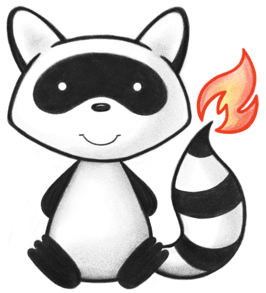
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.BindingStrength; 040import org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory; 041import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 042import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 043import org.hl7.fhir.r4.model.Enumerations.SearchParamType; 044import org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.ChildOrder; 050import ca.uhn.fhir.model.api.annotation.Description; 051import ca.uhn.fhir.model.api.annotation.ResourceDef; 052import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 053 054/** 055 * A formal computable definition of an operation (on the RESTful interface) or 056 * a named query (using the search interaction). 057 */ 058@ResourceDef(name = "OperationDefinition", profile = "http://hl7.org/fhir/StructureDefinition/OperationDefinition") 059@ChildOrder(names = { "url", "version", "name", "title", "status", "kind", "experimental", "date", "publisher", 060 "contact", "description", "useContext", "jurisdiction", "purpose", "affectsState", "code", "comment", "base", 061 "resource", "system", "type", "instance", "inputProfile", "outputProfile", "parameter", "overload" }) 062public class OperationDefinition extends MetadataResource { 063 064 public enum OperationKind { 065 /** 066 * This operation is invoked as an operation. 067 */ 068 OPERATION, 069 /** 070 * This operation is a named query, invoked using the search mechanism. 071 */ 072 QUERY, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static OperationKind fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("operation".equals(codeString)) 082 return OPERATION; 083 if ("query".equals(codeString)) 084 return QUERY; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown OperationKind code '" + codeString + "'"); 089 } 090 091 public String toCode() { 092 switch (this) { 093 case OPERATION: 094 return "operation"; 095 case QUERY: 096 return "query"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case OPERATION: 107 return "http://hl7.org/fhir/operation-kind"; 108 case QUERY: 109 return "http://hl7.org/fhir/operation-kind"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case OPERATION: 120 return "This operation is invoked as an operation."; 121 case QUERY: 122 return "This operation is a named query, invoked using the search mechanism."; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDisplay() { 131 switch (this) { 132 case OPERATION: 133 return "Operation"; 134 case QUERY: 135 return "Query"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 } 143 144 public static class OperationKindEnumFactory implements EnumFactory<OperationKind> { 145 public OperationKind fromCode(String codeString) throws IllegalArgumentException { 146 if (codeString == null || "".equals(codeString)) 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("operation".equals(codeString)) 150 return OperationKind.OPERATION; 151 if ("query".equals(codeString)) 152 return OperationKind.QUERY; 153 throw new IllegalArgumentException("Unknown OperationKind code '" + codeString + "'"); 154 } 155 156 public Enumeration<OperationKind> fromType(PrimitiveType<?> code) throws FHIRException { 157 if (code == null) 158 return null; 159 if (code.isEmpty()) 160 return new Enumeration<OperationKind>(this, OperationKind.NULL, code); 161 String codeString = code.asStringValue(); 162 if (codeString == null || "".equals(codeString)) 163 return new Enumeration<OperationKind>(this, OperationKind.NULL, code); 164 if ("operation".equals(codeString)) 165 return new Enumeration<OperationKind>(this, OperationKind.OPERATION, code); 166 if ("query".equals(codeString)) 167 return new Enumeration<OperationKind>(this, OperationKind.QUERY, code); 168 throw new FHIRException("Unknown OperationKind code '" + codeString + "'"); 169 } 170 171 public String toCode(OperationKind code) { 172 if (code == OperationKind.OPERATION) 173 return "operation"; 174 if (code == OperationKind.QUERY) 175 return "query"; 176 return "?"; 177 } 178 179 public String toSystem(OperationKind code) { 180 return code.getSystem(); 181 } 182 } 183 184 public enum OperationParameterUse { 185 /** 186 * This is an input parameter. 187 */ 188 IN, 189 /** 190 * This is an output parameter. 191 */ 192 OUT, 193 /** 194 * added to help the parsers with the generic types 195 */ 196 NULL; 197 198 public static OperationParameterUse fromCode(String codeString) throws FHIRException { 199 if (codeString == null || "".equals(codeString)) 200 return null; 201 if ("in".equals(codeString)) 202 return IN; 203 if ("out".equals(codeString)) 204 return OUT; 205 if (Configuration.isAcceptInvalidEnums()) 206 return null; 207 else 208 throw new FHIRException("Unknown OperationParameterUse code '" + codeString + "'"); 209 } 210 211 public String toCode() { 212 switch (this) { 213 case IN: 214 return "in"; 215 case OUT: 216 return "out"; 217 case NULL: 218 return null; 219 default: 220 return "?"; 221 } 222 } 223 224 public String getSystem() { 225 switch (this) { 226 case IN: 227 return "http://hl7.org/fhir/operation-parameter-use"; 228 case OUT: 229 return "http://hl7.org/fhir/operation-parameter-use"; 230 case NULL: 231 return null; 232 default: 233 return "?"; 234 } 235 } 236 237 public String getDefinition() { 238 switch (this) { 239 case IN: 240 return "This is an input parameter."; 241 case OUT: 242 return "This is an output parameter."; 243 case NULL: 244 return null; 245 default: 246 return "?"; 247 } 248 } 249 250 public String getDisplay() { 251 switch (this) { 252 case IN: 253 return "In"; 254 case OUT: 255 return "Out"; 256 case NULL: 257 return null; 258 default: 259 return "?"; 260 } 261 } 262 } 263 264 public static class OperationParameterUseEnumFactory implements EnumFactory<OperationParameterUse> { 265 public OperationParameterUse fromCode(String codeString) throws IllegalArgumentException { 266 if (codeString == null || "".equals(codeString)) 267 if (codeString == null || "".equals(codeString)) 268 return null; 269 if ("in".equals(codeString)) 270 return OperationParameterUse.IN; 271 if ("out".equals(codeString)) 272 return OperationParameterUse.OUT; 273 throw new IllegalArgumentException("Unknown OperationParameterUse code '" + codeString + "'"); 274 } 275 276 public Enumeration<OperationParameterUse> fromType(PrimitiveType<?> code) throws FHIRException { 277 if (code == null) 278 return null; 279 if (code.isEmpty()) 280 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.NULL, code); 281 String codeString = code.asStringValue(); 282 if (codeString == null || "".equals(codeString)) 283 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.NULL, code); 284 if ("in".equals(codeString)) 285 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.IN, code); 286 if ("out".equals(codeString)) 287 return new Enumeration<OperationParameterUse>(this, OperationParameterUse.OUT, code); 288 throw new FHIRException("Unknown OperationParameterUse code '" + codeString + "'"); 289 } 290 291 public String toCode(OperationParameterUse code) { 292 if (code == OperationParameterUse.IN) 293 return "in"; 294 if (code == OperationParameterUse.OUT) 295 return "out"; 296 return "?"; 297 } 298 299 public String toSystem(OperationParameterUse code) { 300 return code.getSystem(); 301 } 302 } 303 304 @Block() 305 public static class OperationDefinitionParameterComponent extends BackboneElement implements IBaseBackboneElement { 306 /** 307 * The name of used to identify the parameter. 308 */ 309 @Child(name = "name", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 310 @Description(shortDefinition = "Name in Parameters.parameter.name or in URL", formalDefinition = "The name of used to identify the parameter.") 311 protected CodeType name; 312 313 /** 314 * Whether this is an input or an output parameter. 315 */ 316 @Child(name = "use", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 317 @Description(shortDefinition = "in | out", formalDefinition = "Whether this is an input or an output parameter.") 318 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/operation-parameter-use") 319 protected Enumeration<OperationParameterUse> use; 320 321 /** 322 * The minimum number of times this parameter SHALL appear in the request or 323 * response. 324 */ 325 @Child(name = "min", type = { IntegerType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 326 @Description(shortDefinition = "Minimum Cardinality", formalDefinition = "The minimum number of times this parameter SHALL appear in the request or response.") 327 protected IntegerType min; 328 329 /** 330 * The maximum number of times this element is permitted to appear in the 331 * request or response. 332 */ 333 @Child(name = "max", type = { StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 334 @Description(shortDefinition = "Maximum Cardinality (a number or *)", formalDefinition = "The maximum number of times this element is permitted to appear in the request or response.") 335 protected StringType max; 336 337 /** 338 * Describes the meaning or use of this parameter. 339 */ 340 @Child(name = "documentation", type = { 341 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 342 @Description(shortDefinition = "Description of meaning/use", formalDefinition = "Describes the meaning or use of this parameter.") 343 protected StringType documentation; 344 345 /** 346 * The type for this parameter. 347 */ 348 @Child(name = "type", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 349 @Description(shortDefinition = "What type this parameter has", formalDefinition = "The type for this parameter.") 350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/all-types") 351 protected CodeType type; 352 353 /** 354 * Used when the type is "Reference" or "canonical", and identifies a profile 355 * structure or implementation Guide that applies to the target of the reference 356 * this parameter refers to. If any profiles are specified, then the content 357 * must conform to at least one of them. The URL can be a local reference - to a 358 * contained StructureDefinition, or a reference to another StructureDefinition 359 * or Implementation Guide by a canonical URL. When an implementation guide is 360 * specified, the target resource SHALL conform to at least one profile defined 361 * in the implementation guide. 362 */ 363 @Child(name = "targetProfile", type = { 364 CanonicalType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 365 @Description(shortDefinition = "If type is Reference | canonical, allowed targets", formalDefinition = "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.") 366 protected List<CanonicalType> targetProfile; 367 368 /** 369 * How the parameter is understood as a search parameter. This is only used if 370 * the parameter type is 'string'. 371 */ 372 @Child(name = "searchType", type = { 373 CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 374 @Description(shortDefinition = "number | date | string | token | reference | composite | quantity | uri | special", formalDefinition = "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.") 375 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/search-param-type") 376 protected Enumeration<SearchParamType> searchType; 377 378 /** 379 * Binds to a value set if this parameter is coded (code, Coding, 380 * CodeableConcept). 381 */ 382 @Child(name = "binding", type = {}, order = 9, min = 0, max = 1, modifier = false, summary = false) 383 @Description(shortDefinition = "ValueSet details if this is coded", formalDefinition = "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).") 384 protected OperationDefinitionParameterBindingComponent binding; 385 386 /** 387 * Identifies other resource parameters within the operation invocation that are 388 * expected to resolve to this resource. 389 */ 390 @Child(name = "referencedFrom", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 391 @Description(shortDefinition = "References to this parameter", formalDefinition = "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.") 392 protected List<OperationDefinitionParameterReferencedFromComponent> referencedFrom; 393 394 /** 395 * The parts of a nested Parameter. 396 */ 397 @Child(name = "part", type = { 398 OperationDefinitionParameterComponent.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 399 @Description(shortDefinition = "Parts of a nested Parameter", formalDefinition = "The parts of a nested Parameter.") 400 protected List<OperationDefinitionParameterComponent> part; 401 402 private static final long serialVersionUID = 1715661531L; 403 404 /** 405 * Constructor 406 */ 407 public OperationDefinitionParameterComponent() { 408 super(); 409 } 410 411 /** 412 * Constructor 413 */ 414 public OperationDefinitionParameterComponent(CodeType name, Enumeration<OperationParameterUse> use, IntegerType min, 415 StringType max) { 416 super(); 417 this.name = name; 418 this.use = use; 419 this.min = min; 420 this.max = max; 421 } 422 423 /** 424 * @return {@link #name} (The name of used to identify the parameter.). This is 425 * the underlying object with id, value and extensions. The accessor 426 * "getName" gives direct access to the value 427 */ 428 public CodeType getNameElement() { 429 if (this.name == null) 430 if (Configuration.errorOnAutoCreate()) 431 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.name"); 432 else if (Configuration.doAutoCreate()) 433 this.name = new CodeType(); // bb 434 return this.name; 435 } 436 437 public boolean hasNameElement() { 438 return this.name != null && !this.name.isEmpty(); 439 } 440 441 public boolean hasName() { 442 return this.name != null && !this.name.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #name} (The name of used to identify the parameter.). 447 * This is the underlying object with id, value and extensions. The 448 * accessor "getName" gives direct access to the value 449 */ 450 public OperationDefinitionParameterComponent setNameElement(CodeType value) { 451 this.name = value; 452 return this; 453 } 454 455 /** 456 * @return The name of used to identify the parameter. 457 */ 458 public String getName() { 459 return this.name == null ? null : this.name.getValue(); 460 } 461 462 /** 463 * @param value The name of used to identify the parameter. 464 */ 465 public OperationDefinitionParameterComponent setName(String value) { 466 if (this.name == null) 467 this.name = new CodeType(); 468 this.name.setValue(value); 469 return this; 470 } 471 472 /** 473 * @return {@link #use} (Whether this is an input or an output parameter.). This 474 * is the underlying object with id, value and extensions. The accessor 475 * "getUse" gives direct access to the value 476 */ 477 public Enumeration<OperationParameterUse> getUseElement() { 478 if (this.use == null) 479 if (Configuration.errorOnAutoCreate()) 480 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.use"); 481 else if (Configuration.doAutoCreate()) 482 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); // bb 483 return this.use; 484 } 485 486 public boolean hasUseElement() { 487 return this.use != null && !this.use.isEmpty(); 488 } 489 490 public boolean hasUse() { 491 return this.use != null && !this.use.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #use} (Whether this is an input or an output parameter.). 496 * This is the underlying object with id, value and extensions. The 497 * accessor "getUse" gives direct access to the value 498 */ 499 public OperationDefinitionParameterComponent setUseElement(Enumeration<OperationParameterUse> value) { 500 this.use = value; 501 return this; 502 } 503 504 /** 505 * @return Whether this is an input or an output parameter. 506 */ 507 public OperationParameterUse getUse() { 508 return this.use == null ? null : this.use.getValue(); 509 } 510 511 /** 512 * @param value Whether this is an input or an output parameter. 513 */ 514 public OperationDefinitionParameterComponent setUse(OperationParameterUse value) { 515 if (this.use == null) 516 this.use = new Enumeration<OperationParameterUse>(new OperationParameterUseEnumFactory()); 517 this.use.setValue(value); 518 return this; 519 } 520 521 /** 522 * @return {@link #min} (The minimum number of times this parameter SHALL appear 523 * in the request or response.). This is the underlying object with id, 524 * value and extensions. The accessor "getMin" gives direct access to 525 * the value 526 */ 527 public IntegerType getMinElement() { 528 if (this.min == null) 529 if (Configuration.errorOnAutoCreate()) 530 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.min"); 531 else if (Configuration.doAutoCreate()) 532 this.min = new IntegerType(); // bb 533 return this.min; 534 } 535 536 public boolean hasMinElement() { 537 return this.min != null && !this.min.isEmpty(); 538 } 539 540 public boolean hasMin() { 541 return this.min != null && !this.min.isEmpty(); 542 } 543 544 /** 545 * @param value {@link #min} (The minimum number of times this parameter SHALL 546 * appear in the request or response.). This is the underlying 547 * object with id, value and extensions. The accessor "getMin" 548 * gives direct access to the value 549 */ 550 public OperationDefinitionParameterComponent setMinElement(IntegerType value) { 551 this.min = value; 552 return this; 553 } 554 555 /** 556 * @return The minimum number of times this parameter SHALL appear in the 557 * request or response. 558 */ 559 public int getMin() { 560 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 561 } 562 563 /** 564 * @param value The minimum number of times this parameter SHALL appear in the 565 * request or response. 566 */ 567 public OperationDefinitionParameterComponent setMin(int value) { 568 if (this.min == null) 569 this.min = new IntegerType(); 570 this.min.setValue(value); 571 return this; 572 } 573 574 /** 575 * @return {@link #max} (The maximum number of times this element is permitted 576 * to appear in the request or response.). This is the underlying object 577 * with id, value and extensions. The accessor "getMax" gives direct 578 * access to the value 579 */ 580 public StringType getMaxElement() { 581 if (this.max == null) 582 if (Configuration.errorOnAutoCreate()) 583 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.max"); 584 else if (Configuration.doAutoCreate()) 585 this.max = new StringType(); // bb 586 return this.max; 587 } 588 589 public boolean hasMaxElement() { 590 return this.max != null && !this.max.isEmpty(); 591 } 592 593 public boolean hasMax() { 594 return this.max != null && !this.max.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #max} (The maximum number of times this element is 599 * permitted to appear in the request or response.). This is the 600 * underlying object with id, value and extensions. The accessor 601 * "getMax" gives direct access to the value 602 */ 603 public OperationDefinitionParameterComponent setMaxElement(StringType value) { 604 this.max = value; 605 return this; 606 } 607 608 /** 609 * @return The maximum number of times this element is permitted to appear in 610 * the request or response. 611 */ 612 public String getMax() { 613 return this.max == null ? null : this.max.getValue(); 614 } 615 616 /** 617 * @param value The maximum number of times this element is permitted to appear 618 * in the request or response. 619 */ 620 public OperationDefinitionParameterComponent setMax(String value) { 621 if (this.max == null) 622 this.max = new StringType(); 623 this.max.setValue(value); 624 return this; 625 } 626 627 /** 628 * @return {@link #documentation} (Describes the meaning or use of this 629 * parameter.). This is the underlying object with id, value and 630 * extensions. The accessor "getDocumentation" gives direct access to 631 * the value 632 */ 633 public StringType getDocumentationElement() { 634 if (this.documentation == null) 635 if (Configuration.errorOnAutoCreate()) 636 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.documentation"); 637 else if (Configuration.doAutoCreate()) 638 this.documentation = new StringType(); // bb 639 return this.documentation; 640 } 641 642 public boolean hasDocumentationElement() { 643 return this.documentation != null && !this.documentation.isEmpty(); 644 } 645 646 public boolean hasDocumentation() { 647 return this.documentation != null && !this.documentation.isEmpty(); 648 } 649 650 /** 651 * @param value {@link #documentation} (Describes the meaning or use of this 652 * parameter.). This is the underlying object with id, value and 653 * extensions. The accessor "getDocumentation" gives direct access 654 * to the value 655 */ 656 public OperationDefinitionParameterComponent setDocumentationElement(StringType value) { 657 this.documentation = value; 658 return this; 659 } 660 661 /** 662 * @return Describes the meaning or use of this parameter. 663 */ 664 public String getDocumentation() { 665 return this.documentation == null ? null : this.documentation.getValue(); 666 } 667 668 /** 669 * @param value Describes the meaning or use of this parameter. 670 */ 671 public OperationDefinitionParameterComponent setDocumentation(String value) { 672 if (Utilities.noString(value)) 673 this.documentation = null; 674 else { 675 if (this.documentation == null) 676 this.documentation = new StringType(); 677 this.documentation.setValue(value); 678 } 679 return this; 680 } 681 682 /** 683 * @return {@link #type} (The type for this parameter.). This is the underlying 684 * object with id, value and extensions. The accessor "getType" gives 685 * direct access to the value 686 */ 687 public CodeType getTypeElement() { 688 if (this.type == null) 689 if (Configuration.errorOnAutoCreate()) 690 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.type"); 691 else if (Configuration.doAutoCreate()) 692 this.type = new CodeType(); // bb 693 return this.type; 694 } 695 696 public boolean hasTypeElement() { 697 return this.type != null && !this.type.isEmpty(); 698 } 699 700 public boolean hasType() { 701 return this.type != null && !this.type.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #type} (The type for this parameter.). This is the 706 * underlying object with id, value and extensions. The accessor 707 * "getType" gives direct access to the value 708 */ 709 public OperationDefinitionParameterComponent setTypeElement(CodeType value) { 710 this.type = value; 711 return this; 712 } 713 714 /** 715 * @return The type for this parameter. 716 */ 717 public String getType() { 718 return this.type == null ? null : this.type.getValue(); 719 } 720 721 /** 722 * @param value The type for this parameter. 723 */ 724 public OperationDefinitionParameterComponent setType(String value) { 725 if (Utilities.noString(value)) 726 this.type = null; 727 else { 728 if (this.type == null) 729 this.type = new CodeType(); 730 this.type.setValue(value); 731 } 732 return this; 733 } 734 735 /** 736 * @return {@link #targetProfile} (Used when the type is "Reference" or 737 * "canonical", and identifies a profile structure or implementation 738 * Guide that applies to the target of the reference this parameter 739 * refers to. If any profiles are specified, then the content must 740 * conform to at least one of them. The URL can be a local reference - 741 * to a contained StructureDefinition, or a reference to another 742 * StructureDefinition or Implementation Guide by a canonical URL. When 743 * an implementation guide is specified, the target resource SHALL 744 * conform to at least one profile defined in the implementation guide.) 