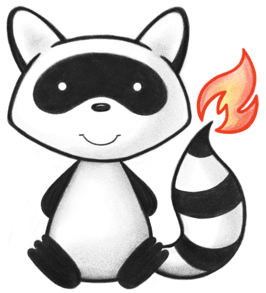
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * This resource allows for the definition of various types of plans as a 052 * sharable, consumable, and executable artifact. The resource is general enough 053 * to support the description of a broad range of clinical artifacts such as 054 * clinical decision support rules, order sets and protocols. 055 */ 056@ResourceDef(name = "PlanDefinition", profile = "http://hl7.org/fhir/StructureDefinition/PlanDefinition") 057@ChildOrder(names = { "url", "identifier", "version", "name", "title", "subtitle", "type", "status", "experimental", 058 "subject[x]", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "usage", 059 "copyright", "approvalDate", "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", 060 "endorser", "relatedArtifact", "library", "goal", "action" }) 061public class PlanDefinition extends MetadataResource { 062 063 public enum RequestPriority { 064 /** 065 * The request has normal priority. 066 */ 067 ROUTINE, 068 /** 069 * The request should be actioned promptly - higher priority than routine. 070 */ 071 URGENT, 072 /** 073 * The request should be actioned as soon as possible - higher priority than 074 * urgent. 075 */ 076 ASAP, 077 /** 078 * The request should be actioned immediately - highest possible priority. E.g. 079 * an emergency. 080 */ 081 STAT, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 087 public static RequestPriority fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("routine".equals(codeString)) 091 return ROUTINE; 092 if ("urgent".equals(codeString)) 093 return URGENT; 094 if ("asap".equals(codeString)) 095 return ASAP; 096 if ("stat".equals(codeString)) 097 return STAT; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case ROUTINE: 107 return "routine"; 108 case URGENT: 109 return "urgent"; 110 case ASAP: 111 return "asap"; 112 case STAT: 113 return "stat"; 114 case NULL: 115 return null; 116 default: 117 return "?"; 118 } 119 } 120 121 public String getSystem() { 122 switch (this) { 123 case ROUTINE: 124 return "http://hl7.org/fhir/request-priority"; 125 case URGENT: 126 return "http://hl7.org/fhir/request-priority"; 127 case ASAP: 128 return "http://hl7.org/fhir/request-priority"; 129 case STAT: 130 return "http://hl7.org/fhir/request-priority"; 131 case NULL: 132 return null; 133 default: 134 return "?"; 135 } 136 } 137 138 public String getDefinition() { 139 switch (this) { 140 case ROUTINE: 141 return "The request has normal priority."; 142 case URGENT: 143 return "The request should be actioned promptly - higher priority than routine."; 144 case ASAP: 145 return "The request should be actioned as soon as possible - higher priority than urgent."; 146 case STAT: 147 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDisplay() { 156 switch (this) { 157 case ROUTINE: 158 return "Routine"; 159 case URGENT: 160 return "Urgent"; 161 case ASAP: 162 return "ASAP"; 163 case STAT: 164 return "STAT"; 165 case NULL: 166 return null; 167 default: 168 return "?"; 169 } 170 } 171 } 172 173 public static class RequestPriorityEnumFactory implements EnumFactory<RequestPriority> { 174 public RequestPriority fromCode(String codeString) throws IllegalArgumentException { 175 if (codeString == null || "".equals(codeString)) 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("routine".equals(codeString)) 179 return RequestPriority.ROUTINE; 180 if ("urgent".equals(codeString)) 181 return RequestPriority.URGENT; 182 if ("asap".equals(codeString)) 183 return RequestPriority.ASAP; 184 if ("stat".equals(codeString)) 185 return RequestPriority.STAT; 186 throw new IllegalArgumentException("Unknown RequestPriority code '" + codeString + "'"); 187 } 188 189 public Enumeration<RequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 190 if (code == null) 191 return null; 192 if (code.isEmpty()) 193 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 194 String codeString = code.asStringValue(); 195 if (codeString == null || "".equals(codeString)) 196 return new Enumeration<RequestPriority>(this, RequestPriority.NULL, code); 197 if ("routine".equals(codeString)) 198 return new Enumeration<RequestPriority>(this, RequestPriority.ROUTINE, code); 199 if ("urgent".equals(codeString)) 200 return new Enumeration<RequestPriority>(this, RequestPriority.URGENT, code); 201 if ("asap".equals(codeString)) 202 return new Enumeration<RequestPriority>(this, RequestPriority.ASAP, code); 203 if ("stat".equals(codeString)) 204 return new Enumeration<RequestPriority>(this, RequestPriority.STAT, code); 205 throw new FHIRException("Unknown RequestPriority code '" + codeString + "'"); 206 } 207 208 public String toCode(RequestPriority code) { 209 if (code == RequestPriority.ROUTINE) 210 return "routine"; 211 if (code == RequestPriority.URGENT) 212 return "urgent"; 213 if (code == RequestPriority.ASAP) 214 return "asap"; 215 if (code == RequestPriority.STAT) 216 return "stat"; 217 return "?"; 218 } 219 220 public String toSystem(RequestPriority code) { 221 return code.getSystem(); 222 } 223 } 224 225 public enum ActionConditionKind { 226 /** 227 * The condition describes whether or not a given action is applicable. 228 */ 229 APPLICABILITY, 230 /** 231 * The condition is a starting condition for the action. 232 */ 233 START, 234 /** 235 * The condition is a stop, or exit condition for the action. 236 */ 237 STOP, 238 /** 239 * added to help the parsers with the generic types 240 */ 241 NULL; 242 243 public static ActionConditionKind fromCode(String codeString) throws FHIRException { 244 if (codeString == null || "".equals(codeString)) 245 return null; 246 if ("applicability".equals(codeString)) 247 return APPLICABILITY; 248 if ("start".equals(codeString)) 249 return START; 250 if ("stop".equals(codeString)) 251 return STOP; 252 if (Configuration.isAcceptInvalidEnums()) 253 return null; 254 else 255 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 256 } 257 258 public String toCode() { 259 switch (this) { 260 case APPLICABILITY: 261 return "applicability"; 262 case START: 263 return "start"; 264 case STOP: 265 return "stop"; 266 case NULL: 267 return null; 268 default: 269 return "?"; 270 } 271 } 272 273 public String getSystem() { 274 switch (this) { 275 case APPLICABILITY: 276 return "http://hl7.org/fhir/action-condition-kind"; 277 case START: 278 return "http://hl7.org/fhir/action-condition-kind"; 279 case STOP: 280 return "http://hl7.org/fhir/action-condition-kind"; 281 case NULL: 282 return null; 283 default: 284 return "?"; 285 } 286 } 287 288 public String getDefinition() { 289 switch (this) { 290 case APPLICABILITY: 291 return "The condition describes whether or not a given action is applicable."; 292 case START: 293 return "The condition is a starting condition for the action."; 294 case STOP: 295 return "The condition is a stop, or exit condition for the action."; 296 case NULL: 297 return null; 298 default: 299 return "?"; 300 } 301 } 302 303 public String getDisplay() { 304 switch (this) { 305 case APPLICABILITY: 306 return "Applicability"; 307 case START: 308 return "Start"; 309 case STOP: 310 return "Stop"; 311 case NULL: 312 return null; 313 default: 314 return "?"; 315 } 316 } 317 } 318 319 public static class ActionConditionKindEnumFactory implements EnumFactory<ActionConditionKind> { 320 public ActionConditionKind fromCode(String codeString) throws IllegalArgumentException { 321 if (codeString == null || "".equals(codeString)) 322 if (codeString == null || "".equals(codeString)) 323 return null; 324 if ("applicability".equals(codeString)) 325 return ActionConditionKind.APPLICABILITY; 326 if ("start".equals(codeString)) 327 return ActionConditionKind.START; 328 if ("stop".equals(codeString)) 329 return ActionConditionKind.STOP; 330 throw new IllegalArgumentException("Unknown ActionConditionKind code '" + codeString + "'"); 331 } 332 333 public Enumeration<ActionConditionKind> fromType(PrimitiveType<?> code) throws FHIRException { 334 if (code == null) 335 return null; 336 if (code.isEmpty()) 337 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 338 String codeString = code.asStringValue(); 339 if (codeString == null || "".equals(codeString)) 340 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.NULL, code); 341 if ("applicability".equals(codeString)) 342 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.APPLICABILITY, code); 343 if ("start".equals(codeString)) 344 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.START, code); 345 if ("stop".equals(codeString)) 346 return new Enumeration<ActionConditionKind>(this, ActionConditionKind.STOP, code); 347 throw new FHIRException("Unknown ActionConditionKind code '" + codeString + "'"); 348 } 349 350 public String toCode(ActionConditionKind code) { 351 if (code == ActionConditionKind.APPLICABILITY) 352 return "applicability"; 353 if (code == ActionConditionKind.START) 354 return "start"; 355 if (code == ActionConditionKind.STOP) 356 return "stop"; 357 return "?"; 358 } 359 360 public String toSystem(ActionConditionKind code) { 361 return code.getSystem(); 362 } 363 } 364 365 public enum ActionRelationshipType { 366 /** 367 * The action must be performed before the start of the related action. 368 */ 369 BEFORESTART, 370 /** 371 * The action must be performed before the related action. 372 */ 373 BEFORE, 374 /** 375 * The action must be performed before the end of the related action. 376 */ 377 BEFOREEND, 378 /** 379 * The action must be performed concurrent with the start of the related action. 380 */ 381 CONCURRENTWITHSTART, 382 /** 383 * The action must be performed concurrent with the related action. 384 */ 385 CONCURRENT, 386 /** 387 * The action must be performed concurrent with the end of the related action. 388 */ 389 CONCURRENTWITHEND, 390 /** 391 * The action must be performed after the start of the related action. 392 */ 393 AFTERSTART, 394 /** 395 * The action must be performed after the related action. 396 */ 397 AFTER, 398 /** 399 * The action must be performed after the end of the related action. 400 */ 401 AFTEREND, 402 /** 403 * added to help the parsers with the generic types 404 */ 405 NULL; 406 407 public static ActionRelationshipType fromCode(String codeString) throws FHIRException { 408 if (codeString == null || "".equals(codeString)) 409 return null; 410 if ("before-start".equals(codeString)) 411 return BEFORESTART; 412 if ("before".equals(codeString)) 413 return BEFORE; 414 if ("before-end".equals(codeString)) 415 return BEFOREEND; 416 if ("concurrent-with-start".equals(codeString)) 417 return CONCURRENTWITHSTART; 418 if ("concurrent".equals(codeString)) 419 return CONCURRENT; 420 if ("concurrent-with-end".equals(codeString)) 421 return CONCURRENTWITHEND; 422 if ("after-start".equals(codeString)) 423 return AFTERSTART; 424 if ("after".equals(codeString)) 425 return AFTER; 426 if ("after-end".equals(codeString)) 427 return AFTEREND; 428 if (Configuration.isAcceptInvalidEnums()) 429 return null; 430 else 431 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 432 } 433 434 public String toCode() { 435 switch (this) { 436 case BEFORESTART: 437 return "before-start"; 438 case BEFORE: 439 return "before"; 440 case BEFOREEND: 441 return "before-end"; 442 case CONCURRENTWITHSTART: 443 return "concurrent-with-start"; 444 case CONCURRENT: 445 return "concurrent"; 446 case CONCURRENTWITHEND: 447 return "concurrent-with-end"; 448 case AFTERSTART: 449 return "after-start"; 450 case AFTER: 451 return "after"; 452 case AFTEREND: 453 return "after-end"; 454 case NULL: 455 return null; 456 default: 457 return "?"; 458 } 459 } 460 461 public String getSystem() { 462 switch (this) { 463 case BEFORESTART: 464 return "http://hl7.org/fhir/action-relationship-type"; 465 case BEFORE: 466 return "http://hl7.org/fhir/action-relationship-type"; 467 case BEFOREEND: 468 return "http://hl7.org/fhir/action-relationship-type"; 469 case CONCURRENTWITHSTART: 470 return "http://hl7.org/fhir/action-relationship-type"; 471 case CONCURRENT: 472 return "http://hl7.org/fhir/action-relationship-type"; 473 case CONCURRENTWITHEND: 474 return "http://hl7.org/fhir/action-relationship-type"; 475 case AFTERSTART: 476 return "http://hl7.org/fhir/action-relationship-type"; 477 case AFTER: 478 return "http://hl7.org/fhir/action-relationship-type"; 479 case AFTEREND: 480 return "http://hl7.org/fhir/action-relationship-type"; 481 case NULL: 482 return null; 483 default: 484 return "?"; 485 } 486 } 487 488 public String getDefinition() { 489 switch (this) { 490 case BEFORESTART: 491 return "The action must be performed before the start of the related action."; 492 case BEFORE: 493 return "The action must be performed before the related action."; 494 case BEFOREEND: 495 return "The action must be performed before the end of the related action."; 496 case CONCURRENTWITHSTART: 497 return "The action must be performed concurrent with the start of the related action."; 498 case CONCURRENT: 499 return "The action must be performed concurrent with the related action."; 500 case CONCURRENTWITHEND: 501 return "The action must be performed concurrent with the end of the related action."; 502 case AFTERSTART: 503 return "The action must be performed after the start of the related action."; 504 case AFTER: 505 return "The action must be performed after the related action."; 506 case AFTEREND: 507 return "The action must be performed after the end of the related action."; 508 case NULL: 509 return null; 510 default: 511 return "?"; 512 } 513 } 514 515 public String getDisplay() { 516 switch (this) { 517 case BEFORESTART: 518 return "Before Start"; 519 case BEFORE: 520 return "Before"; 521 case BEFOREEND: 522 return "Before End"; 523 case CONCURRENTWITHSTART: 524 return "Concurrent With Start"; 525 case CONCURRENT: 526 return "Concurrent"; 527 case CONCURRENTWITHEND: 528 return "Concurrent With End"; 529 case AFTERSTART: 530 return "After Start"; 531 case AFTER: 532 return "After"; 533 case AFTEREND: 534 return "After End"; 535 case NULL: 536 return null; 537 default: 538 return "?"; 539 } 540 } 541 } 542 543 public static class ActionRelationshipTypeEnumFactory implements EnumFactory<ActionRelationshipType> { 544 public ActionRelationshipType fromCode(String codeString) throws IllegalArgumentException { 545 if (codeString == null || "".equals(codeString)) 546 if (codeString == null || "".equals(codeString)) 547 return null; 548 if ("before-start".equals(codeString)) 549 return ActionRelationshipType.BEFORESTART; 550 if ("before".equals(codeString)) 551 return ActionRelationshipType.BEFORE; 552 if ("before-end".equals(codeString)) 553 return ActionRelationshipType.BEFOREEND; 554 if ("concurrent-with-start".equals(codeString)) 555 return ActionRelationshipType.CONCURRENTWITHSTART; 556 if ("concurrent".equals(codeString)) 557 return ActionRelationshipType.CONCURRENT; 558 if ("concurrent-with-end".equals(codeString)) 559 return ActionRelationshipType.CONCURRENTWITHEND; 560 if ("after-start".equals(codeString)) 561 return ActionRelationshipType.AFTERSTART; 562 if ("after".equals(codeString)) 563 return ActionRelationshipType.AFTER; 564 if ("after-end".equals(codeString)) 565 return ActionRelationshipType.AFTEREND; 566 throw new IllegalArgumentException("Unknown ActionRelationshipType code '" + codeString + "'"); 567 } 568 569 public Enumeration<ActionRelationshipType> fromType(PrimitiveType<?> code) throws FHIRException { 570 if (code == null) 571 return null; 572 if (code.isEmpty()) 573 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 574 String codeString = code.asStringValue(); 575 if (codeString == null || "".equals(codeString)) 576 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.NULL, code); 577 if ("before-start".equals(codeString)) 578 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORESTART, code); 579 if ("before".equals(codeString)) 580 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFORE, code); 581 if ("before-end".equals(codeString)) 582 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.BEFOREEND, code); 583 if ("concurrent-with-start".equals(codeString)) 584 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHSTART, code); 585 if ("concurrent".equals(codeString)) 586 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENT, code); 587 if ("concurrent-with-end".equals(codeString)) 588 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.CONCURRENTWITHEND, code); 589 if ("after-start".equals(codeString)) 590 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTERSTART, code); 591 if ("after".equals(codeString)) 592 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTER, code); 593 if ("after-end".equals(codeString)) 594 return new Enumeration<ActionRelationshipType>(this, ActionRelationshipType.AFTEREND, code); 595 throw new FHIRException("Unknown ActionRelationshipType code '" + codeString + "'"); 596 } 597 598 public String toCode(ActionRelationshipType code) { 599 if (code == ActionRelationshipType.BEFORESTART) 600 return "before-start"; 601 if (code == ActionRelationshipType.BEFORE) 602 return "before"; 603 if (code == ActionRelationshipType.BEFOREEND) 604 return "before-end"; 605 if (code == ActionRelationshipType.CONCURRENTWITHSTART) 606 return "concurrent-with-start"; 607 if (code == ActionRelationshipType.CONCURRENT) 608 return "concurrent"; 609 if (code == ActionRelationshipType.CONCURRENTWITHEND) 610 return "concurrent-with-end"; 611 if (code == ActionRelationshipType.AFTERSTART) 612 return "after-start"; 613 if (code == ActionRelationshipType.AFTER) 614 return "after"; 615 if (code == ActionRelationshipType.AFTEREND) 616 return "after-end"; 617 return "?"; 618 } 619 620 public String toSystem(ActionRelationshipType code) { 621 return code.getSystem(); 622 } 623 } 624 625 public enum ActionParticipantType { 626 /** 627 * The participant is the patient under evaluation. 628 */ 629 PATIENT, 630 /** 631 * The participant is a practitioner involved in the patient's care. 632 */ 633 PRACTITIONER, 634 /** 635 * The participant is a person related to the patient. 636 */ 637 RELATEDPERSON, 638 /** 639 * The participant is a system or device used in the care of the patient. 640 */ 641 DEVICE, 642 /** 643 * added to help the parsers with the generic types 644 */ 645 NULL; 646 647 public static ActionParticipantType fromCode(String codeString) throws FHIRException { 648 if (codeString == null || "".equals(codeString)) 649 return null; 650 if ("patient".equals(codeString)) 651 return PATIENT; 652 if ("practitioner".equals(codeString)) 653 return PRACTITIONER; 654 if ("related-person".equals(codeString)) 655 return RELATEDPERSON; 656 if ("device".equals(codeString)) 657 return DEVICE; 658 if (Configuration.isAcceptInvalidEnums()) 659 return null; 660 else 661 throw new FHIRException("Unknown ActionParticipantType code '" + codeString + "'"); 662 } 663 664 public String toCode() { 665 switch (this) { 666 case PATIENT: 667 return "patient"; 668 case PRACTITIONER: 669 return "practitioner"; 670 case RELATEDPERSON: 671 return "related-person"; 672 case DEVICE: 673 return "device"; 674 case NULL: 675 return null; 676 default: 677 return "?"; 678 } 679 } 680 681 public String getSystem() { 682 switch (this) { 683 case PATIENT: 684 return "http://hl7.org/fhir/action-participant-type"; 685 case PRACTITIONER: 686 return "http://hl7.org/fhir/action-participant-type"; 687 case RELATEDPERSON: 688 return "http://hl7.org/fhir/action-participant-type"; 689 case DEVICE: 690 return "http://hl7.org/fhir/action-participant-type"; 691 case NULL: 692 return null; 693 default: 694 return "?"; 695 } 696 } 697 698 public String getDefinition() { 699 switch (this) { 700 case PATIENT: 701 return "The participant is the patient under evaluation."; 702 case PRACTITIONER: 703 return "The participant is a practitioner involved in the patient's care."; 704 case RELATEDPERSON: 705 return "The participant is a person related to the patient."; 706 case DEVICE: 707 return "The participant is a system or device used in the care of the patient."; 708 case NULL: 709 return null; 710 default: 711 return "?"; 712 } 713 } 714 715 public String getDisplay() { 716 switch (this) { 717 case PATIENT: 718 return "Patient"; 719 case PRACTITIONER: 720 return "Practitioner"; 721 case RELATEDPERSON: 722 return "Related Person"; 723 case DEVICE: 724 return "Device"; 725 case NULL: 726 return null; 727 default: 728 return "?"; 729 } 730 } 731 } 732 733 public static class ActionParticipantTypeEnumFactory implements EnumFactory<ActionParticipantType> { 734 public ActionParticipantType fromCode(String codeString) throws IllegalArgumentException { 735 if (codeString == null || "".equals(codeString)) 736 if (codeString == null || "".equals(codeString)) 737 return null; 738 if ("patient".equals(codeString)) 739 return ActionParticipantType.PATIENT; 740 if ("practitioner".equals(codeString)) 741 return ActionParticipantType.PRACTITIONER; 742 if ("related-person".equals(codeString)) 743 return ActionParticipantType.RELATEDPERSON; 744 if ("device".equals(codeString)) 745 return ActionParticipantType.DEVICE; 746 throw new IllegalArgumentException("Unknown ActionParticipantType code '" + codeString + "'"); 747 } 748 749 public Enumeration<ActionParticipantType> fromType(PrimitiveType<?> code) throws FHIRException { 750 if (code == null) 751 return null; 752 if (code.isEmpty()) 753 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.NULL, code); 754 String codeString = code.asStringValue(); 755 if (codeString == null || "".equals(codeString)) 756 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.NULL, code); 757 if ("patient".equals(codeString)) 758 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.PATIENT, code); 759 if ("practitioner".equals(codeString)) 760 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.PRACTITIONER, code); 761 if ("related-person".equals(codeString)) 762 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.RELATEDPERSON, code); 763 if ("device".equals(codeString)) 764 return new Enumeration<ActionParticipantType>(this, ActionParticipantType.DEVICE, code); 765 throw new FHIRException("Unknown ActionParticipantType code '" + codeString + "'"); 766 } 767 768 public String toCode(ActionParticipantType code) { 769 if (code == ActionParticipantType.PATIENT) 770 return "patient"; 771 if (code == ActionParticipantType.PRACTITIONER) 772 return "practitioner"; 773 if (code == ActionParticipantType.RELATEDPERSON) 774 return "related-person"; 775 if (code == ActionParticipantType.DEVICE) 776 return "device"; 777 return "?"; 778 } 779 780 public String toSystem(ActionParticipantType code) { 781 return code.getSystem(); 782 } 783 } 784 785 public enum ActionGroupingBehavior { 786 /** 787 * Any group marked with this behavior should be displayed as a visual group to 788 * the end user. 789 */ 790 VISUALGROUP, 791 /** 792 * A group with this behavior logically groups its sub-elements, and may be 793 * shown as a visual group to the end user, but it is not required to do so. 794 */ 795 LOGICALGROUP, 796 /** 797 * A group of related alternative actions is a sentence group if the target 798 * referenced by the action is the same in all the actions and each action 799 * simply constitutes a different variation on how to specify the details for 800 * the target. For example, two actions that could be in a SentenceGroup are 801 * "aspirin, 500 mg, 2 times per day" and "aspirin, 300 mg, 3 times per day". In 802 * both cases, aspirin is the target referenced by the action, and the two 803 * actions represent different options for how aspirin might be ordered for the 804 * patient. Note that a SentenceGroup would almost always have an associated 805 * selection behavior of "AtMostOne", unless it's a required action, in which 806 * case, it would be "ExactlyOne". 807 */ 808 SENTENCEGROUP, 809 /** 810 * added to help the parsers with the generic types 811 */ 812 NULL; 813 814 public static ActionGroupingBehavior fromCode(String codeString) throws FHIRException { 815 if (codeString == null || "".equals(codeString)) 816 return null; 817 if ("visual-group".equals(codeString)) 818 return VISUALGROUP; 819 if ("logical-group".equals(codeString)) 820 return LOGICALGROUP; 821 if ("sentence-group".equals(codeString)) 822 return SENTENCEGROUP; 823 if (Configuration.isAcceptInvalidEnums()) 824 return null; 825 else 826 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 827 } 828 829 public String toCode() { 830 switch (this) { 831 case VISUALGROUP: 832 return "visual-group"; 833 case LOGICALGROUP: 834 return "logical-group"; 835 case SENTENCEGROUP: 836 return "sentence-group"; 837 case NULL: 838 return null; 839 default: 840 return "?"; 841 } 842 } 843 844 public String getSystem() { 845 switch (this) { 846 case VISUALGROUP: 847 return "http://hl7.org/fhir/action-grouping-behavior"; 848 case LOGICALGROUP: 849 return "http://hl7.org/fhir/action-grouping-behavior"; 850 case SENTENCEGROUP: 851 return "http://hl7.org/fhir/action-grouping-behavior"; 852 case NULL: 853 return null; 854 default: 855 return "?"; 856 } 857 } 858 859 public String getDefinition() { 860 switch (this) { 861 case VISUALGROUP: 862 return "Any group marked with this behavior should be displayed as a visual group to the end user."; 863 case LOGICALGROUP: 864 return "A group with this behavior logically groups its sub-elements, and may be shown as a visual group to the end user, but it is not required to do so."; 865 case SENTENCEGROUP: 866 return "A group of related alternative actions is a sentence group if the target referenced by the action is the same in all the actions and each action simply constitutes a different variation on how to specify the details for the target. For example, two actions that could be in a SentenceGroup are \"aspirin, 500 mg, 2 times per day\" and \"aspirin, 300 mg, 3 times per day\". In both cases, aspirin is the target referenced by the action, and the two actions represent different options for how aspirin might be ordered for the patient. Note that a SentenceGroup would almost always have an associated selection behavior of \"AtMostOne\", unless it's a required action, in which case, it would be \"ExactlyOne\"."; 867 case NULL: 868 return null; 869 default: 870 return "?"; 871 } 872 } 873 874 public String getDisplay() { 875 switch (this) { 876 case VISUALGROUP: 877 return "Visual Group"; 878 case LOGICALGROUP: 879 return "Logical Group"; 880 case SENTENCEGROUP: 881 return "Sentence Group"; 882 case NULL: 883 return null; 884 default: 885 return "?"; 886 } 887 } 888 } 889 890 public static class ActionGroupingBehaviorEnumFactory implements EnumFactory<ActionGroupingBehavior> { 891 public ActionGroupingBehavior fromCode(String codeString) throws IllegalArgumentException { 892 if (codeString == null || "".equals(codeString)) 893 if (codeString == null || "".equals(codeString)) 894 return null; 895 if ("visual-group".equals(codeString)) 896 return ActionGroupingBehavior.VISUALGROUP; 897 if ("logical-group".equals(codeString)) 898 return ActionGroupingBehavior.LOGICALGROUP; 899 if ("sentence-group".equals(codeString)) 900 return ActionGroupingBehavior.SENTENCEGROUP; 901 throw new IllegalArgumentException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 902 } 903 904 public Enumeration<ActionGroupingBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 905 if (code == null) 906 return null; 907 if (code.isEmpty()) 908 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 909 String codeString = code.asStringValue(); 910 if (codeString == null || "".equals(codeString)) 911 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.NULL, code); 912 if ("visual-group".equals(codeString)) 913 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.VISUALGROUP, code); 914 if ("logical-group".equals(codeString)) 915 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.LOGICALGROUP, code); 916 if ("sentence-group".equals(codeString)) 917 return new Enumeration<ActionGroupingBehavior>(this, ActionGroupingBehavior.SENTENCEGROUP, code); 918 throw new FHIRException("Unknown ActionGroupingBehavior code '" + codeString + "'"); 919 } 920 921 public String toCode(ActionGroupingBehavior code) { 922 if (code == ActionGroupingBehavior.VISUALGROUP) 923 return "visual-group"; 924 if (code == ActionGroupingBehavior.LOGICALGROUP) 925 return "logical-group"; 926 if (code == ActionGroupingBehavior.SENTENCEGROUP) 927 return "sentence-group"; 928 return "?"; 929 } 930 931 public String toSystem(ActionGroupingBehavior code) { 932 return code.getSystem(); 933 } 934 } 935 936 public enum ActionSelectionBehavior { 937 /** 938 * Any number of the actions in the group may be chosen, from zero to all. 939 */ 940 ANY, 941 /** 942 * All the actions in the group must be selected as a single unit. 943 */ 944 ALL, 945 /** 946 * All the actions in the group are meant to be chosen as a single unit: either 947 * all must be selected by the end user, or none may be selected. 948 */ 949 ALLORNONE, 950 /** 951 * The end user must choose one and only one of the selectable actions in the 952 * group. The user SHALL NOT choose none of the actions in the group. 953 */ 954 EXACTLYONE, 955 /** 956 * The end user may choose zero or at most one of the actions in the group. 957 */ 958 ATMOSTONE, 959 /** 960 * The end user must choose a minimum of one, and as many additional as desired. 961 */ 962 ONEORMORE, 963 /** 964 * added to help the parsers with the generic types 965 */ 966 NULL; 967 968 public static ActionSelectionBehavior fromCode(String codeString) throws FHIRException { 969 if (codeString == null || "".equals(codeString)) 970 return null; 971 if ("any".equals(codeString)) 972 return ANY; 973 if ("all".equals(codeString)) 974 return ALL; 975 if ("all-or-none".equals(codeString)) 976 return ALLORNONE; 977 if ("exactly-one".equals(codeString)) 978 return EXACTLYONE; 979 if ("at-most-one".equals(codeString)) 980 return ATMOSTONE; 981 if ("one-or-more".equals(codeString)) 982 return ONEORMORE; 983 if (Configuration.isAcceptInvalidEnums()) 984 return null; 985 else 986 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 987 } 988 989 public String toCode() { 990 switch (this) { 991 case ANY: 992 return "any"; 993 case ALL: 994 return "all"; 995 case ALLORNONE: 996 return "all-or-none"; 997 case EXACTLYONE: 998 return "exactly-one"; 999 case ATMOSTONE: 1000 return "at-most-one"; 1001 case ONEORMORE: 1002 return "one-or-more"; 1003 case NULL: 1004 return null; 1005 default: 1006 return "?"; 1007 } 1008 } 1009 1010 public String getSystem() { 1011 switch (this) { 1012 case ANY: 1013 return "http://hl7.org/fhir/action-selection-behavior"; 1014 case ALL: 1015 return "http://hl7.org/fhir/action-selection-behavior"; 1016 case ALLORNONE: 1017 return "http://hl7.org/fhir/action-selection-behavior"; 1018 case EXACTLYONE: 1019 return "http://hl7.org/fhir/action-selection-behavior"; 1020 case ATMOSTONE: 1021 return "http://hl7.org/fhir/action-selection-behavior"; 1022 case ONEORMORE: 1023 return "http://hl7.org/fhir/action-selection-behavior"; 1024 case NULL: 1025 return null; 1026 default: 1027 return "?"; 1028 } 1029 } 1030 1031 public String getDefinition() { 1032 switch (this) { 1033 case ANY: 1034 return "Any number of the actions in the group may be chosen, from zero to all."; 1035 case ALL: 1036 return "All the actions in the group must be selected as a single unit."; 1037 case ALLORNONE: 1038 return "All the actions in the group are meant to be chosen as a single unit: either all must be selected by the end user, or none may be selected."; 1039 case EXACTLYONE: 1040 return "The end user must choose one and only one of the selectable actions in the group. The user SHALL NOT choose none of the actions in the group."; 1041 case ATMOSTONE: 1042 return "The end user may choose zero or at most one of the actions in the group."; 1043 case ONEORMORE: 1044 return "The end user must choose a minimum of one, and as many additional as desired."; 1045 case NULL: 1046 return null; 1047 default: 1048 return "?"; 1049 } 1050 } 1051 1052 public String getDisplay() { 1053 switch (this) { 1054 case ANY: 1055 return "Any"; 1056 case ALL: 1057 return "All"; 1058 case ALLORNONE: 1059 return "All Or None"; 1060 case EXACTLYONE: 1061 return "Exactly One"; 1062 case ATMOSTONE: 1063 return "At Most One"; 1064 case ONEORMORE: 1065 return "One Or More"; 1066 case NULL: 1067 return null; 1068 default: 1069 return "?"; 1070 } 1071 } 1072 } 1073 1074 public static class ActionSelectionBehaviorEnumFactory implements EnumFactory<ActionSelectionBehavior> { 1075 public ActionSelectionBehavior fromCode(String codeString) throws IllegalArgumentException { 1076 if (codeString == null || "".equals(codeString)) 1077 if (codeString == null || "".equals(codeString)) 1078 return null; 1079 if ("any".equals(codeString)) 1080 return ActionSelectionBehavior.ANY; 1081 if ("all".equals(codeString)) 1082 return ActionSelectionBehavior.ALL; 1083 if ("all-or-none".equals(codeString)) 1084 return ActionSelectionBehavior.ALLORNONE; 1085 if ("exactly-one".equals(codeString)) 1086 return ActionSelectionBehavior.EXACTLYONE; 1087 if ("at-most-one".equals(codeString)) 1088 return ActionSelectionBehavior.ATMOSTONE; 1089 if ("one-or-more".equals(codeString)) 1090 return ActionSelectionBehavior.ONEORMORE; 1091 throw new IllegalArgumentException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1092 } 1093 1094 public Enumeration<ActionSelectionBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1095 if (code == null) 1096 return null; 1097 if (code.isEmpty()) 1098 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1099 String codeString = code.asStringValue(); 1100 if (codeString == null || "".equals(codeString)) 1101 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.NULL, code); 1102 if ("any".equals(codeString)) 1103 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ANY, code); 1104 if ("all".equals(codeString)) 1105 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALL, code); 1106 if ("all-or-none".equals(codeString)) 1107 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ALLORNONE, code); 1108 if ("exactly-one".equals(codeString)) 1109 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.EXACTLYONE, code); 1110 if ("at-most-one".equals(codeString)) 1111 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ATMOSTONE, code); 1112 if ("one-or-more".equals(codeString)) 1113 return new Enumeration<ActionSelectionBehavior>(this, ActionSelectionBehavior.ONEORMORE, code); 1114 throw new FHIRException("Unknown ActionSelectionBehavior code '" + codeString + "'"); 1115 } 1116 1117 public String toCode(ActionSelectionBehavior code) { 1118 if (code == ActionSelectionBehavior.ANY) 1119 return "any"; 1120 if (code == ActionSelectionBehavior.ALL) 1121 return "all"; 1122 if (code == ActionSelectionBehavior.ALLORNONE) 1123 return "all-or-none"; 1124 if (code == ActionSelectionBehavior.EXACTLYONE) 1125 return "exactly-one"; 1126 if (code == ActionSelectionBehavior.ATMOSTONE) 1127 return "at-most-one"; 1128 if (code == ActionSelectionBehavior.ONEORMORE) 1129 return "one-or-more"; 1130 return "?"; 1131 } 1132 1133 public String toSystem(ActionSelectionBehavior code) { 1134 return code.getSystem(); 1135 } 1136 } 1137 1138 public enum ActionRequiredBehavior { 1139 /** 1140 * An action with this behavior must be included in the actions processed by the 1141 * end user; the end user SHALL NOT choose not to include this action. 1142 */ 1143 MUST, 1144 /** 1145 * An action with this behavior may be included in the set of actions processed 1146 * by the end user. 1147 */ 1148 COULD, 1149 /** 1150 * An action with this behavior must be included in the set of actions processed 1151 * by the end user, unless the end user provides documentation as to why the 1152 * action was not included. 1153 */ 1154 MUSTUNLESSDOCUMENTED, 1155 /** 1156 * added to help the parsers with the generic types 1157 */ 1158 NULL; 1159 1160 public static ActionRequiredBehavior fromCode(String codeString) throws FHIRException { 1161 if (codeString == null || "".equals(codeString)) 1162 return null; 1163 if ("must".equals(codeString)) 1164 return MUST; 1165 if ("could".equals(codeString)) 1166 return COULD; 1167 if ("must-unless-documented".equals(codeString)) 1168 return MUSTUNLESSDOCUMENTED; 1169 if (Configuration.isAcceptInvalidEnums()) 1170 return null; 1171 else 1172 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1173 } 1174 1175 public String toCode() { 1176 switch (this) { 1177 case MUST: 1178 return "must"; 1179 case COULD: 1180 return "could"; 1181 case MUSTUNLESSDOCUMENTED: 1182 return "must-unless-documented"; 1183 case NULL: 1184 return null; 1185 default: 1186 return "?"; 1187 } 1188 } 1189 1190 public String getSystem() { 1191 switch (this) { 1192 case MUST: 1193 return "http://hl7.org/fhir/action-required-behavior"; 1194 case COULD: 1195 return "http://hl7.org/fhir/action-required-behavior"; 1196 case MUSTUNLESSDOCUMENTED: 1197 return "http://hl7.org/fhir/action-required-behavior"; 1198 case NULL: 1199 return null; 1200 default: 1201 return "?"; 1202 } 1203 } 1204 1205 public String getDefinition() { 1206 switch (this) { 1207 case MUST: 1208 return "An action with this behavior must be included in the actions processed by the end user; the end user SHALL NOT choose not to include this action."; 1209 case COULD: 1210 return "An action with this behavior may be included in the set of actions processed by the end user."; 1211 case MUSTUNLESSDOCUMENTED: 1212 return "An action with this behavior must be included in the set of actions processed by the end user, unless the end user provides documentation as to why the action was not included."; 1213 case NULL: 1214 return null; 1215 default: 1216 return "?"; 1217 } 1218 } 1219 1220 public String getDisplay() { 1221 switch (this) { 1222 case MUST: 1223 return "Must"; 1224 case COULD: 1225 return "Could"; 1226 case MUSTUNLESSDOCUMENTED: 1227 return "Must Unless Documented"; 1228 case NULL: 1229 return null; 1230 default: 1231 return "?"; 1232 } 1233 } 1234 } 1235 1236 public static class ActionRequiredBehaviorEnumFactory implements EnumFactory<ActionRequiredBehavior> { 1237 public ActionRequiredBehavior fromCode(String codeString) throws IllegalArgumentException { 1238 if (codeString == null || "".equals(codeString)) 1239 if (codeString == null || "".equals(codeString)) 1240 return null; 1241 if ("must".equals(codeString)) 1242 return ActionRequiredBehavior.MUST; 1243 if ("could".equals(codeString)) 1244 return ActionRequiredBehavior.COULD; 1245 if ("must-unless-documented".equals(codeString)) 1246 return ActionRequiredBehavior.MUSTUNLESSDOCUMENTED; 1247 throw new IllegalArgumentException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1248 } 1249 1250 public Enumeration<ActionRequiredBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1251 if (code == null) 1252 return null; 1253 if (code.isEmpty()) 1254 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1255 String codeString = code.asStringValue(); 1256 if (codeString == null || "".equals(codeString)) 1257 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.NULL, code); 1258 if ("must".equals(codeString)) 1259 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUST, code); 1260 if ("could".equals(codeString)) 1261 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.COULD, code); 1262 if ("must-unless-documented".equals(codeString)) 1263 return new Enumeration<ActionRequiredBehavior>(this, ActionRequiredBehavior.MUSTUNLESSDOCUMENTED, code); 1264 throw new FHIRException("Unknown ActionRequiredBehavior code '" + codeString + "'"); 1265 } 1266 1267 public String toCode(ActionRequiredBehavior code) { 1268 if (code == ActionRequiredBehavior.MUST) 1269 return "must"; 1270 if (code == ActionRequiredBehavior.COULD) 1271 return "could"; 1272 if (code == ActionRequiredBehavior.MUSTUNLESSDOCUMENTED) 1273 return "must-unless-documented"; 1274 return "?"; 1275 } 1276 1277 public String toSystem(ActionRequiredBehavior code) { 1278 return code.getSystem(); 1279 } 1280 } 1281 1282 public enum ActionPrecheckBehavior { 1283 /** 1284 * An action with this behavior is one of the most frequent action that is, or 1285 * should be, included by an end user, for the particular context in which the 1286 * action occurs. The system displaying the action to the end user should 1287 * consider "pre-checking" such an action as a convenience for the user. 1288 */ 1289 YES, 1290 /** 1291 * An action with this behavior is one of the less frequent actions included by 1292 * the end user, for the particular context in which the action occurs. The 1293 * system displaying the actions to the end user would typically not "pre-check" 1294 * such an action. 1295 */ 1296 NO, 1297 /** 1298 * added to help the parsers with the generic types 1299 */ 1300 NULL; 1301 1302 public static ActionPrecheckBehavior fromCode(String codeString) throws FHIRException { 1303 if (codeString == null || "".equals(codeString)) 1304 return null; 1305 if ("yes".equals(codeString)) 1306 return YES; 1307 if ("no".equals(codeString)) 1308 return NO; 1309 if (Configuration.isAcceptInvalidEnums()) 1310 return null; 1311 else 1312 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1313 } 1314 1315 public String toCode() { 1316 switch (this) { 1317 case YES: 1318 return "yes"; 1319 case NO: 1320 return "no"; 1321 case NULL: 1322 return null; 1323 default: 1324 return "?"; 1325 } 1326 } 1327 1328 public String getSystem() { 1329 switch (this) { 1330 case YES: 1331 return "http://hl7.org/fhir/action-precheck-behavior"; 1332 case NO: 1333 return "http://hl7.org/fhir/action-precheck-behavior"; 1334 case NULL: 1335 return null; 1336 default: 1337 return "?"; 1338 } 1339 } 1340 1341 public String getDefinition() { 1342 switch (this) { 1343 case YES: 1344 return "An action with this behavior is one of the most frequent action that is, or should be, included by an end user, for the particular context in which the action occurs. The system displaying the action to the end user should consider \"pre-checking\" such an action as a convenience for the user."; 1345 case NO: 1346 return "An action with this behavior is one of the less frequent actions included by the end user, for the particular context in which the action occurs. The system displaying the actions to the end user would typically not \"pre-check\" such an action."; 1347 case NULL: 1348 return null; 1349 default: 1350 return "?"; 1351 } 1352 } 1353 1354 public String getDisplay() { 1355 switch (this) { 1356 case YES: 1357 return "Yes"; 1358 case NO: 1359 return "No"; 1360 case NULL: 1361 return null; 1362 default: 1363 return "?"; 1364 } 1365 } 1366 } 1367 1368 public static class ActionPrecheckBehaviorEnumFactory implements EnumFactory<ActionPrecheckBehavior> { 1369 public ActionPrecheckBehavior fromCode(String codeString) throws IllegalArgumentException { 1370 if (codeString == null || "".equals(codeString)) 1371 if (codeString == null || "".equals(codeString)) 1372 return null; 1373 if ("yes".equals(codeString)) 1374 return ActionPrecheckBehavior.YES; 1375 if ("no".equals(codeString)) 1376 return ActionPrecheckBehavior.NO; 1377 throw new IllegalArgumentException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1378 } 1379 1380 public Enumeration<ActionPrecheckBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1381 if (code == null) 1382 return null; 1383 if (code.isEmpty()) 1384 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1385 String codeString = code.asStringValue(); 1386 if (codeString == null || "".equals(codeString)) 1387 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NULL, code); 1388 if ("yes".equals(codeString)) 1389 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.YES, code); 1390 if ("no".equals(codeString)) 1391 return new Enumeration<ActionPrecheckBehavior>(this, ActionPrecheckBehavior.NO, code); 1392 throw new FHIRException("Unknown ActionPrecheckBehavior code '" + codeString + "'"); 1393 } 1394 1395 public String toCode(ActionPrecheckBehavior code) { 1396 if (code == ActionPrecheckBehavior.YES) 1397 return "yes"; 1398 if (code == ActionPrecheckBehavior.NO) 1399 return "no"; 1400 return "?"; 1401 } 1402 1403 public String toSystem(ActionPrecheckBehavior code) { 1404 return code.getSystem(); 1405 } 1406 } 1407 1408 public enum ActionCardinalityBehavior { 1409 /** 1410 * The action may only be selected one time. 1411 */ 1412 SINGLE, 1413 /** 1414 * The action may be selected multiple times. 