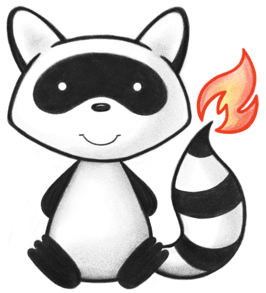
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Demographics and administrative information about a person independent of a 050 * specific health-related context. 051 */ 052@ResourceDef(name = "Person", profile = "http://hl7.org/fhir/StructureDefinition/Person") 053public class Person extends DomainResource { 054 055 public enum IdentityAssuranceLevel { 056 /** 057 * Little or no confidence in the asserted identity's accuracy. 058 */ 059 LEVEL1, 060 /** 061 * Some confidence in the asserted identity's accuracy. 062 */ 063 LEVEL2, 064 /** 065 * High confidence in the asserted identity's accuracy. 066 */ 067 LEVEL3, 068 /** 069 * Very high confidence in the asserted identity's accuracy. 070 */ 071 LEVEL4, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 077 public static IdentityAssuranceLevel fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("level1".equals(codeString)) 081 return LEVEL1; 082 if ("level2".equals(codeString)) 083 return LEVEL2; 084 if ("level3".equals(codeString)) 085 return LEVEL3; 086 if ("level4".equals(codeString)) 087 return LEVEL4; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown IdentityAssuranceLevel code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case LEVEL1: 097 return "level1"; 098 case LEVEL2: 099 return "level2"; 100 case LEVEL3: 101 return "level3"; 102 case LEVEL4: 103 return "level4"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 switch (this) { 113 case LEVEL1: 114 return "http://hl7.org/fhir/identity-assuranceLevel"; 115 case LEVEL2: 116 return "http://hl7.org/fhir/identity-assuranceLevel"; 117 case LEVEL3: 118 return "http://hl7.org/fhir/identity-assuranceLevel"; 119 case LEVEL4: 120 return "http://hl7.org/fhir/identity-assuranceLevel"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case LEVEL1: 131 return "Little or no confidence in the asserted identity's accuracy."; 132 case LEVEL2: 133 return "Some confidence in the asserted identity's accuracy."; 134 case LEVEL3: 135 return "High confidence in the asserted identity's accuracy."; 136 case LEVEL4: 137 return "Very high confidence in the asserted identity's accuracy."; 138 case NULL: 139 return null; 140 default: 141 return "?"; 142 } 143 } 144 145 public String getDisplay() { 146 switch (this) { 147 case LEVEL1: 148 return "Level 1"; 149 case LEVEL2: 150 return "Level 2"; 151 case LEVEL3: 152 return "Level 3"; 153 case LEVEL4: 154 return "Level 4"; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 } 162 163 public static class IdentityAssuranceLevelEnumFactory implements EnumFactory<IdentityAssuranceLevel> { 164 public IdentityAssuranceLevel fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("level1".equals(codeString)) 169 return IdentityAssuranceLevel.LEVEL1; 170 if ("level2".equals(codeString)) 171 return IdentityAssuranceLevel.LEVEL2; 172 if ("level3".equals(codeString)) 173 return IdentityAssuranceLevel.LEVEL3; 174 if ("level4".equals(codeString)) 175 return IdentityAssuranceLevel.LEVEL4; 176 throw new IllegalArgumentException("Unknown IdentityAssuranceLevel code '" + codeString + "'"); 177 } 178 179 public Enumeration<IdentityAssuranceLevel> fromType(PrimitiveType<?> code) throws FHIRException { 180 if (code == null) 181 return null; 182 if (code.isEmpty()) 183 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.NULL, code); 184 String codeString = code.asStringValue(); 185 if (codeString == null || "".equals(codeString)) 186 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.NULL, code); 187 if ("level1".equals(codeString)) 188 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL1, code); 189 if ("level2".equals(codeString)) 190 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL2, code); 191 if ("level3".equals(codeString)) 192 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL3, code); 193 if ("level4".equals(codeString)) 194 return new Enumeration<IdentityAssuranceLevel>(this, IdentityAssuranceLevel.LEVEL4, code); 195 throw new FHIRException("Unknown IdentityAssuranceLevel code '" + codeString + "'"); 196 } 197 198 public String toCode(IdentityAssuranceLevel code) { 199 if (code == IdentityAssuranceLevel.NULL) 200 return null; 201 if (code == IdentityAssuranceLevel.LEVEL1) 202 return "level1"; 203 if (code == IdentityAssuranceLevel.LEVEL2) 204 return "level2"; 205 if (code == IdentityAssuranceLevel.LEVEL3) 206 return "level3"; 207 if (code == IdentityAssuranceLevel.LEVEL4) 208 return "level4"; 209 return "?"; 210 } 211 212 public String toSystem(IdentityAssuranceLevel code) { 213 return code.getSystem(); 214 } 215 } 216 217 @Block() 218 public static class PersonLinkComponent extends BackboneElement implements IBaseBackboneElement { 219 /** 220 * The resource to which this actual person is associated. 221 */ 222 @Child(name = "target", type = { Patient.class, Practitioner.class, RelatedPerson.class, 223 Person.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 224 @Description(shortDefinition = "The resource to which this actual person is associated", formalDefinition = "The resource to which this actual person is associated.") 225 protected Reference target; 226 227 /** 228 * The actual object that is the target of the reference (The resource to which 229 * this actual person is associated.) 230 */ 231 protected Resource targetTarget; 232 233 /** 234 * Level of assurance that this link is associated with the target resource. 235 */ 236 @Child(name = "assurance", type = { 237 CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 238 @Description(shortDefinition = "level1 | level2 | level3 | level4", formalDefinition = "Level of assurance that this link is associated with the target resource.") 239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/identity-assuranceLevel") 240 protected Enumeration<IdentityAssuranceLevel> assurance; 241 242 private static final long serialVersionUID = 508763647L; 243 244 /** 245 * Constructor 246 */ 247 public PersonLinkComponent() { 248 super(); 249 } 250 251 /** 252 * Constructor 253 */ 254 public PersonLinkComponent(Reference target) { 255 super(); 256 this.target = target; 257 } 258 259 /** 260 * @return {@link #target} (The resource to which this actual person is 261 * associated.) 262 */ 263 public Reference getTarget() { 264 if (this.target == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create PersonLinkComponent.target"); 267 else if (Configuration.doAutoCreate()) 268 this.target = new Reference(); // cc 269 return this.target; 270 } 271 272 public boolean hasTarget() { 273 return this.target != null && !this.target.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #target} (The resource to which this actual person is 278 * associated.) 279 */ 280 public PersonLinkComponent setTarget(Reference value) { 281 this.target = value; 282 return this; 283 } 284 285 /** 286 * @return {@link #target} The actual object that is the target of the 287 * reference. The reference library doesn't populate this, but you can 288 * use it to hold the resource if you resolve it. (The resource to which 289 * this actual person is associated.) 290 */ 291 public Resource getTargetTarget() { 292 return this.targetTarget; 293 } 294 295 /** 296 * @param value {@link #target} The actual object that is the target of the 297 * reference. The reference library doesn't use these, but you can 298 * use it to hold the resource if you resolve it. (The resource to 299 * which this actual person is associated.) 