietTextureComponent.foodType"); 1541 else if (Configuration.doAutoCreate()) 1542 this.foodType = new CodeableConcept(); // cc 1543 return this.foodType; 1544 } 1545 1546 public boolean hasFoodType() { 1547 return this.foodType != null && !this.foodType.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #foodType} (The food type(s) (e.g. meats, all foods) that 1552 * the texture modification applies to. This could be all foods 1553 * types.) 1554 */ 1555 public NutritionOrderOralDietTextureComponent setFoodType(CodeableConcept value) { 1556 this.foodType = value; 1557 return this; 1558 } 1559 1560 protected void listChildren(List<Property> children) { 1561 super.listChildren(children); 1562 children.add(new Property("modifier", "CodeableConcept", 1563 "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 1564 0, 1, modifier)); 1565 children.add(new Property("foodType", "CodeableConcept", 1566 "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 1567 0, 1, foodType)); 1568 } 1569 1570 @Override 1571 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1572 switch (_hash) { 1573 case -615513385: 1574 /* modifier */ return new Property("modifier", "CodeableConcept", 1575 "Any texture modifications (for solid foods) that should be made, e.g. easy to chew, chopped, ground, and pureed.", 1576 0, 1, modifier); 1577 case 379498680: 1578 /* foodType */ return new Property("foodType", "CodeableConcept", 1579 "The food type(s) (e.g. meats, all foods) that the texture modification applies to. This could be all foods types.", 1580 0, 1, foodType); 1581 default: 1582 return super.getNamedProperty(_hash, _name, _checkValid); 1583 } 1584 1585 } 1586 1587 @Override 1588 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1589 switch (hash) { 1590 case -615513385: 1591 /* modifier */ return this.modifier == null ? new Base[0] : new Base[] { this.modifier }; // CodeableConcept 1592 case 379498680: 1593 /* foodType */ return this.foodType == null ? new Base[0] : new Base[] { this.foodType }; // CodeableConcept 1594 default: 1595 return super.getProperty(hash, name, checkValid); 1596 } 1597 1598 } 1599 1600 @Override 1601 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1602 switch (hash) { 1603 case -615513385: // modifier 1604 this.modifier = castToCodeableConcept(value); // CodeableConcept 1605 return value; 1606 case 379498680: // foodType 1607 this.foodType = castToCodeableConcept(value); // CodeableConcept 1608 return value; 1609 default: 1610 return super.setProperty(hash, name, value); 1611 } 1612 1613 } 1614 1615 @Override 1616 public Base setProperty(String name, Base value) throws FHIRException { 1617 if (name.equals("modifier")) { 1618 this.modifier = castToCodeableConcept(value); // CodeableConcept 1619 } else if (name.equals("foodType")) { 1620 this.foodType = castToCodeableConcept(value); // CodeableConcept 1621 } else 1622 return super.setProperty(name, value); 1623 return value; 1624 } 1625 1626 @Override 1627 public void removeChild(String name, Base value) throws FHIRException { 1628 if (name.equals("modifier")) { 1629 this.modifier = null; 1630 } else if (name.equals("foodType")) { 1631 this.foodType = null; 1632 } else 1633 super.removeChild(name, value); 1634 1635 } 1636 1637 @Override 1638 public Base makeProperty(int hash, String name) throws FHIRException { 1639 switch (hash) { 1640 case -615513385: 1641 return getModifier(); 1642 case 379498680: 1643 return getFoodType(); 1644 default: 1645 return super.makeProperty(hash, name); 1646 } 1647 1648 } 1649 1650 @Override 1651 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1652 switch (hash) { 1653 case -615513385: 1654 /* modifier */ return new String[] { "CodeableConcept" }; 1655 case 379498680: 1656 /* foodType */ return new String[] { "CodeableConcept" }; 1657 default: 1658 return super.getTypesForProperty(hash, name); 1659 } 1660 1661 } 1662 1663 @Override 1664 public Base addChild(String name) throws FHIRException { 1665 if (name.equals("modifier")) { 1666 this.modifier = new CodeableConcept(); 1667 return this.modifier; 1668 } else if (name.equals("foodType")) { 1669 this.foodType = new CodeableConcept(); 1670 return this.foodType; 1671 } else 1672 return super.addChild(name); 1673 } 1674 1675 public NutritionOrderOralDietTextureComponent copy() { 1676 NutritionOrderOralDietTextureComponent dst = new NutritionOrderOralDietTextureComponent(); 1677 copyValues(dst); 1678 return dst; 1679 } 1680 1681 public void copyValues(NutritionOrderOralDietTextureComponent dst) { 1682 super.copyValues(dst); 1683 dst.modifier = modifier == null ? null : modifier.copy(); 1684 dst.foodType = foodType == null ? null : foodType.copy(); 1685 } 1686 1687 @Override 1688 public boolean equalsDeep(Base other_) { 1689 if (!super.equalsDeep(other_)) 1690 return false; 1691 if (!(other_ instanceof NutritionOrderOralDietTextureComponent)) 1692 return false; 1693 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other_; 1694 return compareDeep(modifier, o.modifier, true) && compareDeep(foodType, o.foodType, true); 1695 } 1696 1697 @Override 1698 public boolean equalsShallow(Base other_) { 1699 if (!super.equalsShallow(other_)) 1700 return false; 1701 if (!(other_ instanceof NutritionOrderOralDietTextureComponent)) 1702 return false; 1703 NutritionOrderOralDietTextureComponent o = (NutritionOrderOralDietTextureComponent) other_; 1704 return true; 1705 } 1706 1707 public boolean isEmpty() { 1708 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifier, foodType); 1709 } 1710 1711 public String fhirType() { 1712 return "NutritionOrder.oralDiet.texture"; 1713 1714 } 1715 1716 } 1717 1718 @Block() 1719 public static class NutritionOrderSupplementComponent extends BackboneElement implements IBaseBackboneElement { 1720 /** 1721 * The kind of nutritional supplement product required such as a high protein or 1722 * pediatric clear liquid supplement. 1723 */ 1724 @Child(name = "type", type = { 1725 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1726 @Description(shortDefinition = "Type of supplement product requested", formalDefinition = "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.") 1727 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/supplement-type") 1728 protected CodeableConcept type; 1729 1730 /** 1731 * The product or brand name of the nutritional supplement such as "Acme Protein 1732 * Shake". 1733 */ 1734 @Child(name = "productName", type = { 1735 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1736 @Description(shortDefinition = "Product or brand name of the nutritional supplement", formalDefinition = "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".") 1737 protected StringType productName; 1738 1739 /** 1740 * The time period and frequency at which the supplement(s) should be given. The 1741 * supplement should be given for the combination of all schedules if more than 1742 * one schedule is present. 1743 */ 1744 @Child(name = "schedule", type = { 1745 Timing.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1746 @Description(shortDefinition = "Scheduled frequency of supplement", formalDefinition = "The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.") 1747 protected List<Timing> schedule; 1748 1749 /** 1750 * The amount of the nutritional supplement to be given. 1751 */ 1752 @Child(name = "quantity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1753 @Description(shortDefinition = "Amount of the nutritional supplement", formalDefinition = "The amount of the nutritional supplement to be given.") 1754 protected Quantity quantity; 1755 1756 /** 1757 * Free text or additional instructions or information pertaining to the oral 1758 * supplement. 1759 */ 1760 @Child(name = "instruction", type = { 1761 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1762 @Description(shortDefinition = "Instructions or additional information about the oral supplement", formalDefinition = "Free text or additional instructions or information pertaining to the oral supplement.") 1763 protected StringType instruction; 1764 1765 private static final long serialVersionUID = -37646618L; 1766 1767 /** 1768 * Constructor 1769 */ 1770 public NutritionOrderSupplementComponent() { 1771 super(); 1772 } 1773 1774 /** 1775 * @return {@link #type} (The kind of nutritional supplement product required 1776 * such as a high protein or pediatric clear liquid supplement.) 1777 */ 1778 public CodeableConcept getType() { 1779 if (this.type == null) 1780 if (Configuration.errorOnAutoCreate()) 1781 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.type"); 1782 else if (Configuration.doAutoCreate()) 1783 this.type = new CodeableConcept(); // cc 1784 return this.type; 1785 } 1786 1787 public boolean hasType() { 1788 return this.type != null && !this.type.isEmpty(); 1789 } 1790 1791 /** 1792 * @param value {@link #type} (The kind of nutritional supplement product 1793 * required such as a high protein or pediatric clear liquid 1794 * supplement.) 1795 */ 1796 public NutritionOrderSupplementComponent setType(CodeableConcept value) { 1797 this.type = value; 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #productName} (The product or brand name of the nutritional 1803 * supplement such as "Acme Protein Shake".). This is the underlying 1804 * object with id, value and extensions. The accessor "getProductName" 1805 * gives direct access to the value 1806 */ 1807 public StringType getProductNameElement() { 1808 if (this.productName == null) 1809 if (Configuration.errorOnAutoCreate()) 1810 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.productName"); 1811 else if (Configuration.doAutoCreate()) 1812 this.productName = new StringType(); // bb 1813 return this.productName; 1814 } 1815 1816 public boolean hasProductNameElement() { 1817 return this.productName != null && !this.productName.isEmpty(); 1818 } 1819 1820 public boolean hasProductName() { 1821 return this.productName != null && !this.productName.isEmpty(); 1822 } 1823 1824 /** 1825 * @param value {@link #productName} (The product or brand name of the 1826 * nutritional supplement such as "Acme Protein Shake".). This is 1827 * the underlying object with id, value and extensions. The 1828 * accessor "getProductName" gives direct access to the value 1829 */ 1830 public NutritionOrderSupplementComponent setProductNameElement(StringType value) { 1831 this.productName = value; 1832 return this; 1833 } 1834 1835 /** 1836 * @return The product or brand name of the nutritional supplement such as "Acme 1837 * Protein Shake". 1838 */ 1839 public String getProductName() { 1840 return this.productName == null ? null : this.productName.getValue(); 1841 } 1842 1843 /** 1844 * @param value The product or brand name of the nutritional supplement such as 1845 * "Acme Protein Shake". 1846 */ 1847 public NutritionOrderSupplementComponent setProductName(String value) { 1848 if (Utilities.noString(value)) 1849 this.productName = null; 1850 else { 1851 if (this.productName == null) 1852 this.productName = new StringType(); 1853 this.productName.setValue(value); 1854 } 1855 return this; 1856 } 1857 1858 /** 1859 * @return {@link #schedule} (The time period and frequency at which the 1860 * supplement(s) should be given. The supplement should be given for the 1861 * combination of all schedules if more than one schedule is present.) 1862 */ 1863 public List<Timing> getSchedule() { 1864 if (this.schedule == null) 1865 this.schedule = new ArrayList<Timing>(); 1866 return this.schedule; 1867 } 1868 1869 /** 1870 * @return Returns a reference to <code>this</code> for easy method chaining 1871 */ 1872 public NutritionOrderSupplementComponent setSchedule(List<Timing> theSchedule) { 1873 this.schedule = theSchedule; 1874 return this; 1875 } 1876 1877 public boolean hasSchedule() { 1878 if (this.schedule == null) 1879 return false; 1880 for (Timing item : this.schedule) 1881 if (!item.isEmpty()) 1882 return true; 1883 return false; 1884 } 1885 1886 public Timing addSchedule() { // 3 1887 Timing t = new Timing(); 1888 if (this.schedule == null) 1889 this.schedule = new ArrayList<Timing>(); 1890 this.schedule.add(t); 1891 return t; 1892 } 1893 1894 public NutritionOrderSupplementComponent addSchedule(Timing t) { // 3 1895 if (t == null) 1896 return this; 1897 if (this.schedule == null) 1898 this.schedule = new ArrayList<Timing>(); 1899 this.schedule.add(t); 1900 return this; 1901 } 1902 1903 /** 1904 * @return The first repetition of repeating field {@link #schedule}, creating 1905 * it if it does not already exist 1906 */ 1907 public Timing getScheduleFirstRep() { 1908 if (getSchedule().isEmpty()) { 1909 addSchedule(); 1910 } 1911 return getSchedule().get(0); 1912 } 1913 1914 /** 1915 * @return {@link #quantity} (The amount of the nutritional supplement to be 1916 * given.) 1917 */ 1918 public Quantity getQuantity() { 1919 if (this.quantity == null) 1920 if (Configuration.errorOnAutoCreate()) 1921 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.quantity"); 1922 else if (Configuration.doAutoCreate()) 1923 this.quantity = new Quantity(); // cc 1924 return this.quantity; 1925 } 1926 1927 public boolean hasQuantity() { 1928 return this.quantity != null && !this.quantity.isEmpty(); 1929 } 1930 1931 /** 1932 * @param value {@link #quantity} (The amount of the nutritional supplement to 1933 * be given.) 1934 */ 1935 public NutritionOrderSupplementComponent setQuantity(Quantity value) { 1936 this.quantity = value; 1937 return this; 1938 } 1939 1940 /** 1941 * @return {@link #instruction} (Free text or additional instructions or 1942 * information pertaining to the oral supplement.). This is the 1943 * underlying object with id, value and extensions. The accessor 1944 * "getInstruction" gives direct access to the value 1945 */ 1946 public StringType getInstructionElement() { 1947 if (this.instruction == null) 1948 if (Configuration.errorOnAutoCreate()) 1949 throw new Error("Attempt to auto-create NutritionOrderSupplementComponent.instruction"); 1950 else if (Configuration.doAutoCreate()) 1951 this.instruction = new StringType(); // bb 1952 return this.instruction; 1953 } 1954 1955 public boolean hasInstructionElement() { 1956 return this.instruction != null && !this.instruction.isEmpty(); 1957 } 1958 1959 public boolean hasInstruction() { 1960 return this.instruction != null && !this.instruction.isEmpty(); 1961 } 1962 1963 /** 1964 * @param value {@link #instruction} (Free text or additional instructions or 1965 * information pertaining to the oral supplement.). This is the 1966 * underlying object with id, value and extensions. The accessor 1967 * "getInstruction" gives direct access to the value 1968 */ 1969 public NutritionOrderSupplementComponent setInstructionElement(StringType value) { 1970 this.instruction = value; 1971 return this; 1972 } 1973 1974 /** 1975 * @return Free text or additional instructions or information pertaining to the 1976 * oral supplement. 1977 */ 1978 public String getInstruction() { 1979 return this.instruction == null ? null : this.instruction.getValue(); 1980 } 1981 1982 /** 1983 * @param value Free text or additional instructions or information pertaining 1984 * to the oral supplement. 1985 */ 1986 public NutritionOrderSupplementComponent setInstruction(String value) { 1987 if (Utilities.noString(value)) 1988 this.instruction = null; 1989 else { 1990 if (this.instruction == null) 1991 this.instruction = new StringType(); 1992 this.instruction.setValue(value); 1993 } 1994 return this; 1995 } 1996 1997 protected void listChildren(List<Property> children) { 1998 super.listChildren(children); 1999 children.add(new Property("type", "CodeableConcept", 2000 "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 2001 0, 1, type)); 2002 children.add(new Property("productName", "string", 2003 "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1, 2004 productName)); 2005 children.add(new Property("schedule", "Timing", 2006 "The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.", 2007 0, java.lang.Integer.MAX_VALUE, schedule)); 2008 children.add(new Property("quantity", "SimpleQuantity", "The amount of the nutritional supplement to be given.", 2009 0, 1, quantity)); 2010 children.add(new Property("instruction", "string", 2011 "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1, instruction)); 2012 } 2013 2014 @Override 2015 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2016 switch (_hash) { 2017 case 3575610: 2018 /* type */ return new Property("type", "CodeableConcept", 2019 "The kind of nutritional supplement product required such as a high protein or pediatric clear liquid supplement.", 2020 0, 1, type); 2021 case -1491817446: 2022 /* productName */ return new Property("productName", "string", 2023 "The product or brand name of the nutritional supplement such as \"Acme Protein Shake\".", 0, 1, 2024 productName); 2025 case -697920873: 2026 /* schedule */ return new Property("schedule", "Timing", 2027 "The time period and frequency at which the supplement(s) should be given. The supplement should be given for the combination of all schedules if more than one schedule is present.", 2028 0, java.lang.Integer.MAX_VALUE, schedule); 2029 case -1285004149: 2030 /* quantity */ return new Property("quantity", "SimpleQuantity", 2031 "The amount of the nutritional supplement to be given.", 0, 1, quantity); 2032 case 301526158: 2033 /* instruction */ return new Property("instruction", "string", 2034 "Free text or additional instructions or information pertaining to the oral supplement.", 0, 1, 2035 instruction); 2036 default: 2037 return super.getNamedProperty(_hash, _name, _checkValid); 2038 } 2039 2040 } 2041 2042 @Override 2043 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2044 switch (hash) { 2045 case 3575610: 2046 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2047 case -1491817446: 2048 /* productName */ return this.productName == null ? new Base[0] : new Base[] { this.productName }; // StringType 2049 case -697920873: 2050 /* schedule */ return this.schedule == null ? new Base[0] 2051 : this.schedule.toArray(new Base[this.schedule.size()]); // Timing 2052 case -1285004149: 2053 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 2054 case 301526158: 2055 /* instruction */ return this.instruction == null ? new Base[0] : new Base[] { this.instruction }; // StringType 2056 default: 2057 return super.getProperty(hash, name, checkValid); 2058 } 2059 2060 } 2061 2062 @Override 2063 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2064 switch (hash) { 2065 case 3575610: // type 2066 this.type = castToCodeableConcept(value); // CodeableConcept 2067 return value; 2068 case -1491817446: // productName 2069 this.productName = castToString(value); // StringType 2070 return value; 2071 case -697920873: // schedule 2072 this.getSchedule().add(castToTiming(value)); // Timing 2073 return value; 2074 case -1285004149: // quantity 2075 this.quantity = castToQuantity(value); // Quantity 2076 return value; 2077 case 301526158: // instruction 2078 this.instruction = castToString(value); // StringType 2079 return value; 2080 default: 2081 return super.setProperty(hash, name, value); 2082 } 2083 2084 } 2085 2086 @Override 2087 