1415 */ 1416 MULTIPLE, 1417 /** 1418 * added to help the parsers with the generic types 1419 */ 1420 NULL; 1421 1422 public static ActionCardinalityBehavior fromCode(String codeString) throws FHIRException { 1423 if (codeString == null || "".equals(codeString)) 1424 return null; 1425 if ("single".equals(codeString)) 1426 return SINGLE; 1427 if ("multiple".equals(codeString)) 1428 return MULTIPLE; 1429 if (Configuration.isAcceptInvalidEnums()) 1430 return null; 1431 else 1432 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1433 } 1434 1435 public String toCode() { 1436 switch (this) { 1437 case SINGLE: 1438 return "single"; 1439 case MULTIPLE: 1440 return "multiple"; 1441 case NULL: 1442 return null; 1443 default: 1444 return "?"; 1445 } 1446 } 1447 1448 public String getSystem() { 1449 switch (this) { 1450 case SINGLE: 1451 return "http://hl7.org/fhir/action-cardinality-behavior"; 1452 case MULTIPLE: 1453 return "http://hl7.org/fhir/action-cardinality-behavior"; 1454 case NULL: 1455 return null; 1456 default: 1457 return "?"; 1458 } 1459 } 1460 1461 public String getDefinition() { 1462 switch (this) { 1463 case SINGLE: 1464 return "The action may only be selected one time."; 1465 case MULTIPLE: 1466 return "The action may be selected multiple times."; 1467 case NULL: 1468 return null; 1469 default: 1470 return "?"; 1471 } 1472 } 1473 1474 public String getDisplay() { 1475 switch (this) { 1476 case SINGLE: 1477 return "Single"; 1478 case MULTIPLE: 1479 return "Multiple"; 1480 case NULL: 1481 return null; 1482 default: 1483 return "?"; 1484 } 1485 } 1486 } 1487 1488 public static class ActionCardinalityBehaviorEnumFactory implements EnumFactory<ActionCardinalityBehavior> { 1489 public ActionCardinalityBehavior fromCode(String codeString) throws IllegalArgumentException { 1490 if (codeString == null || "".equals(codeString)) 1491 if (codeString == null || "".equals(codeString)) 1492 return null; 1493 if ("single".equals(codeString)) 1494 return ActionCardinalityBehavior.SINGLE; 1495 if ("multiple".equals(codeString)) 1496 return ActionCardinalityBehavior.MULTIPLE; 1497 throw new IllegalArgumentException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1498 } 1499 1500 public Enumeration<ActionCardinalityBehavior> fromType(PrimitiveType<?> code) throws FHIRException { 1501 if (code == null) 1502 return null; 1503 if (code.isEmpty()) 1504 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1505 String codeString = code.asStringValue(); 1506 if (codeString == null || "".equals(codeString)) 1507 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.NULL, code); 1508 if ("single".equals(codeString)) 1509 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.SINGLE, code); 1510 if ("multiple".equals(codeString)) 1511 return new Enumeration<ActionCardinalityBehavior>(this, ActionCardinalityBehavior.MULTIPLE, code); 1512 throw new FHIRException("Unknown ActionCardinalityBehavior code '" + codeString + "'"); 1513 } 1514 1515 public String toCode(ActionCardinalityBehavior code) { 1516 if (code == ActionCardinalityBehavior.SINGLE) 1517 return "single"; 1518 if (code == ActionCardinalityBehavior.MULTIPLE) 1519 return "multiple"; 1520 return "?"; 1521 } 1522 1523 public String toSystem(ActionCardinalityBehavior code) { 1524 return code.getSystem(); 1525 } 1526 } 1527 1528 @Block() 1529 public static class PlanDefinitionGoalComponent extends BackboneElement implements IBaseBackboneElement { 1530 /** 1531 * Indicates a category the goal falls within. 1532 */ 1533 @Child(name = "category", type = { 1534 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1535 @Description(shortDefinition = "E.g. Treatment, dietary, behavioral", formalDefinition = "Indicates a category the goal falls within.") 1536 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-category") 1537 protected CodeableConcept category; 1538 1539 /** 1540 * Human-readable and/or coded description of a specific desired objective of 1541 * care, such as "control blood pressure" or "negotiate an obstacle course" or 1542 * "dance with child at wedding". 1543 */ 1544 @Child(name = "description", type = { 1545 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1546 @Description(shortDefinition = "Code or text describing the goal", formalDefinition = "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".") 1547 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 1548 protected CodeableConcept description; 1549 1550 /** 1551 * Identifies the expected level of importance associated with 1552 * reaching/sustaining the defined goal. 1553 */ 1554 @Child(name = "priority", type = { 1555 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1556 @Description(shortDefinition = "high-priority | medium-priority | low-priority", formalDefinition = "Identifies the expected level of importance associated with reaching/sustaining the defined goal.") 1557 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-priority") 1558 protected CodeableConcept priority; 1559 1560 /** 1561 * The event after which the goal should begin being pursued. 1562 */ 1563 @Child(name = "start", type = { 1564 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1565 @Description(shortDefinition = "When goal pursuit begins", formalDefinition = "The event after which the goal should begin being pursued.") 1566 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/goal-start-event") 1567 protected CodeableConcept start; 1568 1569 /** 1570 * Identifies problems, conditions, issues, or concerns the goal is intended to 1571 * address. 1572 */ 1573 @Child(name = "addresses", type = { 1574 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1575 @Description(shortDefinition = "What does the goal address", formalDefinition = "Identifies problems, conditions, issues, or concerns the goal is intended to address.") 1576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 1577 protected List<CodeableConcept> addresses; 1578 1579 /** 1580 * Didactic or other informational resources associated with the goal that 1581 * provide further supporting information about the goal. Information resources 1582 * can include inline text commentary and links to web resources. 1583 */ 1584 @Child(name = "documentation", type = { 1585 RelatedArtifact.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1586 @Description(shortDefinition = "Supporting documentation for the goal", formalDefinition = "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.") 1587 protected List<RelatedArtifact> documentation; 1588 1589 /** 1590 * Indicates what should be done and within what timeframe. 1591 */ 1592 @Child(name = "target", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1593 @Description(shortDefinition = "Target outcome for the goal", formalDefinition = "Indicates what should be done and within what timeframe.") 1594 protected List<PlanDefinitionGoalTargetComponent> target; 1595 1596 private static final long serialVersionUID = -795308926L; 1597 1598 /** 1599 * Constructor 1600 */ 1601 public PlanDefinitionGoalComponent() { 1602 super(); 1603 } 1604 1605 /** 1606 * Constructor 1607 */ 1608 public PlanDefinitionGoalComponent(CodeableConcept description) { 1609 super(); 1610 this.description = description; 1611 } 1612 1613 /** 1614 * @return {@link #category} (Indicates a category the goal falls within.) 1615 */ 1616 public CodeableConcept getCategory() { 1617 if (this.category == null) 1618 if (Configuration.errorOnAutoCreate()) 1619 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.category"); 1620 else if (Configuration.doAutoCreate()) 1621 this.category = new CodeableConcept(); // cc 1622 return this.category; 1623 } 1624 1625 public boolean hasCategory() { 1626 return this.category != null && !this.category.isEmpty(); 1627 } 1628 1629 /** 1630 * @param value {@link #category} (Indicates a category the goal falls within.) 1631 */ 1632 public PlanDefinitionGoalComponent setCategory(CodeableConcept value) { 1633 this.category = value; 1634 return this; 1635 } 1636 1637 /** 1638 * @return {@link #description} (Human-readable and/or coded description of a 1639 * specific desired objective of care, such as "control blood pressure" 1640 * or "negotiate an obstacle course" or "dance with child at wedding".) 1641 */ 1642 public CodeableConcept getDescription() { 1643 if (this.description == null) 1644 if (Configuration.errorOnAutoCreate()) 1645 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.description"); 1646 else if (Configuration.doAutoCreate()) 1647 this.description = new CodeableConcept(); // cc 1648 return this.description; 1649 } 1650 1651 public boolean hasDescription() { 1652 return this.description != null && !this.description.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #description} (Human-readable and/or coded description of 1657 * a specific desired objective of care, such as "control blood 1658 * pressure" or "negotiate an obstacle course" or "dance with child 1659 * at wedding".) 1660 */ 1661 public PlanDefinitionGoalComponent setDescription(CodeableConcept value) { 1662 this.description = value; 1663 return this; 1664 } 1665 1666 /** 1667 * @return {@link #priority} (Identifies the expected level of importance 1668 * associated with reaching/sustaining the defined goal.) 1669 */ 1670 public CodeableConcept getPriority() { 1671 if (this.priority == null) 1672 if (Configuration.errorOnAutoCreate()) 1673 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.priority"); 1674 else if (Configuration.doAutoCreate()) 1675 this.priority = new CodeableConcept(); // cc 1676 return this.priority; 1677 } 1678 1679 public boolean hasPriority() { 1680 return this.priority != null && !this.priority.isEmpty(); 1681 } 1682 1683 /** 1684 * @param value {@link #priority} (Identifies the expected level of importance 1685 * associated with reaching/sustaining the defined goal.) 1686 */ 1687 public PlanDefinitionGoalComponent setPriority(CodeableConcept value) { 1688 this.priority = value; 1689 return this; 1690 } 1691 1692 /** 1693 * @return {@link #start} (The event after which the goal should begin being 1694 * pursued.) 1695 */ 1696 public CodeableConcept getStart() { 1697 if (this.start == null) 1698 if (Configuration.errorOnAutoCreate()) 1699 throw new Error("Attempt to auto-create PlanDefinitionGoalComponent.start"); 1700 else if (Configuration.doAutoCreate()) 1701 this.start = new CodeableConcept(); // cc 1702 return this.start; 1703 } 1704 1705 public boolean hasStart() { 1706 return this.start != null && !this.start.isEmpty(); 1707 } 1708 1709 /** 1710 * @param value {@link #start} (The event after which the goal should begin 1711 * being pursued.) 1712 */ 1713 public PlanDefinitionGoalComponent setStart(CodeableConcept value) { 1714 this.start = value; 1715 return this; 1716 } 1717 1718 /** 1719 * @return {@link #addresses} (Identifies problems, conditions, issues, or 1720 * concerns the goal is intended to address.) 1721 */ 1722 public List<CodeableConcept> getAddresses() { 1723 if (this.addresses == null) 1724 this.addresses = new ArrayList<CodeableConcept>(); 1725 return this.addresses; 1726 } 1727 1728 /** 1729 * @return Returns a reference to <code>this</code> for easy method chaining 1730 */ 1731 public PlanDefinitionGoalComponent setAddresses(List<CodeableConcept> theAddresses) { 1732 this.addresses = theAddresses; 1733 return this; 1734 } 1735 1736 public boolean hasAddresses() { 1737 if (this.addresses == null) 1738 return false; 1739 for (CodeableConcept item : this.addresses) 1740 if (!item.isEmpty()) 1741 return true; 1742 return false; 1743 } 1744 1745 public CodeableConcept addAddresses() { // 3 1746 CodeableConcept t = new CodeableConcept(); 1747 if (this.addresses == null) 1748 this.addresses = new ArrayList<CodeableConcept>(); 1749 this.addresses.add(t); 1750 return t; 1751 } 1752 1753 public PlanDefinitionGoalComponent addAddresses(CodeableConcept t) { // 3 1754 if (t == null) 1755 return this; 1756 if (this.addresses == null) 1757 this.addresses = new ArrayList<CodeableConcept>(); 1758 this.addresses.add(t); 1759 return this; 1760 } 1761 1762 /** 1763 * @return The first repetition of repeating field {@link #addresses}, creating 1764 * it if it does not already exist 1765 */ 1766 public CodeableConcept getAddressesFirstRep() { 1767 if (getAddresses().isEmpty()) { 1768 addAddresses(); 1769 } 1770 return getAddresses().get(0); 1771 } 1772 1773 /** 1774 * @return {@link #documentation} (Didactic or other informational resources 1775 * associated with the goal that provide further supporting information 1776 * about the goal. Information resources can include inline text 1777 * commentary and links to web resources.) 1778 */ 1779 public List<RelatedArtifact> getDocumentation() { 1780 if (this.documentation == null) 1781 this.documentation = new ArrayList<RelatedArtifact>(); 1782 return this.documentation; 1783 } 1784 1785 /** 1786 * @return Returns a reference to <code>this</code> for easy method chaining 1787 */ 1788 public PlanDefinitionGoalComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 1789 this.documentation = theDocumentation; 1790 return this; 1791 } 1792 1793 public boolean hasDocumentation() { 1794 if (this.documentation == null) 1795 return false; 1796 for (RelatedArtifact item : this.documentation) 1797 if (!item.isEmpty()) 1798 return true; 1799 return false; 1800 } 1801 1802 public RelatedArtifact addDocumentation() { // 3 1803 RelatedArtifact t = new RelatedArtifact(); 1804 if (this.documentation == null) 1805 this.documentation = new ArrayList<RelatedArtifact>(); 1806 this.documentation.add(t); 1807 return t; 1808 } 1809 1810 public PlanDefinitionGoalComponent addDocumentation(RelatedArtifact t) { // 3 1811 if (t == null) 1812 return this; 1813 if (this.documentation == null) 1814 this.documentation = new ArrayList<RelatedArtifact>(); 1815 this.documentation.add(t); 1816 return this; 1817 } 1818 1819 /** 1820 * @return The first repetition of repeating field {@link #documentation}, 1821 * creating it if it does not already exist 1822 */ 1823 public RelatedArtifact getDocumentationFirstRep() { 1824 if (getDocumentation().isEmpty()) { 1825 addDocumentation(); 1826 } 1827 return getDocumentation().get(0); 1828 } 1829 1830 /** 1831 * @return {@link #target} (Indicates what should be done and within what 1832 * timeframe.) 1833 */ 1834 public List<PlanDefinitionGoalTargetComponent> getTarget() { 1835 if (this.target == null) 1836 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1837 return this.target; 1838 } 1839 1840 /** 1841 * @return Returns a reference to <code>this</code> for easy method chaining 1842 */ 1843 public PlanDefinitionGoalComponent setTarget(List<PlanDefinitionGoalTargetComponent> theTarget) { 1844 this.target = theTarget; 1845 return this; 1846 } 1847 1848 public boolean hasTarget() { 1849 if (this.target == null) 1850 return false; 1851 for (PlanDefinitionGoalTargetComponent item : this.target) 1852 if (!item.isEmpty()) 1853 return true; 1854 return false; 1855 } 1856 1857 public PlanDefinitionGoalTargetComponent addTarget() { // 3 1858 PlanDefinitionGoalTargetComponent t = new PlanDefinitionGoalTargetComponent(); 1859 if (this.target == null) 1860 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1861 this.target.add(t); 1862 return t; 1863 } 1864 1865 public PlanDefinitionGoalComponent addTarget(PlanDefinitionGoalTargetComponent t) { // 3 1866 if (t == null) 1867 return this; 1868 if (this.target == null) 1869 this.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 1870 this.target.add(t); 1871 return this; 1872 } 1873 1874 /** 1875 * @return The first repetition of repeating field {@link #target}, creating it 1876 * if it does not already exist 1877 */ 1878 public PlanDefinitionGoalTargetComponent getTargetFirstRep() { 1879 if (getTarget().isEmpty()) { 1880 addTarget(); 1881 } 1882 return getTarget().get(0); 1883 } 1884 1885 protected void listChildren(List<Property> children) { 1886 super.listChildren(children); 1887 children.add( 1888 new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, 1, category)); 1889 children.add(new Property("description", "CodeableConcept", 1890 "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 1891 0, 1, description)); 1892 children.add(new Property("priority", "CodeableConcept", 1893 "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, 1894 priority)); 1895 children.add(new Property("start", "CodeableConcept", 1896 "The event after which the goal should begin being pursued.", 0, 1, start)); 1897 children.add(new Property("addresses", "CodeableConcept", 1898 "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, 1899 java.lang.Integer.MAX_VALUE, addresses)); 1900 children.add(new Property("documentation", "RelatedArtifact", 1901 "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 1902 0, java.lang.Integer.MAX_VALUE, documentation)); 1903 children.add(new Property("target", "", "Indicates what should be done and within what timeframe.", 0, 1904 java.lang.Integer.MAX_VALUE, target)); 1905 } 1906 1907 @Override 1908 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1909 switch (_hash) { 1910 case 50511102: 1911 /* category */ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 1912 0, 1, category); 1913 case -1724546052: 1914 /* description */ return new Property("description", "CodeableConcept", 1915 "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 1916 0, 1, description); 1917 case -1165461084: 1918 /* priority */ return new Property("priority", "CodeableConcept", 1919 "Identifies the expected level of importance associated with reaching/sustaining the defined goal.", 0, 1, 1920 priority); 1921 case 109757538: 1922 /* start */ return new Property("start", "CodeableConcept", 1923 "The event after which the goal should begin being pursued.", 0, 1, start); 1924 case 874544034: 1925 /* addresses */ return new Property("addresses", "CodeableConcept", 1926 "Identifies problems, conditions, issues, or concerns the goal is intended to address.", 0, 1927 java.lang.Integer.MAX_VALUE, addresses); 1928 case 1587405498: 1929 /* documentation */ return new Property("documentation", "RelatedArtifact", 1930 "Didactic or other informational resources associated with the goal that provide further supporting information about the goal. Information resources can include inline text commentary and links to web resources.", 1931 0, java.lang.Integer.MAX_VALUE, documentation); 1932 case -880905839: 1933 /* target */ return new Property("target", "", "Indicates what should be done and within what timeframe.", 0, 1934 java.lang.Integer.MAX_VALUE, target); 1935 default: 1936 return super.getNamedProperty(_hash, _name, _checkValid); 1937 } 1938 1939 } 1940 1941 @Override 1942 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1943 switch (hash) { 1944 case 50511102: 1945 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1946 case -1724546052: 1947 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // CodeableConcept 1948 case -1165461084: 1949 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 1950 case 109757538: 1951 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // CodeableConcept 1952 case 874544034: 1953 /* addresses */ return this.addresses == null ? new Base[0] 1954 : this.addresses.toArray(new Base[this.addresses.size()]); // CodeableConcept 1955 case 1587405498: 1956 /* documentation */ return this.documentation == null ? new Base[0] 1957 : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 1958 case -880905839: 1959 /* target */ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // PlanDefinitionGoalTargetComponent 1960 default: 1961 return super.getProperty(hash, name, checkValid); 1962 } 1963 1964 } 1965 1966 @Override 1967 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1968 switch (hash) { 1969 case 50511102: // category 1970 this.category = castToCodeableConcept(value); // CodeableConcept 1971 return value; 1972 case -1724546052: // description 1973 this.description = castToCodeableConcept(value); // CodeableConcept 1974 return value; 1975 case -1165461084: // priority 1976 this.priority = castToCodeableConcept(value); // CodeableConcept 1977 return value; 1978 case 109757538: // start 1979 this.start = castToCodeableConcept(value); // CodeableConcept 1980 return value; 1981 case 874544034: // addresses 1982 this.getAddresses().add(castToCodeableConcept(value)); // CodeableConcept 1983 return value; 1984 case 1587405498: // documentation 1985 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 1986 return value; 1987 case -880905839: // target 1988 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); // PlanDefinitionGoalTargetComponent 1989 return value; 1990 default: 1991 return super.setProperty(hash, name, value); 1992 } 1993 1994 } 1995 1996 @Override 1997 public Base setProperty(String name, Base value) throws FHIRException { 1998 if (name.equals("category")) { 1999 this.category = castToCodeableConcept(value); // CodeableConcept 2000 } else if (name.equals("description")) { 2001 this.description = castToCodeableConcept(value); // CodeableConcept 2002 } else if (name.equals("priority")) { 2003 this.priority = castToCodeableConcept(value); // CodeableConcept 2004 } else if (name.equals("start")) { 2005 this.start = castToCodeableConcept(value); // CodeableConcept 2006 } else if (name.equals("addresses")) { 2007 this.getAddresses().add(castToCodeableConcept(value)); 2008 } else if (name.equals("documentation")) { 2009 this.getDocumentation().add(castToRelatedArtifact(value)); 2010 } else if (name.equals("target")) { 2011 this.getTarget().add((PlanDefinitionGoalTargetComponent) value); 2012 } else 2013 return super.setProperty(name, value); 2014 return value; 2015 } 2016 2017 @Override 2018 public Base makeProperty(int hash, String name) throws FHIRException { 2019 switch (hash) { 2020 case 50511102: 2021 return getCategory(); 2022 case -1724546052: 2023 return getDescription(); 2024 case -1165461084: 2025 return getPriority(); 2026 case 109757538: 2027 return getStart(); 2028 case 874544034: 2029 return addAddresses(); 2030 case 1587405498: 2031 return addDocumentation(); 2032 case -880905839: 2033 return addTarget(); 2034 default: 2035 return super.makeProperty(hash, name); 2036 } 2037 2038 } 2039 2040 @Override 2041 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2042 switch (hash) { 2043 case 50511102: 2044 /* category */ return new String[] { "CodeableConcept" }; 2045 case -1724546052: 2046 /* description */ return new String[] { "CodeableConcept" }; 2047 case -1165461084: 2048 /* priority */ return new String[] { "CodeableConcept" }; 2049 case 109757538: 2050 /* start */ return new String[] { "CodeableConcept" }; 2051 case 874544034: 2052 /* addresses */ return new String[] { "CodeableConcept" }; 2053 case 1587405498: 2054 /* documentation */ return new String[] { "RelatedArtifact" }; 2055 case -880905839: 2056 /* target */ return new String[] {}; 2057 default: 2058 return super.getTypesForProperty(hash, name); 2059 } 2060 2061 } 2062 2063 @Override 2064 public Base addChild(String name) throws FHIRException { 2065 if (name.equals("category")) { 2066 this.category = new CodeableConcept(); 2067 return this.category; 2068 } else if (name.equals("description")) { 2069 this.description = new CodeableConcept(); 2070 return this.description; 2071 } else if (name.equals("priority")) { 2072 this.priority = new CodeableConcept(); 2073 return this.priority; 2074 } else if (name.equals("start")) { 2075 this.start = new CodeableConcept(); 2076 return this.start; 2077 } else if (name.equals("addresses")) { 2078 return addAddresses(); 2079 } else if (name.equals("documentation")) { 2080 return addDocumentation(); 2081 } else if (name.equals("target")) { 2082 return addTarget(); 2083 } else 2084 return super.addChild(name); 2085 } 2086 2087 public PlanDefinitionGoalComponent copy() { 2088 PlanDefinitionGoalComponent dst = new PlanDefinitionGoalComponent(); 2089 copyValues(dst); 2090 return dst; 2091 } 2092 2093 public void copyValues(PlanDefinitionGoalComponent dst) { 2094 super.copyValues(dst); 2095 dst.category = category == null ? null : category.copy(); 2096 dst.description = description == null ? null : description.copy(); 2097 dst.priority = priority == null ? null : priority.copy(); 2098 dst.start = start == null ? null : start.copy(); 2099 if (addresses != null) { 2100 dst.addresses = new ArrayList<CodeableConcept>(); 2101 for (CodeableConcept i : addresses) 2102 dst.addresses.add(i.copy()); 2103 } 2104 ; 2105 if (documentation != null) { 2106 dst.documentation = new ArrayList<RelatedArtifact>(); 2107 for (RelatedArtifact i : documentation) 2108 dst.documentation.add(i.copy()); 2109 } 2110 ; 2111 if (target != null) { 2112 dst.target = new ArrayList<PlanDefinitionGoalTargetComponent>(); 2113 for (PlanDefinitionGoalTargetComponent i : target) 2114 dst.target.add(i.copy()); 2115 } 2116 ; 2117 } 2118 2119 @Override 2120 public boolean equalsDeep(Base other_) { 2121 if (!super.equalsDeep(other_)) 2122 return false; 2123 if (!(other_ instanceof PlanDefinitionGoalComponent)) 2124 return false; 2125 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 2126 return compareDeep(category, o.category, true) && compareDeep(description, o.description, true) 2127 && compareDeep(priority, o.priority, true) && compareDeep(start, o.start, true) 2128 && compareDeep(addresses, o.addresses, true) && compareDeep(documentation, o.documentation, true) 2129 && compareDeep(target, o.target, true); 2130 } 2131 2132 @Override 2133 public boolean equalsShallow(Base other_) { 2134 if (!super.equalsShallow(other_)) 2135 return false; 2136 if (!(other_ instanceof PlanDefinitionGoalComponent)) 2137 return false; 2138 PlanDefinitionGoalComponent o = (PlanDefinitionGoalComponent) other_; 2139 return true; 2140 } 2141 2142 public boolean isEmpty() { 2143 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, description, priority, start, addresses, 2144 documentation, target); 2145 } 2146 2147 public String fhirType() { 2148 return "PlanDefinition.goal"; 2149 2150 } 2151 2152 } 2153 2154 @Block() 2155 public static class PlanDefinitionGoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 2156 /** 2157 * The parameter whose value is to be tracked, e.g. body weight, blood pressure, 2158 * or hemoglobin A1c level. 2159 */ 2160 @Child(name = "measure", type = { 2161 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2162 @Description(shortDefinition = "The parameter whose value is to be tracked", formalDefinition = "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.") 2163 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 2164 protected CodeableConcept measure; 2165 2166 /** 2167 * The target value of the measure to be achieved to signify fulfillment of the 2168 * goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the 2169 * range can be specified. When a low value is missing, it indicates that the 2170 * goal is achieved at any value at or below the high value. Similarly, if the 2171 * high value is missing, it indicates that the goal is achieved at any value at 2172 * or above the low value. 2173 */ 2174 @Child(name = "detail", type = { Quantity.class, Range.class, 2175 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2176 @Description(shortDefinition = "The target value to be achieved", formalDefinition = "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.") 2177 protected Type detail; 2178 2179 /** 2180 * Indicates the timeframe after the start of the goal in which the goal should 2181 * be met. 2182 */ 2183 @Child(name = "due", type = { Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2184 @Description(shortDefinition = "Reach goal within", formalDefinition = "Indicates the timeframe after the start of the goal in which the goal should be met.") 2185 protected Duration due; 2186 2187 private static final long serialVersionUID = -131874144L; 2188 2189 /** 2190 * Constructor 2191 */ 2192 public PlanDefinitionGoalTargetComponent() { 2193 super(); 2194 } 2195 2196 /** 2197 * @return {@link #measure} (The parameter whose value is to be tracked, e.g. 2198 * body weight, blood pressure, or hemoglobin A1c level.) 2199 */ 2200 public CodeableConcept getMeasure() { 2201 if (this.measure == null) 2202 if (Configuration.errorOnAutoCreate()) 2203 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.measure"); 2204 else if (Configuration.doAutoCreate()) 2205 this.measure = new CodeableConcept(); // cc 2206 return this.measure; 2207 } 2208 2209 public boolean hasMeasure() { 2210 return this.measure != null && !this.measure.isEmpty(); 2211 } 2212 2213 /** 2214 * @param value {@link #measure} (The parameter whose value is to be tracked, 2215 * e.g. body weight, blood pressure, or hemoglobin A1c level.) 2216 */ 2217 public PlanDefinitionGoalTargetComponent setMeasure(CodeableConcept value) { 2218 this.measure = value; 2219 return this; 2220 } 2221 2222 /** 2223 * @return {@link #detail} (The target value of the measure to be achieved to 2224 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2225 * high or low or both values of the range can be specified. When a low 2226 * value is missing, it indicates that the goal is achieved at any value 2227 * at or below the high value. Similarly, if the high value is missing, 2228 * it indicates that the goal is achieved at any value at or above the 2229 * low value.) 2230 */ 2231 public Type getDetail() { 2232 return this.detail; 2233 } 2234 2235 /** 2236 * @return {@link #detail} (The target value of the measure to be achieved to 2237 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2238 * high or low or both values of the range can be specified. When a low 2239 * value is missing, it indicates that the goal is achieved at any value 2240 * at or below the high value. Similarly, if the high value is missing, 2241 * it indicates that the goal is achieved at any value at or above the 2242 * low value.) 2243 */ 2244 public Quantity getDetailQuantity() throws FHIRException { 2245 if (this.detail == null) 2246 this.detail = new Quantity(); 2247 if (!(this.detail instanceof Quantity)) 2248 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.detail.getClass().getName() 2249 + " was encountered"); 2250 return (Quantity) this.detail; 2251 } 2252 2253 public boolean hasDetailQuantity() { 2254 return this != null && this.detail instanceof Quantity; 2255 } 2256 2257 /** 2258 * @return {@link #detail} (The target value of the measure to be achieved to 2259 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2260 * high or low or both values of the range can be specified. When a low 2261 * value is missing, it indicates that the goal is achieved at any value 2262 * at or below the high value. Similarly, if the high value is missing, 2263 * it indicates that the goal is achieved at any value at or above the 2264 * low value.) 2265 */ 2266 public Range getDetailRange() throws FHIRException { 2267 if (this.detail == null) 2268 this.detail = new Range(); 2269 if (!(this.detail instanceof Range)) 2270 throw new FHIRException( 2271 "Type mismatch: the type Range was expected, but " + this.detail.getClass().getName() + " was encountered"); 2272 return (Range) this.detail; 2273 } 2274 2275 public boolean hasDetailRange() { 2276 return this != null && this.detail instanceof Range; 2277 } 2278 2279 /** 2280 * @return {@link #detail} (The target value of the measure to be achieved to 2281 * signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the 2282 * high or low or both values of the range can be specified. When a low 2283 * value is missing, it indicates that the goal is achieved at any value 2284 * at or below the high value. Similarly, if the high value is missing, 2285 * it indicates that the goal is achieved at any value at or above the 2286 * low value.) 2287 */ 2288 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 2289 if (this.detail == null) 2290 this.detail = new CodeableConcept(); 2291 if (!(this.detail instanceof CodeableConcept)) 2292 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2293 + this.detail.getClass().getName() + " was encountered"); 2294 return (CodeableConcept) this.detail; 2295 } 2296 2297 public boolean hasDetailCodeableConcept() { 2298 return this != null && this.detail instanceof CodeableConcept; 2299 } 2300 2301 public boolean hasDetail() { 2302 return this.detail != null && !this.detail.isEmpty(); 2303 } 2304 2305 /** 2306 * @param value {@link #detail} (The target value of the measure to be achieved 2307 * to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. 2308 * Either the high or low or both values of the range can be 2309 * specified. When a low value is missing, it indicates that the 2310 * goal is achieved at any value at or below the high value. 2311 * Similarly, if the high value is missing, it indicates that the 2312 * goal is achieved at any value at or above the low value.) 2313 */ 2314 public PlanDefinitionGoalTargetComponent setDetail(Type value) { 2315 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept)) 2316 throw new Error("Not the right type for PlanDefinition.goal.target.detail[x]: " + value.fhirType()); 2317 this.detail = value; 2318 return this; 2319 } 2320 2321 /** 2322 * @return {@link #due} (Indicates the timeframe after the start of the goal in 2323 * which the goal should be met.) 2324 */ 2325 public Duration getDue() { 2326 if (this.due == null) 2327 if (Configuration.errorOnAutoCreate()) 2328 throw new Error("Attempt to auto-create PlanDefinitionGoalTargetComponent.due"); 2329 else if (Configuration.doAutoCreate()) 2330 this.due = new Duration(); // cc 2331 return this.due; 2332 } 2333 2334 public boolean hasDue() { 2335 return this.due != null && !this.due.isEmpty(); 2336 } 2337 2338 /** 2339 * @param value {@link #due} (Indicates the timeframe after the start of the 2340 * goal in which the goal should be met.) 2341 */ 2342 public PlanDefinitionGoalTargetComponent setDue(Duration value) { 2343 this.due = value; 2344 return this; 2345 } 2346 2347 protected void listChildren(List<Property> children) { 2348 super.listChildren(children); 2349 children.add(new Property("measure", "CodeableConcept", 2350 "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 2351 1, measure)); 2352 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept", 2353 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2354 0, 1, detail)); 2355 children.add(new Property("due", "Duration", 2356 "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due)); 2357 } 2358 2359 @Override 2360 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2361 switch (_hash) { 2362 case 938321246: 2363 /* measure */ return new Property("measure", "CodeableConcept", 2364 "The parameter whose value is to be tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 2365 1, measure); 2366 case -1973084529: 2367 /* detail[x] */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2368 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2369 0, 1, detail); 2370 case -1335224239: 2371 /* detail */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2372 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2373 0, 1, detail); 2374 case -1313079300: 2375 /* detailQuantity */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2376 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2377 0, 1, detail); 2378 case -2062632084: 2379 /* detailRange */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2380 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2381 0, 1, detail); 2382 case -175586544: 2383 /* detailCodeableConcept */ return new Property("detail[x]", "Quantity|Range|CodeableConcept", 2384 "The target value of the measure to be achieved to signify fulfillment of the goal, e.g. 150 pounds or 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any value at or above the low value.", 2385 0, 1, detail); 2386 case 99828: 2387 /* due */ return new Property("due", "Duration", 2388 "Indicates the timeframe after the start of the goal in which the goal should be met.", 0, 1, due); 2389 default: 2390 return super.getNamedProperty(_hash, _name, _checkValid); 2391 } 2392 2393 } 2394 2395 @Override 2396 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2397 switch (hash) { 2398 case 938321246: 2399 /* measure */ return this.measure == null ? new Base[0] : new Base[] { this.measure }; // CodeableConcept 2400 case -1335224239: 2401 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // Type 2402 case 99828: 2403 /* due */ return this.due == null ? new Base[0] : new Base[] { this.due }; // Duration 2404 default: 2405 return super.getProperty(hash, name, checkValid); 2406 } 2407 2408 } 2409 2410 @Override 2411 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2412 switch (hash) { 2413 case 938321246: // measure 2414 this.measure = castToCodeableConcept(value); // CodeableConcept 2415 return value; 2416 case -1335224239: // detail 2417 this.detail = castToType(value); // Type 2418 return value; 2419 case 99828: // due 2420 this.due = castToDuration(value); // Duration 2421 return value; 2422 default: 2423 return super.setProperty(hash, name, value); 2424 } 2425 2426 } 2427 2428 @Override 2429 public Base setProperty(String name, Base value) throws FHIRException { 2430 if (name.equals("measure")) { 2431 this.measure = castToCodeableConcept(value); // CodeableConcept 2432 } else if (name.equals("detail[x]")) { 2433 this.detail = castToType(value); // Type 2434 } else if (name.equals("due")) { 2435 this.due = castToDuration(value); // Duration 2436 } else 2437 return super.setProperty(name, value); 2438 return value; 2439 } 2440 2441 @Override 2442 public Base makeProperty(int hash, String name) throws FHIRException { 2443 switch (hash) { 2444 case 938321246: 2445 return getMeasure(); 2446 case -1973084529: 2447 return getDetail(); 2448 case -1335224239: 2449 return getDetail(); 2450 case 99828: 2451 return getDue(); 2452 default: 2453 return super.makeProperty(hash, name); 2454 } 2455 2456 } 2457 2458 @Override 2459 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2460 switch (hash) { 2461 case 938321246: 2462 /* measure */ return new String[] { "CodeableConcept" }; 2463 case -1335224239: 2464 /* detail */ return new String[] { "Quantity", "Range", "CodeableConcept" }; 2465 case 99828: 2466 /* due */ return new String[] { "Duration" }; 2467 default: 2468 return super.getTypesForProperty(hash, name); 2469 } 2470 2471 } 2472 2473 @Override 2474 public Base addChild(String name) throws FHIRException { 2475 if (name.equals("measure")) { 2476 this.measure = new CodeableConcept(); 2477 return this.measure; 2478 } else if (name.equals("detailQuantity")) { 2479 this.detail = new Quantity(); 2480 return this.detail; 2481 } else if (name.equals("detailRange")) { 2482 this.detail = new Range(); 2483 return this.detail; 2484 } else if (name.equals("detailCodeableConcept")) { 2485 this.detail = new CodeableConcept(); 2486 return this.detail; 2487 } else if (name.equals("due")) { 2488 this.due = new Duration(); 2489 return this.due; 2490 } else 2491 return super.addChild(name); 2492 } 2493 2494 public PlanDefinitionGoalTargetComponent copy() { 2495 PlanDefinitionGoalTargetComponent dst = new PlanDefinitionGoalTargetComponent(); 2496 copyValues(dst); 2497 return dst; 2498 } 2499 2500 public void copyValues(PlanDefinitionGoalTargetComponent dst) { 2501 super.copyValues(dst); 2502 dst.measure = measure == null ? null : measure.copy(); 2503 dst.detail = detail == null ? null : detail.copy(); 2504 dst.due = due == null ? null : due.copy(); 2505 } 2506 2507 @Override 2508 public boolean equalsDeep(Base other_) { 2509 if (!super.equalsDeep(other_)) 2510 return false; 2511 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 2512 return false; 2513 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 2514 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) 2515 && compareDeep(due, o.due, true); 2516 } 2517 2518 @Override 2519 public boolean equalsShallow(Base other_) { 2520 if (!super.equalsShallow(other_)) 2521 return false; 2522 if (!(other_ instanceof PlanDefinitionGoalTargetComponent)) 2523 return false; 2524 PlanDefinitionGoalTargetComponent o = (PlanDefinitionGoalTargetComponent) other_; 2525 return true; 2526 } 2527 2528 public boolean isEmpty() { 2529 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 2530 } 2531 2532 public String fhirType() { 2533 return "PlanDefinition.goal.target"; 2534 2535 } 2536 2537 } 2538 2539 @Block() 2540 public static class PlanDefinitionActionComponent extends BackboneElement implements IBaseBackboneElement { 2541 /** 2542 * A user-visible prefix for the action. 2543 */ 2544 @Child(name = "prefix", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2545 @Description(shortDefinition = "User-visible prefix for the action (e.g. 1. or A.)", formalDefinition = "A user-visible prefix for the action.") 2546 protected StringType prefix; 2547 2548 /** 2549 * The title of the action displayed to a user. 2550 */ 2551 @Child(name = "title", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2552 @Description(shortDefinition = "User-visible title", formalDefinition = "The title of the action displayed to a user.") 2553 protected StringType title; 2554 2555 /** 2556 * A brief description of the action used to provide a summary to display to the 2557 * user. 2558 */ 2559 @Child(name = "description", type = { 2560 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2561 @Description(shortDefinition = "Brief description of the action", formalDefinition = "A brief description of the action used to provide a summary to display to the user.") 2562 protected StringType description; 2563 2564 /** 2565 * A text equivalent of the action to be performed. This provides a 2566 * human-interpretable description of the action when the definition is consumed 2567 * by a system that might not be capable of interpreting it dynamically. 2568 */ 2569 @Child(name = "textEquivalent", type = { 2570 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2571 @Description(shortDefinition = "Static text equivalent of the action, used if the dynamic aspects cannot be interpreted by the receiving system", formalDefinition = "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.") 2572 protected StringType textEquivalent; 2573 2574 /** 2575 * Indicates how quickly the action should be addressed with respect to other 2576 * actions. 2577 */ 2578 @Child(name = "priority", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2579 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the action should be addressed with respect to other actions.") 2580 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 2581 protected Enumeration<RequestPriority> priority; 2582 2583 /** 2584 * A code that provides meaning for the action or action group. For example, a 2585 * section may have a LOINC code for the section of a documentation template. 2586 */ 2587 @Child(name = "code", type = { 2588 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2589 @Description(shortDefinition = "Code representing the meaning of the action or sub-actions", formalDefinition = "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for the section of a documentation template.") 2590 protected List<CodeableConcept> code; 2591 2592 /** 2593 * A description of why this action is necessary or appropriate. 2594 */ 2595 @Child(name = "reason", type = { 2596 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2597 @Description(shortDefinition = "Why the action should be performed", formalDefinition = "A description of why this action is necessary or appropriate.") 2598 protected List<CodeableConcept> reason; 2599 2600 /** 2601 * Didactic or other informational resources associated with the action that can 2602 * be provided to the CDS recipient. Information resources can include inline 2603 * text commentary and links to web resources. 2604 */ 2605 @Child(name = "documentation", type = { 2606 RelatedArtifact.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2607 @Description(shortDefinition = "Supporting documentation for the intended performer of the action", formalDefinition = "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.") 2608 protected List<RelatedArtifact> documentation; 2609 2610 /** 2611 * Identifies goals that this action supports. The reference must be to a goal 2612 * element defined within this plan definition. 2613 */ 2614 @Child(name = "goalId", type = { 2615 IdType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2616 @Description(shortDefinition = "What goals this action supports", formalDefinition = "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.") 2617 protected List<IdType> goalId; 2618 2619 /** 2620 * A code or group definition that describes the intended subject of the action 2621 * and its children, if any. 2622 */ 2623 @Child(name = "subject", type = { CodeableConcept.class, 2624 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2625 @Description(shortDefinition = "Type of individual the action is focused on", formalDefinition = "A code or group definition that describes the intended subject of the action and its children, if any.") 2626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 2627 protected Type subject; 2628 2629 /** 2630 * A description of when the action should be triggered. 