300 */ 301 public PersonLinkComponent setTargetTarget(Resource value) { 302 this.targetTarget = value; 303 return this; 304 } 305 306 /** 307 * @return {@link #assurance} (Level of assurance that this link is associated 308 * with the target resource.). This is the underlying object with id, 309 * value and extensions. The accessor "getAssurance" gives direct access 310 * to the value 311 */ 312 public Enumeration<IdentityAssuranceLevel> getAssuranceElement() { 313 if (this.assurance == null) 314 if (Configuration.errorOnAutoCreate()) 315 throw new Error("Attempt to auto-create PersonLinkComponent.assurance"); 316 else if (Configuration.doAutoCreate()) 317 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); // bb 318 return this.assurance; 319 } 320 321 public boolean hasAssuranceElement() { 322 return this.assurance != null && !this.assurance.isEmpty(); 323 } 324 325 public boolean hasAssurance() { 326 return this.assurance != null && !this.assurance.isEmpty(); 327 } 328 329 /** 330 * @param value {@link #assurance} (Level of assurance that this link is 331 * associated with the target resource.). This is the underlying 332 * object with id, value and extensions. The accessor 333 * "getAssurance" gives direct access to the value 334 */ 335 public PersonLinkComponent setAssuranceElement(Enumeration<IdentityAssuranceLevel> value) { 336 this.assurance = value; 337 return this; 338 } 339 340 /** 341 * @return Level of assurance that this link is associated with the target 342 * resource. 343 */ 344 public IdentityAssuranceLevel getAssurance() { 345 return this.assurance == null ? null : this.assurance.getValue(); 346 } 347 348 /** 349 * @param value Level of assurance that this link is associated with the target 350 * resource. 351 */ 352 public PersonLinkComponent setAssurance(IdentityAssuranceLevel value) { 353 if (value == null) 354 this.assurance = null; 355 else { 356 if (this.assurance == null) 357 this.assurance = new Enumeration<IdentityAssuranceLevel>(new IdentityAssuranceLevelEnumFactory()); 358 this.assurance.setValue(value); 359 } 360 return this; 361 } 362 363 protected void listChildren(List<Property> children) { 364 super.listChildren(children); 365 children.add(new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", 366 "The resource to which this actual person is associated.", 0, 1, target)); 367 children.add(new Property("assurance", "code", 368 "Level of assurance that this link is associated with the target resource.", 0, 1, assurance)); 369 } 370 371 @Override 372 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 373 switch (_hash) { 374 case -880905839: 375 /* target */ return new Property("target", "Reference(Patient|Practitioner|RelatedPerson|Person)", 376 "The resource to which this actual person is associated.", 0, 1, target); 377 case 1771900717: 378 /* assurance */ return new Property("assurance", "code", 379 "Level of assurance that this link is associated with the target resource.", 0, 1, assurance); 380 default: 381 return super.getNamedProperty(_hash, _name, _checkValid); 382 } 383 384 } 385 386 @Override 387 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 388 switch (hash) { 389 case -880905839: 390 /* target */ return this.target == null ? new Base[0] : new Base[] { this.target }; // Reference 391 case 1771900717: 392 /* assurance */ return this.assurance == null ? new Base[0] : new Base[] { this.assurance }; // Enumeration<IdentityAssuranceLevel> 393 default: 394 return super.getProperty(hash, name, checkValid); 395 } 396 397 } 398 399 @Override 400 public Base setProperty(int hash, String name, Base value) throws FHIRException { 401 switch (hash) { 402 case -880905839: // target 403 this.target = castToReference(value); // Reference 404 return value; 405 case 1771900717: // assurance 406 value = new IdentityAssuranceLevelEnumFactory().fromType(castToCode(value)); 407 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 408 return value; 409 default: 410 return super.setProperty(hash, name, value); 411 } 412 413 } 414 415 @Override 416 public Base setProperty(String name, Base value) throws FHIRException { 417 if (name.equals("target")) { 418 this.target = castToReference(value); // Reference 419 } else if (name.equals("assurance")) { 420 value = new IdentityAssuranceLevelEnumFactory().fromType(castToCode(value)); 421 this.assurance = (Enumeration) value; // Enumeration<IdentityAssuranceLevel> 422 } else 423 return super.setProperty(name, value); 424 return value; 425 } 426 427 @Override 428 public void removeChild(String name, Base value) throws FHIRException { 429 if (name.equals("target")) { 430 this.target = null; 431 } else if (name.equals("assurance")) { 432 this.assurance = null; 433 } else 434 super.removeChild(name, value); 435 436 } 437 438 @Override 439 public Base makeProperty(int hash, String name) throws FHIRException { 440 switch (hash) { 441 case -880905839: 442 return getTarget(); 443 case 1771900717: 444 return getAssuranceElement(); 445 default: 446 return super.makeProperty(hash, name); 447 } 448 449 } 450 451 @Override 452 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 453 switch (hash) { 454 case -880905839: 455 /* target */ return new String[] { "Reference" }; 456 case 1771900717: 457 /* assurance */ return new String[] { "code" }; 458 default: 459 return super.getTypesForProperty(hash, name); 460 } 461 462 } 463 464 @Override 465 public Base addChild(String name) throws FHIRException { 466 if (name.equals("target")) { 467 this.target = new Reference(); 468 return this.target; 469 } else if (name.equals("assurance")) { 470 throw new FHIRException("Cannot call addChild on a singleton property Person.assurance"); 471 } else 472 return super.addChild(name); 473 } 474 475 public PersonLinkComponent copy() { 476 PersonLinkComponent dst = new PersonLinkComponent(); 477 copyValues(dst); 478 return dst; 479 } 480 481 public void copyValues(PersonLinkComponent dst) { 482 super.copyValues(dst); 483 dst.target = target == null ? null : target.copy(); 484 dst.assurance = assurance == null ? null : assurance.copy(); 485 } 486 487 @Override 488 public boolean equalsDeep(Base other_) { 489 if (!super.equalsDeep(other_)) 490 return false; 491 if (!(other_ instanceof PersonLinkComponent)) 492 return false; 493 PersonLinkComponent o = (PersonLinkComponent) other_; 494 return compareDeep(target, o.target, true) && compareDeep(assurance, o.assurance, true); 495 } 496 497 @Override 498 public boolean equalsShallow(Base other_) { 499 if (!super.equalsShallow(other_)) 500 return false; 501 if (!(other_ instanceof PersonLinkComponent)) 502 return false; 503 PersonLinkComponent o = (PersonLinkComponent) other_; 504 return compareValues(assurance, o.assurance, true); 505 } 506 507 public boolean isEmpty() { 508 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(target, assurance); 509 } 510 511 public String fhirType() { 512 return "Person.link"; 513 514 } 515 516 } 517 518 /** 519 * Identifier for a person within a particular scope. 520 */ 521 @Child(name = "identifier", type = { 522 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 523 @Description(shortDefinition = "A human identifier for this person", formalDefinition = "Identifier for a person within a particular scope.") 524 protected List<Identifier> identifier; 525 526 /** 527 * A name associated with the person. 528 */ 529 @Child(name = "name", type = { 530 HumanName.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 531 @Description(shortDefinition = "A name associated with the person", formalDefinition = "A name associated with the person.") 532 protected List<HumanName> name; 533 534 /** 535 * A contact detail for the person, e.g. a telephone number or an email address. 536 */ 537 @Child(name = "telecom", type = { 538 ContactPoint.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 539 @Description(shortDefinition = "A contact detail for the person", formalDefinition = "A contact detail for the person, e.g. a telephone number or an email address.") 540 protected List<ContactPoint> telecom; 541 542 /** 543 * Administrative Gender. 544 */ 545 @Child(name = "gender", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 546 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender.") 547 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 548 protected Enumeration<AdministrativeGender> gender; 549 550 /** 551 * The birth date for the person. 