public Base setProperty(String name, Base value) throws FHIRException { 2088 if (name.equals("type")) { 2089 this.type = castToCodeableConcept(value); // CodeableConcept 2090 } else if (name.equals("productName")) { 2091 this.productName = castToString(value); // StringType 2092 } else if (name.equals("schedule")) { 2093 this.getSchedule().add(castToTiming(value)); 2094 } else if (name.equals("quantity")) { 2095 this.quantity = castToQuantity(value); // Quantity 2096 } else if (name.equals("instruction")) { 2097 this.instruction = castToString(value); // StringType 2098 } else 2099 return super.setProperty(name, value); 2100 return value; 2101 } 2102 2103 @Override 2104 public void removeChild(String name, Base value) throws FHIRException { 2105 if (name.equals("type")) { 2106 this.type = null; 2107 } else if (name.equals("productName")) { 2108 this.productName = null; 2109 } else if (name.equals("schedule")) { 2110 this.getSchedule().remove(castToTiming(value)); 2111 } else if (name.equals("quantity")) { 2112 this.quantity = null; 2113 } else if (name.equals("instruction")) { 2114 this.instruction = null; 2115 } else 2116 super.removeChild(name, value); 2117 2118 } 2119 2120 @Override 2121 public Base makeProperty(int hash, String name) throws FHIRException { 2122 switch (hash) { 2123 case 3575610: 2124 return getType(); 2125 case -1491817446: 2126 return getProductNameElement(); 2127 case -697920873: 2128 return addSchedule(); 2129 case -1285004149: 2130 return getQuantity(); 2131 case 301526158: 2132 return getInstructionElement(); 2133 default: 2134 return super.makeProperty(hash, name); 2135 } 2136 2137 } 2138 2139 @Override 2140 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2141 switch (hash) { 2142 case 3575610: 2143 /* type */ return new String[] { "CodeableConcept" }; 2144 case -1491817446: 2145 /* productName */ return new String[] { "string" }; 2146 case -697920873: 2147 /* schedule */ return new String[] { "Timing" }; 2148 case -1285004149: 2149 /* quantity */ return new String[] { "SimpleQuantity" }; 2150 case 301526158: 2151 /* instruction */ return new String[] { "string" }; 2152 default: 2153 return super.getTypesForProperty(hash, name); 2154 } 2155 2156 } 2157 2158 @Override 2159 public Base addChild(String name) throws FHIRException { 2160 if (name.equals("type")) { 2161 this.type = new CodeableConcept(); 2162 return this.type; 2163 } else if (name.equals("productName")) { 2164 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.productName"); 2165 } else if (name.equals("schedule")) { 2166 return addSchedule(); 2167 } else if (name.equals("quantity")) { 2168 this.quantity = new Quantity(); 2169 return this.quantity; 2170 } else if (name.equals("instruction")) { 2171 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instruction"); 2172 } else 2173 return super.addChild(name); 2174 } 2175 2176 public NutritionOrderSupplementComponent copy() { 2177 NutritionOrderSupplementComponent dst = new NutritionOrderSupplementComponent(); 2178 copyValues(dst); 2179 return dst; 2180 } 2181 2182 public void copyValues(NutritionOrderSupplementComponent dst) { 2183 super.copyValues(dst); 2184 dst.type = type == null ? null : type.copy(); 2185 dst.productName = productName == null ? null : productName.copy(); 2186 if (schedule != null) { 2187 dst.schedule = new ArrayList<Timing>(); 2188 for (Timing i : schedule) 2189 dst.schedule.add(i.copy()); 2190 } 2191 ; 2192 dst.quantity = quantity == null ? null : quantity.copy(); 2193 dst.instruction = instruction == null ? null : instruction.copy(); 2194 } 2195 2196 @Override 2197 public boolean equalsDeep(Base other_) { 2198 if (!super.equalsDeep(other_)) 2199 return false; 2200 if (!(other_ instanceof NutritionOrderSupplementComponent)) 2201 return false; 2202 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other_; 2203 return compareDeep(type, o.type, true) && compareDeep(productName, o.productName, true) 2204 && compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) 2205 && compareDeep(instruction, o.instruction, true); 2206 } 2207 2208 @Override 2209 public boolean equalsShallow(Base other_) { 2210 if (!super.equalsShallow(other_)) 2211 return false; 2212 if (!(other_ instanceof NutritionOrderSupplementComponent)) 2213 return false; 2214 NutritionOrderSupplementComponent o = (NutritionOrderSupplementComponent) other_; 2215 return compareValues(productName, o.productName, true) && compareValues(instruction, o.instruction, true); 2216 } 2217 2218 public boolean isEmpty() { 2219 return super.isEmpty() 2220 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, productName, schedule, quantity, instruction); 2221 } 2222 2223 public String fhirType() { 2224 return "NutritionOrder.supplement"; 2225 2226 } 2227 2228 } 2229 2230 @Block() 2231 public static class NutritionOrderEnteralFormulaComponent extends BackboneElement implements IBaseBackboneElement { 2232 /** 2233 * The type of enteral or infant formula such as an adult standard formula with 2234 * fiber or a soy-based infant formula. 2235 */ 2236 @Child(name = "baseFormulaType", type = { 2237 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2238 @Description(shortDefinition = "Type of enteral or infant formula", formalDefinition = "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.") 2239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/entformula-type") 2240 protected CodeableConcept baseFormulaType; 2241 2242 /** 2243 * The product or brand name of the enteral or infant formula product such as 2244 * "ACME Adult Standard Formula". 2245 */ 2246 @Child(name = "baseFormulaProductName", type = { 2247 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2248 @Description(shortDefinition = "Product or brand name of the enteral or infant formula", formalDefinition = "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".") 2249 protected StringType baseFormulaProductName; 2250 2251 /** 2252 * Indicates the type of modular component such as protein, carbohydrate, fat or 2253 * fiber to be provided in addition to or mixed with the base formula. 2254 */ 2255 @Child(name = "additiveType", type = { 2256 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2257 @Description(shortDefinition = "Type of modular component to add to the feeding", formalDefinition = "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.") 2258 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/entformula-additive") 2259 protected CodeableConcept additiveType; 2260 2261 /** 2262 * The product or brand name of the type of modular component to be added to the 2263 * formula. 2264 */ 2265 @Child(name = "additiveProductName", type = { 2266 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2267 @Description(shortDefinition = "Product or brand name of the modular additive", formalDefinition = "The product or brand name of the type of modular component to be added to the formula.") 2268 protected StringType additiveProductName; 2269 2270 /** 2271 * The amount of energy (calories) that the formula should provide per specified 2272 * volume, typically per mL or fluid oz. For example, an infant may require a 2273 * formula that provides 24 calories per fluid ounce or an adult may require an 2274 * enteral formula that provides 1.5 calorie/mL. 2275 */ 2276 @Child(name = "caloricDensity", type = { 2277 Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2278 @Description(shortDefinition = "Amount of energy per specified volume that is required", formalDefinition = "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.") 2279 protected Quantity caloricDensity; 2280 2281 /** 2282 * The route or physiological path of administration into the patient's 2283 * gastrointestinal tract for purposes of providing the formula feeding, e.g. 2284 * nasogastric tube. 2285 */ 2286 @Child(name = "routeofAdministration", type = { 2287 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2288 @Description(shortDefinition = "How the formula should enter the patient's gastrointestinal tract", formalDefinition = "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.") 2289 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/enteral-route") 2290 protected CodeableConcept routeofAdministration; 2291 2292 /** 2293 * Formula administration instructions as structured data. This repeating 2294 * structure allows for changing the administration rate or volume over time for 2295 * both bolus and continuous feeding. An example of this would be an instruction 2296 * to increase the rate of continuous feeding every 2 hours. 2297 */ 2298 @Child(name = "administration", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2299 @Description(shortDefinition = "Formula feeding instruction as structured data", formalDefinition = "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.") 2300 protected List<NutritionOrderEnteralFormulaAdministrationComponent> administration; 2301 2302 /** 2303 * The maximum total quantity of formula that may be administered to a subject 2304 * over the period of time, e.g. 1440 mL over 24 hours. 2305 */ 2306 @Child(name = "maxVolumeToDeliver", type = { 2307 Quantity.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2308 @Description(shortDefinition = "Upper limit on formula volume per unit of time", formalDefinition = "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.") 2309 protected Quantity maxVolumeToDeliver; 2310 2311 /** 2312 * Free text formula administration, feeding instructions or additional 2313 * instructions or information. 2314 */ 2315 @Child(name = "administrationInstruction", type = { 2316 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2317 @Description(shortDefinition = "Formula feeding instructions expressed as text", formalDefinition = "Free text formula administration, feeding instructions or additional instructions or information.") 2318 protected StringType administrationInstruction; 2319 2320 private static final long serialVersionUID = -124511395L; 2321 2322 /** 2323 * Constructor 2324 */ 2325 public NutritionOrderEnteralFormulaComponent() { 2326 super(); 2327 } 2328 2329 /** 2330 * @return {@link #baseFormulaType} (The type of enteral or infant formula such 2331 * as an adult standard formula with fiber or a soy-based infant 2332 * formula.) 2333 */ 2334 public CodeableConcept getBaseFormulaType() { 2335 if (this.baseFormulaType == null) 2336 if (Configuration.errorOnAutoCreate()) 2337 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaType"); 2338 else if (Configuration.doAutoCreate()) 2339 this.baseFormulaType = new CodeableConcept(); // cc 2340 return this.baseFormulaType; 2341 } 2342 2343 public boolean hasBaseFormulaType() { 2344 return this.baseFormulaType != null && !this.baseFormulaType.isEmpty(); 2345 } 2346 2347 /** 2348 * @param value {@link #baseFormulaType} (The type of enteral or infant formula 2349 * such as an adult standard formula with fiber or a soy-based 2350 * infant formula.) 2351 */ 2352 public NutritionOrderEnteralFormulaComponent setBaseFormulaType(CodeableConcept value) { 2353 this.baseFormulaType = value; 2354 return this; 2355 } 2356 2357 /** 2358 * @return {@link #baseFormulaProductName} (The product or brand name of the 2359 * enteral or infant formula product such as "ACME Adult Standard 2360 * Formula".). This is the underlying object with id, value and 2361 * extensions. The accessor "getBaseFormulaProductName" gives direct 2362 * access to the value 2363 */ 2364 public StringType getBaseFormulaProductNameElement() { 2365 if (this.baseFormulaProductName == null) 2366 if (Configuration.errorOnAutoCreate()) 2367 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.baseFormulaProductName"); 2368 else if (Configuration.doAutoCreate()) 2369 this.baseFormulaProductName = new StringType(); // bb 2370 return this.baseFormulaProductName; 2371 } 2372 2373 public boolean hasBaseFormulaProductNameElement() { 2374 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 2375 } 2376 2377 public boolean hasBaseFormulaProductName() { 2378 return this.baseFormulaProductName != null && !this.baseFormulaProductName.isEmpty(); 2379 } 2380 2381 /** 2382 * @param value {@link #baseFormulaProductName} (The product or brand name of 2383 * the enteral or infant formula product such as "ACME Adult 2384 * Standard Formula".). This is the underlying object with id, 2385 * value and extensions. The accessor "getBaseFormulaProductName" 2386 * gives direct access to the value 2387 */ 2388 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductNameElement(StringType value) { 2389 this.baseFormulaProductName = value; 2390 return this; 2391 } 2392 2393 /** 2394 * @return The product or brand name of the enteral or infant formula product 2395 * such as "ACME Adult Standard Formula". 2396 */ 2397 public String getBaseFormulaProductName() { 2398 return this.baseFormulaProductName == null ? null : this.baseFormulaProductName.getValue(); 2399 } 2400 2401 /** 2402 * @param value The product or brand name of the enteral or infant formula 2403 * product such as "ACME Adult Standard Formula". 2404 */ 2405 public NutritionOrderEnteralFormulaComponent setBaseFormulaProductName(String value) { 2406 if (Utilities.noString(value)) 2407 this.baseFormulaProductName = null; 2408 else { 2409 if (this.baseFormulaProductName == null) 2410 this.baseFormulaProductName = new StringType(); 2411 this.baseFormulaProductName.setValue(value); 2412 } 2413 return this; 2414 } 2415 2416 /** 2417 * @return {@link #additiveType} (Indicates the type of modular component such 2418 * as protein, carbohydrate, fat or fiber to be provided in addition to 2419 * or mixed with the base formula.) 2420 */ 2421 public CodeableConcept getAdditiveType() { 2422 if (this.additiveType == null) 2423 if (Configuration.errorOnAutoCreate()) 2424 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveType"); 2425 else if (Configuration.doAutoCreate()) 2426 this.additiveType = new CodeableConcept(); // cc 2427 return this.additiveType; 2428 } 2429 2430 public boolean hasAdditiveType() { 2431 return this.additiveType != null && !this.additiveType.isEmpty(); 2432 } 2433 2434 /** 2435 * @param value {@link #additiveType} (Indicates the type of modular component 2436 * such as protein, carbohydrate, fat or fiber to be provided in 2437 * addition to or mixed with the base formula.) 2438 */ 2439 public NutritionOrderEnteralFormulaComponent setAdditiveType(CodeableConcept value) { 2440 this.additiveType = value; 2441 return this; 2442 } 2443 2444 /** 2445 * @return {@link #additiveProductName} (The product or brand name of the type 2446 * of modular component to be added to the formula.). This is the 2447 * underlying object with id, value and extensions. The accessor 2448 * "getAdditiveProductName" gives direct access to the value 2449 */ 2450 public StringType getAdditiveProductNameElement() { 2451 if (this.additiveProductName == null) 2452 if (Configuration.errorOnAutoCreate()) 2453 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.additiveProductName"); 2454 else if (Configuration.doAutoCreate()) 2455 this.additiveProductName = new StringType(); // bb 2456 return this.additiveProductName; 2457 } 2458 2459 public boolean hasAdditiveProductNameElement() { 2460 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 2461 } 2462 2463 public boolean hasAdditiveProductName() { 2464 return this.additiveProductName != null && !this.additiveProductName.isEmpty(); 2465 } 2466 2467 /** 2468 * @param value {@link #additiveProductName} (The product or brand name of the 2469 * type of modular component to be added to the formula.). This is 2470 * the underlying object with id, value and extensions. The 2471 * accessor "getAdditiveProductName" gives direct access to the 2472 * value 2473 */ 2474 public NutritionOrderEnteralFormulaComponent setAdditiveProductNameElement(StringType value) { 2475 this.additiveProductName = value; 2476 return this; 2477 } 2478 2479 /** 2480 * @return The product or brand name of the type of modular component to be 2481 * added to the formula. 2482 */ 2483 public String getAdditiveProductName() { 2484 return this.additiveProductName == null ? null : this.additiveProductName.getValue(); 2485 } 2486 2487 /** 2488 * @param value The product or brand name of the type of modular component to be 2489 * added to the formula. 2490 */ 2491 public NutritionOrderEnteralFormulaComponent setAdditiveProductName(String value) { 2492 if (Utilities.noString(value)) 2493 this.additiveProductName = null; 2494 else { 2495 if (this.additiveProductName == null) 2496 this.additiveProductName = new StringType(); 2497 this.additiveProductName.setValue(value); 2498 } 2499 return this; 2500 } 2501 2502 /** 2503 * @return {@link #caloricDensity} (The amount of energy (calories) that the 2504 * formula should provide per specified volume, typically per mL or 2505 * fluid oz. For example, an infant may require a formula that provides 2506 * 24 calories per fluid ounce or an adult may require an enteral 2507 * formula that provides 1.5 calorie/mL.) 2508 */ 2509 public Quantity getCaloricDensity() { 2510 if (this.caloricDensity == null) 2511 if (Configuration.errorOnAutoCreate()) 2512 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.caloricDensity"); 2513 else if (Configuration.doAutoCreate()) 2514 this.caloricDensity = new Quantity(); // cc 2515 return this.caloricDensity; 2516 } 2517 2518 public boolean hasCaloricDensity() { 2519 return this.caloricDensity != null && !this.caloricDensity.isEmpty(); 2520 } 2521 2522 /** 2523 * @param value {@link #caloricDensity} (The amount of energy (calories) that 2524 * the formula should provide per specified volume, typically per 2525 * mL or fluid oz. For example, an infant may require a formula 2526 * that provides 24 calories per fluid ounce or an adult may 2527 * require an enteral formula that provides 1.5 calorie/mL.) 2528 */ 2529 public NutritionOrderEnteralFormulaComponent setCaloricDensity(Quantity value) { 2530 this.caloricDensity = value; 2531 return this; 2532 } 2533 2534 /** 2535 * @return {@link #routeofAdministration} (The route or physiological path of 2536 * administration into the patient's gastrointestinal tract for purposes 2537 * of providing the formula feeding, e.g. nasogastric tube.) 2538 */ 2539 public CodeableConcept getRouteofAdministration() { 2540 if (this.routeofAdministration == null) 2541 if (Configuration.errorOnAutoCreate()) 2542 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.routeofAdministration"); 2543 else if (Configuration.doAutoCreate()) 2544 this.routeofAdministration = new CodeableConcept(); // cc 2545 return this.routeofAdministration; 2546 } 2547 2548 public boolean hasRouteofAdministration() { 2549 return this.routeofAdministration != null && !this.routeofAdministration.isEmpty(); 2550 } 2551 2552 /** 2553 * @param value {@link #routeofAdministration} (The route or physiological path 2554 * of administration into the patient's gastrointestinal tract for 2555 * purposes of providing the formula feeding, e.g. nasogastric 2556 * tube.) 2557 */ 2558 public NutritionOrderEnteralFormulaComponent setRouteofAdministration(CodeableConcept value) { 2559 this.routeofAdministration = value; 2560 return this; 2561 } 2562 2563 /** 2564 * @return {@link #administration} (Formula administration instructions as 2565 * structured data. This repeating structure allows for changing the 2566 * administration rate or volume over time for both bolus and continuous 2567 * feeding. An example of this would be an instruction to increase the 2568 * rate of continuous feeding every 2 hours.) 