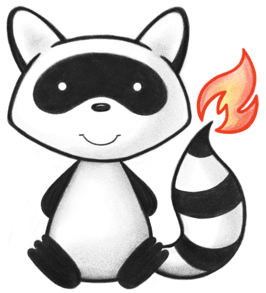
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 041import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048 049/** 050 * Set of definitional characteristics for a kind of observation or measurement 051 * produced or consumed by an orderable health care service. 052 */ 053@ResourceDef(name = "ObservationDefinition", profile = "http://hl7.org/fhir/StructureDefinition/ObservationDefinition") 054public class ObservationDefinition extends DomainResource { 055 056 public enum ObservationDataType { 057 /** 058 * A measured amount. 059 */ 060 QUANTITY, 061 /** 062 * A coded concept from a reference terminology and/or text. 063 */ 064 CODEABLECONCEPT, 065 /** 066 * A sequence of Unicode characters. 067 */ 068 STRING, 069 /** 070 * true or false. 071 */ 072 BOOLEAN, 073 /** 074 * A signed integer. 075 */ 076 INTEGER, 077 /** 078 * A set of values bounded by low and high. 079 */ 080 RANGE, 081 /** 082 * A ratio of two Quantity values - a numerator and a denominator. 083 */ 084 RATIO, 085 /** 086 * A series of measurements taken by a device. 087 */ 088 SAMPLEDDATA, 089 /** 090 * A time during the day, in the format hh:mm:ss. 091 */ 092 TIME, 093 /** 094 * A date, date-time or partial date (e.g. just year or year + month) as used in 095 * human communication. 096 */ 097 DATETIME, 098 /** 099 * A time range defined by start and end date/time. 100 */ 101 PERIOD, 102 /** 103 * added to help the parsers with the generic types 104 */ 105 NULL; 106 107 public static ObservationDataType fromCode(String codeString) throws FHIRException { 108 if (codeString == null || "".equals(codeString)) 109 return null; 110 if ("Quantity".equals(codeString)) 111 return QUANTITY; 112 if ("CodeableConcept".equals(codeString)) 113 return CODEABLECONCEPT; 114 if ("string".equals(codeString)) 115 return STRING; 116 if ("boolean".equals(codeString)) 117 return BOOLEAN; 118 if ("integer".equals(codeString)) 119 return INTEGER; 120 if ("Range".equals(codeString)) 121 return RANGE; 122 if ("Ratio".equals(codeString)) 123 return RATIO; 124 if ("SampledData".equals(codeString)) 125 return SAMPLEDDATA; 126 if ("time".equals(codeString)) 127 return TIME; 128 if ("dateTime".equals(codeString)) 129 return DATETIME; 130 if ("Period".equals(codeString)) 131 return PERIOD; 132 if (Configuration.isAcceptInvalidEnums()) 133 return null; 134 else 135 throw new FHIRException("Unknown ObservationDataType code '" + codeString + "'"); 136 } 137 138 public String toCode() { 139 switch (this) { 140 case QUANTITY: 141 return "Quantity"; 142 case CODEABLECONCEPT: 143 return "CodeableConcept"; 144 case STRING: 145 return "string"; 146 case BOOLEAN: 147 return "boolean"; 148 case INTEGER: 149 return "integer"; 150 case RANGE: 151 return "Range"; 152 case RATIO: 153 return "Ratio"; 154 case SAMPLEDDATA: 155 return "SampledData"; 156 case TIME: 157 return "time"; 158 case DATETIME: 159 return "dateTime"; 160 case PERIOD: 161 return "Period"; 162 case NULL: 163 return null; 164 default: 165 return "?"; 166 } 167 } 168 169 public String getSystem() { 170 switch (this) { 171 case QUANTITY: 172 return "http://hl7.org/fhir/permitted-data-type"; 173 case CODEABLECONCEPT: 174 return "http://hl7.org/fhir/permitted-data-type"; 175 case STRING: 176 return "http://hl7.org/fhir/permitted-data-type"; 177 case BOOLEAN: 178 return "http://hl7.org/fhir/permitted-data-type"; 179 case INTEGER: 180 return "http://hl7.org/fhir/permitted-data-type"; 181 case RANGE: 182 return "http://hl7.org/fhir/permitted-data-type"; 183 case RATIO: 184 return "http://hl7.org/fhir/permitted-data-type"; 185 case SAMPLEDDATA: 186 return "http://hl7.org/fhir/permitted-data-type"; 187 case TIME: 188 return "http://hl7.org/fhir/permitted-data-type"; 189 case DATETIME: 190 return "http://hl7.org/fhir/permitted-data-type"; 191 case PERIOD: 192 return "http://hl7.org/fhir/permitted-data-type"; 193 case NULL: 194 return null; 195 default: 196 return "?"; 197 } 198 } 199 200 public String getDefinition() { 201 switch (this) { 202 case QUANTITY: 203 return "A measured amount."; 204 case CODEABLECONCEPT: 205 return "A coded concept from a reference terminology and/or text."; 206 case STRING: 207 return "A sequence of Unicode characters."; 208 case BOOLEAN: 209 return "true or false."; 210 case INTEGER: 211 return "A signed integer."; 212 case RANGE: 213 return "A set of values bounded by low and high."; 214 case RATIO: 215 return "A ratio of two Quantity values - a numerator and a denominator."; 216 case SAMPLEDDATA: 217 return "A series of measurements taken by a device."; 218 case TIME: 219 return "A time during the day, in the format hh:mm:ss."; 220 case DATETIME: 221 return "A date, date-time or partial date (e.g. just year or year + month) as used in human communication."; 222 case PERIOD: 223 return "A time range defined by start and end date/time."; 224 case NULL: 225 return null; 226 default: 227 return "?"; 228 } 229 } 230 231 public String getDisplay() { 232 switch (this) { 233 case QUANTITY: 234 return "Quantity"; 235 case CODEABLECONCEPT: 236 return "CodeableConcept"; 237 case STRING: 238 return "string"; 239 case BOOLEAN: 240 return "boolean"; 241 case INTEGER: 242 return "integer"; 243 case RANGE: 244 return "Range"; 245 case RATIO: 246 return "Ratio"; 247 case SAMPLEDDATA: 248 return "SampledData"; 249 case TIME: 250 return "time"; 251 case DATETIME: 252 return "dateTime"; 253 case PERIOD: 254 return "Period"; 255 case NULL: 256 return null; 257 default: 258 return "?"; 259 } 260 } 261 } 262 263 public static class ObservationDataTypeEnumFactory implements EnumFactory<ObservationDataType> { 264 public ObservationDataType fromCode(String codeString) throws IllegalArgumentException { 265 if (codeString == null || "".equals(codeString)) 266 if (codeString == null || "".equals(codeString)) 267 return null; 268 if ("Quantity".equals(codeString)) 269 return ObservationDataType.QUANTITY; 270 if ("CodeableConcept".equals(codeString)) 271 return ObservationDataType.CODEABLECONCEPT; 272 if ("string".equals(codeString)) 273 return ObservationDataType.STRING; 274 if ("boolean".equals(codeString)) 275 return ObservationDataType.BOOLEAN; 276 if ("integer".equals(codeString)) 277 return ObservationDataType.INTEGER; 278 if ("Range".equals(codeString)) 279 return ObservationDataType.RANGE; 280 if ("Ratio".equals(codeString)) 281 return ObservationDataType.RATIO; 282 if ("SampledData".equals(codeString)) 283 return ObservationDataType.SAMPLEDDATA; 284 if ("time".equals(codeString)) 285 return ObservationDataType.TIME; 286 if ("dateTime".equals(codeString)) 287 return ObservationDataType.DATETIME; 288 if ("Period".equals(codeString)) 289 return ObservationDataType.PERIOD; 290 throw new IllegalArgumentException("Unknown ObservationDataType code '" + codeString + "'"); 291 } 292 293 public Enumeration<ObservationDataType> fromType(PrimitiveType<?> code) throws FHIRException { 294 if (code == null) 295 return null; 296 if (code.isEmpty()) 297 return new Enumeration<ObservationDataType>(this, ObservationDataType.NULL, code); 298 String codeString = code.asStringValue(); 299 if (codeString == null || "".equals(codeString)) 300 return new Enumeration<ObservationDataType>(this, ObservationDataType.NULL, code); 301 if ("Quantity".equals(codeString)) 302 return new Enumeration<ObservationDataType>(this, ObservationDataType.QUANTITY, code); 303 if ("CodeableConcept".equals(codeString)) 304 return new Enumeration<ObservationDataType>(this, ObservationDataType.CODEABLECONCEPT, code); 305 if ("string".equals(codeString)) 306 return new Enumeration<ObservationDataType>(this, ObservationDataType.STRING, code); 307 if ("boolean".equals(codeString)) 308 return new Enumeration<ObservationDataType>(this, ObservationDataType.BOOLEAN, code); 309 if ("integer".equals(codeString)) 310 return new Enumeration<ObservationDataType>(this, ObservationDataType.INTEGER, code); 311 if ("Range".equals(codeString)) 312 return new Enumeration<ObservationDataType>(this, ObservationDataType.RANGE, code); 313 if ("Ratio".equals(codeString)) 314 return new Enumeration<ObservationDataType>(this, ObservationDataType.RATIO, code); 315 if ("SampledData".equals(codeString)) 316 return new Enumeration<ObservationDataType>(this, ObservationDataType.SAMPLEDDATA, code); 317 if ("time".equals(codeString)) 318 return new Enumeration<ObservationDataType>(this, ObservationDataType.TIME, code); 319 if ("dateTime".equals(codeString)) 320 return new Enumeration<ObservationDataType>(this, ObservationDataType.DATETIME, code); 321 if ("Period".equals(codeString)) 322 return new Enumeration<ObservationDataType>(this, ObservationDataType.PERIOD, code); 323 throw new FHIRException("Unknown ObservationDataType code '" + codeString + "'"); 324 } 325 326 public String toCode(ObservationDataType code) { 327 if (code == ObservationDataType.NULL) 328 return null; 329 if (code == ObservationDataType.QUANTITY) 330 return "Quantity"; 331 if (code == ObservationDataType.CODEABLECONCEPT) 332 return "CodeableConcept"; 333 if (code == ObservationDataType.STRING) 334 return "string"; 335 if (code == ObservationDataType.BOOLEAN) 336 return "boolean"; 337 if (code == ObservationDataType.INTEGER) 338 return "integer"; 339 if (code == ObservationDataType.RANGE) 340 return "Range"; 341 if (code == ObservationDataType.RATIO) 342 return "Ratio"; 343 if (code == ObservationDataType.SAMPLEDDATA) 344 return "SampledData"; 345 if (code == ObservationDataType.TIME) 346 return "time"; 347 if (code == ObservationDataType.DATETIME) 348 return "dateTime"; 349 if (code == ObservationDataType.PERIOD) 350 return "Period"; 351 return "?"; 352 } 353 354 public String toSystem(ObservationDataType code) { 355 return code.getSystem(); 356 } 357 } 358 359 public enum ObservationRangeCategory { 360 /** 361 * Reference (Normal) Range for Ordinal and Continuous Observations. 362 */ 363 REFERENCE, 364 /** 365 * Critical Range for Ordinal and Continuous Observations. 366 */ 367 CRITICAL, 368 /** 369 * Absolute Range for Ordinal and Continuous Observations. Results outside this 370 * range are not possible. 371 */ 372 ABSOLUTE, 373 /** 374 * added to help the parsers with the generic types 375 */ 376 NULL; 377 378 public static ObservationRangeCategory fromCode(String codeString) throws FHIRException { 379 if (codeString == null || "".equals(codeString)) 380 return null; 381 if ("reference".equals(codeString)) 382 return REFERENCE; 383 if ("critical".equals(codeString)) 384 return CRITICAL; 385 if ("absolute".equals(codeString)) 386 return ABSOLUTE; 387 if (Configuration.isAcceptInvalidEnums()) 388 return null; 389 else 390 throw new FHIRException("Unknown ObservationRangeCategory code '" + codeString + "'"); 391 } 392 393 public String toCode() { 394 switch (this) { 395 case REFERENCE: 396 return "reference"; 397 case CRITICAL: 398 return "critical"; 399 case ABSOLUTE: 400 return "absolute"; 401 case NULL: 402 return null; 403 default: 404 return "?"; 405 } 406 } 407 408 public String getSystem() { 409 switch (this) { 410 case REFERENCE: 411 return "http://hl7.org/fhir/observation-range-category"; 412 case CRITICAL: 413 return "http://hl7.org/fhir/observation-range-category"; 414 case ABSOLUTE: 415 return "http://hl7.org/fhir/observation-range-category"; 416 case NULL: 417 return null; 418 default: 419 return "?"; 420 } 421 } 422 423 public String getDefinition() { 424 switch (this) { 425 case REFERENCE: 426 return "Reference (Normal) Range for Ordinal and Continuous Observations."; 427 case CRITICAL: 428 return "Critical Range for Ordinal and Continuous Observations."; 429 case ABSOLUTE: 430 return "Absolute Range for Ordinal and Continuous Observations. Results outside this range are not possible."; 431 case NULL: 432 return null; 433 default: 434 return "?"; 435 } 436 } 437 438 public String getDisplay() { 439 switch (this) { 440 case REFERENCE: 441 return "reference range"; 442 case CRITICAL: 443 return "critical range"; 444 case ABSOLUTE: 445 return "absolute range"; 446 case NULL: 447 return null; 448 default: 449 return "?"; 450 } 451 } 452 } 453 454 public static class ObservationRangeCategoryEnumFactory implements EnumFactory<ObservationRangeCategory> { 455 public ObservationRangeCategory fromCode(String codeString) throws IllegalArgumentException { 456 if (codeString == null || "".equals(codeString)) 457 if (codeString == null || "".equals(codeString)) 458 return null; 459 if ("reference".equals(codeString)) 460 return ObservationRangeCategory.REFERENCE; 461 if ("critical".equals(codeString)) 462 return ObservationRangeCategory.CRITICAL; 463 if ("absolute".equals(codeString)) 464 return ObservationRangeCategory.ABSOLUTE; 465 throw new IllegalArgumentException("Unknown ObservationRangeCategory code '" + codeString + "'"); 466 } 467 468 public Enumeration<ObservationRangeCategory> fromType(PrimitiveType<?> code) throws FHIRException { 469 if (code == null) 470 return null; 471 if (code.isEmpty()) 472 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.NULL, code); 473 String codeString = code.asStringValue(); 474 if (codeString == null || "".equals(codeString)) 475 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.NULL, code); 476 if ("reference".equals(codeString)) 477 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.REFERENCE, code); 478 if ("critical".equals(codeString)) 479 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.CRITICAL, code); 480 if ("absolute".equals(codeString)) 481 return new Enumeration<ObservationRangeCategory>(this, ObservationRangeCategory.ABSOLUTE, code); 482 throw new FHIRException("Unknown ObservationRangeCategory code '" + codeString + "'"); 483 } 484 485 public String toCode(ObservationRangeCategory code) { 486 if (code == ObservationRangeCategory.NULL) 487 return null; 488 if (code == ObservationRangeCategory.REFERENCE) 489 return "reference"; 490 if (code == ObservationRangeCategory.CRITICAL) 491 return "critical"; 492 if (code == ObservationRangeCategory.ABSOLUTE) 493 return "absolute"; 494 return "?"; 495 } 496 497 public String toSystem(ObservationRangeCategory code) { 498 return code.getSystem(); 499 } 500 } 501 502 @Block() 503 public static class ObservationDefinitionQuantitativeDetailsComponent extends BackboneElement 504 implements IBaseBackboneElement { 505 /** 506 * Customary unit used to report quantitative results of observations conforming 507 * to this ObservationDefinition. 