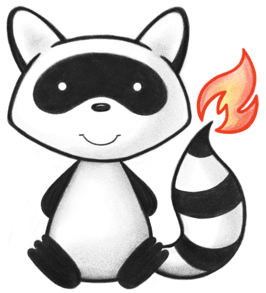
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.BindingStrength; 040import org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.r4.model.Enumerations.SearchParamType; 044import org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.ChildOrder; 050import ca.uhn.fhir.model.api.annotation.Description; 051import ca.uhn.fhir.model.api.annotation.ResourceDef; 052import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 053 054/** 055 * A formal computable definition of an operation (on the RESTful interface) or 056 * a named query (using the search interaction). 057 */ 058@ResourceDef(name = "OperationDefinition", profile = "http://hl7.org/fhir/StructureDefinition/OperationDefinition") 059@ChildOrder(names = { "url", "version", "name", "title", "status", "kind", "experimental", "date", "publisher", 060 "contact", "description", "useContext", "jurisdiction", "purpose", "affectsState", "code", "comment", "base", 061 "resource", "system", "type", "instance", "inputProfile", "outputProfile", "parameter", "overload" }) 062public class OperationDefinition extends MetadataResource { 063 064 public enum OperationKind { 065 /** 066 * This operation is invoked as an operation. 067 */ 068 OPERATION, 069 /** 070 * This operation is a named query, invoked using the search mechanism. 071 */ 072 QUERY, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static OperationKind fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("operation".equals(codeString)) 082 return OPERATION; 083 if ("query".equals(codeString)) 084 return QUERY; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown OperationKind code '" + codeString + "'"); 089 } 090 091 public String toCode() { 092 switch (this) { 093 case OPERATION: 094 return "operation"; 095 case QUERY: 096 return "query"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case OPERATION: 107 return "http://hl7.org/fhir/operation-kind"; 108 case QUERY: 109 return "http://hl7.org/fhir/operation-kind"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case OPERATION: 120 return "This operation is invoked as an operation."; 121 case QUERY: 122 return "This operation is a named query, invoked using the search mechanism."; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDisplay() { 131 switch (this) { 132 case OPERATION: 133 return "Operation"; 134 case QUERY: 135 return "Query"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 } 143 144 public static class OperationKindEnumFactory implements EnumFactory<OperationKind> { 145 public OperationKind fromCode(String codeString) throws IllegalArgumentException { 146 if (codeString == null || "".equals(codeString)) 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("operation".equals(codeString)) 150 return OperationKind.OPERATION; 151 if ("query".equals(codeString)) 152 return OperationKind.QUERY; 153 throw new IllegalArgumentException("Unknown OperationKind code '" + codeString + "'"); 154 } 155 156 public Enumeration<OperationKind> fromType(PrimitiveType<?> code) throws FHIRException { 157 if (code == null) 158 return null; 159 if (code.isEmpty()) 160 return new Enumeration<OperationKind>(this, OperationKind.NULL, code); 161 String codeString = code.asStringValue(); 162 if (codeString == null || "".equals(codeString)) 163 return new Enumeration<OperationKind>(this, OperationKind.NULL, code); 164 if ("operation".equals(codeString)) 165 return new Enumeration<OperationKind>(this, OperationKind.OPERATION, code); 166 if ("query".equals(codeString)) 167 return new Enumeration<OperationKind>(this, OperationKind.QUERY, code); 168 throw new FHIRException("Unknown OperationKind code '" + codeString + "'"); 169 } 170 171 public String toCode(OperationKind code) { 172 if (code == OperationKind.NULL) 173 return null; 174 if (code == OperationKind.OPERATION) 175 return "operation"; 176 if (code == OperationKind.QUERY) 177 return "query"; 178 return "?"; 179 } 180 181 public String toSystem(OperationKind code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum OperationParameterUse { 187 /** 188 * This is an input parameter. 189 */ 190 IN, 191 /** 192 * This is an output parameter. 193 */ 194 OUT, 195 /** 196 * added to help the parsers with the generic types 197 */ 198 NULL; 199 200 public static OperationParameterUse fromCode(String codeString) throws FHIRException { 201 if (codeString == null || "".equals(codeString)) 202 return null; 203 if ("in".equals(codeString)) 204 return IN; 205 if ("out".equals(codeString)) 206 return OUT; 207 if (Configuration.isAcceptInvalidEnums()) 208 return null; 209 else 210 throw new FHIRException("Unknown OperationParameterUse code '" + codeString + "'"); 211 } 212 213 public String toCode() { 214 switch (this) { 215 case IN: 216 return "in"; 217 case OUT: 218 return "out"; 219 case NULL: 220 return null; 221 default: 222 return "?"; 223 } 224 } 225 226 public String getSystem() { 227 switch (this) { 228 case IN: 229 return "http://hl7.org/fhir/operation-parameter-use"; 230 case OUT: 231 return "http://hl7.org/fhir/operation-parameter-use"; 232 case NULL: 233 return null; 234 default: 235 return "?"; 236 } 237 } 238 239 public String getDefinition() { 240 switch (this) { 241 case IN: 242 return "This is an input parameter."; 243 case OUT: 244 return "This is an output parameter."; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getDisplay() { 253 switch (this) { 254 case IN: 255 return "In"; 256 case OUT: 257 return "Out"; 258 case NULL: 259 return null; 260 default: 261 return "?"; 262 } 263 } 264 } 265 266 public static class OperationParameterUseEnumFactory implements EnumFactory<OperationParameterUse> { 267 public OperationParameterUse fromCode(String codeString) throws IllegalArgumentException { 268 if (codeString == null || "".equals(codeString)) 269 if (codeString == null || "".equals(codeString)) 270 return null; 271 if ("in".equals(codeString)) 272 return OperationParameterUse.IN; 273 if ("out".equals(codeString)) 274 return OperationParameterUse.OUT; 275 throw new IllegalArgumentException("Unknown OperationParameterUse code '" + codeString + "'"); 276 } 277 278 public Enumeration<OperationParameterUse> fromType(PrimitiveType<?> code) throws FHIRException { 279 if (code == null) 280 return null; 281 if (code.isEmpty()) 282 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.NULL, code); 283 String codeString = code.asStringValue(); 284 if (codeString == null || "".equals(codeString)) 285 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.NULL, code); 286 if ("in".equals(codeString)) 287 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.IN, code); 288 if ("out".equals(codeString)) 289 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.OUT, code); 290 throw new FHIRException("Unknown OperationParameterUse code '" + codeString + "'"); 291 } 292 293 public String toCode(OperationParameterUse code) { 294 if (code == OperationParameterUse.NULL) 295 return null; 296 if (code == OperationParameterUse.IN) 297 return "in"; 298 if (code == OperationParameterUse.OUT) 299 return "out"; 300 return "?"; 301 } 302 303 public String toSystem(OperationParameterUse code) { 304 return code.getSystem(); 305 } 306 } 307 308 @Block() 309 public static class OperationDefinitionParameterComponent extends BackboneElement implements IBaseBackboneElement { 310 /** 311 * The name of used to identify the parameter. 312 */ 313 @Child(name = "name", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 314 @Description(shortDefinition = "Name in Parameters.parameter.name or in URL", formalDefinition = "The name of used to identify the parameter.") 315 protected CodeType name; 316 317 /** 318 * Whether this is an input or an output parameter. 319 */ 320 @Child(name = "use", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 321 @Description(shortDefinition = "in | out", formalDefinition = "Whether this is an input or an output parameter.") 322 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/operation-parameter-use") 323 protected Enumeration<OperationParameterUse> use; 324 325 /** 326 * The minimum number of times this parameter SHALL appear in the request or 327 * response. 328 */ 329 @Child(name = "min", type = { IntegerType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 330 @Description(shortDefinition = "Minimum Cardinality", formalDefinition = "The minimum number of times this parameter SHALL appear in the request or response.") 331 protected IntegerType min; 332 333 /** 334 * The maximum number of times this element is permitted to appear in the 335 * request or response. 336 */ 337 @Child(name = "max", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 338 @Description(shortDefinition = "Maximum Cardinality (a number or *)", formalDefinition = "The maximum number of times this element is permitted to appear in the request or response.") 339 protected StringType max; 340 341 /** 342 * Describes the meaning or use of this parameter. 343 */ 344 @Child(name = "documentation", type = { 345 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 346 @Description(shortDefinition = "Description of meaning/use", formalDefinition = "Describes the meaning or use of this parameter.") 347 protected StringType documentation; 348 349 /** 350 * The type for this parameter. 351 */ 352 @Child(name = "type", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 353 @Description(shortDefinition = "What type this parameter has", formalDefinition = "The type for this parameter.") 354 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/all-types") 355 protected CodeType type; 356 357 /** 358 * Used when the type is "Reference" or "canonical", and identifies a profile 359 * structure or implementation Guide that applies to the target of the reference 360 * this parameter refers to. If any profiles are specified, then the content 361 * must conform to at least one of them. The URL can be a local reference - to a 362 * contained StructureDefinition, or a reference to another StructureDefinition 363 * or Implementation Guide by a canonical URL. When an implementation guide is 364 * specified, the target resource SHALL conform to at least one profile defined 365 * in the implementation guide. 366 */ 367 @Child(name = "targetProfile", type = { 368 CanonicalType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 369 @Description(shortDefinition = "If type is Reference | canonical, allowed targets", formalDefinition = "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.") 370 protected List<CanonicalType> targetProfile; 371 372 /** 373 * How the parameter is understood as a search parameter. This is only used if 374 * the parameter type is 'string'. 375 */ 376 @Child(name = "searchType", type = { 377 CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 378 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri | special", formalDefinition = "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.") 379 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-param-type") 380 protected Enumeration<SearchParamType> searchType; 381 382 /** 383 * Binds to a value set if this parameter is coded (code, Coding, 384 * CodeableConcept). 385 */ 386 @Child(name = "binding", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 387 @Description(shortDefinition = "ValueSet details if this is coded", formalDefinition = "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).") 388 protected OperationDefinitionParameterBindingComponent binding; 389 390 /** 391 * Identifies other resource parameters within the operation invocation that are 392 * expected to resolve to this resource. 393 */ 394 @Child(name = "referencedFrom", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 395 @Description(shortDefinition = "References to this parameter", formalDefinition = "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.") 396 protected List<OperationDefinitionParameterReferencedFromComponent> referencedFrom; 397 398 /** 399 * The parts of a nested Parameter. 400 */ 401 @Child(name = "part", type = { 402 OperationDefinitionParameterComponent.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 403 @Description(shortDefinition = "Parts of a nested Parameter", formalDefinition = "The parts of a nested Parameter.") 404 protected List<OperationDefinitionParameterComponent> part; 405 406 private static final long serialVersionUID = 1715661531L; 407 408 /** 409 * Constructor 410 */ 411 public OperationDefinitionParameterComponent() { 412 super(); 413 } 414 415 /** 416 * Constructor 417 */ 418 public OperationDefinitionParameterComponent(CodeType name, Enumeration<OperationParameterUse> use, IntegerType min, 419 StringType max) { 420 super(); 421 this.name = name; 422 this.use = use; 423 this.min = min; 424 this.max = max; 425 } 426 427 /** 428 * @return {@link #name} (The name of used to identify the parameter.). This is 429 * the underlying object with id, value and extensions. The accessor 430 * "getName" gives direct access to the value 431 */ 432 public CodeType getNameElement() { 433 if (this.name == null) 434 if (Configuration.errorOnAutoCreate()) 435 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.name"); 436 else if (Configuration.doAutoCreate()) 437 this.name = new CodeType(); // bb 438 return this.name; 439 } 440 441 public boolean hasNameElement() { 442 return this.name != null && !this.name.isEmpty(); 443 } 444 445 public boolean hasName() { 446 return this.name != null && !this.name.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #name} (The name of used to identify the parameter.). 451 * This is the underlying object with id, value and extensions. The 452 * accessor "getName" gives direct access to the value 453 */ 454 public OperationDefinitionParameterComponent setNameElement(CodeType value) { 455 this.name = value; 456 return this; 457 } 458 459 /** 460 * @return The name of used to identify the parameter. 461 */ 462 public String getName() { 463 return this.name == null ? null : this.name.getValue(); 464 } 465 466 /** 467 * @param value The name of used to identify the parameter. 468 */ 469 public OperationDefinitionParameterComponent setName(String value) { 470 if (this.name == null) 471 this.name = new CodeType(); 472 this.name.setValue(value); 473 return this; 474 } 475 476 /** 477 * @return {@link #use} (Whether this is an input or an output parameter.). This 478 * is the underlying object with id, value and extensions. The accessor 479 * "getUse" gives direct access to the value 480 */ 481 public Enumeration<OperationParameterUse> getUseElement() { 482 if (this.use == null) 483 if (Configuration.errorOnAutoCreate()) 484 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.use"); 485 else if (Configuration.doAutoCreate()) 486 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); // bb 487 return this.use; 488 } 489 490 public boolean hasUseElement() { 491 return this.use != null && !this.use.isEmpty(); 492 } 493 494 public boolean hasUse() { 495 return this.use != null && !this.use.isEmpty(); 496 } 497 498 /** 499 * @param value {@link #use} (Whether this is an input or an output parameter.). 500 * This is the underlying object with id, value and extensions. The 501 * accessor "getUse" gives direct access to the value 502 */ 503 public OperationDefinitionParameterComponent setUseElement(Enumeration<OperationParameterUse> value) { 504 this.use = value; 505 return this; 506 } 507 508 /** 509 * @return Whether this is an input or an output parameter. 510 */ 511 public OperationParameterUse getUse() { 512 return this.use == null ? null : this.use.getValue(); 513 } 514 515 /** 516 * @param value Whether this is an input or an output parameter. 517 */ 518 public OperationDefinitionParameterComponent setUse(OperationParameterUse value) { 519 if (this.use == null) 520 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); 521 this.use.setValue(value); 522 return this; 523 } 524 525 /** 526 * @return {@link #min} (The minimum number of times this parameter SHALL appear 527 * in the request or response.). This is the underlying object with id, 528 * value and extensions. The accessor "getMin" gives direct access to 529 * the value 530 */ 531 public IntegerType getMinElement() { 532 if (this.min == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.min"); 535 else if (Configuration.doAutoCreate()) 536 this.min = new IntegerType(); // bb 537 return this.min; 538 } 539 540 public boolean hasMinElement() { 541 return this.min != null && !this.min.isEmpty(); 542 } 543 544 public boolean hasMin() { 545 return this.min != null && !this.min.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #min} (The minimum number of times this parameter SHALL 550 * appear in the request or response.). This is the underlying 551 * object with id, value and extensions. The accessor "getMin" 552 * gives direct access to the value 553 */ 554 public OperationDefinitionParameterComponent setMinElement(IntegerType value) { 555 this.min = value; 556 return this; 557 } 558 559 /** 560 * @return The minimum number of times this parameter SHALL appear in the 561 * request or response. 562 */ 563 public int getMin() { 564 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 565 } 566 567 /** 568 * @param value The minimum number of times this parameter SHALL appear in the 569 * request or response. 570 */ 571 public OperationDefinitionParameterComponent setMin(int value) { 572 if (this.min == null) 573 this.min = new IntegerType(); 574 this.min.setValue(value); 575 return this; 576 } 577 578 /** 579 * @return {@link #max} (The maximum number of times this element is permitted 580 * to appear in the request or response.). This is the underlying object 581 * with id, value and extensions. The accessor "getMax" gives direct 582 * access to the value 583 */ 584 public StringType getMaxElement() { 585 if (this.max == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.max"); 588 else if (Configuration.doAutoCreate()) 589 this.max = new StringType(); // bb 590 return this.max; 591 } 592 593 public boolean hasMaxElement() { 594 return this.max != null && !this.max.isEmpty(); 595 } 596 597 public boolean hasMax() { 598 return this.max != null && !this.max.isEmpty(); 599 } 600 601 /** 602 * @param value {@link #max} (The maximum number of times this element is 603 * permitted to appear in the request or response.). This is the 604 * underlying object with id, value and extensions. The accessor 605 * "getMax" gives direct access to the value 606 */ 607 public OperationDefinitionParameterComponent setMaxElement(StringType value) { 608 this.max = value; 609 return this; 610 } 611 612 /** 613 * @return The maximum number of times this element is permitted to appear in 614 * the request or response. 615 */ 616 public String getMax() { 617 return this.max == null ? null : this.max.getValue(); 618 } 619 620 /** 621 * @param value The maximum number of times this element is permitted to appear 622 * in the request or response. 623 */ 624 public OperationDefinitionParameterComponent setMax(String value) { 625 if (this.max == null) 626 this.max = new StringType(); 627 this.max.setValue(value); 628 return this; 629 } 630 631 /** 632 * @return {@link #documentation} (Describes the meaning or use of this 633 * parameter.). This is the underlying object with id, value and 634 * extensions. The accessor "getDocumentation" gives direct access to 635 * the value 636 */ 637 public StringType getDocumentationElement() { 638 if (this.documentation == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.documentation"); 641 else if (Configuration.doAutoCreate()) 642 this.documentation = new StringType(); // bb 643 return this.documentation; 644 } 645 646 public boolean hasDocumentationElement() { 647 return this.documentation != null && !this.documentation.isEmpty(); 648 } 649 650 public boolean hasDocumentation() { 651 return this.documentation != null && !this.documentation.isEmpty(); 652 } 653 654 /** 655 * @param value {@link #documentation} (Describes the meaning or use of this 656 * parameter.). This is the underlying object with id, value and 657 * extensions. The accessor "getDocumentation" gives direct access 658 * to the value 659 */ 660 public OperationDefinitionParameterComponent setDocumentationElement(StringType value) { 661 this.documentation = value; 662 return this; 663 } 664 665 /** 666 * @return Describes the meaning or use of this parameter. 667 */ 668 public String getDocumentation() { 669 return this.documentation == null ? null : this.documentation.getValue(); 670 } 671 672 /** 673 * @param value Describes the meaning or use of this parameter. 674 */ 675 public OperationDefinitionParameterComponent setDocumentation(String value) { 676 if (Utilities.noString(value)) 677 this.documentation = null; 678 else { 679 if (this.documentation == null) 680 this.documentation = new StringType(); 681 this.documentation.setValue(value); 682 } 683 return this; 684 } 685 686 /** 687 * @return {@link #type} (The type for this parameter.). This is the underlying 688 * object with id, value and extensions. The accessor "getType" gives 689 * direct access to the value 690 */ 691 public CodeType getTypeElement() { 692 if (this.type == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.type"); 695 else if (Configuration.doAutoCreate()) 696 this.type = new CodeType(); // bb 697 return this.type; 698 } 699 700 public boolean hasTypeElement() { 701 return this.type != null && !this.type.isEmpty(); 702 } 703 704 public boolean hasType() { 705 return this.type != null && !this.type.isEmpty(); 706 } 707 708 /** 709 * @param value {@link #type} (The type for this parameter.). This is the 710 * underlying object with id, value and extensions. The accessor 711 * "getType" gives direct access to the value 712 */ 713 public OperationDefinitionParameterComponent setTypeElement(CodeType value) { 714 this.type = value; 715 return this; 716 } 717 718 /** 719 * @return The type for this parameter. 720 */ 721 public String getType() { 722 return this.type == null ? null : this.type.getValue(); 723 } 724 725 /** 726 * @param value The type for this parameter. 727 */ 728 public OperationDefinitionParameterComponent setType(String value) { 729 if (Utilities.noString(value)) 730 this.type = null; 731 else { 732 if (this.type == null) 733 this.type = new CodeType(); 734 this.type.setValue(value); 735 } 736 return this; 737 } 738 739 /** 740 * @return {@link #targetProfile} (Used when the type is "Reference" or 741 * "canonical", and identifies a profile structure or implementation 742 * Guide that applies to the target of the reference this parameter 743 * refers to. If any profiles are specified, then the content must 744 * conform to at least one of them. The URL can be a local reference - 745 * to a contained StructureDefinition, or a reference to another 746 * StructureDefinition or Implementation Guide by a canonical URL. When 747 * an implementation guide is specified, the target resource SHALL 748 * conform to at least one profile defined in the implementation guide.) 749 */ 750 public List<CanonicalType> getTargetProfile() { 751 if (this.targetProfile == null) 752 this.targetProfile = new ArrayList<CanonicalType>(); 753 return this.targetProfile; 754 } 755 756 /** 757 * @return Returns a reference to <code>this</code> for easy method chaining 758 */ 759 public OperationDefinitionParameterComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 760 this.targetProfile = theTargetProfile; 761 return this; 762 } 763 764 public boolean hasTargetProfile() { 765 if (this.targetProfile == null) 766 return false; 767 for (CanonicalType item : this.targetProfile) 768 if (!item.isEmpty()) 769 return true; 770 return false; 771 } 772 773 /** 774 * @return {@link #targetProfile} (Used when the type is "Reference" or 775 * "canonical", and identifies a profile structure or implementation 776 * Guide that applies to the target of the reference this parameter 777 * refers to. If any profiles are specified, then the content must 778 * conform to at least one of them. The URL can be a local reference - 779 * to a contained StructureDefinition, or a reference to another 780 * StructureDefinition or Implementation Guide by a canonical URL. When 781 * an implementation guide is specified, the target resource SHALL 782 * conform to at least one profile defined in the implementation guide.) 