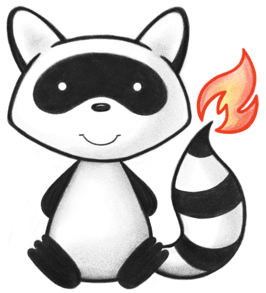
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * The parameters to the module. This collection specifies both the input and 045 * output parameters. Input parameters are provided by the caller as part of the 046 * $evaluate operation. Output parameters are included in the GuidanceResponse. 047 */ 048@DatatypeDef(name = "ParameterDefinition") 049public class ParameterDefinition extends Type implements ICompositeType { 050 051 public enum ParameterUse { 052 /** 053 * This is an input parameter. 054 */ 055 IN, 056 /** 057 * This is an output parameter. 058 */ 059 OUT, 060 /** 061 * added to help the parsers with the generic types 062 */ 063 NULL; 064 065 public static ParameterUse fromCode(String codeString) throws FHIRException { 066 if (codeString == null || "".equals(codeString)) 067 return null; 068 if ("in".equals(codeString)) 069 return IN; 070 if ("out".equals(codeString)) 071 return OUT; 072 if (Configuration.isAcceptInvalidEnums()) 073 return null; 074 else 075 throw new FHIRException("Unknown ParameterUse code '" + codeString + "'"); 076 } 077 078 public String toCode() { 079 switch (this) { 080 case IN: 081 return "in"; 082 case OUT: 083 return "out"; 084 case NULL: 085 return null; 086 default: 087 return "?"; 088 } 089 } 090 091 public String getSystem() { 092 switch (this) { 093 case IN: 094 return "http://hl7.org/fhir/operation-parameter-use"; 095 case OUT: 096 return "http://hl7.org/fhir/operation-parameter-use"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getDefinition() { 105 switch (this) { 106 case IN: 107 return "This is an input parameter."; 108 case OUT: 109 return "This is an output parameter."; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDisplay() { 118 switch (this) { 119 case IN: 120 return "In"; 121 case OUT: 122 return "Out"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 } 130 131 public static class ParameterUseEnumFactory implements EnumFactory<ParameterUse> { 132 public ParameterUse fromCode(String codeString) throws IllegalArgumentException { 133 if (codeString == null || "".equals(codeString)) 134 if (codeString == null || "".equals(codeString)) 135 return null; 136 if ("in".equals(codeString)) 137 return ParameterUse.IN; 138 if ("out".equals(codeString)) 139 return ParameterUse.OUT; 140 throw new IllegalArgumentException("Unknown ParameterUse code '" + codeString + "'"); 141 } 142 143 public Enumeration<ParameterUse> fromType(PrimitiveType<?> code) throws FHIRException { 144 if (code == null) 145 return null; 146 if (code.isEmpty()) 147 return new Enumeration<ParameterUse>(this, ParameterUse.NULL, code); 148 String codeString = code.asStringValue(); 149 if (codeString == null || "".equals(codeString)) 150 return new Enumeration<ParameterUse>(this, ParameterUse.NULL, code); 151 if ("in".equals(codeString)) 152 return new Enumeration<ParameterUse>(this, ParameterUse.IN, code); 153 if ("out".equals(codeString)) 154 return new Enumeration<ParameterUse>(this, ParameterUse.OUT, code); 155 throw new FHIRException("Unknown ParameterUse code '" + codeString + "'"); 156 } 157 158 public String toCode(ParameterUse code) { 159 if (code == ParameterUse.NULL) 160 return null; 161 if (code == ParameterUse.IN) 162 return "in"; 163 if (code == ParameterUse.OUT) 164 return "out"; 165 return "?"; 166 } 167 168 public String toSystem(ParameterUse code) { 169 return code.getSystem(); 170 } 171 } 172 173 /** 174 * The name of the parameter used to allow access to the value of the parameter 175 * in evaluation contexts. 176 */ 177 @Child(name = "name", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 178 @Description(shortDefinition = "Name used to access the parameter value", formalDefinition = "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.") 179 protected CodeType name; 180 181 /** 182 * Whether the parameter is input or output for the module. 183 */ 184 @Child(name = "use", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 185 @Description(shortDefinition = "in | out", formalDefinition = "Whether the parameter is input or output for the module.") 186 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/operation-parameter-use") 187 protected Enumeration<ParameterUse> use; 188 189 /** 190 * The minimum number of times this parameter SHALL appear in the request or 191 * response. 192 */ 193 @Child(name = "min", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 194 @Description(shortDefinition = "Minimum cardinality", formalDefinition = "The minimum number of times this parameter SHALL appear in the request or response.") 195 protected IntegerType min; 196 197 /** 198 * The maximum number of times this element is permitted to appear in the 199 * request or response. 