745 */ 746 public List<CanonicalType> getTargetProfile() { 747 if (this.targetProfile == null) 748 this.targetProfile = new ArrayList<CanonicalType>(); 749 return this.targetProfile; 750 } 751 752 /** 753 * @return Returns a reference to <code>this</code> for easy method chaining 754 */ 755 public OperationDefinitionParameterComponent setTargetProfile(List<CanonicalType> theTargetProfile) { 756 this.targetProfile = theTargetProfile; 757 return this; 758 } 759 760 public boolean hasTargetProfile() { 761 if (this.targetProfile == null) 762 return false; 763 for (CanonicalType item : this.targetProfile) 764 if (!item.isEmpty()) 765 return true; 766 return false; 767 } 768 769 /** 770 * @return {@link #targetProfile} (Used when the type is "Reference" or 771 * "canonical", and identifies a profile structure or implementation 772 * Guide that applies to the target of the reference this parameter 773 * refers to. If any profiles are specified, then the content must 774 * conform to at least one of them. The URL can be a local reference - 775 * to a contained StructureDefinition, or a reference to another 776 * StructureDefinition or Implementation Guide by a canonical URL. When 777 * an implementation guide is specified, the target resource SHALL 778 * conform to at least one profile defined in the implementation guide.) 779 */ 780 public CanonicalType addTargetProfileElement() {// 2 781 CanonicalType t = new CanonicalType(); 782 if (this.targetProfile == null) 783 this.targetProfile = new ArrayList<CanonicalType>(); 784 this.targetProfile.add(t); 785 return t; 786 } 787 788 /** 789 * @param value {@link #targetProfile} (Used when the type is "Reference" or 790 * "canonical", and identifies a profile structure or 791 * implementation Guide that applies to the target of the reference 792 * this parameter refers to. If any profiles are specified, then 793 * the content must conform to at least one of them. The URL can be 794 * a local reference - to a contained StructureDefinition, or a 795 * reference to another StructureDefinition or Implementation Guide 796 * by a canonical URL. When an implementation guide is specified, 797 * the target resource SHALL conform to at least one profile 798 * defined in the implementation guide.) 799 */ 800 public OperationDefinitionParameterComponent addTargetProfile(String value) { // 1 801 CanonicalType t = new CanonicalType(); 802 t.setValue(value); 803 if (this.targetProfile == null) 804 this.targetProfile = new ArrayList<CanonicalType>(); 805 this.targetProfile.add(t); 806 return this; 807 } 808 809 /** 810 * @param value {@link #targetProfile} (Used when the type is "Reference" or 811 * "canonical", and identifies a profile structure or 812 * implementation Guide that applies to the target of the reference 813 * this parameter refers to. If any profiles are specified, then 814 * the content must conform to at least one of them. The URL can be 815 * a local reference - to a contained StructureDefinition, or a 816 * reference to another StructureDefinition or Implementation Guide 817 * by a canonical URL. When an implementation guide is specified, 818 * the target resource SHALL conform to at least one profile 819 * defined in the implementation guide.) 820 */ 821 public boolean hasTargetProfile(String value) { 822 if (this.targetProfile == null) 823 return false; 824 for (CanonicalType v : this.targetProfile) 825 if (v.getValue().equals(value)) // canonical(StructureDefinition) 826 return true; 827 return false; 828 } 829 830 /** 831 * @return {@link #searchType} (How the parameter is understood as a search 832 * parameter. This is only used if the parameter type is 'string'.). 833 * This is the underlying object with id, value and extensions. The 834 * accessor "getSearchType" gives direct access to the value 835 */ 836 public Enumeration<SearchParamType> getSearchTypeElement() { 837 if (this.searchType == null) 838 if (Configuration.errorOnAutoCreate()) 839 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.searchType"); 840 else if (Configuration.doAutoCreate()) 841 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); // bb 842 return this.searchType; 843 } 844 845 public boolean hasSearchTypeElement() { 846 return this.searchType != null && !this.searchType.isEmpty(); 847 } 848 849 public boolean hasSearchType() { 850 return this.searchType != null && !this.searchType.isEmpty(); 851 } 852 853 /** 854 * @param value {@link #searchType} (How the parameter is understood as a search 855 * parameter. This is only used if the parameter type is 856 * 'string'.). This is the underlying object with id, value and 857 * extensions. The accessor "getSearchType" gives direct access to 858 * the value 859 */ 860 public OperationDefinitionParameterComponent setSearchTypeElement(Enumeration<SearchParamType> value) { 861 this.searchType = value; 862 return this; 863 } 864 865 /** 866 * @return How the parameter is understood as a search parameter. This is only 867 * used if the parameter type is 'string'. 868 */ 869 public SearchParamType getSearchType() { 870 return this.searchType == null ? null : this.searchType.getValue(); 871 } 872 873 /** 874 * @param value How the parameter is understood as a search parameter. This is 875 * only used if the parameter type is 'string'. 876 */ 877 public OperationDefinitionParameterComponent setSearchType(SearchParamType value) { 878 if (value == null) 879 this.searchType = null; 880 else { 881 if (this.searchType == null) 882 this.searchType = new Enumeration<SearchParamType>(new SearchParamTypeEnumFactory()); 883 this.searchType.setValue(value); 884 } 885 return this; 886 } 887 888 /** 889 * @return {@link #binding} (Binds to a value set if this parameter is coded 890 * (code, Coding, CodeableConcept).) 891 */ 892 public OperationDefinitionParameterBindingComponent getBinding() { 893 if (this.binding == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create OperationDefinitionParameterComponent.binding"); 896 else if (Configuration.doAutoCreate()) 897 this.binding = new OperationDefinitionParameterBindingComponent(); // cc 898 return this.binding; 899 } 900 901 public boolean hasBinding() { 902 return this.binding != null && !this.binding.isEmpty(); 903 } 904 905 /** 906 * @param value {@link #binding} (Binds to a value set if this parameter is 907 * coded (code, Coding, CodeableConcept).) 908 */ 909 public OperationDefinitionParameterComponent setBinding(OperationDefinitionParameterBindingComponent value) { 910 this.binding = value; 911 return this; 912 } 913 914 /** 915 * @return {@link #referencedFrom} (Identifies other resource parameters within 916 * the operation invocation that are expected to resolve to this 917 * resource.) 918 */ 919 public List<OperationDefinitionParameterReferencedFromComponent> getReferencedFrom() { 920 if (this.referencedFrom == null) 921 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 922 return this.referencedFrom; 923 } 924 925 /** 926 * @return Returns a reference to <code>this</code> for easy method chaining 927 */ 928 public OperationDefinitionParameterComponent setReferencedFrom( 929 List<OperationDefinitionParameterReferencedFromComponent> theReferencedFrom) { 930 this.referencedFrom = theReferencedFrom; 931 return this; 932 } 933 934 public boolean hasReferencedFrom() { 935 if (this.referencedFrom == null) 936 return false; 937 for (OperationDefinitionParameterReferencedFromComponent item : this.referencedFrom) 938 if (!item.isEmpty()) 939 return true; 940 return false; 941 } 942 943 public OperationDefinitionParameterReferencedFromComponent addReferencedFrom() { // 3 944 OperationDefinitionParameterReferencedFromComponent t = new OperationDefinitionParameterReferencedFromComponent(); 945 if (this.referencedFrom == null) 946 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 947 this.referencedFrom.add(t); 948 return t; 949 } 950 951 public OperationDefinitionParameterComponent addReferencedFrom( 952 OperationDefinitionParameterReferencedFromComponent t) { // 3 953 if (t == null) 954 return this; 955 if (this.referencedFrom == null) 956 this.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 957 this.referencedFrom.add(t); 958 return this; 959 } 960 961 /** 962 * @return The first repetition of repeating field {@link #referencedFrom}, 963 * creating it if it does not already exist 964 */ 965 public OperationDefinitionParameterReferencedFromComponent getReferencedFromFirstRep() { 966 if (getReferencedFrom().isEmpty()) { 967 addReferencedFrom(); 968 } 969 return getReferencedFrom().get(0); 970 } 971 972 /** 973 * @return {@link #part} (The parts of a nested Parameter.) 974 */ 975 public List<OperationDefinitionParameterComponent> getPart() { 976 if (this.part == null) 977 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 978 return this.part; 979 } 980 981 /** 982 * @return Returns a reference to <code>this</code> for easy method chaining 983 */ 984 public OperationDefinitionParameterComponent setPart(List<OperationDefinitionParameterComponent> thePart) { 985 this.part = thePart; 986 return this; 987 } 988 989 public boolean hasPart() { 990 if (this.part == null) 991 return false; 992 for (OperationDefinitionParameterComponent item : this.part) 993 if (!item.isEmpty()) 994 return true; 995 return false; 996 } 997 998 public OperationDefinitionParameterComponent addPart() { // 3 999 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 1000 if (this.part == null) 1001 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1002 this.part.add(t); 1003 return t; 1004 } 1005 1006 public OperationDefinitionParameterComponent addPart(OperationDefinitionParameterComponent t) { // 3 1007 if (t == null) 1008 return this; 1009 if (this.part == null) 1010 this.part = new ArrayList<OperationDefinitionParameterComponent>(); 1011 this.part.add(t); 1012 return this; 1013 } 1014 1015 /** 1016 * @return The first repetition of repeating field {@link #part}, creating it if 1017 * it does not already exist 1018 */ 1019 public OperationDefinitionParameterComponent getPartFirstRep() { 1020 if (getPart().isEmpty()) { 1021 addPart(); 1022 } 1023 return getPart().get(0); 1024 } 1025 1026 protected void listChildren(List<Property> children) { 1027 super.listChildren(children); 1028 children.add(new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name)); 1029 children.add(new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use)); 1030 children.add(new Property("min", "integer", 1031 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 1032 children.add(new Property("max", "string", 1033 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 1034 children.add(new Property("documentation", "string", "Describes the meaning or use of this parameter.", 0, 1, 1035 documentation)); 1036 children.add(new Property("type", "code", "The type for this parameter.", 0, 1, type)); 1037 children.add(new Property("targetProfile", "canonical(StructureDefinition)", 1038 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 1039 0, java.lang.Integer.MAX_VALUE, targetProfile)); 1040 children.add(new Property("searchType", "code", 1041 "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.", 1042 0, 1, searchType)); 1043 children.add(new Property("binding", "", 1044 "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding)); 1045 children.add(new Property("referencedFrom", "", 1046 "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.", 1047 0, java.lang.Integer.MAX_VALUE, referencedFrom)); 1048 children.add(new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, 1049 java.lang.Integer.MAX_VALUE, part)); 1050 } 1051 1052 @Override 1053 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1054 switch (_hash) { 1055 case 3373707: 1056 /* name */ return new Property("name", "code", "The name of used to identify the parameter.", 0, 1, name); 1057 case 116103: 1058 /* use */ return new Property("use", "code", "Whether this is an input or an output parameter.", 0, 1, use); 1059 case 108114: 1060 /* min */ return new Property("min", "integer", 1061 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 1062 case 107876: 1063 /* max */ return new Property("max", "string", 1064 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 1065 case 1587405498: 1066 /* documentation */ return new Property("documentation", "string", 1067 "Describes the meaning or use of this parameter.", 0, 1, documentation); 1068 case 3575610: 1069 /* type */ return new Property("type", "code", "The type for this parameter.", 0, 1, type); 1070 case 1994521304: 1071 /* targetProfile */ return new Property("targetProfile", "canonical(StructureDefinition)", 1072 "Used when the type is \"Reference\" or \"canonical\", and identifies a profile structure or implementation Guide that applies to the target of the reference this parameter refers to. If any profiles are specified, then the content must conform to at least one of them. The URL can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the target resource SHALL conform to at least one profile defined in the implementation guide.", 1073 0, java.lang.Integer.MAX_VALUE, targetProfile); 1074 case -710454014: 1075 /* searchType */ return new Property("searchType", "code", 1076 "How the parameter is understood as a search parameter. This is only used if the parameter type is 'string'.", 1077 0, 1, searchType); 1078 case -108220795: 1079 /* binding */ return new Property("binding", "", 1080 "Binds to a value set if this parameter is coded (code, Coding, CodeableConcept).", 0, 1, binding); 1081 case -1896721981: 1082 /* referencedFrom */ return new Property("referencedFrom", "", 1083 "Identifies other resource parameters within the operation invocation that are expected to resolve to this resource.", 1084 0, java.lang.Integer.MAX_VALUE, referencedFrom); 1085 case 3433459: 1086 /* part */ return new Property("part", "@OperationDefinition.parameter", "The parts of a nested Parameter.", 0, 1087 java.lang.Integer.MAX_VALUE, part); 1088 default: 1089 return super.getNamedProperty(_hash, _name, _checkValid); 1090 } 1091 1092 } 1093 1094 @Override 1095 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1096 switch (hash) { 1097 case 3373707: 1098 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // CodeType 1099 case 116103: 1100 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<OperationParameterUse> 1101 case 108114: 1102 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // IntegerType 1103 case 107876: 1104 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 1105 case 1587405498: 1106 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 1107 case 3575610: 1108 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 1109 case 1994521304: 1110 /* targetProfile */ return this.targetProfile == null ? new Base[0] 1111 : this.targetProfile.toArray(new Base[this.targetProfile.size()]); // CanonicalType 1112 case -710454014: 1113 /* searchType */ return this.searchType == null ? new Base[0] : new Base[] { this.searchType }; // Enumeration<SearchParamType> 1114 case -108220795: 1115 /* binding */ return this.binding == null ? new Base[0] : new Base[] { this.binding }; // OperationDefinitionParameterBindingComponent 1116 case -1896721981: 1117 /* referencedFrom */ return this.referencedFrom == null ? new Base[0] 1118 : this.referencedFrom.toArray(new Base[this.referencedFrom.size()]); // OperationDefinitionParameterReferencedFromComponent 1119 case 3433459: 1120 /* part */ return this.part == null ? new Base[0] : this.part.toArray(new Base[this.part.size()]); // OperationDefinitionParameterComponent 1121 default: 1122 return super.getProperty(hash, name, checkValid); 1123 } 1124 1125 } 1126 1127 @Override 1128 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1129 switch (hash) { 1130 case 3373707: // name 1131 this.name = castToCode(value); // CodeType 1132 return value; 1133 case 116103: // use 1134 value = new OperationParameterUseEnumFactory().fromType(castToCode(value)); 1135 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1136 return value; 1137 case 108114: // min 1138 this.min = castToInteger(value); // IntegerType 1139 return value; 1140 case 107876: // max 1141 this.max = castToString(value); // StringType 1142 return value; 1143 case 1587405498: // documentation 1144 this.documentation = castToString(value); // StringType 1145 return value; 1146 case 3575610: // type 1147 this.type = castToCode(value); // CodeType 1148 return value; 1149 case 1994521304: // targetProfile 1150 this.getTargetProfile().add(castToCanonical(value)); // CanonicalType 1151 return value; 1152 case -710454014: // searchType 1153 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 1154 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1155 return value; 1156 case -108220795: // binding 1157 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1158 return value; 1159 case -1896721981: // referencedFrom 1160 this.getReferencedFrom().add((OperationDefinitionParameterReferencedFromComponent) value); // OperationDefinitionParameterReferencedFromComponent 1161 return value; 1162 case 3433459: // part 1163 this.getPart().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 1164 return value; 1165 default: 1166 return super.setProperty(hash, name, value); 1167 } 1168 1169 } 1170 1171 @Override 1172 public Base setProperty(String name, Base value) throws FHIRException { 1173 if (name.equals("name")) { 1174 this.name = castToCode(value); // CodeType 1175 } else if (name.equals("use")) { 1176 value = new OperationParameterUseEnumFactory().fromType(castToCode(value)); 1177 this.use = (Enumeration) value; // Enumeration<OperationParameterUse> 1178 } else if (name.equals("min")) { 1179 this.min = castToInteger(value); // IntegerType 1180 } else if (name.equals("max")) { 1181 this.max = castToString(value); // StringType 1182 } else if (name.equals("documentation")) { 1183 this.documentation = castToString(value); // StringType 1184 } else if (name.equals("type")) { 1185 this.type = castToCode(value); // CodeType 1186 } else if (name.equals("targetProfile")) { 1187 this.getTargetProfile().add(castToCanonical(value)); 1188 } else if (name.equals("searchType")) { 1189 value = new SearchParamTypeEnumFactory().fromType(castToCode(value)); 1190 this.searchType = (Enumeration) value; // Enumeration<SearchParamType> 1191 } else if (name.equals("binding")) { 1192 this.binding = (OperationDefinitionParameterBindingComponent) value; // OperationDefinitionParameterBindingComponent 1193 } else if (name.equals("referencedFrom")) { 1194 this.getReferencedFrom().add((OperationDefinitionParameterReferencedFromComponent) value); 1195 } else if (name.equals("part")) { 1196 this.getPart().add((OperationDefinitionParameterComponent) value); 1197 } else 1198 return super.setProperty(name, value); 1199 return value; 1200 } 1201 1202 @Override 1203 public Base makeProperty(int hash, String name) throws FHIRException { 1204 switch (hash) { 1205 case 3373707: 1206 return getNameElement(); 1207 case 116103: 1208 return getUseElement(); 1209 case 108114: 1210 return getMinElement(); 1211 case 107876: 1212 return getMaxElement(); 1213 case 1587405498: 1214 return getDocumentationElement(); 1215 case 3575610: 1216 return getTypeElement(); 1217 case 1994521304: 1218 return addTargetProfileElement(); 1219 case -710454014: 1220 return getSearchTypeElement(); 1221 case -108220795: 1222 return getBinding(); 1223 case -1896721981: 1224 return addReferencedFrom(); 1225 case 3433459: 1226 return addPart(); 1227 default: 1228 return super.makeProperty(hash, name); 1229 } 1230 1231 } 1232 1233 @Override 1234 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1235 switch (hash) { 1236 case 3373707: 1237 /* name */ return new String[] { "code" }; 1238 case 116103: 1239 /* use */ return new String[] { "code" }; 1240 case 108114: 1241 /* min */ return new String[] { "integer" }; 1242 case 107876: 1243 /* max */ return new String[] { "string" }; 1244 case 1587405498: 1245 /* documentation */ return new String[] { "string" }; 1246 case 3575610: 1247 /* type */ return new String[] { "code" }; 1248 case 1994521304: 1249 /* targetProfile */ return new String[] { "canonical" }; 1250 case -710454014: 1251 /* searchType */ return new String[] { "code" }; 1252 case -108220795: 1253 /* binding */ return new String[] {}; 1254 case -1896721981: 1255 /* referencedFrom */ return new String[] {}; 1256 case 3433459: 1257 /* part */ return new String[] { "@OperationDefinition.parameter" }; 1258 default: 1259 return super.getTypesForProperty(hash, name); 1260 } 1261 1262 } 1263 1264 @Override 1265 public Base addChild(String name) throws FHIRException { 1266 if (name.equals("name")) { 1267 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 1268 } else if (name.equals("use")) { 1269 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.use"); 1270 } else if (name.equals("min")) { 1271 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.min"); 1272 } else if (name.equals("max")) { 1273 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.max"); 1274 } else if (name.equals("documentation")) { 1275 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.documentation"); 1276 } else if (name.equals("type")) { 1277 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 1278 } else if (name.equals("targetProfile")) { 1279 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.targetProfile"); 1280 } else if (name.equals("searchType")) { 1281 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.searchType"); 1282 } else if (name.equals("binding")) { 1283 this.binding = new OperationDefinitionParameterBindingComponent(); 1284 return this.binding; 1285 } else if (name.equals("referencedFrom")) { 1286 return addReferencedFrom(); 1287 } else if (name.equals("part")) { 1288 return addPart(); 1289 } else 1290 return super.addChild(name); 1291 } 1292 1293 public OperationDefinitionParameterComponent copy() { 1294 OperationDefinitionParameterComponent dst = new OperationDefinitionParameterComponent(); 1295 copyValues(dst); 1296 return dst; 1297 } 1298 1299 public void copyValues(OperationDefinitionParameterComponent dst) { 1300 super.copyValues(dst); 1301 dst.name = name == null ? null : name.copy(); 1302 dst.use = use == null ? null : use.copy(); 1303 dst.min = min == null ? null : min.copy(); 1304 dst.max = max == null ? null : max.copy(); 1305 dst.documentation = documentation == null ? null : documentation.copy(); 1306 dst.type = type == null ? null : type.copy(); 1307 if (targetProfile != null) { 1308 dst.targetProfile = new ArrayList<CanonicalType>(); 1309 for (CanonicalType i : targetProfile) 1310 dst.targetProfile.add(i.copy()); 1311 } 1312 ; 1313 dst.searchType = searchType == null ? null : searchType.copy(); 1314 dst.binding = binding == null ? null : binding.copy(); 1315 if (referencedFrom != null) { 1316 dst.referencedFrom = new ArrayList<OperationDefinitionParameterReferencedFromComponent>(); 1317 for (OperationDefinitionParameterReferencedFromComponent i : referencedFrom) 1318 dst.referencedFrom.add(i.copy()); 1319 } 1320 ; 1321 if (part != null) { 1322 dst.part = new ArrayList<OperationDefinitionParameterComponent>(); 1323 for (OperationDefinitionParameterComponent i : part) 1324 dst.part.add(i.copy()); 1325 } 1326 ; 1327 } 1328 1329 @Override 1330 public boolean equalsDeep(Base other_) { 1331 if (!super.equalsDeep(other_)) 1332 return false; 1333 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1334 return false; 1335 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1336 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 1337 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) 1338 && compareDeep(type, o.type, true) && compareDeep(targetProfile, o.targetProfile, true) 1339 && compareDeep(searchType, o.searchType, true) && compareDeep(binding, o.binding, true) 1340 && compareDeep(referencedFrom, o.referencedFrom, true) && compareDeep(part, o.part, true); 1341 } 1342 1343 @Override 1344 public boolean equalsShallow(Base other_) { 1345 if (!super.equalsShallow(other_)) 1346 return false; 1347 if (!