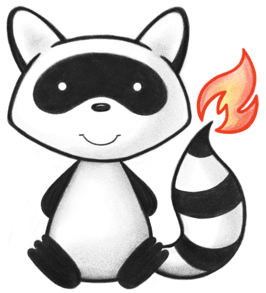
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * A person who is directly or indirectly involved in the provisioning of 050 * healthcare. 051 */ 052@ResourceDef(name = "Practitioner", profile = "http://hl7.org/fhir/StructureDefinition/Practitioner") 053public class Practitioner extends DomainResource { 054 055 @Block() 056 public static class PractitionerQualificationComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * An identifier that applies to this person's qualification in this role. 059 */ 060 @Child(name = "identifier", type = { 061 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 062 @Description(shortDefinition = "An identifier for this qualification for the practitioner", formalDefinition = "An identifier that applies to this person's qualification in this role.") 063 protected List<Identifier> identifier; 064 065 /** 066 * Coded representation of the qualification. 067 */ 068 @Child(name = "code", type = { 069 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 070 @Description(shortDefinition = "Coded representation of the qualification", formalDefinition = "Coded representation of the qualification.") 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-2.7-0360") 072 protected CodeableConcept code; 073 074 /** 075 * Period during which the qualification is valid. 076 */ 077 @Child(name = "period", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 078 @Description(shortDefinition = "Period during which the qualification is valid", formalDefinition = "Period during which the qualification is valid.") 079 protected Period period; 080 081 /** 082 * Organization that regulates and issues the qualification. 083 */ 084 @Child(name = "issuer", type = { 085 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 086 @Description(shortDefinition = "Organization that regulates and issues the qualification", formalDefinition = "Organization that regulates and issues the qualification.") 087 protected Reference issuer; 088 089 /** 090 * The actual object that is the target of the reference (Organization that 091 * regulates and issues the qualification.) 092 */ 093 protected Organization issuerTarget; 094 095 private static final long serialVersionUID = 1095219071L; 096 097 /** 098 * Constructor 099 */ 100 public PractitionerQualificationComponent() { 101 super(); 102 } 103 104 /** 105 * Constructor 106 */ 107 public PractitionerQualificationComponent(CodeableConcept code) { 108 super(); 109 this.code = code; 110 } 111 112 /** 113 * @return {@link #identifier} (An identifier that applies to this person's 114 * qualification in this role.) 115 */ 116 public List<Identifier> getIdentifier() { 117 if (this.identifier == null) 118 this.identifier = new ArrayList<Identifier>(); 119 return this.identifier; 120 } 121 122 /** 123 * @return Returns a reference to <code>this</code> for easy method chaining 124 */ 125 public PractitionerQualificationComponent setIdentifier(List<Identifier> theIdentifier) { 126 this.identifier = theIdentifier; 127 return this; 128 } 129 130 public boolean hasIdentifier() { 131 if (this.identifier == null) 132 return false; 133 for (Identifier item : this.identifier) 134 if (!item.isEmpty()) 135 return true; 136 return false; 137 } 138 139 public Identifier addIdentifier() { // 3 140 Identifier t = new Identifier(); 141 if (this.identifier == null) 142 this.identifier = new ArrayList<Identifier>(); 143 this.identifier.add(t); 144 return t; 145 } 146 147 public PractitionerQualificationComponent addIdentifier(Identifier t) { // 3 148 if (t == null) 149 return this; 150 if (this.identifier == null) 151 this.identifier = new ArrayList<Identifier>(); 152 this.identifier.add(t); 153 return this; 154 } 155 156 /** 157 * @return The first repetition of repeating field {@link #identifier}, creating 158 * it if it does not already exist 159 */ 160 public Identifier getIdentifierFirstRep() { 161 if (getIdentifier().isEmpty()) { 162 addIdentifier(); 163 } 164 return getIdentifier().get(0); 165 } 166 167 /** 168 * @return {@link #code} (Coded representation of the qualification.) 169 */ 170 public CodeableConcept getCode() { 171 if (this.code == null) 172 if (Configuration.errorOnAutoCreate()) 173 throw new Error("Attempt to auto-create PractitionerQualificationComponent.code"); 174 else if (Configuration.doAutoCreate()) 175 this.code = new CodeableConcept(); // cc 176 return this.code; 177 } 178 179 public boolean hasCode() { 180 return this.code != null && !this.code.isEmpty(); 181 } 182 183 /** 184 * @param value {@link #code} (Coded representation of the qualification.) 185 */ 186 public PractitionerQualificationComponent setCode(CodeableConcept value) { 187 this.code = value; 188 return this; 189 } 190 191 /** 192 * @return {@link #period} (Period during which the qualification is valid.) 193 */ 194 public Period getPeriod() { 195 if (this.period == null) 196 if (Configuration.errorOnAutoCreate()) 197 throw new Error("Attempt to auto-create PractitionerQualificationComponent.period"); 198 else if (Configuration.doAutoCreate()) 199 this.period = new Period(); // cc 200 return this.period; 201 } 202 203 public boolean hasPeriod() { 204 return this.period != null && !this.period.isEmpty(); 205 } 206 207 /** 208 * @param value {@link #period} (Period during which the qualification is 209 * valid.) 210 */ 211 public PractitionerQualificationComponent setPeriod(Period value) { 212 this.period = value; 213 return this; 214 } 215 216 /** 217 * @return {@link #issuer} (Organization that regulates and issues the 218 * qualification.) 219 */ 220 public Reference getIssuer() { 221 if (this.issuer == null) 222 if (Configuration.errorOnAutoCreate()) 223 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 224 else if (Configuration.doAutoCreate()) 225 this.issuer = new Reference(); // cc 226 return this.issuer; 227 } 228 229 public boolean hasIssuer() { 230 return this.issuer != null && !this.issuer.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #issuer} (Organization that regulates and issues the 235 * qualification.) 236 */ 237 public PractitionerQualificationComponent setIssuer(Reference value) { 238 this.issuer = value; 239 return this; 240 } 241 242 /** 243 * @return {@link #issuer} The actual object that is the target of the 244 * reference. The reference library doesn't populate this, but you can 245 * use it to hold the resource if you resolve it. (Organization that 246 * regulates and issues the qualification.) 247 */ 248 public Organization getIssuerTarget() { 249 if (this.issuerTarget == null) 250 if (Configuration.errorOnAutoCreate()) 251 throw new Error("Attempt to auto-create PractitionerQualificationComponent.issuer"); 252 else if (Configuration.doAutoCreate()) 253 this.issuerTarget = new Organization(); // aa 254 return this.issuerTarget; 255 } 256 257 /** 258 * @param value {@link #issuer} The actual object that is the target of the 259 * reference. The reference library doesn't use these, but you can 260 * use it to hold the resource if you resolve it. (Organization 261 * that regulates and issues the qualification.) 