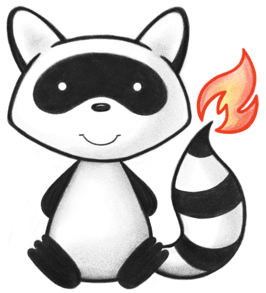
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Demographics and other administrative information about an individual or 050 * animal receiving care or other health-related services. 051 */ 052@ResourceDef(name = "Patient", profile = "http://hl7.org/fhir/StructureDefinition/Patient") 053public class Patient extends DomainResource { 054 055 public enum LinkType { 056 /** 057 * The patient resource containing this link must no longer be used. The link 058 * points forward to another patient resource that must be used in lieu of the 059 * patient resource that contains this link. 060 */ 061 REPLACEDBY, 062 /** 063 * The patient resource containing this link is the current active patient 064 * record. The link points back to an inactive patient resource that has been 065 * merged into this resource, and should be consulted to retrieve additional 066 * referenced information. 067 */ 068 REPLACES, 069 /** 070 * The patient resource containing this link is in use and valid but not 071 * considered the main source of information about a patient. The link points 072 * forward to another patient resource that should be consulted to retrieve 073 * additional patient information. 074 */ 075 REFER, 076 /** 077 * The patient resource containing this link is in use and valid, but points to 078 * another patient resource that is known to contain data about the same person. 079 * Data in this resource might overlap or contradict information found in the 080 * other patient resource. This link does not indicate any relative importance 081 * of the resources concerned, and both should be regarded as equally valid. 082 */ 083 SEEALSO, 084 /** 085 * added to help the parsers with the generic types 086 */ 087 NULL; 088 089 public static LinkType fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("replaced-by".equals(codeString)) 093 return REPLACEDBY; 094 if ("replaces".equals(codeString)) 095 return REPLACES; 096 if ("refer".equals(codeString)) 097 return REFER; 098 if ("seealso".equals(codeString)) 099 return SEEALSO; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 104 } 105 106 public String toCode() { 107 switch (this) { 108 case REPLACEDBY: 109 return "replaced-by"; 110 case REPLACES: 111 return "replaces"; 112 case REFER: 113 return "refer"; 114 case SEEALSO: 115 return "seealso"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getSystem() { 124 switch (this) { 125 case REPLACEDBY: 126 return "http://hl7.org/fhir/link-type"; 127 case REPLACES: 128 return "http://hl7.org/fhir/link-type"; 129 case REFER: 130 return "http://hl7.org/fhir/link-type"; 131 case SEEALSO: 132 return "http://hl7.org/fhir/link-type"; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDefinition() { 141 switch (this) { 142 case REPLACEDBY: 143 return "The patient resource containing this link must no longer be used. The link points forward to another patient resource that must be used in lieu of the patient resource that contains this link."; 144 case REPLACES: 145 return "The patient resource containing this link is the current active patient record. The link points back to an inactive patient resource that has been merged into this resource, and should be consulted to retrieve additional referenced information."; 146 case REFER: 147 return "The patient resource containing this link is in use and valid but not considered the main source of information about a patient. The link points forward to another patient resource that should be consulted to retrieve additional patient information."; 148 case SEEALSO: 149 return "The patient resource containing this link is in use and valid, but points to another patient resource that is known to contain data about the same person. Data in this resource might overlap or contradict information found in the other patient resource. This link does not indicate any relative importance of the resources concerned, and both should be regarded as equally valid."; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 157 public String getDisplay() { 158 switch (this) { 159 case REPLACEDBY: 160 return "Replaced-by"; 161 case REPLACES: 162 return "Replaces"; 163 case REFER: 164 return "Refer"; 165 case SEEALSO: 166 return "See also"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 } 174 175 public static class LinkTypeEnumFactory implements EnumFactory<LinkType> { 176 public LinkType fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("replaced-by".equals(codeString)) 181 return LinkType.REPLACEDBY; 182 if ("replaces".equals(codeString)) 183 return LinkType.REPLACES; 184 if ("refer".equals(codeString)) 185 return LinkType.REFER; 186 if ("seealso".equals(codeString)) 187 return LinkType.SEEALSO; 188 throw new IllegalArgumentException("Unknown LinkType code '" + codeString + "'"); 189 } 190 191 public Enumeration<LinkType> fromType(PrimitiveType<?> code) throws FHIRException { 192 if (code == null) 193 return null; 194 if (code.isEmpty()) 195 return new Enumeration<LinkType>(this, LinkType.NULL, code); 196 String codeString = code.asStringValue(); 197 if (codeString == null || "".equals(codeString)) 198 return new Enumeration<LinkType>(this, LinkType.NULL, code); 199 if ("replaced-by".equals(codeString)) 200 return new Enumeration<LinkType>(this, LinkType.REPLACEDBY, code); 201 if ("replaces".equals(codeString)) 202 return new Enumeration<LinkType>(this, LinkType.REPLACES, code); 203 if ("refer".equals(codeString)) 204 return new Enumeration<LinkType>(this, LinkType.REFER, code); 205 if ("seealso".equals(codeString)) 206 return new Enumeration<LinkType>(this, LinkType.SEEALSO, code); 207 throw new FHIRException("Unknown LinkType code '" + codeString + "'"); 208 } 209 210 public String toCode(LinkType code) { 211 if (code == LinkType.NULL) 212 return null; 213 if (code == LinkType.REPLACEDBY) 214 return "replaced-by"; 215 if (code == LinkType.REPLACES) 216 return "replaces"; 217 if (code == LinkType.REFER) 218 return "refer"; 219 if (code == LinkType.SEEALSO) 220 return "seealso"; 221 return "?"; 222 } 223 224 public String toSystem(LinkType code) { 225 return code.getSystem(); 226 } 227 } 228 229 @Block() 230 public static class ContactComponent extends BackboneElement implements IBaseBackboneElement { 231 /** 232 * The nature of the relationship between the patient and the contact person. 233 */ 234 @Child(name = "relationship", type = { 235 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 236 @Description(shortDefinition = "The kind of relationship", formalDefinition = "The nature of the relationship between the patient and the contact person.") 237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/patient-contactrelationship") 238 protected List<CodeableConcept> relationship; 239 240 /** 241 * A name associated with the contact person. 242 */ 243 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 244 @Description(shortDefinition = "A name associated with the contact person", formalDefinition = "A name associated with the contact person.") 245 protected HumanName name; 246 247 /** 248 * A contact detail for the person, e.g. a telephone number or an email address. 249 */ 250 @Child(name = "telecom", type = { 251 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 252 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 253 protected List<ContactPoint> telecom; 254 255 /** 256 * Address for the contact person. 257 */ 258 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 259 @Description(shortDefinition = "Address for the contact person", formalDefinition = "Address for the contact person.") 260 protected Address address; 261 262 /** 263 * Administrative Gender - the gender that the contact person is considered to 264 * have for administration and record keeping purposes. 265 */ 266 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 267 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.") 268 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 269 protected Enumeration<AdministrativeGender> gender; 270 271 /** 272 * Organization on behalf of which the contact is acting or for which the 273 * contact is working. 274 */ 275 @Child(name = "organization", type = { 276 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 277 @Description(shortDefinition = "Organization that is associated with the contact", formalDefinition = "Organization on behalf of which the contact is acting or for which the contact is working.") 278 protected Reference organization; 279 280 /** 281 * The actual object that is the target of the reference (Organization on behalf 282 * of which the contact is acting or for which the contact is working.) 283 */ 284 protected Organization organizationTarget; 285 286 /** 287 * The period during which this contact person or organization is valid to be 288 * contacted relating to this patient. 289 */ 290 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 291 @Description(shortDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient", formalDefinition = "The period during which this contact person or organization is valid to be contacted relating to this patient.") 292 protected Period period; 293 294 private static final long serialVersionUID = 364269017L; 295 296 /** 297 * Constructor 298 */ 299 public ContactComponent() { 300 super(); 301 } 302 303 /** 304 * @return {@link #relationship} (The nature of the relationship between the 305 * patient and the contact person.) 306 */ 307 public List<CodeableConcept> getRelationship() { 308 if (this.relationship == null) 309 this.relationship = new ArrayList<CodeableConcept>(); 310 return this.relationship; 311 } 312 313 /** 314 * @return Returns a reference to <code>this</code> for easy method chaining 315 */ 316 public ContactComponent setRelationship(List<CodeableConcept> theRelationship) { 317 this.relationship = theRelationship; 318 return this; 319 } 320 321 public boolean hasRelationship() { 322 if (this.relationship == null) 323 return false; 324 for (CodeableConcept item : this.relationship) 325 if (!item.isEmpty()) 326 return true; 327 return false; 328 } 329 330 public CodeableConcept addRelationship() { // 3 331 CodeableConcept t = new CodeableConcept(); 332 if (this.relationship == null) 333 this.relationship = new ArrayList<CodeableConcept>(); 334 this.relationship.add(t); 335 return t; 336 } 337 338 public ContactComponent addRelationship(CodeableConcept t) { // 3 339 if (t == null) 340 return this; 341 if (this.relationship == null) 342 this.relationship = new ArrayList<CodeableConcept>(); 343 this.relationship.add(t); 344 return this; 345 } 346 347 /** 348 * @return The first repetition of repeating field {@link #relationship}, 349 * creating it if it does not already exist 350 */ 351 public CodeableConcept getRelationshipFirstRep() { 352 if (getRelationship().isEmpty()) { 353 addRelationship(); 354 } 355 return getRelationship().get(0); 356 } 357 358 /** 359 * @return {@link #name} (A name associated with the contact person.) 360 */ 361 public HumanName getName() { 362 if (this.name == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create ContactComponent.name"); 365 else if (Configuration.doAutoCreate()) 366 this.name = new HumanName(); // cc 367 return this.name; 368 } 369 370 public boolean hasName() { 371 return this.name != null && !this.name.isEmpty(); 372 } 373 374 /** 375 * @param value {@link #name} (A name associated with the contact person.) 376 */ 377 public ContactComponent setName(HumanName value) { 378 this.name = value; 379 return this; 380 } 381 382 /** 383 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 384 * number or an email address.) 385 */ 386 public List<ContactPoint> getTelecom() { 387 if (this.telecom == null) 388 this.telecom = new ArrayList<ContactPoint>(); 389 return this.telecom; 390 } 391 392 /** 393 * @return Returns a reference to <code>this</code> for easy method chaining 394 */ 395 public ContactComponent setTelecom(List<ContactPoint> theTelecom) { 396 this.telecom = theTelecom; 397 return this; 398 } 399 400 public boolean hasTelecom() { 401 if (this.telecom == null) 402 return false; 403 for (ContactPoint item : this.telecom) 404 if (!item.isEmpty()) 405 return true; 406 return false; 407 } 408 409 public ContactPoint addTelecom() { // 3 410 ContactPoint t = new ContactPoint(); 411 if (this.telecom == null) 412 this.telecom = new ArrayList<ContactPoint>(); 413 this.telecom.add(t); 414 return t; 415 } 416 417 public ContactComponent addTelecom(ContactPoint t) { // 3 418 if (t == null) 419 return this; 420 if (this.telecom == null) 421 this.telecom = new ArrayList<ContactPoint>(); 422 this.telecom.add(t); 423 return this; 424 } 425 426 /** 427 * @return The first repetition of repeating field {@link #telecom}, creating it 428 * if it does not already exist 429 */ 430 public ContactPoint getTelecomFirstRep() { 431 if (getTelecom().isEmpty()) { 432 addTelecom(); 433 } 434 return getTelecom().get(0); 435 } 436 437 /** 438 * @return {@link #address} (Address for the contact person.) 439 */ 440 public Address getAddress() { 441 if (this.address == null) 442 if (Configuration.errorOnAutoCreate()) 443 throw new Error("Attempt to auto-create ContactComponent.address"); 444 else if (Configuration.doAutoCreate()) 445 this.address = new Address(); // cc 446 return this.address; 447 } 448 449 public boolean hasAddress() { 450 return this.address != null && !this.address.isEmpty(); 451 } 452 453 /** 454 * @param value {@link #address} (Address for the contact person.) 455 */ 456 public ContactComponent setAddress(Address value) { 457 this.address = value; 458 return this; 459 } 460 461 /** 462 * @return {@link #gender} (Administrative Gender - the gender that the contact 463 * person is considered to have for administration and record keeping 464 * purposes.). This is the underlying object with id, value and 465 * extensions. The accessor "getGender" gives direct access to the value 466 */ 467 public Enumeration<AdministrativeGender> getGenderElement() { 468 if (this.gender == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create ContactComponent.gender"); 471 else if (Configuration.doAutoCreate()) 472 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 473 return this.gender; 474 } 475 476 public boolean hasGenderElement() { 477 return this.gender != null && !this.gender.isEmpty(); 478 } 479 480 public boolean hasGender() { 481 return this.gender != null && !this.gender.isEmpty(); 482 } 483 484 /** 485 * @param value {@link #gender} (Administrative Gender - the gender that the 486 * contact person is considered to have for administration and 487 * record keeping purposes.). This is the underlying object with 488 * id, value and extensions. The accessor "getGender" gives direct 489 * access to the value 490 */ 491 public ContactComponent setGenderElement(Enumeration<AdministrativeGender> value) { 492 this.gender = value; 493 return this; 494 } 495 496 /** 497 * @return Administrative Gender - the gender that the contact person is 498 * considered to have for administration and record keeping purposes. 499 */ 500 public AdministrativeGender getGender() { 501 return this.gender == null ? null : this.gender.getValue(); 502 } 503 504 /** 505 * @param value Administrative Gender - the gender that the contact person is 506 * considered to have for administration and record keeping 507 * purposes. 508 */ 509 public ContactComponent setGender(AdministrativeGender value) { 510 if (value == null) 511 this.gender = null; 512 else { 513 if (this.gender == null) 514 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 515 this.gender.setValue(value); 516 } 517 return this; 518 } 519 520 /** 521 * @return {@link #organization} (Organization on behalf of which the contact is 522 * acting or for which the contact is working.) 