(other_ instanceof OperationDefinitionParameterComponent)) 1348 return false; 1349 OperationDefinitionParameterComponent o = (OperationDefinitionParameterComponent) other_; 1350 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 1351 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) 1352 && compareValues(type, o.type, true) && compareValues(searchType, o.searchType, true); 1353 } 1354 1355 public boolean isEmpty() { 1356 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, min, max, documentation, type, 1357 targetProfile, searchType, binding, referencedFrom, part); 1358 } 1359 1360 public String fhirType() { 1361 return "OperationDefinition.parameter"; 1362 1363 } 1364 1365 } 1366 1367 @Block() 1368 public static class OperationDefinitionParameterBindingComponent extends BackboneElement 1369 implements IBaseBackboneElement { 1370 /** 1371 * Indicates the degree of conformance expectations associated with this binding 1372 * - that is, the degree to which the provided value set must be adhered to in 1373 * the instances. 1374 */ 1375 @Child(name = "strength", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1376 @Description(shortDefinition = "required | extensible | preferred | example", formalDefinition = "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.") 1377 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/binding-strength") 1378 protected Enumeration<BindingStrength> strength; 1379 1380 /** 1381 * Points to the value set or external definition (e.g. implicit value set) that 1382 * identifies the set of codes to be used. 1383 */ 1384 @Child(name = "valueSet", type = { 1385 CanonicalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1386 @Description(shortDefinition = "Source of value set", formalDefinition = "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.") 1387 protected CanonicalType valueSet; 1388 1389 private static final long serialVersionUID = -2048653907L; 1390 1391 /** 1392 * Constructor 1393 */ 1394 public OperationDefinitionParameterBindingComponent() { 1395 super(); 1396 } 1397 1398 /** 1399 * Constructor 1400 */ 1401 public OperationDefinitionParameterBindingComponent(Enumeration<BindingStrength> strength, CanonicalType valueSet) { 1402 super(); 1403 this.strength = strength; 1404 this.valueSet = valueSet; 1405 } 1406 1407 /** 1408 * @return {@link #strength} (Indicates the degree of conformance expectations 1409 * associated with this binding - that is, the degree to which the 1410 * provided value set must be adhered to in the instances.). This is the 1411 * underlying object with id, value and extensions. The accessor 1412 * "getStrength" gives direct access to the value 1413 */ 1414 public Enumeration<BindingStrength> getStrengthElement() { 1415 if (this.strength == null) 1416 if (Configuration.errorOnAutoCreate()) 1417 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.strength"); 1418 else if (Configuration.doAutoCreate()) 1419 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 1420 return this.strength; 1421 } 1422 1423 public boolean hasStrengthElement() { 1424 return this.strength != null && !this.strength.isEmpty(); 1425 } 1426 1427 public boolean hasStrength() { 1428 return this.strength != null && !this.strength.isEmpty(); 1429 } 1430 1431 /** 1432 * @param value {@link #strength} (Indicates the degree of conformance 1433 * expectations associated with this binding - that is, the degree 1434 * to which the provided value set must be adhered to in the 1435 * instances.). This is the underlying object with id, value and 1436 * extensions. The accessor "getStrength" gives direct access to 1437 * the value 1438 */ 1439 public OperationDefinitionParameterBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 1440 this.strength = value; 1441 return this; 1442 } 1443 1444 /** 1445 * @return Indicates the degree of conformance expectations associated with this 1446 * binding - that is, the degree to which the provided value set must be 1447 * adhered to in the instances. 1448 */ 1449 public BindingStrength getStrength() { 1450 return this.strength == null ? null : this.strength.getValue(); 1451 } 1452 1453 /** 1454 * @param value Indicates the degree of conformance expectations associated with 1455 * this binding - that is, the degree to which the provided value 1456 * set must be adhered to in the instances. 1457 */ 1458 public OperationDefinitionParameterBindingComponent setStrength(BindingStrength value) { 1459 if (this.strength == null) 1460 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 1461 this.strength.setValue(value); 1462 return this; 1463 } 1464 1465 /** 1466 * @return {@link #valueSet} (Points to the value set or external definition 1467 * (e.g. implicit value set) that identifies the set of codes to be 1468 * used.). This is the underlying object with id, value and extensions. 1469 * The accessor "getValueSet" gives direct access to the value 1470 */ 1471 public CanonicalType getValueSetElement() { 1472 if (this.valueSet == null) 1473 if (Configuration.errorOnAutoCreate()) 1474 throw new Error("Attempt to auto-create OperationDefinitionParameterBindingComponent.valueSet"); 1475 else if (Configuration.doAutoCreate()) 1476 this.valueSet = new CanonicalType(); // bb 1477 return this.valueSet; 1478 } 1479 1480 public boolean hasValueSetElement() { 1481 return this.valueSet != null && !this.valueSet.isEmpty(); 1482 } 1483 1484 public boolean hasValueSet() { 1485 return this.valueSet != null && !this.valueSet.isEmpty(); 1486 } 1487 1488 /** 1489 * @param value {@link #valueSet} (Points to the value set or external 1490 * definition (e.g. implicit value set) that identifies the set of 1491 * codes to be used.). This is the underlying object with id, value 1492 * and extensions. The accessor "getValueSet" gives direct access 1493 * to the value 1494 */ 1495 public OperationDefinitionParameterBindingComponent setValueSetElement(CanonicalType value) { 1496 this.valueSet = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return Points to the value set or external definition (e.g. implicit value 1502 * set) that identifies the set of codes to be used. 1503 */ 1504 public String getValueSet() { 1505 return this.valueSet == null ? null : this.valueSet.getValue(); 1506 } 1507 1508 /** 1509 * @param value Points to the value set or external definition (e.g. implicit 1510 * value set) that identifies the set of codes to be used. 1511 */ 1512 public OperationDefinitionParameterBindingComponent setValueSet(String value) { 1513 if (this.valueSet == null) 1514 this.valueSet = new CanonicalType(); 1515 this.valueSet.setValue(value); 1516 return this; 1517 } 1518 1519 protected void listChildren(List<Property> children) { 1520 super.listChildren(children); 1521 children.add(new Property("strength", "code", 1522 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 1523 0, 1, strength)); 1524 children.add(new Property("valueSet", "canonical(ValueSet)", 1525 "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 1526 0, 1, valueSet)); 1527 } 1528 1529 @Override 1530 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1531 switch (_hash) { 1532 case 1791316033: 1533 /* strength */ return new Property("strength", "code", 1534 "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 1535 0, 1, strength); 1536 case -1410174671: 1537 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 1538 "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used.", 1539 0, 1, valueSet); 1540 default: 1541 return super.getNamedProperty(_hash, _name, _checkValid); 1542 } 1543 1544 } 1545 1546 @Override 1547 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1548 switch (hash) { 1549 case 1791316033: 1550 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Enumeration<BindingStrength> 1551 case -1410174671: 1552 /* valueSet */ return this.valueSet == null ? new Base[0] : new Base[] { this.valueSet }; // CanonicalType 1553 default: 1554 return super.getProperty(hash, name, checkValid); 1555 } 1556 1557 } 1558 1559 @Override 1560 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1561 switch (hash) { 1562 case 1791316033: // strength 1563 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 1564 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1565 return value; 1566 case -1410174671: // valueSet 1567 this.valueSet = castToCanonical(value); // CanonicalType 1568 return value; 1569 default: 1570 return super.setProperty(hash, name, value); 1571 } 1572 1573 } 1574 1575 @Override 1576 public Base setProperty(String name, Base value) throws FHIRException { 1577 if (name.equals("strength")) { 1578 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 1579 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 1580 } else if (name.equals("valueSet")) { 1581 this.valueSet = castToCanonical(value); // CanonicalType 1582 } else 1583 return super.setProperty(name, value); 1584 return value; 1585 } 1586 1587 @Override 1588 public Base makeProperty(int hash, String name) throws FHIRException { 1589 switch (hash) { 1590 case 1791316033: 1591 return getStrengthElement(); 1592 case -1410174671: 1593 return getValueSetElement(); 1594 default: 1595 return super.makeProperty(hash, name); 1596 } 1597 1598 } 1599 1600 @Override 1601 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1602 switch (hash) { 1603 case 1791316033: 1604 /* strength */ return new String[] { "code" }; 1605 case -1410174671: 1606 /* valueSet */ return new String[] { "canonical" }; 1607 default: 1608 return super.getTypesForProperty(hash, name); 1609 } 1610 1611 } 1612 1613 @Override 1614 public Base addChild(String name) throws FHIRException { 1615 if (name.equals("strength")) { 1616 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.strength"); 1617 } else if (name.equals("valueSet")) { 1618 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.valueSet"); 1619 } else 1620 return super.addChild(name); 1621 } 1622 1623 public OperationDefinitionParameterBindingComponent copy() { 1624 OperationDefinitionParameterBindingComponent dst = new OperationDefinitionParameterBindingComponent(); 1625 copyValues(dst); 1626 return dst; 1627 } 1628 1629 public void copyValues(OperationDefinitionParameterBindingComponent dst) { 1630 super.copyValues(dst); 1631 dst.strength = strength == null ? null : strength.copy(); 1632 dst.valueSet = valueSet == null ? null : valueSet.copy(); 1633 } 1634 1635 @Override 1636 public boolean equalsDeep(Base other_) { 1637 if (!super.equalsDeep(other_)) 1638 return false; 1639 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1640 return false; 1641 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1642 return compareDeep(strength, o.strength, true) && compareDeep(valueSet, o.valueSet, true); 1643 } 1644 1645 @Override 1646 public boolean equalsShallow(Base other_) { 1647 if (!super.equalsShallow(other_)) 1648 return false; 1649 if (!(other_ instanceof OperationDefinitionParameterBindingComponent)) 1650 return false; 1651 OperationDefinitionParameterBindingComponent o = (OperationDefinitionParameterBindingComponent) other_; 1652 return compareValues(strength, o.strength, true); 1653 } 1654 1655 public boolean isEmpty() { 1656 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, valueSet); 1657 } 1658 1659 public String fhirType() { 1660 return "OperationDefinition.parameter.binding"; 1661 1662 } 1663 1664 } 1665 1666 @Block() 1667 public static class OperationDefinitionParameterReferencedFromComponent extends BackboneElement 1668 implements IBaseBackboneElement { 1669 /** 1670 * The name of the parameter or dot-separated path of parameter names pointing 1671 * to the resource parameter that is expected to contain a reference to this 1672 * resource. 1673 */ 1674 @Child(name = "source", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1675 @Description(shortDefinition = "Referencing parameter", formalDefinition = "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.") 1676 protected StringType source; 1677 1678 /** 1679 * The id of the element in the referencing resource that is expected to resolve 1680 * to this resource. 1681 */ 1682 @Child(name = "sourceId", type = { 1683 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1684 @Description(shortDefinition = "Element id of reference", formalDefinition = "The id of the element in the referencing resource that is expected to resolve to this resource.") 1685 protected StringType sourceId; 1686 1687 private static final long serialVersionUID = -104239783L; 1688 1689 /** 1690 * Constructor 1691 */ 1692 public OperationDefinitionParameterReferencedFromComponent() { 1693 super(); 1694 } 1695 1696 /** 1697 * Constructor 1698 */ 1699 public OperationDefinitionParameterReferencedFromComponent(StringType source) { 1700 super(); 1701 this.source = source; 1702 } 1703 1704 /** 1705 * @return {@link #source} (The name of the parameter or dot-separated path of 1706 * parameter names pointing to the resource parameter that is expected 1707 * to contain a reference to this resource.). This is the underlying 1708 * object with id, value and extensions. The accessor "getSource" gives 1709 * direct access to the value 1710 */ 1711 public StringType getSourceElement() { 1712 if (this.source == null) 1713 if (Configuration.errorOnAutoCreate()) 1714 throw new Error("Attempt to auto-create OperationDefinitionParameterReferencedFromComponent.source"); 1715 else if (Configuration.doAutoCreate()) 1716 this.source = new StringType(); // bb 1717 return this.source; 1718 } 1719 1720 public boolean hasSourceElement() { 1721 return this.source != null && !this.source.isEmpty(); 1722 } 1723 1724 public boolean hasSource() { 1725 return this.source != null && !this.source.isEmpty(); 1726 } 1727 1728 /** 1729 * @param value {@link #source} (The name of the parameter or dot-separated path 1730 * of parameter names pointing to the resource parameter that is 1731 * expected to contain a reference to this resource.). This is the 1732 * underlying object with id, value and extensions. The accessor 1733 * "getSource" gives direct access to the value 1734 */ 1735 public OperationDefinitionParameterReferencedFromComponent setSourceElement(StringType value) { 1736 this.source = value; 1737 return this; 1738 } 1739 1740 /** 1741 * @return The name of the parameter or dot-separated path of parameter names 1742 * pointing to the resource parameter that is expected to contain a 1743 * reference to this resource. 1744 */ 1745 public String getSource() { 1746 return this.source == null ? null : this.source.getValue(); 1747 } 1748 1749 /** 1750 * @param value The name of the parameter or dot-separated path of parameter 1751 * names pointing to the resource parameter that is expected to 1752 * contain a reference to this resource. 1753 */ 1754 public OperationDefinitionParameterReferencedFromComponent setSource(String value) { 1755 if (this.source == null) 1756 this.source = new StringType(); 1757 this.source.setValue(value); 1758 return this; 1759 } 1760 1761 /** 1762 * @return {@link #sourceId} (The id of the element in the referencing resource 1763 * that is expected to resolve to this resource.). This is the 1764 * underlying object with id, value and extensions. The accessor 1765 * "getSourceId" gives direct access to the value 1766 */ 1767 public StringType getSourceIdElement() { 1768 if (this.sourceId == null) 1769 if (Configuration.errorOnAutoCreate()) 1770 throw new Error("Attempt to auto-create OperationDefinitionParameterReferencedFromComponent.sourceId"); 1771 else if (Configuration.doAutoCreate()) 1772 this.sourceId = new StringType(); // bb 1773 return this.sourceId; 1774 } 1775 1776 public boolean hasSourceIdElement() { 1777 return this.sourceId != null && !this.sourceId.isEmpty(); 1778 } 1779 1780 public boolean hasSourceId() { 1781 return this.sourceId != null && !this.sourceId.isEmpty(); 1782 } 1783 1784 /** 1785 * @param value {@link #sourceId} (The id of the element in the referencing 1786 * resource that is expected to resolve to this resource.). This is 1787 * the underlying object with id, value and extensions. The 1788 * accessor "getSourceId" gives direct access to the value 1789 */ 1790 public OperationDefinitionParameterReferencedFromComponent setSourceIdElement(StringType value) { 1791 this.sourceId = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return The id of the element in the referencing resource that is expected to 1797 * resolve to this resource. 1798 */ 1799 public String getSourceId() { 1800 return this.sourceId == null ? null : this.sourceId.getValue(); 1801 } 1802 1803 /** 1804 * @param value The id of the element in the referencing resource that is 1805 * expected to resolve to this resource. 1806 */ 1807 public OperationDefinitionParameterReferencedFromComponent setSourceId(String value) { 1808 if (Utilities.noString(value)) 1809 this.sourceId = null; 1810 else { 1811 if (this.sourceId == null) 1812 this.sourceId = new StringType(); 1813 this.sourceId.setValue(value); 1814 } 1815 return this; 1816 } 1817 1818 protected void listChildren(List<Property> children) { 1819 super.listChildren(children); 1820 children.add(new Property("source", "string", 1821 "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.", 1822 0, 1, source)); 1823 children.add(new Property("sourceId", "string", 1824 "The id of the element in the referencing resource that is expected to resolve to this resource.", 0, 1, 1825 sourceId)); 1826 } 1827 1828 @Override 1829 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1830 switch (_hash) { 1831 case -896505829: 1832 /* source */ return new Property("source", "string", 1833 "The name of the parameter or dot-separated path of parameter names pointing to the resource parameter that is expected to contain a reference to this resource.", 1834 0, 1, source); 1835 case 1746327190: 1836 /* sourceId */ return new Property("sourceId", "string", 1837 "The id of the element in the referencing resource that is expected to resolve to this resource.", 0, 1, 1838 sourceId); 1839 default: 1840 return super.getNamedProperty(_hash, _name, _checkValid); 1841 } 1842 1843 } 1844 1845 @Override 1846 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1847 switch (hash) { 1848 case -896505829: 1849 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // StringType 1850 case 1746327190: 1851 /* sourceId */ return this.sourceId == null ? new Base[0] : new Base[] { this.sourceId }; // StringType 1852 default: 1853 return super.getProperty(hash, name, checkValid); 1854 } 1855 1856 } 1857 1858 @Override 1859 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1860 switch (hash) { 1861 case -896505829: // source 1862 this.source = castToString(value); // StringType 1863 return value; 1864 case 1746327190: // sourceId 1865 this.sourceId = castToString(value); // StringType 1866 return value; 1867 default: 1868 return super.setProperty(hash, name, value); 1869 } 1870 1871 } 1872 1873 @Override 1874 public Base setProperty(String name, Base value) throws FHIRException { 1875 if (name.equals("source")) { 1876 this.source = castToString(value); // StringType 1877 } else if (name.equals("sourceId")) { 1878 this.sourceId = castToString(value); // StringType 1879 } else 1880 return super.setProperty(name, value); 1881 return value; 1882 } 1883 1884 @Override 1885 public Base makeProperty(int hash, String name) throws FHIRException { 1886 switch (hash) { 1887 case -896505829: 1888 return getSourceElement(); 1889 case 1746327190: 1890 return getSourceIdElement(); 1891 default: 1892 return super.makeProperty(hash, name); 1893 } 1894 1895 } 1896 1897 @Override 1898 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1899 switch (hash) { 1900 case -896505829: 1901 /* source */ return new String[] { "string" }; 1902 case 1746327190: 1903 /* sourceId */ return new String[] { "string" }; 1904 default: 1905 return super.getTypesForProperty(hash, name); 1906 } 1907 1908 } 1909 1910 @Override 1911 public Base addChild(String name) throws FHIRException { 1912 if (name.equals("source")) { 1913 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.source"); 1914 } else if (name.equals("sourceId")) { 1915 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.sourceId"); 1916 } else 1917 return super.addChild(name); 1918 } 1919 1920 public OperationDefinitionParameterReferencedFromComponent copy() { 1921 OperationDefinitionParameterReferencedFromComponent dst = new OperationDefinitionParameterReferencedFromComponent(); 1922 copyValues(dst); 1923 return dst; 1924 } 1925 1926 public void copyValues(OperationDefinitionParameterReferencedFromComponent dst) { 1927 super.copyValues(dst); 1928 dst.source = source == null ? null : source.copy(); 1929 dst.sourceId = sourceId == null ? null : sourceId.copy(); 1930 } 1931 1932 @Override 1933 public boolean equalsDeep(Base other_) { 1934 if (!super.equalsDeep(other_)) 1935 return false; 1936 if (!(other_ instanceof OperationDefinitionParameterReferencedFromComponent)) 1937 return false; 1938 OperationDefinitionParameterReferencedFromComponent o = (OperationDefinitionParameterReferencedFromComponent) other_; 1939 return compareDeep(source, o.source, true) && compareDeep(sourceId, o.sourceId, true); 1940 } 1941 1942 @Override 1943 public boolean equalsShallow(Base other_) { 1944 if (!super.equalsShallow(other_)) 1945 return false; 1946 if (!(other_ instanceof OperationDefinitionParameterReferencedFromComponent)) 1947 return false; 1948 OperationDefinitionParameterReferencedFromComponent o = (OperationDefinitionParameterReferencedFromComponent) other_; 1949 return compareValues(source, o.source, true) && compareValues(sourceId, o.sourceId, true); 1950 } 1951 1952 public boolean isEmpty() { 1953 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, sourceId); 1954 } 1955 1956 public String fhirType() { 1957 return "OperationDefinition.parameter.referencedFrom"; 1958 1959 } 1960 1961 } 1962 1963 @Block() 1964 public static class OperationDefinitionOverloadComponent extends BackboneElement implements IBaseBackboneElement { 1965 /** 1966 * Name of parameter to include in overload. 1967 */ 1968 @Child(name = "parameterName", type = { 1969 StringType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1970 @Description(shortDefinition = "Name of parameter to include in overload", formalDefinition = "Name of parameter to include in overload.") 1971 protected List<StringType> parameterName; 1972 1973 /** 1974 * Comments to go on overload. 1975 */ 1976 @Child(name = "comment", type = { 1977 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1978 @Description(shortDefinition = "Comments to go on overload", formalDefinition = "Comments to go on overload.") 1979 protected StringType comment; 1980 1981 private static final long serialVersionUID = -907948545L; 1982 1983 /** 1984 * Constructor 1985 */ 1986 public OperationDefinitionOverloadComponent() { 1987 super(); 1988 } 1989 1990 /** 1991 * @return {@link #parameterName} (Name of parameter to include in overload.) 