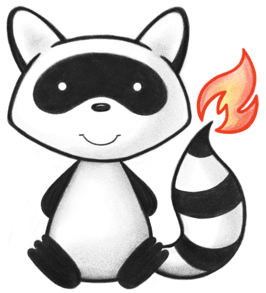
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A specific set of Roles/Locations/specialties/services that a practitioner 048 * may perform at an organization for a period of time. 049 */ 050@ResourceDef(name = "PractitionerRole", profile = "http://hl7.org/fhir/StructureDefinition/PractitionerRole") 051public class PractitionerRole extends DomainResource { 052 053 public enum DaysOfWeek { 054 /** 055 * Monday. 056 */ 057 MON, 058 /** 059 * Tuesday. 060 */ 061 TUE, 062 /** 063 * Wednesday. 064 */ 065 WED, 066 /** 067 * Thursday. 068 */ 069 THU, 070 /** 071 * Friday. 072 */ 073 FRI, 074 /** 075 * Saturday. 076 */ 077 SAT, 078 /** 079 * Sunday. 080 */ 081 SUN, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 087 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("mon".equals(codeString)) 091 return MON; 092 if ("tue".equals(codeString)) 093 return TUE; 094 if ("wed".equals(codeString)) 095 return WED; 096 if ("thu".equals(codeString)) 097 return THU; 098 if ("fri".equals(codeString)) 099 return FRI; 100 if ("sat".equals(codeString)) 101 return SAT; 102 if ("sun".equals(codeString)) 103 return SUN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case MON: 113 return "mon"; 114 case TUE: 115 return "tue"; 116 case WED: 117 return "wed"; 118 case THU: 119 return "thu"; 120 case FRI: 121 return "fri"; 122 case SAT: 123 return "sat"; 124 case SUN: 125 return "sun"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getSystem() { 134 switch (this) { 135 case MON: 136 return "http://hl7.org/fhir/days-of-week"; 137 case TUE: 138 return "http://hl7.org/fhir/days-of-week"; 139 case WED: 140 return "http://hl7.org/fhir/days-of-week"; 141 case THU: 142 return "http://hl7.org/fhir/days-of-week"; 143 case FRI: 144 return "http://hl7.org/fhir/days-of-week"; 145 case SAT: 146 return "http://hl7.org/fhir/days-of-week"; 147 case SUN: 148 return "http://hl7.org/fhir/days-of-week"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDefinition() { 157 switch (this) { 158 case MON: 159 return "Monday."; 160 case TUE: 161 return "Tuesday."; 162 case WED: 163 return "Wednesday."; 164 case THU: 165 return "Thursday."; 166 case FRI: 167 return "Friday."; 168 case SAT: 169 return "Saturday."; 170 case SUN: 171 return "Sunday."; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDisplay() { 180 switch (this) { 181 case MON: 182 return "Monday"; 183 case TUE: 184 return "Tuesday"; 185 case WED: 186 return "Wednesday"; 187 case THU: 188 return "Thursday"; 189 case FRI: 190 return "Friday"; 191 case SAT: 192 return "Saturday"; 193 case SUN: 194 return "Sunday"; 195 case NULL: 196 return null; 197 default: 198 return "?"; 199 } 200 } 201 } 202 203 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 204 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 205 if (codeString == null || "".equals(codeString)) 206 if (codeString == null || "".equals(codeString)) 207 return null; 208 if ("mon".equals(codeString)) 209 return DaysOfWeek.MON; 210 if ("tue".equals(codeString)) 211 return DaysOfWeek.TUE; 212 if ("wed".equals(codeString)) 213 return DaysOfWeek.WED; 214 if ("thu".equals(codeString)) 215 return DaysOfWeek.THU; 216 if ("fri".equals(codeString)) 217 return DaysOfWeek.FRI; 218 if ("sat".equals(codeString)) 219 return DaysOfWeek.SAT; 220 if ("sun".equals(codeString)) 221 return DaysOfWeek.SUN; 222 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 223 } 224 225 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 226 if (code == null) 227 return null; 228 if (code.isEmpty()) 229 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 230 String codeString = code.asStringValue(); 231 if (codeString == null || "".equals(codeString)) 232 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 233 if ("mon".equals(codeString)) 234 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON, code); 235 if ("tue".equals(codeString)) 236 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE, code); 237 if ("wed".equals(codeString)) 238 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED, code); 239 if ("thu".equals(codeString)) 240 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU, code); 241 if ("fri".equals(codeString)) 242 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI, code); 243 if ("sat".equals(codeString)) 244 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT, code); 245 if ("sun".equals(codeString)) 246 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN, code); 247 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 248 } 249 250 public String toCode(DaysOfWeek code) { 251 if (code == DaysOfWeek.NULL) 252 return null; 253 if (code == DaysOfWeek.MON) 254 return "mon"; 255 if (code == DaysOfWeek.TUE) 256 return "tue"; 257 if (code == DaysOfWeek.WED) 258 return "wed"; 259 if (code == DaysOfWeek.THU) 260 return "thu"; 261 if (code == DaysOfWeek.FRI) 262 return "fri"; 263 if (code == DaysOfWeek.SAT) 264 return "sat"; 265 if (code == DaysOfWeek.SUN) 266 return "sun"; 267 return "?"; 268 } 269 270 public String toSystem(DaysOfWeek code) { 271 return code.getSystem(); 272 } 273 } 274 275 @Block() 276 public static class PractitionerRoleAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 277 /** 278 * Indicates which days of the week are available between the start and end 279 * Times. 280 */ 281 @Child(name = "daysOfWeek", type = { 282 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 283 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 285 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 286 287 /** 288 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 289 */ 290 @Child(name = "allDay", type = { 291 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 292 @Description(shortDefinition = "Always available? e.g. 24 hour service", formalDefinition = "Is this always available? (hence times are irrelevant) e.g. 24 hour service.") 293 protected BooleanType allDay; 294 295 /** 296 * The opening time of day. Note: If the AllDay flag is set, then this time is 297 * ignored. 298 */ 299 @Child(name = "availableStartTime", type = { 300 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 301 @Description(shortDefinition = "Opening time of day (ignored if allDay = true)", formalDefinition = "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.") 302 protected TimeType availableStartTime; 303 304 /** 305 * The closing time of day. Note: If the AllDay flag is set, then this time is 306 * ignored. 307 */ 308 @Child(name = "availableEndTime", type = { 309 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 310 @Description(shortDefinition = "Closing time of day (ignored if allDay = true)", formalDefinition = "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.") 311 protected TimeType availableEndTime; 312 313 private static final long serialVersionUID = -2139510127L; 314 315 /** 316 * Constructor 317 */ 318 public PractitionerRoleAvailableTimeComponent() { 319 super(); 320 } 321 322 /** 323 * @return {@link #daysOfWeek} (Indicates which days of the week are available 324 * between the start and end Times.) 325 */ 326 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 327 if (this.daysOfWeek == null) 328 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 329 return this.daysOfWeek; 330 } 331 332 /** 333 * @return Returns a reference to <code>this</code> for easy method chaining 334 */ 335 public PractitionerRoleAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 336 this.daysOfWeek = theDaysOfWeek; 337 return this; 338 } 339 340 public boolean hasDaysOfWeek() { 341 if (this.daysOfWeek == null) 342 return false; 343 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 344 if (!item.isEmpty()) 345 return true; 346 return false; 347 } 348 349 /** 350 * @return {@link #daysOfWeek} (Indicates which days of the week are available 351 * between the start and end Times.) 