523 */ 524 public Reference getOrganization() { 525 if (this.organization == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create ContactComponent.organization"); 528 else if (Configuration.doAutoCreate()) 529 this.organization = new Reference(); // cc 530 return this.organization; 531 } 532 533 public boolean hasOrganization() { 534 return this.organization != null && !this.organization.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #organization} (Organization on behalf of which the 539 * contact is acting or for which the contact is working.) 540 */ 541 public ContactComponent setOrganization(Reference value) { 542 this.organization = value; 543 return this; 544 } 545 546 /** 547 * @return {@link #organization} The actual object that is the target of the 548 * reference. The reference library doesn't populate this, but you can 549 * use it to hold the resource if you resolve it. (Organization on 550 * behalf of which the contact is acting or for which the contact is 551 * working.) 552 */ 553 public Organization getOrganizationTarget() { 554 if (this.organizationTarget == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create ContactComponent.organization"); 557 else if (Configuration.doAutoCreate()) 558 this.organizationTarget = new Organization(); // aa 559 return this.organizationTarget; 560 } 561 562 /** 563 * @param value {@link #organization} The actual object that is the target of 564 * the reference. The reference library doesn't use these, but you 565 * can use it to hold the resource if you resolve it. (Organization 566 * on behalf of which the contact is acting or for which the 567 * contact is working.) 568 */ 569 public ContactComponent setOrganizationTarget(Organization value) { 570 this.organizationTarget = value; 571 return this; 572 } 573 574 /** 575 * @return {@link #period} (The period during which this contact person or 576 * organization is valid to be contacted relating to this patient.) 577 */ 578 public Period getPeriod() { 579 if (this.period == null) 580 if (Configuration.errorOnAutoCreate()) 581 throw new Error("Attempt to auto-create ContactComponent.period"); 582 else if (Configuration.doAutoCreate()) 583 this.period = new Period(); // cc 584 return this.period; 585 } 586 587 public boolean hasPeriod() { 588 return this.period != null && !this.period.isEmpty(); 589 } 590 591 /** 592 * @param value {@link #period} (The period during which this contact person or 593 * organization is valid to be contacted relating to this patient.) 594 */ 595 public ContactComponent setPeriod(Period value) { 596 this.period = value; 597 return this; 598 } 599 600 protected void listChildren(List<Property> children) { 601 super.listChildren(children); 602 children.add(new Property("relationship", "CodeableConcept", 603 "The nature of the relationship between the patient and the contact person.", 0, java.lang.Integer.MAX_VALUE, 604 relationship)); 605 children.add(new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name)); 606 children.add(new Property("telecom", "ContactPoint", 607 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 608 java.lang.Integer.MAX_VALUE, telecom)); 609 children.add(new Property("address", "Address", "Address for the contact person.", 0, 1, address)); 610 children.add(new Property("gender", "code", 611 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 612 0, 1, gender)); 613 children.add(new Property("organization", "Reference(Organization)", 614 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, 615 organization)); 616 children.add(new Property("period", "Period", 617 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 618 0, 1, period)); 619 } 620 621 @Override 622 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 623 switch (_hash) { 624 case -261851592: 625 /* relationship */ return new Property("relationship", "CodeableConcept", 626 "The nature of the relationship between the patient and the contact person.", 0, 627 java.lang.Integer.MAX_VALUE, relationship); 628 case 3373707: 629 /* name */ return new Property("name", "HumanName", "A name associated with the contact person.", 0, 1, name); 630 case -1429363305: 631 /* telecom */ return new Property("telecom", "ContactPoint", 632 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 633 java.lang.Integer.MAX_VALUE, telecom); 634 case -1147692044: 635 /* address */ return new Property("address", "Address", "Address for the contact person.", 0, 1, address); 636 case -1249512767: 637 /* gender */ return new Property("gender", "code", 638 "Administrative Gender - the gender that the contact person is considered to have for administration and record keeping purposes.", 639 0, 1, gender); 640 case 1178922291: 641 /* organization */ return new Property("organization", "Reference(Organization)", 642 "Organization on behalf of which the contact is acting or for which the contact is working.", 0, 1, 643 organization); 644 case -991726143: 645 /* period */ return new Property("period", "Period", 646 "The period during which this contact person or organization is valid to be contacted relating to this patient.", 647 0, 1, period); 648 default: 649 return super.getNamedProperty(_hash, _name, _checkValid); 650 } 651 652 } 653 654 @Override 655 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 656 switch (hash) { 657 case -261851592: 658 /* relationship */ return this.relationship == null ? new Base[0] 659 : this.relationship.toArray(new Base[this.relationship.size()]); // CodeableConcept 660 case 3373707: 661 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // HumanName 662 case -1429363305: 663 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 664 case -1147692044: 665 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 666 case -1249512767: 667 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 668 case 1178922291: 669 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 670 case -991726143: 671 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 672 default: 673 return super.getProperty(hash, name, checkValid); 674 } 675 676 } 677 678 @Override 679 public Base setProperty(int hash, String name, Base value) throws FHIRException { 680 switch (hash) { 681 case -261851592: // relationship 682 this.getRelationship().add(castToCodeableConcept(value)); // CodeableConcept 683 return value; 684 case 3373707: // name 685 this.name = castToHumanName(value); // HumanName 686 return value; 687 case -1429363305: // telecom 688 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 689 return value; 690 case -1147692044: // address 691 this.address = castToAddress(value); // Address 692 return value; 693 case -1249512767: // gender 694 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 695 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 696 return value; 697 case 1178922291: // organization 698 this.organization = castToReference(value); // Reference 699 return value; 700 case -991726143: // period 701 this.period = castToPeriod(value); // Period 702 return value; 703 default: 704 return super.setProperty(hash, name, value); 705 } 706 707 } 708 709 @Override 710 public Base setProperty(String name, Base value) throws FHIRException { 711 if (name.equals("relationship")) { 712 this.getRelationship().add(castToCodeableConcept(value)); 713 } else if (name.equals("name")) { 714 this.name = castToHumanName(value); // HumanName 715 } else if (name.equals("telecom")) { 716 this.getTelecom().add(castToContactPoint(value)); 717 } else if (name.equals("address")) { 718 this.address = castToAddress(value); // Address 719 } else if (name.equals("gender")) { 720 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 721 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 722 } else if (name.equals("organization")) { 723 this.organization = castToReference(value); // Reference 724 } else if (name.equals("period")) { 725 this.period = castToPeriod(value); // Period 726 } else 727 return super.setProperty(name, value); 728 return value; 729 } 730 731 @Override 732 public void removeChild(String name, Base value) throws FHIRException { 733 if (name.equals("relationship")) { 734 this.getRelationship().remove(castToCodeableConcept(value)); 735 } else if (name.equals("name")) { 736 this.name = null; 737 } else if (name.equals("telecom")) { 738 this.getTelecom().remove(castToContactPoint(value)); 739 } else if (name.equals("address")) { 740 this.address = null; 741 } else if (name.equals("gender")) { 742 this.gender = null; 743 } else if (name.equals("organization")) { 744 this.organization = null; 745 } else if (name.equals("period")) { 746 this.period = null; 747 } else 748 super.removeChild(name, value); 749 750 } 751 752 @Override 753 public Base makeProperty(int hash, String name) throws FHIRException { 754 switch (hash) { 755 case -261851592: 756 return addRelationship(); 757 case 3373707: 758 return getName(); 759 case -1429363305: 760 return addTelecom(); 761 case -1147692044: 762 return getAddress(); 763 case -1249512767: 764 return getGenderElement(); 765 case 1178922291: 766 return getOrganization(); 767 case -991726143: 768 return getPeriod(); 769 default: 770 return super.makeProperty(hash, name); 771 } 772 773 } 774 775 @Override 776 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 777 switch (hash) { 778 case -261851592: 779 /* relationship */ return new String[] { "CodeableConcept" }; 780 case 3373707: 781 /* name */ return new String[] { "HumanName" }; 782 case -1429363305: 783 /* telecom */ return new String[] { "ContactPoint" }; 784 case -1147692044: 785 /* address */ return new String[] { "Address" }; 786 case -1249512767: 787 /* gender */ return new String[] { "code" }; 788 case 1178922291: 789 /* organization */ return new String[] { "Reference" }; 790 case -991726143: 791 /* period */ return new String[] { "Period" }; 792 default: 793 return super.getTypesForProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public Base addChild(String name) throws FHIRException { 800 if (name.equals("relationship")) { 801 return addRelationship(); 802 } else if (name.equals("name")) { 803 this.name = new HumanName(); 804 return this.name; 805 } else if (name.equals("telecom")) { 806 return addTelecom(); 807 } else if (name.equals("address")) { 808 this.address = new Address(); 809 return this.address; 810 } else if (name.equals("gender")) { 811 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 812 } else if (name.equals("organization")) { 813 this.organization = new Reference(); 814 return this.organization; 815 } else if (name.equals("period")) { 816 this.period = new Period(); 817 return this.period; 818 } else 819 return super.addChild(name); 820 } 821 822 public ContactComponent copy() { 823 ContactComponent dst = new ContactComponent(); 824 copyValues(dst); 825 return dst; 826 } 827 828 public void copyValues(ContactComponent dst) { 829 super.copyValues(dst); 830 if (relationship != null) { 831 dst.relationship = new ArrayList<CodeableConcept>(); 832 for (CodeableConcept i : relationship) 833 dst.relationship.add(i.copy()); 834 } 835 ; 836 dst.name = name == null ? null : name.copy(); 837 if (telecom != null) { 838 dst.telecom = new ArrayList<ContactPoint>(); 839 for (ContactPoint i : telecom) 840 dst.telecom.add(i.copy()); 841 } 842 ; 843 dst.address = address == null ? null : address.copy(); 844 dst.gender = gender == null ? null : gender.copy(); 845 dst.organization = organization == null ? null : organization.copy(); 846 dst.period = period == null ? null : period.copy(); 847 } 848 849 @Override 850 public boolean equalsDeep(Base other_) { 851 if (!super.equalsDeep(other_)) 852 return false; 853 if (!(other_ instanceof ContactComponent)) 854 return false; 855 ContactComponent o = (ContactComponent) other_; 856 return compareDeep(relationship, o.relationship, true) && compareDeep(name, o.name, true) 857 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true) 858 && compareDeep(gender, o.gender, true) && compareDeep(organization, o.organization, true) 859 && compareDeep(period, o.period, true); 860 } 861 862 @Override 863 public boolean equalsShallow(Base other_) { 864 if (!super.equalsShallow(other_)) 865 return false; 866 if (!(other_ instanceof ContactComponent)) 867 return false; 868 ContactComponent o = (ContactComponent) other_; 869 return compareValues(gender, o.gender, true); 870 } 871 872 public boolean isEmpty() { 873 return super.isEmpty() 874 && ca.uhn.fhir.util.ElementUtil.isEmpty(relationship, name, telecom, address, gender, organization, period); 875 } 876 877 public String fhirType() { 878 return "Patient.contact"; 879 880 } 881 882 } 883 884 @Block() 885 public static class PatientCommunicationComponent extends BackboneElement implements IBaseBackboneElement { 886 /** 887 * The ISO-639-1 alpha 2 code in lower case for the language, optionally 888 * followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper 889 * case; e.g. "en" for English, or "en-US" for American English versus "en-EN" 890 * for England English. 891 */ 892 @Child(name = "language", type = { 893 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 894 @Description(shortDefinition = "The language which can be used to communicate with the patient about his or her health", formalDefinition = "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.") 895 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 896 protected CodeableConcept language; 897 898 /** 899 * Indicates whether or not the patient prefers this language (over other 900 * languages he masters up a certain level). 901 */ 902 @Child(name = "preferred", type = { 903 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 904 @Description(shortDefinition = "Language preference indicator", formalDefinition = "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).") 905 protected BooleanType preferred; 906 907 private static final long serialVersionUID = 633792918L; 908 909 /** 910 * Constructor 911 */ 912 public PatientCommunicationComponent() { 913 super(); 914 } 915 916 /** 917 * Constructor 918 */ 919 public PatientCommunicationComponent(CodeableConcept language) { 920 super(); 921 this.language = language; 922 } 923 924 /** 925 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the 926 * language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 927 * code for the region in upper case; e.g. "en" for English, or "en-US" 928 * for American English versus "en-EN" for England English.) 929 */ 930 public CodeableConcept getLanguage() { 931 if (this.language == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create PatientCommunicationComponent.language"); 934 else if (Configuration.doAutoCreate()) 935 this.language = new CodeableConcept(); // cc 936 return this.language; 937 } 938 939 public boolean hasLanguage() { 940 return this.language != null && !this.language.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for 945 * the language, optionally followed by a hyphen and the ISO-3166-1 946 * alpha 2 code for the region in upper case; e.g. "en" for 947 * English, or "en-US" for American English versus "en-EN" for 948 * England English.) 