1992 */ 1993 public List<StringType> getParameterName() { 1994 if (this.parameterName == null) 1995 this.parameterName = new ArrayList<StringType>(); 1996 return this.parameterName; 1997 } 1998 1999 /** 2000 * @return Returns a reference to <code>this</code> for easy method chaining 2001 */ 2002 public OperationDefinitionOverloadComponent setParameterName(List<StringType> theParameterName) { 2003 this.parameterName = theParameterName; 2004 return this; 2005 } 2006 2007 public boolean hasParameterName() { 2008 if (this.parameterName == null) 2009 return false; 2010 for (StringType item : this.parameterName) 2011 if (!item.isEmpty()) 2012 return true; 2013 return false; 2014 } 2015 2016 /** 2017 * @return {@link #parameterName} (Name of parameter to include in overload.) 2018 */ 2019 public StringType addParameterNameElement() {// 2 2020 StringType t = new StringType(); 2021 if (this.parameterName == null) 2022 this.parameterName = new ArrayList<StringType>(); 2023 this.parameterName.add(t); 2024 return t; 2025 } 2026 2027 /** 2028 * @param value {@link #parameterName} (Name of parameter to include in 2029 * overload.) 2030 */ 2031 public OperationDefinitionOverloadComponent addParameterName(String value) { // 1 2032 StringType t = new StringType(); 2033 t.setValue(value); 2034 if (this.parameterName == null) 2035 this.parameterName = new ArrayList<StringType>(); 2036 this.parameterName.add(t); 2037 return this; 2038 } 2039 2040 /** 2041 * @param value {@link #parameterName} (Name of parameter to include in 2042 * overload.) 2043 */ 2044 public boolean hasParameterName(String value) { 2045 if (this.parameterName == null) 2046 return false; 2047 for (StringType v : this.parameterName) 2048 if (v.getValue().equals(value)) // string 2049 return true; 2050 return false; 2051 } 2052 2053 /** 2054 * @return {@link #comment} (Comments to go on overload.). This is the 2055 * underlying object with id, value and extensions. The accessor 2056 * "getComment" gives direct access to the value 2057 */ 2058 public StringType getCommentElement() { 2059 if (this.comment == null) 2060 if (Configuration.errorOnAutoCreate()) 2061 throw new Error("Attempt to auto-create OperationDefinitionOverloadComponent.comment"); 2062 else if (Configuration.doAutoCreate()) 2063 this.comment = new StringType(); // bb 2064 return this.comment; 2065 } 2066 2067 public boolean hasCommentElement() { 2068 return this.comment != null && !this.comment.isEmpty(); 2069 } 2070 2071 public boolean hasComment() { 2072 return this.comment != null && !this.comment.isEmpty(); 2073 } 2074 2075 /** 2076 * @param value {@link #comment} (Comments to go on overload.). This is the 2077 * underlying object with id, value and extensions. The accessor 2078 * "getComment" gives direct access to the value 2079 */ 2080 public OperationDefinitionOverloadComponent setCommentElement(StringType value) { 2081 this.comment = value; 2082 return this; 2083 } 2084 2085 /** 2086 * @return Comments to go on overload. 2087 */ 2088 public String getComment() { 2089 return this.comment == null ? null : this.comment.getValue(); 2090 } 2091 2092 /** 2093 * @param value Comments to go on overload. 2094 */ 2095 public OperationDefinitionOverloadComponent setComment(String value) { 2096 if (Utilities.noString(value)) 2097 this.comment = null; 2098 else { 2099 if (this.comment == null) 2100 this.comment = new StringType(); 2101 this.comment.setValue(value); 2102 } 2103 return this; 2104 } 2105 2106 protected void listChildren(List<Property> children) { 2107 super.listChildren(children); 2108 children.add(new Property("parameterName", "string", "Name of parameter to include in overload.", 0, 2109 java.lang.Integer.MAX_VALUE, parameterName)); 2110 children.add(new Property("comment", "string", "Comments to go on overload.", 0, 1, comment)); 2111 } 2112 2113 @Override 2114 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2115 switch (_hash) { 2116 case -379607596: 2117 /* parameterName */ return new Property("parameterName", "string", "Name of parameter to include in overload.", 2118 0, java.lang.Integer.MAX_VALUE, parameterName); 2119 case 950398559: 2120 /* comment */ return new Property("comment", "string", "Comments to go on overload.", 0, 1, comment); 2121 default: 2122 return super.getNamedProperty(_hash, _name, _checkValid); 2123 } 2124 2125 } 2126 2127 @Override 2128 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2129 switch (hash) { 2130 case -379607596: 2131 /* parameterName */ return this.parameterName == null ? new Base[0] 2132 : this.parameterName.toArray(new Base[this.parameterName.size()]); // StringType 2133 case 950398559: 2134 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2135 default: 2136 return super.getProperty(hash, name, checkValid); 2137 } 2138 2139 } 2140 2141 @Override 2142 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2143 switch (hash) { 2144 case -379607596: // parameterName 2145 this.getParameterName().add(castToString(value)); // StringType 2146 return value; 2147 case 950398559: // comment 2148 this.comment = castToString(value); // StringType 2149 return value; 2150 default: 2151 return super.setProperty(hash, name, value); 2152 } 2153 2154 } 2155 2156 @Override 2157 public Base setProperty(String name, Base value) throws FHIRException { 2158 if (name.equals("parameterName")) { 2159 this.getParameterName().add(castToString(value)); 2160 } else if (name.equals("comment")) { 2161 this.comment = castToString(value); // StringType 2162 } else 2163 return super.setProperty(name, value); 2164 return value; 2165 } 2166 2167 @Override 2168 public Base makeProperty(int hash, String name) throws FHIRException { 2169 switch (hash) { 2170 case -379607596: 2171 return addParameterNameElement(); 2172 case 950398559: 2173 return getCommentElement(); 2174 default: 2175 return super.makeProperty(hash, name); 2176 } 2177 2178 } 2179 2180 @Override 2181 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2182 switch (hash) { 2183 case -379607596: 2184 /* parameterName */ return new String[] { "string" }; 2185 case 950398559: 2186 /* comment */ return new String[] { "string" }; 2187 default: 2188 return super.getTypesForProperty(hash, name); 2189 } 2190 2191 } 2192 2193 @Override 2194 public Base addChild(String name) throws FHIRException { 2195 if (name.equals("parameterName")) { 2196 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.parameterName"); 2197 } else if (name.equals("comment")) { 2198 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 2199 } else 2200 return super.addChild(name); 2201 } 2202 2203 public OperationDefinitionOverloadComponent copy() { 2204 OperationDefinitionOverloadComponent dst = new OperationDefinitionOverloadComponent(); 2205 copyValues(dst); 2206 return dst; 2207 } 2208 2209 public void copyValues(OperationDefinitionOverloadComponent dst) { 2210 super.copyValues(dst); 2211 if (parameterName != null) { 2212 dst.parameterName = new ArrayList<StringType>(); 2213 for (StringType i : parameterName) 2214 dst.parameterName.add(i.copy()); 2215 } 2216 ; 2217 dst.comment = comment == null ? null : comment.copy(); 2218 } 2219 2220 @Override 2221 public boolean equalsDeep(Base other_) { 2222 if (!super.equalsDeep(other_)) 2223 return false; 2224 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 2225 return false; 2226 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 2227 return compareDeep(parameterName, o.parameterName, true) && compareDeep(comment, o.comment, true); 2228 } 2229 2230 @Override 2231 public boolean equalsShallow(Base other_) { 2232 if (!super.equalsShallow(other_)) 2233 return false; 2234 if (!(other_ instanceof OperationDefinitionOverloadComponent)) 2235 return false; 2236 OperationDefinitionOverloadComponent o = (OperationDefinitionOverloadComponent) other_; 2237 return compareValues(parameterName, o.parameterName, true) && compareValues(comment, o.comment, true); 2238 } 2239 2240 public boolean isEmpty() { 2241 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(parameterName, comment); 2242 } 2243 2244 public String fhirType() { 2245 return "OperationDefinition.overload"; 2246 2247 } 2248 2249 } 2250 2251 /** 2252 * Whether this is an operation or a named query. 2253 */ 2254 @Child(name = "kind", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2255 @Description(shortDefinition = "operation | query", formalDefinition = "Whether this is an operation or a named query.") 2256 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/operation-kind") 2257 protected Enumeration<OperationKind> kind; 2258 2259 /** 2260 * Explanation of why this operation definition is needed and why it has been 2261 * designed as it has. 2262 */ 2263 @Child(name = "purpose", type = { 2264 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2265 @Description(shortDefinition = "Why this operation definition is defined", formalDefinition = "Explanation of why this operation definition is needed and why it has been designed as it has.") 2266 protected MarkdownType purpose; 2267 2268 /** 2269 * Whether the operation affects state. Side effects such as producing audit 2270 * trail entries do not count as 'affecting state'. 2271 */ 2272 @Child(name = "affectsState", type = { 2273 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2274 @Description(shortDefinition = "Whether content is changed by the operation", formalDefinition = "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.") 2275 protected BooleanType affectsState; 2276 2277 /** 2278 * The name used to invoke the operation. 2279 */ 2280 @Child(name = "code", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2281 @Description(shortDefinition = "Name used to invoke the operation", formalDefinition = "The name used to invoke the operation.") 2282 protected CodeType code; 2283 2284 /** 2285 * Additional information about how to use this operation or named query. 2286 */ 2287 @Child(name = "comment", type = { 2288 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2289 @Description(shortDefinition = "Additional information about use", formalDefinition = "Additional information about how to use this operation or named query.") 2290 protected MarkdownType comment; 2291 2292 /** 2293 * Indicates that this operation definition is a constraining profile on the 2294 * base. 2295 */ 2296 @Child(name = "base", type = { CanonicalType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2297 @Description(shortDefinition = "Marks this as a profile of the base", formalDefinition = "Indicates that this operation definition is a constraining profile on the base.") 2298 protected CanonicalType base; 2299 2300 /** 2301 * The types on which this operation can be executed. 2302 */ 2303 @Child(name = "resource", type = { 2304 CodeType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2305 @Description(shortDefinition = "Types this operation applies to", formalDefinition = "The types on which this operation can be executed.") 2306 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 2307 protected List<CodeType> resource; 2308 2309 /** 2310 * Indicates whether this operation or named query can be invoked at the system 2311 * level (e.g. without needing to choose a resource type for the context). 2312 */ 2313 @Child(name = "system", type = { BooleanType.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 2314 @Description(shortDefinition = "Invoke at the system level?", formalDefinition = "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).") 2315 protected BooleanType system; 2316 2317 /** 2318 * Indicates whether this operation or named query can be invoked at the 2319 * resource type level for any given resource type level (e.g. without needing 2320 * to choose a specific resource id for the context). 2321 */ 2322 @Child(name = "type", type = { BooleanType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 2323 @Description(shortDefinition = "Invoke at the type level?", formalDefinition = "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).") 2324 protected BooleanType type; 2325 2326 /** 2327 * Indicates whether this operation can be invoked on a particular instance of 2328 * one of the given types. 2329 */ 2330 @Child(name = "instance", type = { BooleanType.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 2331 @Description(shortDefinition = "Invoke on an instance?", formalDefinition = "Indicates whether this operation can be invoked on a particular instance of one of the given types.") 2332 protected BooleanType instance; 2333 2334 /** 2335 * Additional validation information for the in parameters - a single profile 2336 * that covers all the parameters. The profile is a constraint on the parameters 2337 * resource as a whole. 2338 */ 2339 @Child(name = "inputProfile", type = { 2340 CanonicalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2341 @Description(shortDefinition = "Validation information for in parameters", formalDefinition = "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.") 2342 protected CanonicalType inputProfile; 2343 2344 /** 2345 * Additional validation information for the out parameters - a single profile 2346 * that covers all the parameters. The profile is a constraint on the parameters 2347 * resource. 2348 */ 2349 @Child(name = "outputProfile", type = { 2350 CanonicalType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 2351 @Description(shortDefinition = "Validation information for out parameters", formalDefinition = "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.") 2352 protected CanonicalType outputProfile; 2353 2354 /** 2355 * The parameters for the operation/query. 2356 */ 2357 @Child(name = "parameter", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2358 @Description(shortDefinition = "Parameters for the operation/query", formalDefinition = "The parameters for the operation/query.") 2359 protected List<OperationDefinitionParameterComponent> parameter; 2360 2361 /** 2362 * Defines an appropriate combination of parameters to use when invoking this 2363 * operation, to help code generators when generating overloaded parameter sets 2364 * for this operation. 2365 */ 2366 @Child(name = "overload", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2367 @Description(shortDefinition = "Define overloaded variants for when generating code", formalDefinition = "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.") 2368 protected List<OperationDefinitionOverloadComponent> overload; 2369 2370 private static final long serialVersionUID = 149113671L; 2371 2372 /** 2373 * Constructor 2374 */ 2375 public OperationDefinition() { 2376 super(); 2377 } 2378 2379 /** 2380 * Constructor 2381 */ 2382 public OperationDefinition(StringType name, Enumeration<PublicationStatus> status, Enumeration<OperationKind> kind, 2383 CodeType code, BooleanType system, BooleanType type, BooleanType instance) { 2384 super(); 2385 this.name = name; 2386 this.status = status; 2387 this.kind = kind; 2388 this.code = code; 2389 this.system = system; 2390 this.type = type; 2391 this.instance = instance; 2392 } 2393 2394 /** 2395 * @return {@link #url} (An absolute URI that is used to identify this operation 2396 * definition when it is referenced in a specification, model, design or 2397 * an instance; also called its canonical identifier. This SHOULD be 2398 * globally unique and SHOULD be a literal address at which at which an 2399 * authoritative instance of this operation definition is (or will be) 2400 * published. This URL can be the target of a canonical reference. It 2401 * SHALL remain the same when the operation definition is stored on 2402 * different servers.). This is the underlying object with id, value and 2403 * extensions. The accessor "getUrl" gives direct access to the value 2404 */ 2405 public UriType getUrlElement() { 2406 if (this.url == null) 2407 if (Configuration.errorOnAutoCreate()) 2408 throw new Error("Attempt to auto-create OperationDefinition.url"); 2409 else if (Configuration.doAutoCreate()) 2410 this.url = new UriType(); // bb 2411 return this.url; 2412 } 2413 2414 public boolean hasUrlElement() { 2415 return this.url != null && !this.url.isEmpty(); 2416 } 2417 2418 public boolean hasUrl() { 2419 return this.url != null && !this.url.isEmpty(); 2420 } 2421 2422 /** 2423 * @param value {@link #url} (An absolute URI that is used to identify this 2424 * operation definition when it is referenced in a specification, 2425 * model, design or an instance; also called its canonical 2426 * identifier. This SHOULD be globally unique and SHOULD be a 2427 * literal address at which at which an authoritative instance of 2428 * this operation definition is (or will be) published. This URL 2429 * can be the target of a canonical reference. It SHALL remain the 2430 * same when the operation definition is stored on different 2431 * servers.). This is the underlying object with id, value and 2432 * extensions. The accessor "getUrl" gives direct access to the 2433 * value 2434 */ 2435 public OperationDefinition setUrlElement(UriType value) { 2436 this.url = value; 2437 return this; 2438 } 2439 2440 /** 2441 * @return An absolute URI that is used to identify this operation definition 2442 * when it is referenced in a specification, model, design or an 2443 * instance; also called its canonical identifier. This SHOULD be 2444 * globally unique and SHOULD be a literal address at which at which an 2445 * authoritative instance of this operation definition is (or will be) 2446 * published. This URL can be the target of a canonical reference. It 2447 * SHALL remain the same when the operation definition is stored on 2448 * different servers. 2449 */ 2450 public String getUrl() { 2451 return this.url == null ? null : this.url.getValue(); 2452 } 2453 2454 /** 2455 * @param value An absolute URI that is used to identify this operation 2456 * definition when it is referenced in a specification, model, 2457 * design or an instance; also called its canonical identifier. 2458 * This SHOULD be globally unique and SHOULD be a literal address 2459 * at which at which an authoritative instance of this operation 2460 * definition is (or will be) published. This URL can be the target 2461 * of a canonical reference. It SHALL remain the same when the 2462 * operation definition is stored on different servers. 2463 */ 2464 public OperationDefinition setUrl(String value) { 2465 if (Utilities.noString(value)) 2466 this.url = null; 2467 else { 2468 if (this.url == null) 2469 this.url = new UriType(); 2470 this.url.setValue(value); 2471 } 2472 return this; 2473 } 2474 2475 /** 2476 * @return {@link #version} (The identifier that is used to identify this 2477 * version of the operation definition when it is referenced in a 2478 * specification, model, design or instance. This is an arbitrary value 2479 * managed by the operation definition author and is not expected to be 2480 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 2481 * if a managed version is not available. There is also no expectation 2482 * that versions can be placed in a lexicographical sequence.). This is 2483 * the underlying object with id, value and extensions. The accessor 2484 * "getVersion" gives direct access to the value 2485 */ 2486 public StringType getVersionElement() { 2487 if (this.version == null) 2488 if (Configuration.errorOnAutoCreate()) 2489 throw new Error("Attempt to auto-create OperationDefinition.version"); 2490 else if (Configuration.doAutoCreate()) 2491 this.version = new StringType(); // bb 2492 return this.version; 2493 } 2494 2495 public boolean hasVersionElement() { 2496 return this.version != null && !this.version.isEmpty(); 2497 } 2498 2499 public boolean hasVersion() { 2500 return this.version != null && !this.version.isEmpty(); 2501 } 2502 2503 /** 2504 * @param value {@link #version} (The identifier that is used to identify this 2505 * version of the operation definition when it is referenced in a 2506 * specification, model, design or instance. This is an arbitrary 2507 * value managed by the operation definition author and is not 2508 * expected to be globally unique. For example, it might be a 2509 * timestamp (e.g. yyyymmdd) if a managed version is not available. 2510 * There is also no expectation that versions can be placed in a 2511 * lexicographical sequence.). This is the underlying object with 2512 * id, value and extensions. The accessor "getVersion" gives direct 2513 * access to the value 2514 */ 2515 public OperationDefinition setVersionElement(StringType value) { 2516 this.version = value; 2517 return this; 2518 } 2519 2520 /** 2521 * @return The identifier that is used to identify this version of the operation 2522 * definition when it is referenced in a specification, model, design or 2523 * instance. This is an arbitrary value managed by the operation 2524 * definition author and is not expected to be globally unique. For 2525 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 2526 * is not available. There is also no expectation that versions can be 2527 * placed in a lexicographical sequence. 2528 */ 2529 public String getVersion() { 2530 return this.version == null ? null : this.version.getValue(); 2531 } 2532 2533 /** 2534 * @param value The identifier that is used to identify this version of the 2535 * operation definition when it is referenced in a specification, 2536 * model, design or instance. This is an arbitrary value managed by 2537 * the operation definition author and is not expected to be 2538 * globally unique. For example, it might be a timestamp (e.g. 2539 * yyyymmdd) if a managed version is not available. There is also 2540 * no expectation that versions can be placed in a lexicographical 2541 * sequence. 2542 */ 2543 public OperationDefinition setVersion(String value) { 2544 if (Utilities.noString(value)) 2545 this.version = null; 2546 else { 2547 if (this.version == null) 2548 this.version = new StringType(); 2549 this.version.setValue(value); 2550 } 2551 return this; 2552 } 2553 2554 /** 2555 * @return {@link #name} (A natural language name identifying the operation 2556 * definition. This name should be usable as an identifier for the 2557 * module by machine processing applications such as code generation.). 2558 * This is the underlying object with id, value and extensions. The 2559 * accessor "getName" gives direct access to the value 2560 */ 2561 public StringType getNameElement() { 2562 if (this.name == null) 2563 if (Configuration.errorOnAutoCreate()) 2564 throw new Error("Attempt to auto-create OperationDefinition.name"); 2565 else if (Configuration.doAutoCreate()) 2566 this.name = new StringType(); // bb 2567 return this.name; 2568 } 2569 2570 public boolean hasNameElement() { 2571 return this.name != null && !this.name.isEmpty(); 2572 } 2573 2574 public boolean hasName() { 2575 return this.name != null && !this.name.isEmpty(); 2576 } 2577 2578 /** 2579 * @param value {@link #name} (A natural language name identifying the operation 2580 * definition. This name should be usable as an identifier for the 2581 * module by machine processing applications such as code 2582 * generation.). This is the underlying object with id, value and 2583 * extensions. The accessor "getName" gives direct access to the 2584 * value 2585 */ 2586 public OperationDefinition setNameElement(StringType value) { 2587 this.name = value; 2588 return this; 2589 } 2590 2591 /** 2592 * @return A natural language name identifying the operation definition. This 2593 * name should be usable as an identifier for the module by machine 2594 * processing applications such as code generation. 2595 */ 2596 public String getName() { 2597 return this.name == null ? null : this.name.getValue(); 2598 } 2599 2600 /** 2601 * @param value A natural language name identifying the operation definition. 2602 * This name should be usable as an identifier for the module by 2603 * machine processing applications such as code generation. 