552 */ 553 @Child(name = "birthDate", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 554 @Description(shortDefinition = "The date on which the person was born", formalDefinition = "The birth date for the person.") 555 protected DateType birthDate; 556 557 /** 558 * One or more addresses for the person. 559 */ 560 @Child(name = "address", type = { 561 Address.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 562 @Description(shortDefinition = "One or more addresses for the person", formalDefinition = "One or more addresses for the person.") 563 protected List<Address> address; 564 565 /** 566 * An image that can be displayed as a thumbnail of the person to enhance the 567 * identification of the individual. 568 */ 569 @Child(name = "photo", type = { Attachment.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 570 @Description(shortDefinition = "Image of the person", formalDefinition = "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.") 571 protected Attachment photo; 572 573 /** 574 * The organization that is the custodian of the person record. 575 */ 576 @Child(name = "managingOrganization", type = { 577 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 578 @Description(shortDefinition = "The organization that is the custodian of the person record", formalDefinition = "The organization that is the custodian of the person record.") 579 protected Reference managingOrganization; 580 581 /** 582 * The actual object that is the target of the reference (The organization that 583 * is the custodian of the person record.) 584 */ 585 protected Organization managingOrganizationTarget; 586 587 /** 588 * Whether this person's record is in active use. 589 */ 590 @Child(name = "active", type = { BooleanType.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 591 @Description(shortDefinition = "This person's record is in active use", formalDefinition = "Whether this person's record is in active use.") 592 protected BooleanType active; 593 594 /** 595 * Link to a resource that concerns the same actual person. 596 */ 597 @Child(name = "link", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 598 @Description(shortDefinition = "Link to a resource that concerns the same actual person", formalDefinition = "Link to a resource that concerns the same actual person.") 599 protected List<PersonLinkComponent> link; 600 601 private static final long serialVersionUID = -117464654L; 602 603 /** 604 * Constructor 605 */ 606 public Person() { 607 super(); 608 } 609 610 /** 611 * @return {@link #identifier} (Identifier for a person within a particular 612 * scope.) 613 */ 614 public List<Identifier> getIdentifier() { 615 if (this.identifier == null) 616 this.identifier = new ArrayList<Identifier>(); 617 return this.identifier; 618 } 619 620 /** 621 * @return Returns a reference to <code>this</code> for easy method chaining 622 */ 623 public Person setIdentifier(List<Identifier> theIdentifier) { 624 this.identifier = theIdentifier; 625 return this; 626 } 627 628 public boolean hasIdentifier() { 629 if (this.identifier == null) 630 return false; 631 for (Identifier item : this.identifier) 632 if (!item.isEmpty()) 633 return true; 634 return false; 635 } 636 637 public Identifier addIdentifier() { // 3 638 Identifier t = new Identifier(); 639 if (this.identifier == null) 640 this.identifier = new ArrayList<Identifier>(); 641 this.identifier.add(t); 642 return t; 643 } 644 645 public Person addIdentifier(Identifier t) { // 3 646 if (t == null) 647 return this; 648 if (this.identifier == null) 649 this.identifier = new ArrayList<Identifier>(); 650 this.identifier.add(t); 651 return this; 652 } 653 654 /** 655 * @return The first repetition of repeating field {@link #identifier}, creating 656 * it if it does not already exist 657 */ 658 public Identifier getIdentifierFirstRep() { 659 if (getIdentifier().isEmpty()) { 660 addIdentifier(); 661 } 662 return getIdentifier().get(0); 663 } 664 665 /** 666 * @return {@link #name} (A name associated with the person.) 667 */ 668 public List<HumanName> getName() { 669 if (this.name == null) 670 this.name = new ArrayList<HumanName>(); 671 return this.name; 672 } 673 674 /** 675 * @return Returns a reference to <code>this</code> for easy method chaining 676 */ 677 public Person setName(List<HumanName> theName) { 678 this.name = theName; 679 return this; 680 } 681 682 public boolean hasName() { 683 if (this.name == null) 684 return false; 685 for (HumanName item : this.name) 686 if (!item.isEmpty()) 687 return true; 688 return false; 689 } 690 691 public HumanName addName() { // 3 692 HumanName t = new HumanName(); 693 if (this.name == null) 694 this.name = new ArrayList<HumanName>(); 695 this.name.add(t); 696 return t; 697 } 698 699 public Person addName(HumanName t) { // 3 700 if (t == null) 701 return this; 702 if (this.name == null) 703 this.name = new ArrayList<HumanName>(); 704 this.name.add(t); 705 return this; 706 } 707 708 /** 709 * @return The first repetition of repeating field {@link #name}, creating it if 710 * it does not already exist 711 */ 712 public HumanName getNameFirstRep() { 713 if (getName().isEmpty()) { 714 addName(); 715 } 716 return getName().get(0); 717 } 718 719 /** 720 * @return {@link #telecom} (A contact detail for the person, e.g. a telephone 721 * number or an email address.) 722 */ 723 public List<ContactPoint> getTelecom() { 724 if (this.telecom == null) 725 this.telecom = new ArrayList<ContactPoint>(); 726 return this.telecom; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public Person setTelecom(List<ContactPoint> theTelecom) { 733 this.telecom = theTelecom; 734 return this; 735 } 736 737 public boolean hasTelecom() { 738 if (this.telecom == null) 739 return false; 740 for (ContactPoint item : this.telecom) 741 if (!item.isEmpty()) 742 return true; 743 return false; 744 } 745 746 public ContactPoint addTelecom() { // 3 747 ContactPoint t = new ContactPoint(); 748 if (this.telecom == null) 749 this.telecom = new ArrayList<ContactPoint>(); 750 this.telecom.add(t); 751 return t; 752 } 753 754 public Person addTelecom(ContactPoint t) { // 3 755 if (t == null) 756 return this; 757 if (this.telecom == null) 758 this.telecom = new ArrayList<ContactPoint>(); 759 this.telecom.add(t); 760 return this; 761 } 762 763 /** 764 * @return The first repetition of repeating field {@link #telecom}, creating it 765 * if it does not already exist 766 */ 767 public ContactPoint getTelecomFirstRep() { 768 if (getTelecom().isEmpty()) { 769 addTelecom(); 770 } 771 return getTelecom().get(0); 772 } 773 774 /** 775 * @return {@link #gender} (Administrative Gender.). This is the underlying 776 * object with id, value and extensions. The accessor "getGender" gives 777 * direct access to the value 778 */ 779 public Enumeration<AdministrativeGender> getGenderElement() { 780 if (this.gender == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create Person.gender"); 783 else if (Configuration.doAutoCreate()) 784 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 785 return this.gender; 786 } 787 788 public boolean hasGenderElement() { 789 return this.gender != null && !this.gender.isEmpty(); 790 } 791 792 public boolean hasGender() { 793 return this.gender != null && !this.gender.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #gender} (Administrative Gender.). This is the underlying 798 * object with id, value and extensions. The accessor "getGender" 799 * gives direct access to the value 800 */ 801 public Person setGenderElement(Enumeration<AdministrativeGender> value) { 802 this.gender = value; 803 return this; 804 } 805 806 /** 807 * @return Administrative Gender. 808 */ 809 public AdministrativeGender getGender() { 810 return this.gender == null ? null : this.gender.getValue(); 811 } 812 813 /** 814 * @param value Administrative Gender. 