949 */ 950 public PatientCommunicationComponent setLanguage(CodeableConcept value) { 951 this.language = value; 952 return this; 953 } 954 955 /** 956 * @return {@link #preferred} (Indicates whether or not the patient prefers this 957 * language (over other languages he masters up a certain level).). This 958 * is the underlying object with id, value and extensions. The accessor 959 * "getPreferred" gives direct access to the value 960 */ 961 public BooleanType getPreferredElement() { 962 if (this.preferred == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create PatientCommunicationComponent.preferred"); 965 else if (Configuration.doAutoCreate()) 966 this.preferred = new BooleanType(); // bb 967 return this.preferred; 968 } 969 970 public boolean hasPreferredElement() { 971 return this.preferred != null && !this.preferred.isEmpty(); 972 } 973 974 public boolean hasPreferred() { 975 return this.preferred != null && !this.preferred.isEmpty(); 976 } 977 978 /** 979 * @param value {@link #preferred} (Indicates whether or not the patient prefers 980 * this language (over other languages he masters up a certain 981 * level).). This is the underlying object with id, value and 982 * extensions. The accessor "getPreferred" gives direct access to 983 * the value 984 */ 985 public PatientCommunicationComponent setPreferredElement(BooleanType value) { 986 this.preferred = value; 987 return this; 988 } 989 990 /** 991 * @return Indicates whether or not the patient prefers this language (over 992 * other languages he masters up a certain level). 993 */ 994 public boolean getPreferred() { 995 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 996 } 997 998 /** 999 * @param value Indicates whether or not the patient prefers this language (over 1000 * other languages he masters up a certain level). 1001 */ 1002 public PatientCommunicationComponent setPreferred(boolean value) { 1003 if (this.preferred == null) 1004 this.preferred = new BooleanType(); 1005 this.preferred.setValue(value); 1006 return this; 1007 } 1008 1009 protected void listChildren(List<Property> children) { 1010 super.listChildren(children); 1011 children.add(new Property("language", "CodeableConcept", 1012 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 1013 0, 1, language)); 1014 children.add(new Property("preferred", "boolean", 1015 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1016 0, 1, preferred)); 1017 } 1018 1019 @Override 1020 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1021 switch (_hash) { 1022 case -1613589672: 1023 /* language */ return new Property("language", "CodeableConcept", 1024 "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 1025 0, 1, language); 1026 case -1294005119: 1027 /* preferred */ return new Property("preferred", "boolean", 1028 "Indicates whether or not the patient prefers this language (over other languages he masters up a certain level).", 1029 0, 1, preferred); 1030 default: 1031 return super.getNamedProperty(_hash, _name, _checkValid); 1032 } 1033 1034 } 1035 1036 @Override 1037 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1038 switch (hash) { 1039 case -1613589672: 1040 /* language */ return this.language == null ? new Base[0] : new Base[] { this.language }; // CodeableConcept 1041 case -1294005119: 1042 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 1043 default: 1044 return super.getProperty(hash, name, checkValid); 1045 } 1046 1047 } 1048 1049 @Override 1050 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1051 switch (hash) { 1052 case -1613589672: // language 1053 this.language = castToCodeableConcept(value); // CodeableConcept 1054 return value; 1055 case -1294005119: // preferred 1056 this.preferred = castToBoolean(value); // BooleanType 1057 return value; 1058 default: 1059 return super.setProperty(hash, name, value); 1060 } 1061 1062 } 1063 1064 @Override 1065 public Base setProperty(String name, Base value) throws FHIRException { 1066 if (name.equals("language")) { 1067 this.language = castToCodeableConcept(value); // CodeableConcept 1068 } else if (name.equals("preferred")) { 1069 this.preferred = castToBoolean(value); // BooleanType 1070 } else 1071 return super.setProperty(name, value); 1072 return value; 1073 } 1074 1075 @Override 1076 public void removeChild(String name, Base value) throws FHIRException { 1077 if (name.equals("language")) { 1078 this.language = null; 1079 } else if (name.equals("preferred")) { 1080 this.preferred = null; 1081 } else 1082 super.removeChild(name, value); 1083 1084 } 1085 1086 @Override 1087 public Base makeProperty(int hash, String name) throws FHIRException { 1088 switch (hash) { 1089 case -1613589672: 1090 return getLanguage(); 1091 case -1294005119: 1092 return getPreferredElement(); 1093 default: 1094 return super.makeProperty(hash, name); 1095 } 1096 1097 } 1098 1099 @Override 1100 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1101 switch (hash) { 1102 case -1613589672: 1103 /* language */ return new String[] { "CodeableConcept" }; 1104 case -1294005119: 1105 /* preferred */ return new String[] { "boolean" }; 1106 default: 1107 return super.getTypesForProperty(hash, name); 1108 } 1109 1110 } 1111 1112 @Override 1113 public Base addChild(String name) throws FHIRException { 1114 if (name.equals("language")) { 1115 this.language = new CodeableConcept(); 1116 return this.language; 1117 } else if (name.equals("preferred")) { 1118 throw new FHIRException("Cannot call addChild on a singleton property Patient.preferred"); 1119 } else 1120 return super.addChild(name); 1121 } 1122 1123 public PatientCommunicationComponent copy() { 1124 PatientCommunicationComponent dst = new PatientCommunicationComponent(); 1125 copyValues(dst); 1126 return dst; 1127 } 1128 1129 public void copyValues(PatientCommunicationComponent dst) { 1130 super.copyValues(dst); 1131 dst.language = language == null ? null : language.copy(); 1132 dst.preferred = preferred == null ? null : preferred.copy(); 1133 } 1134 1135 @Override 1136 public boolean equalsDeep(Base other_) { 1137 if (!super.equalsDeep(other_)) 1138 return false; 1139 if (!(other_ instanceof PatientCommunicationComponent)) 1140 return false; 1141 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1142 return compareDeep(language, o.language, true) && compareDeep(preferred, o.preferred, true); 1143 } 1144 1145 @Override 1146 public boolean equalsShallow(Base other_) { 1147 if (!super.equalsShallow(other_)) 1148 return false; 1149 if (!(other_ instanceof PatientCommunicationComponent)) 1150 return false; 1151 PatientCommunicationComponent o = (PatientCommunicationComponent) other_; 1152 return compareValues(preferred, o.preferred, true); 1153 } 1154 1155 public boolean isEmpty() { 1156 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, preferred); 1157 } 1158 1159 public String fhirType() { 1160 return "Patient.communication"; 1161 1162 } 1163 1164 } 1165 1166 @Block() 1167 public static class PatientLinkComponent extends BackboneElement implements IBaseBackboneElement { 1168 /** 1169 * The other patient resource that the link refers to. 1170 */ 1171 @Child(name = "other", type = { Patient.class, 1172 RelatedPerson.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1173 @Description(shortDefinition = "The other patient or related person resource that the link refers to", formalDefinition = "The other patient resource that the link refers to.") 1174 protected Reference other; 1175 1176 /** 1177 * The actual object that is the target of the reference (The other patient 1178 * resource that the link refers to.) 1179 */ 1180 protected Resource otherTarget; 1181 1182 /** 1183 * The type of link between this patient resource and another patient resource. 1184 */ 1185 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1186 @Description(shortDefinition = "replaced-by | replaces | refer | seealso", formalDefinition = "The type of link between this patient resource and another patient resource.") 1187 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/link-type") 1188 protected Enumeration<LinkType> type; 1189 1190 private static final long serialVersionUID = 1083576633L; 1191 1192 /** 1193 * Constructor 1194 */ 1195 public PatientLinkComponent() { 1196 super(); 1197 } 1198 1199 /** 1200 * Constructor 1201 */ 1202 public PatientLinkComponent(Reference other, Enumeration<LinkType> type) { 1203 super(); 1204 this.other = other; 1205 this.type = type; 1206 } 1207 1208 /** 1209 * @return {@link #other} (The other patient resource that the link refers to.) 1210 */ 1211 public Reference getOther() { 1212 if (this.other == null) 1213 if (Configuration.errorOnAutoCreate()) 1214 throw new Error("Attempt to auto-create PatientLinkComponent.other"); 1215 else if (Configuration.doAutoCreate()) 1216 this.other = new Reference(); // cc 1217 return this.other; 1218 } 1219 1220 public boolean hasOther() { 1221 return this.other != null && !this.other.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #other} (The other patient resource that the link refers 1226 * to.) 1227 */ 1228 public PatientLinkComponent setOther(Reference value) { 1229 this.other = value; 1230 return this; 1231 } 1232 1233 /** 1234 * @return {@link #other} The actual object that is the target of the reference. 1235 * The reference library doesn't populate this, but you can use it to 1236 * hold the resource if you resolve it. (The other patient resource that 1237 * the link refers to.) 1238 */ 1239 public Resource getOtherTarget() { 1240 return this.otherTarget; 1241 } 1242 1243 /** 1244 * @param value {@link #other} The actual object that is the target of the 1245 * reference. The reference library doesn't use these, but you can 1246 * use it to hold the resource if you resolve it. (The other 1247 * patient resource that the link refers to.) 1248 */ 1249 public PatientLinkComponent setOtherTarget(Resource value) { 1250 this.otherTarget = value; 1251 return this; 1252 } 1253 1254 /** 1255 * @return {@link #type} (The type of link between this patient resource and 1256 * another patient resource.). This is the underlying object with id, 1257 * value and extensions. The accessor "getType" gives direct access to 1258 * the value 1259 */ 1260 public Enumeration<LinkType> getTypeElement() { 1261 if (this.type == null) 1262 if (Configuration.errorOnAutoCreate()) 1263 throw new Error("Attempt to auto-create PatientLinkComponent.type"); 1264 else if (Configuration.doAutoCreate()) 1265 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); // bb 1266 return this.type; 1267 } 1268 1269 public boolean hasTypeElement() { 1270 return this.type != null && !this.type.isEmpty(); 1271 } 1272 1273 public boolean hasType() { 1274 return this.type != null && !this.type.isEmpty(); 1275 } 1276 1277 /** 1278 * @param value {@link #type} (The type of link between this patient resource 1279 * and another patient resource.). This is the underlying object 1280 * with id, value and extensions. The accessor "getType" gives 1281 * direct access to the value 1282 */ 1283 public PatientLinkComponent setTypeElement(Enumeration<LinkType> value) { 1284 this.type = value; 1285 return this; 1286 } 1287 1288 /** 1289 * @return The type of link between this patient resource and another patient 1290 * resource. 1291 */ 1292 public LinkType getType() { 1293 return this.type == null ? null : this.type.getValue(); 1294 } 1295 1296 /** 1297 * @param value The type of link between this patient resource and another 1298 * patient resource. 1299 */ 1300 public PatientLinkComponent setType(LinkType value) { 1301 if (this.type == null) 1302 this.type = new Enumeration<LinkType>(new LinkTypeEnumFactory()); 1303 this.type.setValue(value); 1304 return this; 1305 } 1306 1307 protected void listChildren(List<Property> children) { 1308 super.listChildren(children); 1309 children.add(new Property("other", "Reference(Patient|RelatedPerson)", 1310 "The other patient resource that the link refers to.", 0, 1, other)); 1311 children.add(new Property("type", "code", 1312 "The type of link between this patient resource and another patient resource.", 0, 1, type)); 1313 } 1314 1315 @Override 1316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1317 switch (_hash) { 1318 case 106069776: 1319 /* other */ return new Property("other", "Reference(Patient|RelatedPerson)", 1320 "The other patient resource that the link refers to.", 0, 1, other); 1321 case 3575610: 1322 /* type */ return new Property("type", "code", 1323 "The type of link between this patient resource and another patient resource.", 0, 1, type); 1324 default: 1325 return super.getNamedProperty(_hash, _name, _checkValid); 1326 } 1327 1328 } 1329 1330 @Override 1331 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1332 switch (hash) { 1333 case 106069776: 1334 /* other */ return this.other == null ? new Base[0] : new Base[] { this.other }; // Reference 1335 case 3575610: 1336 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<LinkType> 1337 default: 1338 return super.getProperty(hash, name, checkValid); 1339 } 1340 1341 } 1342 1343 @Override 1344 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1345 switch (hash) { 1346 case 106069776: // other 1347 this.other = castToReference(value); // Reference 1348 return value; 1349 case 3575610: // type 1350 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1351 this.type = (Enumeration) value; // Enumeration<LinkType> 1352 return value; 1353 default: 1354 return super.setProperty(hash, name, value); 1355 } 1356 1357 } 1358 1359 @Override 1360 public Base setProperty(String name, Base value) throws FHIRException { 1361 if (name.equals("other")) { 1362 this.other = castToReference(value); // Reference 1363 } else if (name.equals("type")) { 1364 value = new LinkTypeEnumFactory().fromType(castToCode(value)); 1365 this.type = (Enumeration) value; // Enumeration<LinkType> 1366 } else 1367 return super.setProperty(name, value); 1368 return value; 1369 } 1370 1371 @Override 1372 public void removeChild(String name, Base value) throws FHIRException { 1373 if (name.equals("other")) { 1374 this.other = null; 1375 } else if (name.equals("type")) { 1376 this.type = null; 1377 } else 1378 super.removeChild(name, value); 1379 1380 } 1381 1382 @Override 1383 public Base makeProperty(int hash, String name) throws FHIRException { 1384 switch (hash) { 1385 case 106069776: 1386 return getOther(); 1387 case 3575610: 1388 return getTypeElement(); 1389 default: 1390 return super.makeProperty(hash, name); 1391 } 1392 1393 } 1394 1395 @Override 1396 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1397 switch (hash) { 1398 case 106069776: 1399 /* other */ return new String[] { "Reference" }; 1400 case 3575610: 1401 /* type */ return new String[] { "code" }; 1402 default: 1403 return super.getTypesForProperty(hash, name); 1404 } 1405 1406 } 1407 1408 @Override 1409 public Base addChild(String name) throws FHIRException { 1410 if (name.equals("other")) { 1411 this.other = new Reference(); 1412 return this.other; 1413 } else if (name.equals("type")) { 1414 throw new FHIRException("Cannot call addChild on a singleton property Patient.type"); 1415 } else 1416 return super.addChild(name); 1417 } 1418 1419 public PatientLinkComponent copy() { 1420 PatientLinkComponent dst = new PatientLinkComponent(); 1421 copyValues(dst); 1422 return dst; 1423 } 1424 1425 public void copyValues(PatientLinkComponent dst) { 1426 super.copyValues(dst); 1427 dst.other = other == null ? null : other.copy(); 1428 dst.type = type == null ? null : type.copy(); 1429 } 1430 1431 @Override 1432 public boolean equalsDeep(Base other_) { 1433 if (!super.equalsDeep(other_)) 1434 return false; 1435 if (!(other_ instanceof PatientLinkComponent)) 1436 return false; 1437 PatientLinkComponent o = (PatientLinkComponent) other_; 1438 return compareDeep(other, o.other, true) && compareDeep(type, o.type, true); 1439 } 1440 1441 @Override 1442 public boolean equalsShallow(Base other_) { 1443 if (!super.equalsShallow(other_)) 1444 return false; 1445 if (!