2631 */ 2632 @Child(name = "trigger", type = { 2633 TriggerDefinition.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2634 @Description(shortDefinition = "When the action should be triggered", formalDefinition = "A description of when the action should be triggered.") 2635 protected List<TriggerDefinition> trigger; 2636 2637 /** 2638 * An expression that describes applicability criteria or start/stop conditions 2639 * for the action. 2640 */ 2641 @Child(name = "condition", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2642 @Description(shortDefinition = "Whether or not the action is applicable", formalDefinition = "An expression that describes applicability criteria or start/stop conditions for the action.") 2643 protected List<PlanDefinitionActionConditionComponent> condition; 2644 2645 /** 2646 * Defines input data requirements for the action. 2647 */ 2648 @Child(name = "input", type = { 2649 DataRequirement.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2650 @Description(shortDefinition = "Input data requirements", formalDefinition = "Defines input data requirements for the action.") 2651 protected List<DataRequirement> input; 2652 2653 /** 2654 * Defines the outputs of the action, if any. 2655 */ 2656 @Child(name = "output", type = { 2657 DataRequirement.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2658 @Description(shortDefinition = "Output data definition", formalDefinition = "Defines the outputs of the action, if any.") 2659 protected List<DataRequirement> output; 2660 2661 /** 2662 * A relationship to another action such as "before" or "30-60 minutes after 2663 * start of". 2664 */ 2665 @Child(name = "relatedAction", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2666 @Description(shortDefinition = "Relationship to another action", formalDefinition = "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".") 2667 protected List<PlanDefinitionActionRelatedActionComponent> relatedAction; 2668 2669 /** 2670 * An optional value describing when the action should be performed. 2671 */ 2672 @Child(name = "timing", type = { DateTimeType.class, Age.class, Period.class, Duration.class, Range.class, 2673 Timing.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2674 @Description(shortDefinition = "When the action should take place", formalDefinition = "An optional value describing when the action should be performed.") 2675 protected Type timing; 2676 2677 /** 2678 * Indicates who should participate in performing the action described. 2679 */ 2680 @Child(name = "participant", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2681 @Description(shortDefinition = "Who should participate in the action", formalDefinition = "Indicates who should participate in performing the action described.") 2682 protected List<PlanDefinitionActionParticipantComponent> participant; 2683 2684 /** 2685 * The type of action to perform (create, update, remove). 2686 */ 2687 @Child(name = "type", type = { 2688 CodeableConcept.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 2689 @Description(shortDefinition = "create | update | remove | fire-event", formalDefinition = "The type of action to perform (create, update, remove).") 2690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-type") 2691 protected CodeableConcept type; 2692 2693 /** 2694 * Defines the grouping behavior for the action and its children. 2695 */ 2696 @Child(name = "groupingBehavior", type = { 2697 CodeType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 2698 @Description(shortDefinition = "visual-group | logical-group | sentence-group", formalDefinition = "Defines the grouping behavior for the action and its children.") 2699 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-grouping-behavior") 2700 protected Enumeration<ActionGroupingBehavior> groupingBehavior; 2701 2702 /** 2703 * Defines the selection behavior for the action and its children. 2704 */ 2705 @Child(name = "selectionBehavior", type = { 2706 CodeType.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 2707 @Description(shortDefinition = "any | all | all-or-none | exactly-one | at-most-one | one-or-more", formalDefinition = "Defines the selection behavior for the action and its children.") 2708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-selection-behavior") 2709 protected Enumeration<ActionSelectionBehavior> selectionBehavior; 2710 2711 /** 2712 * Defines the required behavior for the action. 2713 */ 2714 @Child(name = "requiredBehavior", type = { 2715 CodeType.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 2716 @Description(shortDefinition = "must | could | must-unless-documented", formalDefinition = "Defines the required behavior for the action.") 2717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-required-behavior") 2718 protected Enumeration<ActionRequiredBehavior> requiredBehavior; 2719 2720 /** 2721 * Defines whether the action should usually be preselected. 2722 */ 2723 @Child(name = "precheckBehavior", type = { 2724 CodeType.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 2725 @Description(shortDefinition = "yes | no", formalDefinition = "Defines whether the action should usually be preselected.") 2726 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-precheck-behavior") 2727 protected Enumeration<ActionPrecheckBehavior> precheckBehavior; 2728 2729 /** 2730 * Defines whether the action can be selected multiple times. 2731 */ 2732 @Child(name = "cardinalityBehavior", type = { 2733 CodeType.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 2734 @Description(shortDefinition = "single | multiple", formalDefinition = "Defines whether the action can be selected multiple times.") 2735 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-cardinality-behavior") 2736 protected Enumeration<ActionCardinalityBehavior> cardinalityBehavior; 2737 2738 /** 2739 * A reference to an ActivityDefinition that describes the action to be taken in 2740 * detail, or a PlanDefinition that describes a series of actions to be taken. 2741 */ 2742 @Child(name = "definition", type = { CanonicalType.class, 2743 UriType.class }, order = 24, min = 0, max = 1, modifier = false, summary = false) 2744 @Description(shortDefinition = "Description of the activity to be performed", formalDefinition = "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.") 2745 protected Type definition; 2746 2747 /** 2748 * A reference to a StructureMap resource that defines a transform that can be 2749 * executed to produce the intent resource using the ActivityDefinition instance 2750 * as the input. 2751 */ 2752 @Child(name = "transform", type = { 2753 CanonicalType.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 2754 @Description(shortDefinition = "Transform to apply the template", formalDefinition = "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.") 2755 protected CanonicalType transform; 2756 2757 /** 2758 * Customizations that should be applied to the statically defined resource. For 2759 * example, if the dosage of a medication must be computed based on the 2760 * patient's weight, a customization would be used to specify an expression that 2761 * calculated the weight, and the path on the resource that would contain the 2762 * result. 2763 */ 2764 @Child(name = "dynamicValue", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2765 @Description(shortDefinition = "Dynamic aspects of the definition", formalDefinition = "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.") 2766 protected List<PlanDefinitionActionDynamicValueComponent> dynamicValue; 2767 2768 /** 2769 * Sub actions that are contained within the action. The behavior of this action 2770 * determines the functionality of the sub-actions. For example, a selection 2771 * behavior of at-most-one indicates that of the sub-actions, at most one may be 2772 * chosen as part of realizing the action definition. 2773 */ 2774 @Child(name = "action", type = { 2775 PlanDefinitionActionComponent.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2776 @Description(shortDefinition = "A sub-action", formalDefinition = "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.") 2777 protected List<PlanDefinitionActionComponent> action; 2778 2779 private static final long serialVersionUID = 158605540L; 2780 2781 /** 2782 * Constructor 2783 */ 2784 public PlanDefinitionActionComponent() { 2785 super(); 2786 } 2787 2788 /** 2789 * @return {@link #prefix} (A user-visible prefix for the action.). This is the 2790 * underlying object with id, value and extensions. The accessor 2791 * "getPrefix" gives direct access to the value 2792 */ 2793 public StringType getPrefixElement() { 2794 if (this.prefix == null) 2795 if (Configuration.errorOnAutoCreate()) 2796 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.prefix"); 2797 else if (Configuration.doAutoCreate()) 2798 this.prefix = new StringType(); // bb 2799 return this.prefix; 2800 } 2801 2802 public boolean hasPrefixElement() { 2803 return this.prefix != null && !this.prefix.isEmpty(); 2804 } 2805 2806 public boolean hasPrefix() { 2807 return this.prefix != null && !this.prefix.isEmpty(); 2808 } 2809 2810 /** 2811 * @param value {@link #prefix} (A user-visible prefix for the action.). This is 2812 * the underlying object with id, value and extensions. The 2813 * accessor "getPrefix" gives direct access to the value 2814 */ 2815 public PlanDefinitionActionComponent setPrefixElement(StringType value) { 2816 this.prefix = value; 2817 return this; 2818 } 2819 2820 /** 2821 * @return A user-visible prefix for the action. 2822 */ 2823 public String getPrefix() { 2824 return this.prefix == null ? null : this.prefix.getValue(); 2825 } 2826 2827 /** 2828 * @param value A user-visible prefix for the action. 2829 */ 2830 public PlanDefinitionActionComponent setPrefix(String value) { 2831 if (Utilities.noString(value)) 2832 this.prefix = null; 2833 else { 2834 if (this.prefix == null) 2835 this.prefix = new StringType(); 2836 this.prefix.setValue(value); 2837 } 2838 return this; 2839 } 2840 2841 /** 2842 * @return {@link #title} (The title of the action displayed to a user.). This 2843 * is the underlying object with id, value and extensions. The accessor 2844 * "getTitle" gives direct access to the value 2845 */ 2846 public StringType getTitleElement() { 2847 if (this.title == null) 2848 if (Configuration.errorOnAutoCreate()) 2849 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.title"); 2850 else if (Configuration.doAutoCreate()) 2851 this.title = new StringType(); // bb 2852 return this.title; 2853 } 2854 2855 public boolean hasTitleElement() { 2856 return this.title != null && !this.title.isEmpty(); 2857 } 2858 2859 public boolean hasTitle() { 2860 return this.title != null && !this.title.isEmpty(); 2861 } 2862 2863 /** 2864 * @param value {@link #title} (The title of the action displayed to a user.). 2865 * This is the underlying object with id, value and extensions. The 2866 * accessor "getTitle" gives direct access to the value 2867 */ 2868 public PlanDefinitionActionComponent setTitleElement(StringType value) { 2869 this.title = value; 2870 return this; 2871 } 2872 2873 /** 2874 * @return The title of the action displayed to a user. 2875 */ 2876 public String getTitle() { 2877 return this.title == null ? null : this.title.getValue(); 2878 } 2879 2880 /** 2881 * @param value The title of the action displayed to a user. 2882 */ 2883 public PlanDefinitionActionComponent setTitle(String value) { 2884 if (Utilities.noString(value)) 2885 this.title = null; 2886 else { 2887 if (this.title == null) 2888 this.title = new StringType(); 2889 this.title.setValue(value); 2890 } 2891 return this; 2892 } 2893 2894 /** 2895 * @return {@link #description} (A brief description of the action used to 2896 * provide a summary to display to the user.). This is the underlying 2897 * object with id, value and extensions. The accessor "getDescription" 2898 * gives direct access to the value 2899 */ 2900 public StringType getDescriptionElement() { 2901 if (this.description == null) 2902 if (Configuration.errorOnAutoCreate()) 2903 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.description"); 2904 else if (Configuration.doAutoCreate()) 2905 this.description = new StringType(); // bb 2906 return this.description; 2907 } 2908 2909 public boolean hasDescriptionElement() { 2910 return this.description != null && !this.description.isEmpty(); 2911 } 2912 2913 public boolean hasDescription() { 2914 return this.description != null && !this.description.isEmpty(); 2915 } 2916 2917 /** 2918 * @param value {@link #description} (A brief description of the action used to 2919 * provide a summary to display to the user.). This is the 2920 * underlying object with id, value and extensions. The accessor 2921 * "getDescription" gives direct access to the value 2922 */ 2923 public PlanDefinitionActionComponent setDescriptionElement(StringType value) { 2924 this.description = value; 2925 return this; 2926 } 2927 2928 /** 2929 * @return A brief description of the action used to provide a summary to 2930 * display to the user. 2931 */ 2932 public String getDescription() { 2933 return this.description == null ? null : this.description.getValue(); 2934 } 2935 2936 /** 2937 * @param value A brief description of the action used to provide a summary to 2938 * display to the user. 2939 */ 2940 public PlanDefinitionActionComponent setDescription(String value) { 2941 if (Utilities.noString(value)) 2942 this.description = null; 2943 else { 2944 if (this.description == null) 2945 this.description = new StringType(); 2946 this.description.setValue(value); 2947 } 2948 return this; 2949 } 2950 2951 /** 2952 * @return {@link #textEquivalent} (A text equivalent of the action to be 2953 * performed. This provides a human-interpretable description of the 2954 * action when the definition is consumed by a system that might not be 2955 * capable of interpreting it dynamically.). This is the underlying 2956 * object with id, value and extensions. The accessor 2957 * "getTextEquivalent" gives direct access to the value 2958 */ 2959 public StringType getTextEquivalentElement() { 2960 if (this.textEquivalent == null) 2961 if (Configuration.errorOnAutoCreate()) 2962 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.textEquivalent"); 2963 else if (Configuration.doAutoCreate()) 2964 this.textEquivalent = new StringType(); // bb 2965 return this.textEquivalent; 2966 } 2967 2968 public boolean hasTextEquivalentElement() { 2969 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2970 } 2971 2972 public boolean hasTextEquivalent() { 2973 return this.textEquivalent != null && !this.textEquivalent.isEmpty(); 2974 } 2975 2976 /** 2977 * @param value {@link #textEquivalent} (A text equivalent of the action to be 2978 * performed. This provides a human-interpretable description of 2979 * the action when the definition is consumed by a system that 2980 * might not be capable of interpreting it dynamically.). This is 2981 * the underlying object with id, value and extensions. The 2982 * accessor "getTextEquivalent" gives direct access to the value 2983 */ 2984 public PlanDefinitionActionComponent setTextEquivalentElement(StringType value) { 2985 this.textEquivalent = value; 2986 return this; 2987 } 2988 2989 /** 2990 * @return A text equivalent of the action to be performed. This provides a 2991 * human-interpretable description of the action when the definition is 2992 * consumed by a system that might not be capable of interpreting it 2993 * dynamically. 2994 */ 2995 public String getTextEquivalent() { 2996 return this.textEquivalent == null ? null : this.textEquivalent.getValue(); 2997 } 2998 2999 /** 3000 * @param value A text equivalent of the action to be performed. This provides a 3001 * human-interpretable description of the action when the 3002 * definition is consumed by a system that might not be capable of 3003 * interpreting it dynamically. 3004 */ 3005 public PlanDefinitionActionComponent setTextEquivalent(String value) { 3006 if (Utilities.noString(value)) 3007 this.textEquivalent = null; 3008 else { 3009 if (this.textEquivalent == null) 3010 this.textEquivalent = new StringType(); 3011 this.textEquivalent.setValue(value); 3012 } 3013 return this; 3014 } 3015 3016 /** 3017 * @return {@link #priority} (Indicates how quickly the action should be 3018 * addressed with respect to other actions.). This is the underlying 3019 * object with id, value and extensions. The accessor "getPriority" 3020 * gives direct access to the value 3021 */ 3022 public Enumeration<RequestPriority> getPriorityElement() { 3023 if (this.priority == null) 3024 if (Configuration.errorOnAutoCreate()) 3025 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.priority"); 3026 else if (Configuration.doAutoCreate()) 3027 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); // bb 3028 return this.priority; 3029 } 3030 3031 public boolean hasPriorityElement() { 3032 return this.priority != null && !this.priority.isEmpty(); 3033 } 3034 3035 public boolean hasPriority() { 3036 return this.priority != null && !this.priority.isEmpty(); 3037 } 3038 3039 /** 3040 * @param value {@link #priority} (Indicates how quickly the action should be 3041 * addressed with respect to other actions.). This is the 3042 * underlying object with id, value and extensions. The accessor 3043 * "getPriority" gives direct access to the value 3044 */ 3045 public PlanDefinitionActionComponent setPriorityElement(Enumeration<RequestPriority> value) { 3046 this.priority = value; 3047 return this; 3048 } 3049 3050 /** 3051 * @return Indicates how quickly the action should be addressed with respect to 3052 * other actions. 3053 */ 3054 public RequestPriority getPriority() { 3055 return this.priority == null ? null : this.priority.getValue(); 3056 } 3057 3058 /** 3059 * @param value Indicates how quickly the action should be addressed with 3060 * respect to other actions. 3061 */ 3062 public PlanDefinitionActionComponent setPriority(RequestPriority value) { 3063 if (value == null) 3064 this.priority = null; 3065 else { 3066 if (this.priority == null) 3067 this.priority = new Enumeration<RequestPriority>(new RequestPriorityEnumFactory()); 3068 this.priority.setValue(value); 3069 } 3070 return this; 3071 } 3072 3073 /** 3074 * @return {@link #code} (A code that provides meaning for the action or action 3075 * group. For example, a section may have a LOINC code for the section 3076 * of a documentation template.) 3077 */ 3078 public List<CodeableConcept> getCode() { 3079 if (this.code == null) 3080 this.code = new ArrayList<CodeableConcept>(); 3081 return this.code; 3082 } 3083 3084 /** 3085 * @return Returns a reference to <code>this</code> for easy method chaining 3086 */ 3087 public PlanDefinitionActionComponent setCode(List<CodeableConcept> theCode) { 3088 this.code = theCode; 3089 return this; 3090 } 3091 3092 public boolean hasCode() { 3093 if (this.code == null) 3094 return false; 3095 for (CodeableConcept item : this.code) 3096 if (!item.isEmpty()) 3097 return true; 3098 return false; 3099 } 3100 3101 public CodeableConcept addCode() { // 3 3102 CodeableConcept t = new CodeableConcept(); 3103 if (this.code == null) 3104 this.code = new ArrayList<CodeableConcept>(); 3105 this.code.add(t); 3106 return t; 3107 } 3108 3109 public PlanDefinitionActionComponent addCode(CodeableConcept t) { // 3 3110 if (t == null) 3111 return this; 3112 if (this.code == null) 3113 this.code = new ArrayList<CodeableConcept>(); 3114 this.code.add(t); 3115 return this; 3116 } 3117 3118 /** 3119 * @return The first repetition of repeating field {@link #code}, creating it if 3120 * it does not already exist 3121 */ 3122 public CodeableConcept getCodeFirstRep() { 3123 if (getCode().isEmpty()) { 3124 addCode(); 3125 } 3126 return getCode().get(0); 3127 } 3128 3129 /** 3130 * @return {@link #reason} (A description of why this action is necessary or 3131 * appropriate.) 3132 */ 3133 public List<CodeableConcept> getReason() { 3134 if (this.reason == null) 3135 this.reason = new ArrayList<CodeableConcept>(); 3136 return this.reason; 3137 } 3138 3139 /** 3140 * @return Returns a reference to <code>this</code> for easy method chaining 3141 */ 3142 public PlanDefinitionActionComponent setReason(List<CodeableConcept> theReason) { 3143 this.reason = theReason; 3144 return this; 3145 } 3146 3147 public boolean hasReason() { 3148 if (this.reason == null) 3149 return false; 3150 for (CodeableConcept item : this.reason) 3151 if (!item.isEmpty()) 3152 return true; 3153 return false; 3154 } 3155 3156 public CodeableConcept addReason() { // 3 3157 CodeableConcept t = new CodeableConcept(); 3158 if (this.reason == null) 3159 this.reason = new ArrayList<CodeableConcept>(); 3160 this.reason.add(t); 3161 return t; 3162 } 3163 3164 public PlanDefinitionActionComponent addReason(CodeableConcept t) { // 3 3165 if (t == null) 3166 return this; 3167 if (this.reason == null) 3168 this.reason = new ArrayList<CodeableConcept>(); 3169 this.reason.add(t); 3170 return this; 3171 } 3172 3173 /** 3174 * @return The first repetition of repeating field {@link #reason}, creating it 3175 * if it does not already exist 3176 */ 3177 public CodeableConcept getReasonFirstRep() { 3178 if (getReason().isEmpty()) { 3179 addReason(); 3180 } 3181 return getReason().get(0); 3182 } 3183 3184 /** 3185 * @return {@link #documentation} (Didactic or other informational resources 3186 * associated with the action that can be provided to the CDS recipient. 3187 * Information resources can include inline text commentary and links to 3188 * web resources.) 3189 */ 3190 public List<RelatedArtifact> getDocumentation() { 3191 if (this.documentation == null) 3192 this.documentation = new ArrayList<RelatedArtifact>(); 3193 return this.documentation; 3194 } 3195 3196 /** 3197 * @return Returns a reference to <code>this</code> for easy method chaining 3198 */ 3199 public PlanDefinitionActionComponent setDocumentation(List<RelatedArtifact> theDocumentation) { 3200 this.documentation = theDocumentation; 3201 return this; 3202 } 3203 3204 public boolean hasDocumentation() { 3205 if (this.documentation == null) 3206 return false; 3207 for (RelatedArtifact item : this.documentation) 3208 if (!item.isEmpty()) 3209 return true; 3210 return false; 3211 } 3212 3213 public RelatedArtifact addDocumentation() { // 3 3214 RelatedArtifact t = new RelatedArtifact(); 3215 if (this.documentation == null) 3216 this.documentation = new ArrayList<RelatedArtifact>(); 3217 this.documentation.add(t); 3218 return t; 3219 } 3220 3221 public PlanDefinitionActionComponent addDocumentation(RelatedArtifact t) { // 3 3222 if (t == null) 3223 return this; 3224 if (this.documentation == null) 3225 this.documentation = new ArrayList<RelatedArtifact>(); 3226 this.documentation.add(t); 3227 return this; 3228 } 3229 3230 /** 3231 * @return The first repetition of repeating field {@link #documentation}, 3232 * creating it if it does not already exist 3233 */ 3234 public RelatedArtifact getDocumentationFirstRep() { 3235 if (getDocumentation().isEmpty()) { 3236 addDocumentation(); 3237 } 3238 return getDocumentation().get(0); 3239 } 3240 3241 /** 3242 * @return {@link #goalId} (Identifies goals that this action supports. The 3243 * reference must be to a goal element defined within this plan 3244 * definition.) 3245 */ 3246 public List<IdType> getGoalId() { 3247 if (this.goalId == null) 3248 this.goalId = new ArrayList<IdType>(); 3249 return this.goalId; 3250 } 3251 3252 /** 3253 * @return Returns a reference to <code>this</code> for easy method chaining 3254 */ 3255 public PlanDefinitionActionComponent setGoalId(List<IdType> theGoalId) { 3256 this.goalId = theGoalId; 3257 return this; 3258 } 3259 3260 public boolean hasGoalId() { 3261 if (this.goalId == null) 3262 return false; 3263 for (IdType item : this.goalId) 3264 if (!item.isEmpty()) 3265 return true; 3266 return false; 3267 } 3268 3269 /** 3270 * @return {@link #goalId} (Identifies goals that this action supports. The 3271 * reference must be to a goal element defined within this plan 3272 * definition.) 3273 */ 3274 public IdType addGoalIdElement() {// 2 3275 IdType t = new IdType(); 3276 if (this.goalId == null) 3277 this.goalId = new ArrayList<IdType>(); 3278 this.goalId.add(t); 3279 return t; 3280 } 3281 3282 /** 3283 * @param value {@link #goalId} (Identifies goals that this action supports. The 3284 * reference must be to a goal element defined within this plan 3285 * definition.) 3286 */ 3287 public PlanDefinitionActionComponent addGoalId(String value) { // 1 3288 IdType t = new IdType(); 3289 t.setValue(value); 3290 if (this.goalId == null) 3291 this.goalId = new ArrayList<IdType>(); 3292 this.goalId.add(t); 3293 return this; 3294 } 3295 3296 /** 3297 * @param value {@link #goalId} (Identifies goals that this action supports. The 3298 * reference must be to a goal element defined within this plan 3299 * definition.) 3300 */ 3301 public boolean hasGoalId(String value) { 3302 if (this.goalId == null) 3303 return false; 3304 for (IdType v : this.goalId) 3305 if (v.getValue().equals(value)) // id 3306 return true; 3307 return false; 3308 } 3309 3310 /** 3311 * @return {@link #subject} (A code or group definition that describes the 3312 * intended subject of the action and its children, if any.) 3313 */ 3314 public Type getSubject() { 3315 return this.subject; 3316 } 3317 3318 /** 3319 * @return {@link #subject} (A code or group definition that describes the 3320 * intended subject of the action and its children, if any.) 3321 */ 3322 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 3323 if (this.subject == null) 3324 this.subject = new CodeableConcept(); 3325 if (!(this.subject instanceof CodeableConcept)) 3326 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 3327 + this.subject.getClass().getName() + " was encountered"); 3328 return (CodeableConcept) this.subject; 3329 } 3330 3331 public boolean hasSubjectCodeableConcept() { 3332 return this != null && this.subject instanceof CodeableConcept; 3333 } 3334 3335 /** 3336 * @return {@link #subject} (A code or group definition that describes the 3337 * intended subject of the action and its children, if any.) 3338 */ 3339 public Reference getSubjectReference() throws FHIRException { 3340 if (this.subject == null) 3341 this.subject = new Reference(); 3342 if (!(this.subject instanceof Reference)) 3343 throw new FHIRException("Type mismatch: the type Reference was expected, but " 3344 + this.subject.getClass().getName() + " was encountered"); 3345 return (Reference) this.subject; 3346 } 3347 3348 public boolean hasSubjectReference() { 3349 return this != null && this.subject instanceof Reference; 3350 } 3351 3352 public boolean hasSubject() { 3353 return this.subject != null && !this.subject.isEmpty(); 3354 } 3355 3356 /** 3357 * @param value {@link #subject} (A code or group definition that describes the 3358 * intended subject of the action and its children, if any.) 3359 */ 3360 public PlanDefinitionActionComponent setSubject(Type value) { 3361 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 3362 throw new Error("Not the right type for PlanDefinition.action.subject[x]: " + value.fhirType()); 3363 this.subject = value; 3364 return this; 3365 } 3366 3367 /** 3368 * @return {@link #trigger} (A description of when the action should be 3369 * triggered.) 3370 */ 3371 public List<TriggerDefinition> getTrigger() { 3372 if (this.trigger == null) 3373 this.trigger = new ArrayList<TriggerDefinition>(); 3374 return this.trigger; 3375 } 3376 3377 /** 3378 * @return Returns a reference to <code>this</code> for easy method chaining 3379 */ 3380 public PlanDefinitionActionComponent setTrigger(List<TriggerDefinition> theTrigger) { 3381 this.trigger = theTrigger; 3382 return this; 3383 } 3384 3385 public boolean hasTrigger() { 3386 if (this.trigger == null) 3387 return false; 3388 for (TriggerDefinition item : this.trigger) 3389 if (!item.isEmpty()) 3390 return true; 3391 return false; 3392 } 3393 3394 public TriggerDefinition addTrigger() { // 3 3395 TriggerDefinition t = new TriggerDefinition(); 3396 if (this.trigger == null) 3397 this.trigger = new ArrayList<TriggerDefinition>(); 3398 this.trigger.add(t); 3399 return t; 3400 } 3401 3402 public PlanDefinitionActionComponent addTrigger(TriggerDefinition t) { // 3 3403 if (t == null) 3404 return this; 3405 if (this.trigger == null) 3406 this.trigger = new ArrayList<TriggerDefinition>(); 3407 this.trigger.add(t); 3408 return this; 3409 } 3410 3411 /** 3412 * @return The first repetition of repeating field {@link #trigger}, creating it 3413 * if it does not already exist 3414 */ 3415 public TriggerDefinition getTriggerFirstRep() { 3416 if (getTrigger().isEmpty()) { 3417 addTrigger(); 3418 } 3419 return getTrigger().get(0); 3420 } 3421 3422 /** 3423 * @return {@link #condition} (An expression that describes applicability 3424 * criteria or start/stop conditions for the action.) 3425 */ 3426 public List<PlanDefinitionActionConditionComponent> getCondition() { 3427 if (this.condition == null) 3428 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3429 return this.condition; 3430 } 3431 3432 /** 3433 * @return Returns a reference to <code>this</code> for easy method chaining 3434 */ 3435 public PlanDefinitionActionComponent setCondition(List<PlanDefinitionActionConditionComponent> theCondition) { 3436 this.condition = theCondition; 3437 return this; 3438 } 3439 3440 public boolean hasCondition() { 3441 if (this.condition == null) 3442 return false; 3443 for (PlanDefinitionActionConditionComponent item : this.condition) 3444 if (!item.isEmpty()) 3445 return true; 3446 return false; 3447 } 3448 3449 public PlanDefinitionActionConditionComponent addCondition() { // 3 3450 PlanDefinitionActionConditionComponent t = new PlanDefinitionActionConditionComponent(); 3451 if (this.condition == null) 3452 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3453 this.condition.add(t); 3454 return t; 3455 } 3456 3457 public PlanDefinitionActionComponent addCondition(PlanDefinitionActionConditionComponent t) { // 3 3458 if (t == null) 3459 return this; 3460 if (this.condition == null) 3461 this.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 3462 this.condition.add(t); 3463 return this; 3464 } 3465 3466 /** 3467 * @return The first repetition of repeating field {@link #condition}, creating 3468 * it if it does not already exist 3469 */ 3470 public PlanDefinitionActionConditionComponent getConditionFirstRep() { 3471 if (getCondition().isEmpty()) { 3472 addCondition(); 3473 } 3474 return getCondition().get(0); 3475 } 3476 3477 /** 3478 * @return {@link #input} (Defines input data requirements for the action.) 3479 */ 3480 public List<DataRequirement> getInput() { 3481 if (this.input == null) 3482 this.input = new ArrayList<DataRequirement>(); 3483 return this.input; 3484 } 3485 3486 /** 3487 * @return Returns a reference to <code>this</code> for easy method chaining 3488 */ 3489 public PlanDefinitionActionComponent setInput(List<DataRequirement> theInput) { 3490 this.input = theInput; 3491 return this; 3492 } 3493 3494 public boolean hasInput() { 3495 if (this.input == null) 3496 return false; 3497 for (DataRequirement item : this.input) 3498 if (!item.isEmpty()) 3499 return true; 3500 return false; 3501 } 3502 3503 public DataRequirement addInput() { // 3 3504 DataRequirement t = new DataRequirement(); 3505 if (this.input == null) 3506 this.input = new ArrayList<DataRequirement>(); 3507 this.input.add(t); 3508 return t; 3509 } 3510 3511 public PlanDefinitionActionComponent addInput(DataRequirement t) { // 3 3512 if (t == null) 3513 return this; 3514 if (this.input == null) 3515 this.input = new ArrayList<DataRequirement>(); 3516 this.input.add(t); 3517 return this; 3518 } 3519 3520 /** 3521 * @return The first repetition of repeating field {@link #input}, creating it 3522 * if it does not already exist 3523 */ 3524 public DataRequirement getInputFirstRep() { 3525 if (getInput().isEmpty()) { 3526 addInput(); 3527 } 3528 return getInput().get(0); 3529 } 3530 3531 /** 3532 * @return {@link #output} (Defines the outputs of the action, if any.) 3533 */ 3534 public List<DataRequirement> getOutput() { 3535 if (this.output == null) 3536 this.output = new ArrayList<DataRequirement>(); 3537 return this.output; 3538 } 3539 3540 /** 3541 * @return Returns a reference to <code>this</code> for easy method chaining 3542 */ 3543 public PlanDefinitionActionComponent setOutput(List<DataRequirement> theOutput) { 3544 this.output = theOutput; 3545 return this; 3546 } 3547 3548 public boolean hasOutput() { 3549 if (this.output == null) 3550 return false; 3551 for (DataRequirement item : this.output) 3552 if (!item.isEmpty()) 3553 return true; 3554 return false; 3555 } 3556 3557 public DataRequirement addOutput() { // 3 3558 DataRequirement t = new DataRequirement(); 3559 if (this.output == null) 3560 this.output = new ArrayList<DataRequirement>(); 3561 this.output.add(t); 3562 return t; 3563 } 3564 3565 public PlanDefinitionActionComponent addOutput(DataRequirement t) { // 3 3566 if (t == null) 3567 return this; 3568 if (this.output == null) 3569 this.output = new ArrayList<DataRequirement>(); 3570 this.output.add(t); 3571 return this; 3572 } 3573 3574 /** 3575 * @return The first repetition of repeating field {@link #output}, creating it 3576 * if it does not already exist 3577 */ 3578 public DataRequirement getOutputFirstRep() { 3579 if (getOutput().isEmpty()) { 3580 addOutput(); 3581 } 3582 return getOutput().get(0); 3583 } 3584 3585 /** 3586 * @return {@link #relatedAction} (A relationship to another action such as 3587 * "before" or "30-60 minutes after start of".) 3588 */ 3589 public List<PlanDefinitionActionRelatedActionComponent> getRelatedAction() { 3590 if (this.relatedAction == null) 3591 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3592 return this.relatedAction; 3593 } 3594 3595 /** 3596 * @return Returns a reference to <code>this</code> for easy method chaining 3597 */ 3598 public PlanDefinitionActionComponent setRelatedAction( 3599 List<PlanDefinitionActionRelatedActionComponent> theRelatedAction) { 3600 this.relatedAction = theRelatedAction; 3601 return this; 3602 } 3603 3604 public boolean hasRelatedAction() { 3605 if (this.relatedAction == null) 3606 return false; 3607 for (PlanDefinitionActionRelatedActionComponent item : this.relatedAction) 3608 if (!item.isEmpty()) 3609 return true; 3610 return false; 3611 } 3612 3613 public PlanDefinitionActionRelatedActionComponent addRelatedAction() { // 3 3614 PlanDefinitionActionRelatedActionComponent t = new PlanDefinitionActionRelatedActionComponent(); 3615 if (this.relatedAction == null) 3616 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3617 this.relatedAction.add(t); 3618 return t; 3619 } 3620 3621 public PlanDefinitionActionComponent addRelatedAction(PlanDefinitionActionRelatedActionComponent t) { // 3 3622 if (t == null) 3623 return this; 3624 if (this.relatedAction == null) 3625 this.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 3626 this.relatedAction.add(t); 3627 return this; 3628 } 3629 3630 /** 3631 * @return The first repetition of repeating field {@link #relatedAction}, 3632 * creating it if it does not already exist 3633 */ 3634 public PlanDefinitionActionRelatedActionComponent getRelatedActionFirstRep() { 3635 if (getRelatedAction().isEmpty()) { 3636 addRelatedAction(); 3637 } 3638 return getRelatedAction().get(0); 3639 } 3640 3641 /** 3642 * @return {@link #timing} (An optional value describing when the action should 3643 * be performed.) 3644 */ 3645 public Type getTiming() { 3646 return this.timing; 3647 } 3648 3649 /** 3650 * @return {@link #timing} (An optional value describing when the action should 3651 * be performed.) 3652 */ 3653 public DateTimeType getTimingDateTimeType() throws FHIRException { 3654 if (this.timing == null) 3655 this.timing = new DateTimeType(); 3656 if (!(this.timing instanceof DateTimeType)) 3657 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 3658 + this.timing.getClass().getName() + " was encountered"); 3659 return (DateTimeType) this.timing; 3660 } 3661 3662 public boolean hasTimingDateTimeType() { 3663 return this != null && this.timing instanceof DateTimeType; 3664 } 3665 3666 /** 3667 * @return {@link #timing} (An optional value describing when the action should 3668 * be performed.) 3669 */ 3670 public Age getTimingAge() throws FHIRException { 3671 if (this.timing == null) 3672 this.timing = new Age(); 3673 if (!(this.timing instanceof Age)) 3674 throw new FHIRException( 3675 "Type mismatch: the type Age was expected, but " + this.timing.getClass().getName() + " was encountered"); 3676 return (Age) this.timing; 3677 } 3678 3679 public boolean hasTimingAge() { 3680 return this != null && this.timing instanceof Age; 3681 } 3682 3683 /** 3684 * @return {@link #timing} (An optional value describing when the action should 3685 * be performed.) 3686 */ 3687 public Period getTimingPeriod() throws FHIRException { 3688 if (this.timing == null) 3689 this.timing = new Period(); 3690 if (!(this.timing instanceof Period)) 3691 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() 3692 + " was encountered"); 3693 return (Period) this.timing; 3694 } 3695 3696 public boolean hasTimingPeriod() { 3697 return this != null && this.timing instanceof Period; 3698 } 3699 3700 /** 3701 * @return {@link #timing} (An optional value describing when the action should 3702 * be performed.) 3703 */ 3704 public Duration getTimingDuration() throws FHIRException { 3705 if (this.timing == null) 3706 this.timing = new Duration(); 3707 if (!(this.timing instanceof Duration)) 3708 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.timing.getClass().getName() 3709 + " was encountered"); 3710 return (Duration) this.timing; 3711 } 3712 3713 public boolean hasTimingDuration() { 3714 return this != null && this.timing instanceof Duration; 3715 } 3716 3717 /** 3718 * @return {@link #timing} (An optional value describing when the action should 3719 * be performed.) 3720 */ 3721 public Range getTimingRange() throws FHIRException { 3722 if (this.timing == null) 3723 this.timing = new Range(); 3724 if (!(this.timing instanceof Range)) 3725 throw new FHIRException( 3726 "Type mismatch: the type Range was expected, but " + this.timing.getClass().getName() + " was encountered"); 3727 return (Range) this.timing; 3728 } 3729 3730 public boolean hasTimingRange() { 3731 return this != null && this.timing instanceof Range; 3732 } 3733 3734 /** 3735 * @return {@link #timing} (An optional value describing when the action should 3736 * be performed.) 3737 */ 3738 public Timing getTimingTiming() throws FHIRException { 3739 if (this.timing == null) 3740 this.timing = new Timing(); 3741 if (!(this.timing instanceof Timing)) 3742 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() 3743 + " was encountered"); 3744 return (Timing) this.timing; 3745 } 3746 3747 public boolean hasTimingTiming() { 3748 return this != null && this.timing instanceof Timing; 3749 } 3750 3751 public boolean hasTiming() { 3752 return this.timing != null && !this.timing.isEmpty(); 3753 } 3754 3755 /** 3756 * @param value {@link #timing} (An optional value describing when the action 3757 * should be performed.) 3758 */ 3759 public PlanDefinitionActionComponent setTiming(Type value) { 3760 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 3761 || value instanceof Duration || value instanceof Range || value instanceof Timing)) 3762 throw new Error("Not the right type for PlanDefinition.action.timing[x]: " + value.fhirType()); 3763 this.timing = value; 3764 return this; 3765 } 3766 3767 /** 3768 * @return {@link #participant} (Indicates who should participate in performing 3769 * the action described.) 3770 */ 3771 public List<PlanDefinitionActionParticipantComponent> getParticipant() { 3772 if (this.participant == null) 3773 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3774 return this.participant; 3775 } 3776 3777 /** 3778 * @return Returns a reference to <code>this</code> for easy method chaining 3779 */ 3780 public PlanDefinitionActionComponent setParticipant(List<PlanDefinitionActionParticipantComponent> theParticipant) { 3781 this.participant = theParticipant; 3782 return this; 3783 } 3784 3785 public boolean hasParticipant() { 3786 if (this.participant == null) 3787 return false; 3788 for (PlanDefinitionActionParticipantComponent item : this.participant) 3789 if (!item.isEmpty()) 3790 return true; 3791 return false; 3792 } 3793 3794 public PlanDefinitionActionParticipantComponent addParticipant() { // 3 3795 PlanDefinitionActionParticipantComponent t = new PlanDefinitionActionParticipantComponent(); 3796 if (this.participant == null) 3797 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3798 this.participant.add(t); 3799 return t; 3800 } 3801 3802 public PlanDefinitionActionComponent addParticipant(PlanDefinitionActionParticipantComponent t) { // 3 3803 if (t == null) 3804 return this; 3805 if (this.participant == null) 3806 this.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 3807 this.participant.add(t); 3808 return this; 3809 } 3810 3811 /** 3812 * @return The first repetition of repeating field {@link #participant}, 3813 * creating it if it does not already exist 3814 */ 3815 public PlanDefinitionActionParticipantComponent getParticipantFirstRep() { 3816 if (getParticipant().isEmpty()) { 3817 addParticipant(); 3818 } 3819 return getParticipant().get(0); 3820 } 3821 3822 /** 3823 * @return {@link #type} (The type of action to perform (create, update, 3824 * remove).) 3825 */ 3826 public CodeableConcept getType() { 3827 if (this.type == null) 3828 if (Configuration.errorOnAutoCreate()) 3829 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.type"); 3830 else if (Configuration.doAutoCreate()) 3831 this.type = new CodeableConcept(); // cc 3832 return this.type; 3833 } 3834 3835 public boolean hasType() { 3836 return this.type != null && !this.type.isEmpty(); 3837 } 3838 3839 /** 3840 * @param value {@link #type} (The type of action to perform (create, update, 3841 * remove).) 3842 */ 3843 public PlanDefinitionActionComponent setType(CodeableConcept value) { 3844 this.type = value; 3845 return this; 3846 } 3847 3848 /** 3849 * @return {@link #groupingBehavior} (Defines the grouping behavior for the 3850 * action and its children.). This is the underlying object with id, 3851 * value and extensions. The accessor "getGroupingBehavior" gives direct 3852 * access to the value 3853 */ 3854 public Enumeration<ActionGroupingBehavior> getGroupingBehaviorElement() { 3855 if (this.groupingBehavior == null) 3856 if (Configuration.errorOnAutoCreate()) 3857 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.groupingBehavior"); 3858 else if (Configuration.doAutoCreate()) 3859 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); // bb 3860 return this.groupingBehavior; 3861 } 3862 3863 public boolean hasGroupingBehaviorElement() { 3864 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 3865 } 3866 3867 public boolean hasGroupingBehavior() { 3868 return this.groupingBehavior != null && !this.groupingBehavior.isEmpty(); 3869 } 3870 3871 /** 3872 * @param value {@link #groupingBehavior} (Defines the grouping behavior for the 3873 * action and its children.). This is the underlying object with 3874 * id, value and extensions. The accessor "getGroupingBehavior" 3875 * gives direct access to the value 3876 */ 3877 public PlanDefinitionActionComponent setGroupingBehaviorElement(Enumeration<ActionGroupingBehavior> value) { 3878 this.groupingBehavior = value; 3879 return this; 3880 } 3881 3882 /** 3883 * @return Defines the grouping behavior for the action and its children. 3884 */ 3885 public ActionGroupingBehavior getGroupingBehavior() { 3886 return this.groupingBehavior == null ? null : this.groupingBehavior.getValue(); 3887 } 3888 3889 /** 3890 * @param value Defines the grouping behavior for the action and its children. 