352 */ 353 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 354 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 355 if (this.daysOfWeek == null) 356 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 357 this.daysOfWeek.add(t); 358 return t; 359 } 360 361 /** 362 * @param value {@link #daysOfWeek} (Indicates which days of the week are 363 * available between the start and end Times.) 364 */ 365 public PractitionerRoleAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { // 1 366 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 367 t.setValue(value); 368 if (this.daysOfWeek == null) 369 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 370 this.daysOfWeek.add(t); 371 return this; 372 } 373 374 /** 375 * @param value {@link #daysOfWeek} (Indicates which days of the week are 376 * available between the start and end Times.) 377 */ 378 public boolean hasDaysOfWeek(DaysOfWeek value) { 379 if (this.daysOfWeek == null) 380 return false; 381 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 382 if (v.getValue().equals(value)) // code 383 return true; 384 return false; 385 } 386 387 /** 388 * @return {@link #allDay} (Is this always available? (hence times are 389 * irrelevant) e.g. 24 hour service.). This is the underlying object 390 * with id, value and extensions. The accessor "getAllDay" gives direct 391 * access to the value 392 */ 393 public BooleanType getAllDayElement() { 394 if (this.allDay == null) 395 if (Configuration.errorOnAutoCreate()) 396 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.allDay"); 397 else if (Configuration.doAutoCreate()) 398 this.allDay = new BooleanType(); // bb 399 return this.allDay; 400 } 401 402 public boolean hasAllDayElement() { 403 return this.allDay != null && !this.allDay.isEmpty(); 404 } 405 406 public boolean hasAllDay() { 407 return this.allDay != null && !this.allDay.isEmpty(); 408 } 409 410 /** 411 * @param value {@link #allDay} (Is this always available? (hence times are 412 * irrelevant) e.g. 24 hour service.). This is the underlying 413 * object with id, value and extensions. The accessor "getAllDay" 414 * gives direct access to the value 415 */ 416 public PractitionerRoleAvailableTimeComponent setAllDayElement(BooleanType value) { 417 this.allDay = value; 418 return this; 419 } 420 421 /** 422 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour 423 * service. 424 */ 425 public boolean getAllDay() { 426 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 427 } 428 429 /** 430 * @param value Is this always available? (hence times are irrelevant) e.g. 24 431 * hour service. 432 */ 433 public PractitionerRoleAvailableTimeComponent setAllDay(boolean value) { 434 if (this.allDay == null) 435 this.allDay = new BooleanType(); 436 this.allDay.setValue(value); 437 return this; 438 } 439 440 /** 441 * @return {@link #availableStartTime} (The opening time of day. Note: If the 442 * AllDay flag is set, then this time is ignored.). This is the 443 * underlying object with id, value and extensions. The accessor 444 * "getAvailableStartTime" gives direct access to the value 445 */ 446 public TimeType getAvailableStartTimeElement() { 447 if (this.availableStartTime == null) 448 if (Configuration.errorOnAutoCreate()) 449 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableStartTime"); 450 else if (Configuration.doAutoCreate()) 451 this.availableStartTime = new TimeType(); // bb 452 return this.availableStartTime; 453 } 454 455 public boolean hasAvailableStartTimeElement() { 456 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 457 } 458 459 public boolean hasAvailableStartTime() { 460 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #availableStartTime} (The opening time of day. Note: If 465 * the AllDay flag is set, then this time is ignored.). This is the 466 * underlying object with id, value and extensions. The accessor 467 * "getAvailableStartTime" gives direct access to the value 468 */ 469 public PractitionerRoleAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 470 this.availableStartTime = value; 471 return this; 472 } 473 474 /** 475 * @return The opening time of day. Note: If the AllDay flag is set, then this 476 * time is ignored. 477 */ 478 public String getAvailableStartTime() { 479 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 480 } 481 482 /** 483 * @param value The opening time of day. Note: If the AllDay flag is set, then 484 * this time is ignored. 485 */ 486 public PractitionerRoleAvailableTimeComponent setAvailableStartTime(String value) { 487 if (value == null) 488 this.availableStartTime = null; 489 else { 490 if (this.availableStartTime == null) 491 this.availableStartTime = new TimeType(); 492 this.availableStartTime.setValue(value); 493 } 494 return this; 495 } 496 497 /** 498 * @return {@link #availableEndTime} (The closing time of day. Note: If the 499 * AllDay flag is set, then this time is ignored.). This is the 500 * underlying object with id, value and extensions. The accessor 501 * "getAvailableEndTime" gives direct access to the value 502 */ 503 public TimeType getAvailableEndTimeElement() { 504 if (this.availableEndTime == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableEndTime"); 507 else if (Configuration.doAutoCreate()) 508 this.availableEndTime = new TimeType(); // bb 509 return this.availableEndTime; 510 } 511 512 public boolean hasAvailableEndTimeElement() { 513 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 514 } 515 516 public boolean hasAvailableEndTime() { 517 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #availableEndTime} (The closing time of day. Note: If the 522 * AllDay flag is set, then this time is ignored.). This is the 523 * underlying object with id, value and extensions. The accessor 524 * "getAvailableEndTime" gives direct access to the value 525 */ 526 public PractitionerRoleAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 527 this.availableEndTime = value; 528 return this; 529 } 530 531 /** 532 * @return The closing time of day. Note: If the AllDay flag is set, then this 533 * time is ignored. 534 */ 535 public String getAvailableEndTime() { 536 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 537 } 538 539 /** 540 * @param value The closing time of day. Note: If the AllDay flag is set, then 541 * this time is ignored. 542 */ 543 public PractitionerRoleAvailableTimeComponent setAvailableEndTime(String value) { 544 if (value == null) 545 this.availableEndTime = null; 546 else { 547 if (this.availableEndTime == null) 548 this.availableEndTime = new TimeType(); 549 this.availableEndTime.setValue(value); 550 } 551 return this; 552 } 553 554 protected void listChildren(List<Property> children) { 555 super.listChildren(children); 556 children.add(new Property("daysOfWeek", "code", 557 "Indicates which days of the week are available between the start and end Times.", 0, 558 java.lang.Integer.MAX_VALUE, daysOfWeek)); 559 children.add(new Property("allDay", "boolean", 560 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 561 children.add(new Property("availableStartTime", "time", 562 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 563 availableStartTime)); 564 children.add(new Property("availableEndTime", "time", 565 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 566 availableEndTime)); 567 } 568 569 @Override 570 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 571 switch (_hash) { 572 case 68050338: 573 /* daysOfWeek */ return new Property("daysOfWeek", "code", 574 "Indicates which days of the week are available between the start and end Times.", 0, 575 java.lang.Integer.MAX_VALUE, daysOfWeek); 576 case -1414913477: 577 /* allDay */ return new Property("allDay", "boolean", 578 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 579 case -1039453818: 580 /* availableStartTime */ return new Property("availableStartTime", "time", 581 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 