(other_ instanceof PatientLinkComponent)) 1446 return false; 1447 PatientLinkComponent o = (PatientLinkComponent) other_; 1448 return compareValues(type, o.type, true); 1449 } 1450 1451 public boolean isEmpty() { 1452 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(other, type); 1453 } 1454 1455 public String fhirType() { 1456 return "Patient.link"; 1457 1458 } 1459 1460 } 1461 1462 /** 1463 * An identifier for this patient. 1464 */ 1465 @Child(name = "identifier", type = { 1466 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1467 @Description(shortDefinition = "An identifier for this patient", formalDefinition = "An identifier for this patient.") 1468 protected List<Identifier> identifier; 1469 1470 /** 1471 * Whether this patient record is in active use. Many systems use this property 1472 * to mark as non-current patients, such as those that have not been seen for a 1473 * period of time based on an organization's business rules. 1474 * 1475 * It is often used to filter patient lists to exclude inactive patients 1476 * 1477 * Deceased patients may also be marked as inactive for the same reasons, but 1478 * may be active for some time after death. 1479 */ 1480 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1481 @Description(shortDefinition = "Whether this patient's record is in active use", formalDefinition = "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.") 1482 protected BooleanType active; 1483 1484 /** 1485 * A name associated with the individual. 1486 */ 1487 @Child(name = "name", type = { 1488 HumanName.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1489 @Description(shortDefinition = "A name associated with the patient", formalDefinition = "A name associated with the individual.") 1490 protected List<HumanName> name; 1491 1492 /** 1493 * A contact detail (e.g. a telephone number or an email address) by which the 1494 * individual may be contacted. 1495 */ 1496 @Child(name = "telecom", type = { 1497 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1498 @Description(shortDefinition = "A contact detail for the individual", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.") 1499 protected List<ContactPoint> telecom; 1500 1501 /** 1502 * Administrative Gender - the gender that the patient is considered to have for 1503 * administration and record keeping purposes. 1504 */ 1505 @Child(name = "gender", type = { CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1506 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.") 1507 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 1508 protected Enumeration<AdministrativeGender> gender; 1509 1510 /** 1511 * The date of birth for the individual. 1512 */ 1513 @Child(name = "birthDate", type = { DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1514 @Description(shortDefinition = "The date of birth for the individual", formalDefinition = "The date of birth for the individual.") 1515 protected DateType birthDate; 1516 1517 /** 1518 * Indicates if the individual is deceased or not. 1519 */ 1520 @Child(name = "deceased", type = { BooleanType.class, 1521 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1522 @Description(shortDefinition = "Indicates if the individual is deceased or not", formalDefinition = "Indicates if the individual is deceased or not.") 1523 protected Type deceased; 1524 1525 /** 1526 * An address for the individual. 1527 */ 1528 @Child(name = "address", type = { 1529 Address.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1530 @Description(shortDefinition = "An address for the individual", formalDefinition = "An address for the individual.") 1531 protected List<Address> address; 1532 1533 /** 1534 * This field contains a patient's most recent marital (civil) status. 1535 */ 1536 @Child(name = "maritalStatus", type = { 1537 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1538 @Description(shortDefinition = "Marital (civil) status of a patient", formalDefinition = "This field contains a patient's most recent marital (civil) status.") 1539 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/marital-status") 1540 protected CodeableConcept maritalStatus; 1541 1542 /** 1543 * Indicates whether the patient is part of a multiple (boolean) or indicates 1544 * the actual birth order (integer). 1545 */ 1546 @Child(name = "multipleBirth", type = { BooleanType.class, 1547 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1548 @Description(shortDefinition = "Whether patient is part of a multiple birth", formalDefinition = "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).") 1549 protected Type multipleBirth; 1550 1551 /** 1552 * Image of the patient. 1553 */ 1554 @Child(name = "photo", type = { 1555 Attachment.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1556 @Description(shortDefinition = "Image of the patient", formalDefinition = "Image of the patient.") 1557 protected List<Attachment> photo; 1558 1559 /** 1560 * A contact party (e.g. guardian, partner, friend) for the patient. 1561 */ 1562 @Child(name = "contact", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1563 @Description(shortDefinition = "A contact party (e.g. guardian, partner, friend) for the patient", formalDefinition = "A contact party (e.g. guardian, partner, friend) for the patient.") 1564 protected List<ContactComponent> contact; 1565 1566 /** 1567 * A language which may be used to communicate with the patient about his or her 1568 * health. 1569 */ 1570 @Child(name = "communication", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1571 @Description(shortDefinition = "A language which may be used to communicate with the patient about his or her health", formalDefinition = "A language which may be used to communicate with the patient about his or her health.") 1572 protected List<PatientCommunicationComponent> communication; 1573 1574 /** 1575 * Patient's nominated care provider. 1576 */ 1577 @Child(name = "generalPractitioner", type = { Organization.class, Practitioner.class, 1578 PractitionerRole.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1579 @Description(shortDefinition = "Patient's nominated primary care provider", formalDefinition = "Patient's nominated care provider.") 1580 protected List<Reference> generalPractitioner; 1581 /** 1582 * The actual objects that are the target of the reference (Patient's nominated 1583 * care provider.) 1584 */ 1585 protected List<Resource> generalPractitionerTarget; 1586 1587 /** 1588 * Organization that is the custodian of the patient record. 1589 */ 1590 @Child(name = "managingOrganization", type = { 1591 Organization.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1592 @Description(shortDefinition = "Organization that is the custodian of the patient record", formalDefinition = "Organization that is the custodian of the patient record.") 1593 protected Reference managingOrganization; 1594 1595 /** 1596 * The actual object that is the target of the reference (Organization that is 1597 * the custodian of the patient record.) 1598 */ 1599 protected Organization managingOrganizationTarget; 1600 1601 /** 1602 * Link to another patient resource that concerns the same actual patient. 1603 */ 1604 @Child(name = "link", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = true) 1605 @Description(shortDefinition = "Link to another patient resource that concerns the same actual person", formalDefinition = "Link to another patient resource that concerns the same actual patient.") 1606 protected List<PatientLinkComponent> link; 1607 1608 private static final long serialVersionUID = 2138656939L; 1609 1610 /** 1611 * Constructor 1612 */ 1613 public Patient() { 1614 super(); 1615 } 1616 1617 /** 1618 * @return {@link #identifier} (An identifier for this patient.) 1619 */ 1620 public List<Identifier> getIdentifier() { 1621 if (this.identifier == null) 1622 this.identifier = new ArrayList<Identifier>(); 1623 return this.identifier; 1624 } 1625 1626 /** 1627 * @return Returns a reference to <code>this</code> for easy method chaining 1628 */ 1629 public Patient setIdentifier(List<Identifier> theIdentifier) { 1630 this.identifier = theIdentifier; 1631 return this; 1632 } 1633 1634 public boolean hasIdentifier() { 1635 if (this.identifier == null) 1636 return false; 1637 for (Identifier item : this.identifier) 1638 if (!item.isEmpty()) 1639 return true; 1640 return false; 1641 } 1642 1643 public Identifier addIdentifier() { // 3 1644 Identifier t = new Identifier(); 1645 if (this.identifier == null) 1646 this.identifier = new ArrayList<Identifier>(); 1647 this.identifier.add(t); 1648 return t; 1649 } 1650 1651 public Patient addIdentifier(Identifier t) { // 3 1652 if (t == null) 1653 return this; 1654 if (this.identifier == null) 1655 this.identifier = new ArrayList<Identifier>(); 1656 this.identifier.add(t); 1657 return this; 1658 } 1659 1660 /** 1661 * @return The first repetition of repeating field {@link #identifier}, creating 1662 * it if it does not already exist 1663 */ 1664 public Identifier getIdentifierFirstRep() { 1665 if (getIdentifier().isEmpty()) { 1666 addIdentifier(); 1667 } 1668 return getIdentifier().get(0); 1669 } 1670 1671 /** 1672 * @return {@link #active} (Whether this patient record is in active use. Many 1673 * systems use this property to mark as non-current patients, such as 1674 * those that have not been seen for a period of time based on an 1675 * organization's business rules. 1676 * 1677 * It is often used to filter patient lists to exclude inactive patients 1678 * 1679 * Deceased patients may also be marked as inactive for the same 1680 * reasons, but may be active for some time after death.). This is the 1681 * underlying object with id, value and extensions. The accessor 1682 * "getActive" gives direct access to the value 1683 */ 1684 public BooleanType getActiveElement() { 1685 if (this.active == null) 1686 if (Configuration.errorOnAutoCreate()) 1687 throw new Error("Attempt to auto-create Patient.active"); 1688 else if (Configuration.doAutoCreate()) 1689 this.active = new BooleanType(); // bb 1690 return this.active; 1691 } 1692 1693 public boolean hasActiveElement() { 1694 return this.active != null && !this.active.isEmpty(); 1695 } 1696 1697 public boolean hasActive() { 1698 return this.active != null && !this.active.isEmpty(); 1699 } 1700 1701 /** 1702 * @param value {@link #active} (Whether this patient record is in active use. 1703 * Many systems use this property to mark as non-current patients, 1704 * such as those that have not been seen for a period of time based 1705 * on an organization's business rules. 1706 * 1707 * It is often used to filter patient lists to exclude inactive 1708 * patients 1709 * 1710 * Deceased patients may also be marked as inactive for the same 1711 * reasons, but may be active for some time after death.). This is 1712 * the underlying object with id, value and extensions. The 1713 * accessor "getActive" gives direct access to the value 1714 */ 1715 public Patient setActiveElement(BooleanType value) { 1716 this.active = value; 1717 return this; 1718 } 1719 1720 /** 1721 * @return Whether this patient record is in active use. Many systems use this 1722 * property to mark as non-current patients, such as those that have not 1723 * been seen for a period of time based on an organization's business 1724 * rules. 1725 * 1726 * It is often used to filter patient lists to exclude inactive patients 1727 * 1728 * Deceased patients may also be marked as inactive for the same 1729 * reasons, but may be active for some time after death. 1730 */ 1731 public boolean getActive() { 1732 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1733 } 1734 1735 /** 1736 * @param value Whether this patient record is in active use. Many systems use 1737 * this property to mark as non-current patients, such as those 1738 * that have not been seen for a period of time based on an 1739 * organization's business rules. 1740 * 1741 * It is often used to filter patient lists to exclude inactive 1742 * patients 1743 * 1744 * Deceased patients may also be marked as inactive for the same 1745 * reasons, but may be active for some time after death. 1746 */ 1747 public Patient setActive(boolean value) { 1748 if (this.active == null) 1749 this.active = new BooleanType(); 1750 this.active.setValue(value); 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #name} (A name associated with the individual.) 1756 */ 1757 public List<HumanName> getName() { 1758 if (this.name == null) 1759 this.name = new ArrayList<HumanName>(); 1760 return this.name; 1761 } 1762 1763 /** 1764 * @return Returns a reference to <code>this</code> for easy method chaining 1765 */ 1766 public Patient setName(List<HumanName> theName) { 1767 this.name = theName; 1768 return this; 1769 } 1770 1771 public boolean hasName() { 1772 if (this.name == null) 1773 return false; 1774 for (HumanName item : this.name) 1775 if (!item.isEmpty()) 1776 return true; 1777 return false; 1778 } 1779 1780 public HumanName addName() { // 3 1781 HumanName t = new HumanName(); 1782 if (this.name == null) 1783 this.name = new ArrayList<HumanName>(); 1784 this.name.add(t); 1785 return t; 1786 } 1787 1788 public Patient addName(HumanName t) { // 3 1789 if (t == null) 1790 return this; 1791 if (this.name == null) 1792 this.name = new ArrayList<HumanName>(); 1793 this.name.add(t); 1794 return this; 1795 } 1796 1797 /** 1798 * @return The first repetition of repeating field {@link #name}, creating it if 1799 * it does not already exist 1800 */ 1801 public HumanName getNameFirstRep() { 1802 if (getName().isEmpty()) { 1803 addName(); 1804 } 1805 return getName().get(0); 1806 } 1807 1808 /** 1809 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 1810 * email address) by which the individual may be contacted.) 1811 */ 1812 public List<ContactPoint> getTelecom() { 1813 if (this.telecom == null) 1814 this.telecom = new ArrayList<ContactPoint>(); 1815 return this.telecom; 1816 } 1817 1818 /** 1819 * @return Returns a reference to <code>this</code> for easy method chaining 1820 */ 1821 public Patient setTelecom(List<ContactPoint> theTelecom) { 1822 this.telecom = theTelecom; 1823 return this; 1824 } 1825 1826 public boolean hasTelecom() { 1827 if (this.telecom == null) 1828 return false; 1829 for (ContactPoint item : this.telecom) 1830 if (!item.isEmpty()) 1831 return true; 1832 return false; 1833 } 1834 1835 public ContactPoint addTelecom() { // 3 1836 ContactPoint t = new ContactPoint(); 1837 if (this.telecom == null) 1838 this.telecom = new ArrayList<ContactPoint>(); 1839 this.telecom.add(t); 1840 return t; 1841 } 1842 1843 public Patient addTelecom(ContactPoint t) { // 3 1844 if (t == null) 1845 return this; 1846 if (this.telecom == null) 1847 this.telecom = new ArrayList<ContactPoint>(); 1848 this.telecom.add(t); 1849 return this; 1850 } 1851 1852 /** 1853 * @return The first repetition of repeating field {@link #telecom}, creating it 1854 * if it does not already exist 1855 */ 1856 public ContactPoint getTelecomFirstRep() { 1857 if (getTelecom().isEmpty()) { 1858 addTelecom(); 1859 } 1860 return getTelecom().get(0); 1861 } 1862 1863 /** 1864 * @return {@link #gender} (Administrative Gender - the gender that the patient 1865 * is considered to have for administration and record keeping 1866 * purposes.). This is the underlying object with id, value and 1867 * extensions. The accessor "getGender" gives direct access to the value 1868 */ 1869 public Enumeration<AdministrativeGender> getGenderElement() { 1870 if (this.gender == null) 1871 if (Configuration.errorOnAutoCreate()) 1872 throw new Error("Attempt to auto-create Patient.gender"); 1873 else if (Configuration.doAutoCreate()) 1874 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1875 return this.gender; 1876 } 1877 1878 public boolean hasGenderElement() { 1879 return this.gender != null && !this.gender.isEmpty(); 1880 } 1881 1882 public boolean hasGender() { 1883 return this.gender != null && !this.gender.isEmpty(); 1884 } 1885 1886 /** 1887 * @param value {@link #gender} (Administrative Gender - the gender that the 1888 * patient is considered to have for administration and record 1889 * keeping purposes.). This is the underlying object with id, value 1890 * and extensions. The accessor "getGender" gives direct access to 1891 * the value 1892 */ 1893 public Patient setGenderElement(Enumeration<AdministrativeGender> value) { 1894 this.gender = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return Administrative Gender - the gender that the patient is considered to 1900 * have for administration and record keeping purposes. 