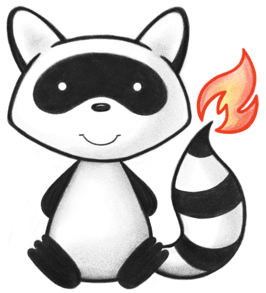
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Measurements and simple assertions made about a patient, device or other 049 * subject. 050 */ 051@ResourceDef(name = "Observation", profile = "http://hl7.org/fhir/StructureDefinition/Observation") 052public class Observation extends DomainResource { 053 054 public enum ObservationStatus { 055 /** 056 * The existence of the observation is registered, but there is no result yet 057 * available. 058 */ 059 REGISTERED, 060 /** 061 * This is an initial or interim observation: data may be incomplete or 062 * unverified. 063 */ 064 PRELIMINARY, 065 /** 066 * The observation is complete and there are no further actions needed. 067 * Additional information such "released", "signed", etc would be represented 068 * using [Provenance](provenance.html) which provides not only the act but also 069 * the actors and dates and other related data. These act states would be 070 * associated with an observation status of `preliminary` until they are all 071 * completed and then a status of `final` would be applied. 072 */ 073 FINAL, 074 /** 075 * Subsequent to being Final, the observation has been modified subsequent. This 076 * includes updates/new information and corrections. 077 */ 078 AMENDED, 079 /** 080 * Subsequent to being Final, the observation has been modified to correct an 081 * error in the test result. 082 */ 083 CORRECTED, 084 /** 085 * The observation is unavailable because the measurement was not started or not 086 * completed (also sometimes called "aborted"). 087 */ 088 CANCELLED, 089 /** 090 * The observation has been withdrawn following previous final release. This 091 * electronic record should never have existed, though it is possible that 092 * real-world decisions were based on it. (If real-world activity has occurred, 093 * the status should be "cancelled" rather than "entered-in-error".). 094 */ 095 ENTEREDINERROR, 096 /** 097 * The authoring/source system does not know which of the status values 098 * currently applies for this observation. Note: This concept is not to be used 099 * for "other" - one of the listed statuses is presumed to apply, but the 100 * authoring/source system does not know which. 101 */ 102 UNKNOWN, 103 /** 104 * added to help the parsers with the generic types 105 */ 106 NULL; 107 108 public static ObservationStatus fromCode(String codeString) throws FHIRException { 109 if (codeString == null || "".equals(codeString)) 110 return null; 111 if ("registered".equals(codeString)) 112 return REGISTERED; 113 if ("preliminary".equals(codeString)) 114 return PRELIMINARY; 115 if ("final".equals(codeString)) 116 return FINAL; 117 if ("amended".equals(codeString)) 118 return AMENDED; 119 if ("corrected".equals(codeString)) 120 return CORRECTED; 121 if ("cancelled".equals(codeString)) 122 return CANCELLED; 123 if ("entered-in-error".equals(codeString)) 124 return ENTEREDINERROR; 125 if ("unknown".equals(codeString)) 126 return UNKNOWN; 127 if (Configuration.isAcceptInvalidEnums()) 128 return null; 129 else 130 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 131 } 132 133 public String toCode() { 134 switch (this) { 135 case REGISTERED: 136 return "registered"; 137 case PRELIMINARY: 138 return "preliminary"; 139 case FINAL: 140 return "final"; 141 case AMENDED: 142 return "amended"; 143 case CORRECTED: 144 return "corrected"; 145 case CANCELLED: 146 return "cancelled"; 147 case ENTEREDINERROR: 148 return "entered-in-error"; 149 case UNKNOWN: 150 return "unknown"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getSystem() { 159 switch (this) { 160 case REGISTERED: 161 return "http://hl7.org/fhir/observation-status"; 162 case PRELIMINARY: 163 return "http://hl7.org/fhir/observation-status"; 164 case FINAL: 165 return "http://hl7.org/fhir/observation-status"; 166 case AMENDED: 167 return "http://hl7.org/fhir/observation-status"; 168 case CORRECTED: 169 return "http://hl7.org/fhir/observation-status"; 170 case CANCELLED: 171 return "http://hl7.org/fhir/observation-status"; 172 case ENTEREDINERROR: 173 return "http://hl7.org/fhir/observation-status"; 174 case UNKNOWN: 175 return "http://hl7.org/fhir/observation-status"; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183 public String getDefinition() { 184 switch (this) { 185 case REGISTERED: 186 return "The existence of the observation is registered, but there is no result yet available."; 187 case PRELIMINARY: 188 return "This is an initial or interim observation: data may be incomplete or unverified."; 189 case FINAL: 190 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 191 case AMENDED: 192 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 193 case CORRECTED: 194 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 195 case CANCELLED: 196 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 197 case ENTEREDINERROR: 198 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 199 case UNKNOWN: 200 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getDisplay() { 209 switch (this) { 210 case REGISTERED: 211 return "Registered"; 212 case PRELIMINARY: 213 return "Preliminary"; 214 case FINAL: 215 return "Final"; 216 case AMENDED: 217 return "Amended"; 218 case CORRECTED: 219 return "Corrected"; 220 case CANCELLED: 221 return "Cancelled"; 222 case ENTEREDINERROR: 223 return "Entered in Error"; 224 case UNKNOWN: 225 return "Unknown"; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 } 233 234 public static class ObservationStatusEnumFactory implements EnumFactory<ObservationStatus> { 235 public ObservationStatus fromCode(String codeString) throws IllegalArgumentException { 236 if (codeString == null || "".equals(codeString)) 237 if (codeString == null || "".equals(codeString)) 238 return null; 239 if ("registered".equals(codeString)) 240 return ObservationStatus.REGISTERED; 241 if ("preliminary".equals(codeString)) 242 return ObservationStatus.PRELIMINARY; 243 if ("final".equals(codeString)) 244 return ObservationStatus.FINAL; 245 if ("amended".equals(codeString)) 246 return ObservationStatus.AMENDED; 247 if ("corrected".equals(codeString)) 248 return ObservationStatus.CORRECTED; 249 if ("cancelled".equals(codeString)) 250 return ObservationStatus.CANCELLED; 251 if ("entered-in-error".equals(codeString)) 252 return ObservationStatus.ENTEREDINERROR; 253 if ("unknown".equals(codeString)) 254 return ObservationStatus.UNKNOWN; 255 throw new IllegalArgumentException("Unknown ObservationStatus code '" + codeString + "'"); 256 } 257 258 public Enumeration<ObservationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 259 if (code == null) 260 return null; 261 if (code.isEmpty()) 262 return new Enumeration<ObservationStatus>(this, ObservationStatus.NULL, code); 263 String codeString = code.asStringValue(); 264 if (codeString == null || "".equals(codeString)) 265 return new Enumeration<ObservationStatus>(this, ObservationStatus.NULL, code); 266 if ("registered".equals(codeString)) 267 return new Enumeration<ObservationStatus>(this, ObservationStatus.REGISTERED, code); 268 if ("preliminary".equals(codeString)) 269 return new Enumeration<ObservationStatus>(this, ObservationStatus.PRELIMINARY, code); 270 if ("final".equals(codeString)) 271 return new Enumeration<ObservationStatus>(this, ObservationStatus.FINAL, code); 272 if ("amended".equals(codeString)) 273 return new Enumeration<ObservationStatus>(this, ObservationStatus.AMENDED, code); 274 if ("corrected".equals(codeString)) 275 return new Enumeration<ObservationStatus>(this, ObservationStatus.CORRECTED, code); 276 if ("cancelled".equals(codeString)) 277 return new Enumeration<ObservationStatus>(this, ObservationStatus.CANCELLED, code); 278 if ("entered-in-error".equals(codeString)) 279 return new Enumeration<ObservationStatus>(this, ObservationStatus.ENTEREDINERROR, code); 280 if ("unknown".equals(codeString)) 281 return new Enumeration<ObservationStatus>(this, ObservationStatus.UNKNOWN, code); 282 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 283 } 284 285 public String toCode(ObservationStatus code) { 286 if (code == ObservationStatus.REGISTERED) 287 return "registered"; 288 if (code == ObservationStatus.PRELIMINARY) 289 return "preliminary"; 290 if (code == ObservationStatus.FINAL) 291 return "final"; 292 if (code == ObservationStatus.AMENDED) 293 return "amended"; 294 if (code == ObservationStatus.CORRECTED) 295 return "corrected"; 296 if (code == ObservationStatus.CANCELLED) 297 return "cancelled"; 298 if (code == ObservationStatus.ENTEREDINERROR) 299 return "entered-in-error"; 300 if (code == ObservationStatus.UNKNOWN) 301 return "unknown"; 302 return "?"; 303 } 304 305 public String toSystem(ObservationStatus code) { 306 return code.getSystem(); 307 } 308 } 309 310 @Block() 311 public static class ObservationReferenceRangeComponent extends BackboneElement implements IBaseBackboneElement { 312 /** 313 * The value of the low bound of the reference range. The low bound of the 314 * reference range endpoint is inclusive of the value (e.g. reference range is 315 * >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless 316 * (e.g. reference range is <=2.3). 317 */ 318 @Child(name = "low", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 319 @Description(shortDefinition = "Low Range, if relevant", formalDefinition = "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).") 320 protected Quantity low; 321 322 /** 323 * The value of the high bound of the reference range. The high bound of the 324 * reference range endpoint is inclusive of the value (e.g. reference range is 325 * >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless 326 * (e.g. reference range is >= 2.3). 327 */ 328 @Child(name = "high", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 329 @Description(shortDefinition = "High Range, if relevant", formalDefinition = "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).") 330 protected Quantity high; 331 332 /** 333 * Codes to indicate the what part of the targeted reference population it 334 * applies to. For example, the normal or therapeutic range. 335 */ 336 @Child(name = "type", type = { 337 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 338 @Description(shortDefinition = "Reference range qualifier", formalDefinition = "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.") 339 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/referencerange-meaning") 340 protected CodeableConcept type; 341 342 /** 343 * Codes to indicate the target population this reference range applies to. For 344 * example, a reference range may be based on the normal population or a 345 * particular sex or race. Multiple `appliesTo` are interpreted as an "AND" of 346 * the target populations. For example, to represent a target population of 347 * African American females, both a code of female and a code for African 348 * American would be used. 349 */ 350 @Child(name = "appliesTo", type = { 351 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 352 @Description(shortDefinition = "Reference range population", formalDefinition = "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.") 353 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/referencerange-appliesto") 354 protected List<CodeableConcept> appliesTo; 355 356 /** 357 * The age at which this reference range is applicable. This is a neonatal age 358 * (e.g. number of weeks at term) if the meaning says so. 359 */ 360 @Child(name = "age", type = { Range.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 361 @Description(shortDefinition = "Applicable age range, if relevant", formalDefinition = "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.") 362 protected Range age; 363 364 /** 365 * Text based reference range in an observation which may be used when a 366 * quantitative range is not appropriate for an observation. An example would be 367 * a reference value of "Negative" or a list or table of "normals". 368 */ 369 @Child(name = "text", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 370 @Description(shortDefinition = "Text based reference range in an observation", formalDefinition = "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".") 371 protected StringType text; 372 373 private static final long serialVersionUID = -305128879L; 374 375 /** 376 * Constructor 377 */ 378 public ObservationReferenceRangeComponent() { 379 super(); 380 } 381 382 /** 383 * @return {@link #low} (The value of the low bound of the reference range. The 384 * low bound of the reference range endpoint is inclusive of the value 385 * (e.g. reference range is >=5 - <=9). If the low bound is omitted, it 386 * is assumed to be meaningless (e.g. reference range is <=2.3).) 387 */ 388 public Quantity getLow() { 389 if (this.low == null) 390 if (Configuration.errorOnAutoCreate()) 391 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.low"); 392 else if (Configuration.doAutoCreate()) 393 this.low = new Quantity(); // cc 394 return this.low; 395 } 396 397 public boolean hasLow() { 398 return this.low != null && !this.low.isEmpty(); 399 } 400 401 /** 402 * @param value {@link #low} (The value of the low bound of the reference range. 403 * The low bound of the reference range endpoint is inclusive of 404 * the value (e.g. reference range is >=5 - <=9). If the low bound 405 * is omitted, it is assumed to be meaningless (e.g. reference 406 * range is <=2.3).) 407 */ 408 public ObservationReferenceRangeComponent setLow(Quantity value) { 409 this.low = value; 410 return this; 411 } 412 413 /** 414 * @return {@link #high} (The value of the high bound of the reference range. 415 * The high bound of the reference range endpoint is inclusive of the 416 * value (e.g. reference range is >=5 - <=9). If the high bound is 417 * omitted, it is assumed to be meaningless (e.g. reference range is >= 418 * 2.3).) 419 */ 420 public Quantity getHigh() { 421 if (this.high == null) 422 if (Configuration.errorOnAutoCreate()) 423 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.high"); 424 else if (Configuration.doAutoCreate()) 425 this.high = new Quantity(); // cc 426 return this.high; 427 } 428 429 public boolean hasHigh() { 430 return this.high != null && !this.high.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #high} (The value of the high bound of the reference 435 * range. The high bound of the reference range endpoint is 436 * inclusive of the value (e.g. reference range is >=5 - <=9). If 437 * the high bound is omitted, it is assumed to be meaningless (e.g. 438 * reference range is >= 2.3).) 439 */ 440 public ObservationReferenceRangeComponent setHigh(Quantity value) { 441 this.high = value; 442 return this; 443 } 444 445 /** 446 * @return {@link #type} (Codes to indicate the what part of the targeted 447 * reference population it applies to. For example, the normal or 448 * therapeutic range.) 449 */ 450 public CodeableConcept getType() { 451 if (this.type == null) 452 if (Configuration.errorOnAutoCreate()) 453 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.type"); 454 else if (Configuration.doAutoCreate()) 455 this.type = new CodeableConcept(); // cc 456 return this.type; 457 } 458 459 public boolean hasType() { 460 return this.type != null && !this.type.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #type} (Codes to indicate the what part of the targeted 465 * reference population it applies to. For example, the normal or 466 * therapeutic range.) 467 */ 468 public ObservationReferenceRangeComponent setType(CodeableConcept value) { 469 this.type = value; 470 return this; 471 } 472 473 /** 474 * @return {@link #appliesTo} (Codes to indicate the target population this 475 * reference range applies to. For example, a reference range may be 476 * based on the normal population or a particular sex or race. Multiple 477 * `appliesTo` are interpreted as an "AND" of the target populations. 478 * For example, to represent a target population of African American 479 * females, both a code of female and a code for African American would 480 * be used.) 481 */ 482 public List<CodeableConcept> getAppliesTo() { 483 if (this.appliesTo == null) 484 this.appliesTo = new ArrayList<CodeableConcept>(); 485 return this.appliesTo; 486 } 487 488 /** 489 * @return Returns a reference to <code>this</code> for easy method chaining 490 */ 491 public ObservationReferenceRangeComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 492 this.appliesTo = theAppliesTo; 493 return this; 494 } 495 496 public boolean hasAppliesTo() { 497 if (this.appliesTo == null) 498 return false; 499 for (CodeableConcept item : this.appliesTo) 500 if (!item.isEmpty()) 501 return true; 502 return false; 503 } 504 505 public CodeableConcept addAppliesTo() { // 3 506 CodeableConcept t = new CodeableConcept(); 507 if (this.appliesTo == null) 508 this.appliesTo = new ArrayList<CodeableConcept>(); 509 this.appliesTo.add(t); 510 return t; 511 } 512 513 public ObservationReferenceRangeComponent addAppliesTo(CodeableConcept t) { // 3 514 if (t == null) 515 return this; 516 if (this.appliesTo == null) 517 this.appliesTo = new ArrayList<CodeableConcept>(); 518 this.appliesTo.add(t); 519 return this; 520 } 521 522 /** 523 * @return The first repetition of repeating field {@link #appliesTo}, creating 524 * it if it does not already exist 525 */ 526 public CodeableConcept getAppliesToFirstRep() { 527 if (getAppliesTo().isEmpty()) { 528 addAppliesTo(); 529 } 530 return getAppliesTo().get(0); 531 } 532 533 /** 534 * @return {@link #age} (The age at which this reference range is applicable. 535 * This is a neonatal age (e.g. number of weeks at term) if the meaning 536 * says so.) 537 */ 538 public Range getAge() { 539 if (this.age == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.age"); 542 else if (Configuration.doAutoCreate()) 543 this.age = new Range(); // cc 544 return this.age; 545 } 546 547 public boolean hasAge() { 548 return this.age != null && !this.age.isEmpty(); 549 } 550 551 /** 552 * @param value {@link #age} (The age at which this reference range is 553 * applicable. This is a neonatal age (e.g. number of weeks at 554 * term) if the meaning says so.) 555 */ 556 public ObservationReferenceRangeComponent setAge(Range value) { 557 this.age = value; 558 return this; 559 } 560 561 /** 562 * @return {@link #text} (Text based reference range in an observation which may 563 * be used when a quantitative range is not appropriate for an 564 * observation. An example would be a reference value of "Negative" or a 565 * list or table of "normals".). This is the underlying object with id, 566 * value and extensions. The accessor "getText" gives direct access to 567 * the value 568 */ 569 public StringType getTextElement() { 570 if (this.text == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.text"); 573 else if (Configuration.doAutoCreate()) 574 this.text = new StringType(); // bb 575 return this.text; 576 } 577 578 public boolean hasTextElement() { 579 return this.text != null && !this.text.isEmpty(); 580 } 581 582 public boolean hasText() { 583 return this.text != null && !this.text.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #text} (Text based reference range in an observation 588 * which may be used when a quantitative range is not appropriate 589 * for an observation. An example would be a reference value of 590 * "Negative" or a list or table of "normals".). This is the 591 * underlying object with id, value and extensions. The accessor 592 * "getText" gives direct access to the value 593 */ 594 public ObservationReferenceRangeComponent setTextElement(StringType value) { 595 this.text = value; 596 return this; 597 } 598 599 /** 600 * @return Text based reference range in an observation which may be used when a 601 * quantitative range is not appropriate for an observation. An example 602 * would be a reference value of "Negative" or a list or table of 603 * "normals". 604 */ 605 public String getText() { 606 return this.text == null ? null : this.text.getValue(); 607 } 608 609 /** 610 * @param value Text based reference range in an observation which may be used 611 * when a quantitative range is not appropriate for an observation. 612 * An example would be a reference value of "Negative" or a list or 613 * table of "normals". 614 */ 615 public ObservationReferenceRangeComponent setText(String value) { 616 if (Utilities.noString(value)) 617 this.text = null; 618 else { 619 if (this.text == null) 620 this.text = new StringType(); 621 this.text.setValue(value); 622 } 623 return this; 624 } 625 626 protected void listChildren(List<Property> children) { 627 super.listChildren(children); 628 children.add(new Property("low", "SimpleQuantity", 629 "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 630 0, 1, low)); 631 children.add(new Property("high", "SimpleQuantity", 632 "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 633 0, 1, high)); 634 children.add(new Property("type", "CodeableConcept", 635 "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 636 0, 1, type)); 637 children.add(new Property("appliesTo", "CodeableConcept", 638 "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 639 0, java.lang.Integer.MAX_VALUE, appliesTo)); 640 children.add(new Property("age", "Range", 641 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 642 0, 1, age)); 643 children.add(new Property("text", "string", 644 "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 645 0, 1, text)); 646 } 647 648 @Override 649 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 650 switch (_hash) { 651 case 107348: 652 /* low */ return new Property("low", "SimpleQuantity", 653 "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 654 0, 1, low); 655 case 3202466: 656 /* high */ return new Property("high", "SimpleQuantity", 657 "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 658 0, 1, high); 659 case 3575610: 660 /* type */ return new Property("type", "CodeableConcept", 661 "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 662 0, 1, type); 663 case -2089924569: 664 /* appliesTo */ return new Property("appliesTo", "CodeableConcept", 665 "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 666 0, java.lang.Integer.MAX_VALUE, appliesTo); 667 case 96511: 668 /* age */ return new Property("age", "Range", 669 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 670 0, 1, age); 671 case 3556653: 672 /* text */ return new Property("text", "string", 673 "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 674 0, 1, text); 675 default: 676 return super.getNamedProperty(_hash, _name, _checkValid); 677 } 678 679 } 680 681 @Override 682 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 683 switch (hash) { 684 case 107348: 685 /* low */ return this.low == null ? new Base[0] : new Base[] { this.low }; // Quantity 686 case 3202466: 687 /* high */ return this.high == null ? new Base[0] : new Base[] { this.high }; // Quantity 688 case 3575610: 689 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 690 case -2089924569: 691 /* appliesTo */ return this.appliesTo == null ? new Base[0] 692 : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 693 case 96511: 694 /* age */ return this.age == null ? new Base[0] : new Base[] { this.age }; // Range 695 case 3556653: 696 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 697 default: 698 return super.getProperty(hash, name, checkValid); 699 } 700 701 } 702 703 @Override 704 public Base setProperty(int hash, String name, Base value) throws FHIRException { 705 switch (hash) { 706 case 107348: // low 707 this.low = castToQuantity(value); // Quantity 708 return value; 709 case 3202466: // high 710 this.high = castToQuantity(value); // Quantity 711 return value; 712 case 3575610: // type 713 this.type = castToCodeableConcept(value); // CodeableConcept 714 return value; 715 case -2089924569: // appliesTo 716 this.getAppliesTo().add(castToCodeableConcept(value)); // CodeableConcept 717 return value; 718 case 96511: // age 719 this.age = castToRange(value); // Range 720 return value; 721 case 3556653: // text 722 this.text = castToString(value); // StringType 723 return value; 724 default: 725 return super.setProperty(hash, name, value); 726 } 727 728 } 729 730 @Override 731 public Base setProperty(String name, Base value) throws FHIRException { 732 if (name.equals("low")) { 733 this.low = castToQuantity(value); // Quantity 734 } else if (name.equals("high")) { 735 this.high = castToQuantity(value); // Quantity 736 } else if (name.equals("type")) { 737 this.type = castToCodeableConcept(value); // CodeableConcept 738 } else if (name.equals("appliesTo")) { 739 this.getAppliesTo().add(castToCodeableConcept(value)); 740 } else if (name.equals("age")) { 741 this.age = castToRange(value); // Range 742 } else if (name.equals("text")) { 743 this.text = castToString(value); // StringType 744 } else 745 return super.setProperty(name, value); 746 return value; 747 } 748 749 @Override 750 public Base makeProperty(int hash, String name) throws FHIRException { 751 switch (hash) { 752 case 107348: 753 return getLow(); 754 case 3202466: 755 return getHigh(); 756 case 3575610: 757 return getType(); 758 case -2089924569: 759 return addAppliesTo(); 760 case 96511: 761 return getAge(); 762 case 3556653: 763 return getTextElement(); 764 default: 765 return super.makeProperty(hash, name); 766 } 767 768 } 769 770 @Override 771 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 772 switch (hash) { 773 case 107348: 774 /* low */ return new String[] { "SimpleQuantity" }; 775 case 3202466: 776 /* high */ return new String[] { "SimpleQuantity" }; 777 case 3575610: 778 /* type */ return new String[] { "CodeableConcept" }; 779 case -2089924569: 780 /* appliesTo */ return new String[] { "CodeableConcept" }; 781 case 96511: 782 /* age */ return new String[] { "Range" }; 783 case 3556653: 784 /* text */ return new String[] { "string" }; 785 default: 786 return super.getTypesForProperty(hash, name); 787 } 788 789 } 790 791 @Override 792 public Base addChild(String name) throws FHIRException { 793 if (name.equals("low")) { 794 this.low = new Quantity(); 795 return this.low; 796 } else if (name.equals("high")) { 797 this.high = new Quantity(); 798 return this.high; 799 } else if (name.equals("type")) { 800 this.type = new CodeableConcept(); 801 return this.type; 802 } else if (name.equals("appliesTo")) { 803 return addAppliesTo(); 804 } else if (name.equals("age")) { 805 this.age = new Range(); 806 return this.age; 807 } else if (name.equals("text")) { 808 throw new FHIRException("Cannot call addChild on a singleton property Observation.text"); 809 } else 810 return super.addChild(name); 811 } 812 813 public ObservationReferenceRangeComponent copy() { 814 ObservationReferenceRangeComponent dst = new ObservationReferenceRangeComponent(); 815 copyValues(dst); 816 return dst; 817 } 818 819 public void copyValues(ObservationReferenceRangeComponent dst) { 820 super.copyValues(dst); 821 dst.low = low == null ? null : low.copy(); 822 dst.high = high == null ? null : high.copy(); 823 dst.type = type == null ? null : type.copy(); 824 if (appliesTo != null) { 825 dst.appliesTo = new ArrayList<CodeableConcept>(); 826 for (CodeableConcept i : appliesTo) 827 dst.appliesTo.add(i.copy()); 828 } 829 ; 830 dst.age = age == null ? null : age.copy(); 831 dst.text = text == null ? null : text.copy(); 832 } 833 834 @Override 835 public boolean equalsDeep(Base other_) { 836 if (!super.equalsDeep(other_)) 837 return false; 838 if (!