3891 */ 3892 public PlanDefinitionActionComponent setGroupingBehavior(ActionGroupingBehavior value) { 3893 if (value == null) 3894 this.groupingBehavior = null; 3895 else { 3896 if (this.groupingBehavior == null) 3897 this.groupingBehavior = new Enumeration<ActionGroupingBehavior>(new ActionGroupingBehaviorEnumFactory()); 3898 this.groupingBehavior.setValue(value); 3899 } 3900 return this; 3901 } 3902 3903 /** 3904 * @return {@link #selectionBehavior} (Defines the selection behavior for the 3905 * action and its children.). This is the underlying object with id, 3906 * value and extensions. The accessor "getSelectionBehavior" gives 3907 * direct access to the value 3908 */ 3909 public Enumeration<ActionSelectionBehavior> getSelectionBehaviorElement() { 3910 if (this.selectionBehavior == null) 3911 if (Configuration.errorOnAutoCreate()) 3912 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.selectionBehavior"); 3913 else if (Configuration.doAutoCreate()) 3914 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); // bb 3915 return this.selectionBehavior; 3916 } 3917 3918 public boolean hasSelectionBehaviorElement() { 3919 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3920 } 3921 3922 public boolean hasSelectionBehavior() { 3923 return this.selectionBehavior != null && !this.selectionBehavior.isEmpty(); 3924 } 3925 3926 /** 3927 * @param value {@link #selectionBehavior} (Defines the selection behavior for 3928 * the action and its children.). This is the underlying object 3929 * with id, value and extensions. The accessor 3930 * "getSelectionBehavior" gives direct access to the value 3931 */ 3932 public PlanDefinitionActionComponent setSelectionBehaviorElement(Enumeration<ActionSelectionBehavior> value) { 3933 this.selectionBehavior = value; 3934 return this; 3935 } 3936 3937 /** 3938 * @return Defines the selection behavior for the action and its children. 3939 */ 3940 public ActionSelectionBehavior getSelectionBehavior() { 3941 return this.selectionBehavior == null ? null : this.selectionBehavior.getValue(); 3942 } 3943 3944 /** 3945 * @param value Defines the selection behavior for the action and its children. 3946 */ 3947 public PlanDefinitionActionComponent setSelectionBehavior(ActionSelectionBehavior value) { 3948 if (value == null) 3949 this.selectionBehavior = null; 3950 else { 3951 if (this.selectionBehavior == null) 3952 this.selectionBehavior = new Enumeration<ActionSelectionBehavior>(new ActionSelectionBehaviorEnumFactory()); 3953 this.selectionBehavior.setValue(value); 3954 } 3955 return this; 3956 } 3957 3958 /** 3959 * @return {@link #requiredBehavior} (Defines the required behavior for the 3960 * action.). This is the underlying object with id, value and 3961 * extensions. The accessor "getRequiredBehavior" gives direct access to 3962 * the value 3963 */ 3964 public Enumeration<ActionRequiredBehavior> getRequiredBehaviorElement() { 3965 if (this.requiredBehavior == null) 3966 if (Configuration.errorOnAutoCreate()) 3967 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.requiredBehavior"); 3968 else if (Configuration.doAutoCreate()) 3969 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); // bb 3970 return this.requiredBehavior; 3971 } 3972 3973 public boolean hasRequiredBehaviorElement() { 3974 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 3975 } 3976 3977 public boolean hasRequiredBehavior() { 3978 return this.requiredBehavior != null && !this.requiredBehavior.isEmpty(); 3979 } 3980 3981 /** 3982 * @param value {@link #requiredBehavior} (Defines the required behavior for the 3983 * action.). This is the underlying object with id, value and 3984 * extensions. The accessor "getRequiredBehavior" gives direct 3985 * access to the value 3986 */ 3987 public PlanDefinitionActionComponent setRequiredBehaviorElement(Enumeration<ActionRequiredBehavior> value) { 3988 this.requiredBehavior = value; 3989 return this; 3990 } 3991 3992 /** 3993 * @return Defines the required behavior for the action. 3994 */ 3995 public ActionRequiredBehavior getRequiredBehavior() { 3996 return this.requiredBehavior == null ? null : this.requiredBehavior.getValue(); 3997 } 3998 3999 /** 4000 * @param value Defines the required behavior for the action. 4001 */ 4002 public PlanDefinitionActionComponent setRequiredBehavior(ActionRequiredBehavior value) { 4003 if (value == null) 4004 this.requiredBehavior = null; 4005 else { 4006 if (this.requiredBehavior == null) 4007 this.requiredBehavior = new Enumeration<ActionRequiredBehavior>(new ActionRequiredBehaviorEnumFactory()); 4008 this.requiredBehavior.setValue(value); 4009 } 4010 return this; 4011 } 4012 4013 /** 4014 * @return {@link #precheckBehavior} (Defines whether the action should usually 4015 * be preselected.). This is the underlying object with id, value and 4016 * extensions. The accessor "getPrecheckBehavior" gives direct access to 4017 * the value 4018 */ 4019 public Enumeration<ActionPrecheckBehavior> getPrecheckBehaviorElement() { 4020 if (this.precheckBehavior == null) 4021 if (Configuration.errorOnAutoCreate()) 4022 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.precheckBehavior"); 4023 else if (Configuration.doAutoCreate()) 4024 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); // bb 4025 return this.precheckBehavior; 4026 } 4027 4028 public boolean hasPrecheckBehaviorElement() { 4029 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 4030 } 4031 4032 public boolean hasPrecheckBehavior() { 4033 return this.precheckBehavior != null && !this.precheckBehavior.isEmpty(); 4034 } 4035 4036 /** 4037 * @param value {@link #precheckBehavior} (Defines whether the action should 4038 * usually be preselected.). This is the underlying object with id, 4039 * value and extensions. The accessor "getPrecheckBehavior" gives 4040 * direct access to the value 4041 */ 4042 public PlanDefinitionActionComponent setPrecheckBehaviorElement(Enumeration<ActionPrecheckBehavior> value) { 4043 this.precheckBehavior = value; 4044 return this; 4045 } 4046 4047 /** 4048 * @return Defines whether the action should usually be preselected. 4049 */ 4050 public ActionPrecheckBehavior getPrecheckBehavior() { 4051 return this.precheckBehavior == null ? null : this.precheckBehavior.getValue(); 4052 } 4053 4054 /** 4055 * @param value Defines whether the action should usually be preselected. 4056 */ 4057 public PlanDefinitionActionComponent setPrecheckBehavior(ActionPrecheckBehavior value) { 4058 if (value == null) 4059 this.precheckBehavior = null; 4060 else { 4061 if (this.precheckBehavior == null) 4062 this.precheckBehavior = new Enumeration<ActionPrecheckBehavior>(new ActionPrecheckBehaviorEnumFactory()); 4063 this.precheckBehavior.setValue(value); 4064 } 4065 return this; 4066 } 4067 4068 /** 4069 * @return {@link #cardinalityBehavior} (Defines whether the action can be 4070 * selected multiple times.). This is the underlying object with id, 4071 * value and extensions. The accessor "getCardinalityBehavior" gives 4072 * direct access to the value 4073 */ 4074 public Enumeration<ActionCardinalityBehavior> getCardinalityBehaviorElement() { 4075 if (this.cardinalityBehavior == null) 4076 if (Configuration.errorOnAutoCreate()) 4077 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.cardinalityBehavior"); 4078 else if (Configuration.doAutoCreate()) 4079 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 4080 new ActionCardinalityBehaviorEnumFactory()); // bb 4081 return this.cardinalityBehavior; 4082 } 4083 4084 public boolean hasCardinalityBehaviorElement() { 4085 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 4086 } 4087 4088 public boolean hasCardinalityBehavior() { 4089 return this.cardinalityBehavior != null && !this.cardinalityBehavior.isEmpty(); 4090 } 4091 4092 /** 4093 * @param value {@link #cardinalityBehavior} (Defines whether the action can be 4094 * selected multiple times.). This is the underlying object with 4095 * id, value and extensions. The accessor "getCardinalityBehavior" 4096 * gives direct access to the value 4097 */ 4098 public PlanDefinitionActionComponent setCardinalityBehaviorElement(Enumeration<ActionCardinalityBehavior> value) { 4099 this.cardinalityBehavior = value; 4100 return this; 4101 } 4102 4103 /** 4104 * @return Defines whether the action can be selected multiple times. 4105 */ 4106 public ActionCardinalityBehavior getCardinalityBehavior() { 4107 return this.cardinalityBehavior == null ? null : this.cardinalityBehavior.getValue(); 4108 } 4109 4110 /** 4111 * @param value Defines whether the action can be selected multiple times. 4112 */ 4113 public PlanDefinitionActionComponent setCardinalityBehavior(ActionCardinalityBehavior value) { 4114 if (value == null) 4115 this.cardinalityBehavior = null; 4116 else { 4117 if (this.cardinalityBehavior == null) 4118 this.cardinalityBehavior = new Enumeration<ActionCardinalityBehavior>( 4119 new ActionCardinalityBehaviorEnumFactory()); 4120 this.cardinalityBehavior.setValue(value); 4121 } 4122 return this; 4123 } 4124 4125 /** 4126 * @return {@link #definition} (A reference to an ActivityDefinition that 4127 * describes the action to be taken in detail, or a PlanDefinition that 4128 * describes a series of actions to be taken.) 4129 */ 4130 public Type getDefinition() { 4131 return this.definition; 4132 } 4133 4134 /** 4135 * @return {@link #definition} (A reference to an ActivityDefinition that 4136 * describes the action to be taken in detail, or a PlanDefinition that 4137 * describes a series of actions to be taken.) 4138 */ 4139 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 4140 if (this.definition == null) 4141 this.definition = new CanonicalType(); 4142 if (!(this.definition instanceof CanonicalType)) 4143 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 4144 + this.definition.getClass().getName() + " was encountered"); 4145 return (CanonicalType) this.definition; 4146 } 4147 4148 public boolean hasDefinitionCanonicalType() { 4149 return this != null && this.definition instanceof CanonicalType; 4150 } 4151 4152 /** 4153 * @return {@link #definition} (A reference to an ActivityDefinition that 4154 * describes the action to be taken in detail, or a PlanDefinition that 4155 * describes a series of actions to be taken.) 4156 */ 4157 public UriType getDefinitionUriType() throws FHIRException { 4158 if (this.definition == null) 4159 this.definition = new UriType(); 4160 if (!(this.definition instanceof UriType)) 4161 throw new FHIRException("Type mismatch: the type UriType was expected, but " 4162 + this.definition.getClass().getName() + " was encountered"); 4163 return (UriType) this.definition; 4164 } 4165 4166 public boolean hasDefinitionUriType() { 4167 return this != null && this.definition instanceof UriType; 4168 } 4169 4170 public boolean hasDefinition() { 4171 return this.definition != null && !this.definition.isEmpty(); 4172 } 4173 4174 /** 4175 * @param value {@link #definition} (A reference to an ActivityDefinition that 4176 * describes the action to be taken in detail, or a PlanDefinition 4177 * that describes a series of actions to be taken.) 4178 */ 4179 public PlanDefinitionActionComponent setDefinition(Type value) { 4180 if (value != null && !(value instanceof CanonicalType || value instanceof UriType)) 4181 throw new Error("Not the right type for PlanDefinition.action.definition[x]: " + value.fhirType()); 4182 this.definition = value; 4183 return this; 4184 } 4185 4186 /** 4187 * @return {@link #transform} (A reference to a StructureMap resource that 4188 * defines a transform that can be executed to produce the intent 4189 * resource using the ActivityDefinition instance as the input.). This 4190 * is the underlying object with id, value and extensions. The accessor 4191 * "getTransform" gives direct access to the value 4192 */ 4193 public CanonicalType getTransformElement() { 4194 if (this.transform == null) 4195 if (Configuration.errorOnAutoCreate()) 4196 throw new Error("Attempt to auto-create PlanDefinitionActionComponent.transform"); 4197 else if (Configuration.doAutoCreate()) 4198 this.transform = new CanonicalType(); // bb 4199 return this.transform; 4200 } 4201 4202 public boolean hasTransformElement() { 4203 return this.transform != null && !this.transform.isEmpty(); 4204 } 4205 4206 public boolean hasTransform() { 4207 return this.transform != null && !this.transform.isEmpty(); 4208 } 4209 4210 /** 4211 * @param value {@link #transform} (A reference to a StructureMap resource that 4212 * defines a transform that can be executed to produce the intent 4213 * resource using the ActivityDefinition instance as the input.). 4214 * This is the underlying object with id, value and extensions. The 4215 * accessor "getTransform" gives direct access to the value 4216 */ 4217 public PlanDefinitionActionComponent setTransformElement(CanonicalType value) { 4218 this.transform = value; 4219 return this; 4220 } 4221 4222 /** 4223 * @return A reference to a StructureMap resource that defines a transform that 4224 * can be executed to produce the intent resource using the 4225 * ActivityDefinition instance as the input. 4226 */ 4227 public String getTransform() { 4228 return this.transform == null ? null : this.transform.getValue(); 4229 } 4230 4231 /** 4232 * @param value A reference to a StructureMap resource that defines a transform 4233 * that can be executed to produce the intent resource using the 4234 * ActivityDefinition instance as the input. 4235 */ 4236 public PlanDefinitionActionComponent setTransform(String value) { 4237 if (Utilities.noString(value)) 4238 this.transform = null; 4239 else { 4240 if (this.transform == null) 4241 this.transform = new CanonicalType(); 4242 this.transform.setValue(value); 4243 } 4244 return this; 4245 } 4246 4247 /** 4248 * @return {@link #dynamicValue} (Customizations that should be applied to the 4249 * statically defined resource. For example, if the dosage of a 4250 * medication must be computed based on the patient's weight, a 4251 * customization would be used to specify an expression that calculated 4252 * the weight, and the path on the resource that would contain the 4253 * result.) 4254 */ 4255 public List<PlanDefinitionActionDynamicValueComponent> getDynamicValue() { 4256 if (this.dynamicValue == null) 4257 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4258 return this.dynamicValue; 4259 } 4260 4261 /** 4262 * @return Returns a reference to <code>this</code> for easy method chaining 4263 */ 4264 public PlanDefinitionActionComponent setDynamicValue( 4265 List<PlanDefinitionActionDynamicValueComponent> theDynamicValue) { 4266 this.dynamicValue = theDynamicValue; 4267 return this; 4268 } 4269 4270 public boolean hasDynamicValue() { 4271 if (this.dynamicValue == null) 4272 return false; 4273 for (PlanDefinitionActionDynamicValueComponent item : this.dynamicValue) 4274 if (!item.isEmpty()) 4275 return true; 4276 return false; 4277 } 4278 4279 public PlanDefinitionActionDynamicValueComponent addDynamicValue() { // 3 4280 PlanDefinitionActionDynamicValueComponent t = new PlanDefinitionActionDynamicValueComponent(); 4281 if (this.dynamicValue == null) 4282 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4283 this.dynamicValue.add(t); 4284 return t; 4285 } 4286 4287 public PlanDefinitionActionComponent addDynamicValue(PlanDefinitionActionDynamicValueComponent t) { // 3 4288 if (t == null) 4289 return this; 4290 if (this.dynamicValue == null) 4291 this.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 4292 this.dynamicValue.add(t); 4293 return this; 4294 } 4295 4296 /** 4297 * @return The first repetition of repeating field {@link #dynamicValue}, 4298 * creating it if it does not already exist 4299 */ 4300 public PlanDefinitionActionDynamicValueComponent getDynamicValueFirstRep() { 4301 if (getDynamicValue().isEmpty()) { 4302 addDynamicValue(); 4303 } 4304 return getDynamicValue().get(0); 4305 } 4306 4307 /** 4308 * @return {@link #action} (Sub actions that are contained within the action. 4309 * The behavior of this action determines the functionality of the 4310 * sub-actions. For example, a selection behavior of at-most-one 4311 * indicates that of the sub-actions, at most one may be chosen as part 4312 * of realizing the action definition.) 4313 */ 4314 public List<PlanDefinitionActionComponent> getAction() { 4315 if (this.action == null) 4316 this.action = new ArrayList<PlanDefinitionActionComponent>(); 4317 return this.action; 4318 } 4319 4320 /** 4321 * @return Returns a reference to <code>this</code> for easy method chaining 4322 */ 4323 public PlanDefinitionActionComponent setAction(List<PlanDefinitionActionComponent> theAction) { 4324 this.action = theAction; 4325 return this; 4326 } 4327 4328 public boolean hasAction() { 4329 if (this.action == null) 4330 return false; 4331 for (PlanDefinitionActionComponent item : this.action) 4332 if (!item.isEmpty()) 4333 return true; 4334 return false; 4335 } 4336 4337 public PlanDefinitionActionComponent addAction() { // 3 4338 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 4339 if (this.action == null) 4340 this.action = new ArrayList<PlanDefinitionActionComponent>(); 4341 this.action.add(t); 4342 return t; 4343 } 4344 4345 public PlanDefinitionActionComponent addAction(PlanDefinitionActionComponent t) { // 3 4346 if (t == null) 4347 return this; 4348 if (this.action == null) 4349 this.action = new ArrayList<PlanDefinitionActionComponent>(); 4350 this.action.add(t); 4351 return this; 4352 } 4353 4354 /** 4355 * @return The first repetition of repeating field {@link #action}, creating it 4356 * if it does not already exist 4357 */ 4358 public PlanDefinitionActionComponent getActionFirstRep() { 4359 if (getAction().isEmpty()) { 4360 addAction(); 4361 } 4362 return getAction().get(0); 4363 } 4364 4365 protected void listChildren(List<Property> children) { 4366 super.listChildren(children); 4367 children.add(new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix)); 4368 children.add(new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title)); 4369 children.add(new Property("description", "string", 4370 "A brief description of the action used to provide a summary to display to the user.", 0, 1, description)); 4371 children.add(new Property("textEquivalent", "string", 4372 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 4373 0, 1, textEquivalent)); 4374 children.add(new Property("priority", "code", 4375 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority)); 4376 children.add(new Property("code", "CodeableConcept", 4377 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for the section of a documentation template.", 4378 0, java.lang.Integer.MAX_VALUE, code)); 4379 children.add(new Property("reason", "CodeableConcept", 4380 "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason)); 4381 children.add(new Property("documentation", "RelatedArtifact", 4382 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 4383 0, java.lang.Integer.MAX_VALUE, documentation)); 4384 children.add(new Property("goalId", "id", 4385 "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.", 4386 0, java.lang.Integer.MAX_VALUE, goalId)); 4387 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 4388 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4389 1, subject)); 4390 children.add(new Property("trigger", "TriggerDefinition", "A description of when the action should be triggered.", 4391 0, java.lang.Integer.MAX_VALUE, trigger)); 4392 children.add(new Property("condition", "", 4393 "An expression that describes applicability criteria or start/stop conditions for the action.", 0, 4394 java.lang.Integer.MAX_VALUE, condition)); 4395 children.add(new Property("input", "DataRequirement", "Defines input data requirements for the action.", 0, 4396 java.lang.Integer.MAX_VALUE, input)); 4397 children.add(new Property("output", "DataRequirement", "Defines the outputs of the action, if any.", 0, 4398 java.lang.Integer.MAX_VALUE, output)); 4399 children.add(new Property("relatedAction", "", 4400 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 4401 java.lang.Integer.MAX_VALUE, relatedAction)); 4402 children.add(new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4403 "An optional value describing when the action should be performed.", 0, 1, timing)); 4404 children 4405 .add(new Property("participant", "", "Indicates who should participate in performing the action described.", 4406 0, java.lang.Integer.MAX_VALUE, participant)); 4407 children.add(new Property("type", "CodeableConcept", "The type of action to perform (create, update, remove).", 0, 4408 1, type)); 4409 children.add(new Property("groupingBehavior", "code", 4410 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior)); 4411 children.add(new Property("selectionBehavior", "code", 4412 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior)); 4413 children.add(new Property("requiredBehavior", "code", "Defines the required behavior for the action.", 0, 1, 4414 requiredBehavior)); 4415 children.add(new Property("precheckBehavior", "code", "Defines whether the action should usually be preselected.", 4416 0, 1, precheckBehavior)); 4417 children.add(new Property("cardinalityBehavior", "code", 4418 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior)); 4419 children.add(new Property("definition[x]", "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4420 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4421 0, 1, definition)); 4422 children.add(new Property("transform", "canonical(StructureMap)", 4423 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 4424 0, 1, transform)); 4425 children.add(new Property("dynamicValue", "", 4426 "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 4427 0, java.lang.Integer.MAX_VALUE, dynamicValue)); 4428 children.add(new Property("action", "@PlanDefinition.action", 4429 "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 4430 0, java.lang.Integer.MAX_VALUE, action)); 4431 } 4432 4433 @Override 4434 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4435 switch (_hash) { 4436 case -980110702: 4437 /* prefix */ return new Property("prefix", "string", "A user-visible prefix for the action.", 0, 1, prefix); 4438 case 110371416: 4439 /* title */ return new Property("title", "string", "The title of the action displayed to a user.", 0, 1, title); 4440 case -1724546052: 4441 /* description */ return new Property("description", "string", 4442 "A brief description of the action used to provide a summary to display to the user.", 0, 1, description); 4443 case -900391049: 4444 /* textEquivalent */ return new Property("textEquivalent", "string", 4445 "A text equivalent of the action to be performed. This provides a human-interpretable description of the action when the definition is consumed by a system that might not be capable of interpreting it dynamically.", 4446 0, 1, textEquivalent); 4447 case -1165461084: 4448 /* priority */ return new Property("priority", "code", 4449 "Indicates how quickly the action should be addressed with respect to other actions.", 0, 1, priority); 4450 case 3059181: 4451 /* code */ return new Property("code", "CodeableConcept", 4452 "A code that provides meaning for the action or action group. For example, a section may have a LOINC code for the section of a documentation template.", 4453 0, java.lang.Integer.MAX_VALUE, code); 4454 case -934964668: 4455 /* reason */ return new Property("reason", "CodeableConcept", 4456 "A description of why this action is necessary or appropriate.", 0, java.lang.Integer.MAX_VALUE, reason); 4457 case 1587405498: 4458 /* documentation */ return new Property("documentation", "RelatedArtifact", 4459 "Didactic or other informational resources associated with the action that can be provided to the CDS recipient. Information resources can include inline text commentary and links to web resources.", 4460 0, java.lang.Integer.MAX_VALUE, documentation); 4461 case -1240658034: 4462 /* goalId */ return new Property("goalId", "id", 4463 "Identifies goals that this action supports. The reference must be to a goal element defined within this plan definition.", 4464 0, java.lang.Integer.MAX_VALUE, goalId); 4465 case -573640748: 4466 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4467 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4468 1, subject); 4469 case -1867885268: 4470 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4471 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4472 1, subject); 4473 case -1257122603: 4474 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4475 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4476 1, subject); 4477 case 772938623: 4478 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 4479 "A code or group definition that describes the intended subject of the action and its children, if any.", 0, 4480 1, subject); 4481 case -1059891784: 4482 /* trigger */ return new Property("trigger", "TriggerDefinition", 4483 "A description of when the action should be triggered.", 0, java.lang.Integer.MAX_VALUE, trigger); 4484 case -861311717: 4485 /* condition */ return new Property("condition", "", 4486 "An expression that describes applicability criteria or start/stop conditions for the action.", 0, 4487 java.lang.Integer.MAX_VALUE, condition); 4488 case 100358090: 4489 /* input */ return new Property("input", "DataRequirement", "Defines input data requirements for the action.", 4490 0, java.lang.Integer.MAX_VALUE, input); 4491 case -1005512447: 4492 /* output */ return new Property("output", "DataRequirement", "Defines the outputs of the action, if any.", 0, 4493 java.lang.Integer.MAX_VALUE, output); 4494 case -384107967: 4495 /* relatedAction */ return new Property("relatedAction", "", 4496 "A relationship to another action such as \"before\" or \"30-60 minutes after start of\".", 0, 4497 java.lang.Integer.MAX_VALUE, relatedAction); 4498 case 164632566: 4499 /* timing[x] */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4500 "An optional value describing when the action should be performed.", 0, 1, timing); 4501 case -873664438: 4502 /* timing */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4503 "An optional value describing when the action should be performed.", 0, 1, timing); 4504 case -1837458939: 4505 /* timingDateTime */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4506 "An optional value describing when the action should be performed.", 0, 1, timing); 4507 case 164607061: 4508 /* timingAge */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4509 "An optional value describing when the action should be performed.", 0, 1, timing); 4510 case -615615829: 4511 /* timingPeriod */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4512 "An optional value describing when the action should be performed.", 0, 1, timing); 4513 case -1327253506: 4514 /* timingDuration */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4515 "An optional value describing when the action should be performed.", 0, 1, timing); 4516 case -710871277: 4517 /* timingRange */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4518 "An optional value describing when the action should be performed.", 0, 1, timing); 4519 case -497554124: 4520 /* timingTiming */ return new Property("timing[x]", "dateTime|Age|Period|Duration|Range|Timing", 4521 "An optional value describing when the action should be performed.", 0, 1, timing); 4522 case 767422259: 4523 /* participant */ return new Property("participant", "", 4524 "Indicates who should participate in performing the action described.", 0, java.lang.Integer.MAX_VALUE, 4525 participant); 4526 case 3575610: 4527 /* type */ return new Property("type", "CodeableConcept", 4528 "The type of action to perform (create, update, remove).", 0, 1, type); 4529 case 586678389: 4530 /* groupingBehavior */ return new Property("groupingBehavior", "code", 4531 "Defines the grouping behavior for the action and its children.", 0, 1, groupingBehavior); 4532 case 168639486: 4533 /* selectionBehavior */ return new Property("selectionBehavior", "code", 4534 "Defines the selection behavior for the action and its children.", 0, 1, selectionBehavior); 4535 case -1163906287: 4536 /* requiredBehavior */ return new Property("requiredBehavior", "code", 4537 "Defines the required behavior for the action.", 0, 1, requiredBehavior); 4538 case -1174249033: 4539 /* precheckBehavior */ return new Property("precheckBehavior", "code", 4540 "Defines whether the action should usually be preselected.", 0, 1, precheckBehavior); 4541 case -922577408: 4542 /* cardinalityBehavior */ return new Property("cardinalityBehavior", "code", 4543 "Defines whether the action can be selected multiple times.", 0, 1, cardinalityBehavior); 4544 case -1139422643: 4545 /* definition[x] */ return new Property("definition[x]", 4546 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4547 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4548 0, 1, definition); 4549 case -1014418093: 4550 /* definition */ return new Property("definition[x]", 4551 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4552 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4553 0, 1, definition); 4554 case 933485793: 4555 /* definitionCanonical */ return new Property("definition[x]", 4556 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4557 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4558 0, 1, definition); 4559 case -1139428583: 4560 /* definitionUri */ return new Property("definition[x]", 4561 "canonical(ActivityDefinition|PlanDefinition|Questionnaire)|uri", 4562 "A reference to an ActivityDefinition that describes the action to be taken in detail, or a PlanDefinition that describes a series of actions to be taken.", 4563 0, 1, definition); 4564 case 1052666732: 4565 /* transform */ return new Property("transform", "canonical(StructureMap)", 4566 "A reference to a StructureMap resource that defines a transform that can be executed to produce the intent resource using the ActivityDefinition instance as the input.", 4567 0, 1, transform); 4568 case 572625010: 4569 /* dynamicValue */ return new Property("dynamicValue", "", 4570 "Customizations that should be applied to the statically defined resource. For example, if the dosage of a medication must be computed based on the patient's weight, a customization would be used to specify an expression that calculated the weight, and the path on the resource that would contain the result.", 4571 0, java.lang.Integer.MAX_VALUE, dynamicValue); 4572 case -1422950858: 4573 /* action */ return new Property("action", "@PlanDefinition.action", 4574 "Sub actions that are contained within the action. The behavior of this action determines the functionality of the sub-actions. For example, a selection behavior of at-most-one indicates that of the sub-actions, at most one may be chosen as part of realizing the action definition.", 4575 0, java.lang.Integer.MAX_VALUE, action); 4576 default: 4577 return super.getNamedProperty(_hash, _name, _checkValid); 4578 } 4579 4580 } 4581 4582 @Override 4583 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4584 switch (hash) { 4585 case -980110702: 4586 /* prefix */ return this.prefix == null ? new Base[0] : new Base[] { this.prefix }; // StringType 4587 case 110371416: 4588 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 4589 case -1724546052: 4590 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 4591 case -900391049: 4592 /* textEquivalent */ return this.textEquivalent == null ? new Base[0] : new Base[] { this.textEquivalent }; // StringType 4593 case -1165461084: 4594 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<RequestPriority> 4595 case 3059181: 4596 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 4597 case -934964668: 4598 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 4599 case 1587405498: 4600 /* documentation */ return this.documentation == null ? new Base[0] 4601 : this.documentation.toArray(new Base[this.documentation.size()]); // RelatedArtifact 4602 case -1240658034: 4603 /* goalId */ return this.goalId == null ? new Base[0] : this.goalId.toArray(new Base[this.goalId.size()]); // IdType 4604 case -1867885268: 4605 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 4606 case -1059891784: 4607 /* trigger */ return this.trigger == null ? new Base[0] : this.trigger.toArray(new Base[this.trigger.size()]); // TriggerDefinition 4608 case -861311717: 4609 /* condition */ return this.condition == null ? new Base[0] 4610 : this.condition.toArray(new Base[this.condition.size()]); // PlanDefinitionActionConditionComponent 4611 case 100358090: 4612 /* input */ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // DataRequirement 4613 case -1005512447: 4614 /* output */ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // DataRequirement 4615 case -384107967: 4616 /* relatedAction */ return this.relatedAction == null ? new Base[0] 4617 : this.relatedAction.toArray(new Base[this.relatedAction.size()]); // PlanDefinitionActionRelatedActionComponent 4618 case -873664438: 4619 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 4620 case 767422259: 4621 /* participant */ return this.participant == null ? new Base[0] 4622 : this.participant.toArray(new Base[this.participant.size()]); // PlanDefinitionActionParticipantComponent 4623 case 3575610: 4624 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 4625 case 586678389: 4626 /* groupingBehavior */ return this.groupingBehavior == null ? new Base[0] 4627 : new Base[] { this.groupingBehavior }; // Enumeration<ActionGroupingBehavior> 4628 case 168639486: 4629 /* selectionBehavior */ return this.selectionBehavior == null ? new Base[0] 4630 : new Base[] { this.selectionBehavior }; // Enumeration<ActionSelectionBehavior> 4631 case -1163906287: 4632 /* requiredBehavior */ return this.requiredBehavior == null ? new Base[0] 4633 : new Base[] { this.requiredBehavior }; // Enumeration<ActionRequiredBehavior> 4634 case -1174249033: 4635 /* precheckBehavior */ return this.precheckBehavior == null ? new Base[0] 4636 : new Base[] { this.precheckBehavior }; // Enumeration<ActionPrecheckBehavior> 4637 case -922577408: 4638 /* cardinalityBehavior */ return this.cardinalityBehavior == null ? new Base[0] 4639 : new Base[] { this.cardinalityBehavior }; // Enumeration<ActionCardinalityBehavior> 4640 case -1014418093: 4641 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Type 4642 case 1052666732: 4643 /* transform */ return this.transform == null ? new Base[0] : new Base[] { this.transform }; // CanonicalType 4644 case 572625010: 4645 /* dynamicValue */ return this.dynamicValue == null ? new Base[0] 4646 : this.dynamicValue.toArray(new Base[this.dynamicValue.size()]); // PlanDefinitionActionDynamicValueComponent 4647 case -1422950858: 4648 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 4649 default: 4650 return super.getProperty(hash, name, checkValid); 4651 } 4652 4653 } 4654 4655 @Override 4656 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4657 switch (hash) { 4658 case -980110702: // prefix 4659 this.prefix = castToString(value); // StringType 4660 return value; 4661 case 110371416: // title 4662 this.title = castToString(value); // StringType 4663 return value; 4664 case -1724546052: // description 4665 this.description = castToString(value); // StringType 4666 return value; 4667 case -900391049: // textEquivalent 4668 this.textEquivalent = castToString(value); // StringType 4669 return value; 4670 case -1165461084: // priority 4671 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4672 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4673 return value; 4674 case 3059181: // code 4675 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 4676 return value; 4677 case -934964668: // reason 4678 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 4679 return value; 4680 case 1587405498: // documentation 4681 this.getDocumentation().add(castToRelatedArtifact(value)); // RelatedArtifact 4682 return value; 4683 case -1240658034: // goalId 4684 this.getGoalId().add(castToId(value)); // IdType 4685 return value; 4686 case -1867885268: // subject 4687 this.subject = castToType(value); // Type 4688 return value; 4689 case -1059891784: // trigger 4690 this.getTrigger().add(castToTriggerDefinition(value)); // TriggerDefinition 4691 return value; 4692 case -861311717: // condition 4693 this.getCondition().add((PlanDefinitionActionConditionComponent) value); // PlanDefinitionActionConditionComponent 4694 return value; 4695 case 100358090: // input 4696 this.getInput().add(castToDataRequirement(value)); // DataRequirement 4697 return value; 4698 case -1005512447: // output 4699 this.getOutput().add(castToDataRequirement(value)); // DataRequirement 4700 return value; 4701 case -384107967: // relatedAction 4702 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); // PlanDefinitionActionRelatedActionComponent 4703 return value; 4704 case -873664438: // timing 4705 this.timing = castToType(value); // Type 4706 return value; 4707 case 767422259: // participant 4708 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); // PlanDefinitionActionParticipantComponent 4709 return value; 4710 case 3575610: // type 4711 this.type = castToCodeableConcept(value); // CodeableConcept 4712 return value; 4713 case 586678389: // groupingBehavior 4714 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 4715 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 4716 return value; 4717 case 168639486: // selectionBehavior 4718 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 4719 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 4720 return value; 4721 case -1163906287: // requiredBehavior 4722 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 4723 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 4724 return value; 4725 case -1174249033: // precheckBehavior 4726 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 4727 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 4728 return value; 4729 case -922577408: // cardinalityBehavior 4730 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 4731 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 4732 return value; 4733 case -1014418093: // definition 4734 this.definition = castToType(value); // Type 4735 return value; 4736 case 1052666732: // transform 4737 this.transform = castToCanonical(value); // CanonicalType 4738 return value; 4739 case 572625010: // dynamicValue 4740 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); // PlanDefinitionActionDynamicValueComponent 4741 return value; 4742 case -1422950858: // action 4743 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 4744 return value; 4745 default: 4746 return super.setProperty(hash, name, value); 4747 } 4748 4749 } 4750 4751 @Override 4752 public Base setProperty(String name, Base value) throws FHIRException { 4753 if (name.equals("prefix")) { 4754 this.prefix = castToString(value); // StringType 4755 } else if (name.equals("title")) { 4756 this.title = castToString(value); // StringType 4757 } else if (name.equals("description")) { 4758 this.description = castToString(value); // StringType 4759 } else if (name.equals("textEquivalent")) { 4760 this.textEquivalent = castToString(value); // StringType 4761 } else if (name.equals("priority")) { 4762 value = new RequestPriorityEnumFactory().fromType(castToCode(value)); 4763 this.priority = (Enumeration) value; // Enumeration<RequestPriority> 4764 } else if (name.equals("code")) { 4765 this.getCode().add(castToCodeableConcept(value)); 4766 } else if (name.equals("reason")) { 4767 this.getReason().add(castToCodeableConcept(value)); 4768 } else if (name.equals("documentation")) { 4769 this.getDocumentation().add(castToRelatedArtifact(value)); 4770 } else if (name.equals("goalId")) { 4771 this.getGoalId().add(castToId(value)); 4772 } else if (name.equals("subject[x]")) { 4773 this.subject = castToType(value); // Type 4774 } else if (name.equals("trigger")) { 4775 this.getTrigger().add(castToTriggerDefinition(value)); 4776 } else if (name.equals("condition")) { 4777 this.getCondition().add((PlanDefinitionActionConditionComponent) value); 4778 } else if (name.equals("input")) { 4779 this.getInput().add(castToDataRequirement(value)); 4780 } else if (name.equals("output")) { 4781 this.getOutput().add(castToDataRequirement(value)); 4782 } else if (name.equals("relatedAction")) { 4783 this.getRelatedAction().add((PlanDefinitionActionRelatedActionComponent) value); 4784 } else if (name.equals("timing[x]")) { 4785 this.timing = castToType(value); // Type 4786 } else if (name.equals("participant")) { 4787 this.getParticipant().add((PlanDefinitionActionParticipantComponent) value); 4788 } else if (name.equals("type")) { 4789 this.type = castToCodeableConcept(value); // CodeableConcept 4790 } else if (name.equals("groupingBehavior")) { 4791 value = new ActionGroupingBehaviorEnumFactory().fromType(castToCode(value)); 4792 this.groupingBehavior = (Enumeration) value; // Enumeration<ActionGroupingBehavior> 4793 } else if (name.equals("selectionBehavior")) { 4794 value = new ActionSelectionBehaviorEnumFactory().fromType(castToCode(value)); 4795 this.selectionBehavior = (Enumeration) value; // Enumeration<ActionSelectionBehavior> 4796 } else if (name.equals("requiredBehavior")) { 4797 value = new ActionRequiredBehaviorEnumFactory().fromType(castToCode(value)); 4798 this.requiredBehavior = (Enumeration) value; // Enumeration<ActionRequiredBehavior> 4799 } else if (name.equals("precheckBehavior")) { 4800 value = new ActionPrecheckBehaviorEnumFactory().fromType(castToCode(value)); 4801 this.precheckBehavior = (Enumeration) value; // Enumeration<ActionPrecheckBehavior> 4802 } else if (name.equals("cardinalityBehavior")) { 4803 value = new ActionCardinalityBehaviorEnumFactory().fromType(castToCode(value)); 4804 this.cardinalityBehavior = (Enumeration) value; // Enumeration<ActionCardinalityBehavior> 4805 } else if (name.equals("definition[x]")) { 4806 this.definition = castToType(value); // Type 4807 } else if (name.equals("transform")) { 4808 this.transform = castToCanonical(value); // CanonicalType 4809 } else if (name.equals("dynamicValue")) { 4810 this.getDynamicValue().add((PlanDefinitionActionDynamicValueComponent) value); 4811 } else if (name.equals("action")) { 4812 this.getAction().add((PlanDefinitionActionComponent) value); 4813 } else 4814 return super.setProperty(name, value); 4815 return value; 4816 } 4817 4818 @Override 4819 public Base makeProperty(int hash, String name) throws FHIRException { 4820 switch (hash) { 4821 case -980110702: 4822 return getPrefixElement(); 4823 case 110371416: 4824 return getTitleElement(); 4825 case -1724546052: 4826 return getDescriptionElement(); 4827 case -900391049: 4828 return getTextEquivalentElement(); 4829 case -1165461084: 4830 return getPriorityElement(); 4831 case 3059181: 4832 return addCode(); 4833 case -934964668: 4834 return addReason(); 4835 case 1587405498: 4836 return addDocumentation(); 4837 case -1240658034: 4838 return addGoalIdElement(); 4839 case -573640748: 4840 return getSubject(); 4841 case -1867885268: 4842 return getSubject(); 4843 case -1059891784: 4844 return addTrigger(); 4845 case -861311717: 4846 return addCondition(); 4847 case 100358090: 4848 return addInput(); 4849 case -1005512447: 4850 return addOutput(); 4851 case -384107967: 4852 return addRelatedAction(); 4853 case 164632566: 4854 return getTiming(); 4855 case -873664438: 4856 return getTiming(); 4857 case 767422259: 4858 return addParticipant(); 4859 case 3575610: 4860 return getType(); 4861 case 586678389: 4862 return getGroupingBehaviorElement(); 4863 case 168639486: 4864 return getSelectionBehaviorElement(); 4865 case -1163906287: 4866 return getRequiredBehaviorElement(); 4867 case -1174249033: 4868 return getPrecheckBehaviorElement(); 4869 case -922577408: 4870 return getCardinalityBehaviorElement(); 4871 case -1139422643: 4872 return getDefinition(); 4873 case -1014418093: 4874 return getDefinition(); 4875 case 1052666732: 4876 return getTransformElement(); 4877 case 572625010: 4878 return addDynamicValue(); 4879 case -1422950858: 4880 return addAction(); 4881 default: 4882 return super.makeProperty(hash, name); 4883 } 4884 4885 } 4886 4887 @Override 4888 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4889 switch (hash) { 4890 case -980110702: 4891 /* prefix */ return new String[] { "string" }; 4892 case 110371416: 4893 /* title */ return new String[] { "string" }; 4894 case -1724546052: 4895 /* description */ return new String[] { "string" }; 4896 case -900391049: 4897 /* textEquivalent */ return new String[] { "string" }; 4898 case -1165461084: 4899 /* priority */ return new String[] { "code" }; 4900 case 3059181: 4901 /* code */ return new String[] { "CodeableConcept" }; 4902 case -934964668: 4903 /* reason */ return new String[] { "CodeableConcept" }; 4904 case 1587405498: 4905 /* documentation */ return new String[] { "RelatedArtifact" }; 4906 case -1240658034: 4907 /* goalId */ return new String[] { "id" }; 4908 case -1867885268: 4909 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 4910 case -1059891784: 4911 /* trigger */ return new String[] { "TriggerDefinition" }; 4912 case -861311717: 4913 /* condition */ return new String[] {}; 4914 case 100358090: 4915 /* input */ return new String[] { "DataRequirement" }; 4916 case -1005512447: 4917 /* output */ return new String[] { "DataRequirement" }; 4918 case -384107967: 4919 /* relatedAction */ return new String[] {}; 4920 case -873664438: 4921 /* timing */ return new String[] { "dateTime", "Age", "Period", "Duration", "Range", "Timing" }; 