2569 */ 2570 public List<NutritionOrderEnteralFormulaAdministrationComponent> getAdministration() { 2571 if (this.administration == null) 2572 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2573 return this.administration; 2574 } 2575 2576 /** 2577 * @return Returns a reference to <code>this</code> for easy method chaining 2578 */ 2579 public NutritionOrderEnteralFormulaComponent setAdministration( 2580 List<NutritionOrderEnteralFormulaAdministrationComponent> theAdministration) { 2581 this.administration = theAdministration; 2582 return this; 2583 } 2584 2585 public boolean hasAdministration() { 2586 if (this.administration == null) 2587 return false; 2588 for (NutritionOrderEnteralFormulaAdministrationComponent item : this.administration) 2589 if (!item.isEmpty()) 2590 return true; 2591 return false; 2592 } 2593 2594 public NutritionOrderEnteralFormulaAdministrationComponent addAdministration() { // 3 2595 NutritionOrderEnteralFormulaAdministrationComponent t = new NutritionOrderEnteralFormulaAdministrationComponent(); 2596 if (this.administration == null) 2597 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2598 this.administration.add(t); 2599 return t; 2600 } 2601 2602 public NutritionOrderEnteralFormulaComponent addAdministration( 2603 NutritionOrderEnteralFormulaAdministrationComponent t) { // 3 2604 if (t == null) 2605 return this; 2606 if (this.administration == null) 2607 this.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 2608 this.administration.add(t); 2609 return this; 2610 } 2611 2612 /** 2613 * @return The first repetition of repeating field {@link #administration}, 2614 * creating it if it does not already exist 2615 */ 2616 public NutritionOrderEnteralFormulaAdministrationComponent getAdministrationFirstRep() { 2617 if (getAdministration().isEmpty()) { 2618 addAdministration(); 2619 } 2620 return getAdministration().get(0); 2621 } 2622 2623 /** 2624 * @return {@link #maxVolumeToDeliver} (The maximum total quantity of formula 2625 * that may be administered to a subject over the period of time, e.g. 2626 * 1440 mL over 24 hours.) 2627 */ 2628 public Quantity getMaxVolumeToDeliver() { 2629 if (this.maxVolumeToDeliver == null) 2630 if (Configuration.errorOnAutoCreate()) 2631 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.maxVolumeToDeliver"); 2632 else if (Configuration.doAutoCreate()) 2633 this.maxVolumeToDeliver = new Quantity(); // cc 2634 return this.maxVolumeToDeliver; 2635 } 2636 2637 public boolean hasMaxVolumeToDeliver() { 2638 return this.maxVolumeToDeliver != null && !this.maxVolumeToDeliver.isEmpty(); 2639 } 2640 2641 /** 2642 * @param value {@link #maxVolumeToDeliver} (The maximum total quantity of 2643 * formula that may be administered to a subject over the period of 2644 * time, e.g. 1440 mL over 24 hours.) 2645 */ 2646 public NutritionOrderEnteralFormulaComponent setMaxVolumeToDeliver(Quantity value) { 2647 this.maxVolumeToDeliver = value; 2648 return this; 2649 } 2650 2651 /** 2652 * @return {@link #administrationInstruction} (Free text formula administration, 2653 * feeding instructions or additional instructions or information.). 2654 * This is the underlying object with id, value and extensions. The 2655 * accessor "getAdministrationInstruction" gives direct access to the 2656 * value 2657 */ 2658 public StringType getAdministrationInstructionElement() { 2659 if (this.administrationInstruction == null) 2660 if (Configuration.errorOnAutoCreate()) 2661 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaComponent.administrationInstruction"); 2662 else if (Configuration.doAutoCreate()) 2663 this.administrationInstruction = new StringType(); // bb 2664 return this.administrationInstruction; 2665 } 2666 2667 public boolean hasAdministrationInstructionElement() { 2668 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 2669 } 2670 2671 public boolean hasAdministrationInstruction() { 2672 return this.administrationInstruction != null && !this.administrationInstruction.isEmpty(); 2673 } 2674 2675 /** 2676 * @param value {@link #administrationInstruction} (Free text formula 2677 * administration, feeding instructions or additional instructions 2678 * or information.). This is the underlying object with id, value 2679 * and extensions. The accessor "getAdministrationInstruction" 2680 * gives direct access to the value 2681 */ 2682 public NutritionOrderEnteralFormulaComponent setAdministrationInstructionElement(StringType value) { 2683 this.administrationInstruction = value; 2684 return this; 2685 } 2686 2687 /** 2688 * @return Free text formula administration, feeding instructions or additional 2689 * instructions or information. 2690 */ 2691 public String getAdministrationInstruction() { 2692 return this.administrationInstruction == null ? null : this.administrationInstruction.getValue(); 2693 } 2694 2695 /** 2696 * @param value Free text formula administration, feeding instructions or 2697 * additional instructions or information. 2698 */ 2699 public NutritionOrderEnteralFormulaComponent setAdministrationInstruction(String value) { 2700 if (Utilities.noString(value)) 2701 this.administrationInstruction = null; 2702 else { 2703 if (this.administrationInstruction == null) 2704 this.administrationInstruction = new StringType(); 2705 this.administrationInstruction.setValue(value); 2706 } 2707 return this; 2708 } 2709 2710 protected void listChildren(List<Property> children) { 2711 super.listChildren(children); 2712 children.add(new Property("baseFormulaType", "CodeableConcept", 2713 "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 2714 0, 1, baseFormulaType)); 2715 children.add(new Property("baseFormulaProductName", "string", 2716 "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 2717 0, 1, baseFormulaProductName)); 2718 children.add(new Property("additiveType", "CodeableConcept", 2719 "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 2720 0, 1, additiveType)); 2721 children.add(new Property("additiveProductName", "string", 2722 "The product or brand name of the type of modular component to be added to the formula.", 0, 1, 2723 additiveProductName)); 2724 children.add(new Property("caloricDensity", "SimpleQuantity", 2725 "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.", 2726 0, 1, caloricDensity)); 2727 children.add(new Property("routeofAdministration", "CodeableConcept", 2728 "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 2729 0, 1, routeofAdministration)); 2730 children.add(new Property("administration", "", 2731 "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 2732 0, java.lang.Integer.MAX_VALUE, administration)); 2733 children.add(new Property("maxVolumeToDeliver", "SimpleQuantity", 2734 "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 2735 0, 1, maxVolumeToDeliver)); 2736 children.add(new Property("administrationInstruction", "string", 2737 "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1, 2738 administrationInstruction)); 2739 } 2740 2741 @Override 2742 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2743 switch (_hash) { 2744 case -138930641: 2745 /* baseFormulaType */ return new Property("baseFormulaType", "CodeableConcept", 2746 "The type of enteral or infant formula such as an adult standard formula with fiber or a soy-based infant formula.", 2747 0, 1, baseFormulaType); 2748 case -1267705979: 2749 /* baseFormulaProductName */ return new Property("baseFormulaProductName", "string", 2750 "The product or brand name of the enteral or infant formula product such as \"ACME Adult Standard Formula\".", 2751 0, 1, baseFormulaProductName); 2752 case -470746842: 2753 /* additiveType */ return new Property("additiveType", "CodeableConcept", 2754 "Indicates the type of modular component such as protein, carbohydrate, fat or fiber to be provided in addition to or mixed with the base formula.", 2755 0, 1, additiveType); 2756 case 488079534: 2757 /* additiveProductName */ return new Property("additiveProductName", "string", 2758 "The product or brand name of the type of modular component to be added to the formula.", 0, 1, 2759 additiveProductName); 2760 case 186983261: 2761 /* caloricDensity */ return new Property("caloricDensity", "SimpleQuantity", 2762 "The amount of energy (calories) that the formula should provide per specified volume, typically per mL or fluid oz. For example, an infant may require a formula that provides 24 calories per fluid ounce or an adult may require an enteral formula that provides 1.5 calorie/mL.", 2763 0, 1, caloricDensity); 2764 case -1710107042: 2765 /* routeofAdministration */ return new Property("routeofAdministration", "CodeableConcept", 2766 "The route or physiological path of administration into the patient's gastrointestinal tract for purposes of providing the formula feeding, e.g. nasogastric tube.", 2767 0, 1, routeofAdministration); 2768 case 1255702622: 2769 /* administration */ return new Property("administration", "", 2770 "Formula administration instructions as structured data. This repeating structure allows for changing the administration rate or volume over time for both bolus and continuous feeding. An example of this would be an instruction to increase the rate of continuous feeding every 2 hours.", 2771 0, java.lang.Integer.MAX_VALUE, administration); 2772 case 2017924652: 2773 /* maxVolumeToDeliver */ return new Property("maxVolumeToDeliver", "SimpleQuantity", 2774 "The maximum total quantity of formula that may be administered to a subject over the period of time, e.g. 1440 mL over 24 hours.", 2775 0, 1, maxVolumeToDeliver); 2776 case 427085136: 2777 /* administrationInstruction */ return new Property("administrationInstruction", "string", 2778 "Free text formula administration, feeding instructions or additional instructions or information.", 0, 1, 2779 administrationInstruction); 2780 default: 2781 return super.getNamedProperty(_hash, _name, _checkValid); 2782 } 2783 2784 } 2785 2786 @Override 2787 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2788 switch (hash) { 2789 case -138930641: 2790 /* baseFormulaType */ return this.baseFormulaType == null ? new Base[0] : new Base[] { this.baseFormulaType }; // CodeableConcept 2791 case -1267705979: 2792 /* baseFormulaProductName */ return this.baseFormulaProductName == null ? new Base[0] 2793 : new Base[] { this.baseFormulaProductName }; // StringType 2794 case -470746842: 2795 /* additiveType */ return this.additiveType == null ? new Base[0] : new Base[] { this.additiveType }; // CodeableConcept 2796 case 488079534: 2797 /* additiveProductName */ return this.additiveProductName == null ? new Base[0] 2798 : new Base[] { this.additiveProductName }; // StringType 2799 case 186983261: 2800 /* caloricDensity */ return this.caloricDensity == null ? new Base[0] : new Base[] { this.caloricDensity }; // Quantity 2801 case -1710107042: 2802 /* routeofAdministration */ return this.routeofAdministration == null ? new Base[0] 2803 : new Base[] { this.routeofAdministration }; // CodeableConcept 2804 case 1255702622: 2805 /* administration */ return this.administration == null ? new Base[0] 2806 : this.administration.toArray(new Base[this.administration.size()]); // NutritionOrderEnteralFormulaAdministrationComponent 2807 case 2017924652: 2808 /* maxVolumeToDeliver */ return this.maxVolumeToDeliver == null ? new Base[0] 2809 : new Base[] { this.maxVolumeToDeliver }; // Quantity 2810 case 427085136: 2811 /* administrationInstruction */ return this.administrationInstruction == null ? new Base[0] 2812 : new Base[] { this.administrationInstruction }; // StringType 2813 default: 2814 return super.getProperty(hash, name, checkValid); 2815 } 2816 2817 } 2818 2819 @Override 2820 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2821 switch (hash) { 2822 case -138930641: // baseFormulaType 2823 this.baseFormulaType = castToCodeableConcept(value); // CodeableConcept 2824 return value; 2825 case -1267705979: // baseFormulaProductName 2826 this.baseFormulaProductName = castToString(value); // StringType 2827 return value; 2828 case -470746842: // additiveType 2829 this.additiveType = castToCodeableConcept(value); // CodeableConcept 2830 return value; 2831 case 488079534: // additiveProductName 2832 this.additiveProductName = castToString(value); // StringType 2833 return value; 2834 case 186983261: // caloricDensity 2835 this.caloricDensity = castToQuantity(value); // Quantity 2836 return value; 2837 case -1710107042: // routeofAdministration 2838 this.routeofAdministration = castToCodeableConcept(value); // CodeableConcept 2839 return value; 2840 case 1255702622: // administration 2841 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); // NutritionOrderEnteralFormulaAdministrationComponent 2842 return value; 2843 case 2017924652: // maxVolumeToDeliver 2844 this.maxVolumeToDeliver = castToQuantity(value); // Quantity 2845 return value; 2846 case 427085136: // administrationInstruction 2847 this.administrationInstruction = castToString(value); // StringType 2848 return value; 2849 default: 2850 return super.setProperty(hash, name, value); 2851 } 2852 2853 } 2854 2855 @Override 2856 public Base setProperty(String name, Base value) throws FHIRException { 2857 if (name.equals("baseFormulaType")) { 2858 this.baseFormulaType = castToCodeableConcept(value); // CodeableConcept 2859 } else if (name.equals("baseFormulaProductName")) { 2860 this.baseFormulaProductName = castToString(value); // StringType 2861 } else if (name.equals("additiveType")) { 2862 this.additiveType = castToCodeableConcept(value); // CodeableConcept 2863 } else if (name.equals("additiveProductName")) { 2864 this.additiveProductName = castToString(value); // StringType 2865 } else if (name.equals("caloricDensity")) { 2866 this.caloricDensity = castToQuantity(value); // Quantity 2867 } else if (name.equals("routeofAdministration")) { 2868 this.routeofAdministration = castToCodeableConcept(value); // CodeableConcept 2869 } else if (name.equals("administration")) { 2870 this.getAdministration().add((NutritionOrderEnteralFormulaAdministrationComponent) value); 2871 } else if (name.equals("maxVolumeToDeliver")) { 2872 this.maxVolumeToDeliver = castToQuantity(value); // Quantity 2873 } else if (name.equals("administrationInstruction")) { 2874 this.administrationInstruction = castToString(value); // StringType 2875 } else 2876 return super.setProperty(name, value); 2877 return value; 2878 } 2879 2880 @Override 2881 public void removeChild(String name, Base value) throws FHIRException { 2882 if (name.equals("baseFormulaType")) { 2883 this.baseFormulaType = null; 2884 } else if (name.equals("baseFormulaProductName")) { 2885 this.baseFormulaProductName = null; 2886 } else if (name.equals("additiveType")) { 2887 this.additiveType = null; 2888 } else if (name.equals("additiveProductName")) { 2889 this.additiveProductName = null; 2890 } else if (name.equals("caloricDensity")) { 2891 this.caloricDensity = null; 2892 } else if (name.equals("routeofAdministration")) { 2893 this.routeofAdministration = null; 2894 } else if (name.equals("administration")) { 2895 this.getAdministration().remove((NutritionOrderEnteralFormulaAdministrationComponent) value); 2896 } else if (name.equals("maxVolumeToDeliver")) { 2897 this.maxVolumeToDeliver = null; 2898 } else if (name.equals("administrationInstruction")) { 2899 this.administrationInstruction = null; 2900 } else 2901 super.removeChild(name, value); 2902 2903 } 2904 2905 @Override 2906 public Base makeProperty(int hash, String name) throws FHIRException { 2907 switch (hash) { 2908 case -138930641: 2909 return getBaseFormulaType(); 2910 case -1267705979: 2911 return getBaseFormulaProductNameElement(); 2912 case -470746842: 2913 return getAdditiveType(); 2914 case 488079534: 2915 return getAdditiveProductNameElement(); 2916 case 186983261: 2917 return getCaloricDensity(); 2918 case -1710107042: 2919 return getRouteofAdministration(); 2920 case 1255702622: 2921 return addAdministration(); 2922 case 2017924652: 2923 return getMaxVolumeToDeliver(); 2924 case 427085136: 2925 return getAdministrationInstructionElement(); 2926 default: 2927 return super.makeProperty(hash, name); 2928 } 2929 2930 } 2931 2932 @Override 2933 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2934 switch (hash) { 2935 case -138930641: 2936 /* baseFormulaType */ return new String[] { "CodeableConcept" }; 2937 case -1267705979: 2938 /* baseFormulaProductName */ return new String[] { "string" }; 2939 case -470746842: 2940 /* additiveType */ return new String[] { "CodeableConcept" }; 2941 case 488079534: 2942 /* additiveProductName */ return new String[] { "string" }; 2943 case 186983261: 2944 /* caloricDensity */ return new String[] { "SimpleQuantity" }; 2945 case -1710107042: 2946 /* routeofAdministration */ return new String[] { "CodeableConcept" }; 2947 case 1255702622: 2948 /* administration */ return new String[] {}; 2949 case 2017924652: 2950 /* maxVolumeToDeliver */ return new String[] { "SimpleQuantity" }; 2951 case 427085136: 2952 /* administrationInstruction */ return new String[] { "string" }; 2953 default: 2954 return super.getTypesForProperty(hash, name); 2955 } 2956 2957 } 2958 2959 @Override 2960 public Base addChild(String name) throws FHIRException { 2961 if (name.equals("baseFormulaType")) { 2962 this.baseFormulaType = new CodeableConcept(); 2963 return this.baseFormulaType; 2964 } else if (name.equals("baseFormulaProductName")) { 2965 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.baseFormulaProductName"); 2966 } else if (name.equals("additiveType")) { 2967 this.additiveType = new CodeableConcept(); 2968 return this.additiveType; 2969 } else if (name.equals("additiveProductName")) { 2970 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.additiveProductName"); 2971 } else if (name.equals("caloricDensity")) { 2972 this.caloricDensity = new Quantity(); 2973 return this.caloricDensity; 2974 } else if (name.equals("routeofAdministration")) { 2975 this.routeofAdministration = new CodeableConcept(); 2976 return this.routeofAdministration; 2977 } else if (name.equals("administration")) { 2978 return addAdministration(); 2979 } else if (name.equals("maxVolumeToDeliver")) { 2980 this.maxVolumeToDeliver = new Quantity(); 2981 return this.maxVolumeToDeliver; 2982 } else if (name.equals("administrationInstruction")) { 2983 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.administrationInstruction"); 2984 } else 2985 return super.addChild(name); 2986 } 2987 2988 public NutritionOrderEnteralFormulaComponent copy() { 2989 NutritionOrderEnteralFormulaComponent dst = new NutritionOrderEnteralFormulaComponent(); 2990 copyValues(dst); 2991 return dst; 2992 } 2993 2994 public void copyValues(NutritionOrderEnteralFormulaComponent dst) { 2995 super.copyValues(dst); 2996 dst.baseFormulaType = baseFormulaType == null ? null : baseFormulaType.copy(); 2997 dst.baseFormulaProductName = baseFormulaProductName == null ? null : baseFormulaProductName.copy(); 2998 dst.additiveType = additiveType == null ? null : additiveType.copy(); 2999 dst.additiveProductName = additiveProductName == null ? null : additiveProductName.copy(); 3000 dst.caloricDensity = caloricDensity == null ? null : caloricDensity.copy(); 3001 dst.routeofAdministration = routeofAdministration == null ? null : routeofAdministration.copy(); 3002 if (administration != null) { 3003 dst.administration = new ArrayList<NutritionOrderEnteralFormulaAdministrationComponent>(); 3004 for (NutritionOrderEnteralFormulaAdministrationComponent i : administration) 3005 dst.administration.add(i.copy()); 3006 } 3007 ; 3008 dst.maxVolumeToDeliver = maxVolumeToDeliver == null ? null : maxVolumeToDeliver.copy(); 3009 dst.administrationInstruction = administrationInstruction == null ? null : administrationInstruction.copy(); 3010 } 3011 3012 @Override 3013 public boolean equalsDeep(Base other_) { 3014 if (!super.equalsDeep(other_)) 3015 return false; 3016 if (!