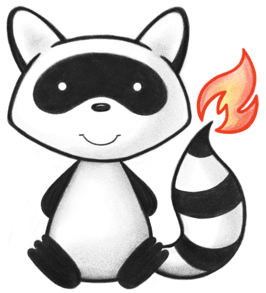
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Demographics and other administrative information about an individual or 050 * animal receiving care or other health-related services. 051 */ 052@ResourceDef(name = "Patient", profile = "http://hl7.org/fhir/StructureDefinition/Patient") 053public class Patient extends DomainResource { 054 055 public enum LinkType { 056 /** 057 * The patient resource containing this link must no longer be used. The link 058 * points forward to another patient resource that must be used in lieu of the 059 * patient resource that contains this link. 060 */ 061 REPLACEDBY, 062 /** 063 * The patient resource containing this link is the current active patient 064 * record. The link points back to an inactive patient resource that has been 065 * merged into this resource, and should be consulted to retrieve additional 066 * referenced information. 067 */ 068 REPLACES, 069 /** 070 * The patient resource containing this link is in use and valid but not 071 * considered the main source of information about a patient. The link points 072 * forward to another patient resource that should be consulted to retrieve 073 * additional patient information. 074 */ 075 REFER, 076 /** 077 * The patient resource containing this link is in use and valid, but points to 078 * another patient resource that is known to contain data about the same person. 079 * Data in this resource might overlap or contradict information found in the 080 * other patient resource. This link does not indicate any relative importance 081 * of the resources concerned, and both should be regarded as equally valid. 082 */ 083 SEEALSO, 084 /** 085 * added to help the parsers with the generic types 086 */ 087 NULL; 088 089 public static LinkType fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("replaced-by".equals(codeString)) 093 return REPLACEDBY; 094 if ("replaces".equals(codeString)) 095 return REPLACES; 096 if ("refer".equals(codeString)) 097 return REFER; 098 if ("seealso".equals(codeString)) 099 return SEEALSO; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 104 } 105 106 public String toCode() { 107 switch (this) { 108 case REPLACEDBY: 109 return "replaced-by"; 110 case REPLACES: 111 return "replaces"; 112 case REFER: 113 return "refer"; 114 case SEEALSO: 115 return "seealso"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case REPLACEDBY: 126 return "http://hl7.org/fhir/link-type"; 127 case REPLACES: 128 return "http://hl7.org/fhir/link-type"; 129 case REFER: 130 return "http://hl7.org/fhir/link-type"; 131 case SEEALSO: 132 return "http://hl7.org/fhir/link-type"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case REPLACEDBY: 143 return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 144 case REPLACES: 145 return "The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information."; 146 case REFER: 147 return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 148 case SEEALSO: 149 return "The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case REPLACEDBY: 160 return "Replaced-by"; 161 case REPLACES: 162 return "Replaces"; 163 case REFER: 164 return "Refer"; 165 case SEEALSO: 166 return "See also"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 } 174 175 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 176 public LinkType fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("replaced-by".equals(codeString)) 181 return LinkType.REPLACEDBY; 182 if ("replaces".equals(codeString)) 183 return LinkType.REPLACES; 184 if ("refer".equals(codeString)) 185 return LinkType.REFER; 186 if ("seealso".equals(codeString)) 187 return LinkType.SEEALSO; 188 throw new IllegalArgumentException("Unknown LinkType code '" + codeString + "'"); 189 } 190 191 public Enumeration<LinkType> fromType(PrimitiveType<?> code) throws FHIRException { 192 if (code == null) 193 return null; 194 if (code.isEmpty()) 195 return new Enumeration<LinkType>(this, LinkType.NULL, code); 196 String codeString = code.asStringValue(); 197 if (codeString == null || "".equals(codeString)) 198 return new Enumeration<LinkType>(this, LinkType.NULL, code); 199 if ("replaced-by".equals(codeString)) 200 return new Enumeration<LinkType>(this, LinkType.REPLACEDBY, code); 201 if ("replaces".equals(codeString)) 202 return new Enumeration<LinkType>(this, LinkType.REPLACES, code); 203 if ("refer".equals(codeString)) 204 return new Enumeration<LinkType>(this, LinkType.REFER, code); 205 if ("seealso".equals(codeString)) 206 return new Enumeration<LinkType>(this, LinkType.SEEALSO, code); 207 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 208 } 209 210 public String toCode(LinkType code) { 211 if (code == LinkType.REPLACEDBY) 212 return "replaced-by"; 213 if (code == LinkType.REPLACES) 214 return "replaces"; 215 if (code == LinkType.REFER) 216 return "refer"; 217 if (code == LinkType.SEEALSO) 218 return "seealso"; 219 return "?"; 220 } 221 222 public String toSystem(LinkType code) { 223 return code.getSystem(); 224 } 225 } 226 227 @Block() 228 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 229 /** 230 * The nature of the relationship between the patient and the contact person. 231 */ 232 @Child(name = "relationship", type = { 233 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 234 @Description(shortDefinition = "The kind of relationship", formalDefinition = "The nature of the relationship between the patient and the contact person.") 235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/patient-contactrelationship") 236 protected List<CodeableConcept> relationship; 237 238 /** 239 * A name associated with the contact person. 240 */ 241 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 242 @Description(shortDefinition = "A name associated with the contact person", formalDefinition = "A name associated with the contact person.") 243 protected HumanName name; 244 245 /** 246 * A contact detail for the person, e.g. a telephone number or an email address. 247 */ 248 @Child(name = "telecom", type = { 249 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 250 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 251 protected List<ContactPoint> telecom; 252 253 /** 254 * Address for the contact person. 255 */ 256 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 257 @Description(shortDefinition = "Address for the contact person", formalDefinition = "Address for the contact person.") 258 protected Address address; 259 260 /** 261 * Administrative Gender - the gender that the contact person is considered to 262 * have for administration and record keeping purposes. 263 */ 264 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 265 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.") 266 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 267 protected Enumeration<AdministrativeGender> gender; 268 269 /** 270 * Organization on behalf of which the contact is acting or for which the 271 * contact is working. 272 */ 273 @Child(name = "organization", type = { 274 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 275 @Description(shortDefinition = "Organization that is associated with the contact", formalDefinition = "Organization on behalf of which the contact is acting or for which the contact is working.") 276 protected Reference organization; 277 278 /** 279 * The actual object that is the target of the reference (Organization on behalf 280 * of which the contact is acting or for which the contact is working.) 281 */ 282 protected Organization organizationTarget; 283 284 /** 285 * The period during which this contact person or organization is valid to be 286 * contacted relating to this patient. 287 */ 288 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient.") 290 protected Period period; 291 292 private static final long serialVersionUID = 364269017L; 293 294 /** 295 * Constructor 296 */ 297 public ContactComponent() { 298 super(); 299 } 300 301 /** 302 * @return {@link #relationship} (The nature of the relationship between the 303 * patient and the contact person.) 304 */ 305 public List<CodeableConcept> getRelationship() { 306 if (this.relationship == null) 307 this.relationship = new ArrayList<CodeableConcept>(); 308 return this.relationship; 309 } 310 311 /** 312 * @return Returns a reference to <code>this</code> for easy method chaining 313 */ 314 public ContactComponent setRelationship(List<CodeableConcept> theRelationship) { 315 this.relationship = theRelationship; 316 return this; 317 } 318 319 public boolean hasRelationship() { 320 if (this.relationship == null) 321 return false; 322 for (CodeableConcept item : this.relationship) 323 if (!item.isEmpty()) 324 return true; 325 return false; 326 } 327 328 public CodeableConcept addRelationship() { // 3 329 CodeableConcept t = new CodeableConcept(); 330 if (this.relationship == null) 331 this.relationship = new ArrayList<CodeableConcept>(); 332 this.relationship.add(t); 333 return t; 334 } 335 336 public ContactComponent addRelationship(CodeableConcept t) { // 3 337 if (t == null) 338 return this; 339 if (this.relationship == null) 340 this.relationship = new ArrayList<CodeableConcept>(); 341 this.relationship.add(t); 342 return this; 343 } 344 345 /** 346 * @return The first repetition of repeating field {@link #relationship}, 347 * creating it if it does not already exist 348 */ 349 public CodeableConcept getRelationshipFirstRep() { 350 if (getRelationship().isEmpty()) { 351 addRelationship(); 352 } 353 return getRelationship().get(0); 354 } 355 356 /** 357 * @return {@link #name} (A name associated with the contact person.) 358 */ 359 public HumanName getName() { 360 if (this.name == null) 361 if (Configuration.errorOnAutoCreate()) 362 throw new Error("Attempt to auto-create ContactComponent.name"); 363 else if (Configuration.doAutoCreate()) 364 this.name = new HumanName(); // cc 365 return this.name; 366 } 367 368 public boolean hasName() { 369 return this.name != null && !this.name.isEmpty(); 370 } 371 372 /** 373 * @param value {@link #name} (A name associated with the contact person.) 374 */ 375 public ContactComponent setName(HumanName value) { 376 this.name = value; 377 return this; 378 } 379 380 /** 381 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 382 * number or an email address.) 383 */ 384 public List<ContactPoint> getTelecom() { 385 if (this.telecom == null) 386 this.telecom = new ArrayList<ContactPoint>(); 387 return this.telecom; 388 } 389 390 /** 391 * @return Returns a reference to <code>this</code> for easy method chaining 392 */ 393 public ContactComponent setTelecom(List<ContactPoint> theTelecom) { 394 this.telecom = theTelecom; 395 return this; 396 } 397 398 public boolean hasTelecom() { 399 if (this.telecom == null) 400 return false; 401 for (ContactPoint item : this.telecom) 402 if (!item.isEmpty()) 403 return true; 404 return false; 405 } 406 407 public ContactPoint addTelecom() { // 3 408 ContactPoint t = new ContactPoint(); 409 if (this.telecom == null) 410 this.telecom = new ArrayList<ContactPoint>(); 411 this.telecom.add(t); 412 return t; 413 } 414 415 public ContactComponent addTelecom(ContactPoint t) { // 3 416 if (t == null) 417 return this; 418 if (this.telecom == null) 419 this.telecom = new ArrayList<ContactPoint>(); 420 this.telecom.add(t); 421 return this; 422 } 423 424 /** 425 * @return The first repetition of repeating field {@link #telecom}, creating it 426 * if it does not already exist 427 */ 428 public ContactPoint getTelecomFirstRep() { 429 if (getTelecom().isEmpty()) { 430 addTelecom(); 431 } 432 return getTelecom().get(0); 433 } 434 435 /** 436 * @return {@link #address} (Address for the contact person.) 437 */ 438 public Address getAddress() { 439 if (this.address == null) 440 if (Configuration.errorOnAutoCreate()) 441 throw new Error("Attempt to auto-create ContactComponent.address"); 442 else if (Configuration.doAutoCreate()) 443 this.address = new Address(); // cc 444 return this.address; 445 } 446 447 public boolean hasAddress() { 448 return this.address != null && !this.address.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #address} (Address for the contact person.) 453 */ 454 public ContactComponent setAddress(Address value) { 455 this.address = value; 456 return this; 457 } 458 459 /** 460 * @return {@link #gender} (Administrative Gender - the gender that the contact 461 * person is considered to have for administration and record keeping 462 * purposes.). This is the underlying object with id, value and 463 * extensions. The accessor "getGender" gives direct access to the value 464 */ 465 public Enumeration<AdministrativeGender> getGenderElement() { 466 if (this.gender == null) 467 if (Configuration.errorOnAutoCreate()) 468 throw new Error("Attempt to auto-create ContactComponent.gender"); 469 else if (Configuration.doAutoCreate()) 470 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 471 return this.gender; 472 } 473 474 public boolean hasGenderElement() { 475 return this.gender != null && !this.gender.isEmpty(); 476 } 477 478 public boolean hasGender() { 479 return this.gender != null && !this.gender.isEmpty(); 480 } 481 482 /** 483 * @param value {@link #gender} (Administrative Gender - the gender that the 484 * contact person is considered to have for administration and 485 * record keeping purposes.). This is the underlying object with 486 * id, value and extensions. The accessor "getGender" gives direct 487 * access to the value 488 */ 489 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 490 this.gender = value; 491 return this; 492 } 493 494 /** 495 * @return Administrative Gender - the gender that the contact person is 496 * considered to have for administration and record keeping purposes. 497 */ 498 public AdministrativeGender getGender() { 499 return this.gender == null ? null : this.gender.getValue(); 500 } 501 502 /** 503 * @param value Administrative Gender - the gender that the contact person is 504 * considered to have for administration and record keeping 505 * purposes. 506 */ 507 public ContactComponent setGender(AdministrativeGender value) { 508 if (value == null) 509 this.gender = null; 510 else { 511 if (this.gender == null) 512 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 513 this.gender.setValue(value); 514 } 515 return this; 516 } 517 518 /** 519 * @return {@link #organization} (Organization on behalf of which the contact is 520 * acting or for which the contact is working.) 521 */ 522 public Reference getOrganization() { 523 if (this.organization == null) 524 if (Configuration.errorOnAutoCreate()) 525 throw new Error("Attempt to auto-create ContactComponent.organization"); 526 else if (Configuration.doAutoCreate()) 527 this.organization = new Reference(); // cc 528 return this.organization; 529 } 530 531 public boolean hasOrganization() { 532 return this.organization != null && !this.organization.isEmpty(); 533 } 534 535 /** 536 * @param value {@link #organization} (Organization on behalf of which the 537 * contact is acting or for which the contact is working.) 