200 */ 201 @Child(name = "max", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 202 @Description(shortDefinition = "Maximum cardinality (a number of *)", formalDefinition = "The maximum number of times this element is permitted to appear in the request or response.") 203 protected StringType max; 204 205 /** 206 * A brief discussion of what the parameter is for and how it is used by the 207 * module. 208 */ 209 @Child(name = "documentation", type = { 210 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 211 @Description(shortDefinition = "A brief description of the parameter", formalDefinition = "A brief discussion of what the parameter is for and how it is used by the module.") 212 protected StringType documentation; 213 214 /** 215 * The type of the parameter. 216 */ 217 @Child(name = "type", type = { CodeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 218 @Description(shortDefinition = "What type of value", formalDefinition = "The type of the parameter.") 219 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/all-types") 220 protected CodeType type; 221 222 /** 223 * If specified, this indicates a profile that the input data must conform to, 224 * or that the output data will conform to. 225 */ 226 @Child(name = "profile", type = { 227 CanonicalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 228 @Description(shortDefinition = "What profile the value is expected to be", formalDefinition = "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.") 229 protected CanonicalType profile; 230 231 private static final long serialVersionUID = -1891730734L; 232 233 /** 234 * Constructor 235 */ 236 public ParameterDefinition() { 237 super(); 238 } 239 240 /** 241 * Constructor 242 */ 243 public ParameterDefinition(Enumeration<ParameterUse> use, CodeType type) { 244 super(); 245 this.use = use; 246 this.type = type; 247 } 248 249 /** 250 * @return {@link #name} (The name of the parameter used to allow access to the 251 * value of the parameter in evaluation contexts.). This is the 252 * underlying object with id, value and extensions. The accessor 253 * "getName" gives direct access to the value 254 */ 255 public CodeType getNameElement() { 256 if (this.name == null) 257 if (Configuration.errorOnAutoCreate()) 258 throw new Error("Attempt to auto-create ParameterDefinition.name"); 259 else if (Configuration.doAutoCreate()) 260 this.name = new CodeType(); // bb 261 return this.name; 262 } 263 264 public boolean hasNameElement() { 265 return this.name != null && !this.name.isEmpty(); 266 } 267 268 public boolean hasName() { 269 return this.name != null && !this.name.isEmpty(); 270 } 271 272 /** 273 * @param value {@link #name} (The name of the parameter used to allow access to 274 * the value of the parameter in evaluation contexts.). This is the 275 * underlying object with id, value and extensions. The accessor 276 * "getName" gives direct access to the value 277 */ 278 public ParameterDefinition setNameElement(CodeType value) { 279 this.name = value; 280 return this; 281 } 282 283 /** 284 * @return The name of the parameter used to allow access to the value of the 285 * parameter in evaluation contexts. 286 */ 287 public String getName() { 288 return this.name == null ? null : this.name.getValue(); 289 } 290 291 /** 292 * @param value The name of the parameter used to allow access to the value of 293 * the parameter in evaluation contexts. 294 */ 295 public ParameterDefinition setName(String value) { 296 if (Utilities.noString(value)) 297 this.name = null; 298 else { 299 if (this.name == null) 300 this.name = new CodeType(); 301 this.name.setValue(value); 302 } 303 return this; 304 } 305 306 /** 307 * @return {@link #use} (Whether the parameter is input or output for the 308 * module.). This is the underlying object with id, value and 309 * extensions. The accessor "getUse" gives direct access to the value 310 */ 311 public Enumeration<ParameterUse> getUseElement() { 312 if (this.use == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create ParameterDefinition.use"); 315 else if (Configuration.doAutoCreate()) 316 this.use = new Enumeration<ParameterUse>(new ParameterUseEnumFactory()); // bb 317 return this.use; 318 } 319 320 public boolean hasUseElement() { 321 return this.use != null && !this.use.isEmpty(); 322 } 323 324 public boolean hasUse() { 325 return this.use != null && !this.use.isEmpty(); 326 } 327 328 /** 329 * @param value {@link #use} (Whether the parameter is input or output for the 330 * module.). This is the underlying object with id, value and 331 * extensions. The accessor "getUse" gives direct access to the 332 * value 333 */ 334 public ParameterDefinition setUseElement(Enumeration<ParameterUse> value) { 335 this.use = value; 336 return this; 337 } 338 339 /** 340 * @return Whether the parameter is input or output for the module. 