582 availableStartTime); 583 case 101151551: 584 /* availableEndTime */ return new Property("availableEndTime", "time", 585 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 586 availableEndTime); 587 default: 588 return super.getNamedProperty(_hash, _name, _checkValid); 589 } 590 591 } 592 593 @Override 594 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 595 switch (hash) { 596 case 68050338: 597 /* daysOfWeek */ return this.daysOfWeek == null ? new Base[0] 598 : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 599 case -1414913477: 600 /* allDay */ return this.allDay == null ? new Base[0] : new Base[] { this.allDay }; // BooleanType 601 case -1039453818: 602 /* availableStartTime */ return this.availableStartTime == null ? new Base[0] 603 : new Base[] { this.availableStartTime }; // TimeType 604 case 101151551: 605 /* availableEndTime */ return this.availableEndTime == null ? new Base[0] 606 : new Base[] { this.availableEndTime }; // TimeType 607 default: 608 return super.getProperty(hash, name, checkValid); 609 } 610 611 } 612 613 @Override 614 public Base setProperty(int hash, String name, Base value) throws FHIRException { 615 switch (hash) { 616 case 68050338: // daysOfWeek 617 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 618 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 619 return value; 620 case -1414913477: // allDay 621 this.allDay = castToBoolean(value); // BooleanType 622 return value; 623 case -1039453818: // availableStartTime 624 this.availableStartTime = castToTime(value); // TimeType 625 return value; 626 case 101151551: // availableEndTime 627 this.availableEndTime = castToTime(value); // TimeType 628 return value; 629 default: 630 return super.setProperty(hash, name, value); 631 } 632 633 } 634 635 @Override 636 public Base setProperty(String name, Base value) throws FHIRException { 637 if (name.equals("daysOfWeek")) { 638 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 639 this.getDaysOfWeek().add((Enumeration) value); 640 } else if (name.equals("allDay")) { 641 this.allDay = castToBoolean(value); // BooleanType 642 } else if (name.equals("availableStartTime")) { 643 this.availableStartTime = castToTime(value); // TimeType 644 } else if (name.equals("availableEndTime")) { 645 this.availableEndTime = castToTime(value); // TimeType 646 } else 647 return super.setProperty(name, value); 648 return value; 649 } 650 651 @Override 652 public void removeChild(String name, Base value) throws FHIRException { 653 if (name.equals("daysOfWeek")) { 654 this.getDaysOfWeek().remove((Enumeration) value); 655 } else if (name.equals("allDay")) { 656 this.allDay = null; 657 } else if (name.equals("availableStartTime")) { 658 this.availableStartTime = null; 659 } else if (name.equals("availableEndTime")) { 660 this.availableEndTime = null; 661 } else 662 super.removeChild(name, value); 663 664 } 665 666 @Override 667 public Base makeProperty(int hash, String name) throws FHIRException { 668 switch (hash) { 669 case 68050338: 670 return addDaysOfWeekElement(); 671 case -1414913477: 672 return getAllDayElement(); 673 case -1039453818: 674 return getAvailableStartTimeElement(); 675 case 101151551: 676 return getAvailableEndTimeElement(); 677 default: 678 return super.makeProperty(hash, name); 679 } 680 681 } 682 683 @Override 684 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 685 switch (hash) { 686 case 68050338: 687 /* daysOfWeek */ return new String[] { "code" }; 688 case -1414913477: 689 /* allDay */ return new String[] { "boolean" }; 690 case -1039453818: 691 /* availableStartTime */ return new String[] { "time" }; 692 case 101151551: 693 /* availableEndTime */ return new String[] { "time" }; 694 default: 695 return super.getTypesForProperty(hash, name); 696 } 697 698 } 699 700 @Override 701 public Base addChild(String name) throws FHIRException { 702 if (name.equals("daysOfWeek")) { 703 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.daysOfWeek"); 704 } else if (name.equals("allDay")) { 705 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.allDay"); 706 } else if (name.equals("availableStartTime")) { 707 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableStartTime"); 708 } else if (name.equals("availableEndTime")) { 709 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableEndTime"); 710 } else 711 return super.addChild(name); 712 } 713 714 public PractitionerRoleAvailableTimeComponent copy() { 715 PractitionerRoleAvailableTimeComponent dst = new PractitionerRoleAvailableTimeComponent(); 716 copyValues(dst); 717 return dst; 718 } 719 720 public void copyValues(PractitionerRoleAvailableTimeComponent dst) { 721 super.copyValues(dst); 722 if (daysOfWeek != null) { 723 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 724 for (Enumeration<DaysOfWeek> i : daysOfWeek) 725 dst.daysOfWeek.add(i.copy()); 726 } 727 ; 728 dst.allDay = allDay == null ? null : allDay.copy(); 729 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 730 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 731 } 732 733 @Override 734 public boolean equalsDeep(Base other_) { 735 if (!super.equalsDeep(other_)) 736 return false; 737 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 738 return false; 739 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 740 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 741 && compareDeep(availableStartTime, o.availableStartTime, true) 742 && compareDeep(availableEndTime, o.availableEndTime, true); 743 } 744 745 @Override 746 public boolean equalsShallow(Base other_) { 747 if (!super.equalsShallow(other_)) 748 return false; 749 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 750 return false; 751 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 752 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 753 && compareValues(availableStartTime, o.availableStartTime, true) 754 && compareValues(availableEndTime, o.availableEndTime, true); 755 } 756 757 public boolean isEmpty() { 758 return super.isEmpty() 759 && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime, availableEndTime); 760 } 761 762 public String fhirType() { 763 return "PractitionerRole.availableTime"; 764 765 } 766 767 } 768 769 @Block() 770 public static class PractitionerRoleNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 771 /** 772 * The reason that can be presented to the user as to why this time is not 773 * available. 774 */ 775 @Child(name = "description", type = { 776 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 777 @Description(shortDefinition = "Reason presented to the user explaining why time not available", formalDefinition = "The reason that can be presented to the user as to why this time is not available.") 778 protected StringType description; 779 780 /** 781 * Service is not available (seasonally or for a public holiday) from this date. 782 */ 783 @Child(name = "during", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 784 @Description(shortDefinition = "Service not available from this date", formalDefinition = "Service is not available (seasonally or for a public holiday) from this date.") 785 protected Period during; 786 787 private static final long serialVersionUID = 310849929L; 788 789 /** 790 * Constructor 791 */ 792 public PractitionerRoleNotAvailableComponent() { 793 super(); 794 } 795 796 /** 797 * Constructor 798 */ 799 public PractitionerRoleNotAvailableComponent(StringType description) { 800 super(); 801 this.description = description; 802 } 803 804 /** 805 * @return {@link #description} (The reason that can be presented to the user as 806 * to why this time is not available.). This is the underlying object 807 * with id, value and extensions. The accessor "getDescription" gives 808 * direct access to the value 809 */ 810 public StringType getDescriptionElement() { 811 if (this.description == null) 812 if (Configuration.errorOnAutoCreate()) 813 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.description"); 814 else if (Configuration.doAutoCreate()) 815 this.description = new StringType(); // bb 816 return this.description; 817 } 818 819 public boolean hasDescriptionElement() { 820 return this.description != null && !this.description.isEmpty(); 821 } 822 823 public boolean hasDescription() { 824 return this.description != null && !this.description.