783 */ 784 public CanonicalType addTargetProfileElement() {// 2 785 CanonicalType t = new CanonicalType(); 786 if (this.targetProfile == null) 787 this.targetProfile = new ArrayList<CanonicalType>(); 788 this.targetProfile.add(t); 789 return t; 790 } 791 792 /** 793 * @param value {@link #targetProfile} (Used when the type is "Reference" or 794 * "canonical", and identifies a profile structure or 795 * implementation Guide that applies to the target of the reference 796 * this parameter refers to. If any profiles are specified, then 797 * the content must conform to at least one of them. The URL can be 798 * a local reference - to a contained StructureDefinition, or a 799 * reference to another StructureDefinition or Implementation Guide 800 * by a canonical URL. When an implementation guide is specified, 801 * the target resource SHALL conform to at least one profile 802 * defined in the implementation guide.) 803 */ 804 public OperationDefinitionParameterComponent addTargetProfile(String value) { // 1 805 CanonicalType t = new CanonicalType(); 806 t.setValue(value); 807 if (this.targetProfile == null) 808 this.targetProfile = new ArrayList<CanonicalType>(); 809 this.targetProfile.add(t); 810 return this; 811 } 812 813 /** 814 * @param value {@link #targetProfile} (Used when the type is "Reference" or 815 * "canonical", and identifies a profile structure or 816 * implementation Guide that applies to the target of the reference 817 * this parameter refers to. If any profiles are specified, then 818 * the content must conform to at least one of them. The URL can be 819 * a local reference - to a contained StructureDefinition, or a 820 * reference to another StructureDefinition or Implementation Guide 821 * by a canonical URL. When an implementation guide is specified, 822 * the target resource SHALL conform to at least one profile 823 * defined in the implementation guide.) 824 */ 825 public boolean hasTargetProfile(String value) { 826 if (this.targetProfile == null) 827 return false; 828 for (CanonicalType v : this.targetProfile) 829 if (v.getValue().equals(value)) // canonical(StructureDefinition) 830 return true; 831 return false; 832 } 833 834 /** 835 * @return {@link #searchType} (How the parameter is understood as a search 836 * parameter. This is only used if the parameter type is 'string'.). 837 * This is the underlying object with id, value and extensions. The 838 * accessor "getSearchType" gives direct access to the value 839 */ 840 public Enumeration<SearchParamType> getSearchTypeElement() { 841 if (this.searchType == null) 842 if (Configuration.errorOnAutoCreate()) 843 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.searchType"); 844 else if (Configuration.doAutoCreate()) 845 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 846 return this.searchType; 847 } 848 849 public boolean hasSearchTypeElement() { 850 return this.searchType != null && !this.searchType.isEmpty(); 851 } 852 853 public boolean hasSearchType() { 854 return this.searchType != null && !this.searchType.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #searchType} (How the parameter is understood as a search 859 * parameter. This is only used if the parameter type is 860 * 'string'.). This is the underlying object with id, value and 861 * extensions. The accessor "getSearchType" gives direct access to 862 * the value 863 */ 864 public OperationDefinitionParameterComponent setSearchTypeElement(Enumeration<SearchParamType> value) { 865 this.searchType = value; 866 return this; 867 } 868 869 /** 870 * @return How the parameter is understood as a search parameter. This is only 871 * used if the parameter type is 'string'. 872 */ 873 public SearchParamType getSearchType() { 874 return this.searchType == null ? null : this.searchType.getValue(); 875 } 876 877 /** 878 * @param value How the parameter is understood as a search parameter. This is 879 * only used if the parameter type is 'string'. 880 */ 881 public OperationDefinitionParameterComponent setSearchType(SearchParamType value) { 882 if (value == null) 883 this.searchType = null; 884 else { 885 if (this.searchType == null) 886 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 887 this.searchType.setValue(value); 888 } 889 return this; 890 } 891 892 /** 893 * @return {@link #binding} (Binds to a value set if this parameter is coded 894 * (code, Coding, CodeableConcept).) 895 */ 896 public OperationDefinitionParameterBindingComponent getBinding() { 897 if (this.binding == null) 898 if (Configuration.errorOnAutoCreate()) 899 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.binding"); 900 else if (Configuration.doAutoCreate()) 901 this.binding = new OperationDefinitionParameterBindingComponent(); // cc 902 return this.binding; 903 } 904 905 public boolean hasBinding() { 906 return this.binding != null && !this.binding.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #binding} (Binds to a value set if this parameter is 911 * coded (code, Coding, CodeableConcept).) 912 */ 913 public OperationDefinitionParameterComponent setBinding(OperationDefinitionParameterBindingComponent value) { 914 this.binding = value; 915 return this; 916 } 917 918 /** 919 * @return {@link #referencedFrom} (Identifies other resource parameters within 920 * the operation invocation that are expected to resolve to this 921 * resource.) 922 */ 923 public List<OperationDefinitionParameterReferencedFromComponent> getReferencedFrom() { 924 if (this.referencedFrom == null) 925 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 926 return this.referencedFrom; 927 } 928 929 /** 930 * @return Returns a reference to <code>this</code> for easy method chaining 931 */ 932 public OperationDefinitionParameterComponent setReferencedFrom( 933 List<OperationDefinitionParameterReferencedFromComponent> theReferencedFrom) { 934 this.referencedFrom = theReferencedFrom; 935 return this; 936 } 937 938 public boolean hasReferencedFrom() { 939 if (this.referencedFrom == null) 940 return false; 941 for (OperationDefinitionParameterReferencedFromComponent item : this.referencedFrom) 942 if (!item.isEmpty()) 943 return true; 944 return false; 945 } 946 947 public OperationDefinitionParameterReferencedFromComponent addReferencedFrom() { // 3 948 OperationDefinitionParameterReferencedFromComponent t = new OperationDefinitionParameterReferencedFromComponent(); 949 if (this.referencedFrom == null) 950 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 951 this.referencedFrom.add(t); 952 return t; 953 } 954 955 public OperationDefinitionParameterComponent addReferencedFrom( 956 OperationDefinitionParameterReferencedFromComponent t) { // 3 957 if (t == null) 958 return this; 959 if (this.referencedFrom == null) 960 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 961 this.referencedFrom.add(t); 962 return this; 963 } 964 965 /** 966 * @return The first repetition of repeating field {@link #referencedFrom}, 967 * creating it if it does not already exist 968 */ 969 public OperationDefinitionParameterReferencedFromComponent getReferencedFromFirstRep() { 970 if (getReferencedFrom().isEmpty()) { 971 addReferencedFrom(); 972 } 973 return getReferencedFrom().get(0); 974 } 975 976 /** 977 * @return {@link #part} (The parts of a nested Parameter.) 978 */ 979 public List<OperationDefinitionParameterComponent> getPart() { 980 if (this.part == null) 981 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 982 return this.part; 983 } 984 985 /** 986 * @return Returns a reference to <code>this</code> for easy method chaining 987 */ 988 public OperationDefinitionParameterComponent setPart(List<OperationDefinitionParameterComponent> thePart) { 989 this.part = thePart; 990 return this; 991 } 992 993 public boolean hasPart() { 994 if (this.part == null) 995 return false; 996 for (OperationDefinitionParameterComponent item : this.part) 997 if (!item.isEmpty()) 998 return true; 999 return false; 1000 } 1001 1002 public OperationDefinitionParameterComponent addPart() { // 3 1003 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 1004 if (this.part == null) 1005 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1006 this.part.add(t); 1007 return t; 1008 } 1009 1010 public OperationDefinitionParameterComponent addPart(OperationDefinitionParameterComponent t) { // 3 1011 if (t == null) 1012 return this; 1013 if (this.part == null) 1014 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1015 this.part.add(t); 1016 return this; 1017 } 1018 1019 /** 1020 * @return The first repetition of repeating field {@link #part}, creating it if 1021 * it does not already exist 1022 */ 1023 public OperationDefinitionParameterComponent getPartFirstRep() { 1024 if (getPart().isEmpty()) { 1025 addPart(); 1026 } 1027 return getPart().get(0); 1028 } 1029 1030 protected void listChildren(List<Property> children) { 1031 super.listChildren(children); 1032 children.add(new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name)); 1033 children.add(new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use)); 1034 children.add(new Property("min", "integer", 1035 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 1036 children.add(new Property("max", "string", 1037 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 1038 children.add(new Property("documentation", "string", "Describes the meaning or use of this parameter.", 0, 1, 1039 documentation)); 1040 children.add(new Property("type", "code", "The type for this parameter.", 0, 1, type)); 1041 children.add(new Property("targetProfile", "canonical(StructureDefinition)", 1042 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 1043 0, java.lang.Integer.MAX_VALUE, targetProfile)); 1044 children.add(new Property("searchType", "code", 1045 "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.", 1046 0, 1, searchType)); 1047 children.add(new Property("binding", "", 1048 "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding)); 1049 children.add(new Property("referencedFrom", "", 1050 "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.", 1051 0, java.lang.Integer.MAX_VALUE, referencedFrom)); 1052 children.add(new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, 1053 java.lang.Integer.MAX_VALUE, part)); 1054 } 1055 1056 @Override 1057 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1058 switch (_hash) { 1059 case 3373707: 1060 /* name */ return new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name); 1061 case 116103: 1062 /* use */ return new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use); 1063 case 108114: 1064 /* min */ return new Property("min", "integer", 1065 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 1066 case 107876: 1067 /* max */ return new Property("max", "string", 1068 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 1069 case 1587405498: 1070 /* documentation */ return new Property("documentation", "string", 1071 "Describes the meaning or use of this parameter.", 0, 1, documentation); 1072 case 3575610: 1073 /* type */ return new Property("type", "code", "The type for this parameter.", 0, 1, type); 1074 case 1994521304: 1075 /* targetProfile */ return new Property("targetProfile", "canonical(StructureDefinition)", 1076 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 1077 0, java.lang.Integer.MAX_VALUE, targetProfile); 1078 case -710454014: 1079 /* searchType */ return new Property("searchType", "code", 1080 "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.", 1081 0, 1, searchType); 1082 case -108220795: 1083 /* binding */ return new Property("binding", "", 1084 "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding); 1085 case -1896721981: 1086 /* referencedFrom */ return new Property("referencedFrom", "", 1087 "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.", 1088 0, java.lang.Integer.MAX_VALUE, referencedFrom); 1089 case 3433459: 1090 /* part */ return new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, 1091 java.lang.Integer.MAX_VALUE, part); 1092 default: 1093 return super.getNamedProperty(_hash, _name, _checkValid); 1094 } 1095 1096 } 1097 1098 @Override 1099 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1100 switch (hash) { 1101 case 3373707: 1102 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // CodeType 1103 case 116103: 1104 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<OperationParameterUse> 1105 case 108114: 1106 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // IntegerType 1107 case 107876: 1108 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 1109 case 1587405498: 1110 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 1111 case 3575610: 1112 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 1113 case 1994521304: 1114 /* targetProfile */ return this.targetProfile == null ? new Base[0] 1115 : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 1116 case -710454014: 1117 /* searchType */ return this.searchType == null ? new Base[0] : new Base[] { this.searchType }; // Enumeration<SearchParamType> 1118 case -108220795: 1119 /* binding */ return this.binding == null ? new Base[0] : new Base[] { this.binding }; // OperationDefinitionParameterBindingComponent 1120 case -1896721981: 1121 /* referencedFrom */ return this.referencedFrom == null ? new Base[0] 1122 : this.referencedFrom.toArray(new Base[this.referencedFrom.size()]); // OperationDefinitionParameterReferencedFromComponent 1123 case 3433459: 1124 /* part */ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // OperationDefinitionParameterComponent 1125 default: 1126 return super.getProperty(hash, name, checkValid); 1127 } 1128 1129 } 1130 1131 @Override 1132 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1133 switch (hash) { 1134 case 3373707: // name 1135 this.name = castToCode(value); // CodeType 1136 return value; 1137 case 116103: // use 1138 value = new OperationParameterUseEnumFactory().fromType(castToCode(value)); 1139 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1140 return value; 1141 case 108114: // min 1142 this.min = castToInteger(value); // IntegerType 1143 return value; 1144 case 107876: // max 1145 this.max = castToString(value); // StringType 1146 return value; 1147 case 1587405498: // documentation 1148 this.documentation = castToString(value); // StringType 1149 return value; 1150 case 3575610: // type 1151 this.type = castToCode(value); // CodeType 1152 return value; 1153 case 1994521304: // targetProfile 1154 this.getTargetProfile().add(castToCanonical(value)); // CanonicalType 1155 return value; 1156 case -710454014: // searchType 1157 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 1158 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1159 return value; 1160 case -108220795: // binding 1161 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1162 return value; 1163 case -1896721981: // referencedFrom 1164 this.getReferencedFrom().add((OperationDefinitionParameterReferencedFromComponent) value); // OperationDefinitionParameterReferencedFromComponent 1165 return value; 1166 case 3433459: // part 1167 this.getPart().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 1168 return value; 1169 default: 1170 return super.setProperty(hash, name, value); 1171 } 1172 1173 } 1174 1175 @Override 1176 public Base setProperty(String name, Base value) throws FHIRException { 1177 if (name.equals("name")) { 1178 this.name = castToCode(value); // CodeType 1179 } else if (name.equals("use")) { 1180 value = new OperationParameterUseEnumFactory().fromType(castToCode(value)); 1181 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1182 } else if (name.equals("min")) { 1183 this.min = castToInteger(value); // IntegerType 1184 } else if (name.equals("max")) { 1185 this.max = castToString(value); // StringType 1186 } else if (name.equals("documentation")) { 1187 this.documentation = castToString(value); // StringType 1188 } else if (name.equals("type")) { 1189 this.type = castToCode(value); // CodeType 1190 } else if (name.equals("targetProfile")) { 1191 this.getTargetProfile().add(castToCanonical(value)); 1192 } else if (name.equals("searchType")) { 1193 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 1194 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1195 } else if (name.equals("binding")) { 1196 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1197 } else if (name.equals("referencedFrom")) { 1198 this.getReferencedFrom().add((OperationDefinitionParameterReferencedFromComponent) value); 1199 } else if (name.equals("part")) { 1200 this.getPart().add((OperationDefinitionParameterComponent) value); 1201 } else 1202 return super.setProperty(name, value); 1203 return value; 1204 } 1205 1206 @Override 1207 public void removeChild(String name, Base value) throws FHIRException { 1208 if (name.equals("name")) { 1209 this.name = null; 1210 } else if (name.equals("use")) { 1211 this.use = null; 1212 } else if (name.equals("min")) { 1213 this.min = null; 1214 } else if (name.equals("max")) { 1215 this.max = null; 1216 } else if (name.equals("documentation")) { 1217 this.documentation = null; 1218 } else if (name.equals("type")) { 1219 this.type = null; 1220 } else if (name.equals("targetProfile")) { 1221 this.getTargetProfile().remove(castToCanonical(value)); 1222 } else if (name.equals("searchType")) { 1223 this.searchType = null; 1224 } else if (name.equals("binding")) { 1225 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1226 } else if (name.equals("referencedFrom")) { 1227 this.getReferencedFrom().remove((OperationDefinitionParameterReferencedFromComponent) value); 1228 } else if (name.equals("part")) { 1229 this.getPart().remove((OperationDefinitionParameterComponent) value); 1230 } else 1231 super.removeChild(name, value); 1232 1233 } 1234 1235 @Override 1236 public Base makeProperty(int hash, String name) throws FHIRException { 1237 switch (hash) { 1238 case 3373707: 1239 return getNameElement(); 1240 case 116103: 1241 return getUseElement(); 1242 case 108114: 1243 return getMinElement(); 1244 case 107876: 1245 return getMaxElement(); 1246 case 1587405498: 1247 return getDocumentationElement(); 1248 case 3575610: 1249 return getTypeElement(); 1250 case 1994521304: 1251 return addTargetProfileElement(); 1252 case -710454014: 1253 return getSearchTypeElement(); 1254 case -108220795: 1255 return getBinding(); 1256 case -1896721981: 1257 return addReferencedFrom(); 1258 case 3433459: 1259 return addPart(); 1260 default: 1261 return super.makeProperty(hash, name); 1262 } 1263 1264 } 1265 1266 @Override 1267 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1268 switch (hash) { 1269 case 3373707: 1270 /* name */ return new String[] { "code" }; 1271 case 116103: 1272 /* use */ return new String[] { "code" }; 1273 case 108114: 1274 /* min */ return new String[] { "integer" }; 1275 case 107876: 1276 /* max */ return new String[] { "string" }; 1277 case 1587405498: 1278 /* documentation */ return new String[] { "string" }; 1279 case 3575610: 1280 /* type */ return new String[] { "code" }; 1281 case 1994521304: 1282 /* targetProfile */ return new String[] { "canonical" }; 1283 case -710454014: 1284 /* searchType */ return new String[] { "code" }; 1285 case -108220795: 1286 /* binding */ return new String[] {}; 1287 case -1896721981: 1288 /* referencedFrom */ return new String[] {}; 1289 case 3433459: 1290 /* part */ return new String[] { "@OperationDefinition.parameter" }; 1291 default: 1292 return super.getTypesForProperty(hash, name); 1293 } 1294 1295 } 1296 1297 @Override 1298 public Base addChild(String name) throws FHIRException { 1299 if (name.equals("name")) { 1300 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 1301 } else if (name.equals("use")) { 1302 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.use"); 1303 } else if (name.equals("min")) { 1304 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.min"); 1305 } else if (name.equals("max")) { 1306 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.max"); 1307 } else if (name.equals("documentation")) { 1308 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.documentation"); 1309 } else if (name.equals("type")) { 1310 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 1311 } else if (name.equals("targetProfile")) { 1312 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.targetProfile"); 1313 } else if (name.equals("searchType")) { 1314 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.searchType"); 1315 } else if (name.equals("binding")) { 1316 this.binding = new OperationDefinitionParameterBindingComponent(); 1317 return this.binding; 1318 } else if (name.equals("referencedFrom")) { 1319 return addReferencedFrom(); 1320 } else if (name.equals("part")) { 1321 return addPart(); 1322 } else 1323 return super.addChild(name); 1324 } 1325 1326 public OperationDefinitionParameterComponent copy() { 1327 OperationDefinitionParameterComponent dst = new OperationDefinitionParameterComponent(); 1328 copyValues(dst); 1329 return dst; 1330 } 1331 1332 public void copyValues(OperationDefinitionParameterComponent dst) { 1333 super.copyValues(dst); 1334 dst.name = name == null ? null : name.copy(); 1335 dst.use = use == null ? null : use.copy(); 1336 dst.min = min == null ? null : min.copy(); 1337 dst.max = max == null ? null : max.copy(); 1338 dst.documentation = documentation == null ? null : documentation.copy(); 1339 dst.type = type == null ? null : type.copy(); 1340 if (targetProfile != null) { 1341 dst.targetProfile = new ArrayList<CanonicalType>(); 1342 for (CanonicalType i : targetProfile) 1343 dst.targetProfile.add(i.copy()); 1344 } 1345 ; 1346 dst.searchType = searchType == null ? null : searchType.copy(); 1347 dst.binding = binding == null ? null : binding.copy(); 1348 if (referencedFrom != null) { 1349 dst.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 1350 for (OperationDefinitionParameterReferencedFromComponent i : referencedFrom) 1351 dst.referencedFrom.add(i.copy()); 1352 } 1353 ; 1354 if (part != null) { 1355 dst.part = new ArrayList<OperationDefinitionParameterComponent>(); 1356 for (OperationDefinitionParameterComponent i : part) 1357 dst.part.add(i.copy()); 1358 } 1359 ; 1360 } 1361 1362 @Override 1363 public boolean equalsDeep(Base other_) { 1364 if (!super.equalsDeep(other_)) 1365 return false; 1366 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1367 return false; 1368 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1369 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 1370 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) 1371 && compareDeep(type, o.type, true) && compareDeep(targetProfile, o.targetProfile, true) 1372 && compareDeep(searchType, o.searchType, true) && compareDeep(binding, o.binding, true) 1373 && compareDeep(referencedFrom, o.referencedFrom, true) && compareDeep(part, o.part, true); 1374 } 1375 1376 @Override 1377 public boolean equalsShallow(Base other_) { 1378 if (!super.equalsShallow(other_)) 1379 return false; 1380 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1381 return false; 1382 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1383 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 1384 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) 1385 && compareValues(type, o.type, true) && compareValues(searchType, o.searchType, true); 1386 } 1387 1388 public boolean isEmpty() { 1389 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, min, max, documentation, type, 1390 targetProfile, searchType, binding, referencedFrom, part); 1391 } 1392 1393 public String fhirType() { 1394 return "OperationDefinition.parameter"; 1395 1396 } 1397 1398 } 1399 1400 @Block() 1401 public static class OperationDefinitionParameterBindingComponent extends BackboneElement 1402 implements IBaseBackboneElement { 1403 /** 1404 * Indicates the degree of conformance expectations associated with this binding 1405 * - that is, the degree to which the provided value set must be adhered to in 1406 * the instances. 