4922 case 767422259: 4923 /* participant */ return new String[] {}; 4924 case 3575610: 4925 /* type */ return new String[] { "CodeableConcept" }; 4926 case 586678389: 4927 /* groupingBehavior */ return new String[] { "code" }; 4928 case 168639486: 4929 /* selectionBehavior */ return new String[] { "code" }; 4930 case -1163906287: 4931 /* requiredBehavior */ return new String[] { "code" }; 4932 case -1174249033: 4933 /* precheckBehavior */ return new String[] { "code" }; 4934 case -922577408: 4935 /* cardinalityBehavior */ return new String[] { "code" }; 4936 case -1014418093: 4937 /* definition */ return new String[] { "canonical", "uri" }; 4938 case 1052666732: 4939 /* transform */ return new String[] { "canonical" }; 4940 case 572625010: 4941 /* dynamicValue */ return new String[] {}; 4942 case -1422950858: 4943 /* action */ return new String[] { "@PlanDefinition.action" }; 4944 default: 4945 return super.getTypesForProperty(hash, name); 4946 } 4947 4948 } 4949 4950 @Override 4951 public Base addChild(String name) throws FHIRException { 4952 if (name.equals("prefix")) { 4953 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.prefix"); 4954 } else if (name.equals("title")) { 4955 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 4956 } else if (name.equals("description")) { 4957 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 4958 } else if (name.equals("textEquivalent")) { 4959 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.textEquivalent"); 4960 } else if (name.equals("priority")) { 4961 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.priority"); 4962 } else if (name.equals("code")) { 4963 return addCode(); 4964 } else if (name.equals("reason")) { 4965 return addReason(); 4966 } else if (name.equals("documentation")) { 4967 return addDocumentation(); 4968 } else if (name.equals("goalId")) { 4969 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.goalId"); 4970 } else if (name.equals("subjectCodeableConcept")) { 4971 this.subject = new CodeableConcept(); 4972 return this.subject; 4973 } else if (name.equals("subjectReference")) { 4974 this.subject = new Reference(); 4975 return this.subject; 4976 } else if (name.equals("trigger")) { 4977 return addTrigger(); 4978 } else if (name.equals("condition")) { 4979 return addCondition(); 4980 } else if (name.equals("input")) { 4981 return addInput(); 4982 } else if (name.equals("output")) { 4983 return addOutput(); 4984 } else if (name.equals("relatedAction")) { 4985 return addRelatedAction(); 4986 } else if (name.equals("timingDateTime")) { 4987 this.timing = new DateTimeType(); 4988 return this.timing; 4989 } else if (name.equals("timingAge")) { 4990 this.timing = new Age(); 4991 return this.timing; 4992 } else if (name.equals("timingPeriod")) { 4993 this.timing = new Period(); 4994 return this.timing; 4995 } else if (name.equals("timingDuration")) { 4996 this.timing = new Duration(); 4997 return this.timing; 4998 } else if (name.equals("timingRange")) { 4999 this.timing = new Range(); 5000 return this.timing; 5001 } else if (name.equals("timingTiming")) { 5002 this.timing = new Timing(); 5003 return this.timing; 5004 } else if (name.equals("participant")) { 5005 return addParticipant(); 5006 } else if (name.equals("type")) { 5007 this.type = new CodeableConcept(); 5008 return this.type; 5009 } else if (name.equals("groupingBehavior")) { 5010 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.groupingBehavior"); 5011 } else if (name.equals("selectionBehavior")) { 5012 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.selectionBehavior"); 5013 } else if (name.equals("requiredBehavior")) { 5014 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.requiredBehavior"); 5015 } else if (name.equals("precheckBehavior")) { 5016 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.precheckBehavior"); 5017 } else if (name.equals("cardinalityBehavior")) { 5018 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.cardinalityBehavior"); 5019 } else if (name.equals("definitionCanonical")) { 5020 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.definition[x]"); 5021 } else if (name.equals("definitionUri")) { 5022 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.definition[x]"); 5023 } else if (name.equals("transform")) { 5024 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.transform"); 5025 } else if (name.equals("dynamicValue")) { 5026 return addDynamicValue(); 5027 } else if (name.equals("action")) { 5028 return addAction(); 5029 } else 5030 return super.addChild(name); 5031 } 5032 5033 public PlanDefinitionActionComponent copy() { 5034 PlanDefinitionActionComponent dst = new PlanDefinitionActionComponent(); 5035 copyValues(dst); 5036 return dst; 5037 } 5038 5039 public void copyValues(PlanDefinitionActionComponent dst) { 5040 super.copyValues(dst); 5041 dst.prefix = prefix == null ? null : prefix.copy(); 5042 dst.title = title == null ? null : title.copy(); 5043 dst.description = description == null ? null : description.copy(); 5044 dst.textEquivalent = textEquivalent == null ? null : textEquivalent.copy(); 5045 dst.priority = priority == null ? null : priority.copy(); 5046 if (code != null) { 5047 dst.code = new ArrayList<CodeableConcept>(); 5048 for (CodeableConcept i : code) 5049 dst.code.add(i.copy()); 5050 } 5051 ; 5052 if (reason != null) { 5053 dst.reason = new ArrayList<CodeableConcept>(); 5054 for (CodeableConcept i : reason) 5055 dst.reason.add(i.copy()); 5056 } 5057 ; 5058 if (documentation != null) { 5059 dst.documentation = new ArrayList<RelatedArtifact>(); 5060 for (RelatedArtifact i : documentation) 5061 dst.documentation.add(i.copy()); 5062 } 5063 ; 5064 if (goalId != null) { 5065 dst.goalId = new ArrayList<IdType>(); 5066 for (IdType i : goalId) 5067 dst.goalId.add(i.copy()); 5068 } 5069 ; 5070 dst.subject = subject == null ? null : subject.copy(); 5071 if (trigger != null) { 5072 dst.trigger = new ArrayList<TriggerDefinition>(); 5073 for (TriggerDefinition i : trigger) 5074 dst.trigger.add(i.copy()); 5075 } 5076 ; 5077 if (condition != null) { 5078 dst.condition = new ArrayList<PlanDefinitionActionConditionComponent>(); 5079 for (PlanDefinitionActionConditionComponent i : condition) 5080 dst.condition.add(i.copy()); 5081 } 5082 ; 5083 if (input != null) { 5084 dst.input = new ArrayList<DataRequirement>(); 5085 for (DataRequirement i : input) 5086 dst.input.add(i.copy()); 5087 } 5088 ; 5089 if (output != null) { 5090 dst.output = new ArrayList<DataRequirement>(); 5091 for (DataRequirement i : output) 5092 dst.output.add(i.copy()); 5093 } 5094 ; 5095 if (relatedAction != null) { 5096 dst.relatedAction = new ArrayList<PlanDefinitionActionRelatedActionComponent>(); 5097 for (PlanDefinitionActionRelatedActionComponent i : relatedAction) 5098 dst.relatedAction.add(i.copy()); 5099 } 5100 ; 5101 dst.timing = timing == null ? null : timing.copy(); 5102 if (participant != null) { 5103 dst.participant = new ArrayList<PlanDefinitionActionParticipantComponent>(); 5104 for (PlanDefinitionActionParticipantComponent i : participant) 5105 dst.participant.add(i.copy()); 5106 } 5107 ; 5108 dst.type = type == null ? null : type.copy(); 5109 dst.groupingBehavior = groupingBehavior == null ? null : groupingBehavior.copy(); 5110 dst.selectionBehavior = selectionBehavior == null ? null : selectionBehavior.copy(); 5111 dst.requiredBehavior = requiredBehavior == null ? null : requiredBehavior.copy(); 5112 dst.precheckBehavior = precheckBehavior == null ? null : precheckBehavior.copy(); 5113 dst.cardinalityBehavior = cardinalityBehavior == null ? null : cardinalityBehavior.copy(); 5114 dst.definition = definition == null ? null : definition.copy(); 5115 dst.transform = transform == null ? null : transform.copy(); 5116 if (dynamicValue != null) { 5117 dst.dynamicValue = new ArrayList<PlanDefinitionActionDynamicValueComponent>(); 5118 for (PlanDefinitionActionDynamicValueComponent i : dynamicValue) 5119 dst.dynamicValue.add(i.copy()); 5120 } 5121 ; 5122 if (action != null) { 5123 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 5124 for (PlanDefinitionActionComponent i : action) 5125 dst.action.add(i.copy()); 5126 } 5127 ; 5128 } 5129 5130 @Override 5131 public boolean equalsDeep(Base other_) { 5132 if (!super.equalsDeep(other_)) 5133 return false; 5134 if (!(other_ instanceof PlanDefinitionActionComponent)) 5135 return false; 5136 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 5137 return compareDeep(prefix, o.prefix, true) && compareDeep(title, o.title, true) 5138 && compareDeep(description, o.description, true) && compareDeep(textEquivalent, o.textEquivalent, true) 5139 && compareDeep(priority, o.priority, true) && compareDeep(code, o.code, true) 5140 && compareDeep(reason, o.reason, true) && compareDeep(documentation, o.documentation, true) 5141 && compareDeep(goalId, o.goalId, true) && compareDeep(subject, o.subject, true) 5142 && compareDeep(trigger, o.trigger, true) && compareDeep(condition, o.condition, true) 5143 && compareDeep(input, o.input, true) && compareDeep(output, o.output, true) 5144 && compareDeep(relatedAction, o.relatedAction, true) && compareDeep(timing, o.timing, true) 5145 && compareDeep(participant, o.participant, true) && compareDeep(type, o.type, true) 5146 && compareDeep(groupingBehavior, o.groupingBehavior, true) 5147 && compareDeep(selectionBehavior, o.selectionBehavior, true) 5148 && compareDeep(requiredBehavior, o.requiredBehavior, true) 5149 && compareDeep(precheckBehavior, o.precheckBehavior, true) 5150 && compareDeep(cardinalityBehavior, o.cardinalityBehavior, true) 5151 && compareDeep(definition, o.definition, true) && compareDeep(transform, o.transform, true) 5152 && compareDeep(dynamicValue, o.dynamicValue, true) && compareDeep(action, o.action, true); 5153 } 5154 5155 @Override 5156 public boolean equalsShallow(Base other_) { 5157 if (!super.equalsShallow(other_)) 5158 return false; 5159 if (!(other_ instanceof PlanDefinitionActionComponent)) 5160 return false; 5161 PlanDefinitionActionComponent o = (PlanDefinitionActionComponent) other_; 5162 return compareValues(prefix, o.prefix, true) && compareValues(title, o.title, true) 5163 && compareValues(description, o.description, true) && compareValues(textEquivalent, o.textEquivalent, true) 5164 && compareValues(priority, o.priority, true) && compareValues(goalId, o.goalId, true) 5165 && compareValues(groupingBehavior, o.groupingBehavior, true) 5166 && compareValues(selectionBehavior, o.selectionBehavior, true) 5167 && compareValues(requiredBehavior, o.requiredBehavior, true) 5168 && compareValues(precheckBehavior, o.precheckBehavior, true) 5169 && compareValues(cardinalityBehavior, o.cardinalityBehavior, true); 5170 } 5171 5172 public boolean isEmpty() { 5173 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(prefix, title, description, textEquivalent, 5174 priority, code, reason, documentation, goalId, subject, trigger, condition, input, output, relatedAction, 5175 timing, participant, type, groupingBehavior, selectionBehavior, requiredBehavior, precheckBehavior, 5176 cardinalityBehavior, definition, transform, dynamicValue, action); 5177 } 5178 5179 public String fhirType() { 5180 return "PlanDefinition.action"; 5181 5182 } 5183 5184 } 5185 5186 @Block() 5187 public static class PlanDefinitionActionConditionComponent extends BackboneElement implements IBaseBackboneElement { 5188 /** 5189 * The kind of condition. 5190 */ 5191 @Child(name = "kind", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5192 @Description(shortDefinition = "applicability | start | stop", formalDefinition = "The kind of condition.") 5193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-condition-kind") 5194 protected Enumeration<ActionConditionKind> kind; 5195 5196 /** 5197 * An expression that returns true or false, indicating whether the condition is 5198 * satisfied. 5199 */ 5200 @Child(name = "expression", type = { 5201 Expression.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5202 @Description(shortDefinition = "Boolean-valued expression", formalDefinition = "An expression that returns true or false, indicating whether the condition is satisfied.") 5203 protected Expression expression; 5204 5205 private static final long serialVersionUID = -455150438L; 5206 5207 /** 5208 * Constructor 5209 */ 5210 public PlanDefinitionActionConditionComponent() { 5211 super(); 5212 } 5213 5214 /** 5215 * Constructor 5216 */ 5217 public PlanDefinitionActionConditionComponent(Enumeration<ActionConditionKind> kind) { 5218 super(); 5219 this.kind = kind; 5220 } 5221 5222 /** 5223 * @return {@link #kind} (The kind of condition.). This is the underlying object 5224 * with id, value and extensions. The accessor "getKind" gives direct 5225 * access to the value 5226 */ 5227 public Enumeration<ActionConditionKind> getKindElement() { 5228 if (this.kind == null) 5229 if (Configuration.errorOnAutoCreate()) 5230 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.kind"); 5231 else if (Configuration.doAutoCreate()) 5232 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); // bb 5233 return this.kind; 5234 } 5235 5236 public boolean hasKindElement() { 5237 return this.kind != null && !this.kind.isEmpty(); 5238 } 5239 5240 public boolean hasKind() { 5241 return this.kind != null && !this.kind.isEmpty(); 5242 } 5243 5244 /** 5245 * @param value {@link #kind} (The kind of condition.). This is the underlying 5246 * object with id, value and extensions. The accessor "getKind" 5247 * gives direct access to the value 5248 */ 5249 public PlanDefinitionActionConditionComponent setKindElement(Enumeration<ActionConditionKind> value) { 5250 this.kind = value; 5251 return this; 5252 } 5253 5254 /** 5255 * @return The kind of condition. 5256 */ 5257 public ActionConditionKind getKind() { 5258 return this.kind == null ? null : this.kind.getValue(); 5259 } 5260 5261 /** 5262 * @param value The kind of condition. 5263 */ 5264 public PlanDefinitionActionConditionComponent setKind(ActionConditionKind value) { 5265 if (this.kind == null) 5266 this.kind = new Enumeration<ActionConditionKind>(new ActionConditionKindEnumFactory()); 5267 this.kind.setValue(value); 5268 return this; 5269 } 5270 5271 /** 5272 * @return {@link #expression} (An expression that returns true or false, 5273 * indicating whether the condition is satisfied.) 5274 */ 5275 public Expression getExpression() { 5276 if (this.expression == null) 5277 if (Configuration.errorOnAutoCreate()) 5278 throw new Error("Attempt to auto-create PlanDefinitionActionConditionComponent.expression"); 5279 else if (Configuration.doAutoCreate()) 5280 this.expression = new Expression(); // cc 5281 return this.expression; 5282 } 5283 5284 public boolean hasExpression() { 5285 return this.expression != null && !this.expression.isEmpty(); 5286 } 5287 5288 /** 5289 * @param value {@link #expression} (An expression that returns true or false, 5290 * indicating whether the condition is satisfied.) 5291 */ 5292 public PlanDefinitionActionConditionComponent setExpression(Expression value) { 5293 this.expression = value; 5294 return this; 5295 } 5296 5297 protected void listChildren(List<Property> children) { 5298 super.listChildren(children); 5299 children.add(new Property("kind", "code", "The kind of condition.", 0, 1, kind)); 5300 children.add(new Property("expression", "Expression", 5301 "An expression that returns true or false, indicating whether the condition is satisfied.", 0, 1, 5302 expression)); 5303 } 5304 5305 @Override 5306 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5307 switch (_hash) { 5308 case 3292052: 5309 /* kind */ return new Property("kind", "code", "The kind of condition.", 0, 1, kind); 5310 case -1795452264: 5311 /* expression */ return new Property("expression", "Expression", 5312 "An expression that returns true or false, indicating whether the condition is satisfied.", 0, 1, 5313 expression); 5314 default: 5315 return super.getNamedProperty(_hash, _name, _checkValid); 5316 } 5317 5318 } 5319 5320 @Override 5321 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5322 switch (hash) { 5323 case 3292052: 5324 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<ActionConditionKind> 5325 case -1795452264: 5326 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 5327 default: 5328 return super.getProperty(hash, name, checkValid); 5329 } 5330 5331 } 5332 5333 @Override 5334 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5335 switch (hash) { 5336 case 3292052: // kind 5337 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 5338 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 5339 return value; 5340 case -1795452264: // expression 5341 this.expression = castToExpression(value); // Expression 5342 return value; 5343 default: 5344 return super.setProperty(hash, name, value); 5345 } 5346 5347 } 5348 5349 @Override 5350 public Base setProperty(String name, Base value) throws FHIRException { 5351 if (name.equals("kind")) { 5352 value = new ActionConditionKindEnumFactory().fromType(castToCode(value)); 5353 this.kind = (Enumeration) value; // Enumeration<ActionConditionKind> 5354 } else if (name.equals("expression")) { 5355 this.expression = castToExpression(value); // Expression 5356 } else 5357 return super.setProperty(name, value); 5358 return value; 5359 } 5360 5361 @Override 5362 public Base makeProperty(int hash, String name) throws FHIRException { 5363 switch (hash) { 5364 case 3292052: 5365 return getKindElement(); 5366 case -1795452264: 5367 return getExpression(); 5368 default: 5369 return super.makeProperty(hash, name); 5370 } 5371 5372 } 5373 5374 @Override 5375 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5376 switch (hash) { 5377 case 3292052: 5378 /* kind */ return new String[] { "code" }; 5379 case -1795452264: 5380 /* expression */ return new String[] { "Expression" }; 5381 default: 5382 return super.getTypesForProperty(hash, name); 5383 } 5384 5385 } 5386 5387 @Override 5388 public Base addChild(String name) throws FHIRException { 5389 if (name.equals("kind")) { 5390 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.kind"); 5391 } else if (name.equals("expression")) { 5392 this.expression = new Expression(); 5393 return this.expression; 5394 } else 5395 return super.addChild(name); 5396 } 5397 5398 public PlanDefinitionActionConditionComponent copy() { 5399 PlanDefinitionActionConditionComponent dst = new PlanDefinitionActionConditionComponent(); 5400 copyValues(dst); 5401 return dst; 5402 } 5403 5404 public void copyValues(PlanDefinitionActionConditionComponent dst) { 5405 super.copyValues(dst); 5406 dst.kind = kind == null ? null : kind.copy(); 5407 dst.expression = expression == null ? null : expression.copy(); 5408 } 5409 5410 @Override 5411 public boolean equalsDeep(Base other_) { 5412 if (!super.equalsDeep(other_)) 5413 return false; 5414 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 5415 return false; 5416 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 5417 return compareDeep(kind, o.kind, true) && compareDeep(expression, o.expression, true); 5418 } 5419 5420 @Override 5421 public boolean equalsShallow(Base other_) { 5422 if (!super.equalsShallow(other_)) 5423 return false; 5424 if (!(other_ instanceof PlanDefinitionActionConditionComponent)) 5425 return false; 5426 PlanDefinitionActionConditionComponent o = (PlanDefinitionActionConditionComponent) other_; 5427 return compareValues(kind, o.kind, true); 5428 } 5429 5430 public boolean isEmpty() { 5431 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, expression); 5432 } 5433 5434 public String fhirType() { 5435 return "PlanDefinition.action.condition"; 5436 5437 } 5438 5439 } 5440 5441 @Block() 5442 public static class PlanDefinitionActionRelatedActionComponent extends BackboneElement 5443 implements IBaseBackboneElement { 5444 /** 5445 * The element id of the related action. 5446 */ 5447 @Child(name = "actionId", type = { IdType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5448 @Description(shortDefinition = "What action is this related to", formalDefinition = "The element id of the related action.") 5449 protected IdType actionId; 5450 5451 /** 5452 * The relationship of this action to the related action. 5453 */ 5454 @Child(name = "relationship", type = { 5455 CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 5456 @Description(shortDefinition = "before-start | before | before-end | concurrent-with-start | concurrent | concurrent-with-end | after-start | after | after-end", formalDefinition = "The relationship of this action to the related action.") 5457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-relationship-type") 5458 protected Enumeration<ActionRelationshipType> relationship; 5459 5460 /** 5461 * A duration or range of durations to apply to the relationship. For example, 5462 * 30-60 minutes before. 5463 */ 5464 @Child(name = "offset", type = { Duration.class, 5465 Range.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 5466 @Description(shortDefinition = "Time offset for the relationship", formalDefinition = "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.") 5467 protected Type offset; 5468 5469 private static final long serialVersionUID = 1063306770L; 5470 5471 /** 5472 * Constructor 5473 */ 5474 public PlanDefinitionActionRelatedActionComponent() { 5475 super(); 5476 } 5477 5478 /** 5479 * Constructor 5480 */ 5481 public PlanDefinitionActionRelatedActionComponent(IdType actionId, 5482 Enumeration<ActionRelationshipType> relationship) { 5483 super(); 5484 this.actionId = actionId; 5485 this.relationship = relationship; 5486 } 5487 5488 /** 5489 * @return {@link #actionId} (The element id of the related action.). This is 5490 * the underlying object with id, value and extensions. The accessor 5491 * "getActionId" gives direct access to the value 5492 */ 5493 public IdType getActionIdElement() { 5494 if (this.actionId == null) 5495 if (Configuration.errorOnAutoCreate()) 5496 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.actionId"); 5497 else if (Configuration.doAutoCreate()) 5498 this.actionId = new IdType(); // bb 5499 return this.actionId; 5500 } 5501 5502 public boolean hasActionIdElement() { 5503 return this.actionId != null && !this.actionId.isEmpty(); 5504 } 5505 5506 public boolean hasActionId() { 5507 return this.actionId != null && !this.actionId.isEmpty(); 5508 } 5509 5510 /** 5511 * @param value {@link #actionId} (The element id of the related action.). This 5512 * is the underlying object with id, value and extensions. The 5513 * accessor "getActionId" gives direct access to the value 5514 */ 5515 public PlanDefinitionActionRelatedActionComponent setActionIdElement(IdType value) { 5516 this.actionId = value; 5517 return this; 5518 } 5519 5520 /** 5521 * @return The element id of the related action. 5522 */ 5523 public String getActionId() { 5524 return this.actionId == null ? null : this.actionId.getValue(); 5525 } 5526 5527 /** 5528 * @param value The element id of the related action. 5529 */ 5530 public PlanDefinitionActionRelatedActionComponent setActionId(String value) { 5531 if (this.actionId == null) 5532 this.actionId = new IdType(); 5533 this.actionId.setValue(value); 5534 return this; 5535 } 5536 5537 /** 5538 * @return {@link #relationship} (The relationship of this action to the related 5539 * action.). This is the underlying object with id, value and 5540 * extensions. The accessor "getRelationship" gives direct access to the 5541 * value 5542 */ 5543 public Enumeration<ActionRelationshipType> getRelationshipElement() { 5544 if (this.relationship == null) 5545 if (Configuration.errorOnAutoCreate()) 5546 throw new Error("Attempt to auto-create PlanDefinitionActionRelatedActionComponent.relationship"); 5547 else if (Configuration.doAutoCreate()) 5548 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); // bb 5549 return this.relationship; 5550 } 5551 5552 public boolean hasRelationshipElement() { 5553 return this.relationship != null && !this.relationship.isEmpty(); 5554 } 5555 5556 public boolean hasRelationship() { 5557 return this.relationship != null && !this.relationship.isEmpty(); 5558 } 5559 5560 /** 5561 * @param value {@link #relationship} (The relationship of this action to the 5562 * related action.). This is the underlying object with id, value 5563 * and extensions. The accessor "getRelationship" gives direct 5564 * access to the value 5565 */ 5566 public PlanDefinitionActionRelatedActionComponent setRelationshipElement( 5567 Enumeration<ActionRelationshipType> value) { 5568 this.relationship = value; 5569 return this; 5570 } 5571 5572 /** 5573 * @return The relationship of this action to the related action. 5574 */ 5575 public ActionRelationshipType getRelationship() { 5576 return this.relationship == null ? null : this.relationship.getValue(); 5577 } 5578 5579 /** 5580 * @param value The relationship of this action to the related action. 5581 */ 5582 public PlanDefinitionActionRelatedActionComponent setRelationship(ActionRelationshipType value) { 5583 if (this.relationship == null) 5584 this.relationship = new Enumeration<ActionRelationshipType>(new ActionRelationshipTypeEnumFactory()); 5585 this.relationship.setValue(value); 5586 return this; 5587 } 5588 5589 /** 5590 * @return {@link #offset} (A duration or range of durations to apply to the 5591 * relationship. For example, 30-60 minutes before.) 5592 */ 5593 public Type getOffset() { 5594 return this.offset; 5595 } 5596 5597 /** 5598 * @return {@link #offset} (A duration or range of durations to apply to the 5599 * relationship. For example, 30-60 minutes before.) 5600 */ 5601 public Duration getOffsetDuration() throws FHIRException { 5602 if (this.offset == null) 5603 this.offset = new Duration(); 5604 if (!(this.offset instanceof Duration)) 5605 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.offset.getClass().getName() 5606 + " was encountered"); 5607 return (Duration) this.offset; 5608 } 5609 5610 public boolean hasOffsetDuration() { 5611 return this != null && this.offset instanceof Duration; 5612 } 5613 5614 /** 5615 * @return {@link #offset} (A duration or range of durations to apply to the 5616 * relationship. For example, 30-60 minutes before.) 5617 */ 5618 public Range getOffsetRange() throws FHIRException { 5619 if (this.offset == null) 5620 this.offset = new Range(); 5621 if (!(this.offset instanceof Range)) 5622 throw new FHIRException( 5623 "Type mismatch: the type Range was expected, but " + this.offset.getClass().getName() + " was encountered"); 5624 return (Range) this.offset; 5625 } 5626 5627 public boolean hasOffsetRange() { 5628 return this != null && this.offset instanceof Range; 5629 } 5630 5631 public boolean hasOffset() { 5632 return this.offset != null && !this.offset.isEmpty(); 5633 } 5634 5635 /** 5636 * @param value {@link #offset} (A duration or range of durations to apply to 5637 * the relationship. For example, 30-60 minutes before.) 5638 */ 5639 public PlanDefinitionActionRelatedActionComponent setOffset(Type value) { 5640 if (value != null && !(value instanceof Duration || value instanceof Range)) 5641 throw new Error("Not the right type for PlanDefinition.action.relatedAction.offset[x]: " + value.fhirType()); 5642 this.offset = value; 5643 return this; 5644 } 5645 5646 protected void listChildren(List<Property> children) { 5647 super.listChildren(children); 5648 children.add(new Property("actionId", "id", "The element id of the related action.", 0, 1, actionId)); 5649 children.add(new Property("relationship", "code", "The relationship of this action to the related action.", 0, 1, 5650 relationship)); 5651 children.add(new Property("offset[x]", "Duration|Range", 5652 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5653 offset)); 5654 } 5655 5656 @Override 5657 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5658 switch (_hash) { 5659 case -1656172047: 5660 /* actionId */ return new Property("actionId", "id", "The element id of the related action.", 0, 1, actionId); 5661 case -261851592: 5662 /* relationship */ return new Property("relationship", "code", 5663 "The relationship of this action to the related action.", 0, 1, relationship); 5664 case -1960684787: 5665 /* offset[x] */ return new Property("offset[x]", "Duration|Range", 5666 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5667 offset); 5668 case -1019779949: 5669 /* offset */ return new Property("offset[x]", "Duration|Range", 5670 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5671 offset); 5672 case 134075207: 5673 /* offsetDuration */ return new Property("offset[x]", "Duration|Range", 5674 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5675 offset); 5676 case 1263585386: 5677 /* offsetRange */ return new Property("offset[x]", "Duration|Range", 5678 "A duration or range of durations to apply to the relationship. For example, 30-60 minutes before.", 0, 1, 5679 offset); 5680 default: 5681 return super.getNamedProperty(_hash, _name, _checkValid); 5682 } 5683 5684 } 5685 5686 @Override 5687 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5688 switch (hash) { 5689 case -1656172047: 5690 /* actionId */ return this.actionId == null ? new Base[0] : new Base[] { this.actionId }; // IdType 5691 case -261851592: 5692 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Enumeration<ActionRelationshipType> 5693 case -1019779949: 5694 /* offset */ return this.offset == null ? new Base[0] : new Base[] { this.offset }; // Type 5695 default: 5696 return super.getProperty(hash, name, checkValid); 5697 } 5698 5699 } 5700 5701 @Override 5702 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5703 switch (hash) { 5704 case -1656172047: // actionId 5705 this.actionId = castToId(value); // IdType 5706 return value; 5707 case -261851592: // relationship 5708 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 5709 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5710 return value; 5711 case -1019779949: // offset 5712 this.offset = castToType(value); // Type 5713 return value; 5714 default: 5715 return super.setProperty(hash, name, value); 5716 } 5717 5718 } 5719 5720 @Override 5721 public Base setProperty(String name, Base value) throws FHIRException { 5722 if (name.equals("actionId")) { 5723 this.actionId = castToId(value); // IdType 5724 } else if (name.equals("relationship")) { 5725 value = new ActionRelationshipTypeEnumFactory().fromType(castToCode(value)); 5726 this.relationship = (Enumeration) value; // Enumeration<ActionRelationshipType> 5727 } else if (name.equals("offset[x]")) { 5728 this.offset = castToType(value); // Type 5729 } else 5730 return super.setProperty(name, value); 5731 return value; 5732 } 5733 5734 @Override 5735 public Base makeProperty(int hash, String name) throws FHIRException { 5736 switch (hash) { 5737 case -1656172047: 5738 return getActionIdElement(); 5739 case -261851592: 5740 return getRelationshipElement(); 5741 case -1960684787: 5742 return getOffset(); 5743 case -1019779949: 5744 return getOffset(); 5745 default: 5746 return super.makeProperty(hash, name); 5747 } 5748 5749 } 5750 5751 @Override 5752 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5753 switch (hash) { 5754 case -1656172047: 5755 /* actionId */ return new String[] { "id" }; 5756 case -261851592: 5757 /* relationship */ return new String[] { "code" }; 5758 case -1019779949: 5759 /* offset */ return new String[] { "Duration", "Range" }; 5760 default: 5761 return super.getTypesForProperty(hash, name); 5762 } 5763 5764 } 5765 5766 @Override 5767 public Base addChild(String name) throws FHIRException { 5768 if (name.equals("actionId")) { 5769 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.actionId"); 5770 } else if (name.equals("relationship")) { 5771 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.relationship"); 5772 } else if (name.equals("offsetDuration")) { 5773 this.offset = new Duration(); 5774 return this.offset; 5775 } else if (name.equals("offsetRange")) { 5776 this.offset = new Range(); 5777 return this.offset; 5778 } else 5779 return super.addChild(name); 5780 } 5781 5782 public PlanDefinitionActionRelatedActionComponent copy() { 5783 PlanDefinitionActionRelatedActionComponent dst = new PlanDefinitionActionRelatedActionComponent(); 5784 copyValues(dst); 5785 return dst; 5786 } 5787 5788 public void copyValues(PlanDefinitionActionRelatedActionComponent dst) { 5789 super.copyValues(dst); 5790 dst.actionId = actionId == null ? null : actionId.copy(); 5791 dst.relationship = relationship == null ? null : relationship.copy(); 5792 dst.offset = offset == null ? null : offset.copy(); 5793 } 5794 5795 @Override 5796 public boolean equalsDeep(Base other_) { 5797 if (!super.equalsDeep(other_)) 5798 return false; 5799 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 5800 return false; 5801 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 5802 return compareDeep(actionId, o.actionId, true) && compareDeep(relationship, o.relationship, true) 5803 && compareDeep(offset, o.offset, true); 5804 } 5805 5806 @Override 5807 public boolean equalsShallow(Base other_) { 5808 if (!super.equalsShallow(other_)) 5809 return false; 5810 if (!(other_ instanceof PlanDefinitionActionRelatedActionComponent)) 5811 return false; 5812 PlanDefinitionActionRelatedActionComponent o = (PlanDefinitionActionRelatedActionComponent) other_; 5813 return compareValues(actionId, o.actionId, true) && compareValues(relationship, o.relationship, true); 5814 } 5815 5816 public boolean isEmpty() { 5817 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actionId, relationship, offset); 5818 } 5819 5820 public String fhirType() { 5821 return "PlanDefinition.action.relatedAction"; 5822 5823 } 5824 5825 } 5826 5827 @Block() 5828 public static class PlanDefinitionActionParticipantComponent extends BackboneElement implements IBaseBackboneElement { 5829 /** 5830 * The type of participant in the action. 5831 */ 5832 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 5833 @Description(shortDefinition = "patient | practitioner | related-person | device", formalDefinition = "The type of participant in the action.") 5834 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-type") 5835 protected Enumeration<ActionParticipantType> type; 5836 5837 /** 5838 * The role the participant should play in performing the described action. 5839 */ 5840 @Child(name = "role", type = { 5841 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5842 @Description(shortDefinition = "E.g. Nurse, Surgeon, Parent", formalDefinition = "The role the participant should play in performing the described action.") 5843 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/action-participant-role") 5844 protected CodeableConcept role; 5845 5846 private static final long serialVersionUID = -1152013659L; 5847 5848 /** 5849 * Constructor 5850 */ 5851 public PlanDefinitionActionParticipantComponent() { 5852 super(); 5853 } 5854 5855 /** 5856 * Constructor 5857 */ 5858 public PlanDefinitionActionParticipantComponent(Enumeration<ActionParticipantType> type) { 5859 super(); 5860 this.type = type; 5861 } 5862 5863 /** 5864 * @return {@link #type} (The type of participant in the action.). This is the 5865 * underlying object with id, value and extensions. The accessor 5866 * "getType" gives direct access to the value 5867 */ 5868 public Enumeration<ActionParticipantType> getTypeElement() { 5869 if (this.type == null) 5870 if (Configuration.errorOnAutoCreate()) 5871 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.type"); 5872 else if (Configuration.doAutoCreate()) 5873 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); // bb 5874 return this.type; 5875 } 5876 5877 public boolean hasTypeElement() { 5878 return this.type != null && !this.type.isEmpty(); 5879 } 5880 5881 public boolean hasType() { 5882 return this.type != null && !this.type.isEmpty(); 5883 } 5884 5885 /** 5886 * @param value {@link #type} (The type of participant in the action.). This is 5887 * the underlying object with id, value and extensions. The 5888 * accessor "getType" gives direct access to the value 5889 */ 5890 public PlanDefinitionActionParticipantComponent setTypeElement(Enumeration<ActionParticipantType> value) { 5891 this.type = value; 5892 return this; 5893 } 5894 5895 /** 5896 * @return The type of participant in the action. 5897 */ 5898 public ActionParticipantType getType() { 5899 return this.type == null ? null : this.type.getValue(); 5900 } 5901 5902 /** 5903 * @param value The type of participant in the action. 5904 */ 5905 public PlanDefinitionActionParticipantComponent setType(ActionParticipantType value) { 5906 if (this.type == null) 5907 this.type = new Enumeration<ActionParticipantType>(new ActionParticipantTypeEnumFactory()); 5908 this.type.setValue(value); 5909 return this; 5910 } 5911 5912 /** 5913 * @return {@link #role} (The role the participant should play in performing the 5914 * described action.) 5915 */ 5916 public CodeableConcept getRole() { 5917 if (this.role == null) 5918 if (Configuration.errorOnAutoCreate()) 5919 throw new Error("Attempt to auto-create PlanDefinitionActionParticipantComponent.role"); 5920 else if (Configuration.doAutoCreate()) 5921 this.role = new CodeableConcept(); // cc 5922 return this.role; 5923 } 5924 5925 public boolean hasRole() { 5926 return this.role != null && !this.role.isEmpty(); 5927 } 5928 5929 /** 5930 * @param value {@link #role} (The role the participant should play in 5931 * performing the described action.) 5932 */ 5933 public PlanDefinitionActionParticipantComponent setRole(CodeableConcept value) { 5934 this.role = value; 5935 return this; 5936 } 5937 5938 protected void listChildren(List<Property> children) { 5939 super.listChildren(children); 5940 children.add(new Property("type", "code", "The type of participant in the action.", 0, 1, type)); 5941 children.add(new Property("role", "CodeableConcept", 5942 "The role the participant should play in performing the described action.", 0, 1, role)); 5943 } 5944 5945 @Override 5946 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5947 switch (_hash) { 5948 case 3575610: 5949 /* type */ return new Property("type", "code", "The type of participant in the action.", 0, 1, type); 5950 case 3506294: 5951 /* role */ return new Property("role", "CodeableConcept", 5952 "The role the participant should play in performing the described action.", 0, 1, role); 5953 default: 5954 return super.getNamedProperty(_hash, _name, _checkValid); 5955 } 5956 5957 } 5958 5959 @Override 5960 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5961 switch (hash) { 5962 case 3575610: 5963 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ActionParticipantType> 5964 case 3506294: 5965 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 5966 default: 5967 return super.getProperty(hash, name, checkValid); 5968 } 5969 5970 } 5971 5972 @Override 5973 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5974 switch (hash) { 5975 case 3575610: // type 5976 value = new ActionParticipantTypeEnumFactory().fromType(castToCode(value)); 5977 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 5978 return value; 5979 case 3506294: // role 5980 this.role = castToCodeableConcept(value); // CodeableConcept 5981 return value; 5982 default: 5983 return super.setProperty(hash, name, value); 5984 } 5985 5986 } 5987 5988 @Override 5989 public Base setProperty(String name, Base value) throws FHIRException { 5990 if (name.equals("type")) { 5991 value = new ActionParticipantTypeEnumFactory().fromType(castToCode(value)); 5992 this.type = (Enumeration) value; // Enumeration<ActionParticipantType> 5993 } else if (name.equals("role")) { 5994 this.role = castToCodeableConcept(value); // CodeableConcept 5995 } else 5996 return super.setProperty(name, value); 5997 return value; 5998 } 5999 6000 @Override 6001 public Base makeProperty(int hash, String name) throws FHIRException { 6002 switch (hash) { 6003 case 3575610: 6004 return getTypeElement(); 6005 case 3506294: 6006 return getRole(); 6007 default: 6008 return super.makeProperty(hash, name); 6009 } 6010 6011 } 6012 6013 @Override 6014 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6015 switch (hash) { 6016 case 3575610: 6017 /* type */ return new String[] { "code" }; 6018 case 3506294: 6019 /* role */ return new String[] { "CodeableConcept" }; 6020 default: 6021 return super.getTypesForProperty(hash, name); 6022 } 6023 6024 } 6025 6026 @Override 6027 public Base addChild(String name) throws FHIRException { 6028 if (name.equals("type")) { 6029 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.type"); 6030 } else if (name.equals("role")) { 6031 this.role = new CodeableConcept(); 6032 return this.role; 6033 } else 6034 return super.addChild(name); 6035 } 6036 6037 public PlanDefinitionActionParticipantComponent copy() { 6038 PlanDefinitionActionParticipantComponent dst = new PlanDefinitionActionParticipantComponent(); 6039 copyValues(dst); 6040 return dst; 6041 } 6042 6043 public void copyValues(PlanDefinitionActionParticipantComponent dst) { 6044 super.copyValues(dst); 6045 dst.type = type == null ? null : type.copy(); 6046 dst.role = role == null ? null : role.copy(); 6047 } 6048 6049 @Override 6050 public boolean equalsDeep(Base other_) { 6051 if (!super.equalsDeep(other_)) 6052 return false; 6053 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 6054 return false; 6055 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 6056 return compareDeep(type, o.type, true) && compareDeep(role, o.role, true); 6057 } 6058 6059 @Override 6060 public boolean equalsShallow(Base other_) { 6061 if (!super.equalsShallow(other_)) 6062 return false; 6063 if (!(other_ instanceof PlanDefinitionActionParticipantComponent)) 6064 return false; 6065 PlanDefinitionActionParticipantComponent o = (PlanDefinitionActionParticipantComponent) other_; 6066 return compareValues(type, o.type, true); 6067 } 6068 6069 public boolean isEmpty() { 6070 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, role); 6071 } 6072 6073 public String fhirType() { 6074 return "PlanDefinition.action.participant"; 6075 6076 } 6077 6078 } 6079 6080 @Block() 6081 public static class PlanDefinitionActionDynamicValueComponent extends BackboneElement 6082 implements IBaseBackboneElement { 6083 /** 6084 * The path to the element to be customized. This is the path on the resource 6085 * that will hold the result of the calculation defined by the expression. The 6086 * specified path SHALL be a FHIRPath resolveable on the specified target type 6087 * of the ActivityDefinition, and SHALL consist only of identifiers, constant 6088 * indexers, and a restricted subset of functions. The path is allowed to 6089 * contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 6090 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 6091 * Profile](fhirpath.html#simple) for full details). 6092 */ 6093 @Child(name = "path", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6094 @Description(shortDefinition = "The path to the element to be set dynamically", formalDefinition = "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).") 6095 protected StringType path; 6096 6097 /** 6098 * An expression specifying the value of the customized element. 6099 */ 6100 @Child(name = "expression", type = { 6101 Expression.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6102 @Description(shortDefinition = "An expression that provides the dynamic value for the customization", formalDefinition = "An expression specifying the value of the customized element.") 