2604 */ 2605 public OperationDefinition setName(String value) { 2606 if (this.name == null) 2607 this.name = new StringType(); 2608 this.name.setValue(value); 2609 return this; 2610 } 2611 2612 /** 2613 * @return {@link #title} (A short, descriptive, user-friendly title for the 2614 * operation definition.). This is the underlying object with id, value 2615 * and extensions. The accessor "getTitle" gives direct access to the 2616 * value 2617 */ 2618 public StringType getTitleElement() { 2619 if (this.title == null) 2620 if (Configuration.errorOnAutoCreate()) 2621 throw new Error("Attempt to auto-create OperationDefinition.title"); 2622 else if (Configuration.doAutoCreate()) 2623 this.title = new StringType(); // bb 2624 return this.title; 2625 } 2626 2627 public boolean hasTitleElement() { 2628 return this.title != null && !this.title.isEmpty(); 2629 } 2630 2631 public boolean hasTitle() { 2632 return this.title != null && !this.title.isEmpty(); 2633 } 2634 2635 /** 2636 * @param value {@link #title} (A short, descriptive, user-friendly title for 2637 * the operation definition.). This is the underlying object with 2638 * id, value and extensions. The accessor "getTitle" gives direct 2639 * access to the value 2640 */ 2641 public OperationDefinition setTitleElement(StringType value) { 2642 this.title = value; 2643 return this; 2644 } 2645 2646 /** 2647 * @return A short, descriptive, user-friendly title for the operation 2648 * definition. 2649 */ 2650 public String getTitle() { 2651 return this.title == null ? null : this.title.getValue(); 2652 } 2653 2654 /** 2655 * @param value A short, descriptive, user-friendly title for the operation 2656 * definition. 2657 */ 2658 public OperationDefinition setTitle(String value) { 2659 if (Utilities.noString(value)) 2660 this.title = null; 2661 else { 2662 if (this.title == null) 2663 this.title = new StringType(); 2664 this.title.setValue(value); 2665 } 2666 return this; 2667 } 2668 2669 /** 2670 * @return {@link #status} (The status of this operation definition. Enables 2671 * tracking the life-cycle of the content.). This is the underlying 2672 * object with id, value and extensions. The accessor "getStatus" gives 2673 * direct access to the value 2674 */ 2675 public Enumeration<PublicationStatus> getStatusElement() { 2676 if (this.status == null) 2677 if (Configuration.errorOnAutoCreate()) 2678 throw new Error("Attempt to auto-create OperationDefinition.status"); 2679 else if (Configuration.doAutoCreate()) 2680 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2681 return this.status; 2682 } 2683 2684 public boolean hasStatusElement() { 2685 return this.status != null && !this.status.isEmpty(); 2686 } 2687 2688 public boolean hasStatus() { 2689 return this.status != null && !this.status.isEmpty(); 2690 } 2691 2692 /** 2693 * @param value {@link #status} (The status of this operation definition. 2694 * Enables tracking the life-cycle of the content.). This is the 2695 * underlying object with id, value and extensions. The accessor 2696 * "getStatus" gives direct access to the value 2697 */ 2698 public OperationDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2699 this.status = value; 2700 return this; 2701 } 2702 2703 /** 2704 * @return The status of this operation definition. Enables tracking the 2705 * life-cycle of the content. 2706 */ 2707 public PublicationStatus getStatus() { 2708 return this.status == null ? null : this.status.getValue(); 2709 } 2710 2711 /** 2712 * @param value The status of this operation definition. Enables tracking the 2713 * life-cycle of the content. 2714 */ 2715 public OperationDefinition setStatus(PublicationStatus value) { 2716 if (this.status == null) 2717 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2718 this.status.setValue(value); 2719 return this; 2720 } 2721 2722 /** 2723 * @return {@link #kind} (Whether this is an operation or a named query.). This 2724 * is the underlying object with id, value and extensions. The accessor 2725 * "getKind" gives direct access to the value 2726 */ 2727 public Enumeration<OperationKind> getKindElement() { 2728 if (this.kind == null) 2729 if (Configuration.errorOnAutoCreate()) 2730 throw new Error("Attempt to auto-create OperationDefinition.kind"); 2731 else if (Configuration.doAutoCreate()) 2732 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); // bb 2733 return this.kind; 2734 } 2735 2736 public boolean hasKindElement() { 2737 return this.kind != null && !this.kind.isEmpty(); 2738 } 2739 2740 public boolean hasKind() { 2741 return this.kind != null && !this.kind.isEmpty(); 2742 } 2743 2744 /** 2745 * @param value {@link #kind} (Whether this is an operation or a named query.). 2746 * This is the underlying object with id, value and extensions. The 2747 * accessor "getKind" gives direct access to the value 2748 */ 2749 public OperationDefinition setKindElement(Enumeration<OperationKind> value) { 2750 this.kind = value; 2751 return this; 2752 } 2753 2754 /** 2755 * @return Whether this is an operation or a named query. 2756 */ 2757 public OperationKind getKind() { 2758 return this.kind == null ? null : this.kind.getValue(); 2759 } 2760 2761 /** 2762 * @param value Whether this is an operation or a named query. 2763 */ 2764 public OperationDefinition setKind(OperationKind value) { 2765 if (this.kind == null) 2766 this.kind = new Enumeration<OperationKind>(new OperationKindEnumFactory()); 2767 this.kind.setValue(value); 2768 return this; 2769 } 2770 2771 /** 2772 * @return {@link #experimental} (A Boolean value to indicate that this 2773 * operation definition is authored for testing purposes (or 2774 * education/evaluation/marketing) and is not intended to be used for 2775 * genuine usage.). This is the underlying object with id, value and 2776 * extensions. The accessor "getExperimental" gives direct access to the 2777 * value 2778 */ 2779 public BooleanType getExperimentalElement() { 2780 if (this.experimental == null) 2781 if (Configuration.errorOnAutoCreate()) 2782 throw new Error("Attempt to auto-create OperationDefinition.experimental"); 2783 else if (Configuration.doAutoCreate()) 2784 this.experimental = new BooleanType(); // bb 2785 return this.experimental; 2786 } 2787 2788 public boolean hasExperimentalElement() { 2789 return this.experimental != null && !this.experimental.isEmpty(); 2790 } 2791 2792 public boolean hasExperimental() { 2793 return this.experimental != null && !this.experimental.isEmpty(); 2794 } 2795 2796 /** 2797 * @param value {@link #experimental} (A Boolean value to indicate that this 2798 * operation definition is authored for testing purposes (or 2799 * education/evaluation/marketing) and is not intended to be used 2800 * for genuine usage.). This is the underlying object with id, 2801 * value and extensions. The accessor "getExperimental" gives 2802 * direct access to the value 2803 */ 2804 public OperationDefinition setExperimentalElement(BooleanType value) { 2805 this.experimental = value; 2806 return this; 2807 } 2808 2809 /** 2810 * @return A Boolean value to indicate that this operation definition is 2811 * authored for testing purposes (or education/evaluation/marketing) and 2812 * is not intended to be used for genuine usage. 2813 */ 2814 public boolean getExperimental() { 2815 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2816 } 2817 2818 /** 2819 * @param value A Boolean value to indicate that this operation definition is 2820 * authored for testing purposes (or 2821 * education/evaluation/marketing) and is not intended to be used 2822 * for genuine usage. 2823 */ 2824 public OperationDefinition setExperimental(boolean value) { 2825 if (this.experimental == null) 2826 this.experimental = new BooleanType(); 2827 this.experimental.setValue(value); 2828 return this; 2829 } 2830 2831 /** 2832 * @return {@link #date} (The date (and optionally time) when the operation 2833 * definition was published. The date must change when the business 2834 * version changes and it must change if the status code changes. In 2835 * addition, it should change when the substantive content of the 2836 * operation definition changes.). This is the underlying object with 2837 * id, value and extensions. The accessor "getDate" gives direct access 2838 * to the value 2839 */ 2840 public DateTimeType getDateElement() { 2841 if (this.date == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create OperationDefinition.date"); 2844 else if (Configuration.doAutoCreate()) 2845 this.date = new DateTimeType(); // bb 2846 return this.date; 2847 } 2848 2849 public boolean hasDateElement() { 2850 return this.date != null && !this.date.isEmpty(); 2851 } 2852 2853 public boolean hasDate() { 2854 return this.date != null && !this.date.isEmpty(); 2855 } 2856 2857 /** 2858 * @param value {@link #date} (The date (and optionally time) when the operation 2859 * definition was published. The date must change when the business 2860 * version changes and it must change if the status code changes. 2861 * In addition, it should change when the substantive content of 2862 * the operation definition changes.). This is the underlying 2863 * object with id, value and extensions. The accessor "getDate" 2864 * gives direct access to the value 2865 */ 2866 public OperationDefinition setDateElement(DateTimeType value) { 2867 this.date = value; 2868 return this; 2869 } 2870 2871 /** 2872 * @return The date (and optionally time) when the operation definition was 2873 * published. The date must change when the business version changes and 2874 * it must change if the status code changes. In addition, it should 2875 * change when the substantive content of the operation definition 2876 * changes. 2877 */ 2878 public Date getDate() { 2879 return this.date == null ? null : this.date.getValue(); 2880 } 2881 2882 /** 2883 * @param value The date (and optionally time) when the operation definition was 2884 * published. The date must change when the business version 2885 * changes and it must change if the status code changes. In 2886 * addition, it should change when the substantive content of the 2887 * operation definition changes. 2888 */ 2889 public OperationDefinition setDate(Date value) { 2890 if (value == null) 2891 this.date = null; 2892 else { 2893 if (this.date == null) 2894 this.date = new DateTimeType(); 2895 this.date.setValue(value); 2896 } 2897 return this; 2898 } 2899 2900 /** 2901 * @return {@link #publisher} (The name of the organization or individual that 2902 * published the operation definition.). This is the underlying object 2903 * with id, value and extensions. The accessor "getPublisher" gives 2904 * direct access to the value 2905 */ 2906 public StringType getPublisherElement() { 2907 if (this.publisher == null) 2908 if (Configuration.errorOnAutoCreate()) 2909 throw new Error("Attempt to auto-create OperationDefinition.publisher"); 2910 else if (Configuration.doAutoCreate()) 2911 this.publisher = new StringType(); // bb 2912 return this.publisher; 2913 } 2914 2915 public boolean hasPublisherElement() { 2916 return this.publisher != null && !this.publisher.isEmpty(); 2917 } 2918 2919 public boolean hasPublisher() { 2920 return this.publisher != null && !this.publisher.isEmpty(); 2921 } 2922 2923 /** 2924 * @param value {@link #publisher} (The name of the organization or individual 2925 * that published the operation definition.). This is the 2926 * underlying object with id, value and extensions. The accessor 2927 * "getPublisher" gives direct access to the value 2928 */ 2929 public OperationDefinition setPublisherElement(StringType value) { 2930 this.publisher = value; 2931 return this; 2932 } 2933 2934 /** 2935 * @return The name of the organization or individual that published the 2936 * operation definition. 2937 */ 2938 public String getPublisher() { 2939 return this.publisher == null ? null : this.publisher.getValue(); 2940 } 2941 2942 /** 2943 * @param value The name of the organization or individual that published the 2944 * operation definition. 2945 */ 2946 public OperationDefinition setPublisher(String value) { 2947 if (Utilities.noString(value)) 2948 this.publisher = null; 2949 else { 2950 if (this.publisher == null) 2951 this.publisher = new StringType(); 2952 this.publisher.setValue(value); 2953 } 2954 return this; 2955 } 2956 2957 /** 2958 * @return {@link #contact} (Contact details to assist a user in finding and 2959 * communicating with the publisher.) 2960 */ 2961 public List<ContactDetail> getContact() { 2962 if (this.contact == null) 2963 this.contact = new ArrayList<ContactDetail>(); 2964 return this.contact; 2965 } 2966 2967 /** 2968 * @return Returns a reference to <code>this</code> for easy method chaining 2969 */ 2970 public OperationDefinition setContact(List<ContactDetail> theContact) { 2971 this.contact = theContact; 2972 return this; 2973 } 2974 2975 public boolean hasContact() { 2976 if (this.contact == null) 2977 return false; 2978 for (ContactDetail item : this.contact) 2979 if (!item.isEmpty()) 2980 return true; 2981 return false; 2982 } 2983 2984 public ContactDetail addContact() { // 3 2985 ContactDetail t = new ContactDetail(); 2986 if (this.contact == null) 2987 this.contact = new ArrayList<ContactDetail>(); 2988 this.contact.add(t); 2989 return t; 2990 } 2991 2992 public OperationDefinition addContact(ContactDetail t) { // 3 2993 if (t == null) 2994 return this; 2995 if (this.contact == null) 2996 this.contact = new ArrayList<ContactDetail>(); 2997 this.contact.add(t); 2998 return this; 2999 } 3000 3001 /** 3002 * @return The first repetition of repeating field {@link #contact}, creating it 3003 * if it does not already exist 3004 */ 3005 public ContactDetail getContactFirstRep() { 3006 if (getContact().isEmpty()) { 3007 addContact(); 3008 } 3009 return getContact().get(0); 3010 } 3011 3012 /** 3013 * @return {@link #description} (A free text natural language description of the 3014 * operation definition from a consumer's perspective.). This is the 3015 * underlying object with id, value and extensions. The accessor 3016 * "getDescription" gives direct access to the value 3017 */ 3018 public MarkdownType getDescriptionElement() { 3019 if (this.description == null) 3020 if (Configuration.errorOnAutoCreate()) 3021 throw new Error("Attempt to auto-create OperationDefinition.description"); 3022 else if (Configuration.doAutoCreate()) 3023 this.description = new MarkdownType(); // bb 3024 return this.description; 3025 } 3026 3027 public boolean hasDescriptionElement() { 3028 return this.description != null && !this.description.isEmpty(); 3029 } 3030 3031 public boolean hasDescription() { 3032 return this.description != null && !this.description.isEmpty(); 3033 } 3034 3035 /** 3036 * @param value {@link #description} (A free text natural language description 3037 * of the operation definition from a consumer's perspective.). 3038 * This is the underlying object with id, value and extensions. The 3039 * accessor "getDescription" gives direct access to the value 3040 */ 3041 public OperationDefinition setDescriptionElement(MarkdownType value) { 3042 this.description = value; 3043 return this; 3044 } 3045 3046 /** 3047 * @return A free text natural language description of the operation definition 3048 * from a consumer's perspective. 3049 */ 3050 public String getDescription() { 3051 return this.description == null ? null : this.description.getValue(); 3052 } 3053 3054 /** 3055 * @param value A free text natural language description of the operation 3056 * definition from a consumer's perspective. 3057 */ 3058 public OperationDefinition setDescription(String value) { 3059 if (value == null) 3060 this.description = null; 3061 else { 3062 if (this.description == null) 3063 this.description = new MarkdownType(); 3064 this.description.setValue(value); 3065 } 3066 return this; 3067 } 3068 3069 /** 3070 * @return {@link #useContext} (The content was developed with a focus and 3071 * intent of supporting the contexts that are listed. These contexts may 3072 * be general categories (gender, age, ...) or may be references to 3073 * specific programs (insurance plans, studies, ...) and may be used to 3074 * assist with indexing and searching for appropriate operation 3075 * definition instances.) 3076 */ 3077 public List<UsageContext> getUseContext() { 3078 if (this.useContext == null) 3079 this.useContext = new ArrayList<UsageContext>(); 3080 return this.useContext; 3081 } 3082 3083 /** 3084 * @return Returns a reference to <code>this</code> for easy method chaining 3085 */ 3086 public OperationDefinition setUseContext(List<UsageContext> theUseContext) { 3087 this.useContext = theUseContext; 3088 return this; 3089 } 3090 3091 public boolean hasUseContext() { 3092 if (this.useContext == null) 3093 return false; 3094 for (UsageContext item : this.useContext) 3095 if (!item.isEmpty()) 3096 return true; 3097 return false; 3098 } 3099 3100 public UsageContext addUseContext() { // 3 3101 UsageContext t = new UsageContext(); 3102 if (this.useContext == null) 3103 this.useContext = new ArrayList<UsageContext>(); 3104 this.useContext.add(t); 3105 return t; 3106 } 3107 3108 public OperationDefinition addUseContext(UsageContext t) { // 3 3109 if (t == null) 3110 return this; 3111 if (this.useContext == null) 3112 this.useContext = new ArrayList<UsageContext>(); 3113 this.useContext.add(t); 3114 return this; 3115 } 3116 3117 /** 3118 * @return The first repetition of repeating field {@link #useContext}, creating 3119 * it if it does not already exist 3120 */ 3121 public UsageContext getUseContextFirstRep() { 3122 if (getUseContext().isEmpty()) { 3123 addUseContext(); 3124 } 3125 return getUseContext().get(0); 3126 } 3127 3128 /** 3129 * @return {@link #jurisdiction} (A legal or geographic region in which the 3130 * operation definition is intended to be used.) 3131 */ 3132 public List<CodeableConcept> getJurisdiction() { 3133 if (this.jurisdiction == null) 3134 this.jurisdiction = new ArrayList<CodeableConcept>(); 3135 return this.jurisdiction; 3136 } 3137 3138 /** 3139 * @return Returns a reference to <code>this</code> for easy method chaining 3140 */ 3141 public OperationDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 3142 this.jurisdiction = theJurisdiction; 3143 return this; 3144 } 3145 3146 public boolean hasJurisdiction() { 3147 if (this.jurisdiction == null) 3148 return false; 3149 for (CodeableConcept item : this.jurisdiction) 3150 if (!item.isEmpty()) 3151 return true; 3152 return false; 3153 } 3154 3155 public CodeableConcept addJurisdiction() { // 3 3156 CodeableConcept t = new CodeableConcept(); 3157 if (this.jurisdiction == null) 3158 this.jurisdiction = new ArrayList<CodeableConcept>(); 3159 this.jurisdiction.add(t); 3160 return t; 3161 } 3162 3163 public OperationDefinition addJurisdiction(CodeableConcept t) { // 3 3164 if (t == null) 3165 return this; 3166 if (this.jurisdiction == null) 3167 this.jurisdiction = new ArrayList<CodeableConcept>(); 3168 this.jurisdiction.add(t); 3169 return this; 3170 } 3171 3172 /** 3173 * @return The first repetition of repeating field {@link #jurisdiction}, 3174 * creating it if it does not already exist 3175 */ 3176 public CodeableConcept getJurisdictionFirstRep() { 3177 if (getJurisdiction().isEmpty()) { 3178 addJurisdiction(); 3179 } 3180 return getJurisdiction().get(0); 3181 } 3182 3183 /** 3184 * @return {@link #purpose} (Explanation of why this operation definition is 3185 * needed and why it has been designed as it has.). This is the 3186 * underlying object with id, value and extensions. The accessor 3187 * "getPurpose" gives direct access to the value 3188 */ 3189 public MarkdownType getPurposeElement() { 3190 if (this.purpose == null) 3191 if (Configuration.errorOnAutoCreate()) 3192 throw new Error("Attempt to auto-create OperationDefinition.purpose"); 3193 else if (Configuration.doAutoCreate()) 3194 this.purpose = new MarkdownType(); // bb 3195 return this.purpose; 3196 } 3197 3198 public boolean hasPurposeElement() { 3199 return this.purpose != null && !this.purpose.isEmpty(); 3200 } 3201 3202 public boolean hasPurpose() { 3203 return this.purpose != null && !this.purpose.isEmpty(); 3204 } 3205 3206 /** 3207 * @param value {@link #purpose} (Explanation of why this operation definition 3208 * is needed and why it has been designed as it has.). This is the 3209 * underlying object with id, value and extensions. The accessor 3210 * "getPurpose" gives direct access to the value 3211 */ 3212 public OperationDefinition setPurposeElement(MarkdownType value) { 3213 this.purpose = value; 3214 return this; 3215 } 3216 3217 /** 3218 * @return Explanation of why this operation definition is needed and why it has 3219 * been designed as it has. 3220 */ 3221 public String getPurpose() { 3222 return this.purpose == null ? null : this.purpose.getValue(); 3223 } 3224 3225 /** 3226 * @param value Explanation of why this operation definition is needed and why 3227 * it has been designed as it has. 3228 */ 3229 public OperationDefinition setPurpose(String value) { 3230 if (value == null) 3231 this.purpose = null; 3232 else { 3233 if (this.purpose == null) 3234 this.purpose = new MarkdownType(); 3235 this.purpose.setValue(value); 3236 } 3237 return this; 3238 } 3239 3240 /** 3241 * @return {@link #affectsState} (Whether the operation affects state. Side 3242 * effects such as producing audit trail entries do not count as 3243 * 'affecting state'.). This is the underlying object with id, value and 3244 * extensions. The accessor "getAffectsState" gives direct access to the 3245 * value 3246 */ 3247 public BooleanType getAffectsStateElement() { 3248 if (this.affectsState == null) 3249 if (Configuration.errorOnAutoCreate()) 3250 throw new Error("Attempt to auto-create OperationDefinition.affectsState"); 3251 else if (Configuration.doAutoCreate()) 3252 this.affectsState = new BooleanType(); // bb 3253 return this.affectsState; 3254 } 3255 3256 public boolean hasAffectsStateElement() { 3257 return this.affectsState != null && !this.affectsState.isEmpty(); 3258 } 3259 3260 public boolean hasAffectsState() { 3261 return this.affectsState != null && !this.affectsState.isEmpty(); 3262 } 3263 3264 /** 3265 * @param value {@link #affectsState} (Whether the operation affects state. Side 3266 * effects such as producing audit trail entries do not count as 3267 * 'affecting state'.). This is the underlying object with id, 3268 * value and extensions. The accessor "getAffectsState" gives 3269 * direct access to the value 3270 */ 3271 public OperationDefinition setAffectsStateElement(BooleanType value) { 3272 this.affectsState = value; 3273 return this; 3274 } 3275 3276 /** 3277 * @return Whether the operation affects state. Side effects such as producing 3278 * audit trail entries do not count as 'affecting state'. 3279 */ 3280 public boolean getAffectsState() { 3281 return this.affectsState == null || this.affectsState.isEmpty() ? false : this.affectsState.getValue(); 3282 } 3283 3284 /** 3285 * @param value Whether the operation affects state. Side effects such as 3286 * producing audit trail entries do not count as 'affecting state'. 