341 */ 342 public ParameterUse getUse() { 343 return this.use == null ? null : this.use.getValue(); 344 } 345 346 /** 347 * @param value Whether the parameter is input or output for the module. 348 */ 349 public ParameterDefinition setUse(ParameterUse value) { 350 if (this.use == null) 351 this.use = new Enumeration<ParameterUse>(new ParameterUseEnumFactory()); 352 this.use.setValue(value); 353 return this; 354 } 355 356 /** 357 * @return {@link #min} (The minimum number of times this parameter SHALL appear 358 * in the request or response.). This is the underlying object with id, 359 * value and extensions. The accessor "getMin" gives direct access to 360 * the value 361 */ 362 public IntegerType getMinElement() { 363 if (this.min == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create ParameterDefinition.min"); 366 else if (Configuration.doAutoCreate()) 367 this.min = new IntegerType(); // bb 368 return this.min; 369 } 370 371 public boolean hasMinElement() { 372 return this.min != null && !this.min.isEmpty(); 373 } 374 375 public boolean hasMin() { 376 return this.min != null && !this.min.isEmpty(); 377 } 378 379 /** 380 * @param value {@link #min} (The minimum number of times this parameter SHALL 381 * appear in the request or response.). This is the underlying 382 * object with id, value and extensions. The accessor "getMin" 383 * gives direct access to the value 384 */ 385 public ParameterDefinition setMinElement(IntegerType value) { 386 this.min = value; 387 return this; 388 } 389 390 /** 391 * @return The minimum number of times this parameter SHALL appear in the 392 * request or response. 393 */ 394 public int getMin() { 395 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 396 } 397 398 /** 399 * @param value The minimum number of times this parameter SHALL appear in the 400 * request or response. 401 */ 402 public ParameterDefinition setMin(int value) { 403 if (this.min == null) 404 this.min = new IntegerType(); 405 this.min.setValue(value); 406 return this; 407 } 408 409 /** 410 * @return {@link #max} (The maximum number of times this element is permitted 411 * to appear in the request or response.). This is the underlying object 412 * with id, value and extensions. The accessor "getMax" gives direct 413 * access to the value 414 */ 415 public StringType getMaxElement() { 416 if (this.max == null) 417 if (Configuration.errorOnAutoCreate()) 418 throw new Error("Attempt to auto-create ParameterDefinition.max"); 419 else if (Configuration.doAutoCreate()) 420 this.max = new StringType(); // bb 421 return this.max; 422 } 423 424 public boolean hasMaxElement() { 425 return this.max != null && !this.max.isEmpty(); 426 } 427 428 public boolean hasMax() { 429 return this.max != null && !this.max.isEmpty(); 430 } 431 432 /** 433 * @param value {@link #max} (The maximum number of times this element is 434 * permitted to appear in the request or response.). This is the 435 * underlying object with id, value and extensions. The accessor 436 * "getMax" gives direct access to the value 437 */ 438 public ParameterDefinition setMaxElement(StringType value) { 439 this.max = value; 440 return this; 441 } 442 443 /** 444 * @return The maximum number of times this element is permitted to appear in 445 * the request or response. 446 */ 447 public String getMax() { 448 return this.max == null ? null : this.max.getValue(); 449 } 450 451 /** 452 * @param value The maximum number of times this element is permitted to appear 453 * in the request or response. 454 */ 455 public ParameterDefinition setMax(String value) { 456 if (Utilities.noString(value)) 457 this.max = null; 458 else { 459 if (this.max == null) 460 this.max = new StringType(); 461 this.max.setValue(value); 462 } 463 return this; 464 } 465 466 /** 467 * @return {@link #documentation} (A brief discussion of what the parameter is 468 * for and how it is used by the module.). This is the underlying object 469 * with id, value and extensions. The accessor "getDocumentation" gives 470 * direct access to the value 471 */ 472 public StringType getDocumentationElement() { 473 if (this.documentation == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create ParameterDefinition.documentation"); 476 else if (Configuration.doAutoCreate()) 477 this.documentation = new StringType(); // bb 478 return this.documentation; 479 } 480 481 public boolean hasDocumentationElement() { 482 return this.documentation != null && !this.documentation.isEmpty(); 483 } 484 485 public boolean hasDocumentation() { 486 return this.documentation != null && !this.documentation.