(other_ instanceof ObservationReferenceRangeComponent)) 839 return false; 840 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 841 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true) && compareDeep(type, o.type, true) 842 && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(age, o.age, true) 843 && compareDeep(text, o.text, true); 844 } 845 846 @Override 847 public boolean equalsShallow(Base other_) { 848 if (!super.equalsShallow(other_)) 849 return false; 850 if (!(other_ instanceof ObservationReferenceRangeComponent)) 851 return false; 852 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 853 return compareValues(text, o.text, true); 854 } 855 856 public boolean isEmpty() { 857 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high, type, appliesTo, age, text); 858 } 859 860 public String fhirType() { 861 return "Observation.referenceRange"; 862 863 } 864 865 } 866 867 @Block() 868 public static class ObservationComponentComponent extends BackboneElement implements IBaseBackboneElement { 869 /** 870 * Describes what was observed. Sometimes this is called the observation "code". 871 */ 872 @Child(name = "code", type = { 873 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 874 @Description(shortDefinition = "Type of component observation (code / type)", formalDefinition = "Describes what was observed. Sometimes this is called the observation \"code\".") 875 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 876 protected CodeableConcept code; 877 878 /** 879 * The information determined as a result of making the observation, if the 880 * information has a simple value. 881 */ 882 @Child(name = "value", type = { Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, 883 IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, 884 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 885 @Description(shortDefinition = "Actual component result", formalDefinition = "The information determined as a result of making the observation, if the information has a simple value.") 886 protected Type value; 887 888 /** 889 * Provides a reason why the expected value in the element 890 * Observation.component.value[x] is missing. 891 */ 892 @Child(name = "dataAbsentReason", type = { 893 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 894 @Description(shortDefinition = "Why the component result is missing", formalDefinition = "Provides a reason why the expected value in the element Observation.component.value[x] is missing.") 895 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/data-absent-reason") 896 protected CodeableConcept dataAbsentReason; 897 898 /** 899 * A categorical assessment of an observation value. For example, high, low, 900 * normal. 901 */ 902 @Child(name = "interpretation", type = { 903 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 904 @Description(shortDefinition = "High, low, normal, etc.", formalDefinition = "A categorical assessment of an observation value. For example, high, low, normal.") 905 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-interpretation") 906 protected List<CodeableConcept> interpretation; 907 908 /** 909 * Guidance on how to interpret the value by comparison to a normal or 910 * recommended range. 911 */ 912 @Child(name = "referenceRange", type = { 913 ObservationReferenceRangeComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 914 @Description(shortDefinition = "Provides guide for interpretation of component result", formalDefinition = "Guidance on how to interpret the value by comparison to a normal or recommended range.") 915 protected List<ObservationReferenceRangeComponent> referenceRange; 916 917 private static final long serialVersionUID = 576590931L; 918 919 /** 920 * Constructor 921 */ 922 public ObservationComponentComponent() { 923 super(); 924 } 925 926 /** 927 * Constructor 928 */ 929 public ObservationComponentComponent(CodeableConcept code) { 930 super(); 931 this.code = code; 932 } 933 934 /** 935 * @return {@link #code} (Describes what was observed. Sometimes this is called 936 * the observation "code".) 937 */ 938 public CodeableConcept getCode() { 939 if (this.code == null) 940 if (Configuration.errorOnAutoCreate()) 941 throw new Error("Attempt to auto-create ObservationComponentComponent.code"); 942 else if (Configuration.doAutoCreate()) 943 this.code = new CodeableConcept(); // cc 944 return this.code; 945 } 946 947 public boolean hasCode() { 948 return this.code != null && !this.code.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #code} (Describes what was observed. Sometimes this is 953 * called the observation "code".) 954 */ 955 public ObservationComponentComponent setCode(CodeableConcept value) { 956 this.code = value; 957 return this; 958 } 959 960 /** 961 * @return {@link #value} (The information determined as a result of making the 962 * observation, if the information has a simple value.) 963 */ 964 public Type getValue() { 965 return this.value; 966 } 967 968 /** 969 * @return {@link #value} (The information determined as a result of making the 970 * observation, if the information has a simple value.) 971 */ 972 public Quantity getValueQuantity() throws FHIRException { 973 if (this.value == null) 974 this.value = new Quantity(); 975 if (!(this.value instanceof Quantity)) 976 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 977 + " was encountered"); 978 return (Quantity) this.value; 979 } 980 981 public boolean hasValueQuantity() { 982 return this != null && this.value instanceof Quantity; 983 } 984 985 /** 986 * @return {@link #value} (The information determined as a result of making the 987 * observation, if the information has a simple value.) 988 */ 989 public CodeableConcept getValueCodeableConcept() throws FHIRException { 990 if (this.value == null) 991 this.value = new CodeableConcept(); 992 if (!(this.value instanceof CodeableConcept)) 993 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 994 + this.value.getClass().getName() + " was encountered"); 995 return (CodeableConcept) this.value; 996 } 997 998 public boolean hasValueCodeableConcept() { 999 return this != null && this.value instanceof CodeableConcept; 1000 } 1001 1002 /** 1003 * @return {@link #value} (The information determined as a result of making the 1004 * observation, if the information has a simple value.) 1005 */ 1006 public StringType getValueStringType() throws FHIRException { 1007 if (this.value == null) 1008 this.value = new StringType(); 1009 if (!(this.value instanceof StringType)) 1010 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1011 + this.value.getClass().getName() + " was encountered"); 1012 return (StringType) this.value; 1013 } 1014 1015 public boolean hasValueStringType() { 1016 return this != null && this.value instanceof StringType; 1017 } 1018 1019 /** 1020 * @return {@link #value} (The information determined as a result of making the 1021 * observation, if the information has a simple value.) 1022 */ 1023 public BooleanType getValueBooleanType() throws FHIRException { 1024 if (this.value == null) 1025 this.value = new BooleanType(); 1026 if (!(this.value instanceof BooleanType)) 1027 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1028 + this.value.getClass().getName() + " was encountered"); 1029 return (BooleanType) this.value; 1030 } 1031 1032 public boolean hasValueBooleanType() { 1033 return this != null && this.value instanceof BooleanType; 1034 } 1035 1036 /** 1037 * @return {@link #value} (The information determined as a result of making the 1038 * observation, if the information has a simple value.) 1039 */ 1040 public IntegerType getValueIntegerType() throws FHIRException { 1041 if (this.value == null) 1042 this.value = new IntegerType(); 1043 if (!(this.value instanceof IntegerType)) 1044 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 1045 + this.value.getClass().getName() + " was encountered"); 1046 return (IntegerType) this.value; 1047 } 1048 1049 public boolean hasValueIntegerType() { 1050 return this != null && this.value instanceof IntegerType; 1051 } 1052 1053 /** 1054 * @return {@link #value} (The information determined as a result of making the 1055 * observation, if the information has a simple value.) 1056 */ 1057 public Range getValueRange() throws FHIRException { 1058 if (this.value == null) 1059 this.value = new Range(); 1060 if (!(this.value instanceof Range)) 1061 throw new FHIRException( 1062 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 1063 return (Range) this.value; 1064 } 1065 1066 public boolean hasValueRange() { 1067 return this != null && this.value instanceof Range; 1068 } 1069 1070 /** 1071 * @return {@link #value} (The information determined as a result of making the 1072 * observation, if the information has a simple value.) 1073 */ 1074 public Ratio getValueRatio() throws FHIRException { 1075 if (this.value == null) 1076 this.value = new Ratio(); 1077 if (!(this.value instanceof Ratio)) 1078 throw new FHIRException( 1079 "Type mismatch: the type Ratio was expected, but " + this.value.getClass().getName() + " was encountered"); 1080 return (Ratio) this.value; 1081 } 1082 1083 public boolean hasValueRatio() { 1084 return this != null && this.value instanceof Ratio; 1085 } 1086 1087 /** 1088 * @return {@link #value} (The information determined as a result of making the 1089 * observation, if the information has a simple value.) 1090 */ 1091 public SampledData getValueSampledData() throws FHIRException { 1092 if (this.value == null) 1093 this.value = new SampledData(); 1094 if (!(this.value instanceof SampledData)) 1095 throw new FHIRException("Type mismatch: the type SampledData was expected, but " 1096 + this.value.getClass().getName() + " was encountered"); 1097 return (SampledData) this.value; 1098 } 1099 1100 public boolean hasValueSampledData() { 1101 return this != null && this.value instanceof SampledData; 1102 } 1103 1104 /** 1105 * @return {@link #value} (The information determined as a result of making the 1106 * observation, if the information has a simple value.) 1107 */ 1108 public TimeType getValueTimeType() throws FHIRException { 1109 if (this.value == null) 1110 this.value = new TimeType(); 1111 if (!(this.value instanceof TimeType)) 1112 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 1113 + " was encountered"); 1114 return (TimeType) this.value; 1115 } 1116 1117 public boolean hasValueTimeType() { 1118 return this != null && this.value instanceof TimeType; 1119 } 1120 1121 /** 1122 * @return {@link #value} (The information determined as a result of making the 1123 * observation, if the information has a simple value.) 1124 */ 1125 public DateTimeType getValueDateTimeType() throws FHIRException { 1126 if (this.value == null) 1127 this.value = new DateTimeType(); 1128 if (!(this.value instanceof DateTimeType)) 1129 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1130 + this.value.getClass().getName() + " was encountered"); 1131 return (DateTimeType) this.value; 1132 } 1133 1134 public boolean hasValueDateTimeType() { 1135 return this != null && this.value instanceof DateTimeType; 1136 } 1137 1138 /** 1139 * @return {@link #value} (The information determined as a result of making the 1140 * observation, if the information has a simple value.) 1141 */ 1142 public Period getValuePeriod() throws FHIRException { 1143 if (this.value == null) 1144 this.value = new Period(); 1145 if (!(this.value instanceof Period)) 1146 throw new FHIRException( 1147 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 1148 return (Period) this.value; 1149 } 1150 1151 public boolean hasValuePeriod() { 1152 return this != null && this.value instanceof Period; 1153 } 1154 1155 public boolean hasValue() { 1156 return this.value != null && !this.value.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #value} (The information determined as a result of making 1161 * the observation, if the information has a simple value.) 1162 */ 1163 public ObservationComponentComponent setValue(Type value) { 1164 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept 1165 || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType 1166 || value instanceof Range || value instanceof Ratio || value instanceof SampledData 1167 || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period)) 1168 throw new Error("Not the right type for Observation.component.value[x]: " + value.fhirType()); 1169 this.value = value; 1170 return this; 1171 } 1172 1173 /** 1174 * @return {@link #dataAbsentReason} (Provides a reason why the expected value 1175 * in the element Observation.component.value[x] is missing.) 1176 */ 1177 public CodeableConcept getDataAbsentReason() { 1178 if (this.dataAbsentReason == null) 1179 if (Configuration.errorOnAutoCreate()) 1180 throw new Error("Attempt to auto-create ObservationComponentComponent.dataAbsentReason"); 1181 else if (Configuration.doAutoCreate()) 1182 this.dataAbsentReason = new CodeableConcept(); // cc 1183 return this.dataAbsentReason; 1184 } 1185 1186 public boolean hasDataAbsentReason() { 1187 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1188 } 1189 1190 /** 1191 * @param value {@link #dataAbsentReason} (Provides a reason why the expected 1192 * value in the element Observation.component.value[x] is missing.) 1193 */ 1194 public ObservationComponentComponent setDataAbsentReason(CodeableConcept value) { 1195 this.dataAbsentReason = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #interpretation} (A categorical assessment of an observation 1201 * value. For example, high, low, normal.) 1202 */ 1203 public List<CodeableConcept> getInterpretation() { 1204 if (this.interpretation == null) 1205 this.interpretation = new ArrayList<CodeableConcept>(); 1206 return this.interpretation; 1207 } 1208 1209 /** 1210 * @return Returns a reference to <code>this</code> for easy method chaining 1211 */ 1212 public ObservationComponentComponent setInterpretation(List<CodeableConcept> theInterpretation) { 1213 this.interpretation = theInterpretation; 1214 return this; 1215 } 1216 1217 public boolean hasInterpretation() { 1218 if (this.interpretation == null) 1219 return false; 1220 for (CodeableConcept item : this.interpretation) 1221 if (!item.isEmpty()) 1222 return true; 1223 return false; 1224 } 1225 1226 public CodeableConcept addInterpretation() { // 3 1227 CodeableConcept t = new CodeableConcept(); 1228 if (this.interpretation == null) 1229 this.interpretation = new ArrayList<CodeableConcept>(); 1230 this.interpretation.add(t); 1231 return t; 1232 } 1233 1234 public ObservationComponentComponent addInterpretation(CodeableConcept t) { // 3 1235 if (t == null) 1236 return this; 1237 if (this.interpretation == null) 1238 this.interpretation = new ArrayList<CodeableConcept>(); 1239 this.interpretation.add(t); 1240 return this; 1241 } 1242 1243 /** 1244 * @return The first repetition of repeating field {@link #interpretation}, 1245 * creating it if it does not already exist 1246 */ 1247 public CodeableConcept getInterpretationFirstRep() { 1248 if (getInterpretation().isEmpty()) { 1249 addInterpretation(); 1250 } 1251 return getInterpretation().get(0); 1252 } 1253 1254 /** 1255 * @return {@link #referenceRange} (Guidance on how to interpret the value by 1256 * comparison to a normal or recommended range.) 1257 */ 1258 public List<ObservationReferenceRangeComponent> getReferenceRange() { 1259 if (this.referenceRange == null) 1260 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1261 return this.referenceRange; 1262 } 1263 1264 /** 1265 * @return Returns a reference to <code>this</code> for easy method chaining 1266 */ 1267 public ObservationComponentComponent setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 1268 this.referenceRange = theReferenceRange; 1269 return this; 1270 } 1271 1272 public boolean hasReferenceRange() { 1273 if (this.referenceRange == null) 1274 return false; 1275 for (ObservationReferenceRangeComponent item : this.referenceRange) 1276 if (!item.isEmpty()) 1277 return true; 1278 return false; 1279 } 1280 1281 public ObservationReferenceRangeComponent addReferenceRange() { // 3 1282 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 1283 if (this.referenceRange == null) 1284 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1285 this.referenceRange.add(t); 1286 return t; 1287 } 1288 1289 public ObservationComponentComponent addReferenceRange(ObservationReferenceRangeComponent t) { // 3 1290 if (t == null) 1291 return this; 1292 if (this.referenceRange == null) 1293 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1294 this.referenceRange.add(t); 1295 return this; 1296 } 1297 1298 /** 1299 * @return The first repetition of repeating field {@link #referenceRange}, 1300 * creating it if it does not already exist 1301 */ 1302 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 1303 if (getReferenceRange().isEmpty()) { 1304 addReferenceRange(); 1305 } 1306 return getReferenceRange().get(0); 1307 } 1308 1309 protected void listChildren(List<Property> children) { 1310 super.listChildren(children); 1311 children.add(new Property("code", "CodeableConcept", 1312 "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code)); 1313 children.add(new Property("value[x]", 1314 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1315 "The information determined as a result of making the observation, if the information has a simple value.", 0, 1316 1, value)); 1317 children.add(new Property("dataAbsentReason", "CodeableConcept", 1318 "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, 1319 dataAbsentReason)); 1320 children.add(new Property("interpretation", "CodeableConcept", 1321 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 1322 java.lang.Integer.MAX_VALUE, interpretation)); 1323 children.add(new Property("referenceRange", "@Observation.referenceRange", 1324 "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, 1325 java.lang.Integer.MAX_VALUE, referenceRange)); 1326 } 1327 1328 @Override 1329 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1330 switch (_hash) { 1331 case 3059181: 1332 /* code */ return new Property("code", "CodeableConcept", 1333 "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code); 1334 case -1410166417: 1335 /* value[x] */ return new Property("value[x]", 1336 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1337 "The information determined as a result of making the observation, if the information has a simple value.", 1338 0, 1, value); 1339 case 111972721: 1340 /* value */ return new Property("value[x]", 1341 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1342 "The information determined as a result of making the observation, if the information has a simple value.", 1343 0, 1, value); 1344 case -2029823716: 1345 /* valueQuantity */ return new Property("value[x]", 1346 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1347 "The information determined as a result of making the observation, if the information has a simple value.", 1348 0, 1, value); 1349 case 924902896: 1350 /* valueCodeableConcept */ return new Property("value[x]", 1351 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1352 "The information determined as a result of making the observation, if the information has a simple value.", 1353 0, 1, value); 1354 case -1424603934: 1355 /* valueString */ return new Property("value[x]", 1356 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1357 "The information determined as a result of making the observation, if the information has a simple value.", 1358 0, 1, value); 1359 case 733421943: 1360 /* valueBoolean */ return new Property("value[x]", 1361 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1362 "The information determined as a result of making the observation, if the information has a simple value.", 1363 0, 1, value); 1364 case -1668204915: 1365 /* valueInteger */ return new Property("value[x]", 1366 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1367 "The information determined as a result of making the observation, if the information has a simple value.", 1368 0, 1, value); 1369 case 2030761548: 1370 /* valueRange */ return new Property("value[x]", 1371 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1372 "The information determined as a result of making the observation, if the information has a simple value.", 1373 0, 1, value); 1374 case 2030767386: 1375 /* valueRatio */ return new Property("value[x]", 1376 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1377 "The information determined as a result of making the observation, if the information has a simple value.", 1378 0, 1, value); 1379 case -962229101: 1380 /* valueSampledData */ return new Property("value[x]", 1381 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1382 "The information determined as a result of making the observation, if the information has a simple value.", 1383 0, 1, value); 1384 case -765708322: 1385 /* valueTime */ return new Property("value[x]", 1386 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1387 "The information determined as a result of making the observation, if the information has a simple value.", 1388 0, 1, value); 1389 case 1047929900: 1390 /* valueDateTime */ return new Property("value[x]", 1391 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1392 "The information determined as a result of making the observation, if the information has a simple value.", 