262 */ 263 public PractitionerQualificationComponent setIssuerTarget(Organization value) { 264 this.issuerTarget = value; 265 return this; 266 } 267 268 protected void listChildren(List<Property> children) { 269 super.listChildren(children); 270 children.add(new Property("identifier", "Identifier", 271 "An identifier that applies to this person's qualification in this role.", 0, java.lang.Integer.MAX_VALUE, 272 identifier)); 273 children.add(new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, code)); 274 children.add(new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, period)); 275 children.add(new Property("issuer", "Reference(Organization)", 276 "Organization that regulates and issues the qualification.", 0, 1, issuer)); 277 } 278 279 @Override 280 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 281 switch (_hash) { 282 case -1618432855: 283 /* identifier */ return new Property("identifier", "Identifier", 284 "An identifier that applies to this person's qualification in this role.", 0, java.lang.Integer.MAX_VALUE, 285 identifier); 286 case 3059181: 287 /* code */ return new Property("code", "CodeableConcept", "Coded representation of the qualification.", 0, 1, 288 code); 289 case -991726143: 290 /* period */ return new Property("period", "Period", "Period during which the qualification is valid.", 0, 1, 291 period); 292 case -1179159879: 293 /* issuer */ return new Property("issuer", "Reference(Organization)", 294 "Organization that regulates and issues the qualification.", 0, 1, issuer); 295 default: 296 return super.getNamedProperty(_hash, _name, _checkValid); 297 } 298 299 } 300 301 @Override 302 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 303 switch (hash) { 304 case -1618432855: 305 /* identifier */ return this.identifier == null ? new Base[0] 306 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 307 case 3059181: 308 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 309 case -991726143: 310 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 311 case -1179159879: 312 /* issuer */ return this.issuer == null ? new Base[0] : new Base[] { this.issuer }; // Reference 313 default: 314 return super.getProperty(hash, name, checkValid); 315 } 316 317 } 318 319 @Override 320 public Base setProperty(int hash, String name, Base value) throws FHIRException { 321 switch (hash) { 322 case -1618432855: // identifier 323 this.getIdentifier().add(castToIdentifier(value)); // Identifier 324 return value; 325 case 3059181: // code 326 this.code = castToCodeableConcept(value); // CodeableConcept 327 return value; 328 case -991726143: // period 329 this.period = castToPeriod(value); // Period 330 return value; 331 case -1179159879: // issuer 332 this.issuer = castToReference(value); // Reference 333 return value; 334 default: 335 return super.setProperty(hash, name, value); 336 } 337 338 } 339 340 @Override 341 public Base setProperty(String name, Base value) throws FHIRException { 342 if (name.equals("identifier")) { 343 this.getIdentifier().add(castToIdentifier(value)); 344 } else if (name.equals("code")) { 345 this.code = castToCodeableConcept(value); // CodeableConcept 346 } else if (name.equals("period")) { 347 this.period = castToPeriod(value); // Period 348 } else if (name.equals("issuer")) { 349 this.issuer = castToReference(value); // Reference 350 } else 351 return super.setProperty(name, value); 352 return value; 353 } 354 355 @Override 356 public void removeChild(String name, Base value) throws FHIRException { 357 if (name.equals("identifier")) { 358 this.getIdentifier().remove(castToIdentifier(value)); 359 } else if (name.equals("code")) { 360 this.code = null; 361 } else if (name.equals("period")) { 362 this.period = null; 363 } else if (name.equals("issuer")) { 364 this.issuer = null; 365 } else 366 super.removeChild(name, value); 367 368 } 369 370 @Override 371 public Base makeProperty(int hash, String name) throws FHIRException { 372 switch (hash) { 373 case -1618432855: 374 return addIdentifier(); 375 case 3059181: 376 return getCode(); 377 case -991726143: 378 return getPeriod(); 379 case -1179159879: 380 return getIssuer(); 381 default: 382 return super.makeProperty(hash, name); 383 } 384 385 } 386 387 @Override 388 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 389 switch (hash) { 390 case -1618432855: 391 /* identifier */ return new String[] { "Identifier" }; 392 case 3059181: 393 /* code */ return new String[] { "CodeableConcept" }; 394 case -991726143: 395 /* period */ return new String[] { "Period" }; 396 case -1179159879: 397 /* issuer */ return new String[] { "Reference" }; 398 default: 399 return super.getTypesForProperty(hash, name); 400 } 401 402 } 403 404 @Override 405 public Base addChild(String name) throws FHIRException { 406 if (name.equals("identifier")) { 407 return addIdentifier(); 408 } else if (name.equals("code")) { 409 this.code = new CodeableConcept(); 410 return this.code; 411 } else if (name.equals("period")) { 412 this.period = new Period(); 413 return this.period; 414 } else if (name.equals("issuer")) { 415 this.issuer = new Reference(); 416 return this.issuer; 417 } else 418 return super.addChild(name); 419 } 420 421 public PractitionerQualificationComponent copy() { 422 PractitionerQualificationComponent dst = new PractitionerQualificationComponent(); 423 copyValues(dst); 424 return dst; 425 } 426 427 public void copyValues(PractitionerQualificationComponent dst) { 428 super.copyValues(dst); 429 if (identifier != null) { 430 dst.identifier = new ArrayList<Identifier>(); 431 for (Identifier i : identifier) 432 dst.identifier.add(i.copy()); 433 } 434 ; 435 dst.code = code == null ? null : code.copy(); 436 dst.period = period == null ? null : period.copy(); 437 dst.issuer = issuer == null ? null : issuer.copy(); 438 } 439 440 @Override 441 public boolean equalsDeep(Base other_) { 442 if (!super.equalsDeep(other_)) 443 return false; 444 if (!(other_ instanceof PractitionerQualificationComponent)) 445 return false; 446 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other_; 447 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 448 && compareDeep(period, o.period, true) && compareDeep(issuer, o.issuer, true); 449 } 450 451 @Override 452 public boolean equalsShallow(Base other_) { 453 if (!super.equalsShallow(other_)) 454 return false; 455 if (!(other_ instanceof PractitionerQualificationComponent)) 456 return false; 457 PractitionerQualificationComponent o = (PractitionerQualificationComponent) other_; 458 return true; 459 } 460 461 public boolean isEmpty() { 462 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, period, issuer); 463 } 464 465 public String fhirType() { 466 return "Practitioner.qualification"; 467 468 } 469 470 } 471 472 /** 473 * An identifier that applies to this person in this role. 474 */ 475 @Child(name = "identifier", type = { 476 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 477 @Description(shortDefinition = "An identifier for the person as this agent", formalDefinition = "An identifier that applies to this person in this role.") 478 protected List<Identifier> identifier; 479 480 /** 481 * Whether this practitioner's record is in active use. 482 */ 483 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 484 @Description(shortDefinition = "Whether this practitioner's record is in active use", formalDefinition = "Whether this practitioner's record is in active use.") 485 protected BooleanType active; 486 487 /** 488 * The name(s) associated with the practitioner. 489 */ 490 @Child(name = "name", type = { 491 HumanName.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 492 @Description(shortDefinition = "The name(s) associated with the practitioner", formalDefinition = "The name(s) associated with the practitioner.") 493 protected List<HumanName> name; 494 495 /** 496 * A contact detail for the practitioner, e.g. a telephone number or an email 497 * address. 498 */ 499 @Child(name = "telecom", type = { 500 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 501 @Description(shortDefinition = "A contact detail for the practitioner (that apply to all roles)", formalDefinition = "A contact detail for the practitioner, e.g. a telephone number or an email address.") 502 protected List<ContactPoint> telecom; 503 504 /** 505 * Address(es) of the practitioner that are not role specific (typically home 506 * address). Work addresses are not typically entered in this property as they 507 * are usually role dependent. 508 */ 509 @Child(name = "address", type = { 510 Address.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 511 @Description(shortDefinition = "Address(es) of the practitioner that are not role specific (typically home address)", formalDefinition = "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.") 