538 */ 539 public ContactComponent setOrganization(Reference value) { 540 this.organization = value; 541 return this; 542 } 543 544 /** 545 * @return {@link #organization} The actual object that is the target of the 546 * reference. The reference library doesn't populate this, but you can 547 * use it to hold the resource if you resolve it. (Organization on 548 * behalf of which the contact is acting or for which the contact is 549 * working.) 550 */ 551 public Organization getOrganizationTarget() { 552 if (this.organizationTarget == null) 553 if (Configuration.errorOnAutoCreate()) 554 throw new Error("Attempt to auto-create ContactComponent.organization"); 555 else if (Configuration.doAutoCreate()) 556 this.organizationTarget = new Organization(); // aa 557 return this.organizationTarget; 558 } 559 560 /** 561 * @param value {@link #organization} The actual object that is the target of 562 * the reference. The reference library doesn't use these, but you 563 * can use it to hold the resource if you resolve it. (Organization 564 * on behalf of which the contact is acting or for which the 565 * contact is working.) 566 */ 567 public ContactComponent setOrganizationTarget(Organization value) { 568 this.organizationTarget = value; 569 return this; 570 } 571 572 /** 573 * @return {@link #period} (The period during which this contact person or 574 * organization is valid to be contacted relating to this patient.) 575 */ 576 public Period getPeriod() { 577 if (this.period == null) 578 if (Configuration.errorOnAutoCreate()) 579 throw new Error("Attempt to auto-create ContactComponent.period"); 580 else if (Configuration.doAutoCreate()) 581 this.period = new Period(); // cc 582 return this.period; 583 } 584 585 public boolean hasPeriod() { 586 return this.period != null && !this.period.isEmpty(); 587 } 588 589 /** 590 * @param value {@link #period} (The period during which this contact person or 591 * organization is valid to be contacted relating to this patient.) 592 */ 593 public ContactComponent setPeriod(Period value) { 594 this.period = value; 595 return this; 596 } 597 598 protected void listChildren(List<Property> children) { 599 super.listChildren(children); 600 children.add(new Property("relationship", "CodeableConcept", 601 "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, 602 relationship)); 603 children.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name)); 604 children.add(new Property("telecom", "ContactPoint", 605 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 606 java.lang.Integer.MAX_VALUE, telecom)); 607 children.add(new Property("address", "Address", "Address for the contact person.", 0, 1, address)); 608 children.add(new Property("gender", "code", 609 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 610 0, 1, gender)); 611 children.add(new Property("organization", "Reference(Organization)", 612 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, 613 organization)); 614 children.add(new Property("period", "Period", 615 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 616 0, 1, period)); 617 } 618 619 @Override 620 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 621 switch (_hash) { 622 case -261851592: 623 /* relationship */ return new Property("relationship", "CodeableConcept", 624 "The nature of the relationship between the patient and the contact person.", 0, 625 java.lang.Integer.MAX_VALUE, relationship); 626 case 3373707: 627 /* name */ return new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name); 628 case -1429363305: 629 /* telecom */ return new Property("telecom", "ContactPoint", 630 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 631 java.lang.Integer.MAX_VALUE, telecom); 632 case -1147692044: 633 /* address */ return new Property("address", "Address", "Address for the contact person.", 0, 1, address); 634 case -1249512767: 635 /* gender */ return new Property("gender", "code", 636 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 637 0, 1, gender); 638 case 1178922291: 639 /* organization */ return new Property("organization", "Reference(Organization)", 640 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, 641 organization); 642 case -991726143: 643 /* period */ return new Property("period", "Period", 644 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 645 0, 1, period); 646 default: 647 return super.getNamedProperty(_hash, _name, _checkValid); 648 } 649 650 } 651 652 @Override 653 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 654 switch (hash) { 655 case -261851592: 656 /* relationship */ return this.relationship == null ? new Base[0] 657 : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 658 case 3373707: 659 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // HumanName 660 case -1429363305: 661 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 662 case -1147692044: 663 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 664 case -1249512767: 665 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 666 case 1178922291: 667 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 668 case -991726143: 669 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 670 default: 671 return super.getProperty(hash, name, checkValid); 672 } 673 674 } 675 676 @Override 677 public Base setProperty(int hash, String name, Base value) throws FHIRException { 678 switch (hash) { 679 case -261851592: // relationship 680 this.getRelationship().add(castToCodeableConcept(value)); // CodeableConcept 681 return value; 682 case 3373707: // name 683 this.name = castToHumanName(value); // HumanName 684 return value; 685 case -1429363305: // telecom 686 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 687 return value; 688 case -1147692044: // address 689 this.address = castToAddress(value); // Address 690 return value; 691 case -1249512767: // gender 692 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 693 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 694 return value; 695 case 1178922291: // organization 696 this.organization = castToReference(value); // Reference 697 return value; 698 case -991726143: // period 699 this.period = castToPeriod(value); // Period 700 return value; 701 default: 702 return super.setProperty(hash, name, value); 703 } 704 705 } 706 707 @Override 708 public Base setProperty(String name, Base value) throws FHIRException { 709 if (name.equals("relationship")) { 710 this.getRelationship().add(castToCodeableConcept(value)); 711 } else if (name.equals("name")) { 712 this.name = castToHumanName(value); // HumanName 713 } else if (name.equals("telecom")) { 714 this.getTelecom().add(castToContactPoint(value)); 715 } else if (name.equals("address")) { 716 this.address = castToAddress(value); // Address 717 } else if (name.equals("gender")) { 718 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 719 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 720 } else if (name.equals("organization")) { 721 this.organization = castToReference(value); // Reference 722 } else if (name.equals("period")) { 723 this.period = castToPeriod(value); // Period 724 } else 725 return super.setProperty(name, value); 726 return value; 727 } 728 729 @Override 730 public void removeChild(String name, Base value) throws FHIRException { 731 if (name.equals("relationship")) { 732 this.getRelationship().remove(castToCodeableConcept(value)); 733 } else if (name.equals("name")) { 734 this.name = null; 735 } else if (name.equals("telecom")) { 736 this.getTelecom().remove(castToContactPoint(value)); 737 } else if (name.equals("address")) { 738 this.address = null; 739 } else if (name.equals("gender")) { 740 this.gender = null; 741 } else if (name.equals("organization")) { 742 this.organization = null; 743 } else if (name.equals("period")) { 744 this.period = null; 745 } else 746 super.removeChild(name, value); 747 748 } 749 750 @Override 751 public Base makeProperty(int hash, String name) throws FHIRException { 752 switch (hash) { 753 case -261851592: 754 return addRelationship(); 755 case 3373707: 756 return getName(); 757 case -1429363305: 758 return addTelecom(); 759 case -1147692044: 760 return getAddress(); 761 case -1249512767: 762 return getGenderElement(); 763 case 1178922291: 764 return getOrganization(); 765 case -991726143: 766 return getPeriod(); 767 default: 768 return super.makeProperty(hash, name); 769 } 770 771 } 772 773 @Override 774 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 775 switch (hash) { 776 case -261851592: 777 /* relationship */ return new String[] { "CodeableConcept" }; 778 case 3373707: 779 /* name */ return new String[] { "HumanName" }; 780 case -1429363305: 781 /* telecom */ return new String[] { "ContactPoint" }; 782 case -1147692044: 783 /* address */ return new String[] { "Address" }; 784 case -1249512767: 785 /* gender */ return new String[] { "code" }; 786 case 1178922291: 787 /* organization */ return new String[] { "Reference" }; 788 case -991726143: 789 /* period */ return new String[] { "Period" }; 790 default: 791 return super.getTypesForProperty(hash, name); 792 } 793 794 } 795 796 @Override 797 public Base addChild(String name) throws FHIRException { 798 if (name.equals("relationship")) { 799 return addRelationship(); 800 } else if (name.equals("name")) { 801 this.name = new HumanName(); 802 return this.name; 803 } else if (name.equals("telecom")) { 804 return addTelecom(); 805 } else if (name.equals("address")) { 806 this.address = new Address(); 807 return this.address; 808 } else if (name.equals("gender")) { 809 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 810 } else if (name.equals("organization")) { 811 this.organization = new Reference(); 812 return this.organization; 813 } else if (name.equals("period")) { 814 this.period = new Period(); 815 return this.period; 816 } else 817 return super.addChild(name); 818 } 819 820 public ContactComponent copy() { 821 ContactComponent dst = new ContactComponent(); 822 copyValues(dst); 823 return dst; 824 } 825 826 public void copyValues(ContactComponent dst) { 827 super.copyValues(dst); 828 if (relationship != null) { 829 dst.relationship = new ArrayList<CodeableConcept>(); 830 for (CodeableConcept i : relationship) 831 dst.relationship.add(i.copy()); 832 } 833 ; 834 dst.name = name == null ? null : name.copy(); 835 if (telecom != null) { 836 dst.telecom = new ArrayList<ContactPoint>(); 837 for (ContactPoint i : telecom) 838 dst.telecom.add(i.copy()); 839 } 840 ; 841 dst.address = address == null ? null : address.copy(); 842 dst.gender = gender == null ? null : gender.copy(); 843 dst.organization = organization == null ? null : organization.copy(); 844 dst.period = period == null ? null : period.copy(); 845 } 846 847 @Override 848 public boolean equalsDeep(Base other_) { 849 if (!super.equalsDeep(other_)) 850 return false; 851 if (!(other_ instanceof ContactComponent)) 852 return false; 853 ContactComponent o = (ContactComponent) other_; 854 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) 855 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) 856 && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 857 && compareDeep(period, o.period, true); 858 } 859 860 @Override 861 public boolean equalsShallow(Base other_) { 862 if (!super.equalsShallow(other_)) 863 return false; 864 if (!(other_ instanceof ContactComponent)) 865 return false; 866 ContactComponent o = (ContactComponent) other_; 867 return compareValues(gender, o.gender, true); 868 } 869 870 public boolean isEmpty() { 871 return super.isEmpty() 872 && ca.uhn.fhir.util.ElementUtil.isEmpty(relationship, name, telecom, address, gender, organization, period); 873 } 874 875 public String fhirType() { 876 return "Patient.contact"; 877 878 } 879 880 } 881 882 @Block() 883 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 884 /** 885 * The ISO-639-1 alpha 2 code in lower case for the language, optionally 886 * followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper 887 * case; e.g. "en" for English, or "en-US" for American English versus "en-EN" 888 * for England English. 889 */ 890 @Child(name = "language", type = { 891 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 892 @Description(shortDefinition = "The language which can be used to communicate with the patient about his or her health", formalDefinition = "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.") 893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 894 protected CodeableConcept language; 895 896 /** 897 * Indicates whether or not the patient prefers this language (over other 898 * languages he masters up a certain level). 899 */ 900 @Child(name = "preferred", type = { 901 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 902 @Description(shortDefinition = "Language preference indicator", formalDefinition = "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).") 903 protected BooleanType preferred; 904 905 private static final long serialVersionUID = 633792918L; 906 907 /** 908 * Constructor 909 */ 910 public PatientCommunicationComponent() { 911 super(); 912 } 913 914 /** 915 * Constructor 916 */ 917 public PatientCommunicationComponent(CodeableConcept language) { 918 super(); 919 this.language = language; 920 } 921 922 /** 923 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the 924 * language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 925 * code for the region in upper case; e.g. "en" for English, or "en-US" 926 * for American English versus "en-EN" for England English.) 927 */ 928 public CodeableConcept getLanguage() { 929 if (this.language == null) 930 if (Configuration.errorOnAutoCreate()) 931 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 932 else if (Configuration.doAutoCreate()) 933 this.language = new CodeableConcept(); // cc 934 return this.language; 935 } 936 937 public boolean hasLanguage() { 938 return this.language != null && !this.language.isEmpty(); 939 } 940 941 /** 942 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for 943 * the language, optionally followed by a hyphen and the ISO-3166-1 944 * alpha 2 code for the region in upper case; e.g. "en" for 945 * English, or "en-US" for American English versus "en-EN" for 946 * England English.) 947 */ 948 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 949 this.language = value; 950 return this; 951 } 952 953 /** 954 * @return {@link #preferred} (Indicates whether or not the patient prefers this 955 * language (over other languages he masters up a certain level).). This 956 * is the underlying object with id, value and extensions. The accessor 957 * "getPreferred" gives direct access to the value 958 */ 959 public BooleanType getPreferredElement() { 960 if (this.preferred == null) 961 if (Configuration.errorOnAutoCreate()) 962 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 963 else if (Configuration.doAutoCreate()) 964 this.preferred = new BooleanType(); // bb 965 return this.preferred; 966 } 967 968 public boolean hasPreferredElement() { 969 return this.preferred != null && !this.preferred.isEmpty(); 970 } 971 972 public boolean hasPreferred() { 973 return this.preferred != null && !this.preferred.isEmpty(); 974 } 975 976 /** 977 * @param value {@link #preferred} (Indicates whether or not the patient prefers 978 * this language (over other languages he masters up a certain 979 * level).). This is the underlying object with id, value and 980 * extensions. The accessor "getPreferred" gives direct access to 981 * the value 982 */ 983 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 984 this.preferred = value; 985 return this; 986 } 987 988 /** 989 * @return Indicates whether or not the patient prefers this language (over 990 * other languages he masters up a certain level). 