1393 0, 1, value); 1394 case -1524344174: 1395 /* valuePeriod */ return new Property("value[x]", 1396 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1397 "The information determined as a result of making the observation, if the information has a simple value.", 1398 0, 1, value); 1399 case 1034315687: 1400 /* dataAbsentReason */ return new Property("dataAbsentReason", "CodeableConcept", 1401 "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, 1402 dataAbsentReason); 1403 case -297950712: 1404 /* interpretation */ return new Property("interpretation", "CodeableConcept", 1405 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 1406 java.lang.Integer.MAX_VALUE, interpretation); 1407 case -1912545102: 1408 /* referenceRange */ return new Property("referenceRange", "@Observation.referenceRange", 1409 "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, 1410 java.lang.Integer.MAX_VALUE, referenceRange); 1411 default: 1412 return super.getNamedProperty(_hash, _name, _checkValid); 1413 } 1414 1415 } 1416 1417 @Override 1418 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1419 switch (hash) { 1420 case 3059181: 1421 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1422 case 111972721: 1423 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1424 case 1034315687: 1425 /* dataAbsentReason */ return this.dataAbsentReason == null ? new Base[0] 1426 : new Base[] { this.dataAbsentReason }; // CodeableConcept 1427 case -297950712: 1428 /* interpretation */ return this.interpretation == null ? new Base[0] 1429 : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 1430 case -1912545102: 1431 /* referenceRange */ return this.referenceRange == null ? new Base[0] 1432 : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 1433 default: 1434 return super.getProperty(hash, name, checkValid); 1435 } 1436 1437 } 1438 1439 @Override 1440 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1441 switch (hash) { 1442 case 3059181: // code 1443 this.code = castToCodeableConcept(value); // CodeableConcept 1444 return value; 1445 case 111972721: // value 1446 this.value = castToType(value); // Type 1447 return value; 1448 case 1034315687: // dataAbsentReason 1449 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1450 return value; 1451 case -297950712: // interpretation 1452 this.getInterpretation().add(castToCodeableConcept(value)); // CodeableConcept 1453 return value; 1454 case -1912545102: // referenceRange 1455 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 1456 return value; 1457 default: 1458 return super.setProperty(hash, name, value); 1459 } 1460 1461 } 1462 1463 @Override 1464 public Base setProperty(String name, Base value) throws FHIRException { 1465 if (name.equals("code")) { 1466 this.code = castToCodeableConcept(value); // CodeableConcept 1467 } else if (name.equals("value[x]")) { 1468 this.value = castToType(value); // Type 1469 } else if (name.equals("dataAbsentReason")) { 1470 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1471 } else if (name.equals("interpretation")) { 1472 this.getInterpretation().add(castToCodeableConcept(value)); 1473 } else if (name.equals("referenceRange")) { 1474 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 1475 } else 1476 return super.setProperty(name, value); 1477 return value; 1478 } 1479 1480 @Override 1481 public Base makeProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case 3059181: 1484 return getCode(); 1485 case -1410166417: 1486 return getValue(); 1487 case 111972721: 1488 return getValue(); 1489 case 1034315687: 1490 return getDataAbsentReason(); 1491 case -297950712: 1492 return addInterpretation(); 1493 case -1912545102: 1494 return addReferenceRange(); 1495 default: 1496 return super.makeProperty(hash, name); 1497 } 1498 1499 } 1500 1501 @Override 1502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1503 switch (hash) { 1504 case 3059181: 1505 /* code */ return new String[] { "CodeableConcept" }; 1506 case 111972721: 1507 /* value */ return new String[] { "Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", 1508 "Ratio", "SampledData", "time", "dateTime", "Period" }; 1509 case 1034315687: 1510 /* dataAbsentReason */ return new String[] { "CodeableConcept" }; 1511 case -297950712: 1512 /* interpretation */ return new String[] { "CodeableConcept" }; 1513 case -1912545102: 1514 /* referenceRange */ return new String[] { "@Observation.referenceRange" }; 1515 default: 1516 return super.getTypesForProperty(hash, name); 1517 } 1518 1519 } 1520 1521 @Override 1522 public Base addChild(String name) throws FHIRException { 1523 if (name.equals("code")) { 1524 this.code = new CodeableConcept(); 1525 return this.code; 1526 } else if (name.equals("valueQuantity")) { 1527 this.value = new Quantity(); 1528 return this.value; 1529 } else if (name.equals("valueCodeableConcept")) { 1530 this.value = new CodeableConcept(); 1531 return this.value; 1532 } else if (name.equals("valueString")) { 1533 this.value = new StringType(); 1534 return this.value; 1535 } else if (name.equals("valueBoolean")) { 1536 this.value = new BooleanType(); 1537 return this.value; 1538 } else if (name.equals("valueInteger")) { 1539 this.value = new IntegerType(); 1540 return this.value; 1541 } else if (name.equals("valueRange")) { 1542 this.value = new Range(); 1543 return this.value; 1544 } else if (name.equals("valueRatio")) { 1545 this.value = new Ratio(); 1546 return this.value; 1547 } else if (name.equals("valueSampledData")) { 1548 this.value = new SampledData(); 1549 return this.value; 1550 } else if (name.equals("valueTime")) { 1551 this.value = new TimeType(); 1552 return this.value; 1553 } else if (name.equals("valueDateTime")) { 1554 this.value = new DateTimeType(); 1555 return this.value; 1556 } else if (name.equals("valuePeriod")) { 1557 this.value = new Period(); 1558 return this.value; 1559 } else if (name.equals("dataAbsentReason")) { 1560 this.dataAbsentReason = new CodeableConcept(); 1561 return this.dataAbsentReason; 1562 } else if (name.equals("interpretation")) { 1563 return addInterpretation(); 1564 } else if (name.equals("referenceRange")) { 1565 return addReferenceRange(); 1566 } else 1567 return super.addChild(name); 1568 } 1569 1570 public ObservationComponentComponent copy() { 1571 ObservationComponentComponent dst = new ObservationComponentComponent(); 1572 copyValues(dst); 1573 return dst; 1574 } 1575 1576 public void copyValues(ObservationComponentComponent dst) { 1577 super.copyValues(dst); 1578 dst.code = code == null ? null : code.copy(); 1579 dst.value = value == null ? null : value.copy(); 1580 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 1581 if (interpretation != null) { 1582 dst.interpretation = new ArrayList<CodeableConcept>(); 1583 for (CodeableConcept i : interpretation) 1584 dst.interpretation.add(i.copy()); 1585 } 1586 ; 1587 if (referenceRange != null) { 1588 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1589 for (ObservationReferenceRangeComponent i : referenceRange) 1590 dst.referenceRange.add(i.copy()); 1591 } 1592 ; 1593 } 1594 1595 @Override 1596 public boolean equalsDeep(Base other_) { 1597 if (!super.equalsDeep(other_)) 1598 return false; 1599 if (!(other_ instanceof ObservationComponentComponent)) 1600 return false; 1601 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1602 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 1603 && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 1604 && compareDeep(interpretation, o.interpretation, true) && compareDeep(referenceRange, o.referenceRange, true); 1605 } 1606 1607 @Override 1608 public boolean equalsShallow(Base other_) { 1609 if (!super.equalsShallow(other_)) 1610 return false; 1611 if (!(other_ instanceof ObservationComponentComponent)) 1612 return false; 1613 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1614 return true; 1615 } 1616 1617 public boolean isEmpty() { 1618 return super.isEmpty() 1619 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, dataAbsentReason, interpretation, referenceRange); 1620 } 1621 1622 public String fhirType() { 1623 return "Observation.component"; 1624 1625 } 1626 1627 } 1628 1629 /** 1630 * A unique identifier assigned to this observation. 1631 */ 1632 @Child(name = "identifier", type = { 1633 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1634 @Description(shortDefinition = "Business Identifier for observation", formalDefinition = "A unique identifier assigned to this observation.") 1635 protected List<Identifier> identifier; 1636 1637 /** 1638 * A plan, proposal or order that is fulfilled in whole or in part by this 1639 * event. For example, a MedicationRequest may require a patient to have 1640 * laboratory test performed before it is dispensed. 1641 */ 1642 @Child(name = "basedOn", type = { CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, 1643 MedicationRequest.class, NutritionOrder.class, 1644 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1645 @Description(shortDefinition = "Fulfills plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.") 1646 protected List<Reference> basedOn; 1647 /** 1648 * The actual objects that are the target of the reference (A plan, proposal or 1649 * order that is fulfilled in whole or in part by this event. For example, a 1650 * MedicationRequest may require a patient to have laboratory test performed 1651 * before it is dispensed.) 1652 */ 1653 protected List<Resource> basedOnTarget; 1654 1655 /** 1656 * A larger event of which this particular Observation is a component or step. 1657 * For example, an observation as part of a procedure. 1658 */ 1659 @Child(name = "partOf", type = { MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, 1660 Procedure.class, Immunization.class, 1661 ImagingStudy.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1662 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.") 1663 protected List<Reference> partOf; 1664 /** 1665 * The actual objects that are the target of the reference (A larger event of 1666 * which this particular Observation is a component or step. For example, an 1667 * observation as part of a procedure.) 1668 */ 1669 protected List<Resource> partOfTarget; 1670 1671 /** 1672 * The status of the result value. 1673 */ 1674 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 1675 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "The status of the result value.") 1676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1677 protected Enumeration<ObservationStatus> status; 1678 1679 /** 1680 * A code that classifies the general type of observation being made. 1681 */ 1682 @Child(name = "category", type = { 1683 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1684 @Description(shortDefinition = "Classification of type of observation", formalDefinition = "A code that classifies the general type of observation being made.") 1685 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-category") 1686 protected List<CodeableConcept> category; 1687 1688 /** 1689 * Describes what was observed. Sometimes this is called the observation "name". 1690 */ 1691 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 1692 @Description(shortDefinition = "Type of observation (code / type)", formalDefinition = "Describes what was observed. Sometimes this is called the observation \"name\".") 1693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 1694 protected CodeableConcept code; 1695 1696 /** 1697 * The patient, or group of patients, location, or device this observation is 1698 * about and into whose record the observation is placed. If the actual focus of 1699 * the observation is different from the subject (or a sample of, part, or 1700 * region of the subject), the `focus` element or the `code` itself specifies 1701 * the actual focus of the observation. 1702 */ 1703 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 1704 Location.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1705 @Description(shortDefinition = "Who and/or what the observation is about", formalDefinition = "The patient, or group of patients, location, or device this observation is about and into whose record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.") 1706 protected Reference subject; 1707 1708 /** 1709 * The actual object that is the target of the reference (The patient, or group 1710 * of patients, location, or device this observation is about and into whose 1711 * record the observation is placed. If the actual focus of the observation is 1712 * different from the subject (or a sample of, part, or region of the subject), 1713 * the `focus` element or the `code` itself specifies the actual focus of the 1714 * observation.) 1715 */ 1716 protected Resource subjectTarget; 1717 1718 /** 1719 * The actual focus of an observation when it is not the patient of record 1720 * representing something or someone associated with the patient such as a 1721 * spouse, parent, fetus, or donor. For example, fetus observations in a 1722 * mother's record. The focus of an observation could also be an existing 1723 * condition, an intervention, the subject's diet, another observation of the 1724 * subject, or a body structure such as tumor or implanted device. An example 1725 * use case would be using the Observation resource to capture whether the 1726 * mother is trained to change her child's tracheostomy tube. In this example, 1727 * the child is the patient of record and the mother is the focus. 1728 */ 1729 @Child(name = "focus", type = { 1730 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1731 @Description(shortDefinition = "What the observation is about, when it is not about the subject of record", formalDefinition = "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.") 1732 protected List<Reference> focus; 1733 /** 1734 * The actual objects that are the target of the reference (The actual focus of 1735 * an observation when it is not the patient of record representing something or 1736 * someone associated with the patient such as a spouse, parent, fetus, or 1737 * donor. For example, fetus observations in a mother's record. The focus of an 1738 * observation could also be an existing condition, an intervention, the 1739 * subject's diet, another observation of the subject, or a body structure such 1740 * as tumor or implanted device. An example use case would be using the 1741 * Observation resource to capture whether the mother is trained to change her 1742 * child's tracheostomy tube. In this example, the child is the patient of 1743 * record and the mother is the focus.) 1744 */ 1745 protected List<Resource> focusTarget; 1746 1747 /** 1748 * The healthcare event (e.g. a patient and healthcare provider interaction) 1749 * during which this observation is made. 1750 */ 1751 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1752 @Description(shortDefinition = "Healthcare event during which this observation is made", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.") 1753 protected Reference encounter; 1754 1755 /** 1756 * The actual object that is the target of the reference (The healthcare event 1757 * (e.g. a patient and healthcare provider interaction) during which this 1758 * observation is made.) 1759 */ 1760 protected Encounter encounterTarget; 1761 1762 /** 1763 * The time or time-period the observed value is asserted as being true. For 1764 * biological subjects - e.g. human patients - this is usually called the 1765 * "physiologically relevant time". This is usually either the time of the 1766 * procedure or of specimen collection, but very often the source of the 1767 * date/time is not known, only the date/time itself. 1768 */ 1769 @Child(name = "effective", type = { DateTimeType.class, Period.class, Timing.class, 1770 InstantType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1771 @Description(shortDefinition = "Clinically relevant time/time-period for observation", formalDefinition = "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.") 1772 protected Type effective; 1773 1774 /** 1775 * The date and time this version of the observation was made available to 1776 * providers, typically after the results have been reviewed and verified. 1777 */ 1778 @Child(name = "issued", type = { InstantType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1779 @Description(shortDefinition = "Date/Time this version was made available", formalDefinition = "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.") 1780 protected InstantType issued; 1781 1782 /** 1783 * Who was responsible for asserting the observed value as "true". 1784 */ 1785 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 1786 Patient.class, 1787 RelatedPerson.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1788 @Description(shortDefinition = "Who is responsible for the observation", formalDefinition = "Who was responsible for asserting the observed value as \"true\".") 1789 protected List<Reference> performer; 1790 /** 1791 * The actual objects that are the target of the reference (Who was responsible 1792 * for asserting the observed value as "true".) 1793 */ 1794 protected List<Resource> performerTarget; 1795 1796 /** 1797 * The information determined as a result of making the observation, if the 1798 * information has a simple value. 1799 */ 1800 @Child(name = "value", type = { Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, 1801 IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, 1802 Period.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1803 @Description(shortDefinition = "Actual result", formalDefinition = "The information determined as a result of making the observation, if the information has a simple value.") 1804 protected Type value; 1805 1806 /** 1807 * Provides a reason why the expected value in the element Observation.value[x] 1808 * is missing. 1809 */ 1810 @Child(name = "dataAbsentReason", type = { 1811 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1812 @Description(shortDefinition = "Why the result is missing", formalDefinition = "Provides a reason why the expected value in the element Observation.value[x] is missing.") 1813 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/data-absent-reason") 1814 protected CodeableConcept dataAbsentReason; 1815 1816 /** 1817 * A categorical assessment of an observation value. For example, high, low, 1818 * normal. 1819 */ 1820 @Child(name = "interpretation", type = { 1821 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1822 @Description(shortDefinition = "High, low, normal, etc.", formalDefinition = "A categorical assessment of an observation value. For example, high, low, normal.") 1823 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-interpretation") 1824 protected List<CodeableConcept> interpretation; 1825 1826 /** 1827 * Comments about the observation or the results. 1828 */ 1829 @Child(name = "note", type = { 1830 Annotation.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1831 @Description(shortDefinition = "Comments about the observation", formalDefinition = "Comments about the observation or the results.") 1832 protected List<Annotation> note; 1833 1834 /** 1835 * Indicates the site on the subject's body where the observation was made (i.e. 1836 * the target site). 1837 */ 1838 @Child(name = "bodySite", type = { 1839 CodeableConcept.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1840 @Description(shortDefinition = "Observed body part", formalDefinition = "Indicates the site on the subject's body where the observation was made (i.e. the target site).") 1841 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 1842 protected CodeableConcept bodySite; 1843 1844 /** 1845 * Indicates the mechanism used to perform the observation. 1846 */ 1847 @Child(name = "method", type = { 1848 CodeableConcept.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1849 @Description(shortDefinition = "How it was done", formalDefinition = "Indicates the mechanism used to perform the observation.") 1850 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-methods") 1851 protected CodeableConcept method; 1852 1853 /** 1854 * The specimen that was used when this observation was made. 1855 */ 1856 @Child(name = "specimen", type = { Specimen.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 1857 @Description(shortDefinition = "Specimen used for this observation", formalDefinition = "The specimen that was used when this observation was made.") 1858 protected Reference specimen; 1859 1860 /** 1861 * The actual object that is the target of the reference (The specimen that was 1862 * used when this observation was made.) 1863 */ 1864 protected Specimen specimenTarget; 1865 1866 /** 1867 * The device used to generate the observation data. 1868 */ 1869 @Child(name = "device", type = { Device.class, 1870 DeviceMetric.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 1871 @Description(shortDefinition = "(Measurement) Device", formalDefinition = "The device used to generate the observation data.") 1872 protected Reference device; 1873 1874 /** 1875 * The actual object that is the target of the reference (The device used to 1876 * generate the observation data.) 1877 */ 1878 protected Resource deviceTarget; 1879 1880 /** 1881 * Guidance on how to interpret the value by comparison to a normal or 1882 * recommended range. Multiple reference ranges are interpreted as an "OR". In 1883 * other words, to represent two distinct target populations, two 1884 * `referenceRange` elements would be used. 1885 */ 1886 @Child(name = "referenceRange", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1887 @Description(shortDefinition = "Provides guide for interpretation", formalDefinition = "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.") 1888 protected List<ObservationReferenceRangeComponent> referenceRange; 1889 1890 /** 1891 * This observation is a group observation (e.g. a battery, a panel of tests, a 1892 * set of vital sign measurements) that includes the target as a member of the 1893 * group. 1894 */ 1895 @Child(name = "hasMember", type = { Observation.class, QuestionnaireResponse.class, 1896 MolecularSequence.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1897 @Description(shortDefinition = "Related resource that belongs to the Observation group", formalDefinition = "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.") 1898 protected List<Reference> hasMember; 1899 /** 1900 * The actual objects that are the target of the reference (This observation is 1901 * a group observation (e.g. a battery, a panel of tests, a set of vital sign 1902 * measurements) that includes the target as a member of the group.) 1903 */ 1904 protected List<Resource> hasMemberTarget; 1905 1906 /** 1907 * The target resource that represents a measurement from which this observation 1908 * value is derived. For example, a calculated anion gap or a fetal measurement 1909 * based on an ultrasound image. 1910 */ 1911 @Child(name = "derivedFrom", type = { DocumentReference.class, ImagingStudy.class, Media.class, 1912 QuestionnaireResponse.class, Observation.class, 1913 MolecularSequence.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1914 @Description(shortDefinition = "Related measurements the observation is made from", formalDefinition = "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.") 1915 protected List<Reference> derivedFrom; 1916 /** 1917 * The actual objects that are the target of the reference (The target resource 1918 * that represents a measurement from which this observation value is derived. 1919 * For example, a calculated anion gap or a fetal measurement based on an 1920 * ultrasound image.) 1921 */ 1922 protected List<Resource> derivedFromTarget; 1923 1924 /** 1925 * Some observations have multiple component observations. These component 1926 * observations are expressed as separate code value pairs that share the same 1927 * attributes. Examples include systolic and diastolic component observations 1928 * for blood pressure measurement and multiple component observations for 1929 * genetics observations. 1930 */ 1931 @Child(name = "component", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1932 @Description(shortDefinition = "Component results", formalDefinition = "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.") 