(other_ instanceof NutritionOrderEnteralFormulaComponent)) 3017 return false; 3018 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other_; 3019 return compareDeep(baseFormulaType, o.baseFormulaType, true) 3020 && compareDeep(baseFormulaProductName, o.baseFormulaProductName, true) 3021 && compareDeep(additiveType, o.additiveType, true) 3022 && compareDeep(additiveProductName, o.additiveProductName, true) 3023 && compareDeep(caloricDensity, o.caloricDensity, true) 3024 && compareDeep(routeofAdministration, o.routeofAdministration, true) 3025 && compareDeep(administration, o.administration, true) 3026 && compareDeep(maxVolumeToDeliver, o.maxVolumeToDeliver, true) 3027 && compareDeep(administrationInstruction, o.administrationInstruction, true); 3028 } 3029 3030 @Override 3031 public boolean equalsShallow(Base other_) { 3032 if (!super.equalsShallow(other_)) 3033 return false; 3034 if (!(other_ instanceof NutritionOrderEnteralFormulaComponent)) 3035 return false; 3036 NutritionOrderEnteralFormulaComponent o = (NutritionOrderEnteralFormulaComponent) other_; 3037 return compareValues(baseFormulaProductName, o.baseFormulaProductName, true) 3038 && compareValues(additiveProductName, o.additiveProductName, true) 3039 && compareValues(administrationInstruction, o.administrationInstruction, true); 3040 } 3041 3042 public boolean isEmpty() { 3043 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(baseFormulaType, baseFormulaProductName, 3044 additiveType, additiveProductName, caloricDensity, routeofAdministration, administration, maxVolumeToDeliver, 3045 administrationInstruction); 3046 } 3047 3048 public String fhirType() { 3049 return "NutritionOrder.enteralFormula"; 3050 3051 } 3052 3053 } 3054 3055 @Block() 3056 public static class NutritionOrderEnteralFormulaAdministrationComponent extends BackboneElement 3057 implements IBaseBackboneElement { 3058 /** 3059 * The time period and frequency at which the enteral formula should be 3060 * delivered to the patient. 3061 */ 3062 @Child(name = "schedule", type = { Timing.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 3063 @Description(shortDefinition = "Scheduled frequency of enteral feeding", formalDefinition = "The time period and frequency at which the enteral formula should be delivered to the patient.") 3064 protected Timing schedule; 3065 3066 /** 3067 * The volume of formula to provide to the patient per the specified 3068 * administration schedule. 3069 */ 3070 @Child(name = "quantity", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 3071 @Description(shortDefinition = "The volume of formula to provide", formalDefinition = "The volume of formula to provide to the patient per the specified administration schedule.") 3072 protected Quantity quantity; 3073 3074 /** 3075 * The rate of administration of formula via a feeding pump, e.g. 60 mL per 3076 * hour, according to the specified schedule. 3077 */ 3078 @Child(name = "rate", type = { Quantity.class, 3079 Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3080 @Description(shortDefinition = "Speed with which the formula is provided per period of time", formalDefinition = "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.") 3081 protected Type rate; 3082 3083 private static final long serialVersionUID = 673093291L; 3084 3085 /** 3086 * Constructor 3087 */ 3088 public NutritionOrderEnteralFormulaAdministrationComponent() { 3089 super(); 3090 } 3091 3092 /** 3093 * @return {@link #schedule} (The time period and frequency at which the enteral 3094 * formula should be delivered to the patient.) 3095 */ 3096 public Timing getSchedule() { 3097 if (this.schedule == null) 3098 if (Configuration.errorOnAutoCreate()) 3099 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.schedule"); 3100 else if (Configuration.doAutoCreate()) 3101 this.schedule = new Timing(); // cc 3102 return this.schedule; 3103 } 3104 3105 public boolean hasSchedule() { 3106 return this.schedule != null && !this.schedule.isEmpty(); 3107 } 3108 3109 /** 3110 * @param value {@link #schedule} (The time period and frequency at which the 3111 * enteral formula should be delivered to the patient.) 3112 */ 3113 public NutritionOrderEnteralFormulaAdministrationComponent setSchedule(Timing value) { 3114 this.schedule = value; 3115 return this; 3116 } 3117 3118 /** 3119 * @return {@link #quantity} (The volume of formula to provide to the patient 3120 * per the specified administration schedule.) 3121 */ 3122 public Quantity getQuantity() { 3123 if (this.quantity == null) 3124 if (Configuration.errorOnAutoCreate()) 3125 throw new Error("Attempt to auto-create NutritionOrderEnteralFormulaAdministrationComponent.quantity"); 3126 else if (Configuration.doAutoCreate()) 3127 this.quantity = new Quantity(); // cc 3128 return this.quantity; 3129 } 3130 3131 public boolean hasQuantity() { 3132 return this.quantity != null && !this.quantity.isEmpty(); 3133 } 3134 3135 /** 3136 * @param value {@link #quantity} (The volume of formula to provide to the 3137 * patient per the specified administration schedule.) 3138 */ 3139 public NutritionOrderEnteralFormulaAdministrationComponent setQuantity(Quantity value) { 3140 this.quantity = value; 3141 return this; 3142 } 3143 3144 /** 3145 * @return {@link #rate} (The rate of administration of formula via a feeding 3146 * pump, e.g. 60 mL per hour, according to the specified schedule.) 3147 */ 3148 public Type getRate() { 3149 return this.rate; 3150 } 3151 3152 /** 3153 * @return {@link #rate} (The rate of administration of formula via a feeding 3154 * pump, e.g. 60 mL per hour, according to the specified schedule.) 3155 */ 3156 public Quantity getRateQuantity() throws FHIRException { 3157 if (this.rate == null) 3158 this.rate = new Quantity(); 3159 if (!(this.rate instanceof Quantity)) 3160 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.rate.getClass().getName() 3161 + " was encountered"); 3162 return (Quantity) this.rate; 3163 } 3164 3165 public boolean hasRateQuantity() { 3166 return this != null && this.rate instanceof Quantity; 3167 } 3168 3169 /** 3170 * @return {@link #rate} (The rate of administration of formula via a feeding 3171 * pump, e.g. 60 mL per hour, according to the specified schedule.) 3172 */ 3173 public Ratio getRateRatio() throws FHIRException { 3174 if (this.rate == null) 3175 this.rate = new Ratio(); 3176 if (!(this.rate instanceof Ratio)) 3177 throw new FHIRException( 3178 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 3179 return (Ratio) this.rate; 3180 } 3181 3182 public boolean hasRateRatio() { 3183 return this != null && this.rate instanceof Ratio; 3184 } 3185 3186 public boolean hasRate() { 3187 return this.rate != null && !this.rate.isEmpty(); 3188 } 3189 3190 /** 3191 * @param value {@link #rate} (The rate of administration of formula via a 3192 * feeding pump, e.g. 60 mL per hour, according to the specified 3193 * schedule.) 3194 */ 3195 public NutritionOrderEnteralFormulaAdministrationComponent setRate(Type value) { 3196 if (value != null && !(value instanceof Quantity || value instanceof Ratio)) 3197 throw new Error( 3198 "Not the right type for NutritionOrder.enteralFormula.administration.rate[x]: " + value.fhirType()); 3199 this.rate = value; 3200 return this; 3201 } 3202 3203 protected void listChildren(List<Property> children) { 3204 super.listChildren(children); 3205 children.add(new Property("schedule", "Timing", 3206 "The time period and frequency at which the enteral formula should be delivered to the patient.", 0, 1, 3207 schedule)); 3208 children.add(new Property("quantity", "SimpleQuantity", 3209 "The volume of formula to provide to the patient per the specified administration schedule.", 0, 1, 3210 quantity)); 3211 children.add(new Property("rate[x]", "SimpleQuantity|Ratio", 3212 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 3213 0, 1, rate)); 3214 } 3215 3216 @Override 3217 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3218 switch (_hash) { 3219 case -697920873: 3220 /* schedule */ return new Property("schedule", "Timing", 3221 "The time period and frequency at which the enteral formula should be delivered to the patient.", 0, 1, 3222 schedule); 3223 case -1285004149: 3224 /* quantity */ return new Property("quantity", "SimpleQuantity", 3225 "The volume of formula to provide to the patient per the specified administration schedule.", 0, 1, 3226 quantity); 3227 case 983460768: 3228 /* rate[x] */ return new Property("rate[x]", "SimpleQuantity|Ratio", 3229 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 3230 0, 1, rate); 3231 case 3493088: 3232 /* rate */ return new Property("rate[x]", "SimpleQuantity|Ratio", 3233 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 3234 0, 1, rate); 3235 case -1085459061: 3236 /* rateQuantity */ return new Property("rate[x]", "SimpleQuantity|Ratio", 3237 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 3238 0, 1, rate); 3239 case 204021515: 3240 /* rateRatio */ return new Property("rate[x]", "SimpleQuantity|Ratio", 3241 "The rate of administration of formula via a feeding pump, e.g. 60 mL per hour, according to the specified schedule.", 3242 0, 1, rate); 3243 default: 3244 return super.getNamedProperty(_hash, _name, _checkValid); 3245 } 3246 3247 } 3248 3249 @Override 3250 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3251 switch (hash) { 3252 case -697920873: 3253 /* schedule */ return this.schedule == null ? new Base[0] : new Base[] { this.schedule }; // Timing 3254 case -1285004149: 3255 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 3256 case 3493088: 3257 /* rate */ return this.rate == null ? new Base[0] : new Base[] { this.rate }; // Type 3258 default: 3259 return super.getProperty(hash, name, checkValid); 3260 } 3261 3262 } 3263 3264 @Override 3265 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3266 switch (hash) { 3267 case -697920873: // schedule 3268 this.schedule = castToTiming(value); // Timing 3269 return value; 3270 case -1285004149: // quantity 3271 this.quantity = castToQuantity(value); // Quantity 3272 return value; 3273 case 3493088: // rate 3274 this.rate = castToType(value); // Type 3275 return value; 3276 default: 3277 return super.setProperty(hash, name, value); 3278 } 3279 3280 } 3281 3282 @Override 3283 public Base setProperty(String name, Base value) throws FHIRException { 3284 if (name.equals("schedule")) { 3285 this.schedule = castToTiming(value); // Timing 3286 } else if (name.equals("quantity")) { 3287 this.quantity = castToQuantity(value); // Quantity 3288 } else if (name.equals("rate[x]")) { 3289 this.rate = castToType(value); // Type 3290 } else 3291 return super.setProperty(name, value); 3292 return value; 3293 } 3294 3295 @Override 3296 public void removeChild(String name, Base value) throws FHIRException { 3297 if (name.equals("schedule")) { 3298 this.schedule = null; 3299 } else if (name.equals("quantity")) { 3300 this.quantity = null; 3301 } else if (name.equals("rate[x]")) { 3302 this.rate = null; 3303 } else 3304 super.removeChild(name, value); 3305 3306 } 3307 3308 @Override 3309 public Base makeProperty(int hash, String name) throws FHIRException { 3310 switch (hash) { 3311 case -697920873: 3312 return getSchedule(); 3313 case -1285004149: 3314 return getQuantity(); 3315 case 983460768: 3316 return getRate(); 3317 case 3493088: 3318 return getRate(); 3319 default: 3320 return super.makeProperty(hash, name); 3321 } 3322 3323 } 3324 3325 @Override 3326 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3327 switch (hash) { 3328 case -697920873: 3329 /* schedule */ return new String[] { "Timing" }; 3330 case -1285004149: 3331 /* quantity */ return new String[] { "SimpleQuantity" }; 3332 case 3493088: 3333 /* rate */ return new String[] { "SimpleQuantity", "Ratio" }; 3334 default: 3335 return super.getTypesForProperty(hash, name); 3336 } 3337 3338 } 3339 3340 @Override 3341 public Base addChild(String name) throws FHIRException { 3342 if (name.equals("schedule")) { 3343 this.schedule = new Timing(); 3344 return this.schedule; 3345 } else if (name.equals("quantity")) { 3346 this.quantity = new Quantity(); 3347 return this.quantity; 3348 } else if (name.equals("rateQuantity")) { 3349 this.rate = new Quantity(); 3350 return this.rate; 3351 } else if (name.equals("rateRatio")) { 3352 this.rate = new Ratio(); 3353 return this.rate; 3354 } else 3355 return super.addChild(name); 3356 } 3357 3358 public NutritionOrderEnteralFormulaAdministrationComponent copy() { 3359 NutritionOrderEnteralFormulaAdministrationComponent dst = new NutritionOrderEnteralFormulaAdministrationComponent(); 3360 copyValues(dst); 3361 return dst; 3362 } 3363 3364 public void copyValues(NutritionOrderEnteralFormulaAdministrationComponent dst) { 3365 super.copyValues(dst); 3366 dst.schedule = schedule == null ? null : schedule.copy(); 3367 dst.quantity = quantity == null ? null : quantity.copy(); 3368 dst.rate = rate == null ? null : rate.copy(); 3369 } 3370 3371 @Override 3372 public boolean equalsDeep(Base other_) { 3373 if (!super.equalsDeep(other_)) 3374 return false; 3375 if (!(other_ instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 3376 return false; 3377 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other_; 3378 return compareDeep(schedule, o.schedule, true) && compareDeep(quantity, o.quantity, true) 3379 && compareDeep(rate, o.rate, true); 3380 } 3381 3382 @Override 3383 public boolean equalsShallow(Base other_) { 3384 if (!super.equalsShallow(other_)) 3385 return false; 3386 if (!(other_ instanceof NutritionOrderEnteralFormulaAdministrationComponent)) 3387 return false; 3388 NutritionOrderEnteralFormulaAdministrationComponent o = (NutritionOrderEnteralFormulaAdministrationComponent) other_; 3389 return true; 3390 } 3391 3392 public boolean isEmpty() { 3393 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(schedule, quantity, rate); 3394 } 3395 3396 public String fhirType() { 3397 return "NutritionOrder.enteralFormula.administration"; 3398 3399 } 3400 3401 } 3402 3403 /** 3404 * Identifiers assigned to this order by the order sender or by the order 3405 * receiver. 3406 */ 3407 @Child(name = "identifier", type = { 3408 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3409 @Description(shortDefinition = "Identifiers assigned to this order", formalDefinition = "Identifiers assigned to this order by the order sender or by the order receiver.") 3410 protected List<Identifier> identifier; 3411 3412 /** 3413 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other 3414 * definition that is adhered to in whole or in part by this NutritionOrder. 3415 */ 3416 @Child(name = "instantiatesCanonical", type = { 3417 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3418 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.") 3419 protected List<CanonicalType> instantiatesCanonical; 3420 3421 /** 3422 * The URL pointing to an externally maintained protocol, guideline, orderset or 3423 * other definition that is adhered to in whole or in part by this 3424 * NutritionOrder. 3425 */ 3426 @Child(name = "instantiatesUri", type = { 3427 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3428 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.") 3429 protected List<UriType> instantiatesUri; 3430 3431 /** 3432 * The URL pointing to a protocol, guideline, orderset or other definition that 3433 * is adhered to in whole or in part by this NutritionOrder. 3434 */ 3435 @Child(name = "instantiates", type = { 3436 UriType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3437 @Description(shortDefinition = "Instantiates protocol or definition", formalDefinition = "The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.") 3438 protected List<UriType> instantiates; 3439 3440 /** 3441 * The workflow status of the nutrition order/request. 3442 */ 3443 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 3444 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The workflow status of the nutrition order/request.") 3445 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 3446 protected Enumeration<NutritionOrderStatus> status; 3447 3448 /** 3449 * Indicates the level of authority/intentionality associated with the 3450 * NutrionOrder and where the request fits into the workflow chain. 3451 */ 3452 @Child(name = "intent", type = { CodeType.class }, order = 5, min = 1, max = 1, modifier = true, summary = true) 3453 @Description(shortDefinition = "proposal | plan | directive | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.") 3454 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-intent") 3455 protected Enumeration<NutritiionOrderIntent> intent; 3456 3457 /** 3458 * The person (patient) who needs the nutrition order for an oral diet, 3459 * nutritional supplement and/or enteral or formula feeding. 3460 */ 3461 @Child(name = "patient", type = { Patient.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 3462 @Description(shortDefinition = "The person who requires the diet, formula or nutritional supplement", formalDefinition = "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.") 3463 protected Reference patient; 3464 3465 /** 3466 * The actual object that is the target of the reference (The person (patient) 3467 * who needs the nutrition order for an oral diet, nutritional supplement and/or 3468 * enteral or formula feeding.) 3469 */ 3470 protected Patient patientTarget; 3471 3472 /** 3473 * An encounter that provides additional information about the healthcare 3474 * context in which this request is made. 3475 */ 3476 @Child(name = "encounter", type = { Encounter.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 3477 @Description(shortDefinition = "The encounter associated with this nutrition order", formalDefinition = "An encounter that provides additional information about the healthcare context in which this request is made.") 3478 protected Reference encounter; 3479 3480 /** 3481 * The actual object that is the target of the reference (An encounter that 3482 * provides additional information about the healthcare context in which this 3483 * request is made.) 3484 */ 3485 protected Encounter encounterTarget; 3486 3487 /** 3488 * The date and time that this nutrition order was requested. 