991 */ 992 public boolean getPreferred() { 993 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 994 } 995 996 /** 997 * @param value Indicates whether or not the patient prefers this language (over 998 * other languages he masters up a certain level). 999 */ 1000 public PatientCommunicationComponent setPreferred(boolean value) { 1001 if (this.preferred == null) 1002 this.preferred = new BooleanType(); 1003 this.preferred.setValue(value); 1004 return this; 1005 } 1006 1007 protected void listChildren(List<Property> children) { 1008 super.listChildren(children); 1009 children.add(new Property("language", "CodeableConcept", 1010 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 1011 0, 1, language)); 1012 children.add(new Property("preferred", "boolean", 1013 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1014 0, 1, preferred)); 1015 } 1016 1017 @Override 1018 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1019 switch (_hash) { 1020 case -1613589672: 1021 /* language */ return new Property("language", "CodeableConcept", 1022 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 1023 0, 1, language); 1024 case -1294005119: 1025 /* preferred */ return new Property("preferred", "boolean", 1026 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1027 0, 1, preferred); 1028 default: 1029 return super.getNamedProperty(_hash, _name, _checkValid); 1030 } 1031 1032 } 1033 1034 @Override 1035 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1036 switch (hash) { 1037 case -1613589672: 1038 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 1039 case -1294005119: 1040 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 1041 default: 1042 return super.getProperty(hash, name, checkValid); 1043 } 1044 1045 } 1046 1047 @Override 1048 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1049 switch (hash) { 1050 case -1613589672: // language 1051 this.language = castToCodeableConcept(value); // CodeableConcept 1052 return value; 1053 case -1294005119: // preferred 1054 this.preferred = castToBoolean(value); // BooleanType 1055 return value; 1056 default: 1057 return super.setProperty(hash, name, value); 1058 } 1059 1060 } 1061 1062 @Override 1063 public Base setProperty(String name, Base value) throws FHIRException { 1064 if (name.equals("language")) { 1065 this.language = castToCodeableConcept(value); // CodeableConcept 1066 } else if (name.equals("preferred")) { 1067 this.preferred = castToBoolean(value); // BooleanType 1068 } else 1069 return super.setProperty(name, value); 1070 return value; 1071 } 1072 1073 @Override 1074 public void removeChild(String name, Base value) throws FHIRException { 1075 if (name.equals("language")) { 1076 this.language = null; 1077 } else if (name.equals("preferred")) { 1078 this.preferred = null; 1079 } else 1080 super.removeChild(name, value); 1081 1082 } 1083 1084 @Override 1085 public Base makeProperty(int hash, String name) throws FHIRException { 1086 switch (hash) { 1087 case -1613589672: 1088 return getLanguage(); 1089 case -1294005119: 1090 return getPreferredElement(); 1091 default: 1092 return super.makeProperty(hash, name); 1093 } 1094 1095 } 1096 1097 @Override 1098 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1099 switch (hash) { 1100 case -1613589672: 1101 /* language */ return new String[] { "CodeableConcept" }; 1102 case -1294005119: 1103 /* preferred */ return new String[] { "boolean" }; 1104 default: 1105 return super.getTypesForProperty(hash, name); 1106 } 1107 1108 } 1109 1110 @Override 1111 public Base addChild(String name) throws FHIRException { 1112 if (name.equals("language")) { 1113 this.language = new CodeableConcept(); 1114 return this.language; 1115 } else if (name.equals("preferred")) { 1116 throw new FHIRException("Cannot call addChild on a singleton property Patient.preferred"); 1117 } else 1118 return super.addChild(name); 1119 } 1120 1121 public PatientCommunicationComponent copy() { 1122 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 1123 copyValues(dst); 1124 return dst; 1125 } 1126 1127 public void copyValues(PatientCommunicationComponent dst) { 1128 super.copyValues(dst); 1129 dst.language = language == null ? null : language.copy(); 1130 dst.preferred = preferred == null ? null : preferred.copy(); 1131 } 1132 1133 @Override 1134 public boolean equalsDeep(Base other_) { 1135 if (!super.equalsDeep(other_)) 1136 return false; 1137 if (!(other_ instanceof PatientCommunicationComponent)) 1138 return false; 1139 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1140 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 1141 } 1142 1143 @Override 1144 public boolean equalsShallow(Base other_) { 1145 if (!super.equalsShallow(other_)) 1146 return false; 1147 if (!(other_ instanceof PatientCommunicationComponent)) 1148 return false; 1149 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1150 return compareValues(preferred, o.preferred, true); 1151 } 1152 1153 public boolean isEmpty() { 1154 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 1155 } 1156 1157 public String fhirType() { 1158 return "Patient.communication"; 1159 1160 } 1161 1162 } 1163 1164 @Block() 1165 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 1166 /** 1167 * The other patient resource that the link refers to. 1168 */ 1169 @Child(name = "other", type = { Patient.class, 1170 RelatedPerson.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1171 @Description(shortDefinition = "The other patient or related person resource that the link refers to", formalDefinition = "The other patient resource that the link refers to.") 1172 protected Reference other; 1173 1174 /** 1175 * The actual object that is the target of the reference (The other patient 1176 * resource that the link refers to.) 1177 */ 1178 protected Resource otherTarget; 1179 1180 /** 1181 * The type of link between this patient resource and another patient resource. 1182 */ 1183 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1184 @Description(shortDefinition = "replaced-by | replaces | refer | seealso", formalDefinition = "The type of link between this patient resource and another patient resource.") 1185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/link-type") 1186 protected Enumeration<LinkType> type; 1187 1188 private static final long serialVersionUID = 1083576633L; 1189 1190 /** 1191 * Constructor 1192 */ 1193 public PatientLinkComponent() { 1194 super(); 1195 } 1196 1197 /** 1198 * Constructor 1199 */ 1200 public PatientLinkComponent(Reference other, Enumeration<LinkType> type) { 1201 super(); 1202 this.other = other; 1203 this.type = type; 1204 } 1205 1206 /** 1207 * @return {@link #other} (The other patient resource that the link refers to.) 1208 */ 1209 public Reference getOther() { 1210 if (this.other == null) 1211 if (Configuration.errorOnAutoCreate()) 1212 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1213 else if (Configuration.doAutoCreate()) 1214 this.other = new Reference(); // cc 1215 return this.other; 1216 } 1217 1218 public boolean hasOther() { 1219 return this.other != null && !this.other.isEmpty(); 1220 } 1221 1222 /** 1223 * @param value {@link #other} (The other patient resource that the link refers 1224 * to.) 1225 */ 1226 public PatientLinkComponent setOther(Reference value) { 1227 this.other = value; 1228 return this; 1229 } 1230 1231 /** 1232 * @return {@link #other} The actual object that is the target of the reference. 1233 * The reference library doesn't populate this, but you can use it to 1234 * hold the resource if you resolve it. (The other patient resource that 1235 * the link refers to.) 1236 */ 1237 public Resource getOtherTarget() { 1238 return this.otherTarget; 1239 } 1240 1241 /** 1242 * @param value {@link #other} The actual object that is the target of the 1243 * reference. The reference library doesn't use these, but you can 1244 * use it to hold the resource if you resolve it. (The other 1245 * patient resource that the link refers to.) 1246 */ 1247 public PatientLinkComponent setOtherTarget(Resource value) { 1248 this.otherTarget = value; 1249 return this; 1250 } 1251 1252 /** 1253 * @return {@link #type} (The type of link between this patient resource and 1254 * another patient resource.). This is the underlying object with id, 1255 * value and extensions. The accessor "getType" gives direct access to 1256 * the value 1257 */ 1258 public Enumeration<LinkType> getTypeElement() { 1259 if (this.type == null) 1260 if (Configuration.errorOnAutoCreate()) 1261 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1262 else if (Configuration.doAutoCreate()) 1263 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1264 return this.type; 1265 } 1266 1267 public boolean hasTypeElement() { 1268 return this.type != null && !this.type.isEmpty(); 1269 } 1270 1271 public boolean hasType() { 1272 return this.type != null && !this.type.isEmpty(); 1273 } 1274 1275 /** 1276 * @param value {@link #type} (The type of link between this patient resource 1277 * and another patient resource.). This is the underlying object 1278 * with id, value and extensions. The accessor "getType" gives 1279 * direct access to the value 1280 */ 1281 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1282 this.type = value; 1283 return this; 1284 } 1285 1286 /** 1287 * @return The type of link between this patient resource and another patient 1288 * resource. 1289 */ 1290 public LinkType getType() { 1291 return this.type == null ? null : this.type.getValue(); 1292 } 1293 1294 /** 1295 * @param value The type of link between this patient resource and another 1296 * patient resource. 1297 */ 1298 public PatientLinkComponent setType(LinkType value) { 1299 if (this.type == null) 1300 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1301 this.type.setValue(value); 1302 return this; 1303 } 1304 1305 protected void listChildren(List<Property> children) { 1306 super.listChildren(children); 1307 children.add(new Property("other", "Reference(Patient|RelatedPerson)", 1308 "The other patient resource that the link refers to.", 0, 1, other)); 1309 children.add(new Property("type", "code", 1310 "The type of link between this patient resource and another patient resource.", 0, 1, type)); 1311 } 1312 1313 @Override 1314 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1315 switch (_hash) { 1316 case 106069776: 1317 /* other */ return new Property("other", "Reference(Patient|RelatedPerson)", 1318 "The other patient resource that the link refers to.", 0, 1, other); 1319 case 3575610: 1320 /* type */ return new Property("type", "code", 1321 "The type of link between this patient resource and another patient resource.", 0, 1, type); 1322 default: 1323 return super.getNamedProperty(_hash, _name, _checkValid); 1324 } 1325 1326 } 1327 1328 @Override 1329 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1330 switch (hash) { 1331 case 106069776: 1332 /* other */ return this.other == null ? new Base[0] : new Base[] { this.other }; // Reference 1333 case 3575610: 1334 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<LinkType> 1335 default: 1336 return super.getProperty(hash, name, checkValid); 1337 } 1338 1339 } 1340 1341 @Override 1342 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1343 switch (hash) { 1344 case 106069776: // other 1345 this.other = castToReference(value); // Reference 1346 return value; 1347 case 3575610: // type 1348 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1349 this.type = (Enumeration) value; // Enumeration<LinkType> 1350 return value; 1351 default: 1352 return super.setProperty(hash, name, value); 1353 } 1354 1355 } 1356 1357 @Override 1358 public Base setProperty(String name, Base value) throws FHIRException { 1359 if (name.equals("other")) { 1360 this.other = castToReference(value); // Reference 1361 } else if (name.equals("type")) { 1362 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1363 this.type = (Enumeration) value; // Enumeration<LinkType> 1364 } else 1365 return super.setProperty(name, value); 1366 return value; 1367 } 1368 1369 @Override 1370 public void removeChild(String name, Base value) throws FHIRException { 1371 if (name.equals("other")) { 1372 this.other = null; 1373 } else if (name.equals("type")) { 1374 this.type = null; 1375 } else 1376 super.removeChild(name, value); 1377 1378 } 1379 1380 @Override 1381 public Base makeProperty(int hash, String name) throws FHIRException { 1382 switch (hash) { 1383 case 106069776: 1384 return getOther(); 1385 case 3575610: 1386 return getTypeElement(); 1387 default: 1388 return super.makeProperty(hash, name); 1389 } 1390 1391 } 1392 1393 @Override 1394 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1395 switch (hash) { 1396 case 106069776: 1397 /* other */ return new String[] { "Reference" }; 1398 case 3575610: 1399 /* type */ return new String[] { "code" }; 1400 default: 1401 return super.getTypesForProperty(hash, name); 1402 } 1403 1404 } 1405 1406 @Override 1407 public Base addChild(String name) throws FHIRException { 1408 if (name.equals("other")) { 1409 this.other = new Reference(); 1410 return this.other; 1411 } else if (name.equals("type")) { 1412 throw new FHIRException("Cannot call addChild on a singleton property Patient.type"); 1413 } else 1414 return super.addChild(name); 1415 } 1416 1417 public PatientLinkComponent copy() { 1418 PatientLinkComponent dst = new PatientLinkComponent(); 1419 copyValues(dst); 1420 return dst; 1421 } 1422 1423 public void copyValues(PatientLinkComponent dst) { 1424 super.copyValues(dst); 1425 dst.other = other == null ? null : other.copy(); 1426 dst.type = type == null ? null : type.copy(); 1427 } 1428 1429 @Override 1430 public boolean equalsDeep(Base other_) { 1431 if (!super.equalsDeep(other_)) 1432 return false; 1433 if (!(other_ instanceof PatientLinkComponent)) 1434 return false; 1435 PatientLinkComponent o = (PatientLinkComponent) other_; 1436 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1437 } 1438 1439 @Override 1440 public boolean equalsShallow(Base other_) { 1441 if (!super.equalsShallow(other_)) 1442 return false; 1443 if (!(other_ instanceof PatientLinkComponent)) 1444 return false; 1445 PatientLinkComponent o = (PatientLinkComponent) other_; 1446 return compareValues(type, o.type, true); 1447 } 1448 1449 public boolean isEmpty() { 1450 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(other, type); 1451 } 1452 1453 public String fhirType() { 1454 return "Patient.link"; 1455 1456 } 1457 1458 } 1459 1460 /** 1461 * An identifier for this patient. 1462 */ 1463 @Child(name = "identifier", type = { 1464 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1465 @Description(shortDefinition = "An identifier for this patient", formalDefinition = "An identifier for this patient.") 