1933 protected List<ObservationComponentComponent> component; 1934 1935 private static final long serialVersionUID = -2036786355L; 1936 1937 /** 1938 * Constructor 1939 */ 1940 public Observation() { 1941 super(); 1942 } 1943 1944 /** 1945 * Constructor 1946 */ 1947 public Observation(Enumeration<ObservationStatus> status, CodeableConcept code) { 1948 super(); 1949 this.status = status; 1950 this.code = code; 1951 } 1952 1953 /** 1954 * @return {@link #identifier} (A unique identifier assigned to this 1955 * observation.) 1956 */ 1957 public List<Identifier> getIdentifier() { 1958 if (this.identifier == null) 1959 this.identifier = new ArrayList<Identifier>(); 1960 return this.identifier; 1961 } 1962 1963 /** 1964 * @return Returns a reference to <code>this</code> for easy method chaining 1965 */ 1966 public Observation setIdentifier(List<Identifier> theIdentifier) { 1967 this.identifier = theIdentifier; 1968 return this; 1969 } 1970 1971 public boolean hasIdentifier() { 1972 if (this.identifier == null) 1973 return false; 1974 for (Identifier item : this.identifier) 1975 if (!item.isEmpty()) 1976 return true; 1977 return false; 1978 } 1979 1980 public Identifier addIdentifier() { // 3 1981 Identifier t = new Identifier(); 1982 if (this.identifier == null) 1983 this.identifier = new ArrayList<Identifier>(); 1984 this.identifier.add(t); 1985 return t; 1986 } 1987 1988 public Observation addIdentifier(Identifier t) { // 3 1989 if (t == null) 1990 return this; 1991 if (this.identifier == null) 1992 this.identifier = new ArrayList<Identifier>(); 1993 this.identifier.add(t); 1994 return this; 1995 } 1996 1997 /** 1998 * @return The first repetition of repeating field {@link #identifier}, creating 1999 * it if it does not already exist 2000 */ 2001 public Identifier getIdentifierFirstRep() { 2002 if (getIdentifier().isEmpty()) { 2003 addIdentifier(); 2004 } 2005 return getIdentifier().get(0); 2006 } 2007 2008 /** 2009 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 2010 * whole or in part by this event. For example, a MedicationRequest may 2011 * require a patient to have laboratory test performed before it is 2012 * dispensed.) 2013 */ 2014 public List<Reference> getBasedOn() { 2015 if (this.basedOn == null) 2016 this.basedOn = new ArrayList<Reference>(); 2017 return this.basedOn; 2018 } 2019 2020 /** 2021 * @return Returns a reference to <code>this</code> for easy method chaining 2022 */ 2023 public Observation setBasedOn(List<Reference> theBasedOn) { 2024 this.basedOn = theBasedOn; 2025 return this; 2026 } 2027 2028 public boolean hasBasedOn() { 2029 if (this.basedOn == null) 2030 return false; 2031 for (Reference item : this.basedOn) 2032 if (!item.isEmpty()) 2033 return true; 2034 return false; 2035 } 2036 2037 public Reference addBasedOn() { // 3 2038 Reference t = new Reference(); 2039 if (this.basedOn == null) 2040 this.basedOn = new ArrayList<Reference>(); 2041 this.basedOn.add(t); 2042 return t; 2043 } 2044 2045 public Observation addBasedOn(Reference t) { // 3 2046 if (t == null) 2047 return this; 2048 if (this.basedOn == null) 2049 this.basedOn = new ArrayList<Reference>(); 2050 this.basedOn.add(t); 2051 return this; 2052 } 2053 2054 /** 2055 * @return The first repetition of repeating field {@link #basedOn}, creating it 2056 * if it does not already exist 2057 */ 2058 public Reference getBasedOnFirstRep() { 2059 if (getBasedOn().isEmpty()) { 2060 addBasedOn(); 2061 } 2062 return getBasedOn().get(0); 2063 } 2064 2065 /** 2066 * @deprecated Use Reference#setResource(IBaseResource) instead 2067 */ 2068 @Deprecated 2069 public List<Resource> getBasedOnTarget() { 2070 if (this.basedOnTarget == null) 2071 this.basedOnTarget = new ArrayList<Resource>(); 2072 return this.basedOnTarget; 2073 } 2074 2075 /** 2076 * @return {@link #partOf} (A larger event of which this particular Observation 2077 * is a component or step. For example, an observation as part of a 2078 * procedure.) 2079 */ 2080 public List<Reference> getPartOf() { 2081 if (this.partOf == null) 2082 this.partOf = new ArrayList<Reference>(); 2083 return this.partOf; 2084 } 2085 2086 /** 2087 * @return Returns a reference to <code>this</code> for easy method chaining 2088 */ 2089 public Observation setPartOf(List<Reference> thePartOf) { 2090 this.partOf = thePartOf; 2091 return this; 2092 } 2093 2094 public boolean hasPartOf() { 2095 if (this.partOf == null) 2096 return false; 2097 for (Reference item : this.partOf) 2098 if (!item.isEmpty()) 2099 return true; 2100 return false; 2101 } 2102 2103 public Reference addPartOf() { // 3 2104 Reference t = new Reference(); 2105 if (this.partOf == null) 2106 this.partOf = new ArrayList<Reference>(); 2107 this.partOf.add(t); 2108 return t; 2109 } 2110 2111 public Observation addPartOf(Reference t) { // 3 2112 if (t == null) 2113 return this; 2114 if (this.partOf == null) 2115 this.partOf = new ArrayList<Reference>(); 2116 this.partOf.add(t); 2117 return this; 2118 } 2119 2120 /** 2121 * @return The first repetition of repeating field {@link #partOf}, creating it 2122 * if it does not already exist 2123 */ 2124 public Reference getPartOfFirstRep() { 2125 if (getPartOf().isEmpty()) { 2126 addPartOf(); 2127 } 2128 return getPartOf().get(0); 2129 } 2130 2131 /** 2132 * @deprecated Use Reference#setResource(IBaseResource) instead 2133 */ 2134 @Deprecated 2135 public List<Resource> getPartOfTarget() { 2136 if (this.partOfTarget == null) 2137 this.partOfTarget = new ArrayList<Resource>(); 2138 return this.partOfTarget; 2139 } 2140 2141 /** 2142 * @return {@link #status} (The status of the result value.). This is the 2143 * underlying object with id, value and extensions. The accessor 2144 * "getStatus" gives direct access to the value 2145 */ 2146 public Enumeration<ObservationStatus> getStatusElement() { 2147 if (this.status == null) 2148 if (Configuration.errorOnAutoCreate()) 2149 throw new Error("Attempt to auto-create Observation.status"); 2150 else if (Configuration.doAutoCreate()) 2151 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 2152 return this.status; 2153 } 2154 2155 public boolean hasStatusElement() { 2156 return this.status != null && !this.status.isEmpty(); 2157 } 2158 2159 public boolean hasStatus() { 2160 return this.status != null && !this.status.isEmpty(); 2161 } 2162 2163 /** 2164 * @param value {@link #status} (The status of the result value.). This is the 2165 * underlying object with id, value and extensions. The accessor 2166 * "getStatus" gives direct access to the value 2167 */ 2168 public Observation setStatusElement(Enumeration<ObservationStatus> value) { 2169 this.status = value; 2170 return this; 2171 } 2172 2173 /** 2174 * @return The status of the result value. 2175 */ 2176 public ObservationStatus getStatus() { 2177 return this.status == null ? null : this.status.getValue(); 2178 } 2179 2180 /** 2181 * @param value The status of the result value. 2182 */ 2183 public Observation setStatus(ObservationStatus value) { 2184 if (this.status == null) 2185 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 2186 this.status.setValue(value); 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #category} (A code that classifies the general type of 2192 * observation being made.) 2193 */ 2194 public List<CodeableConcept> getCategory() { 2195 if (this.category == null) 2196 this.category = new ArrayList<CodeableConcept>(); 2197 return this.category; 2198 } 2199 2200 /** 2201 * @return Returns a reference to <code>this</code> for easy method chaining 2202 */ 2203 public Observation setCategory(List<CodeableConcept> theCategory) { 2204 this.category = theCategory; 2205 return this; 2206 } 2207 2208 public boolean hasCategory() { 2209 if (this.category == null) 2210 return false; 2211 for (CodeableConcept item : this.category) 2212 if (!item.isEmpty()) 2213 return true; 2214 return false; 2215 } 2216 2217 public CodeableConcept addCategory() { // 3 2218 CodeableConcept t = new CodeableConcept(); 2219 if (this.category == null) 2220 this.category = new ArrayList<CodeableConcept>(); 2221 this.category.add(t); 2222 return t; 2223 } 2224 2225 public Observation addCategory(CodeableConcept t) { // 3 2226 if (t == null) 2227 return this; 2228 if (this.category == null) 2229 this.category = new ArrayList<CodeableConcept>(); 2230 this.category.add(t); 2231 return this; 2232 } 2233 2234 /** 2235 * @return The first repetition of repeating field {@link #category}, creating 2236 * it if it does not already exist 2237 */ 2238 public CodeableConcept getCategoryFirstRep() { 2239 if (getCategory().isEmpty()) { 2240 addCategory(); 2241 } 2242 return getCategory().get(0); 2243 } 2244 2245 /** 2246 * @return {@link #code} (Describes what was observed. Sometimes this is called 2247 * the observation "name".) 2248 */ 2249 public CodeableConcept getCode() { 2250 if (this.code == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create Observation.code"); 2253 else if (Configuration.doAutoCreate()) 2254 this.code = new CodeableConcept(); // cc 2255 return this.code; 2256 } 2257 2258 public boolean hasCode() { 2259 return this.code != null && !this.code.isEmpty(); 2260 } 2261 2262 /** 2263 * @param value {@link #code} (Describes what was observed. Sometimes this is 2264 * called the observation "name".) 2265 */ 2266 public Observation setCode(CodeableConcept value) { 2267 this.code = value; 2268 return this; 2269 } 2270 2271 /** 2272 * @return {@link #subject} (The patient, or group of patients, location, or 2273 * device this observation is about and into whose record the 2274 * observation is placed. If the actual focus of the observation is 2275 * different from the subject (or a sample of, part, or region of the 2276 * subject), the `focus` element or the `code` itself specifies the 2277 * actual focus of the observation.) 2278 */ 2279 public Reference getSubject() { 2280 if (this.subject == null) 2281 if (Configuration.errorOnAutoCreate()) 2282 throw new Error("Attempt to auto-create Observation.subject"); 2283 else if (Configuration.doAutoCreate()) 2284 this.subject = new Reference(); // cc 2285 return this.subject; 2286 } 2287 2288 public boolean hasSubject() { 2289 return this.subject != null && !this.subject.isEmpty(); 2290 } 2291 2292 /** 2293 * @param value {@link #subject} (The patient, or group of patients, location, 2294 * or device this observation is about and into whose record the 2295 * observation is placed. If the actual focus of the observation is 2296 * different from the subject (or a sample of, part, or region of 2297 * the subject), the `focus` element or the `code` itself specifies 2298 * the actual focus of the observation.) 2299 */ 2300 public Observation setSubject(Reference value) { 2301 this.subject = value; 2302 return this; 2303 } 2304 2305 /** 2306 * @return {@link #subject} The actual object that is the target of the 2307 * reference. The reference library doesn't populate this, but you can 2308 * use it to hold the resource if you resolve it. (The patient, or group 2309 * of patients, location, or device this observation is about and into 2310 * whose record the observation is placed. If the actual focus of the 2311 * observation is different from the subject (or a sample of, part, or 2312 * region of the subject), the `focus` element or the `code` itself 2313 * specifies the actual focus of the observation.) 2314 */ 2315 public Resource getSubjectTarget() { 2316 return this.subjectTarget; 2317 } 2318 2319 /** 2320 * @param value {@link #subject} The actual object that is the target of the 2321 * reference. The reference library doesn't use these, but you can 2322 * use it to hold the resource if you resolve it. (The patient, or 2323 * group of patients, location, or device this observation is about 2324 * and into whose record the observation is placed. If the actual 2325 * focus of the observation is different from the subject (or a 2326 * sample of, part, or region of the subject), the `focus` element 2327 * or the `code` itself specifies the actual focus of the 2328 * observation.) 2329 */ 2330 public Observation setSubjectTarget(Resource value) { 2331 this.subjectTarget = value; 2332 return this; 2333 } 2334 2335 /** 2336 * @return {@link #focus} (The actual focus of an observation when it is not the 2337 * patient of record representing something or someone associated with 2338 * the patient such as a spouse, parent, fetus, or donor. For example, 2339 * fetus observations in a mother's record. The focus of an observation 2340 * could also be an existing condition, an intervention, the subject's 2341 * diet, another observation of the subject, or a body structure such as 2342 * tumor or implanted device. An example use case would be using the 2343 * Observation resource to capture whether the mother is trained to 2344 * change her child's tracheostomy tube. In this example, the child is 2345 * the patient of record and the mother is the focus.) 2346 */ 2347 public List<Reference> getFocus() { 2348 if (this.focus == null) 2349 this.focus = new ArrayList<Reference>(); 2350 return this.focus; 2351 } 2352 2353 /** 2354 * @return Returns a reference to <code>this</code> for easy method chaining 2355 */ 2356 public Observation setFocus(List<Reference> theFocus) { 2357 this.focus = theFocus; 2358 return this; 2359 } 2360 2361 public boolean hasFocus() { 2362 if (this.focus == null) 2363 return false; 2364 for (Reference item : this.focus) 2365 if (!item.isEmpty()) 2366 return true; 2367 return false; 2368 } 2369 2370 public Reference addFocus() { // 3 2371 Reference t = new Reference(); 2372 if (this.focus == null) 2373 this.focus = new ArrayList<Reference>(); 2374 this.focus.add(t); 2375 return t; 2376 } 2377 2378 public Observation addFocus(Reference t) { // 3 2379 if (t == null) 2380 return this; 2381 if (this.focus == null) 2382 this.focus = new ArrayList<Reference>(); 2383 this.focus.add(t); 2384 return this; 2385 } 2386 2387 /** 2388 * @return The first repetition of repeating field {@link #focus}, creating it 2389 * if it does not already exist 2390 */ 2391 public Reference getFocusFirstRep() { 2392 if (getFocus().isEmpty()) { 2393 addFocus(); 2394 } 2395 return getFocus().get(0); 2396 } 2397 2398 /** 2399 * @deprecated Use Reference#setResource(IBaseResource) instead 2400 */ 2401 @Deprecated 2402 public List<Resource> getFocusTarget() { 2403 if (this.focusTarget == null) 2404 this.focusTarget = new ArrayList<Resource>(); 2405 return this.focusTarget; 2406 } 2407 2408 /** 2409 * @return {@link #encounter} (The healthcare event (e.g. a patient and 2410 * healthcare provider interaction) during which this observation is 2411 * made.) 2412 */ 2413 public Reference getEncounter() { 2414 if (this.encounter == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create Observation.encounter"); 2417 else if (Configuration.doAutoCreate()) 2418 this.encounter = new Reference(); // cc 2419 return this.encounter; 2420 } 2421 2422 public boolean hasEncounter() { 2423 return this.encounter != null && !this.encounter.isEmpty(); 2424 } 2425 2426 /** 2427 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 2428 * healthcare provider interaction) during which this observation 2429 * is made.) 2430 */ 2431 public Observation setEncounter(Reference value) { 2432 this.encounter = value; 2433 return this; 2434 } 2435 2436 /** 2437 * @return {@link #encounter} The actual object that is the target of the 2438 * reference. The reference library doesn't populate this, but you can 2439 * use it to hold the resource if you resolve it. (The healthcare event 2440 * (e.g. a patient and healthcare provider interaction) during which 2441 * this observation is made.) 2442 */ 2443 public Encounter getEncounterTarget() { 2444 if (this.encounterTarget == null) 2445 if (Configuration.errorOnAutoCreate()) 2446 throw new Error("Attempt to auto-create Observation.encounter"); 2447 else if (Configuration.doAutoCreate()) 2448 this.encounterTarget = new Encounter(); // aa 2449 return this.encounterTarget; 2450 } 2451 2452 /** 2453 * @param value {@link #encounter} The actual object that is the target of the 2454 * reference. The reference library doesn't use these, but you can 2455 * use it to hold the resource if you resolve it. (The healthcare 2456 * event (e.g. a patient and healthcare provider interaction) 2457 * during which this observation is made.) 2458 */ 2459 public Observation setEncounterTarget(Encounter value) { 2460 this.encounterTarget = value; 2461 return this; 2462 } 2463 2464 /** 2465 * @return {@link #effective} (The time or time-period the observed value is 2466 * asserted as being true. For biological subjects - e.g. human patients 2467 * - this is usually called the "physiologically relevant time". This is 2468 * usually either the time of the procedure or of specimen collection, 2469 * but very often the source of the date/time is not known, only the 2470 * date/time itself.) 2471 */ 2472 public Type getEffective() { 2473 return this.effective; 2474 } 2475 2476 /** 2477 * @return {@link #effective} (The time or time-period the observed value is 2478 * asserted as being true. For biological subjects - e.g. human patients 2479 * - this is usually called the "physiologically relevant time". This is 2480 * usually either the time of the procedure or of specimen collection, 2481 * but very often the source of the date/time is not known, only the 2482 * date/time itself.) 2483 */ 2484 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 2485 if (this.effective == null) 2486 this.effective = new DateTimeType(); 2487 if (!(this.effective instanceof DateTimeType)) 2488 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2489 + this.effective.getClass().getName() + " was encountered"); 2490 return (DateTimeType) this.effective; 2491 } 2492 2493 public boolean hasEffectiveDateTimeType() { 2494 return this != null && this.effective instanceof DateTimeType; 2495 } 2496 2497 /** 2498 * @return {@link #effective} (The time or time-period the observed value is 2499 * asserted as being true. For biological subjects - e.g. human patients 2500 * - this is usually called the "physiologically relevant time". This is 2501 * usually either the time of the procedure or of specimen collection, 2502 * but very often the source of the date/time is not known, only the 2503 * date/time itself.) 2504 */ 2505 public Period getEffectivePeriod() throws FHIRException { 2506 if (this.effective == null) 2507 this.effective = new Period(); 2508 if (!(this.effective instanceof Period)) 2509 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 2510 + " was encountered"); 2511 return (Period) this.effective; 2512 } 2513 2514 public boolean hasEffectivePeriod() { 2515 return this != null && this.effective instanceof Period; 2516 } 2517 2518 /** 2519 * @return {@link #effective} (The time or time-period the observed value is 2520 * asserted as being true. For biological subjects - e.g. human patients 2521 * - this is usually called the "physiologically relevant time". This is 2522 * usually either the time of the procedure or of specimen collection, 2523 * but very often the source of the date/time is not known, only the 2524 * date/time itself.) 2525 */ 2526 public Timing getEffectiveTiming() throws FHIRException { 2527 if (this.effective == null) 2528 this.effective = new Timing(); 2529 if (!(this.effective instanceof Timing)) 2530 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.effective.getClass().getName() 2531 + " was encountered"); 2532 return (Timing) this.effective; 2533 } 2534 2535 public boolean hasEffectiveTiming() { 2536 return this != null && this.effective instanceof Timing; 2537 } 2538 2539 /** 2540 * @return {@link #effective} (The time or time-period the observed value is 2541 * asserted as being true. For biological subjects - e.g. human patients 2542 * - this is usually called the "physiologically relevant time". This is 2543 * usually either the time of the procedure or of specimen collection, 2544 * but very often the source of the date/time is not known, only the 2545 * date/time itself.) 2546 */ 2547 public InstantType getEffectiveInstantType() throws FHIRException { 2548 if (this.effective == null) 2549 this.effective = new InstantType(); 2550 if (!(this.effective instanceof InstantType)) 2551 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 2552 + this.effective.getClass().getName() + " was encountered"); 2553 return (InstantType) this.effective; 2554 } 2555 2556 public boolean hasEffectiveInstantType() { 2557 return this != null && this.effective instanceof InstantType; 2558 } 2559 2560 public boolean hasEffective() { 2561 return this.effective != null && !this.effective.isEmpty(); 2562 } 2563 2564 /** 2565 * @param value {@link #effective} (The time or time-period the observed value 2566 * is asserted as being true. For biological subjects - e.g. human 2567 * patients - this is usually called the "physiologically relevant 2568 * time". This is usually either the time of the procedure or of 2569 * specimen collection, but very often the source of the date/time 2570 * is not known, only the date/time itself.) 2571 */ 2572 public Observation setEffective(Type value) { 2573 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing 2574 || value instanceof InstantType)) 2575 throw new Error("Not the right type for Observation.effective[x]: " + value.fhirType()); 2576 this.effective = value; 2577 return this; 2578 } 2579 2580 /** 2581 * @return {@link #issued} (The date and time this version of the observation 2582 * was made available to providers, typically after the results have 2583 * been reviewed and verified.). This is the underlying object with id, 2584 * value and extensions. The accessor "getIssued" gives direct access to 2585 * the value 2586 */ 2587 public InstantType getIssuedElement() { 2588 if (this.issued == null) 2589 if (Configuration.errorOnAutoCreate()) 2590 throw new Error("Attempt to auto-create Observation.issued"); 2591 else if (Configuration.doAutoCreate()) 2592 this.issued = new InstantType(); // bb 2593 return this.issued; 2594 } 2595 2596 public boolean hasIssuedElement() { 2597 return this.issued != null && !this.issued.isEmpty(); 2598 } 2599 2600 public boolean hasIssued() { 2601 return this.issued != null && !this.issued.isEmpty(); 2602 } 2603 2604 /** 2605 * @param value {@link #issued} (The date and time this version of the 2606 * observation was made available to providers, typically after the 2607 * results have been reviewed and verified.). This is the 2608 * underlying object with id, value and extensions. The accessor 2609 * "getIssued" gives direct access to the value 2610 */ 2611 public Observation setIssuedElement(InstantType value) { 2612 this.issued = value; 2613 return this; 2614 } 2615 2616 /** 2617 * @return The date and time this version of the observation was made available 2618 * to providers, typically after the results have been reviewed and 2619 * verified. 2620 */ 2621 public Date getIssued() { 2622 return this.issued == null ? null : this.issued.getValue(); 2623 } 2624 2625 /** 2626 * @param value The date and time this version of the observation was made 2627 * available to providers, typically after the results have been 2628 * reviewed and verified. 2629 */ 2630 public Observation setIssued(Date value) { 2631 if (value == null) 2632 this.issued = null; 2633 else { 2634 if (this.issued == null) 2635 this.issued = new InstantType(); 2636 this.issued.setValue(value); 2637 } 2638 return this; 2639 } 2640 2641 /** 2642 * @return {@link #performer} (Who was responsible for asserting the observed 2643 * value as "true".) 2644 */ 2645 public List<Reference> getPerformer() { 2646 if (this.performer == null) 2647 this.performer = new ArrayList<Reference>(); 2648 return this.performer; 2649 } 2650 2651 /** 2652 * @return Returns a reference to <code>this</code> for easy method chaining 2653 */ 2654 public Observation setPerformer(List<Reference> thePerformer) { 2655 this.performer = thePerformer; 2656 return this; 2657 } 2658 2659 public boolean hasPerformer() { 2660 if (this.performer == null) 2661 return false; 2662 for (Reference item : this.performer) 2663 if (!item.isEmpty()) 2664 return true; 2665 return false; 2666 } 2667 2668 public Reference addPerformer() { // 3 2669 Reference t = new Reference(); 2670 if (this.performer == null) 2671 this.performer = new ArrayList<Reference>(); 2672 this.performer.add(t); 2673 return t; 2674 } 2675 2676 public Observation addPerformer(Reference t) { // 3 2677 if (t == null) 2678 return this; 2679 if (this.performer == null) 2680 this.performer = new ArrayList<Reference>(); 2681 this.performer.add(t); 2682 return this; 2683 } 2684 2685 /** 2686 * @return The first repetition of repeating field {@link #performer}, creating 2687 * it if it does not already exist 2688 */ 2689 public Reference getPerformerFirstRep() { 2690 if (getPerformer().isEmpty()) { 2691 addPerformer(); 2692 } 2693 return getPerformer().get(0); 2694 } 2695 2696 /** 2697 * @deprecated Use Reference#setResource(IBaseResource) instead 2698 */ 2699 @Deprecated 2700 public List<Resource> getPerformerTarget() { 2701 if (this.performerTarget == null) 2702 this.performerTarget = new ArrayList<Resource>(); 2703 return this.performerTarget; 2704 } 2705 2706 /** 2707 * @return {@link #value} (The information determined as a result of making the 2708 * observation, if the information has a simple value.) 