512 protected List<Address> address; 513 514 /** 515 * Administrative Gender - the gender that the person is considered to have for 516 * administration and record keeping purposes. 517 */ 518 @Child(name = "gender", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 519 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.") 520 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 521 protected Enumeration<AdministrativeGender> gender; 522 523 /** 524 * The date of birth for the practitioner. 525 */ 526 @Child(name = "birthDate", type = { DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 527 @Description(shortDefinition = "The date on which the practitioner was born", formalDefinition = "The date of birth for the practitioner.") 528 protected DateType birthDate; 529 530 /** 531 * Image of the person. 532 */ 533 @Child(name = "photo", type = { 534 Attachment.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 535 @Description(shortDefinition = "Image of the person", formalDefinition = "Image of the person.") 536 protected List<Attachment> photo; 537 538 /** 539 * The official certifications, training, and licenses that authorize or 540 * otherwise pertain to the provision of care by the practitioner. For example, 541 * a medical license issued by a medical board authorizing the practitioner to 542 * practice medicine within a certian locality. 543 */ 544 @Child(name = "qualification", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 545 @Description(shortDefinition = "Certification, licenses, or training pertaining to the provision of care", formalDefinition = "The official certifications, training, and licenses that authorize or otherwise pertain to the provision of care by the practitioner. For example, a medical license issued by a medical board authorizing the practitioner to practice medicine within a certian locality.") 546 protected List<PractitionerQualificationComponent> qualification; 547 548 /** 549 * A language the practitioner can use in patient communication. 550 */ 551 @Child(name = "communication", type = { 552 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 553 @Description(shortDefinition = "A language the practitioner can use in patient communication", formalDefinition = "A language the practitioner can use in patient communication.") 554 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 555 protected List<CodeableConcept> communication; 556 557 private static final long serialVersionUID = 2128349259L; 558 559 /** 560 * Constructor 561 */ 562 public Practitioner() { 563 super(); 564 } 565 566 /** 567 * @return {@link #identifier} (An identifier that applies to this person in 568 * this role.) 569 */ 570 public List<Identifier> getIdentifier() { 571 if (this.identifier == null) 572 this.identifier = new ArrayList<Identifier>(); 573 return this.identifier; 574 } 575 576 /** 577 * @return Returns a reference to <code>this</code> for easy method chaining 578 */ 579 public Practitioner setIdentifier(List<Identifier> theIdentifier) { 580 this.identifier = theIdentifier; 581 return this; 582 } 583 584 public boolean hasIdentifier() { 585 if (this.identifier == null) 586 return false; 587 for (Identifier item : this.identifier) 588 if (!item.isEmpty()) 589 return true; 590 return false; 591 } 592 593 public Identifier addIdentifier() { // 3 594 Identifier t = new Identifier(); 595 if (this.identifier == null) 596 this.identifier = new ArrayList<Identifier>(); 597 this.identifier.add(t); 598 return t; 599 } 600 601 public Practitioner addIdentifier(Identifier t) { // 3 602 if (t == null) 603 return this; 604 if (this.identifier == null) 605 this.identifier = new ArrayList<Identifier>(); 606 this.identifier.add(t); 607 return this; 608 } 609 610 /** 611 * @return The first repetition of repeating field {@link #identifier}, creating 612 * it if it does not already exist 613 */ 614 public Identifier getIdentifierFirstRep() { 615 if (getIdentifier().isEmpty()) { 616 addIdentifier(); 617 } 618 return getIdentifier().get(0); 619 } 620 621 /** 622 * @return {@link #active} (Whether this practitioner's record is in active 623 * use.). This is the underlying object with id, value and extensions. 624 * The accessor "getActive" gives direct access to the value 625 */ 626 public BooleanType getActiveElement() { 627 if (this.active == null) 628 if (Configuration.errorOnAutoCreate()) 629 throw new Error("Attempt to auto-create Practitioner.active"); 630 else if (Configuration.doAutoCreate()) 631 this.active = new BooleanType(); // bb 632 return this.active; 633 } 634 635 public boolean hasActiveElement() { 636 return this.active != null && !this.active.isEmpty(); 637 } 638 639 public boolean hasActive() { 640 return this.active != null && !this.active.isEmpty(); 641 } 642 643 /** 644 * @param value {@link #active} (Whether this practitioner's record is in active 645 * use.). This is the underlying object with id, value and 646 * extensions. The accessor "getActive" gives direct access to the 647 * value 648 */ 649 public Practitioner setActiveElement(BooleanType value) { 650 this.active = value; 651 return this; 652 } 653 654 /** 655 * @return Whether this practitioner's record is in active use. 656 */ 657 public boolean getActive() { 658 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 659 } 660 661 /** 662 * @param value Whether this practitioner's record is in active use. 663 */ 664 public Practitioner setActive(boolean value) { 665 if (this.active == null) 666 this.active = new BooleanType(); 667 this.active.setValue(value); 668 return this; 669 } 670 671 /** 672 * @return {@link #name} (The name(s) associated with the practitioner.) 673 */ 674 public List<HumanName> getName() { 675 if (this.name == null) 676 this.name = new ArrayList<HumanName>(); 677 return this.name; 678 } 679 680 /** 681 * @return Returns a reference to <code>this</code> for easy method chaining 682 */ 683 public Practitioner setName(List<HumanName> theName) { 684 this.name = theName; 685 return this; 686 } 687 688 public boolean hasName() { 689 if (this.name == null) 690 return false; 691 for (HumanName item : this.name) 692 if (!item.isEmpty()) 693 return true; 694 return false; 695 } 696 697 public HumanName addName() { // 3 698 HumanName t = new HumanName(); 699 if (this.name == null) 700 this.name = new ArrayList<HumanName>(); 701 this.name.add(t); 702 return t; 703 } 704 705 public Practitioner addName(HumanName t) { // 3 706 if (t == null) 707 return this; 708 if (this.name == null) 709 this.name = new ArrayList<HumanName>(); 710 this.name.add(t); 711 return this; 712 } 713 714 /** 715 * @return The first repetition of repeating field {@link #name}, creating it if 716 * it does not already exist 717 */ 718 public HumanName getNameFirstRep() { 719 if (getName().isEmpty()) { 720 addName(); 721 } 722 return getName().get(0); 723 } 724 725 /** 726 * @return {@link #telecom} (A contact detail for the practitioner, e.g. a 727 * telephone number or an email address.) 728 */ 729 public List<ContactPoint> getTelecom() { 730 if (this.telecom == null) 731 this.telecom = new ArrayList<ContactPoint>(); 732 return this.telecom; 733 } 734 735 /** 736 * @return Returns a reference to <code>this</code> for easy method chaining 737 */ 738 public Practitioner setTelecom(List<ContactPoint> theTelecom) { 739 this.telecom = theTelecom; 740 return this; 741 } 742 743 public boolean hasTelecom() { 744 if (this.telecom == null) 745 return false; 746 for (ContactPoint item : this.telecom) 747 if (!item.isEmpty()) 748 return true; 749 return false; 750 } 751 752 public ContactPoint addTelecom() { // 3 753 ContactPoint t = new ContactPoint(); 754 if (this.telecom == null) 755 this.telecom = new ArrayList<ContactPoint>(); 756 this.telecom.add(t); 757 return t; 758 } 759 760 public Practitioner addTelecom(ContactPoint t) { // 3 761 if (t == null) 762 return this; 763 if (this.telecom == null) 764 this.telecom = new ArrayList<ContactPoint>(); 765 this.telecom.add(t); 766 return this; 767 } 768 769 /** 770 * @return The first repetition of repeating field {@link #telecom}, creating it 771 * if it does not already exist 772 */ 773 public ContactPoint getTelecomFirstRep() { 774 if (getTelecom().isEmpty()) { 775 addTelecom(); 776 } 777 return getTelecom().get(0); 778 } 779 780 /** 781 * @return {@link #address} (Address(es) of the practitioner that are not role 782 * specific (typically home address). Work addresses are not typically 783 * entered in this property as they are usually role dependent.) 