6103 protected Expression expression; 6104 6105 private static final long serialVersionUID = 1064529082L; 6106 6107 /** 6108 * Constructor 6109 */ 6110 public PlanDefinitionActionDynamicValueComponent() { 6111 super(); 6112 } 6113 6114 /** 6115 * @return {@link #path} (The path to the element to be customized. This is the 6116 * path on the resource that will hold the result of the calculation 6117 * defined by the expression. The specified path SHALL be a FHIRPath 6118 * resolveable on the specified target type of the ActivityDefinition, 6119 * and SHALL consist only of identifiers, constant indexers, and a 6120 * restricted subset of functions. The path is allowed to contain 6121 * qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 6122 * traverse multiple-cardinality sub-elements (see the [Simple FHIRPath 6123 * Profile](fhirpath.html#simple) for full details).). This is the 6124 * underlying object with id, value and extensions. The accessor 6125 * "getPath" gives direct access to the value 6126 */ 6127 public StringType getPathElement() { 6128 if (this.path == null) 6129 if (Configuration.errorOnAutoCreate()) 6130 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.path"); 6131 else if (Configuration.doAutoCreate()) 6132 this.path = new StringType(); // bb 6133 return this.path; 6134 } 6135 6136 public boolean hasPathElement() { 6137 return this.path != null && !this.path.isEmpty(); 6138 } 6139 6140 public boolean hasPath() { 6141 return this.path != null && !this.path.isEmpty(); 6142 } 6143 6144 /** 6145 * @param value {@link #path} (The path to the element to be customized. This is 6146 * the path on the resource that will hold the result of the 6147 * calculation defined by the expression. The specified path SHALL 6148 * be a FHIRPath resolveable on the specified target type of the 6149 * ActivityDefinition, and SHALL consist only of identifiers, 6150 * constant indexers, and a restricted subset of functions. The 6151 * path is allowed to contain qualifiers (.) to traverse 6152 * sub-elements, as well as indexers ([x]) to traverse 6153 * multiple-cardinality sub-elements (see the [Simple FHIRPath 6154 * Profile](fhirpath.html#simple) for full details).). This is the 6155 * underlying object with id, value and extensions. The accessor 6156 * "getPath" gives direct access to the value 6157 */ 6158 public PlanDefinitionActionDynamicValueComponent setPathElement(StringType value) { 6159 this.path = value; 6160 return this; 6161 } 6162 6163 /** 6164 * @return The path to the element to be customized. This is the path on the 6165 * resource that will hold the result of the calculation defined by the 6166 * expression. The specified path SHALL be a FHIRPath resolveable on the 6167 * specified target type of the ActivityDefinition, and SHALL consist 6168 * only of identifiers, constant indexers, and a restricted subset of 6169 * functions. The path is allowed to contain qualifiers (.) to traverse 6170 * sub-elements, as well as indexers ([x]) to traverse 6171 * multiple-cardinality sub-elements (see the [Simple FHIRPath 6172 * Profile](fhirpath.html#simple) for full details). 6173 */ 6174 public String getPath() { 6175 return this.path == null ? null : this.path.getValue(); 6176 } 6177 6178 /** 6179 * @param value The path to the element to be customized. This is the path on 6180 * the resource that will hold the result of the calculation 6181 * defined by the expression. The specified path SHALL be a 6182 * FHIRPath resolveable on the specified target type of the 6183 * ActivityDefinition, and SHALL consist only of identifiers, 6184 * constant indexers, and a restricted subset of functions. The 6185 * path is allowed to contain qualifiers (.) to traverse 6186 * sub-elements, as well as indexers ([x]) to traverse 6187 * multiple-cardinality sub-elements (see the [Simple FHIRPath 6188 * Profile](fhirpath.html#simple) for full details). 6189 */ 6190 public PlanDefinitionActionDynamicValueComponent setPath(String value) { 6191 if (Utilities.noString(value)) 6192 this.path = null; 6193 else { 6194 if (this.path == null) 6195 this.path = new StringType(); 6196 this.path.setValue(value); 6197 } 6198 return this; 6199 } 6200 6201 /** 6202 * @return {@link #expression} (An expression specifying the value of the 6203 * customized element.) 6204 */ 6205 public Expression getExpression() { 6206 if (this.expression == null) 6207 if (Configuration.errorOnAutoCreate()) 6208 throw new Error("Attempt to auto-create PlanDefinitionActionDynamicValueComponent.expression"); 6209 else if (Configuration.doAutoCreate()) 6210 this.expression = new Expression(); // cc 6211 return this.expression; 6212 } 6213 6214 public boolean hasExpression() { 6215 return this.expression != null && !this.expression.isEmpty(); 6216 } 6217 6218 /** 6219 * @param value {@link #expression} (An expression specifying the value of the 6220 * customized element.) 6221 */ 6222 public PlanDefinitionActionDynamicValueComponent setExpression(Expression value) { 6223 this.expression = value; 6224 return this; 6225 } 6226 6227 protected void listChildren(List<Property> children) { 6228 super.listChildren(children); 6229 children.add(new Property("path", "string", 6230 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 6231 0, 1, path)); 6232 children.add(new Property("expression", "Expression", 6233 "An expression specifying the value of the customized element.", 0, 1, expression)); 6234 } 6235 6236 @Override 6237 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6238 switch (_hash) { 6239 case 3433509: 6240 /* path */ return new Property("path", "string", 6241 "The path to the element to be customized. This is the path on the resource that will hold the result of the calculation defined by the expression. The specified path SHALL be a FHIRPath resolveable on the specified target type of the ActivityDefinition, and SHALL consist only of identifiers, constant indexers, and a restricted subset of functions. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 6242 0, 1, path); 6243 case -1795452264: 6244 /* expression */ return new Property("expression", "Expression", 6245 "An expression specifying the value of the customized element.", 0, 1, expression); 6246 default: 6247 return super.getNamedProperty(_hash, _name, _checkValid); 6248 } 6249 6250 } 6251 6252 @Override 6253 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6254 switch (hash) { 6255 case 3433509: 6256 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 6257 case -1795452264: 6258 /* expression */ return this.expression == null ? new Base[0] : new Base[] { this.expression }; // Expression 6259 default: 6260 return super.getProperty(hash, name, checkValid); 6261 } 6262 6263 } 6264 6265 @Override 6266 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6267 switch (hash) { 6268 case 3433509: // path 6269 this.path = castToString(value); // StringType 6270 return value; 6271 case -1795452264: // expression 6272 this.expression = castToExpression(value); // Expression 6273 return value; 6274 default: 6275 return super.setProperty(hash, name, value); 6276 } 6277 6278 } 6279 6280 @Override 6281 public Base setProperty(String name, Base value) throws FHIRException { 6282 if (name.equals("path")) { 6283 this.path = castToString(value); // StringType 6284 } else if (name.equals("expression")) { 6285 this.expression = castToExpression(value); // Expression 6286 } else 6287 return super.setProperty(name, value); 6288 return value; 6289 } 6290 6291 @Override 6292 public Base makeProperty(int hash, String name) throws FHIRException { 6293 switch (hash) { 6294 case 3433509: 6295 return getPathElement(); 6296 case -1795452264: 6297 return getExpression(); 6298 default: 6299 return super.makeProperty(hash, name); 6300 } 6301 6302 } 6303 6304 @Override 6305 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6306 switch (hash) { 6307 case 3433509: 6308 /* path */ return new String[] { "string" }; 6309 case -1795452264: 6310 /* expression */ return new String[] { "Expression" }; 6311 default: 6312 return super.getTypesForProperty(hash, name); 6313 } 6314 6315 } 6316 6317 @Override 6318 public Base addChild(String name) throws FHIRException { 6319 if (name.equals("path")) { 6320 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.path"); 6321 } else if (name.equals("expression")) { 6322 this.expression = new Expression(); 6323 return this.expression; 6324 } else 6325 return super.addChild(name); 6326 } 6327 6328 public PlanDefinitionActionDynamicValueComponent copy() { 6329 PlanDefinitionActionDynamicValueComponent dst = new PlanDefinitionActionDynamicValueComponent(); 6330 copyValues(dst); 6331 return dst; 6332 } 6333 6334 public void copyValues(PlanDefinitionActionDynamicValueComponent dst) { 6335 super.copyValues(dst); 6336 dst.path = path == null ? null : path.copy(); 6337 dst.expression = expression == null ? null : expression.copy(); 6338 } 6339 6340 @Override 6341 public boolean equalsDeep(Base other_) { 6342 if (!super.equalsDeep(other_)) 6343 return false; 6344 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 6345 return false; 6346 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 6347 return compareDeep(path, o.path, true) && compareDeep(expression, o.expression, true); 6348 } 6349 6350 @Override 6351 public boolean equalsShallow(Base other_) { 6352 if (!super.equalsShallow(other_)) 6353 return false; 6354 if (!(other_ instanceof PlanDefinitionActionDynamicValueComponent)) 6355 return false; 6356 PlanDefinitionActionDynamicValueComponent o = (PlanDefinitionActionDynamicValueComponent) other_; 6357 return compareValues(path, o.path, true); 6358 } 6359 6360 public boolean isEmpty() { 6361 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, expression); 6362 } 6363 6364 public String fhirType() { 6365 return "PlanDefinition.action.dynamicValue"; 6366 6367 } 6368 6369 } 6370 6371 /** 6372 * A formal identifier that is used to identify this plan definition when it is 6373 * represented in other formats, or referenced in a specification, model, design 6374 * or an instance. 6375 */ 6376 @Child(name = "identifier", type = { 6377 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6378 @Description(shortDefinition = "Additional identifier for the plan definition", formalDefinition = "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 6379 protected List<Identifier> identifier; 6380 6381 /** 6382 * An explanatory or alternate title for the plan definition giving additional 6383 * information about its content. 6384 */ 6385 @Child(name = "subtitle", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6386 @Description(shortDefinition = "Subordinate title of the plan definition", formalDefinition = "An explanatory or alternate title for the plan definition giving additional information about its content.") 6387 protected StringType subtitle; 6388 6389 /** 6390 * A high-level category for the plan definition that distinguishes the kinds of 6391 * systems that would be interested in the plan definition. 6392 */ 6393 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 6394 @Description(shortDefinition = "order-set | clinical-protocol | eca-rule | workflow-definition", formalDefinition = "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.") 6395 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/plan-definition-type") 6396 protected CodeableConcept type; 6397 6398 /** 6399 * A code or group definition that describes the intended subject of the plan 6400 * definition. 6401 */ 6402 @Child(name = "subject", type = { CodeableConcept.class, 6403 Group.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6404 @Description(shortDefinition = "Type of individual the plan definition is focused on", formalDefinition = "A code or group definition that describes the intended subject of the plan definition.") 6405 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 6406 protected Type subject; 6407 6408 /** 6409 * Explanation of why this plan definition is needed and why it has been 6410 * designed as it has. 6411 */ 6412 @Child(name = "purpose", type = { 6413 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6414 @Description(shortDefinition = "Why this plan definition is defined", formalDefinition = "Explanation of why this plan definition is needed and why it has been designed as it has.") 6415 protected MarkdownType purpose; 6416 6417 /** 6418 * A detailed description of how the plan definition is used from a clinical 6419 * perspective. 6420 */ 6421 @Child(name = "usage", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6422 @Description(shortDefinition = "Describes the clinical usage of the plan", formalDefinition = "A detailed description of how the plan definition is used from a clinical perspective.") 6423 protected StringType usage; 6424 6425 /** 6426 * A copyright statement relating to the plan definition and/or its contents. 6427 * Copyright statements are generally legal restrictions on the use and 6428 * publishing of the plan definition. 6429 */ 6430 @Child(name = "copyright", type = { 6431 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6432 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.") 6433 protected MarkdownType copyright; 6434 6435 /** 6436 * The date on which the resource content was approved by the publisher. 6437 * Approval happens once when the content is officially approved for usage. 6438 */ 6439 @Child(name = "approvalDate", type = { 6440 DateType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6441 @Description(shortDefinition = "When the plan definition was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 6442 protected DateType approvalDate; 6443 6444 /** 6445 * The date on which the resource content was last reviewed. Review happens 6446 * periodically after approval but does not change the original approval date. 6447 */ 6448 @Child(name = "lastReviewDate", type = { 6449 DateType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6450 @Description(shortDefinition = "When the plan definition was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 6451 protected DateType lastReviewDate; 6452 6453 /** 6454 * The period during which the plan definition content was or is planned to be 6455 * in active use. 6456 */ 6457 @Child(name = "effectivePeriod", type = { 6458 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 6459 @Description(shortDefinition = "When the plan definition is expected to be used", formalDefinition = "The period during which the plan definition content was or is planned to be in active use.") 6460 protected Period effectivePeriod; 6461 6462 /** 6463 * Descriptive topics related to the content of the plan definition. Topics 6464 * provide a high-level categorization of the definition that can be useful for 6465 * filtering and searching. 6466 */ 6467 @Child(name = "topic", type = { 6468 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6469 @Description(shortDefinition = "E.g. Education, Treatment, Assessment", formalDefinition = "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.") 6470 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 6471 protected List<CodeableConcept> topic; 6472 6473 /** 6474 * An individiual or organization primarily involved in the creation and 6475 * maintenance of the content. 6476 */ 6477 @Child(name = "author", type = { 6478 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6479 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 6480 protected List<ContactDetail> author; 6481 6482 /** 6483 * An individual or organization primarily responsible for internal coherence of 6484 * the content. 6485 */ 6486 @Child(name = "editor", type = { 6487 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6488 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 6489 protected List<ContactDetail> editor; 6490 6491 /** 6492 * An individual or organization primarily responsible for review of some aspect 6493 * of the content. 6494 */ 6495 @Child(name = "reviewer", type = { 6496 ContactDetail.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6497 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 6498 protected List<ContactDetail> reviewer; 6499 6500 /** 6501 * An individual or organization responsible for officially endorsing the 6502 * content for use in some setting. 6503 */ 6504 @Child(name = "endorser", type = { 6505 ContactDetail.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6506 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 6507 protected List<ContactDetail> endorser; 6508 6509 /** 6510 * Related artifacts such as additional documentation, justification, or 6511 * bibliographic references. 6512 */ 6513 @Child(name = "relatedArtifact", type = { 6514 RelatedArtifact.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6515 @Description(shortDefinition = "Additional documentation, citations", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 6516 protected List<RelatedArtifact> relatedArtifact; 6517 6518 /** 6519 * A reference to a Library resource containing any formal logic used by the 6520 * plan definition. 6521 */ 6522 @Child(name = "library", type = { 6523 CanonicalType.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6524 @Description(shortDefinition = "Logic used by the plan definition", formalDefinition = "A reference to a Library resource containing any formal logic used by the plan definition.") 6525 protected List<CanonicalType> library; 6526 6527 /** 6528 * Goals that describe what the activities within the plan are intended to 6529 * achieve. For example, weight loss, restoring an activity of daily living, 6530 * obtaining herd immunity via immunization, meeting a process improvement 6531 * objective, etc. 6532 */ 6533 @Child(name = "goal", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6534 @Description(shortDefinition = "What the plan is trying to accomplish", formalDefinition = "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.") 6535 protected List<PlanDefinitionGoalComponent> goal; 6536 6537 /** 6538 * An action or group of actions to be taken as part of the plan. 6539 */ 6540 @Child(name = "action", type = {}, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6541 @Description(shortDefinition = "Action defined by the plan", formalDefinition = "An action or group of actions to be taken as part of the plan.") 6542 protected List<PlanDefinitionActionComponent> action; 6543 6544 private static final long serialVersionUID = -1725695645L; 6545 6546 /** 6547 * Constructor 6548 */ 6549 public PlanDefinition() { 6550 super(); 6551 } 6552 6553 /** 6554 * Constructor 6555 */ 6556 public PlanDefinition(Enumeration<PublicationStatus> status) { 6557 super(); 6558 this.status = status; 6559 } 6560 6561 /** 6562 * @return {@link #url} (An absolute URI that is used to identify this plan 6563 * definition when it is referenced in a specification, model, design or 6564 * an instance; also called its canonical identifier. This SHOULD be 6565 * globally unique and SHOULD be a literal address at which at which an 6566 * authoritative instance of this plan definition is (or will be) 6567 * published. This URL can be the target of a canonical reference. It 6568 * SHALL remain the same when the plan definition is stored on different 6569 * servers.). This is the underlying object with id, value and 6570 * extensions. The accessor "getUrl" gives direct access to the value 6571 */ 6572 public UriType getUrlElement() { 6573 if (this.url == null) 6574 if (Configuration.errorOnAutoCreate()) 6575 throw new Error("Attempt to auto-create PlanDefinition.url"); 6576 else if (Configuration.doAutoCreate()) 6577 this.url = new UriType(); // bb 6578 return this.url; 6579 } 6580 6581 public boolean hasUrlElement() { 6582 return this.url != null && !this.url.isEmpty(); 6583 } 6584 6585 public boolean hasUrl() { 6586 return this.url != null && !this.url.isEmpty(); 6587 } 6588 6589 /** 6590 * @param value {@link #url} (An absolute URI that is used to identify this plan 6591 * definition when it is referenced in a specification, model, 6592 * design or an instance; also called its canonical identifier. 6593 * This SHOULD be globally unique and SHOULD be a literal address 6594 * at which at which an authoritative instance of this plan 6595 * definition is (or will be) published. This URL can be the target 6596 * of a canonical reference. It SHALL remain the same when the plan 6597 * definition is stored on different servers.). This is the 6598 * underlying object with id, value and extensions. The accessor 6599 * "getUrl" gives direct access to the value 6600 */ 6601 public PlanDefinition setUrlElement(UriType value) { 6602 this.url = value; 6603 return this; 6604 } 6605 6606 /** 6607 * @return An absolute URI that is used to identify this plan definition when it 6608 * is referenced in a specification, model, design or an instance; also 6609 * called its canonical identifier. This SHOULD be globally unique and 6610 * SHOULD be a literal address at which at which an authoritative 6611 * instance of this plan definition is (or will be) published. This URL 6612 * can be the target of a canonical reference. It SHALL remain the same 6613 * when the plan definition is stored on different servers. 6614 */ 6615 public String getUrl() { 6616 return this.url == null ? null : this.url.getValue(); 6617 } 6618 6619 /** 6620 * @param value An absolute URI that is used to identify this plan definition 6621 * when it is referenced in a specification, model, design or an 6622 * instance; also called its canonical identifier. This SHOULD be 6623 * globally unique and SHOULD be a literal address at which at 6624 * which an authoritative instance of this plan definition is (or 6625 * will be) published. This URL can be the target of a canonical 6626 * reference. It SHALL remain the same when the plan definition is 6627 * stored on different servers. 6628 */ 6629 public PlanDefinition setUrl(String value) { 6630 if (Utilities.noString(value)) 6631 this.url = null; 6632 else { 6633 if (this.url == null) 6634 this.url = new UriType(); 6635 this.url.setValue(value); 6636 } 6637 return this; 6638 } 6639 6640 /** 6641 * @return {@link #identifier} (A formal identifier that is used to identify 6642 * this plan definition when it is represented in other formats, or 6643 * referenced in a specification, model, design or an instance.) 6644 */ 6645 public List<Identifier> getIdentifier() { 6646 if (this.identifier == null) 6647 this.identifier = new ArrayList<Identifier>(); 6648 return this.identifier; 6649 } 6650 6651 /** 6652 * @return Returns a reference to <code>this</code> for easy method chaining 6653 */ 6654 public PlanDefinition setIdentifier(List<Identifier> theIdentifier) { 6655 this.identifier = theIdentifier; 6656 return this; 6657 } 6658 6659 public boolean hasIdentifier() { 6660 if (this.identifier == null) 6661 return false; 6662 for (Identifier item : this.identifier) 6663 if (!item.isEmpty()) 6664 return true; 6665 return false; 6666 } 6667 6668 public Identifier addIdentifier() { // 3 6669 Identifier t = new Identifier(); 6670 if (this.identifier == null) 6671 this.identifier = new ArrayList<Identifier>(); 6672 this.identifier.add(t); 6673 return t; 6674 } 6675 6676 public PlanDefinition addIdentifier(Identifier t) { // 3 6677 if (t == null) 6678 return this; 6679 if (this.identifier == null) 6680 this.identifier = new ArrayList<Identifier>(); 6681 this.identifier.add(t); 6682 return this; 6683 } 6684 6685 /** 6686 * @return The first repetition of repeating field {@link #identifier}, creating 6687 * it if it does not already exist 6688 */ 6689 public Identifier getIdentifierFirstRep() { 6690 if (getIdentifier().isEmpty()) { 6691 addIdentifier(); 6692 } 6693 return getIdentifier().get(0); 6694 } 6695 6696 /** 6697 * @return {@link #version} (The identifier that is used to identify this 6698 * version of the plan definition when it is referenced in a 6699 * specification, model, design or instance. This is an arbitrary value 6700 * managed by the plan definition author and is not expected to be 6701 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 6702 * if a managed version is not available. There is also no expectation 6703 * that versions can be placed in a lexicographical sequence. To provide 6704 * a version consistent with the Decision Support Service specification, 6705 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 6706 * information on versioning knowledge assets, refer to the Decision 6707 * Support Service specification. Note that a version is required for 6708 * non-experimental active artifacts.). This is the underlying object 6709 * with id, value and extensions. The accessor "getVersion" gives direct 6710 * access to the value 6711 */ 6712 public StringType getVersionElement() { 6713 if (this.version == null) 6714 if (Configuration.errorOnAutoCreate()) 6715 throw new Error("Attempt to auto-create PlanDefinition.version"); 6716 else if (Configuration.doAutoCreate()) 6717 this.version = new StringType(); // bb 6718 return this.version; 6719 } 6720 6721 public boolean hasVersionElement() { 6722 return this.version != null && !this.version.isEmpty(); 6723 } 6724 6725 public boolean hasVersion() { 6726 return this.version != null && !this.version.isEmpty(); 6727 } 6728 6729 /** 6730 * @param value {@link #version} (The identifier that is used to identify this 6731 * version of the plan definition when it is referenced in a 6732 * specification, model, design or instance. This is an arbitrary 6733 * value managed by the plan definition author and is not expected 6734 * to be globally unique. For example, it might be a timestamp 6735 * (e.g. yyyymmdd) if a managed version is not available. There is 6736 * also no expectation that versions can be placed in a 6737 * lexicographical sequence. To provide a version consistent with 6738 * the Decision Support Service specification, use the format 6739 * Major.Minor.Revision (e.g. 1.0.0). For more information on 6740 * versioning knowledge assets, refer to the Decision Support 6741 * Service specification. Note that a version is required for 6742 * non-experimental active artifacts.). This is the underlying 6743 * object with id, value and extensions. The accessor "getVersion" 6744 * gives direct access to the value 6745 */ 6746 public PlanDefinition setVersionElement(StringType value) { 6747 this.version = value; 6748 return this; 6749 } 6750 6751 /** 6752 * @return The identifier that is used to identify this version of the plan 6753 * definition when it is referenced in a specification, model, design or 6754 * instance. This is an arbitrary value managed by the plan definition 6755 * author and is not expected to be globally unique. For example, it 6756 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 6757 * available. There is also no expectation that versions can be placed 6758 * in a lexicographical sequence. To provide a version consistent with 6759 * the Decision Support Service specification, use the format 6760 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 6761 * knowledge assets, refer to the Decision Support Service 6762 * specification. Note that a version is required for non-experimental 6763 * active artifacts. 6764 */ 6765 public String getVersion() { 6766 return this.version == null ? null : this.version.getValue(); 6767 } 6768 6769 /** 6770 * @param value The identifier that is used to identify this version of the plan 6771 * definition when it is referenced in a specification, model, 6772 * design or instance. This is an arbitrary value managed by the 6773 * plan definition author and is not expected to be globally 6774 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 6775 * a managed version is not available. There is also no expectation 6776 * that versions can be placed in a lexicographical sequence. To 6777 * provide a version consistent with the Decision Support Service 6778 * specification, use the format Major.Minor.Revision (e.g. 1.0.0). 6779 * For more information on versioning knowledge assets, refer to 6780 * the Decision Support Service specification. Note that a version 6781 * is required for non-experimental active artifacts. 6782 */ 6783 public PlanDefinition setVersion(String value) { 6784 if (Utilities.noString(value)) 6785 this.version = null; 6786 else { 6787 if (this.version == null) 6788 this.version = new StringType(); 6789 this.version.setValue(value); 6790 } 6791 return this; 6792 } 6793 6794 /** 6795 * @return {@link #name} (A natural language name identifying the plan 6796 * definition. This name should be usable as an identifier for the 6797 * module by machine processing applications such as code generation.). 6798 * This is the underlying object with id, value and extensions. The 6799 * accessor "getName" gives direct access to the value 6800 */ 6801 public StringType getNameElement() { 6802 if (this.name == null) 6803 if (Configuration.errorOnAutoCreate()) 6804 throw new Error("Attempt to auto-create PlanDefinition.name"); 6805 else if (Configuration.doAutoCreate()) 6806 this.name = new StringType(); // bb 6807 return this.name; 6808 } 6809 6810 public boolean hasNameElement() { 6811 return this.name != null && !this.name.isEmpty(); 6812 } 6813 6814 public boolean hasName() { 6815 return this.name != null && !this.name.isEmpty(); 6816 } 6817 6818 /** 6819 * @param value {@link #name} (A natural language name identifying the plan 6820 * definition. This name should be usable as an identifier for the 6821 * module by machine processing applications such as code 6822 * generation.). This is the underlying object with id, value and 6823 * extensions. The accessor "getName" gives direct access to the 6824 * value 6825 */ 6826 public PlanDefinition setNameElement(StringType value) { 6827 this.name = value; 6828 return this; 6829 } 6830 6831 /** 6832 * @return A natural language name identifying the plan definition. This name 6833 * should be usable as an identifier for the module by machine 6834 * processing applications such as code generation. 6835 */ 6836 public String getName() { 6837 return this.name == null ? null : this.name.getValue(); 6838 } 6839 6840 /** 6841 * @param value A natural language name identifying the plan definition. This 6842 * name should be usable as an identifier for the module by machine 6843 * processing applications such as code generation. 6844 */ 6845 public PlanDefinition setName(String value) { 6846 if (Utilities.noString(value)) 6847 this.name = null; 6848 else { 6849 if (this.name == null) 6850 this.name = new StringType(); 6851 this.name.setValue(value); 6852 } 6853 return this; 6854 } 6855 6856 /** 6857 * @return {@link #title} (A short, descriptive, user-friendly title for the 6858 * plan definition.). This is the underlying object with id, value and 6859 * extensions. The accessor "getTitle" gives direct access to the value 6860 */ 6861 public StringType getTitleElement() { 6862 if (this.title == null) 6863 if (Configuration.errorOnAutoCreate()) 6864 throw new Error("Attempt to auto-create PlanDefinition.title"); 6865 else if (Configuration.doAutoCreate()) 6866 this.title = new StringType(); // bb 6867 return this.title; 6868 } 6869 6870 public boolean hasTitleElement() { 6871 return this.title != null && !this.title.isEmpty(); 6872 } 6873 6874 public boolean hasTitle() { 6875 return this.title != null && !this.title.isEmpty(); 6876 } 6877 6878 /** 6879 * @param value {@link #title} (A short, descriptive, user-friendly title for 6880 * the plan definition.). This is the underlying object with id, 6881 * value and extensions. The accessor "getTitle" gives direct 6882 * access to the value 6883 */ 6884 public PlanDefinition setTitleElement(StringType value) { 6885 this.title = value; 6886 return this; 6887 } 6888 6889 /** 6890 * @return A short, descriptive, user-friendly title for the plan definition. 6891 */ 6892 public String getTitle() { 6893 return this.title == null ? null : this.title.getValue(); 6894 } 6895 6896 /** 6897 * @param value A short, descriptive, user-friendly title for the plan 6898 * definition. 6899 */ 6900 public PlanDefinition setTitle(String value) { 6901 if (Utilities.noString(value)) 6902 this.title = null; 6903 else { 6904 if (this.title == null) 6905 this.title = new StringType(); 6906 this.title.setValue(value); 6907 } 6908 return this; 6909 } 6910 6911 /** 6912 * @return {@link #subtitle} (An explanatory or alternate title for the plan 6913 * definition giving additional information about its content.). This is 6914 * the underlying object with id, value and extensions. The accessor 6915 * "getSubtitle" gives direct access to the value 6916 */ 6917 public StringType getSubtitleElement() { 6918 if (this.subtitle == null) 6919 if (Configuration.errorOnAutoCreate()) 6920 throw new Error("Attempt to auto-create PlanDefinition.subtitle"); 6921 else if (Configuration.doAutoCreate()) 6922 this.subtitle = new StringType(); // bb 6923 return this.subtitle; 6924 } 6925 6926 public boolean hasSubtitleElement() { 6927 return this.subtitle != null && !this.subtitle.isEmpty(); 6928 } 6929 6930 public boolean hasSubtitle() { 6931 return this.subtitle != null && !this.subtitle.isEmpty(); 6932 } 6933 6934 /** 6935 * @param value {@link #subtitle} (An explanatory or alternate title for the 6936 * plan definition giving additional information about its 6937 * content.). This is the underlying object with id, value and 6938 * extensions. The accessor "getSubtitle" gives direct access to 6939 * the value 6940 */ 6941 public PlanDefinition setSubtitleElement(StringType value) { 6942 this.subtitle = value; 6943 return this; 6944 } 6945 6946 /** 6947 * @return An explanatory or alternate title for the plan definition giving 6948 * additional information about its content. 6949 */ 6950 public String getSubtitle() { 6951 return this.subtitle == null ? null : this.subtitle.getValue(); 6952 } 6953 6954 /** 6955 * @param value An explanatory or alternate title for the plan definition giving 6956 * additional information about its content. 6957 */ 6958 public PlanDefinition setSubtitle(String value) { 6959 if (Utilities.noString(value)) 6960 this.subtitle = null; 6961 else { 6962 if (this.subtitle == null) 6963 this.subtitle = new StringType(); 6964 this.subtitle.setValue(value); 6965 } 6966 return this; 6967 } 6968 6969 /** 6970 * @return {@link #type} (A high-level category for the plan definition that 6971 * distinguishes the kinds of systems that would be interested in the 6972 * plan definition.) 6973 */ 6974 public CodeableConcept getType() { 6975 if (this.type == null) 6976 if (Configuration.errorOnAutoCreate()) 6977 throw new Error("Attempt to auto-create PlanDefinition.type"); 6978 else if (Configuration.doAutoCreate()) 6979 this.type = new CodeableConcept(); // cc 6980 return this.type; 6981 } 6982 6983 public boolean hasType() { 6984 return this.type != null && !this.type.isEmpty(); 6985 } 6986 6987 /** 6988 * @param value {@link #type} (A high-level category for the plan definition 6989 * that distinguishes the kinds of systems that would be interested 6990 * in the plan definition.) 6991 */ 6992 public PlanDefinition setType(CodeableConcept value) { 6993 this.type = value; 6994 return this; 6995 } 6996 6997 /** 6998 * @return {@link #status} (The status of this plan definition. Enables tracking 6999 * the life-cycle of the content.). This is the underlying object with 7000 * id, value and extensions. The accessor "getStatus" gives direct 7001 * access to the value 7002 */ 7003 public Enumeration<PublicationStatus> getStatusElement() { 7004 if (this.status == null) 7005 if (Configuration.errorOnAutoCreate()) 7006 throw new Error("Attempt to auto-create PlanDefinition.status"); 7007 else if (Configuration.doAutoCreate()) 7008 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 7009 return this.status; 7010 } 7011 7012 public boolean hasStatusElement() { 7013 return this.status != null && !this.status.isEmpty(); 7014 } 7015 7016 public boolean hasStatus() { 7017 return this.status != null && !this.status.isEmpty(); 7018 } 7019 7020 /** 7021 * @param value {@link #status} (The status of this plan definition. Enables 7022 * tracking the life-cycle of the content.). This is the underlying 7023 * object with id, value and extensions. The accessor "getStatus" 7024 * gives direct access to the value 7025 */ 7026 public PlanDefinition setStatusElement(Enumeration<PublicationStatus> value) { 7027 this.status = value; 7028 return this; 7029 } 7030 7031 /** 7032 * @return The status of this plan definition. Enables tracking the life-cycle 7033 * of the content. 7034 */ 7035 public PublicationStatus getStatus() { 7036 return this.status == null ? null : this.status.getValue(); 7037 } 7038 7039 /** 7040 * @param value The status of this plan definition. Enables tracking the 7041 * life-cycle of the content. 7042 */ 7043 public PlanDefinition setStatus(PublicationStatus value) { 7044 if (this.status == null) 7045 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 7046 this.status.setValue(value); 7047 return this; 7048 } 7049 7050 /** 7051 * @return {@link #experimental} (A Boolean value to indicate that this plan 7052 * definition is authored for testing purposes (or 7053 * education/evaluation/marketing) and is not intended to be used for 7054 * genuine usage.). This is the underlying object with id, value and 7055 * extensions. The accessor "getExperimental" gives direct access to the 7056 * value 7057 */ 7058 public BooleanType getExperimentalElement() { 7059 if (this.experimental == null) 7060 if (Configuration.errorOnAutoCreate()) 7061 throw new Error("Attempt to auto-create PlanDefinition.experimental"); 7062 else if (Configuration.doAutoCreate()) 7063 this.experimental = new BooleanType(); // bb 7064 return this.experimental; 7065 } 7066 7067 public boolean hasExperimentalElement() { 7068 return this.experimental != null && !this.experimental.isEmpty(); 7069 } 7070 7071 public boolean hasExperimental() { 7072 return this.experimental != null && !this.experimental.isEmpty(); 7073 } 7074 7075 /** 7076 * @param value {@link #experimental} (A Boolean value to indicate that this 7077 * plan definition is authored for testing purposes (or 7078 * education/evaluation/marketing) and is not intended to be used 7079 * for genuine usage.). This is the underlying object with id, 7080 * value and extensions. The accessor "getExperimental" gives 7081 * direct access to the value 7082 */ 7083 public PlanDefinition setExperimentalElement(BooleanType value) { 7084 this.experimental = value; 7085 return this; 7086 } 7087 7088 /** 7089 * @return A Boolean value to indicate that this plan definition is authored for 7090 * testing purposes (or education/evaluation/marketing) and is not 7091 * intended to be used for genuine usage. 7092 */ 7093 public boolean getExperimental() { 7094 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7095 } 7096 7097 /** 7098 * @param value A Boolean value to indicate that this plan definition is 7099 * authored for testing purposes (or 7100 * education/evaluation/marketing) and is not intended to be used 7101 * for genuine usage. 7102 */ 7103 public PlanDefinition setExperimental(boolean value) { 7104 if (this.experimental == null) 7105 this.experimental = new BooleanType(); 7106 this.experimental.setValue(value); 7107 return this; 7108 } 7109 7110 /** 7111 * @return {@link #subject} (A code or group definition that describes the 7112 * intended subject of the plan definition.) 7113 */ 7114 public Type getSubject() { 7115 return this.subject; 7116 } 7117 7118 /** 7119 * @return {@link #subject} (A code or group definition that describes the 7120 * intended subject of the plan definition.) 7121 */ 7122 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 7123 if (this.subject == null) 7124 this.subject = new CodeableConcept(); 7125 if (!(this.subject instanceof CodeableConcept)) 7126 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 7127 + this.subject.getClass().getName() + " was encountered"); 7128 return (CodeableConcept) this.subject; 7129 } 7130 7131 public boolean hasSubjectCodeableConcept() { 7132 return this != null && this.subject instanceof CodeableConcept; 7133 } 7134 7135 /** 7136 * @return {@link #subject} (A code or group definition that describes the 7137 * intended subject of the plan definition.) 7138 */ 7139 public Reference getSubjectReference() throws FHIRException { 7140 if (this.subject == null) 7141 this.subject = new Reference(); 7142 if (!(this.subject instanceof Reference)) 7143 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 7144 + " was encountered"); 7145 return (Reference) this.subject; 7146 } 7147 7148 public boolean hasSubjectReference() { 7149 return this != null && this.subject instanceof Reference; 7150 } 7151 7152 public boolean hasSubject() { 7153 return this.subject != null && !this.subject.isEmpty(); 7154 } 7155 7156 /** 7157 * @param value {@link #subject} (A code or group definition that describes the 7158 * intended subject of the plan definition.) 7159 */ 7160 public PlanDefinition setSubject(Type value) { 7161 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 7162 throw new Error("Not the right type for PlanDefinition.subject[x]: " + value.fhirType()); 7163 this.subject = value; 7164 return this; 7165 } 7166 7167 /** 7168 * @return {@link #date} (The date (and optionally time) when the plan 7169 * definition was published. The date must change when the business 7170 * version changes and it must change if the status code changes. In 7171 * addition, it should change when the substantive content of the plan 7172 * definition changes.). This is the underlying object with id, value 7173 * and extensions. The accessor "getDate" gives direct access to the 7174 * value 7175 */ 7176 public DateTimeType getDateElement() { 7177 if (this.date == null) 7178 if (Configuration.errorOnAutoCreate()) 7179 throw new Error("Attempt to auto-create PlanDefinition.date"); 7180 else if (Configuration.doAutoCreate()) 7181 this.date = new DateTimeType(); // bb 7182 return this.date; 7183 } 7184 7185 public boolean hasDateElement() { 7186 return this.date != null && !this.date.isEmpty(); 7187 } 7188 7189 public boolean hasDate() { 7190 return this.date != null && !this.date.isEmpty(); 7191 } 7192 7193 /** 7194 * @param value {@link #date} (The date (and optionally time) when the plan 7195 * definition was published. The date must change when the business 7196 * version changes and it must change if the status code changes. 7197 * In addition, it should change when the substantive content of 7198 * the plan definition changes.). This is the underlying object 7199 * with id, value and extensions. The accessor "getDate" gives 7200 * direct access to the value 7201 */ 7202 public PlanDefinition setDateElement(DateTimeType value) { 7203 this.date = value; 7204 return this; 7205 } 7206 7207 /** 7208 * @return The date (and optionally time) when the plan definition was 7209 * published. The date must change when the business version changes and 7210 * it must change if the status code changes. In addition, it should 7211 * change when the substantive content of the plan definition changes. 7212 */ 7213 public Date getDate() { 7214 return this.date == null ? null : this.date.getValue(); 7215 } 7216 7217 /** 7218 * @param value The date (and optionally time) when the plan definition was 7219 * published. The date must change when the business version 7220 * changes and it must change if the status code changes. In 7221 * addition, it should change when the substantive content of the 7222 * plan definition changes. 7223 */ 7224 public PlanDefinition setDate(Date value) { 7225 if (value == null) 7226 this.date = null; 7227 else { 7228 if (this.date == null) 7229 this.date = new DateTimeType(); 7230 this.date.setValue(value); 7231 } 7232 return this; 7233 } 7234 7235 /** 7236 * @return {@link #publisher} (The name of the organization or individual that 7237 * published the plan definition.). This is the underlying object with 7238 * id, value and extensions. The accessor "getPublisher" gives direct 7239 * access to the value 7240 */ 7241 public StringType getPublisherElement() { 7242 if (this.publisher == null) 7243 if (Configuration.errorOnAutoCreate()) 7244 throw new Error("Attempt to auto-create PlanDefinition.publisher"); 7245 else if (Configuration.doAutoCreate()) 7246 this.publisher = new StringType(); // bb 7247 return this.publisher; 7248 } 7249 7250 public boolean hasPublisherElement() { 7251 return this.publisher != null && !this.publisher.isEmpty(); 7252 } 7253 7254 public boolean hasPublisher() { 7255 return this.publisher != null && !this.publisher.isEmpty(); 7256 } 7257 7258 /** 7259 * @param value {@link #publisher} (The name of the organization or individual 7260 * that published the plan definition.). This is the underlying 7261 * object with id, value and extensions. The accessor 7262 * "getPublisher" gives direct access to the value 7263 */ 7264 public PlanDefinition setPublisherElement(StringType value) { 7265 this.publisher = value; 7266 return this; 7267 } 7268 7269 /** 7270 * @return The name of the organization or individual that published the plan 7271 * definition. 7272 */ 7273 public String getPublisher() { 7274 return this.publisher == null ? null : this.publisher.getValue(); 7275 } 7276 7277 /** 7278 * @param value The name of the organization or individual that published the 7279 * plan definition. 7280 */ 7281 public PlanDefinition setPublisher(String value) { 7282 if (Utilities.noString(value)) 7283 this.publisher = null; 7284 else { 7285 if (this.publisher == null) 7286 this.publisher = new StringType(); 7287 this.publisher.setValue(value); 7288 } 7289 return this; 7290 } 7291 7292 /** 7293 * @return {@link #contact} (Contact details to assist a user in finding and 7294 * communicating with the publisher.) 