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #description} (The reason that can be presented to the 829 * user as to why this time is not available.). This is the 830 * underlying object with id, value and extensions. The accessor 831 * "getDescription" gives direct access to the value 832 */ 833 public PractitionerRoleNotAvailableComponent setDescriptionElement(StringType value) { 834 this.description = value; 835 return this; 836 } 837 838 /** 839 * @return The reason that can be presented to the user as to why this time is 840 * not available. 841 */ 842 public String getDescription() { 843 return this.description == null ? null : this.description.getValue(); 844 } 845 846 /** 847 * @param value The reason that can be presented to the user as to why this time 848 * is not available. 849 */ 850 public PractitionerRoleNotAvailableComponent setDescription(String value) { 851 if (this.description == null) 852 this.description = new StringType(); 853 this.description.setValue(value); 854 return this; 855 } 856 857 /** 858 * @return {@link #during} (Service is not available (seasonally or for a public 859 * holiday) from this date.) 860 */ 861 public Period getDuring() { 862 if (this.during == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.during"); 865 else if (Configuration.doAutoCreate()) 866 this.during = new Period(); // cc 867 return this.during; 868 } 869 870 public boolean hasDuring() { 871 return this.during != null && !this.during.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #during} (Service is not available (seasonally or for a 876 * public holiday) from this date.) 877 */ 878 public PractitionerRoleNotAvailableComponent setDuring(Period value) { 879 this.during = value; 880 return this; 881 } 882 883 protected void listChildren(List<Property> children) { 884 super.listChildren(children); 885 children.add(new Property("description", "string", 886 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 887 children.add(new Property("during", "Period", 888 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 889 } 890 891 @Override 892 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 893 switch (_hash) { 894 case -1724546052: 895 /* description */ return new Property("description", "string", 896 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 897 case -1320499647: 898 /* during */ return new Property("during", "Period", 899 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 900 default: 901 return super.getNamedProperty(_hash, _name, _checkValid); 902 } 903 904 } 905 906 @Override 907 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 908 switch (hash) { 909 case -1724546052: 910 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 911 case -1320499647: 912 /* during */ return this.during == null ? new Base[0] : new Base[] { this.during }; // Period 913 default: 914 return super.getProperty(hash, name, checkValid); 915 } 916 917 } 918 919 @Override 920 public Base setProperty(int hash, String name, Base value) throws FHIRException { 921 switch (hash) { 922 case -1724546052: // description 923 this.description = castToString(value); // StringType 924 return value; 925 case -1320499647: // during 926 this.during = castToPeriod(value); // Period 927 return value; 928 default: 929 return super.setProperty(hash, name, value); 930 } 931 932 } 933 934 @Override 935 public Base setProperty(String name, Base value) throws FHIRException { 936 if (name.equals("description")) { 937 this.description = castToString(value); // StringType 938 } else if (name.equals("during")) { 939 this.during = castToPeriod(value); // Period 940 } else 941 return super.setProperty(name, value); 942 return value; 943 } 944 945 @Override 946 public void removeChild(String name, Base value) throws FHIRException { 947 if (name.equals("description")) { 948 this.description = null; 949 } else if (name.equals("during")) { 950 this.during = null; 951 } else 952 super.removeChild(name, value); 953 954 } 955 956 @Override 957 public Base makeProperty(int hash, String name) throws FHIRException { 958 switch (hash) { 959 case -1724546052: 960 return getDescriptionElement(); 961 case -1320499647: 962 return getDuring(); 963 default: 964 return super.makeProperty(hash, name); 965 } 966 967 } 968 969 @Override 970 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 971 switch (hash) { 972 case -1724546052: 973 /* description */ return new String[] { "string" }; 974 case -1320499647: 975 /* during */ return new String[] { "Period" }; 976 default: 977 return super.getTypesForProperty(hash, name); 978 } 979 980 } 981 982 @Override 983 public Base addChild(String name) throws FHIRException { 984 if (name.equals("description")) { 985 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.description"); 986 } else if (name.equals("during")) { 987 this.during = new Period(); 988 return this.during; 989 } else 990 return super.addChild(name); 991 } 992 993 public PractitionerRoleNotAvailableComponent copy() { 994 PractitionerRoleNotAvailableComponent dst = new PractitionerRoleNotAvailableComponent(); 995 copyValues(dst); 996 return dst; 997 } 998 999 public void copyValues(PractitionerRoleNotAvailableComponent dst) { 1000 super.copyValues(dst); 1001 dst.description = description == null ? null : description.copy(); 1002 dst.during = during == null ? null : during.copy(); 1003 } 1004 1005 @Override 1006 public boolean equalsDeep(Base other_) { 1007 if (!super.equalsDeep(other_)) 1008 return false; 1009 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 1010 return false; 1011 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 1012 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 1013 } 1014 1015 @Override 1016 public boolean equalsShallow(Base other_) { 1017 if (!super.equalsShallow(other_)) 1018 return false; 1019 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 1020 return false; 1021 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 1022 return compareValues(description, o.description, true); 1023 } 1024 1025 public boolean isEmpty() { 1026 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 1027 } 1028 1029 public String fhirType() { 1030 return "PractitionerRole.notAvailable"; 1031 1032 } 1033 1034 } 1035 1036 /** 1037 * Business Identifiers that are specific to a role/location. 1038 */ 1039 @Child(name = "identifier", type = { 1040 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1041 @Description(shortDefinition = "Business Identifiers that are specific to a role/location", formalDefinition = "Business Identifiers that are specific to a role/location.") 1042 protected List<Identifier> identifier; 1043 1044 /** 1045 * Whether this practitioner role record is in active use. 1046 */ 1047 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1048 @Description(shortDefinition = "Whether this practitioner role record is in active use", formalDefinition = "Whether this practitioner role record is in active use.") 1049 protected BooleanType active; 1050 1051 /** 1052 * The period during which the person is authorized to act as a practitioner in 1053 * these role(s) for the organization. 1054 */ 1055 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1056 @Description(shortDefinition = "The period during which the practitioner is authorized to perform in these role(s)", formalDefinition = "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.") 