784 */ 785 public List<Address> getAddress() { 786 if (this.address == null) 787 this.address = new ArrayList<Address>(); 788 return this.address; 789 } 790 791 /** 792 * @return Returns a reference to <code>this</code> for easy method chaining 793 */ 794 public Practitioner setAddress(List<Address> theAddress) { 795 this.address = theAddress; 796 return this; 797 } 798 799 public boolean hasAddress() { 800 if (this.address == null) 801 return false; 802 for (Address item : this.address) 803 if (!item.isEmpty()) 804 return true; 805 return false; 806 } 807 808 public Address addAddress() { // 3 809 Address t = new Address(); 810 if (this.address == null) 811 this.address = new ArrayList<Address>(); 812 this.address.add(t); 813 return t; 814 } 815 816 public Practitioner addAddress(Address t) { // 3 817 if (t == null) 818 return this; 819 if (this.address == null) 820 this.address = new ArrayList<Address>(); 821 this.address.add(t); 822 return this; 823 } 824 825 /** 826 * @return The first repetition of repeating field {@link #address}, creating it 827 * if it does not already exist 828 */ 829 public Address getAddressFirstRep() { 830 if (getAddress().isEmpty()) { 831 addAddress(); 832 } 833 return getAddress().get(0); 834 } 835 836 /** 837 * @return {@link #gender} (Administrative Gender - the gender that the person 838 * is considered to have for administration and record keeping 839 * purposes.). This is the underlying object with id, value and 840 * extensions. The accessor "getGender" gives direct access to the value 841 */ 842 public Enumeration<AdministrativeGender> getGenderElement() { 843 if (this.gender == null) 844 if (Configuration.errorOnAutoCreate()) 845 throw new Error("Attempt to auto-create Practitioner.gender"); 846 else if (Configuration.doAutoCreate()) 847 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 848 return this.gender; 849 } 850 851 public boolean hasGenderElement() { 852 return this.gender != null && !this.gender.isEmpty(); 853 } 854 855 public boolean hasGender() { 856 return this.gender != null && !this.gender.isEmpty(); 857 } 858 859 /** 860 * @param value {@link #gender} (Administrative Gender - the gender that the 861 * person is considered to have for administration and record 862 * keeping purposes.). This is the underlying object with id, value 863 * and extensions. The accessor "getGender" gives direct access to 864 * the value 865 */ 866 public Practitioner setGenderElement(Enumeration<AdministrativeGender> value) { 867 this.gender = value; 868 return this; 869 } 870 871 /** 872 * @return Administrative Gender - the gender that the person is considered to 873 * have for administration and record keeping purposes. 874 */ 875 public AdministrativeGender getGender() { 876 return this.gender == null ? null : this.gender.getValue(); 877 } 878 879 /** 880 * @param value Administrative Gender - the gender that the person is considered 881 * to have for administration and record keeping purposes. 882 */ 883 public Practitioner setGender(AdministrativeGender value) { 884 if (value == null) 885 this.gender = null; 886 else { 887 if (this.gender == null) 888 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 889 this.gender.setValue(value); 890 } 891 return this; 892 } 893 894 /** 895 * @return {@link #birthDate} (The date of birth for the practitioner.). This is 896 * the underlying object with id, value and extensions. The accessor 897 * "getBirthDate" gives direct access to the value 898 */ 899 public DateType getBirthDateElement() { 900 if (this.birthDate == null) 901 if (Configuration.errorOnAutoCreate()) 902 throw new Error("Attempt to auto-create Practitioner.birthDate"); 903 else if (Configuration.doAutoCreate()) 904 this.birthDate = new DateType(); // bb 905 return this.birthDate; 906 } 907 908 public boolean hasBirthDateElement() { 909 return this.birthDate != null && !this.birthDate.isEmpty(); 910 } 911 912 public boolean hasBirthDate() { 913 return this.birthDate != null && !this.birthDate.isEmpty(); 914 } 915 916 /** 917 * @param value {@link #birthDate} (The date of birth for the practitioner.). 918 * This is the underlying object with id, value and extensions. The 919 * accessor "getBirthDate" gives direct access to the value 920 */ 921 public Practitioner setBirthDateElement(DateType value) { 922 this.birthDate = value; 923 return this; 924 } 925 926 /** 927 * @return The date of birth for the practitioner. 928 */ 929 public Date getBirthDate() { 930 return this.birthDate == null ? null : this.birthDate.getValue(); 931 } 932 933 /** 934 * @param value The date of birth for the practitioner. 935 */ 936 public Practitioner setBirthDate(Date value) { 937 if (value == null) 938 this.birthDate = null; 939 else { 940 if (this.birthDate == null) 941 this.birthDate = new DateType(); 942 this.birthDate.setValue(value); 943 } 944 return this; 945 } 946 947 /** 948 * @return {@link #photo} (Image of the person.) 949 */ 950 public List<Attachment> getPhoto() { 951 if (this.photo == null) 952 this.photo = new ArrayList<Attachment>(); 953 return this.photo; 954 } 955 956 /** 957 * @return Returns a reference to <code>this</code> for easy method chaining 958 */ 959 public Practitioner setPhoto(List<Attachment> thePhoto) { 960 this.photo = thePhoto; 961 return this; 962 } 963 964 public boolean hasPhoto() { 965 if (this.photo == null) 966 return false; 967 for (Attachment item : this.photo) 968 if (!item.isEmpty()) 969 return true; 970 return false; 971 } 972 973 public Attachment addPhoto() { // 3 974 Attachment t = new Attachment(); 975 if (this.photo == null) 976 this.photo = new ArrayList<Attachment>(); 977 this.photo.add(t); 978 return t; 979 } 980 981 public Practitioner addPhoto(Attachment t) { // 3 982 if (t == null) 983 return this; 984 if (this.photo == null) 985 this.photo = new ArrayList<Attachment>(); 986 this.photo.add(t); 987 return this; 988 } 989 990 /** 991 * @return The first repetition of repeating field {@link #photo}, creating it 992 * if it does not already exist 993 */ 994 public Attachment getPhotoFirstRep() { 995 if (getPhoto().isEmpty()) { 996 addPhoto(); 997 } 998 return getPhoto().get(0); 999 } 1000 1001 /** 1002 * @return {@link #qualification} (The official certifications, training, and 1003 * licenses that authorize or otherwise pertain to the provision of care 1004 * by the practitioner. For example, a medical license issued by a 1005 * medical board authorizing the practitioner to practice medicine 1006 * within a certian locality.) 