3489 */ 3490 @Child(name = "dateTime", type = { 3491 DateTimeType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 3492 @Description(shortDefinition = "Date and time the nutrition order was requested", formalDefinition = "The date and time that this nutrition order was requested.") 3493 protected DateTimeType dateTime; 3494 3495 /** 3496 * The practitioner that holds legal responsibility for ordering the diet, 3497 * nutritional supplement, or formula feedings. 3498 */ 3499 @Child(name = "orderer", type = { Practitioner.class, 3500 PractitionerRole.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3501 @Description(shortDefinition = "Who ordered the diet, formula or nutritional supplement", formalDefinition = "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.") 3502 protected Reference orderer; 3503 3504 /** 3505 * The actual object that is the target of the reference (The practitioner that 3506 * holds legal responsibility for ordering the diet, nutritional supplement, or 3507 * formula feedings.) 3508 */ 3509 protected Resource ordererTarget; 3510 3511 /** 3512 * A link to a record of allergies or intolerances which should be included in 3513 * the nutrition order. 3514 */ 3515 @Child(name = "allergyIntolerance", type = { 3516 AllergyIntolerance.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3517 @Description(shortDefinition = "List of the patient's food and nutrition-related allergies and intolerances", formalDefinition = "A link to a record of allergies or intolerances which should be included in the nutrition order.") 3518 protected List<Reference> allergyIntolerance; 3519 /** 3520 * The actual objects that are the target of the reference (A link to a record 3521 * of allergies or intolerances which should be included in the nutrition 3522 * order.) 3523 */ 3524 protected List<AllergyIntolerance> allergyIntoleranceTarget; 3525 3526 /** 3527 * This modifier is used to convey order-specific modifiers about the type of 3528 * food that should be given. These can be derived from patient allergies, 3529 * intolerances, or preferences such as Halal, Vegan or Kosher. This modifier 3530 * applies to the entire nutrition order inclusive of the oral diet, nutritional 3531 * supplements and enteral formula feedings. 3532 */ 3533 @Child(name = "foodPreferenceModifier", type = { 3534 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3535 @Description(shortDefinition = "Order-specific modifier about the type of food that should be given", formalDefinition = "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.") 3536 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-diet") 3537 protected List<CodeableConcept> foodPreferenceModifier; 3538 3539 /** 3540 * This modifier is used to convey Order-specific modifier about the type of 3541 * oral food or oral fluids that should not be given. These can be derived from 3542 * patient allergies, intolerances, or preferences such as No Red Meat, No Soy 3543 * or No Wheat or Gluten-Free. While it should not be necessary to repeat 3544 * allergy or intolerance information captured in the referenced 3545 * AllergyIntolerance resource in the excludeFoodModifier, this element may be 3546 * used to convey additional specificity related to foods that should be 3547 * eliminated from the patient?s diet for any reason. This modifier applies to 3548 * the entire nutrition order inclusive of the oral diet, nutritional 3549 * supplements and enteral formula feedings. 3550 */ 3551 @Child(name = "excludeFoodModifier", type = { 3552 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3553 @Description(shortDefinition = "Order-specific modifier about the type of food that should not be given", formalDefinition = "This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.") 3554 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/food-type") 3555 protected List<CodeableConcept> excludeFoodModifier; 3556 3557 /** 3558 * Diet given orally in contrast to enteral (tube) feeding. 3559 */ 3560 @Child(name = "oralDiet", type = {}, order = 13, min = 0, max = 1, modifier = false, summary = false) 3561 @Description(shortDefinition = "Oral diet components", formalDefinition = "Diet given orally in contrast to enteral (tube) feeding.") 3562 protected NutritionOrderOralDietComponent oralDiet; 3563 3564 /** 3565 * Oral nutritional products given in order to add further nutritional value to 3566 * the patient's diet. 3567 */ 3568 @Child(name = "supplement", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3569 @Description(shortDefinition = "Supplement components", formalDefinition = "Oral nutritional products given in order to add further nutritional value to the patient's diet.") 3570 protected List<NutritionOrderSupplementComponent> supplement; 3571 3572 /** 3573 * Feeding provided through the gastrointestinal tract via a tube, catheter, or 3574 * stoma that delivers nutrition distal to the oral cavity. 3575 */ 3576 @Child(name = "enteralFormula", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = false) 3577 @Description(shortDefinition = "Enteral formula components", formalDefinition = "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.") 3578 protected NutritionOrderEnteralFormulaComponent enteralFormula; 3579 3580 /** 3581 * Comments made about the {{title}} by the requester, performer, subject or 3582 * other participants. 3583 */ 3584 @Child(name = "note", type = { 3585 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3586 @Description(shortDefinition = "Comments", formalDefinition = "Comments made about the {{title}} by the requester, performer, subject or other participants.") 3587 protected List<Annotation> note; 3588 3589 private static final long serialVersionUID = 1746744267L; 3590 3591 /** 3592 * Constructor 3593 */ 3594 public NutritionOrder() { 3595 super(); 3596 } 3597 3598 /** 3599 * Constructor 3600 */ 3601 public NutritionOrder(Enumeration<NutritionOrderStatus> status, Enumeration<NutritiionOrderIntent> intent, 3602 Reference patient, DateTimeType dateTime) { 3603 super(); 3604 this.status = status; 3605 this.intent = intent; 3606 this.patient = patient; 3607 this.dateTime = dateTime; 3608 } 3609 3610 /** 3611 * @return {@link #identifier} (Identifiers assigned to this order by the order 3612 * sender or by the order receiver.) 3613 */ 3614 public List<Identifier> getIdentifier() { 3615 if (this.identifier == null) 3616 this.identifier = new ArrayList<Identifier>(); 3617 return this.identifier; 3618 } 3619 3620 /** 3621 * @return Returns a reference to <code>this</code> for easy method chaining 3622 */ 3623 public NutritionOrder setIdentifier(List<Identifier> theIdentifier) { 3624 this.identifier = theIdentifier; 3625 return this; 3626 } 3627 3628 public boolean hasIdentifier() { 3629 if (this.identifier == null) 3630 return false; 3631 for (Identifier item : this.identifier) 3632 if (!item.isEmpty()) 3633 return true; 3634 return false; 3635 } 3636 3637 public Identifier addIdentifier() { // 3 3638 Identifier t = new Identifier(); 3639 if (this.identifier == null) 3640 this.identifier = new ArrayList<Identifier>(); 3641 this.identifier.add(t); 3642 return t; 3643 } 3644 3645 public NutritionOrder addIdentifier(Identifier t) { // 3 3646 if (t == null) 3647 return this; 3648 if (this.identifier == null) 3649 this.identifier = new ArrayList<Identifier>(); 3650 this.identifier.add(t); 3651 return this; 3652 } 3653 3654 /** 3655 * @return The first repetition of repeating field {@link #identifier}, creating 3656 * it if it does not already exist 3657 */ 3658 public Identifier getIdentifierFirstRep() { 3659 if (getIdentifier().isEmpty()) { 3660 addIdentifier(); 3661 } 3662 return getIdentifier().get(0); 3663 } 3664 3665 /** 3666 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 3667 * protocol, guideline, orderset or other definition that is adhered to 3668 * in whole or in part by this NutritionOrder.) 3669 */ 3670 public List<CanonicalType> getInstantiatesCanonical() { 3671 if (this.instantiatesCanonical == null) 3672 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3673 return this.instantiatesCanonical; 3674 } 3675 3676 /** 3677 * @return Returns a reference to <code>this</code> for easy method chaining 3678 */ 3679 public NutritionOrder setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 3680 this.instantiatesCanonical = theInstantiatesCanonical; 3681 return this; 3682 } 3683 3684 public boolean hasInstantiatesCanonical() { 3685 if (this.instantiatesCanonical == null) 3686 return false; 3687 for (CanonicalType item : this.instantiatesCanonical) 3688 if (!item.isEmpty()) 3689 return true; 3690 return false; 3691 } 3692 3693 /** 3694 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 3695 * protocol, guideline, orderset or other definition that is adhered to 3696 * in whole or in part by this NutritionOrder.) 3697 */ 3698 public CanonicalType addInstantiatesCanonicalElement() {// 2 3699 CanonicalType t = new CanonicalType(); 3700 if (this.instantiatesCanonical == null) 3701 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3702 this.instantiatesCanonical.add(t); 3703 return t; 3704 } 3705 3706 /** 3707 * @param value {@link #instantiatesCanonical} (The URL pointing to a 3708 * FHIR-defined protocol, guideline, orderset or other definition 3709 * that is adhered to in whole or in part by this NutritionOrder.) 3710 */ 3711 public NutritionOrder addInstantiatesCanonical(String value) { // 1 3712 CanonicalType t = new CanonicalType(); 3713 t.setValue(value); 3714 if (this.instantiatesCanonical == null) 3715 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3716 this.instantiatesCanonical.add(t); 3717 return this; 3718 } 3719 3720 /** 3721 * @param value {@link #instantiatesCanonical} (The URL pointing to a 3722 * FHIR-defined protocol, guideline, orderset or other definition 3723 * that is adhered to in whole or in part by this NutritionOrder.) 3724 */ 3725 public boolean hasInstantiatesCanonical(String value) { 3726 if (this.instantiatesCanonical == null) 3727 return false; 3728 for (CanonicalType v : this.instantiatesCanonical) 3729 if (v.getValue().equals(value)) // canonical(ActivityDefinition|PlanDefinition) 3730 return true; 3731 return false; 3732 } 3733 3734 /** 3735 * @return {@link #instantiatesUri} (The URL pointing to an externally 3736 * maintained protocol, guideline, orderset or other definition that is 3737 * adhered to in whole or in part by this NutritionOrder.) 3738 */ 3739 public List<UriType> getInstantiatesUri() { 3740 if (this.instantiatesUri == null) 3741 this.instantiatesUri = new ArrayList<UriType>(); 3742 return this.instantiatesUri; 3743 } 3744 3745 /** 3746 * @return Returns a reference to <code>this</code> for easy method chaining 3747 */ 3748 public NutritionOrder setInstantiatesUri(List<UriType> theInstantiatesUri) { 3749 this.instantiatesUri = theInstantiatesUri; 3750 return this; 3751 } 3752 3753 public boolean hasInstantiatesUri() { 3754 if (this.instantiatesUri == null) 3755 return false; 3756 for (UriType item : this.instantiatesUri) 3757 if (!item.isEmpty()) 3758 return true; 3759 return false; 3760 } 3761 3762 /** 3763 * @return {@link #instantiatesUri} (The URL pointing to an externally 3764 * maintained protocol, guideline, orderset or other definition that is 3765 * adhered to in whole or in part by this NutritionOrder.) 3766 */ 3767 public UriType addInstantiatesUriElement() {// 2 3768 UriType t = new UriType(); 3769 if (this.instantiatesUri == null) 3770 this.instantiatesUri = new ArrayList<UriType>(); 3771 this.instantiatesUri.add(t); 3772 return t; 3773 } 3774 3775 /** 3776 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3777 * maintained protocol, guideline, orderset or other definition 3778 * that is adhered to in whole or in part by this NutritionOrder.) 3779 */ 3780 public NutritionOrder addInstantiatesUri(String value) { // 1 3781 UriType t = new UriType(); 3782 t.setValue(value); 3783 if (this.instantiatesUri == null) 3784 this.instantiatesUri = new ArrayList<UriType>(); 3785 this.instantiatesUri.add(t); 3786 return this; 3787 } 3788 3789 /** 3790 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3791 * maintained protocol, guideline, orderset or other definition 3792 * that is adhered to in whole or in part by this NutritionOrder.) 3793 */ 3794 public boolean hasInstantiatesUri(String value) { 3795 if (this.instantiatesUri == null) 3796 return false; 3797 for (UriType v : this.instantiatesUri) 3798 if (v.getValue().equals(value)) // uri 3799 return true; 3800 return false; 3801 } 3802 3803 /** 3804 * @return {@link #instantiates} (The URL pointing to a protocol, guideline, 3805 * orderset or other definition that is adhered to in whole or in part 3806 * by this NutritionOrder.) 3807 */ 3808 public List<UriType> getInstantiates() { 3809 if (this.instantiates == null) 3810 this.instantiates = new ArrayList<UriType>(); 3811 return this.instantiates; 3812 } 3813 3814 /** 3815 * @return Returns a reference to <code>this</code> for easy method chaining 3816 */ 3817 public NutritionOrder setInstantiates(List<UriType> theInstantiates) { 3818 this.instantiates = theInstantiates; 3819 return this; 3820 } 3821 3822 public boolean hasInstantiates() { 3823 if (this.instantiates == null) 3824 return false; 3825 for (UriType item : this.instantiates) 3826 if (!item.isEmpty()) 3827 return true; 3828 return false; 3829 } 3830 3831 /** 3832 * @return {@link #instantiates} (The URL pointing to a protocol, guideline, 3833 * orderset or other definition that is adhered to in whole or in part 3834 * by this NutritionOrder.) 3835 */ 3836 public UriType addInstantiatesElement() {// 2 3837 UriType t = new UriType(); 3838 if (this.instantiates == null) 3839 this.instantiates = new ArrayList<UriType>(); 3840 this.instantiates.add(t); 3841 return t; 3842 } 3843 3844 /** 3845 * @param value {@link #instantiates} (The URL pointing to a protocol, 3846 * guideline, orderset or other definition that is adhered to in 3847 * whole or in part by this NutritionOrder.) 3848 */ 3849 public NutritionOrder addInstantiates(String value) { // 1 3850 UriType t = new UriType(); 3851 t.setValue(value); 3852 if (this.instantiates == null) 3853 this.instantiates = new ArrayList<UriType>(); 3854 this.instantiates.add(t); 3855 return this; 3856 } 3857 3858 /** 3859 * @param value {@link #instantiates} (The URL pointing to a protocol, 3860 * guideline, orderset or other definition that is adhered to in 3861 * whole or in part by this NutritionOrder.) 3862 */ 3863 public boolean hasInstantiates(String value) { 3864 if (this.instantiates == null) 3865 return false; 3866 for (UriType v : this.instantiates) 3867 if (v.getValue().equals(value)) // uri 3868 return true; 3869 return false; 3870 } 3871 3872 /** 3873 * @return {@link #status} (The workflow status of the nutrition 3874 * order/request.). This is the underlying object with id, value and 3875 * extensions. The accessor "getStatus" gives direct access to the value 3876 */ 3877 public Enumeration<NutritionOrderStatus> getStatusElement() { 3878 if (this.status == null) 3879 if (Configuration.errorOnAutoCreate()) 3880 throw new Error("Attempt to auto-create NutritionOrder.status"); 3881 else if (Configuration.doAutoCreate()) 3882 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); // bb 3883 return this.status; 3884 } 3885 3886 public boolean hasStatusElement() { 3887 return this.status != null && !this.status.isEmpty(); 3888 } 3889 3890 public boolean hasStatus() { 3891 return this.status != null && !this.status.isEmpty(); 3892 } 3893 3894 /** 3895 * @param value {@link #status} (The workflow status of the nutrition 3896 * order/request.). This is the underlying object with id, value 3897 * and extensions. The accessor "getStatus" gives direct access to 3898 * the value 3899 */ 3900 public NutritionOrder setStatusElement(Enumeration<NutritionOrderStatus> value) { 3901 this.status = value; 3902 return this; 3903 } 3904 3905 /** 3906 * @return The workflow status of the nutrition order/request. 3907 */ 3908 public NutritionOrderStatus getStatus() { 3909 return this.status == null ? null : this.status.getValue(); 3910 } 3911 3912 /** 3913 * @param value The workflow status of the nutrition order/request. 3914 */ 3915 public NutritionOrder setStatus(NutritionOrderStatus value) { 3916 if (this.status == null) 3917 this.status = new Enumeration<NutritionOrderStatus>(new NutritionOrderStatusEnumFactory()); 3918 this.status.setValue(value); 3919 return this; 3920 } 3921 3922 /** 3923 * @return {@link #intent} (Indicates the level of authority/intentionality 3924 * associated with the NutrionOrder and where the request fits into the 3925 * workflow chain.). This is the underlying object with id, value and 3926 * extensions. The accessor "getIntent" gives direct access to the value 3927 */ 3928 public Enumeration<NutritiionOrderIntent> getIntentElement() { 3929 if (this.intent == null) 3930 if (Configuration.errorOnAutoCreate()) 3931 throw new Error("Attempt to auto-create NutritionOrder.intent"); 3932 else if (Configuration.doAutoCreate()) 3933 this.intent = new Enumeration<NutritiionOrderIntent>(new NutritiionOrderIntentEnumFactory()); // bb 3934 return this.intent; 3935 } 3936 3937 public boolean hasIntentElement() { 3938 return this.intent != null && !this.intent.isEmpty(); 3939 } 3940 3941 public boolean hasIntent() { 3942 return this.intent != null && !this.intent.isEmpty(); 3943 } 3944 3945 /** 3946 * @param value {@link #intent} (Indicates the level of authority/intentionality 3947 * associated with the NutrionOrder and where the request fits into 3948 * the workflow chain.). This is the underlying object with id, 3949 * value and extensions. The accessor "getIntent" gives direct 3950 * access to the value 3951 */ 3952 public NutritionOrder setIntentElement(Enumeration<NutritiionOrderIntent> value) { 3953 this.intent = value; 3954 return this; 3955 } 3956 3957 /** 3958 * @return Indicates the level of authority/intentionality associated with the 3959 * NutrionOrder and where the request fits into the workflow chain. 3960 */ 3961 public NutritiionOrderIntent getIntent() { 3962 return this.intent == null ? null : this.intent.getValue(); 3963 } 3964 3965 /** 3966 * @param value Indicates the level of authority/intentionality associated with 3967 * the NutrionOrder and where the request fits into the workflow 3968 * chain. 3969 */ 3970 public NutritionOrder setIntent(NutritiionOrderIntent value) { 3971 if (this.intent == null) 3972 this.intent = new Enumeration<NutritiionOrderIntent>(new NutritiionOrderIntentEnumFactory()); 3973 this.intent.setValue(value); 3974 return this; 3975 } 3976 3977 /** 3978 * @return {@link #patient} (The person (patient) who needs the nutrition order 3979 * for an oral diet, nutritional supplement and/or enteral or formula 3980 * feeding.) 