2709 */ 2710 public Type getValue() { 2711 return this.value; 2712 } 2713 2714 /** 2715 * @return {@link #value} (The information determined as a result of making the 2716 * observation, if the information has a simple value.) 2717 */ 2718 public Quantity getValueQuantity() throws FHIRException { 2719 if (this.value == null) 2720 this.value = new Quantity(); 2721 if (!(this.value instanceof Quantity)) 2722 throw new FHIRException( 2723 "Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() + " was encountered"); 2724 return (Quantity) this.value; 2725 } 2726 2727 public boolean hasValueQuantity() { 2728 return this != null && this.value instanceof Quantity; 2729 } 2730 2731 /** 2732 * @return {@link #value} (The information determined as a result of making the 2733 * observation, if the information has a simple value.) 2734 */ 2735 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2736 if (this.value == null) 2737 this.value = new CodeableConcept(); 2738 if (!(this.value instanceof CodeableConcept)) 2739 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2740 + this.value.getClass().getName() + " was encountered"); 2741 return (CodeableConcept) this.value; 2742 } 2743 2744 public boolean hasValueCodeableConcept() { 2745 return this != null && this.value instanceof CodeableConcept; 2746 } 2747 2748 /** 2749 * @return {@link #value} (The information determined as a result of making the 2750 * observation, if the information has a simple value.) 2751 */ 2752 public StringType getValueStringType() throws FHIRException { 2753 if (this.value == null) 2754 this.value = new StringType(); 2755 if (!(this.value instanceof StringType)) 2756 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.value.getClass().getName() 2757 + " was encountered"); 2758 return (StringType) this.value; 2759 } 2760 2761 public boolean hasValueStringType() { 2762 return this != null && this.value instanceof StringType; 2763 } 2764 2765 /** 2766 * @return {@link #value} (The information determined as a result of making the 2767 * observation, if the information has a simple value.) 2768 */ 2769 public BooleanType getValueBooleanType() throws FHIRException { 2770 if (this.value == null) 2771 this.value = new BooleanType(); 2772 if (!(this.value instanceof BooleanType)) 2773 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " + this.value.getClass().getName() 2774 + " was encountered"); 2775 return (BooleanType) this.value; 2776 } 2777 2778 public boolean hasValueBooleanType() { 2779 return this != null && this.value instanceof BooleanType; 2780 } 2781 2782 /** 2783 * @return {@link #value} (The information determined as a result of making the 2784 * observation, if the information has a simple value.) 2785 */ 2786 public IntegerType getValueIntegerType() throws FHIRException { 2787 if (this.value == null) 2788 this.value = new IntegerType(); 2789 if (!(this.value instanceof IntegerType)) 2790 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " + this.value.getClass().getName() 2791 + " was encountered"); 2792 return (IntegerType) this.value; 2793 } 2794 2795 public boolean hasValueIntegerType() { 2796 return this != null && this.value instanceof IntegerType; 2797 } 2798 2799 /** 2800 * @return {@link #value} (The information determined as a result of making the 2801 * observation, if the information has a simple value.) 2802 */ 2803 public Range getValueRange() throws FHIRException { 2804 if (this.value == null) 2805 this.value = new Range(); 2806 if (!(this.value instanceof Range)) 2807 throw new FHIRException( 2808 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 2809 return (Range) this.value; 2810 } 2811 2812 public boolean hasValueRange() { 2813 return this != null && this.value instanceof Range; 2814 } 2815 2816 /** 2817 * @return {@link #value} (The information determined as a result of making the 2818 * observation, if the information has a simple value.) 2819 */ 2820 public Ratio getValueRatio() throws FHIRException { 2821 if (this.value == null) 2822 this.value = new Ratio(); 2823 if (!(this.value instanceof Ratio)) 2824 throw new FHIRException( 2825 "Type mismatch: the type Ratio was expected, but " + this.value.getClass().getName() + " was encountered"); 2826 return (Ratio) this.value; 2827 } 2828 2829 public boolean hasValueRatio() { 2830 return this != null && this.value instanceof Ratio; 2831 } 2832 2833 /** 2834 * @return {@link #value} (The information determined as a result of making the 2835 * observation, if the information has a simple value.) 2836 */ 2837 public SampledData getValueSampledData() throws FHIRException { 2838 if (this.value == null) 2839 this.value = new SampledData(); 2840 if (!(this.value instanceof SampledData)) 2841 throw new FHIRException("Type mismatch: the type SampledData was expected, but " + this.value.getClass().getName() 2842 + " was encountered"); 2843 return (SampledData) this.value; 2844 } 2845 2846 public boolean hasValueSampledData() { 2847 return this != null && this.value instanceof SampledData; 2848 } 2849 2850 /** 2851 * @return {@link #value} (The information determined as a result of making the 2852 * observation, if the information has a simple value.) 2853 */ 2854 public TimeType getValueTimeType() throws FHIRException { 2855 if (this.value == null) 2856 this.value = new TimeType(); 2857 if (!(this.value instanceof TimeType)) 2858 throw new FHIRException( 2859 "Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() + " was encountered"); 2860 return (TimeType) this.value; 2861 } 2862 2863 public boolean hasValueTimeType() { 2864 return this != null && this.value instanceof TimeType; 2865 } 2866 2867 /** 2868 * @return {@link #value} (The information determined as a result of making the 2869 * observation, if the information has a simple value.) 2870 */ 2871 public DateTimeType getValueDateTimeType() throws FHIRException { 2872 if (this.value == null) 2873 this.value = new DateTimeType(); 2874 if (!(this.value instanceof DateTimeType)) 2875 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2876 + this.value.getClass().getName() + " was encountered"); 2877 return (DateTimeType) this.value; 2878 } 2879 2880 public boolean hasValueDateTimeType() { 2881 return this != null && this.value instanceof DateTimeType; 2882 } 2883 2884 /** 2885 * @return {@link #value} (The information determined as a result of making the 2886 * observation, if the information has a simple value.) 2887 */ 2888 public Period getValuePeriod() throws FHIRException { 2889 if (this.value == null) 2890 this.value = new Period(); 2891 if (!(this.value instanceof Period)) 2892 throw new FHIRException( 2893 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 2894 return (Period) this.value; 2895 } 2896 2897 public boolean hasValuePeriod() { 2898 return this != null && this.value instanceof Period; 2899 } 2900 2901 public boolean hasValue() { 2902 return this.value != null && !this.value.isEmpty(); 2903 } 2904 2905 /** 2906 * @param value {@link #value} (The information determined as a result of making 2907 * the observation, if the information has a simple value.) 2908 */ 2909 public Observation setValue(Type value) { 2910 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType 2911 || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range 2912 || value instanceof Ratio || value instanceof SampledData || value instanceof TimeType 2913 || value instanceof DateTimeType || value instanceof Period)) 2914 throw new Error("Not the right type for Observation.value[x]: " + value.fhirType()); 2915 this.value = value; 2916 return this; 2917 } 2918 2919 /** 2920 * @return {@link #dataAbsentReason} (Provides a reason why the expected value 2921 * in the element Observation.value[x] is missing.) 2922 */ 2923 public CodeableConcept getDataAbsentReason() { 2924 if (this.dataAbsentReason == null) 2925 if (Configuration.errorOnAutoCreate()) 2926 throw new Error("Attempt to auto-create Observation.dataAbsentReason"); 2927 else if (Configuration.doAutoCreate()) 2928 this.dataAbsentReason = new CodeableConcept(); // cc 2929 return this.dataAbsentReason; 2930 } 2931 2932 public boolean hasDataAbsentReason() { 2933 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 2934 } 2935 2936 /** 2937 * @param value {@link #dataAbsentReason} (Provides a reason why the expected 2938 * value in the element Observation.value[x] is missing.) 2939 */ 2940 public Observation setDataAbsentReason(CodeableConcept value) { 2941 this.dataAbsentReason = value; 2942 return this; 2943 } 2944 2945 /** 2946 * @return {@link #interpretation} (A categorical assessment of an observation 2947 * value. For example, high, low, normal.) 2948 */ 2949 public List<CodeableConcept> getInterpretation() { 2950 if (this.interpretation == null) 2951 this.interpretation = new ArrayList<CodeableConcept>(); 2952 return this.interpretation; 2953 } 2954 2955 /** 2956 * @return Returns a reference to <code>this</code> for easy method chaining 2957 */ 2958 public Observation setInterpretation(List<CodeableConcept> theInterpretation) { 2959 this.interpretation = theInterpretation; 2960 return this; 2961 } 2962 2963 public boolean hasInterpretation() { 2964 if (this.interpretation == null) 2965 return false; 2966 for (CodeableConcept item : this.interpretation) 2967 if (!item.isEmpty()) 2968 return true; 2969 return false; 2970 } 2971 2972 public CodeableConcept addInterpretation() { // 3 2973 CodeableConcept t = new CodeableConcept(); 2974 if (this.interpretation == null) 2975 this.interpretation = new ArrayList<CodeableConcept>(); 2976 this.interpretation.add(t); 2977 return t; 2978 } 2979 2980 public Observation addInterpretation(CodeableConcept t) { // 3 2981 if (t == null) 2982 return this; 2983 if (this.interpretation == null) 2984 this.interpretation = new ArrayList<CodeableConcept>(); 2985 this.interpretation.add(t); 2986 return this; 2987 } 2988 2989 /** 2990 * @return The first repetition of repeating field {@link #interpretation}, 2991 * creating it if it does not already exist 2992 */ 2993 public CodeableConcept getInterpretationFirstRep() { 2994 if (getInterpretation().isEmpty()) { 2995 addInterpretation(); 2996 } 2997 return getInterpretation().get(0); 2998 } 2999 3000 /** 3001 * @return {@link #note} (Comments about the observation or the results.) 3002 */ 3003 public List<Annotation> getNote() { 3004 if (this.note == null) 3005 this.note = new ArrayList<Annotation>(); 3006 return this.note; 3007 } 3008 3009 /** 3010 * @return Returns a reference to <code>this</code> for easy method chaining 3011 */ 3012 public Observation setNote(List<Annotation> theNote) { 3013 this.note = theNote; 3014 return this; 3015 } 3016 3017 public boolean hasNote() { 3018 if (this.note == null) 3019 return false; 3020 for (Annotation item : this.note) 3021 if (!item.isEmpty()) 3022 return true; 3023 return false; 3024 } 3025 3026 public Annotation addNote() { // 3 3027 Annotation t = new Annotation(); 3028 if (this.note == null) 3029 this.note = new ArrayList<Annotation>(); 3030 this.note.add(t); 3031 return t; 3032 } 3033 3034 public Observation addNote(Annotation t) { // 3 3035 if (t == null) 3036 return this; 3037 if (this.note == null) 3038 this.note = new ArrayList<Annotation>(); 3039 this.note.add(t); 3040 return this; 3041 } 3042 3043 /** 3044 * @return The first repetition of repeating field {@link #note}, creating it if 3045 * it does not already exist 3046 */ 3047 public Annotation getNoteFirstRep() { 3048 if (getNote().isEmpty()) { 3049 addNote(); 3050 } 3051 return getNote().get(0); 3052 } 3053 3054 /** 3055 * @return {@link #bodySite} (Indicates the site on the subject's body where the 3056 * observation was made (i.e. the target site).) 3057 */ 3058 public CodeableConcept getBodySite() { 3059 if (this.bodySite == null) 3060 if (Configuration.errorOnAutoCreate()) 3061 throw new Error("Attempt to auto-create Observation.bodySite"); 3062 else if (Configuration.doAutoCreate()) 3063 this.bodySite = new CodeableConcept(); // cc 3064 return this.bodySite; 3065 } 3066 3067 public boolean hasBodySite() { 3068 return this.bodySite != null && !this.bodySite.isEmpty(); 3069 } 3070 3071 /** 3072 * @param value {@link #bodySite} (Indicates the site on the subject's body 3073 * where the observation was made (i.e. the target site).) 3074 */ 3075 public Observation setBodySite(CodeableConcept value) { 3076 this.bodySite = value; 3077 return this; 3078 } 3079 3080 /** 3081 * @return {@link #method} (Indicates the mechanism used to perform the 3082 * observation.) 3083 */ 3084 public CodeableConcept getMethod() { 3085 if (this.method == null) 3086 if (Configuration.errorOnAutoCreate()) 3087 throw new Error("Attempt to auto-create Observation.method"); 3088 else if (Configuration.doAutoCreate()) 3089 this.method = new CodeableConcept(); // cc 3090 return this.method; 3091 } 3092 3093 public boolean hasMethod() { 3094 return this.method != null && !this.method.isEmpty(); 3095 } 3096 3097 /** 3098 * @param value {@link #method} (Indicates the mechanism used to perform the 3099 * observation.) 3100 */ 3101 public Observation setMethod(CodeableConcept value) { 3102 this.method = value; 3103 return this; 3104 } 3105 3106 /** 3107 * @return {@link #specimen} (The specimen that was used when this observation 3108 * was made.) 3109 */ 3110 public Reference getSpecimen() { 3111 if (this.specimen == null) 3112 if (Configuration.errorOnAutoCreate()) 3113 throw new Error("Attempt to auto-create Observation.specimen"); 3114 else if (Configuration.doAutoCreate()) 3115 this.specimen = new Reference(); // cc 3116 return this.specimen; 3117 } 3118 3119 public boolean hasSpecimen() { 3120 return this.specimen != null && !this.specimen.isEmpty(); 3121 } 3122 3123 /** 3124 * @param value {@link #specimen} (The specimen that was used when this 3125 * observation was made.) 3126 */ 3127 public Observation setSpecimen(Reference value) { 3128 this.specimen = value; 3129 return this; 3130 } 3131 3132 /** 3133 * @return {@link #specimen} The actual object that is the target of the 3134 * reference. The reference library doesn't populate this, but you can 3135 * use it to hold the resource if you resolve it. (The specimen that was 3136 * used when this observation was made.) 3137 */ 3138 public Specimen getSpecimenTarget() { 3139 if (this.specimenTarget == null) 3140 if (Configuration.errorOnAutoCreate()) 3141 throw new Error("Attempt to auto-create Observation.specimen"); 3142 else if (Configuration.doAutoCreate()) 3143 this.specimenTarget = new Specimen(); // aa 3144 return this.specimenTarget; 3145 } 3146 3147 /** 3148 * @param value {@link #specimen} The actual object that is the target of the 3149 * reference. The reference library doesn't use these, but you can 3150 * use it to hold the resource if you resolve it. (The specimen 3151 * that was used when this observation was made.) 3152 */ 3153 public Observation setSpecimenTarget(Specimen value) { 3154 this.specimenTarget = value; 3155 return this; 3156 } 3157 3158 /** 3159 * @return {@link #device} (The device used to generate the observation data.) 3160 */ 3161 public Reference getDevice() { 3162 if (this.device == null) 3163 if (Configuration.errorOnAutoCreate()) 3164 throw new Error("Attempt to auto-create Observation.device"); 3165 else if (Configuration.doAutoCreate()) 3166 this.device = new Reference(); // cc 3167 return this.device; 3168 } 3169 3170 public boolean hasDevice() { 3171 return this.device != null && !this.device.isEmpty(); 3172 } 3173 3174 /** 3175 * @param value {@link #device} (The device used to generate the observation 3176 * data.) 3177 */ 3178 public Observation setDevice(Reference value) { 3179 this.device = value; 3180 return this; 3181 } 3182 3183 /** 3184 * @return {@link #device} The actual object that is the target of the 3185 * reference. The reference library doesn't populate this, but you can 3186 * use it to hold the resource if you resolve it. (The device used to 3187 * generate the observation data.) 3188 */ 3189 public Resource getDeviceTarget() { 3190 return this.deviceTarget; 3191 } 3192 3193 /** 3194 * @param value {@link #device} The actual object that is the target of the 3195 * reference. The reference library doesn't use these, but you can 3196 * use it to hold the resource if you resolve it. (The device used 3197 * to generate the observation data.) 3198 */ 3199 public Observation setDeviceTarget(Resource value) { 3200 this.deviceTarget = value; 3201 return this; 3202 } 3203 3204 /** 3205 * @return {@link #referenceRange} (Guidance on how to interpret the value by 3206 * comparison to a normal or recommended range. Multiple reference 3207 * ranges are interpreted as an "OR". In other words, to represent two 3208 * distinct target populations, two `referenceRange` elements would be 3209 * used.) 3210 */ 3211 public List<ObservationReferenceRangeComponent> getReferenceRange() { 3212 if (this.referenceRange == null) 3213 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3214 return this.referenceRange; 3215 } 3216 3217 /** 3218 * @return Returns a reference to <code>this</code> for easy method chaining 3219 */ 3220 public Observation setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 3221 this.referenceRange = theReferenceRange; 3222 return this; 3223 } 3224 3225 public boolean hasReferenceRange() { 3226 if (this.referenceRange == null) 3227 return false; 3228 for (ObservationReferenceRangeComponent item : this.referenceRange) 3229 if (!item.isEmpty()) 3230 return true; 3231 return false; 3232 } 3233 3234 public ObservationReferenceRangeComponent addReferenceRange() { // 3 3235 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 3236 if (this.referenceRange == null) 3237 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3238 this.referenceRange.add(t); 3239 return t; 3240 } 3241 3242 public Observation addReferenceRange(ObservationReferenceRangeComponent t) { // 3 3243 if (t == null) 3244 return this; 3245 if (this.referenceRange == null) 3246 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3247 this.referenceRange.add(t); 3248 return this; 3249 } 3250 3251 /** 3252 * @return The first repetition of repeating field {@link #referenceRange}, 3253 * creating it if it does not already exist 3254 */ 3255 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 3256 if (getReferenceRange().isEmpty()) { 3257 addReferenceRange(); 3258 } 3259 return getReferenceRange().get(0); 3260 } 3261 3262 /** 3263 * @return {@link #hasMember} (This observation is a group observation (e.g. a 3264 * battery, a panel of tests, a set of vital sign measurements) that 3265 * includes the target as a member of the group.) 3266 */ 3267 public List<Reference> getHasMember() { 3268 if (this.hasMember == null) 3269 this.hasMember = new ArrayList<Reference>(); 3270 return this.hasMember; 3271 } 3272 3273 /** 3274 * @return Returns a reference to <code>this</code> for easy method chaining 3275 */ 3276 public Observation setHasMember(List<Reference> theHasMember) { 3277 this.hasMember = theHasMember; 3278 return this; 3279 } 3280 3281 public boolean hasHasMember() { 3282 if (this.hasMember == null) 3283 return false; 3284 for (Reference item : this.hasMember) 3285 if (!item.isEmpty()) 3286 return true; 3287 return false; 3288 } 3289 3290 public Reference addHasMember() { // 3 3291 Reference t = new Reference(); 3292 if (this.hasMember == null) 3293 this.hasMember = new ArrayList<Reference>(); 3294 this.hasMember.add(t); 3295 return t; 3296 } 3297 3298 public Observation addHasMember(Reference t) { // 3 3299 if (t == null) 3300 return this; 3301 if (this.hasMember == null) 3302 this.hasMember = new ArrayList<Reference>(); 3303 this.hasMember.add(t); 3304 return this; 3305 } 3306 3307 /** 3308 * @return The first repetition of repeating field {@link #hasMember}, creating 3309 * it if it does not already exist 3310 */ 3311 public Reference getHasMemberFirstRep() { 3312 if (getHasMember().isEmpty()) { 3313 addHasMember(); 3314 } 3315 return getHasMember().get(0); 3316 } 3317 3318 /** 3319 * @deprecated Use Reference#setResource(IBaseResource) instead 3320 */ 3321 @Deprecated 3322 public List<Resource> getHasMemberTarget() { 3323 if (this.hasMemberTarget == null) 3324 this.hasMemberTarget = new ArrayList<Resource>(); 3325 return this.hasMemberTarget; 3326 } 3327 3328 /** 3329 * @return {@link #derivedFrom} (The target resource that represents a 3330 * measurement from which this observation value is derived. For 3331 * example, a calculated anion gap or a fetal measurement based on an 3332 * ultrasound image.) 3333 */ 3334 public List<Reference> getDerivedFrom() { 3335 if (this.derivedFrom == null) 3336 this.derivedFrom = new ArrayList<Reference>(); 3337 return this.derivedFrom; 3338 } 3339 3340 /** 3341 * @return Returns a reference to <code>this</code> for easy method chaining 3342 */ 3343 public Observation setDerivedFrom(List<Reference> theDerivedFrom) { 3344 this.derivedFrom = theDerivedFrom; 3345 return this; 3346 } 3347 3348 public boolean hasDerivedFrom() { 3349 if (this.derivedFrom == null) 3350 return false; 3351 for (Reference item : this.derivedFrom) 3352 if (!item.isEmpty()) 3353 return true; 3354 return false; 3355 } 3356 3357 public Reference addDerivedFrom() { // 3 3358 Reference t = new Reference(); 3359 if (this.derivedFrom == null) 3360 this.derivedFrom = new ArrayList<Reference>(); 3361 this.derivedFrom.add(t); 3362 return t; 3363 } 3364 3365 public Observation addDerivedFrom(Reference t) { // 3 3366 if (t == null) 3367 return this; 3368 if (this.derivedFrom == null) 3369 this.derivedFrom = new ArrayList<Reference>(); 3370 this.derivedFrom.add(t); 3371 return this; 3372 } 3373 3374 /** 3375 * @return The first repetition of repeating field {@link #derivedFrom}, 3376 * creating it if it does not already exist 3377 */ 3378 public Reference getDerivedFromFirstRep() { 3379 if (getDerivedFrom().isEmpty()) { 3380 addDerivedFrom(); 3381 } 3382 return getDerivedFrom().get(0); 3383 } 3384 3385 /** 3386 * @deprecated Use Reference#setResource(IBaseResource) instead 3387 */ 3388 @Deprecated 3389 public List<Resource> getDerivedFromTarget() { 3390 if (this.derivedFromTarget == null) 3391 this.derivedFromTarget = new ArrayList<Resource>(); 3392 return this.derivedFromTarget; 3393 } 3394 3395 /** 3396 * @return {@link #component} (Some observations have multiple component 3397 * observations. These component observations are expressed as separate 3398 * code value pairs that share the same attributes. Examples include 3399 * systolic and diastolic component observations for blood pressure 3400 * measurement and multiple component observations for genetics 3401 * observations.) 