1057 protected Period period; 1058 1059 /** 1060 * Practitioner that is able to provide the defined services for the 1061 * organization. 1062 */ 1063 @Child(name = "practitioner", type = { 1064 Practitioner.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1065 @Description(shortDefinition = "Practitioner that is able to provide the defined services for the organization", formalDefinition = "Practitioner that is able to provide the defined services for the organization.") 1066 protected Reference practitioner; 1067 1068 /** 1069 * The actual object that is the target of the reference (Practitioner that is 1070 * able to provide the defined services for the organization.) 1071 */ 1072 protected Practitioner practitionerTarget; 1073 1074 /** 1075 * The organization where the Practitioner performs the roles associated. 1076 */ 1077 @Child(name = "organization", type = { 1078 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1079 @Description(shortDefinition = "Organization where the roles are available", formalDefinition = "The organization where the Practitioner performs the roles associated.") 1080 protected Reference organization; 1081 1082 /** 1083 * The actual object that is the target of the reference (The organization where 1084 * the Practitioner performs the roles associated.) 1085 */ 1086 protected Organization organizationTarget; 1087 1088 /** 1089 * Roles which this practitioner is authorized to perform for the organization. 1090 */ 1091 @Child(name = "code", type = { 1092 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1093 @Description(shortDefinition = "Roles which this practitioner may perform", formalDefinition = "Roles which this practitioner is authorized to perform for the organization.") 1094 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/practitioner-role") 1095 protected List<CodeableConcept> code; 1096 1097 /** 1098 * Specific specialty of the practitioner. 1099 */ 1100 @Child(name = "specialty", type = { 1101 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1102 @Description(shortDefinition = "Specific specialty of the practitioner", formalDefinition = "Specific specialty of the practitioner.") 1103 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 1104 protected List<CodeableConcept> specialty; 1105 1106 /** 1107 * The location(s) at which this practitioner provides care. 1108 */ 1109 @Child(name = "location", type = { 1110 Location.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1111 @Description(shortDefinition = "The location(s) at which this practitioner provides care", formalDefinition = "The location(s) at which this practitioner provides care.") 1112 protected List<Reference> location; 1113 /** 1114 * The actual objects that are the target of the reference (The location(s) at 1115 * which this practitioner provides care.) 1116 */ 1117 protected List<Location> locationTarget; 1118 1119 /** 1120 * The list of healthcare services that this worker provides for this role's 1121 * Organization/Location(s). 1122 */ 1123 @Child(name = "healthcareService", type = { 1124 HealthcareService.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1125 @Description(shortDefinition = "The list of healthcare services that this worker provides for this role's Organization/Location(s)", formalDefinition = "The list of healthcare services that this worker provides for this role's Organization/Location(s).") 1126 protected List<Reference> healthcareService; 1127 /** 1128 * The actual objects that are the target of the reference (The list of 1129 * healthcare services that this worker provides for this role's 1130 * Organization/Location(s).) 1131 */ 1132 protected List<HealthcareService> healthcareServiceTarget; 1133 1134 /** 1135 * Contact details that are specific to the role/location/service. 1136 */ 1137 @Child(name = "telecom", type = { 1138 ContactPoint.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1139 @Description(shortDefinition = "Contact details that are specific to the role/location/service", formalDefinition = "Contact details that are specific to the role/location/service.") 1140 protected List<ContactPoint> telecom; 1141 1142 /** 1143 * A collection of times the practitioner is available or performing this role 1144 * at the location and/or healthcareservice. 1145 */ 1146 @Child(name = "availableTime", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1147 @Description(shortDefinition = "Times the Service Site is available", formalDefinition = "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.") 1148 protected List<PractitionerRoleAvailableTimeComponent> availableTime; 1149 1150 /** 1151 * The practitioner is not available or performing this role during this period 1152 * of time due to the provided reason. 1153 */ 1154 @Child(name = "notAvailable", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1155 @Description(shortDefinition = "Not available during this time due to provided reason", formalDefinition = "The practitioner is not available or performing this role during this period of time due to the provided reason.") 1156 protected List<PractitionerRoleNotAvailableComponent> notAvailable; 1157 1158 /** 1159 * A description of site availability exceptions, e.g. public holiday 1160 * availability. Succinctly describing all possible exceptions to normal site 1161 * availability as details in the available Times and not available Times. 1162 */ 1163 @Child(name = "availabilityExceptions", type = { 1164 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1165 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.") 1166 protected StringType availabilityExceptions; 1167 1168 /** 1169 * Technical endpoints providing access to services operated for the 1170 * practitioner with this role. 1171 */ 1172 @Child(name = "endpoint", type = { 1173 Endpoint.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1174 @Description(shortDefinition = "Technical endpoints providing access to services operated for the practitioner with this role", formalDefinition = "Technical endpoints providing access to services operated for the practitioner with this role.") 1175 protected List<Reference> endpoint; 1176 /** 1177 * The actual objects that are the target of the reference (Technical endpoints 1178 * providing access to services operated for the practitioner with this role.) 1179 */ 1180 protected List<Endpoint> endpointTarget; 1181 1182 private static final long serialVersionUID = 423338051L; 1183 1184 /** 1185 * Constructor 1186 */ 1187 public PractitionerRole() { 1188 super(); 1189 } 1190 1191 /** 1192 * @return {@link #identifier} (Business Identifiers that are specific to a 1193 * role/location.) 1194 */ 1195 public List<Identifier> getIdentifier() { 1196 if (this.identifier == null) 1197 this.identifier = new ArrayList<Identifier>(); 1198 return this.identifier; 1199 } 1200 1201 /** 1202 * @return Returns a reference to <code>this</code> for easy method chaining 1203 */ 1204 public PractitionerRole setIdentifier(List<Identifier> theIdentifier) { 1205 this.identifier = theIdentifier; 1206 return this; 1207 } 1208 1209 public boolean hasIdentifier() { 1210 if (this.identifier == null) 1211 return false; 1212 for (Identifier item : this.identifier) 1213 if (!item.isEmpty()) 1214 return true; 1215 return false; 1216 } 1217 1218 public Identifier addIdentifier() { // 3 1219 Identifier t = new Identifier(); 1220 if (this.identifier == null) 1221 this.identifier = new ArrayList<Identifier>(); 1222 this.identifier.add(t); 1223 return t; 1224 } 1225 1226 public PractitionerRole addIdentifier(Identifier t) { // 3 1227 if (t == null) 1228 return this; 1229 if (this.identifier == null) 1230 this.identifier = new ArrayList<Identifier>(); 1231 this.identifier.add(t); 1232 return this; 1233 } 1234 1235 /** 1236 * @return The first repetition of repeating field {@link #identifier}, creating 1237 * it if it does not already exist 1238 */ 1239 public Identifier getIdentifierFirstRep() { 1240 if (getIdentifier().isEmpty()) { 1241 addIdentifier(); 1242 } 1243 return getIdentifier().get(0); 1244 } 1245 1246 /** 1247 * @return {@link #active} (Whether this practitioner role record is in active 1248 * use.). This is the underlying object with id, value and extensions. 