815 */ 816 public Person setGender(AdministrativeGender value) { 817 if (value == null) 818 this.gender = null; 819 else { 820 if (this.gender == null) 821 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 822 this.gender.setValue(value); 823 } 824 return this; 825 } 826 827 /** 828 * @return {@link #birthDate} (The birth date for the person.). This is the 829 * underlying object with id, value and extensions. The accessor 830 * "getBirthDate" gives direct access to the value 831 */ 832 public DateType getBirthDateElement() { 833 if (this.birthDate == null) 834 if (Configuration.errorOnAutoCreate()) 835 throw new Error("Attempt to auto-create Person.birthDate"); 836 else if (Configuration.doAutoCreate()) 837 this.birthDate = new DateType(); // bb 838 return this.birthDate; 839 } 840 841 public boolean hasBirthDateElement() { 842 return this.birthDate != null && !this.birthDate.isEmpty(); 843 } 844 845 public boolean hasBirthDate() { 846 return this.birthDate != null && !this.birthDate.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #birthDate} (The birth date for the person.). This is the 851 * underlying object with id, value and extensions. The accessor 852 * "getBirthDate" gives direct access to the value 853 */ 854 public Person setBirthDateElement(DateType value) { 855 this.birthDate = value; 856 return this; 857 } 858 859 /** 860 * @return The birth date for the person. 861 */ 862 public Date getBirthDate() { 863 return this.birthDate == null ? null : this.birthDate.getValue(); 864 } 865 866 /** 867 * @param value The birth date for the person. 868 */ 869 public Person setBirthDate(Date value) { 870 if (value == null) 871 this.birthDate = null; 872 else { 873 if (this.birthDate == null) 874 this.birthDate = new DateType(); 875 this.birthDate.setValue(value); 876 } 877 return this; 878 } 879 880 /** 881 * @return {@link #address} (One or more addresses for the person.) 882 */ 883 public List<Address> getAddress() { 884 if (this.address == null) 885 this.address = new ArrayList<Address>(); 886 return this.address; 887 } 888 889 /** 890 * @return Returns a reference to <code>this</code> for easy method chaining 891 */ 892 public Person setAddress(List<Address> theAddress) { 893 this.address = theAddress; 894 return this; 895 } 896 897 public boolean hasAddress() { 898 if (this.address == null) 899 return false; 900 for (Address item : this.address) 901 if (!item.isEmpty()) 902 return true; 903 return false; 904 } 905 906 public Address addAddress() { // 3 907 Address t = new Address(); 908 if (this.address == null) 909 this.address = new ArrayList<Address>(); 910 this.address.add(t); 911 return t; 912 } 913 914 public Person addAddress(Address t) { // 3 915 if (t == null) 916 return this; 917 if (this.address == null) 918 this.address = new ArrayList<Address>(); 919 this.address.add(t); 920 return this; 921 } 922 923 /** 924 * @return The first repetition of repeating field {@link #address}, creating it 925 * if it does not already exist 926 */ 927 public Address getAddressFirstRep() { 928 if (getAddress().isEmpty()) { 929 addAddress(); 930 } 931 return getAddress().get(0); 932 } 933 934 /** 935 * @return {@link #photo} (An image that can be displayed as a thumbnail of the 936 * person to enhance the identification of the individual.) 937 */ 938 public Attachment getPhoto() { 939 if (this.photo == null) 940 if (Configuration.errorOnAutoCreate()) 941 throw new Error("Attempt to auto-create Person.photo"); 942 else if (Configuration.doAutoCreate()) 943 this.photo = new Attachment(); // cc 944 return this.photo; 945 } 946 947 public boolean hasPhoto() { 948 return this.photo != null && !this.photo.isEmpty(); 949 } 950 951 /** 952 * @param value {@link #photo} (An image that can be displayed as a thumbnail of 953 * the person to enhance the identification of the individual.) 954 */ 955 public Person setPhoto(Attachment value) { 956 this.photo = value; 957 return this; 958 } 959 960 /** 961 * @return {@link #managingOrganization} (The organization that is the custodian 962 * of the person record.) 963 */ 964 public Reference getManagingOrganization() { 965 if (this.managingOrganization == null) 966 if (Configuration.errorOnAutoCreate()) 967 throw new Error("Attempt to auto-create Person.managingOrganization"); 968 else if (Configuration.doAutoCreate()) 969 this.managingOrganization = new Reference(); // cc 970 return this.managingOrganization; 971 } 972 973 public boolean hasManagingOrganization() { 974 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 975 } 976 977 /** 978 * @param value {@link #managingOrganization} (The organization that is the 979 * custodian of the person record.) 980 */ 981 public Person setManagingOrganization(Reference value) { 982 this.managingOrganization = value; 983 return this; 984 } 985 986 /** 987 * @return {@link #managingOrganization} The actual object that is the target of 988 * the reference. The reference library doesn't populate this, but you 989 * can use it to hold the resource if you resolve it. (The organization 990 * that is the custodian of the person record.) 991 */ 992 public Organization getManagingOrganizationTarget() { 993 if (this.managingOrganizationTarget == null) 994 if (Configuration.errorOnAutoCreate()) 995 throw new Error("Attempt to auto-create Person.managingOrganization"); 996 else if (Configuration.doAutoCreate()) 997 this.managingOrganizationTarget = new Organization(); // aa 998 return this.managingOrganizationTarget; 999 } 1000 1001 /** 1002 * @param value {@link #managingOrganization} The actual object that is the 1003 * target of the reference. The reference library doesn't use 1004 * these, but you can use it to hold the resource if you resolve 1005 * it. (The organization that is the custodian of the person 1006 * record.) 1007 */ 1008 public Person setManagingOrganizationTarget(Organization value) { 1009 this.managingOrganizationTarget = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return {@link #active} (Whether this person's record is in active use.). 1015 * This is the underlying object with id, value and extensions. The 1016 * accessor "getActive" gives direct access to the value 1017 */ 1018 public BooleanType getActiveElement() { 1019 if (this.active == null) 1020 if (Configuration.errorOnAutoCreate()) 1021 throw new Error("Attempt to auto-create Person.active"); 1022 else if (Configuration.doAutoCreate()) 1023 this.active = new BooleanType(); // bb 1024 return this.active; 1025 } 1026 1027 public boolean hasActiveElement() { 1028 return this.active != null && !this.active.isEmpty(); 1029 } 1030 1031 public boolean hasActive() { 1032 return this.active != null && !this.active.isEmpty(); 1033 } 1034 1035 /** 1036 * @param value {@link #active} (Whether this person's record is in active 1037 * use.). This is the underlying object with id, value and 1038 * extensions. The accessor "getActive" gives direct access to the 1039 * value 1040 */ 1041 public Person setActiveElement(BooleanType value) { 1042 this.active = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return Whether this person's record is in active use. 1048 */ 1049 public boolean getActive() { 1050 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1051 } 1052 1053 /** 1054 * @param value Whether this person's record is in active use. 1055 */ 1056 public Person setActive(boolean value) { 1057 if (this.active == null) 1058 this.active = new BooleanType(); 1059 this.active.setValue(value); 1060 return this; 1061 } 1062 1063 /** 1064 * @return {@link #link} (Link to a resource that concerns the same actual 1065 * person.) 