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #documentation} (A brief discussion of what the parameter 491 * is for and how it is used by the module.). This is the 492 * underlying object with id, value and extensions. The accessor 493 * "getDocumentation" gives direct access to the value 494 */ 495 public ParameterDefinition setDocumentationElement(StringType value) { 496 this.documentation = value; 497 return this; 498 } 499 500 /** 501 * @return A brief discussion of what the parameter is for and how it is used by 502 * the module. 503 */ 504 public String getDocumentation() { 505 return this.documentation == null ? null : this.documentation.getValue(); 506 } 507 508 /** 509 * @param value A brief discussion of what the parameter is for and how it is 510 * used by the module. 511 */ 512 public ParameterDefinition setDocumentation(String value) { 513 if (Utilities.noString(value)) 514 this.documentation = null; 515 else { 516 if (this.documentation == null) 517 this.documentation = new StringType(); 518 this.documentation.setValue(value); 519 } 520 return this; 521 } 522 523 /** 524 * @return {@link #type} (The type of the parameter.). This is the underlying 525 * object with id, value and extensions. The accessor "getType" gives 526 * direct access to the value 527 */ 528 public CodeType getTypeElement() { 529 if (this.type == null) 530 if (Configuration.errorOnAutoCreate()) 531 throw new Error("Attempt to auto-create ParameterDefinition.type"); 532 else if (Configuration.doAutoCreate()) 533 this.type = new CodeType(); // bb 534 return this.type; 535 } 536 537 public boolean hasTypeElement() { 538 return this.type != null && !this.type.isEmpty(); 539 } 540 541 public boolean hasType() { 542 return this.type != null && !this.type.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #type} (The type of the parameter.). This is the 547 * underlying object with id, value and extensions. The accessor 548 * "getType" gives direct access to the value 549 */ 550 public ParameterDefinition setTypeElement(CodeType value) { 551 this.type = value; 552 return this; 553 } 554 555 /** 556 * @return The type of the parameter. 557 */ 558 public String getType() { 559 return this.type == null ? null : this.type.getValue(); 560 } 561 562 /** 563 * @param value The type of the parameter. 564 */ 565 public ParameterDefinition setType(String value) { 566 if (this.type == null) 567 this.type = new CodeType(); 568 this.type.setValue(value); 569 return this; 570 } 571 572 /** 573 * @return {@link #profile} (If specified, this indicates a profile that the 574 * input data must conform to, or that the output data will conform 575 * to.). This is the underlying object with id, value and extensions. 576 * The accessor "getProfile" gives direct access to the value 577 */ 578 public CanonicalType getProfileElement() { 579 if (this.profile == null) 580 if (Configuration.errorOnAutoCreate()) 581 throw new Error("Attempt to auto-create ParameterDefinition.profile"); 582 else if (Configuration.doAutoCreate()) 583 this.profile = new CanonicalType(); // bb 584 return this.profile; 585 } 586 587 public boolean hasProfileElement() { 588 return this.profile != null && !this.profile.isEmpty(); 589 } 590 591 public boolean hasProfile() { 592 return this.profile != null && !this.profile.isEmpty(); 593 } 594 595 /** 596 * @param value {@link #profile} (If specified, this indicates a profile that 597 * the input data must conform to, or that the output data will 598 * conform to.). This is the underlying object with id, value and 599 * extensions. The accessor "getProfile" gives direct access to the 600 * value 601 */ 602 public ParameterDefinition setProfileElement(CanonicalType value) { 603 this.profile = value; 604 return this; 605 } 606 607 /** 608 * @return If specified, this indicates a profile that the input data must 609 * conform to, or that the output data will conform to. 610 */ 611 public String getProfile() { 612 return this.profile == null ? null : this.profile.getValue(); 613 } 614 615 /** 616 * @param value If specified, this indicates a profile that the input data must 617 * conform to, or that the output data will conform to. 618 */ 619 public ParameterDefinition setProfile(String value) { 620 if (Utilities.noString(value)) 621 this.profile = null; 622 else { 623 if (this.profile == null) 624 this.profile = new CanonicalType(); 625 this.profile.setValue(value); 626 } 627 return this; 628 } 629 630 protected void listChildren(List<Property> children) { 631 super.listChildren(children); 632 children.add(new Property("name", "code", 633 "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.", 