1407 */ 1408 @Child(name = "strength", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1409 @Description(shortDefinition = "required | extensible | preferred | example", formalDefinition = "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.") 1410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/binding-strength") 1411 protected Enumeration<BindingStrength> strength; 1412 1413 /** 1414 * Points to the value set or external definition (e.g. implicit value set) that 1415 * identifies the set of codes to be used. 1416 */ 1417 @Child(name = "valueSet", type = { 1418 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1419 @Description(shortDefinition = "Source of value set", formalDefinition = "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.") 1420 protected CanonicalType valueSet; 1421 1422 private static final long serialVersionUID = -2048653907L; 1423 1424 /** 1425 * Constructor 1426 */ 1427 public OperationDefinitionParameterBindingComponent() { 1428 super(); 1429 } 1430 1431 /** 1432 * Constructor 1433 */ 1434 public OperationDefinitionParameterBindingComponent(Enumeration<BindingStrength> strength, CanonicalType valueSet) { 1435 super(); 1436 this.strength = strength; 1437 this.valueSet = valueSet; 1438 } 1439 1440 /** 1441 * @return {@link #strength} (Indicates the degree of conformance expectations 1442 * associated with this binding - that is, the degree to which the 1443 * provided value set must be adhered to in the instances.). This is the 1444 * underlying object with id, value and extensions. The accessor 1445 * "getStrength" gives direct access to the value 1446 */ 1447 public Enumeration<BindingStrength> getStrengthElement() { 1448 if (this.strength == null) 1449 if (Configuration.errorOnAutoCreate()) 1450 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.strength"); 1451 else if (Configuration.doAutoCreate()) 1452 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 1453 return this.strength; 1454 } 1455 1456 public boolean hasStrengthElement() { 1457 return this.strength != null && !this.strength.isEmpty(); 1458 } 1459 1460 public boolean hasStrength() { 1461 return this.strength != null && !this.strength.isEmpty(); 1462 } 1463 1464 /** 1465 * @param value {@link #strength} (Indicates the degree of conformance 1466 * expectations associated with this binding - that is, the degree 1467 * to which the provided value set must be adhered to in the 1468 * instances.). This is the underlying object with id, value and 1469 * extensions. The accessor "getStrength" gives direct access to 1470 * the value 1471 */ 1472 public OperationDefinitionParameterBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 1473 this.strength = value; 1474 return this; 1475 } 1476 1477 /** 1478 * @return Indicates the degree of conformance expectations associated with this 1479 * binding - that is, the degree to which the provided value set must be 1480 * adhered to in the instances. 1481 */ 1482 public BindingStrength getStrength() { 1483 return this.strength == null ? null : this.strength.getValue(); 1484 } 1485 1486 /** 1487 * @param value Indicates the degree of conformance expectations associated with 1488 * this binding - that is, the degree to which the provided value 1489 * set must be adhered to in the instances. 1490 */ 1491 public OperationDefinitionParameterBindingComponent setStrength(BindingStrength value) { 1492 if (this.strength == null) 1493 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 1494 this.strength.setValue(value); 1495 return this; 1496 } 1497 1498 /** 1499 * @return {@link #valueSet} (Points to the value set or external definition 1500 * (e.g. implicit value set) that identifies the set of codes to be 1501 * used.). This is the underlying object with id, value and extensions. 1502 * The accessor "getValueSet" gives direct access to the value 1503 */ 1504 public CanonicalType getValueSetElement() { 1505 if (this.valueSet == null) 1506 if (Configuration.errorOnAutoCreate()) 1507 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.valueSet"); 1508 else if (Configuration.doAutoCreate()) 1509 this.valueSet = new CanonicalType(); // bb 1510 return this.valueSet; 1511 } 1512 1513 public boolean hasValueSetElement() { 1514 return this.valueSet != null && !this.valueSet.isEmpty(); 1515 } 1516 1517 public boolean hasValueSet() { 1518 return this.valueSet != null && !this.valueSet.isEmpty(); 1519 } 1520 1521 /** 1522 * @param value {@link #valueSet} (Points to the value set or external 1523 * definition (e.g. implicit value set) that identifies the set of 1524 * codes to be used.). This is the underlying object with id, value 1525 * and extensions. The accessor "getValueSet" gives direct access 1526 * to the value 1527 */ 1528 public OperationDefinitionParameterBindingComponent setValueSetElement(CanonicalType value) { 1529 this.valueSet = value; 1530 return this; 1531 } 1532 1533 /** 1534 * @return Points to the value set or external definition (e.g. implicit value 1535 * set) that identifies the set of codes to be used. 1536 */ 1537 public String getValueSet() { 1538 return this.valueSet == null ? null : this.valueSet.getValue(); 1539 } 1540 1541 /** 1542 * @param value Points to the value set or external definition (e.g. implicit 1543 * value set) that identifies the set of codes to be used. 1544 */ 1545 public OperationDefinitionParameterBindingComponent setValueSet(String value) { 1546 if (this.valueSet == null) 1547 this.valueSet = new CanonicalType(); 1548 this.valueSet.setValue(value); 1549 return this; 1550 } 1551 1552 protected void listChildren(List<Property> children) { 1553 super.listChildren(children); 1554 children.add(new Property("strength", "code", 1555 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 1556 0, 1, strength)); 1557 children.add(new Property("valueSet", "canonical(ValueSet)", 1558 "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 1559 0, 1, valueSet)); 1560 } 1561 1562 @Override 1563 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1564 switch (_hash) { 1565 case 1791316033: 1566 /* strength */ return new Property("strength", "code", 1567 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 1568 0, 1, strength); 1569 case -1410174671: 1570 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 1571 "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 1572 0, 1, valueSet); 1573 default: 1574 return super.getNamedProperty(_hash, _name, _checkValid); 1575 } 1576 1577 } 1578 1579 @Override 1580 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1581 switch (hash) { 1582 case 1791316033: 1583 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Enumeration<BindingStrength> 1584 case -1410174671: 1585 /* valueSet */ return this.valueSet == null ? new Base[0] : new Base[] { this.valueSet }; // CanonicalType 1586 default: 1587 return super.getProperty(hash, name, checkValid); 1588 } 1589 1590 } 1591 1592 @Override 1593 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1594 switch (hash) { 1595 case 1791316033: // strength 1596 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 1597 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1598 return value; 1599 case -1410174671: // valueSet 1600 this.valueSet = castToCanonical(value); // CanonicalType 1601 return value; 1602 default: 1603 return super.setProperty(hash, name, value); 1604 } 1605 1606 } 1607 1608 @Override 1609 public Base setProperty(String name, Base value) throws FHIRException { 1610 if (name.equals("strength")) { 1611 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 1612 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1613 } else if (name.equals("valueSet")) { 1614 this.valueSet = castToCanonical(value); // CanonicalType 1615 } else 1616 return super.setProperty(name, value); 1617 return value; 1618 } 1619 1620 @Override 1621 public void removeChild(String name, Base value) throws FHIRException { 1622 if (name.equals("strength")) { 1623 this.strength = null; 1624 } else if (name.equals("valueSet")) { 1625 this.valueSet = null; 1626 } else 1627 super.removeChild(name, value); 1628 1629 } 1630 1631 @Override 1632 public Base makeProperty(int hash, String name) throws FHIRException { 1633 switch (hash) { 1634 case 1791316033: 1635 return getStrengthElement(); 1636 case -1410174671: 1637 return getValueSetElement(); 1638 default: 1639 return super.makeProperty(hash, name); 1640 } 1641 1642 } 1643 1644 @Override 1645 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1646 switch (hash) { 1647 case 1791316033: 1648 /* strength */ return new String[] { "code" }; 1649 case -1410174671: 1650 /* valueSet */ return new String[] { "canonical" }; 1651 default: 1652 return super.getTypesForProperty(hash, name); 1653 } 1654 1655 } 1656 1657 @Override 1658 public Base addChild(String name) throws FHIRException { 1659 if (name.equals("strength")) { 1660 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.strength"); 1661 } else if (name.equals("valueSet")) { 1662 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.valueSet"); 1663 } else 1664 return super.addChild(name); 1665 } 1666 1667 public OperationDefinitionParameterBindingComponent copy() { 1668 OperationDefinitionParameterBindingComponent dst = new OperationDefinitionParameterBindingComponent(); 1669 copyValues(dst); 1670 return dst; 1671 } 1672 1673 public void copyValues(OperationDefinitionParameterBindingComponent dst) { 1674 super.copyValues(dst); 1675 dst.strength = strength == null ? null : strength.copy(); 1676 dst.valueSet = valueSet == null ? null : valueSet.copy(); 1677 } 1678 1679 @Override 1680 public boolean equalsDeep(Base other_) { 1681 if (!super.equalsDeep(other_)) 1682 return false; 1683 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1684 return false; 1685 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1686 return compareDeep(strength, o.strength, true) && compareDeep(valueSet, o.valueSet, true); 1687 } 1688 1689 @Override 1690 public boolean equalsShallow(Base other_) { 1691 if (!super.equalsShallow(other_)) 1692 return false; 1693 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1694 return false; 1695 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1696 return compareValues(strength, o.strength, true); 1697 } 1698 1699 public boolean isEmpty() { 1700 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, valueSet); 1701 } 1702 1703 public String fhirType() { 1704 return "OperationDefinition.parameter.binding"; 1705 1706 } 1707 1708 } 1709 1710 @Block() 1711 public static class OperationDefinitionParameterReferencedFromComponent extends BackboneElement 1712 implements IBaseBackboneElement { 1713 /** 1714 * The name of the parameter or dot-separated path of parameter names pointing 1715 * to the resource parameter that is expected to contain a reference to this 1716 * resource. 1717 */ 1718 @Child(name = "source", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1719 @Description(shortDefinition = "Referencing parameter", formalDefinition = "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.") 1720 protected StringType source; 1721 1722 /** 1723 * The id of the element in the referencing resource that is expected to resolve 1724 * to this resource. 1725 */ 1726 @Child(name = "sourceId", type = { 1727 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1728 @Description(shortDefinition = "Element id of reference", formalDefinition = "The id of the element in the referencing resource that is expected to resolve to this resource.") 1729 protected StringType sourceId; 1730 1731 private static final long serialVersionUID = -104239783L; 1732 1733 /** 1734 * Constructor 1735 */ 1736 public OperationDefinitionParameterReferencedFromComponent() { 1737 super(); 1738 } 1739 1740 /** 1741 * Constructor 1742 */ 1743 public OperationDefinitionParameterReferencedFromComponent(StringType source) { 1744 super(); 1745 this.source = source; 1746 } 1747 1748 /** 1749 * @return {@link #source} (The name of the parameter or dot-separated path of 1750 * parameter names pointing to the resource parameter that is expected 1751 * to contain a reference to this resource.). This is the underlying 1752 * object with id, value and extensions. The accessor "getSource" gives 1753 * direct access to the value 1754 */ 1755 public StringType getSourceElement() { 1756 if (this.source == null) 1757 if (Configuration.errorOnAutoCreate()) 1758 throw new Error("Attempt to auto-create OperationDefinitionParameterReferencedFromComponent.source"); 1759 else if (Configuration.doAutoCreate()) 1760 this.source = new StringType(); // bb 1761 return this.source; 1762 } 1763 1764 public boolean hasSourceElement() { 1765 return this.source != null && !this.source.isEmpty(); 1766 } 1767 1768 public boolean hasSource() { 1769 return this.source != null && !this.source.isEmpty(); 1770 } 1771 1772 /** 1773 * @param value {@link #source} (The name of the parameter or dot-separated path 1774 * of parameter names pointing to the resource parameter that is 1775 * expected to contain a reference to this resource.). This is the 1776 * underlying object with id, value and extensions. The accessor 1777 * "getSource" gives direct access to the value 1778 */ 1779 public OperationDefinitionParameterReferencedFromComponent setSourceElement(StringType value) { 1780 this.source = value; 1781 return this; 1782 } 1783 1784 /** 1785 * @return The name of the parameter or dot-separated path of parameter names 1786 * pointing to the resource parameter that is expected to contain a 1787 * reference to this resource. 1788 */ 1789 public String getSource() { 1790 return this.source == null ? null : this.source.getValue(); 1791 } 1792 1793 /** 1794 * @param value The name of the parameter or dot-separated path of parameter 1795 * names pointing to the resource parameter that is expected to 1796 * contain a reference to this resource. 1797 */ 1798 public OperationDefinitionParameterReferencedFromComponent setSource(String value) { 1799 if (this.source == null) 1800 this.source = new StringType(); 1801 this.source.setValue(value); 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #sourceId} (The id of the element in the referencing resource 1807 * that is expected to resolve to this resource.). This is the 1808 * underlying object with id, value and extensions. The accessor 1809 * "getSourceId" gives direct access to the value 1810 */ 1811 public StringType getSourceIdElement() { 1812 if (this.sourceId == null) 1813 if (Configuration.errorOnAutoCreate()) 1814 throw new Error("Attempt to auto-create OperationDefinitionParameterReferencedFromComponent.sourceId"); 1815 else if (Configuration.doAutoCreate()) 1816 this.sourceId = new StringType(); // bb 1817 return this.sourceId; 1818 } 1819 1820 public boolean hasSourceIdElement() { 1821 return this.sourceId != null && !this.sourceId.isEmpty(); 1822 } 1823 1824 public boolean hasSourceId() { 1825 return this.sourceId != null && !this.sourceId.isEmpty(); 1826 } 1827 1828 /** 1829 * @param value {@link #sourceId} (The id of the element in the referencing 1830 * resource that is expected to resolve to this resource.). This is 1831 * the underlying object with id, value and extensions. The 1832 * accessor "getSourceId" gives direct access to the value 1833 */ 1834 public OperationDefinitionParameterReferencedFromComponent setSourceIdElement(StringType value) { 1835 this.sourceId = value; 1836 return this; 1837 } 1838 1839 /** 1840 * @return The id of the element in the referencing resource that is expected to 1841 * resolve to this resource. 1842 */ 1843 public String getSourceId() { 1844 return this.sourceId == null ? null : this.sourceId.getValue(); 1845 } 1846 1847 /** 1848 * @param value The id of the element in the referencing resource that is 1849 * expected to resolve to this resource. 1850 */ 1851 public OperationDefinitionParameterReferencedFromComponent setSourceId(String value) { 1852 if (Utilities.noString(value)) 1853 this.sourceId = null; 1854 else { 1855 if (this.sourceId == null) 1856 this.sourceId = new StringType(); 1857 this.sourceId.setValue(value); 1858 } 1859 return this; 1860 } 1861 1862 protected void listChildren(List<Property> children) { 1863 super.listChildren(children); 1864 children.add(new Property("source", "string", 1865 "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.", 1866 0, 1, source)); 1867 children.add(new Property("sourceId", "string", 1868 "The id of the element in the referencing resource that is expected to resolve to this resource.", 0, 1, 1869 sourceId)); 1870 } 1871 1872 @Override 1873 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1874 switch (_hash) { 1875 case -896505829: 1876 /* source */ return new Property("source", "string", 1877 "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.", 1878 0, 1, source); 1879 case 1746327190: 1880 /* sourceId */ return new Property("sourceId", "string", 1881 "The id of the element in the referencing resource that is expected to resolve to this resource.", 0, 1, 1882 sourceId); 1883 default: 1884 return super.getNamedProperty(_hash, _name, _checkValid); 1885 } 1886 1887 } 1888 1889 @Override 1890 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1891 switch (hash) { 1892 case -896505829: 1893 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // StringType 1894 case 1746327190: 1895 /* sourceId */ return this.sourceId == null ? new Base[0] : new Base[] { this.sourceId }; // StringType 1896 default: 1897 return super.getProperty(hash, name, checkValid); 1898 } 1899 1900 } 1901 1902 @Override 1903 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1904 switch (hash) { 1905 case -896505829: // source 1906 this.source = castToString(value); // StringType 1907 return value; 1908 case 1746327190: // sourceId 1909 this.sourceId = castToString(value); // StringType 1910 return value; 1911 default: 1912 return super.setProperty(hash, name, value); 1913 } 1914 1915 } 1916 1917 @Override 1918 public Base setProperty(String name, Base value) throws FHIRException { 1919 if (name.equals("source")) { 1920 this.source = castToString(value); // StringType 1921 } else if (name.equals("sourceId")) { 1922 this.sourceId = castToString(value); // StringType 1923 } else 1924 return super.setProperty(name, value); 1925 return value; 1926 } 1927 1928 @Override 1929 public void removeChild(String name, Base value) throws FHIRException { 1930 if (name.equals("source")) { 1931 this.source = null; 1932 } else if (name.equals("sourceId")) { 1933 this.sourceId = null; 1934 } else 1935 super.removeChild(name, value); 1936 1937 } 1938 1939 @Override 1940 public Base makeProperty(int hash, String name) throws FHIRException { 1941 switch (hash) { 1942 case -896505829: 1943 return getSourceElement(); 1944 case 1746327190: 1945 return getSourceIdElement(); 1946 default: 1947 return super.makeProperty(hash, name); 1948 } 1949 1950 } 1951 1952 @Override 1953 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1954 switch (hash) { 1955 case -896505829: 1956 /* source */ return new String[] { "string" }; 1957 case 1746327190: 1958 /* sourceId */ return new String[] { "string" }; 1959 default: 1960 return super.getTypesForProperty(hash, name); 1961 } 1962 1963 } 1964 1965 @Override 1966 public Base addChild(String name) throws FHIRException { 1967 if (name.equals("source")) { 1968 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.source"); 1969 } else if (name.equals("sourceId")) { 1970 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.sourceId"); 1971 } else 1972 return super.addChild(name); 1973 } 1974 1975 public OperationDefinitionParameterReferencedFromComponent copy() { 1976 OperationDefinitionParameterReferencedFromComponent dst = new OperationDefinitionParameterReferencedFromComponent(); 1977 copyValues(dst); 1978 return dst; 1979 } 1980 1981 public void copyValues(OperationDefinitionParameterReferencedFromComponent dst) { 1982 super.copyValues(dst); 1983 dst.source = source == null ? null : source.copy(); 1984 dst.sourceId = sourceId == null ? null : sourceId.copy(); 1985 } 1986 1987 @Override 1988 public boolean equalsDeep(Base other_) { 1989 if (!super.equalsDeep(other_)) 1990 return false; 1991 if (!(other_ instanceof OperationDefinitionParameterReferencedFromComponent)) 1992 return false; 1993 OperationDefinitionParameterReferencedFromComponent o = (OperationDefinitionParameterReferencedFromComponent) other_; 1994 return compareDeep(source, o.source, true) && compareDeep(sourceId, o.sourceId, true); 1995 } 1996 1997 @Override 1998 public boolean equalsShallow(Base other_) { 1999 if (!super.equalsShallow(other_)) 2000 return false; 2001 if (!(other_ instanceof OperationDefinitionParameterReferencedFromComponent)) 2002 return false; 2003 OperationDefinitionParameterReferencedFromComponent o = (OperationDefinitionParameterReferencedFromComponent) other_; 2004 return compareValues(source, o.source, true) && compareValues(sourceId, o.sourceId, true); 2005 } 2006 2007 public boolean isEmpty() { 2008 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, sourceId); 2009 } 2010 2011 public String fhirType() { 2012 return "OperationDefinition.parameter.referencedFrom"; 2013 2014 } 2015 2016 } 2017 2018 @Block() 2019 public static class OperationDefinitionOverloadComponent extends BackboneElement implements IBaseBackboneElement { 2020 /** 2021 * Name of parameter to include in overload. 2022 */ 2023 @Child(name = "parameterName", type = { 2024 StringType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2025 @Description(shortDefinition = "Name of parameter to include in overload", formalDefinition = "Name of parameter to include in overload.") 2026 protected List<StringType> parameterName; 2027 2028 /** 2029 * Comments to go on overload. 2030 */ 2031 @Child(name = "comment", type = { 2032 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2033 @Description(shortDefinition = "Comments to go on overload", formalDefinition = "Comments to go on overload.") 2034 protected StringType comment; 2035 2036 private static final long serialVersionUID = -907948545L; 2037 2038 /** 2039 * Constructor 2040 */ 2041 public OperationDefinitionOverloadComponent() { 2042 super(); 2043 } 2044 2045 /** 2046 * @return {@link #parameterName} (Name of parameter to include in overload.) 2047 */ 2048 public List<StringType> getParameterName() { 2049 if (this.parameterName == null) 2050 this.parameterName = new ArrayList<StringType>(); 2051 return this.parameterName; 2052 } 2053 2054 /** 2055 * @return Returns a reference to <code>this</code> for easy method chaining 2056 */ 2057 public OperationDefinitionOverloadComponent setParameterName(List<StringType> theParameterName) { 2058 this.parameterName = theParameterName; 2059 return this; 2060 } 2061 2062 public boolean hasParameterName() { 2063 if (this.parameterName == null) 2064 return false; 2065 for (StringType item : this.parameterName) 2066 if (!item.isEmpty()) 2067 return true; 2068 return false; 2069 } 2070 2071 /** 2072 * @return {@link #parameterName} (Name of parameter to include in overload.) 