508 */ 509 @Child(name = "customaryUnit", type = { 510 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 511 @Description(shortDefinition = "Customary unit for quantitative results", formalDefinition = "Customary unit used to report quantitative results of observations conforming to this ObservationDefinition.") 512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 513 protected CodeableConcept customaryUnit; 514 515 /** 516 * SI unit used to report quantitative results of observations conforming to 517 * this ObservationDefinition. 518 */ 519 @Child(name = "unit", type = { 520 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 521 @Description(shortDefinition = "SI unit for quantitative results", formalDefinition = "SI unit used to report quantitative results of observations conforming to this ObservationDefinition.") 522 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ucum-units") 523 protected CodeableConcept unit; 524 525 /** 526 * Factor for converting value expressed with SI unit to value expressed with 527 * customary unit. 528 */ 529 @Child(name = "conversionFactor", type = { 530 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 531 @Description(shortDefinition = "SI to Customary unit conversion factor", formalDefinition = "Factor for converting value expressed with SI unit to value expressed with customary unit.") 532 protected DecimalType conversionFactor; 533 534 /** 535 * Number of digits after decimal separator when the results of such 536 * observations are of type Quantity. 537 */ 538 @Child(name = "decimalPrecision", type = { 539 IntegerType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 540 @Description(shortDefinition = "Decimal precision of observation quantitative results", formalDefinition = "Number of digits after decimal separator when the results of such observations are of type Quantity.") 541 protected IntegerType decimalPrecision; 542 543 private static final long serialVersionUID = 1790019610L; 544 545 /** 546 * Constructor 547 */ 548 public ObservationDefinitionQuantitativeDetailsComponent() { 549 super(); 550 } 551 552 /** 553 * @return {@link #customaryUnit} (Customary unit used to report quantitative 554 * results of observations conforming to this ObservationDefinition.) 555 */ 556 public CodeableConcept getCustomaryUnit() { 557 if (this.customaryUnit == null) 558 if (Configuration.errorOnAutoCreate()) 559 throw new Error("Attempt to auto-create ObservationDefinitionQuantitativeDetailsComponent.customaryUnit"); 560 else if (Configuration.doAutoCreate()) 561 this.customaryUnit = new CodeableConcept(); // cc 562 return this.customaryUnit; 563 } 564 565 public boolean hasCustomaryUnit() { 566 return this.customaryUnit != null && !this.customaryUnit.isEmpty(); 567 } 568 569 /** 570 * @param value {@link #customaryUnit} (Customary unit used to report 571 * quantitative results of observations conforming to this 572 * ObservationDefinition.) 573 */ 574 public ObservationDefinitionQuantitativeDetailsComponent setCustomaryUnit(CodeableConcept value) { 575 this.customaryUnit = value; 576 return this; 577 } 578 579 /** 580 * @return {@link #unit} (SI unit used to report quantitative results of 581 * observations conforming to this ObservationDefinition.) 582 */ 583 public CodeableConcept getUnit() { 584 if (this.unit == null) 585 if (Configuration.errorOnAutoCreate()) 586 throw new Error("Attempt to auto-create ObservationDefinitionQuantitativeDetailsComponent.unit"); 587 else if (Configuration.doAutoCreate()) 588 this.unit = new CodeableConcept(); // cc 589 return this.unit; 590 } 591 592 public boolean hasUnit() { 593 return this.unit != null && !this.unit.isEmpty(); 594 } 595 596 /** 597 * @param value {@link #unit} (SI unit used to report quantitative results of 598 * observations conforming to this ObservationDefinition.) 599 */ 600 public ObservationDefinitionQuantitativeDetailsComponent setUnit(CodeableConcept value) { 601 this.unit = value; 602 return this; 603 } 604 605 /** 606 * @return {@link #conversionFactor} (Factor for converting value expressed with 607 * SI unit to value expressed with customary unit.). This is the 608 * underlying object with id, value and extensions. The accessor 609 * "getConversionFactor" gives direct access to the value 610 */ 611 public DecimalType getConversionFactorElement() { 612 if (this.conversionFactor == null) 613 if (Configuration.errorOnAutoCreate()) 614 throw new Error("Attempt to auto-create ObservationDefinitionQuantitativeDetailsComponent.conversionFactor"); 615 else if (Configuration.doAutoCreate()) 616 this.conversionFactor = new DecimalType(); // bb 617 return this.conversionFactor; 618 } 619 620 public boolean hasConversionFactorElement() { 621 return this.conversionFactor != null && !this.conversionFactor.isEmpty(); 622 } 623 624 public boolean hasConversionFactor() { 625 return this.conversionFactor != null && !this.conversionFactor.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #conversionFactor} (Factor for converting value expressed 630 * with SI unit to value expressed with customary unit.). This is 631 * the underlying object with id, value and extensions. The 632 * accessor "getConversionFactor" gives direct access to the value 633 */ 634 public ObservationDefinitionQuantitativeDetailsComponent setConversionFactorElement(DecimalType value) { 635 this.conversionFactor = value; 636 return this; 637 } 638 639 /** 640 * @return Factor for converting value expressed with SI unit to value expressed 641 * with customary unit. 642 */ 643 public BigDecimal getConversionFactor() { 644 return this.conversionFactor == null ? null : this.conversionFactor.getValue(); 645 } 646 647 /** 648 * @param value Factor for converting value expressed with SI unit to value 649 * expressed with customary unit. 650 */ 651 public ObservationDefinitionQuantitativeDetailsComponent setConversionFactor(BigDecimal value) { 652 if (value == null) 653 this.conversionFactor = null; 654 else { 655 if (this.conversionFactor == null) 656 this.conversionFactor = new DecimalType(); 657 this.conversionFactor.setValue(value); 658 } 659 return this; 660 } 661 662 /** 663 * @param value Factor for converting value expressed with SI unit to value 664 * expressed with customary unit. 665 */ 666 public ObservationDefinitionQuantitativeDetailsComponent setConversionFactor(long value) { 667 this.conversionFactor = new DecimalType(); 668 this.conversionFactor.setValue(value); 669 return this; 670 } 671 672 /** 673 * @param value Factor for converting value expressed with SI unit to value 674 * expressed with customary unit. 675 */ 676 public ObservationDefinitionQuantitativeDetailsComponent setConversionFactor(double value) { 677 this.conversionFactor = new DecimalType(); 678 this.conversionFactor.setValue(value); 679 return this; 680 } 681 682 /** 683 * @return {@link #decimalPrecision} (Number of digits after decimal separator 684 * when the results of such observations are of type Quantity.). This is 685 * the underlying object with id, value and extensions. The accessor 686 * "getDecimalPrecision" gives direct access to the value 687 */ 688 public IntegerType getDecimalPrecisionElement() { 689 if (this.decimalPrecision == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create ObservationDefinitionQuantitativeDetailsComponent.decimalPrecision"); 692 else if (Configuration.doAutoCreate()) 693 this.decimalPrecision = new IntegerType(); // bb 694 return this.decimalPrecision; 695 } 696 697 public boolean hasDecimalPrecisionElement() { 698 return this.decimalPrecision != null && !this.decimalPrecision.isEmpty(); 699 } 700 701 public boolean hasDecimalPrecision() { 702 return this.decimalPrecision != null && !this.decimalPrecision.isEmpty(); 703 } 704 705 /** 706 * @param value {@link #decimalPrecision} (Number of digits after decimal 707 * separator when the results of such observations are of type 708 * Quantity.). This is the underlying object with id, value and 709 * extensions. The accessor "getDecimalPrecision" gives direct 710 * access to the value 711 */ 712 public ObservationDefinitionQuantitativeDetailsComponent setDecimalPrecisionElement(IntegerType value) { 713 this.decimalPrecision = value; 714 return this; 715 } 716 717 /** 718 * @return Number of digits after decimal separator when the results of such 719 * observations are of type Quantity. 720 */ 721 public int getDecimalPrecision() { 722 return this.decimalPrecision == null || this.decimalPrecision.isEmpty() ? 0 : this.decimalPrecision.getValue(); 723 } 724 725 /** 726 * @param value Number of digits after decimal separator when the results of 727 * such observations are of type Quantity. 728 */ 729 public ObservationDefinitionQuantitativeDetailsComponent setDecimalPrecision(int value) { 730 if (this.decimalPrecision == null) 731 this.decimalPrecision = new IntegerType(); 732 this.decimalPrecision.setValue(value); 733 return this; 734 } 735 736 protected void listChildren(List<Property> children) { 737 super.listChildren(children); 738 children.add(new Property("customaryUnit", "CodeableConcept", 739 "Customary unit used to report quantitative results of observations conforming to this ObservationDefinition.", 740 0, 1, customaryUnit)); 741 children.add(new Property("unit", "CodeableConcept", 742 "SI unit used to report quantitative results of observations conforming to this ObservationDefinition.", 0, 1, 743 unit)); 744 children.add(new Property("conversionFactor", "decimal", 745 "Factor for converting value expressed with SI unit to value expressed with customary unit.", 0, 1, 746 conversionFactor)); 747 children.add(new Property("decimalPrecision", "integer", 748 "Number of digits after decimal separator when the results of such observations are of type Quantity.", 0, 1, 749 decimalPrecision)); 750 } 751 752 @Override 753 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 754 switch (_hash) { 755 case -1375586437: 756 /* customaryUnit */ return new Property("customaryUnit", "CodeableConcept", 757 "Customary unit used to report quantitative results of observations conforming to this ObservationDefinition.", 758 0, 1, customaryUnit); 759 case 3594628: 760 /* unit */ return new Property("unit", "CodeableConcept", 761 "SI unit used to report quantitative results of observations conforming to this ObservationDefinition.", 0, 762 1, unit); 763 case 1438876165: 764 /* conversionFactor */ return new Property("conversionFactor", "decimal", 765 "Factor for converting value expressed with SI unit to value expressed with customary unit.", 0, 1, 766 conversionFactor); 767 case -1564447699: 768 /* decimalPrecision */ return new Property("decimalPrecision", "integer", 769 "Number of digits after decimal separator when the results of such observations are of type Quantity.", 0, 770 1, decimalPrecision); 771 default: 772 return super.getNamedProperty(_hash, _name, _checkValid); 773 } 774 775 } 776 777 @Override 778 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 779 switch (hash) { 780 case -1375586437: 781 /* customaryUnit */ return this.customaryUnit == null ? new Base[0] : new Base[] { this.customaryUnit }; // CodeableConcept 782 case 3594628: 783 /* unit */ return this.unit == null ? new Base[0] : new Base[] { this.unit }; // CodeableConcept 784 case 1438876165: 785 /* conversionFactor */ return this.conversionFactor == null ? new Base[0] 786 : new Base[] { this.conversionFactor }; // DecimalType 787 case -1564447699: 788 /* decimalPrecision */ return this.decimalPrecision == null ? new Base[0] 789 : new Base[] { this.decimalPrecision }; // IntegerType 790 default: 791 return super.getProperty(hash, name, checkValid); 792 } 793 794 } 795 796 @Override 797 public Base setProperty(int hash, String name, Base value) throws FHIRException { 798 switch (hash) { 799 case -1375586437: // customaryUnit 800 this.customaryUnit = castToCodeableConcept(value); // CodeableConcept 801 return value; 802 case 3594628: // unit 803 this.unit = castToCodeableConcept(value); // CodeableConcept 804 return value; 805 case 1438876165: // conversionFactor 806 this.conversionFactor = castToDecimal(value); // DecimalType 807 return value; 808 case -1564447699: // decimalPrecision 809 this.decimalPrecision = castToInteger(value); // IntegerType 810 return value; 811 default: 812 return super.setProperty(hash, name, value); 813 } 814 815 } 816 817 @Override 818 public Base setProperty(String name, Base value) throws FHIRException { 819 if (name.equals("customaryUnit")) { 820 this.customaryUnit = castToCodeableConcept(value); // CodeableConcept 821 } else if (name.equals("unit")) { 822 this.unit = castToCodeableConcept(value); // CodeableConcept 823 } else if (name.equals("conversionFactor")) { 824 this.conversionFactor = castToDecimal(value); // DecimalType 825 } else if (name.equals("decimalPrecision")) { 826 this.decimalPrecision = castToInteger(value); // IntegerType 827 } else 828 return super.setProperty(name, value); 829 return value; 830 } 831 832 @Override 833 public void removeChild(String name, Base value) throws FHIRException { 834 if (name.equals("customaryUnit")) { 835 this.customaryUnit = null; 836 } else if (name.equals("unit")) { 837 this.unit = null; 838 } else if (name.equals("conversionFactor")) { 839 this.conversionFactor = null; 840 } else if (name.equals("decimalPrecision")) { 841 this.decimalPrecision = null; 842 } else 843 super.removeChild(name, value); 844 845 } 846 847 @Override 848 public Base makeProperty(int hash, String name) throws FHIRException { 849 switch (hash) { 850 case -1375586437: 851 return getCustomaryUnit(); 