1466 protected List<Identifier> identifier; 1467 1468 /** 1469 * Whether this patient record is in active use. Many systems use this property 1470 * to mark as non-current patients, such as those that have not been seen for a 1471 * period of time based on an organization's business rules. 1472 * 1473 * It is often used to filter patient lists to exclude inactive patients 1474 * 1475 * Deceased patients may also be marked as inactive for the same reasons, but 1476 * may be active for some time after death. 1477 */ 1478 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1479 @Description(shortDefinition = "Whether this patient's record is in active use", formalDefinition = "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.") 1480 protected BooleanType active; 1481 1482 /** 1483 * A name associated with the individual. 1484 */ 1485 @Child(name = "name", type = { 1486 HumanName.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1487 @Description(shortDefinition = "A name associated with the patient", formalDefinition = "A name associated with the individual.") 1488 protected List<HumanName> name; 1489 1490 /** 1491 * A contact detail (e.g. a telephone number or an email address) by which the 1492 * individual may be contacted. 1493 */ 1494 @Child(name = "telecom", type = { 1495 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1496 @Description(shortDefinition = "A contact detail for the individual", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.") 1497 protected List<ContactPoint> telecom; 1498 1499 /** 1500 * Administrative Gender - the gender that the patient is considered to have for 1501 * administration and record keeping purposes. 1502 */ 1503 @Child(name = "gender", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1504 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.") 1505 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 1506 protected Enumeration<AdministrativeGender> gender; 1507 1508 /** 1509 * The date of birth for the individual. 1510 */ 1511 @Child(name = "birthDate", type = { DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1512 @Description(shortDefinition = "The date of birth for the individual", formalDefinition = "The date of birth for the individual.") 1513 protected DateType birthDate; 1514 1515 /** 1516 * Indicates if the individual is deceased or not. 1517 */ 1518 @Child(name = "deceased", type = { BooleanType.class, 1519 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1520 @Description(shortDefinition = "Indicates if the individual is deceased or not", formalDefinition = "Indicates if the individual is deceased or not.") 1521 protected Type deceased; 1522 1523 /** 1524 * An address for the individual. 1525 */ 1526 @Child(name = "address", type = { 1527 Address.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1528 @Description(shortDefinition = "An address for the individual", formalDefinition = "An address for the individual.") 1529 protected List<Address> address; 1530 1531 /** 1532 * This field contains a patient's most recent marital (civil) status. 1533 */ 1534 @Child(name = "maritalStatus", type = { 1535 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1536 @Description(shortDefinition = "Marital (civil) status of a patient", formalDefinition = "This field contains a patient's most recent marital (civil) status.") 1537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/marital-status") 1538 protected CodeableConcept maritalStatus; 1539 1540 /** 1541 * Indicates whether the patient is part of a multiple (boolean) or indicates 1542 * the actual birth order (integer). 1543 */ 1544 @Child(name = "multipleBirth", type = { BooleanType.class, 1545 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1546 @Description(shortDefinition = "Whether patient is part of a multiple birth", formalDefinition = "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).") 1547 protected Type multipleBirth; 1548 1549 /** 1550 * Image of the patient. 1551 */ 1552 @Child(name = "photo", type = { 1553 Attachment.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1554 @Description(shortDefinition = "Image of the patient", formalDefinition = "Image of the patient.") 1555 protected List<Attachment> photo; 1556 1557 /** 1558 * A contact party (e.g. guardian, partner, friend) for the patient. 1559 */ 1560 @Child(name = "contact", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1561 @Description(shortDefinition = "A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition = "A contact party (e.g. guardian, partner, friend) for the patient.") 1562 protected List<ContactComponent> contact; 1563 1564 /** 1565 * A language which may be used to communicate with the patient about his or her 1566 * health. 1567 */ 1568 @Child(name = "communication", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1569 @Description(shortDefinition = "A language which may be used to communicate with the patient about his or her health", formalDefinition = "A language which may be used to communicate with the patient about his or her health.") 1570 protected List<PatientCommunicationComponent> communication; 1571 1572 /** 1573 * Patient's nominated care provider. 1574 */ 1575 @Child(name = "generalPractitioner", type = { Organization.class, Practitioner.class, 1576 PractitionerRole.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1577 @Description(shortDefinition = "Patient's nominated primary care provider", formalDefinition = "Patient's nominated care provider.") 1578 protected List<Reference> generalPractitioner; 1579 /** 1580 * The actual objects that are the target of the reference (Patient's nominated 1581 * care provider.) 1582 */ 1583 protected List<Resource> generalPractitionerTarget; 1584 1585 /** 1586 * Organization that is the custodian of the patient record. 1587 */ 1588 @Child(name = "managingOrganization", type = { 1589 Organization.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1590 @Description(shortDefinition = "Organization that is the custodian of the patient record", formalDefinition = "Organization that is the custodian of the patient record.") 1591 protected Reference managingOrganization; 1592 1593 /** 1594 * The actual object that is the target of the reference (Organization that is 1595 * the custodian of the patient record.) 1596 */ 1597 protected Organization managingOrganizationTarget; 1598 1599 /** 1600 * Link to another patient resource that concerns the same actual patient. 1601 */ 1602 @Child(name = "link", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = true) 1603 @Description(shortDefinition = "Link to another patient resource that concerns the same actual person", formalDefinition = "Link to another patient resource that concerns the same actual patient.") 1604 protected List<PatientLinkComponent> link; 1605 1606 private static final long serialVersionUID = 2138656939L; 1607 1608 /** 1609 * Constructor 1610 */ 1611 public Patient() { 1612 super(); 1613 } 1614 1615 /** 1616 * @return {@link #identifier} (An identifier for this patient.) 1617 */ 1618 public List<Identifier> getIdentifier() { 1619 if (this.identifier == null) 1620 this.identifier = new ArrayList<Identifier>(); 1621 return this.identifier; 1622 } 1623 1624 /** 1625 * @return Returns a reference to <code>this</code> for easy method chaining 1626 */ 1627 public Patient setIdentifier(List<Identifier> theIdentifier) { 1628 this.identifier = theIdentifier; 1629 return this; 1630 } 1631 1632 public boolean hasIdentifier() { 1633 if (this.identifier == null) 1634 return false; 1635 for (Identifier item : this.identifier) 1636 if (!item.isEmpty()) 1637 return true; 1638 return false; 1639 } 1640 1641 public Identifier addIdentifier() { // 3 1642 Identifier t = new Identifier(); 1643 if (this.identifier == null) 1644 this.identifier = new ArrayList<Identifier>(); 1645 this.identifier.add(t); 1646 return t; 1647 } 1648 1649 public Patient addIdentifier(Identifier t) { // 3 1650 if (t == null) 1651 return this; 1652 if (this.identifier == null) 1653 this.identifier = new ArrayList<Identifier>(); 1654 this.identifier.add(t); 1655 return this; 1656 } 1657 1658 /** 1659 * @return The first repetition of repeating field {@link #identifier}, creating 1660 * it if it does not already exist 1661 */ 1662 public Identifier getIdentifierFirstRep() { 1663 if (getIdentifier().isEmpty()) { 1664 addIdentifier(); 1665 } 1666 return getIdentifier().get(0); 1667 } 1668 1669 /** 1670 * @return {@link #active} (Whether this patient record is in active use. Many 1671 * systems use this property to mark as non-current patients, such as 1672 * those that have not been seen for a period of time based on an 1673 * organization's business rules. 1674 * 1675 * It is often used to filter patient lists to exclude inactive patients 1676 * 1677 * Deceased patients may also be marked as inactive for the same 1678 * reasons, but may be active for some time after death.). This is the 1679 * underlying object with id, value and extensions. The accessor 1680 * "getActive" gives direct access to the value 1681 */ 1682 public BooleanType getActiveElement() { 1683 if (this.active == null) 1684 if (Configuration.errorOnAutoCreate()) 1685 throw new Error("Attempt to auto-create Patient.active"); 1686 else if (Configuration.doAutoCreate()) 1687 this.active = new BooleanType(); // bb 1688 return this.active; 1689 } 1690 1691 public boolean hasActiveElement() { 1692 return this.active != null && !this.active.isEmpty(); 1693 } 1694 1695 public boolean hasActive() { 1696 return this.active != null && !this.active.isEmpty(); 1697 } 1698 1699 /** 1700 * @param value {@link #active} (Whether this patient record is in active use. 1701 * Many systems use this property to mark as non-current patients, 1702 * such as those that have not been seen for a period of time based 1703 * on an organization's business rules. 1704 * 1705 * It is often used to filter patient lists to exclude inactive 1706 * patients 1707 * 1708 * Deceased patients may also be marked as inactive for the same 1709 * reasons, but may be active for some time after death.). This is 1710 * the underlying object with id, value and extensions. The 1711 * accessor "getActive" gives direct access to the value 1712 */ 1713 public Patient setActiveElement(BooleanType value) { 1714 this.active = value; 1715 return this; 1716 } 1717 1718 /** 1719 * @return Whether this patient record is in active use. Many systems use this 1720 * property to mark as non-current patients, such as those that have not 1721 * been seen for a period of time based on an organization's business 1722 * rules. 1723 * 1724 * It is often used to filter patient lists to exclude inactive patients 1725 * 1726 * Deceased patients may also be marked as inactive for the same 1727 * reasons, but may be active for some time after death. 1728 */ 1729 public boolean getActive() { 1730 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1731 } 1732 1733 /** 1734 * @param value Whether this patient record is in active use. Many systems use 1735 * this property to mark as non-current patients, such as those 1736 * that have not been seen for a period of time based on an 1737 * organization's business rules. 1738 * 1739 * It is often used to filter patient lists to exclude inactive 1740 * patients 1741 * 1742 * Deceased patients may also be marked as inactive for the same 1743 * reasons, but may be active for some time after death. 1744 */ 1745 public Patient setActive(boolean value) { 1746 if (this.active == null) 1747 this.active = new BooleanType(); 1748 this.active.setValue(value); 1749 return this; 1750 } 1751 1752 /** 1753 * @return {@link #name} (A name associated with the individual.) 1754 */ 1755 public List<HumanName> getName() { 1756 if (this.name == null) 1757 this.name = new ArrayList<HumanName>(); 1758 return this.name; 1759 } 1760 1761 /** 1762 * @return Returns a reference to <code>this</code> for easy method chaining 1763 */ 1764 public Patient setName(List<HumanName> theName) { 1765 this.name = theName; 1766 return this; 1767 } 1768 1769 public boolean hasName() { 1770 if (this.name == null) 1771 return false; 1772 for (HumanName item : this.name) 1773 if (!item.isEmpty()) 1774 return true; 1775 return false; 1776 } 1777 1778 public HumanName addName() { // 3 1779 HumanName t = new HumanName(); 1780 if (this.name == null) 1781 this.name = new ArrayList<HumanName>(); 1782 this.name.add(t); 1783 return t; 1784 } 1785 1786 public Patient addName(HumanName t) { // 3 1787 if (t == null) 1788 return this; 1789 if (this.name == null) 1790 this.name = new ArrayList<HumanName>(); 1791 this.name.add(t); 1792 return this; 1793 } 1794 1795 /** 1796 * @return The first repetition of repeating field {@link #name}, creating it if 1797 * it does not already exist 1798 */ 1799 public HumanName getNameFirstRep() { 1800 if (getName().isEmpty()) { 1801 addName(); 1802 } 1803 return getName().get(0); 1804 } 1805 1806 /** 1807 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1808 * email address) by which the individual may be contacted.) 1809 */ 1810 public List<ContactPoint> getTelecom() { 1811 if (this.telecom == null) 1812 this.telecom = new ArrayList<ContactPoint>(); 1813 return this.telecom; 1814 } 1815 1816 /** 1817 * @return Returns a reference to <code>this</code> for easy method chaining 1818 */ 1819 public Patient setTelecom(List<ContactPoint> theTelecom) { 1820 this.telecom = theTelecom; 1821 return this; 1822 } 1823 1824 public boolean hasTelecom() { 1825 if (this.telecom == null) 1826 return false; 1827 for (ContactPoint item : this.telecom) 1828 if (!item.isEmpty()) 1829 return true; 1830 return false; 1831 } 1832 1833 public ContactPoint addTelecom() { // 3 1834 ContactPoint t = new ContactPoint(); 1835 if (this.telecom == null) 1836 this.telecom = new ArrayList<ContactPoint>(); 1837 this.telecom.add(t); 1838 return t; 1839 } 1840 1841 public Patient addTelecom(ContactPoint t) { // 3 1842 if (t == null) 1843 return this; 1844 if (this.telecom == null) 1845 this.telecom = new ArrayList<ContactPoint>(); 1846 this.telecom.add(t); 1847 return this; 1848 } 1849 1850 /** 1851 * @return The first repetition of repeating field {@link #telecom}, creating it 1852 * if it does not already exist 1853 */ 1854 public ContactPoint getTelecomFirstRep() { 1855 if (getTelecom().isEmpty()) { 1856 addTelecom(); 1857 } 1858 return getTelecom().get(0); 1859 } 1860 1861 /** 1862 * @return {@link #gender} (Administrative Gender - the gender that the patient 1863 * is considered to have for administration and record keeping 1864 * purposes.). This is the underlying object with id, value and 1865 * extensions. The accessor "getGender" gives direct access to the value 1866 */ 1867 public Enumeration<AdministrativeGender> getGenderElement() { 1868 if (this.gender == null) 1869 if (Configuration.errorOnAutoCreate()) 1870 throw new Error("Attempt to auto-create Patient.gender"); 1871 else if (Configuration.doAutoCreate()) 1872 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1873 return this.gender; 1874 } 1875 1876 public boolean hasGenderElement() { 1877 return this.gender != null && !this.gender.isEmpty(); 1878 } 1879 1880 public boolean hasGender() { 1881 return this.gender != null && !this.gender.isEmpty(); 1882 } 1883 1884 /** 1885 * @param value {@link #gender} (Administrative Gender - the gender that the 1886 * patient is considered to have for administration and record 1887 * keeping purposes.). This is the underlying object with id, value 1888 * and extensions. The accessor "getGender" gives direct access to 1889 * the value 1890 */ 1891 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1892 this.gender = value; 1893 return this; 1894 } 1895 1896 /** 1897 * @return Administrative Gender - the gender that the patient is considered to 1898 * have for administration and record keeping purposes. 