3287 */ 3288 public OperationDefinition setAffectsState(boolean value) { 3289 if (this.affectsState == null) 3290 this.affectsState = new BooleanType(); 3291 this.affectsState.setValue(value); 3292 return this; 3293 } 3294 3295 /** 3296 * @return {@link #code} (The name used to invoke the operation.). This is the 3297 * underlying object with id, value and extensions. The accessor 3298 * "getCode" gives direct access to the value 3299 */ 3300 public CodeType getCodeElement() { 3301 if (this.code == null) 3302 if (Configuration.errorOnAutoCreate()) 3303 throw new Error("Attempt to auto-create OperationDefinition.code"); 3304 else if (Configuration.doAutoCreate()) 3305 this.code = new CodeType(); // bb 3306 return this.code; 3307 } 3308 3309 public boolean hasCodeElement() { 3310 return this.code != null && !this.code.isEmpty(); 3311 } 3312 3313 public boolean hasCode() { 3314 return this.code != null && !this.code.isEmpty(); 3315 } 3316 3317 /** 3318 * @param value {@link #code} (The name used to invoke the operation.). This is 3319 * the underlying object with id, value and extensions. The 3320 * accessor "getCode" gives direct access to the value 3321 */ 3322 public OperationDefinition setCodeElement(CodeType value) { 3323 this.code = value; 3324 return this; 3325 } 3326 3327 /** 3328 * @return The name used to invoke the operation. 3329 */ 3330 public String getCode() { 3331 return this.code == null ? null : this.code.getValue(); 3332 } 3333 3334 /** 3335 * @param value The name used to invoke the operation. 3336 */ 3337 public OperationDefinition setCode(String value) { 3338 if (this.code == null) 3339 this.code = new CodeType(); 3340 this.code.setValue(value); 3341 return this; 3342 } 3343 3344 /** 3345 * @return {@link #comment} (Additional information about how to use this 3346 * operation or named query.). This is the underlying object with id, 3347 * value and extensions. The accessor "getComment" gives direct access 3348 * to the value 3349 */ 3350 public MarkdownType getCommentElement() { 3351 if (this.comment == null) 3352 if (Configuration.errorOnAutoCreate()) 3353 throw new Error("Attempt to auto-create OperationDefinition.comment"); 3354 else if (Configuration.doAutoCreate()) 3355 this.comment = new MarkdownType(); // bb 3356 return this.comment; 3357 } 3358 3359 public boolean hasCommentElement() { 3360 return this.comment != null && !this.comment.isEmpty(); 3361 } 3362 3363 public boolean hasComment() { 3364 return this.comment != null && !this.comment.isEmpty(); 3365 } 3366 3367 /** 3368 * @param value {@link #comment} (Additional information about how to use this 3369 * operation or named query.). This is the underlying object with 3370 * id, value and extensions. The accessor "getComment" gives direct 3371 * access to the value 3372 */ 3373 public OperationDefinition setCommentElement(MarkdownType value) { 3374 this.comment = value; 3375 return this; 3376 } 3377 3378 /** 3379 * @return Additional information about how to use this operation or named 3380 * query. 3381 */ 3382 public String getComment() { 3383 return this.comment == null ? null : this.comment.getValue(); 3384 } 3385 3386 /** 3387 * @param value Additional information about how to use this operation or named 3388 * query. 3389 */ 3390 public OperationDefinition setComment(String value) { 3391 if (value == null) 3392 this.comment = null; 3393 else { 3394 if (this.comment == null) 3395 this.comment = new MarkdownType(); 3396 this.comment.setValue(value); 3397 } 3398 return this; 3399 } 3400 3401 /** 3402 * @return {@link #base} (Indicates that this operation definition is a 3403 * constraining profile on the base.). This is the underlying object 3404 * with id, value and extensions. The accessor "getBase" gives direct 3405 * access to the value 3406 */ 3407 public CanonicalType getBaseElement() { 3408 if (this.base == null) 3409 if (Configuration.errorOnAutoCreate()) 3410 throw new Error("Attempt to auto-create OperationDefinition.base"); 3411 else if (Configuration.doAutoCreate()) 3412 this.base = new CanonicalType(); // bb 3413 return this.base; 3414 } 3415 3416 public boolean hasBaseElement() { 3417 return this.base != null && !this.base.isEmpty(); 3418 } 3419 3420 public boolean hasBase() { 3421 return this.base != null && !this.base.isEmpty(); 3422 } 3423 3424 /** 3425 * @param value {@link #base} (Indicates that this operation definition is a 3426 * constraining profile on the base.). This is the underlying 3427 * object with id, value and extensions. The accessor "getBase" 3428 * gives direct access to the value 3429 */ 3430 public OperationDefinition setBaseElement(CanonicalType value) { 3431 this.base = value; 3432 return this; 3433 } 3434 3435 /** 3436 * @return Indicates that this operation definition is a constraining profile on 3437 * the base. 3438 */ 3439 public String getBase() { 3440 return this.base == null ? null : this.base.getValue(); 3441 } 3442 3443 /** 3444 * @param value Indicates that this operation definition is a constraining 3445 * profile on the base. 3446 */ 3447 public OperationDefinition setBase(String value) { 3448 if (Utilities.noString(value)) 3449 this.base = null; 3450 else { 3451 if (this.base == null) 3452 this.base = new CanonicalType(); 3453 this.base.setValue(value); 3454 } 3455 return this; 3456 } 3457 3458 /** 3459 * @return {@link #resource} (The types on which this operation can be 3460 * executed.) 3461 */ 3462 public List<CodeType> getResource() { 3463 if (this.resource == null) 3464 this.resource = new ArrayList<CodeType>(); 3465 return this.resource; 3466 } 3467 3468 /** 3469 * @return Returns a reference to <code>this</code> for easy method chaining 3470 */ 3471 public OperationDefinition setResource(List<CodeType> theResource) { 3472 this.resource = theResource; 3473 return this; 3474 } 3475 3476 public boolean hasResource() { 3477 if (this.resource == null) 3478 return false; 3479 for (CodeType item : this.resource) 3480 if (!item.isEmpty()) 3481 return true; 3482 return false; 3483 } 3484 3485 /** 3486 * @return {@link #resource} (The types on which this operation can be 3487 * executed.) 3488 */ 3489 public CodeType addResourceElement() {// 2 3490 CodeType t = new CodeType(); 3491 if (this.resource == null) 3492 this.resource = new ArrayList<CodeType>(); 3493 this.resource.add(t); 3494 return t; 3495 } 3496 3497 /** 3498 * @param value {@link #resource} (The types on which this operation can be 3499 * executed.) 3500 */ 3501 public OperationDefinition addResource(String value) { // 1 3502 CodeType t = new CodeType(); 3503 t.setValue(value); 3504 if (this.resource == null) 3505 this.resource = new ArrayList<CodeType>(); 3506 this.resource.add(t); 3507 return this; 3508 } 3509 3510 /** 3511 * @param value {@link #resource} (The types on which this operation can be 3512 * executed.) 3513 */ 3514 public boolean hasResource(String value) { 3515 if (this.resource == null) 3516 return false; 3517 for (CodeType v : this.resource) 3518 if (v.getValue().equals(value)) // code 3519 return true; 3520 return false; 3521 } 3522 3523 /** 3524 * @return {@link #system} (Indicates whether this operation or named query can 3525 * be invoked at the system level (e.g. without needing to choose a 3526 * resource type for the context).). This is the underlying object with 3527 * id, value and extensions. The accessor "getSystem" gives direct 3528 * access to the value 3529 */ 3530 public BooleanType getSystemElement() { 3531 if (this.system == null) 3532 if (Configuration.errorOnAutoCreate()) 3533 throw new Error("Attempt to auto-create OperationDefinition.system"); 3534 else if (Configuration.doAutoCreate()) 3535 this.system = new BooleanType(); // bb 3536 return this.system; 3537 } 3538 3539 public boolean hasSystemElement() { 3540 return this.system != null && !this.system.isEmpty(); 3541 } 3542 3543 public boolean hasSystem() { 3544 return this.system != null && !this.system.isEmpty(); 3545 } 3546 3547 /** 3548 * @param value {@link #system} (Indicates whether this operation or named query 3549 * can be invoked at the system level (e.g. without needing to 3550 * choose a resource type for the context).). This is the 3551 * underlying object with id, value and extensions. The accessor 3552 * "getSystem" gives direct access to the value 3553 */ 3554 public OperationDefinition setSystemElement(BooleanType value) { 3555 this.system = value; 3556 return this; 3557 } 3558 3559 /** 3560 * @return Indicates whether this operation or named query can be invoked at the 3561 * system level (e.g. without needing to choose a resource type for the 3562 * context). 3563 */ 3564 public boolean getSystem() { 3565 return this.system == null || this.system.isEmpty() ? false : this.system.getValue(); 3566 } 3567 3568 /** 3569 * @param value Indicates whether this operation or named query can be invoked 3570 * at the system level (e.g. without needing to choose a resource 3571 * type for the context). 3572 */ 3573 public OperationDefinition setSystem(boolean value) { 3574 if (this.system == null) 3575 this.system = new BooleanType(); 3576 this.system.setValue(value); 3577 return this; 3578 } 3579 3580 /** 3581 * @return {@link #type} (Indicates whether this operation or named query can be 3582 * invoked at the resource type level for any given resource type level 3583 * (e.g. without needing to choose a specific resource id for the 3584 * context).). This is the underlying object with id, value and 3585 * extensions. The accessor "getType" gives direct access to the value 3586 */ 3587 public BooleanType getTypeElement() { 3588 if (this.type == null) 3589 if (Configuration.errorOnAutoCreate()) 3590 throw new Error("Attempt to auto-create OperationDefinition.type"); 3591 else if (Configuration.doAutoCreate()) 3592 this.type = new BooleanType(); // bb 3593 return this.type; 3594 } 3595 3596 public boolean hasTypeElement() { 3597 return this.type != null && !this.type.isEmpty(); 3598 } 3599 3600 public boolean hasType() { 3601 return this.type != null && !this.type.isEmpty(); 3602 } 3603 3604 /** 3605 * @param value {@link #type} (Indicates whether this operation or named query 3606 * can be invoked at the resource type level for any given resource 3607 * type level (e.g. without needing to choose a specific resource 3608 * id for the context).). This is the underlying object with id, 3609 * value and extensions. The accessor "getType" gives direct access 3610 * to the value 3611 */ 3612 public OperationDefinition setTypeElement(BooleanType value) { 3613 this.type = value; 3614 return this; 3615 } 3616 3617 /** 3618 * @return Indicates whether this operation or named query can be invoked at the 3619 * resource type level for any given resource type level (e.g. without 3620 * needing to choose a specific resource id for the context). 3621 */ 3622 public boolean getType() { 3623 return this.type == null || this.type.isEmpty() ? false : this.type.getValue(); 3624 } 3625 3626 /** 3627 * @param value Indicates whether this operation or named query can be invoked 3628 * at the resource type level for any given resource type level 3629 * (e.g. without needing to choose a specific resource id for the 3630 * context). 3631 */ 3632 public OperationDefinition setType(boolean value) { 3633 if (this.type == null) 3634 this.type = new BooleanType(); 3635 this.type.setValue(value); 3636 return this; 3637 } 3638 3639 /** 3640 * @return {@link #instance} (Indicates whether this operation can be invoked on 3641 * a particular instance of one of the given types.). This is the 3642 * underlying object with id, value and extensions. The accessor 3643 * "getInstance" gives direct access to the value 3644 */ 3645 public BooleanType getInstanceElement() { 3646 if (this.instance == null) 3647 if (Configuration.errorOnAutoCreate()) 3648 throw new Error("Attempt to auto-create OperationDefinition.instance"); 3649 else if (Configuration.doAutoCreate()) 3650 this.instance = new BooleanType(); // bb 3651 return this.instance; 3652 } 3653 3654 public boolean hasInstanceElement() { 3655 return this.instance != null && !this.instance.isEmpty(); 3656 } 3657 3658 public boolean hasInstance() { 3659 return this.instance != null && !this.instance.isEmpty(); 3660 } 3661 3662 /** 3663 * @param value {@link #instance} (Indicates whether this operation can be 3664 * invoked on a particular instance of one of the given types.). 3665 * This is the underlying object with id, value and extensions. The 3666 * accessor "getInstance" gives direct access to the value 3667 */ 3668 public OperationDefinition setInstanceElement(BooleanType value) { 3669 this.instance = value; 3670 return this; 3671 } 3672 3673 /** 3674 * @return Indicates whether this operation can be invoked on a particular 3675 * instance of one of the given types. 3676 */ 3677 public boolean getInstance() { 3678 return this.instance == null || this.instance.isEmpty() ? false : this.instance.getValue(); 3679 } 3680 3681 /** 3682 * @param value Indicates whether this operation can be invoked on a particular 3683 * instance of one of the given types. 3684 */ 3685 public OperationDefinition setInstance(boolean value) { 3686 if (this.instance == null) 3687 this.instance = new BooleanType(); 3688 this.instance.setValue(value); 3689 return this; 3690 } 3691 3692 /** 3693 * @return {@link #inputProfile} (Additional validation information for the in 3694 * parameters - a single profile that covers all the parameters. The 3695 * profile is a constraint on the parameters resource as a whole.). This 3696 * is the underlying object with id, value and extensions. The accessor 3697 * "getInputProfile" gives direct access to the value 3698 */ 3699 public CanonicalType getInputProfileElement() { 3700 if (this.inputProfile == null) 3701 if (Configuration.errorOnAutoCreate()) 3702 throw new Error("Attempt to auto-create OperationDefinition.inputProfile"); 3703 else if (Configuration.doAutoCreate()) 3704 this.inputProfile = new CanonicalType(); // bb 3705 return this.inputProfile; 3706 } 3707 3708 public boolean hasInputProfileElement() { 3709 return this.inputProfile != null && !this.inputProfile.isEmpty(); 3710 } 3711 3712 public boolean hasInputProfile() { 3713 return this.inputProfile != null && !this.inputProfile.isEmpty(); 3714 } 3715 3716 /** 3717 * @param value {@link #inputProfile} (Additional validation information for the 3718 * in parameters - a single profile that covers all the parameters. 3719 * The profile is a constraint on the parameters resource as a 3720 * whole.). This is the underlying object with id, value and 3721 * extensions. The accessor "getInputProfile" gives direct access 3722 * to the value 3723 */ 3724 public OperationDefinition setInputProfileElement(CanonicalType value) { 3725 this.inputProfile = value; 3726 return this; 3727 } 3728 3729 /** 3730 * @return Additional validation information for the in parameters - a single 3731 * profile that covers all the parameters. The profile is a constraint 3732 * on the parameters resource as a whole. 3733 */ 3734 public String getInputProfile() { 3735 return this.inputProfile == null ? null : this.inputProfile.getValue(); 3736 } 3737 3738 /** 3739 * @param value Additional validation information for the in parameters - a 3740 * single profile that covers all the parameters. The profile is a 3741 * constraint on the parameters resource as a whole. 3742 */ 3743 public OperationDefinition setInputProfile(String value) { 3744 if (Utilities.noString(value)) 3745 this.inputProfile = null; 3746 else { 3747 if (this.inputProfile == null) 3748 this.inputProfile = new CanonicalType(); 3749 this.inputProfile.setValue(value); 3750 } 3751 return this; 3752 } 3753 3754 /** 3755 * @return {@link #outputProfile} (Additional validation information for the out 3756 * parameters - a single profile that covers all the parameters. The 3757 * profile is a constraint on the parameters resource.). This is the 3758 * underlying object with id, value and extensions. The accessor 3759 * "getOutputProfile" gives direct access to the value 3760 */ 3761 public CanonicalType getOutputProfileElement() { 3762 if (this.outputProfile == null) 3763 if (Configuration.errorOnAutoCreate()) 3764 throw new Error("Attempt to auto-create OperationDefinition.outputProfile"); 3765 else if (Configuration.doAutoCreate()) 3766 this.outputProfile = new CanonicalType(); // bb 3767 return this.outputProfile; 3768 } 3769 3770 public boolean hasOutputProfileElement() { 3771 return this.outputProfile != null && !this.outputProfile.isEmpty(); 3772 } 3773 3774 public boolean hasOutputProfile() { 3775 return this.outputProfile != null && !this.outputProfile.isEmpty(); 3776 } 3777 3778 /** 3779 * @param value {@link #outputProfile} (Additional validation information for 3780 * the out parameters - a single profile that covers all the 3781 * parameters. The profile is a constraint on the parameters 3782 * resource.). This is the underlying object with id, value and 3783 * extensions. The accessor "getOutputProfile" gives direct access 3784 * to the value 3785 */ 3786 public OperationDefinition setOutputProfileElement(CanonicalType value) { 3787 this.outputProfile = value; 3788 return this; 3789 } 3790 3791 /** 3792 * @return Additional validation information for the out parameters - a single 3793 * profile that covers all the parameters. The profile is a constraint 3794 * on the parameters resource. 3795 */ 3796 public String getOutputProfile() { 3797 return this.outputProfile == null ? null : this.outputProfile.getValue(); 3798 } 3799 3800 /** 3801 * @param value Additional validation information for the out parameters - a 3802 * single profile that covers all the parameters. The profile is a 3803 * constraint on the parameters resource. 3804 */ 3805 public OperationDefinition setOutputProfile(String value) { 3806 if (Utilities.noString(value)) 3807 this.outputProfile = null; 3808 else { 3809 if (this.outputProfile == null) 3810 this.outputProfile = new CanonicalType(); 3811 this.outputProfile.setValue(value); 3812 } 3813 return this; 3814 } 3815 3816 /** 3817 * @return {@link #parameter} (The parameters for the operation/query.) 3818 */ 3819 public List<OperationDefinitionParameterComponent> getParameter() { 3820 if (this.parameter == null) 3821 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3822 return this.parameter; 3823 } 3824 3825 /** 3826 * @return Returns a reference to <code>this</code> for easy method chaining 3827 */ 3828 public OperationDefinition setParameter(List<OperationDefinitionParameterComponent> theParameter) { 3829 this.parameter = theParameter; 3830 return this; 3831 } 3832 3833 public boolean hasParameter() { 3834 if (this.parameter == null) 3835 return false; 3836 for (OperationDefinitionParameterComponent item : this.parameter) 3837 if (!item.isEmpty()) 3838 return true; 3839 return false; 3840 } 3841 3842 public OperationDefinitionParameterComponent addParameter() { // 3 3843 OperationDefinitionParameterComponent t = new OperationDefinitionParameterComponent(); 3844 if (this.parameter == null) 3845 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3846 this.parameter.add(t); 3847 return t; 3848 } 3849 3850 public OperationDefinition addParameter(OperationDefinitionParameterComponent t) { // 3 3851 if (t == null) 3852 return this; 3853 if (this.parameter == null) 3854 this.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 3855 this.parameter.add(t); 3856 return this; 3857 } 3858 3859 /** 3860 * @return The first repetition of repeating field {@link #parameter}, creating 3861 * it if it does not already exist 3862 */ 3863 public OperationDefinitionParameterComponent getParameterFirstRep() { 3864 if (getParameter().isEmpty()) { 3865 addParameter(); 3866 } 3867 return getParameter().get(0); 3868 } 3869 3870 /** 3871 * @return {@link #overload} (Defines an appropriate combination of parameters 3872 * to use when invoking this operation, to help code generators when 3873 * generating overloaded parameter sets for this operation.) 3874 */ 3875 public List<OperationDefinitionOverloadComponent> getOverload() { 3876 if (this.overload == null) 3877 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3878 return this.overload; 3879 } 3880 3881 /** 3882 * @return Returns a reference to <code>this</code> for easy method chaining 3883 */ 3884 public OperationDefinition setOverload(List<OperationDefinitionOverloadComponent> theOverload) { 3885 this.overload = theOverload; 3886 return this; 3887 } 3888 3889 public boolean hasOverload() { 3890 if (this.overload == null) 3891 return false; 3892 for (OperationDefinitionOverloadComponent item : this.overload) 3893 if (!item.isEmpty()) 3894 return true; 3895 return false; 3896 } 3897 3898 public OperationDefinitionOverloadComponent addOverload() { // 3 3899 OperationDefinitionOverloadComponent t = new OperationDefinitionOverloadComponent(); 3900 if (this.overload == null) 3901 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3902 this.overload.add(t); 3903 return t; 3904 } 3905 3906 public OperationDefinition addOverload(OperationDefinitionOverloadComponent t) { // 3 3907 if (t == null) 3908 return this; 3909 if (this.overload == null) 3910 this.