3402 */ 3403 public List<ObservationComponentComponent> getComponent() { 3404 if (this.component == null) 3405 this.component = new ArrayList<ObservationComponentComponent>(); 3406 return this.component; 3407 } 3408 3409 /** 3410 * @return Returns a reference to <code>this</code> for easy method chaining 3411 */ 3412 public Observation setComponent(List<ObservationComponentComponent> theComponent) { 3413 this.component = theComponent; 3414 return this; 3415 } 3416 3417 public boolean hasComponent() { 3418 if (this.component == null) 3419 return false; 3420 for (ObservationComponentComponent item : this.component) 3421 if (!item.isEmpty()) 3422 return true; 3423 return false; 3424 } 3425 3426 public ObservationComponentComponent addComponent() { // 3 3427 ObservationComponentComponent t = new ObservationComponentComponent(); 3428 if (this.component == null) 3429 this.component = new ArrayList<ObservationComponentComponent>(); 3430 this.component.add(t); 3431 return t; 3432 } 3433 3434 public Observation addComponent(ObservationComponentComponent t) { // 3 3435 if (t == null) 3436 return this; 3437 if (this.component == null) 3438 this.component = new ArrayList<ObservationComponentComponent>(); 3439 this.component.add(t); 3440 return this; 3441 } 3442 3443 /** 3444 * @return The first repetition of repeating field {@link #component}, creating 3445 * it if it does not already exist 3446 */ 3447 public ObservationComponentComponent getComponentFirstRep() { 3448 if (getComponent().isEmpty()) { 3449 addComponent(); 3450 } 3451 return getComponent().get(0); 3452 } 3453 3454 protected void listChildren(List<Property> children) { 3455 super.listChildren(children); 3456 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, 3457 java.lang.Integer.MAX_VALUE, identifier)); 3458 children.add(new Property("basedOn", 3459 "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 3460 "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 3461 0, java.lang.Integer.MAX_VALUE, basedOn)); 3462 children.add(new Property("partOf", 3463 "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy)", 3464 "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 3465 0, java.lang.Integer.MAX_VALUE, partOf)); 3466 children.add(new Property("status", "code", "The status of the result value.", 0, 1, status)); 3467 children.add(new Property("category", "CodeableConcept", 3468 "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, 3469 category)); 3470 children.add(new Property("code", "CodeableConcept", 3471 "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 3472 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 3473 "The patient, or group of patients, location, or device this observation is about and into whose record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 3474 0, 1, subject)); 3475 children.add(new Property("focus", "Reference(Any)", 3476 "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 3477 0, java.lang.Integer.MAX_VALUE, focus)); 3478 children.add(new Property("encounter", "Reference(Encounter)", 3479 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 3480 0, 1, encounter)); 3481 children.add(new Property("effective[x]", "dateTime|Period|Timing|instant", 3482 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3483 0, 1, effective)); 3484 children.add(new Property("issued", "instant", 3485 "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 3486 0, 1, issued)); 3487 children.add(new Property("performer", 3488 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", 3489 "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, 3490 performer)); 3491 children.add(new Property("value[x]", 3492 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3493 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3494 1, value)); 3495 children.add(new Property("dataAbsentReason", "CodeableConcept", 3496 "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, 3497 dataAbsentReason)); 3498 children.add(new Property("interpretation", "CodeableConcept", 3499 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 3500 java.lang.Integer.MAX_VALUE, interpretation)); 3501 children.add(new Property("note", "Annotation", "Comments about the observation or the results.", 0, 3502 java.lang.Integer.MAX_VALUE, note)); 3503 children.add(new Property("bodySite", "CodeableConcept", 3504 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 3505 bodySite)); 3506 children.add(new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 3507 0, 1, method)); 3508 children.add(new Property("specimen", "Reference(Specimen)", 3509 "The specimen that was used when this observation was made.", 0, 1, specimen)); 3510 children.add(new Property("device", "Reference(Device|DeviceMetric)", 3511 "The device used to generate the observation data.", 0, 1, device)); 3512 children.add(new Property("referenceRange", "", 3513 "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 3514 0, java.lang.Integer.MAX_VALUE, referenceRange)); 3515 children.add(new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", 3516 "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 3517 0, java.lang.Integer.MAX_VALUE, hasMember)); 3518 children.add(new Property("derivedFrom", 3519 "Reference(DocumentReference|ImagingStudy|Media|QuestionnaireResponse|Observation|MolecularSequence)", 3520 "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 3521 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 3522 children.add(new Property("component", "", 3523 "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 3524 0, java.lang.Integer.MAX_VALUE, component)); 3525 } 3526 3527 @Override 3528 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3529 switch (_hash) { 3530 case -1618432855: 3531 /* identifier */ return new Property("identifier", "Identifier", 3532 "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier); 3533 case -332612366: 3534 /* basedOn */ return new Property("basedOn", 3535 "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 3536 "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 3537 0, java.lang.Integer.MAX_VALUE, basedOn); 3538 case -995410646: 3539 /* partOf */ return new Property("partOf", 3540 "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy)", 3541 "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 3542 0, java.lang.Integer.MAX_VALUE, partOf); 3543 case -892481550: 3544 /* status */ return new Property("status", "code", "The status of the result value.", 0, 1, status); 3545 case 50511102: 3546 /* category */ return new Property("category", "CodeableConcept", 3547 "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, 3548 category); 3549 case 3059181: 3550 /* code */ return new Property("code", "CodeableConcept", 3551 "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code); 3552 case -1867885268: 3553 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Location)", 3554 "The patient, or group of patients, location, or device this observation is about and into whose record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 3555 0, 1, subject); 3556 case 97604824: 3557 /* focus */ return new Property("focus", "Reference(Any)", 3558 "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 3559 0, java.lang.Integer.MAX_VALUE, focus); 3560 case 1524132147: 3561 /* encounter */ return new Property("encounter", "Reference(Encounter)", 3562 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 3563 0, 1, encounter); 3564 case 247104889: 3565 /* effective[x] */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3566 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3567 0, 1, effective); 3568 case -1468651097: 3569 /* effective */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3570 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3571 0, 1, effective); 3572 case -275306910: 3573 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3574 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3575 0, 1, effective); 3576 case -403934648: 3577 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3578 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3579 0, 1, effective); 3580 case -285872943: 3581 /* effectiveTiming */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3582 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3583 0, 1, effective); 3584 case -1295730118: 3585 /* effectiveInstant */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3586 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3587 0, 1, effective); 3588 case -1179159893: 3589 /* issued */ return new Property("issued", "instant", 3590 "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 3591 0, 1, issued); 3592 case 481140686: 3593 /* performer */ return new Property("performer", 3594 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", 3595 "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, 3596 performer); 3597 case -1410166417: 3598 /* value[x] */ return new Property("value[x]", 3599 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3600 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3601 1, value); 3602 case 111972721: 3603 /* value */ return new Property("value[x]", 3604 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3605 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3606 1, value); 3607 case -2029823716: 3608 /* valueQuantity */ return new Property("value[x]", 3609 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3610 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3611 1, value); 3612 case 924902896: 3613 /* valueCodeableConcept */ return new Property("value[x]", 3614 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3615 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3616 1, value); 3617 case -1424603934: 3618 /* valueString */ return new Property("value[x]", 3619 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3620 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3621 1, value); 3622 case 733421943: 3623 /* valueBoolean */ return new Property("value[x]", 3624 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3625 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3626 1, value); 3627 case -1668204915: 3628 /* valueInteger */ return new Property("value[x]", 3629 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3630 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3631 1, value); 3632 case 2030761548: 3633 /* valueRange */ return new Property("value[x]", 3634 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3635 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3636 1, value); 3637 case 2030767386: 3638 /* valueRatio */ return new Property("value[x]", 3639 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3640 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3641 1, value); 3642 case -962229101: 3643 /* valueSampledData */ return new Property("value[x]", 3644 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3645 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3646 1, value); 3647 case -765708322: 3648 /* valueTime */ return new Property("value[x]", 3649 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3650 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3651 1, value); 3652 case 1047929900: 3653 /* valueDateTime */ return new Property("value[x]", 3654 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3655 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3656 1, value); 3657 case -1524344174: 3658 /* valuePeriod */ return new Property("value[x]", 3659 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3660 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3661 1, value); 3662 case 1034315687: 3663 /* dataAbsentReason */ return new Property("dataAbsentReason", "CodeableConcept", 3664 "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, 3665 dataAbsentReason); 3666 case -297950712: 3667 /* interpretation */ return new Property("interpretation", "CodeableConcept", 3668 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 3669 java.lang.Integer.MAX_VALUE, interpretation); 3670 case 3387378: 3671 /* note */ return new Property("note", "Annotation", "Comments about the observation or the results.", 0, 3672 java.lang.Integer.MAX_VALUE, note); 3673 case 1702620169: 3674 /* bodySite */ return new Property("bodySite", "CodeableConcept", 3675 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 3676 bodySite); 3677 case -1077554975: 3678 /* method */ return new Property("method", "CodeableConcept", 3679 "Indicates the mechanism used to perform the observation.", 0, 1, method); 3680 case -2132868344: 3681 /* specimen */ return new Property("specimen", "Reference(Specimen)", 3682 "The specimen that was used when this observation was made.", 0, 1, specimen); 3683 case -1335157162: 3684 /* device */ return new Property("device", "Reference(Device|DeviceMetric)", 3685 "The device used to generate the observation data.", 0, 1, device); 3686 case -1912545102: 3687 /* referenceRange */ return new Property("referenceRange", "", 3688 "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 3689 0, java.lang.Integer.MAX_VALUE, referenceRange); 3690 case -458019372: 3691 /* hasMember */ return new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", 3692 "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 3693 0, java.lang.Integer.MAX_VALUE, hasMember); 3694 case 1077922663: 3695 /* derivedFrom */ return new Property("derivedFrom", 3696 "Reference(DocumentReference|ImagingStudy|Media|QuestionnaireResponse|Observation|MolecularSequence)", 3697 "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 3698 0, java.lang.Integer.MAX_VALUE, derivedFrom); 3699 case -1399907075: 3700 /* component */ return new Property("component", "", 3701 "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 3702 0, java.lang.Integer.MAX_VALUE, component); 3703 default: 3704 return super.getNamedProperty(_hash, _name, _checkValid); 3705 } 3706 3707 } 3708 3709 @Override 3710 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3711 switch (hash) { 3712 case -1618432855: 3713 /* identifier */ return this.identifier == null ? new Base[0] 3714 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3715 case -332612366: 3716 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3717 case -995410646: 3718 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3719 case -892481550: 3720 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ObservationStatus> 3721 case 50511102: 3722 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3723 case 3059181: 3724 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3725 case -1867885268: 3726 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 3727 case 97604824: 3728 /* focus */ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 3729 case 1524132147: 3730 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3731 case -1468651097: 3732 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 3733 case -1179159893: 3734 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // InstantType 3735 case 481140686: 3736 /* performer */ return this.performer == null ? new Base[0] 3737 : this.performer.toArray(new Base[this.performer.size()]); // Reference 3738 case 111972721: 3739 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3740 case 1034315687: 3741 /* dataAbsentReason */ return this.dataAbsentReason == null ? new Base[0] : new Base[] { this.dataAbsentReason }; // CodeableConcept 3742 case -297950712: 3743 /* interpretation */ return this.interpretation == null ? new Base[0] 3744 : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 3745 case 3387378: 3746 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3747 case 1702620169: 3748 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 3749 case -1077554975: 3750 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 3751 case -2132868344: 3752 /* specimen */ return this.specimen == null ? new Base[0] : new Base[] { this.specimen }; // Reference 3753 case -1335157162: 3754 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 3755 case -1912545102: 3756 /* referenceRange */ return this.referenceRange == null ? new Base[0] 3757 : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 3758 case -458019372: 3759 /* hasMember */ return this.hasMember == null ? new Base[0] 3760 : this.hasMember.toArray(new Base[this.hasMember.size()]); // Reference 3761 case 1077922663: 3762 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] 3763 : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 3764 case -1399907075: 3765 /* component */ return this.component == null ? new Base[0] 3766 : this.component.toArray(new Base[this.component.size()]); // ObservationComponentComponent 3767 default: 3768 return super.getProperty(hash, name, checkValid); 3769 } 3770 3771 } 3772 3773 @Override 3774 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3775 switch (hash) { 3776 case -1618432855: // identifier 3777 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3778 return value; 3779 case -332612366: // basedOn 3780 this.getBasedOn().add(castToReference(value)); // Reference 3781 return value; 3782 case -995410646: // partOf 3783 this.getPartOf().add(castToReference(value)); // Reference 3784 return value; 3785 case -892481550: // status 3786 value = new ObservationStatusEnumFactory().fromType(castToCode(value)); 3787 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3788 return value; 3789 case 50511102: // category 3790 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3791 return value; 3792 case 3059181: // code 3793 this.code = castToCodeableConcept(value); // CodeableConcept 3794 return value; 3795 case -1867885268: // subject 3796 this.subject = castToReference(value); // Reference 3797 return value; 3798 case 97604824: // focus 3799 this.getFocus().add(castToReference(value)); // Reference 3800 return value; 3801 case 1524132147: // encounter 3802 this.encounter = castToReference(value); // Reference 3803 return value; 3804 case -1468651097: // effective 3805 this.effective = castToType(value); // Type 3806 return value; 3807 case -1179159893: // issued 3808 this.issued = castToInstant(value); // InstantType 3809 return value; 3810 case 481140686: // performer 3811 this.getPerformer().add(castToReference(value)); // Reference 3812 return value; 3813 case 111972721: // value 3814 this.value = castToType(value); // Type 3815 return value; 3816 case 1034315687: // dataAbsentReason 3817 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 3818 return value; 3819 case -297950712: // interpretation 3820 this.getInterpretation().add(castToCodeableConcept(value)); // CodeableConcept 3821 return value; 3822 case 3387378: // note 3823 this.getNote().add(castToAnnotation(value)); // Annotation 3824 return value; 3825 case 1702620169: // bodySite 3826 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3827 return value; 3828 case -1077554975: // method 3829 this.method = castToCodeableConcept(value); // CodeableConcept 3830 return value; 3831 case -2132868344: // specimen 3832 this.specimen = castToReference(value); // Reference 3833 return value; 3834 case -1335157162: // device 3835 this.device = castToReference(value); // Reference 3836 return value; 3837 case -1912545102: // referenceRange 3838 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 3839 return value; 3840 case -458019372: // hasMember 3841 this.getHasMember().add(castToReference(value)); // Reference 3842 return value; 3843 case 1077922663: // derivedFrom 3844 this.getDerivedFrom().add(castToReference(value)); // Reference 3845 return value; 3846 case -1399907075: // component 3847 this.getComponent().add((ObservationComponentComponent) value); // ObservationComponentComponent 3848 return value; 3849 default: 3850 return super.setProperty(hash, name, value); 3851 } 3852 3853 } 3854 3855 @Override 3856 public Base setProperty(String name, Base value) throws FHIRException { 3857 if (name.equals("identifier")) { 3858 this.getIdentifier().add(castToIdentifier(value)); 3859 } else if (name.equals("basedOn")) { 3860 this.getBasedOn().add(castToReference(value)); 3861 } else if (name.equals("partOf")) { 3862 this.getPartOf().add(castToReference(value)); 3863 } else if (name.equals("status")) { 3864 value = new ObservationStatusEnumFactory().fromType(castToCode(value)); 3865 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3866 } else if (name.equals("category")) { 3867 this.getCategory().add(castToCodeableConcept(value)); 3868 } else if (name.equals("code")) { 3869 this.code = castToCodeableConcept(value); // CodeableConcept 3870 } else if (name.equals("subject")) { 3871 this.subject = castToReference(value); // Reference 3872 } else if (name.equals("focus")) { 3873 this.getFocus().add(castToReference(value)); 3874 } else if (name.equals("encounter")) { 3875 this.encounter = castToReference(value); // Reference 3876 } else if (name.equals("effective[x]")) { 3877 this.effective = castToType(value); // Type 3878 } else if (name.equals("issued")) { 3879 this.issued = castToInstant(value); // InstantType 3880 } else if (name.equals("performer")) { 3881 this.getPerformer().add(castToReference(value)); 3882 } else if (name.equals("value[x]")) { 3883 this.value = castToType(value); // Type 3884 } else if (name.equals("dataAbsentReason")) { 3885 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 3886 } else if (name.equals("interpretation")) { 3887 this.getInterpretation().add(castToCodeableConcept(value)); 3888 } else if (name.equals("note")) { 3889 this.getNote().add(castToAnnotation(value)); 3890 } else if (name.equals("bodySite")) { 3891 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3892 } else if (name.equals("method")) { 3893 this.method = castToCodeableConcept(value); // CodeableConcept 3894 } else if (name.equals("specimen")) { 3895 this.specimen = castToReference(value); // Reference 3896 } else if (name.equals("device")) { 3897 this.device = castToReference(value); // Reference 3898 } else if (name.equals("referenceRange")) { 3899 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 3900 } else if (name.equals("hasMember")) { 3901 this.getHasMember().add(castToReference(value)); 3902 } else if (name.equals("derivedFrom")) { 3903 this.getDerivedFrom().add(castToReference(value)); 3904 } else if (name.equals("component")) { 3905 this.getComponent().add((ObservationComponentComponent) value); 3906 } else 3907 return super.setProperty(name, value); 3908 return value; 3909 } 3910 3911 @Override 3912 public Base makeProperty(int hash, String name) throws FHIRException { 3913 switch (hash) { 3914 case -1618432855: 3915 return addIdentifier(); 3916 case -332612366: 3917 return addBasedOn(); 3918 case -995410646: 3919 return addPartOf(); 3920 case -892481550: 3921 return getStatusElement(); 3922 case 50511102: 3923 return addCategory(); 3924 case 3059181: 3925 return getCode(); 3926 case -1867885268: 3927 return getSubject(); 3928 case 97604824: 3929 return addFocus(); 3930 case 1524132147: 3931 return getEncounter(); 3932 case 247104889: 3933 return getEffective(); 3934 case -1468651097: 3935 return getEffective(); 3936 case -1179159893: 3937 return getIssuedElement(); 3938 case 481140686: 3939 return addPerformer(); 3940 case -1410166417: 3941 return getValue(); 3942 case 111972721: 3943 return getValue(); 3944 case 1034315687: 3945 return getDataAbsentReason(); 3946 case -297950712: 3947 return addInterpretation(); 3948 case 3387378: 3949 return addNote(); 3950 case 1702620169: 3951 return getBodySite(); 3952 case -1077554975: 3953 return getMethod(); 3954 case -2132868344: 3955 return getSpecimen(); 3956 case -1335157162: 3957 return getDevice(); 3958 case -1912545102: 3959 return addReferenceRange(); 3960 case -458019372: 3961 return addHasMember(); 3962 case 1077922663: 3963 return addDerivedFrom(); 3964 case -1399907075: 3965 return addComponent(); 3966 default: 3967 return super.makeProperty(hash, name); 3968 } 3969 3970 } 3971 3972 @Override 3973 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3974 switch (hash) { 3975 case -1618432855: 3976 /* identifier */ return new String[] { "Identifier" }; 3977 case -332612366: 3978 /* basedOn */ return new String[] { "Reference" }; 3979 case -995410646: 3980 /* partOf */ return new String[] { "Reference" }; 3981 case -892481550: 3982 /* status */ return new String[] { "code" }; 3983 case 50511102: 3984 /* category */ return new String[] { "CodeableConcept" }; 3985 case 3059181: 3986 /* code */ return new String[] { "CodeableConcept" }; 3987 case -1867885268: 3988 /* subject */ return new String[] { "Reference" }; 3989 case 97604824: 3990 /* focus */ return new String[] { "Reference" }; 3991 case 1524132147: 3992 /* encounter */ return new String[] { "Reference" }; 3993 case -1468651097: 3994 /* effective */ return new String[] { "dateTime", "Period", "Timing", "instant" }; 3995 case -1179159893: 3996 /* issued */ return new String[] { "instant" }; 3997 case 481140686: 3998 /* performer */ return new String[] { "Reference" }; 3999 case 111972721: 4000 /* value */ return new String[] { "Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", 4001 "SampledData", "time", "dateTime", "Period" }; 4002 case 1034315687: 4003 /* dataAbsentReason */ return new String[] { "CodeableConcept" }; 4004 case -297950712: 4005 /* interpretation */ return new String[] { "CodeableConcept" }; 4006 case 3387378: 4007 /* note */ return new