2073 */ 2074 public StringType addParameterNameElement() {// 2 2075 StringType t = new StringType(); 2076 if (this.parameterName == null) 2077 this.parameterName = new ArrayList<StringType>(); 2078 this.parameterName.add(t); 2079 return t; 2080 } 2081 2082 /** 2083 * @param value {@link #parameterName} (Name of parameter to include in 2084 * overload.) 2085 */ 2086 public OperationDefinitionOverloadComponent addParameterName(String value) { // 1 2087 StringType t = new StringType(); 2088 t.setValue(value); 2089 if (this.parameterName == null) 2090 this.parameterName = new ArrayList<StringType>(); 2091 this.parameterName.add(t); 2092 return this; 2093 } 2094 2095 /** 2096 * @param value {@link #parameterName} (Name of parameter to include in 2097 * overload.) 2098 */ 2099 public boolean hasParameterName(String value) { 2100 if (this.parameterName == null) 2101 return false; 2102 for (StringType v : this.parameterName) 2103 if (v.getValue().equals(value)) // string 2104 return true; 2105 return false; 2106 } 2107 2108 /** 2109 * @return {@link #comment} (Comments to go on overload.). This is the 2110 * underlying object with id, value and extensions. The accessor 2111 * "getComment" gives direct access to the value 2112 */ 2113 public StringType getCommentElement() { 2114 if (this.comment == null) 2115 if (Configuration.errorOnAutoCreate()) 2116 throw new Error("Attempt to auto-create OperationDefinitionOverloadComponent.comment"); 2117 else if (Configuration.doAutoCreate()) 2118 this.comment = new StringType(); // bb 2119 return this.comment; 2120 } 2121 2122 public boolean hasCommentElement() { 2123 return this.comment != null && !this.comment.isEmpty(); 2124 } 2125 2126 public boolean hasComment() { 2127 return this.comment != null && !this.comment.isEmpty(); 2128 } 2129 2130 /** 2131 * @param value {@link #comment} (Comments to go on overload.). This is the 2132 * underlying object with id, value and extensions. The accessor 2133 * "getComment" gives direct access to the value 2134 */ 2135 public OperationDefinitionOverloadComponent setCommentElement(StringType value) { 2136 this.comment = value; 2137 return this; 2138 } 2139 2140 /** 2141 * @return Comments to go on overload. 2142 */ 2143 public String getComment() { 2144 return this.comment == null ? null : this.comment.getValue(); 2145 } 2146 2147 /** 2148 * @param value Comments to go on overload. 2149 */ 2150 public OperationDefinitionOverloadComponent setComment(String value) { 2151 if (Utilities.noString(value)) 2152 this.comment = null; 2153 else { 2154 if (this.comment == null) 2155 this.comment = new StringType(); 2156 this.comment.setValue(value); 2157 } 2158 return this; 2159 } 2160 2161 protected void listChildren(List<Property> children) { 2162 super.listChildren(children); 2163 children.add(new Property("parameterName", "string", "Name of parameter to include in overload.", 0, 2164 java.lang.Integer.MAX_VALUE, parameterName)); 2165 children.add(new Property("comment", "string", "Comments to go on overload.", 0, 1, comment)); 2166 } 2167 2168 @Override 2169 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2170 switch (_hash) { 2171 case -379607596: 2172 /* parameterName */ return new Property("parameterName", "string", "Name of parameter to include in overload.", 2173 0, java.lang.Integer.MAX_VALUE, parameterName); 2174 case 950398559: 2175 /* comment */ return new Property("comment", "string", "Comments to go on overload.", 0, 1, comment); 2176 default: 2177 return super.getNamedProperty(_hash, _name, _checkValid); 2178 } 2179 2180 } 2181 2182 @Override 2183 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2184 switch (hash) { 2185 case -379607596: 2186 /* parameterName */ return this.parameterName == null ? new Base[0] 2187 : this.parameterName.toArray(new Base[this.parameterName.size()]); // StringType 2188 case 950398559: 2189 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2190 default: 2191 return super.getProperty(hash, name, checkValid); 2192 } 2193 2194 } 2195 2196 @Override 2197 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2198 switch (hash) { 2199 case -379607596: // parameterName 2200 this.getParameterName().add(castToString(value)); // StringType 2201 return value; 2202 case 950398559: // comment 2203 this.comment = castToString(value); // StringType 2204 return value; 2205 default: 2206 return super.setProperty(hash, name, value); 2207 } 2208 2209 } 2210 2211 @Override 2212 public Base setProperty(String name, Base value) throws FHIRException { 2213 if (name.equals("parameterName")) { 2214 this.getParameterName().add(castToString(value)); 2215 } else if (name.equals("comment")) { 2216 this.comment = castToString(value); // StringType 2217 } else 2218 return super.setProperty(name, value); 2219 return value; 2220 } 2221 2222 @Override 2223 public void removeChild(String name, Base value) throws FHIRException { 2224 if (name.equals("parameterName")) { 2225 this.getParameterName().remove(castToString(value)); 2226 } else if (name.equals("comment")) { 2227 this.comment = null; 2228 } else 2229 super.removeChild(name, value); 2230 2231 } 2232 2233 @Override 2234 public Base makeProperty(int hash, String name) throws FHIRException { 2235 switch (hash) { 2236 case -379607596: 2237 return addParameterNameElement(); 2238 case 950398559: 2239 return getCommentElement(); 2240 default: 2241 return super.makeProperty(hash, name); 2242 } 2243 2244 } 2245 2246 @Override 2247 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2248 switch (hash) { 2249 case -379607596: 2250 /* parameterName */ return new String[] { "string" }; 2251 case 950398559: 2252 /* comment */ return new String[] { "string" }; 2253 default: 2254 return super.getTypesForProperty(hash, name); 2255 } 2256 2257 } 2258 2259 @Override 2260 public Base addChild(String name) throws FHIRException { 2261 if (name.equals("parameterName")) { 2262 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameterName"); 2263 } else if (name.equals("comment")) { 2264 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 2265 } else 2266 return super.addChild(name); 2267 } 2268 2269 public OperationDefinitionOverloadComponent copy() { 2270 OperationDefinitionOverloadComponent dst = new OperationDefinitionOverloadComponent(); 2271 copyValues(dst); 2272 return dst; 2273 } 2274 2275 public void copyValues(OperationDefinitionOverloadComponent dst) { 2276 super.copyValues(dst); 2277 if (parameterName != null) { 2278 dst.parameterName = new ArrayList<StringType>(); 2279 for (StringType i : parameterName) 2280 dst.parameterName.add(i.copy()); 2281 } 2282 ; 2283 dst.comment = comment == null ? null : comment.copy(); 2284 } 2285 2286 @Override 2287 public boolean equalsDeep(Base other_) { 2288 if (!super.equalsDeep(other_)) 2289 return false; 2290 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 2291 return false; 2292 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 2293 return compareDeep(parameterName, o.parameterName, true) && compareDeep(comment, o.comment, true); 2294 } 2295 2296 @Override 2297 public boolean equalsShallow(Base other_) { 2298 if (!super.equalsShallow(other_)) 2299 return false; 2300 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 2301 return false; 2302 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 2303 return compareValues(parameterName, o.parameterName, true) && compareValues(comment, o.comment, true); 2304 } 2305 2306 public boolean isEmpty() { 2307 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameterName, comment); 2308 } 2309 2310 public String fhirType() { 2311 return "OperationDefinition.overload"; 2312 2313 } 2314 2315 } 2316 2317 /** 2318 * Whether this is an operation or a named query. 2319 */ 2320 @Child(name = "kind", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2321 @Description(shortDefinition = "operation | query", formalDefinition = "Whether this is an operation or a named query.") 2322 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/operation-kind") 2323 protected Enumeration<OperationKind> kind; 2324 2325 /** 2326 * Explanation of why this operation definition is needed and why it has been 2327 * designed as it has. 2328 */ 2329 @Child(name = "purpose", type = { 2330 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2331 @Description(shortDefinition = "Why this operation definition is defined", formalDefinition = "Explanation of why this operation definition is needed and why it has been designed as it has.") 2332 protected MarkdownType purpose; 2333 2334 /** 2335 * Whether the operation affects state. Side effects such as producing audit 2336 * trail entries do not count as 'affecting state'. 2337 */ 2338 @Child(name = "affectsState", type = { 2339 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2340 @Description(shortDefinition = "Whether content is changed by the operation", formalDefinition = "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.") 2341 protected BooleanType affectsState; 2342 2343 /** 2344 * The name used to invoke the operation. 2345 */ 2346 @Child(name = "code", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2347 @Description(shortDefinition = "Name used to invoke the operation", formalDefinition = "The name used to invoke the operation.") 2348 protected CodeType code; 2349 2350 /** 2351 * Additional information about how to use this operation or named query. 2352 */ 2353 @Child(name = "comment", type = { 2354 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2355 @Description(shortDefinition = "Additional information about use", formalDefinition = "Additional information about how to use this operation or named query.") 2356 protected MarkdownType comment; 2357 2358 /** 2359 * Indicates that this operation definition is a constraining profile on the 2360 * base. 2361 */ 2362 @Child(name = "base", type = { CanonicalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2363 @Description(shortDefinition = "Marks this as a profile of the base", formalDefinition = "Indicates that this operation definition is a constraining profile on the base.") 2364 protected CanonicalType base; 2365 2366 /** 2367 * The types on which this operation can be executed. 2368 */ 2369 @Child(name = "resource", type = { 2370 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2371 @Description(shortDefinition = "Types this operation applies to", formalDefinition = "The types on which this operation can be executed.") 2372 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 2373 protected List<CodeType> resource; 2374 2375 /** 2376 * Indicates whether this operation or named query can be invoked at the system 2377 * level (e.g. without needing to choose a resource type for the context). 2378 */ 2379 @Child(name = "system", type = { BooleanType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 2380 @Description(shortDefinition = "Invoke at the system level?", formalDefinition = "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).") 2381 protected BooleanType system; 2382 2383 /** 2384 * Indicates whether this operation or named query can be invoked at the 2385 * resource type level for any given resource type level (e.g. without needing 2386 * to choose a specific resource id for the context). 2387 */ 2388 @Child(name = "type", type = { BooleanType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 2389 @Description(shortDefinition = "Invoke at the type level?", formalDefinition = "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).") 2390 protected BooleanType type; 2391 2392 /** 2393 * Indicates whether this operation can be invoked on a particular instance of 2394 * one of the given types. 2395 */ 2396 @Child(name = "instance", type = { BooleanType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 2397 @Description(shortDefinition = "Invoke on an instance?", formalDefinition = "Indicates whether this operation can be invoked on a particular instance of one of the given types.") 2398 protected BooleanType instance; 2399 2400 /** 2401 * Additional validation information for the in parameters - a single profile 2402 * that covers all the parameters. The profile is a constraint on the parameters 2403 * resource as a whole. 2404 */ 2405 @Child(name = "inputProfile", type = { 2406 CanonicalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2407 @Description(shortDefinition = "Validation information for in parameters", formalDefinition = "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.") 2408 protected CanonicalType inputProfile; 2409 2410 /** 2411 * Additional validation information for the out parameters - a single profile 2412 * that covers all the parameters. The profile is a constraint on the parameters 2413 * resource. 2414 */ 2415 @Child(name = "outputProfile", type = { 2416 CanonicalType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 2417 @Description(shortDefinition = "Validation information for out parameters", formalDefinition = "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.") 2418 protected CanonicalType outputProfile; 2419 2420 /** 2421 * The parameters for the operation/query. 2422 */ 2423 @Child(name = "parameter", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2424 @Description(shortDefinition = "Parameters for the operation/query", formalDefinition = "The parameters for the operation/query.") 2425 protected List<OperationDefinitionParameterComponent> parameter; 2426 2427 /** 2428 * Defines an appropriate combination of parameters to use when invoking this 2429 * operation, to help code generators when generating overloaded parameter sets 2430 * for this operation. 2431 */ 2432 @Child(name = "overload", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2433 @Description(shortDefinition = "Define overloaded variants for when generating code", formalDefinition = "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.") 2434 protected List<OperationDefinitionOverloadComponent> overload; 2435 2436 private static final long serialVersionUID = 149113671L; 2437 2438 /** 2439 * Constructor 2440 */ 2441 public OperationDefinition() { 2442 super(); 2443 } 2444 2445 /** 2446 * Constructor 2447 */ 2448 public OperationDefinition(StringType name, Enumeration<PublicationStatus> status, Enumeration<OperationKind> kind, 2449 CodeType code, BooleanType system, BooleanType type, BooleanType instance) { 2450 super(); 2451 this.name = name; 2452 this.status = status; 2453 this.kind = kind; 2454 this.code = code; 2455 this.system = system; 2456 this.type = type; 2457 this.instance = instance; 2458 } 2459 2460 /** 2461 * @return {@link #url} (An absolute URI that is used to identify this operation 2462 * definition when it is referenced in a specification, model, design or 2463 * an instance; also called its canonical identifier. This SHOULD be 2464 * globally unique and SHOULD be a literal address at which at which an 2465 * authoritative instance of this operation definition is (or will be) 2466 * published. This URL can be the target of a canonical reference. It 2467 * SHALL remain the same when the operation definition is stored on 2468 * different servers.). This is the underlying object with id, value and 2469 * extensions. The accessor "getUrl" gives direct access to the value 2470 */ 2471 public UriType getUrlElement() { 2472 if (this.url == null) 2473 if (Configuration.errorOnAutoCreate()) 2474 throw new Error("Attempt to auto-create OperationDefinition.url"); 2475 else if (Configuration.doAutoCreate()) 2476 this.url = new UriType(); // bb 2477 return this.url; 2478 } 2479 2480 public boolean hasUrlElement() { 2481 return this.url != null && !this.url.isEmpty(); 2482 } 2483 2484 public boolean hasUrl() { 2485 return this.url != null && !this.url.isEmpty(); 2486 } 2487 2488 /** 2489 * @param value {@link #url} (An absolute URI that is used to identify this 2490 * operation definition when it is referenced in a specification, 2491 * model, design or an instance; also called its canonical 2492 * identifier. This SHOULD be globally unique and SHOULD be a 2493 * literal address at which at which an authoritative instance of 2494 * this operation definition is (or will be) published. This URL 2495 * can be the target of a canonical reference. It SHALL remain the 2496 * same when the operation definition is stored on different 2497 * servers.). This is the underlying object with id, value and 2498 * extensions. The accessor "getUrl" gives direct access to the 2499 * value 2500 */ 2501 public OperationDefinition setUrlElement(UriType value) { 2502 this.url = value; 2503 return this; 2504 } 2505 2506 /** 2507 * @return An absolute URI that is used to identify this operation definition 2508 * when it is referenced in a specification, model, design or an 2509 * instance; also called its canonical identifier. This SHOULD be 2510 * globally unique and SHOULD be a literal address at which at which an 2511 * authoritative instance of this operation definition is (or will be) 2512 * published. This URL can be the target of a canonical reference. It 2513 * SHALL remain the same when the operation definition is stored on 2514 * different servers. 2515 */ 2516 public String getUrl() { 2517 return this.url == null ? null : this.url.getValue(); 2518 } 2519 2520 /** 2521 * @param value An absolute URI that is used to identify this operation 2522 * definition when it is referenced in a specification, model, 2523 * design or an instance; also called its canonical identifier. 2524 * This SHOULD be globally unique and SHOULD be a literal address 2525 * at which at which an authoritative instance of this operation 2526 * definition is (or will be) published. This URL can be the target 2527 * of a canonical reference. It SHALL remain the same when the 2528 * operation definition is stored on different servers. 2529 */ 2530 public OperationDefinition setUrl(String value) { 2531 if (Utilities.noString(value)) 2532 this.url = null; 2533 else { 2534 if (this.url == null) 2535 this.url = new UriType(); 2536 this.url.setValue(value); 2537 } 2538 return this; 2539 } 2540 2541 /** 2542 * @return {@link #version} (The identifier that is used to identify this 2543 * version of the operation definition when it is referenced in a 2544 * specification, model, design or instance. This is an arbitrary value 2545 * managed by the operation definition author and is not expected to be 2546 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 2547 * if a managed version is not available. There is also no expectation 2548 * that versions can be placed in a lexicographical sequence.). This is 2549 * the underlying object with id, value and extensions. The accessor 2550 * "getVersion" gives direct access to the value 2551 */ 2552 public StringType getVersionElement() { 2553 if (this.version == null) 2554 if (Configuration.errorOnAutoCreate()) 2555 throw new Error("Attempt to auto-create OperationDefinition.version"); 2556 else if (Configuration.doAutoCreate()) 2557 this.version = new StringType(); // bb 2558 return this.version; 2559 } 2560 2561 public boolean hasVersionElement() { 2562 return this.version != null && !this.version.isEmpty(); 2563 } 2564 2565 public boolean hasVersion() { 2566 return this.version != null && !this.version.isEmpty(); 2567 } 2568 2569 /** 2570 * @param value {@link #version} (The identifier that is used to identify this 2571 * version of the operation definition when it is referenced in a 2572 * specification, model, design or instance. This is an arbitrary 2573 * value managed by the operation definition author and is not 2574 * expected to be globally unique. For example, it might be a 2575 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2576 * There is also no expectation that versions can be placed in a 2577 * lexicographical sequence.). This is the underlying object with 2578 * id, value and extensions. The accessor "getVersion" gives direct 2579 * access to the value 2580 */ 2581 public OperationDefinition setVersionElement(StringType value) { 2582 this.version = value; 2583 return this; 2584 } 2585 2586 /** 2587 * @return The identifier that is used to identify this version of the operation 2588 * definition when it is referenced in a specification, model, design or 2589 * instance. This is an arbitrary value managed by the operation 2590 * definition author and is not expected to be globally unique. For 2591 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 2592 * is not available. There is also no expectation that versions can be 2593 * placed in a lexicographical sequence. 2594 */ 2595 public String getVersion() { 2596 return this.version == null ? null : this.version.getValue(); 2597 } 2598 2599 /** 2600 * @param value The identifier that is used to identify this version of the 2601 * operation definition when it is referenced in a specification, 2602 * model, design or instance. This is an arbitrary value managed by 2603 * the operation definition author and is not expected to be 2604 * globally unique. For example, it might be a timestamp (e.g. 2605 * yyyymmdd) if a managed version is not available. There is also 2606 * no expectation that versions can be placed in a lexicographical 2607 * sequence. 2608 */ 2609 public OperationDefinition setVersion(String value) { 2610 if (Utilities.noString(value)) 2611 this.version = null; 2612 else { 2613 if (this.version == null) 2614 this.version = new StringType(); 2615 this.version.setValue(value); 2616 } 2617 return this; 2618 } 2619 2620 /** 2621 * @return {@link #name} (A natural language name identifying the operation 2622 * definition. This name should be usable as an identifier for the 2623 * module by machine processing applications such as code generation.). 2624 * This is the underlying object with id, value and extensions. The 2625 * accessor "getName" gives direct access to the value 2626 */ 2627 public StringType getNameElement() { 2628 if (this.name == null) 2629 if (Configuration.errorOnAutoCreate()) 2630 throw new Error("Attempt to auto-create OperationDefinition.name"); 2631 else if (Configuration.doAutoCreate()) 2632 this.name = new StringType(); // bb 2633 return this.name; 2634 } 2635 2636 public boolean hasNameElement() { 2637 return this.name != null && !this.name.isEmpty(); 2638 } 2639 2640 public boolean hasName() { 2641 return this.name != null && !this.name.isEmpty(); 2642 } 2643 2644 /** 2645 * @param value {@link #name} (A natural language name identifying the operation 2646 * definition. This name should be usable as an identifier for the 2647 * module by machine processing applications such as code 2648 * generation.). This is the underlying object with id, value and 2649 * extensions. The accessor "getName" gives direct access to the 2650 * value 2651 */ 2652 public OperationDefinition setNameElement(StringType value) { 2653 this.name = value; 2654 return this; 2655 } 2656 2657 /** 2658 * @return A natural language name identifying the operation definition. This 2659 * name should be usable as an identifier for the module by machine 2660 * processing applications such as code generation. 2661 */ 2662 public String getName() { 2663 return this.name == null ? null : this.name.getValue(); 2664 } 2665 2666 /** 2667 * @param value A natural language name identifying the operation definition. 2668 * This name should be usable as an identifier for the module by 2669 * machine processing applications such as code generation. 