1901 */ 1902 public AdministrativeGender getGender() { 1903 return this.gender == null ? null : this.gender.getValue(); 1904 } 1905 1906 /** 1907 * @param value Administrative Gender - the gender that the patient is 1908 * considered to have for administration and record keeping 1909 * purposes. 1910 */ 1911 public Patient setGender(AdministrativeGender value) { 1912 if (value == null) 1913 this.gender = null; 1914 else { 1915 if (this.gender == null) 1916 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1917 this.gender.setValue(value); 1918 } 1919 return this; 1920 } 1921 1922 /** 1923 * @return {@link #birthDate} (The date of birth for the individual.). This is 1924 * the underlying object with id, value and extensions. The accessor 1925 * "getBirthDate" gives direct access to the value 1926 */ 1927 public DateType getBirthDateElement() { 1928 if (this.birthDate == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create Patient.birthDate"); 1931 else if (Configuration.doAutoCreate()) 1932 this.birthDate = new DateType(); // bb 1933 return this.birthDate; 1934 } 1935 1936 public boolean hasBirthDateElement() { 1937 return this.birthDate != null && !this.birthDate.isEmpty(); 1938 } 1939 1940 public boolean hasBirthDate() { 1941 return this.birthDate != null && !this.birthDate.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #birthDate} (The date of birth for the individual.). This 1946 * is the underlying object with id, value and extensions. The 1947 * accessor "getBirthDate" gives direct access to the value 1948 */ 1949 public Patient setBirthDateElement(DateType value) { 1950 this.birthDate = value; 1951 return this; 1952 } 1953 1954 /** 1955 * @return The date of birth for the individual. 1956 */ 1957 public Date getBirthDate() { 1958 return this.birthDate == null ? null : this.birthDate.getValue(); 1959 } 1960 1961 /** 1962 * @param value The date of birth for the individual. 1963 */ 1964 public Patient setBirthDate(Date value) { 1965 if (value == null) 1966 this.birthDate = null; 1967 else { 1968 if (this.birthDate == null) 1969 this.birthDate = new DateType(); 1970 this.birthDate.setValue(value); 1971 } 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1977 */ 1978 public Type getDeceased() { 1979 return this.deceased; 1980 } 1981 1982 /** 1983 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 1984 */ 1985 public BooleanType getDeceasedBooleanType() throws FHIRException { 1986 if (this.deceased == null) 1987 this.deceased = new BooleanType(); 1988 if (!(this.deceased instanceof BooleanType)) 1989 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1990 + this.deceased.getClass().getName() + " was encountered"); 1991 return (BooleanType) this.deceased; 1992 } 1993 1994 public boolean hasDeceasedBooleanType() { 1995 return this != null && this.deceased instanceof BooleanType; 1996 } 1997 1998 /** 1999 * @return {@link #deceased} (Indicates if the individual is deceased or not.) 2000 */ 2001 public DateTimeType getDeceasedDateTimeType() throws FHIRException { 2002 if (this.deceased == null) 2003 this.deceased = new DateTimeType(); 2004 if (!(this.deceased instanceof DateTimeType)) 2005 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2006 + this.deceased.getClass().getName() + " was encountered"); 2007 return (DateTimeType) this.deceased; 2008 } 2009 2010 public boolean hasDeceasedDateTimeType() { 2011 return this != null && this.deceased instanceof DateTimeType; 2012 } 2013 2014 public boolean hasDeceased() { 2015 return this.deceased != null && !this.deceased.isEmpty(); 2016 } 2017 2018 /** 2019 * @param value {@link #deceased} (Indicates if the individual is deceased or 2020 * not.) 2021 */ 2022 public Patient setDeceased(Type value) { 2023 if (value != null && !(value instanceof BooleanType || value instanceof DateTimeType)) 2024 throw new Error("Not the right type for Patient.deceased[x]: " + value.fhirType()); 2025 this.deceased = value; 2026 return this; 2027 } 2028 2029 /** 2030 * @return {@link #address} (An address for the individual.) 2031 */ 2032 public List<Address> getAddress() { 2033 if (this.address == null) 2034 this.address = new ArrayList<Address>(); 2035 return this.address; 2036 } 2037 2038 /** 2039 * @return Returns a reference to <code>this</code> for easy method chaining 2040 */ 2041 public Patient setAddress(List<Address> theAddress) { 2042 this.address = theAddress; 2043 return this; 2044 } 2045 2046 public boolean hasAddress() { 2047 if (this.address == null) 2048 return false; 2049 for (Address item : this.address) 2050 if (!item.isEmpty()) 2051 return true; 2052 return false; 2053 } 2054 2055 public Address addAddress() { // 3 2056 Address t = new Address(); 2057 if (this.address == null) 2058 this.address = new ArrayList<Address>(); 2059 this.address.add(t); 2060 return t; 2061 } 2062 2063 public Patient addAddress(Address t) { // 3 2064 if (t == null) 2065 return this; 2066 if (this.address == null) 2067 this.address = new ArrayList<Address>(); 2068 this.address.add(t); 2069 return this; 2070 } 2071 2072 /** 2073 * @return The first repetition of repeating field {@link #address}, creating it 2074 * if it does not already exist 2075 */ 2076 public Address getAddressFirstRep() { 2077 if (getAddress().isEmpty()) { 2078 addAddress(); 2079 } 2080 return getAddress().get(0); 2081 } 2082 2083 /** 2084 * @return {@link #maritalStatus} (This field contains a patient's most recent 2085 * marital (civil) status.) 2086 */ 2087 public CodeableConcept getMaritalStatus() { 2088 if (this.maritalStatus == null) 2089 if (Configuration.errorOnAutoCreate()) 2090 throw new Error("Attempt to auto-create Patient.maritalStatus"); 2091 else if (Configuration.doAutoCreate()) 2092 this.maritalStatus = new CodeableConcept(); // cc 2093 return this.maritalStatus; 2094 } 2095 2096 public boolean hasMaritalStatus() { 2097 return this.maritalStatus != null && !this.maritalStatus.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #maritalStatus} (This field contains a patient's most 2102 * recent marital (civil) status.) 2103 */ 2104 public Patient setMaritalStatus(CodeableConcept value) { 2105 this.maritalStatus = value; 2106 return this; 2107 } 2108 2109 /** 2110 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2111 * multiple (boolean) or indicates the actual birth order (integer).) 2112 */ 2113 public Type getMultipleBirth() { 2114 return this.multipleBirth; 2115 } 2116 2117 /** 2118 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2119 * multiple (boolean) or indicates the actual birth order (integer).) 2120 */ 2121 public BooleanType getMultipleBirthBooleanType() throws FHIRException { 2122 if (this.multipleBirth == null) 2123 this.multipleBirth = new BooleanType(); 2124 if (!(this.multipleBirth instanceof BooleanType)) 2125 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 2126 + this.multipleBirth.getClass().getName() + " was encountered"); 2127 return (BooleanType) this.multipleBirth; 2128 } 2129 2130 public boolean hasMultipleBirthBooleanType() { 2131 return this != null && this.multipleBirth instanceof BooleanType; 2132 } 2133 2134 /** 2135 * @return {@link #multipleBirth} (Indicates whether the patient is part of a 2136 * multiple (boolean) or indicates the actual birth order (integer).) 2137 */ 2138 public IntegerType getMultipleBirthIntegerType() throws FHIRException { 2139 if (this.multipleBirth == null) 2140 this.multipleBirth = new IntegerType(); 2141 if (!(this.multipleBirth instanceof IntegerType)) 2142 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 2143 + this.multipleBirth.getClass().getName() + " was encountered"); 2144 return (IntegerType) this.multipleBirth; 2145 } 2146 2147 public boolean hasMultipleBirthIntegerType() { 2148 return this != null && this.multipleBirth instanceof IntegerType; 2149 } 2150 2151 public boolean hasMultipleBirth() { 2152 return this.multipleBirth != null && !this.multipleBirth.isEmpty(); 2153 } 2154 2155 /** 2156 * @param value {@link #multipleBirth} (Indicates whether the patient is part of 2157 * a multiple (boolean) or indicates the actual birth order 2158 * (integer).) 2159 */ 2160 public Patient setMultipleBirth(Type value) { 2161 if (value != null && !(value instanceof BooleanType || value instanceof IntegerType)) 2162 throw new Error("Not the right type for Patient.multipleBirth[x]: " + value.fhirType()); 2163 this.multipleBirth = value; 2164 return this; 2165 } 2166 2167 /** 2168 * @return {@link #photo} (Image of the patient.) 2169 */ 2170 public List<Attachment> getPhoto() { 2171 if (this.photo == null) 2172 this.photo = new ArrayList<Attachment>(); 2173 return this.photo; 2174 } 2175 2176 /** 2177 * @return Returns a reference to <code>this</code> for easy method chaining 2178 */ 2179 public Patient setPhoto(List<Attachment> thePhoto) { 2180 this.photo = thePhoto; 2181 return this; 2182 } 2183 2184 public boolean hasPhoto() { 2185 if (this.photo == null) 2186 return false; 2187 for (Attachment item : this.photo) 2188 if (!item.isEmpty()) 2189 return true; 2190 return false; 2191 } 2192 2193 public Attachment addPhoto() { // 3 2194 Attachment t = new Attachment(); 2195 if (this.photo == null) 2196 this.photo = new ArrayList<Attachment>(); 2197 this.photo.add(t); 2198 return t; 2199 } 2200 2201 public Patient addPhoto(Attachment t) { // 3 2202 if (t == null) 2203 return this; 2204 if (this.photo == null) 2205 this.photo = new ArrayList<Attachment>(); 2206 this.photo.add(t); 2207 return this; 2208 } 2209 2210 /** 2211 * @return The first repetition of repeating field {@link #photo}, creating it 2212 * if it does not already exist 2213 */ 2214 public Attachment getPhotoFirstRep() { 2215 if (getPhoto().isEmpty()) { 2216 addPhoto(); 2217 } 2218 return getPhoto().get(0); 2219 } 2220 2221 /** 2222 * @return {@link #contact} (A contact party (e.g. guardian, partner, friend) 2223 * for the patient.) 2224 */ 2225 public List<ContactComponent> getContact() { 2226 if (this.contact == null) 2227 this.contact = new ArrayList<ContactComponent>(); 2228 return this.contact; 2229 } 2230 2231 /** 2232 * @return Returns a reference to <code>this</code> for easy method chaining 2233 */ 2234 public Patient setContact(List<ContactComponent> theContact) { 2235 this.contact = theContact; 2236 return this; 2237 } 2238 2239 public boolean hasContact() { 2240 if (this.contact == null) 2241 return false; 2242 for (ContactComponent item : this.contact) 2243 if (!item.isEmpty()) 2244 return true; 2245 return false; 2246 } 2247 2248 public ContactComponent addContact() { // 3 2249 ContactComponent t = new ContactComponent(); 2250 if (this.contact == null) 2251 this.contact = new ArrayList<ContactComponent>(); 2252 this.contact.add(t); 2253 return t; 2254 } 2255 2256 public Patient addContact(ContactComponent t) { // 3 2257 if (t == null) 2258 return this; 2259 if (this.contact == null) 2260 this.contact = new ArrayList<ContactComponent>(); 2261 this.contact.add(t); 2262 return this; 2263 } 2264 2265 /** 2266 * @return The first repetition of repeating field {@link #contact}, creating it 2267 * if it does not already exist 2268 */ 2269 public ContactComponent getContactFirstRep() { 2270 if (getContact().isEmpty()) { 2271 addContact(); 2272 } 2273 return getContact().get(0); 2274 } 2275 2276 /** 2277 * @return {@link #communication} (A language which may be used to communicate 2278 * with the patient about his or her health.) 2279 */ 2280 public List<PatientCommunicationComponent> getCommunication() { 2281 if (this.communication == null) 2282 this.communication = new ArrayList<PatientCommunicationComponent>(); 2283 return this.communication; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public Patient setCommunication(List<PatientCommunicationComponent> theCommunication) { 2290 this.communication = theCommunication; 2291 return this; 2292 } 2293 2294 public boolean hasCommunication() { 2295 if (this.communication == null) 2296 return false; 2297 for (PatientCommunicationComponent item : this.communication) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public PatientCommunicationComponent addCommunication() { // 3 2304 PatientCommunicationComponent t = new PatientCommunicationComponent(); 2305 if (this.communication == null) 2306 this.communication = new ArrayList<PatientCommunicationComponent>(); 2307 this.communication.add(t); 2308 return t; 2309 } 2310 2311 public Patient addCommunication(PatientCommunicationComponent t) { // 3 2312 if (t == null) 2313 return this; 2314 if (this.communication == null) 2315 this.communication = new ArrayList<PatientCommunicationComponent>(); 2316 this.communication.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #communication}, 2322 * creating it if it does not already exist 2323 */ 2324 public PatientCommunicationComponent getCommunicationFirstRep() { 2325 if (getCommunication().isEmpty()) { 2326 addCommunication(); 2327 } 2328 return getCommunication().get(0); 2329 } 2330 2331 /** 2332 * @return {@link #generalPractitioner} (Patient's nominated care provider.) 2333 */ 2334 public List<Reference> getGeneralPractitioner() { 2335 if (this.generalPractitioner == null) 2336 this.generalPractitioner = new ArrayList<Reference>(); 2337 return this.generalPractitioner; 2338 } 2339 2340 /** 2341 * @return Returns a reference to <code>this</code> for easy method chaining 2342 */ 2343 public Patient setGeneralPractitioner(List<Reference> theGeneralPractitioner) { 2344 this.generalPractitioner = theGeneralPractitioner; 2345 return this; 2346 } 2347 2348 public boolean hasGeneralPractitioner() { 2349 if (this.generalPractitioner == null) 2350 return false; 2351 for (Reference item : this.generalPractitioner) 2352 if (!item.isEmpty()) 2353 return true; 2354 return false; 2355 } 2356 2357 public Reference addGeneralPractitioner() { // 3 2358 Reference t = new Reference(); 2359 if (this.generalPractitioner == null) 2360 this.generalPractitioner = new ArrayList<Reference>(); 2361 this.generalPractitioner.add(t); 2362 return t; 2363 } 2364 2365 public Patient addGeneralPractitioner(Reference t) { // 3 2366 if (t == null) 2367 return this; 2368 if (this.generalPractitioner == null) 2369 this.generalPractitioner = new ArrayList<Reference>(); 2370 this.generalPractitioner.add(t); 2371 return this; 2372 } 2373 2374 /** 2375 * @return The first repetition of repeating field {@link #generalPractitioner}, 2376 * creating it if it does not already exist 2377 */ 2378 public Reference getGeneralPractitionerFirstRep() { 2379 if (getGeneralPractitioner().isEmpty()) { 2380 addGeneralPractitioner(); 2381 } 2382 return getGeneralPractitioner().get(0); 2383 } 2384 2385 /** 2386 * @deprecated Use Reference#setResource(IBaseResource) instead 2387 */ 2388 @Deprecated 2389 public List<Resource> getGeneralPractitionerTarget() { 2390 if (this.generalPractitionerTarget == null) 2391 this.generalPractitionerTarget = new ArrayList<Resource>(); 2392 return this.generalPractitionerTarget; 2393 } 2394 2395 /** 2396 * @return {@link #managingOrganization} (Organization that is the custodian of 2397 * the patient record.) 