7295 */ 7296 public List<ContactDetail> getContact() { 7297 if (this.contact == null) 7298 this.contact = new ArrayList<ContactDetail>(); 7299 return this.contact; 7300 } 7301 7302 /** 7303 * @return Returns a reference to <code>this</code> for easy method chaining 7304 */ 7305 public PlanDefinition setContact(List<ContactDetail> theContact) { 7306 this.contact = theContact; 7307 return this; 7308 } 7309 7310 public boolean hasContact() { 7311 if (this.contact == null) 7312 return false; 7313 for (ContactDetail item : this.contact) 7314 if (!item.isEmpty()) 7315 return true; 7316 return false; 7317 } 7318 7319 public ContactDetail addContact() { // 3 7320 ContactDetail t = new ContactDetail(); 7321 if (this.contact == null) 7322 this.contact = new ArrayList<ContactDetail>(); 7323 this.contact.add(t); 7324 return t; 7325 } 7326 7327 public PlanDefinition addContact(ContactDetail t) { // 3 7328 if (t == null) 7329 return this; 7330 if (this.contact == null) 7331 this.contact = new ArrayList<ContactDetail>(); 7332 this.contact.add(t); 7333 return this; 7334 } 7335 7336 /** 7337 * @return The first repetition of repeating field {@link #contact}, creating it 7338 * if it does not already exist 7339 */ 7340 public ContactDetail getContactFirstRep() { 7341 if (getContact().isEmpty()) { 7342 addContact(); 7343 } 7344 return getContact().get(0); 7345 } 7346 7347 /** 7348 * @return {@link #description} (A free text natural language description of the 7349 * plan definition from a consumer's perspective.). This is the 7350 * underlying object with id, value and extensions. The accessor 7351 * "getDescription" gives direct access to the value 7352 */ 7353 public MarkdownType getDescriptionElement() { 7354 if (this.description == null) 7355 if (Configuration.errorOnAutoCreate()) 7356 throw new Error("Attempt to auto-create PlanDefinition.description"); 7357 else if (Configuration.doAutoCreate()) 7358 this.description = new MarkdownType(); // bb 7359 return this.description; 7360 } 7361 7362 public boolean hasDescriptionElement() { 7363 return this.description != null && !this.description.isEmpty(); 7364 } 7365 7366 public boolean hasDescription() { 7367 return this.description != null && !this.description.isEmpty(); 7368 } 7369 7370 /** 7371 * @param value {@link #description} (A free text natural language description 7372 * of the plan definition from a consumer's perspective.). This is 7373 * the underlying object with id, value and extensions. The 7374 * accessor "getDescription" gives direct access to the value 7375 */ 7376 public PlanDefinition setDescriptionElement(MarkdownType value) { 7377 this.description = value; 7378 return this; 7379 } 7380 7381 /** 7382 * @return A free text natural language description of the plan definition from 7383 * a consumer's perspective. 7384 */ 7385 public String getDescription() { 7386 return this.description == null ? null : this.description.getValue(); 7387 } 7388 7389 /** 7390 * @param value A free text natural language description of the plan definition 7391 * from a consumer's perspective. 7392 */ 7393 public PlanDefinition setDescription(String value) { 7394 if (value == null) 7395 this.description = null; 7396 else { 7397 if (this.description == null) 7398 this.description = new MarkdownType(); 7399 this.description.setValue(value); 7400 } 7401 return this; 7402 } 7403 7404 /** 7405 * @return {@link #useContext} (The content was developed with a focus and 7406 * intent of supporting the contexts that are listed. These contexts may 7407 * be general categories (gender, age, ...) or may be references to 7408 * specific programs (insurance plans, studies, ...) and may be used to 7409 * assist with indexing and searching for appropriate plan definition 7410 * instances.) 7411 */ 7412 public List<UsageContext> getUseContext() { 7413 if (this.useContext == null) 7414 this.useContext = new ArrayList<UsageContext>(); 7415 return this.useContext; 7416 } 7417 7418 /** 7419 * @return Returns a reference to <code>this</code> for easy method chaining 7420 */ 7421 public PlanDefinition setUseContext(List<UsageContext> theUseContext) { 7422 this.useContext = theUseContext; 7423 return this; 7424 } 7425 7426 public boolean hasUseContext() { 7427 if (this.useContext == null) 7428 return false; 7429 for (UsageContext item : this.useContext) 7430 if (!item.isEmpty()) 7431 return true; 7432 return false; 7433 } 7434 7435 public UsageContext addUseContext() { // 3 7436 UsageContext t = new UsageContext(); 7437 if (this.useContext == null) 7438 this.useContext = new ArrayList<UsageContext>(); 7439 this.useContext.add(t); 7440 return t; 7441 } 7442 7443 public PlanDefinition addUseContext(UsageContext t) { // 3 7444 if (t == null) 7445 return this; 7446 if (this.useContext == null) 7447 this.useContext = new ArrayList<UsageContext>(); 7448 this.useContext.add(t); 7449 return this; 7450 } 7451 7452 /** 7453 * @return The first repetition of repeating field {@link #useContext}, creating 7454 * it if it does not already exist 7455 */ 7456 public UsageContext getUseContextFirstRep() { 7457 if (getUseContext().isEmpty()) { 7458 addUseContext(); 7459 } 7460 return getUseContext().get(0); 7461 } 7462 7463 /** 7464 * @return {@link #jurisdiction} (A legal or geographic region in which the plan 7465 * definition is intended to be used.) 7466 */ 7467 public List<CodeableConcept> getJurisdiction() { 7468 if (this.jurisdiction == null) 7469 this.jurisdiction = new ArrayList<CodeableConcept>(); 7470 return this.jurisdiction; 7471 } 7472 7473 /** 7474 * @return Returns a reference to <code>this</code> for easy method chaining 7475 */ 7476 public PlanDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 7477 this.jurisdiction = theJurisdiction; 7478 return this; 7479 } 7480 7481 public boolean hasJurisdiction() { 7482 if (this.jurisdiction == null) 7483 return false; 7484 for (CodeableConcept item : this.jurisdiction) 7485 if (!item.isEmpty()) 7486 return true; 7487 return false; 7488 } 7489 7490 public CodeableConcept addJurisdiction() { // 3 7491 CodeableConcept t = new CodeableConcept(); 7492 if (this.jurisdiction == null) 7493 this.jurisdiction = new ArrayList<CodeableConcept>(); 7494 this.jurisdiction.add(t); 7495 return t; 7496 } 7497 7498 public PlanDefinition addJurisdiction(CodeableConcept t) { // 3 7499 if (t == null) 7500 return this; 7501 if (this.jurisdiction == null) 7502 this.jurisdiction = new ArrayList<CodeableConcept>(); 7503 this.jurisdiction.add(t); 7504 return this; 7505 } 7506 7507 /** 7508 * @return The first repetition of repeating field {@link #jurisdiction}, 7509 * creating it if it does not already exist 7510 */ 7511 public CodeableConcept getJurisdictionFirstRep() { 7512 if (getJurisdiction().isEmpty()) { 7513 addJurisdiction(); 7514 } 7515 return getJurisdiction().get(0); 7516 } 7517 7518 /** 7519 * @return {@link #purpose} (Explanation of why this plan definition is needed 7520 * and why it has been designed as it has.). This is the underlying 7521 * object with id, value and extensions. The accessor "getPurpose" gives 7522 * direct access to the value 7523 */ 7524 public MarkdownType getPurposeElement() { 7525 if (this.purpose == null) 7526 if (Configuration.errorOnAutoCreate()) 7527 throw new Error("Attempt to auto-create PlanDefinition.purpose"); 7528 else if (Configuration.doAutoCreate()) 7529 this.purpose = new MarkdownType(); // bb 7530 return this.purpose; 7531 } 7532 7533 public boolean hasPurposeElement() { 7534 return this.purpose != null && !this.purpose.isEmpty(); 7535 } 7536 7537 public boolean hasPurpose() { 7538 return this.purpose != null && !this.purpose.isEmpty(); 7539 } 7540 7541 /** 7542 * @param value {@link #purpose} (Explanation of why this plan definition is 7543 * needed and why it has been designed as it has.). This is the 7544 * underlying object with id, value and extensions. The accessor 7545 * "getPurpose" gives direct access to the value 7546 */ 7547 public PlanDefinition setPurposeElement(MarkdownType value) { 7548 this.purpose = value; 7549 return this; 7550 } 7551 7552 /** 7553 * @return Explanation of why this plan definition is needed and why it has been 7554 * designed as it has. 7555 */ 7556 public String getPurpose() { 7557 return this.purpose == null ? null : this.purpose.getValue(); 7558 } 7559 7560 /** 7561 * @param value Explanation of why this plan definition is needed and why it has 7562 * been designed as it has. 7563 */ 7564 public PlanDefinition setPurpose(String value) { 7565 if (value == null) 7566 this.purpose = null; 7567 else { 7568 if (this.purpose == null) 7569 this.purpose = new MarkdownType(); 7570 this.purpose.setValue(value); 7571 } 7572 return this; 7573 } 7574 7575 /** 7576 * @return {@link #usage} (A detailed description of how the plan definition is 7577 * used from a clinical perspective.). This is the underlying object 7578 * with id, value and extensions. The accessor "getUsage" gives direct 7579 * access to the value 7580 */ 7581 public StringType getUsageElement() { 7582 if (this.usage == null) 7583 if (Configuration.errorOnAutoCreate()) 7584 throw new Error("Attempt to auto-create PlanDefinition.usage"); 7585 else if (Configuration.doAutoCreate()) 7586 this.usage = new StringType(); // bb 7587 return this.usage; 7588 } 7589 7590 public boolean hasUsageElement() { 7591 return this.usage != null && !this.usage.isEmpty(); 7592 } 7593 7594 public boolean hasUsage() { 7595 return this.usage != null && !this.usage.isEmpty(); 7596 } 7597 7598 /** 7599 * @param value {@link #usage} (A detailed description of how the plan 7600 * definition is used from a clinical perspective.). This is the 7601 * underlying object with id, value and extensions. The accessor 7602 * "getUsage" gives direct access to the value 7603 */ 7604 public PlanDefinition setUsageElement(StringType value) { 7605 this.usage = value; 7606 return this; 7607 } 7608 7609 /** 7610 * @return A detailed description of how the plan definition is used from a 7611 * clinical perspective. 7612 */ 7613 public String getUsage() { 7614 return this.usage == null ? null : this.usage.getValue(); 7615 } 7616 7617 /** 7618 * @param value A detailed description of how the plan definition is used from a 7619 * clinical perspective. 7620 */ 7621 public PlanDefinition setUsage(String value) { 7622 if (Utilities.noString(value)) 7623 this.usage = null; 7624 else { 7625 if (this.usage == null) 7626 this.usage = new StringType(); 7627 this.usage.setValue(value); 7628 } 7629 return this; 7630 } 7631 7632 /** 7633 * @return {@link #copyright} (A copyright statement relating to the plan 7634 * definition and/or its contents. Copyright statements are generally 7635 * legal restrictions on the use and publishing of the plan 7636 * definition.). This is the underlying object with id, value and 7637 * extensions. The accessor "getCopyright" gives direct access to the 7638 * value 7639 */ 7640 public MarkdownType getCopyrightElement() { 7641 if (this.copyright == null) 7642 if (Configuration.errorOnAutoCreate()) 7643 throw new Error("Attempt to auto-create PlanDefinition.copyright"); 7644 else if (Configuration.doAutoCreate()) 7645 this.copyright = new MarkdownType(); // bb 7646 return this.copyright; 7647 } 7648 7649 public boolean hasCopyrightElement() { 7650 return this.copyright != null && !this.copyright.isEmpty(); 7651 } 7652 7653 public boolean hasCopyright() { 7654 return this.copyright != null && !this.copyright.isEmpty(); 7655 } 7656 7657 /** 7658 * @param value {@link #copyright} (A copyright statement relating to the plan 7659 * definition and/or its contents. Copyright statements are 7660 * generally legal restrictions on the use and publishing of the 7661 * plan definition.). This is the underlying object with id, value 7662 * and extensions. The accessor "getCopyright" gives direct access 7663 * to the value 7664 */ 7665 public PlanDefinition setCopyrightElement(MarkdownType value) { 7666 this.copyright = value; 7667 return this; 7668 } 7669 7670 /** 7671 * @return A copyright statement relating to the plan definition and/or its 7672 * contents. Copyright statements are generally legal restrictions on 7673 * the use and publishing of the plan definition. 7674 */ 7675 public String getCopyright() { 7676 return this.copyright == null ? null : this.copyright.getValue(); 7677 } 7678 7679 /** 7680 * @param value A copyright statement relating to the plan definition and/or its 7681 * contents. Copyright statements are generally legal restrictions 7682 * on the use and publishing of the plan definition. 7683 */ 7684 public PlanDefinition setCopyright(String value) { 7685 if (value == null) 7686 this.copyright = null; 7687 else { 7688 if (this.copyright == null) 7689 this.copyright = new MarkdownType(); 7690 this.copyright.setValue(value); 7691 } 7692 return this; 7693 } 7694 7695 /** 7696 * @return {@link #approvalDate} (The date on which the resource content was 7697 * approved by the publisher. Approval happens once when the content is 7698 * officially approved for usage.). This is the underlying object with 7699 * id, value and extensions. The accessor "getApprovalDate" gives direct 7700 * access to the value 7701 */ 7702 public DateType getApprovalDateElement() { 7703 if (this.approvalDate == null) 7704 if (Configuration.errorOnAutoCreate()) 7705 throw new Error("Attempt to auto-create PlanDefinition.approvalDate"); 7706 else if (Configuration.doAutoCreate()) 7707 this.approvalDate = new DateType(); // bb 7708 return this.approvalDate; 7709 } 7710 7711 public boolean hasApprovalDateElement() { 7712 return this.approvalDate != null && !this.approvalDate.isEmpty(); 7713 } 7714 7715 public boolean hasApprovalDate() { 7716 return this.approvalDate != null && !this.approvalDate.isEmpty(); 7717 } 7718 7719 /** 7720 * @param value {@link #approvalDate} (The date on which the resource content 7721 * was approved by the publisher. Approval happens once when the 7722 * content is officially approved for usage.). This is the 7723 * underlying object with id, value and extensions. The accessor 7724 * "getApprovalDate" gives direct access to the value 7725 */ 7726 public PlanDefinition setApprovalDateElement(DateType value) { 7727 this.approvalDate = value; 7728 return this; 7729 } 7730 7731 /** 7732 * @return The date on which the resource content was approved by the publisher. 7733 * Approval happens once when the content is officially approved for 7734 * usage. 7735 */ 7736 public Date getApprovalDate() { 7737 return this.approvalDate == null ? null : this.approvalDate.getValue(); 7738 } 7739 7740 /** 7741 * @param value The date on which the resource content was approved by the 7742 * publisher. Approval happens once when the content is officially 7743 * approved for usage. 7744 */ 7745 public PlanDefinition setApprovalDate(Date value) { 7746 if (value == null) 7747 this.approvalDate = null; 7748 else { 7749 if (this.approvalDate == null) 7750 this.approvalDate = new DateType(); 7751 this.approvalDate.setValue(value); 7752 } 7753 return this; 7754 } 7755 7756 /** 7757 * @return {@link #lastReviewDate} (The date on which the resource content was 7758 * last reviewed. Review happens periodically after approval but does 7759 * not change the original approval date.). This is the underlying 7760 * object with id, value and extensions. The accessor 7761 * "getLastReviewDate" gives direct access to the value 7762 */ 7763 public DateType getLastReviewDateElement() { 7764 if (this.lastReviewDate == null) 7765 if (Configuration.errorOnAutoCreate()) 7766 throw new Error("Attempt to auto-create PlanDefinition.lastReviewDate"); 7767 else if (Configuration.doAutoCreate()) 7768 this.lastReviewDate = new DateType(); // bb 7769 return this.lastReviewDate; 7770 } 7771 7772 public boolean hasLastReviewDateElement() { 7773 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 7774 } 7775 7776 public boolean hasLastReviewDate() { 7777 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 7778 } 7779 7780 /** 7781 * @param value {@link #lastReviewDate} (The date on which the resource content 7782 * was last reviewed. Review happens periodically after approval 7783 * but does not change the original approval date.). This is the 7784 * underlying object with id, value and extensions. The accessor 7785 * "getLastReviewDate" gives direct access to the value 7786 */ 7787 public PlanDefinition setLastReviewDateElement(DateType value) { 7788 this.lastReviewDate = value; 7789 return this; 7790 } 7791 7792 /** 7793 * @return The date on which the resource content was last reviewed. Review 7794 * happens periodically after approval but does not change the original 7795 * approval date. 7796 */ 7797 public Date getLastReviewDate() { 7798 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 7799 } 7800 7801 /** 7802 * @param value The date on which the resource content was last reviewed. Review 7803 * happens periodically after approval but does not change the 7804 * original approval date. 7805 */ 7806 public PlanDefinition setLastReviewDate(Date value) { 7807 if (value == null) 7808 this.lastReviewDate = null; 7809 else { 7810 if (this.lastReviewDate == null) 7811 this.lastReviewDate = new DateType(); 7812 this.lastReviewDate.setValue(value); 7813 } 7814 return this; 7815 } 7816 7817 /** 7818 * @return {@link #effectivePeriod} (The period during which the plan definition 7819 * content was or is planned to be in active use.) 7820 */ 7821 public Period getEffectivePeriod() { 7822 if (this.effectivePeriod == null) 7823 if (Configuration.errorOnAutoCreate()) 7824 throw new Error("Attempt to auto-create PlanDefinition.effectivePeriod"); 7825 else if (Configuration.doAutoCreate()) 7826 this.effectivePeriod = new Period(); // cc 7827 return this.effectivePeriod; 7828 } 7829 7830 public boolean hasEffectivePeriod() { 7831 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 7832 } 7833 7834 /** 7835 * @param value {@link #effectivePeriod} (The period during which the plan 7836 * definition content was or is planned to be in active use.) 7837 */ 7838 public PlanDefinition setEffectivePeriod(Period value) { 7839 this.effectivePeriod = value; 7840 return this; 7841 } 7842 7843 /** 7844 * @return {@link #topic} (Descriptive topics related to the content of the plan 7845 * definition. Topics provide a high-level categorization of the 7846 * definition that can be useful for filtering and searching.) 7847 */ 7848 public List<CodeableConcept> getTopic() { 7849 if (this.topic == null) 7850 this.topic = new ArrayList<CodeableConcept>(); 7851 return this.topic; 7852 } 7853 7854 /** 7855 * @return Returns a reference to <code>this</code> for easy method chaining 7856 */ 7857 public PlanDefinition setTopic(List<CodeableConcept> theTopic) { 7858 this.topic = theTopic; 7859 return this; 7860 } 7861 7862 public boolean hasTopic() { 7863 if (this.topic == null) 7864 return false; 7865 for (CodeableConcept item : this.topic) 7866 if (!item.isEmpty()) 7867 return true; 7868 return false; 7869 } 7870 7871 public CodeableConcept addTopic() { // 3 7872 CodeableConcept t = new CodeableConcept(); 7873 if (this.topic == null) 7874 this.topic = new ArrayList<CodeableConcept>(); 7875 this.topic.add(t); 7876 return t; 7877 } 7878 7879 public PlanDefinition addTopic(CodeableConcept t) { // 3 7880 if (t == null) 7881 return this; 7882 if (this.topic == null) 7883 this.topic = new ArrayList<CodeableConcept>(); 7884 this.topic.add(t); 7885 return this; 7886 } 7887 7888 /** 7889 * @return The first repetition of repeating field {@link #topic}, creating it 7890 * if it does not already exist 7891 */ 7892 public CodeableConcept getTopicFirstRep() { 7893 if (getTopic().isEmpty()) { 7894 addTopic(); 7895 } 7896 return getTopic().get(0); 7897 } 7898 7899 /** 7900 * @return {@link #author} (An individiual or organization primarily involved in 7901 * the creation and maintenance of the content.) 7902 */ 7903 public List<ContactDetail> getAuthor() { 7904 if (this.author == null) 7905 this.author = new ArrayList<ContactDetail>(); 7906 return this.author; 7907 } 7908 7909 /** 7910 * @return Returns a reference to <code>this</code> for easy method chaining 7911 */ 7912 public PlanDefinition setAuthor(List<ContactDetail> theAuthor) { 7913 this.author = theAuthor; 7914 return this; 7915 } 7916 7917 public boolean hasAuthor() { 7918 if (this.author == null) 7919 return false; 7920 for (ContactDetail item : this.author) 7921 if (!item.isEmpty()) 7922 return true; 7923 return false; 7924 } 7925 7926 public ContactDetail addAuthor() { // 3 7927 ContactDetail t = new ContactDetail(); 7928 if (this.author == null) 7929 this.author = new ArrayList<ContactDetail>(); 7930 this.author.add(t); 7931 return t; 7932 } 7933 7934 public PlanDefinition addAuthor(ContactDetail t) { // 3 7935 if (t == null) 7936 return this; 7937 if (this.author == null) 7938 this.author = new ArrayList<ContactDetail>(); 7939 this.author.add(t); 7940 return this; 7941 } 7942 7943 /** 7944 * @return The first repetition of repeating field {@link #author}, creating it 7945 * if it does not already exist 7946 */ 7947 public ContactDetail getAuthorFirstRep() { 7948 if (getAuthor().isEmpty()) { 7949 addAuthor(); 7950 } 7951 return getAuthor().get(0); 7952 } 7953 7954 /** 7955 * @return {@link #editor} (An individual or organization primarily responsible 7956 * for internal coherence of the content.) 7957 */ 7958 public List<ContactDetail> getEditor() { 7959 if (this.editor == null) 7960 this.editor = new ArrayList<ContactDetail>(); 7961 return this.editor; 7962 } 7963 7964 /** 7965 * @return Returns a reference to <code>this</code> for easy method chaining 7966 */ 7967 public PlanDefinition setEditor(List<ContactDetail> theEditor) { 7968 this.editor = theEditor; 7969 return this; 7970 } 7971 7972 public boolean hasEditor() { 7973 if (this.editor == null) 7974 return false; 7975 for (ContactDetail item : this.editor) 7976 if (!item.isEmpty()) 7977 return true; 7978 return false; 7979 } 7980 7981 public ContactDetail addEditor() { // 3 7982 ContactDetail t = new ContactDetail(); 7983 if (this.editor == null) 7984 this.editor = new ArrayList<ContactDetail>(); 7985 this.editor.add(t); 7986 return t; 7987 } 7988 7989 public PlanDefinition addEditor(ContactDetail t) { // 3 7990 if (t == null) 7991 return this; 7992 if (this.editor == null) 7993 this.editor = new ArrayList<ContactDetail>(); 7994 this.editor.add(t); 7995 return this; 7996 } 7997 7998 /** 7999 * @return The first repetition of repeating field {@link #editor}, creating it 8000 * if it does not already exist 8001 */ 8002 public ContactDetail getEditorFirstRep() { 8003 if (getEditor().isEmpty()) { 8004 addEditor(); 8005 } 8006 return getEditor().get(0); 8007 } 8008 8009 /** 8010 * @return {@link #reviewer} (An individual or organization primarily 8011 * responsible for review of some aspect of the content.) 8012 */ 8013 public List<ContactDetail> getReviewer() { 8014 if (this.reviewer == null) 8015 this.reviewer = new ArrayList<ContactDetail>(); 8016 return this.reviewer; 8017 } 8018 8019 /** 8020 * @return Returns a reference to <code>this</code> for easy method chaining 8021 */ 8022 public PlanDefinition setReviewer(List<ContactDetail> theReviewer) { 8023 this.reviewer = theReviewer; 8024 return this; 8025 } 8026 8027 public boolean hasReviewer() { 8028 if (this.reviewer == null) 8029 return false; 8030 for (ContactDetail item : this.reviewer) 8031 if (!item.isEmpty()) 8032 return true; 8033 return false; 8034 } 8035 8036 public ContactDetail addReviewer() { // 3 8037 ContactDetail t = new ContactDetail(); 8038 if (this.reviewer == null) 8039 this.reviewer = new ArrayList<ContactDetail>(); 8040 this.reviewer.add(t); 8041 return t; 8042 } 8043 8044 public PlanDefinition addReviewer(ContactDetail t) { // 3 8045 if (t == null) 8046 return this; 8047 if (this.reviewer == null) 8048 this.reviewer = new ArrayList<ContactDetail>(); 8049 this.reviewer.add(t); 8050 return this; 8051 } 8052 8053 /** 8054 * @return The first repetition of repeating field {@link #reviewer}, creating 8055 * it if it does not already exist 8056 */ 8057 public ContactDetail getReviewerFirstRep() { 8058 if (getReviewer().isEmpty()) { 8059 addReviewer(); 8060 } 8061 return getReviewer().get(0); 8062 } 8063 8064 /** 8065 * @return {@link #endorser} (An individual or organization responsible for 8066 * officially endorsing the content for use in some setting.) 8067 */ 8068 public List<ContactDetail> getEndorser() { 8069 if (this.endorser == null) 8070 this.endorser = new ArrayList<ContactDetail>(); 8071 return this.endorser; 8072 } 8073 8074 /** 8075 * @return Returns a reference to <code>this</code> for easy method chaining 8076 */ 8077 public PlanDefinition setEndorser(List<ContactDetail> theEndorser) { 8078 this.endorser = theEndorser; 8079 return this; 8080 } 8081 8082 public boolean hasEndorser() { 8083 if (this.endorser == null) 8084 return false; 8085 for (ContactDetail item : this.endorser) 8086 if (!item.isEmpty()) 8087 return true; 8088 return false; 8089 } 8090 8091 public ContactDetail addEndorser() { // 3 8092 ContactDetail t = new ContactDetail(); 8093 if (this.endorser == null) 8094 this.endorser = new ArrayList<ContactDetail>(); 8095 this.endorser.add(t); 8096 return t; 8097 } 8098 8099 public PlanDefinition addEndorser(ContactDetail t) { // 3 8100 if (t == null) 8101 return this; 8102 if (this.endorser == null) 8103 this.endorser = new ArrayList<ContactDetail>(); 8104 this.endorser.add(t); 8105 return this; 8106 } 8107 8108 /** 8109 * @return The first repetition of repeating field {@link #endorser}, creating 8110 * it if it does not already exist 8111 */ 8112 public ContactDetail getEndorserFirstRep() { 8113 if (getEndorser().isEmpty()) { 8114 addEndorser(); 8115 } 8116 return getEndorser().get(0); 8117 } 8118 8119 /** 8120 * @return {@link #relatedArtifact} (Related artifacts such as additional 8121 * documentation, justification, or bibliographic references.) 8122 */ 8123 public List<RelatedArtifact> getRelatedArtifact() { 8124 if (this.relatedArtifact == null) 8125 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 8126 return this.relatedArtifact; 8127 } 8128 8129 /** 8130 * @return Returns a reference to <code>this</code> for easy method chaining 8131 */ 8132 public PlanDefinition setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 8133 this.relatedArtifact = theRelatedArtifact; 8134 return this; 8135 } 8136 8137 public boolean hasRelatedArtifact() { 8138 if (this.relatedArtifact == null) 8139 return false; 8140 for (RelatedArtifact item : this.relatedArtifact) 8141 if (!item.isEmpty()) 8142 return true; 8143 return false; 8144 } 8145 8146 public RelatedArtifact addRelatedArtifact() { // 3 8147 RelatedArtifact t = new RelatedArtifact(); 8148 if (this.relatedArtifact == null) 8149 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 8150 this.relatedArtifact.add(t); 8151 return t; 8152 } 8153 8154 public PlanDefinition addRelatedArtifact(RelatedArtifact t) { // 3 8155 if (t == null) 8156 return this; 8157 if (this.relatedArtifact == null) 8158 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 8159 this.relatedArtifact.add(t); 8160 return this; 8161 } 8162 8163 /** 8164 * @return The first repetition of repeating field {@link #relatedArtifact}, 8165 * creating it if it does not already exist 8166 */ 8167 public RelatedArtifact getRelatedArtifactFirstRep() { 8168 if (getRelatedArtifact().isEmpty()) { 8169 addRelatedArtifact(); 8170 } 8171 return getRelatedArtifact().get(0); 8172 } 8173 8174 /** 8175 * @return {@link #library} (A reference to a Library resource containing any 8176 * formal logic used by the plan definition.) 8177 */ 8178 public List<CanonicalType> getLibrary() { 8179 if (this.library == null) 8180 this.library = new ArrayList<CanonicalType>(); 8181 return this.library; 8182 } 8183 8184 /** 8185 * @return Returns a reference to <code>this</code> for easy method chaining 8186 */ 8187 public PlanDefinition setLibrary(List<CanonicalType> theLibrary) { 8188 this.library = theLibrary; 8189 return this; 8190 } 8191 8192 public boolean hasLibrary() { 8193 if (this.library == null) 8194 return false; 8195 for (CanonicalType item : this.library) 8196 if (!item.isEmpty()) 8197 return true; 8198 return false; 8199 } 8200 8201 /** 8202 * @return {@link #library} (A reference to a Library resource containing any 8203 * formal logic used by the plan definition.) 8204 */ 8205 public CanonicalType addLibraryElement() {// 2 8206 CanonicalType t = new CanonicalType(); 8207 if (this.library == null) 8208 this.library = new ArrayList<CanonicalType>(); 8209 this.library.add(t); 8210 return t; 8211 } 8212 8213 /** 8214 * @param value {@link #library} (A reference to a Library resource containing 8215 * any formal logic used by the plan definition.) 8216 */ 8217 public PlanDefinition addLibrary(String value) { // 1 8218 CanonicalType t = new CanonicalType(); 8219 t.setValue(value); 8220 if (this.library == null) 8221 this.library = new ArrayList<CanonicalType>(); 8222 this.library.add(t); 8223 return this; 8224 } 8225 8226 /** 8227 * @param value {@link #library} (A reference to a Library resource containing 8228 * any formal logic used by the plan definition.) 8229 */ 8230 public boolean hasLibrary(String value) { 8231 if (this.library == null) 8232 return false; 8233 for (CanonicalType v : this.library) 8234 if (v.getValue().equals(value)) // canonical(Library) 8235 return true; 8236 return false; 8237 } 8238 8239 /** 8240 * @return {@link #goal} (Goals that describe what the activities within the 8241 * plan are intended to achieve. For example, weight loss, restoring an 8242 * activity of daily living, obtaining herd immunity via immunization, 8243 * meeting a process improvement objective, etc.) 8244 */ 8245 public List<PlanDefinitionGoalComponent> getGoal() { 8246 if (this.goal == null) 8247 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8248 return this.goal; 8249 } 8250 8251 /** 8252 * @return Returns a reference to <code>this</code> for easy method chaining 8253 */ 8254 public PlanDefinition setGoal(List<PlanDefinitionGoalComponent> theGoal) { 8255 this.goal = theGoal; 8256 return this; 8257 } 8258 8259 public boolean hasGoal() { 8260 if (this.goal == null) 8261 return false; 8262 for (PlanDefinitionGoalComponent item : this.goal) 8263 if (!item.isEmpty()) 8264 return true; 8265 return false; 8266 } 8267 8268 public PlanDefinitionGoalComponent addGoal() { // 3 8269 PlanDefinitionGoalComponent t = new PlanDefinitionGoalComponent(); 8270 if (this.goal == null) 8271 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8272 this.goal.add(t); 8273 return t; 8274 } 8275 8276 public PlanDefinition addGoal(PlanDefinitionGoalComponent t) { // 3 8277 if (t == null) 8278 return this; 8279 if (this.goal == null) 8280 this.goal = new ArrayList<PlanDefinitionGoalComponent>(); 8281 this.goal.add(t); 8282 return this; 8283 } 8284 8285 /** 8286 * @return The first repetition of repeating field {@link #goal}, creating it if 8287 * it does not already exist 8288 */ 8289 public PlanDefinitionGoalComponent getGoalFirstRep() { 8290 if (getGoal().isEmpty()) { 8291 addGoal(); 8292 } 8293 return getGoal().get(0); 8294 } 8295 8296 /** 8297 * @return {@link #action} (An action or group of actions to be taken as part of 8298 * the plan.) 8299 */ 8300 public List<PlanDefinitionActionComponent> getAction() { 8301 if (this.action == null) 8302 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8303 return this.action; 8304 } 8305 8306 /** 8307 * @return Returns a reference to <code>this</code> for easy method chaining 8308 */ 8309 public PlanDefinition setAction(List<PlanDefinitionActionComponent> theAction) { 8310 this.action = theAction; 8311 return this; 8312 } 8313 8314 public boolean hasAction() { 8315 if (this.action == null) 8316 return false; 8317 for (PlanDefinitionActionComponent item : this.action) 8318 if (!item.isEmpty()) 8319 return true; 8320 return false; 8321 } 8322 8323 public PlanDefinitionActionComponent addAction() { // 3 8324 PlanDefinitionActionComponent t = new PlanDefinitionActionComponent(); 8325 if (this.action == null) 8326 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8327 this.action.add(t); 8328 return t; 8329 } 8330 8331 public PlanDefinition addAction(PlanDefinitionActionComponent t) { // 3 8332 if (t == null) 8333 return this; 8334 if (this.action == null) 8335 this.action = new ArrayList<PlanDefinitionActionComponent>(); 8336 this.action.add(t); 8337 return this; 8338 } 8339 8340 /** 8341 * @return The first repetition of repeating field {@link #action}, creating it 8342 * if it does not already exist 8343 */ 8344 public PlanDefinitionActionComponent getActionFirstRep() { 8345 if (getAction().isEmpty()) { 8346 addAction(); 8347 } 8348 return getAction().get(0); 8349 } 8350 8351 protected void listChildren(List<Property> children) { 8352 super.listChildren(children); 8353 children.add(new Property("url", "uri", 8354 "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.", 8355 0, 1, url)); 8356 children.add(new Property("identifier", "Identifier", 8357 "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8358 0, java.lang.Integer.MAX_VALUE, identifier)); 8359 children.add(new Property("version", "string", 8360 "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 8361 0, 1, version)); 8362 children.add(new Property("name", "string", 8363 "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8364 0, 1, name)); 8365 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the plan definition.", 8366 0, 1, title)); 8367 children.add(new Property("subtitle", "string", 8368 "An explanatory or alternate title for the plan definition giving additional information about its content.", 0, 8369 1, subtitle)); 8370 children.add(new Property("type", "CodeableConcept", 8371 "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.", 8372 0, 1, type)); 8373 children.add(new Property("status", "code", 8374 "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 8375 children.add(new Property("experimental", "boolean", 8376 "A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8377 0, 1, experimental)); 8378 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 8379 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject)); 8380 children.add(new Property("date", "dateTime", 8381 "The date (and optionally time) when the plan definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 8382 0, 1, date)); 8383 children.add(new Property("publisher", "string", 8384 "The name of the organization or individual that published the plan definition.", 0, 1, publisher)); 8385 children.add(new Property("contact", "ContactDetail", 8386 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8387 java.lang.Integer.MAX_VALUE, contact)); 8388 children.add(new Property("description", "markdown", 8389 "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, 8390 description)); 8391 children.add(new Property("useContext", "UsageContext", 8392 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.", 8393 0, java.lang.Integer.MAX_VALUE, useContext)); 8394 children.add(new Property("jurisdiction", "CodeableConcept", 8395 "A legal or geographic region in which the plan definition is intended to be used.", 0, 8396 java.lang.Integer.MAX_VALUE, jurisdiction)); 8397 children.add(new Property("purpose", "markdown", 8398 "Explanation of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose)); 8399 children.add(new Property("usage", "string", 8400 "A detailed description of how the plan definition is used from a clinical perspective.", 0, 1, usage)); 8401 children.add(new Property("copyright", "markdown", 8402 "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 8403 0, 1, copyright)); 8404 children.add(new Property("approvalDate", "date", 8405 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 8406 0, 1, approvalDate)); 8407 children.add(new Property("lastReviewDate", "date", 8408 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 8409 0, 1, lastReviewDate)); 8410 children.add(new Property("effectivePeriod", "Period", 8411 "The period during which the plan definition content was or is planned to be in active use.", 0, 1, 8412 effectivePeriod)); 8413 children.add(new Property("topic", "CodeableConcept", 8414 "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 8415 0, java.lang.Integer.MAX_VALUE, topic)); 8416 children.add(new Property("author", "ContactDetail", 8417 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 8418 java.lang.Integer.MAX_VALUE, author)); 8419 children.add(new Property("editor", "ContactDetail", 8420 "An individual or organization primarily responsible for internal coherence of the content.", 0, 8421 java.lang.Integer.MAX_VALUE, editor)); 8422 children.add(new Property("reviewer", "ContactDetail", 8423 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 8424 java.lang.Integer.MAX_VALUE, reviewer)); 8425 children.add(new Property("endorser", "ContactDetail", 8426 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 8427 java.lang.Integer.MAX_VALUE, endorser)); 8428 children.add(new Property("relatedArtifact", "RelatedArtifact", 8429 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 8430 java.lang.Integer.MAX_VALUE, relatedArtifact)); 8431 children.add(new Property("library", "canonical(Library)", 8432 "A reference to a Library resource containing any formal logic used by the plan definition.", 0, 8433 java.lang.Integer.MAX_VALUE, library)); 8434 children.add(new Property("goal", "", 8435 "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.", 8436 0, java.lang.Integer.MAX_VALUE, goal)); 8437 children.add(new Property("action", "", "An action or group of actions to be taken as part of the plan.", 0, 8438 java.lang.Integer.MAX_VALUE, action)); 8439 } 8440 8441 @Override 8442 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8443 switch (_hash) { 8444 case 116079: 8445 /* url */ return new Property("url", "uri", 8446 "An absolute URI that is used to identify this plan definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this plan definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the plan definition is stored on different servers.", 8447 0, 1, url); 8448 case -1618432855: 8449 /* identifier */ return new Property("identifier", "Identifier", 8450 "A formal identifier that is used to identify this plan definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8451 0, java.lang.Integer.MAX_VALUE, identifier); 8452 case 351608024: 8453 /* version */ return new Property("version", "string", 8454 "The identifier that is used to identify this version of the plan definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the plan definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 8455 0, 1, version); 8456 case 3373707: 8457 /* name */ return new Property("name", "string", 8458 "A natural language name identifying the plan definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8459 0, 1, name); 8460 case 110371416: 8461 /* title */ return new Property("title", "string", 8462 "A short, descriptive, user-friendly title for the plan definition.", 0, 1, title); 8463 case -2060497896: 8464 /* subtitle */ return new Property("subtitle", "string", 8465 "An explanatory or alternate title for the plan definition giving additional information about its content.", 8466 0, 1, subtitle); 8467 case 3575610: 8468 /* type */ return new Property("type", "CodeableConcept", 8469 "A high-level category for the plan definition that distinguishes the kinds of systems that would be interested in the plan definition.", 8470 0, 1, type); 8471 case -892481550: 8472 /* status */ return new Property("status", "code", 8473 "The status of this plan definition. Enables tracking the life-cycle of the content.", 0, 1, status); 8474 case -404562712: 8475 /* experimental */ return new Property("experimental", "boolean", 8476 "A Boolean value to indicate that this plan definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8477 0, 1, experimental); 8478 case -573640748: 8479 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8480 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8481 case -1867885268: 8482 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8483 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8484 case -1257122603: 8485 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8486 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8487 case 772938623: 8488 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 8489 "A code or group definition that describes the intended subject of the plan definition.", 0, 1, subject); 8490 case 3076014: 8491 /* date */ return new Property("date", "dateTime", 8492 "The date (and optionally time) when the plan definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the plan definition changes.", 8493 0, 1, date); 8494 case 1447404028: 8495 /* publisher */ return new Property("publisher", "string", 8496 "The name of the organization or individual that published the plan definition.", 0, 1, publisher); 8497 case 951526432: 8498 /* contact */ return new Property("contact", "ContactDetail", 8499 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8500 java.lang.Integer.MAX_VALUE, contact); 8501 case -1724546052: 8502 /* description */ return new Property("description", "markdown", 8503 "A free text natural language description of the plan definition from a consumer's perspective.", 0, 1, 8504 description); 8505 case -669707736: 8506 /* useContext */ return new Property("useContext", "UsageContext", 8507 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate plan definition instances.", 8508 0, java.lang.Integer.MAX_VALUE, useContext); 8509 case -507075711: 8510 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 8511 "A legal or geographic region in which the plan definition is intended to be used.", 0, 8512 java.lang.Integer.MAX_VALUE, jurisdiction); 8513 case -220463842: 8514 /* purpose */ return new Property("purpose", "markdown", 8515 "Explanation of why this plan definition is needed and why it has been designed as it has.", 0, 1, purpose); 8516 case 111574433: 8517 /* usage */ return new Property("usage", "string", 8518 "A detailed description of how the plan definition is used from a clinical perspective.", 0, 1, usage); 8519 case 1522889671: 8520 /* copyright */ return new Property("copyright", "markdown", 8521 "A copyright statement relating to the plan definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the plan definition.", 8522 0, 1, copyright); 8523 case 223539345: 8524 /* approvalDate */ return new Property("approvalDate", "date", 8525 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 8526 0, 1, approvalDate); 8527 case -1687512484: 8528 /* lastReviewDate */ return new Property("lastReviewDate", "date", 8529 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 8530 0, 1, lastReviewDate); 8531 case -403934648: 8532 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 8533 "The period during which the plan definition content was or is planned to be in active use.", 0, 1, 8534 effectivePeriod); 8535 case 110546223: 8536 /* topic */ return new Property("topic", "CodeableConcept", 8537 "Descriptive topics related to the content of the plan definition. Topics provide a high-level categorization of the definition that can be useful for filtering and searching.", 8538 0, java.lang.Integer.MAX_VALUE, topic); 8539 case -1406328437: 8540 /* author */ return new Property("author", "ContactDetail", 8541 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 8542 java.lang.Integer.MAX_VALUE, author); 8543 case -1307827859: 8544 /* editor */ return new Property("editor", "ContactDetail", 8545 "An individual or organization primarily responsible for internal coherence of the content.", 0, 8546 java.lang.Integer.MAX_VALUE, editor); 8547 case -261190139: 8548 /* reviewer */ return new Property("reviewer", "ContactDetail", 8549 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 8550 java.lang.Integer.MAX_VALUE, reviewer); 8551 case 1740277666: 8552 /* endorser */ return new Property("endorser", "ContactDetail", 8553 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 8554 java.lang.Integer.MAX_VALUE, endorser); 8555 case 666807069: 8556 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 8557 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 8558 java.lang.Integer.MAX_VALUE, relatedArtifact); 8559 case 166208699: 8560 /* library */ return new Property("library", "canonical(Library)", 8561 "A reference to a Library resource containing any formal logic used by the plan definition.", 0, 8562 java.lang.Integer.MAX_VALUE, library); 8563 case 3178259: 8564 /* goal */ return new Property("goal", "", 8565 "Goals that describe what the activities within the plan are intended to achieve. For example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc.", 8566 0, java.lang.Integer.MAX_VALUE, goal); 8567 case -1422950858: 8568 /* action */ return new Property("action", "", "An action or group of actions to be taken as part of the plan.", 8569 0, java.lang.Integer.MAX_VALUE, action); 8570 