3981 */ 3982 public Reference getPatient() { 3983 if (this.patient == null) 3984 if (Configuration.errorOnAutoCreate()) 3985 throw new Error("Attempt to auto-create NutritionOrder.patient"); 3986 else if (Configuration.doAutoCreate()) 3987 this.patient = new Reference(); // cc 3988 return this.patient; 3989 } 3990 3991 public boolean hasPatient() { 3992 return this.patient != null && !this.patient.isEmpty(); 3993 } 3994 3995 /** 3996 * @param value {@link #patient} (The person (patient) who needs the nutrition 3997 * order for an oral diet, nutritional supplement and/or enteral or 3998 * formula feeding.) 3999 */ 4000 public NutritionOrder setPatient(Reference value) { 4001 this.patient = value; 4002 return this; 4003 } 4004 4005 /** 4006 * @return {@link #patient} The actual object that is the target of the 4007 * reference. The reference library doesn't populate this, but you can 4008 * use it to hold the resource if you resolve it. (The person (patient) 4009 * who needs the nutrition order for an oral diet, nutritional 4010 * supplement and/or enteral or formula feeding.) 4011 */ 4012 public Patient getPatientTarget() { 4013 if (this.patientTarget == null) 4014 if (Configuration.errorOnAutoCreate()) 4015 throw new Error("Attempt to auto-create NutritionOrder.patient"); 4016 else if (Configuration.doAutoCreate()) 4017 this.patientTarget = new Patient(); // aa 4018 return this.patientTarget; 4019 } 4020 4021 /** 4022 * @param value {@link #patient} The actual object that is the target of the 4023 * reference. The reference library doesn't use these, but you can 4024 * use it to hold the resource if you resolve it. (The person 4025 * (patient) who needs the nutrition order for an oral diet, 4026 * nutritional supplement and/or enteral or formula feeding.) 4027 */ 4028 public NutritionOrder setPatientTarget(Patient value) { 4029 this.patientTarget = value; 4030 return this; 4031 } 4032 4033 /** 4034 * @return {@link #encounter} (An encounter that provides additional information 4035 * about the healthcare context in which this request is made.) 4036 */ 4037 public Reference getEncounter() { 4038 if (this.encounter == null) 4039 if (Configuration.errorOnAutoCreate()) 4040 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 4041 else if (Configuration.doAutoCreate()) 4042 this.encounter = new Reference(); // cc 4043 return this.encounter; 4044 } 4045 4046 public boolean hasEncounter() { 4047 return this.encounter != null && !this.encounter.isEmpty(); 4048 } 4049 4050 /** 4051 * @param value {@link #encounter} (An encounter that provides additional 4052 * information about the healthcare context in which this request 4053 * is made.) 4054 */ 4055 public NutritionOrder setEncounter(Reference value) { 4056 this.encounter = value; 4057 return this; 4058 } 4059 4060 /** 4061 * @return {@link #encounter} The actual object that is the target of the 4062 * reference. The reference library doesn't populate this, but you can 4063 * use it to hold the resource if you resolve it. (An encounter that 4064 * provides additional information about the healthcare context in which 4065 * this request is made.) 4066 */ 4067 public Encounter getEncounterTarget() { 4068 if (this.encounterTarget == null) 4069 if (Configuration.errorOnAutoCreate()) 4070 throw new Error("Attempt to auto-create NutritionOrder.encounter"); 4071 else if (Configuration.doAutoCreate()) 4072 this.encounterTarget = new Encounter(); // aa 4073 return this.encounterTarget; 4074 } 4075 4076 /** 4077 * @param value {@link #encounter} The actual object that is the target of the 4078 * reference. The reference library doesn't use these, but you can 4079 * use it to hold the resource if you resolve it. (An encounter 4080 * that provides additional information about the healthcare 4081 * context in which this request is made.) 4082 */ 4083 public NutritionOrder setEncounterTarget(Encounter value) { 4084 this.encounterTarget = value; 4085 return this; 4086 } 4087 4088 /** 4089 * @return {@link #dateTime} (The date and time that this nutrition order was 4090 * requested.). This is the underlying object with id, value and 4091 * extensions. The accessor "getDateTime" gives direct access to the 4092 * value 4093 */ 4094 public DateTimeType getDateTimeElement() { 4095 if (this.dateTime == null) 4096 if (Configuration.errorOnAutoCreate()) 4097 throw new Error("Attempt to auto-create NutritionOrder.dateTime"); 4098 else if (Configuration.doAutoCreate()) 4099 this.dateTime = new DateTimeType(); // bb 4100 return this.dateTime; 4101 } 4102 4103 public boolean hasDateTimeElement() { 4104 return this.dateTime != null && !this.dateTime.isEmpty(); 4105 } 4106 4107 public boolean hasDateTime() { 4108 return this.dateTime != null && !this.dateTime.isEmpty(); 4109 } 4110 4111 /** 4112 * @param value {@link #dateTime} (The date and time that this nutrition order 4113 * was requested.). This is the underlying object with id, value 4114 * and extensions. The accessor "getDateTime" gives direct access 4115 * to the value 4116 */ 4117 public NutritionOrder setDateTimeElement(DateTimeType value) { 4118 this.dateTime = value; 4119 return this; 4120 } 4121 4122 /** 4123 * @return The date and time that this nutrition order was requested. 4124 */ 4125 public Date getDateTime() { 4126 return this.dateTime == null ? null : this.dateTime.getValue(); 4127 } 4128 4129 /** 4130 * @param value The date and time that this nutrition order was requested. 4131 */ 4132 public NutritionOrder setDateTime(Date value) { 4133 if (this.dateTime == null) 4134 this.dateTime = new DateTimeType(); 4135 this.dateTime.setValue(value); 4136 return this; 4137 } 4138 4139 /** 4140 * @return {@link #orderer} (The practitioner that holds legal responsibility 4141 * for ordering the diet, nutritional supplement, or formula feedings.) 4142 */ 4143 public Reference getOrderer() { 4144 if (this.orderer == null) 4145 if (Configuration.errorOnAutoCreate()) 4146 throw new Error("Attempt to auto-create NutritionOrder.orderer"); 4147 else if (Configuration.doAutoCreate()) 4148 this.orderer = new Reference(); // cc 4149 return this.orderer; 4150 } 4151 4152 public boolean hasOrderer() { 4153 return this.orderer != null && !this.orderer.isEmpty(); 4154 } 4155 4156 /** 4157 * @param value {@link #orderer} (The practitioner that holds legal 4158 * responsibility for ordering the diet, nutritional supplement, or 4159 * formula feedings.) 4160 */ 4161 public NutritionOrder setOrderer(Reference value) { 4162 this.orderer = value; 4163 return this; 4164 } 4165 4166 /** 4167 * @return {@link #orderer} The actual object that is the target of the 4168 * reference. The reference library doesn't populate this, but you can 4169 * use it to hold the resource if you resolve it. (The practitioner that 4170 * holds legal responsibility for ordering the diet, nutritional 4171 * supplement, or formula feedings.) 4172 */ 4173 public Resource getOrdererTarget() { 4174 return this.ordererTarget; 4175 } 4176 4177 /** 4178 * @param value {@link #orderer} The actual object that is the target of the 4179 * reference. The reference library doesn't use these, but you can 4180 * use it to hold the resource if you resolve it. (The practitioner 4181 * that holds legal responsibility for ordering the diet, 4182 * nutritional supplement, or formula feedings.) 4183 */ 4184 public NutritionOrder setOrdererTarget(Resource value) { 4185 this.ordererTarget = value; 4186 return this; 4187 } 4188 4189 /** 4190 * @return {@link #allergyIntolerance} (A link to a record of allergies or 4191 * intolerances which should be included in the nutrition order.) 4192 */ 4193 public List<Reference> getAllergyIntolerance() { 4194 if (this.allergyIntolerance == null) 4195 this.allergyIntolerance = new ArrayList<Reference>(); 4196 return this.allergyIntolerance; 4197 } 4198 4199 /** 4200 * @return Returns a reference to <code>this</code> for easy method chaining 4201 */ 4202 public NutritionOrder setAllergyIntolerance(List<Reference> theAllergyIntolerance) { 4203 this.allergyIntolerance = theAllergyIntolerance; 4204 return this; 4205 } 4206 4207 public boolean hasAllergyIntolerance() { 4208 if (this.allergyIntolerance == null) 4209 return false; 4210 for (Reference item : this.allergyIntolerance) 4211 if (!item.isEmpty()) 4212 return true; 4213 return false; 4214 } 4215 4216 public Reference addAllergyIntolerance() { // 3 4217 Reference t = new Reference(); 4218 if (this.allergyIntolerance == null) 4219 this.allergyIntolerance = new ArrayList<Reference>(); 4220 this.allergyIntolerance.add(t); 4221 return t; 4222 } 4223 4224 public NutritionOrder addAllergyIntolerance(Reference t) { // 3 4225 if (t == null) 4226 return this; 4227 if (this.allergyIntolerance == null) 4228 this.allergyIntolerance = new ArrayList<Reference>(); 4229 this.allergyIntolerance.add(t); 4230 return this; 4231 } 4232 4233 /** 4234 * @return The first repetition of repeating field {@link #allergyIntolerance}, 4235 * creating it if it does not already exist 4236 */ 4237 public Reference getAllergyIntoleranceFirstRep() { 4238 if (getAllergyIntolerance().isEmpty()) { 4239 addAllergyIntolerance(); 4240 } 4241 return getAllergyIntolerance().get(0); 4242 } 4243 4244 /** 4245 * @deprecated Use Reference#setResource(IBaseResource) instead 4246 */ 4247 @Deprecated 4248 public List<AllergyIntolerance> getAllergyIntoleranceTarget() { 4249 if (this.allergyIntoleranceTarget == null) 4250 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 4251 return this.allergyIntoleranceTarget; 4252 } 4253 4254 /** 4255 * @deprecated Use Reference#setResource(IBaseResource) instead 4256 */ 4257 @Deprecated 4258 public AllergyIntolerance addAllergyIntoleranceTarget() { 4259 AllergyIntolerance r = new AllergyIntolerance(); 4260 if (this.allergyIntoleranceTarget == null) 4261 this.allergyIntoleranceTarget = new ArrayList<AllergyIntolerance>(); 4262 this.allergyIntoleranceTarget.add(r); 4263 return r; 4264 } 4265 4266 /** 4267 * @return {@link #foodPreferenceModifier} (This modifier is used to convey 4268 * order-specific modifiers about the type of food that should be given. 4269 * These can be derived from patient allergies, intolerances, or 4270 * preferences such as Halal, Vegan or Kosher. This modifier applies to 4271 * the entire nutrition order inclusive of the oral diet, nutritional 4272 * supplements and enteral formula feedings.) 4273 */ 4274 public List<CodeableConcept> getFoodPreferenceModifier() { 4275 if (this.foodPreferenceModifier == null) 4276 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 4277 return this.foodPreferenceModifier; 4278 } 4279 4280 /** 4281 * @return Returns a reference to <code>this</code> for easy method chaining 4282 */ 4283 public NutritionOrder setFoodPreferenceModifier(List<CodeableConcept> theFoodPreferenceModifier) { 4284 this.foodPreferenceModifier = theFoodPreferenceModifier; 4285 return this; 4286 } 4287 4288 public boolean hasFoodPreferenceModifier() { 4289 if (this.foodPreferenceModifier == null) 4290 return false; 4291 for (CodeableConcept item : this.foodPreferenceModifier) 4292 if (!item.isEmpty()) 4293 return true; 4294 return false; 4295 } 4296 4297 public CodeableConcept addFoodPreferenceModifier() { // 3 4298 CodeableConcept t = new CodeableConcept(); 4299 if (this.foodPreferenceModifier == null) 4300 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 4301 this.foodPreferenceModifier.add(t); 4302 return t; 4303 } 4304 4305 public NutritionOrder addFoodPreferenceModifier(CodeableConcept t) { // 3 4306 if (t == null) 4307 return this; 4308 if (this.foodPreferenceModifier == null) 4309 this.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 4310 this.foodPreferenceModifier.add(t); 4311 return this; 4312 } 4313 4314 /** 4315 * @return The first repetition of repeating field 4316 * {@link #foodPreferenceModifier}, creating it if it does not already 4317 * exist 4318 */ 4319 public CodeableConcept getFoodPreferenceModifierFirstRep() { 4320 if (getFoodPreferenceModifier().isEmpty()) { 4321 addFoodPreferenceModifier(); 4322 } 4323 return getFoodPreferenceModifier().get(0); 4324 } 4325 4326 /** 4327 * @return {@link #excludeFoodModifier} (This modifier is used to convey 4328 * Order-specific modifier about the type of oral food or oral fluids 4329 * that should not be given. These can be derived from patient 4330 * allergies, intolerances, or preferences such as No Red Meat, No Soy 4331 * or No Wheat or Gluten-Free. While it should not be necessary to 4332 * repeat allergy or intolerance information captured in the referenced 4333 * AllergyIntolerance resource in the excludeFoodModifier, this element 4334 * may be used to convey additional specificity related to foods that 4335 * should be eliminated from the patient?s diet for any reason. This 4336 * modifier applies to the entire nutrition order inclusive of the oral 4337 * diet, nutritional supplements and enteral formula feedings.) 4338 */ 4339 public List<CodeableConcept> getExcludeFoodModifier() { 4340 if (this.excludeFoodModifier == null) 4341 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 4342 return this.excludeFoodModifier; 4343 } 4344 4345 /** 4346 * @return Returns a reference to <code>this</code> for easy method chaining 4347 */ 4348 public NutritionOrder setExcludeFoodModifier(List<CodeableConcept> theExcludeFoodModifier) { 4349 this.excludeFoodModifier = theExcludeFoodModifier; 4350 return this; 4351 } 4352 4353 public boolean hasExcludeFoodModifier() { 4354 if (this.excludeFoodModifier == null) 4355 return false; 4356 for (CodeableConcept item : this.excludeFoodModifier) 4357 if (!item.isEmpty()) 4358 return true; 4359 return false; 4360 } 4361 4362 public CodeableConcept addExcludeFoodModifier() { // 3 4363 CodeableConcept t = new CodeableConcept(); 4364 if (this.excludeFoodModifier == null) 4365 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 4366 this.excludeFoodModifier.add(t); 4367 return t; 4368 } 4369 4370 public NutritionOrder addExcludeFoodModifier(CodeableConcept t) { // 3 4371 if (t == null) 4372 return this; 4373 if (this.excludeFoodModifier == null) 4374 this.excludeFoodModifier = new ArrayList<CodeableConcept>(); 4375 this.excludeFoodModifier.add(t); 4376 return this; 4377 } 4378 4379 /** 4380 * @return The first repetition of repeating field {@link #excludeFoodModifier}, 4381 * creating it if it does not already exist 4382 */ 4383 public CodeableConcept getExcludeFoodModifierFirstRep() { 4384 if (getExcludeFoodModifier().isEmpty()) { 4385 addExcludeFoodModifier(); 4386 } 4387 return getExcludeFoodModifier().get(0); 4388 } 4389 4390 /** 4391 * @return {@link #oralDiet} (Diet given orally in contrast to enteral (tube) 4392 * feeding.) 4393 */ 4394 public NutritionOrderOralDietComponent getOralDiet() { 4395 if (this.oralDiet == null) 4396 if (Configuration.errorOnAutoCreate()) 4397 throw new Error("Attempt to auto-create NutritionOrder.oralDiet"); 4398 else if (Configuration.doAutoCreate()) 4399 this.oralDiet = new NutritionOrderOralDietComponent(); // cc 4400 return this.oralDiet; 4401 } 4402 4403 public boolean hasOralDiet() { 4404 return this.oralDiet != null && !this.oralDiet.isEmpty(); 4405 } 4406 4407 /** 4408 * @param value {@link #oralDiet} (Diet given orally in contrast to enteral 4409 * (tube) feeding.) 4410 */ 4411 public NutritionOrder setOralDiet(NutritionOrderOralDietComponent value) { 4412 this.oralDiet = value; 4413 return this; 4414 } 4415 4416 /** 4417 * @return {@link #supplement} (Oral nutritional products given in order to add 4418 * further nutritional value to the patient's diet.) 4419 */ 4420 public List<NutritionOrderSupplementComponent> getSupplement() { 4421 if (this.supplement == null) 4422 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 4423 return this.supplement; 4424 } 4425 4426 /** 4427 * @return Returns a reference to <code>this</code> for easy method chaining 4428 */ 4429 public NutritionOrder setSupplement(List<NutritionOrderSupplementComponent> theSupplement) { 4430 this.supplement = theSupplement; 4431 return this; 4432 } 4433 4434 public boolean hasSupplement() { 4435 if (this.supplement == null) 4436 return false; 4437 for (NutritionOrderSupplementComponent item : this.supplement) 4438 if (!item.isEmpty()) 4439 return true; 4440 return false; 4441 } 4442 4443 public NutritionOrderSupplementComponent addSupplement() { // 3 4444 NutritionOrderSupplementComponent t = new NutritionOrderSupplementComponent(); 4445 if (this.supplement == null) 4446 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 4447 this.supplement.add(t); 4448 return t; 4449 } 4450 4451 public NutritionOrder addSupplement(NutritionOrderSupplementComponent t) { // 3 4452 if (t == null) 4453 return this; 4454 if (this.supplement == null) 4455 this.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 4456 this.supplement.add(t); 4457 return this; 4458 } 4459 4460 /** 4461 * @return The first repetition of repeating field {@link #supplement}, creating 4462 * it if it does not already exist 4463 */ 4464 public NutritionOrderSupplementComponent getSupplementFirstRep() { 4465 if (getSupplement().isEmpty()) { 4466 addSupplement(); 4467 } 4468 return getSupplement().get(0); 4469 } 4470 4471 /** 4472 * @return {@link #enteralFormula} (Feeding provided through the 4473 * gastrointestinal tract via a tube, catheter, or stoma that delivers 4474 * nutrition distal to the oral cavity.) 4475 */ 4476 public NutritionOrderEnteralFormulaComponent getEnteralFormula() { 4477 if (this.enteralFormula == null) 4478 if (Configuration.errorOnAutoCreate()) 4479 throw new Error("Attempt to auto-create NutritionOrder.enteralFormula"); 4480 else if (Configuration.doAutoCreate()) 4481 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); // cc 4482 return this.enteralFormula; 4483 } 4484 4485 public boolean hasEnteralFormula() { 4486 return this.enteralFormula != null && !this.enteralFormula.isEmpty(); 4487 } 4488 4489 /** 4490 * @param value {@link #enteralFormula} (Feeding provided through the 4491 * gastrointestinal tract via a tube, catheter, or stoma that 4492 * delivers nutrition distal to the oral cavity.) 4493 */ 4494 public NutritionOrder setEnteralFormula(NutritionOrderEnteralFormulaComponent value) { 4495 this.enteralFormula = value; 4496 return this; 4497 } 4498 4499 /** 4500 * @return {@link #note} (Comments made about the {{title}} by the requester, 4501 * performer, subject or other participants.) 