1066 */ 1067 public List<PersonLinkComponent> getLink() { 1068 if (this.link == null) 1069 this.link = new ArrayList<PersonLinkComponent>(); 1070 return this.link; 1071 } 1072 1073 /** 1074 * @return Returns a reference to <code>this</code> for easy method chaining 1075 */ 1076 public Person setLink(List<PersonLinkComponent> theLink) { 1077 this.link = theLink; 1078 return this; 1079 } 1080 1081 public boolean hasLink() { 1082 if (this.link == null) 1083 return false; 1084 for (PersonLinkComponent item : this.link) 1085 if (!item.isEmpty()) 1086 return true; 1087 return false; 1088 } 1089 1090 public PersonLinkComponent addLink() { // 3 1091 PersonLinkComponent t = new PersonLinkComponent(); 1092 if (this.link == null) 1093 this.link = new ArrayList<PersonLinkComponent>(); 1094 this.link.add(t); 1095 return t; 1096 } 1097 1098 public Person addLink(PersonLinkComponent t) { // 3 1099 if (t == null) 1100 return this; 1101 if (this.link == null) 1102 this.link = new ArrayList<PersonLinkComponent>(); 1103 this.link.add(t); 1104 return this; 1105 } 1106 1107 /** 1108 * @return The first repetition of repeating field {@link #link}, creating it if 1109 * it does not already exist 1110 */ 1111 public PersonLinkComponent getLinkFirstRep() { 1112 if (getLink().isEmpty()) { 1113 addLink(); 1114 } 1115 return getLink().get(0); 1116 } 1117 1118 protected void listChildren(List<Property> children) { 1119 super.listChildren(children); 1120 children.add(new Property("identifier", "Identifier", "Identifier for a person within a particular scope.", 0, 1121 java.lang.Integer.MAX_VALUE, identifier)); 1122 children.add( 1123 new Property("name", "HumanName", "A name associated with the person.", 0, java.lang.Integer.MAX_VALUE, name)); 1124 children.add(new Property("telecom", "ContactPoint", 1125 "A contact detail for the person, e.g. a telephone number or an email address.", 0, java.lang.Integer.MAX_VALUE, 1126 telecom)); 1127 children.add(new Property("gender", "code", "Administrative Gender.", 0, 1, gender)); 1128 children.add(new Property("birthDate", "date", "The birth date for the person.", 0, 1, birthDate)); 1129 children.add(new Property("address", "Address", "One or more addresses for the person.", 0, 1130 java.lang.Integer.MAX_VALUE, address)); 1131 children.add(new Property("photo", "Attachment", 1132 "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 1133 0, 1, photo)); 1134 children.add(new Property("managingOrganization", "Reference(Organization)", 1135 "The organization that is the custodian of the person record.", 0, 1, managingOrganization)); 1136 children.add(new Property("active", "boolean", "Whether this person's record is in active use.", 0, 1, active)); 1137 children.add(new Property("link", "", "Link to a resource that concerns the same actual person.", 0, 1138 java.lang.Integer.MAX_VALUE, link)); 1139 } 1140 1141 @Override 1142 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1143 switch (_hash) { 1144 case -1618432855: 1145 /* identifier */ return new Property("identifier", "Identifier", 1146 "Identifier for a person within a particular scope.", 0, java.lang.Integer.MAX_VALUE, identifier); 1147 case 3373707: 1148 /* name */ return new Property("name", "HumanName", "A name associated with the person.", 0, 1149 java.lang.Integer.MAX_VALUE, name); 1150 case -1429363305: 1151 /* telecom */ return new Property("telecom", "ContactPoint", 1152 "A contact detail for the person, e.g. a telephone number or an email address.", 0, 1153 java.lang.Integer.MAX_VALUE, telecom); 1154 case -1249512767: 1155 /* gender */ return new Property("gender", "code", "Administrative Gender.", 0, 1, gender); 1156 case -1210031859: 1157 /* birthDate */ return new Property("birthDate", "date", "The birth date for the person.", 0, 1, birthDate); 1158 case -1147692044: 1159 /* address */ return new Property("address", "Address", "One or more addresses for the person.", 0, 1160 java.lang.Integer.MAX_VALUE, address); 1161 case 106642994: 1162 /* photo */ return new Property("photo", "Attachment", 1163 "An image that can be displayed as a thumbnail of the person to enhance the identification of the individual.", 1164 0, 1, photo); 1165 case -2058947787: 1166 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1167 "The organization that is the custodian of the person record.", 0, 1, managingOrganization); 1168 case -1422950650: 1169 /* active */ return new Property("active", "boolean", "Whether this person's record is in active use.", 0, 1, 1170 active); 1171 case 3321850: 1172 /* link */ return new Property("link", "", "Link to a resource that concerns the same actual person.", 0, 1173 java.lang.Integer.MAX_VALUE, link); 1174 default: 1175 return super.getNamedProperty(_hash, _name, _checkValid); 1176 } 1177 1178 } 1179 1180 @Override 1181 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1182 switch (hash) { 1183 case -1618432855: 1184 /* identifier */ return this.identifier == null ? new Base[0] 1185 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1186 case 3373707: 1187 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1188 case -1429363305: 1189 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1190 case -1249512767: 1191 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 1192 case -1210031859: 1193 /* birthDate */ return this.birthDate == null ? new Base[0] : new Base[] { this.birthDate }; // DateType 1194 case -1147692044: 1195 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1196 case 106642994: 1197 /* photo */ return this.photo == null ? new Base[0] : new Base[] { this.photo }; // Attachment 1198 case -2058947787: 1199 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1200 : new Base[] { this.managingOrganization }; // Reference 1201 case -1422950650: 1202 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1203 case 3321850: 1204 /* link */ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // PersonLinkComponent 1205 default: 1206 return super.getProperty(hash, name, checkValid); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1213 switch (hash) { 1214 case -1618432855: // identifier 1215 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1216 return value; 1217 case 3373707: // name 1218 this.getName().add(castToHumanName(value)); // HumanName 1219 return value; 1220 case -1429363305: // telecom 1221 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1222 return value; 1223 case -1249512767: // gender 1224 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1225 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1226 return value; 1227 case -1210031859: // birthDate 1228 this.birthDate = castToDate(value); // DateType 1229 return value; 1230 case -1147692044: // address 1231 this.getAddress().add(castToAddress(value)); // Address 1232 return value; 1233 case 106642994: // photo 1234 this.photo = castToAttachment(value); // Attachment 1235 return value; 1236 case -2058947787: // managingOrganization 1237 this.managingOrganization = castToReference(value); // Reference 1238 return value; 1239 case -1422950650: // active 1240 this.active = castToBoolean(value); // BooleanType 1241 return value; 1242 case 3321850: // link 1243 this.getLink().add((PersonLinkComponent) value); // PersonLinkComponent 1244 return value; 1245 default: 1246 return super.setProperty(hash, name, value); 1247 } 1248 1249 } 1250 1251 @Override 1252 public Base setProperty(String name, Base value) throws FHIRException { 1253 if (name.equals("identifier")) { 1254 this.getIdentifier().add(castToIdentifier(value)); 1255 } else if (name.equals("name")) { 1256 this.getName().add(castToHumanName(value)); 1257 } else if (name.equals("telecom")) { 1258 this.getTelecom().add(castToContactPoint(value)); 1259 } else if (name.equals("gender")) { 1260 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1261 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1262 } else if (name.equals("birthDate")) { 1263 this.birthDate = castToDate(value); // DateType 1264 } else if (name.equals("address")) { 1265 this.getAddress().add(castToAddress(value)); 1266 } else if (name.equals("photo")) { 1267 this.photo = castToAttachment(value); // Attachment 1268 } else if (name.equals("managingOrganization")) { 1269 this.managingOrganization = castToReference(value); // Reference 