default: 8571 return super.getNamedProperty(_hash, _name, _checkValid); 8572 } 8573 8574 } 8575 8576 @Override 8577 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8578 switch (hash) { 8579 case 116079: 8580 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 8581 case -1618432855: 8582 /* identifier */ return this.identifier == null ? new Base[0] 8583 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8584 case 351608024: 8585 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 8586 case 3373707: 8587 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 8588 case 110371416: 8589 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 8590 case -2060497896: 8591 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 8592 case 3575610: 8593 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 8594 case -892481550: 8595 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 8596 case -404562712: 8597 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 8598 case -1867885268: 8599 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 8600 case 3076014: 8601 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 8602 case 1447404028: 8603 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 8604 case 951526432: 8605 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 8606 case -1724546052: 8607 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 8608 case -669707736: 8609 /* useContext */ return this.useContext == null ? new Base[0] 8610 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 8611 case -507075711: 8612 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 8613 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 8614 case -220463842: 8615 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 8616 case 111574433: 8617 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 8618 case 1522889671: 8619 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 8620 case 223539345: 8621 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 8622 case -1687512484: 8623 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 8624 case -403934648: 8625 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 8626 case 110546223: 8627 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 8628 case -1406328437: 8629 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 8630 case -1307827859: 8631 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 8632 case -261190139: 8633 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 8634 case 1740277666: 8635 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 8636 case 666807069: 8637 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 8638 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 8639 case 166208699: 8640 /* library */ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 8641 case 3178259: 8642 /* goal */ return this.goal == null ? new Base[0] : this.goal.toArray(new Base[this.goal.size()]); // PlanDefinitionGoalComponent 8643 case -1422950858: 8644 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // PlanDefinitionActionComponent 8645 default: 8646 return super.getProperty(hash, name, checkValid); 8647 } 8648 8649 } 8650 8651 @Override 8652 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8653 switch (hash) { 8654 case 116079: // url 8655 this.url = castToUri(value); // UriType 8656 return value; 8657 case -1618432855: // identifier 8658 this.getIdentifier().add(castToIdentifier(value)); // Identifier 8659 return value; 8660 case 351608024: // version 8661 this.version = castToString(value); // StringType 8662 return value; 8663 case 3373707: // name 8664 this.name = castToString(value); // StringType 8665 return value; 8666 case 110371416: // title 8667 this.title = castToString(value); // StringType 8668 return value; 8669 case -2060497896: // subtitle 8670 this.subtitle = castToString(value); // StringType 8671 return value; 8672 case 3575610: // type 8673 this.type = castToCodeableConcept(value); // CodeableConcept 8674 return value; 8675 case -892481550: // status 8676 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8677 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8678 return value; 8679 case -404562712: // experimental 8680 this.experimental = castToBoolean(value); // BooleanType 8681 return value; 8682 case -1867885268: // subject 8683 this.subject = castToType(value); // Type 8684 return value; 8685 case 3076014: // date 8686 this.date = castToDateTime(value); // DateTimeType 8687 return value; 8688 case 1447404028: // publisher 8689 this.publisher = castToString(value); // StringType 8690 return value; 8691 case 951526432: // contact 8692 this.getContact().add(castToContactDetail(value)); // ContactDetail 8693 return value; 8694 case -1724546052: // description 8695 this.description = castToMarkdown(value); // MarkdownType 8696 return value; 8697 case -669707736: // useContext 8698 this.getUseContext().add(castToUsageContext(value)); // UsageContext 8699 return value; 8700 case -507075711: // jurisdiction 8701 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 8702 return value; 8703 case -220463842: // purpose 8704 this.purpose = castToMarkdown(value); // MarkdownType 8705 return value; 8706 case 111574433: // usage 8707 this.usage = castToString(value); // StringType 8708 return value; 8709 case 1522889671: // copyright 8710 this.copyright = castToMarkdown(value); // MarkdownType 8711 return value; 8712 case 223539345: // approvalDate 8713 this.approvalDate = castToDate(value); // DateType 8714 return value; 8715 case -1687512484: // lastReviewDate 8716 this.lastReviewDate = castToDate(value); // DateType 8717 return value; 8718 case -403934648: // effectivePeriod 8719 this.effectivePeriod = castToPeriod(value); // Period 8720 return value; 8721 case 110546223: // topic 8722 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 8723 return value; 8724 case -1406328437: // author 8725 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 8726 return value; 8727 case -1307827859: // editor 8728 this.getEditor().add(castToContactDetail(value)); // ContactDetail 8729 return value; 8730 case -261190139: // reviewer 8731 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 8732 return value; 8733 case 1740277666: // endorser 8734 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 8735 return value; 8736 case 666807069: // relatedArtifact 8737 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 8738 return value; 8739 case 166208699: // library 8740 this.getLibrary().add(castToCanonical(value)); // CanonicalType 8741 return value; 8742 case 3178259: // goal 8743 this.getGoal().add((PlanDefinitionGoalComponent) value); // PlanDefinitionGoalComponent 8744 return value; 8745 case -1422950858: // action 8746 this.getAction().add((PlanDefinitionActionComponent) value); // PlanDefinitionActionComponent 8747 return value; 8748 default: 8749 return super.setProperty(hash, name, value); 8750 } 8751 8752 } 8753 8754 @Override 8755 public Base setProperty(String name, Base value) throws FHIRException { 8756 if (name.equals("url")) { 8757 this.url = castToUri(value); // UriType 8758 } else if (name.equals("identifier")) { 8759 this.getIdentifier().add(castToIdentifier(value)); 8760 } else if (name.equals("version")) { 8761 this.version = castToString(value); // StringType 8762 } else if (name.equals("name")) { 8763 this.name = castToString(value); // StringType 8764 } else if (name.equals("title")) { 8765 this.title = castToString(value); // StringType 8766 } else if (name.equals("subtitle")) { 8767 this.subtitle = castToString(value); // StringType 8768 } else if (name.equals("type")) { 8769 this.type = castToCodeableConcept(value); // CodeableConcept 8770 } else if (name.equals("status")) { 8771 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8772 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8773 } else if (name.equals("experimental")) { 8774 this.experimental = castToBoolean(value); // BooleanType 8775 } else if (name.equals("subject[x]")) { 8776 this.subject = castToType(value); // Type 8777 } else if (name.equals("date")) { 8778 this.date = castToDateTime(value); // DateTimeType 8779 } else if (name.equals("publisher")) { 8780 this.publisher = castToString(value); // StringType 8781 } else if (name.equals("contact")) { 8782 this.getContact().add(castToContactDetail(value)); 8783 } else if (name.equals("description")) { 8784 this.description = castToMarkdown(value); // MarkdownType 8785 } else if (name.equals("useContext")) { 8786 this.getUseContext().add(castToUsageContext(value)); 8787 } else if (name.equals("jurisdiction")) { 8788 this.getJurisdiction().add(castToCodeableConcept(value)); 8789 } else if (name.equals("purpose")) { 8790 this.purpose = castToMarkdown(value); // MarkdownType 8791 } else if (name.equals("usage")) { 8792 this.usage = castToString(value); // StringType 8793 } else if (name.equals("copyright")) { 8794 this.copyright = castToMarkdown(value); // MarkdownType 8795 } else if (name.equals("approvalDate")) { 8796 this.approvalDate = castToDate(value); // DateType 8797 } else if (name.equals("lastReviewDate")) { 8798 this.lastReviewDate = castToDate(value); // DateType 8799 } else if (name.equals("effectivePeriod")) { 8800 this.effectivePeriod = castToPeriod(value); // Period 8801 } else if (name.equals("topic")) { 8802 this.getTopic().add(castToCodeableConcept(value)); 8803 } else if (name.equals("author")) { 8804 this.getAuthor().add(castToContactDetail(value)); 8805 } else if (name.equals("editor")) { 8806 this.getEditor().add(castToContactDetail(value)); 8807 } else if (name.equals("reviewer")) { 8808 this.getReviewer().add(castToContactDetail(value)); 8809 } else if (name.equals("endorser")) { 8810 this.getEndorser().add(castToContactDetail(value)); 8811 } else if (name.equals("relatedArtifact")) { 8812 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 8813 } else if (name.equals("library")) { 8814 this.getLibrary().add(castToCanonical(value)); 8815 } else if (name.equals("goal")) { 8816 this.getGoal().add((PlanDefinitionGoalComponent) value); 8817 } else if (name.equals("action")) { 8818 this.getAction().add((PlanDefinitionActionComponent) value); 8819 } else 8820 return super.setProperty(name, value); 8821 return value; 8822 } 8823 8824 @Override 8825 public Base makeProperty(int hash, String name) throws FHIRException { 8826 switch (hash) { 8827 case 116079: 8828 return getUrlElement(); 8829 case -1618432855: 8830 return addIdentifier(); 8831 case 351608024: 8832 return getVersionElement(); 8833 case 3373707: 8834 return getNameElement(); 8835 case 110371416: 8836 return getTitleElement(); 8837 case -2060497896: 8838 return getSubtitleElement(); 8839 case 3575610: 8840 return getType(); 8841 case -892481550: 8842 return getStatusElement(); 8843 case -404562712: 8844 return getExperimentalElement(); 8845 case -573640748: 8846 return getSubject(); 8847 case -1867885268: 8848 return getSubject(); 8849 case 3076014: 8850 return getDateElement(); 8851 case 1447404028: 8852 return getPublisherElement(); 8853 case 951526432: 8854 return addContact(); 8855 case -1724546052: 8856 return getDescriptionElement(); 8857 case -669707736: 8858 return addUseContext(); 8859 case -507075711: 8860 return addJurisdiction(); 8861 case -220463842: 8862 return getPurposeElement(); 8863 case 111574433: 8864 return getUsageElement(); 8865 case 1522889671: 8866 return getCopyrightElement(); 8867 case 223539345: 8868 return getApprovalDateElement(); 8869 case -1687512484: 8870 return getLastReviewDateElement(); 8871 case -403934648: 8872 return getEffectivePeriod(); 8873 case 110546223: 8874 return addTopic(); 8875 case -1406328437: 8876 return addAuthor(); 8877 case -1307827859: 8878 return addEditor(); 8879 case -261190139: 8880 return addReviewer(); 8881 case 1740277666: 8882 return addEndorser(); 8883 case 666807069: 8884 return addRelatedArtifact(); 8885 case 166208699: 8886 return addLibraryElement(); 8887 case 3178259: 8888 return addGoal(); 8889 case -1422950858: 8890 return addAction(); 8891 default: 8892 return super.makeProperty(hash, name); 8893 } 8894 8895 } 8896 8897 @Override 8898 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8899 switch (hash) { 8900 case 116079: 8901 /* url */ return new String[] { "uri" }; 8902 case -1618432855: 8903 /* identifier */ return new String[] { "Identifier" }; 8904 case 351608024: 8905 /* version */ return new String[] { "string" }; 8906 case 3373707: 8907 /* name */ return new String[] { "string" }; 8908 case 110371416: 8909 /* title */ return new String[] { "string" }; 8910 case -2060497896: 8911 /* subtitle */ return new String[] { "string" }; 8912 case 3575610: 8913 /* type */ return new String[] { "CodeableConcept" }; 8914 case -892481550: 8915 /* status */ return new String[] { "code" }; 8916 case -404562712: 8917 /* experimental */ return new String[] { "boolean" }; 8918 case -1867885268: 8919 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 8920 case 3076014: 8921 /* date */ return new String[] { "dateTime" }; 8922 case 1447404028: 8923 /* publisher */ return new String[] { "string" }; 8924 case 951526432: 8925 /* contact */ return new String[] { "ContactDetail" }; 8926 case -1724546052: 8927 /* description */ return new String[] { "markdown" }; 8928 case -669707736: 8929 /* useContext */ return new String[] { "UsageContext" }; 8930 case -507075711: 8931 /* jurisdiction */ return new String[] { "CodeableConcept" }; 8932 case -220463842: 8933 /* purpose */ return new String[] { "markdown" }; 8934 case 111574433: 8935 /* usage */ return new String[] { "string" }; 8936 case 1522889671: 8937 /* copyright */ return new String[] { "markdown" }; 8938 case 223539345: 8939 /* approvalDate */ return new String[] { "date" }; 8940 case -1687512484: 8941 /* lastReviewDate */ return new String[] { "date" }; 8942 case -403934648: 8943 /* effectivePeriod */ return new String[] { "Period" }; 8944 case 110546223: 8945 /* topic */ return new String[] { "CodeableConcept" }; 8946 case -1406328437: 8947 /* author */ return new String[] { "ContactDetail" }; 8948 case -1307827859: 8949 /* editor */ return new String[] { "ContactDetail" }; 8950 case -261190139: 8951 /* reviewer */ return new String[] { "ContactDetail" }; 8952 case 1740277666: 8953 /* endorser */ return new String[] { "ContactDetail" }; 8954 case 666807069: 8955 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 8956 case 166208699: 8957 /* library */ return new String[] { "canonical" }; 8958 case 3178259: 8959 /* goal */ return new String[] {}; 8960 case -1422950858: 8961 /* action */ return new String[] {}; 8962 default: 8963 return super.getTypesForProperty(hash, name); 8964 } 8965 8966 } 8967 8968 @Override 8969 public Base addChild(String name) throws FHIRException { 8970 if (name.equals("url")) { 8971 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.url"); 8972 } else if (name.equals("identifier")) { 8973 return addIdentifier(); 8974 } else if (name.equals("version")) { 8975 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.version"); 8976 } else if (name.equals("name")) { 8977 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.name"); 8978 } else if (name.equals("title")) { 8979 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.title"); 8980 } else if (name.equals("subtitle")) { 8981 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.subtitle"); 8982 } else if (name.equals("type")) { 8983 this.type = new CodeableConcept(); 8984 return this.type; 8985 } else if (name.equals("status")) { 8986 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.status"); 8987 } else if (name.equals("experimental")) { 8988 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.experimental"); 8989 } else if (name.equals("subjectCodeableConcept")) { 8990 this.subject = new CodeableConcept(); 8991 return this.subject; 8992 } else if (name.equals("subjectReference")) { 8993 this.subject = new Reference(); 8994 return this.subject; 8995 } else if (name.equals("date")) { 8996 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.date"); 8997 } else if (name.equals("publisher")) { 8998 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.publisher"); 8999 } else if (name.equals("contact")) { 9000 return addContact(); 9001 } else if (name.equals("description")) { 9002 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.description"); 9003 } else if (name.equals("useContext")) { 9004 return addUseContext(); 9005 } else if (name.equals("jurisdiction")) { 9006 return addJurisdiction(); 9007 } else if (name.equals("purpose")) { 9008 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.purpose"); 9009 } else if (name.equals("usage")) { 9010 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.usage"); 9011 } else if (name.equals("copyright")) { 9012 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.copyright"); 9013 } else if (name.equals("approvalDate")) { 9014 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.approvalDate"); 9015 } else if (name.equals("lastReviewDate")) { 9016 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.lastReviewDate"); 9017 } else if (name.equals("effectivePeriod")) { 9018 this.effectivePeriod = new Period(); 9019 return this.effectivePeriod; 9020 } else if (name.equals("topic")) { 9021 return addTopic(); 9022 } else if (name.equals("author")) { 9023 return addAuthor(); 9024 } else if (name.equals("editor")) { 9025 return addEditor(); 9026 } else if (name.equals("reviewer")) { 9027 return addReviewer(); 9028 } else if (name.equals("endorser")) { 9029 return addEndorser(); 9030 } else if (name.equals("relatedArtifact")) { 9031 return addRelatedArtifact(); 9032 } else if (name.equals("library")) { 9033 throw new FHIRException("Cannot call addChild on a singleton property PlanDefinition.library"); 9034 } else if (name.equals("goal")) { 9035 return addGoal(); 9036 } else if (name.equals("action")) { 9037 return addAction(); 9038 } else 9039 return super.addChild(name); 9040 } 9041 9042 public String fhirType() { 9043 return "PlanDefinition"; 9044 9045 } 9046 9047 public PlanDefinition copy() { 9048 PlanDefinition dst = new PlanDefinition(); 9049 copyValues(dst); 9050 return dst; 9051 } 9052 9053 public void copyValues(PlanDefinition dst) { 9054 super.copyValues(dst); 9055 dst.url = url == null ? null : url.copy(); 9056 if (identifier != null) { 9057 dst.identifier = new ArrayList<Identifier>(); 9058 for (Identifier i : identifier) 9059 dst.identifier.add(i.copy()); 9060 } 9061 ; 9062 dst.version = version == null ? null : version.copy(); 9063 dst.name = name == null ? null : name.copy(); 9064 dst.title = title == null ? null : title.copy(); 9065 dst.subtitle = subtitle == null ? null : subtitle.copy(); 9066 dst.type = type == null ? null : type.copy(); 9067 dst.status = status == null ? null : status.copy(); 9068 dst.experimental = experimental == null ? null : experimental.copy(); 9069 dst.subject = subject == null ? null : subject.copy(); 9070 dst.date = date == null ? null : date.copy(); 9071 dst.publisher = publisher == null ? null : publisher.copy(); 9072 if (contact != null) { 9073 dst.contact = new ArrayList<ContactDetail>(); 9074 for (ContactDetail i : contact) 9075 dst.contact.add(i.copy()); 9076 } 9077 ; 9078 dst.description = description == null ? null : description.copy(); 9079 if (useContext != null) { 9080 dst.useContext = new ArrayList<UsageContext>(); 9081 for (UsageContext i : useContext) 9082 dst.useContext.add(i.copy()); 9083 } 9084 ; 9085 if (jurisdiction != null) { 9086 dst.jurisdiction = new ArrayList<CodeableConcept>(); 9087 for (CodeableConcept i : jurisdiction) 9088 dst.jurisdiction.add(i.copy()); 9089 } 9090 ; 9091 dst.purpose = purpose == null ? null : purpose.copy(); 9092 dst.usage = usage == null ? null : usage.copy(); 9093 dst.copyright = copyright == null ? null : copyright.copy(); 9094 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 9095 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 9096 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 9097 if (topic != null) { 9098 dst.topic = new ArrayList<CodeableConcept>(); 9099 for (CodeableConcept i : topic) 9100 dst.topic.add(i.copy()); 9101 } 9102 ; 9103 if (author != null) { 9104 dst.author = new ArrayList<ContactDetail>(); 9105 for (ContactDetail i : author) 9106 dst.author.add(i.copy()); 9107 } 9108 ; 9109 if (editor != null) { 9110 dst.editor = new ArrayList<ContactDetail>(); 9111 for (ContactDetail i : editor) 9112 dst.editor.add(i.copy()); 9113 } 9114 ; 9115 if (reviewer != null) { 9116 dst.reviewer = new ArrayList<ContactDetail>(); 9117 for (ContactDetail i : reviewer) 9118 dst.reviewer.add(i.copy()); 9119 } 9120 ; 9121 if (endorser != null) { 9122 dst.endorser = new ArrayList<ContactDetail>(); 9123 for (ContactDetail i : endorser) 9124 dst.endorser.add(i.copy()); 9125 } 9126 ; 9127 if (relatedArtifact != null) { 9128 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 9129 for (RelatedArtifact i : relatedArtifact) 9130 dst.relatedArtifact.add(i.copy()); 9131 } 9132 ; 9133 if (library != null) { 9134 dst.library = new ArrayList<CanonicalType>(); 9135 for (CanonicalType i : library) 9136 dst.library.add(i.copy()); 9137 } 9138 ; 9139 if (goal != null) { 9140 dst.goal = new ArrayList<PlanDefinitionGoalComponent>(); 9141 for (PlanDefinitionGoalComponent i : goal) 9142 dst.goal.add(i.copy()); 9143 } 9144 ; 9145 if (action != null) { 9146 dst.action = new ArrayList<PlanDefinitionActionComponent>(); 9147 for (PlanDefinitionActionComponent i : action) 9148 dst.action.add(i.copy()); 9149 } 9150 ; 9151 } 9152 9153 protected PlanDefinition typedCopy() { 9154 return copy(); 9155 } 9156 9157 @Override 9158 public boolean equalsDeep(Base other_) { 9159 if (!super.equalsDeep(other_)) 9160 return false; 9161 if (!(other_ instanceof PlanDefinition)) 9162 return false; 9163 PlanDefinition o = (PlanDefinition) other_; 9164 return compareDeep(identifier, o.identifier, true) && compareDeep(subtitle, o.subtitle, true) 9165 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) 9166 && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 9167 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 9168 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 9169 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 9170 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 9171 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 9172 && compareDeep(library, o.library, true) && compareDeep(goal, o.goal, true) 9173 && compareDeep(action, o.action, true); 9174 } 9175 9176 @Override 9177 public boolean equalsShallow(Base other_) { 9178 if (!super.equalsShallow(other_)) 9179 return false; 9180 if (!(other_ instanceof PlanDefinition)) 9181 return false; 9182 PlanDefinition o = (PlanDefinition) other_; 9183 return compareValues(subtitle, o.subtitle, true) && compareValues(purpose, o.purpose, true) 9184 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) 9185 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true); 9186 } 9187 9188 public boolean isEmpty() { 9189 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, subtitle, type, subject, purpose, usage, 9190 copyright, approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, 9191 relatedArtifact, library, goal, action); 9192 } 9193 9194 @Override 9195 public ResourceType getResourceType() { 9196 return ResourceType.PlanDefinition; 9197 } 9198 9199 /** 9200 * Search parameter: <b>date</b> 9201 * <p> 9202 * Description: <b>The plan definition publication date</b><br> 9203 * Type: <b>date</b><br> 9204 * Path: <b>PlanDefinition.date</b><br> 9205 * </p> 9206 */ 9207 @SearchParamDefinition(name = "date", path = "PlanDefinition.date", description = "The plan definition publication date", type = "date") 9208 public static final String SP_DATE = "date"; 9209 /** 9210 * <b>Fluent Client</b> search parameter constant for <b>date</b> 9211 * <p> 9212 * Description: <b>The plan definition publication date</b><br> 9213 * Type: <b>date</b><br> 9214 * Path: <b>PlanDefinition.date</b><br> 9215 * </p> 9216 */ 9217 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 9218 SP_DATE); 9219 9220 /** 9221 * Search parameter: <b>identifier</b> 9222 * <p> 9223 * Description: <b>External identifier for the plan definition</b><br> 9224 * Type: <b>token</b><br> 9225 * Path: <b>PlanDefinition.identifier</b><br> 9226 * </p> 9227 */ 9228 @SearchParamDefinition(name = "identifier", path = "PlanDefinition.identifier", description = "External identifier for the plan definition", type = "token") 9229 public static final String SP_IDENTIFIER = "identifier"; 9230 /** 9231 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 9232 * <p> 9233 * Description: <b>External identifier for the plan definition</b><br> 9234 * Type: <b>token</b><br> 9235 * Path: <b>PlanDefinition.identifier</b><br> 9236 * </p> 9237 */ 9238 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9239 SP_IDENTIFIER); 9240 9241 /** 9242 * Search parameter: <b>successor</b> 9243 * <p> 9244 * Description: <b>What resource is being referenced</b><br> 9245 * Type: <b>reference</b><br> 9246 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9247 * </p> 9248 */ 9249 @SearchParamDefinition(name = "successor", path = "PlanDefinition.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 9250 public static final String SP_SUCCESSOR = "successor"; 9251 /** 9252 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 9253 * <p> 9254 * Description: <b>What resource is being referenced</b><br> 9255 * Type: <b>reference</b><br> 9256 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9257 * </p> 9258 */ 9259 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9260 SP_SUCCESSOR); 9261 9262 /** 9263 * Constant for fluent queries to be used to add include statements. Specifies 9264 * the path value of "<b>PlanDefinition:successor</b>". 9265 */ 9266 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 9267 "PlanDefinition:successor").toLocked(); 9268 9269 /** 9270 * Search parameter: <b>context-type-value</b> 9271 * <p> 9272 * Description: <b>A use context type and value assigned to the plan 9273 * definition</b><br> 9274 * Type: <b>composite</b><br> 9275 * Path: <b></b><br> 9276 * </p> 9277 */ 9278 @SearchParamDefinition(name = "context-type-value", path = "PlanDefinition.useContext", description = "A use context type and value assigned to the plan definition", type = "composite", compositeOf = { 9279 "context-type", "context" }) 9280 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 9281 /** 9282 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 9283 * <p> 9284 * Description: <b>A use context type and value assigned to the plan 9285 * definition</b><br> 9286 * Type: <b>composite</b><br> 9287 * Path: <b></b><br> 9288 * </p> 9289 */ 9290 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 9291 SP_CONTEXT_TYPE_VALUE); 9292 9293 /** 9294 * Search parameter: <b>jurisdiction</b> 9295 * <p> 9296 * Description: <b>Intended jurisdiction for the plan definition</b><br> 9297 * Type: <b>token</b><br> 9298 * Path: <b>PlanDefinition.jurisdiction</b><br> 9299 * </p> 9300 */ 9301 @SearchParamDefinition(name = "jurisdiction", path = "PlanDefinition.jurisdiction", description = "Intended jurisdiction for the plan definition", type = "token") 9302 public static final String SP_JURISDICTION = "jurisdiction"; 9303 /** 9304 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 9305 * <p> 9306 * Description: <b>Intended jurisdiction for the plan definition</b><br> 9307 * Type: <b>token</b><br> 9308 * Path: <b>PlanDefinition.jurisdiction</b><br> 9309 * </p> 9310 */ 9311 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9312 SP_JURISDICTION); 9313 9314 /** 9315 * Search parameter: <b>description</b> 9316 * <p> 9317 * Description: <b>The description of the plan definition</b><br> 9318 * Type: <b>string</b><br> 9319 * Path: <b>PlanDefinition.description</b><br> 9320 * </p> 9321 */ 9322 @SearchParamDefinition(name = "description", path = "PlanDefinition.description", description = "The description of the plan definition", type = "string") 9323 public static final String SP_DESCRIPTION = "description"; 9324 /** 9325 * <b>Fluent Client</b> search parameter constant for <b>description</b> 9326 * <p> 9327 * Description: <b>The description of the plan definition</b><br> 9328 * Type: <b>string</b><br> 9329 * Path: <b>PlanDefinition.description</b><br> 9330 * </p> 9331 */ 9332 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 9333 SP_DESCRIPTION); 9334 9335 /** 9336 * Search parameter: <b>derived-from</b> 9337 * <p> 9338 * Description: <b>What resource is being referenced</b><br> 9339 * Type: <b>reference</b><br> 9340 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9341 * </p> 9342 */ 9343 @SearchParamDefinition(name = "derived-from", path = "PlanDefinition.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 9344 public static final String SP_DERIVED_FROM = "derived-from"; 9345 /** 9346 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 9347 * <p> 9348 * Description: <b>What resource is being referenced</b><br> 9349 * Type: <b>reference</b><br> 9350 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9351 * </p> 9352 */ 9353 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9354 SP_DERIVED_FROM); 9355 9356 /** 9357 * Constant for fluent queries to be used to add include statements. Specifies 9358 * the path value of "<b>PlanDefinition:derived-from</b>". 9359 */ 9360 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 9361 "PlanDefinition:derived-from").toLocked(); 9362 9363 /** 9364 * Search parameter: <b>context-type</b> 9365 * <p> 9366 * Description: <b>A type of use context assigned to the plan definition</b><br> 9367 * Type: <b>token</b><br> 9368 * Path: <b>PlanDefinition.useContext.code</b><br> 9369 * </p> 9370 */ 9371 @SearchParamDefinition(name = "context-type", path = "PlanDefinition.useContext.code", description = "A type of use context assigned to the plan definition", type = "token") 9372 public static final String SP_CONTEXT_TYPE = "context-type"; 9373 /** 9374 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 9375 * <p> 9376 * Description: <b>A type of use context assigned to the plan definition</b><br> 9377 * Type: <b>token</b><br> 9378 * Path: <b>PlanDefinition.useContext.code</b><br> 9379 * </p> 9380 */ 9381 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9382 SP_CONTEXT_TYPE); 9383 9384 /** 9385 * Search parameter: <b>predecessor</b> 9386 * <p> 9387 * Description: <b>What resource is being referenced</b><br> 9388 * Type: <b>reference</b><br> 9389 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9390 * </p> 9391 */ 9392 @SearchParamDefinition(name = "predecessor", path = "PlanDefinition.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 9393 public static final String SP_PREDECESSOR = "predecessor"; 9394 /** 9395 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 9396 * <p> 9397 * Description: <b>What resource is being referenced</b><br> 9398 * Type: <b>reference</b><br> 9399 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9400 * </p> 9401 */ 9402 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9403 SP_PREDECESSOR); 9404 9405 /** 9406 * Constant for fluent queries to be used to add include statements. Specifies 9407 * the path value of "<b>PlanDefinition:predecessor</b>". 9408 */ 9409 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 9410 "PlanDefinition:predecessor").toLocked(); 9411 9412 /** 9413 * Search parameter: <b>title</b> 9414 * <p> 9415 * Description: <b>The human-friendly name of the plan definition</b><br> 9416 * Type: <b>string</b><br> 9417 * Path: <b>PlanDefinition.title</b><br> 9418 * </p> 9419 */ 9420 @SearchParamDefinition(name = "title", path = "PlanDefinition.title", description = "The human-friendly name of the plan definition", type = "string") 9421 public static final String SP_TITLE = "title"; 9422 /** 9423 * <b>Fluent Client</b> search parameter constant for <b>title</b> 9424 * <p> 9425 * Description: <b>The human-friendly name of the plan definition</b><br> 9426 * Type: <b>string</b><br> 9427 * Path: <b>PlanDefinition.title</b><br> 9428 * </p> 9429 */ 9430 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 9431 SP_TITLE); 9432 9433 /** 9434 * Search parameter: <b>composed-of</b> 9435 * <p> 9436 * Description: <b>What resource is being referenced</b><br> 9437 * Type: <b>reference</b><br> 9438 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9439 * </p> 9440 */ 9441 @SearchParamDefinition(name = "composed-of", path = "PlanDefinition.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 9442 public static final String SP_COMPOSED_OF = "composed-of"; 9443 /** 9444 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 9445 * <p> 9446 * Description: <b>What resource is being referenced</b><br> 9447 * Type: <b>reference</b><br> 9448 * Path: <b>PlanDefinition.relatedArtifact.resource</b><br> 9449 * </p> 9450 */ 9451 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9452 SP_COMPOSED_OF); 9453 9454 /** 9455 * Constant for fluent queries to be used to add include statements. Specifies 9456 * the path value of "<b>PlanDefinition:composed-of</b>". 9457 */ 9458 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 9459 "PlanDefinition:composed-of").toLocked(); 9460 9461 /** 9462 * Search parameter: <b>type</b> 9463 * <p> 9464 * Description: <b>The type of artifact the plan (e.g. order-set, eca-rule, 9465 * protocol)</b><br> 9466 * Type: <b>token</b><br> 9467 * Path: <b>PlanDefinition.type</b><br> 9468 * </p> 9469 */ 9470 @SearchParamDefinition(name = "type", path = "PlanDefinition.type", description = "The type of artifact the plan (e.g. order-set, eca-rule, protocol)", type = "token") 9471 public static final String SP_TYPE = "type"; 9472 /** 9473 * <b>Fluent Client</b> search parameter constant for <b>type</b> 9474 * <p> 9475 * Description: <b>The type of artifact the plan (e.g. order-set, eca-rule, 9476 * protocol)</b><br> 9477 * Type: <b>token</b><br> 9478 * Path: <b>PlanDefinition.type</b><br> 9479 * </p> 9480 */ 9481 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9482 SP_TYPE); 9483 9484 /** 9485 * Search parameter: <b>version</b> 9486 * <p> 9487 * Description: <b>The business version of the plan definition</b><br> 9488 * Type: <b>token</b><br> 9489 * Path: <b>PlanDefinition.version</b><br> 9490 * </p> 9491 */ 9492 @SearchParamDefinition(name = "version", path = "PlanDefinition.version", description = "The business version of the plan definition", type = "token") 9493 public static final String SP_VERSION = "version"; 9494 /** 9495 * <b>Fluent Client</b> search parameter constant for <b>version</b> 9496 * <p> 9497 * Description: <b>The business version of the plan definition</b><br> 9498 * Type: <b>token</b><br> 9499 * Path: <b>PlanDefinition.version</b><br> 9500 * </p> 9501 */ 9502 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9503 SP_VERSION); 9504 9505 /** 9506 * Search parameter: <b>url</b> 9507 * <p> 9508 * Description: <b>The uri that identifies the plan definition</b><br> 9509 * Type: <b>uri</b><br> 9510 * Path: <b>PlanDefinition.url</b><br> 9511 * </p> 9512 */ 9513 @SearchParamDefinition(name = "url", path = "PlanDefinition.url", description = "The uri that identifies the plan definition", type = "uri") 9514 public static final String SP_URL = "url"; 9515 /** 9516 * <b>Fluent Client</b> search parameter constant for <b>url</b> 9517 * <p> 9518 * Description: <b>The uri that identifies the plan definition</b><br> 9519 * Type: <b>uri</b><br> 9520 * Path: <b>PlanDefinition.url</b><br> 9521 * </p> 9522 */ 9523 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 9524 9525 /** 9526 * Search parameter: <b>context-quantity</b> 9527 * <p> 9528 * Description: <b>A quantity- or range-valued use context assigned to the plan 9529 * definition</b><br> 9530 * Type: <b>quantity</b><br> 9531 * Path: <b>PlanDefinition.useContext.valueQuantity, 9532 * PlanDefinition.useContext.valueRange</b><br> 9533 * </p> 9534 */ 9535 @SearchParamDefinition(name = "context-quantity", path = "(PlanDefinition.useContext.value as Quantity) | (PlanDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the plan definition", type = "quantity") 9536 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 9537 /** 9538 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 9539 * <p> 9540 * Description: <b>A quantity- or range-valued use context assigned to the plan 9541 * definition</b><br> 9542 * Type: <b>quantity</b><br> 9543 * Path: <b>PlanDefinition.useContext.valueQuantity, 9544 * PlanDefinition.useContext.valueRange</b><br> 9545 * </p> 9546 */ 9547 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 9548 SP_CONTEXT_QUANTITY); 9549 9550 /** 9551 * Search parameter: <b>effective</b> 9552 * <p> 9553 * Description: <b>The time during which the plan definition is intended to be 9554 * in use</b><br> 9555 * Type: <b>date</b><br> 9556 * Path: <b>PlanDefinition.effectivePeriod</b><br> 9557 * </p> 9558 */ 9559 @SearchParamDefinition(name = "effective", path = "PlanDefinition.effectivePeriod", description = "The time during which the plan definition is intended to be in use", type = "date") 9560 public static final String SP_EFFECTIVE = "effective"; 9561 /** 9562 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 9563 * <p> 9564 * Description: <b>The time during which the plan definition is intended to be 9565 * in use</b><br> 9566 * Type: <b>date</b><br> 9567 * Path: <b>PlanDefinition.effectivePeriod</b><br> 9568 * </p> 9569 */ 9570 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 9571 SP_EFFECTIVE); 9572 9573 /** 9574 * Search parameter: <b>depends-on</b> 9575 * <p> 9576 * Description: <b>What resource is being referenced</b><br> 9577 * Type: <b>reference</b><br> 9578 * Path: <b>PlanDefinition.relatedArtifact.resource, 9579 * PlanDefinition.library</b><br> 9580 * </p> 9581 */ 9582 @SearchParamDefinition(name = "depends-on", path = "PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description = "What resource is being referenced", type = "reference") 9583 public static final String SP_DEPENDS_ON = "depends-on"; 9584 /** 9585 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 9586 * <p> 9587 * Description: <b>What resource is being referenced</b><br> 9588 * Type: <b>reference</b><br> 9589 * Path: <b>PlanDefinition.relatedArtifact.resource, 9590 * PlanDefinition.library</b><br> 9591 * </p> 9592 */ 9593 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9594 SP_DEPENDS_ON); 9595 9596 /** 9597 * Constant for fluent queries to be used to add include statements. Specifies 9598 * the path value of "<b>PlanDefinition:depends-on</b>". 9599 */ 9600 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 9601 "PlanDefinition:depends-on").toLocked(); 9602 9603 /** 9604 * Search parameter: <b>name</b> 9605 * <p> 9606 * Description: <b>Computationally friendly name of the plan definition</b><br> 9607 * Type: <b>string</b><br> 9608 * Path: <b>PlanDefinition.name</b><br> 9609 * </p> 9610 */ 9611 @SearchParamDefinition(name = "name", path = "PlanDefinition.name", description = "Computationally friendly name of the plan definition", type = "string") 9612 public static final String SP_NAME = "name"; 9613 /** 9614 * <b>Fluent Client</b> search parameter constant for <b>name</b> 9615 * <p> 9616 * Description: <b>Computationally friendly name of the plan definition</b><br> 9617 * Type: <b>string</b><br> 9618 * Path: <b>PlanDefinition.name</b><br> 9619 * </p> 9620 */ 9621 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 9622 SP_NAME); 9623 9624 /** 9625 * Search parameter: <b>context</b> 9626 * <p> 9627 * Description: <b>A use context assigned to the plan definition</b><br> 9628 * Type: <b>token</b><br> 9629 * Path: <b>PlanDefinition.useContext.valueCodeableConcept</b><br> 9630 * </p> 9631 */ 9632 @SearchParamDefinition(name = "context", path = "(PlanDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the plan definition", type = "token") 9633 public static final String SP_CONTEXT = "context"; 9634 /** 9635 * <b>Fluent Client</b> search parameter constant for <b>context</b> 9636 * <p> 9637 * Description: <b>A use context assigned to the plan definition</b><br> 9638 * Type: <b>token</b><br> 9639 * Path: <b>PlanDefinition.useContext.valueCodeableConcept</b><br> 9640 * </p> 9641 */ 9642 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9643 SP_CONTEXT); 9644 9645 /** 9646 * Search parameter: <b>publisher</b> 9647 * <p> 9648 * Description: <b>Name of the publisher of the plan definition</b><br> 9649 * Type: <b>string</b><br> 9650 * Path: <b>PlanDefinition.publisher</b><br> 9651 * </p> 9652 */ 9653 @SearchParamDefinition(name = "publisher", path = "PlanDefinition.publisher", description = "Name of the publisher of the plan definition", type = "string") 9654 public static final String SP_PUBLISHER = "publisher"; 9655 /** 9656 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 9657 * <p> 9658 * Description: <b>Name of the publisher of the plan definition</b><br> 9659 * Type: <b>string</b><br> 9660 * Path: <b>PlanDefinition.publisher</b><br> 9661 * </p> 9662 */ 9663 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 9664 SP_PUBLISHER); 9665 9666 /** 9667 * Search parameter: <b>topic</b> 9668 * <p> 9669 * Description: <b>Topics associated with the module</b><br> 9670 * Type: <b>token</b><br> 9671 * Path: <b>PlanDefinition.topic</b><br> 9672 * </p> 9673 */ 9674 @SearchParamDefinition(name = "topic", path = "PlanDefinition.topic", description = "Topics associated with the module", type = "token") 9675 public static final String SP_TOPIC = "topic"; 9676 /** 9677 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 9678 * <p> 9679 * Description: <b>Topics associated with the module</b><br> 9680 * Type: <b>token</b><br> 9681 * Path: <b>PlanDefinition.topic</b><br> 9682 * </p> 9683 */ 9684 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9685 SP_TOPIC); 9686 9687 /** 9688 * Search parameter: <b>definition</b> 9689 * <p> 9690 * Description: <b>Activity or plan definitions used by plan definition</b><br> 9691 * Type: <b>reference</b><br> 9692 * Path: <b>PlanDefinition.action.definition[x]</b><br> 9693 * </p> 9694 */ 9695 @SearchParamDefinition(name = "definition", path = "PlanDefinition.action.definition", description = "Activity or plan definitions used by plan definition", type = "reference", target = { 9696 ActivityDefinition.class, PlanDefinition.class, Questionnaire.class }) 9697 public static final String SP_DEFINITION = "definition"; 9698 /** 9699 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 9700 * <p> 9701 * Description: <b>Activity or plan definitions used by plan definition</b><br> 9702 * Type: <b>reference</b><br> 9703 * Path: <b>PlanDefinition.action.definition[x]</b><br> 9704 * </p> 9705 */ 9706 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 9707 SP_DEFINITION); 9708 9709 /** 9710 * Constant for fluent queries to be used to add include statements. Specifies 9711 * the path value of "<b>PlanDefinition:definition</b>". 9712 */ 9713 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include( 9714 "PlanDefinition:definition").toLocked(); 9715 9716 /** 9717 * Search parameter: <b>context-type-quantity</b> 9718 * <p> 9719 * Description: <b>A use context type and quantity- or range-based value 9720 * assigned to the plan definition</b><br> 9721 * Type: <b>composite</b><br> 9722 * Path: <b></b><br> 9723 * </p> 9724 */ 9725 @SearchParamDefinition(name = "context-type-quantity", path = "PlanDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the plan definition", type = "composite", compositeOf = { 9726 "context-type", "context-quantity" }) 9727 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 9728 /** 9729 * <b>Fluent Client</b> search parameter constant for 9730 * <b>context-type-quantity</b> 9731 * <p> 9732 * Description: <b>A use context type and quantity- or range-based value 9733 * assigned to the plan definition</b><br> 9734 * Type: <b>composite</b><br> 9735 * Path: <b></b><br> 9736 * </p> 9737 */ 9738 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 9739 SP_CONTEXT_TYPE_QUANTITY); 9740 9741 /** 9742 * Search parameter: <b>status</b> 9743 * <p> 9744 * Description: <b>The current status of the plan definition</b><br> 9745 * Type: <b>token</b><br> 9746 * Path: <b>PlanDefinition.status</b><br> 9747 * </p> 9748 */ 9749 @SearchParamDefinition(name = "status", path = "PlanDefinition.status", description = "The current status of the plan definition", type = "token") 9750 public static final String SP_STATUS = "status"; 9751 /** 9752 * <b>Fluent Client</b> search parameter constant for <b>status</b> 9753 * <p> 9754 * Description: <b>The current status of the plan definition</b><br> 9755 * Type: <b>token</b><br> 9756 * Path: <b>PlanDefinition.status</b><br> 9757 * </p> 9758 */ 9759 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9760 SP_STATUS); 9761 9762}