852 case 3594628: 853 return getUnit(); 854 case 1438876165: 855 return getConversionFactorElement(); 856 case -1564447699: 857 return getDecimalPrecisionElement(); 858 default: 859 return super.makeProperty(hash, name); 860 } 861 862 } 863 864 @Override 865 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 866 switch (hash) { 867 case -1375586437: 868 /* customaryUnit */ return new String[] { "CodeableConcept" }; 869 case 3594628: 870 /* unit */ return new String[] { "CodeableConcept" }; 871 case 1438876165: 872 /* conversionFactor */ return new String[] { "decimal" }; 873 case -1564447699: 874 /* decimalPrecision */ return new String[] { "integer" }; 875 default: 876 return super.getTypesForProperty(hash, name); 877 } 878 879 } 880 881 @Override 882 public Base addChild(String name) throws FHIRException { 883 if (name.equals("customaryUnit")) { 884 this.customaryUnit = new CodeableConcept(); 885 return this.customaryUnit; 886 } else if (name.equals("unit")) { 887 this.unit = new CodeableConcept(); 888 return this.unit; 889 } else if (name.equals("conversionFactor")) { 890 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.conversionFactor"); 891 } else if (name.equals("decimalPrecision")) { 892 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.decimalPrecision"); 893 } else 894 return super.addChild(name); 895 } 896 897 public ObservationDefinitionQuantitativeDetailsComponent copy() { 898 ObservationDefinitionQuantitativeDetailsComponent dst = new ObservationDefinitionQuantitativeDetailsComponent(); 899 copyValues(dst); 900 return dst; 901 } 902 903 public void copyValues(ObservationDefinitionQuantitativeDetailsComponent dst) { 904 super.copyValues(dst); 905 dst.customaryUnit = customaryUnit == null ? null : customaryUnit.copy(); 906 dst.unit = unit == null ? null : unit.copy(); 907 dst.conversionFactor = conversionFactor == null ? null : conversionFactor.copy(); 908 dst.decimalPrecision = decimalPrecision == null ? null : decimalPrecision.copy(); 909 } 910 911 @Override 912 public boolean equalsDeep(Base other_) { 913 if (!super.equalsDeep(other_)) 914 return false; 915 if (!(other_ instanceof ObservationDefinitionQuantitativeDetailsComponent)) 916 return false; 917 ObservationDefinitionQuantitativeDetailsComponent o = (ObservationDefinitionQuantitativeDetailsComponent) other_; 918 return compareDeep(customaryUnit, o.customaryUnit, true) && compareDeep(unit, o.unit, true) 919 && compareDeep(conversionFactor, o.conversionFactor, true) 920 && compareDeep(decimalPrecision, o.decimalPrecision, true); 921 } 922 923 @Override 924 public boolean equalsShallow(Base other_) { 925 if (!super.equalsShallow(other_)) 926 return false; 927 if (!(other_ instanceof ObservationDefinitionQuantitativeDetailsComponent)) 928 return false; 929 ObservationDefinitionQuantitativeDetailsComponent o = (ObservationDefinitionQuantitativeDetailsComponent) other_; 930 return compareValues(conversionFactor, o.conversionFactor, true) 931 && compareValues(decimalPrecision, o.decimalPrecision, true); 932 } 933 934 public boolean isEmpty() { 935 return super.isEmpty() 936 && ca.uhn.fhir.util.ElementUtil.isEmpty(customaryUnit, unit, conversionFactor, decimalPrecision); 937 } 938 939 public String fhirType() { 940 return "ObservationDefinition.quantitativeDetails"; 941 942 } 943 944 } 945 946 @Block() 947 public static class ObservationDefinitionQualifiedIntervalComponent extends BackboneElement 948 implements IBaseBackboneElement { 949 /** 950 * The category of interval of values for continuous or ordinal observations 951 * conforming to this ObservationDefinition. 952 */ 953 @Child(name = "category", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 954 @Description(shortDefinition = "reference | critical | absolute", formalDefinition = "The category of interval of values for continuous or ordinal observations conforming to this ObservationDefinition.") 955 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-range-category") 956 protected Enumeration<ObservationRangeCategory> category; 957 958 /** 959 * The low and high values determining the interval. There may be only one of 960 * the two. 961 */ 962 @Child(name = "range", type = { Range.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 963 @Description(shortDefinition = "The interval itself, for continuous or ordinal observations", formalDefinition = "The low and high values determining the interval. There may be only one of the two.") 964 protected Range range; 965 966 /** 967 * Codes to indicate the health context the range applies to. For example, the 968 * normal or therapeutic range. 969 */ 970 @Child(name = "context", type = { 971 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 972 @Description(shortDefinition = "Range context qualifier", formalDefinition = "Codes to indicate the health context the range applies to. For example, the normal or therapeutic range.") 973 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/referencerange-meaning") 974 protected CodeableConcept context; 975 976 /** 977 * Codes to indicate the target population this reference range applies to. 978 */ 979 @Child(name = "appliesTo", type = { 980 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 981 @Description(shortDefinition = "Targetted population of the range", formalDefinition = "Codes to indicate the target population this reference range applies to.") 982 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/referencerange-appliesto") 983 protected List<CodeableConcept> appliesTo; 984 985 /** 986 * Sex of the population the range applies to. 987 */ 988 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 989 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Sex of the population the range applies to.") 990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 991 protected Enumeration<AdministrativeGender> gender; 992 993 /** 994 * The age at which this reference range is applicable. This is a neonatal age 995 * (e.g. number of weeks at term) if the meaning says so. 996 */ 997 @Child(name = "age", type = { Range.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 998 @Description(shortDefinition = "Applicable age range, if relevant", formalDefinition = "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.") 999 protected Range age; 1000 1001 /** 1002 * The gestational age to which this reference range is applicable, in the 1003 * context of pregnancy. 1004 */ 1005 @Child(name = "gestationalAge", type = { 1006 Range.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1007 @Description(shortDefinition = "Applicable gestational age range, if relevant", formalDefinition = "The gestational age to which this reference range is applicable, in the context of pregnancy.") 1008 protected Range gestationalAge; 1009 1010 /** 1011 * Text based condition for which the reference range is valid. 1012 */ 1013 @Child(name = "condition", type = { 1014 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1015 @Description(shortDefinition = "Condition associated with the reference range", formalDefinition = "Text based condition for which the reference range is valid.") 1016 protected StringType condition; 1017 1018 private static final long serialVersionUID = -416423468L; 1019 1020 /** 1021 * Constructor 1022 */ 1023 public ObservationDefinitionQualifiedIntervalComponent() { 1024 super(); 1025 } 1026 1027 /** 1028 * @return {@link #category} (The category of interval of values for continuous 1029 * or ordinal observations conforming to this ObservationDefinition.). 1030 * This is the underlying object with id, value and extensions. The 1031 * accessor "getCategory" gives direct access to the value 1032 */ 1033 public Enumeration<ObservationRangeCategory> getCategoryElement() { 1034 if (this.category == null) 1035 if (Configuration.errorOnAutoCreate()) 1036 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.category"); 1037 else if (Configuration.doAutoCreate()) 1038 this.category = new Enumeration<ObservationRangeCategory>(new ObservationRangeCategoryEnumFactory()); // bb 1039 return this.category; 1040 } 1041 1042 public boolean hasCategoryElement() { 1043 return this.category != null && !this.category.isEmpty(); 1044 } 1045 1046 public boolean hasCategory() { 1047 return this.category != null && !this.category.isEmpty(); 1048 } 1049 1050 /** 1051 * @param value {@link #category} (The category of interval of values for 1052 * continuous or ordinal observations conforming to this 1053 * ObservationDefinition.). This is the underlying object with id, 1054 * value and extensions. The accessor "getCategory" gives direct 1055 * access to the value 1056 */ 1057 public ObservationDefinitionQualifiedIntervalComponent setCategoryElement( 1058 Enumeration<ObservationRangeCategory> value) { 1059 this.category = value; 1060 return this; 1061 } 1062 1063 /** 1064 * @return The category of interval of values for continuous or ordinal 1065 * observations conforming to this ObservationDefinition. 1066 */ 1067 public ObservationRangeCategory getCategory() { 1068 return this.category == null ? null : this.category.getValue(); 1069 } 1070 1071 /** 1072 * @param value The category of interval of values for continuous or ordinal 1073 * observations conforming to this ObservationDefinition. 1074 */ 1075 public ObservationDefinitionQualifiedIntervalComponent setCategory(ObservationRangeCategory value) { 1076 if (value == null) 1077 this.category = null; 1078 else { 1079 if (this.category == null) 1080 this.category = new Enumeration<ObservationRangeCategory>(new ObservationRangeCategoryEnumFactory()); 1081 this.category.setValue(value); 1082 } 1083 return this; 1084 } 1085 1086 /** 1087 * @return {@link #range} (The low and high values determining the interval. 1088 * There may be only one of the two.) 1089 */ 1090 public Range getRange() { 1091 if (this.range == null) 1092 if (Configuration.errorOnAutoCreate()) 1093 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.range"); 1094 else if (Configuration.doAutoCreate()) 1095 this.range = new Range(); // cc 1096 return this.range; 1097 } 1098 1099 public boolean hasRange() { 1100 return this.range != null && !this.range.isEmpty(); 1101 } 1102 1103 /** 1104 * @param value {@link #range} (The low and high values determining the 1105 * interval. There may be only one of the two.) 1106 */ 1107 public ObservationDefinitionQualifiedIntervalComponent setRange(Range value) { 1108 this.range = value; 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #context} (Codes to indicate the health context the range 1114 * applies to. For example, the normal or therapeutic range.) 1115 */ 1116 public CodeableConcept getContext() { 1117 if (this.context == null) 1118 if (Configuration.errorOnAutoCreate()) 1119 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.context"); 1120 else if (Configuration.doAutoCreate()) 1121 this.context = new CodeableConcept(); // cc 1122 return this.context; 1123 } 1124 1125 public boolean hasContext() { 1126 return this.context != null && !this.context.isEmpty(); 1127 } 1128 1129 /** 1130 * @param value {@link #context} (Codes to indicate the health context the range 1131 * applies to. For example, the normal or therapeutic range.) 1132 */ 1133 public ObservationDefinitionQualifiedIntervalComponent setContext(CodeableConcept value) { 1134 this.context = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return {@link #appliesTo} (Codes to indicate the target population this 1140 * reference range applies to.) 1141 */ 1142 public List<CodeableConcept> getAppliesTo() { 1143 if (this.appliesTo == null) 1144 this.appliesTo = new ArrayList<CodeableConcept>(); 1145 return this.appliesTo; 1146 } 1147 1148 /** 1149 * @return Returns a reference to <code>this</code> for easy method chaining 1150 */ 1151 public ObservationDefinitionQualifiedIntervalComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 1152 this.appliesTo = theAppliesTo; 1153 return this; 1154 } 1155 1156 public boolean hasAppliesTo() { 1157 if (this.appliesTo == null) 1158 return false; 1159 for (CodeableConcept item : this.appliesTo) 1160 if (!item.isEmpty()) 1161 return true; 1162 return false; 1163 } 1164 1165 public CodeableConcept addAppliesTo() { // 3 1166 CodeableConcept t = new CodeableConcept(); 1167 if (this.appliesTo == null) 1168 this.appliesTo = new ArrayList<CodeableConcept>(); 1169 this.appliesTo.add(t); 1170 return t; 1171 } 1172 1173 public ObservationDefinitionQualifiedIntervalComponent addAppliesTo(CodeableConcept t) { // 3 1174 if (t == null) 1175 return this; 1176 if (this.appliesTo == null) 1177 this.appliesTo = new ArrayList<CodeableConcept>(); 1178 this.appliesTo.add(t); 1179 return this; 1180 } 1181 1182 /** 1183 * @return The first repetition of repeating field {@link #appliesTo}, creating 1184 * it if it does not already exist 1185 */ 1186 public CodeableConcept getAppliesToFirstRep() { 1187 if (getAppliesTo().isEmpty()) { 1188 addAppliesTo(); 1189 } 1190 return getAppliesTo().get(0); 1191 } 1192 1193 /** 1194 * @return {@link #gender} (Sex of the population the range applies to.). This 1195 * is the underlying object with id, value and extensions. The accessor 1196 * "getGender" gives direct access to the value 1197 */ 1198 public Enumeration<AdministrativeGender> getGenderElement() { 1199 if (this.gender == null) 1200 if (Configuration.errorOnAutoCreate()) 1201 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.gender"); 1202 else if (Configuration.doAutoCreate()) 1203 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1204 return this.gender; 1205 } 1206 1207 public boolean hasGenderElement() { 1208 return this.gender != null && !this.gender.isEmpty(); 1209 } 1210 1211 public boolean hasGender() { 1212 return this.gender != null && !this.gender.isEmpty(); 1213 } 1214 1215 /** 1216 * @param value {@link #gender} (Sex of the population the range applies to.). 