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 3911 this.overload.add(t); 3912 return this; 3913 } 3914 3915 /** 3916 * @return The first repetition of repeating field {@link #overload}, creating 3917 * it if it does not already exist 3918 */ 3919 public OperationDefinitionOverloadComponent getOverloadFirstRep() { 3920 if (getOverload().isEmpty()) { 3921 addOverload(); 3922 } 3923 return getOverload().get(0); 3924 } 3925 3926 protected void listChildren(List<Property> children) { 3927 super.listChildren(children); 3928 children.add(new Property("url", "uri", 3929 "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.", 3930 0, 1, url)); 3931 children.add(new Property("version", "string", 3932 "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 3933 0, 1, version)); 3934 children.add(new Property("name", "string", 3935 "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3936 0, 1, name)); 3937 children.add(new Property("title", "string", 3938 "A short, descriptive, user-friendly title for the operation definition.", 0, 1, title)); 3939 children.add(new Property("status", "code", 3940 "The status of this operation definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3941 children.add(new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind)); 3942 children.add(new Property("experimental", "boolean", 3943 "A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 3944 0, 1, experimental)); 3945 children.add(new Property("date", "dateTime", 3946 "The date (and optionally time) when the operation definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 3947 0, 1, date)); 3948 children.add(new Property("publisher", "string", 3949 "The name of the organization or individual that published the operation definition.", 0, 1, publisher)); 3950 children.add(new Property("contact", "ContactDetail", 3951 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3952 java.lang.Integer.MAX_VALUE, contact)); 3953 children.add(new Property("description", "markdown", 3954 "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, 3955 description)); 3956 children.add(new Property("useContext", "UsageContext", 3957 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition instances.", 3958 0, java.lang.Integer.MAX_VALUE, useContext)); 3959 children.add(new Property("jurisdiction", "CodeableConcept", 3960 "A legal or geographic region in which the operation definition is intended to be used.", 0, 3961 java.lang.Integer.MAX_VALUE, jurisdiction)); 3962 children.add(new Property("purpose", "markdown", 3963 "Explanation of why this operation definition is needed and why it has been designed as it has.", 0, 1, 3964 purpose)); 3965 children.add(new Property("affectsState", "boolean", 3966 "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.", 3967 0, 1, affectsState)); 3968 children.add(new Property("code", "code", "The name used to invoke the operation.", 0, 1, code)); 3969 children.add(new Property("comment", "markdown", 3970 "Additional information about how to use this operation or named query.", 0, 1, comment)); 3971 children.add(new Property("base", "canonical(OperationDefinition)", 3972 "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base)); 3973 children.add(new Property("resource", "code", "The types on which this operation can be executed.", 0, 3974 java.lang.Integer.MAX_VALUE, resource)); 3975 children.add(new Property("system", "boolean", 3976 "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 3977 0, 1, system)); 3978 children.add(new Property("type", "boolean", 3979 "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 3980 0, 1, type)); 3981 children.add(new Property("instance", "boolean", 3982 "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, 3983 instance)); 3984 children.add(new Property("inputProfile", "canonical(StructureDefinition)", 3985 "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.", 3986 0, 1, inputProfile)); 3987 children.add(new Property("outputProfile", "canonical(StructureDefinition)", 3988 "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.", 3989 0, 1, outputProfile)); 3990 children.add(new Property("parameter", "", "The parameters for the operation/query.", 0, 3991 java.lang.Integer.MAX_VALUE, parameter)); 3992 children.add(new Property("overload", "", 3993 "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 3994 0, java.lang.Integer.MAX_VALUE, overload)); 3995 } 3996 3997 @Override 3998 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3999 switch (_hash) { 4000 case 116079: 4001 /* url */ return new Property("url", "uri", 4002 "An absolute URI that is used to identify this operation definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this operation definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the operation definition is stored on different servers.", 4003 0, 1, url); 4004 case 351608024: 4005 /* version */ return new Property("version", "string", 4006 "The identifier that is used to identify this version of the operation definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the operation definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 4007 0, 1, version); 4008 case 3373707: 4009 /* name */ return new Property("name", "string", 4010 "A natural language name identifying the operation definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 4011 0, 1, name); 4012 case 110371416: 4013 /* title */ return new Property("title", "string", 4014 "A short, descriptive, user-friendly title for the operation definition.", 0, 1, title); 4015 case -892481550: 4016 /* status */ return new Property("status", "code", 4017 "The status of this operation definition. Enables tracking the life-cycle of the content.", 0, 1, status); 4018 case 3292052: 4019 /* kind */ return new Property("kind", "code", "Whether this is an operation or a named query.", 0, 1, kind); 4020 case -404562712: 4021 /* experimental */ return new Property("experimental", "boolean", 4022 "A Boolean value to indicate that this operation definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 4023 0, 1, experimental); 4024 case 3076014: 4025 /* date */ return new Property("date", "dateTime", 4026 "The date (and optionally time) when the operation definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the operation definition changes.", 4027 0, 1, date); 4028 case 1447404028: 4029 /* publisher */ return new Property("publisher", "string", 4030 "The name of the organization or individual that published the operation definition.", 0, 1, publisher); 4031 case 951526432: 4032 /* contact */ return new Property("contact", "ContactDetail", 4033 "Contact details to assist a user in finding and communicating with the publisher.", 0, 4034 java.lang.Integer.MAX_VALUE, contact); 4035 case -1724546052: 4036 /* description */ return new Property("description", "markdown", 4037 "A free text natural language description of the operation definition from a consumer's perspective.", 0, 1, 4038 description); 4039 case -669707736: 4040 /* useContext */ return new Property("useContext", "UsageContext", 4041 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate operation definition instances.", 4042 0, java.lang.Integer.MAX_VALUE, useContext); 4043 case -507075711: 4044 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 4045 "A legal or geographic region in which the operation definition is intended to be used.", 0, 4046 java.lang.Integer.MAX_VALUE, jurisdiction); 4047 case -220463842: 4048 /* purpose */ return new Property("purpose", "markdown", 4049 "Explanation of why this operation definition is needed and why it has been designed as it has.", 0, 1, 4050 purpose); 4051 case -14805197: 4052 /* affectsState */ return new Property("affectsState", "boolean", 4053 "Whether the operation affects state. Side effects such as producing audit trail entries do not count as 'affecting state'.", 4054 0, 1, affectsState); 4055 case 3059181: 4056 /* code */ return new Property("code", "code", "The name used to invoke the operation.", 0, 1, code); 4057 case 950398559: 4058 /* comment */ return new Property("comment", "markdown", 4059 "Additional information about how to use this operation or named query.", 0, 1, comment); 4060 case 3016401: 4061 /* base */ return new Property("base", "canonical(OperationDefinition)", 4062 "Indicates that this operation definition is a constraining profile on the base.", 0, 1, base); 4063 case -341064690: 4064 /* resource */ return new Property("resource", "code", "The types on which this operation can be executed.", 0, 4065 java.lang.Integer.MAX_VALUE, resource); 4066 case -887328209: 4067 /* system */ return new Property("system", "boolean", 4068 "Indicates whether this operation or named query can be invoked at the system level (e.g. without needing to choose a resource type for the context).", 4069 0, 1, system); 4070 case 3575610: 4071 /* type */ return new Property("type", "boolean", 4072 "Indicates whether this operation or named query can be invoked at the resource type level for any given resource type level (e.g. without needing to choose a specific resource id for the context).", 4073 0, 1, type); 4074 case 555127957: 4075 /* instance */ return new Property("instance", "boolean", 4076 "Indicates whether this operation can be invoked on a particular instance of one of the given types.", 0, 1, 4077 instance); 4078 case 676942463: 4079 /* inputProfile */ return new Property("inputProfile", "canonical(StructureDefinition)", 4080 "Additional validation information for the in parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource as a whole.", 4081 0, 1, inputProfile); 4082 case 1826166120: 4083 /* outputProfile */ return new Property("outputProfile", "canonical(StructureDefinition)", 4084 "Additional validation information for the out parameters - a single profile that covers all the parameters. The profile is a constraint on the parameters resource.", 4085 0, 1, outputProfile); 4086 case 1954460585: 4087 /* parameter */ return new Property("parameter", "", "The parameters for the operation/query.", 0, 4088 java.lang.Integer.MAX_VALUE, parameter); 4089 case 529823674: 4090 /* overload */ return new Property("overload", "", 4091 "Defines an appropriate combination of parameters to use when invoking this operation, to help code generators when generating overloaded parameter sets for this operation.", 4092 0, java.lang.Integer.MAX_VALUE, overload); 4093 default: 4094 return super.getNamedProperty(_hash, _name, _checkValid); 4095 } 4096 4097 } 4098 4099 @Override 4100 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4101 switch (hash) { 4102 case 116079: 4103 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 4104 case 351608024: 4105 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 4106 case 3373707: 4107 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4108 case 110371416: 4109 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4110 case -892481550: 4111 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4112 case 3292052: 4113 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<OperationKind> 4114 case -404562712: 4115 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 4116 case 3076014: 4117 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 4118 case 1447404028: 4119 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 4120 case 951526432: 4121 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 4122 case -1724546052: 4123 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4124 case -669707736: 4125 /* useContext */ return this.useContext == null ? new Base[0] 4126 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 4127 case -507075711: 4128 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 4129 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 4130 case -220463842: 4131 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 4132 case -14805197: 4133 /* affectsState */ return this.affectsState == null ? new Base[0] : new Base[] { this.affectsState }; // BooleanType 4134 case 3059181: 4135 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 4136 case 950398559: 4137 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // MarkdownType 4138 case 3016401: 4139 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // CanonicalType 4140 case -341064690: 4141 /* resource */ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CodeType 4142 case -887328209: 4143 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // BooleanType 4144 case 3575610: 4145 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // BooleanType 4146 case 555127957: 4147 /* instance */ return this.instance == null ? new Base[0] : new Base[] { this.instance }; // BooleanType 4148 case 676942463: 4149 /* inputProfile */ return this.inputProfile == null ? new Base[0] : new Base[] { this.inputProfile }; // CanonicalType 4150 case 1826166120: 4151 /* outputProfile */ return this.outputProfile == null ? new Base[0] : new Base[] { this.outputProfile }; // CanonicalType 4152 case 1954460585: 4153 /* parameter */ return this.parameter == null ? new Base[0] 4154 : this.parameter.toArray(new Base[this.parameter.size()]); // OperationDefinitionParameterComponent 4155 case 529823674: 4156 /* overload */ return this.overload == null ? new Base[0] : this.overload.toArray(new Base[this.overload.size()]); // OperationDefinitionOverloadComponent 4157 default: 4158 return super.getProperty(hash, name, checkValid); 4159 } 4160 4161 } 4162 4163 @Override 4164 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4165 switch (hash) { 4166 case 116079: // url 4167 this.url = castToUri(value); // UriType 4168 return value; 4169 case 351608024: // version 4170 this.version = castToString(value); // StringType 4171 return value; 4172 case 3373707: // name 4173 this.name = castToString(value); // StringType 4174 return value; 4175 case 110371416: // title 4176 this.title = castToString(value); // StringType 4177 return value; 4178 case -892481550: // status 4179 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4180 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4181 return value; 4182 case 3292052: // kind 4183 value = new OperationKindEnumFactory().fromType(castToCode(value)); 4184 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4185 return value; 4186 case -404562712: // experimental 4187 this.experimental = castToBoolean(value); // BooleanType 4188 return value; 4189 case 3076014: // date 4190 this.date = castToDateTime(value); // DateTimeType 4191 return value; 4192 case 1447404028: // publisher 4193 this.publisher = castToString(value); // StringType 4194 return value; 4195 case 951526432: // contact 4196 this.getContact().add(castToContactDetail(value)); // ContactDetail 4197 return value; 4198 case -1724546052: // description 4199 this.description = castToMarkdown(value); // MarkdownType 4200 return value; 4201 case -669707736: // useContext 4202 this.getUseContext().add(castToUsageContext(value)); // UsageContext 4203 return value; 4204 case -507075711: // jurisdiction 4205 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 4206 return value; 4207 case -220463842: // purpose 4208 this.purpose = castToMarkdown(value); // MarkdownType 4209 return value; 4210 case -14805197: // affectsState 4211 this.affectsState = castToBoolean(value); // BooleanType 4212 return value; 4213 case 3059181: // code 4214 this.code = castToCode(value); // CodeType 4215 return value; 4216 case 950398559: // comment 4217 this.comment = castToMarkdown(value); // MarkdownType 4218 return value; 4219 case 3016401: // base 4220 this.base = castToCanonical(value); // CanonicalType 4221 return value; 4222 case -341064690: // resource 4223 this.getResource().add(castToCode(value)); // CodeType 4224 return value; 4225 case -887328209: // system 4226 this.system = castToBoolean(value); // BooleanType 4227 return value; 4228 case 3575610: // type 4229 this.type = castToBoolean(value); // BooleanType 4230 return value; 4231 case 555127957: // instance 4232 this.instance = castToBoolean(value); // BooleanType 4233 return value; 4234 case 676942463: // inputProfile 4235 this.inputProfile = castToCanonical(value); // CanonicalType 4236 return value; 4237 case 1826166120: // outputProfile 4238 this.outputProfile = castToCanonical(value); // CanonicalType 4239 return value; 4240 case 1954460585: // parameter 4241 this.getParameter().add((OperationDefinitionParameterComponent) value); // OperationDefinitionParameterComponent 4242 return value; 4243 case 529823674: // overload 4244 this.getOverload().add((OperationDefinitionOverloadComponent) value); // OperationDefinitionOverloadComponent 4245 return value; 4246 default: 4247 return super.setProperty(hash, name, value); 4248 } 4249 4250 } 4251 4252 @Override 4253 public Base setProperty(String name, Base value) throws FHIRException { 4254 if (name.equals("url")) { 4255 this.url = castToUri(value); // UriType 4256 } else if (name.equals("version")) { 4257 this.version = castToString(value); // StringType 4258 } else if (name.equals("name")) { 4259 this.name = castToString(value); // StringType 4260 } else if (name.equals("title")) { 4261 this.title = castToString(value); // StringType 4262 } else if (name.equals("status")) { 4263 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4264 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4265 } else if (name.equals("kind")) { 4266 value = new OperationKindEnumFactory().fromType(castToCode(value)); 4267 this.kind = (Enumeration) value; // Enumeration<OperationKind> 4268 } else if (name.equals("experimental")) { 4269 this.experimental = castToBoolean(value); // BooleanType 4270 } else if (name.equals("date")) { 4271 this.date = castToDateTime(value); // DateTimeType 4272 } else if (name.equals("publisher")) { 4273 this.publisher = castToString(value); // StringType 4274 } else if (name.equals("contact")) { 4275 this.getContact().add(castToContactDetail(value)); 4276 } else if (name.equals("description")) { 4277 this.description = castToMarkdown(value); // MarkdownType 4278 } else if (name.equals("useContext")) { 4279 this.getUseContext().add(castToUsageContext(value)); 4280 } else if (name.equals("jurisdiction")) { 4281 this.getJurisdiction().add(castToCodeableConcept(value)); 4282 } else if (name.equals("purpose")) { 4283 this.purpose = castToMarkdown(value); // MarkdownType 4284 } else if (name.equals("affectsState")) { 4285 this.affectsState = castToBoolean(value); // BooleanType 4286 } else if (name.equals("code")) { 4287 this.code = castToCode(value); // CodeType 4288 } else if (name.equals("comment")) { 4289 this.comment = castToMarkdown(value); // MarkdownType 4290 } else if (name.equals("base")) { 4291 this.base = castToCanonical(value); // CanonicalType 4292 } else if (name.equals("resource")) { 4293 this.getResource().add(castToCode(value)); 4294 } else if (name.equals("system")) { 4295 this.system = castToBoolean(value); // BooleanType 4296 } else if (name.equals("type")) { 4297 this.type = castToBoolean(value); // BooleanType 4298 } else if (name.equals("instance")) { 4299 this.instance = castToBoolean(value); // BooleanType 4300 } else if (name.equals("inputProfile")) { 4301 this.inputProfile = castToCanonical(value); // CanonicalType 4302 } else if (name.equals("outputProfile")) { 4303 this.outputProfile = castToCanonical(value); // CanonicalType 4304 } else if (name.equals("parameter")) { 4305 this.getParameter().add((OperationDefinitionParameterComponent) value); 4306 } else if (name.equals("overload")) { 4307 this.getOverload().add((OperationDefinitionOverloadComponent) value); 4308 } else 4309 return super.setProperty(name, value); 4310 return value; 4311 } 4312 4313 @Override 4314 public Base makeProperty(int hash, String name) throws FHIRException { 4315 switch (hash) { 4316 case 116079: 4317 return getUrlElement(); 4318 case 351608024: 4319 return getVersionElement(); 4320 case 3373707: 4321 return getNameElement(); 4322 case 110371416: 4323 return getTitleElement(); 4324 case -892481550: 4325 return getStatusElement(); 4326 case 3292052: 4327 return getKindElement(); 4328 case -404562712: 4329 return getExperimentalElement(); 4330 case 3076014: 4331 return getDateElement(); 4332 case 1447404028: 4333 return getPublisherElement(); 4334 case 951526432: 4335 return addContact(); 4336 case -1724546052: 4337 return getDescriptionElement(); 4338 case -669707736: 4339 return addUseContext(); 4340 case -507075711: 4341 return addJurisdiction(); 4342 case -220463842: 4343 return getPurposeElement(); 4344 case -14805197: 4345 return getAffectsStateElement(); 4346 case 3059181: 4347 return getCodeElement(); 4348 case 950398559: 4349 return getCommentElement(); 4350 case 3016401: 4351 return getBaseElement(); 4352 case -341064690: 4353 return addResourceElement(); 4354 case -887328209: 4355 return getSystemElement(); 4356 case 3575610: 4357 return getTypeElement(); 4358 case 555127957: 4359 return getInstanceElement(); 4360 case 676942463: 4361 return getInputProfileElement(); 4362 case 1826166120: 4363 return getOutputProfileElement(); 4364 case 1954460585: 4365 return addParameter(); 4366 case 529823674: 4367 return addOverload(); 4368 default: 4369 return super.makeProperty(hash, name); 4370 } 4371 4372 } 4373 4374 @Override 4375 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4376 switch (hash) { 4377 case 116079: 4378 /* url */ return new String[] { "uri" }; 4379 case 351608024: 4380 /* version */ return new String[] { "string" }; 4381 case 3373707: 4382 /* name */ return new String[] { "string" }; 4383 case 110371416: 4384 /* title */ return new String[] { "string" }; 4385 case -892481550: 4386 /* status */ return new String[] { "code" }; 4387 case 3292052: 4388 /* kind */ return new String[] { "code" }; 4389 case -404562712: 4390 /* experimental */ return new String[] { "boolean" }; 4391 case 3076014: 4392 /* date */ return new String[] { "dateTime" }; 4393 case 1447404028: 4394 /* publisher */ return new String[] { "string" }; 4395 case 951526432: 4396 /* contact */ return new String[] { "ContactDetail" }; 4397 case -1724546052: 4398 /* description */ return new String[] { "markdown" }; 4399 case -669707736: 4400 /* useContext */ return new String[] { "UsageContext" }; 4401 case -507075711: 4402 /* jurisdiction */ return new String[] { "CodeableConcept" }; 4403 case -220463842: 4404 /* purpose */ return new String[] { "markdown" }; 4405 case -14805197: 4406 /* affectsState */ return new String[] { "boolean" }; 4407 case 3059181: 4408 /* code */ return new String[] { "code" }; 4409 case 950398559: 4410 /* comment */ return new String[] { "markdown" }; 4411 case 3016401: 4412 /* base */ return new String[] { "canonical" }; 4413 case -341064690: 4414 /* resource */ return new String[] { "code" }; 4415 case -887328209: 4416 /* system */ return new String[] { "boolean" }; 4417 case 3575610: 4418 /* type */ return new String[] { "boolean" }; 4419 case 555127957: 4420 /* instance */ return new String[] { "boolean" }; 4421 case 676942463: 4422 /* inputProfile */ return new String[] { "canonical" }; 4423 case 1826166120: 4424 /* outputProfile */ return new String[] { "canonical" }; 4425 case 1954460585: 4426 /* parameter */ return new String[] {}; 4427 case 529823674: 4428 /* overload */ return new String[] {}; 4429 default: 4430 return super.getTypesForProperty(hash, name); 4431 } 4432 4433 } 4434 4435 @Override 4436 public Base addChild(String name) throws FHIRException { 4437 if (name.equals("url")) { 4438 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.url"); 4439 } else if (name.equals("version")) { 4440 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.version"); 4441 } else if (name.equals("name")) { 4442 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.name"); 4443 } else if (name.equals("title")) { 4444 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.title"); 4445 } else if (name.equals("status")) { 4446 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.status"); 4447 } else if (name.equals("kind")) { 4448 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.kind"); 4449 } else if (name.equals("experimental")) { 4450 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.experimental"); 4451 } else if (name.equals("date")) { 4452 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.date"); 4453 } else if (name.equals("publisher")) { 4454 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.publisher"); 4455 } else if (name.equals("contact")) { 4456 return addContact(); 4457 } else if (name.equals("description")) { 4458 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.description"); 4459 } else if (name.equals("useContext")) { 4460 return addUseContext(); 4461 } else if (name.equals("jurisdiction")) { 4462 return addJurisdiction(); 4463 } else if (name.equals("purpose")) { 4464 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.purpose"); 4465 } else if (name.equals("affectsState")) { 4466 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.affectsState"); 4467 } else if (name.equals("code")) { 4468 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.code"); 4469 } else if (name.equals("comment")) { 4470 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.comment"); 4471 } else if (name.equals("base")) { 4472 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.base"); 4473 } else if (name.equals("resource")) { 4474 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.resource"); 4475 } else if (name.equals("system")) { 4476 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.system"); 4477 } else if (name.equals("type")) { 4478 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.type"); 4479 } else if (name.equals("instance")) { 4480 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.instance"); 4481 } else if (name.equals("inputProfile")) { 4482 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.inputProfile"); 4483 } else if (name.equals("outputProfile")) { 4484 throw new FHIRException("Cannot call addChild on a singleton property OperationDefinition.outputProfile"); 4485 } else if (name.equals("parameter")) { 4486 return addParameter(); 4487 } else if (name.equals("overload")) { 4488 return addOverload(); 4489 } else 4490 return super.addChild(name); 4491 } 4492 4493 public String fhirType() { 4494 return "OperationDefinition"; 4495 4496 } 4497 4498 public OperationDefinition copy() { 4499 OperationDefinition dst = new OperationDefinition(); 4500 copyValues(dst); 4501 return dst; 4502 } 4503 4504 public void copyValues(OperationDefinition dst) { 4505 super.copyValues(dst); 4506 dst.url = url == null ? null : url.copy(); 4507 dst.version = version == null ? null : version.copy(); 4508 dst.name = name == null ? null : name.copy(); 4509 dst.title = title == null ? null : title.copy(); 4510 dst.status = status == null ? null : status.copy(); 4511 dst.kind = kind == null ? null : kind.copy(); 4512 dst.experimental = experimental == null ? null : experimental.copy(); 4513 dst.date = date == null ? null : date.copy(); 4514 dst.publisher = publisher == null ? null : publisher.copy(); 4515 if (contact != null) { 4516 dst.contact = new ArrayList<ContactDetail>(); 4517 for (ContactDetail i : contact) 4518 dst.contact.add(i.copy()); 4519 } 4520 ; 4521 dst.description = description == null ? null : description.copy(); 4522 if (useContext != null) { 4523 dst.useContext = new ArrayList<UsageContext>(); 4524 for (UsageContext i : useContext) 4525 dst.useContext.add(i.copy()); 4526 } 4527 ; 4528 if (jurisdiction != null) { 4529 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4530 for (CodeableConcept i : jurisdiction) 4531 dst.jurisdiction.add(i.copy()); 4532 } 4533 ; 4534 dst.purpose = purpose == null ? null : purpose.copy(); 4535 dst.affectsState = affectsState == null ? null : affectsState.copy(); 4536 dst.code = code == null ? null : code.copy(); 4537 dst.comment = comment == null ? null : comment.copy(); 4538 dst.base = base == null ? null : base.copy(); 4539 if (resource != null) { 4540 dst.resource = new ArrayList<CodeType>(); 4541 for (CodeType i : resource) 4542 dst.resource.add(i.copy()); 4543 } 4544 ; 4545 dst.system = system == null ? null : system.copy(); 4546 dst.type = type == null ? null : type.copy(); 4547 dst.instance = instance == null ? null : instance.copy(); 4548 dst.inputProfile = inputProfile == null ? null : inputProfile.copy(); 4549 dst.outputProfile = outputProfile == null ? null : outputProfile.copy(); 4550 if (parameter != null) { 4551 dst.parameter = new ArrayList<OperationDefinitionParameterComponent>(); 4552 for (OperationDefinitionParameterComponent i : parameter) 4553 dst.parameter.add(i.copy()); 4554 } 4555 ; 4556 if (overload != null) { 4557 dst.overload = new ArrayList<OperationDefinitionOverloadComponent>(); 4558 for (OperationDefinitionOverloadComponent i : overload) 4559 dst.overload.add(i.copy()); 4560 } 4561 ; 4562 } 4563 4564 protected OperationDefinition typedCopy() { 4565 return copy(); 4566 } 4567 4568 @Override 4569 public boolean equalsDeep(Base other_) { 4570 if (!super.equalsDeep(other_)) 4571 return false; 4572 if (!