1249 * The accessor "getActive" gives direct access to the value 1250 */ 1251 public BooleanType getActiveElement() { 1252 if (this.active == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create PractitionerRole.active"); 1255 else if (Configuration.doAutoCreate()) 1256 this.active = new BooleanType(); // bb 1257 return this.active; 1258 } 1259 1260 public boolean hasActiveElement() { 1261 return this.active != null && !this.active.isEmpty(); 1262 } 1263 1264 public boolean hasActive() { 1265 return this.active != null && !this.active.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #active} (Whether this practitioner role record is in 1270 * active use.). This is the underlying object with id, value and 1271 * extensions. The accessor "getActive" gives direct access to the 1272 * value 1273 */ 1274 public PractitionerRole setActiveElement(BooleanType value) { 1275 this.active = value; 1276 return this; 1277 } 1278 1279 /** 1280 * @return Whether this practitioner role record is in active use. 1281 */ 1282 public boolean getActive() { 1283 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1284 } 1285 1286 /** 1287 * @param value Whether this practitioner role record is in active use. 1288 */ 1289 public PractitionerRole setActive(boolean value) { 1290 if (this.active == null) 1291 this.active = new BooleanType(); 1292 this.active.setValue(value); 1293 return this; 1294 } 1295 1296 /** 1297 * @return {@link #period} (The period during which the person is authorized to 1298 * act as a practitioner in these role(s) for the organization.) 1299 */ 1300 public Period getPeriod() { 1301 if (this.period == null) 1302 if (Configuration.errorOnAutoCreate()) 1303 throw new Error("Attempt to auto-create PractitionerRole.period"); 1304 else if (Configuration.doAutoCreate()) 1305 this.period = new Period(); // cc 1306 return this.period; 1307 } 1308 1309 public boolean hasPeriod() { 1310 return this.period != null && !this.period.isEmpty(); 1311 } 1312 1313 /** 1314 * @param value {@link #period} (The period during which the person is 1315 * authorized to act as a practitioner in these role(s) for the 1316 * organization.) 1317 */ 1318 public PractitionerRole setPeriod(Period value) { 1319 this.period = value; 1320 return this; 1321 } 1322 1323 /** 1324 * @return {@link #practitioner} (Practitioner that is able to provide the 1325 * defined services for the organization.) 1326 */ 1327 public Reference getPractitioner() { 1328 if (this.practitioner == null) 1329 if (Configuration.errorOnAutoCreate()) 1330 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1331 else if (Configuration.doAutoCreate()) 1332 this.practitioner = new Reference(); // cc 1333 return this.practitioner; 1334 } 1335 1336 public boolean hasPractitioner() { 1337 return this.practitioner != null && !this.practitioner.isEmpty(); 1338 } 1339 1340 /** 1341 * @param value {@link #practitioner} (Practitioner that is able to provide the 1342 * defined services for the organization.) 1343 */ 1344 public PractitionerRole setPractitioner(Reference value) { 1345 this.practitioner = value; 1346 return this; 1347 } 1348 1349 /** 1350 * @return {@link #practitioner} The actual object that is the target of the 1351 * reference. The reference library doesn't populate this, but you can 1352 * use it to hold the resource if you resolve it. (Practitioner that is 1353 * able to provide the defined services for the organization.) 1354 */ 1355 public Practitioner getPractitionerTarget() { 1356 if (this.practitionerTarget == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1359 else if (Configuration.doAutoCreate()) 1360 this.practitionerTarget = new Practitioner(); // aa 1361 return this.practitionerTarget; 1362 } 1363 1364 /** 1365 * @param value {@link #practitioner} The actual object that is the target of 1366 * the reference. The reference library doesn't use these, but you 1367 * can use it to hold the resource if you resolve it. (Practitioner 1368 * that is able to provide the defined services for the 1369 * organization.) 1370 */ 1371 public PractitionerRole setPractitionerTarget(Practitioner value) { 1372 this.practitionerTarget = value; 1373 return this; 1374 } 1375 1376 /** 1377 * @return {@link #organization} (The organization where the Practitioner 1378 * performs the roles associated.) 1379 */ 1380 public Reference getOrganization() { 1381 if (this.organization == null) 1382 if (Configuration.errorOnAutoCreate()) 1383 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1384 else if (Configuration.doAutoCreate()) 1385 this.organization = new Reference(); // cc 1386 return this.organization; 1387 } 1388 1389 public boolean hasOrganization() { 1390 return this.organization != null && !this.organization.isEmpty(); 1391 } 1392 1393 /** 1394 * @param value {@link #organization} (The organization where the Practitioner 1395 * performs the roles associated.) 1396 */ 1397 public PractitionerRole setOrganization(Reference value) { 1398 this.organization = value; 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #organization} The actual object that is the target of the 1404 * reference. The reference library doesn't populate this, but you can 1405 * use it to hold the resource if you resolve it. (The organization 1406 * where the Practitioner performs the roles associated.) 1407 */ 1408 public Organization getOrganizationTarget() { 1409 if (this.organizationTarget == null) 1410 if (Configuration.errorOnAutoCreate()) 1411 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1412 else if (Configuration.doAutoCreate()) 1413 this.organizationTarget = new Organization(); // aa 1414 return this.organizationTarget; 1415 } 1416 1417 /** 1418 * @param value {@link #organization} The actual object that is the target of 1419 * the reference. The reference library doesn't use these, but you 1420 * can use it to hold the resource if you resolve it. (The 1421 * organization where the Practitioner performs the roles 1422 * associated.) 1423 */ 1424 public PractitionerRole setOrganizationTarget(Organization value) { 1425 this.organizationTarget = value; 1426 return this; 1427 } 1428 1429 /** 1430 * @return {@link #code} (Roles which this practitioner is authorized to perform 1431 * for the organization.) 1432 */ 1433 public List<CodeableConcept> getCode() { 1434 if (this.code == null) 1435 this.code = new ArrayList<CodeableConcept>(); 1436 return this.code; 1437 } 1438 1439 /** 1440 * @return Returns a reference to <code>this</code> for easy method chaining 1441 */ 1442 public PractitionerRole setCode(List<CodeableConcept> theCode) { 1443 this.code = theCode; 1444 return this; 1445 } 1446 1447 public boolean hasCode() { 1448 if (this.code == null) 1449 return false; 1450 for (CodeableConcept item : this.code) 1451 if (!item.isEmpty()) 1452 return true; 1453 return false; 1454 } 1455 1456 public CodeableConcept addCode() { // 3 1457 CodeableConcept t = new CodeableConcept(); 1458 if (this.code == null) 1459 this.code = new ArrayList<CodeableConcept>(); 1460 this.code.add(t); 1461 return t; 1462 } 1463 1464 public PractitionerRole addCode(CodeableConcept t) { // 3 1465 if (t == null) 1466 return this; 1467 if (this.code == null) 1468 this.code = new ArrayList<CodeableConcept>(); 1469 this.code.add(t); 1470 return this; 1471 } 1472 1473 /** 1474 * @return The first repetition of repeating field {@link #code}, creating it if 1475 * it does not already exist 1476 */ 1477 public CodeableConcept getCodeFirstRep() { 1478 if (getCode().isEmpty()) { 1479 addCode(); 1480 } 1481 return getCode().get(0); 1482 } 1483 1484 /** 1485 * @return {@link #specialty} (Specific specialty of the practitioner.) 