2670 */ 2671 public OperationDefinition setName(String value) { 2672 if (this.name == null) 2673 this.name = new StringType(); 2674 this.name.setValue(value); 2675 return this; 2676 } 2677 2678 /** 2679 * @return {@link #title} (A short, descriptive, user-friendly title for the 2680 * operation definition.). This is the underlying object with id, value 2681 * and extensions. The accessor "getTitle" gives direct access to the 2682 * value 2683 */ 2684 public StringType getTitleElement() { 2685 if (this.title == null) 2686 if (Configuration.errorOnAutoCreate()) 2687 throw new Error("Attempt to auto-create OperationDefinition.title"); 2688 else if (Configuration.doAutoCreate()) 2689 this.title = new StringType(); // bb 2690 return this.title; 2691 } 2692 2693 public boolean hasTitleElement() { 2694 return this.title != null && !this.title.isEmpty(); 2695 } 2696 2697 public boolean hasTitle() { 2698 return this.title != null && !this.title.isEmpty(); 2699 } 2700 2701 /** 2702 * @param value {@link #title} (A short, descriptive, user-friendly title for 2703 * the operation definition.). This is the underlying object with 2704 * id, value and extensions. The accessor "getTitle" gives direct 2705 * access to the value 2706 */ 2707 public OperationDefinition setTitleElement(StringType value) { 2708 this.title = value; 2709 return this; 2710 } 2711 2712 /** 2713 * @return A short, descriptive, user-friendly title for the operation 2714 * definition. 2715 */ 2716 public String getTitle() { 2717 return this.title == null ? null : this.title.getValue(); 2718 } 2719 2720 /** 2721 * @param value A short, descriptive, user-friendly title for the operation 2722 * definition. 2723 */ 2724 public OperationDefinition setTitle(String value) { 2725 if (Utilities.noString(value)) 2726 this.title = null; 2727 else { 2728 if (this.title == null) 2729 this.title = new StringType(); 2730 this.title.setValue(value); 2731 } 2732 return this; 2733 } 2734 2735 /** 2736 * @return {@link #status} (The status of this operation definition. Enables 2737 * tracking the life-cycle of the content.). This is the underlying 2738 * object with id, value and extensions. The accessor "getStatus" gives 2739 * direct access to the value 2740 */ 2741 public Enumeration<PublicationStatus> getStatusElement() { 2742 if (this.status == null) 2743 if (Configuration.errorOnAutoCreate()) 2744 throw new Error("Attempt to auto-create OperationDefinition.status"); 2745 else if (Configuration.doAutoCreate()) 2746 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2747 return this.status; 2748 } 2749 2750 public boolean hasStatusElement() { 2751 return this.status != null && !this.status.isEmpty(); 2752 } 2753 2754 public boolean hasStatus() { 2755 return this.status != null && !this.status.isEmpty(); 2756 } 2757 2758 /** 2759 * @param value {@link #status} (The status of this operation definition. 2760 * Enables tracking the life-cycle of the content.). This is the 2761 * underlying object with id, value and extensions. The accessor 2762 * "getStatus" gives direct access to the value 2763 */ 2764 public OperationDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2765 this.status = value; 2766 return this; 2767 } 2768 2769 /** 2770 * @return The status of this operation definition. Enables tracking the 2771 * life-cycle of the content. 2772 */ 2773 public PublicationStatus getStatus() { 2774 return this.status == null ? null : this.status.getValue(); 2775 } 2776 2777 /** 2778 * @param value The status of this operation definition. Enables tracking the 2779 * life-cycle of the content. 2780 */ 2781 public OperationDefinition setStatus(PublicationStatus value) { 2782 if (this.status == null) 2783 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2784 this.status.setValue(value); 2785 return this; 2786 } 2787 2788 /** 2789 * @return {@link #kind} (Whether this is an operation or a named query.). This 2790 * is the underlying object with id, value and extensions. The accessor 2791 * "getKind" gives direct access to the value 2792 */ 2793 public Enumeration<OperationKind> getKindElement() { 2794 if (this.kind == null) 2795 if (Configuration.errorOnAutoCreate()) 2796 throw new Error("Attempt to auto-create OperationDefinition.kind"); 2797 else if (Configuration.doAutoCreate()) 2798 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); // bb 2799 return this.kind; 2800 } 2801 2802 public boolean hasKindElement() { 2803 return this.kind != null && !this.kind.isEmpty(); 2804 } 2805 2806 public boolean hasKind() { 2807 return this.kind != null && !this.kind.isEmpty(); 2808 } 2809 2810 /** 2811 * @param value {@link #kind} (Whether this is an operation or a named query.). 2812 * This is the underlying object with id, value and extensions. The 2813 * accessor "getKind" gives direct access to the value 2814 */ 2815 public OperationDefinition setKindElement(Enumeration<OperationKind> value) { 2816 this.kind = value; 2817 return this; 2818 } 2819 2820 /** 2821 * @return Whether this is an operation or a named query. 2822 */ 2823 public OperationKind getKind() { 2824 return this.kind == null ? null : this.kind.getValue(); 2825 } 2826 2827 /** 2828 * @param value Whether this is an operation or a named query. 2829 */ 2830 public OperationDefinition setKind(OperationKind value) { 2831 if (this.kind == null) 2832 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); 2833 this.kind.setValue(value); 2834 return this; 2835 } 2836 2837 /** 2838 * @return {@link #experimental} (A Boolean value to indicate that this 2839 * operation definition is authored for testing purposes (or 2840 * education/evaluation/marketing) and is not intended to be used for 2841 * genuine usage.). This is the underlying object with id, value and 2842 * extensions. The accessor "getExperimental" gives direct access to the 2843 * value 2844 */ 2845 public BooleanType getExperimentalElement() { 2846 if (this.experimental == null) 2847 if (Configuration.errorOnAutoCreate()) 2848 throw new Error("Attempt to auto-create OperationDefinition.experimental"); 2849 else if (Configuration.doAutoCreate()) 2850 this.experimental = new BooleanType(); // bb 2851 return this.experimental; 2852 } 2853 2854 public boolean hasExperimentalElement() { 2855 return this.experimental != null && !this.experimental.isEmpty(); 2856 } 2857 2858 public boolean hasExperimental() { 2859 return this.experimental != null && !this.experimental.isEmpty(); 2860 } 2861 2862 /** 2863 * @param value {@link #experimental} (A Boolean value to indicate that this 2864 * operation definition is authored for testing purposes (or 2865 * education/evaluation/marketing) and is not intended to be used 2866 * for genuine usage.). This is the underlying object with id, 2867 * value and extensions. The accessor "getExperimental" gives 2868 * direct access to the value 2869 */ 2870 public OperationDefinition setExperimentalElement(BooleanType value) { 2871 this.experimental = value; 2872 return this; 2873 } 2874 2875 /** 2876 * @return A Boolean value to indicate that this operation definition is 2877 * authored for testing purposes (or education/evaluation/marketing) and 2878 * is not intended to be used for genuine usage. 2879 */ 2880 public boolean getExperimental() { 2881 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2882 } 2883 2884 /** 2885 * @param value A Boolean value to indicate that this operation definition is 2886 * authored for testing purposes (or 2887 * education/evaluation/marketing) and is not intended to be used 2888 * for genuine usage. 2889 */ 2890 public OperationDefinition setExperimental(boolean value) { 2891 if (this.experimental == null) 2892 this.experimental = new BooleanType(); 2893 this.experimental.setValue(value); 2894 return this; 2895 } 2896 2897 /** 2898 * @return {@link #date} (The date (and optionally time) when the operation 2899 * definition was published. The date must change when the business 2900 * version changes and it must change if the status code changes. In 2901 * addition, it should change when the substantive content of the 2902 * operation definition changes.). This is the underlying object with 2903 * id, value and extensions. The accessor "getDate" gives direct access 2904 * to the value 2905 */ 2906 public DateTimeType getDateElement() { 2907 if (this.date == null) 2908 if (Configuration.errorOnAutoCreate()) 2909 throw new Error("Attempt to auto-create OperationDefinition.date"); 2910 else if (Configuration.doAutoCreate()) 2911 this.date = new DateTimeType(); // bb 2912 return this.date; 2913 } 2914 2915 public boolean hasDateElement() { 2916 return this.date != null && !this.date.isEmpty(); 2917 } 2918 2919 public boolean hasDate() { 2920 return this.date != null && !this.date.isEmpty(); 2921 } 2922 2923 /** 2924 * @param value {@link #date} (The date (and optionally time) when the operation 2925 * definition was published. The date must change when the business 2926 * version changes and it must change if the status code changes. 2927 * In addition, it should change when the substantive content of 2928 * the operation definition changes.). This is the underlying 2929 * object with id, value and extensions. The accessor "getDate" 2930 * gives direct access to the value 2931 */ 2932 public OperationDefinition setDateElement(DateTimeType value) { 2933 this.date = value; 2934 return this; 2935 } 2936 2937 /** 2938 * @return The date (and optionally time) when the operation definition was 2939 * published. The date must change when the business version changes and 2940 * it must change if the status code changes. In addition, it should 2941 * change when the substantive content of the operation definition 2942 * changes. 2943 */ 2944 public Date getDate() { 2945 return this.date == null ? null : this.date.getValue(); 2946 } 2947 2948 /** 2949 * @param value The date (and optionally time) when the operation definition was 2950 * published. The date must change when the business version 2951 * changes and it must change if the status code changes. In 2952 * addition, it should change when the substantive content of the 2953 * operation definition changes. 2954 */ 2955 public OperationDefinition setDate(Date value) { 2956 if (value == null) 2957 this.date = null; 2958 else { 2959 if (this.date == null) 2960 this.date = new DateTimeType(); 2961 this.date.setValue(value); 2962 } 2963 return this; 2964 } 2965 2966 /** 2967 * @return {@link #publisher} (The name of the organization or individual that 2968 * published the operation definition.). This is the underlying object 2969 * with id, value and extensions. The accessor "getPublisher" gives 2970 * direct access to the value 2971 */ 2972 public StringType getPublisherElement() { 2973 if (this.publisher == null) 2974 if (Configuration.errorOnAutoCreate()) 2975 throw new Error("Attempt to auto-create OperationDefinition.publisher"); 2976 else if (Configuration.doAutoCreate()) 2977 this.publisher = new StringType(); // bb 2978 return this.publisher; 2979 } 2980 2981 public boolean hasPublisherElement() { 2982 return this.publisher != null && !this.publisher.isEmpty(); 2983 } 2984 2985 public boolean hasPublisher() { 2986 return this.publisher != null && !this.publisher.isEmpty(); 2987 } 2988 2989 /** 2990 * @param value {@link #publisher} (The name of the organization or individual 2991 * that published the operation definition.). This is the 2992 * underlying object with id, value and extensions. The accessor 2993 * "getPublisher" gives direct access to the value 2994 */ 2995 public OperationDefinition setPublisherElement(StringType value) { 2996 this.publisher = value; 2997 return this; 2998 } 2999 3000 /** 3001 * @return The name of the organization or individual that published the 3002 * operation definition. 3003 */ 3004 public String getPublisher() { 3005 return this.publisher == null ? null : this.publisher.getValue(); 3006 } 3007 3008 /** 3009 * @param value The name of the organization or individual that published the 3010 * operation definition. 3011 */ 3012 public OperationDefinition setPublisher(String value) { 3013 if (Utilities.noString(value)) 3014 this.publisher = null; 3015 else { 3016 if (this.publisher == null) 3017 this.publisher = new StringType(); 3018 this.publisher.setValue(value); 3019 } 3020 return this; 3021 } 3022 3023 /** 3024 * @return {@link #contact} (Contact details to assist a user in finding and 3025 * communicating with the publisher.) 3026 */ 3027 public List<ContactDetail> getContact() { 3028 if (this.contact == null) 3029 this.contact = new ArrayList<ContactDetail>(); 3030 return this.contact; 3031 } 3032 3033 /** 3034 * @return Returns a reference to <code>this</code> for easy method chaining 3035 */ 3036 public OperationDefinition setContact(List<ContactDetail> theContact) { 3037 this.contact = theContact; 3038 return this; 3039 } 3040 3041 public boolean hasContact() { 3042 if (this.contact == null) 3043 return false; 3044 for (ContactDetail item : this.contact) 3045 if (!item.isEmpty()) 3046 return true; 3047 return false; 3048 } 3049 3050 public ContactDetail addContact() { // 3 3051 ContactDetail t = new ContactDetail(); 3052 if (this.contact == null) 3053 this.contact = new ArrayList<ContactDetail>(); 3054 this.contact.add(t); 3055 return t; 3056 } 3057 3058 public OperationDefinition addContact(ContactDetail t) { // 3 3059 if (t == null) 3060 return this; 3061 if (this.contact == null) 3062 this.contact = new ArrayList<ContactDetail>(); 3063 this.contact.add(t); 3064 return this; 3065 } 3066 3067 /** 3068 * @return The first repetition of repeating field {@link #contact}, creating it 3069 * if it does not already exist 3070 */ 3071 public ContactDetail getContactFirstRep() { 3072 if (getContact().isEmpty()) { 3073 addContact(); 3074 } 3075 return getContact().get(0); 3076 } 3077 3078 /** 3079 * @return {@link #description} (A free text natural language description of the 3080 * operation definition from a consumer's perspective.). This is the 3081 * underlying object with id, value and extensions. The accessor 3082 * "getDescription" gives direct access to the value 3083 */ 3084 public MarkdownType getDescriptionElement() { 3085 if (this.description == null) 3086 if (Configuration.errorOnAutoCreate()) 3087 throw new Error("Attempt to auto-create OperationDefinition.description"); 3088 else if (Configuration.doAutoCreate()) 3089 this.description = new MarkdownType(); // bb 3090 return this.description; 3091 } 3092 3093 public boolean hasDescriptionElement() { 3094 return this.description != null && !this.description.isEmpty(); 3095 } 3096 3097 public boolean hasDescription() { 3098 return this.description != null && !this.description.isEmpty(); 3099 } 3100 3101 /** 3102 * @param value {@link #description} (A free text natural language description 3103 * of the operation definition from a consumer's perspective.). 3104 * This is the underlying object with id, value and extensions. The 3105 * accessor "getDescription" gives direct access to the value 3106 */ 3107 public OperationDefinition setDescriptionElement(MarkdownType value) { 3108 this.description = value; 3109 return this; 3110 } 3111 3112 /** 3113 * @return A free text natural language description of the operation definition 3114 * from a consumer's perspective. 3115 */ 3116 public String getDescription() { 3117 return this.description == null ? null : this.description.getValue(); 3118 } 3119 3120 /** 3121 * @param value A free text natural language description of the operation 3122 * definition from a consumer's perspective. 3123 */ 3124 public OperationDefinition setDescription(String value) { 3125 if (value == null) 3126 this.description = null; 3127 else { 3128 if (this.description == null) 3129 this.description = new MarkdownType(); 3130 this.description.setValue(value); 3131 } 3132 return this; 3133 } 3134 3135 /** 3136 * @return {@link #useContext} (The content was developed with a focus and 3137 * intent of supporting the contexts that are listed. These contexts may 3138 * be general categories (gender, age, ...) or may be references to 3139 * specific programs (insurance plans, studies, ...) and may be used to 3140 * assist with indexing and searching for appropriate operation 3141 * definition instances.) 3142 */ 3143 public List<UsageContext> getUseContext() { 3144 if (this.useContext == null) 3145 this.useContext = new ArrayList<UsageContext>(); 3146 return this.useContext; 3147 } 3148 3149 /** 3150 * @return Returns a reference to <code>this</code> for easy method chaining 3151 */ 3152 public OperationDefinition setUseContext(List<UsageContext> theUseContext) { 3153 this.useContext = theUseContext; 3154 return this; 3155 } 3156 3157 public boolean hasUseContext() { 3158 if (this.useContext == null) 3159 return false; 3160 for (UsageContext item : this.useContext) 3161 if (!item.isEmpty()) 3162 return true; 3163 return false; 3164 } 3165 3166 public UsageContext addUseContext() { // 3 3167 UsageContext t = new UsageContext(); 3168 if (this.useContext == null) 3169 this.useContext = new ArrayList<UsageContext>(); 3170 this.useContext.add(t); 3171 return t; 3172 } 3173 3174 public OperationDefinition addUseContext(UsageContext t) { // 3 3175 if (t == null) 3176 return this; 3177 if (this.useContext == null) 3178 this.useContext = new ArrayList<UsageContext>(); 3179 this.useContext.add(t); 3180 return this; 3181 } 3182 3183 /** 3184 * @return The first repetition of repeating field {@link #useContext}, creating 3185 * it if it does not already exist 3186 */ 3187 public UsageContext getUseContextFirstRep() { 3188 if (getUseContext().isEmpty()) { 3189 addUseContext(); 3190 } 3191 return getUseContext().get(0); 3192 } 3193 3194 /** 3195 * @return {@link #jurisdiction} (A legal or geographic region in which the 3196 * operation definition is intended to be used.) 3197 */ 3198 public List<CodeableConcept> getJurisdiction() { 3199 if (this.jurisdiction == null) 3200 this.jurisdiction = new ArrayList<CodeableConcept>(); 3201 return this.jurisdiction; 3202 } 3203 3204 /** 3205 * @return Returns a reference to <code>this</code> for easy method chaining 3206 */ 3207 public OperationDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3208 this.jurisdiction = theJurisdiction; 3209 return this; 3210 } 3211 3212 public boolean hasJurisdiction() { 3213 if (this.jurisdiction == null) 3214 return false; 3215 for (CodeableConcept item : this.jurisdiction) 3216 if (!item.isEmpty()) 3217 return true; 3218 return false; 3219 } 3220 3221 public CodeableConcept addJurisdiction() { // 3 3222 CodeableConcept t = new CodeableConcept(); 3223 if (this.jurisdiction == null) 3224 this.jurisdiction = new ArrayList<CodeableConcept>(); 3225 this.jurisdiction.add(t); 3226 return t; 3227 } 3228 3229 public OperationDefinition addJurisdiction(CodeableConcept t) { // 3 3230 if (t == null) 3231 return this; 3232 if (this.jurisdiction == null) 3233 this.jurisdiction = new ArrayList<CodeableConcept>(); 3234 this.jurisdiction.add(t); 3235 return this; 3236 } 3237 3238 /** 3239 * @return The first repetition of repeating field {@link #jurisdiction}, 3240 * creating it if it does not already exist 3241 */ 3242 public CodeableConcept getJurisdictionFirstRep() { 3243 if (getJurisdiction().isEmpty()) { 3244 addJurisdiction(); 3245 } 3246 return getJurisdiction().get(0); 3247 } 3248 3249 /** 3250 * @return {@link #purpose} (Explanation of why this operation definition is 3251 * needed and why it has been designed as it has.). This is the 3252 * underlying object with id, value and extensions. The accessor 3253 * "getPurpose" gives direct access to the value 3254 */ 3255 public MarkdownType getPurposeElement() { 3256 if (this.purpose == null) 3257 if (Configuration.errorOnAutoCreate()) 3258 throw new Error("Attempt to auto-create OperationDefinition.purpose"); 3259 else if (Configuration.doAutoCreate()) 3260 this.purpose = new MarkdownType(); // bb 3261 return this.purpose; 3262 } 3263 3264 public boolean hasPurposeElement() { 3265 return this.purpose != null && !this.purpose.isEmpty(); 3266 } 3267 3268 public boolean hasPurpose() { 3269 return this.purpose != null && !this.purpose.isEmpty(); 3270 } 3271 3272 /** 3273 * @param value {@link #purpose} (Explanation of why this operation definition 3274 * is needed and why it has been designed as it has.). This is the 3275 * underlying object with id, value and extensions. The accessor 3276 * "getPurpose" gives direct access to the value 3277 */ 3278 public OperationDefinition setPurposeElement(MarkdownType value) { 3279 this.purpose = value; 3280 return this; 3281 } 3282 3283 /** 3284 * @return Explanation of why this operation definition is needed and why it has 3285 * been designed as it has. 3286 */ 3287 public String getPurpose() { 3288 return this.purpose == null ? null : this.purpose.getValue(); 3289 } 3290 3291 /** 3292 * @param value Explanation of why this operation definition is needed and why 3293 * it has been designed as it has. 3294 */ 3295 public OperationDefinition setPurpose(String value) { 3296 if (value == null) 3297 this.purpose = null; 3298 else { 3299 if (this.purpose == null) 3300 this.purpose = new MarkdownType(); 3301 this.purpose.setValue(value); 3302 } 3303 return this; 3304 } 3305 3306 /** 3307 * @return {@link #affectsState} (Whether the operation affects state. Side 3308 * effects such as producing audit trail entries do not count as 3309 * 'affecting state'.). This is the underlying object with id, value and 3310 * extensions. The accessor "getAffectsState" gives direct access to the 3311 * value 3312 */ 3313 public BooleanType getAffectsStateElement() { 3314 if (this.affectsState == null) 3315 if (Configuration.errorOnAutoCreate()) 3316 throw new Error("Attempt to auto-create OperationDefinition.affectsState"); 3317 else if (Configuration.doAutoCreate()) 3318 this.affectsState = new BooleanType(); // bb 3319 return this.affectsState; 3320 } 3321 3322 public boolean hasAffectsStateElement() { 3323 return this.affectsState != null && !this.affectsState.isEmpty(); 3324 } 3325 3326 public boolean hasAffectsState() { 3327 return this.affectsState != null && !this.affectsState.isEmpty(); 3328 } 3329 3330 /** 3331 * @param value {@link #affectsState} (Whether the operation affects state. Side 3332 * effects such as producing audit trail entries do not count as 3333 * 'affecting state'.). This is the underlying object with id, 3334 * value and extensions. The accessor "getAffectsState" gives 3335 * direct access to the value 3336 */ 3337 public OperationDefinition setAffectsStateElement(BooleanType value) { 3338 this.affectsState = value; 3339 return this; 3340 } 3341 3342 /** 3343 * @return Whether the operation affects state. Side effects such as producing 3344 * audit trail entries do not count as 'affecting state'. 3345 */ 3346 public boolean getAffectsState() { 3347 return this.affectsState == null || this.affectsState.isEmpty() ? false : this.affectsState.getValue(); 3348 } 3349 3350 /** 3351 * @param value Whether the operation affects state. Side effects such as 3352 * producing audit trail entries do not count as 'affecting state'. 3353 */ 3354 public OperationDefinition setAffectsState(boolean value) { 3355 if (this.affectsState == null) 3356 this.affectsState = new BooleanType(); 3357 this.affectsState.setValue(value); 3358 return this; 3359 } 3360 3361 /** 3362 * @return {@link #code} (The name used to invoke the operation.). This is the 3363 * underlying object with id, value and extensions. The accessor 3364 * "getCode" gives direct access to the value 3365 */ 3366 public CodeType getCodeElement() { 3367 if (this.code == null) 3368 if (Configuration.errorOnAutoCreate()) 3369 throw new Error("Attempt to auto-create OperationDefinition.code"); 3370 else if (Configuration.doAutoCreate()) 3371 this.code = new CodeType(); // bb 3372 return this.code; 3373 } 3374 3375 public boolean hasCodeElement() { 3376 return this.code != null && !this.code.isEmpty(); 3377 } 3378 3379 public boolean hasCode() { 3380 return this.code != null && !this.code.isEmpty(); 3381 } 3382 3383 /** 3384 * @param value {@link #code} (The name used to invoke the operation.). This is 3385 * the underlying object with id, value and extensions. The 3386 * accessor "getCode" gives direct access to the value 3387 */ 3388 public OperationDefinition setCodeElement(CodeType value) { 3389 this.code = value; 3390 return this; 3391 } 3392 3393 /** 3394 * @return The name used to invoke the operation. 