4502 */ 4503 public List<Annotation> getNote() { 4504 if (this.note == null) 4505 this.note = new ArrayList<Annotation>(); 4506 return this.note; 4507 } 4508 4509 /** 4510 * @return Returns a reference to <code>this</code> for easy method chaining 4511 */ 4512 public NutritionOrder setNote(List<Annotation> theNote) { 4513 this.note = theNote; 4514 return this; 4515 } 4516 4517 public boolean hasNote() { 4518 if (this.note == null) 4519 return false; 4520 for (Annotation item : this.note) 4521 if (!item.isEmpty()) 4522 return true; 4523 return false; 4524 } 4525 4526 public Annotation addNote() { // 3 4527 Annotation t = new Annotation(); 4528 if (this.note == null) 4529 this.note = new ArrayList<Annotation>(); 4530 this.note.add(t); 4531 return t; 4532 } 4533 4534 public NutritionOrder addNote(Annotation t) { // 3 4535 if (t == null) 4536 return this; 4537 if (this.note == null) 4538 this.note = new ArrayList<Annotation>(); 4539 this.note.add(t); 4540 return this; 4541 } 4542 4543 /** 4544 * @return The first repetition of repeating field {@link #note}, creating it if 4545 * it does not already exist 4546 */ 4547 public Annotation getNoteFirstRep() { 4548 if (getNote().isEmpty()) { 4549 addNote(); 4550 } 4551 return getNote().get(0); 4552 } 4553 4554 protected void listChildren(List<Property> children) { 4555 super.listChildren(children); 4556 children.add(new Property("identifier", "Identifier", 4557 "Identifiers assigned to this order by the order sender or by the order receiver.", 0, 4558 java.lang.Integer.MAX_VALUE, identifier)); 4559 children.add(new Property("instantiatesCanonical", "canonical(ActivityDefinition|PlanDefinition)", 4560 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 4561 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 4562 children.add(new Property("instantiatesUri", "uri", 4563 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 4564 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 4565 children.add(new Property("instantiates", "uri", 4566 "The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 4567 0, java.lang.Integer.MAX_VALUE, instantiates)); 4568 children.add(new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 1, status)); 4569 children.add(new Property("intent", "code", 4570 "Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.", 4571 0, 1, intent)); 4572 children.add(new Property("patient", "Reference(Patient)", 4573 "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 4574 0, 1, patient)); 4575 children.add(new Property("encounter", "Reference(Encounter)", 4576 "An encounter that provides additional information about the healthcare context in which this request is made.", 4577 0, 1, encounter)); 4578 children.add(new Property("dateTime", "dateTime", "The date and time that this nutrition order was requested.", 0, 4579 1, dateTime)); 4580 children.add(new Property("orderer", "Reference(Practitioner|PractitionerRole)", 4581 "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 4582 0, 1, orderer)); 4583 children.add(new Property("allergyIntolerance", "Reference(AllergyIntolerance)", 4584 "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, 4585 java.lang.Integer.MAX_VALUE, allergyIntolerance)); 4586 children.add(new Property("foodPreferenceModifier", "CodeableConcept", 4587 "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 4588 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier)); 4589 children.add(new Property("excludeFoodModifier", "CodeableConcept", 4590 "This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 4591 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier)); 4592 children 4593 .add(new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 1, oralDiet)); 4594 children.add(new Property("supplement", "", 4595 "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, 4596 java.lang.Integer.MAX_VALUE, supplement)); 4597 children.add(new Property("enteralFormula", "", 4598 "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 4599 0, 1, enteralFormula)); 4600 children.add(new Property("note", "Annotation", 4601 "Comments made about the {{title}} by the requester, performer, subject or other participants.", 0, 4602 java.lang.Integer.MAX_VALUE, note)); 4603 } 4604 4605 @Override 4606 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4607 switch (_hash) { 4608 case -1618432855: 4609 /* identifier */ return new Property("identifier", "Identifier", 4610 "Identifiers assigned to this order by the order sender or by the order receiver.", 0, 4611 java.lang.Integer.MAX_VALUE, identifier); 4612 case 8911915: 4613 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 4614 "canonical(ActivityDefinition|PlanDefinition)", 4615 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 4616 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 4617 case -1926393373: 4618 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 4619 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 4620 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 4621 case -246883639: 4622 /* instantiates */ return new Property("instantiates", "uri", 4623 "The URL pointing to a protocol, guideline, orderset or other definition that is adhered to in whole or in part by this NutritionOrder.", 4624 0, java.lang.Integer.MAX_VALUE, instantiates); 4625 case -892481550: 4626 /* status */ return new Property("status", "code", "The workflow status of the nutrition order/request.", 0, 1, 4627 status); 4628 case -1183762788: 4629 /* intent */ return new Property("intent", "code", 4630 "Indicates the level of authority/intentionality associated with the NutrionOrder and where the request fits into the workflow chain.", 4631 0, 1, intent); 4632 case -791418107: 4633 /* patient */ return new Property("patient", "Reference(Patient)", 4634 "The person (patient) who needs the nutrition order for an oral diet, nutritional supplement and/or enteral or formula feeding.", 4635 0, 1, patient); 4636 case 1524132147: 4637 /* encounter */ return new Property("encounter", "Reference(Encounter)", 4638 "An encounter that provides additional information about the healthcare context in which this request is made.", 4639 0, 1, encounter); 4640 case 1792749467: 4641 /* dateTime */ return new Property("dateTime", "dateTime", 4642 "The date and time that this nutrition order was requested.", 0, 1, dateTime); 4643 case -1207109509: 4644 /* orderer */ return new Property("orderer", "Reference(Practitioner|PractitionerRole)", 4645 "The practitioner that holds legal responsibility for ordering the diet, nutritional supplement, or formula feedings.", 4646 0, 1, orderer); 4647 case -120164120: 4648 /* allergyIntolerance */ return new Property("allergyIntolerance", "Reference(AllergyIntolerance)", 4649 "A link to a record of allergies or intolerances which should be included in the nutrition order.", 0, 4650 java.lang.Integer.MAX_VALUE, allergyIntolerance); 4651 case 659473872: 4652 /* foodPreferenceModifier */ return new Property("foodPreferenceModifier", "CodeableConcept", 4653 "This modifier is used to convey order-specific modifiers about the type of food that should be given. These can be derived from patient allergies, intolerances, or preferences such as Halal, Vegan or Kosher. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 4654 0, java.lang.Integer.MAX_VALUE, foodPreferenceModifier); 4655 case 1760260175: 4656 /* excludeFoodModifier */ return new Property("excludeFoodModifier", "CodeableConcept", 4657 "This modifier is used to convey Order-specific modifier about the type of oral food or oral fluids that should not be given. These can be derived from patient allergies, intolerances, or preferences such as No Red Meat, No Soy or No Wheat or Gluten-Free. While it should not be necessary to repeat allergy or intolerance information captured in the referenced AllergyIntolerance resource in the excludeFoodModifier, this element may be used to convey additional specificity related to foods that should be eliminated from the patient?s diet for any reason. This modifier applies to the entire nutrition order inclusive of the oral diet, nutritional supplements and enteral formula feedings.", 4658 0, java.lang.Integer.MAX_VALUE, excludeFoodModifier); 4659 case 1153521250: 4660 /* oralDiet */ return new Property("oralDiet", "", "Diet given orally in contrast to enteral (tube) feeding.", 0, 4661 1, oralDiet); 4662 case -711993159: 4663 /* supplement */ return new Property("supplement", "", 4664 "Oral nutritional products given in order to add further nutritional value to the patient's diet.", 0, 4665 java.lang.Integer.MAX_VALUE, supplement); 4666 case -671083805: 4667 /* enteralFormula */ return new Property("enteralFormula", "", 4668 "Feeding provided through the gastrointestinal tract via a tube, catheter, or stoma that delivers nutrition distal to the oral cavity.", 4669 0, 1, enteralFormula); 4670 case 3387378: 4671 /* note */ return new Property("note", "Annotation", 4672 "Comments made about the {{title}} by the requester, performer, subject or other participants.", 0, 4673 java.lang.Integer.MAX_VALUE, note); 4674 default: 4675 return super.getNamedProperty(_hash, _name, _checkValid); 4676 } 4677 4678 } 4679 4680 @Override 4681 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4682 switch (hash) { 4683 case -1618432855: 4684 /* identifier */ return this.identifier == null ? new Base[0] 4685 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4686 case 8911915: 4687 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 4688 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 4689 case -1926393373: 4690 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 4691 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 4692 case -246883639: 4693 /* instantiates */ return this.instantiates == null ? new Base[0] 4694 : this.instantiates.toArray(new Base[this.instantiates.size()]); // UriType 4695 case -892481550: 4696 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<NutritionOrderStatus> 4697 case -1183762788: 4698 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<NutritiionOrderIntent> 4699 case -791418107: 4700 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 4701 case 1524132147: 4702 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 4703 case 1792749467: 4704 /* dateTime */ return this.dateTime == null ? new Base[0] : new Base[] { this.dateTime }; // DateTimeType 4705 case -1207109509: 4706 /* orderer */ return this.orderer == null ? new Base[0] : new Base[] { this.orderer }; // Reference 4707 case -120164120: 4708 /* allergyIntolerance */ return this.allergyIntolerance == null ? new Base[0] 4709 : this.allergyIntolerance.toArray(new Base[this.allergyIntolerance.size()]); // Reference 4710 case 659473872: 4711 /* foodPreferenceModifier */ return this.foodPreferenceModifier == null ? new Base[0] 4712 : this.foodPreferenceModifier.toArray(new Base[this.foodPreferenceModifier.size()]); // CodeableConcept 4713 case 1760260175: 4714 /* excludeFoodModifier */ return this.excludeFoodModifier == null ? new Base[0] 4715 : this.excludeFoodModifier.toArray(new Base[this.excludeFoodModifier.size()]); // CodeableConcept 4716 case 1153521250: 4717 /* oralDiet */ return this.oralDiet == null ? new Base[0] : new Base[] { this.oralDiet }; // NutritionOrderOralDietComponent 4718 case -711993159: 4719 /* supplement */ return this.supplement == null ? new Base[0] 4720 : this.supplement.toArray(new Base[this.supplement.size()]); // NutritionOrderSupplementComponent 4721 case -671083805: 4722 /* enteralFormula */ return this.enteralFormula == null ? new Base[0] : new Base[] { this.enteralFormula }; // NutritionOrderEnteralFormulaComponent 4723 case 3387378: 4724 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4725 default: 4726 return super.getProperty(hash, name, checkValid); 4727 } 4728 4729 } 4730 4731 @Override 4732 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4733 switch (hash) { 4734 case -1618432855: // identifier 4735 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4736 return value; 4737 case 8911915: // instantiatesCanonical 4738 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 4739 return value; 4740 case -1926393373: // instantiatesUri 4741 this.getInstantiatesUri().add(castToUri(value)); // UriType 4742 return value; 4743 case -246883639: // instantiates 4744 this.getInstantiates().add(castToUri(value)); // UriType 4745 return value; 4746 case -892481550: // status 4747 value = new NutritionOrderStatusEnumFactory().fromType(castToCode(value)); 4748 this.status = (Enumeration) value; // Enumeration<NutritionOrderStatus> 4749 return value; 4750 case -1183762788: // intent 4751 value = new NutritiionOrderIntentEnumFactory().fromType(castToCode(value)); 4752 this.intent = (Enumeration) value; // Enumeration<NutritiionOrderIntent> 4753 return value; 4754 case -791418107: // patient 4755 this.patient = castToReference(value); // Reference 4756 return value; 4757 case 1524132147: // encounter 4758 this.encounter = castToReference(value); // Reference 4759 return value; 4760 case 1792749467: // dateTime 4761 this.dateTime = castToDateTime(value); // DateTimeType 4762 return value; 4763 case -1207109509: // orderer 4764 this.orderer = castToReference(value); // Reference 4765 return value; 4766 case -120164120: // allergyIntolerance 4767 this.getAllergyIntolerance().add(castToReference(value)); // Reference 4768 return value; 4769 case 659473872: // foodPreferenceModifier 4770 this.getFoodPreferenceModifier().add(castToCodeableConcept(value)); // CodeableConcept 4771 return value; 4772 case 1760260175: // excludeFoodModifier 4773 this.getExcludeFoodModifier().add(castToCodeableConcept(value)); // CodeableConcept 4774 return value; 4775 case 1153521250: // oralDiet 4776 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 4777 return value; 4778 case -711993159: // supplement 4779 this.getSupplement().add((NutritionOrderSupplementComponent) value); // NutritionOrderSupplementComponent 4780 return value; 4781 case -671083805: // enteralFormula 4782 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 4783 return value; 4784 case 3387378: // note 4785 this.getNote().add(castToAnnotation(value)); // Annotation 4786 return value; 4787 default: 4788 return super.setProperty(hash, name, value); 4789 } 4790 4791 } 4792 4793 @Override 4794 public Base setProperty(String name, Base value) throws FHIRException { 4795 if (name.equals("identifier")) { 4796 this.getIdentifier().add(castToIdentifier(value)); 4797 } else if (name.equals("instantiatesCanonical")) { 4798 this.getInstantiatesCanonical().add(castToCanonical(value)); 4799 } else if (name.equals("instantiatesUri")) { 4800 this.getInstantiatesUri().add(castToUri(value)); 4801 } else if (name.equals("instantiates")) { 4802 this.getInstantiates().add(castToUri(value)); 4803 } else if (name.equals("status")) { 4804 value = new NutritionOrderStatusEnumFactory().fromType(castToCode(value)); 4805 this.status = (Enumeration) value; // Enumeration<NutritionOrderStatus> 4806 } else if (name.equals("intent")) { 4807 value = new NutritiionOrderIntentEnumFactory().fromType(castToCode(value)); 4808 this.intent = (Enumeration) value; // Enumeration<NutritiionOrderIntent> 4809 } else if (name.equals("patient")) { 4810 this.patient = castToReference(value); // Reference 4811 } else if (name.equals("encounter")) { 4812 this.encounter = castToReference(value); // Reference 4813 } else if (name.equals("dateTime")) { 4814 this.dateTime = castToDateTime(value); // DateTimeType 4815 } else if (name.equals("orderer")) { 4816 this.orderer = castToReference(value); // Reference 4817 } else if (name.equals("allergyIntolerance")) { 4818 this.getAllergyIntolerance().add(castToReference(value)); 4819 } else if (name.equals("foodPreferenceModifier")) { 4820 this.getFoodPreferenceModifier().add(castToCodeableConcept(value)); 4821 } else if (name.equals("excludeFoodModifier")) { 4822 this.getExcludeFoodModifier().add(castToCodeableConcept(value)); 4823 } else if (name.equals("oralDiet")) { 4824 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 4825 } else if (name.equals("supplement")) { 4826 this.getSupplement().add((NutritionOrderSupplementComponent) value); 4827 } else if (name.equals("enteralFormula")) { 4828 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 4829 } else if (name.equals("note")) { 4830 this.getNote().add(castToAnnotation(value)); 4831 } else 4832 return super.setProperty(name, value); 4833 return value; 4834 } 4835 4836 @Override 4837 public void removeChild(String name, Base value) throws FHIRException { 4838 if (name.equals("identifier")) { 4839 this.getIdentifier().remove(castToIdentifier(value)); 4840 } else if (name.equals("instantiatesCanonical")) { 4841 this.getInstantiatesCanonical().remove(castToCanonical(value)); 4842 } else if (name.equals("instantiatesUri")) { 4843 this.getInstantiatesUri().remove(castToUri(value)); 4844 } else if (name.equals("instantiates")) { 4845 this.getInstantiates().remove(castToUri(value)); 4846 } else if (name.equals("status")) { 4847 this.status = null; 4848 } else if (name.equals("intent")) { 4849 this.intent = null; 4850 } else if (name.equals("patient")) { 4851 this.patient = null; 4852 } else if (name.equals("encounter")) { 4853 this.encounter = null; 4854 } else if (name.equals("dateTime")) { 4855 this.dateTime = null; 4856 } else if (name.equals("orderer")) { 4857 this.orderer = null; 4858 } else if (name.equals("allergyIntolerance")) { 4859 this.getAllergyIntolerance().remove(castToReference(value)); 4860 } else if (name.equals("foodPreferenceModifier")) { 4861 this.getFoodPreferenceModifier().remove(castToCodeableConcept(value)); 4862 } else if (name.equals("excludeFoodModifier")) { 4863 this.getExcludeFoodModifier().remove(castToCodeableConcept(value)); 4864 } else if (name.equals("oralDiet")) { 4865 this.oralDiet = (NutritionOrderOralDietComponent) value; // NutritionOrderOralDietComponent 4866 } else if (name.equals("supplement")) { 4867 this.getSupplement().remove((NutritionOrderSupplementComponent) value); 4868 } else if (name.equals("enteralFormula")) { 4869 this.enteralFormula = (NutritionOrderEnteralFormulaComponent) value; // NutritionOrderEnteralFormulaComponent 4870 } else if (name.equals("note")) { 4871 this.getNote().remove(castToAnnotation(value)); 4872 } else 4873 super.removeChild(name, value); 4874 4875 } 4876 4877 @Override 4878 public Base makeProperty(int hash, String name) throws FHIRException { 4879 switch (hash) { 4880 case -1618432855: 4881 return addIdentifier(); 4882 case 8911915: 4883 return addInstantiatesCanonicalElement(); 4884 case -1926393373: 4885 return addInstantiatesUriElement(); 4886 case -246883639: 4887 return addInstantiatesElement(); 4888 case -892481550: 4889 return getStatusElement(); 4890 case -1183762788: 4891 return getIntentElement(); 4892 case -791418107: 4893 return getPatient(); 4894 case 1524132147: 4895 return getEncounter(); 4896 case 1792749467: 4897 return getDateTimeElement(); 4898 case -1207109509: 4899 return getOrderer(); 4900 case -120164120: 4901 return addAllergyIntolerance(); 4902 case 659473872: 4903 return addFoodPreferenceModifier(); 4904 case 1760260175: 4905 return addExcludeFoodModifier(); 4906 case 1153521250: 4907 return getOralDiet(); 4908 case -711993159: 4909 return addSupplement(); 4910 case -671083805: 4911 return getEnteralFormula(); 4912 case 3387378: 4913 return addNote(); 4914 default: 4915 return super.makeProperty(hash, name); 4916 } 4917 4918 } 4919 4920 @Override 4921 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4922 switch (hash) { 4923 case -1618432855: 4924 /* identifier */ return new String[] { "Identifier" }; 4925 case 8911915: 4926 /* instantiatesCanonical */ return new String[] { "canonical" }; 4927 case -1926393373: 4928 /* instantiatesUri */ return new String[] { "uri" }; 4929 case -246883639: 4930 /* instantiates */ return new String[] { "uri" }; 4931 case -892481550: 4932 /* status */ return new String[] { "code" }; 4933 case -1183762788: 4934 /* intent */ return new String[] { "code" }; 4935 case -791418107: 4936 /* patient */ return new String[] { "Reference" }; 4937 