1217 * This is the underlying object with id, value and extensions. The 1218 * accessor "getGender" gives direct access to the value 1219 */ 1220 public ObservationDefinitionQualifiedIntervalComponent setGenderElement(Enumeration<AdministrativeGender> value) { 1221 this.gender = value; 1222 return this; 1223 } 1224 1225 /** 1226 * @return Sex of the population the range applies to. 1227 */ 1228 public AdministrativeGender getGender() { 1229 return this.gender == null ? null : this.gender.getValue(); 1230 } 1231 1232 /** 1233 * @param value Sex of the population the range applies to. 1234 */ 1235 public ObservationDefinitionQualifiedIntervalComponent setGender(AdministrativeGender value) { 1236 if (value == null) 1237 this.gender = null; 1238 else { 1239 if (this.gender == null) 1240 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1241 this.gender.setValue(value); 1242 } 1243 return this; 1244 } 1245 1246 /** 1247 * @return {@link #age} (The age at which this reference range is applicable. 1248 * This is a neonatal age (e.g. number of weeks at term) if the meaning 1249 * says so.) 1250 */ 1251 public Range getAge() { 1252 if (this.age == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.age"); 1255 else if (Configuration.doAutoCreate()) 1256 this.age = new Range(); // cc 1257 return this.age; 1258 } 1259 1260 public boolean hasAge() { 1261 return this.age != null && !this.age.isEmpty(); 1262 } 1263 1264 /** 1265 * @param value {@link #age} (The age at which this reference range is 1266 * applicable. This is a neonatal age (e.g. number of weeks at 1267 * term) if the meaning says so.) 1268 */ 1269 public ObservationDefinitionQualifiedIntervalComponent setAge(Range value) { 1270 this.age = value; 1271 return this; 1272 } 1273 1274 /** 1275 * @return {@link #gestationalAge} (The gestational age to which this reference 1276 * range is applicable, in the context of pregnancy.) 1277 */ 1278 public Range getGestationalAge() { 1279 if (this.gestationalAge == null) 1280 if (Configuration.errorOnAutoCreate()) 1281 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.gestationalAge"); 1282 else if (Configuration.doAutoCreate()) 1283 this.gestationalAge = new Range(); // cc 1284 return this.gestationalAge; 1285 } 1286 1287 public boolean hasGestationalAge() { 1288 return this.gestationalAge != null && !this.gestationalAge.isEmpty(); 1289 } 1290 1291 /** 1292 * @param value {@link #gestationalAge} (The gestational age to which this 1293 * reference range is applicable, in the context of pregnancy.) 1294 */ 1295 public ObservationDefinitionQualifiedIntervalComponent setGestationalAge(Range value) { 1296 this.gestationalAge = value; 1297 return this; 1298 } 1299 1300 /** 1301 * @return {@link #condition} (Text based condition for which the reference 1302 * range is valid.). This is the underlying object with id, value and 1303 * extensions. The accessor "getCondition" gives direct access to the 1304 * value 1305 */ 1306 public StringType getConditionElement() { 1307 if (this.condition == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create ObservationDefinitionQualifiedIntervalComponent.condition"); 1310 else if (Configuration.doAutoCreate()) 1311 this.condition = new StringType(); // bb 1312 return this.condition; 1313 } 1314 1315 public boolean hasConditionElement() { 1316 return this.condition != null && !this.condition.isEmpty(); 1317 } 1318 1319 public boolean hasCondition() { 1320 return this.condition != null && !this.condition.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #condition} (Text based condition for which the reference 1325 * range is valid.). This is the underlying object with id, value 1326 * and extensions. The accessor "getCondition" gives direct access 1327 * to the value 1328 */ 1329 public ObservationDefinitionQualifiedIntervalComponent setConditionElement(StringType value) { 1330 this.condition = value; 1331 return this; 1332 } 1333 1334 /** 1335 * @return Text based condition for which the reference range is valid. 1336 */ 1337 public String getCondition() { 1338 return this.condition == null ? null : this.condition.getValue(); 1339 } 1340 1341 /** 1342 * @param value Text based condition for which the reference range is valid. 1343 */ 1344 public ObservationDefinitionQualifiedIntervalComponent setCondition(String value) { 1345 if (Utilities.noString(value)) 1346 this.condition = null; 1347 else { 1348 if (this.condition == null) 1349 this.condition = new StringType(); 1350 this.condition.setValue(value); 1351 } 1352 return this; 1353 } 1354 1355 protected void listChildren(List<Property> children) { 1356 super.listChildren(children); 1357 children.add(new Property("category", "code", 1358 "The category of interval of values for continuous or ordinal observations conforming to this ObservationDefinition.", 1359 0, 1, category)); 1360 children.add(new Property("range", "Range", 1361 "The low and high values determining the interval. There may be only one of the two.", 0, 1, range)); 1362 children.add(new Property("context", "CodeableConcept", 1363 "Codes to indicate the health context the range applies to. For example, the normal or therapeutic range.", 0, 1364 1, context)); 1365 children.add(new Property("appliesTo", "CodeableConcept", 1366 "Codes to indicate the target population this reference range applies to.", 0, java.lang.Integer.MAX_VALUE, 1367 appliesTo)); 1368 children.add(new Property("gender", "code", "Sex of the population the range applies to.", 0, 1, gender)); 1369 children.add(new Property("age", "Range", 1370 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 1371 0, 1, age)); 1372 children.add(new Property("gestationalAge", "Range", 1373 "The gestational age to which this reference range is applicable, in the context of pregnancy.", 0, 1, 1374 gestationalAge)); 1375 children.add(new Property("condition", "string", "Text based condition for which the reference range is valid.", 1376 0, 1, condition)); 1377 } 1378 1379 @Override 1380 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1381 switch (_hash) { 1382 case 50511102: 1383 /* category */ return new Property("category", "code", 1384 "The category of interval of values for continuous or ordinal observations conforming to this ObservationDefinition.", 1385 0, 1, category); 1386 case 108280125: 1387 /* range */ return new Property("range", "Range", 1388 "The low and high values determining the interval. There may be only one of the two.", 0, 1, range); 1389 case 951530927: 1390 /* context */ return new Property("context", "CodeableConcept", 1391 "Codes to indicate the health context the range applies to. For example, the normal or therapeutic range.", 1392 0, 1, context); 1393 case -2089924569: 1394 /* appliesTo */ return new Property("appliesTo", "CodeableConcept", 1395 "Codes to indicate the target population this reference range applies to.", 0, java.lang.Integer.MAX_VALUE, 1396 appliesTo); 1397 case -1249512767: 1398 /* gender */ return new Property("gender", "code", "Sex of the population the range applies to.", 0, 1, gender); 1399 case 96511: 1400 /* age */ return new Property("age", "Range", 1401 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 1402 0, 1, age); 1403 case -241217538: 1404 /* gestationalAge */ return new Property("gestationalAge", "Range", 1405 "The gestational age to which this reference range is applicable, in the context of pregnancy.", 0, 1, 1406 gestationalAge); 1407 case -861311717: 1408 /* condition */ return new Property("condition", "string", 1409 "Text based condition for which the reference range is valid.", 0, 1, condition); 1410 default: 1411 return super.getNamedProperty(_hash, _name, _checkValid); 1412 } 1413 1414 } 1415 1416 @Override 1417 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1418 switch (hash) { 1419 case 50511102: 1420 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // Enumeration<ObservationRangeCategory> 1421 case 108280125: 1422 /* range */ return this.range == null ? new Base[0] : new Base[] { this.range }; // Range 1423 case 951530927: 1424 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // CodeableConcept 1425 case -2089924569: 1426 /* appliesTo */ return this.appliesTo == null ? new Base[0] 1427 : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 1428 case -1249512767: 1429 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 1430 case 96511: 1431 /* age */ return this.age == null ? new Base[0] : new Base[] { this.age }; // Range 1432 case -241217538: 1433 /* gestationalAge */ return this.gestationalAge == null ? new Base[0] : new Base[] { this.gestationalAge }; // Range 1434 case -861311717: 1435 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // StringType 1436 default: 1437 return super.getProperty(hash, name, checkValid); 1438 } 1439 1440 } 1441 1442 @Override 1443 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1444 switch (hash) { 1445 case 50511102: // category 1446 value = new ObservationRangeCategoryEnumFactory().fromType(castToCode(value)); 1447 this.category = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1448 return value; 1449 case 108280125: // range 1450 this.range = castToRange(value); // Range 1451 return value; 1452 case 951530927: // context 1453 this.context = castToCodeableConcept(value); // CodeableConcept 1454 return value; 1455 case -2089924569: // appliesTo 1456 this.getAppliesTo().add(castToCodeableConcept(value)); // CodeableConcept 1457 return value; 1458 case -1249512767: // gender 1459 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1460 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1461 return value; 1462 case 96511: // age 1463 this.age = castToRange(value); // Range 1464 return value; 1465 case -241217538: // gestationalAge 1466 this.gestationalAge = castToRange(value); // Range 1467 return value; 1468 case -861311717: // condition 1469 this.condition = castToString(value); // StringType 1470 return value; 1471 default: 1472 return super.setProperty(hash, name, value); 1473 } 1474 1475 } 1476 1477 @Override 1478 public Base setProperty(String name, Base value) throws FHIRException { 1479 if (name.equals("category")) { 1480 value = new ObservationRangeCategoryEnumFactory().fromType(castToCode(value)); 1481 this.category = (Enumeration) value; // Enumeration<ObservationRangeCategory> 1482 } else if (name.equals("range")) { 1483 this.range = castToRange(value); // Range 1484 } else if (name.equals("context")) { 1485 this.context = castToCodeableConcept(value); // CodeableConcept 1486 } else if (name.equals("appliesTo")) { 1487 this.getAppliesTo().add(castToCodeableConcept(value)); 1488 } else if (name.equals("gender")) { 1489 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1490 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1491 } else if (name.equals("age")) { 1492 this.age = castToRange(value); // Range 1493 } else if (name.equals("gestationalAge")) { 1494 this.gestationalAge = castToRange(value); // Range 1495 } else if (name.equals("condition")) { 1496 this.condition = castToString(value); // StringType 1497 } else 1498 return super.setProperty(name, value); 1499 return value; 1500 } 1501 1502 @Override 1503 public void removeChild(String name, Base value) throws FHIRException { 1504 if (name.equals("category")) { 1505 this.category = null; 1506 } else if (name.equals("range")) { 1507 this.range = null; 1508 } else if (name.equals("context")) { 1509 this.context = null; 1510 } else if (name.equals("appliesTo")) { 1511 this.getAppliesTo().remove(castToCodeableConcept(value)); 1512 } else if (name.equals("gender")) { 1513 this.gender = null; 1514 } else if (name.equals("age")) { 1515 this.age = null; 1516 } else if (name.equals("gestationalAge")) { 1517 this.gestationalAge = null; 1518 } else if (name.equals("condition")) { 1519 this.condition = null; 1520 } else 1521 super.removeChild(name, value); 1522 1523 } 1524 1525 @Override 1526 public Base makeProperty(int hash, String name) throws FHIRException { 1527 switch (hash) { 1528 case 50511102: 1529 return getCategoryElement(); 1530 case 108280125: 1531 return getRange(); 1532 case 951530927: 1533 return getContext(); 1534 case -2089924569: 1535 return addAppliesTo(); 1536 case -1249512767: 1537 return getGenderElement(); 1538 case 96511: 1539 return getAge(); 1540 case -241217538: 1541 return getGestationalAge(); 1542 case -861311717: 1543 return getConditionElement(); 1544 default: 1545 return super.makeProperty(hash, name); 1546 } 1547 1548 } 1549 1550 @Override 1551 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1552 switch (hash) { 1553 case 50511102: 1554 /* category */ return new String[] { "code" }; 1555 case 108280125: 1556 /* range */ return new String[] { "Range" }; 1557 case 951530927: 1558 /* context */ return new String[] { "CodeableConcept" }; 1559 case -2089924569: 1560 /* appliesTo */ return new String[] { "CodeableConcept" }; 1561 case -1249512767: 1562 /* gender */ return new String[] { "code" }; 1563 case 96511: 1564 /* age */ return new String[] { "Range" }; 1565 case -241217538: 1566 /* gestationalAge */ return new String[] { "Range" }; 1567 case -861311717: 1568 /* condition */ return new String[] { "string" }; 1569 default: 1570 return super.getTypesForProperty(hash, name); 1571 } 1572 1573 } 1574 1575 @Override 1576 public Base addChild(String name) throws FHIRException { 1577 if (name.equals("category")) { 1578 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.category"); 1579 } else if (name.equals("range")) { 1580 this.range = new Range(); 1581 return this.range; 1582 } else if (name.equals("context")) { 1583 this.context = new CodeableConcept(); 1584 return this.context; 1585 } else if (name.equals("appliesTo")) { 1586 return addAppliesTo(); 1587 } else if (name.equals("gender")) { 1588 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.gender"); 1589 } else if (name.equals("age")) { 1590 this.age = new Range(); 1591 return this.age; 1592 } else if (name.equals("gestationalAge")) { 1593 this.gestationalAge = new Range(); 1594 return this.gestationalAge; 1595 } else if (name.equals("condition")) { 1596 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.condition"); 1597 } else 1598 return super.addChild(name); 1599 } 1600 1601 public ObservationDefinitionQualifiedIntervalComponent copy() { 1602 ObservationDefinitionQualifiedIntervalComponent dst = new ObservationDefinitionQualifiedIntervalComponent(); 1603 copyValues(dst); 1604 return dst; 1605 } 1606 1607 public void copyValues(ObservationDefinitionQualifiedIntervalComponent dst) { 1608 super.copyValues(dst); 1609 dst.category = category == null ? null : category.copy(); 1610 dst.range = range == null ? null : range.copy(); 1611 dst.context = context == null ? null : context.copy(); 1612 if (appliesTo != null) { 1613 dst.appliesTo = new ArrayList<CodeableConcept>(); 1614 for (CodeableConcept i : appliesTo) 1615 dst.appliesTo.add(i.copy()); 1616 } 1617 ; 1618 dst.gender = gender == null ? null : gender.copy(); 1619 dst.age = age == null ? null : age.copy(); 1620 dst.gestationalAge = gestationalAge == null ? null : gestationalAge.copy(); 1621 dst.condition = condition == null ? null : condition.copy(); 1622 } 1623 1624 @Override 1625 public boolean equalsDeep(Base other_) { 1626 if (!super.equalsDeep(other_)) 1627 return false; 1628 if (!