0, 1, 634 name)); 635 children.add(new Property("use", "code", "Whether the parameter is input or output for the module.", 0, 1, use)); 636 children.add(new Property("min", "integer", 637 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min)); 638 children.add(new Property("max", "string", 639 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max)); 640 children.add(new Property("documentation", "string", 641 "A brief discussion of what the parameter is for and how it is used by the module.", 0, 1, documentation)); 642 children.add(new Property("type", "code", "The type of the parameter.", 0, 1, type)); 643 children.add(new Property("profile", "canonical(StructureDefinition)", 644 "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.", 645 0, 1, profile)); 646 } 647 648 @Override 649 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 650 switch (_hash) { 651 case 3373707: 652 /* name */ return new Property("name", "code", 653 "The name of the parameter used to allow access to the value of the parameter in evaluation contexts.", 0, 1, 654 name); 655 case 116103: 656 /* use */ return new Property("use", "code", "Whether the parameter is input or output for the module.", 0, 1, 657 use); 658 case 108114: 659 /* min */ return new Property("min", "integer", 660 "The minimum number of times this parameter SHALL appear in the request or response.", 0, 1, min); 661 case 107876: 662 /* max */ return new Property("max", "string", 663 "The maximum number of times this element is permitted to appear in the request or response.", 0, 1, max); 664 case 1587405498: 665 /* documentation */ return new Property("documentation", "string", 666 "A brief discussion of what the parameter is for and how it is used by the module.", 0, 1, documentation); 667 case 3575610: 668 /* type */ return new Property("type", "code", "The type of the parameter.", 0, 1, type); 669 case -309425751: 670 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 671 "If specified, this indicates a profile that the input data must conform to, or that the output data will conform to.", 672 0, 1, profile); 673 default: 674 return super.getNamedProperty(_hash, _name, _checkValid); 675 } 676 677 } 678 679 @Override 680 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 681 switch (hash) { 682 case 3373707: 683 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // CodeType 684 case 116103: 685 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<ParameterUse> 686 case 108114: 687 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // IntegerType 688 case 107876: 689 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 690 case 1587405498: 691 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 692 case 3575610: 693 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 694 case -309425751: 695 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 696 default: 697 return super.getProperty(hash, name, checkValid); 698 } 699 700 } 701 702 @Override 703 public Base setProperty(int hash, String name, Base value) throws FHIRException { 704 switch (hash) { 705 case 3373707: // name 706 this.name = castToCode(value); // CodeType 707 return value; 708 case 116103: // use 709 value = new ParameterUseEnumFactory().fromType(castToCode(value)); 710 this.use = (Enumeration) value; // Enumeration<ParameterUse> 711 return value; 712 case 108114: // min 713 this.min = castToInteger(value); // IntegerType 714 return value; 715 case 107876: // max 716 this.max = castToString(value); // StringType 717 return value; 718 case 1587405498: // documentation 719 this.documentation = castToString(value); // StringType 720 return value; 721 case 3575610: // type 722 this.type = castToCode(value); // CodeType 723 return value; 724 case -309425751: // profile 725 this.profile = castToCanonical(value); // CanonicalType 726 return value; 727 default: 728 return super.setProperty(hash, name, value); 729 } 730 731 } 732 733 @Override 734 public Base setProperty(String name, Base value) throws FHIRException { 735 if (name.equals("name")) { 736 this.name = castToCode(value); // CodeType 737 } else if (name.equals("use")) { 738 value = new ParameterUseEnumFactory().fromType(castToCode(value)); 739 this.use = (Enumeration) value; // Enumeration<ParameterUse> 740 } else if (name.equals("min")) { 741 this.min = castToInteger(value); // IntegerType 742 } else if (name.equals("max")) { 743 this.max = castToString(value); // StringType 744 } else if (name.equals("documentation")) { 745 this.documentation = castToString(value); // StringType 746 } else if (name.equals("type")) { 747 this.type = castToCode(value); // CodeType 748 } else if (name.equals("profile")) { 749 this.profile = castToCanonical(value); // CanonicalType 750 } else 751 return super.setProperty(name, value); 752 return value; 753 } 754 755 @Override 756 public void removeChild(String name, Base value) throws FHIRException { 757 if (name.equals("name")) { 758 this.name = null; 759 } else if (name.equals("use")) { 760 this.use = null; 761 } else if (name.equals("min")) { 762 this.min = null; 763 } else if (name.equals("max")) { 764 this.max = null; 765 } else if (name.equals("documentation")) { 766 this.documentation = null; 767 } else if (name.equals("type")) { 768 this.type = null; 769 } else if (name.equals("profile")) { 770 this.profile = null; 771 } else 772 super.removeChild(name, value); 773 774 } 775 776 @Override 777 public Base makeProperty(int hash, String name) throws FHIRException { 778 switch (hash) { 779 case 3373707: 780 return getNameElement(); 781 case 116103: 782 return getUseElement(); 783 case 108114: 784 return getMinElement(); 785 case 107876: 786 return getMaxElement(); 787 case 1587405498: 788 return getDocumentationElement(); 789 case 3575610: 790 return getTypeElement(); 791 case -309425751: 792 return getProfileElement(); 793 default: 794 return super.makeProperty(hash, name); 795 } 796 797 } 798 799 @Override 800 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 801 switch (hash) { 802 case 3373707: 803 /* name */ return new String[] { "code" }; 804 case 116103: 805 /* use */ return new String[] { "code" }; 806 case 108114: 807 /* min */ return new String[] { "integer" }; 808 case 107876: 809 /* max */ return new String[] { "string" }; 810 case 1587405498: 811 /* documentation */ return new String[] { "string" }; 812 case 3575610: 813 /* type */ return new String[] { "code" }; 814 case -309425751: 815 /* profile */ return new String[] { "canonical" }; 816 default: 817 return super.getTypesForProperty(hash, name); 818 } 819 820 } 821 822 @Override 823 public Base addChild(String name) throws FHIRException { 824 if (name.equals("name")) { 825 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.name"); 826 } else if (name.equals("use")) { 827 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.use"); 828 } else if (name.equals("min")) { 829 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.min"); 830 } else if (name.equals("max")) { 831 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.max"); 832 } else if (name.equals("documentation")) { 833 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.documentation"); 834 } else if (name.equals("type")) { 835 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.type"); 836 } else if (name.equals("profile")) { 837 throw new FHIRException("Cannot call addChild on a singleton property ParameterDefinition.profile"); 838 } else 839 return super.addChild(name); 840 } 841 842 public String fhirType() { 843 return "ParameterDefinition"; 844 845 } 846 847 public ParameterDefinition copy() { 848 ParameterDefinition dst = new ParameterDefinition(); 849 copyValues(dst); 850 return dst; 851 } 852 853 public void copyValues(ParameterDefinition dst) { 854 super.copyValues(dst); 855 dst.name = name == null ? null : name.copy(); 856 dst.use = use == null ? null : use.copy(); 857 dst.min = min == null ? null : min.copy(); 858 dst.max = max == null ? null : max.copy(); 859 dst.documentation = documentation == null ? null : documentation.copy(); 860 dst.type = type == null ? null : type.copy(); 861 dst.profile = profile == null ? null : profile.copy(); 862 } 863 864 protected ParameterDefinition typedCopy() { 865 return copy(); 866 } 867 868 @Override 869 public boolean equalsDeep(Base other_) { 870 if (!super.equalsDeep(other_)) 871 return false; 872 if (!(other_ instanceof ParameterDefinition)) 873 return false; 874 ParameterDefinition o = (ParameterDefinition) other_; 875 return compareDeep(name, o.name, true) && compareDeep(use, o.use, true) && compareDeep(min, o.min, true) 876 && compareDeep(max, o.max, true) && compareDeep(documentation, o.documentation, true) 877 && compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true); 878 } 879 880 @Override 881 public boolean equalsShallow(Base other_) { 882 if (!super.equalsShallow(other_)) 883 return false; 884 if (!(other_ instanceof ParameterDefinition)) 885 return false; 886 ParameterDefinition o = (ParameterDefinition) other_; 887 return compareValues(name, o.name, true) && compareValues(use, o.use, true) && compareValues(min, o.min, true) 888 && compareValues(max, o.max, true) && compareValues(documentation, o.documentation, true) 889 && compareValues(type, o.type, true); 890 } 891 892 public boolean isEmpty() { 893 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, use, min, max, documentation, type, profile); 894 } 895 896}