String[] { "Annotation" }; 4008 case 1702620169: 4009 /* bodySite */ return new String[] { "CodeableConcept" }; 4010 case -1077554975: 4011 /* method */ return new String[] { "CodeableConcept" }; 4012 case -2132868344: 4013 /* specimen */ return new String[] { "Reference" }; 4014 case -1335157162: 4015 /* device */ return new String[] { "Reference" }; 4016 case -1912545102: 4017 /* referenceRange */ return new String[] {}; 4018 case -458019372: 4019 /* hasMember */ return new String[] { "Reference" }; 4020 case 1077922663: 4021 /* derivedFrom */ return new String[] { "Reference" }; 4022 case -1399907075: 4023 /* component */ return new String[] {}; 4024 default: 4025 return super.getTypesForProperty(hash, name); 4026 } 4027 4028 } 4029 4030 @Override 4031 public Base addChild(String name) throws FHIRException { 4032 if (name.equals("identifier")) { 4033 return addIdentifier(); 4034 } else if (name.equals("basedOn")) { 4035 return addBasedOn(); 4036 } else if (name.equals("partOf")) { 4037 return addPartOf(); 4038 } else if (name.equals("status")) { 4039 throw new FHIRException("Cannot call addChild on a singleton property Observation.status"); 4040 } else if (name.equals("category")) { 4041 return addCategory(); 4042 } else if (name.equals("code")) { 4043 this.code = new CodeableConcept(); 4044 return this.code; 4045 } else if (name.equals("subject")) { 4046 this.subject = new Reference(); 4047 return this.subject; 4048 } else if (name.equals("focus")) { 4049 return addFocus(); 4050 } else if (name.equals("encounter")) { 4051 this.encounter = new Reference(); 4052 return this.encounter; 4053 } else if (name.equals("effectiveDateTime")) { 4054 this.effective = new DateTimeType(); 4055 return this.effective; 4056 } else if (name.equals("effectivePeriod")) { 4057 this.effective = new Period(); 4058 return this.effective; 4059 } else if (name.equals("effectiveTiming")) { 4060 this.effective = new Timing(); 4061 return this.effective; 4062 } else if (name.equals("effectiveInstant")) { 4063 this.effective = new InstantType(); 4064 return this.effective; 4065 } else if (name.equals("issued")) { 4066 throw new FHIRException("Cannot call addChild on a singleton property Observation.issued"); 4067 } else if (name.equals("performer")) { 4068 return addPerformer(); 4069 } else if (name.equals("valueQuantity")) { 4070 this.value = new Quantity(); 4071 return this.value; 4072 } else if (name.equals("valueCodeableConcept")) { 4073 this.value = new CodeableConcept(); 4074 return this.value; 4075 } else if (name.equals("valueString")) { 4076 this.value = new StringType(); 4077 return this.value; 4078 } else if (name.equals("valueBoolean")) { 4079 this.value = new BooleanType(); 4080 return this.value; 4081 } else if (name.equals("valueInteger")) { 4082 this.value = new IntegerType(); 4083 return this.value; 4084 } else if (name.equals("valueRange")) { 4085 this.value = new Range(); 4086 return this.value; 4087 } else if (name.equals("valueRatio")) { 4088 this.value = new Ratio(); 4089 return this.value; 4090 } else if (name.equals("valueSampledData")) { 4091 this.value = new SampledData(); 4092 return this.value; 4093 } else if (name.equals("valueTime")) { 4094 this.value = new TimeType(); 4095 return this.value; 4096 } else if (name.equals("valueDateTime")) { 4097 this.value = new DateTimeType(); 4098 return this.value; 4099 } else if (name.equals("valuePeriod")) { 4100 this.value = new Period(); 4101 return this.value; 4102 } else if (name.equals("dataAbsentReason")) { 4103 this.dataAbsentReason = new CodeableConcept(); 4104 return this.dataAbsentReason; 4105 } else if (name.equals("interpretation")) { 4106 return addInterpretation(); 4107 } else if (name.equals("note")) { 4108 return addNote(); 4109 } else if (name.equals("bodySite")) { 4110 this.bodySite = new CodeableConcept(); 4111 return this.bodySite; 4112 } else if (name.equals("method")) { 4113 this.method = new CodeableConcept(); 4114 return this.method; 4115 } else if (name.equals("specimen")) { 4116 this.specimen = new Reference(); 4117 return this.specimen; 4118 } else if (name.equals("device")) { 4119 this.device = new Reference(); 4120 return this.device; 4121 } else if (name.equals("referenceRange")) { 4122 return addReferenceRange(); 4123 } else if (name.equals("hasMember")) { 4124 return addHasMember(); 4125 } else if (name.equals("derivedFrom")) { 4126 return addDerivedFrom(); 4127 } else if (name.equals("component")) { 4128 return addComponent(); 4129 } else 4130 return super.addChild(name); 4131 } 4132 4133 public String fhirType() { 4134 return "Observation"; 4135 4136 } 4137 4138 public Observation copy() { 4139 Observation dst = new Observation(); 4140 copyValues(dst); 4141 return dst; 4142 } 4143 4144 public void copyValues(Observation dst) { 4145 super.copyValues(dst); 4146 if (identifier != null) { 4147 dst.identifier = new ArrayList<Identifier>(); 4148 for (Identifier i : identifier) 4149 dst.identifier.add(i.copy()); 4150 } 4151 ; 4152 if (basedOn != null) { 4153 dst.basedOn = new ArrayList<Reference>(); 4154 for (Reference i : basedOn) 4155 dst.basedOn.add(i.copy()); 4156 } 4157 ; 4158 if (partOf != null) { 4159 dst.partOf = new ArrayList<Reference>(); 4160 for (Reference i : partOf) 4161 dst.partOf.add(i.copy()); 4162 } 4163 ; 4164 dst.status = status == null ? null : status.copy(); 4165 if (category != null) { 4166 dst.category = new ArrayList<CodeableConcept>(); 4167 for (CodeableConcept i : category) 4168 dst.category.add(i.copy()); 4169 } 4170 ; 4171 dst.code = code == null ? null : code.copy(); 4172 dst.subject = subject == null ? null : subject.copy(); 4173 if (focus != null) { 4174 dst.focus = new ArrayList<Reference>(); 4175 for (Reference i : focus) 4176 dst.focus.add(i.copy()); 4177 } 4178 ; 4179 dst.encounter = encounter == null ? null : encounter.copy(); 4180 dst.effective = effective == null ? null : effective.copy(); 4181 dst.issued = issued == null ? null : issued.copy(); 4182 if (performer != null) { 4183 dst.performer = new ArrayList<Reference>(); 4184 for (Reference i : performer) 4185 dst.performer.add(i.copy()); 4186 } 4187 ; 4188 dst.value = value == null ? null : value.copy(); 4189 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 4190 if (interpretation != null) { 4191 dst.interpretation = new ArrayList<CodeableConcept>(); 4192 for (CodeableConcept i : interpretation) 4193 dst.interpretation.add(i.copy()); 4194 } 4195 ; 4196 if (note != null) { 4197 dst.note = new ArrayList<Annotation>(); 4198 for (Annotation i : note) 4199 dst.note.add(i.copy()); 4200 } 4201 ; 4202 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4203 dst.method = method == null ? null : method.copy(); 4204 dst.specimen = specimen == null ? null : specimen.copy(); 4205 dst.device = device == null ? null : device.copy(); 4206 if (referenceRange != null) { 4207 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 4208 for (ObservationReferenceRangeComponent i : referenceRange) 4209 dst.referenceRange.add(i.copy()); 4210 } 4211 ; 4212 if (hasMember != null) { 4213 dst.hasMember = new ArrayList<Reference>(); 4214 for (Reference i : hasMember) 4215 dst.hasMember.add(i.copy()); 4216 } 4217 ; 4218 if (derivedFrom != null) { 4219 dst.derivedFrom = new ArrayList<Reference>(); 4220 for (Reference i : derivedFrom) 4221 dst.derivedFrom.add(i.copy()); 4222 } 4223 ; 4224 if (component != null) { 4225 dst.component = new ArrayList<ObservationComponentComponent>(); 4226 for (ObservationComponentComponent i : component) 4227 dst.component.add(i.copy()); 4228 } 4229 ; 4230 } 4231 4232 protected Observation typedCopy() { 4233 return copy(); 4234 } 4235 4236 @Override 4237 public boolean equalsDeep(Base other_) { 4238 if (!super.equalsDeep(other_)) 4239 return false; 4240 if (!(other_ instanceof Observation)) 4241 return false; 4242 Observation o = (Observation) other_; 4243 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 4244 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 4245 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 4246 && compareDeep(subject, o.subject, true) && compareDeep(focus, o.focus, true) 4247 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) 4248 && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) 4249 && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 4250 && compareDeep(interpretation, o.interpretation, true) && compareDeep(note, o.note, true) 4251 && compareDeep(bodySite, o.bodySite, true) && compareDeep(method, o.method, true) 4252 && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 4253 && compareDeep(referenceRange, o.referenceRange, true) && compareDeep(hasMember, o.hasMember, true) 4254 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(component, o.component, true); 4255 } 4256 4257 @Override 4258 public boolean equalsShallow(Base other_) { 4259 if (!super.equalsShallow(other_)) 4260 return false; 4261 if (!(other_ instanceof Observation)) 4262 return false; 4263 Observation o = (Observation) other_; 4264 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true); 4265 } 4266 4267 public boolean isEmpty() { 4268 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, category, code, 4269 subject, focus, encounter, effective, issued, performer, value, dataAbsentReason, interpretation, note, 4270 bodySite, method, specimen, device, referenceRange, hasMember, derivedFrom, component); 4271 } 4272 4273 @Override 4274 public ResourceType getResourceType() { 4275 return ResourceType.Observation; 4276 } 4277 4278 /** 4279 * Search parameter: <b>date</b> 4280 * <p> 4281 * Description: <b>Obtained date/time. If the obtained element is a period, a 4282 * date that falls in the period</b><br> 4283 * Type: <b>date</b><br> 4284 * Path: <b>Observation.effective[x]</b><br> 4285 * </p> 4286 */ 4287 @SearchParamDefinition(name = "date", path = "Observation.effective", description = "Obtained date/time. If the obtained element is a period, a date that falls in the period", type = "date") 4288 public static final String SP_DATE = "date"; 4289 /** 4290 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4291 * <p> 4292 * Description: <b>Obtained date/time. If the obtained element is a period, a 4293 * date that falls in the period</b><br> 4294 * Type: <b>date</b><br> 4295 * Path: <b>Observation.effective[x]</b><br> 4296 * </p> 4297 */ 4298 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4299 SP_DATE); 4300 4301 /** 4302 * Search parameter: <b>combo-data-absent-reason</b> 4303 * <p> 4304 * Description: <b>The reason why the expected value in the element 4305 * Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4306 * Type: <b>token</b><br> 4307 * Path: <b>Observation.dataAbsentReason, 4308 * Observation.component.dataAbsentReason</b><br> 4309 * </p> 4310 */ 4311 @SearchParamDefinition(name = "combo-data-absent-reason", path = "Observation.dataAbsentReason | Observation.component.dataAbsentReason", description = "The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.", type = "token") 4312 public static final String SP_COMBO_DATA_ABSENT_REASON = "combo-data-absent-reason"; 4313 /** 4314 * <b>Fluent Client</b> search parameter constant for 4315 * <b>combo-data-absent-reason</b> 4316 * <p> 4317 * Description: <b>The reason why the expected value in the element 4318 * Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4319 * Type: <b>token</b><br> 4320 * Path: <b>Observation.dataAbsentReason, 4321 * Observation.component.dataAbsentReason</b><br> 4322 * </p> 4323 */ 4324 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4325 SP_COMBO_DATA_ABSENT_REASON); 4326 4327 /** 4328 * Search parameter: <b>code</b> 4329 * <p> 4330 * Description: <b>The code of the observation type</b><br> 4331 * Type: <b>token</b><br> 4332 * Path: <b>Observation.code</b><br> 4333 * </p> 4334 */ 4335 @SearchParamDefinition(name = "code", path = "Observation.code", description = "The code of the observation type", type = "token") 4336 public static final String SP_CODE = "code"; 4337 /** 4338 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4339 * <p> 4340 * Description: <b>The code of the observation type</b><br> 4341 * Type: <b>token</b><br> 4342 * Path: <b>Observation.code</b><br> 4343 * </p> 4344 */ 4345 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4346 SP_CODE); 4347 4348 /** 4349 * Search parameter: <b>combo-code-value-quantity</b> 4350 * <p> 4351 * Description: <b>Code and quantity value parameter pair, including in 4352 * components</b><br> 4353 * Type: <b>composite</b><br> 4354 * Path: <b></b><br> 4355 * </p> 4356 */ 4357 @SearchParamDefinition(name = "combo-code-value-quantity", path = "Observation | Observation.component", description = "Code and quantity value parameter pair, including in components", type = "composite", compositeOf = { 4358 "combo-code", "combo-value-quantity" }) 4359 public static final String SP_COMBO_CODE_VALUE_QUANTITY = "combo-code-value-quantity"; 4360 /** 4361 * <b>Fluent Client</b> search parameter constant for 4362 * <b>combo-code-value-quantity</b> 4363 * <p> 4364 * Description: <b>Code and quantity value parameter pair, including in 4365 * components</b><br> 4366 * Type: <b>composite</b><br> 4367 * Path: <b></b><br> 4368 * </p> 4369 */ 4370 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMBO_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4371 SP_COMBO_CODE_VALUE_QUANTITY); 4372 4373 /** 4374 * Search parameter: <b>subject</b> 4375 * <p> 4376 * Description: <b>The subject that the observation is about</b><br> 4377 * Type: <b>reference</b><br> 4378 * Path: <b>Observation.subject</b><br> 4379 * </p> 4380 */ 4381 @SearchParamDefinition(name = "subject", path = "Observation.subject", description = "The subject that the observation is about", type = "reference", providesMembershipIn = { 4382 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4383 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 4384 Location.class, Patient.class }) 4385 public static final String SP_SUBJECT = "subject"; 4386 /** 4387 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4388 * <p> 4389 * Description: <b>The subject that the observation is about</b><br> 4390 * Type: <b>reference</b><br> 4391 * Path: <b>Observation.subject</b><br> 4392 * </p> 4393 */ 4394 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4395 SP_SUBJECT); 4396 4397 /** 4398 * Constant for fluent queries to be used to add include statements. Specifies 4399 * the path value of "<b>Observation:subject</b>". 4400 */ 4401 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 4402 "Observation:subject").toLocked(); 4403 4404 /** 4405 * Search parameter: <b>component-data-absent-reason</b> 4406 * <p> 4407 * Description: <b>The reason why the expected value in the element 4408 * Observation.component.value[x] is missing.</b><br> 4409 * Type: <b>token</b><br> 4410 * Path: <b>Observation.component.dataAbsentReason</b><br> 4411 * </p> 4412 */ 4413 @SearchParamDefinition(name = "component-data-absent-reason", path = "Observation.component.dataAbsentReason", description = "The reason why the expected value in the element Observation.component.value[x] is missing.", type = "token") 4414 public static final String SP_COMPONENT_DATA_ABSENT_REASON = "component-data-absent-reason"; 4415 /** 4416 * <b>Fluent Client</b> search parameter constant for 4417 * <b>component-data-absent-reason</b> 4418 * <p> 4419 * Description: <b>The reason why the expected value in the element 4420 * Observation.component.value[x] is missing.</b><br> 4421 * Type: <b>token</b><br> 4422 * Path: <b>Observation.component.dataAbsentReason</b><br> 4423 * </p> 4424 */ 4425 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4426 SP_COMPONENT_DATA_ABSENT_REASON); 4427 4428 /** 4429 * Search parameter: <b>value-concept</b> 4430 * <p> 4431 * Description: <b>The value of the observation, if the value is a 4432 * CodeableConcept</b><br> 4433 * Type: <b>token</b><br> 4434 * Path: <b>Observation.valueCodeableConcept</b><br> 4435 * </p> 4436 */ 4437 @SearchParamDefinition(name = "value-concept", path = "(Observation.value as CodeableConcept)", description = "The value of the observation, if the value is a CodeableConcept", type = "token") 4438 public static final String SP_VALUE_CONCEPT = "value-concept"; 4439 /** 4440 * <b>Fluent Client</b> search parameter constant for <b>value-concept</b> 4441 * <p> 4442 * Description: <b>The value of the observation, if the value is a 4443 * CodeableConcept</b><br> 4444 * Type: <b>token</b><br> 4445 * Path: <b>Observation.valueCodeableConcept</b><br> 4446 * </p> 4447 */ 4448 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4449 SP_VALUE_CONCEPT); 4450 4451 /** 4452 * Search parameter: <b>value-date</b> 4453 * <p> 4454 * Description: <b>The value of the observation, if the value is a date or 4455 * period of time</b><br> 4456 * Type: <b>date</b><br> 4457 * Path: <b>Observation.valueDateTime, Observation.valuePeriod</b><br> 4458 * </p> 4459 */ 4460 @SearchParamDefinition(name = "value-date", path = "(Observation.value as dateTime) | (Observation.value as Period)", description = "The value of the observation, if the value is a date or period of time", type = "date") 4461 public static final String SP_VALUE_DATE = "value-date"; 4462 /** 4463 * <b>Fluent Client</b> search parameter constant for <b>value-date</b> 4464 * <p> 4465 * Description: <b>The value of the observation, if the value is a date or 4466 * period of time</b><br> 4467 * Type: <b>date</b><br> 4468 * Path: <b>Observation.valueDateTime, Observation.valuePeriod</b><br> 4469 * </p> 4470 */ 4471 public static final ca.uhn.fhir.rest.gclient.DateClientParam VALUE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4472 SP_VALUE_DATE); 4473 4474 /** 4475 * Search parameter: <b>focus</b> 4476 * <p> 4477 * Description: <b>The focus of an observation when the focus is not the patient 4478 * of record.</b><br> 4479 * Type: <b>reference</b><br> 4480 * Path: <b>Observation.focus</b><br> 4481 * </p> 4482 */ 4483 @SearchParamDefinition(name = "focus", path = "Observation.focus", description = "The focus of an observation when the focus is not the patient of record.", type = "reference") 4484 public static final String SP_FOCUS = "focus"; 4485 /** 4486 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4487 * <p> 4488 * Description: <b>The focus of an observation when the focus is not the patient 4489 * of record.</b><br> 4490 * Type: <b>reference</b><br> 4491 * Path: <b>Observation.focus</b><br> 4492 * </p> 4493 */ 4494 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4495 SP_FOCUS); 4496 4497 /** 4498 * Constant for fluent queries to be used to add include statements. Specifies 4499 * the path value of "<b>Observation:focus</b>". 4500 */ 4501 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include( 4502 "Observation:focus").toLocked(); 4503 4504 /** 4505 * Search parameter: <b>derived-from</b> 4506 * <p> 4507 * Description: <b>Related measurements the observation is made from</b><br> 4508 * Type: <b>reference</b><br> 4509 * Path: <b>Observation.derivedFrom</b><br> 4510 * </p> 4511 */ 4512 @SearchParamDefinition(name = "derived-from", path = "Observation.derivedFrom", description = "Related measurements the observation is made from", type = "reference", target = { 4513 DocumentReference.class, ImagingStudy.class, Media.class, MolecularSequence.class, Observation.class, 4514 QuestionnaireResponse.class }) 4515 public static final String SP_DERIVED_FROM = "derived-from"; 4516 /** 4517 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4518 * <p> 4519 * Description: <b>Related measurements the observation is made from</b><br> 4520 * Type: <b>reference</b><br> 4521 * Path: <b>Observation.derivedFrom</b><br> 4522 * </p> 4523 */ 4524 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4525 SP_DERIVED_FROM); 4526 4527 /** 4528 * Constant for fluent queries to be used to add include statements. Specifies 4529 * the path value of "<b>Observation:derived-from</b>". 4530 */ 4531 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 4532 "Observation:derived-from").toLocked(); 4533 4534 /** 4535 * Search parameter: <b>part-of</b> 4536 * <p> 4537 * Description: <b>Part of referenced event</b><br> 4538 * Type: <b>reference</b><br> 4539 * Path: <b>Observation.partOf</b><br> 4540 * </p> 4541 */ 4542 @SearchParamDefinition(name = "part-of", path = "Observation.partOf", description = "Part of referenced event", type = "reference", target = { 4543 ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, 4544 MedicationStatement.class, Procedure.class }) 4545 public static final String SP_PART_OF = "part-of"; 4546 /** 4547 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4548 * <p> 4549 * Description: <b>Part of referenced event</b><br> 4550 * Type: <b>reference</b><br> 4551 * Path: <b>Observation.partOf</b><br> 4552 * </p> 4553 */ 4554 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4555 SP_PART_OF); 4556 4557 /** 4558 * Constant for fluent queries to be used to add include statements. Specifies 4559 * the path value of "<b>Observation:part-of</b>". 4560 */ 4561 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 4562 "Observation:part-of").toLocked(); 4563 4564 /** 4565 * Search parameter: <b>has-member</b> 4566 * <p> 4567 * Description: <b>Related resource that belongs to the Observation 4568 * group</b><br> 4569 * Type: <b>reference</b><br> 4570 * Path: <b>Observation.hasMember</b><br> 4571 * </p> 4572 */ 4573 @SearchParamDefinition(name = "has-member", path = "Observation.hasMember", description = "Related resource that belongs to the Observation group", type = "reference", target = { 4574 MolecularSequence.class, Observation.class, QuestionnaireResponse.class }) 4575 public static final String SP_HAS_MEMBER = "has-member"; 4576 /** 4577 * <b>Fluent Client</b> search parameter constant for <b>has-member</b> 4578 * <p> 4579 * Description: <b>Related resource that belongs to the Observation 4580 * group</b><br> 4581 * Type: <b>reference</b><br> 4582 * Path: <b>Observation.hasMember</b><br> 4583 * </p> 4584 */ 4585 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HAS_MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4586 SP_HAS_MEMBER); 4587 4588 /** 4589 * Constant for fluent queries to be used to add include statements. Specifies 4590 * the path value of "<b>Observation:has-member</b>". 4591 */ 4592 public static final ca.uhn.fhir.model.api.Include INCLUDE_HAS_MEMBER = new ca.uhn.fhir.model.api.Include( 4593 "Observation:has-member").toLocked(); 4594 4595 /** 4596 * Search parameter: <b>code-value-string</b> 4597 * <p> 4598 * Description: <b>Code and string value parameter pair</b><br> 4599 * Type: <b>composite</b><br> 4600 * Path: <b></b><br> 4601 * </p> 4602 */ 4603 @SearchParamDefinition(name = "code-value-string", path = "Observation", description = "Code and string value parameter pair", type = "composite", compositeOf = { 4604 "code", "value-string" }) 4605 public static final String SP_CODE_VALUE_STRING = "code-value-string"; 4606 /** 4607 * <b>Fluent Client</b> search parameter constant for <b>code-value-string</b> 4608 * <p> 4609 * Description: <b>Code and string value parameter pair</b><br> 4610 * Type: <b>composite</b><br> 4611 * Path: <b></b><br> 4612 * </p> 4613 */ 4614 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> CODE_VALUE_STRING = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>( 4615 SP_CODE_VALUE_STRING); 4616 4617 /** 4618 * Search parameter: <b>component-code-value-quantity</b> 4619 * <p> 4620 * Description: <b>Component code and component quantity value parameter 4621 * pair</b><br> 4622 * Type: <b>composite</b><br> 4623 * Path: <b></b><br> 4624 * </p> 4625 */ 4626 @SearchParamDefinition(name = "component-code-value-quantity", path = "Observation.component", description = "Component code and component quantity value parameter pair", type = "composite", compositeOf = { 4627 "component-code", "component-value-quantity" }) 4628 public static final String SP_COMPONENT_CODE_VALUE_QUANTITY = "component-code-value-quantity"; 4629 /** 4630 * <b>Fluent Client</b> search parameter constant for 4631 * <b>component-code-value-quantity</b> 4632 * <p> 4633 * Description: <b>Component code and component quantity value parameter 4634 * pair</b><br> 4635 * Type: <b>composite</b><br> 4636 * Path: <b></b><br> 4637 * </p> 4638 */ 4639 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMPONENT_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4640 SP_COMPONENT_CODE_VALUE_QUANTITY); 4641 4642 /** 4643 * Search parameter: <b>based-on</b> 4644 * <p> 4645 * Description: <b>Reference to the service request.