(other_ instanceof ObservationDefinitionQualifiedIntervalComponent)) 1629 return false; 1630 ObservationDefinitionQualifiedIntervalComponent o = (ObservationDefinitionQualifiedIntervalComponent) other_; 1631 return compareDeep(category, o.category, true) && compareDeep(range, o.range, true) 1632 && compareDeep(context, o.context, true) && compareDeep(appliesTo, o.appliesTo, true) 1633 && compareDeep(gender, o.gender, true) && compareDeep(age, o.age, true) 1634 && compareDeep(gestationalAge, o.gestationalAge, true) && compareDeep(condition, o.condition, true); 1635 } 1636 1637 @Override 1638 public boolean equalsShallow(Base other_) { 1639 if (!super.equalsShallow(other_)) 1640 return false; 1641 if (!(other_ instanceof ObservationDefinitionQualifiedIntervalComponent)) 1642 return false; 1643 ObservationDefinitionQualifiedIntervalComponent o = (ObservationDefinitionQualifiedIntervalComponent) other_; 1644 return compareValues(category, o.category, true) && compareValues(gender, o.gender, true) 1645 && compareValues(condition, o.condition, true); 1646 } 1647 1648 public boolean isEmpty() { 1649 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, range, context, appliesTo, gender, age, 1650 gestationalAge, condition); 1651 } 1652 1653 public String fhirType() { 1654 return "ObservationDefinition.qualifiedInterval"; 1655 1656 } 1657 1658 } 1659 1660 /** 1661 * A code that classifies the general type of observation. 1662 */ 1663 @Child(name = "category", type = { 1664 CodeableConcept.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1665 @Description(shortDefinition = "Category of observation", formalDefinition = "A code that classifies the general type of observation.") 1666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-category") 1667 protected List<CodeableConcept> category; 1668 1669 /** 1670 * Describes what will be observed. Sometimes this is called the observation 1671 * "name". 1672 */ 1673 @Child(name = "code", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1674 @Description(shortDefinition = "Type of observation (code / type)", formalDefinition = "Describes what will be observed. Sometimes this is called the observation \"name\".") 1675 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 1676 protected CodeableConcept code; 1677 1678 /** 1679 * A unique identifier assigned to this ObservationDefinition artifact. 1680 */ 1681 @Child(name = "identifier", type = { 1682 Identifier.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1683 @Description(shortDefinition = "Business identifier for this ObservationDefinition instance", formalDefinition = "A unique identifier assigned to this ObservationDefinition artifact.") 1684 protected List<Identifier> identifier; 1685 1686 /** 1687 * The data types allowed for the value element of the instance observations 1688 * conforming to this ObservationDefinition. 1689 */ 1690 @Child(name = "permittedDataType", type = { 1691 CodeType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1692 @Description(shortDefinition = "Quantity | CodeableConcept | string | boolean | integer | Range | Ratio | SampledData | time | dateTime | Period", formalDefinition = "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.") 1693 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/permitted-data-type") 1694 protected List<Enumeration<ObservationDataType>> permittedDataType; 1695 1696 /** 1697 * Multiple results allowed for observations conforming to this 1698 * ObservationDefinition. 1699 */ 1700 @Child(name = "multipleResultsAllowed", type = { 1701 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1702 @Description(shortDefinition = "Multiple results allowed", formalDefinition = "Multiple results allowed for observations conforming to this ObservationDefinition.") 1703 protected BooleanType multipleResultsAllowed; 1704 1705 /** 1706 * The method or technique used to perform the observation. 1707 */ 1708 @Child(name = "method", type = { 1709 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1710 @Description(shortDefinition = "Method used to produce the observation", formalDefinition = "The method or technique used to perform the observation.") 1711 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-methods") 1712 protected CodeableConcept method; 1713 1714 /** 1715 * The preferred name to be used when reporting the results of observations 1716 * conforming to this ObservationDefinition. 1717 */ 1718 @Child(name = "preferredReportName", type = { 1719 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1720 @Description(shortDefinition = "Preferred report name", formalDefinition = "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.") 1721 protected StringType preferredReportName; 1722 1723 /** 1724 * Characteristics for quantitative results of this observation. 1725 */ 1726 @Child(name = "quantitativeDetails", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 1727 @Description(shortDefinition = "Characteristics of quantitative results", formalDefinition = "Characteristics for quantitative results of this observation.") 1728 protected ObservationDefinitionQuantitativeDetailsComponent quantitativeDetails; 1729 1730 /** 1731 * Multiple ranges of results qualified by different contexts for ordinal or 1732 * continuous observations conforming to this ObservationDefinition. 1733 */ 1734 @Child(name = "qualifiedInterval", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1735 @Description(shortDefinition = "Qualified range for continuous and ordinal observation results", formalDefinition = "Multiple ranges of results qualified by different contexts for ordinal or continuous observations conforming to this ObservationDefinition.") 1736 protected List<ObservationDefinitionQualifiedIntervalComponent> qualifiedInterval; 1737 1738 /** 1739 * The set of valid coded results for the observations conforming to this 1740 * ObservationDefinition. 1741 */ 1742 @Child(name = "validCodedValueSet", type = { 1743 ValueSet.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1744 @Description(shortDefinition = "Value set of valid coded values for the observations conforming to this ObservationDefinition", formalDefinition = "The set of valid coded results for the observations conforming to this ObservationDefinition.") 1745 protected Reference validCodedValueSet; 1746 1747 /** 1748 * The actual object that is the target of the reference (The set of valid coded 1749 * results for the observations conforming to this ObservationDefinition.) 1750 */ 1751 protected ValueSet validCodedValueSetTarget; 1752 1753 /** 1754 * The set of normal coded results for the observations conforming to this 1755 * ObservationDefinition. 1756 */ 1757 @Child(name = "normalCodedValueSet", type = { 1758 ValueSet.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1759 @Description(shortDefinition = "Value set of normal coded values for the observations conforming to this ObservationDefinition", formalDefinition = "The set of normal coded results for the observations conforming to this ObservationDefinition.") 1760 protected Reference normalCodedValueSet; 1761 1762 /** 1763 * The actual object that is the target of the reference (The set of normal 1764 * coded results for the observations conforming to this ObservationDefinition.) 1765 */ 1766 protected ValueSet normalCodedValueSetTarget; 1767 1768 /** 1769 * The set of abnormal coded results for the observation conforming to this 1770 * ObservationDefinition. 1771 */ 1772 @Child(name = "abnormalCodedValueSet", type = { 1773 ValueSet.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1774 @Description(shortDefinition = "Value set of abnormal coded values for the observations conforming to this ObservationDefinition", formalDefinition = "The set of abnormal coded results for the observation conforming to this ObservationDefinition.") 1775 protected Reference abnormalCodedValueSet; 1776 1777 /** 1778 * The actual object that is the target of the reference (The set of abnormal 1779 * coded results for the observation conforming to this ObservationDefinition.) 1780 */ 1781 protected ValueSet abnormalCodedValueSetTarget; 1782 1783 /** 1784 * The set of critical coded results for the observation conforming to this 1785 * ObservationDefinition. 1786 */ 1787 @Child(name = "criticalCodedValueSet", type = { 1788 ValueSet.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1789 @Description(shortDefinition = "Value set of critical coded values for the observations conforming to this ObservationDefinition", formalDefinition = "The set of critical coded results for the observation conforming to this ObservationDefinition.") 1790 protected Reference criticalCodedValueSet; 1791 1792 /** 1793 * The actual object that is the target of the reference (The set of critical 1794 * coded results for the observation conforming to this ObservationDefinition.) 1795 */ 1796 protected ValueSet criticalCodedValueSetTarget; 1797 1798 private static final long serialVersionUID = 2136752757L; 1799 1800 /** 1801 * Constructor 1802 */ 1803 public ObservationDefinition() { 1804 super(); 1805 } 1806 1807 /** 1808 * Constructor 1809 */ 1810 public ObservationDefinition(CodeableConcept code) { 1811 super(); 1812 this.code = code; 1813 } 1814 1815 /** 1816 * @return {@link #category} (A code that classifies the general type of 1817 * observation.) 1818 */ 1819 public List<CodeableConcept> getCategory() { 1820 if (this.category == null) 1821 this.category = new ArrayList<CodeableConcept>(); 1822 return this.category; 1823 } 1824 1825 /** 1826 * @return Returns a reference to <code>this</code> for easy method chaining 1827 */ 1828 public ObservationDefinition setCategory(List<CodeableConcept> theCategory) { 1829 this.category = theCategory; 1830 return this; 1831 } 1832 1833 public boolean hasCategory() { 1834 if (this.category == null) 1835 return false; 1836 for (CodeableConcept item : this.category) 1837 if (!item.isEmpty()) 1838 return true; 1839 return false; 1840 } 1841 1842 public CodeableConcept addCategory() { // 3 1843 CodeableConcept t = new CodeableConcept(); 1844 if (this.category == null) 1845 this.category = new ArrayList<CodeableConcept>(); 1846 this.category.add(t); 1847 return t; 1848 } 1849 1850 public ObservationDefinition addCategory(CodeableConcept t) { // 3 1851 if (t == null) 1852 return this; 1853 if (this.category == null) 1854 this.category = new ArrayList<CodeableConcept>(); 1855 this.category.add(t); 1856 return this; 1857 } 1858 1859 /** 1860 * @return The first repetition of repeating field {@link #category}, creating 1861 * it if it does not already exist 1862 */ 1863 public CodeableConcept getCategoryFirstRep() { 1864 if (getCategory().isEmpty()) { 1865 addCategory(); 1866 } 1867 return getCategory().get(0); 1868 } 1869 1870 /** 1871 * @return {@link #code} (Describes what will be observed. Sometimes this is 1872 * called the observation "name".) 1873 */ 1874 public CodeableConcept getCode() { 1875 if (this.code == null) 1876 if (Configuration.errorOnAutoCreate()) 1877 throw new Error("Attempt to auto-create ObservationDefinition.code"); 1878 else if (Configuration.doAutoCreate()) 1879 this.code = new CodeableConcept(); // cc 1880 return this.code; 1881 } 1882 1883 public boolean hasCode() { 1884 return this.code != null && !this.code.isEmpty(); 1885 } 1886 1887 /** 1888 * @param value {@link #code} (Describes what will be observed. Sometimes this 1889 * is called the observation "name".) 1890 */ 1891 public ObservationDefinition setCode(CodeableConcept value) { 1892 this.code = value; 1893 return this; 1894 } 1895 1896 /** 1897 * @return {@link #identifier} (A unique identifier assigned to this 1898 * ObservationDefinition artifact.) 1899 */ 1900 public List<Identifier> getIdentifier() { 1901 if (this.identifier == null) 1902 this.identifier = new ArrayList<Identifier>(); 1903 return this.identifier; 1904 } 1905 1906 /** 1907 * @return Returns a reference to <code>this</code> for easy method chaining 1908 */ 1909 public ObservationDefinition setIdentifier(List<Identifier> theIdentifier) { 1910 this.identifier = theIdentifier; 1911 return this; 1912 } 1913 1914 public boolean hasIdentifier() { 1915 if (this.identifier == null) 1916 return false; 1917 for (Identifier item : this.identifier) 1918 if (!item.isEmpty()) 1919 return true; 1920 return false; 1921 } 1922 1923 public Identifier addIdentifier() { // 3 1924 Identifier t = new Identifier(); 1925 if (this.identifier == null) 1926 this.identifier = new ArrayList<Identifier>(); 1927 this.identifier.add(t); 1928 return t; 1929 } 1930 1931 public ObservationDefinition addIdentifier(Identifier t) { // 3 1932 if (t == null) 1933 return this; 1934 if (this.identifier == null) 1935 this.identifier = new ArrayList<Identifier>(); 1936 this.identifier.add(t); 1937 return this; 1938 } 1939 1940 /** 1941 * @return The first repetition of repeating field {@link #identifier}, creating 1942 * it if it does not already exist 1943 */ 1944 public Identifier getIdentifierFirstRep() { 1945 if (getIdentifier().isEmpty()) { 1946 addIdentifier(); 1947 } 1948 return getIdentifier().get(0); 1949 } 1950 1951 /** 1952 * @return {@link #permittedDataType} (The data types allowed for the value 1953 * element of the instance observations conforming to this 1954 * ObservationDefinition.) 