1486 */ 1487 public List<CodeableConcept> getSpecialty() { 1488 if (this.specialty == null) 1489 this.specialty = new ArrayList<CodeableConcept>(); 1490 return this.specialty; 1491 } 1492 1493 /** 1494 * @return Returns a reference to <code>this</code> for easy method chaining 1495 */ 1496 public PractitionerRole setSpecialty(List<CodeableConcept> theSpecialty) { 1497 this.specialty = theSpecialty; 1498 return this; 1499 } 1500 1501 public boolean hasSpecialty() { 1502 if (this.specialty == null) 1503 return false; 1504 for (CodeableConcept item : this.specialty) 1505 if (!item.isEmpty()) 1506 return true; 1507 return false; 1508 } 1509 1510 public CodeableConcept addSpecialty() { // 3 1511 CodeableConcept t = new CodeableConcept(); 1512 if (this.specialty == null) 1513 this.specialty = new ArrayList<CodeableConcept>(); 1514 this.specialty.add(t); 1515 return t; 1516 } 1517 1518 public PractitionerRole addSpecialty(CodeableConcept t) { // 3 1519 if (t == null) 1520 return this; 1521 if (this.specialty == null) 1522 this.specialty = new ArrayList<CodeableConcept>(); 1523 this.specialty.add(t); 1524 return this; 1525 } 1526 1527 /** 1528 * @return The first repetition of repeating field {@link #specialty}, creating 1529 * it if it does not already exist 1530 */ 1531 public CodeableConcept getSpecialtyFirstRep() { 1532 if (getSpecialty().isEmpty()) { 1533 addSpecialty(); 1534 } 1535 return getSpecialty().get(0); 1536 } 1537 1538 /** 1539 * @return {@link #location} (The location(s) at which this practitioner 1540 * provides care.) 1541 */ 1542 public List<Reference> getLocation() { 1543 if (this.location == null) 1544 this.location = new ArrayList<Reference>(); 1545 return this.location; 1546 } 1547 1548 /** 1549 * @return Returns a reference to <code>this</code> for easy method chaining 1550 */ 1551 public PractitionerRole setLocation(List<Reference> theLocation) { 1552 this.location = theLocation; 1553 return this; 1554 } 1555 1556 public boolean hasLocation() { 1557 if (this.location == null) 1558 return false; 1559 for (Reference item : this.location) 1560 if (!item.isEmpty()) 1561 return true; 1562 return false; 1563 } 1564 1565 public Reference addLocation() { // 3 1566 Reference t = new Reference(); 1567 if (this.location == null) 1568 this.location = new ArrayList<Reference>(); 1569 this.location.add(t); 1570 return t; 1571 } 1572 1573 public PractitionerRole addLocation(Reference t) { // 3 1574 if (t == null) 1575 return this; 1576 if (this.location == null) 1577 this.location = new ArrayList<Reference>(); 1578 this.location.add(t); 1579 return this; 1580 } 1581 1582 /** 1583 * @return The first repetition of repeating field {@link #location}, creating 1584 * it if it does not already exist 1585 */ 1586 public Reference getLocationFirstRep() { 1587 if (getLocation().isEmpty()) { 1588 addLocation(); 1589 } 1590 return getLocation().get(0); 1591 } 1592 1593 /** 1594 * @deprecated Use Reference#setResource(IBaseResource) instead 1595 */ 1596 @Deprecated 1597 public List<Location> getLocationTarget() { 1598 if (this.locationTarget == null) 1599 this.locationTarget = new ArrayList<Location>(); 1600 return this.locationTarget; 1601 } 1602 1603 /** 1604 * @deprecated Use Reference#setResource(IBaseResource) instead 1605 */ 1606 @Deprecated 1607 public Location addLocationTarget() { 1608 Location r = new Location(); 1609 if (this.locationTarget == null) 1610 this.locationTarget = new ArrayList<Location>(); 1611 this.locationTarget.add(r); 1612 return r; 1613 } 1614 1615 /** 1616 * @return {@link #healthcareService} (The list of healthcare services that this 1617 * worker provides for this role's Organization/Location(s).) 1618 */ 1619 public List<Reference> getHealthcareService() { 1620 if (this.healthcareService == null) 1621 this.healthcareService = new ArrayList<Reference>(); 1622 return this.healthcareService; 1623 } 1624 1625 /** 1626 * @return Returns a reference to <code>this</code> for easy method chaining 1627 */ 1628 public PractitionerRole setHealthcareService(List<Reference> theHealthcareService) { 1629 this.healthcareService = theHealthcareService; 1630 return this; 1631 } 1632 1633 public boolean hasHealthcareService() { 1634 if (this.healthcareService == null) 1635 return false; 1636 for (Reference item : this.healthcareService) 1637 if (!item.isEmpty()) 1638 return true; 1639 return false; 1640 } 1641 1642 public Reference addHealthcareService() { // 3 1643 Reference t = new Reference(); 1644 if (this.healthcareService == null) 1645 this.healthcareService = new ArrayList<Reference>(); 1646 this.healthcareService.add(t); 1647 return t; 1648 } 1649 1650 public PractitionerRole addHealthcareService(Reference t) { // 3 1651 if (t == null) 1652 return this; 1653 if (this.healthcareService == null) 1654 this.healthcareService = new ArrayList<Reference>(); 1655 this.healthcareService.add(t); 1656 return this; 1657 } 1658 1659 /** 1660 * @return The first repetition of repeating field {@link #healthcareService}, 1661 * creating it if it does not already exist 1662 */ 1663 public Reference getHealthcareServiceFirstRep() { 1664 if (getHealthcareService().isEmpty()) { 1665 addHealthcareService(); 1666 } 1667 return getHealthcareService().get(0); 1668 } 1669 1670 /** 1671 * @deprecated Use Reference#setResource(IBaseResource) instead 1672 */ 1673 @Deprecated 1674 public List<HealthcareService> getHealthcareServiceTarget() { 1675 if (this.healthcareServiceTarget == null) 1676 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 1677 return this.healthcareServiceTarget; 1678 } 1679 1680 /** 1681 * @deprecated Use Reference#setResource(IBaseResource) instead 1682 */ 1683 @Deprecated 1684 public HealthcareService addHealthcareServiceTarget() { 1685 HealthcareService r = new HealthcareService(); 1686 if (this.healthcareServiceTarget == null) 1687 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 1688 this.healthcareServiceTarget.add(r); 1689 return r; 1690 } 1691 1692 /** 1693 * @return {@link #telecom} (Contact details that are specific to the 1694 * role/location/service.) 1695 */ 1696 public List<ContactPoint> getTelecom() { 1697 if (this.telecom == null) 1698 this.telecom = new ArrayList<ContactPoint>(); 1699 return this.telecom; 1700 } 1701 1702 /** 1703 * @return Returns a reference to <code>this</code> for easy method chaining 1704 */ 1705 public PractitionerRole setTelecom(List<ContactPoint> theTelecom) { 1706 this.telecom = theTelecom; 1707 return this; 1708 } 1709 1710 public boolean hasTelecom() { 1711 if (this.telecom == null) 1712 return false; 1713 for (ContactPoint item : this.telecom) 1714 if (!item.isEmpty()) 1715 return true; 1716 return false; 1717 } 1718 1719 public ContactPoint addTelecom() { // 3 1720 ContactPoint t = new ContactPoint(); 1721 if (this.telecom == null) 1722 this.telecom = new ArrayList<ContactPoint>(); 1723 this.telecom.add(t); 1724 return t; 1725 } 1726 1727 public PractitionerRole addTelecom(ContactPoint t) { // 3 1728 if (t == null) 1729 return this; 1730 if (this.telecom == null) 1731 this.telecom = new ArrayList<ContactPoint>(); 1732 this.telecom.add(t); 1733 return this; 1734 } 1735 1736 /** 1737 * @return The first repetition of repeating field {@link #telecom}, creating it 1738 * if it does not already exist 1739 */ 1740 public ContactPoint getTelecomFirstRep() { 1741 if (getTelecom().isEmpty()) { 1742 addTelecom(); 1743 } 1744 return getTelecom().get(0); 1745 } 1746 1747 /** 1748 * @return {@link #availableTime} (A collection of times the practitioner is 1749 * available or performing this role at the location and/or 1750 * healthcareservice.) 