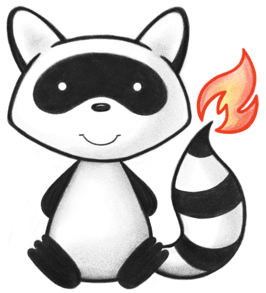
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.Date; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038 039import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * A time period defined by a start and end date and optionally time. 046 */ 047@DatatypeDef(name = "Period") 048public class Period extends Type implements ICompositeType { 049 050 /** 051 * The start of the period. The boundary is inclusive. 052 */ 053 @Child(name = "start", type = { DateTimeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "Starting time with inclusive boundary", formalDefinition = "The start of the period. The boundary is inclusive.") 055 protected DateTimeType start; 056 057 /** 058 * The end of the period. If the end of the period is missing, it means no end 059 * was known or planned at the time the instance was created. The start may be 060 * in the past, and the end date in the future, which means that period is 061 * expected/planned to end at that time. 062 */ 063 @Child(name = "end", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 064 @Description(shortDefinition = "End time with inclusive boundary, if not ongoing", formalDefinition = "The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.") 065 protected DateTimeType end; 066 067 private static final long serialVersionUID = 649791751L; 068 069 /** 070 * Constructor 071 */ 072 public Period() { 073 super(); 074 } 075 076 /** 077 * @return {@link #start} (The start of the period. The boundary is inclusive.). 078 * This is the underlying object with id, value and extensions. The 079 * accessor "getStart" gives direct access to the value 080 */ 081 public DateTimeType getStartElement() { 082 if (this.start == null) 083 if (Configuration.errorOnAutoCreate()) 084 throw new Error("Attempt to auto-create Period.start"); 085 else if (Configuration.doAutoCreate()) 086 this.start = new DateTimeType(); // bb 087 return this.start; 088 } 089 090 public boolean hasStartElement() { 091 return this.start != null && !this.start.isEmpty(); 092 } 093 094 public boolean hasStart() { 095 return this.start != null && !this.start.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #start} (The start of the period. The boundary is 100 * inclusive.). This is the underlying object with id, value and 101 * extensions. The accessor "getStart" gives direct access to the 102 * value 103 */ 104 public Period setStartElement(DateTimeType value) { 105 this.start = value; 106 return this; 107 } 108 109 /** 110 * @return The start of the period. The boundary is inclusive. 111 */ 112 public Date getStart() { 113 return this.start == null ? null : this.start.getValue(); 114 } 115 116 /** 117 * @param value The start of the period. The boundary is inclusive. 118 */ 119 public Period setStart(Date value) { 120 if (value == null) 121 this.start = null; 122 else { 123 if (this.start == null) 124 this.start = new DateTimeType(); 125 this.start.setValue(value); 126 } 127 return this; 128 } 129 130 /** 131 * @return {@link #end} (The end of the period. If the end of the period is 132 * missing, it means no end was known or planned at the time the 133 * instance was created. The start may be in the past, and the end date 134 * in the future, which means that period is expected/planned to end at 135 * that time.). This is the underlying object with id, value and 136 * extensions. The accessor "getEnd" gives direct access to the value 137 */ 138 public DateTimeType getEndElement() { 139 if (this.end == null) 140 if (Configuration.errorOnAutoCreate()) 141 throw new Error("Attempt to auto-create Period.end"); 142 else if (Configuration.doAutoCreate()) 143 this.end = new DateTimeType(); // bb 144 return this.end; 145 } 146 147 public boolean hasEndElement() { 148 return this.end != null && !this.end.isEmpty(); 149 } 150 151 public boolean hasEnd() { 152 return this.end != null && !this.end.isEmpty(); 153 } 154 155 /** 156 * @param value {@link #end} (The end of the period. If the end of the period is 157 * missing, it means no end was known or planned at the time the 158 * instance was created. The start may be in the past, and the end 159 * date in the future, which means that period is expected/planned 160 * to end at that time.). This is the underlying object with id, 161 * value and extensions. The accessor "getEnd" gives direct access 162 * to the value 163 */ 164 public Period setEndElement(DateTimeType value) { 165 this.end = value; 166 return this; 167 } 168 169 /** 170 * @return The end of the period. If the end of the period is missing, it means 171 * no end was known or planned at the time the instance was created. The 172 * start may be in the past, and the end date in the future, which means 173 * that period is expected/planned to end at that time. 174 */ 175 public Date getEnd() { 176 return this.end == null ? null : this.end.getValue(); 177 } 178 179 /** 180 * @param value The end of the period. If the end of the period is missing, it 181 * means no end was known or planned at the time the instance was 182 * created. The start may be in the past, and the end date in the 183 * future, which means that period is expected/planned to end at 184 * that time. 185 */ 186 public Period setEnd(Date value) { 187 if (value == null) 188 this.end = null; 189 else { 190 if (this.end == null) 191 this.end = new DateTimeType(); 192 this.end.setValue(value); 193 } 194 return this; 195 } 196 197 /** 198 * Sets the value for <b>start</b> () 199 * 200 * <p> 201 * <b>Definition:</b> The start of the period. The boundary is inclusive. 