1899 */ 1900 public AdministrativeGender getGender() { 1901 return this.gender == null ? null : this.gender.getValue(); 1902 } 1903 1904 /** 1905 * @param value Administrative Gender - the gender that the patient is 1906 * considered to have for administration and record keeping 1907 * purposes. 1908 */ 1909 public Patient setGender(AdministrativeGender value) { 1910 if (value == null) 1911 this.gender = null; 1912 else { 1913 if (this.gender == null) 1914 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1915 this.gender.setValue(value); 1916 } 1917 return this; 1918 } 1919 1920 /** 1921 * @return {@link #birthDate} (The date of birth for the individual.). This is 1922 * the underlying object with id, value and extensions. The accessor 1923 * "getBirthDate" gives direct access to the value 1924 */ 1925 public DateType getBirthDateElement() { 1926 if (this.birthDate == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create Patient.birthDate"); 1929 else if (Configuration.doAutoCreate()) 1930 this.birthDate = new DateType(); // bb 1931 return this.birthDate; 1932 } 1933 1934 public boolean hasBirthDateElement() { 1935 return this.birthDate != null && !this.birthDate.isEmpty(); 1936 } 1937 1938 public boolean hasBirthDate() { 1939 return this.birthDate != null && !this.birthDate.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #birthDate} (The date of birth for the individual.). This 1944 * is the underlying object with id, value and extensions. The 1945 * accessor "getBirthDate" gives direct access to the value 1946 */ 1947 public Patient setBirthDateElement(DateType value) { 1948 this.birthDate = value; 1949 return this; 1950 } 1951 1952 /** 1953 * @return The date of birth for the individual. 1954 */ 1955 public Date getBirthDate() { 1956 return this.birthDate == null ? null : this.birthDate.getValue(); 1957 } 1958 1959 /** 1960 * @param value The date of birth for the individual. 1961 */ 1962 public Patient setBirthDate(Date value) { 1963 if (value == null) 1964 this.birthDate = null; 1965 else { 1966 if (this.birthDate == null) 1967 this.birthDate = new DateType(); 1968 this.birthDate.setValue(value); 1969 } 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1975 */ 1976 public Type getDeceased() { 1977 return this.deceased; 1978 } 1979 1980 /** 1981 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1982 */ 1983 public BooleanType getDeceasedBooleanType() throws FHIRException { 1984 if (this.deceased == null) 1985 this.deceased = new BooleanType(); 1986 if (!(this.deceased instanceof BooleanType)) 1987 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1988 + this.deceased.getClass().getName() + " was encountered"); 1989 return (BooleanType) this.deceased; 1990 } 1991 1992 public boolean hasDeceasedBooleanType() { 1993 return this != null && this.deceased instanceof BooleanType; 1994 } 1995 1996 /** 1997 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1998 */ 1999 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 2000 if (this.deceased == null) 2001 this.deceased = new DateTimeType(); 2002 if (!(this.deceased instanceof DateTimeType)) 2003 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2004 + this.deceased.getClass().getName() + " was encountered"); 2005 return (DateTimeType) this.deceased; 2006 } 2007 2008 public boolean hasDeceasedDateTimeType() { 2009 return this != null && this.deceased instanceof DateTimeType; 2010 } 2011 2012 public boolean hasDeceased() { 2013 return this.deceased != null && !this.deceased.isEmpty(); 2014 } 2015 2016 /** 2017 * @param value {@link #deceased} (Indicates if the individual is deceased or 2018 * not.) 2019 */ 2020 public Patient setDeceased(Type value) { 2021 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 2022 throw new Error("Not the right type for Patient.deceased[x]: " + value.fhirType()); 2023 this.deceased = value; 2024 return this; 2025 } 2026 2027 /** 2028 * @return {@link #address} (An address for the individual.) 2029 */ 2030 public List<Address> getAddress() { 2031 if (this.address == null) 2032 this.address = new ArrayList<Address>(); 2033 return this.address; 2034 } 2035 2036 /** 2037 * @return Returns a reference to <code>this</code> for easy method chaining 2038 */ 2039 public Patient setAddress(List<Address> theAddress) { 2040 this.address = theAddress; 2041 return this; 2042 } 2043 2044 public boolean hasAddress() { 2045 if (this.address == null) 2046 return false; 2047 for (Address item : this.address) 2048 if (!item.isEmpty()) 2049 return true; 2050 return false; 2051 } 2052 2053 public Address addAddress() { // 3 2054 Address t = new Address(); 2055 if (this.address == null) 2056 this.address = new ArrayList<Address>(); 2057 this.address.add(t); 2058 return t; 2059 } 2060 2061 public Patient addAddress(Address t) { // 3 2062 if (t == null) 2063 return this; 2064 if (this.address == null) 2065 this.address = new ArrayList<Address>(); 2066 this.address.add(t); 2067 return this; 2068 } 2069 2070 /** 2071 * @return The first repetition of repeating field {@link #address}, creating it 2072 * if it does not already exist 2073 */ 2074 public Address getAddressFirstRep() { 2075 if (getAddress().isEmpty()) { 2076 addAddress(); 2077 } 2078 return getAddress().get(0); 2079 } 2080 2081 /** 2082 * @return {@link #maritalStatus} (This field contains a patient's most recent 2083 * marital (civil) status.) 2084 */ 2085 public CodeableConcept getMaritalStatus() { 2086 if (this.maritalStatus == null) 2087 if (Configuration.errorOnAutoCreate()) 2088 throw new Error("Attempt to auto-create Patient.maritalStatus"); 2089 else if (Configuration.doAutoCreate()) 2090 this.maritalStatus = new CodeableConcept(); // cc 2091 return this.maritalStatus; 2092 } 2093 2094 public boolean hasMaritalStatus() { 2095 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 2096 } 2097 2098 /** 2099 * @param value {@link #maritalStatus} (This field contains a patient's most 2100 * recent marital (civil) status.) 2101 */ 2102 public Patient setMaritalStatus(CodeableConcept value) { 2103 this.maritalStatus = value; 2104 return this; 2105 } 2106 2107 /** 2108 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2109 * multiple (boolean) or indicates the actual birth order (integer).) 2110 */ 2111 public Type getMultipleBirth() { 2112 return this.multipleBirth; 2113 } 2114 2115 /** 2116 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2117 * multiple (boolean) or indicates the actual birth order (integer).) 2118 */ 2119 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 2120 if (this.multipleBirth == null) 2121 this.multipleBirth = new BooleanType(); 2122 if (!(this.multipleBirth instanceof BooleanType)) 2123 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 2124 + this.multipleBirth.getClass().getName() + " was encountered"); 2125 return (BooleanType) this.multipleBirth; 2126 } 2127 2128 public boolean hasMultipleBirthBooleanType() { 2129 return this != null && this.multipleBirth instanceof BooleanType; 2130 } 2131 2132 /** 2133 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2134 * multiple (boolean) or indicates the actual birth order (integer).) 2135 */ 2136 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 2137 if (this.multipleBirth == null) 2138 this.multipleBirth = new IntegerType(); 2139 if (!(this.multipleBirth instanceof IntegerType)) 2140 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 2141 + this.multipleBirth.getClass().getName() + " was encountered"); 2142 return (IntegerType) this.multipleBirth; 2143 } 2144 2145 public boolean hasMultipleBirthIntegerType() { 2146 return this != null && this.multipleBirth instanceof IntegerType; 2147 } 2148 2149 public boolean hasMultipleBirth() { 2150 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 2151 } 2152 2153 /** 2154 * @param value {@link #multipleBirth} (Indicates whether the patient is part of 2155 * a multiple (boolean) or indicates the actual birth order 2156 * (integer).) 2157 */ 2158 public Patient setMultipleBirth(Type value) { 2159 if (value != null && !(value instanceof BooleanType || value instanceof IntegerType)) 2160 throw new Error("Not the right type for Patient.multipleBirth[x]: " + value.fhirType()); 2161 this.multipleBirth = value; 2162 return this; 2163 } 2164 2165 /** 2166 * @return {@link #photo} (Image of the patient.) 2167 */ 2168 public List<Attachment> getPhoto() { 2169 if (this.photo == null) 2170 this.photo = new ArrayList<Attachment>(); 2171 return this.photo; 2172 } 2173 2174 /** 2175 * @return Returns a reference to <code>this</code> for easy method chaining 2176 */ 2177 public Patient setPhoto(List<Attachment> thePhoto) { 2178 this.photo = thePhoto; 2179 return this; 2180 } 2181 2182 public boolean hasPhoto() { 2183 if (this.photo == null) 2184 return false; 2185 for (Attachment item : this.photo) 2186 if (!item.isEmpty()) 2187 return true; 2188 return false; 2189 } 2190 2191 public Attachment addPhoto() { // 3 2192 Attachment t = new Attachment(); 2193 if (this.photo == null) 2194 this.photo = new ArrayList<Attachment>(); 2195 this.photo.add(t); 2196 return t; 2197 } 2198 2199 public Patient addPhoto(Attachment t) { // 3 2200 if (t == null) 2201 return this; 2202 if (this.photo == null) 2203 this.photo = new ArrayList<Attachment>(); 2204 this.photo.add(t); 2205 return this; 2206 } 2207 2208 /** 2209 * @return The first repetition of repeating field {@link #photo}, creating it 2210 * if it does not already exist 2211 */ 2212 public Attachment getPhotoFirstRep() { 2213 if (getPhoto().isEmpty()) { 2214 addPhoto(); 2215 } 2216 return getPhoto().get(0); 2217 } 2218 2219 /** 2220 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 2221 * for the patient.) 2222 */ 2223 public List<ContactComponent> getContact() { 2224 if (this.contact == null) 2225 this.contact = new ArrayList<ContactComponent>(); 2226 return this.contact; 2227 } 2228 2229 /** 2230 * @return Returns a reference to <code>this</code> for easy method chaining 2231 */ 2232 public Patient setContact(List<ContactComponent> theContact) { 2233 this.contact = theContact; 2234 return this; 2235 } 2236 2237 public boolean hasContact() { 2238 if (this.contact == null) 2239 return false; 2240 for (ContactComponent item : this.contact) 2241 if (!item.isEmpty()) 2242 return true; 2243 return false; 2244 } 2245 2246 public ContactComponent addContact() { // 3 2247 ContactComponent t = new ContactComponent(); 2248 if (this.contact == null) 2249 this.contact = new ArrayList<ContactComponent>(); 2250 this.contact.add(t); 2251 return t; 2252 } 2253 2254 public Patient addContact(ContactComponent t) { // 3 2255 if (t == null) 2256 return this; 2257 if (this.contact == null) 2258 this.contact = new ArrayList<ContactComponent>(); 2259 this.contact.add(t); 2260 return this; 2261 } 2262 2263 /** 2264 * @return The first repetition of repeating field {@link #contact}, creating it 2265 * if it does not already exist 2266 */ 2267 public ContactComponent getContactFirstRep() { 2268 if (getContact().isEmpty()) { 2269 addContact(); 2270 } 2271 return getContact().get(0); 2272 } 2273 2274 /** 2275 * @return {@link #communication} (A language which may be used to communicate 2276 * with the patient about his or her health.) 2277 */ 2278 public List<PatientCommunicationComponent> getCommunication() { 2279 if (this.communication == null) 2280 this.communication = new ArrayList<PatientCommunicationComponent>(); 2281 return this.communication; 2282 } 2283 2284 /** 2285 * @return Returns a reference to <code>this</code> for easy method chaining 2286 */ 2287 public Patient setCommunication(List<PatientCommunicationComponent> theCommunication) { 2288 this.communication = theCommunication; 2289 return this; 2290 } 2291 2292 public boolean hasCommunication() { 2293 if (this.communication == null) 2294 return false; 2295 for (PatientCommunicationComponent item : this.communication) 2296 if (!item.isEmpty()) 2297 return true; 2298 return false; 2299 } 2300 2301 public PatientCommunicationComponent addCommunication() { // 3 2302 PatientCommunicationComponent t = new PatientCommunicationComponent(); 2303 if (this.communication == null) 2304 this.communication = new ArrayList<PatientCommunicationComponent>(); 2305 this.communication.add(t); 2306 return t; 2307 } 2308 2309 public Patient addCommunication(PatientCommunicationComponent t) { // 3 2310 if (t == null) 2311 return this; 2312 if (this.communication == null) 2313 this.communication = new ArrayList<PatientCommunicationComponent>(); 2314 this.communication.add(t); 2315 return this; 2316 } 2317 2318 /** 2319 * @return The first repetition of repeating field {@link #communication}, 2320 * creating it if it does not already exist 2321 */ 2322 public PatientCommunicationComponent getCommunicationFirstRep() { 2323 if (getCommunication().isEmpty()) { 2324 addCommunication(); 2325 } 2326 return getCommunication().get(0); 2327 } 2328 2329 /** 2330 * @return {@link #generalPractitioner} (Patient's nominated care provider.) 2331 */ 2332 public List<Reference> getGeneralPractitioner() { 2333 if (this.generalPractitioner == null) 2334 this.generalPractitioner = new ArrayList<Reference>(); 2335 return this.generalPractitioner; 2336 } 2337 2338 /** 2339 * @return Returns a reference to <code>this</code> for easy method chaining 2340 */ 2341 public Patient setGeneralPractitioner(List<Reference> theGeneralPractitioner) { 2342 this.generalPractitioner = theGeneralPractitioner; 2343 return this; 2344 } 2345 2346 public boolean hasGeneralPractitioner() { 2347 if (this.generalPractitioner == null) 2348 return false; 2349 for (Reference item : this.generalPractitioner) 2350 if (!item.isEmpty()) 2351 return true; 2352 return false; 2353 } 2354 2355 public Reference addGeneralPractitioner() { // 3 2356 Reference t = new Reference(); 2357 if (this.generalPractitioner == null) 2358 this.generalPractitioner = new ArrayList<Reference>(); 2359 this.generalPractitioner.add(t); 2360 return t; 2361 } 2362 2363 public Patient addGeneralPractitioner(Reference t) { // 3 2364 if (t == null) 2365 return this; 2366 if (this.generalPractitioner == null) 2367 this.generalPractitioner = new ArrayList<Reference>(); 2368 this.generalPractitioner.add(t); 2369 return this; 2370 } 2371 2372 /** 2373 * @return The first repetition of repeating field {@link #generalPractitioner}, 2374 * creating it if it does not already exist 2375 */ 2376 public Reference getGeneralPractitionerFirstRep() { 2377 if (getGeneralPractitioner().isEmpty()) { 2378 addGeneralPractitioner(); 2379 } 2380 return getGeneralPractitioner().get(0); 2381 } 2382 2383 /** 2384 * @deprecated Use Reference#setResource(IBaseResource) instead 2385 */ 2386 @Deprecated 2387 public List<Resource> getGeneralPractitionerTarget() { 2388 if (this.generalPractitionerTarget == null) 2389 this.generalPractitionerTarget = new ArrayList<Resource>(); 2390 return this.generalPractitionerTarget; 2391 } 2392 2393 /** 2394 * @return {@link #managingOrganization} (Organization that is the custodian of 2395 * the patient record.) 