1955 */ 1956 public List<Enumeration<ObservationDataType>> getPermittedDataType() { 1957 if (this.permittedDataType == null) 1958 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1959 return this.permittedDataType; 1960 } 1961 1962 /** 1963 * @return Returns a reference to <code>this</code> for easy method chaining 1964 */ 1965 public ObservationDefinition setPermittedDataType(List<Enumeration<ObservationDataType>> thePermittedDataType) { 1966 this.permittedDataType = thePermittedDataType; 1967 return this; 1968 } 1969 1970 public boolean hasPermittedDataType() { 1971 if (this.permittedDataType == null) 1972 return false; 1973 for (Enumeration<ObservationDataType> item : this.permittedDataType) 1974 if (!item.isEmpty()) 1975 return true; 1976 return false; 1977 } 1978 1979 /** 1980 * @return {@link #permittedDataType} (The data types allowed for the value 1981 * element of the instance observations conforming to this 1982 * ObservationDefinition.) 1983 */ 1984 public Enumeration<ObservationDataType> addPermittedDataTypeElement() {// 2 1985 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 1986 if (this.permittedDataType == null) 1987 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 1988 this.permittedDataType.add(t); 1989 return t; 1990 } 1991 1992 /** 1993 * @param value {@link #permittedDataType} (The data types allowed for the value 1994 * element of the instance observations conforming to this 1995 * ObservationDefinition.) 1996 */ 1997 public ObservationDefinition addPermittedDataType(ObservationDataType value) { // 1 1998 Enumeration<ObservationDataType> t = new Enumeration<ObservationDataType>(new ObservationDataTypeEnumFactory()); 1999 t.setValue(value); 2000 if (this.permittedDataType == null) 2001 this.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 2002 this.permittedDataType.add(t); 2003 return this; 2004 } 2005 2006 /** 2007 * @param value {@link #permittedDataType} (The data types allowed for the value 2008 * element of the instance observations conforming to this 2009 * ObservationDefinition.) 2010 */ 2011 public boolean hasPermittedDataType(ObservationDataType value) { 2012 if (this.permittedDataType == null) 2013 return false; 2014 for (Enumeration<ObservationDataType> v : this.permittedDataType) 2015 if (v.getValue().equals(value)) // code 2016 return true; 2017 return false; 2018 } 2019 2020 /** 2021 * @return {@link #multipleResultsAllowed} (Multiple results allowed for 2022 * observations conforming to this ObservationDefinition.). This is the 2023 * underlying object with id, value and extensions. The accessor 2024 * "getMultipleResultsAllowed" gives direct access to the value 2025 */ 2026 public BooleanType getMultipleResultsAllowedElement() { 2027 if (this.multipleResultsAllowed == null) 2028 if (Configuration.errorOnAutoCreate()) 2029 throw new Error("Attempt to auto-create ObservationDefinition.multipleResultsAllowed"); 2030 else if (Configuration.doAutoCreate()) 2031 this.multipleResultsAllowed = new BooleanType(); // bb 2032 return this.multipleResultsAllowed; 2033 } 2034 2035 public boolean hasMultipleResultsAllowedElement() { 2036 return this.multipleResultsAllowed != null && !this.multipleResultsAllowed.isEmpty(); 2037 } 2038 2039 public boolean hasMultipleResultsAllowed() { 2040 return this.multipleResultsAllowed != null && !this.multipleResultsAllowed.isEmpty(); 2041 } 2042 2043 /** 2044 * @param value {@link #multipleResultsAllowed} (Multiple results allowed for 2045 * observations conforming to this ObservationDefinition.). This is 2046 * the underlying object with id, value and extensions. The 2047 * accessor "getMultipleResultsAllowed" gives direct access to the 2048 * value 2049 */ 2050 public ObservationDefinition setMultipleResultsAllowedElement(BooleanType value) { 2051 this.multipleResultsAllowed = value; 2052 return this; 2053 } 2054 2055 /** 2056 * @return Multiple results allowed for observations conforming to this 2057 * ObservationDefinition. 2058 */ 2059 public boolean getMultipleResultsAllowed() { 2060 return this.multipleResultsAllowed == null || this.multipleResultsAllowed.isEmpty() ? false 2061 : this.multipleResultsAllowed.getValue(); 2062 } 2063 2064 /** 2065 * @param value Multiple results allowed for observations conforming to this 2066 * ObservationDefinition. 2067 */ 2068 public ObservationDefinition setMultipleResultsAllowed(boolean value) { 2069 if (this.multipleResultsAllowed == null) 2070 this.multipleResultsAllowed = new BooleanType(); 2071 this.multipleResultsAllowed.setValue(value); 2072 return this; 2073 } 2074 2075 /** 2076 * @return {@link #method} (The method or technique used to perform the 2077 * observation.) 2078 */ 2079 public CodeableConcept getMethod() { 2080 if (this.method == null) 2081 if (Configuration.errorOnAutoCreate()) 2082 throw new Error("Attempt to auto-create ObservationDefinition.method"); 2083 else if (Configuration.doAutoCreate()) 2084 this.method = new CodeableConcept(); // cc 2085 return this.method; 2086 } 2087 2088 public boolean hasMethod() { 2089 return this.method != null && !this.method.isEmpty(); 2090 } 2091 2092 /** 2093 * @param value {@link #method} (The method or technique used to perform the 2094 * observation.) 2095 */ 2096 public ObservationDefinition setMethod(CodeableConcept value) { 2097 this.method = value; 2098 return this; 2099 } 2100 2101 /** 2102 * @return {@link #preferredReportName} (The preferred name to be used when 2103 * reporting the results of observations conforming to this 2104 * ObservationDefinition.). This is the underlying object with id, value 2105 * and extensions. The accessor "getPreferredReportName" gives direct 2106 * access to the value 2107 */ 2108 public StringType getPreferredReportNameElement() { 2109 if (this.preferredReportName == null) 2110 if (Configuration.errorOnAutoCreate()) 2111 throw new Error("Attempt to auto-create ObservationDefinition.preferredReportName"); 2112 else if (Configuration.doAutoCreate()) 2113 this.preferredReportName = new StringType(); // bb 2114 return this.preferredReportName; 2115 } 2116 2117 public boolean hasPreferredReportNameElement() { 2118 return this.preferredReportName != null && !this.preferredReportName.isEmpty(); 2119 } 2120 2121 public boolean hasPreferredReportName() { 2122 return this.preferredReportName != null && !this.preferredReportName.isEmpty(); 2123 } 2124 2125 /** 2126 * @param value {@link #preferredReportName} (The preferred name to be used when 2127 * reporting the results of observations conforming to this 2128 * ObservationDefinition.). This is the underlying object with id, 2129 * value and extensions. The accessor "getPreferredReportName" 2130 * gives direct access to the value 2131 */ 2132 public ObservationDefinition setPreferredReportNameElement(StringType value) { 2133 this.preferredReportName = value; 2134 return this; 2135 } 2136 2137 /** 2138 * @return The preferred name to be used when reporting the results of 2139 * observations conforming to this ObservationDefinition. 2140 */ 2141 public String getPreferredReportName() { 2142 return this.preferredReportName == null ? null : this.preferredReportName.getValue(); 2143 } 2144 2145 /** 2146 * @param value The preferred name to be used when reporting the results of 2147 * observations conforming to this ObservationDefinition. 2148 */ 2149 public ObservationDefinition setPreferredReportName(String value) { 2150 if (Utilities.noString(value)) 2151 this.preferredReportName = null; 2152 else { 2153 if (this.preferredReportName == null) 2154 this.preferredReportName = new StringType(); 2155 this.preferredReportName.setValue(value); 2156 } 2157 return this; 2158 } 2159 2160 /** 2161 * @return {@link #quantitativeDetails} (Characteristics for quantitative 2162 * results of this observation.) 2163 */ 2164 public ObservationDefinitionQuantitativeDetailsComponent getQuantitativeDetails() { 2165 if (this.quantitativeDetails == null) 2166 if (Configuration.errorOnAutoCreate()) 2167 throw new Error("Attempt to auto-create ObservationDefinition.quantitativeDetails"); 2168 else if (Configuration.doAutoCreate()) 2169 this.quantitativeDetails = new ObservationDefinitionQuantitativeDetailsComponent(); // cc 2170 return this.quantitativeDetails; 2171 } 2172 2173 public boolean hasQuantitativeDetails() { 2174 return this.quantitativeDetails != null && !this.quantitativeDetails.isEmpty(); 2175 } 2176 2177 /** 2178 * @param value {@link #quantitativeDetails} (Characteristics for quantitative 2179 * results of this observation.) 2180 */ 2181 public ObservationDefinition setQuantitativeDetails(ObservationDefinitionQuantitativeDetailsComponent value) { 2182 this.quantitativeDetails = value; 2183 return this; 2184 } 2185 2186 /** 2187 * @return {@link #qualifiedInterval} (Multiple ranges of results qualified by 2188 * different contexts for ordinal or continuous observations conforming 2189 * to this ObservationDefinition.) 2190 */ 2191 public List<ObservationDefinitionQualifiedIntervalComponent> getQualifiedInterval() { 2192 if (this.qualifiedInterval == null) 2193 this.qualifiedInterval = new ArrayList<ObservationDefinitionQualifiedIntervalComponent>(); 2194 return this.qualifiedInterval; 2195 } 2196 2197 /** 2198 * @return Returns a reference to <code>this</code> for easy method chaining 2199 */ 2200 public ObservationDefinition setQualifiedInterval( 2201 List<ObservationDefinitionQualifiedIntervalComponent> theQualifiedInterval) { 2202 this.qualifiedInterval = theQualifiedInterval; 2203 return this; 2204 } 2205 2206 public boolean hasQualifiedInterval() { 2207 if (this.qualifiedInterval == null) 2208 return false; 2209 for (ObservationDefinitionQualifiedIntervalComponent item : this.qualifiedInterval) 2210 if (!item.isEmpty()) 2211 return true; 2212 return false; 2213 } 2214 2215 public ObservationDefinitionQualifiedIntervalComponent addQualifiedInterval() { // 3 2216 ObservationDefinitionQualifiedIntervalComponent t = new ObservationDefinitionQualifiedIntervalComponent(); 2217 if (this.qualifiedInterval == null) 2218 this.qualifiedInterval = new ArrayList<ObservationDefinitionQualifiedIntervalComponent>(); 2219 this.qualifiedInterval.add(t); 2220 return t; 2221 } 2222 2223 public ObservationDefinition addQualifiedInterval(ObservationDefinitionQualifiedIntervalComponent t) { // 3 2224 if (t == null) 2225 return this; 2226 if (this.qualifiedInterval == null) 2227 this.qualifiedInterval = new ArrayList<ObservationDefinitionQualifiedIntervalComponent>(); 2228 this.qualifiedInterval.add(t); 2229 return this; 2230 } 2231 2232 /** 2233 * @return The first repetition of repeating field {@link #qualifiedInterval}, 2234 * creating it if it does not already exist 2235 */ 2236 public ObservationDefinitionQualifiedIntervalComponent getQualifiedIntervalFirstRep() { 2237 if (getQualifiedInterval().isEmpty()) { 2238 addQualifiedInterval(); 2239 } 2240 return getQualifiedInterval().get(0); 2241 } 2242 2243 /** 2244 * @return {@link #validCodedValueSet} (The set of valid coded results for the 2245 * observations conforming to this ObservationDefinition.) 2246 */ 2247 public Reference getValidCodedValueSet() { 2248 if (this.validCodedValueSet == null) 2249 if (Configuration.errorOnAutoCreate()) 2250 throw new Error("Attempt to auto-create ObservationDefinition.validCodedValueSet"); 2251 else if (Configuration.doAutoCreate()) 2252 this.validCodedValueSet = new Reference(); // cc 2253 return this.validCodedValueSet; 2254 } 2255 2256 public boolean hasValidCodedValueSet() { 2257 return this.validCodedValueSet != null && !this.validCodedValueSet.isEmpty(); 2258 } 2259 2260 /** 2261 * @param value {@link #validCodedValueSet} (The set of valid coded results for 2262 * the observations conforming to this ObservationDefinition.) 2263 */ 2264 public ObservationDefinition setValidCodedValueSet(Reference value) { 2265 this.validCodedValueSet = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return {@link #validCodedValueSet} The actual object that is the target of 2271 * the reference. The reference library doesn't populate this, but you 2272 * can use it to hold the resource if you resolve it. (The set of valid 2273 * coded results for the observations conforming to this 2274 * ObservationDefinition.) 2275 */ 2276 public ValueSet getValidCodedValueSetTarget() { 2277 if (this.validCodedValueSetTarget == null) 2278 if (Configuration.errorOnAutoCreate()) 2279 throw new Error("Attempt to auto-create ObservationDefinition.validCodedValueSet"); 2280 else if (Configuration.doAutoCreate()) 2281 this.validCodedValueSetTarget = new ValueSet(); // aa 2282 return this.validCodedValueSetTarget; 2283 } 2284 2285 /** 2286 * @param value {@link #validCodedValueSet} The actual object that is the target 2287 * of the reference. The reference library doesn't use these, but 2288 * you can use it to hold the resource if you resolve it. (The set 2289 * of valid coded results for the observations conforming to this 2290 * ObservationDefinition.) 2291 */ 2292 public ObservationDefinition setValidCodedValueSetTarget(ValueSet value) { 2293 this.validCodedValueSetTarget = value; 2294 return this; 2295 } 2296 2297 /** 2298 * @return {@link #normalCodedValueSet} (The set of normal coded results for the 2299 * observations conforming to this ObservationDefinition.) 