1270 } else if (name.equals("active")) { 1271 this.active = castToBoolean(value); // BooleanType 1272 } else if (name.equals("link")) { 1273 this.getLink().add((PersonLinkComponent) value); 1274 } else 1275 return super.setProperty(name, value); 1276 return value; 1277 } 1278 1279 @Override 1280 public void removeChild(String name, Base value) throws FHIRException { 1281 if (name.equals("identifier")) { 1282 this.getIdentifier().remove(castToIdentifier(value)); 1283 } else if (name.equals("name")) { 1284 this.getName().remove(castToHumanName(value)); 1285 } else if (name.equals("telecom")) { 1286 this.getTelecom().remove(castToContactPoint(value)); 1287 } else if (name.equals("gender")) { 1288 this.gender = null; 1289 } else if (name.equals("birthDate")) { 1290 this.birthDate = null; 1291 } else if (name.equals("address")) { 1292 this.getAddress().remove(castToAddress(value)); 1293 } else if (name.equals("photo")) { 1294 this.photo = null; 1295 } else if (name.equals("managingOrganization")) { 1296 this.managingOrganization = null; 1297 } else if (name.equals("active")) { 1298 this.active = null; 1299 } else if (name.equals("link")) { 1300 this.getLink().remove((PersonLinkComponent) value); 1301 } else 1302 super.removeChild(name, value); 1303 1304 } 1305 1306 @Override 1307 public Base makeProperty(int hash, String name) throws FHIRException { 1308 switch (hash) { 1309 case -1618432855: 1310 return addIdentifier(); 1311 case 3373707: 1312 return addName(); 1313 case -1429363305: 1314 return addTelecom(); 1315 case -1249512767: 1316 return getGenderElement(); 1317 case -1210031859: 1318 return getBirthDateElement(); 1319 case -1147692044: 1320 return addAddress(); 1321 case 106642994: 1322 return getPhoto(); 1323 case -2058947787: 1324 return getManagingOrganization(); 1325 case -1422950650: 1326 return getActiveElement(); 1327 case 3321850: 1328 return addLink(); 1329 default: 1330 return super.makeProperty(hash, name); 1331 } 1332 1333 } 1334 1335 @Override 1336 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1337 switch (hash) { 1338 case -1618432855: 1339 /* identifier */ return new String[] { "Identifier" }; 1340 case 3373707: 1341 /* name */ return new String[] { "HumanName" }; 1342 case -1429363305: 1343 /* telecom */ return new String[] { "ContactPoint" }; 1344 case -1249512767: 1345 /* gender */ return new String[] { "code" }; 1346 case -1210031859: 1347 /* birthDate */ return new String[] { "date" }; 1348 case -1147692044: 1349 /* address */ return new String[] { "Address" }; 1350 case 106642994: 1351 /* photo */ return new String[] { "Attachment" }; 1352 case -2058947787: 1353 /* managingOrganization */ return new String[] { "Reference" }; 1354 case -1422950650: 1355 /* active */ return new String[] { "boolean" }; 1356 case 3321850: 1357 /* link */ return new String[] {}; 1358 default: 1359 return super.getTypesForProperty(hash, name); 1360 } 1361 1362 } 1363 1364 @Override 1365 public Base addChild(String name) throws FHIRException { 1366 if (name.equals("identifier")) { 1367 return addIdentifier(); 1368 } else if (name.equals("name")) { 1369 return addName(); 1370 } else if (name.equals("telecom")) { 1371 return addTelecom(); 1372 } else if (name.equals("gender")) { 1373 throw new FHIRException("Cannot call addChild on a singleton property Person.gender"); 1374 } else if (name.equals("birthDate")) { 1375 throw new FHIRException("Cannot call addChild on a singleton property Person.birthDate"); 1376 } else if (name.equals("address")) { 1377 return addAddress(); 1378 } else if (name.equals("photo")) { 1379 this.photo = new Attachment(); 1380 return this.photo; 1381 } else if (name.equals("managingOrganization")) { 1382 this.managingOrganization = new Reference(); 1383 return this.managingOrganization; 1384 } else if (name.equals("active")) { 1385 throw new FHIRException("Cannot call addChild on a singleton property Person.active"); 1386 } else if (name.equals("link")) { 1387 return addLink(); 1388 } else 1389 return super.addChild(name); 1390 } 1391 1392 public String fhirType() { 1393 return "Person"; 1394 1395 } 1396 1397 public Person copy() { 1398 Person dst = new Person(); 1399 copyValues(dst); 1400 return dst; 1401 } 1402 1403 public void copyValues(Person dst) { 1404 super.copyValues(dst); 1405 if (identifier != null) { 1406 dst.identifier = new ArrayList<Identifier>(); 1407 for (Identifier i : identifier) 1408 dst.identifier.add(i.copy()); 1409 } 1410 ; 1411 if (name != null) { 1412 dst.name = new ArrayList<HumanName>(); 1413 for (HumanName i : name) 1414 dst.name.add(i.copy()); 1415 } 1416 ; 1417 if (telecom != null) { 1418 dst.telecom = new ArrayList<ContactPoint>(); 1419 for (ContactPoint i : telecom) 1420 dst.telecom.add(i.copy()); 1421 } 1422 ; 1423 dst.gender = gender == null ? null : gender.copy(); 1424 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1425 if (address != null) { 1426 dst.address = new ArrayList<Address>(); 1427 for (Address i : address) 1428 dst.address.add(i.copy()); 1429 } 1430 ; 1431 dst.photo = photo == null ? null : photo.copy(); 1432 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1433 dst.active = active == null ? null : active.copy(); 1434 if (link != null) { 1435 dst.link = new ArrayList<PersonLinkComponent>(); 1436 for (PersonLinkComponent i : link) 1437 dst.link.add(i.copy()); 1438 } 1439 ; 1440 } 1441 1442 protected Person typedCopy() { 1443 return copy(); 1444 } 1445 1446 @Override 1447 public boolean equalsDeep(Base other_) { 1448 if (!super.equalsDeep(other_)) 1449 return false; 1450 if (!(other_ instanceof Person)) 1451 return false; 1452 Person o = (Person) other_; 1453 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 1454 && compareDeep(telecom, o.telecom, true) && compareDeep(gender, o.gender, true) 1455 && compareDeep(birthDate, o.birthDate, true) && compareDeep(address, o.address, true) 1456 && compareDeep(photo, o.photo, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1457 && compareDeep(active, o.active, true) && compareDeep(link, o.link, true); 1458 } 1459 1460 @Override 1461 public boolean equalsShallow(Base other_) { 1462 if (!super.equalsShallow(other_)) 1463 return false; 1464 if (!(other_ instanceof Person)) 1465 return false; 1466 Person o = (Person) other_; 1467 return compareValues(gender, o.gender, true) && compareValues(birthDate, o.birthDate, true) 1468 && compareValues(active, o.active, true); 1469 } 1470 1471 public boolean isEmpty() { 1472 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, telecom, gender, birthDate, 1473 address, photo, managingOrganization, active, link); 1474 } 1475 1476 @Override 1477 public ResourceType getResourceType() { 1478 return ResourceType.Person; 1479 } 1480 1481 /** 1482 * Search parameter: <b>identifier</b> 1483 * <p> 1484 * Description: <b>A person Identifier</b><br> 1485 * Type: <b>token</b><br> 1486 * Path: <b>Person.identifier</b><br> 1487 * </p> 1488 */ 1489 @SearchParamDefinition(name = "identifier", path = "Person.identifier", description = "A person Identifier", type = "token") 1490 public static final String SP_IDENTIFIER = "identifier"; 1491 /** 1492 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1493 * <p> 1494 * Description: <b>A person Identifier</b><br> 1495 * Type: <b>token</b><br> 1496 * Path: <b>Person.identifier</b><br> 1497 * </p> 1498 */ 1499 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1500 SP_IDENTIFIER); 1501 1502 /** 1503 * Search parameter: <b>address</b> 1504 * <p> 1505 * Description: <b>A server defined search that may match any of the string 1506 * fields in the Address, including line, city, district, state, country, 1507 * postalCode, and/or text</b><br> 1508 * Type: <b>string</b><br> 1509 * Path: <b>Person.address</b><br> 1510 * </p> 1511 */ 1512 @SearchParamDefinition(name = "address", path = "Person.