1007 */ 1008 public List<PractitionerQualificationComponent> getQualification() { 1009 if (this.qualification == null) 1010 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1011 return this.qualification; 1012 } 1013 1014 /** 1015 * @return Returns a reference to <code>this</code> for easy method chaining 1016 */ 1017 public Practitioner setQualification(List<PractitionerQualificationComponent> theQualification) { 1018 this.qualification = theQualification; 1019 return this; 1020 } 1021 1022 public boolean hasQualification() { 1023 if (this.qualification == null) 1024 return false; 1025 for (PractitionerQualificationComponent item : this.qualification) 1026 if (!item.isEmpty()) 1027 return true; 1028 return false; 1029 } 1030 1031 public PractitionerQualificationComponent addQualification() { // 3 1032 PractitionerQualificationComponent t = new PractitionerQualificationComponent(); 1033 if (this.qualification == null) 1034 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1035 this.qualification.add(t); 1036 return t; 1037 } 1038 1039 public Practitioner addQualification(PractitionerQualificationComponent t) { // 3 1040 if (t == null) 1041 return this; 1042 if (this.qualification == null) 1043 this.qualification = new ArrayList<PractitionerQualificationComponent>(); 1044 this.qualification.add(t); 1045 return this; 1046 } 1047 1048 /** 1049 * @return The first repetition of repeating field {@link #qualification}, 1050 * creating it if it does not already exist 1051 */ 1052 public PractitionerQualificationComponent getQualificationFirstRep() { 1053 if (getQualification().isEmpty()) { 1054 addQualification(); 1055 } 1056 return getQualification().get(0); 1057 } 1058 1059 /** 1060 * @return {@link #communication} (A language the practitioner can use in 1061 * patient communication.) 1062 */ 1063 public List<CodeableConcept> getCommunication() { 1064 if (this.communication == null) 1065 this.communication = new ArrayList<CodeableConcept>(); 1066 return this.communication; 1067 } 1068 1069 /** 1070 * @return Returns a reference to <code>this</code> for easy method chaining 1071 */ 1072 public Practitioner setCommunication(List<CodeableConcept> theCommunication) { 1073 this.communication = theCommunication; 1074 return this; 1075 } 1076 1077 public boolean hasCommunication() { 1078 if (this.communication == null) 1079 return false; 1080 for (CodeableConcept item : this.communication) 1081 if (!item.isEmpty()) 1082 return true; 1083 return false; 1084 } 1085 1086 public CodeableConcept addCommunication() { // 3 1087 CodeableConcept t = new CodeableConcept(); 1088 if (this.communication == null) 1089 this.communication = new ArrayList<CodeableConcept>(); 1090 this.communication.add(t); 1091 return t; 1092 } 1093 1094 public Practitioner addCommunication(CodeableConcept t) { // 3 1095 if (t == null) 1096 return this; 1097 if (this.communication == null) 1098 this.communication = new ArrayList<CodeableConcept>(); 1099 this.communication.add(t); 1100 return this; 1101 } 1102 1103 /** 1104 * @return The first repetition of repeating field {@link #communication}, 1105 * creating it if it does not already exist 1106 */ 1107 public CodeableConcept getCommunicationFirstRep() { 1108 if (getCommunication().isEmpty()) { 1109 addCommunication(); 1110 } 1111 return getCommunication().get(0); 1112 } 1113 1114 protected void listChildren(List<Property> children) { 1115 super.listChildren(children); 1116 children.add(new Property("identifier", "Identifier", "An identifier that applies to this person in this role.", 0, 1117 java.lang.Integer.MAX_VALUE, identifier)); 1118 children 1119 .add(new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1, active)); 1120 children.add(new Property("name", "HumanName", "The name(s) associated with the practitioner.", 0, 1121 java.lang.Integer.MAX_VALUE, name)); 1122 children.add(new Property("telecom", "ContactPoint", 1123 "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, 1124 java.lang.Integer.MAX_VALUE, telecom)); 1125 children.add(new Property("address", "Address", 1126 "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.", 1127 0, java.lang.Integer.MAX_VALUE, address)); 1128 children.add(new Property("gender", "code", 1129 "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 1130 0, 1, gender)); 1131 children.add(new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1, birthDate)); 1132 children.add(new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, photo)); 1133 children.add(new Property("qualification", "", 1134 "The official certifications, training, and licenses that authorize or otherwise pertain to the provision of care by the practitioner. For example, a medical license issued by a medical board authorizing the practitioner to practice medicine within a certian locality.", 1135 0, java.lang.Integer.MAX_VALUE, qualification)); 1136 children.add(new Property("communication", "CodeableConcept", 1137 "A language the practitioner can use in patient communication.", 0, java.lang.Integer.MAX_VALUE, 1138 communication)); 1139 } 1140 1141 @Override 1142 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1143 switch (_hash) { 1144 case -1618432855: 1145 /* identifier */ return new Property("identifier", "Identifier", 1146 "An identifier that applies to this person in this role.", 0, java.lang.Integer.MAX_VALUE, identifier); 1147 case -1422950650: 1148 /* active */ return new Property("active", "boolean", "Whether this practitioner's record is in active use.", 0, 1149 1, active); 1150 case 3373707: 1151 /* name */ return new Property("name", "HumanName", "The name(s) associated with the practitioner.", 0, 1152 java.lang.Integer.MAX_VALUE, name); 1153 case -1429363305: 1154 /* telecom */ return new Property("telecom", "ContactPoint", 1155 "A contact detail for the practitioner, e.g. a telephone number or an email address.", 0, 1156 java.lang.Integer.MAX_VALUE, telecom); 1157 case -1147692044: 1158 /* address */ return new Property("address", "Address", 1159 "Address(es) of the practitioner that are not role specific (typically home address). \rWork addresses are not typically entered in this property as they are usually role dependent.", 1160 0, java.lang.Integer.MAX_VALUE, address); 1161 case -1249512767: 1162 /* gender */ return new Property("gender", "code", 1163 "Administrative Gender - the gender that the person is considered to have for administration and record keeping purposes.", 1164 0, 1, gender); 1165 case -1210031859: 1166 /* birthDate */ return new Property("birthDate", "date", "The date of birth for the practitioner.", 0, 1, 1167 birthDate); 1168 case 106642994: 1169 /* photo */ return new Property("photo", "Attachment", "Image of the person.", 0, java.lang.Integer.MAX_VALUE, 1170 photo); 1171 case -631333393: 1172 /* qualification */ return new Property("qualification", "", 1173 "The official certifications, training, and licenses that authorize or otherwise pertain to the provision of care by the practitioner. For example, a medical license issued by a medical board authorizing the practitioner to practice medicine within a certian locality.", 1174 0, java.lang.Integer.MAX_VALUE, qualification); 1175 case -1035284522: 1176 /* communication */ return new Property("communication", "CodeableConcept", 1177 "A language the practitioner can use in patient communication.", 0, java.lang.Integer.MAX_VALUE, 1178 communication); 1179 default: 1180 return super.getNamedProperty(_hash, _name, _checkValid); 1181 } 1182 1183 } 1184 1185 @Override 1186 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1187 switch (hash) { 1188 case -1618432855: 1189 /* identifier */ return this.identifier == null ? new Base[0] 1190 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1191 case -1422950650: 1192 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1193 case 3373707: 1194 /* name */ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 1195 case -1429363305: 1196 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1197 case -1147692044: 1198 /* address */ return this.address == null ? new Base[0] : this.address.toArray(new Base[this.address.size()]); // Address 1199 case -1249512767: 1200 /* gender */ return this.gender == null ? new Base[0] : new Base[] { this.gender }; // Enumeration<AdministrativeGender> 1201 case -1210031859: 1202 /* birthDate */ return this.birthDate == null ? new Base[0] : new Base[] { this.birthDate }; // DateType 1203 case 106642994: 1204 /* photo */ return this.photo == null ? new Base[0] : this.photo.toArray(new Base[this.photo.size()]); // Attachment 1205 case -631333393: 1206 /* qualification */ return this.qualification == null ? new Base[0] 1207 : this.qualification.toArray(new Base[this.qualification.size()]); // PractitionerQualificationComponent 1208 case -1035284522: 1209 /* communication */ return this.communication == null ? new Base[0] 1210 : this.communication.toArray(new Base[this.communication.size()]); // CodeableConcept 1211 default: 1212 return super.getProperty(hash, name, checkValid); 1213 } 1214 1215 } 1216 1217 @Override 1218 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1219 switch (hash) { 1220 case -1618432855: // identifier 1221 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1222 return value; 1223 case -1422950650: // active 1224 this.active = castToBoolean(value); // BooleanType 1225 return value; 1226 case 3373707: // name 1227 this.getName().add(castToHumanName(value)); // HumanName 1228 return value; 1229 case -1429363305: // telecom 1230 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1231 return value; 1232 case -1147692044: // address 1233 this.getAddress().add(castToAddress(value)); // Address 1234 return value; 1235 case -1249512767: // gender 1236 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1237 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1238 return value; 1239 case -1210031859: // birthDate 1240 this.birthDate = castToDate(value); // DateType 1241 return value; 1242 case 106642994: // photo 1243 this.getPhoto().add(castToAttachment(value)); // Attachment 1244 return value; 1245 case -631333393: // qualification 1246 this.getQualification().add((PractitionerQualificationComponent) value); // PractitionerQualificationComponent 1247 return value; 1248 case -1035284522: // communication 1249 this.getCommunication().add(castToCodeableConcept(value)); // CodeableConcept 1250 return value; 1251 default: 1252 return super.setProperty(hash, name, value); 1253 } 1254 1255 } 1256 1257 @Override 1258 public Base setProperty(String name, Base value) throws FHIRException { 1259 if (name.equals("identifier")) { 1260 this.getIdentifier().add(castToIdentifier(value)); 1261 } else if (name.equals("active")) { 1262 this.active = castToBoolean(value); // BooleanType 1263 } else if (name.equals("name")) { 1264 this.getName().add(castToHumanName(value)); 1265 } else if (name.equals("telecom")) { 1266 this.getTelecom().add(castToContactPoint(value)); 1267 } else if (name.equals("address")) { 1268 this.getAddress().add(castToAddress(value)); 1269 } else if (name.equals("gender")) { 1270 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1271 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1272 } else if (name.equals("birthDate")) { 1273 this.birthDate = castToDate(value); // DateType 1274 } else if (name.equals("photo")) { 1275 this.getPhoto().add(castToAttachment(value)); 1276 } else if (name.equals("qualification")) { 1277 this.getQualification().add((PractitionerQualificationComponent) value); 1278 } else if (name.equals("communication")) { 1279 this.getCommunication().add(castToCodeableConcept(value)); 1280 } else 1281 return super.setProperty(name, value); 1282 return value; 1283 } 1284 1285 @Override 1286 public void removeChild(String name, Base value) throws FHIRException { 1287 if (name.equals("identifier")) { 1288 this.getIdentifier().remove(castToIdentifier(value)); 1289 } else if (name.equals("active")) { 1290 this.active = null; 1291 } else if (name.equals("name")) { 1292 this.getName().remove(castToHumanName(value)); 1293 } else if (name.equals("telecom")) { 1294 this.getTelecom().remove(castToContactPoint(value)); 1295 } else if (name.equals("address")) { 1296 this.getAddress().remove(castToAddress(value)); 1297 } else if (name.equals("gender")) { 1298 this.gender = null; 1299 } else if (name.equals("birthDate")) { 1300 this.birthDate = null; 1301 } else if (name.equals("photo")) { 1302 this.getPhoto().remove(castToAttachment(value)); 1303 } else if (name.equals("qualification")) { 1304 this.getQualification().remove((PractitionerQualificationComponent) value); 1305 } else if (name.equals("communication")) { 1306 this.getCommunication().remove(castToCodeableConcept(value)); 1307 } else 1308 super.removeChild(name, value); 1309 1310 } 1311 1312 @Override 1313 public Base makeProperty(int hash, String name) throws FHIRException { 1314 switch (hash) { 1315 case -1618432855: 1316 return addIdentifier(); 1317 case -1422950650: 1318 return getActiveElement(); 1319 case 3373707: 1320 return addName(); 1321 case -1429363305: 1322 return addTelecom(); 1323 case -1147692044: 1324 return addAddress(); 1325 case -1249512767: 1326 return getGenderElement(); 1327 case -1210031859: 1328 return getBirthDateElement(); 1329 case 106642994: 1330 return addPhoto(); 1331 case -631333393: 1332 return addQualification(); 1333 case -1035284522: 1334 return addCommunication(); 1335 default: 1336 return super.makeProperty(hash, name); 1337 } 1338 1339 } 1340 1341 @Override 1342 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1343 switch (hash) { 1344 case -1618432855: 1345 /* identifier */ return new String[] { "Identifier" }; 1346 case -1422950650: 1347 /* active */ return new String[] { "boolean" }; 1348 case 3373707: 1349 /* name */ return new String[] { "HumanName" }; 1350 case -1429363305: 1351 /* telecom */ return new String[] { "ContactPoint" }; 1352 case -1147692044: 1353 /* address */ return new String[] { "Address" }; 1354 case -1249512767: 1355 /* gender */ return new String[] { "code" }; 1356 case -1210031859: 1357 /* birthDate */ return new String[] { "date" }; 1358 case 106642994: 1359 /* photo */ return new String[] { "Attachment" }; 1360 case -631333393: 1361 /* qualification */ return new String[] {}; 1362 case -1035284522: 1363 /* communication */ return new String[] { "CodeableConcept" }; 1364 default: 1365 return super.getTypesForProperty(hash, name); 1366 } 1367 1368 } 1369 1370 @Override 1371 public Base addChild(String name) throws FHIRException { 1372 if (name.equals("identifier")) { 1373 return addIdentifier(); 1374 } else if (name.equals("active")) { 1375 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.active"); 1376 } else if (name.equals("name")) { 1377 return addName(); 1378 } else if (name.equals("telecom")) { 1379 return addTelecom(); 1380 } else if (name.equals("address")) { 1381 return addAddress(); 1382 } else if (name.equals("gender")) { 1383 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.gender"); 1384 } else if (name.equals("birthDate")) { 1385 throw new FHIRException("Cannot call addChild on a singleton property Practitioner.birthDate"); 1386 } else if (name.equals("photo")) { 1387 return addPhoto(); 1388 } else if (name.equals("qualification")) { 1389 return addQualification(); 1390 } else if (name.equals("communication")) { 1391 return addCommunication(); 1392 } else 1393 return super.addChild(name); 1394 } 1395 1396 public String fhirType() { 1397 return "Practitioner"; 1398 1399 } 1400 1401 public Practitioner copy() { 1402 Practitioner dst = new Practitioner(); 1403 copyValues(dst); 1404 return dst; 1405 } 1406 1407 public void copyValues(Practitioner dst) { 1408 super.copyValues(dst); 1409 if (identifier != null) { 1410 dst.identifier = new ArrayList<Identifier>(); 1411 for (Identifier i : identifier) 1412 dst.identifier.add(i.copy()); 1413 } 1414 ; 1415 dst.active = active == null ? null : active.copy(); 1416 if (name != null) { 1417 dst.name = new ArrayList<HumanName>(); 1418 for (HumanName i : name) 1419 dst.name.add(i.copy()); 1420 } 1421 ; 1422 if (telecom != null) { 1423 dst.telecom = new ArrayList<ContactPoint>(); 1424 for (ContactPoint i : telecom) 1425 dst.telecom.add(i.copy()); 1426 } 1427 ; 1428 if (address != null) { 1429 dst.address = new ArrayList<Address>(); 1430 for (Address i : address) 1431 dst.address.add(i.copy()); 1432 } 1433 ; 1434 dst.gender = gender == null ? null : gender.copy(); 1435 dst.birthDate = birthDate == null ? null : birthDate.copy(); 1436 if (photo != null) { 1437 dst.photo = new ArrayList<Attachment>(); 1438 for (Attachment i : photo) 1439 dst.photo.add(i.copy()); 1440 } 1441 ; 1442 if (qualification != null) { 1443 dst.qualification = new ArrayList<PractitionerQualificationComponent>(); 1444 for (PractitionerQualificationComponent i : qualification) 1445 dst.qualification.add(i.copy()); 1446 } 1447 ; 1448 if (communication != null) { 1449 dst.communication = new ArrayList<CodeableConcept>(); 1450 for (CodeableConcept i : communication) 1451 dst.communication.add(i.copy()); 1452 } 1453 ; 1454 } 1455 1456 protected Practitioner typedCopy() { 1457 return copy(); 1458 } 1459 1460 @Override 1461 public boolean equalsDeep(Base other_) { 1462 if (!super.equalsDeep(other_)) 1463 return false; 1464 if (!