2300 */ 2301 public Reference getNormalCodedValueSet() { 2302 if (this.normalCodedValueSet == null) 2303 if (Configuration.errorOnAutoCreate()) 2304 throw new Error("Attempt to auto-create ObservationDefinition.normalCodedValueSet"); 2305 else if (Configuration.doAutoCreate()) 2306 this.normalCodedValueSet = new Reference(); // cc 2307 return this.normalCodedValueSet; 2308 } 2309 2310 public boolean hasNormalCodedValueSet() { 2311 return this.normalCodedValueSet != null && !this.normalCodedValueSet.isEmpty(); 2312 } 2313 2314 /** 2315 * @param value {@link #normalCodedValueSet} (The set of normal coded results 2316 * for the observations conforming to this ObservationDefinition.) 2317 */ 2318 public ObservationDefinition setNormalCodedValueSet(Reference value) { 2319 this.normalCodedValueSet = value; 2320 return this; 2321 } 2322 2323 /** 2324 * @return {@link #normalCodedValueSet} The actual object that is the target of 2325 * the reference. The reference library doesn't populate this, but you 2326 * can use it to hold the resource if you resolve it. (The set of normal 2327 * coded results for the observations conforming to this 2328 * ObservationDefinition.) 2329 */ 2330 public ValueSet getNormalCodedValueSetTarget() { 2331 if (this.normalCodedValueSetTarget == null) 2332 if (Configuration.errorOnAutoCreate()) 2333 throw new Error("Attempt to auto-create ObservationDefinition.normalCodedValueSet"); 2334 else if (Configuration.doAutoCreate()) 2335 this.normalCodedValueSetTarget = new ValueSet(); // aa 2336 return this.normalCodedValueSetTarget; 2337 } 2338 2339 /** 2340 * @param value {@link #normalCodedValueSet} The actual object that is the 2341 * target of the reference. The reference library doesn't use 2342 * these, but you can use it to hold the resource if you resolve 2343 * it. (The set of normal coded results for the observations 2344 * conforming to this ObservationDefinition.) 2345 */ 2346 public ObservationDefinition setNormalCodedValueSetTarget(ValueSet value) { 2347 this.normalCodedValueSetTarget = value; 2348 return this; 2349 } 2350 2351 /** 2352 * @return {@link #abnormalCodedValueSet} (The set of abnormal coded results for 2353 * the observation conforming to this ObservationDefinition.) 2354 */ 2355 public Reference getAbnormalCodedValueSet() { 2356 if (this.abnormalCodedValueSet == null) 2357 if (Configuration.errorOnAutoCreate()) 2358 throw new Error("Attempt to auto-create ObservationDefinition.abnormalCodedValueSet"); 2359 else if (Configuration.doAutoCreate()) 2360 this.abnormalCodedValueSet = new Reference(); // cc 2361 return this.abnormalCodedValueSet; 2362 } 2363 2364 public boolean hasAbnormalCodedValueSet() { 2365 return this.abnormalCodedValueSet != null && !this.abnormalCodedValueSet.isEmpty(); 2366 } 2367 2368 /** 2369 * @param value {@link #abnormalCodedValueSet} (The set of abnormal coded 2370 * results for the observation conforming to this 2371 * ObservationDefinition.) 2372 */ 2373 public ObservationDefinition setAbnormalCodedValueSet(Reference value) { 2374 this.abnormalCodedValueSet = value; 2375 return this; 2376 } 2377 2378 /** 2379 * @return {@link #abnormalCodedValueSet} The actual object that is the target 2380 * of the reference. The reference library doesn't populate this, but 2381 * you can use it to hold the resource if you resolve it. (The set of 2382 * abnormal coded results for the observation conforming to this 2383 * ObservationDefinition.) 2384 */ 2385 public ValueSet getAbnormalCodedValueSetTarget() { 2386 if (this.abnormalCodedValueSetTarget == null) 2387 if (Configuration.errorOnAutoCreate()) 2388 throw new Error("Attempt to auto-create ObservationDefinition.abnormalCodedValueSet"); 2389 else if (Configuration.doAutoCreate()) 2390 this.abnormalCodedValueSetTarget = new ValueSet(); // aa 2391 return this.abnormalCodedValueSetTarget; 2392 } 2393 2394 /** 2395 * @param value {@link #abnormalCodedValueSet} The actual object that is the 2396 * target of the reference. The reference library doesn't use 2397 * these, but you can use it to hold the resource if you resolve 2398 * it. (The set of abnormal coded results for the observation 2399 * conforming to this ObservationDefinition.) 2400 */ 2401 public ObservationDefinition setAbnormalCodedValueSetTarget(ValueSet value) { 2402 this.abnormalCodedValueSetTarget = value; 2403 return this; 2404 } 2405 2406 /** 2407 * @return {@link #criticalCodedValueSet} (The set of critical coded results for 2408 * the observation conforming to this ObservationDefinition.) 2409 */ 2410 public Reference getCriticalCodedValueSet() { 2411 if (this.criticalCodedValueSet == null) 2412 if (Configuration.errorOnAutoCreate()) 2413 throw new Error("Attempt to auto-create ObservationDefinition.criticalCodedValueSet"); 2414 else if (Configuration.doAutoCreate()) 2415 this.criticalCodedValueSet = new Reference(); // cc 2416 return this.criticalCodedValueSet; 2417 } 2418 2419 public boolean hasCriticalCodedValueSet() { 2420 return this.criticalCodedValueSet != null && !this.criticalCodedValueSet.isEmpty(); 2421 } 2422 2423 /** 2424 * @param value {@link #criticalCodedValueSet} (The set of critical coded 2425 * results for the observation conforming to this 2426 * ObservationDefinition.) 2427 */ 2428 public ObservationDefinition setCriticalCodedValueSet(Reference value) { 2429 this.criticalCodedValueSet = value; 2430 return this; 2431 } 2432 2433 /** 2434 * @return {@link #criticalCodedValueSet} The actual object that is the target 2435 * of the reference. The reference library doesn't populate this, but 2436 * you can use it to hold the resource if you resolve it. (The set of 2437 * critical coded results for the observation conforming to this 2438 * ObservationDefinition.) 2439 */ 2440 public ValueSet getCriticalCodedValueSetTarget() { 2441 if (this.criticalCodedValueSetTarget == null) 2442 if (Configuration.errorOnAutoCreate()) 2443 throw new Error("Attempt to auto-create ObservationDefinition.criticalCodedValueSet"); 2444 else if (Configuration.doAutoCreate()) 2445 this.criticalCodedValueSetTarget = new ValueSet(); // aa 2446 return this.criticalCodedValueSetTarget; 2447 } 2448 2449 /** 2450 * @param value {@link #criticalCodedValueSet} The actual object that is the 2451 * target of the reference. The reference library doesn't use 2452 * these, but you can use it to hold the resource if you resolve 2453 * it. (The set of critical coded results for the observation 2454 * conforming to this ObservationDefinition.) 2455 */ 2456 public ObservationDefinition setCriticalCodedValueSetTarget(ValueSet value) { 2457 this.criticalCodedValueSetTarget = value; 2458 return this; 2459 } 2460 2461 protected void listChildren(List<Property> children) { 2462 super.listChildren(children); 2463 children.add(new Property("category", "CodeableConcept", "A code that classifies the general type of observation.", 2464 0, java.lang.Integer.MAX_VALUE, category)); 2465 children.add(new Property("code", "CodeableConcept", 2466 "Describes what will be observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 2467 children.add( 2468 new Property("identifier", "Identifier", "A unique identifier assigned to this ObservationDefinition artifact.", 2469 0, java.lang.Integer.MAX_VALUE, identifier)); 2470 children.add(new Property("permittedDataType", "code", 2471 "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.", 2472 0, java.lang.Integer.MAX_VALUE, permittedDataType)); 2473 children.add(new Property("multipleResultsAllowed", "boolean", 2474 "Multiple results allowed for observations conforming to this ObservationDefinition.", 0, 1, 2475 multipleResultsAllowed)); 2476 children.add(new Property("method", "CodeableConcept", "The method or technique used to perform the observation.", 2477 0, 1, method)); 2478 children.add(new Property("preferredReportName", "string", 2479 "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.", 2480 0, 1, preferredReportName)); 2481 children.add(new Property("quantitativeDetails", "", 2482 "Characteristics for quantitative results of this observation.", 0, 1, quantitativeDetails)); 2483 children.add(new Property("qualifiedInterval", "", 2484 "Multiple ranges of results qualified by different contexts for ordinal or continuous observations conforming to this ObservationDefinition.", 2485 0, java.lang.Integer.MAX_VALUE, qualifiedInterval)); 2486 children.add(new Property("validCodedValueSet", "Reference(ValueSet)", 2487 "The set of valid coded results for the observations conforming to this ObservationDefinition.", 0, 1, 2488 validCodedValueSet)); 2489 children.add(new Property("normalCodedValueSet", "Reference(ValueSet)", 2490 "The set of normal coded results for the observations conforming to this ObservationDefinition.", 0, 1, 2491 normalCodedValueSet)); 2492 children.add(new Property("abnormalCodedValueSet", "Reference(ValueSet)", 2493 "The set of abnormal coded results for the observation conforming to this ObservationDefinition.", 0, 1, 2494 abnormalCodedValueSet)); 2495 children.add(new Property("criticalCodedValueSet", "Reference(ValueSet)", 2496 "The set of critical coded results for the observation conforming to this ObservationDefinition.", 0, 1, 2497 criticalCodedValueSet)); 2498 } 2499 2500 @Override 2501 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2502 switch (_hash) { 2503 case 50511102: 2504 /* category */ return new Property("category", "CodeableConcept", 2505 "A code that classifies the general type of observation.", 0, java.lang.Integer.MAX_VALUE, category); 2506 case 3059181: 2507 /* code */ return new Property("code", "CodeableConcept", 2508 "Describes what will be observed. Sometimes this is called the observation \"name\".", 0, 1, code); 2509 case -1618432855: 2510 /* identifier */ return new Property("identifier", "Identifier", 2511 "A unique identifier assigned to this ObservationDefinition artifact.", 0, java.lang.Integer.MAX_VALUE, 2512 identifier); 2513 case -99492804: 2514 /* permittedDataType */ return new Property("permittedDataType", "code", 2515 "The data types allowed for the value element of the instance observations conforming to this ObservationDefinition.", 2516 0, java.lang.Integer.MAX_VALUE, permittedDataType); 2517 case -2102414590: 2518 /* multipleResultsAllowed */ return new Property("multipleResultsAllowed", "boolean", 2519 "Multiple results allowed for observations conforming to this ObservationDefinition.", 0, 1, 2520 multipleResultsAllowed); 2521 case -1077554975: 2522 /* method */ return new Property("method", "CodeableConcept", 2523 "The method or technique used to perform the observation.", 0, 1, method); 2524 case -1851030208: 2525 /* preferredReportName */ return new Property("preferredReportName", "string", 2526 "The preferred name to be used when reporting the results of observations conforming to this ObservationDefinition.", 2527 0, 1, preferredReportName); 2528 case 842150763: 2529 /* quantitativeDetails */ return new Property("quantitativeDetails", "", 2530 "Characteristics for quantitative results of this observation.", 0, 1, quantitativeDetails); 2531 case 1882971521: 2532 /* qualifiedInterval */ return new Property("qualifiedInterval", "", 2533 "Multiple ranges of results qualified by different contexts for ordinal or continuous observations conforming to this ObservationDefinition.", 2534 0, java.lang.Integer.MAX_VALUE, qualifiedInterval); 2535 case 1374640076: 2536 /* validCodedValueSet */ return new Property("validCodedValueSet", "Reference(ValueSet)", 2537 "The set of valid coded results for the observations conforming to this ObservationDefinition.", 0, 1, 2538 validCodedValueSet); 2539 case -837500735: 2540 /* normalCodedValueSet */ return new Property("normalCodedValueSet", "Reference(ValueSet)", 2541 "The set of normal coded results for the observations conforming to this ObservationDefinition.", 0, 1, 2542 normalCodedValueSet); 2543 case 1073600256: 2544 /* abnormalCodedValueSet */ return new Property("abnormalCodedValueSet", "Reference(ValueSet)", 2545 "The set of abnormal coded results for the observation conforming to this ObservationDefinition.", 0, 1, 2546 abnormalCodedValueSet); 2547 case 2568457: 2548 /* criticalCodedValueSet */ return new Property("criticalCodedValueSet", "Reference(ValueSet)", 2549 "The set of critical coded results for the observation conforming to this ObservationDefinition.", 0, 1, 2550 criticalCodedValueSet); 2551 default: 2552 return super.getNamedProperty(_hash, _name, _checkValid); 2553 } 2554 2555 } 2556 2557 @Override 2558 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2559 switch (hash) { 2560 case 50511102: 2561 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2562 case 3059181: 2563 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2564 case -1618432855: 2565 /* identifier */ return this.identifier == null ? new Base[0] 2566 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2567 case -99492804: 2568 /* permittedDataType */ return this.permittedDataType == null ? new Base[0] 2569 : this.permittedDataType.toArray(new Base[this.permittedDataType.size()]); // Enumeration<ObservationDataType> 2570 case -2102414590: 2571 /* multipleResultsAllowed */ return this.multipleResultsAllowed == null ? new Base[0] 2572 : new Base[] { this.multipleResultsAllowed }; // BooleanType 2573 case -1077554975: 2574 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 2575 case -1851030208: 2576 /* preferredReportName */ return this.preferredReportName == null ? new Base[0] 2577 : new Base[] { this.preferredReportName }; // StringType 2578 case 842150763: 2579 /* quantitativeDetails */ return this.quantitativeDetails == null ? new Base[0] 2580 : new Base[] { this.quantitativeDetails }; // ObservationDefinitionQuantitativeDetailsComponent 2581 case 1882971521: 2582 /* qualifiedInterval */ return this.qualifiedInterval == null ? new Base[0] 2583 : this.qualifiedInterval.toArray(new Base[this.qualifiedInterval.size()]); // ObservationDefinitionQualifiedIntervalComponent 2584 case 1374640076: 2585 /* validCodedValueSet */ return this.validCodedValueSet == null ? new Base[0] 2586 : new Base[] { this.validCodedValueSet }; // Reference 2587 case -837500735: 2588 /* normalCodedValueSet */ return this.normalCodedValueSet == null ? new Base[0] 2589 : new Base[] { this.normalCodedValueSet }; // Reference 2590 case 1073600256: 2591 /* abnormalCodedValueSet */ return this.abnormalCodedValueSet == null ? new Base[0] 2592 : new Base[] { this.abnormalCodedValueSet }; // Reference 2593 case 2568457: 2594 /* criticalCodedValueSet */ return this.criticalCodedValueSet == null ? new Base[0] 2595 : new Base[] { this.criticalCodedValueSet }; // Reference 2596 default: 2597 return super.getProperty(hash, name, checkValid); 2598 } 2599 2600 } 2601 2602 @Override 2603 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2604 switch (hash) { 2605 case 50511102: // category 2606 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2607 return value; 2608 case 3059181: // code 2609 this.code = castToCodeableConcept(value); // CodeableConcept 2610 return value; 2611 case -1618432855: // identifier 2612 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2613 return value; 2614 case -99492804: // permittedDataType 2615 value = new ObservationDataTypeEnumFactory().fromType(castToCode(value)); 2616 this.getPermittedDataType().add((Enumeration) value); // Enumeration<ObservationDataType> 2617 return value; 2618 case -2102414590: // multipleResultsAllowed 2619 this.multipleResultsAllowed = castToBoolean(value); // BooleanType 2620 return value; 2621 case -1077554975: // method 2622 this.method = castToCodeableConcept(value); // CodeableConcept 2623 return value; 2624 case -1851030208: // preferredReportName 2625 this.preferredReportName = castToString(value); // StringType 2626 return value; 2627 case 842150763: // quantitativeDetails 2628 this.quantitativeDetails = (ObservationDefinitionQuantitativeDetailsComponent) value; // ObservationDefinitionQuantitativeDetailsComponent 2629 return value; 2630 case 1882971521: // qualifiedInterval 2631 this.getQualifiedInterval().add((ObservationDefinitionQualifiedIntervalComponent) value); // ObservationDefinitionQualifiedIntervalComponent 2632 return value; 2633 case 1374640076: // validCodedValueSet 2634 this.validCodedValueSet = castToReference(value); // Reference 2635 return value; 2636 case -837500735: // normalCodedValueSet 2637 this.normalCodedValueSet = castToReference(value); // Reference 2638 return value; 2639 case 1073600256: // abnormalCodedValueSet 2640 this.abnormalCodedValueSet = castToReference(value); // Reference 2641 return value; 2642 case 2568457: // criticalCodedValueSet 2643 this.criticalCodedValueSet = castToReference(value); // Reference 2644 return value; 2645 default: 2646 return super.setProperty(hash, name, value); 2647 } 2648 2649 } 2650 2651 @Override 2652 public Base setProperty(String name, Base value) throws FHIRException { 2653 if (name.equals("category")) { 2654 this.getCategory().add(castToCodeableConcept(value)); 2655 } else if (name.equals("code")) { 2656 this.code = castToCodeableConcept(value); // CodeableConcept 2657 } else if (name.equals("identifier")) { 2658 this.getIdentifier().add(castToIdentifier(value)); 2659 } else if (name.equals("permittedDataType")) { 2660 value = new ObservationDataTypeEnumFactory().fromType(castToCode(value)); 2661 this.getPermittedDataType().add((Enumeration) value); 2662 } else if (name.equals("multipleResultsAllowed")) { 2663 this.multipleResultsAllowed = castToBoolean(value); // BooleanType 2664 } else if (name.equals("method")) { 2665 this.method = castToCodeableConcept(value); // CodeableConcept 2666 } else if (name.equals("preferredReportName")) { 2667 this.preferredReportName = castToString(value); // StringType 2668 } else if (name.equals("quantitativeDetails")) { 2669 this.quantitativeDetails = (ObservationDefinitionQuantitativeDetailsComponent) value; // ObservationDefinitionQuantitativeDetailsComponent 2670 } else if (name.equals("qualifiedInterval")) { 2671 this.getQualifiedInterval().add((ObservationDefinitionQualifiedIntervalComponent) value); 2672 } else if (name.equals("validCodedValueSet")) { 2673 this.validCodedValueSet = castToReference(value); // Reference 2674 } else if (name.equals("normalCodedValueSet")) { 2675 this.normalCodedValueSet = castToReference(value); // Reference 2676 } else if (name.equals("abnormalCodedValueSet")) { 2677 this.abnormalCodedValueSet = castToReference(value); // Reference 2678 } else if (name.equals("criticalCodedValueSet")) { 2679 this.criticalCodedValueSet = castToReference(value); // Reference 2680 } else 2681 return super.setProperty(name, value); 2682 return value; 2683 } 2684 2685 @Override 2686 public void removeChild(String name, Base value) throws FHIRException { 2687 if (name.equals("category")) { 2688 this.getCategory().remove(castToCodeableConcept(value)); 2689 } else if (name.equals("code")) { 2690 this.code = null; 2691 } else if (name.equals("identifier")) { 2692 this.getIdentifier().remove(castToIdentifier(value)); 2693 } else if (name.equals("permittedDataType")) { 2694 this.getPermittedDataType().remove((Enumeration) value); 2695 } else if (name.equals("multipleResultsAllowed")) { 2696 this.multipleResultsAllowed = null; 2697 } else if (name.equals("method")) { 2698 this.method = null; 2699 } else if (name.equals("preferredReportName")) { 2700 this.preferredReportName = null; 2701 } else if (name.equals("quantitativeDetails")) { 2702 this.quantitativeDetails = (ObservationDefinitionQuantitativeDetailsComponent) value; // ObservationDefinitionQuantitativeDetailsComponent 2703 } else if (name.equals("qualifiedInterval")) { 2704 this.getQualifiedInterval().remove((ObservationDefinitionQualifiedIntervalComponent) value); 2705 } else if (name.equals("validCodedValueSet")) { 2706 this.validCodedValueSet = null; 2707 } else if (name.equals("normalCodedValueSet")) { 2708 this.normalCodedValueSet = null; 2709 } else if (name.equals("abnormalCodedValueSet")) { 2710 this.abnormalCodedValueSet = null; 2711 } else if (name.equals("criticalCodedValueSet")) { 2712 this.criticalCodedValueSet = null; 2713 } else 2714 super.removeChild(name, value); 2715 2716 } 2717 2718 @Override 2719 public Base makeProperty(int hash, String name) throws FHIRException { 2720 switch (hash) { 2721 case 50511102: 2722 return addCategory(); 2723 case 3059181: 2724 return getCode(); 2725 case -1618432855: 2726 return addIdentifier(); 2727 case -99492804: 2728 return addPermittedDataTypeElement(); 2729 case -2102414590: 2730 return getMultipleResultsAllowedElement(); 2731 case -1077554975: 2732 return getMethod(); 2733 case -1851030208: 2734 return getPreferredReportNameElement(); 2735 case 842150763: 2736 return getQuantitativeDetails(); 2737 case 1882971521: 2738 return addQualifiedInterval(); 2739 case 1374640076: 2740 return getValidCodedValueSet(); 2741 case -837500735: 2742 return getNormalCodedValueSet(); 2743 case 1073600256: 2744 return getAbnormalCodedValueSet(); 2745 case 2568457: 2746 return getCriticalCodedValueSet(); 2747 default: 2748 return super.makeProperty(hash, name); 2749 } 2750 2751 } 2752 2753 @Override 2754 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2755 switch (hash) { 2756 case 50511102: 2757 /* category */ return new String[] { "CodeableConcept" }; 2758 case 3059181: 2759 /* code */ return new String[] { "CodeableConcept" }; 2760 case -1618432855: 2761 /* identifier */ return new String[] { "Identifier" }; 2762 case -99492804: 2763 /* permittedDataType */ return new String[] { "code" }; 2764 case -2102414590: 2765 /* multipleResultsAllowed */ return new String[] { "boolean" }; 2766 case -1077554975: 2767 /* method */ return new String[] { "CodeableConcept" }; 2768 case -1851030208: 2769 /* preferredReportName */ return new String[] { "string" }; 2770 case 842150763: 2771 /* quantitativeDetails */ return new String[] {}; 2772 case 1882971521: 2773 /* qualifiedInterval */ return new String[] {}; 2774 case 1374640076: 2775 /* validCodedValueSet */ return new String[] { "Reference" }; 2776 case -837500735: 2777 /* normalCodedValueSet */ return new String[] { "Reference" }; 2778 case 1073600256: 2779 /* abnormalCodedValueSet */ return new String[] { "Reference" }; 2780 case 2568457: 2781 /* criticalCodedValueSet */ return new String[] { "Reference" }; 2782 default: 2783 return super.getTypesForProperty(hash, name); 2784 } 2785 2786 } 2787 2788 @Override 2789 public Base addChild(String name) throws FHIRException { 2790 if (name.equals("category")) { 2791 return addCategory(); 2792 } else if (name.equals("code")) { 2793 this.code = new CodeableConcept(); 2794 return this.code; 2795 } else if (name.equals("identifier")) { 2796 return addIdentifier(); 2797 } else if (name.equals("permittedDataType")) { 2798 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.permittedDataType"); 2799 } else if (name.equals("multipleResultsAllowed")) { 2800 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.multipleResultsAllowed"); 2801 } else if (name.equals("method")) { 2802 this.method = new CodeableConcept(); 2803 return this.method; 2804 } else if (name.equals("preferredReportName")) { 2805 throw new FHIRException("Cannot call addChild on a singleton property ObservationDefinition.preferredReportName"); 2806 } else if (name.equals("quantitativeDetails")) { 2807 this.quantitativeDetails = new ObservationDefinitionQuantitativeDetailsComponent(); 2808 return this.quantitativeDetails; 2809 } else if (name.equals("qualifiedInterval")) { 2810 return addQualifiedInterval(); 2811 } else if (name.equals("validCodedValueSet")) { 2812 this.validCodedValueSet = new Reference(); 2813 return this.validCodedValueSet; 2814 } else if (name.equals("normalCodedValueSet")) { 2815 this.normalCodedValueSet = new Reference(); 2816 return this.normalCodedValueSet; 2817 } else if (name.equals("abnormalCodedValueSet")) { 2818 this.abnormalCodedValueSet = new Reference(); 2819 return this.abnormalCodedValueSet; 2820 } else if (name.equals("criticalCodedValueSet")) { 2821 this.criticalCodedValueSet = new Reference(); 2822 return this.criticalCodedValueSet; 2823 } else 2824 return super.addChild(name); 2825 } 2826 2827 public String fhirType() { 2828 return "ObservationDefinition"; 2829 2830 } 2831 2832 public ObservationDefinition copy() { 2833 ObservationDefinition dst = new ObservationDefinition(); 2834 copyValues(dst); 2835 return dst; 2836 } 2837 2838 public void copyValues(ObservationDefinition dst) { 2839 super.copyValues(dst); 2840 if (category != null) { 2841 dst.category = new ArrayList<CodeableConcept>(); 2842 for (CodeableConcept i : category) 2843 dst.category.add(i.copy()); 2844 } 2845 ; 2846 dst.code = code == null ? null : code.copy(); 2847 if (identifier != null) { 2848 dst.identifier = new ArrayList<Identifier>(); 2849 for (Identifier i : identifier) 2850 dst.identifier.add(i.copy()); 2851 } 2852 ; 2853 if (permittedDataType != null) { 2854 dst.permittedDataType = new ArrayList<Enumeration<ObservationDataType>>(); 2855 for (Enumeration<ObservationDataType> i : permittedDataType) 2856 dst.permittedDataType.add(i.copy()); 2857 } 2858 ; 2859 dst.multipleResultsAllowed = multipleResultsAllowed == null ? null : multipleResultsAllowed.copy(); 2860 dst.method = method == null ? null : method.copy(); 2861 dst.preferredReportName = preferredReportName == null ? null : preferredReportName.copy(); 2862 dst.quantitativeDetails = quantitativeDetails == null ? null : quantitativeDetails.copy(); 2863 if (qualifiedInterval != null) { 2864 dst.qualifiedInterval = new ArrayList<ObservationDefinitionQualifiedIntervalComponent>(); 2865 for (ObservationDefinitionQualifiedIntervalComponent i : qualifiedInterval) 2866 dst.qualifiedInterval.add(i.copy()); 2867 } 2868 ; 2869 dst.validCodedValueSet = validCodedValueSet == null ? null : validCodedValueSet.copy(); 2870 dst.normalCodedValueSet = normalCodedValueSet == null ? null : normalCodedValueSet.copy(); 2871 dst.abnormalCodedValueSet = abnormalCodedValueSet == null ? null : abnormalCodedValueSet.copy(); 2872 dst.criticalCodedValueSet = criticalCodedValueSet == null ? null : criticalCodedValueSet.copy(); 2873 } 2874 2875 protected ObservationDefinition typedCopy() { 2876 return copy(); 2877 } 2878 2879 @Override 2880 public boolean equalsDeep(Base other_) { 2881 if (!super.equalsDeep(other_)) 2882 return false; 2883 if (!(other_ instanceof ObservationDefinition)) 2884 return false; 2885 ObservationDefinition o = (ObservationDefinition) other_; 2886 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 2887 && compareDeep(identifier, o.identifier, true) && compareDeep(permittedDataType, o.permittedDataType, true) 2888 && compareDeep(multipleResultsAllowed, o.multipleResultsAllowed, true) && compareDeep(method, o.method, true) 2889 && compareDeep(preferredReportName, o.preferredReportName, true) 2890 && compareDeep(quantitativeDetails, o.quantitativeDetails, true) 2891 && compareDeep(qualifiedInterval, o.qualifiedInterval, true) 2892 && compareDeep(validCodedValueSet, o.validCodedValueSet, true) 2893 && compareDeep(normalCodedValueSet, o.normalCodedValueSet, true) 2894 && compareDeep(abnormalCodedValueSet, o.abnormalCodedValueSet, true) 2895 && compareDeep(criticalCodedValueSet, o.criticalCodedValueSet, true); 2896 } 2897 2898 @Override 2899 public boolean equalsShallow(Base other_) { 2900 if (!super.equalsShallow(other_)) 2901 return false; 2902 if (!(other_ instanceof ObservationDefinition)) 2903 return false; 2904 ObservationDefinition o = (ObservationDefinition) other_; 2905 return compareValues(permittedDataType, o.permittedDataType, true) 2906 && compareValues(multipleResultsAllowed, o.multipleResultsAllowed, true) 2907 && compareValues(preferredReportName, o.preferredReportName, true); 2908 } 2909 2910 public boolean isEmpty() { 2911 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, code, identifier, permittedDataType, 2912 multipleResultsAllowed, method, preferredReportName, quantitativeDetails, qualifiedInterval, validCodedValueSet, 2913 normalCodedValueSet, abnormalCodedValueSet, criticalCodedValueSet); 2914 } 2915 2916 @Override 2917 public ResourceType getResourceType() { 2918 return ResourceType.ObservationDefinition; 2919 } 2920 2921}