3395 */ 3396 public String getCode() { 3397 return this.code == null ? null : this.code.getValue(); 3398 } 3399 3400 /** 3401 * @param value The name used to invoke the operation. 3402 */ 3403 public OperationDefinition setCode(String value) { 3404 if (this.code == null) 3405 this.code = new CodeType(); 3406 this.code.setValue(value); 3407 return this; 3408 } 3409 3410 /** 3411 * @return {@link #comment} (Additional information about how to use this 3412 * operation or named query.). This is the underlying object with id, 3413 * value and extensions. The accessor "getComment" gives direct access 3414 * to the value 3415 */ 3416 public MarkdownType getCommentElement() { 3417 if (this.comment == null) 3418 if (Configuration.errorOnAutoCreate()) 3419 throw new Error("Attempt to auto-create OperationDefinition.comment"); 3420 else if (Configuration.doAutoCreate()) 3421 this.comment = new MarkdownType(); // bb 3422 return this.comment; 3423 } 3424 3425 public boolean hasCommentElement() { 3426 return this.comment != null && !this.comment.isEmpty(); 3427 } 3428 3429 public boolean hasComment() { 3430 return this.comment != null && !this.comment.isEmpty(); 3431 } 3432 3433 /** 3434 * @param value {@link #comment} (Additional information about how to use this 3435 * operation or named query.). This is the underlying object with 3436 * id, value and extensions. The accessor "getComment" gives direct 3437 * access to the value 3438 */ 3439 public OperationDefinition setCommentElement(MarkdownType value) { 3440 this.comment = value; 3441 return this; 3442 } 3443 3444 /** 3445 * @return Additional information about how to use this operation or named 3446 * query. 3447 */ 3448 public String getComment() { 3449 return this.comment == null ? null : this.comment.getValue(); 3450 } 3451 3452 /** 3453 * @param value Additional information about how to use this operation or named 3454 * query. 3455 */ 3456 public OperationDefinition setComment(String value) { 3457 if (value == null) 3458 this.comment = null; 3459 else { 3460 if (this.comment == null) 3461 this.comment = new MarkdownType(); 3462 this.comment.setValue(value); 3463 } 3464 return this; 3465 } 3466 3467 /** 3468 * @return {@link #base} (Indicates that this operation definition is a 3469 * constraining profile on the base.). This is the underlying object 3470 * with id, value and extensions. The accessor "getBase" gives direct 3471 * access to the value 3472 */ 3473 public CanonicalType getBaseElement() { 3474 if (this.base == null) 3475 if (Configuration.errorOnAutoCreate()) 3476 throw new Error("Attempt to auto-create OperationDefinition.base"); 3477 else if (Configuration.doAutoCreate()) 3478 this.base = new CanonicalType(); // bb 3479 return this.base; 3480 } 3481 3482 public boolean hasBaseElement() { 3483 return this.base != null && !this.base.isEmpty(); 3484 } 3485 3486 public boolean hasBase() { 3487 return this.base != null && !this.base.isEmpty(); 3488 } 3489 3490 /** 3491 * @param value {@link #base} (Indicates that this operation definition is a 3492 * constraining profile on the base.). This is the underlying 3493 * object with id, value and extensions. The accessor "getBase" 3494 * gives direct access to the value 3495 */ 3496 public OperationDefinition setBaseElement(CanonicalType value) { 3497 this.base = value; 3498 return this; 3499 } 3500 3501 /** 3502 * @return Indicates that this operation definition is a constraining profile on 3503 * the base. 3504 */ 3505 public String getBase() { 3506 return this.base == null ? null : this.base.getValue(); 3507 } 3508 3509 /** 3510 * @param value Indicates that this operation definition is a constraining 3511 * profile on the base. 3512 */ 3513 public OperationDefinition setBase(String value) { 3514 if (Utilities.noString(value)) 3515 this.base = null; 3516 else { 3517 if (this.base == null) 3518 this.base = new CanonicalType(); 3519 this.base.setValue(value); 3520 } 3521 return this; 3522 } 3523 3524 /** 3525 * @return {@link #resource} (The types on which this operation can be 3526 * executed.) 3527 */ 3528 public List<CodeType> getResource() { 3529 if (this.resource == null) 3530 this.resource = new ArrayList<CodeType>(); 3531 return this.resource; 3532 } 3533 3534 /** 3535 * @return Returns a reference to <code>this</code> for easy method chaining 3536 */ 3537 public OperationDefinition setResource(List<CodeType> theResource) { 3538 this.resource = theResource; 3539 return this; 3540 } 3541 3542 public boolean hasResource() { 3543 if (this.resource == null) 3544 return false; 3545 for (CodeType item : this.resource) 3546 if (!item.isEmpty()) 3547 return true; 3548 return false; 3549 } 3550 3551 /** 3552 * @return {@link #resource} (The types on which this operation can be 3553 * executed.) 3554 */ 3555 public CodeType addResourceElement() {// 2 3556 CodeType t = new CodeType(); 3557 if (this.resource == null) 3558 this.resource = new ArrayList<CodeType>(); 3559 this.resource.add(t); 3560 return t; 3561 } 3562 3563 /** 3564 * @param value {@link #resource} (The types on which this operation can be 3565 * executed.) 3566 */ 3567 public OperationDefinition addResource(String value) { // 1 3568 CodeType t = new CodeType(); 3569 t.setValue(value); 3570 if (this.resource == null) 3571 this.resource = new ArrayList<CodeType>(); 3572 this.resource.add(t); 3573 return this; 3574 } 3575 3576 /** 3577 * @param value {@link #resource} (The types on which this operation can be 3578 * executed.) 3579 */ 3580 public boolean hasResource(String value) { 3581 if (this.resource == null) 3582 return false; 3583 for (CodeType v : this.resource) 3584 if (v.getValue().equals(value)) // code 3585 return true; 3586 return false; 3587 } 3588 3589 /** 3590 * @return {@link #system} (Indicates whether this operation or named query can 3591 * be invoked at the system level (e.g. without needing to choose a 3592 * resource type for the context).). This is the underlying object with 3593 * id, value and extensions. The accessor "getSystem" gives direct 3594 * access to the value 3595 */ 3596 public BooleanType getSystemElement() { 3597 if (this.system == null) 3598 if (Configuration.errorOnAutoCreate()) 3599 throw new Error("Attempt to auto-create OperationDefinition.system"); 3600 else if (Configuration.doAutoCreate()) 3601 this.system = new BooleanType(); // bb 3602 return this.system; 3603 } 3604 3605 public boolean hasSystemElement() { 3606 return this.system != null && !this.system.isEmpty(); 3607 } 3608 3609 public boolean hasSystem() { 3610 return this.system != null && !this.system.isEmpty(); 3611 } 3612 3613 /** 3614 * @param value {@link #system} (Indicates whether this operation or named query 3615 * can be invoked at the system level (e.g. without needing to 3616 * choose a resource type for the context).). This is the 3617 * underlying object with id, value and extensions. The accessor 3618 * "getSystem" gives direct access to the value 3619 */ 3620 public OperationDefinition setSystemElement(BooleanType value) { 3621 this.system = value; 3622 return this; 3623 } 3624 3625 /** 3626 * @return Indicates whether this operation or named query can be invoked at the 3627 * system level (e.g. without needing to choose a resource type for the 3628 * context). 3629 */ 3630 public boolean getSystem() { 3631 return this.system == null || this.system.isEmpty() ? false : this.system.getValue(); 3632 } 3633 3634 /** 3635 * @param value Indicates whether this operation or named query can be invoked 3636 * at the system level (e.g. without needing to choose a resource 3637 * type for the context). 3638 */ 3639 public OperationDefinition setSystem(boolean value) { 3640 if (this.system == null) 3641 this.system = new BooleanType(); 3642 this.system.setValue(value); 3643 return this; 3644 } 3645 3646 /** 3647 * @return {@link #type} (Indicates whether this operation or named query can be 3648 * invoked at the resource type level for any given resource type level 3649 * (e.g. without needing to choose a specific resource id for the 3650 * context).). This is the underlying object with id, value and 3651 * extensions. The accessor "getType" gives direct access to the value 3652 */ 3653 public BooleanType getTypeElement() { 3654 if (this.type == null) 3655 if (Configuration.errorOnAutoCreate()) 3656 throw new Error("Attempt to auto-create OperationDefinition.type"); 3657 else if (Configuration.doAutoCreate()) 3658 this.type = new BooleanType(); // bb 3659 return this.type; 3660 } 3661 3662 public boolean hasTypeElement() { 3663 return this.type != null && !this.type.isEmpty(); 3664 } 3665 3666 public boolean hasType() { 3667 return this.type != null && !this.type.isEmpty(); 3668 } 3669 3670 /** 3671 * @param value {@link #type} (Indicates whether this operation or named query 3672 * can be invoked at the resource type level for any given resource 3673 * type level (e.g. without needing to choose a specific resource 3674 * id for the context).). This is the underlying object with id, 3675 * value and extensions. The accessor "getType" gives direct access 3676 * to the value 3677 */ 3678 public OperationDefinition setTypeElement(BooleanType value) { 3679 this.type = value; 3680 return this; 3681 } 3682 3683 /** 3684 * @return Indicates whether this operation or named query can be invoked at the 3685 * resource type level for any given resource type level (e.g. without 3686 * needing to choose a specific resource id for the context). 3687 */ 3688 public boolean getType() { 3689 return this.type == null || this.type.isEmpty() ? false : this.type.getValue(); 3690 } 3691 3692 /** 3693 * @param value Indicates whether this operation or named query can be invoked 3694 * at the resource type level for any given resource type level 3695 * (e.g. without needing to choose a specific resource id for the 3696 * context). 3697 */ 3698 public OperationDefinition setType(boolean value) { 3699 if (this.type == null) 3700 this.type = new BooleanType(); 3701 this.type.setValue(value); 3702 return this; 3703 } 3704 3705 /** 3706 * @return {@link #instance} (Indicates whether this operation can be invoked on 3707 * a particular instance of one of the given types.). This is the 3708 * underlying object with id, value and extensions. The accessor 3709 * "getInstance" gives direct access to the value 3710 */ 3711 public BooleanType getInstanceElement() { 3712 if (this.instance == null) 3713 if (Configuration.errorOnAutoCreate()) 3714 throw new Error("Attempt to auto-create OperationDefinition.instance"); 3715 else if (Configuration.doAutoCreate()) 3716 this.instance = new BooleanType(); // bb 3717 return this.instance; 3718 } 3719 3720 public boolean hasInstanceElement() { 3721 return this.instance != null && !this.instance.isEmpty(); 3722 } 3723 3724 public boolean hasInstance() { 3725 return this.instance != null && !this.instance.isEmpty(); 3726 } 3727 3728 /** 3729 * @param value {@link #instance} (Indicates whether this operation can be 3730 * invoked on a particular instance of one of the given types.). 3731 * This is the underlying object with id, value and extensions. The 3732 * accessor "getInstance" gives direct access to the value 3733 */ 3734 public OperationDefinition setInstanceElement(BooleanType value) { 3735 this.instance = value; 3736 return this; 3737 } 3738 3739 /** 3740 * @return Indicates whether this operation can be invoked on a particular 3741 * instance of one of the given types. 3742 */ 3743 public boolean getInstance() { 3744 return this.instance == null || this.instance.isEmpty() ? false : this.instance.getValue(); 3745 } 3746 3747 /** 3748 * @param value Indicates whether this operation can be invoked on a particular 3749 * instance of one of the given types. 3750 */ 3751 public OperationDefinition setInstance(boolean value) { 3752 if (this.instance == null) 3753 this.instance = new BooleanType(); 3754 this.instance.setValue(value); 3755 return this; 3756 } 3757 3758 /** 3759 * @return {@link #inputProfile} (Additional validation information for the in 3760 * parameters - a single profile that covers all the parameters. The 3761 * profile is a constraint on the parameters resource as a whole.). This 3762 * is the underlying object with id, value and extensions. The accessor 3763 * "getInputProfile" gives direct access to the value 3764 */ 3765 public CanonicalType getInputProfileElement() { 3766 if (this.inputProfile == null) 3767 if (Configuration.errorOnAutoCreate()) 3768 throw new Error("Attempt to auto-create OperationDefinition.inputProfile"); 3769 else if (Configuration.doAutoCreate()) 3770 this.inputProfile = new CanonicalType(); // bb 3771 return this.inputProfile; 3772 } 3773 3774 public boolean hasInputProfileElement() { 3775 return this.inputProfile != null && !this.inputProfile.isEmpty(); 3776 } 3777 3778 public boolean hasInputProfile() { 3779 return this.inputProfile != null && !this.inputProfile.isEmpty(); 3780 } 3781 3782 /** 3783 * @param value {@link #inputProfile} (Additional validation information for the 3784 * in parameters - a single profile that covers all the parameters. 3785 * The profile is a constraint on the parameters resource as a 3786 * whole.). This is the underlying object with id, value and 3787 * extensions. The accessor "getInputProfile" gives direct access 3788 * to the value 3789 */ 3790 public OperationDefinition setInputProfileElement(CanonicalType value) { 3791 this.inputProfile = value; 3792 return this; 3793 } 3794 3795 /** 3796 * @return Additional validation information for the in parameters - a single 3797 * profile that covers all the parameters. The profile is a constraint 3798 * on the parameters resource as a whole. 3799 */ 3800 public String getInputProfile() { 3801 return this.inputProfile == null ? null : this.inputProfile.getValue(); 3802 } 3803 3804 /** 3805 * @param value Additional validation information for the in parameters - a 3806 * single profile that covers all the parameters. The profile is a 3807 * constraint on the parameters resource as a whole. 3808 */ 3809 public OperationDefinition setInputProfile(String value) { 3810 if (Utilities.noString(value)) 3811 this.inputProfile = null; 3812 else { 3813 if (this.inputProfile == null) 3814 this.inputProfile = new CanonicalType(); 3815 this.inputProfile.setValue(value); 3816 } 3817 return this; 3818 } 3819 3820 /** 3821 * @return {@link #outputProfile} (Additional validation information for the out 3822 * parameters - a single profile that covers all the parameters. The 3823 * profile is a constraint on the parameters resource.). This is the 3824 * underlying object with id, value and extensions. The accessor 3825 * "getOutputProfile" gives direct access to the value 3826 */ 3827 public CanonicalType getOutputProfileElement() { 3828 if (this.outputProfile == null) 3829 if (Configuration.errorOnAutoCreate()) 3830 throw new Error("Attempt to auto-create OperationDefinition.outputProfile"); 3831 else if (Configuration.doAutoCreate()) 3832 this.outputProfile = new CanonicalType(); // bb 3833 return this.outputProfile; 3834 } 3835 3836 public boolean hasOutputProfileElement() { 3837 return this.outputProfile != null && !this.outputProfile.isEmpty(); 3838 } 3839 3840 public boolean hasOutputProfile() { 3841 return this.outputProfile != null && !this.outputProfile.isEmpty(); 3842 } 3843 3844 /** 3845 * @param value {@link #outputProfile} (Additional validation information for 3846 * the out parameters - a single profile that covers all the 3847 * parameters. The profile is a constraint on the parameters 3848 * resource.). This is the underlying object with id, value and 3849 * extensions. The accessor "getOutputProfile" gives direct access 3850 * to the value 3851 */ 3852 public OperationDefinition setOutputProfileElement(CanonicalType value) { 3853 this.outputProfile = value; 3854 return this; 3855 } 3856 3857 /** 3858 * @return Additional validation information for the out parameters - a single 3859 * profile that covers all the parameters. The profile is a constraint 3860 * on the parameters resource. 3861 */ 3862 public String getOutputProfile() { 3863 return this.outputProfile == null ? null : this.outputProfile.getValue(); 3864 } 3865 3866 /** 3867 * @param value Additional validation information for the out parameters - a 3868 * single profile that covers all the parameters. The profile is a 3869 * constraint on the parameters resource. 3870 */ 3871 public OperationDefinition setOutputProfile(String value) { 3872 if (Utilities.noString(value)) 3873 this.outputProfile = null; 3874 else { 3875 if (this.outputProfile == null) 3876 this.outputProfile = new CanonicalType(); 3877 this.outputProfile.setValue(value); 3878 } 3879 return this; 3880 } 3881 3882 /** 3883 * @return {@link #parameter} (The parameters for the operation/query.) 3884 */ 3885 public List<OperationDefinitionParameterComponent> getParameter() { 3886 if (this.parameter == null) 3887 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3888 return this.parameter; 3889 } 3890 3891 /** 3892 * @return Returns a reference to <code>this</code> for easy method chaining 3893 */ 3894 public OperationDefinition setParameter(List<OperationDefinitionParameterComponent> theParameter) { 3895 this.parameter = theParameter; 3896 return this; 3897 } 3898 3899 public boolean hasParameter() { 3900 if (this.parameter == null) 3901 return false; 3902 for (OperationDefinitionParameterComponent item : this.parameter) 3903 if (!item.isEmpty()) 3904 return true; 3905 return false; 3906 } 3907 3908 public OperationDefinitionParameterComponent addParameter() { // 3 3909 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 3910 if (this.parameter == null) 3911 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3912 this.parameter.add(t); 3913 return t; 3914 } 3915 3916 public OperationDefinition addParameter(OperationDefinitionParameterComponent t) { // 3 3917 if (t == null) 3918 return this; 3919 if (this.parameter == null) 3920 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3921 this.parameter.add(t); 3922 return this; 3923 } 3924 3925 /** 3926 * @return The first repetition of repeating field {@link #parameter}, creating 3927 * it if it does not already exist 3928 */ 3929 public OperationDefinitionParameterComponent getParameterFirstRep() { 3930 if (getParameter().isEmpty()) { 3931 addParameter(); 3932 } 3933 return getParameter().get(0); 3934 } 3935 3936 /** 3937 * @return {@link #overload} (Defines an appropriate combination of parameters 3938 * to use when invoking this operation, to help code generators when 3939 * generating overloaded parameter sets for this operation.) 3940 */ 3941 public List<OperationDefinitionOverloadComponent> getOverload() { 3942 if (this.overload == null) 3943 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3944 return this.overload; 3945 } 3946 3947 /** 3948 * @return Returns a reference to <code>this</code> for easy method chaining 3949 */ 3950 public OperationDefinition setOverload(List<OperationDefinitionOverloadComponent> theOverload) { 3951 this.overload = theOverload; 3952 return this; 3953 } 3954 3955 public boolean hasOverload() { 3956 if (this.overload == null) 3957 return false; 3958 for (OperationDefinitionOverloadComponent item : this.overload) 3959 if (!item.isEmpty()) 3960 return true; 3961 return false; 3962 } 3963 3964 public OperationDefinitionOverloadComponent addOverload() { // 3 3965 OperationDefinitionOverloadComponent t = new OperationDefinitionOverloadComponent(); 3966 if (this.overload == null) 3967 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3968 this.overload.add(t); 3969 return t; 3970 } 3971 3972 public OperationDefinition addOverload(OperationDefinitionOverloadComponent t) { // 3 3973 if (t == null) 3974 return this; 3975 if (this.overload == null) 3976 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3977 this.overload.add(t); 3978 return this; 3979 } 3980 3981 /** 3982 * @return The first repetition of repeating field {@link #overload}, creating 3983 * it if it does not already exist 3984 */ 3985 public OperationDefinitionOverloadComponent getOverloadFirstRep() { 3986 if (getOverload().isEmpty()) { 3987 addOverload(); 3988 } 3989 return getOverload().get(0); 3990 } 3991 3992 protected void listChildren(List<Property> children) { 3993 super.listChildren(children); 3994 children.add(new Property("url", "uri", 3995 "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.", 3996 0, 1, url)); 3997 children.add(new Property("version", "string", 3998 "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3999 0, 1, version)); 4000 children.add(new Property("name", "string", 4001 "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4002 0, 1, name)); 4003 children.add(new Property("title", "string", 4004 "A short, descriptive, user-friendly title for the operation definition.", 0, 1, title)); 4005 children.add(new Property("status", "code", 4006 "The status of this operation definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 4007 children.add(new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind)); 4008 children.add(new Property("experimental", "boolean", 4009 "A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4010 0, 1, experimental)); 4011 children.add(new Property("date", "dateTime", 4012 "The date (and optionally time) when the operation definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 4013 0, 1, date)); 4014 children.add(new Property("publisher", "string", 4015 "The name of the organization or individual that published the operation definition.", 0, 1, publisher)); 4016 children.add(new Property("contact", "ContactDetail", 4017 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4018 java.lang.Integer.MAX_VALUE, contact)); 4019 children.add(new Property("description", "markdown", 4020 "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, 4021 description)); 4022 children.add(new Property("useContext", "UsageContext", 4023 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition instances.", 4024 0, java.lang.Integer.MAX_VALUE, useContext)); 4025 children.add(new Property("jurisdiction", "CodeableConcept", 4026 "A legal or geographic region in which the operation definition is intended to be used.", 0, 4027 java.lang.Integer.MAX_VALUE, jurisdiction)); 4028 children.add(new Property("purpose", "markdown", 4029 "Explanation of why this operation definition is needed and why it has been designed as it has.", 0, 1, 4030 purpose)); 4031 children.add(new Property("affectsState", "boolean", 4032 "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.", 4033 0, 1, affectsState)); 4034 children.add(new Property("code", "code", "The name used to invoke the operation.", 0, 1, code)); 4035 children.add(new Property("comment", "markdown", 4036 "Additional information about how to use this operation or named query.", 0, 1, comment)); 4037 children.add(new Property("base", "canonical(OperationDefinition)", 4038 "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base)); 4039 children.add(new Property("resource", "code", "The types on which this operation can be executed.", 0, 4040 java.lang.Integer.MAX_VALUE, resource)); 4041 children.add(new Property("system", "boolean", 4042 "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 