case 1524132147: 4938 /* encounter */ return new String[] { "Reference" }; 4939 case 1792749467: 4940 /* dateTime */ return new String[] { "dateTime" }; 4941 case -1207109509: 4942 /* orderer */ return new String[] { "Reference" }; 4943 case -120164120: 4944 /* allergyIntolerance */ return new String[] { "Reference" }; 4945 case 659473872: 4946 /* foodPreferenceModifier */ return new String[] { "CodeableConcept" }; 4947 case 1760260175: 4948 /* excludeFoodModifier */ return new String[] { "CodeableConcept" }; 4949 case 1153521250: 4950 /* oralDiet */ return new String[] {}; 4951 case -711993159: 4952 /* supplement */ return new String[] {}; 4953 case -671083805: 4954 /* enteralFormula */ return new String[] {}; 4955 case 3387378: 4956 /* note */ return new String[] { "Annotation" }; 4957 default: 4958 return super.getTypesForProperty(hash, name); 4959 } 4960 4961 } 4962 4963 @Override 4964 public Base addChild(String name) throws FHIRException { 4965 if (name.equals("identifier")) { 4966 return addIdentifier(); 4967 } else if (name.equals("instantiatesCanonical")) { 4968 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instantiatesCanonical"); 4969 } else if (name.equals("instantiatesUri")) { 4970 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instantiatesUri"); 4971 } else if (name.equals("instantiates")) { 4972 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.instantiates"); 4973 } else if (name.equals("status")) { 4974 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.status"); 4975 } else if (name.equals("intent")) { 4976 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.intent"); 4977 } else if (name.equals("patient")) { 4978 this.patient = new Reference(); 4979 return this.patient; 4980 } else if (name.equals("encounter")) { 4981 this.encounter = new Reference(); 4982 return this.encounter; 4983 } else if (name.equals("dateTime")) { 4984 throw new FHIRException("Cannot call addChild on a singleton property NutritionOrder.dateTime"); 4985 } else if (name.equals("orderer")) { 4986 this.orderer = new Reference(); 4987 return this.orderer; 4988 } else if (name.equals("allergyIntolerance")) { 4989 return addAllergyIntolerance(); 4990 } else if (name.equals("foodPreferenceModifier")) { 4991 return addFoodPreferenceModifier(); 4992 } else if (name.equals("excludeFoodModifier")) { 4993 return addExcludeFoodModifier(); 4994 } else if (name.equals("oralDiet")) { 4995 this.oralDiet = new NutritionOrderOralDietComponent(); 4996 return this.oralDiet; 4997 } else if (name.equals("supplement")) { 4998 return addSupplement(); 4999 } else if (name.equals("enteralFormula")) { 5000 this.enteralFormula = new NutritionOrderEnteralFormulaComponent(); 5001 return this.enteralFormula; 5002 } else if (name.equals("note")) { 5003 return addNote(); 5004 } else 5005 return super.addChild(name); 5006 } 5007 5008 public String fhirType() { 5009 return "NutritionOrder"; 5010 5011 } 5012 5013 public NutritionOrder copy() { 5014 NutritionOrder dst = new NutritionOrder(); 5015 copyValues(dst); 5016 return dst; 5017 } 5018 5019 public void copyValues(NutritionOrder dst) { 5020 super.copyValues(dst); 5021 if (identifier != null) { 5022 dst.identifier = new ArrayList<Identifier>(); 5023 for (Identifier i : identifier) 5024 dst.identifier.add(i.copy()); 5025 } 5026 ; 5027 if (instantiatesCanonical != null) { 5028 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 5029 for (CanonicalType i : instantiatesCanonical) 5030 dst.instantiatesCanonical.add(i.copy()); 5031 } 5032 ; 5033 if (instantiatesUri != null) { 5034 dst.instantiatesUri = new ArrayList<UriType>(); 5035 for (UriType i : instantiatesUri) 5036 dst.instantiatesUri.add(i.copy()); 5037 } 5038 ; 5039 if (instantiates != null) { 5040 dst.instantiates = new ArrayList<UriType>(); 5041 for (UriType i : instantiates) 5042 dst.instantiates.add(i.copy()); 5043 } 5044 ; 5045 dst.status = status == null ? null : status.copy(); 5046 dst.intent = intent == null ? null : intent.copy(); 5047 dst.patient = patient == null ? null : patient.copy(); 5048 dst.encounter = encounter == null ? null : encounter.copy(); 5049 dst.dateTime = dateTime == null ? null : dateTime.copy(); 5050 dst.orderer = orderer == null ? null : orderer.copy(); 5051 if (allergyIntolerance != null) { 5052 dst.allergyIntolerance = new ArrayList<Reference>(); 5053 for (Reference i : allergyIntolerance) 5054 dst.allergyIntolerance.add(i.copy()); 5055 } 5056 ; 5057 if (foodPreferenceModifier != null) { 5058 dst.foodPreferenceModifier = new ArrayList<CodeableConcept>(); 5059 for (CodeableConcept i : foodPreferenceModifier) 5060 dst.foodPreferenceModifier.add(i.copy()); 5061 } 5062 ; 5063 if (excludeFoodModifier != null) { 5064 dst.excludeFoodModifier = new ArrayList<CodeableConcept>(); 5065 for (CodeableConcept i : excludeFoodModifier) 5066 dst.excludeFoodModifier.add(i.copy()); 5067 } 5068 ; 5069 dst.oralDiet = oralDiet == null ? null : oralDiet.copy(); 5070 if (supplement != null) { 5071 dst.supplement = new ArrayList<NutritionOrderSupplementComponent>(); 5072 for (NutritionOrderSupplementComponent i : supplement) 5073 dst.supplement.add(i.copy()); 5074 } 5075 ; 5076 dst.enteralFormula = enteralFormula == null ? null : enteralFormula.copy(); 5077 if (note != null) { 5078 dst.note = new ArrayList<Annotation>(); 5079 for (Annotation i : note) 5080 dst.note.add(i.copy()); 5081 } 5082 ; 5083 } 5084 5085 protected NutritionOrder typedCopy() { 5086 return copy(); 5087 } 5088 5089 @Override 5090 public boolean equalsDeep(Base other_) { 5091 if (!super.equalsDeep(other_)) 5092 return false; 5093 if (!(other_ instanceof NutritionOrder)) 5094 return false; 5095 NutritionOrder o = (NutritionOrder) other_; 5096 return compareDeep(identifier, o.identifier, true) 5097 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 5098 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(instantiates, o.instantiates, true) 5099 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) 5100 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) 5101 && compareDeep(dateTime, o.dateTime, true) && compareDeep(orderer, o.orderer, true) 5102 && compareDeep(allergyIntolerance, o.allergyIntolerance, true) 5103 && compareDeep(foodPreferenceModifier, o.foodPreferenceModifier, true) 5104 && compareDeep(excludeFoodModifier, o.excludeFoodModifier, true) && compareDeep(oralDiet, o.oralDiet, true) 5105 && compareDeep(supplement, o.supplement, true) && compareDeep(enteralFormula, o.enteralFormula, true) 5106 && compareDeep(note, o.note, true); 5107 } 5108 5109 @Override 5110 public boolean equalsShallow(Base other_) { 5111 if (!super.equalsShallow(other_)) 5112 return false; 5113 if (!(other_ instanceof NutritionOrder)) 5114 return false; 5115 NutritionOrder o = (NutritionOrder) other_; 5116 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(instantiates, o.instantiates, true) 5117 && compareValues(status, o.status, true) && compareValues(intent, o.intent, true) 5118 && compareValues(dateTime, o.dateTime, true); 5119 } 5120 5121 public boolean isEmpty() { 5122 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 5123 instantiates, status, intent, patient, encounter, dateTime, orderer, allergyIntolerance, foodPreferenceModifier, 5124 excludeFoodModifier, oralDiet, supplement, enteralFormula, note); 5125 } 5126 5127 @Override 5128 public ResourceType getResourceType() { 5129 return ResourceType.NutritionOrder; 5130 } 5131 5132 /** 5133 * Search parameter: <b>identifier</b> 5134 * <p> 5135 * Description: <b>Return nutrition orders with this external identifier</b><br> 5136 * Type: <b>token</b><br> 5137 * Path: <b>NutritionOrder.identifier</b><br> 5138 * </p> 5139 */ 5140 @SearchParamDefinition(name = "identifier", path = "NutritionOrder.identifier", description = "Return nutrition orders with this external identifier", type = "token") 5141 public static final String SP_IDENTIFIER = "identifier"; 5142 /** 5143 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5144 * <p> 5145 * Description: <b>Return nutrition orders with this external identifier</b><br> 5146 * Type: <b>token</b><br> 5147 * Path: <b>NutritionOrder.identifier</b><br> 5148 * </p> 5149 */ 5150 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5151 SP_IDENTIFIER); 5152 5153 /** 5154 * Search parameter: <b>datetime</b> 5155 * <p> 5156 * Description: <b>Return nutrition orders requested on this date</b><br> 5157 * Type: <b>date</b><br> 5158 * Path: <b>NutritionOrder.dateTime</b><br> 5159 * </p> 5160 */ 5161 @SearchParamDefinition(name = "datetime", path = "NutritionOrder.dateTime", description = "Return nutrition orders requested on this date", type = "date") 5162 public static final String SP_DATETIME = "datetime"; 5163 /** 5164 * <b>Fluent Client</b> search parameter constant for <b>datetime</b> 5165 * <p> 5166 * Description: <b>Return nutrition orders requested on this date</b><br> 5167 * Type: <b>date</b><br> 5168 * Path: <b>NutritionOrder.dateTime</b><br> 5169 * </p> 5170 */ 5171 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATETIME = new ca.uhn.fhir.rest.gclient.DateClientParam( 5172 SP_DATETIME); 5173 5174 /** 5175 * Search parameter: <b>provider</b> 5176 * <p> 5177 * Description: <b>The identity of the provider who placed the nutrition 5178 * order</b><br> 5179 * Type: <b>reference</b><br> 5180 * Path: <b>NutritionOrder.orderer</b><br> 5181 * </p> 5182 */ 5183 @SearchParamDefinition(name = "provider", path = "NutritionOrder.orderer", description = "The identity of the provider who placed the nutrition order", type = "reference", providesMembershipIn = { 5184 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class, 5185 PractitionerRole.class }) 5186 public static final String SP_PROVIDER = "provider"; 5187 /** 5188 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 5189 * <p> 5190 * Description: <b>The identity of the provider who placed the nutrition 5191 * order</b><br> 5192 * Type: <b>reference</b><br> 5193 * Path: <b>NutritionOrder.orderer</b><br> 5194 * </p> 5195 */ 5196 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5197 SP_PROVIDER); 5198 5199 /** 5200 * Constant for fluent queries to be used to add include statements. Specifies 5201 * the path value of "<b>NutritionOrder:provider</b>". 5202 */ 5203 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include( 5204 "NutritionOrder:provider").toLocked(); 5205 5206 /** 5207 * Search parameter: <b>patient</b> 5208 * <p> 5209 * Description: <b>The identity of the person who requires the diet, formula or 5210 * nutritional supplement</b><br> 5211 * Type: <b>reference</b><br> 5212 * Path: <b>NutritionOrder.patient</b><br> 5213 * </p> 5214 */ 5215 @SearchParamDefinition(name = "patient", path = "NutritionOrder.patient", description = "The identity of the person who requires the diet, formula or nutritional supplement", type = "reference", providesMembershipIn = { 5216 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 5217 public static final String SP_PATIENT = "patient"; 5218 /** 5219 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5220 * <p> 5221 * Description: <b>The identity of the person who requires the diet, formula or 5222 * nutritional supplement</b><br> 5223 * Type: <b>reference</b><br> 5224 * Path: <b>NutritionOrder.patient</b><br> 5225 * </p> 5226 */ 5227 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5228 SP_PATIENT); 5229 5230 /** 5231 * Constant for fluent queries to be used to add include statements. Specifies 5232 * the path value of "<b>NutritionOrder:patient</b>". 5233 */ 5234 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 5235 "NutritionOrder:patient").toLocked(); 5236 5237 /** 5238 * Search parameter: <b>supplement</b> 5239 * <p> 5240 * Description: <b>Type of supplement product requested</b><br> 5241 * Type: <b>token</b><br> 5242 * Path: <b>NutritionOrder.supplement.type</b><br> 5243 * </p> 5244 */ 5245 @SearchParamDefinition(name = "supplement", path = "NutritionOrder.supplement.type", description = "Type of supplement product requested", type = "token") 5246 public static final String SP_SUPPLEMENT = "supplement"; 5247 /** 5248 * <b>Fluent Client</b> search parameter constant for <b>supplement</b> 5249 * <p> 5250 * Description: <b>Type of supplement product requested</b><br> 5251 * Type: <b>token</b><br> 5252 * Path: <b>NutritionOrder.supplement.type</b><br> 5253 * </p> 5254 */ 5255 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUPPLEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5256 SP_SUPPLEMENT); 5257 5258 /** 5259 * Search parameter: <b>formula</b> 5260 * <p> 5261 * Description: <b>Type of enteral or infant formula</b><br> 5262 * Type: <b>token</b><br> 5263 * Path: <b>NutritionOrder.enteralFormula.baseFormulaType</b><br> 5264 * </p> 5265 */ 5266 @SearchParamDefinition(name = "formula", path = "NutritionOrder.enteralFormula.baseFormulaType", description = "Type of enteral or infant formula", type = "token") 5267 public static final String SP_FORMULA = "formula"; 5268 /** 5269 * <b>Fluent Client</b> search parameter constant for <b>formula</b> 5270 * <p> 5271 * Description: <b>Type of enteral or infant formula</b><br> 5272 * Type: <b>token</b><br> 5273 * Path: <b>NutritionOrder.enteralFormula.baseFormulaType</b><br> 5274 * </p> 5275 */ 5276 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORMULA = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5277 SP_FORMULA); 5278 5279 /** 5280 * Search parameter: <b>instantiates-canonical</b> 5281 * <p> 5282 * Description: <b>Instantiates FHIR protocol or definition</b><br> 5283 * Type: <b>reference</b><br> 5284 * Path: <b>NutritionOrder.instantiatesCanonical</b><br> 5285 * </p> 5286 */ 5287 @SearchParamDefinition(name = "instantiates-canonical", path = "NutritionOrder.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 5288 ActivityDefinition.class, PlanDefinition.class }) 5289 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 5290 /** 5291 * <b>Fluent Client</b> search parameter constant for 5292 * <b>instantiates-canonical</b> 5293 * <p> 5294 * Description: <b>Instantiates FHIR protocol or definition</b><br> 5295 * Type: <b>reference</b><br> 5296 * Path: <b>NutritionOrder.instantiatesCanonical</b><br> 5297 * </p> 5298 */ 5299 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5300 SP_INSTANTIATES_CANONICAL); 5301 5302 /** 5303 * Constant for fluent queries to be used to add include statements. Specifies 5304 * the path value of "<b>NutritionOrder:instantiates-canonical</b>". 5305 */ 5306 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 5307 "NutritionOrder:instantiates-canonical").toLocked(); 5308 5309 /** 5310 * Search parameter: <b>instantiates-uri</b> 5311 * <p> 5312 * Description: <b>Instantiates external protocol or definition</b><br> 5313 * Type: <b>uri</b><br> 5314 * Path: <b>NutritionOrder.instantiatesUri</b><br> 5315 * </p> 5316 */ 5317 @SearchParamDefinition(name = "instantiates-uri", path = "NutritionOrder.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 5318 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 5319 /** 5320 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 5321 * <p> 5322 * Description: <b>Instantiates external protocol or definition</b><br> 5323 * Type: <b>uri</b><br> 5324 * Path: <b>NutritionOrder.instantiatesUri</b><br> 5325 * </p> 5326 */ 5327 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 5328 SP_INSTANTIATES_URI); 5329 5330 /** 5331 * Search parameter: <b>encounter</b> 5332 * <p> 5333 * Description: <b>Return nutrition orders with this encounter 5334 * identifier</b><br> 5335 * Type: <b>reference</b><br> 5336 * Path: <b>NutritionOrder.encounter</b><br> 5337 * </p> 5338 */ 5339 @SearchParamDefinition(name = "encounter", path = "NutritionOrder.encounter", description = "Return nutrition orders with this encounter identifier", type = "reference", providesMembershipIn = { 5340 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 5341 public static final String SP_ENCOUNTER = "encounter"; 5342 /** 5343 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5344 * <p> 5345 * Description: <b>Return nutrition orders with this encounter 5346 * identifier</b><br> 5347 * Type: <b>reference</b><br> 5348 * Path: <b>NutritionOrder.encounter</b><br> 5349 * </p> 5350 */ 5351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5352 SP_ENCOUNTER); 5353 5354 /** 5355 * Constant for fluent queries to be used to add include statements. Specifies 5356 * the path value of "<b>NutritionOrder:encounter</b>". 5357 */ 5358 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5359 "NutritionOrder:encounter").toLocked(); 5360 5361 /** 5362 * Search parameter: <b>oraldiet</b> 5363 * <p> 5364 * Description: <b>Type of diet that can be consumed orally (i.e., take via the 5365 * mouth).</b><br> 5366 * Type: <b>token</b><br> 5367 * Path: <b>NutritionOrder.oralDiet.type</b><br> 5368 * </p> 5369 */ 5370 @SearchParamDefinition(name = "oraldiet", path = "NutritionOrder.oralDiet.type", description = "Type of diet that can be consumed orally (i.e., take via the mouth).", type = "token") 5371 public static final String SP_ORALDIET = "oraldiet"; 5372 /** 5373 * <b>Fluent Client</b> search parameter constant for <b>oraldiet</b> 5374 * <p> 5375 * Description: <b>Type of diet that can be consumed orally (i.e., take via the 5376 * mouth).</b><br> 5377 * Type: <b>token</b><br> 5378 * Path: <b>NutritionOrder.oralDiet.type</b><br> 5379 * </p> 5380 */ 5381 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ORALDIET = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5382 SP_ORALDIET); 5383 5384 /** 5385 * Search parameter: <b>status</b> 5386 * <p> 5387 * Description: <b>Status of the nutrition order.</b><br> 5388 * Type: <b>token</b><br> 5389 * Path: <b>NutritionOrder.status</b><br> 5390 * </p> 5391 */ 5392 @SearchParamDefinition(name = "status", path = "NutritionOrder.status", description = "Status of the nutrition order.", type = "token") 5393 public static final String SP_STATUS = "status"; 5394 /** 5395 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5396 * <p> 5397 * Description: <b>Status of the nutrition order.</b><br> 5398 * Type: <b>token</b><br> 5399 * Path: <b>NutritionOrder.status</b><br> 5400 * </p> 5401 */ 5402 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5403 SP_STATUS); 5404 5405 /** 5406 * Search parameter: <b>additive</b> 5407 * <p> 5408 * Description: <b>Type of module component to add to the feeding</b><br> 5409 * Type: <b>token</b><br> 5410 * Path: <b>NutritionOrder.enteralFormula.additiveType</b><br> 5411 * </p> 5412 */ 5413 @SearchParamDefinition(name = "additive", path = "NutritionOrder.enteralFormula.additiveType", description = "Type of module component to add to the feeding", type = "token") 5414 public static final String SP_ADDITIVE = "additive"; 5415 /** 5416 * <b>Fluent Client</b> search parameter constant for <b>additive</b> 5417 * <p> 5418 * Description: <b>Type of module component to add to the feeding</b><br> 5419 * Type: <b>token</b><br> 5420 * Path: <b>NutritionOrder.enteralFormula.additiveType</b><br> 5421 * </p> 5422 */ 5423 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDITIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5424 SP_ADDITIVE); 5425 5426}