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 1513 public static final String SP_ADDRESS = "address"; 1514 /** 1515 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1516 * <p> 1517 * Description: <b>A server defined search that may match any of the string 1518 * fields in the Address, including line, city, district, state, country, 1519 * postalCode, and/or text</b><br> 1520 * Type: <b>string</b><br> 1521 * Path: <b>Person.address</b><br> 1522 * </p> 1523 */ 1524 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 1525 SP_ADDRESS); 1526 1527 /** 1528 * Search parameter: <b>birthdate</b> 1529 * <p> 1530 * Description: <b>The person's date of birth</b><br> 1531 * Type: <b>date</b><br> 1532 * Path: <b>Person.birthDate</b><br> 1533 * </p> 1534 */ 1535 @SearchParamDefinition(name = "birthdate", path = "Person.birthDate", description = "The person's date of birth", type = "date") 1536 public static final String SP_BIRTHDATE = "birthdate"; 1537 /** 1538 * <b>Fluent Client</b> search parameter constant for <b>birthdate</b> 1539 * <p> 1540 * Description: <b>The person's date of birth</b><br> 1541 * Type: <b>date</b><br> 1542 * Path: <b>Person.birthDate</b><br> 1543 * </p> 1544 */ 1545 public static final ca.uhn.fhir.rest.gclient.DateClientParam BIRTHDATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1546 SP_BIRTHDATE); 1547 1548 /** 1549 * Search parameter: <b>address-state</b> 1550 * <p> 1551 * Description: <b>A state specified in an address</b><br> 1552 * Type: <b>string</b><br> 1553 * Path: <b>Person.address.state</b><br> 1554 * </p> 1555 */ 1556 @SearchParamDefinition(name = "address-state", path = "Person.address.state", description = "A state specified in an address", type = "string") 1557 public static final String SP_ADDRESS_STATE = "address-state"; 1558 /** 1559 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1560 * <p> 1561 * Description: <b>A state specified in an address</b><br> 1562 * Type: <b>string</b><br> 1563 * Path: <b>Person.address.state</b><br> 1564 * </p> 1565 */ 1566 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1567 SP_ADDRESS_STATE); 1568 1569 /** 1570 * Search parameter: <b>gender</b> 1571 * <p> 1572 * Description: <b>The gender of the person</b><br> 1573 * Type: <b>token</b><br> 1574 * Path: <b>Person.gender</b><br> 1575 * </p> 1576 */ 1577 @SearchParamDefinition(name = "gender", path = "Person.gender", description = "The gender of the person", type = "token") 1578 public static final String SP_GENDER = "gender"; 1579 /** 1580 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1581 * <p> 1582 * Description: <b>The gender of the person</b><br> 1583 * Type: <b>token</b><br> 1584 * Path: <b>Person.gender</b><br> 1585 * </p> 1586 */ 1587 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1588 SP_GENDER); 1589 1590 /** 1591 * Search parameter: <b>practitioner</b> 1592 * <p> 1593 * Description: <b>The Person links to this Practitioner</b><br> 1594 * Type: <b>reference</b><br> 1595 * Path: <b>Person.link.target</b><br> 1596 * </p> 1597 */ 1598 @SearchParamDefinition(name = "practitioner", path = "Person.link.target.where(resolve() is Practitioner)", description = "The Person links to this Practitioner", type = "reference", providesMembershipIn = { 1599 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 1600 public static final String SP_PRACTITIONER = "practitioner"; 1601 /** 1602 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 1603 * <p> 1604 * Description: <b>The Person links to this Practitioner</b><br> 1605 * Type: <b>reference</b><br> 1606 * Path: <b>Person.link.target</b><br> 1607 * </p> 1608 */ 1609 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1610 SP_PRACTITIONER); 1611 1612 /** 1613 * Constant for fluent queries to be used to add include statements. Specifies 1614 * the path value of "<b>Person:practitioner</b>". 1615 */ 1616 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 1617 "Person:practitioner").toLocked(); 1618 1619 /** 1620 * Search parameter: <b>link</b> 1621 * <p> 1622 * Description: <b>Any link has this Patient, Person, RelatedPerson or 1623 * Practitioner reference</b><br> 1624 * Type: <b>reference</b><br> 1625 * Path: <b>Person.link.target</b><br> 1626 * </p> 1627 */ 1628 @SearchParamDefinition(name = "link", path = "Person.link.target", description = "Any link has this Patient, Person, RelatedPerson or Practitioner reference", type = "reference", providesMembershipIn = { 1629 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, Person.class, 1630 Practitioner.class, RelatedPerson.class }) 1631 public static final String SP_LINK = "link"; 1632 /** 1633 * <b>Fluent Client</b> search parameter constant for <b>link</b> 1634 * <p> 1635 * Description: <b>Any link has this Patient, Person, RelatedPerson or 1636 * Practitioner reference</b><br> 1637 * Type: <b>reference</b><br> 1638 * Path: <b>Person.link.target</b><br> 1639 * </p> 1640 */ 1641 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LINK = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1642 SP_LINK); 1643 1644 /** 1645 * Constant for fluent queries to be used to add include statements. Specifies 1646 * the path value of "<b>Person:link</b>". 1647 */ 1648 public static final ca.uhn.fhir.model.api.Include INCLUDE_LINK = new ca.uhn.fhir.model.api.Include("Person:link") 1649 .toLocked(); 1650 1651 /** 1652 * Search parameter: <b>relatedperson</b> 1653 * <p> 1654 * Description: <b>The Person links to this RelatedPerson</b><br> 1655 * Type: <b>reference</b><br> 1656 * Path: <b>Person.link.target</b><br> 1657 * </p> 1658 */ 1659 @SearchParamDefinition(name = "relatedperson", path = "Person.link.target.where(resolve() is RelatedPerson)", description = "The Person links to this RelatedPerson", type = "reference", target = { 1660 RelatedPerson.class }) 1661 public static final String SP_RELATEDPERSON = "relatedperson"; 1662 /** 1663 * <b>Fluent Client</b> search parameter constant for <b>relatedperson</b> 1664 * <p> 1665 * Description: <b>The Person links to this RelatedPerson</b><br> 1666 * Type: <b>reference</b><br> 1667 * Path: <b>Person.link.target</b><br> 1668 * </p> 1669 */ 1670 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATEDPERSON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1671 SP_RELATEDPERSON); 1672 1673 /** 1674 * Constant for fluent queries to be used to add include statements. Specifies 1675 * the path value of "<b>Person:relatedperson</b>". 1676 */ 1677 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATEDPERSON = new ca.uhn.fhir.model.api.Include( 1678 "Person:relatedperson").toLocked(); 1679 1680 /** 1681 * Search parameter: <b>address-postalcode</b> 1682 * <p> 1683 * Description: <b>A postal code specified in an address</b><br> 1684 * Type: <b>string</b><br> 1685 * Path: <b>Person.address.postalCode</b><br> 1686 * </p> 1687 */ 1688 @SearchParamDefinition(name = "address-postalcode", path = "Person.address.postalCode", description = "A postal code specified in an address", type = "string") 1689 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1690 /** 1691 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1692 * <p> 1693 * Description: <b>A postal code specified in an address</b><br> 1694 * Type: <b>string</b><br> 1695 * Path: <b>Person.address.postalCode</b><br> 1696 * </p> 1697 */ 1698 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1699 SP_ADDRESS_POSTALCODE); 1700 1701 /** 1702 * Search parameter: <b>address-country</b> 1703 * <p> 1704 * Description: <b>A country specified in an address</b><br> 1705 * Type: <b>string</b><br> 1706 * Path: <b>Person.address.country</b><br> 1707 * </p> 1708 */ 1709 @SearchParamDefinition(name = "address-country", path = "Person.address.country", description = "A country specified in an address", type = "string") 1710 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1711 /** 1712 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1713 * <p> 1714 * Description: <b>A country specified in an address</b><br> 1715 * Type: <b>string</b><br> 1716 * Path: <b>Person.address.country</b><br> 1717 * </p> 1718 */ 1719 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1720 SP_ADDRESS_COUNTRY); 1721 1722 /** 1723 * Search parameter: <b>phonetic</b> 1724 * <p> 1725 * Description: <b>A portion of name using some kind of phonetic matching 1726 * algorithm</b><br> 1727 * Type: <b>string</b><br> 1728 * Path: <b>Person.name</b><br> 1729 * </p> 1730 */ 1731 @SearchParamDefinition(name = "phonetic", path = "Person.name", description = "A portion of name using some kind of phonetic matching algorithm", type = "string") 1732 public static final String SP_PHONETIC = "phonetic"; 1733 /** 1734 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1735 * <p> 1736 * Description: <b>A portion of name using some kind of phonetic matching 1737 * algorithm</b><br> 1738 * Type: <b>string</b><br> 1739 * Path: <b>Person.name</b><br> 1740 * </p> 1741 */ 1742 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 1743 SP_PHONETIC); 1744 1745 /** 1746 * Search parameter: <b>phone</b> 1747 * <p> 1748 * Description: <b>A value in a phone contact</b><br> 1749 * Type: <b>token</b><br> 1750 * Path: <b>Person.telecom(system=phone)</b><br> 1751 * </p> 1752 */ 1753 @SearchParamDefinition(name = "phone", path = "Person.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 1754 public static final String SP_PHONE = "phone"; 1755 /** 1756 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1757 * <p> 1758 * Description: <b>A value in a phone contact</b><br> 1759 * Type: <b>token</b><br> 1760 * Path: <b>Person.telecom(system=phone)</b><br> 1761 * </p> 1762 */ 1763 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1764 SP_PHONE); 1765 1766 /** 1767 * Search parameter: <b>patient</b> 1768 * <p> 1769 * Description: <b>The Person links to this Patient</b><br> 1770 * Type: <b>reference</b><br> 1771 * Path: <b>Person.link.target</b><br> 1772 * </p> 1773 */ 1774 @SearchParamDefinition(name = "patient", path = "Person.link.target.where(resolve() is Patient)", description = "The Person links to this Patient", type = "reference", providesMembershipIn = { 1775 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1776 public static final String SP_PATIENT = "patient"; 1777 /** 1778 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1779 * <p> 1780 * Description: <b>The Person links to this Patient</b><br> 1781 * Type: <b>reference</b><br> 1782 * Path: <b>Person.link.target</b><br> 1783 * </p> 1784 */ 1785 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1786 SP_PATIENT); 1787 1788 /** 1789 * Constant for fluent queries to be used to add include statements. Specifies 1790 * the path value of "<b>Person:patient</b>". 1791 */ 1792 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1793 "Person:patient").toLocked(); 1794 1795 /** 1796 * Search parameter: <b>organization</b> 1797 * <p> 1798 * Description: <b>The organization at which this person record is being 1799 * managed</b><br> 1800 * Type: <b>reference</b><br> 1801 * Path: <b>Person.managingOrganization</b><br> 1802 * </p> 1803 */ 1804 @SearchParamDefinition(name = "organization", path = "Person.managingOrganization", description = "The organization at which this person record is being managed", type = "reference", target = { 1805 Organization.class }) 1806 public static final String SP_ORGANIZATION = "organization"; 1807 /** 1808 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1809 * <p> 1810 * Description: <b>The organization at which this person record is being 1811 * managed</b><br> 1812 * Type: <b>reference</b><br> 1813 * Path: <b>Person.managingOrganization</b><br> 1814 * </p> 1815 */ 1816 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1817 SP_ORGANIZATION); 1818 1819 /** 1820 * Constant for fluent queries to be used to add include statements. Specifies 1821 * the path value of "<b>Person:organization</b>". 1822 */ 1823 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 1824 "Person:organization").toLocked(); 1825 1826 /** 1827 * Search parameter: <b>name</b> 1828 * <p> 1829 * Description: <b>A server defined search that may match any of the string 1830 * fields in the HumanName, including family, give, prefix, suffix, suffix, 1831 * and/or text</b><br> 1832 * Type: <b>string</b><br> 1833 * Path: <b>Person.name</b><br> 1834 * </p> 1835 */ 1836 @SearchParamDefinition(name = "name", path = "Person.name", description = "A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type = "string") 1837 public static final String SP_NAME = "name"; 1838 /** 1839 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1840 * <p> 1841 * Description: <b>A server defined search that may match any of the string 1842 * fields in the HumanName, including family, give, prefix, suffix, suffix, 1843 * and/or text</b><br> 1844 * Type: <b>string</b><br> 1845 * Path: <b>Person.name</b><br> 1846 * </p> 1847 */ 1848 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 1849 SP_NAME); 1850 1851 /** 1852 * Search parameter: <b>address-use</b> 1853 * <p> 1854 * Description: <b>A use code specified in an address</b><br> 1855 * Type: <b>token</b><br> 1856 * Path: <b>Person.address.use</b><br> 1857 * </p> 1858 */ 1859 @SearchParamDefinition(name = "address-use", path = "Person.address.use", description = "A use code specified in an address", type = "token") 1860 public static final String SP_ADDRESS_USE = "address-use"; 1861 /** 1862 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1863 * <p> 1864 * Description: <b>A use code specified in an address</b><br> 1865 * Type: <b>token</b><br> 1866 * Path: <b>Person.address.use</b><br> 1867 * </p> 1868 */ 1869 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1870 SP_ADDRESS_USE); 1871 1872 /** 1873 * Search parameter: <b>telecom</b> 1874 * <p> 1875 * Description: <b>The value in any kind of contact</b><br> 1876 * Type: <b>token</b><br> 1877 * Path: <b>Person.telecom</b><br> 1878 * </p> 1879 */ 1880 @SearchParamDefinition(name = "telecom", path = "Person.telecom", description = "The value in any kind of contact", type = "token") 1881 public static final String SP_TELECOM = "telecom"; 1882 /** 1883 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1884 * <p> 1885 * Description: <b>The value in any kind of contact</b><br> 1886 * Type: <b>token</b><br> 1887 * Path: <b>Person.telecom</b><br> 1888 * </p> 1889 */ 1890 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1891 SP_TELECOM); 1892 1893 /** 1894 * Search parameter: <b>address-city</b> 1895 * <p> 1896 * Description: <b>A city specified in an address</b><br> 1897 * Type: <b>string</b><br> 1898 * Path: <b>Person.address.city</b><br> 1899 * </p> 1900 */ 1901 @SearchParamDefinition(name = "address-city", path = "Person.address.city", description = "A city specified in an address", type = "string") 1902 public static final String SP_ADDRESS_CITY = "address-city"; 1903 /** 1904 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1905 * <p> 1906 * Description: <b>A city specified in an address</b><br> 1907 * Type: <b>string</b><br> 1908 * Path: <b>Person.address.city</b><br> 1909 * </p> 1910 */ 1911 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1912 SP_ADDRESS_CITY); 1913 1914 /** 1915 * Search parameter: <b>email</b> 1916 * <p> 1917 * Description: <b>A value in an email contact</b><br> 1918 * Type: <b>token</b><br> 1919 * Path: <b>Person.telecom(system=email)</b><br> 1920 * </p> 1921 */ 1922 @SearchParamDefinition(name = "email", path = "Person.telecom.where(system='email')", description = "A value in an email contact", type = "token") 1923 public static final String SP_EMAIL = "email"; 1924 /** 1925 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1926 * <p> 1927 * Description: <b>A value in an email contact</b><br> 1928 * Type: <b>token</b><br> 1929 * Path: <b>Person.telecom(system=email)</b><br> 1930 * </p> 1931 */ 1932 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1933 SP_EMAIL); 1934 1935}