</b><br> 4646 * Type: <b>reference</b><br> 4647 * Path: <b>Observation.basedOn</b><br> 4648 * </p> 4649 */ 4650 @SearchParamDefinition(name = "based-on", path = "Observation.basedOn", description = "Reference to the service request.", type = "reference", target = { 4651 CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, 4652 NutritionOrder.class, ServiceRequest.class }) 4653 public static final String SP_BASED_ON = "based-on"; 4654 /** 4655 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 4656 * <p> 4657 * Description: <b>Reference to the service request.</b><br> 4658 * Type: <b>reference</b><br> 4659 * Path: <b>Observation.basedOn</b><br> 4660 * </p> 4661 */ 4662 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4663 SP_BASED_ON); 4664 4665 /** 4666 * Constant for fluent queries to be used to add include statements. Specifies 4667 * the path value of "<b>Observation:based-on</b>". 4668 */ 4669 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 4670 "Observation:based-on").toLocked(); 4671 4672 /** 4673 * Search parameter: <b>code-value-date</b> 4674 * <p> 4675 * Description: <b>Code and date/time value parameter pair</b><br> 4676 * Type: <b>composite</b><br> 4677 * Path: <b></b><br> 4678 * </p> 4679 */ 4680 @SearchParamDefinition(name = "code-value-date", path = "Observation", description = "Code and date/time value parameter pair", type = "composite", compositeOf = { 4681 "code", "value-date" }) 4682 public static final String SP_CODE_VALUE_DATE = "code-value-date"; 4683 /** 4684 * <b>Fluent Client</b> search parameter constant for <b>code-value-date</b> 4685 * <p> 4686 * Description: <b>Code and date/time value parameter pair</b><br> 4687 * Type: <b>composite</b><br> 4688 * Path: <b></b><br> 4689 * </p> 4690 */ 4691 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam> CODE_VALUE_DATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam>( 4692 SP_CODE_VALUE_DATE); 4693 4694 /** 4695 * Search parameter: <b>patient</b> 4696 * <p> 4697 * Description: <b>The subject that the observation is about (if 4698 * patient)</b><br> 4699 * Type: <b>reference</b><br> 4700 * Path: <b>Observation.subject</b><br> 4701 * </p> 4702 */ 4703 @SearchParamDefinition(name = "patient", path = "Observation.subject.where(resolve() is Patient)", description = "The subject that the observation is about (if patient)", type = "reference", target = { 4704 Patient.class }) 4705 public static final String SP_PATIENT = "patient"; 4706 /** 4707 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4708 * <p> 4709 * Description: <b>The subject that the observation is about (if 4710 * patient)</b><br> 4711 * Type: <b>reference</b><br> 4712 * Path: <b>Observation.subject</b><br> 4713 * </p> 4714 */ 4715 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4716 SP_PATIENT); 4717 4718 /** 4719 * Constant for fluent queries to be used to add include statements. Specifies 4720 * the path value of "<b>Observation:patient</b>". 4721 */ 4722 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4723 "Observation:patient").toLocked(); 4724 4725 /** 4726 * Search parameter: <b>specimen</b> 4727 * <p> 4728 * Description: <b>Specimen used for this observation</b><br> 4729 * Type: <b>reference</b><br> 4730 * Path: <b>Observation.specimen</b><br> 4731 * </p> 4732 */ 4733 @SearchParamDefinition(name = "specimen", path = "Observation.specimen", description = "Specimen used for this observation", type = "reference", target = { 4734 Specimen.class }) 4735 public static final String SP_SPECIMEN = "specimen"; 4736 /** 4737 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 4738 * <p> 4739 * Description: <b>Specimen used for this observation</b><br> 4740 * Type: <b>reference</b><br> 4741 * Path: <b>Observation.specimen</b><br> 4742 * </p> 4743 */ 4744 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4745 SP_SPECIMEN); 4746 4747 /** 4748 * Constant for fluent queries to be used to add include statements. Specifies 4749 * the path value of "<b>Observation:specimen</b>". 4750 */ 4751 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include( 4752 "Observation:specimen").toLocked(); 4753 4754 /** 4755 * Search parameter: <b>component-code</b> 4756 * <p> 4757 * Description: <b>The component code of the observation type</b><br> 4758 * Type: <b>token</b><br> 4759 * Path: <b>Observation.component.code</b><br> 4760 * </p> 4761 */ 4762 @SearchParamDefinition(name = "component-code", path = "Observation.component.code", description = "The component code of the observation type", type = "token") 4763 public static final String SP_COMPONENT_CODE = "component-code"; 4764 /** 4765 * <b>Fluent Client</b> search parameter constant for <b>component-code</b> 4766 * <p> 4767 * Description: <b>The component code of the observation type</b><br> 4768 * Type: <b>token</b><br> 4769 * Path: <b>Observation.component.code</b><br> 4770 * </p> 4771 */ 4772 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4773 SP_COMPONENT_CODE); 4774 4775 /** 4776 * Search parameter: <b>code-value-quantity</b> 4777 * <p> 4778 * Description: <b>Code and quantity value parameter pair</b><br> 4779 * Type: <b>composite</b><br> 4780 * Path: <b></b><br> 4781 * </p> 4782 */ 4783 @SearchParamDefinition(name = "code-value-quantity", path = "Observation", description = "Code and quantity value parameter pair", type = "composite", compositeOf = { 4784 "code", "value-quantity" }) 4785 public static final String SP_CODE_VALUE_QUANTITY = "code-value-quantity"; 4786 /** 4787 * <b>Fluent Client</b> search parameter constant for <b>code-value-quantity</b> 4788 * <p> 4789 * Description: <b>Code and quantity value parameter pair</b><br> 4790 * Type: <b>composite</b><br> 4791 * Path: <b></b><br> 4792 * </p> 4793 */ 4794 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4795 SP_CODE_VALUE_QUANTITY); 4796 4797 /** 4798 * Search parameter: <b>combo-code-value-concept</b> 4799 * <p> 4800 * Description: <b>Code and coded value parameter pair, including in 4801 * components</b><br> 4802 * Type: <b>composite</b><br> 4803 * Path: <b></b><br> 4804 * </p> 4805 */ 4806 @SearchParamDefinition(name = "combo-code-value-concept", path = "Observation | Observation.component", description = "Code and coded value parameter pair, including in components", type = "composite", compositeOf = { 4807 "combo-code", "combo-value-concept" }) 4808 public static final String SP_COMBO_CODE_VALUE_CONCEPT = "combo-code-value-concept"; 4809 /** 4810 * <b>Fluent Client</b> search parameter constant for 4811 * <b>combo-code-value-concept</b> 4812 * <p> 4813 * Description: <b>Code and coded value parameter pair, including in 4814 * components</b><br> 4815 * Type: <b>composite</b><br> 4816 * Path: <b></b><br> 4817 * </p> 4818 */ 4819 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMBO_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4820 SP_COMBO_CODE_VALUE_CONCEPT); 4821 4822 /** 4823 * Search parameter: <b>value-string</b> 4824 * <p> 4825 * Description: <b>The value of the observation, if the value is a string, and 4826 * also searches in CodeableConcept.text</b><br> 4827 * Type: <b>string</b><br> 4828 * Path: <b>Observation.value[x]</b><br> 4829 * </p> 4830 */ 4831 @SearchParamDefinition(name = "value-string", path = "(Observation.value as string) | (Observation.value as CodeableConcept).text", description = "The value of the observation, if the value is a string, and also searches in CodeableConcept.text", type = "string") 4832 public static final String SP_VALUE_STRING = "value-string"; 4833 /** 4834 * <b>Fluent Client</b> search parameter constant for <b>value-string</b> 4835 * <p> 4836 * Description: <b>The value of the observation, if the value is a string, and 4837 * also searches in CodeableConcept.text</b><br> 4838 * Type: <b>string</b><br> 4839 * Path: <b>Observation.value[x]</b><br> 4840 * </p> 4841 */ 4842 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE_STRING = new ca.uhn.fhir.rest.gclient.StringClientParam( 4843 SP_VALUE_STRING); 4844 4845 /** 4846 * Search parameter: <b>identifier</b> 4847 * <p> 4848 * Description: <b>The unique id for a particular observation</b><br> 4849 * Type: <b>token</b><br> 4850 * Path: <b>Observation.identifier</b><br> 4851 * </p> 4852 */ 4853 @SearchParamDefinition(name = "identifier", path = "Observation.identifier", description = "The unique id for a particular observation", type = "token") 4854 public static final String SP_IDENTIFIER = "identifier"; 4855 /** 4856 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4857 * <p> 4858 * Description: <b>The unique id for a particular observation</b><br> 4859 * Type: <b>token</b><br> 4860 * Path: <b>Observation.identifier</b><br> 4861 * </p> 4862 */ 4863 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4864 SP_IDENTIFIER); 4865 4866 /** 4867 * Search parameter: <b>performer</b> 4868 * <p> 4869 * Description: <b>Who performed the observation</b><br> 4870 * Type: <b>reference</b><br> 4871 * Path: <b>Observation.performer</b><br> 4872 * </p> 4873 */ 4874 @SearchParamDefinition(name = "performer", path = "Observation.performer", description = "Who performed the observation", type = "reference", providesMembershipIn = { 4875 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4876 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 4877 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, 4878 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4879 public static final String SP_PERFORMER = "performer"; 4880 /** 4881 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4882 * <p> 4883 * Description: <b>Who performed the observation</b><br> 4884 * Type: <b>reference</b><br> 4885 * Path: <b>Observation.performer</b><br> 4886 * </p> 4887 */ 4888 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4889 SP_PERFORMER); 4890 4891 /** 4892 * Constant for fluent queries to be used to add include statements. Specifies 4893 * the path value of "<b>Observation:performer</b>". 4894 */ 4895 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 4896 "Observation:performer").toLocked(); 4897 4898 /** 4899 * Search parameter: <b>combo-code</b> 4900 * <p> 4901 * Description: <b>The code of the observation type or component type</b><br> 4902 * Type: <b>token</b><br> 4903 * Path: <b>Observation.code, Observation.component.code</b><br> 4904 * </p> 4905 */ 4906 @SearchParamDefinition(name = "combo-code", path = "Observation.code | Observation.component.code", description = "The code of the observation type or component type", type = "token") 4907 public static final String SP_COMBO_CODE = "combo-code"; 4908 /** 4909 * <b>Fluent Client</b> search parameter constant for <b>combo-code</b> 4910 * <p> 4911 * Description: <b>The code of the observation type or component type</b><br> 4912 * Type: <b>token</b><br> 4913 * Path: <b>Observation.code, Observation.component.code</b><br> 4914 * </p> 4915 */ 4916 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4917 SP_COMBO_CODE); 4918 4919 /** 4920 * Search parameter: <b>method</b> 4921 * <p> 4922 * Description: <b>The method used for the observation</b><br> 4923 * Type: <b>token</b><br> 4924 * Path: <b>Observation.method</b><br> 4925 * </p> 4926 */ 4927 @SearchParamDefinition(name = "method", path = "Observation.method", description = "The method used for the observation", type = "token") 4928 public static final String SP_METHOD = "method"; 4929 /** 4930 * <b>Fluent Client</b> search parameter constant for <b>method</b> 4931 * <p> 4932 * Description: <b>The method used for the observation</b><br> 4933 * Type: <b>token</b><br> 4934 * Path: <b>Observation.method</b><br> 4935 * </p> 4936 */ 4937 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4938 SP_METHOD); 4939 4940 /** 4941 * Search parameter: <b>value-quantity</b> 4942 * <p> 4943 * Description: <b>The value of the observation, if the value is a Quantity, or 4944 * a SampledData (just search on the bounds of the values in sampled 4945 * data)</b><br> 4946 * Type: <b>quantity</b><br> 4947 * Path: <b>Observation.value[x]</b><br> 4948 * </p> 4949 */ 4950 @SearchParamDefinition(name = "value-quantity", path = "(Observation.value as Quantity) | (Observation.value as SampledData)", description = "The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 4951 public static final String SP_VALUE_QUANTITY = "value-quantity"; 4952 /** 4953 * <b>Fluent Client</b> search parameter constant for <b>value-quantity</b> 4954 * <p> 4955 * Description: <b>The value of the observation, if the value is a Quantity, or 4956 * a SampledData (just search on the bounds of the values in sampled 4957 * data)</b><br> 4958 * Type: <b>quantity</b><br> 4959 * Path: <b>Observation.value[x]</b><br> 4960 * </p> 4961 */ 4962 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4963 SP_VALUE_QUANTITY); 4964 4965 /** 4966 * Search parameter: <b>component-value-quantity</b> 4967 * <p> 4968 * Description: <b>The value of the component observation, if the value is a 4969 * Quantity, or a SampledData (just search on the bounds of the values in 4970 * sampled data)</b><br> 4971 * Type: <b>quantity</b><br> 4972 * Path: <b>Observation.component.value[x]</b><br> 4973 * </p> 4974 */ 4975 @SearchParamDefinition(name = "component-value-quantity", path = "(Observation.component.value as Quantity) | (Observation.component.value as SampledData)", description = "The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 4976 public static final String SP_COMPONENT_VALUE_QUANTITY = "component-value-quantity"; 4977 /** 4978 * <b>Fluent Client</b> search parameter constant for 4979 * <b>component-value-quantity</b> 4980 * <p> 4981 * Description: <b>The value of the component observation, if the value is a 4982 * Quantity, or a SampledData (just search on the bounds of the values in 4983 * sampled data)</b><br> 4984 * Type: <b>quantity</b><br> 4985 * Path: <b>Observation.component.value[x]</b><br> 4986 * </p> 4987 */ 4988 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMPONENT_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4989 SP_COMPONENT_VALUE_QUANTITY); 4990 4991 /** 4992 * Search parameter: <b>data-absent-reason</b> 4993 * <p> 4994 * Description: <b>The reason why the expected value in the element 4995 * Observation.value[x] is missing.</b><br> 4996 * Type: <b>token</b><br> 4997 * Path: <b>Observation.dataAbsentReason</b><br> 4998 * </p> 4999 */ 5000 @SearchParamDefinition(name = "data-absent-reason", path = "Observation.dataAbsentReason", description = "The reason why the expected value in the element Observation.value[x] is missing.", type = "token") 5001 public static final String SP_DATA_ABSENT_REASON = "data-absent-reason"; 5002 /** 5003 * <b>Fluent Client</b> search parameter constant for <b>data-absent-reason</b> 5004 * <p> 5005 * Description: <b>The reason why the expected value in the element 5006 * Observation.value[x] is missing.</b><br> 5007 * Type: <b>token</b><br> 5008 * Path: <b>Observation.dataAbsentReason</b><br> 5009 * </p> 5010 */ 5011 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5012 SP_DATA_ABSENT_REASON); 5013 5014 /** 5015 * Search parameter: <b>combo-value-quantity</b> 5016 * <p> 5017 * Description: <b>The value or component value of the observation, if the value 5018 * is a Quantity, or a SampledData (just search on the bounds of the values in 5019 * sampled data)</b><br> 5020 * Type: <b>quantity</b><br> 5021 * Path: <b>Observation.value[x]</b><br> 5022 * </p> 5023 */ 5024 @SearchParamDefinition(name = "combo-value-quantity", path = "(Observation.value as Quantity) | (Observation.value as SampledData) | (Observation.component.value as Quantity) | (Observation.component.value as SampledData)", description = "The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 5025 public static final String SP_COMBO_VALUE_QUANTITY = "combo-value-quantity"; 5026 /** 5027 * <b>Fluent Client</b> search parameter constant for 5028 * <b>combo-value-quantity</b> 5029 * <p> 5030 * Description: <b>The value or component value of the observation, if the value 5031 * is a Quantity, or a SampledData (just search on the bounds of the values in 5032 * sampled data)</b><br> 5033 * Type: <b>quantity</b><br> 5034 * Path: <b>Observation.value[x]</b><br> 5035 * </p> 5036 */ 5037 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMBO_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5038 SP_COMBO_VALUE_QUANTITY); 5039 5040 /** 5041 * Search parameter: <b>encounter</b> 5042 * <p> 5043 * Description: <b>Encounter related to the observation</b><br> 5044 * Type: <b>reference</b><br> 5045 * Path: <b>Observation.encounter</b><br> 5046 * </p> 5047 */ 5048 @SearchParamDefinition(name = "encounter", path = "Observation.encounter", description = "Encounter related to the observation", type = "reference", providesMembershipIn = { 5049 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 5050 public static final String SP_ENCOUNTER = "encounter"; 5051 /** 5052 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5053 * <p> 5054 * Description: <b>Encounter related to the observation</b><br> 5055 * Type: <b>reference</b><br> 5056 * Path: <b>Observation.encounter</b><br> 5057 * </p> 5058 */ 5059 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5060 SP_ENCOUNTER); 5061 5062 /** 5063 * Constant for fluent queries to be used to add include statements. Specifies 5064 * the path value of "<b>Observation:encounter</b>". 5065 */ 5066 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5067 "Observation:encounter").toLocked(); 5068 5069 /** 5070 * Search parameter: <b>code-value-concept</b> 5071 * <p> 5072 * Description: <b>Code and coded value parameter pair</b><br> 5073 * Type: <b>composite</b><br> 5074 * Path: <b></b><br> 5075 * </p> 5076 */ 5077 @SearchParamDefinition(name = "code-value-concept", path = "Observation", description = "Code and coded value parameter pair", type = "composite", compositeOf = { 5078 "code", "value-concept" }) 5079 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 5080 /** 5081 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 5082 * <p> 5083 * Description: <b>Code and coded value parameter pair</b><br> 5084 * Type: <b>composite</b><br> 5085 * Path: <b></b><br> 5086 * </p> 5087 */ 5088 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5089 SP_CODE_VALUE_CONCEPT); 5090 5091 /** 5092 * Search parameter: <b>component-code-value-concept</b> 5093 * <p> 5094 * Description: <b>Component code and component coded value parameter 5095 * pair</b><br> 5096 * Type: <b>composite</b><br> 5097 * Path: <b></b><br> 5098 * </p> 5099 */ 5100 @SearchParamDefinition(name = "component-code-value-concept", path = "Observation.component", description = "Component code and component coded value parameter pair", type = "composite", compositeOf = { 5101 "component-code", "component-value-concept" }) 5102 public static final String SP_COMPONENT_CODE_VALUE_CONCEPT = "component-code-value-concept"; 5103 /** 5104 * <b>Fluent Client</b> search parameter constant for 5105 * <b>component-code-value-concept</b> 5106 * <p> 5107 * Description: <b>Component code and component coded value parameter 5108 * pair</b><br> 5109 * Type: <b>composite</b><br> 5110 * Path: <b></b><br> 5111 * </p> 5112 */ 5113 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMPONENT_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5114 SP_COMPONENT_CODE_VALUE_CONCEPT); 5115 5116 /** 5117 * Search parameter: <b>component-value-concept</b> 5118 * <p> 5119 * Description: <b>The value of the component observation, if the value is a 5120 * CodeableConcept</b><br> 5121 * Type: <b>token</b><br> 5122 * Path: <b>Observation.component.valueCodeableConcept</b><br> 5123 * </p> 5124 */ 5125 @SearchParamDefinition(name = "component-value-concept", path = "(Observation.component.value as CodeableConcept)", description = "The value of the component observation, if the value is a CodeableConcept", type = "token") 5126 public static final String SP_COMPONENT_VALUE_CONCEPT = "component-value-concept"; 5127 /** 5128 * <b>Fluent Client</b> search parameter constant for 5129 * <b>component-value-concept</b> 5130 * <p> 5131 * Description: <b>The value of the component observation, if the value is a 5132 * CodeableConcept</b><br> 5133 * Type: <b>token</b><br> 5134 * Path: <b>Observation.component.valueCodeableConcept</b><br> 5135 * </p> 5136 */ 5137 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5138 SP_COMPONENT_VALUE_CONCEPT); 5139 5140 /** 5141 * Search parameter: <b>category</b> 5142 * <p> 5143 * Description: <b>The classification of the type of observation</b><br> 5144 * Type: <b>token</b><br> 5145 * Path: <b>Observation.category</b><br> 5146 * </p> 5147 */ 5148 @SearchParamDefinition(name = "category", path = "Observation.category", description = "The classification of the type of observation", type = "token") 5149 public static final String SP_CATEGORY = "category"; 5150 /** 5151 * <b>Fluent Client</b> search parameter constant for <b>category</b> 5152 * <p> 5153 * Description: <b>The classification of the type of observation</b><br> 5154 * Type: <b>token</b><br> 5155 * Path: <b>Observation.category</b><br> 5156 * </p> 5157 */ 5158 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5159 SP_CATEGORY); 5160 5161 /** 5162 * Search parameter: <b>device</b> 5163 * <p> 5164 * Description: <b>The Device that generated the observation data.</b><br> 5165 * Type: <b>reference</b><br> 5166 * Path: <b>Observation.device</b><br> 5167 * </p> 5168 */ 5169 @SearchParamDefinition(name = "device", path = "Observation.device", description = "The Device that generated the observation data.", type = "reference", providesMembershipIn = { 5170 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class, DeviceMetric.class }) 5171 public static final String SP_DEVICE = "device"; 5172 /** 5173 * <b>Fluent Client</b> search parameter constant for <b>device</b> 5174 * <p> 5175 * Description: <b>The Device that generated the observation data.</b><br> 5176 * Type: <b>reference</b><br> 5177 * Path: <b>Observation.device</b><br> 5178 * </p> 5179 */ 5180 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5181 SP_DEVICE); 5182 5183 /** 5184 * Constant for fluent queries to be used to add include statements. Specifies 5185 * the path value of "<b>Observation:device</b>". 5186 */ 5187 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 5188 "Observation:device").toLocked(); 5189 5190 /** 5191 * Search parameter: <b>combo-value-concept</b> 5192 * <p> 5193 * Description: <b>The value or component value of the observation, if the value 5194 * is a CodeableConcept</b><br> 5195 * Type: <b>token</b><br> 5196 * Path: <b>Observation.valueCodeableConcept, 5197 * Observation.component.valueCodeableConcept</b><br> 5198 * </p> 5199 */ 5200 @SearchParamDefinition(name = "combo-value-concept", path = "(Observation.value as CodeableConcept) | (Observation.component.value as CodeableConcept)", description = "The value or component value of the observation, if the value is a CodeableConcept", type = "token") 5201 public static final String SP_COMBO_VALUE_CONCEPT = "combo-value-concept"; 5202 /** 5203 * <b>Fluent Client</b> search parameter constant for <b>combo-value-concept</b> 5204 * <p> 5205 * Description: <b>The value or component value of the observation, if the value 5206 * is a CodeableConcept</b><br> 5207 * Type: <b>token</b><br> 5208 * Path: <b>Observation.valueCodeableConcept, 5209 * Observation.component.valueCodeableConcept</b><br> 5210 * </p> 5211 */ 5212 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5213 SP_COMBO_VALUE_CONCEPT); 5214 5215 /** 5216 * Search parameter: <b>status</b> 5217 * <p> 5218 * Description: <b>The status of the observation</b><br> 5219 * Type: <b>token</b><br> 5220 * Path: <b>Observation.status</b><br> 5221 * </p> 5222 */ 5223 @SearchParamDefinition(name = "status", path = "Observation.status", description = "The status of the observation", type = "token") 5224 public static final String SP_STATUS = "status"; 5225 /** 5226 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5227 * <p> 5228 * Description: <b>The status of the observation</b><br> 5229 * Type: <b>token</b><br> 5230 * Path: <b>Observation.status</b><br> 5231 * </p> 5232 */ 5233 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5234 SP_STATUS); 5235 5236}