4043 0, 1, system)); 4044 children.add(new Property("type", "boolean", 4045 "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 4046 0, 1, type)); 4047 children.add(new Property("instance", "boolean", 4048 "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, 4049 instance)); 4050 children.add(new Property("inputProfile", "canonical(StructureDefinition)", 4051 "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.", 4052 0, 1, inputProfile)); 4053 children.add(new Property("outputProfile", "canonical(StructureDefinition)", 4054 "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.", 4055 0, 1, outputProfile)); 4056 children.add(new Property("parameter", "", "The parameters for the operation/query.", 0, 4057 java.lang.Integer.MAX_VALUE, parameter)); 4058 children.add(new Property("overload", "", 4059 "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 4060 0, java.lang.Integer.MAX_VALUE, overload)); 4061 } 4062 4063 @Override 4064 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4065 switch (_hash) { 4066 case 116079: 4067 /* url */ return new Property("url", "uri", 4068 "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.", 4069 0, 1, url); 4070 case 351608024: 4071 /* version */ return new Property("version", "string", 4072 "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4073 0, 1, version); 4074 case 3373707: 4075 /* name */ return new Property("name", "string", 4076 "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4077 0, 1, name); 4078 case 110371416: 4079 /* title */ return new Property("title", "string", 4080 "A short, descriptive, user-friendly title for the operation definition.", 0, 1, title); 4081 case -892481550: 4082 /* status */ return new Property("status", "code", 4083 "The status of this operation definition. Enables tracking the life-cycle of the content.", 0, 1, status); 4084 case 3292052: 4085 /* kind */ return new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind); 4086 case -404562712: 4087 /* experimental */ return new Property("experimental", "boolean", 4088 "A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4089 0, 1, experimental); 4090 case 3076014: 4091 /* date */ return new Property("date", "dateTime", 4092 "The date (and optionally time) when the operation definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 4093 0, 1, date); 4094 case 1447404028: 4095 /* publisher */ return new Property("publisher", "string", 4096 "The name of the organization or individual that published the operation definition.", 0, 1, publisher); 4097 case 951526432: 4098 /* contact */ return new Property("contact", "ContactDetail", 4099 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4100 java.lang.Integer.MAX_VALUE, contact); 4101 case -1724546052: 4102 /* description */ return new Property("description", "markdown", 4103 "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, 4104 description); 4105 case -669707736: 4106 /* useContext */ return new Property("useContext", "UsageContext", 4107 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition instances.", 4108 0, java.lang.Integer.MAX_VALUE, useContext); 4109 case -507075711: 4110 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4111 "A legal or geographic region in which the operation definition is intended to be used.", 0, 4112 java.lang.Integer.MAX_VALUE, jurisdiction); 4113 case -220463842: 4114 /* purpose */ return new Property("purpose", "markdown", 4115 "Explanation of why this operation definition is needed and why it has been designed as it has.", 0, 1, 4116 purpose); 4117 case -14805197: 4118 /* affectsState */ return new Property("affectsState", "boolean", 4119 "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.", 4120 0, 1, affectsState); 4121 case 3059181: 4122 /* code */ return new Property("code", "code", "The name used to invoke the operation.", 0, 1, code); 4123 case 950398559: 4124 /* comment */ return new Property("comment", "markdown", 4125 "Additional information about how to use this operation or named query.", 0, 1, comment); 4126 case 3016401: 4127 /* base */ return new Property("base", "canonical(OperationDefinition)", 4128 "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base); 4129 case -341064690: 4130 /* resource */ return new Property("resource", "code", "The types on which this operation can be executed.", 0, 4131 java.lang.Integer.MAX_VALUE, resource); 4132 case -887328209: 4133 /* system */ return new Property("system", "boolean", 4134 "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 4135 0, 1, system); 4136 case 3575610: 4137 /* type */ return new Property("type", "boolean", 4138 "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 4139 0, 1, type); 4140 case 555127957: 4141 /* instance */ return new Property("instance", "boolean", 4142 "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, 4143 instance); 4144 case 676942463: 4145 /* inputProfile */ return new Property("inputProfile", "canonical(StructureDefinition)", 4146 "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.", 4147 0, 1, inputProfile); 4148 case 1826166120: 4149 /* outputProfile */ return new Property("outputProfile", "canonical(StructureDefinition)", 4150 "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.", 4151 0, 1, outputProfile); 4152 case 1954460585: 4153 /* parameter */ return new Property("parameter", "", "The parameters for the operation/query.", 0, 4154 java.lang.Integer.MAX_VALUE, parameter); 4155 case 529823674: 4156 /* overload */ return new Property("overload", "", 4157 "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 4158 0, java.lang.Integer.MAX_VALUE, overload); 4159 default: 4160 return super.getNamedProperty(_hash, _name, _checkValid); 4161 } 4162 4163 } 4164 4165 @Override 4166 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4167 switch (hash) { 4168 case 116079: 4169 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4170 case 351608024: 4171 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4172 case 3373707: 4173 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4174 case 110371416: 4175 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4176 case -892481550: 4177 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4178 case 3292052: 4179 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<OperationKind> 4180 case -404562712: 4181 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4182 case 3076014: 4183 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4184 case 1447404028: 4185 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4186 case 951526432: 4187 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4188 case -1724546052: 4189 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4190 case -669707736: 4191 /* useContext */ return this.useContext == null ? new Base[0] 4192 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4193 case -507075711: 4194 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4195 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4196 case -220463842: 4197 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 4198 case -14805197: 4199 /* affectsState */ return this.affectsState == null ? new Base[0] : new Base[] { this.affectsState }; // BooleanType 4200 case 3059181: 4201 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 4202 case 950398559: 4203 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // MarkdownType 4204 case 3016401: 4205 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // CanonicalType 4206 case -341064690: 4207 /* resource */ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CodeType 4208 case -887328209: 4209 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // BooleanType 4210 case 3575610: 4211 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // BooleanType 4212 case 555127957: 4213 /* instance */ return this.instance == null ? new Base[0] : new Base[] { this.instance }; // BooleanType 4214 case 676942463: 4215 /* inputProfile */ return this.inputProfile == null ? new Base[0] : new Base[] { this.inputProfile }; // CanonicalType 4216 case 1826166120: 4217 /* outputProfile */ return this.outputProfile == null ? new Base[0] : new Base[] { this.outputProfile }; // CanonicalType 4218 case 1954460585: 4219 /* parameter */ return this.parameter == null ? new Base[0] 4220 : this.parameter.toArray(new Base[this.parameter.size()]); // OperationDefinitionParameterComponent 4221 case 529823674: 4222 /* overload */ return this.overload == null ? new Base[0] : this.overload.toArray(new Base[this.overload.size()]); // OperationDefinitionOverloadComponent 4223 default: 4224 return super.getProperty(hash, name, checkValid); 4225 } 4226 4227 } 4228 4229 @Override 4230 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4231 switch (hash) { 4232 case 116079: // url 4233 this.url = castToUri(value); // UriType 4234 return value; 4235 case 351608024: // version 4236 this.version = castToString(value); // StringType 4237 return value; 4238 case 3373707: // name 4239 this.name = castToString(value); // StringType 4240 return value; 4241 case 110371416: // title 4242 this.title = castToString(value); // StringType 4243 return value; 4244 case -892481550: // status 4245 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4246 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4247 return value; 4248 case 3292052: // kind 4249 value = new OperationKindEnumFactory().fromType(castToCode(value)); 4250 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4251 return value; 4252 case -404562712: // experimental 4253 this.experimental = castToBoolean(value); // BooleanType 4254 return value; 4255 case 3076014: // date 4256 this.date = castToDateTime(value); // DateTimeType 4257 return value; 4258 case 1447404028: // publisher 4259 this.publisher = castToString(value); // StringType 4260 return value; 4261 case 951526432: // contact 4262 this.getContact().add(castToContactDetail(value)); // ContactDetail 4263 return value; 4264 case -1724546052: // description 4265 this.description = castToMarkdown(value); // MarkdownType 4266 return value; 4267 case -669707736: // useContext 4268 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4269 return value; 4270 case -507075711: // jurisdiction 4271 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4272 return value; 4273 case -220463842: // purpose 4274 this.purpose = castToMarkdown(value); // MarkdownType 4275 return value; 4276 case -14805197: // affectsState 4277 this.affectsState = castToBoolean(value); // BooleanType 4278 return value; 4279 case 3059181: // code 4280 this.code = castToCode(value); // CodeType 4281 return value; 4282 case 950398559: // comment 4283 this.comment = castToMarkdown(value); // MarkdownType 4284 return value; 4285 case 3016401: // base 4286 this.base = castToCanonical(value); // CanonicalType 4287 return value; 4288 case -341064690: // resource 4289 this.getResource().add(castToCode(value)); // CodeType 4290 return value; 4291 case -887328209: // system 4292 this.system = castToBoolean(value); // BooleanType 4293 return value; 4294 case 3575610: // type 4295 this.type = castToBoolean(value); // BooleanType 4296 return value; 4297 case 555127957: // instance 4298 this.instance = castToBoolean(value); // BooleanType 4299 return value; 4300 case 676942463: // inputProfile 4301 this.inputProfile = castToCanonical(value); // CanonicalType 4302 return value; 4303 case 1826166120: // outputProfile 4304 this.outputProfile = castToCanonical(value); // CanonicalType 4305 return value; 4306 case 1954460585: // parameter 4307 this.getParameter().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 4308 return value; 4309 case 529823674: // overload 4310 this.getOverload().add((OperationDefinitionOverloadComponent) value); // OperationDefinitionOverloadComponent 4311 return value; 4312 default: 4313 return super.setProperty(hash, name, value); 4314 } 4315 4316 } 4317 4318 @Override 4319 public Base setProperty(String name, Base value) throws FHIRException { 4320 if (name.equals("url")) { 4321 this.url = castToUri(value); // UriType 4322 } else if (name.equals("version")) { 4323 this.version = castToString(value); // StringType 4324 } else if (name.equals("name")) { 4325 this.name = castToString(value); // StringType 4326 } else if (name.equals("title")) { 4327 this.title = castToString(value); // StringType 4328 } else if (name.equals("status")) { 4329 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4330 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4331 } else if (name.equals("kind")) { 4332 value = new OperationKindEnumFactory().fromType(castToCode(value)); 4333 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4334 } else if (name.equals("experimental")) { 4335 this.experimental = castToBoolean(value); // BooleanType 4336 } else if (name.equals("date")) { 4337 this.date = castToDateTime(value); // DateTimeType 4338 } else if (name.equals("publisher")) { 4339 this.publisher = castToString(value); // StringType 4340 } else if (name.equals("contact")) { 4341 this.getContact().add(castToContactDetail(value)); 4342 } else if (name.equals("description")) { 4343 this.description = castToMarkdown(value); // MarkdownType 4344 } else if (name.equals("useContext")) { 4345 this.getUseContext().add(castToUsageContext(value)); 4346 } else if (name.equals("jurisdiction")) { 4347 this.getJurisdiction().add(castToCodeableConcept(value)); 4348 } else if (name.equals("purpose")) { 4349 this.purpose = castToMarkdown(value); // MarkdownType 4350 } else if (name.equals("affectsState")) { 4351 this.affectsState = castToBoolean(value); // BooleanType 4352 } else if (name.equals("code")) { 4353 this.code = castToCode(value); // CodeType 4354 } else if (name.equals("comment")) { 4355 this.comment = castToMarkdown(value); // MarkdownType 4356 } else if (name.equals("base")) { 4357 this.base = castToCanonical(value); // CanonicalType 4358 } else if (name.equals("resource")) { 4359 this.getResource().add(castToCode(value)); 4360 } else if (name.equals("system")) { 4361 this.system = castToBoolean(value); // BooleanType 4362 } else if (name.equals("type")) { 4363 this.type = castToBoolean(value); // BooleanType 4364 } else if (name.equals("instance")) { 4365 this.instance = castToBoolean(value); // BooleanType 4366 } else if (name.equals("inputProfile")) { 4367 this.inputProfile = castToCanonical(value); // CanonicalType 4368 } else if (name.equals("outputProfile")) { 4369 this.outputProfile = castToCanonical(value); // CanonicalType 4370 } else if (name.equals("parameter")) { 4371 this.getParameter().add((OperationDefinitionParameterComponent) value); 4372 } else if (name.equals("overload")) { 4373 this.getOverload().add((OperationDefinitionOverloadComponent) value); 4374 } else 4375 return super.setProperty(name, value); 4376 return value; 4377 } 4378 4379 @Override 4380 public void removeChild(String name, Base value) throws FHIRException { 4381 if (name.equals("url")) { 4382 this.url = null; 4383 } else if (name.equals("version")) { 4384 this.version = null; 4385 } else if (name.equals("name")) { 4386 this.name = null; 4387 } else if (name.equals("title")) { 4388 this.title = null; 4389 } else if (name.equals("status")) { 4390 this.status = null; 4391 } else if (name.equals("kind")) { 4392 this.kind = null; 4393 } else if (name.equals("experimental")) { 4394 this.experimental = null; 4395 } else if (name.equals("date")) { 4396 this.date = null; 4397 } else if (name.equals("publisher")) { 4398 this.publisher = null; 4399 } else if (name.equals("contact")) { 4400 this.getContact().remove(castToContactDetail(value)); 4401 } else if (name.equals("description")) { 4402 this.description = null; 4403 } else if (name.equals("useContext")) { 4404 this.getUseContext().remove(castToUsageContext(value)); 4405 } else if (name.equals("jurisdiction")) { 4406 this.getJurisdiction().remove(castToCodeableConcept(value)); 4407 } else if (name.equals("purpose")) { 4408 this.purpose = null; 4409 } else if (name.equals("affectsState")) { 4410 this.affectsState = null; 4411 } else if (name.equals("code")) { 4412 this.code = null; 4413 } else if (name.equals("comment")) { 4414 this.comment = null; 4415 } else if (name.equals("base")) { 4416 this.base = null; 4417 } else if (name.equals("resource")) { 4418 this.getResource().remove(castToCode(value)); 4419 } else if (name.equals("system")) { 4420 this.system = null; 4421 } else if (name.equals("type")) { 4422 this.type = null; 4423 } else if (name.equals("instance")) { 4424 this.instance = null; 4425 } else if (name.equals("inputProfile")) { 4426 this.inputProfile = null; 4427 } else if (name.equals("outputProfile")) { 4428 this.outputProfile = null; 4429 } else if (name.equals("parameter")) { 4430 this.getParameter().remove((OperationDefinitionParameterComponent) value); 4431 } else if (name.equals("overload")) { 4432 this.getOverload().remove((OperationDefinitionOverloadComponent) value); 4433 } else 4434 super.removeChild(name, value); 4435 4436 } 4437 4438 @Override 4439 public Base makeProperty(int hash, String name) throws FHIRException { 4440 switch (hash) { 4441 case 116079: 4442 return getUrlElement(); 4443 case 351608024: 4444 return getVersionElement(); 4445 case 3373707: 4446 return getNameElement(); 4447 case 110371416: 4448 return getTitleElement(); 4449 case -892481550: 4450 return getStatusElement(); 4451 case 3292052: 4452 return getKindElement(); 4453 case -404562712: 4454 return getExperimentalElement(); 4455 case 3076014: 4456 return getDateElement(); 4457 case 1447404028: 4458 return getPublisherElement(); 4459 case 951526432: 4460 return addContact(); 4461 case -1724546052: 4462 return getDescriptionElement(); 4463 case -669707736: 4464 return addUseContext(); 4465 case -507075711: 4466 return addJurisdiction(); 4467 case -220463842: 4468 return getPurposeElement(); 4469 case -14805197: 4470 return getAffectsStateElement(); 4471 case 3059181: 4472 return getCodeElement(); 4473 case 950398559: 4474 return getCommentElement(); 4475 case 3016401: 4476 return getBaseElement(); 4477 case -341064690: 4478 return addResourceElement(); 4479 case -887328209: 4480 return getSystemElement(); 4481 case 3575610: 4482 return getTypeElement(); 4483 case 555127957: 4484 return getInstanceElement(); 4485 case 676942463: 4486 return getInputProfileElement(); 4487 case 1826166120: 4488 return getOutputProfileElement(); 4489 case 1954460585: 4490 return addParameter(); 4491 case 529823674: 4492 return addOverload(); 4493 default: 4494 return super.makeProperty(hash, name); 4495 } 4496 4497 } 4498 4499 @Override 4500 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4501 switch (hash) { 4502 case 116079: 4503 /* url */ return new String[] { "uri" }; 4504 case 351608024: 4505 /* version */ return new String[] { "string" }; 4506 case 3373707: 4507 /* name */ return new String[] { "string" }; 4508 case 110371416: 4509 /* title */ return new String[] { "string" }; 4510 case -892481550: 4511 /* status */ return new String[] { "code" }; 4512 case 3292052: 4513 /* kind */ return new String[] { "code" }; 4514 case -404562712: 4515 /* experimental */ return new String[] { "boolean" }; 4516 case 3076014: 4517 /* date */ return new String[] { "dateTime" }; 4518 case 1447404028: 4519 /* publisher */ return new String[] { "string" }; 4520 case 951526432: 4521 /* contact */ return new String[] { "ContactDetail" }; 4522 case -1724546052: 4523 /* description */ return new String[] { "markdown" }; 4524 case -669707736: 4525 /* useContext */ return new String[] { "UsageContext" }; 4526 case -507075711: 4527 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4528 case -220463842: 4529 /* purpose */ return new String[] { "markdown" }; 4530 case -14805197: 4531 /* affectsState */ return new String[] { "boolean" }; 4532 case 3059181: 4533 /* code */ return new String[] { "code" }; 4534 case 950398559: 4535 /* comment */ return new String[] { "markdown" }; 4536 case 3016401: 4537 /* base */ return new String[] { "canonical" }; 4538 case -341064690: 4539 /* resource */ return new String[] { "code" }; 4540 case -887328209: 4541 /* system */ return new String[] { "boolean" }; 4542 case 3575610: 4543 /* type */ return new String[] { "boolean" }; 4544 case 555127957: 4545 /* instance */ return new String[] { "boolean" }; 4546 case 676942463: 4547 /* inputProfile */ return new String[] { "canonical" }; 4548 case 1826166120: 4549 /* outputProfile */ return new String[] { "canonical" }; 4550 case 1954460585: 4551 /* parameter */ return new String[] {}; 4552 case 529823674: 4553 /* overload */ return new String[] {}; 4554 default: 4555 return super.getTypesForProperty(hash, name); 4556 } 4557 4558 } 4559 4560 @Override 4561 public Base addChild(String name) throws FHIRException { 4562 if (name.equals("url")) { 4563 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.url"); 4564 } else if (name.equals("version")) { 4565 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.version"); 4566 } else if (name.equals("name")) { 4567 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 4568 } else if (name.equals("title")) { 4569 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.title"); 4570 } else if (name.equals("status")) { 4571 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.status"); 4572 } else if (name.equals("kind")) { 4573 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.kind"); 4574 } else if (name.equals("experimental")) { 4575 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.experimental"); 4576 } else if (name.equals("date")) { 4577 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.date"); 4578 } else if (name.equals("publisher")) { 4579 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.publisher"); 4580 } else if (name.equals("contact")) { 4581 return addContact(); 4582 } else if (name.equals("description")) { 4583 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.description"); 4584 } else if (name.equals("useContext")) { 4585 return addUseContext(); 4586 } else if (name.equals("jurisdiction")) { 4587 return addJurisdiction(); 4588 } else if (name.equals("purpose")) { 4589 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.purpose"); 4590 } else if (name.equals("affectsState")) { 4591 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.affectsState"); 4592 } else if (name.equals("code")) { 4593 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.code"); 4594 } else if (name.equals("comment")) { 4595 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 4596 } else if (name.equals("base")) { 4597 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.base"); 4598 } else if (name.equals("resource")) { 4599 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.resource"); 4600 } else if (name.equals("system")) { 4601 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.system"); 4602 } else if (name.equals("type")) { 4603 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 4604 } else if (name.equals("instance")) { 4605 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.instance"); 4606 } else if (name.equals("inputProfile")) { 4607 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.inputProfile"); 4608 } else if (name.equals("outputProfile")) { 4609 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.outputProfile"); 4610 } else if (name.equals("parameter")) { 4611 return addParameter(); 4612 } else if (name.equals("overload")) { 4613 return addOverload(); 4614 } else 4615 return super.addChild(name); 4616 } 4617 4618 public String fhirType() { 4619 return "OperationDefinition"; 4620 4621 } 4622 4623 public OperationDefinition copy() { 4624 OperationDefinition dst = new OperationDefinition(); 4625 copyValues(dst); 4626 return dst; 4627 } 4628 4629 public void copyValues(OperationDefinition dst) { 4630 super.copyValues(dst); 4631 dst.url = url == null ? null : url.copy(); 4632 dst.version = version == null ? null : version.copy(); 4633 dst.name = name == null ? null : name.copy(); 4634 dst.title = title == null ? null : title.copy(); 4635 dst.status = status == null ? null : status.copy(); 4636 dst.kind = kind == null ? null : kind.copy(); 4637 dst.experimental = experimental == null ? null : experimental.copy(); 4638 dst.date = date == null ? null : date.copy(); 4639 dst.publisher = publisher == null ? null : publisher.copy(); 4640 if (contact != null) { 4641 dst.contact = new ArrayList<ContactDetail>(); 4642 for (ContactDetail i : contact) 4643 dst.contact.add(i.copy()); 4644 } 4645 ; 4646 dst.description = description == null ? null : description.copy(); 4647 if (useContext != null) { 4648 dst.useContext = new ArrayList<UsageContext>(); 4649 for (UsageContext i : useContext) 4650 dst.useContext.add(i.copy()); 4651 } 4652 ; 4653 if (jurisdiction != null) { 4654 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4655 for (CodeableConcept i : jurisdiction) 4656 dst.jurisdiction.add(i.copy()); 4657 } 4658 ; 4659 dst.purpose = purpose == null ? null : purpose.copy(); 4660 dst.affectsState = affectsState == null ? null : affectsState.copy(); 4661 dst.code = code == null ? null : code.copy(); 4662 dst.comment = comment == null ? null : comment.copy(); 4663 dst.base = base == null ? null : base.copy(); 4664 if (resource != null) { 4665 dst.resource = new ArrayList<CodeType>(); 4666 for (CodeType i : resource) 4667 dst.resource.add(i.copy()); 4668 } 4669 ; 4670 dst.system = system == null ? null : system.copy(); 4671 dst.type = type == null ? null : type.copy(); 4672 dst.instance = instance == null ? null : instance.copy(); 4673 dst.inputProfile = inputProfile == null ? null : inputProfile.copy(); 4674 dst.outputProfile = outputProfile == null ? null : outputProfile.copy(); 4675 if (parameter != null) { 4676 dst.