2396 */ 2397 public Reference getManagingOrganization() { 2398 if (this.managingOrganization == null) 2399 if (Configuration.errorOnAutoCreate()) 2400 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2401 else if (Configuration.doAutoCreate()) 2402 this.managingOrganization = new Reference(); // cc 2403 return this.managingOrganization; 2404 } 2405 2406 public boolean hasManagingOrganization() { 2407 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2408 } 2409 2410 /** 2411 * @param value {@link #managingOrganization} (Organization that is the 2412 * custodian of the patient record.) 2413 */ 2414 public Patient setManagingOrganization(Reference value) { 2415 this.managingOrganization = value; 2416 return this; 2417 } 2418 2419 /** 2420 * @return {@link #managingOrganization} The actual object that is the target of 2421 * the reference. The reference library doesn't populate this, but you 2422 * can use it to hold the resource if you resolve it. (Organization that 2423 * is the custodian of the patient record.) 2424 */ 2425 public Organization getManagingOrganizationTarget() { 2426 if (this.managingOrganizationTarget == null) 2427 if (Configuration.errorOnAutoCreate()) 2428 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2429 else if (Configuration.doAutoCreate()) 2430 this.managingOrganizationTarget = new Organization(); // aa 2431 return this.managingOrganizationTarget; 2432 } 2433 2434 /** 2435 * @param value {@link #managingOrganization} The actual object that is the 2436 * target of the reference. The reference library doesn't use 2437 * these, but you can use it to hold the resource if you resolve 2438 * it. (Organization that is the custodian of the patient record.) 2439 */ 2440 public Patient setManagingOrganizationTarget(Organization value) { 2441 this.managingOrganizationTarget = value; 2442 return this; 2443 } 2444 2445 /** 2446 * @return {@link #link} (Link to another patient resource that concerns the 2447 * same actual patient.) 2448 */ 2449 public List<PatientLinkComponent> getLink() { 2450 if (this.link == null) 2451 this.link = new ArrayList<PatientLinkComponent>(); 2452 return this.link; 2453 } 2454 2455 /** 2456 * @return Returns a reference to <code>this</code> for easy method chaining 2457 */ 2458 public Patient setLink(List<PatientLinkComponent> theLink) { 2459 this.link = theLink; 2460 return this; 2461 } 2462 2463 public boolean hasLink() { 2464 if (this.link == null) 2465 return false; 2466 for (PatientLinkComponent item : this.link) 2467 if (!item.isEmpty()) 2468 return true; 2469 return false; 2470 } 2471 2472 public PatientLinkComponent addLink() { // 3 2473 PatientLinkComponent t = new PatientLinkComponent(); 2474 if (this.link == null) 2475 this.link = new ArrayList<PatientLinkComponent>(); 2476 this.link.add(t); 2477 return t; 2478 } 2479 2480 public Patient addLink(PatientLinkComponent t) { // 3 2481 if (t == null) 2482 return this; 2483 if (this.link == null) 2484 this.link = new ArrayList<PatientLinkComponent>(); 2485 this.link.add(t); 2486 return this; 2487 } 2488 2489 /** 2490 * @return The first repetition of repeating field {@link #link}, creating it if 2491 * it does not already exist 2492 */ 2493 public PatientLinkComponent getLinkFirstRep() { 2494 if (getLink().isEmpty()) { 2495 addLink(); 2496 } 2497 return getLink().get(0); 2498 } 2499 2500 protected void listChildren(List<Property> children) { 2501 super.listChildren(children); 2502 children.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2503 java.lang.Integer.MAX_VALUE, identifier)); 2504 children.add(new Property("active", "boolean", 2505 "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 2506 0, 1, active)); 2507 children.add(new Property("name", "HumanName", "A name associated with the individual.", 0, 2508 java.lang.Integer.MAX_VALUE, name)); 2509 children.add(new Property("telecom", "ContactPoint", 2510 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2511 java.lang.Integer.MAX_VALUE, telecom)); 2512 children.add(new Property("gender", "code", 2513 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2514 0, 1, gender)); 2515 children.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate)); 2516 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 2517 1, deceased)); 2518 children.add( 2519 new Property("address", "Address", "An address for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2520 children.add(new Property("maritalStatus", "CodeableConcept", 2521 "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus)); 2522 children.add(new Property("multipleBirth[x]", "boolean|integer", 2523 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2524 0, 1, multipleBirth)); 2525 children.add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2526 children.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, 2527 java.lang.Integer.MAX_VALUE, contact)); 2528 children.add(new Property("communication", "", 2529 "A language which may be used to communicate with the patient about his or her health.", 0, 2530 java.lang.Integer.MAX_VALUE, communication)); 2531 children.add(new Property("generalPractitioner", "Reference(Organization|Practitioner|PractitionerRole)", 2532 "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner)); 2533 children.add(new Property("managingOrganization", "Reference(Organization)", 2534 "Organization that is the custodian of the patient record.", 0, 1, managingOrganization)); 2535 children.add(new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 0, 2536 java.lang.Integer.MAX_VALUE, link)); 2537 } 2538 2539 @Override 2540 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2541 switch (_hash) { 2542 case -1618432855: 2543 /* identifier */ return new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2544 java.lang.Integer.MAX_VALUE, identifier); 2545 case -1422950650: 2546 /* active */ return new Property("active", "boolean", 2547 "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 2548 0, 1, active); 2549 case 3373707: 2550 /* name */ return new Property("name", "HumanName", "A name associated with the individual.", 0, 2551 java.lang.Integer.MAX_VALUE, name); 2552 case -1429363305: 2553 /* telecom */ return new Property("telecom", "ContactPoint", 2554 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2555 java.lang.Integer.MAX_VALUE, telecom); 2556 case -1249512767: 2557 /* gender */ return new Property("gender", "code", 2558 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2559 0, 1, gender); 2560 case -1210031859: 2561 /* birthDate */ return new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, 2562 birthDate); 2563 case -1311442804: 2564 /* deceased[x] */ return new Property("deceased[x]", "boolean|dateTime", 2565 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2566 case 561497972: 2567 /* deceased */ return new Property("deceased[x]", "boolean|dateTime", 2568 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2569 case 497463828: 2570 /* deceasedBoolean */ return new Property("deceased[x]", "boolean|dateTime", 2571 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2572 case -1971804369: 2573 /* deceasedDateTime */ return new Property("deceased[x]", "boolean|dateTime", 2574 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2575 case -1147692044: 2576 /* address */ return new Property("address", "Address", "An address for the individual.", 0, 2577 java.lang.Integer.MAX_VALUE, address); 2578 case 1756919302: 2579 /* maritalStatus */ return new Property("maritalStatus", "CodeableConcept", 2580 "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus); 2581 case -1764672111: 2582 /* multipleBirth[x] */ return new Property("multipleBirth[x]", "boolean|integer", 2583 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2584 0, 1, multipleBirth); 2585 case -677369713: 2586 /* multipleBirth */ return new Property("multipleBirth[x]", "boolean|integer", 2587 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2588 0, 1, multipleBirth); 2589 case -247534439: 2590 /* multipleBirthBoolean */ return new Property("multipleBirth[x]", "boolean|integer", 2591 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2592 0, 1, multipleBirth); 2593 case 1645805999: 2594 /* multipleBirthInteger */ return new Property("multipleBirth[x]", "boolean|integer", 2595 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2596 0, 1, multipleBirth); 2597 case 106642994: 2598 /* photo */ return new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, 2599 photo); 2600 case 951526432: 2601 /* contact */ return new Property("contact", "", 2602 "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact); 2603 case -1035284522: 2604 /* communication */ return new Property("communication", "", 2605 "A language which may be used to communicate with the patient about his or her health.", 0, 2606 java.lang.Integer.MAX_VALUE, communication); 2607 case 1488292898: 2608 /* generalPractitioner */ return new Property("generalPractitioner", 2609 "Reference(Organization|Practitioner|PractitionerRole)", "Patient's nominated care provider.", 0, 2610 java.lang.Integer.MAX_VALUE, generalPractitioner); 2611 case -2058947787: 2612 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 2613 "Organization that is the custodian of the patient record.", 0, 1, managingOrganization); 2614 case 3321850: 2615 /* link */ return new Property("link", "", 2616 "Link to another patient resource that concerns the same actual patient.", 0, java.lang.Integer.MAX_VALUE, 2617 link); 2618 default: 2619 return super.getNamedProperty(_hash, _name, _checkValid); 2620 } 2621 2622 } 2623 2624 @Override 2625 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2626 switch (hash) { 2627 case -1618432855: 2628 /* identifier */ return this.identifier == null ? new Base[0] 2629 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2630 case -1422950650: 2631 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 2632 case 3373707: 2633 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 2634 case -1429363305: 2635 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2636 case -1249512767: 2637 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 2638 case -1210031859: 2639 /* birthDate */ return this.birthDate == null ? new Base[0] : new Base[] { this.birthDate }; // DateType 2640 case 561497972: 2641 /* deceased */ return this.deceased == null ? new Base[0] : new Base[] { this.deceased }; // Type 2642 case -1147692044: 2643 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 2644 case 1756919302: 2645 /* maritalStatus */ return this.maritalStatus == null ? new Base[0] : new Base[] { this.maritalStatus }; // CodeableConcept 2646 case -677369713: 2647 /* multipleBirth */ return this.multipleBirth == null ? new Base[0] : new Base[] { this.multipleBirth }; // Type 2648 case 106642994: 2649 /* photo */ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 2650 case 951526432: 2651 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactComponent 2652 case -1035284522: 2653 /* communication */ return this.communication == null ? new Base[0] 2654 : this.communication.toArray(new Base[this.communication.size()]); // PatientCommunicationComponent 2655 case 1488292898: 2656 /* generalPractitioner */ return this.generalPractitioner == null ? new Base[0] 2657 : this.generalPractitioner.toArray(new Base[this.generalPractitioner.size()]); // Reference 2658 case -2058947787: 2659 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 2660 : new Base[] { this.managingOrganization }; // Reference 2661 case 3321850: 2662 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PatientLinkComponent 2663 default: 2664 return super.getProperty(hash, name, checkValid); 2665 } 2666 2667 } 2668 2669 @Override 2670 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2671 switch (hash) { 2672 case -1618432855: // identifier 2673 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2674 return value; 2675 case -1422950650: // active 2676 this.active = castToBoolean(value); // BooleanType 2677 return value; 2678 case 3373707: // name 2679 this.getName().add(castToHumanName(value)); // HumanName 2680 return value; 2681 case -1429363305: // telecom 2682 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2683 return value; 2684 case -1249512767: // gender 2685 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2686 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2687 return value; 2688 case -1210031859: // birthDate 2689 this.birthDate = castToDate(value); // DateType 2690 return value; 2691 case 561497972: // deceased 2692 this.deceased = castToType(value); // Type 2693 return value; 2694 case -1147692044: // address 2695 this.getAddress().add(castToAddress(value)); // Address 2696 return value; 2697 case 1756919302: // maritalStatus 2698 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2699 return value; 2700 case -677369713: // multipleBirth 2701 this.multipleBirth = castToType(value); // Type 2702 return value; 2703 case 106642994: // photo 2704 this.getPhoto().add(castToAttachment(value)); // Attachment 2705 return value; 2706 case 951526432: // contact 2707 this.getContact().add((ContactComponent) value); // ContactComponent 2708 return value; 2709 case -1035284522: // communication 2710 this.getCommunication().add((PatientCommunicationComponent) value); // PatientCommunicationComponent 2711 return value; 2712 case 1488292898: // generalPractitioner 2713 this.getGeneralPractitioner().add(castToReference(value)); // Reference 2714 return value; 2715 case -2058947787: // managingOrganization 2716 this.managingOrganization = castToReference(value); // Reference 2717 return value; 2718 case 3321850: // link 2719 this.getLink().add((PatientLinkComponent) value); // PatientLinkComponent 2720 return value; 2721 default: 2722 return super.setProperty(hash, name, value); 2723 } 2724 2725 } 2726 2727 @Override 2728 public Base setProperty(String name, Base value) throws FHIRException { 2729 if (name.equals("identifier")) { 2730 this.getIdentifier().add(castToIdentifier(value)); 2731 } else if (name.equals("active")) { 2732 this.active = castToBoolean(value); // BooleanType 2733 } else if (name.equals("name")) { 2734 this.getName().add(castToHumanName(value)); 2735 } else if (name.equals("telecom")) { 2736 this.getTelecom().add(castToContactPoint(value)); 2737 } else if (name.equals("gender")) { 2738 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2739 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2740 } else if (name.equals("birthDate")) { 2741 this.birthDate = castToDate(value); // DateType 2742 } else if (name.equals("deceased[x]")) { 2743 this.deceased = castToType(value); // Type 2744 } else if (name.equals("address")) { 2745 this.getAddress().add(castToAddress(value)); 2746 } else if (name.equals("maritalStatus")) { 2747 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2748 } else if (name.equals("multipleBirth[x]")) { 2749 this.multipleBirth = castToType(value); // Type 2750 } else if (name.equals("photo")) { 2751 this.getPhoto().add(castToAttachment(value)); 2752 } else if (name.equals("contact")) { 2753 this.getContact().add((ContactComponent) value); 2754 } else if (name.equals("communication")) { 2755 this.getCommunication().add((PatientCommunicationComponent) value); 2756 } else if (name.equals("generalPractitioner")) { 2757 this.getGeneralPractitioner().add(castToReference(value)); 2758 } else if (name.equals("managingOrganization")) { 2759 this.managingOrganization = castToReference(value); // Reference 2760 } else if (name.equals("link")) { 2761 this.getLink().add((PatientLinkComponent) value); 2762 } else 2763 return super.setProperty(name, value); 2764 return value; 2765 } 2766 2767 @Override 2768 public void removeChild(String name, Base value) throws FHIRException { 2769 if (name.equals("identifier")) { 2770 this.getIdentifier().remove(castToIdentifier(value)); 2771 } else if (name.equals("active")) { 2772 this.active = null; 2773 } else if (name.equals("name")) { 2774 this.getName().remove(castToHumanName(value)); 2775 } else if (name.equals("telecom")) { 2776 this.getTelecom().remove(castToContactPoint(value)); 2777 } else if (name.equals("gender")) { 2778 this.gender = null; 2779 } else if (name.equals("birthDate")) { 2780 this.birthDate = null; 2781 } else if (name.equals("deceased[x]")) { 2782 this.deceased = null; 2783 } else if (name.equals("address")) { 2784 this.getAddress().remove(castToAddress(value)); 2785 } else if (name.equals("maritalStatus")) { 2786 this.maritalStatus = null; 2787 } else if (name.equals("multipleBirth[x]")) { 2788 this.multipleBirth = null; 2789 } else if (name.equals("photo")) { 2790 this.getPhoto().remove(castToAttachment(value)); 2791 } else if (name.equals("contact")) { 2792 this.getContact().remove((ContactComponent) value); 2793 } else if (name.equals("communication")) { 2794 this.getCommunication().remove((PatientCommunicationComponent) value); 2795 } else if (name.equals("generalPractitioner")) { 2796 this.getGeneralPractitioner().remove(castToReference(value)); 2797 } else if (name.equals("managingOrganization")) { 2798 this.managingOrganization = null; 2799 } else if (name.equals("link")) { 2800 this.getLink().remove((PatientLinkComponent) value); 2801 } else 2802 super.removeChild(name, value); 2803 2804 } 2805 2806 @Override 2807 public Base makeProperty(int hash, String name) throws FHIRException { 2808 switch (hash) { 2809 case -1618432855: 2810 return addIdentifier(); 2811 case -1422950650: 2812 return getActiveElement(); 2813 case 3373707: 2814 return addName(); 2815 case -1429363305: 2816 return addTelecom(); 2817 case -1249512767: 2818 return getGenderElement(); 2819 case -1210031859: 2820 return getBirthDateElement(); 2821 case -1311442804: 2822 return getDeceased(); 2823 case 561497972: 2824 return getDeceased(); 2825 case -1147692044: 2826 return addAddress(); 2827 case 1756919302: 2828 return getMaritalStatus(); 2829 case -1764672111: 2830 return getMultipleBirth(); 2831 case -677369713: 2832 return getMultipleBirth(); 2833 case 106642994: 2834 return addPhoto(); 2835 case 951526432: 2836 return addContact(); 2837 case -1035284522: 2838 return addCommunication(); 2839 case 1488292898: 2840 return addGeneralPractitioner(); 2841 case -2058947787: 2842 return getManagingOrganization(); 2843 case 3321850: 2844 return addLink(); 2845 default: 2846 return super.makeProperty(hash, name); 2847 } 2848 2849 } 2850 2851 @Override 2852 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2853 switch (hash) { 2854 case -1618432855: 2855 /* identifier */ return new String[] { "Identifier" }; 2856 case -1422950650: 2857 /* active */ return new String[] { "boolean" }; 2858 case 3373707: 2859 /* name */ return new String[] { "HumanName" }; 2860 case -1429363305: 2861 /* telecom */ return new String[] { "ContactPoint" }; 2862 case -1249512767: 2863 /* gender */ return new String[] { "code" }; 2864 case -1210031859: 2865 /* birthDate */ return new String[] { "date" }; 2866 case 561497972: 2867 /* deceased */ return new String[] { "boolean", "dateTime" }; 2868 case -1147692044: 2869 /* address */ return new String[] { "Address" }; 2870 case 1756919302: 2871 /* maritalStatus */ return new String[] { "CodeableConcept" }; 2872 case -677369713: 2873 /* multipleBirth */ return new String[] { "boolean", "integer" }; 2874 case 106642994: 2875 /* photo */ return new String[] { "Attachment" }; 2876 case 951526432: 2877 /* contact */ return new String[] {}; 2878 case -1035284522: 2879 /* communication */ return new String[] {}; 2880 case 1488292898: 2881 /* generalPractitioner */ return new String[] { "Reference" }; 2882 case -2058947787: 2883 /* managingOrganization */ return new String[] { "Reference" }; 2884 case 3321850: 2885 /* link */ return new String[] {}; 2886 default: 2887 return super.getTypesForProperty(hash, name); 2888 } 2889 2890 } 2891 2892 @Override 2893 public Base addChild(String name) throws FHIRException { 2894 if (name.equals("identifier")) { 2895 return addIdentifier(); 2896 } else if (name.equals("active")) { 2897 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2898 } else if (name.equals("name")) { 2899 return addName(); 2900 } else if (name.equals("telecom")) { 2901 return addTelecom(); 2902 } else if (name.equals("gender")) { 2903 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2904 } else if (name.equals("birthDate")) { 2905 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2906 } else if (name.equals("deceasedBoolean")) { 2907 this.deceased = new BooleanType(); 2908 return this.deceased; 2909 } else if (name.equals("deceasedDateTime")) { 2910 this.deceased = new DateTimeType(); 2911 return this.deceased; 2912 } else if (name.equals("address")) { 2913 return addAddress(); 2914 } else if (name.equals("maritalStatus")) { 2915 this.maritalStatus = new CodeableConcept(); 2916 return this.maritalStatus; 2917 } else if (name.equals("multipleBirthBoolean")) { 2918 this.multipleBirth = new BooleanType(); 2919 return this.multipleBirth; 2920 } else if (name.equals("multipleBirthInteger")) { 2921 this.multipleBirth = new IntegerType(); 2922 return this.multipleBirth; 2923 } else if (name.equals("photo")) { 2924 return addPhoto(); 2925 } else if (name.equals("contact")) { 2926 return addContact(); 2927 } else if (name.equals("communication")) { 2928 return addCommunication(); 2929 } else if (name.equals("generalPractitioner")) { 2930 return addGeneralPractitioner(); 2931 } else if (name.equals("managingOrganization")) { 2932 this.managingOrganization = new Reference(); 2933 return this.managingOrganization; 2934 } else if (name.equals("link")) { 2935 return addLink(); 2936 } else 2937 return super.addChild(name); 2938 } 2939 2940 public String fhirType() { 2941 return "Patient"; 2942 2943 } 2944 2945 public Patient copy() { 2946 Patient dst = new Patient(); 2947 copyValues(dst); 2948 return dst; 2949 } 2950 2951 public void copyValues(Patient dst) { 2952 super.copyValues(dst); 2953 if (identifier != null) { 2954 dst.identifier = new ArrayList<Identifier>(); 2955 for (Identifier i : identifier) 2956 dst.identifier.add(i.copy()); 2957 } 2958 ; 2959 dst.active = active == null ? null : active.copy(); 2960 if (name != null) { 2961 dst.name = new ArrayList<HumanName>(); 2962 for (HumanName i : name) 2963 dst.name.add(i.copy()); 2964 } 2965 ; 2966 if (telecom != null) { 2967 dst.telecom = new ArrayList<ContactPoint>(); 2968 for (ContactPoint i : telecom) 2969 dst.telecom.add(i.copy()); 2970 } 2971 ; 2972 dst.gender = gender == null ? null : gender.copy(); 2973 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2974 dst.deceased = deceased == null ? null : deceased.copy(); 2975 if (address != null) { 2976 dst.address = new ArrayList<Address>(); 2977 for (Address i : address) 2978 dst.address.add(i.copy()); 2979 } 2980 ; 2981 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2982 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2983 if (photo != null) { 2984 dst.photo = new ArrayList<Attachment>(); 2985 for (Attachment i : photo) 2986 dst.photo.add(i.copy()); 2987 } 2988 ; 2989 if (contact != null) { 2990 dst.contact = new ArrayList<ContactComponent>(); 2991 for (ContactComponent i : contact) 2992 dst.contact.add(i.copy()); 2993 } 2994 ; 2995 if (communication != null) { 2996 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2997 for (PatientCommunicationComponent i : communication) 2998 dst.communication.add(i.copy()); 2999 } 3000 ; 3001 if (generalPractitioner != null) { 3002 dst.generalPractitioner = new ArrayList<Reference>(); 3003 for (Reference i : generalPractitioner) 3004 dst.generalPractitioner.add(i.copy()); 3005 } 3006 ; 3007 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 3008 if (link != null) { 3009 dst.link = new ArrayList<PatientLinkComponent>(); 3010 for (PatientLinkComponent i : link) 3011 dst.link.add(i.copy()); 3012 } 3013 ; 3014 } 3015 3016 protected Patient typedCopy() { 3017 return copy(); 3018 } 3019 3020 @Override 3021 public boolean equalsDeep(Base other_) { 3022 if (!super.equalsDeep(other_)) 3023 return false; 3024 if (!(other_ instanceof Patient)) 3025 return false; 3026 Patient o = (Patient) other_; 3027 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 3028 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 3029 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 3030 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) 3031 && compareDeep(maritalStatus, o.maritalStatus, true) && compareDeep(multipleBirth, o.multipleBirth, true) 3032 && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 3033 && compareDeep(communication, o.communication, true) 3034 && compareDeep(generalPractitioner, o.generalPractitioner, true) 3035 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true); 3036 } 3037 3038 @Override 3039 public boolean equalsShallow(Base other_) { 3040 if (!super.equalsShallow(other_)) 3041 return false; 3042 if (!(other_ instanceof Patient)) 3043 return false; 3044 Patient o = (Patient) other_; 3045 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 3046 && compareValues(birthDate, o.birthDate, true); 3047 } 3048 3049 public boolean isEmpty() { 3050 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name, telecom, gender, birthDate, 3051 deceased, address, maritalStatus, multipleBirth, photo, contact, communication, generalPractitioner, 3052 managingOrganization, link); 3053 } 3054 3055 @Override 3056 public ResourceType getResourceType() { 3057 return ResourceType.Patient; 3058 } 3059 3060 /** 3061 * Search parameter: <b>identifier</b> 3062 * <p> 3063 * Description: <b>A patient identifier</b><br> 3064 * Type: <b>token</b><br> 3065 * Path: <b>Patient.identifier</b><br> 3066 * </p> 3067 */ 3068 @SearchParamDefinition(name = "identifier", path = "Patient.identifier", description = "A patient identifier", type = "token") 3069 public static final String SP_IDENTIFIER = "identifier"; 3070 /** 3071 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3072 * <p> 3073 * Description: <b>A patient identifier</b><br> 3074 * Type: <b>token</b><br> 3075 * Path: <b>Patient.identifier</b><br> 3076 * </p> 3077 */ 3078 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3079 SP_IDENTIFIER); 3080 3081 /** 3082 * Search parameter: <b>given</b> 3083 * <p> 3084 * Description: <b>A portion of the given name of the patient</b><br> 3085 * Type: <b>string</b><br> 3086 * Path: <b>Patient.name.given</b><br> 3087 * </p> 3088 */ 3089 @SearchParamDefinition(name = "given", path = "Patient.name.given", description = "A portion of the given name of the patient", type = "string") 3090 public static final String SP_GIVEN = "given"; 3091 /** 3092 * <b>Fluent Client</b> search parameter constant for <b>given</b> 3093 * <p> 3094 * Description: <b>A portion of the given name of the patient</b><br> 3095 * Type: <b>string</b><br> 3096 * Path: <b>Patient.name.given</b><br> 3097 * </p> 3098 */ 3099 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam( 3100 SP_GIVEN); 3101 3102 /** 3103 * Search parameter: <b>address</b> 3104 * <p> 3105 * Description: <b>A server defined search that may match any of the string 3106 * fields in the Address, including line, city, district, state, country, 3107 * postalCode, and/or text</b><br> 3108 * Type: <b>string</b><br> 3109 * Path: <b>Patient.address</b><br> 3110 * </p> 3111 */ 3112 @SearchParamDefinition(name = "address", path = "Patient.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 3113 public static final String SP_ADDRESS = "address"; 3114 /** 3115 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3116 * <p> 3117 * Description: <b>A server defined search that may match any of the string 3118 * fields in the Address, including line, city, district, state, country, 3119 * postalCode, and/or text</b><br> 3120 * Type: <b>string</b><br> 3121 * Path: <b>Patient.address</b><br> 3122 * </p> 3123 */ 3124 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 3125 SP_ADDRESS); 3126 3127 /** 3128 * Search parameter: <b>birthdate</b> 3129 * <p> 3130 * Description: <b>The patient's date of birth</b><br> 3131 * Type: <b>date</b><br> 3132 * Path: <b>Patient.birthDate</b><br> 3133 * </p> 3134 */ 3135 @SearchParamDefinition(name = "birthdate", path = "Patient.birthDate", description = "The patient's date of birth", type = "date") 3136 public static final String SP_BIRTHDATE = "birthdate"; 3137 /** 3138 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 3139 * <p> 3140 * Description: <b>The patient's date of birth</b><br> 3141 * Type: <b>date</b><br> 3142 * Path: <b>Patient.birthDate</b><br> 3143 * </p> 3144 */ 3145 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3146 SP_BIRTHDATE); 3147 3148 /** 3149 * Search parameter: <b>deceased</b> 3150 * <p> 3151 * Description: <b>This patient has been marked as deceased, or as a death date 3152 * entered</b><br> 3153 * Type: <b>token</b><br> 3154 * Path: <b>Patient.deceased[x]</b><br> 3155 * </p> 3156 */ 3157 @SearchParamDefinition(name = "deceased", path = "Patient.deceased.exists() and Patient.deceased != false", description = "This patient has been marked as deceased, or as a death date entered", type = "token") 3158 public static final String SP_DECEASED = "deceased"; 3159 /** 3160 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 3161 * <p> 3162 * Description: <b>This patient has been marked as deceased, or as a death date 3163 * entered</b><br> 3164 * Type: <b>token</b><br> 3165 * Path: <b>Patient.deceased[x]</b><br> 3166 * </p> 3167 */ 3168 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3169 SP_DECEASED); 3170 3171 /** 3172 * Search parameter: <b>address-state</b> 3173 * <p> 3174 * Description: <b>A state specified in an address</b><br> 3175 * Type: <b>string</b><br> 3176 * Path: <b>Patient.address.state</b><br> 3177 * </p> 3178 */ 3179 @SearchParamDefinition(name = "address-state", path = "Patient.address.state", description = "A state specified in an address", type = "string") 3180 public static final String SP_ADDRESS_STATE = "address-state"; 3181 /** 3182 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 3183 * <p> 3184 * Description: <b>A state specified in an address</b><br> 3185 * Type: <b>string</b><br> 3186 * Path: <b>Patient.address.state</b><br> 3187 * </p> 3188 */ 3189 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3190 SP_ADDRESS_STATE); 3191 3192 /** 3193 * Search parameter: <b>gender</b> 3194 * <p> 3195 * Description: <b>Gender of the patient</b><br> 3196 * Type: <b>token</b><br> 3197 * Path: <b>Patient.gender</b><br> 3198 * </p> 3199 */ 3200 @SearchParamDefinition(name = "gender", path = "Patient.gender", description = "Gender of the patient", type = "token") 3201 public static final String SP_GENDER = "gender"; 3202 /** 3203 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 3204 * <p> 3205 * Description: <b>Gender of the patient</b><br> 3206 * Type: <b>token</b><br> 3207 * Path: <b>Patient.gender</b><br> 3208 * </p> 3209 */ 3210 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3211 SP_GENDER); 3212 3213 /** 3214 * Search parameter: <b>general-practitioner</b> 3215 * <p> 3216 * Description: <b>Patient's nominated general practitioner, not the 3217 * organization that manages the record</b><br> 3218 * Type: <b>reference</b><br> 3219 * Path: <b>Patient.generalPractitioner</b><br> 3220 * </p> 3221 */ 3222 @SearchParamDefinition(name = "general-practitioner", path = "Patient.generalPractitioner", description = "Patient's nominated general practitioner, not the organization that manages the record", type = "reference", providesMembershipIn = { 3223 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3224 Practitioner.class, PractitionerRole.class }) 3225 public static final String SP_GENERAL_PRACTITIONER = "general-practitioner"; 3226 /** 3227 * <b>Fluent Client</b> search parameter constant for 3228 * <b>general-practitioner</b> 3229 * <p> 3230 * Description: <b>Patient's nominated general practitioner, not the 3231 * organization that manages the record</b><br> 3232 * Type: <b>reference</b><br> 3233 * Path: <b>Patient.generalPractitioner</b><br> 3234 * </p> 3235 */ 3236 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GENERAL_PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3237 SP_GENERAL_PRACTITIONER); 3238 3239 /** 3240 * Constant for fluent queries to be used to add include statements. Specifies 3241 * the path value of "<b>Patient:general-practitioner</b>". 3242 */ 3243 public static final ca.uhn.fhir.model.api.Include INCLUDE_GENERAL_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 3244 "Patient:general-practitioner").toLocked(); 3245 3246 /** 3247 * Search parameter: <b>link</b> 3248 * <p> 3249 * Description: <b>All patients linked to the given patient</b><br> 3250 * Type: <b>reference</b><br> 3251 * Path: <b>Patient.link.other</b><br> 3252 * </p> 3253 */ 3254 @SearchParamDefinition(name = "link", path = "Patient.link.other", description = "All patients linked to the given patient", type = "reference", providesMembershipIn = { 3255 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3256 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 3257 RelatedPerson.class }) 3258 public static final String SP_LINK = "link"; 3259 /** 3260 * <b>Fluent Client</b> search parameter constant for <b>link</b> 3261 * <p> 3262 * Description: <b>All patients linked to the given patient</b><br> 3263 * Type: <b>reference</b><br> 3264 * Path: <b>Patient.link.other</b><br> 3265 * </p> 3266 */ 3267 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3268 SP_LINK); 3269 3270 /** 3271 * Constant for fluent queries to be used to add include statements. Specifies 3272 * the path value of "<b>Patient:link</b>". 3273 */ 3274 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Patient:link") 3275 .toLocked(); 3276 3277 /** 3278 * Search parameter: <b>active</b> 3279 * <p> 3280 * Description: <b>Whether the patient record is active</b><br> 3281 * Type: <b>token</b><br> 3282 * Path: <b>Patient.active</b><br> 3283 * </p> 3284 */ 3285 @SearchParamDefinition(name = "active", path = "Patient.active", description = "Whether the patient record is active", type = "token") 3286 public static final String SP_ACTIVE = "active"; 3287 /** 3288 * <b>Fluent Client</b> search parameter constant for <b>active</b> 3289 * <p> 3290 * Description: <b>Whether the patient record is active</b><br> 3291 * Type: <b>token</b><br> 3292 * Path: <b>Patient.active</b><br> 3293 * </p> 3294 */ 3295 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3296 SP_ACTIVE); 3297 3298 /** 3299 * Search parameter: <b>language</b> 3300 * <p> 3301 * Description: <b>Language code (irrespective of use value)</b><br> 3302 * Type: <b>token</b><br> 3303 * Path: <b>Patient.communication.language</b><br> 3304 * </p> 3305 */ 3306 @SearchParamDefinition(name = "language", path = "Patient.communication.language", description = "Language code (irrespective of use value)", type = "token") 3307 public static final String SP_LANGUAGE = "language"; 3308 /** 3309 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3310 * <p> 3311 * Description: <b>Language code (irrespective of use value)</b><br> 3312 * Type: <b>token</b><br> 3313 * Path: <b>Patient.communication.language</b><br> 3314 * </p> 3315 */ 3316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3317 SP_LANGUAGE); 3318 3319 /** 3320 * Search parameter: <b>address-postalcode</b> 3321 * <p> 3322 * Description: <b>A postalCode specified in an address</b><br> 3323 * Type: <b>string</b><br> 3324 * Path: <b>Patient.address.postalCode</b><br> 3325 * </p> 3326 */ 3327 @SearchParamDefinition(name = "address-postalcode", path = "Patient.address.postalCode", description = "A postalCode specified in an address", type = "string") 3328 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3329 /** 3330 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3331 * <p> 3332 * Description: <b>A postalCode specified in an address</b><br> 3333 * Type: <b>string</b><br> 3334 * Path: <b>Patient.address.postalCode</b><br> 3335 * </p> 3336 */ 3337 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3338 SP_ADDRESS_POSTALCODE); 3339 3340 /** 3341 * Search parameter: <b>address-country</b> 3342 * <p> 3343 * Description: <b>A country specified in an address</b><br> 3344 * Type: <b>string</b><br> 3345 * Path: <b>Patient.address.country</b><br> 3346 * </p> 3347 */ 3348 @SearchParamDefinition(name = "address-country", path = "Patient.address.country", description = "A country specified in an address", type = "string") 3349 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3350 /** 3351 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3352 * <p> 3353 * Description: <b>A country specified in an address</b><br> 3354 * Type: <b>string</b><br> 3355 * Path: <b>Patient.address.country</b><br> 3356 * </p> 3357 */ 3358 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3359 SP_ADDRESS_COUNTRY); 3360 3361 /** 3362 * Search parameter: <b>death-date</b> 3363 * <p> 3364 * Description: <b>The date of death has been provided and satisfies this search 3365 * value</b><br> 3366 * Type: <b>date</b><br> 3367 * Path: <b>Patient.deceasedDateTime</b><br> 3368 * </p> 3369 */ 3370 @SearchParamDefinition(name = "death-date", path = "(Patient.deceased as dateTime)", description = "The date of death has been provided and satisfies this search value", type = "date") 3371 public static final String SP_DEATH_DATE = "death-date"; 3372 /** 3373 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 3374 * <p> 3375 * Description: <b>The date of death has been provided and satisfies this search 3376 * value</b><br> 3377 * Type: <b>date</b><br> 3378 * Path: <b>Patient.deceasedDateTime</b><br> 3379 * </p> 3380 */ 3381 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3382 SP_DEATH_DATE); 3383 3384 /** 3385 * Search parameter: <b>phonetic</b> 3386 * <p> 3387 * Description: <b>A portion of either family or given name using some kind of 3388 * phonetic matching algorithm</b><br> 3389 * Type: <b>string</b><br> 3390 * Path: <b>Patient.name</b><br> 3391 * </p> 3392 */ 3393 @SearchParamDefinition(name = "phonetic", path = "Patient.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 3394 public static final String SP_PHONETIC = "phonetic"; 3395 /** 3396 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 3397 * <p> 3398 * Description: <b>A portion of either family or given name using some kind of 3399 * phonetic matching algorithm</b><br> 3400 * Type: <b>string</b><br> 3401 * Path: <b>Patient.name</b><br> 3402 * </p> 3403 */ 3404 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 3405 SP_PHONETIC); 3406 3407 /** 3408 * Search parameter: <b>phone</b> 3409 * <p> 3410 * Description: <b>A value in a phone contact</b><br> 3411 * Type: <b>token</b><br> 3412 * Path: <b>Patient.telecom(system=phone)</b><br> 3413 * </p> 3414 */ 3415 @SearchParamDefinition(name = "phone", path = "Patient.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 3416 public static final String SP_PHONE = "phone"; 3417 /** 3418 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 3419 * <p> 3420 * Description: <b>A value in a phone contact</b><br> 3421 * Type: <b>token</b><br> 3422 * Path: <b>Patient.telecom(system=phone)</b><br> 3423 * </p> 3424 */ 3425 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3426 SP_PHONE); 3427 3428 /** 3429 * Search parameter: <b>organization</b> 3430 * <p> 3431 * Description: <b>The organization that is the custodian of the patient 3432 * record</b><br> 3433 * Type: <b>reference</b><br> 3434 * Path: <b>Patient.managingOrganization</b><br> 3435 * </p> 3436 */ 3437 @SearchParamDefinition(name = "organization", path = "Patient.managingOrganization", description = "The organization that is the custodian of the patient record", type = "reference", target = { 3438 Organization.class }) 3439 public static final String SP_ORGANIZATION = "organization"; 3440 /** 3441 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3442 * <p> 3443 * Description: <b>The organization that is the custodian of the patient 3444 * record</b><br> 3445 * Type: <b>reference</b><br> 3446 * Path: <b>Patient.managingOrganization</b><br> 3447 * </p> 3448 */ 3449 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3450 SP_ORGANIZATION); 3451 3452 /** 3453 * Constant for fluent queries to be used to add include statements. Specifies 3454 * the path value of "<b>Patient:organization</b>". 3455 */ 3456 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3457 "Patient:organization").toLocked(); 3458 3459 /** 3460 * Search parameter: <b>name</b> 3461 * <p> 3462 * Description: <b>A server defined search that may match any of the string 3463 * fields in the HumanName, including family, give, prefix, suffix, suffix, 3464 * and/or text</b><br> 3465 * Type: <b>string</b><br> 3466 * Path: <b>Patient.name</b><br> 3467 * </p> 3468 */ 3469 @SearchParamDefinition(name = "name", path = "Patient.name", description = "A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type = "string") 3470 public static final String SP_NAME = "name"; 3471 /** 3472 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3473 * <p> 3474 * Description: <b>A server defined search that may match any of the string 3475 * fields in the HumanName, including family, give, prefix, suffix, suffix, 3476 * and/or text</b><br> 3477 * Type: <b>string</b><br> 3478 * Path: <b>Patient.name</b><br> 3479 * </p> 3480 */ 3481 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3482 SP_NAME); 3483 3484 /** 3485 * Search parameter: <b>address-use</b> 3486 * <p> 3487 * Description: <b>A use code specified in an address</b><br> 3488 * Type: <b>token</b><br> 3489 * Path: <b>Patient.address.use</b><br> 3490 * </p> 3491 */ 3492 @SearchParamDefinition(name = "address-use", path = "Patient.address.use", description = "A use code specified in an address", type = "token") 3493 public static final String SP_ADDRESS_USE = "address-use"; 3494 /** 3495 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3496 * <p> 3497 * Description: <b>A use code specified in an address</b><br> 3498 * Type: <b>token</b><br> 3499 * Path: <b>Patient.address.use</b><br> 3500 * </p> 3501 */ 3502 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3503 SP_ADDRESS_USE); 3504 3505 /** 3506 * Search parameter: <b>telecom</b> 3507 * <p> 3508 * Description: <b>The value in any kind of telecom details of the 3509 * patient</b><br> 3510 * Type: <b>token</b><br> 3511 * Path: <b>Patient.telecom</b><br> 3512 * </p> 3513 */ 3514 @SearchParamDefinition(name = "telecom", path = "Patient.telecom", description = "The value in any kind of telecom details of the patient", type = "token") 3515 public static final String SP_TELECOM = "telecom"; 3516 /** 3517 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 3518 * <p> 3519 * Description: <b>The value in any kind of telecom details of the 3520 * patient</b><br> 3521 * Type: <b>token</b><br> 3522 * Path: <b>Patient.telecom</b><br> 3523 * </p> 3524 */ 3525 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3526 SP_TELECOM); 3527 3528 /** 3529 * Search parameter: <b>family</b> 3530 * <p> 3531 * Description: <b>A portion of the family name of the patient</b><br> 3532 * Type: <b>string</b><br> 3533 * Path: <b>Patient.name.family</b><br> 3534 * </p> 3535 */ 3536 @SearchParamDefinition(name = "family", path = "Patient.name.family", description = "A portion of the family name of the patient", type = "string") 3537 public static final String SP_FAMILY = "family"; 3538 /** 3539 * <b>Fluent Client</b> search parameter constant for <b>family</b> 3540 * <p> 3541 * Description: <b>A portion of the family name of the patient</b><br> 3542 * Type: <b>string</b><br> 3543 * Path: <b>Patient.name.family</b><br> 3544 * </p> 3545 */ 3546 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3547 SP_FAMILY); 3548 3549 /** 3550 * Search parameter: <b>address-city</b> 3551 * <p> 3552 * Description: <b>A city specified in an address</b><br> 3553 * Type: <b>string</b><br> 3554 * Path: <b>Patient.address.city</b><br> 3555 * </p> 3556 */ 3557 @SearchParamDefinition(name = "address-city", path = "Patient.address.city", description = "A city specified in an address", type = "string") 3558 public static final String SP_ADDRESS_CITY = "address-city"; 3559 /** 3560 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3561 * <p> 3562 * Description: <b>A city specified in an address</b><br> 3563 * Type: <b>string</b><br> 3564 * Path: <b>Patient.address.city</b><br> 3565 * </p> 3566 */ 3567 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3568 SP_ADDRESS_CITY); 3569 3570 /** 3571 * Search parameter: <b>email</b> 3572 * <p> 3573 * Description: <b>A value in an email contact</b><br> 3574 * Type: <b>token</b><br> 3575 * Path: <b>Patient.telecom(system=email)</b><br> 3576 * </p> 3577 */ 3578 @SearchParamDefinition(name = "email", path = "Patient.telecom.where(system='email')", description = "A value in an email contact", type = "token") 3579 public static final String SP_EMAIL = "email"; 3580 /** 3581 * <b>Fluent Client</b> search parameter constant for <b>email</b> 3582 * <p> 3583 * Description: <b>A value in an email contact</b><br> 3584 * Type: <b>token</b><br> 3585 * Path: <b>Patient.telecom(system=email)</b><br> 3586 * </p> 3587 */ 3588 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3589 SP_EMAIL); 3590 3591}