202 * </p> 203 */ 204 public Period setStart(Date theDate, TemporalPrecisionEnum thePrecision) { 205 start = new DateTimeType(theDate, thePrecision); 206 return this; 207 } 208 209 /** 210 * Sets the value for <b>end</b> () 211 * 212 * <p> 213 * <b>Definition:</b> The end of the period. The boundary is inclusive. 214 * </p> 215 */ 216 public Period setEnd(Date theDate, TemporalPrecisionEnum thePrecision) { 217 end = new DateTimeType(theDate, thePrecision); 218 return this; 219 } 220 221 protected void listChildren(List<Property> children) { 222 super.listChildren(children); 223 children.add(new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 1, start)); 224 children.add(new Property("end", "dateTime", 225 "The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 226 0, 1, end)); 227 } 228 229 @Override 230 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 231 switch (_hash) { 232 case 109757538: 233 /* start */ return new Property("start", "dateTime", "The start of the period. The boundary is inclusive.", 0, 1, 234 start); 235 case 100571: 236 /* end */ return new Property("end", "dateTime", 237 "The end of the period. If the end of the period is missing, it means no end was known or planned at the time the instance was created. The start may be in the past, and the end date in the future, which means that period is expected/planned to end at that time.", 238 0, 1, end); 239 default: 240 return super.getNamedProperty(_hash, _name, _checkValid); 241 } 242 243 } 244 245 @Override 246 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 247 switch (hash) { 248 case 109757538: 249 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // DateTimeType 250 case 100571: 251 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // DateTimeType 252 default: 253 return super.getProperty(hash, name, checkValid); 254 } 255 256 } 257 258 @Override 259 public Base setProperty(int hash, String name, Base value) throws FHIRException { 260 switch (hash) { 261 case 109757538: // start 262 this.start = castToDateTime(value); // DateTimeType 263 return value; 264 case 100571: // end 265 this.end = castToDateTime(value); // DateTimeType 266 return value; 267 default: 268 return super.setProperty(hash, name, value); 269 } 270 271 } 272 273 @Override 274 public Base setProperty(String name, Base value) throws FHIRException { 275 if (name.equals("start")) { 276 this.start = castToDateTime(value); // DateTimeType 277 } else if (name.equals("end")) { 278 this.end = castToDateTime(value); // DateTimeType 279 } else 280 return super.setProperty(name, value); 281 return value; 282 } 283 284 @Override 285 public void removeChild(String name, Base value) throws FHIRException { 286 if (name.equals("start")) { 287 this.start = null; 288 } else if (name.equals("end")) { 289 this.end = null; 290 } else 291 super.removeChild(name, value); 292 293 } 294 295 @Override 296 public Base makeProperty(int hash, String name) throws FHIRException { 297 switch (hash) { 298 case 109757538: 299 return getStartElement(); 300 case 100571: 301 return getEndElement(); 302 default: 303 return super.makeProperty(hash, name); 304 } 305 306 } 307 308 @Override 309 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 310 switch (hash) { 311 case 109757538: 312 /* start */ return new String[] { "dateTime" }; 313 case 100571: 314 /* end */ return new String[] { "dateTime" }; 315 default: 316 return super.getTypesForProperty(hash, name); 317 } 318 319 } 320 321 @Override 322 public Base addChild(String name) throws FHIRException { 323 if (name.equals("start")) { 324 throw new FHIRException("Cannot call addChild on a singleton property Period.start"); 325 } else if (name.equals("end")) { 326 throw new FHIRException("Cannot call addChild on a singleton property Period.end"); 327 } else 328 return super.addChild(name); 329 } 330 331 public String fhirType() { 332 return "Period"; 333 334 } 335 336 public Period copy() { 337 Period dst = new Period(); 338 copyValues(dst); 339 return dst; 340 } 341 342 public void copyValues(Period dst) { 343 super.copyValues(dst); 344 dst.start = start == null ? null : start.copy(); 345 dst.end = end == null ? null : end.copy(); 346 } 347 348 protected Period typedCopy() { 349 return copy(); 350 } 351 352 @Override 353 public boolean equalsDeep(Base other_) { 354 if (!super.equalsDeep(other_)) 355 return false; 356 if (!(other_ instanceof Period)) 357 return false; 358 Period o = (Period) other_; 359 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 360 } 361 362 @Override 363 public boolean equalsShallow(Base other_) { 364 if (!super.equalsShallow(other_)) 365 return false; 366 if (!(other_ instanceof Period)) 367 return false; 368 Period o = (Period) other_; 369 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 370 } 371 372 public boolean isEmpty() { 373 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 374 } 375 376}