(other_ instanceof OperationDefinition)) 4573 return false; 4574 OperationDefinition o = (OperationDefinition) other_; 4575 return compareDeep(kind, o.kind, true) && compareDeep(purpose, o.purpose, true) 4576 && compareDeep(affectsState, o.affectsState, true) && compareDeep(code, o.code, true) 4577 && compareDeep(comment, o.comment, true) && compareDeep(base, o.base, true) 4578 && compareDeep(resource, o.resource, true) && compareDeep(system, o.system, true) 4579 && compareDeep(type, o.type, true) && compareDeep(instance, o.instance, true) 4580 && compareDeep(inputProfile, o.inputProfile, true) && compareDeep(outputProfile, o.outputProfile, true) 4581 && compareDeep(parameter, o.parameter, true) && compareDeep(overload, o.overload, true); 4582 } 4583 4584 @Override 4585 public boolean equalsShallow(Base other_) { 4586 if (!super.equalsShallow(other_)) 4587 return false; 4588 if (!(other_ instanceof OperationDefinition)) 4589 return false; 4590 OperationDefinition o = (OperationDefinition) other_; 4591 return compareValues(kind, o.kind, true) && compareValues(purpose, o.purpose, true) 4592 && compareValues(affectsState, o.affectsState, true) && compareValues(code, o.code, true) 4593 && compareValues(comment, o.comment, true) && compareValues(resource, o.resource, true) 4594 && compareValues(system, o.system, true) && compareValues(type, o.type, true) 4595 && compareValues(instance, o.instance, true); 4596 } 4597 4598 public boolean isEmpty() { 4599 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, purpose, affectsState, code, comment, base, 4600 resource, system, type, instance, inputProfile, outputProfile, parameter, overload); 4601 } 4602 4603 @Override 4604 public ResourceType getResourceType() { 4605 return ResourceType.OperationDefinition; 4606 } 4607 4608 /** 4609 * Search parameter: <b>date</b> 4610 * <p> 4611 * Description: <b>The operation definition publication date</b><br> 4612 * Type: <b>date</b><br> 4613 * Path: <b>OperationDefinition.date</b><br> 4614 * </p> 4615 */ 4616 @SearchParamDefinition(name = "date", path = "OperationDefinition.date", description = "The operation definition publication date", type = "date") 4617 public static final String SP_DATE = "date"; 4618 /** 4619 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4620 * <p> 4621 * Description: <b>The operation definition publication date</b><br> 4622 * Type: <b>date</b><br> 4623 * Path: <b>OperationDefinition.date</b><br> 4624 * </p> 4625 */ 4626 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4627 SP_DATE); 4628 4629 /** 4630 * Search parameter: <b>code</b> 4631 * <p> 4632 * Description: <b>Name used to invoke the operation</b><br> 4633 * Type: <b>token</b><br> 4634 * Path: <b>OperationDefinition.code</b><br> 4635 * </p> 4636 */ 4637 @SearchParamDefinition(name = "code", path = "OperationDefinition.code", description = "Name used to invoke the operation", type = "token") 4638 public static final String SP_CODE = "code"; 4639 /** 4640 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4641 * <p> 4642 * Description: <b>Name used to invoke the operation</b><br> 4643 * Type: <b>token</b><br> 4644 * Path: <b>OperationDefinition.code</b><br> 4645 * </p> 4646 */ 4647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4648 SP_CODE); 4649 4650 /** 4651 * Search parameter: <b>instance</b> 4652 * <p> 4653 * Description: <b>Invoke on an instance?</b><br> 4654 * Type: <b>token</b><br> 4655 * Path: <b>OperationDefinition.instance</b><br> 4656 * </p> 4657 */ 4658 @SearchParamDefinition(name = "instance", path = "OperationDefinition.instance", description = "Invoke on an instance?", type = "token") 4659 public static final String SP_INSTANCE = "instance"; 4660 /** 4661 * <b>Fluent Client</b> search parameter constant for <b>instance</b> 4662 * <p> 4663 * Description: <b>Invoke on an instance?</b><br> 4664 * Type: <b>token</b><br> 4665 * Path: <b>OperationDefinition.instance</b><br> 4666 * </p> 4667 */ 4668 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INSTANCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4669 SP_INSTANCE); 4670 4671 /** 4672 * Search parameter: <b>context-type-value</b> 4673 * <p> 4674 * Description: <b>A use context type and value assigned to the operation 4675 * definition</b><br> 4676 * Type: <b>composite</b><br> 4677 * Path: <b></b><br> 4678 * </p> 4679 */ 4680 @SearchParamDefinition(name = "context-type-value", path = "OperationDefinition.useContext", description = "A use context type and value assigned to the operation definition", type = "composite", compositeOf = { 4681 "context-type", "context" }) 4682 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 4683 /** 4684 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 4685 * <p> 4686 * Description: <b>A use context type and value assigned to the operation 4687 * definition</b><br> 4688 * Type: <b>composite</b><br> 4689 * Path: <b></b><br> 4690 * </p> 4691 */ 4692 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4693 SP_CONTEXT_TYPE_VALUE); 4694 4695 /** 4696 * Search parameter: <b>kind</b> 4697 * <p> 4698 * Description: <b>operation | query</b><br> 4699 * Type: <b>token</b><br> 4700 * Path: <b>OperationDefinition.kind</b><br> 4701 * </p> 4702 */ 4703 @SearchParamDefinition(name = "kind", path = "OperationDefinition.kind", description = "operation | query", type = "token") 4704 public static final String SP_KIND = "kind"; 4705 /** 4706 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 4707 * <p> 4708 * Description: <b>operation | query</b><br> 4709 * Type: <b>token</b><br> 4710 * Path: <b>OperationDefinition.kind</b><br> 4711 * </p> 4712 */ 4713 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4714 SP_KIND); 4715 4716 /** 4717 * Search parameter: <b>jurisdiction</b> 4718 * <p> 4719 * Description: <b>Intended jurisdiction for the operation definition</b><br> 4720 * Type: <b>token</b><br> 4721 * Path: <b>OperationDefinition.jurisdiction</b><br> 4722 * </p> 4723 */ 4724 @SearchParamDefinition(name = "jurisdiction", path = "OperationDefinition.jurisdiction", description = "Intended jurisdiction for the operation definition", type = "token") 4725 public static final String SP_JURISDICTION = "jurisdiction"; 4726 /** 4727 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4728 * <p> 4729 * Description: <b>Intended jurisdiction for the operation definition</b><br> 4730 * Type: <b>token</b><br> 4731 * Path: <b>OperationDefinition.jurisdiction</b><br> 4732 * </p> 4733 */ 4734 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4735 SP_JURISDICTION); 4736 4737 /** 4738 * Search parameter: <b>description</b> 4739 * <p> 4740 * Description: <b>The description of the operation definition</b><br> 4741 * Type: <b>string</b><br> 4742 * Path: <b>OperationDefinition.description</b><br> 4743 * </p> 4744 */ 4745 @SearchParamDefinition(name = "description", path = "OperationDefinition.description", description = "The description of the operation definition", type = "string") 4746 public static final String SP_DESCRIPTION = "description"; 4747 /** 4748 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4749 * <p> 4750 * Description: <b>The description of the operation definition</b><br> 4751 * Type: <b>string</b><br> 4752 * Path: <b>OperationDefinition.description</b><br> 4753 * </p> 4754 */ 4755 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4756 SP_DESCRIPTION); 4757 4758 /** 4759 * Search parameter: <b>context-type</b> 4760 * <p> 4761 * Description: <b>A type of use context assigned to the operation 4762 * definition</b><br> 4763 * Type: <b>token</b><br> 4764 * Path: <b>OperationDefinition.useContext.code</b><br> 4765 * </p> 4766 */ 4767 @SearchParamDefinition(name = "context-type", path = "OperationDefinition.useContext.code", description = "A type of use context assigned to the operation definition", type = "token") 4768 public static final String SP_CONTEXT_TYPE = "context-type"; 4769 /** 4770 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4771 * <p> 4772 * Description: <b>A type of use context assigned to the operation 4773 * definition</b><br> 4774 * Type: <b>token</b><br> 4775 * Path: <b>OperationDefinition.useContext.code</b><br> 4776 * </p> 4777 */ 4778 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4779 SP_CONTEXT_TYPE); 4780 4781 /** 4782 * Search parameter: <b>title</b> 4783 * <p> 4784 * Description: <b>The human-friendly name of the operation definition</b><br> 4785 * Type: <b>string</b><br> 4786 * Path: <b>OperationDefinition.title</b><br> 4787 * </p> 4788 */ 4789 @SearchParamDefinition(name = "title", path = "OperationDefinition.title", description = "The human-friendly name of the operation definition", type = "string") 4790 public static final String SP_TITLE = "title"; 4791 /** 4792 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4793 * <p> 4794 * Description: <b>The human-friendly name of the operation definition</b><br> 4795 * Type: <b>string</b><br> 4796 * Path: <b>OperationDefinition.title</b><br> 4797 * </p> 4798 */ 4799 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4800 SP_TITLE); 4801 4802 /** 4803 * Search parameter: <b>type</b> 4804 * <p> 4805 * Description: <b>Invoke at the type level?</b><br> 4806 * Type: <b>token</b><br> 4807 * Path: <b>OperationDefinition.type</b><br> 4808 * </p> 4809 */ 4810 @SearchParamDefinition(name = "type", path = "OperationDefinition.type", description = "Invoke at the type level?", type = "token") 4811 public static final String SP_TYPE = "type"; 4812 /** 4813 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4814 * <p> 4815 * Description: <b>Invoke at the type level?</b><br> 4816 * Type: <b>token</b><br> 4817 * Path: <b>OperationDefinition.type</b><br> 4818 * </p> 4819 */ 4820 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4821 SP_TYPE); 4822 4823 /** 4824 * Search parameter: <b>version</b> 4825 * <p> 4826 * Description: <b>The business version of the operation definition</b><br> 4827 * Type: <b>token</b><br> 4828 * Path: <b>OperationDefinition.version</b><br> 4829 * </p> 4830 */ 4831 @SearchParamDefinition(name = "version", path = "OperationDefinition.version", description = "The business version of the operation definition", type = "token") 4832 public static final String SP_VERSION = "version"; 4833 /** 4834 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4835 * <p> 4836 * Description: <b>The business version of the operation definition</b><br> 4837 * Type: <b>token</b><br> 4838 * Path: <b>OperationDefinition.version</b><br> 4839 * </p> 4840 */ 4841 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4842 SP_VERSION); 4843 4844 /** 4845 * Search parameter: <b>url</b> 4846 * <p> 4847 * Description: <b>The uri that identifies the operation definition</b><br> 4848 * Type: <b>uri</b><br> 4849 * Path: <b>OperationDefinition.url</b><br> 4850 * </p> 4851 */ 4852 @SearchParamDefinition(name = "url", path = "OperationDefinition.url", description = "The uri that identifies the operation definition", type = "uri") 4853 public static final String SP_URL = "url"; 4854 /** 4855 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4856 * <p> 4857 * Description: <b>The uri that identifies the operation definition</b><br> 4858 * Type: <b>uri</b><br> 4859 * Path: <b>OperationDefinition.url</b><br> 4860 * </p> 4861 */ 4862 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4863 4864 /** 4865 * Search parameter: <b>context-quantity</b> 4866 * <p> 4867 * Description: <b>A quantity- or range-valued use context assigned to the 4868 * operation definition</b><br> 4869 * Type: <b>quantity</b><br> 4870 * Path: <b>OperationDefinition.useContext.valueQuantity, 4871 * OperationDefinition.useContext.valueRange</b><br> 4872 * </p> 4873 */ 4874 @SearchParamDefinition(name = "context-quantity", path = "(OperationDefinition.useContext.value as Quantity) | (OperationDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the operation definition", type = "quantity") 4875 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4876 /** 4877 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4878 * <p> 4879 * Description: <b>A quantity- or range-valued use context assigned to the 4880 * operation definition</b><br> 4881 * Type: <b>quantity</b><br> 4882 * Path: <b>OperationDefinition.useContext.valueQuantity, 4883 * OperationDefinition.useContext.valueRange</b><br> 4884 * </p> 4885 */ 4886 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4887 SP_CONTEXT_QUANTITY); 4888 4889 /** 4890 * Search parameter: <b>input-profile</b> 4891 * <p> 4892 * Description: <b>Validation information for in parameters</b><br> 4893 * Type: <b>reference</b><br> 4894 * Path: <b>OperationDefinition.inputProfile</b><br> 4895 * </p> 4896 */ 4897 @SearchParamDefinition(name = "input-profile", path = "OperationDefinition.inputProfile", description = "Validation information for in parameters", type = "reference", target = { 4898 StructureDefinition.class }) 4899 public static final String SP_INPUT_PROFILE = "input-profile"; 4900 /** 4901 * <b>Fluent Client</b> search parameter constant for <b>input-profile</b> 4902 * <p> 4903 * Description: <b>Validation information for in parameters</b><br> 4904 * Type: <b>reference</b><br> 4905 * Path: <b>OperationDefinition.inputProfile</b><br> 4906 * </p> 4907 */ 4908 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INPUT_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4909 SP_INPUT_PROFILE); 4910 4911 /** 4912 * Constant for fluent queries to be used to add include statements. Specifies 4913 * the path value of "<b>OperationDefinition:input-profile</b>". 4914 */ 4915 public static final ca.uhn.fhir.model.api.Include INCLUDE_INPUT_PROFILE = new ca.uhn.fhir.model.api.Include( 4916 "OperationDefinition:input-profile").toLocked(); 4917 4918 /** 4919 * Search parameter: <b>output-profile</b> 4920 * <p> 4921 * Description: <b>Validation information for out parameters</b><br> 4922 * Type: <b>reference</b><br> 4923 * Path: <b>OperationDefinition.outputProfile</b><br> 4924 * </p> 4925 */ 4926 @SearchParamDefinition(name = "output-profile", path = "OperationDefinition.outputProfile", description = "Validation information for out parameters", type = "reference", target = { 4927 StructureDefinition.class }) 4928 public static final String SP_OUTPUT_PROFILE = "output-profile"; 4929 /** 4930 * <b>Fluent Client</b> search parameter constant for <b>output-profile</b> 4931 * <p> 4932 * Description: <b>Validation information for out parameters</b><br> 4933 * Type: <b>reference</b><br> 4934 * Path: <b>OperationDefinition.outputProfile</b><br> 4935 * </p> 4936 */ 4937 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OUTPUT_PROFILE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4938 SP_OUTPUT_PROFILE); 4939 4940 /** 4941 * Constant for fluent queries to be used to add include statements. Specifies 4942 * the path value of "<b>OperationDefinition:output-profile</b>". 4943 */ 4944 public static final ca.uhn.fhir.model.api.Include INCLUDE_OUTPUT_PROFILE = new ca.uhn.fhir.model.api.Include( 4945 "OperationDefinition:output-profile").toLocked(); 4946 4947 /** 4948 * Search parameter: <b>system</b> 4949 * <p> 4950 * Description: <b>Invoke at the system level?</b><br> 4951 * Type: <b>token</b><br> 4952 * Path: <b>OperationDefinition.system</b><br> 4953 * </p> 4954 */ 4955 @SearchParamDefinition(name = "system", path = "OperationDefinition.system", description = "Invoke at the system level?", type = "token") 4956 public static final String SP_SYSTEM = "system"; 4957 /** 4958 * <b>Fluent Client</b> search parameter constant for <b>system</b> 4959 * <p> 4960 * Description: <b>Invoke at the system level?</b><br> 4961 * Type: <b>token</b><br> 4962 * Path: <b>OperationDefinition.system</b><br> 4963 * </p> 4964 */ 4965 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SYSTEM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4966 SP_SYSTEM); 4967 4968 /** 4969 * Search parameter: <b>name</b> 4970 * <p> 4971 * Description: <b>Computationally friendly name of the operation 4972 * definition</b><br> 4973 * Type: <b>string</b><br> 4974 * Path: <b>OperationDefinition.name</b><br> 4975 * </p> 4976 */ 4977 @SearchParamDefinition(name = "name", path = "OperationDefinition.name", description = "Computationally friendly name of the operation definition", type = "string") 4978 public static final String SP_NAME = "name"; 4979 /** 4980 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4981 * <p> 4982 * Description: <b>Computationally friendly name of the operation 4983 * definition</b><br> 4984 * Type: <b>string</b><br> 4985 * Path: <b>OperationDefinition.name</b><br> 4986 * </p> 4987 */ 4988 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4989 SP_NAME); 4990 4991 /** 4992 * Search parameter: <b>context</b> 4993 * <p> 4994 * Description: <b>A use context assigned to the operation definition</b><br> 4995 * Type: <b>token</b><br> 4996 * Path: <b>OperationDefinition.useContext.valueCodeableConcept</b><br> 4997 * </p> 4998 */ 4999 @SearchParamDefinition(name = "context", path = "(OperationDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the operation definition", type = "token") 5000 public static final String SP_CONTEXT = "context"; 5001 /** 5002 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5003 * <p> 5004 * Description: <b>A use context assigned to the operation definition</b><br> 5005 * Type: <b>token</b><br> 5006 * Path: <b>OperationDefinition.useContext.valueCodeableConcept</b><br> 5007 * </p> 5008 */ 5009 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5010 SP_CONTEXT); 5011 5012 /** 5013 * Search parameter: <b>publisher</b> 5014 * <p> 5015 * Description: <b>Name of the publisher of the operation definition</b><br> 5016 * Type: <b>string</b><br> 5017 * Path: <b>OperationDefinition.publisher</b><br> 5018 * </p> 5019 */ 5020 @SearchParamDefinition(name = "publisher", path = "OperationDefinition.publisher", description = "Name of the publisher of the operation definition", type = "string") 5021 public static final String SP_PUBLISHER = "publisher"; 5022 /** 5023 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 5024 * <p> 5025 * Description: <b>Name of the publisher of the operation definition</b><br> 5026 * Type: <b>string</b><br> 5027 * Path: <b>OperationDefinition.publisher</b><br> 5028 * </p> 5029 */ 5030 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 5031 SP_PUBLISHER); 5032 5033 /** 5034 * Search parameter: <b>context-type-quantity</b> 5035 * <p> 5036 * Description: <b>A use context type and quantity- or range-based value 5037 * assigned to the operation definition</b><br> 5038 * Type: <b>composite</b><br> 5039 * Path: <b></b><br> 5040 * </p> 5041 */ 5042 @SearchParamDefinition(name = "context-type-quantity", path = "OperationDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the operation definition", type = "composite", compositeOf = { 5043 "context-type", "context-quantity" }) 5044 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5045 /** 5046 * <b>Fluent Client</b> search parameter constant for 5047 * <b>context-type-quantity</b> 5048 * <p> 5049 * Description: <b>A use context type and quantity- or range-based value 5050 * assigned to the operation definition</b><br> 5051 * Type: <b>composite</b><br> 5052 * Path: <b></b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 5056 SP_CONTEXT_TYPE_QUANTITY); 5057 5058 /** 5059 * Search parameter: <b>status</b> 5060 * <p> 5061 * Description: <b>The current status of the operation definition</b><br> 5062 * Type: <b>token</b><br> 5063 * Path: <b>OperationDefinition.status</b><br> 5064 * </p> 5065 */ 5066 @SearchParamDefinition(name = "status", path = "OperationDefinition.status", description = "The current status of the operation definition", type = "token") 5067 public static final String SP_STATUS = "status"; 5068 /** 5069 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5070 * <p> 5071 * Description: <b>The current status of the operation definition</b><br> 5072 * Type: <b>token</b><br> 5073 * Path: <b>OperationDefinition.status</b><br> 5074 * </p> 5075 */ 5076 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5077 SP_STATUS); 5078 5079 /** 5080 * Search parameter: <b>base</b> 5081 * <p> 5082 * Description: <b>Marks this as a profile of the base</b><br> 5083 * Type: <b>reference</b><br> 5084 * Path: <b>OperationDefinition.base</b><br> 5085 * </p> 5086 */ 5087 @SearchParamDefinition(name = "base", path = "OperationDefinition.base", description = "Marks this as a profile of the base", type = "reference", target = { 5088 OperationDefinition.class }) 5089 public static final String SP_BASE = "base"; 5090 /** 5091 * <b>Fluent Client</b> search parameter constant for <b>base</b> 5092 * <p> 5093 * Description: <b>Marks this as a profile of the base</b><br> 5094 * Type: <b>reference</b><br> 5095 * Path: <b>OperationDefinition.base</b><br> 5096 * </p> 5097 */ 5098 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5099 SP_BASE); 5100 5101 /** 5102 * Constant for fluent queries to be used to add include statements. Specifies 5103 * the path value of "<b>OperationDefinition:base</b>". 5104 */ 5105 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASE = new ca.uhn.fhir.model.api.Include( 5106 "OperationDefinition:base").toLocked(); 5107 5108}