(other_ instanceof Practitioner)) 1465 return false; 1466 Practitioner o = (Practitioner) other_; 1467 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 1468 && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 1469 && compareDeep(address, o.address, true) && compareDeep(gender, o.gender, true) 1470 && compareDeep(birthDate, o.birthDate, true) && compareDeep(photo, o.photo, true) 1471 && compareDeep(qualification, o.qualification, true) && compareDeep(communication, o.communication, true); 1472 } 1473 1474 @Override 1475 public boolean equalsShallow(Base other_) { 1476 if (!super.equalsShallow(other_)) 1477 return false; 1478 if (!(other_ instanceof Practitioner)) 1479 return false; 1480 Practitioner o = (Practitioner) other_; 1481 return compareValues(active, o.active, true) && compareValues(gender, o.gender, true) 1482 && compareValues(birthDate, o.birthDate, true); 1483 } 1484 1485 public boolean isEmpty() { 1486 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, name, telecom, address, gender, 1487 birthDate, photo, qualification, communication); 1488 } 1489 1490 @Override 1491 public ResourceType getResourceType() { 1492 return ResourceType.Practitioner; 1493 } 1494 1495 /** 1496 * Search parameter: <b>identifier</b> 1497 * <p> 1498 * Description: <b>A practitioner's Identifier</b><br> 1499 * Type: <b>token</b><br> 1500 * Path: <b>Practitioner.identifier</b><br> 1501 * </p> 1502 */ 1503 @SearchParamDefinition(name = "identifier", path = "Practitioner.identifier", description = "A practitioner's Identifier", type = "token") 1504 public static final String SP_IDENTIFIER = "identifier"; 1505 /** 1506 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1507 * <p> 1508 * Description: <b>A practitioner's Identifier</b><br> 1509 * Type: <b>token</b><br> 1510 * Path: <b>Practitioner.identifier</b><br> 1511 * </p> 1512 */ 1513 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1514 SP_IDENTIFIER); 1515 1516 /** 1517 * Search parameter: <b>given</b> 1518 * <p> 1519 * Description: <b>A portion of the given name</b><br> 1520 * Type: <b>string</b><br> 1521 * Path: <b>Practitioner.name.given</b><br> 1522 * </p> 1523 */ 1524 @SearchParamDefinition(name = "given", path = "Practitioner.name.given", description = "A portion of the given name", type = "string") 1525 public static final String SP_GIVEN = "given"; 1526 /** 1527 * <b>Fluent Client</b> search parameter constant for <b>given</b> 1528 * <p> 1529 * Description: <b>A portion of the given name</b><br> 1530 * Type: <b>string</b><br> 1531 * Path: <b>Practitioner.name.given</b><br> 1532 * </p> 1533 */ 1534 public static final ca.uhn.fhir.rest.gclient.StringClientParam GIVEN = new ca.uhn.fhir.rest.gclient.StringClientParam( 1535 SP_GIVEN); 1536 1537 /** 1538 * Search parameter: <b>address</b> 1539 * <p> 1540 * Description: <b>A server defined search that may match any of the string 1541 * fields in the Address, including line, city, district, state, country, 1542 * postalCode, and/or text</b><br> 1543 * Type: <b>string</b><br> 1544 * Path: <b>Practitioner.address</b><br> 1545 * </p> 1546 */ 1547 @SearchParamDefinition(name = "address", path = "Practitioner.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 1548 public static final String SP_ADDRESS = "address"; 1549 /** 1550 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1551 * <p> 1552 * Description: <b>A server defined search that may match any of the string 1553 * fields in the Address, including line, city, district, state, country, 1554 * postalCode, and/or text</b><br> 1555 * Type: <b>string</b><br> 1556 * Path: <b>Practitioner.address</b><br> 1557 * </p> 1558 */ 1559 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 1560 SP_ADDRESS); 1561 1562 /** 1563 * Search parameter: <b>address-state</b> 1564 * <p> 1565 * Description: <b>A state specified in an address</b><br> 1566 * Type: <b>string</b><br> 1567 * Path: <b>Practitioner.address.state</b><br> 1568 * </p> 1569 */ 1570 @SearchParamDefinition(name = "address-state", path = "Practitioner.address.state", description = "A state specified in an address", type = "string") 1571 public static final String SP_ADDRESS_STATE = "address-state"; 1572 /** 1573 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1574 * <p> 1575 * Description: <b>A state specified in an address</b><br> 1576 * Type: <b>string</b><br> 1577 * Path: <b>Practitioner.address.state</b><br> 1578 * </p> 1579 */ 1580 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1581 SP_ADDRESS_STATE); 1582 1583 /** 1584 * Search parameter: <b>gender</b> 1585 * <p> 1586 * Description: <b>Gender of the practitioner</b><br> 1587 * Type: <b>token</b><br> 1588 * Path: <b>Practitioner.gender</b><br> 1589 * </p> 1590 */ 1591 @SearchParamDefinition(name = "gender", path = "Practitioner.gender", description = "Gender of the practitioner", type = "token") 1592 public static final String SP_GENDER = "gender"; 1593 /** 1594 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 1595 * <p> 1596 * Description: <b>Gender of the practitioner</b><br> 1597 * Type: <b>token</b><br> 1598 * Path: <b>Practitioner.gender</b><br> 1599 * </p> 1600 */ 1601 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1602 SP_GENDER); 1603 1604 /** 1605 * Search parameter: <b>active</b> 1606 * <p> 1607 * Description: <b>Whether the practitioner record is active</b><br> 1608 * Type: <b>token</b><br> 1609 * Path: <b>Practitioner.active</b><br> 1610 * </p> 1611 */ 1612 @SearchParamDefinition(name = "active", path = "Practitioner.active", description = "Whether the practitioner record is active", type = "token") 1613 public static final String SP_ACTIVE = "active"; 1614 /** 1615 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1616 * <p> 1617 * Description: <b>Whether the practitioner record is active</b><br> 1618 * Type: <b>token</b><br> 1619 * Path: <b>Practitioner.active</b><br> 1620 * </p> 1621 */ 1622 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1623 SP_ACTIVE); 1624 1625 /** 1626 * Search parameter: <b>address-postalcode</b> 1627 * <p> 1628 * Description: <b>A postalCode specified in an address</b><br> 1629 * Type: <b>string</b><br> 1630 * Path: <b>Practitioner.address.postalCode</b><br> 1631 * </p> 1632 */ 1633 @SearchParamDefinition(name = "address-postalcode", path = "Practitioner.address.postalCode", description = "A postalCode specified in an address", type = "string") 1634 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1635 /** 1636 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1637 * <p> 1638 * Description: <b>A postalCode specified in an address</b><br> 1639 * Type: <b>string</b><br> 1640 * Path: <b>Practitioner.address.postalCode</b><br> 1641 * </p> 1642 */ 1643 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 1644 SP_ADDRESS_POSTALCODE); 1645 1646 /** 1647 * Search parameter: <b>address-country</b> 1648 * <p> 1649 * Description: <b>A country specified in an address</b><br> 1650 * Type: <b>string</b><br> 1651 * Path: <b>Practitioner.address.country</b><br> 1652 * </p> 1653 */ 1654 @SearchParamDefinition(name = "address-country", path = "Practitioner.address.country", description = "A country specified in an address", type = "string") 1655 