2398 */ 2399 public Reference getManagingOrganization() { 2400 if (this.managingOrganization == null) 2401 if (Configuration.errorOnAutoCreate()) 2402 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2403 else if (Configuration.doAutoCreate()) 2404 this.managingOrganization = new Reference(); // cc 2405 return this.managingOrganization; 2406 } 2407 2408 public boolean hasManagingOrganization() { 2409 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2410 } 2411 2412 /** 2413 * @param value {@link #managingOrganization} (Organization that is the 2414 * custodian of the patient record.) 2415 */ 2416 public Patient setManagingOrganization(Reference value) { 2417 this.managingOrganization = value; 2418 return this; 2419 } 2420 2421 /** 2422 * @return {@link #managingOrganization} The actual object that is the target of 2423 * the reference. The reference library doesn't populate this, but you 2424 * can use it to hold the resource if you resolve it. (Organization that 2425 * is the custodian of the patient record.) 2426 */ 2427 public Organization getManagingOrganizationTarget() { 2428 if (this.managingOrganizationTarget == null) 2429 if (Configuration.errorOnAutoCreate()) 2430 throw new Error("Attempt to auto-create Patient.managingOrganization"); 2431 else if (Configuration.doAutoCreate()) 2432 this.managingOrganizationTarget = new Organization(); // aa 2433 return this.managingOrganizationTarget; 2434 } 2435 2436 /** 2437 * @param value {@link #managingOrganization} The actual object that is the 2438 * target of the reference. The reference library doesn't use 2439 * these, but you can use it to hold the resource if you resolve 2440 * it. (Organization that is the custodian of the patient record.) 2441 */ 2442 public Patient setManagingOrganizationTarget(Organization value) { 2443 this.managingOrganizationTarget = value; 2444 return this; 2445 } 2446 2447 /** 2448 * @return {@link #link} (Link to another patient resource that concerns the 2449 * same actual patient.) 2450 */ 2451 public List<PatientLinkComponent> getLink() { 2452 if (this.link == null) 2453 this.link = new ArrayList<PatientLinkComponent>(); 2454 return this.link; 2455 } 2456 2457 /** 2458 * @return Returns a reference to <code>this</code> for easy method chaining 2459 */ 2460 public Patient setLink(List<PatientLinkComponent> theLink) { 2461 this.link = theLink; 2462 return this; 2463 } 2464 2465 public boolean hasLink() { 2466 if (this.link == null) 2467 return false; 2468 for (PatientLinkComponent item : this.link) 2469 if (!item.isEmpty()) 2470 return true; 2471 return false; 2472 } 2473 2474 public PatientLinkComponent addLink() { // 3 2475 PatientLinkComponent t = new PatientLinkComponent(); 2476 if (this.link == null) 2477 this.link = new ArrayList<PatientLinkComponent>(); 2478 this.link.add(t); 2479 return t; 2480 } 2481 2482 public Patient addLink(PatientLinkComponent t) { // 3 2483 if (t == null) 2484 return this; 2485 if (this.link == null) 2486 this.link = new ArrayList<PatientLinkComponent>(); 2487 this.link.add(t); 2488 return this; 2489 } 2490 2491 /** 2492 * @return The first repetition of repeating field {@link #link}, creating it if 2493 * it does not already exist 2494 */ 2495 public PatientLinkComponent getLinkFirstRep() { 2496 if (getLink().isEmpty()) { 2497 addLink(); 2498 } 2499 return getLink().get(0); 2500 } 2501 2502 protected void listChildren(List<Property> children) { 2503 super.listChildren(children); 2504 children.add(new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2505 java.lang.Integer.MAX_VALUE, identifier)); 2506 children.add(new Property("active", "boolean", 2507 "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 2508 0, 1, active)); 2509 children.add(new Property("name", "HumanName", "A name associated with the individual.", 0, 2510 java.lang.Integer.MAX_VALUE, name)); 2511 children.add(new Property("telecom", "ContactPoint", 2512 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2513 java.lang.Integer.MAX_VALUE, telecom)); 2514 children.add(new Property("gender", "code", 2515 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2516 0, 1, gender)); 2517 children.add(new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, birthDate)); 2518 children.add(new Property("deceased[x]", "boolean|dateTime", "Indicates if the individual is deceased or not.", 0, 2519 1, deceased)); 2520 children.add( 2521 new Property("address", "Address", "An address for the individual.", 0, java.lang.Integer.MAX_VALUE, address)); 2522 children.add(new Property("maritalStatus", "CodeableConcept", 2523 "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus)); 2524 children.add(new Property("multipleBirth[x]", "boolean|integer", 2525 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2526 0, 1, multipleBirth)); 2527 children.add(new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, photo)); 2528 children.add(new Property("contact", "", "A contact party (e.g. guardian, partner, friend) for the patient.", 0, 2529 java.lang.Integer.MAX_VALUE, contact)); 2530 children.add(new Property("communication", "", 2531 "A language which may be used to communicate with the patient about his or her health.", 0, 2532 java.lang.Integer.MAX_VALUE, communication)); 2533 children.add(new Property("generalPractitioner", "Reference(Organization|Practitioner|PractitionerRole)", 2534 "Patient's nominated care provider.", 0, java.lang.Integer.MAX_VALUE, generalPractitioner)); 2535 children.add(new Property("managingOrganization", "Reference(Organization)", 2536 "Organization that is the custodian of the patient record.", 0, 1, managingOrganization)); 2537 children.add(new Property("link", "", "Link to another patient resource that concerns the same actual patient.", 0, 2538 java.lang.Integer.MAX_VALUE, link)); 2539 } 2540 2541 @Override 2542 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2543 switch (_hash) { 2544 case -1618432855: 2545 /* identifier */ return new Property("identifier", "Identifier", "An identifier for this patient.", 0, 2546 java.lang.Integer.MAX_VALUE, identifier); 2547 case -1422950650: 2548 /* active */ return new Property("active", "boolean", 2549 "Whether this patient record is in active use. \nMany systems use this property to mark as non-current patients, such as those that have not been seen for a period of time based on an organization's business rules.\n\nIt is often used to filter patient lists to exclude inactive patients\n\nDeceased patients may also be marked as inactive for the same reasons, but may be active for some time after death.", 2550 0, 1, active); 2551 case 3373707: 2552 /* name */ return new Property("name", "HumanName", "A name associated with the individual.", 0, 2553 java.lang.Integer.MAX_VALUE, name); 2554 case -1429363305: 2555 /* telecom */ return new Property("telecom", "ContactPoint", 2556 "A contact detail (e.g. a telephone number or an email address) by which the individual may be contacted.", 0, 2557 java.lang.Integer.MAX_VALUE, telecom); 2558 case -1249512767: 2559 /* gender */ return new Property("gender", "code", 2560 "Administrative Gender - the gender that the patient is considered to have for administration and record keeping purposes.", 2561 0, 1, gender); 2562 case -1210031859: 2563 /* birthDate */ return new Property("birthDate", "date", "The date of birth for the individual.", 0, 1, 2564 birthDate); 2565 case -1311442804: 2566 /* deceased[x] */ return new Property("deceased[x]", "boolean|dateTime", 2567 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2568 case 561497972: 2569 /* deceased */ return new Property("deceased[x]", "boolean|dateTime", 2570 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2571 case 497463828: 2572 /* deceasedBoolean */ return new Property("deceased[x]", "boolean|dateTime", 2573 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2574 case -1971804369: 2575 /* deceasedDateTime */ return new Property("deceased[x]", "boolean|dateTime", 2576 "Indicates if the individual is deceased or not.", 0, 1, deceased); 2577 case -1147692044: 2578 /* address */ return new Property("address", "Address", "An address for the individual.", 0, 2579 java.lang.Integer.MAX_VALUE, address); 2580 case 1756919302: 2581 /* maritalStatus */ return new Property("maritalStatus", "CodeableConcept", 2582 "This field contains a patient's most recent marital (civil) status.", 0, 1, maritalStatus); 2583 case -1764672111: 2584 /* multipleBirth[x] */ return new Property("multipleBirth[x]", "boolean|integer", 2585 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2586 0, 1, multipleBirth); 2587 case -677369713: 2588 /* multipleBirth */ return new Property("multipleBirth[x]", "boolean|integer", 2589 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2590 0, 1, multipleBirth); 2591 case -247534439: 2592 /* multipleBirthBoolean */ return new Property("multipleBirth[x]", "boolean|integer", 2593 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2594 0, 1, multipleBirth); 2595 case 1645805999: 2596 /* multipleBirthInteger */ return new Property("multipleBirth[x]", "boolean|integer", 2597 "Indicates whether the patient is part of a multiple (boolean) or indicates the actual birth order (integer).", 2598 0, 1, multipleBirth); 2599 case 106642994: 2600 /* photo */ return new Property("photo", "Attachment", "Image of the patient.", 0, java.lang.Integer.MAX_VALUE, 2601 photo); 2602 case 951526432: 2603 /* contact */ return new Property("contact", "", 2604 "A contact party (e.g. guardian, partner, friend) for the patient.", 0, java.lang.Integer.MAX_VALUE, contact); 2605 case -1035284522: 2606 /* communication */ return new Property("communication", "", 2607 "A language which may be used to communicate with the patient about his or her health.", 0, 2608 java.lang.Integer.MAX_VALUE, communication); 2609 case 1488292898: 2610 /* generalPractitioner */ return new Property("generalPractitioner", 2611 "Reference(Organization|Practitioner|PractitionerRole)", "Patient's nominated care provider.", 0, 2612 java.lang.Integer.MAX_VALUE, generalPractitioner); 2613 case -2058947787: 2614 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 2615 "Organization that is the custodian of the patient record.", 0, 1, managingOrganization); 2616 case 3321850: 2617 /* link */ return new Property("link", "", 2618 "Link to another patient resource that concerns the same actual patient.", 0, java.lang.Integer.MAX_VALUE, 2619 link); 2620 default: 2621 return super.getNamedProperty(_hash, _name, _checkValid); 2622 } 2623 2624 } 2625 2626 @Override 2627 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2628 switch (hash) { 2629 case -1618432855: 2630 /* identifier */ return this.identifier == null ? new Base[0] 2631 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2632 case -1422950650: 2633 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 2634 case 3373707: 2635 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 2636 case -1429363305: 2637 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2638 case -1249512767: 2639 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 2640 case -1210031859: 2641 /* birthDate */ return this.birthDate == null ? new Base[0] : new Base[] { this.birthDate }; // DateType 2642 case 561497972: 2643 /* deceased */ return this.deceased == null ? new Base[0] : new Base[] { this.deceased }; // Type 2644 case -1147692044: 2645 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 2646 case 1756919302: 2647 /* maritalStatus */ return this.maritalStatus == null ? new Base[0] : new Base[] { this.maritalStatus }; // CodeableConcept 2648 case -677369713: 2649 /* multipleBirth */ return this.multipleBirth == null ? new Base[0] : new Base[] { this.multipleBirth }; // Type 2650 case 106642994: 2651 /* photo */ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 2652 case 951526432: 2653 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactComponent 2654 case -1035284522: 2655 /* communication */ return this.communication == null ? new Base[0] 2656 : this.communication.toArray(new Base[this.communication.size()]); // PatientCommunicationComponent 2657 case 1488292898: 2658 /* generalPractitioner */ return this.generalPractitioner == null ? new Base[0] 2659 : this.generalPractitioner.toArray(new Base[this.generalPractitioner.size()]); // Reference 2660 case -2058947787: 2661 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 2662 : new Base[] { this.managingOrganization }; // Reference 2663 case 3321850: 2664 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PatientLinkComponent 2665 default: 2666 return super.getProperty(hash, name, checkValid); 2667 } 2668 2669 } 2670 2671 @Override 2672 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2673 switch (hash) { 2674 case -1618432855: // identifier 2675 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2676 return value; 2677 case -1422950650: // active 2678 this.active = castToBoolean(value); // BooleanType 2679 return value; 2680 case 3373707: // name 2681 this.getName().add(castToHumanName(value)); // HumanName 2682 return value; 2683 case -1429363305: // telecom 2684 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2685 return value; 2686 case -1249512767: // gender 2687 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2688 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2689 return value; 2690 case -1210031859: // birthDate 2691 this.birthDate = castToDate(value); // DateType 2692 return value; 2693 case 561497972: // deceased 2694 this.deceased = castToType(value); // Type 2695 return value; 2696 case -1147692044: // address 2697 this.getAddress().add(castToAddress(value)); // Address 2698 return value; 2699 case 1756919302: // maritalStatus 2700 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2701 return value; 2702 case -677369713: // multipleBirth 2703 this.multipleBirth = castToType(value); // Type 2704 return value; 2705 case 106642994: // photo 2706 this.getPhoto().add(castToAttachment(value)); // Attachment 2707 return value; 2708 case 951526432: // contact 2709 this.getContact().add((ContactComponent) value); // ContactComponent 2710 return value; 2711 case -1035284522: // communication 2712 this.getCommunication().add((PatientCommunicationComponent) value); // PatientCommunicationComponent 2713 return value; 2714 case 1488292898: // generalPractitioner 2715 this.getGeneralPractitioner().add(castToReference(value)); // Reference 2716 return value; 2717 case -2058947787: // managingOrganization 2718 this.managingOrganization = castToReference(value); // Reference 2719 return value; 2720 case 3321850: // link 2721 this.getLink().add((PatientLinkComponent) value); // PatientLinkComponent 2722 return value; 2723 default: 2724 return super.setProperty(hash, name, value); 2725 } 2726 2727 } 2728 2729 @Override 2730 public Base setProperty(String name, Base value) throws FHIRException { 2731 if (name.equals("identifier")) { 2732 this.getIdentifier().add(castToIdentifier(value)); 2733 } else if (name.equals("active")) { 2734 this.active = castToBoolean(value); // BooleanType 2735 } else if (name.equals("name")) { 2736 this.getName().add(castToHumanName(value)); 2737 } else if (name.equals("telecom")) { 2738 this.getTelecom().add(castToContactPoint(value)); 2739 } else if (name.equals("gender")) { 2740 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 2741 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 2742 } else if (name.equals("birthDate")) { 2743 this.birthDate = castToDate(value); // DateType 2744 } else if (name.equals("deceased[x]")) { 2745 this.deceased = castToType(value); // Type 2746 } else if (name.equals("address")) { 2747 this.getAddress().add(castToAddress(value)); 2748 } else if (name.equals("maritalStatus")) { 2749 this.maritalStatus = castToCodeableConcept(value); // CodeableConcept 2750 } else if (name.equals("multipleBirth[x]")) { 2751 this.multipleBirth = castToType(value); // Type 2752 } else if (name.equals("photo")) { 2753 this.getPhoto().add(castToAttachment(value)); 2754 } else if (name.equals("contact")) { 2755 this.getContact().add((ContactComponent) value); 2756 } else if (name.equals("communication")) { 2757 this.getCommunication().add((PatientCommunicationComponent) value); 2758 } else if (name.equals("generalPractitioner")) { 2759 this.getGeneralPractitioner().add(castToReference(value)); 2760 } else if (name.equals("managingOrganization")) { 2761 this.managingOrganization = castToReference(value); // Reference 2762 } else if (name.equals("link")) { 2763 this.getLink().add((PatientLinkComponent) value); 2764 } else 2765 return super.setProperty(name, value); 2766 return value; 2767 } 2768 2769 @Override 2770 public void removeChild(String name, Base value) throws FHIRException { 2771 if (name.equals("identifier")) { 2772 this.getIdentifier().remove(castToIdentifier(value)); 2773 } else if (name.equals("active")) { 2774 this.active = null; 2775 } else if (name.equals("name")) { 2776 this.getName().remove(castToHumanName(value)); 2777 } else if (name.equals("telecom")) { 2778 this.getTelecom().remove(castToContactPoint(value)); 2779 } else if (name.equals("gender")) { 2780 this.gender = null; 2781 } else if (name.equals("birthDate")) { 2782 this.birthDate = null; 2783 } else if (name.equals("deceased[x]")) { 2784 this.deceased = null; 2785 } else if (name.equals("address")) { 2786 this.getAddress().remove(castToAddress(value)); 2787 } else if (name.equals("maritalStatus")) { 2788 this.maritalStatus = null; 2789 } else if (name.equals("multipleBirth[x]")) { 2790 this.multipleBirth = null; 2791 } else if (name.equals("photo")) { 2792 this.getPhoto().remove(castToAttachment(value)); 2793 } else if (name.equals("contact")) { 2794 this.getContact().remove((ContactComponent) value); 2795 } else if (name.equals("communication")) { 2796 this.getCommunication().remove((PatientCommunicationComponent) value); 2797 } else if (name.equals("generalPractitioner")) { 2798 this.getGeneralPractitioner().remove(castToReference(value)); 2799 } else if (name.equals("managingOrganization")) { 2800 this.managingOrganization = null; 2801 } else if (name.equals("link")) { 2802 this.getLink().remove((PatientLinkComponent) value); 2803 } else 2804 super.removeChild(name, value); 2805 2806 } 2807 2808 @Override 2809 public Base makeProperty(int hash, String name) throws FHIRException { 2810 switch (hash) { 2811 case -1618432855: 2812 return addIdentifier(); 2813 case -1422950650: 2814 return getActiveElement(); 2815 case 3373707: 2816 return addName(); 2817 case -1429363305: 2818 return addTelecom(); 2819 case -1249512767: 2820 return getGenderElement(); 2821 case -1210031859: 2822 return getBirthDateElement(); 2823 case -1311442804: 2824 return getDeceased(); 2825 case 561497972: 2826 return getDeceased(); 2827 case -1147692044: 2828 return addAddress(); 2829 case 1756919302: 2830 return getMaritalStatus(); 2831 case -1764672111: 2832 return getMultipleBirth(); 2833 case -677369713: 2834 return getMultipleBirth(); 2835 case 106642994: 2836 return addPhoto(); 2837 case 951526432: 2838 return addContact(); 2839 case -1035284522: 2840 return addCommunication(); 2841 case 1488292898: 2842 return addGeneralPractitioner(); 2843 case -2058947787: 2844 return getManagingOrganization(); 2845 case 3321850: 2846 return addLink(); 2847 default: 2848 return super.makeProperty(hash, name); 2849 } 2850 2851 } 2852 2853 @Override 2854 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2855 switch (hash) { 2856 case -1618432855: 2857 /* identifier */ return new String[] { "Identifier" }; 2858 case -1422950650: 2859 /* active */ return new String[] { "boolean" }; 2860 case 3373707: 2861 /* name */ return new String[] { "HumanName" }; 2862 case -1429363305: 2863 /* telecom */ return new String[] { "ContactPoint" }; 2864 case -1249512767: 2865 /* gender */ return new String[] { "code" }; 2866 case -1210031859: 2867 /* birthDate */ return new String[] { "date" }; 2868 case 561497972: 2869 /* deceased */ return new String[] { "boolean", "dateTime" }; 2870 case -1147692044: 2871 /* address */ return new String[] { "Address" }; 2872 case 1756919302: 2873 /* maritalStatus */ return new String[] { "CodeableConcept" }; 2874 case -677369713: 2875 /* multipleBirth */ return new String[] { "boolean", "integer" }; 2876 case 106642994: 2877 /* photo */ return new String[] { "Attachment" }; 2878 case 951526432: 2879 /* contact */ return new String[] {}; 2880 case -1035284522: 2881 /* communication */ return new String[] {}; 2882 case 1488292898: 2883 /* generalPractitioner */ return new String[] { "Reference" }; 2884 case -2058947787: 2885 /* managingOrganization */ return new String[] { "Reference" }; 2886 case 3321850: 2887 /* link */ return new String[] {}; 2888 default: 2889 return super.getTypesForProperty(hash, name); 2890 } 2891 2892 } 2893 2894 @Override 2895 public Base addChild(String name) throws FHIRException { 2896 if (name.equals("identifier")) { 2897 return addIdentifier(); 2898 } else if (name.equals("active")) { 2899 throw new FHIRException("Cannot call addChild on a singleton property Patient.active"); 2900 } else if (name.equals("name")) { 2901 return addName(); 2902 } else if (name.equals("telecom")) { 2903 return addTelecom(); 2904 } else if (name.equals("gender")) { 2905 throw new FHIRException("Cannot call addChild on a singleton property Patient.gender"); 2906 } else if (name.equals("birthDate")) { 2907 throw new FHIRException("Cannot call addChild on a singleton property Patient.birthDate"); 2908 } else if (name.equals("deceasedBoolean")) { 2909 this.deceased = new BooleanType(); 2910 return this.deceased; 2911 } else if (name.equals("deceasedDateTime")) { 2912 this.deceased = new DateTimeType(); 2913 return this.deceased; 2914 } else if (name.equals("address")) { 2915 return addAddress(); 2916 } else if (name.equals("maritalStatus")) { 2917 this.maritalStatus = new CodeableConcept(); 2918 return this.maritalStatus; 2919 } else if (name.equals("multipleBirthBoolean")) { 2920 this.multipleBirth = new BooleanType(); 2921 return this.multipleBirth; 2922 } else if (name.equals("multipleBirthInteger")) { 2923 this.multipleBirth = new IntegerType(); 2924 return this.multipleBirth; 2925 } else if (name.equals("photo")) { 2926 return addPhoto(); 2927 } else if (name.equals("contact")) { 2928 return addContact(); 2929 } else if (name.equals("communication")) { 2930 return addCommunication(); 2931 } else if (name.equals("generalPractitioner")) { 2932 return addGeneralPractitioner(); 2933 } else if (name.equals("managingOrganization")) { 2934 this.managingOrganization = new Reference(); 2935 return this.managingOrganization; 2936 } else if (name.equals("link")) { 2937 return addLink(); 2938 } else 2939 return super.addChild(name); 2940 } 2941 2942 public String fhirType() { 2943 return "Patient"; 2944 2945 } 2946 2947 public Patient copy() { 2948 Patient dst = new Patient(); 2949 copyValues(dst); 2950 return dst; 2951 } 2952 2953 public void copyValues(Patient dst) { 2954 super.copyValues(dst); 2955 if (identifier != null) { 2956 dst.identifier = new ArrayList<Identifier>(); 2957 for (Identifier i : identifier) 2958 dst.identifier.add(i.copy()); 2959 } 2960 ; 2961 dst.active = active == null ? null : active.copy(); 2962 if (name != null) { 2963 dst.name = new ArrayList<HumanName>(); 2964 for (HumanName i : name) 2965 dst.name.add(i.copy()); 2966 } 2967 ; 2968 if (telecom != null) { 2969 dst.telecom = new ArrayList<ContactPoint>(); 2970 for (ContactPoint i : telecom) 2971 dst.telecom.add(i.copy()); 2972 } 2973 ; 2974 dst.gender = gender == null ? null : gender.copy(); 2975 dst.birthDate = birthDate == null ? null : birthDate.copy(); 2976 dst.deceased = deceased == null ? null : deceased.copy(); 2977 if (address != null) { 2978 dst.address = new ArrayList<Address>(); 2979 for (Address i : address) 2980 dst.address.add(i.copy()); 2981 } 2982 ; 2983 dst.maritalStatus = maritalStatus == null ? null : maritalStatus.copy(); 2984 dst.multipleBirth = multipleBirth == null ? null : multipleBirth.copy(); 2985 if (photo != null) { 2986 dst.photo = new ArrayList<Attachment>(); 2987 for (Attachment i : photo) 2988 dst.photo.add(i.copy()); 2989 } 2990 ; 2991 if (contact != null) { 2992 dst.contact = new ArrayList<ContactComponent>(); 2993 for (ContactComponent i : contact) 2994 dst.contact.add(i.copy()); 2995 } 2996 ; 2997 if (communication != null) { 2998 dst.communication = new ArrayList<PatientCommunicationComponent>(); 2999 for (PatientCommunicationComponent i : communication) 3000 dst.communication.add(i.copy()); 3001 } 3002 ; 3003 if (generalPractitioner != null) { 3004 dst.generalPractitioner = new ArrayList<Reference>(); 3005 for (Reference i : generalPractitioner) 3006 dst.generalPractitioner.add(i.copy()); 3007 } 3008 ; 3009 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 3010 if (link != null) { 3011 dst.link = new ArrayList<PatientLinkComponent>(); 3012 for (PatientLinkComponent i : link) 3013 dst.link.add(i.copy()); 3014 } 3015 ; 3016 } 3017 3018 protected Patient typedCopy() { 3019 return copy(); 3020 } 3021 3022 @Override 3023 public boolean equalsDeep(Base other_) { 3024 if (!super.equalsDeep(other_)) 3025 return false; 3026 if (!(other_ instanceof Patient)) 3027 return false; 3028 Patient o = (Patient) other_; 3029 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 3030 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 3031 && compareDeep(gender, o.gender, true) && compareDeep(birthDate, o.birthDate, true) 3032 && compareDeep(deceased, o.deceased, true) && compareDeep(address, o.address, true) 3033 && compareDeep(maritalStatus, o.maritalStatus, true) && compareDeep(multipleBirth, o.multipleBirth, true) 3034 && compareDeep(photo, o.photo, true) && compareDeep(contact, o.contact, true) 3035 && compareDeep(communication, o.communication, true) 3036 && compareDeep(generalPractitioner, o.generalPractitioner, true) 3037 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(link, o.link, true); 3038 } 3039 3040 @Override 3041 public boolean equalsShallow(Base other_) { 3042 if (!super.equalsShallow(other_)) 3043 return false; 3044 if (!(other_ instanceof Patient)) 3045 return false; 3046 Patient o = (Patient) other_; 3047 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 3048 && compareValues(birthDate, o.birthDate, true); 3049 } 3050 3051 public boolean isEmpty() { 3052 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name, telecom, gender, birthDate, 3053 deceased, address, maritalStatus, multipleBirth, photo, contact, communication, generalPractitioner, 3054 managingOrganization, link); 3055 } 3056 3057 @Override 3058 public ResourceType getResourceType() { 3059 return ResourceType.Patient; 3060 } 3061 3062 /** 3063 * Search parameter: <b>identifier</b> 3064 * <p> 3065 * Description: <b>A patient identifier</b><br> 3066 * Type: <b>token</b><br> 3067 * Path: <b>Patient.identifier</b><br> 3068 * </p> 3069 */ 3070 @SearchParamDefinition(name = "identifier", path = "Patient.identifier", description = "A patient identifier", type = "token") 3071 public static final String SP_IDENTIFIER = "identifier"; 3072 /** 3073 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3074 * <p> 3075 * Description: <b>A patient identifier</b><br> 3076 * Type: <b>token</b><br> 3077 * Path: <b>Patient.identifier</b><br> 3078 * </p> 3079 */ 3080 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3081 SP_IDENTIFIER); 3082 3083 /** 3084 * Search parameter: <b>given</b> 3085 * <p> 3086 * Description: <b>A portion of the given name of the patient</b><br> 3087 * Type: <b>string</b><br> 3088 * Path: <b>Patient.name.given</b><br> 3089 * </p> 3090 */ 3091 @SearchParamDefinition(name = "given", path = "Patient.name.given", description = "A portion of the given name of the patient", type = "string") 3092 public static final String SP_GIVEN = "given"; 3093 /** 3094 * <b>Fluent Client</b> search parameter constant for <b>given</b> 3095 * <p> 3096 * Description: <b>A portion of the given name of the patient</b><br> 3097 * Type: <b>string</b><br> 3098 * Path: <b>Patient.name.given</b><br> 3099 * </p> 3100 */ 3101 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam( 3102 SP_GIVEN); 3103 3104 /** 3105 * Search parameter: <b>address</b> 3106 * <p> 3107 * Description: <b>A server defined search that may match any of the string 3108 * fields in the Address, including line, city, district, state, country, 3109 * postalCode, and/or text</b><br> 3110 * Type: <b>string</b><br> 3111 * Path: <b>Patient.address</b><br> 3112 * </p> 3113 */ 3114 @SearchParamDefinition(name = "address", path = "Patient.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 3115 public static final String SP_ADDRESS = "address"; 3116 /** 3117 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3118 * <p> 3119 * Description: <b>A server defined search that may match any of the string 3120 * fields in the Address, including line, city, district, state, country, 3121 * postalCode, and/or text</b><br> 3122 * Type: <b>string</b><br> 3123 * Path: <b>Patient.address</b><br> 3124 * </p> 3125 */ 3126 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 3127 SP_ADDRESS); 3128 3129 /** 3130 * Search parameter: <b>birthdate</b> 3131 * <p> 3132 * Description: <b>The patient's date of birth</b><br> 3133 * Type: <b>date</b><br> 3134 * Path: <b>Patient.birthDate</b><br> 3135 * </p> 3136 */ 3137 @SearchParamDefinition(name = "birthdate", path = "Patient.birthDate", description = "The patient's date of birth", type = "date") 3138 public static final String SP_BIRTHDATE = "birthdate"; 3139 /** 3140 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 3141 * <p> 3142 * Description: <b>The patient's date of birth</b><br> 3143 * Type: <b>date</b><br> 3144 * Path: <b>Patient.birthDate</b><br> 3145 * </p> 3146 */ 3147 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3148 SP_BIRTHDATE); 3149 3150 /** 3151 * Search parameter: <b>deceased</b> 3152 * <p> 3153 * Description: <b>This patient has been marked as deceased, or as a death date 3154 * entered</b><br> 3155 * Type: <b>token</b><br> 3156 * Path: <b>Patient.deceased[x]</b><br> 3157 * </p> 3158 */ 3159 @SearchParamDefinition(name = "deceased", path = "Patient.deceased.exists() and Patient.deceased != false", description = "This patient has been marked as deceased, or as a death date entered", type = "token") 3160 public static final String SP_DECEASED = "deceased"; 3161 /** 3162 * <b>Fluent Client</b> search parameter constant for <b>deceased</b> 3163 * <p> 3164 * Description: <b>This patient has been marked as deceased, or as a death date 3165 * entered</b><br> 3166 * Type: <b>token</b><br> 3167 * Path: <b>Patient.deceased[x]</b><br> 3168 * </p> 3169 */ 3170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DECEASED = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3171 SP_DECEASED); 3172 3173 /** 3174 * Search parameter: <b>address-state</b> 3175 * <p> 3176 * Description: <b>A state specified in an address</b><br> 3177 * Type: <b>string</b><br> 3178 * Path: <b>Patient.address.state</b><br> 3179 * </p> 3180 */ 3181 @SearchParamDefinition(name = "address-state", path = "Patient.address.state", description = "A state specified in an address", type = "string") 3182 public static final String SP_ADDRESS_STATE = "address-state"; 3183 /** 3184 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 3185 * <p> 3186 * Description: <b>A state specified in an address</b><br> 3187 * Type: <b>string</b><br> 3188 * Path: <b>Patient.address.state</b><br> 3189 * </p> 3190 */ 3191 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3192 SP_ADDRESS_STATE); 3193 3194 /** 3195 * Search parameter: <b>gender</b> 3196 * <p> 3197 * Description: <b>Gender of the patient</b><br> 3198 * Type: <b>token</b><br> 3199 * Path: <b>Patient.gender</b><br> 3200 * </p> 3201 */ 3202 @SearchParamDefinition(name = "gender", path = "Patient.gender", description = "Gender of the patient", type = "token") 3203 public static final String SP_GENDER = "gender"; 3204 /** 3205 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 3206 * <p> 3207 * Description: <b>Gender of the patient</b><br> 3208 * Type: <b>token</b><br> 3209 * Path: <b>Patient.gender</b><br> 3210 * </p> 3211 */ 3212 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3213 SP_GENDER); 3214 3215 /** 3216 * Search parameter: <b>general-practitioner</b> 3217 * <p> 3218 * Description: <b>Patient's nominated general practitioner, not the 3219 * organization that manages the record</b><br> 3220 * Type: <b>reference</b><br> 3221 * Path: <b>Patient.generalPractitioner</b><br> 3222 * </p> 3223 */ 3224 @SearchParamDefinition(name = "general-practitioner", path = "Patient.generalPractitioner", description = "Patient's nominated general practitioner, not the organization that manages the record", type = "reference", providesMembershipIn = { 3225 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 3226 Practitioner.class, PractitionerRole.class }) 3227 public static final String SP_GENERAL_PRACTITIONER = "general-practitioner"; 3228 /** 3229 * <b>Fluent Client</b> search parameter constant for 3230 * <b>general-practitioner</b> 3231 * <p> 3232 * Description: <b>Patient's nominated general practitioner, not the 3233 * organization that manages the record</b><br> 3234 * Type: <b>reference</b><br> 3235 * Path: <b>Patient.generalPractitioner</b><br> 3236 * </p> 3237 */ 3238 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GENERAL_PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3239 SP_GENERAL_PRACTITIONER); 3240 3241 /** 3242 * Constant for fluent queries to be used to add include statements. Specifies 3243 * the path value of "<b>Patient:general-practitioner</b>". 3244 */ 3245 public static final ca.uhn.fhir.model.api.Include INCLUDE_GENERAL_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 3246 "Patient:general-practitioner").toLocked(); 3247 3248 /** 3249 * Search parameter: <b>link</b> 3250 * <p> 3251 * Description: <b>All patients linked to the given patient</b><br> 3252 * Type: <b>reference</b><br> 3253 * Path: <b>Patient.link.other</b><br> 3254 * </p> 3255 */ 3256 @SearchParamDefinition(name = "link", path = "Patient.link.other", description = "All patients linked to the given patient", type = "reference", providesMembershipIn = { 3257 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3258 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 3259 RelatedPerson.class }) 3260 public static final String SP_LINK = "link"; 3261 /** 3262 * <b>Fluent Client</b> search parameter constant for <b>link</b> 3263 * <p> 3264 * Description: <b>All patients linked to the given patient</b><br> 3265 * Type: <b>reference</b><br> 3266 * Path: <b>Patient.link.other</b><br> 3267 * </p> 3268 */ 3269 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3270 SP_LINK); 3271 3272 /** 3273 * Constant for fluent queries to be used to add include statements. Specifies 3274 * the path value of "<b>Patient:link</b>". 3275 */ 3276 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Patient:link") 3277 .toLocked(); 3278 3279 /** 3280 * Search parameter: <b>active</b> 3281 * <p> 3282 * Description: <b>Whether the patient record is active</b><br> 3283 * Type: <b>token</b><br> 3284 * Path: <b>Patient.active</b><br> 3285 * </p> 3286 */ 3287 @SearchParamDefinition(name = "active", path = "Patient.active", description = "Whether the patient record is active", type = "token") 3288 public static final String SP_ACTIVE = "active"; 3289 /** 3290 * <b>Fluent Client</b> search parameter constant for <b>active</b> 3291 * <p> 3292 * Description: <b>Whether the patient record is active</b><br> 3293 * Type: <b>token</b><br> 3294 * Path: <b>Patient.active</b><br> 3295 * </p> 3296 */ 3297 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3298 SP_ACTIVE); 3299 3300 /** 3301 * Search parameter: <b>language</b> 3302 * <p> 3303 * Description: <b>Language code (irrespective of use value)</b><br> 3304 * Type: <b>token</b><br> 3305 * Path: <b>Patient.communication.language</b><br> 3306 * </p> 3307 */ 3308 @SearchParamDefinition(name = "language", path = "Patient.communication.language", description = "Language code (irrespective of use value)", type = "token") 3309 public static final String SP_LANGUAGE = "language"; 3310 /** 3311 * <b>Fluent Client</b> search parameter constant for <b>language</b> 3312 * <p> 3313 * Description: <b>Language code (irrespective of use value)</b><br> 3314 * Type: <b>token</b><br> 3315 * Path: <b>Patient.communication.language</b><br> 3316 * </p> 3317 */ 3318 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LANGUAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3319 SP_LANGUAGE); 3320 3321 /** 3322 * Search parameter: <b>address-postalcode</b> 3323 * <p> 3324 * Description: <b>A postalCode specified in an address</b><br> 3325 * Type: <b>string</b><br> 3326 * Path: <b>Patient.address.postalCode</b><br> 3327 * </p> 3328 */ 3329 @SearchParamDefinition(name = "address-postalcode", path = "Patient.address.postalCode", description = "A postalCode specified in an address", type = "string") 3330 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3331 /** 3332 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3333 * <p> 3334 * Description: <b>A postalCode specified in an address</b><br> 3335 * Type: <b>string</b><br> 3336 * Path: <b>Patient.address.postalCode</b><br> 3337 * </p> 3338 */ 3339 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3340 SP_ADDRESS_POSTALCODE); 3341 3342 /** 3343 * Search parameter: <b>address-country</b> 3344 * <p> 3345 * Description: <b>A country specified in an address</b><br> 3346 * Type: <b>string</b><br> 3347 * Path: <b>Patient.address.country</b><br> 3348 * </p> 3349 */ 3350 @SearchParamDefinition(name = "address-country", path = "Patient.address.country", description = "A country specified in an address", type = "string") 3351 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3352 /** 3353 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3354 * <p> 3355 * Description: <b>A country specified in an address</b><br> 3356 * Type: <b>string</b><br> 3357 * Path: <b>Patient.address.country</b><br> 3358 * </p> 3359 */ 3360 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3361 SP_ADDRESS_COUNTRY); 3362 3363 /** 3364 * Search parameter: <b>death-date</b> 3365 * <p> 3366 * Description: <b>The date of death has been provided and satisfies this search 3367 * value</b><br> 3368 * Type: <b>date</b><br> 3369 * Path: <b>Patient.deceasedDateTime</b><br> 3370 * </p> 3371 */ 3372 @SearchParamDefinition(name = "death-date", path = "(Patient.deceased as dateTime)", description = "The date of death has been provided and satisfies this search value", type = "date") 3373 public static final String SP_DEATH_DATE = "death-date"; 3374 /** 3375 * <b>Fluent Client</b> search parameter constant for <b>death-date</b> 3376 * <p> 3377 * Description: <b>The date of death has been provided and satisfies this search 3378 * value</b><br> 3379 * Type: <b>date</b><br> 3380 * Path: <b>Patient.deceasedDateTime</b><br> 3381 * </p> 3382 */ 3383 public static final ca.uhn.fhir.rest.gclient.DateClientParam DEATH_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3384 SP_DEATH_DATE); 3385 3386 /** 3387 * Search parameter: <b>phonetic</b> 3388 * <p> 3389 * Description: <b>A portion of either family or given name using some kind of 3390 * phonetic matching algorithm</b><br> 3391 * Type: <b>string</b><br> 3392 * Path: <b>Patient.name</b><br> 3393 * </p> 3394 */ 3395 @SearchParamDefinition(name = "phonetic", path = "Patient.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 3396 public static final String SP_PHONETIC = "phonetic"; 3397 /** 3398 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 3399 * <p> 3400 * Description: <b>A portion of either family or given name using some kind of 3401 * phonetic matching algorithm</b><br> 3402 * Type: <b>string</b><br> 3403 * Path: <b>Patient.name</b><br> 3404 * </p> 3405 */ 3406 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 3407 SP_PHONETIC); 3408 3409 /** 3410 * Search parameter: <b>phone</b> 3411 * <p> 3412 * Description: <b>A value in a phone contact</b><br> 3413 * Type: <b>token</b><br> 3414 * Path: <b>Patient.telecom(system=phone)</b><br> 3415 * </p> 3416 */ 3417 @SearchParamDefinition(name = "phone", path = "Patient.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 3418 public static final String SP_PHONE = "phone"; 3419 /** 3420 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 3421 * <p> 3422 * Description: <b>A value in a phone contact</b><br> 3423 * Type: <b>token</b><br> 3424 * Path: <b>Patient.telecom(system=phone)</b><br> 3425 * </p> 3426 */ 3427 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3428 SP_PHONE); 3429 3430 /** 3431 * Search parameter: <b>organization</b> 3432 * <p> 3433 * Description: <b>The organization that is the custodian of the patient 3434 * record</b><br> 3435 * Type: <b>reference</b><br> 3436 * Path: <b>Patient.managingOrganization</b><br> 3437 * </p> 3438 */ 3439 @SearchParamDefinition(name = "organization", path = "Patient.managingOrganization", description = "The organization that is the custodian of the patient record", type = "reference", target = { 3440 Organization.class }) 3441 public static final String SP_ORGANIZATION = "organization"; 3442 /** 3443 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3444 * <p> 3445 * Description: <b>The organization that is the custodian of the patient 3446 * record</b><br> 3447 * Type: <b>reference</b><br> 3448 * Path: <b>Patient.managingOrganization</b><br> 3449 * </p> 3450 */ 3451 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3452 SP_ORGANIZATION); 3453 3454 /** 3455 * Constant for fluent queries to be used to add include statements. Specifies 3456 * the path value of "<b>Patient:organization</b>". 3457 */ 3458 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3459 "Patient:organization").toLocked(); 3460 3461 /** 3462 * Search parameter: <b>name</b> 3463 * <p> 3464 * Description: <b>A server defined search that may match any of the string 3465 * fields in the HumanName, including family, give, prefix, suffix, suffix, 3466 * and/or text</b><br> 3467 * Type: <b>string</b><br> 3468 * Path: <b>Patient.name</b><br> 3469 * </p> 3470 */ 3471 @SearchParamDefinition(name = "name", path = "Patient.name", description = "A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type = "string") 3472 public static final String SP_NAME = "name"; 3473 /** 3474 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3475 * <p> 3476 * Description: <b>A server defined search that may match any of the string 3477 * fields in the HumanName, including family, give, prefix, suffix, suffix, 3478 * and/or text</b><br> 3479 * Type: <b>string</b><br> 3480 * Path: <b>Patient.name</b><br> 3481 * </p> 3482 */ 3483 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3484 SP_NAME); 3485 3486 /** 3487 * Search parameter: <b>address-use</b> 3488 * <p> 3489 * Description: <b>A use code specified in an address</b><br> 3490 * Type: <b>token</b><br> 3491 * Path: <b>Patient.address.use</b><br> 3492 * </p> 3493 */ 3494 @SearchParamDefinition(name = "address-use", path = "Patient.address.use", description = "A use code specified in an address", type = "token") 3495 public static final String SP_ADDRESS_USE = "address-use"; 3496 /** 3497 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3498 * <p> 3499 * Description: <b>A use code specified in an address</b><br> 3500 * Type: <b>token</b><br> 3501 * Path: <b>Patient.address.use</b><br> 3502 * </p> 3503 */ 3504 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3505 SP_ADDRESS_USE); 3506 3507 /** 3508 * Search parameter: <b>telecom</b> 3509 * <p> 3510 * Description: <b>The value in any kind of telecom details of the 3511 * patient</b><br> 3512 * Type: <b>token</b><br> 3513 * Path: <b>Patient.telecom</b><br> 3514 * </p> 3515 */ 3516 @SearchParamDefinition(name = "telecom", path = "Patient.telecom", description = "The value in any kind of telecom details of the patient", type = "token") 3517 public static final String SP_TELECOM = "telecom"; 3518 /** 3519 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 3520 * <p> 3521 * Description: <b>The value in any kind of telecom details of the 3522 * patient</b><br> 3523 * Type: <b>token</b><br> 3524 * Path: <b>Patient.telecom</b><br> 3525 * </p> 3526 */ 3527 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3528 SP_TELECOM); 3529 3530 /** 3531 * Search parameter: <b>family</b> 3532 * <p> 3533 * Description: <b>A portion of the family name of the patient</b><br> 3534 * Type: <b>string</b><br> 3535 * Path: <b>Patient.name.family</b><br> 3536 * </p> 3537 */ 3538 @SearchParamDefinition(name = "family", path = "Patient.name.family", description = "A portion of the family name of the patient", type = "string") 3539 public static final String SP_FAMILY = "family"; 3540 /** 3541 * <b>Fluent Client</b> search parameter constant for <b>family</b> 3542 * <p> 3543 * Description: <b>A portion of the family name of the patient</b><br> 3544 * Type: <b>string</b><br> 3545 * Path: <b>Patient.name.family</b><br> 3546 * </p> 3547 */ 3548 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3549 SP_FAMILY); 3550 3551 /** 3552 * Search parameter: <b>address-city</b> 3553 * <p> 3554 * Description: <b>A city specified in an address</b><br> 3555 * Type: <b>string</b><br> 3556 * Path: <b>Patient.address.city</b><br> 3557 * </p> 3558 */ 3559 @SearchParamDefinition(name = "address-city", path = "Patient.address.city", description = "A city specified in an address", type = "string") 3560 public static final String SP_ADDRESS_CITY = "address-city"; 3561 /** 3562 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3563 * <p> 3564 * Description: <b>A city specified in an address</b><br> 3565 * Type: <b>string</b><br> 3566 * Path: <b>Patient.address.city</b><br> 3567 * </p> 3568 */ 3569 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3570 SP_ADDRESS_CITY); 3571 3572 /** 3573 * Search parameter: <b>email</b> 3574 * <p> 3575 * Description: <b>A value in an email contact</b><br> 3576 * Type: <b>token</b><br> 3577 * Path: <b>Patient.telecom(system=email)</b><br> 3578 * </p> 3579 */ 3580 @SearchParamDefinition(name = "email", path = "Patient.telecom.where(system='email')", description = "A value in an email contact", type = "token") 3581 public static final String SP_EMAIL = "email"; 3582 /** 3583 * <b>Fluent Client</b> search parameter constant for <b>email</b> 3584 * <p> 3585 * Description: <b>A value in an email contact</b><br> 3586 * Type: <b>token</b><br> 3587 * Path: <b>Patient.telecom(system=email)</b><br> 3588 * </p> 3589 */ 3590 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3591 SP_EMAIL); 3592 3593}