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 4677 for (OperationDefinitionParameterComponent i : parameter) 4678 dst.parameter.add(i.copy()); 4679 } 4680 ; 4681 if (overload != null) { 4682 dst.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 4683 for (OperationDefinitionOverloadComponent i : overload) 4684 dst.overload.add(i.copy()); 4685 } 4686 ; 4687 } 4688 4689 protected OperationDefinition typedCopy() { 4690 return copy(); 4691 } 4692 4693 @Override 4694 public boolean equalsDeep(Base other_) { 4695 if (!super.equalsDeep(other_)) 4696 return false; 4697 if (!(other_ instanceof OperationDefinition)) 4698 return false; 4699 OperationDefinition o = (OperationDefinition) other_; 4700 return compareDeep(kind, o.kind, true) && compareDeep(purpose, o.purpose, true) 4701 && compareDeep(affectsState, o.affectsState, true) && compareDeep(code, o.code, true) 4702 && compareDeep(comment, o.comment, true) && compareDeep(base, o.base, true) 4703 && compareDeep(resource, o.resource, true) && compareDeep(system, o.system, true) 4704 && compareDeep(type, o.type, true) && compareDeep(instance, o.instance, true) 4705 && compareDeep(inputProfile, o.inputProfile, true) && compareDeep(outputProfile, o.outputProfile, true) 4706 && compareDeep(parameter, o.parameter, true) && compareDeep(overload, o.overload, true); 4707 } 4708 4709 @Override 4710 public boolean equalsShallow(Base other_) { 4711 if (!super.equalsShallow(other_)) 4712 return false; 4713 if (!(other_ instanceof OperationDefinition)) 4714 return false; 4715 OperationDefinition o = (OperationDefinition) other_; 4716 return compareValues(kind, o.kind, true) && compareValues(purpose, o.purpose, true) 4717 && compareValues(affectsState, o.affectsState, true) && compareValues(code, o.code, true) 4718 && compareValues(comment, o.comment, true) && compareValues(resource, o.resource, true) 4719 && compareValues(system, o.system, true) && compareValues(type, o.type, true) 4720 && compareValues(instance, o.instance, true); 4721 } 4722 4723 public boolean isEmpty() { 4724 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, purpose, affectsState, code, comment, base, 4725 resource, system, type, instance, inputProfile, outputProfile, parameter, overload); 4726 } 4727 4728 @Override 4729 public ResourceType getResourceType() { 4730 return ResourceType.OperationDefinition; 4731 } 4732 4733 /** 4734 * Search parameter: <b>date</b> 4735 * <p> 4736 * Description: <b>The operation definition publication date</b><br> 4737 * Type: <b>date</b><br> 4738 * Path: <b>OperationDefinition.date</b><br> 4739 * </p> 4740 */ 4741 @SearchParamDefinition(name = "date", path = "OperationDefinition.date", description = "The operation definition publication date", type = "date") 4742 public static final String SP_DATE = "date"; 4743 /** 4744 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4745 * <p> 4746 * Description: <b>The operation definition publication date</b><br> 4747 * Type: <b>date</b><br> 4748 * Path: <b>OperationDefinition.date</b><br> 4749 * </p> 4750 */ 4751 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4752 SP_DATE); 4753 4754 /** 4755 * Search parameter: <b>code</b> 4756 * <p> 4757 * Description: <b>Name used to invoke the operation</b><br> 4758 * Type: <b>token</b><br> 4759 * Path: <b>OperationDefinition.code</b><br> 4760 * </p> 4761 */ 4762 @SearchParamDefinition(name = "code", path = "OperationDefinition.code", description = "Name used to invoke the operation", type = "token") 4763 public static final String SP_CODE = "code"; 4764 /** 4765 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4766 * <p> 4767 * Description: <b>Name used to invoke the operation</b><br> 4768 * Type: <b>token</b><br> 4769 * Path: <b>OperationDefinition.code</b><br> 4770 * </p> 4771 */ 4772 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4773 SP_CODE); 4774 4775 /** 4776 * Search parameter: <b>instance</b> 4777 * <p> 4778 * Description: <b>Invoke on an instance?</b><br> 4779 * Type: <b>token</b><br> 4780 * Path: <b>OperationDefinition.instance</b><br> 4781 * </p> 4782 */ 4783 @SearchParamDefinition(name = "instance", path = "OperationDefinition.instance", description = "Invoke on an instance?", type = "token") 4784 public static final String SP_INSTANCE = "instance"; 4785 /** 4786 * <b>Fluent Client</b> search parameter constant for <b>instance</b> 4787 * <p> 4788 * Description: <b>Invoke on an instance?</b><br> 4789 * Type: <b>token</b><br> 4790 * Path: <b>OperationDefinition.instance</b><br> 4791 * </p> 4792 */ 4793 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INSTANCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4794 SP_INSTANCE); 4795 4796 /** 4797 * Search parameter: <b>context-type-value</b> 4798 * <p> 4799 * Description: <b>A use context type and value assigned to the operation 4800 * definition</b><br> 4801 * Type: <b>composite</b><br> 4802 * Path: <b></b><br> 4803 * </p> 4804 */ 4805 @SearchParamDefinition(name = "context-type-value", path = "OperationDefinition.useContext", description = "A use context type and value assigned to the operation definition", type = "composite", compositeOf = { 4806 "context-type", "context" }) 4807 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4808 /** 4809 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4810 * <p> 4811 * Description: <b>A use context type and value assigned to the operation 4812 * definition</b><br> 4813 * Type: <b>composite</b><br> 4814 * Path: <b></b><br> 4815 * </p> 4816 */ 4817 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4818 SP_CONTEXT_TYPE_VALUE); 4819 4820 /** 4821 * Search parameter: <b>kind</b> 4822 * <p> 4823 * Description: <b>operation | query</b><br> 4824 * Type: <b>token</b><br> 4825 * Path: <b>OperationDefinition.kind</b><br> 4826 * </p> 4827 */ 4828 @SearchParamDefinition(name = "kind", path = "OperationDefinition.kind", description = "operation | query", type = "token") 4829 public static final String SP_KIND = "kind"; 4830 /** 4831 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 4832 * <p> 4833 * Description: <b>operation | query</b><br> 4834 * Type: <b>token</b><br> 4835 * Path: <b>OperationDefinition.kind</b><br> 4836 * </p> 4837 */ 4838 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4839 SP_KIND); 4840 4841 /** 4842 * Search parameter: <b>jurisdiction</b> 4843 * <p> 4844 * Description: <b>Intended jurisdiction for the operation definition</b><br> 4845 * Type: <b>token</b><br> 4846 * Path: <b>OperationDefinition.jurisdiction</b><br> 4847 * </p> 4848 */ 4849 @SearchParamDefinition(name = "jurisdiction", path = "OperationDefinition.jurisdiction", description = "Intended jurisdiction for the operation definition", type = "token") 4850 public static final String SP_JURISDICTION = "jurisdiction"; 4851 /** 4852 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4853 * <p> 4854 * Description: <b>Intended jurisdiction for the operation definition</b><br> 4855 * Type: <b>token</b><br> 4856 * Path: <b>OperationDefinition.jurisdiction</b><br> 4857 * </p> 4858 */ 4859 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4860 SP_JURISDICTION); 4861 4862 /** 4863 * Search parameter: <b>description</b> 4864 * <p> 4865 * Description: <b>The description of the operation definition</b><br> 4866 * Type: <b>string</b><br> 4867 * Path: <b>OperationDefinition.description</b><br> 4868 * </p> 4869 */ 4870 @SearchParamDefinition(name = "description", path = "OperationDefinition.description", description = "The description of the operation definition", type = "string") 4871 public static final String SP_DESCRIPTION = "description"; 4872 /** 4873 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4874 * <p> 4875 * Description: <b>The description of the operation definition</b><br> 4876 * Type: <b>string</b><br> 4877 * Path: <b>OperationDefinition.description</b><br> 4878 * </p> 4879 */ 4880 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4881 SP_DESCRIPTION); 4882 4883 /** 4884 * Search parameter: <b>context-type</b> 4885 * <p> 4886 * Description: <b>A type of use context assigned to the operation 4887 * definition</b><br> 4888 * Type: <b>token</b><br> 4889 * Path: <b>OperationDefinition.useContext.code</b><br> 4890 * </p> 4891 */ 4892 @SearchParamDefinition(name = "context-type", path = "OperationDefinition.useContext.code", description = "A type of use context assigned to the operation definition", type = "token") 4893 public static final String SP_CONTEXT_TYPE = "context-type"; 4894 /** 4895 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4896 * <p> 4897 * Description: <b>A type of use context assigned to the operation 4898 * definition</b><br> 4899 * Type: <b>token</b><br> 4900 * Path: <b>OperationDefinition.useContext.code</b><br> 4901 * </p> 4902 */ 4903 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4904 SP_CONTEXT_TYPE); 4905 4906 /** 4907 * Search parameter: <b>title</b> 4908 * <p> 4909 * Description: <b>The human-friendly name of the operation definition</b><br> 4910 * Type: <b>string</b><br> 4911 * Path: <b>OperationDefinition.title</b><br> 4912 * </p> 4913 */ 4914 @SearchParamDefinition(name = "title", path = "OperationDefinition.title", description = "The human-friendly name of the operation definition", type = "string") 4915 public static final String SP_TITLE = "title"; 4916 /** 4917 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4918 * <p> 4919 * Description: <b>The human-friendly name of the operation definition</b><br> 4920 * Type: <b>string</b><br> 4921 * Path: <b>OperationDefinition.title</b><br> 4922 * </p> 4923 */ 4924 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4925 SP_TITLE); 4926 4927 /** 4928 * Search parameter: <b>type</b> 4929 * <p> 4930 * Description: <b>Invoke at the type level?</b><br> 4931 * Type: <b>token</b><br> 4932 * Path: <b>OperationDefinition.type</b><br> 4933 * </p> 4934 */ 4935 @SearchParamDefinition(name = "type", path = "OperationDefinition.type", description = "Invoke at the type level?", type = "token") 4936 public static final String SP_TYPE = "type"; 4937 /** 4938 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4939 * <p> 4940 * Description: <b>Invoke at the type level?</b><br> 4941 * Type: <b>token</b><br> 4942 * Path: <b>OperationDefinition.type</b><br> 4943 * </p> 4944 */ 4945 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4946 SP_TYPE); 4947 4948 /** 4949 * Search parameter: <b>version</b> 4950 * <p> 4951 * Description: <b>The business version of the operation definition</b><br> 4952 * Type: <b>token</b><br> 4953 * Path: <b>OperationDefinition.version</b><br> 4954 * </p> 4955 */ 4956 @SearchParamDefinition(name = "version", path = "OperationDefinition.version", description = "The business version of the operation definition", type = "token") 4957 public static final String SP_VERSION = "version"; 4958 /** 4959 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4960 * <p> 4961 * Description: <b>The business version of the operation definition</b><br> 4962 * Type: <b>token</b><br> 4963 * Path: <b>OperationDefinition.version</b><br> 4964 * </p> 4965 */ 4966 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4967 SP_VERSION); 4968 4969 /** 4970 * Search parameter: <b>url</b> 4971 * <p> 4972 * Description: <b>The uri that identifies the operation definition</b><br> 4973 * Type: <b>uri</b><br> 4974 * Path: <b>OperationDefinition.url</b><br> 4975 * </p> 4976 */ 4977 @SearchParamDefinition(name = "url", path = "OperationDefinition.url", description = "The uri that identifies the operation definition", type = "uri") 4978 public static final String SP_URL = "url"; 4979 /** 4980 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4981 * <p> 4982 * Description: <b>The uri that identifies the operation definition</b><br> 4983 * Type: <b>uri</b><br> 4984 * Path: <b>OperationDefinition.url</b><br> 4985 * </p> 4986 */ 4987 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4988 4989 /** 4990 * Search parameter: <b>context-quantity</b> 4991 * <p> 4992 * Description: <b>A quantity- or range-valued use context assigned to the 4993 * operation definition</b><br> 4994 * Type: <b>quantity</b><br> 4995 * Path: <b>OperationDefinition.useContext.valueQuantity, 4996 * OperationDefinition.useContext.valueRange</b><br> 4997 * </p> 4998 */ 4999 @SearchParamDefinition(name = "context-quantity", path = "(OperationDefinition.useContext.value as Quantity) | (OperationDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the operation definition", type = "quantity") 5000 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5001 /** 5002 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5003 * <p> 5004 * Description: <b>A quantity- or range-valued use context assigned to the 5005 * operation definition</b><br> 5006 * Type: <b>quantity</b><br> 5007 * Path: <b>OperationDefinition.useContext.valueQuantity, 5008 * OperationDefinition.useContext.valueRange</b><br> 5009 * </p> 5010 */ 5011 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5012 SP_CONTEXT_QUANTITY); 5013 5014 /** 5015 * Search parameter: <b>input-profile</b> 5016 * <p> 5017 * Description: <b>Validation information for in parameters</b><br> 5018 * Type: <b>reference</b><br> 5019 * Path: <b>OperationDefinition.inputProfile</b><br> 5020 * </p> 5021 */ 5022 @SearchParamDefinition(name = "input-profile", path = "OperationDefinition.inputProfile", description = "Validation information for in parameters", type = "reference", target = { 5023 StructureDefinition.class }) 5024 public static final String SP_INPUT_PROFILE = "input-profile"; 5025 /** 5026 * <b>Fluent Client</b> search parameter constant for <b>input-profile</b> 5027 * <p> 5028 * Description: <b>Validation information for in parameters</b><br> 5029 * Type: <b>reference</b><br> 5030 * Path: <b>OperationDefinition.inputProfile</b><br> 5031 * </p> 5032 */ 5033 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INPUT_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5034 SP_INPUT_PROFILE); 5035 5036 /** 5037 * Constant for fluent queries to be used to add include statements. Specifies 5038 * the path value of "<b>OperationDefinition:input-profile</b>". 5039 */ 5040 public static final ca.uhn.fhir.model.api.Include INCLUDE_INPUT_PROFILE = new ca.uhn.fhir.model.api.Include( 5041 "OperationDefinition:input-profile").toLocked(); 5042 5043 /** 5044 * Search parameter: <b>output-profile</b> 5045 * <p> 5046 * Description: <b>Validation information for out parameters</b><br> 5047 * Type: <b>reference</b><br> 5048 * Path: <b>OperationDefinition.outputProfile</b><br> 5049 * </p> 5050 */ 5051 @SearchParamDefinition(name = "output-profile", path = "OperationDefinition.outputProfile", description = "Validation information for out parameters", type = "reference", target = { 5052 StructureDefinition.class }) 5053 public static final String SP_OUTPUT_PROFILE = "output-profile"; 5054 /** 5055 * <b>Fluent Client</b> search parameter constant for <b>output-profile</b> 5056 * <p> 5057 * Description: <b>Validation information for out parameters</b><br> 5058 * Type: <b>reference</b><br> 5059 * Path: <b>OperationDefinition.outputProfile</b><br> 5060 * </p> 5061 */ 5062 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OUTPUT_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5063 SP_OUTPUT_PROFILE); 5064 5065 /** 5066 * Constant for fluent queries to be used to add include statements. Specifies 5067 * the path value of "<b>OperationDefinition:output-profile</b>". 5068 */ 5069 public static final ca.uhn.fhir.model.api.Include INCLUDE_OUTPUT_PROFILE = new ca.uhn.fhir.model.api.Include( 5070 "OperationDefinition:output-profile").toLocked(); 5071 5072 /** 5073 * Search parameter: <b>system</b> 5074 * <p> 5075 * Description: <b>Invoke at the system level?</b><br> 5076 * Type: <b>token</b><br> 5077 * Path: <b>OperationDefinition.system</b><br> 5078 * </p> 5079 */ 5080 @SearchParamDefinition(name = "system", path = "OperationDefinition.system", description = "Invoke at the system level?", type = "token") 5081 public static final String SP_SYSTEM = "system"; 5082 /** 5083 * <b>Fluent Client</b> search parameter constant for <b>system</b> 5084 * <p> 5085 * Description: <b>Invoke at the system level?</b><br> 5086 * Type: <b>token</b><br> 5087 * Path: <b>OperationDefinition.system</b><br> 5088 * </p> 5089 */ 5090 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5091 SP_SYSTEM); 5092 5093 /** 5094 * Search parameter: <b>name</b> 5095 * <p> 5096 * Description: <b>Computationally friendly name of the operation 5097 * definition</b><br> 5098 * Type: <b>string</b><br> 5099 * Path: <b>OperationDefinition.name</b><br> 5100 * </p> 5101 */ 5102 @SearchParamDefinition(name = "name", path = "OperationDefinition.name", description = "Computationally friendly name of the operation definition", type = "string") 5103 public static final String SP_NAME = "name"; 5104 /** 5105 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5106 * <p> 5107 * Description: <b>Computationally friendly name of the operation 5108 * definition</b><br> 5109 * Type: <b>string</b><br> 5110 * Path: <b>OperationDefinition.name</b><br> 5111 * </p> 5112 */ 5113 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5114 SP_NAME); 5115 5116 /** 5117 * Search parameter: <b>context</b> 5118 * <p> 5119 * Description: <b>A use context assigned to the operation definition</b><br> 5120 * Type: <b>token</b><br> 5121 * Path: <b>OperationDefinition.useContext.valueCodeableConcept</b><br> 5122 * </p> 5123 */ 5124 @SearchParamDefinition(name = "context", path = "(OperationDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the operation definition", type = "token") 5125 public static final String SP_CONTEXT = "context"; 5126 /** 5127 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5128 * <p> 5129 * Description: <b>A use context assigned to the operation definition</b><br> 5130 * Type: <b>token</b><br> 5131 * Path: <b>OperationDefinition.useContext.valueCodeableConcept</b><br> 5132 * </p> 5133 */ 5134 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5135 SP_CONTEXT); 5136 5137 /** 5138 * Search parameter: <b>publisher</b> 5139 * <p> 5140 * Description: <b>Name of the publisher of the operation definition</b><br> 5141 * Type: <b>string</b><br> 5142 * Path: <b>OperationDefinition.publisher</b><br> 5143 * </p> 5144 */ 5145 @SearchParamDefinition(name = "publisher", path = "OperationDefinition.publisher", description = "Name of the publisher of the operation definition", type = "string") 5146 public static final String SP_PUBLISHER = "publisher"; 5147 /** 5148 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5149 * <p> 5150 * Description: <b>Name of the publisher of the operation definition</b><br> 5151 * Type: <b>string</b><br> 5152 * Path: <b>OperationDefinition.publisher</b><br> 5153 * </p> 5154 */ 5155 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5156 SP_PUBLISHER); 5157 5158 /** 5159 * Search parameter: <b>context-type-quantity</b> 5160 * <p> 5161 * Description: <b>A use context type and quantity- or range-based value 5162 * assigned to the operation definition</b><br> 5163 * Type: <b>composite</b><br> 5164 * Path: <b></b><br> 5165 * </p> 5166 */ 5167 @SearchParamDefinition(name = "context-type-quantity", path = "OperationDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the operation definition", type = "composite", compositeOf = { 5168 "context-type", "context-quantity" }) 5169 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5170 /** 5171 * <b>Fluent Client</b> search parameter constant for 5172 * <b>context-type-quantity</b> 5173 * <p> 5174 * Description: <b>A use context type and quantity- or range-based value 5175 * assigned to the operation definition</b><br> 5176 * Type: <b>composite</b><br> 5177 * Path: <b></b><br> 5178 * </p> 5179 */ 5180 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 5181 SP_CONTEXT_TYPE_QUANTITY); 5182 5183 /** 5184 * Search parameter: <b>status</b> 5185 * <p> 5186 * Description: <b>The current status of the operation definition</b><br> 5187 * Type: <b>token</b><br> 5188 * Path: <b>OperationDefinition.status</b><br> 5189 * </p> 5190 */ 5191 @SearchParamDefinition(name = "status", path = "OperationDefinition.status", description = "The current status of the operation definition", type = "token") 5192 public static final String SP_STATUS = "status"; 5193 /** 5194 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5195 * <p> 5196 * Description: <b>The current status of the operation definition</b><br> 5197 * Type: <b>token</b><br> 5198 * Path: <b>OperationDefinition.status</b><br> 5199 * </p> 5200 */ 5201 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5202 SP_STATUS); 5203 5204 /** 5205 * Search parameter: <b>base</b> 5206 * <p> 5207 * Description: <b>Marks this as a profile of the base</b><br> 5208 * Type: <b>reference</b><br> 5209 * Path: <b>OperationDefinition.base</b><br> 5210 * </p> 5211 */ 5212 @SearchParamDefinition(name = "base", path = "OperationDefinition.base", description = "Marks this as a profile of the base", type = "reference", target = { 5213 OperationDefinition.class }) 5214 public static final String SP_BASE = "base"; 5215 /** 5216 * <b>Fluent Client</b> search parameter constant for <b>base</b> 5217 * <p> 5218 * Description: <b>Marks this as a profile of the base</b><br> 5219 * Type: <b>reference</b><br> 5220 * Path: <b>OperationDefinition.base</b><br> 5221 * </p> 5222 */ 5223 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5224 SP_BASE); 5225 5226 /** 5227 * Constant for fluent queries to be used to add include statements. Specifies 5228 * the path value of "<b>OperationDefinition:base</b>". 5229 */ 5230 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include( 5231 "OperationDefinition:base").toLocked(); 5232 5233}