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1656 /** 1657 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1658 * <p> 1659 * Description: <b>A country specified in an address</b><br> 1660 * Type: <b>string</b><br> 1661 * Path: <b>Practitioner.address.country</b><br> 1662 * </p> 1663 */ 1664 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1665 SP_ADDRESS_COUNTRY); 1666 1667 /** 1668 * Search parameter: <b>phonetic</b> 1669 * <p> 1670 * Description: <b>A portion of either family or given name using some kind of 1671 * phonetic matching algorithm</b><br> 1672 * Type: <b>string</b><br> 1673 * Path: <b>Practitioner.name</b><br> 1674 * </p> 1675 */ 1676 @SearchParamDefinition(name = "phonetic", path = "Practitioner.name", description = "A portion of either family or given name using some kind of phonetic matching algorithm", type = "string") 1677 public static final String SP_PHONETIC = "phonetic"; 1678 /** 1679 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 1680 * <p> 1681 * Description: <b>A portion of either family or given name using some kind of 1682 * phonetic matching algorithm</b><br> 1683 * Type: <b>string</b><br> 1684 * Path: <b>Practitioner.name</b><br> 1685 * </p> 1686 */ 1687 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 1688 SP_PHONETIC); 1689 1690 /** 1691 * Search parameter: <b>phone</b> 1692 * <p> 1693 * Description: <b>A value in a phone contact</b><br> 1694 * Type: <b>token</b><br> 1695 * Path: <b>Practitioner.telecom(system=phone)</b><br> 1696 * </p> 1697 */ 1698 @SearchParamDefinition(name = "phone", path = "Practitioner.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 1699 public static final String SP_PHONE = "phone"; 1700 /** 1701 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 1702 * <p> 1703 * Description: <b>A value in a phone contact</b><br> 1704 * Type: <b>token</b><br> 1705 * Path: <b>Practitioner.telecom(system=phone)</b><br> 1706 * </p> 1707 */ 1708 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1709 SP_PHONE); 1710 1711 /** 1712 * Search parameter: <b>name</b> 1713 * <p> 1714 * Description: <b>A server defined search that may match any of the string 1715 * fields in the HumanName, including family, give, prefix, suffix, suffix, 1716 * and/or text</b><br> 1717 * Type: <b>string</b><br> 1718 * Path: <b>Practitioner.name</b><br> 1719 * </p> 1720 */ 1721 @SearchParamDefinition(name = "name", path = "Practitioner.name", description = "A server defined search that may match any of the string fields in the HumanName, including family, give, prefix, suffix, suffix, and/or text", type = "string") 1722 public static final String SP_NAME = "name"; 1723 /** 1724 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1725 * <p> 1726 * Description: <b>A server defined search that may match any of the string 1727 * fields in the HumanName, including family, give, prefix, suffix, suffix, 1728 * and/or text</b><br> 1729 * Type: <b>string</b><br> 1730 * Path: <b>Practitioner.name</b><br> 1731 * </p> 1732 */ 1733 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 1734 SP_NAME); 1735 1736 /** 1737 * Search parameter: <b>address-use</b> 1738 * <p> 1739 * Description: <b>A use code specified in an address</b><br> 1740 * Type: <b>token</b><br> 1741 * Path: <b>Practitioner.address.use</b><br> 1742 * </p> 1743 */ 1744 @SearchParamDefinition(name = "address-use", path = "Practitioner.address.use", description = "A use code specified in an address", type = "token") 1745 public static final String SP_ADDRESS_USE = "address-use"; 1746 /** 1747 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 1748 * <p> 1749 * Description: <b>A use code specified in an address</b><br> 1750 * Type: <b>token</b><br> 1751 * Path: <b>Practitioner.address.use</b><br> 1752 * </p> 1753 */ 1754 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1755 SP_ADDRESS_USE); 1756 1757 /** 1758 * Search parameter: <b>telecom</b> 1759 * <p> 1760 * Description: <b>The value in any kind of contact</b><br> 1761 * Type: <b>token</b><br> 1762 * Path: <b>Practitioner.telecom</b><br> 1763 * </p> 1764 */ 1765 @SearchParamDefinition(name = "telecom", path = "Practitioner.telecom", description = "The value in any kind of contact", type = "token") 1766 public static final String SP_TELECOM = "telecom"; 1767 /** 1768 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 1769 * <p> 1770 * Description: <b>The value in any kind of contact</b><br> 1771 * Type: <b>token</b><br> 1772 * Path: <b>Practitioner.telecom</b><br> 1773 * </p> 1774 */ 1775 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1776 SP_TELECOM); 1777 1778 /** 1779 * Search parameter: <b>family</b> 1780 * <p> 1781 * Description: <b>A portion of the family name</b><br> 1782 * Type: <b>string</b><br> 1783 * Path: <b>Practitioner.name.family</b><br> 1784 * </p> 1785 */ 1786 @SearchParamDefinition(name = "family", path = "Practitioner.name.family", description = "A portion of the family name", type = "string") 1787 public static final String SP_FAMILY = "family"; 1788 /** 1789 * <b>Fluent Client</b> search parameter constant for <b>family</b> 1790 * <p> 1791 * Description: <b>A portion of the family name</b><br> 1792 * Type: <b>string</b><br> 1793 * Path: <b>Practitioner.name.family</b><br> 1794 * </p> 1795 */ 1796 public static final ca.uhn.fhir.rest.gclient.StringClientParam FAMILY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1797 SP_FAMILY); 1798 1799 /** 1800 * Search parameter: <b>address-city</b> 1801 * <p> 1802 * Description: <b>A city specified in an address</b><br> 1803 * Type: <b>string</b><br> 1804 * Path: <b>Practitioner.address.city</b><br> 1805 * </p> 1806 */ 1807 @SearchParamDefinition(name = "address-city", path = "Practitioner.address.city", description = "A city specified in an address", type = "string") 1808 public static final String SP_ADDRESS_CITY = "address-city"; 1809 /** 1810 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 1811 * <p> 1812 * Description: <b>A city specified in an address</b><br> 1813 * Type: <b>string</b><br> 1814 * Path: <b>Practitioner.address.city</b><br> 1815 * </p> 1816 */ 1817 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 1818 SP_ADDRESS_CITY); 1819 1820 /** 1821 * Search parameter: <b>communication</b> 1822 * <p> 1823 * Description: <b>One of the languages that the practitioner can communicate 1824 * with</b><br> 1825 * Type: <b>token</b><br> 1826 * Path: <b>Practitioner.communication</b><br> 1827 * </p> 1828 */ 1829 @SearchParamDefinition(name = "communication", path = "Practitioner.communication", description = "One of the languages that the practitioner can communicate with", type = "token") 1830 public static final String SP_COMMUNICATION = "communication"; 1831 /** 1832 * <b>Fluent Client</b> search parameter constant for <b>communication</b> 1833 * <p> 1834 * Description: <b>One of the languages that the practitioner can communicate 1835 * with</b><br> 1836 * Type: <b>token</b><br> 1837 * Path: <b>Practitioner.communication</b><br> 1838 * </p> 1839 */ 1840 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMMUNICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1841 SP_COMMUNICATION); 1842 1843 /** 1844 * Search parameter: <b>email</b> 1845 * <p> 1846 * Description: <b>A value in an email contact</b><br> 1847 * Type: <b>token</b><br> 1848 * Path: <b>Practitioner.telecom(system=email)</b><br> 1849 * </p> 1850 */ 1851 @SearchParamDefinition(name = "email", path = "Practitioner.telecom.where(system='email')", description = "A value in an email contact", type = "token") 1852 public static final String SP_EMAIL = "email"; 1853 /** 1854 * <b>Fluent Client</b> search parameter constant for <b>email</b> 1855 * <p> 1856 * Description: <b>A value in an email contact</b><br> 1857 * Type: <b>token</b><br> 1858 * Path: <b>Practitioner.telecom(system=email)</b><br> 1859 * </p> 1860 */ 1861 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1862 SP_EMAIL); 1863 1864}