1751 */ 1752 public List<PractitionerRoleAvailableTimeComponent> getAvailableTime() { 1753 if (this.availableTime == null) 1754 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1755 return this.availableTime; 1756 } 1757 1758 /** 1759 * @return Returns a reference to <code>this</code> for easy method chaining 1760 */ 1761 public PractitionerRole setAvailableTime(List<PractitionerRoleAvailableTimeComponent> theAvailableTime) { 1762 this.availableTime = theAvailableTime; 1763 return this; 1764 } 1765 1766 public boolean hasAvailableTime() { 1767 if (this.availableTime == null) 1768 return false; 1769 for (PractitionerRoleAvailableTimeComponent item : this.availableTime) 1770 if (!item.isEmpty()) 1771 return true; 1772 return false; 1773 } 1774 1775 public PractitionerRoleAvailableTimeComponent addAvailableTime() { // 3 1776 PractitionerRoleAvailableTimeComponent t = new PractitionerRoleAvailableTimeComponent(); 1777 if (this.availableTime == null) 1778 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1779 this.availableTime.add(t); 1780 return t; 1781 } 1782 1783 public PractitionerRole addAvailableTime(PractitionerRoleAvailableTimeComponent t) { // 3 1784 if (t == null) 1785 return this; 1786 if (this.availableTime == null) 1787 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1788 this.availableTime.add(t); 1789 return this; 1790 } 1791 1792 /** 1793 * @return The first repetition of repeating field {@link #availableTime}, 1794 * creating it if it does not already exist 1795 */ 1796 public PractitionerRoleAvailableTimeComponent getAvailableTimeFirstRep() { 1797 if (getAvailableTime().isEmpty()) { 1798 addAvailableTime(); 1799 } 1800 return getAvailableTime().get(0); 1801 } 1802 1803 /** 1804 * @return {@link #notAvailable} (The practitioner is not available or 1805 * performing this role during this period of time due to the provided 1806 * reason.) 1807 */ 1808 public List<PractitionerRoleNotAvailableComponent> getNotAvailable() { 1809 if (this.notAvailable == null) 1810 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1811 return this.notAvailable; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public PractitionerRole setNotAvailable(List<PractitionerRoleNotAvailableComponent> theNotAvailable) { 1818 this.notAvailable = theNotAvailable; 1819 return this; 1820 } 1821 1822 public boolean hasNotAvailable() { 1823 if (this.notAvailable == null) 1824 return false; 1825 for (PractitionerRoleNotAvailableComponent item : this.notAvailable) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 public PractitionerRoleNotAvailableComponent addNotAvailable() { // 3 1832 PractitionerRoleNotAvailableComponent t = new PractitionerRoleNotAvailableComponent(); 1833 if (this.notAvailable == null) 1834 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1835 this.notAvailable.add(t); 1836 return t; 1837 } 1838 1839 public PractitionerRole addNotAvailable(PractitionerRoleNotAvailableComponent t) { // 3 1840 if (t == null) 1841 return this; 1842 if (this.notAvailable == null) 1843 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1844 this.notAvailable.add(t); 1845 return this; 1846 } 1847 1848 /** 1849 * @return The first repetition of repeating field {@link #notAvailable}, 1850 * creating it if it does not already exist 1851 */ 1852 public PractitionerRoleNotAvailableComponent getNotAvailableFirstRep() { 1853 if (getNotAvailable().isEmpty()) { 1854 addNotAvailable(); 1855 } 1856 return getNotAvailable().get(0); 1857 } 1858 1859 /** 1860 * @return {@link #availabilityExceptions} (A description of site availability 1861 * exceptions, e.g. public holiday availability. Succinctly describing 1862 * all possible exceptions to normal site availability as details in the 1863 * available Times and not available Times.). This is the underlying 1864 * object with id, value and extensions. The accessor 1865 * "getAvailabilityExceptions" gives direct access to the value 1866 */ 1867 public StringType getAvailabilityExceptionsElement() { 1868 if (this.availabilityExceptions == null) 1869 if (Configuration.errorOnAutoCreate()) 1870 throw new Error("Attempt to auto-create PractitionerRole.availabilityExceptions"); 1871 else if (Configuration.doAutoCreate()) 1872 this.availabilityExceptions = new StringType(); // bb 1873 return this.availabilityExceptions; 1874 } 1875 1876 public boolean hasAvailabilityExceptionsElement() { 1877 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1878 } 1879 1880 public boolean hasAvailabilityExceptions() { 1881 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1882 } 1883 1884 /** 1885 * @param value {@link #availabilityExceptions} (A description of site 1886 * availability exceptions, e.g. public holiday availability. 1887 * Succinctly describing all possible exceptions to normal site 1888 * availability as details in the available Times and not available 1889 * Times.). This is the underlying object with id, value and 1890 * extensions. The accessor "getAvailabilityExceptions" gives 1891 * direct access to the value 1892 */ 1893 public PractitionerRole setAvailabilityExceptionsElement(StringType value) { 1894 this.availabilityExceptions = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return A description of site availability exceptions, e.g. public holiday 1900 * availability. Succinctly describing all possible exceptions to normal 1901 * site availability as details in the available Times and not available 1902 * Times. 1903 */ 1904 public String getAvailabilityExceptions() { 1905 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 1906 } 1907 1908 /** 1909 * @param value A description of site availability exceptions, e.g. public 1910 * holiday availability. Succinctly describing all possible 1911 * exceptions to normal site availability as details in the 1912 * available Times and not available Times. 1913 */ 1914 public PractitionerRole setAvailabilityExceptions(String value) { 1915 if (Utilities.noString(value)) 1916 this.availabilityExceptions = null; 1917 else { 1918 if (this.availabilityExceptions == null) 1919 this.availabilityExceptions = new StringType(); 1920 this.availabilityExceptions.setValue(value); 1921 } 1922 return this; 1923 } 1924 1925 /** 1926 * @return {@link #endpoint} (Technical endpoints providing access to services 1927 * operated for the practitioner with this role.) 1928 */ 1929 public List<Reference> getEndpoint() { 1930 if (this.endpoint == null) 1931 this.endpoint = new ArrayList<Reference>(); 1932 return this.endpoint; 1933 } 1934 1935 /** 1936 * @return Returns a reference to <code>this</code> for easy method chaining 1937 */ 1938 public PractitionerRole setEndpoint(List<Reference> theEndpoint) { 1939 this.endpoint = theEndpoint; 1940 return this; 1941 } 1942 1943 public boolean hasEndpoint() { 1944 if (this.endpoint == null) 1945 return false; 1946 for (Reference item : this.endpoint) 1947 if (!item.isEmpty()) 1948 return true; 1949 return false; 1950 } 1951 1952 public Reference addEndpoint() { // 3 1953 Reference t = new Reference(); 1954 if (this.endpoint == null) 1955 this.endpoint = new ArrayList<Reference>(); 1956 this.endpoint.add(t); 1957 return t; 1958 } 1959 1960 public PractitionerRole addEndpoint(Reference t) { // 3 1961 if (t == null) 1962 return this; 1963 if (this.endpoint == null) 1964 this.endpoint = new ArrayList<Reference>(); 1965 this.endpoint.add(t); 1966 return this; 1967 } 1968 1969 /** 1970 * @return The first repetition of repeating field {@link #endpoint}, creating 1971 * it if it does not already exist 1972 */ 1973 public Reference getEndpointFirstRep() { 1974 if (getEndpoint().isEmpty()) { 1975 addEndpoint(); 1976 } 1977 return getEndpoint().get(0); 1978 } 1979 1980 /** 1981 * @deprecated Use Reference#setResource(IBaseResource) instead 1982 */ 1983 @Deprecated 1984 public List<Endpoint> getEndpointTarget() { 1985 if (this.endpointTarget == null) 1986 this.endpointTarget = new ArrayList<Endpoint>(); 1987 return this.endpointTarget; 1988 } 1989 1990 /** 1991 * @deprecated Use Reference#setResource(IBaseResource) instead 1992 */ 1993 @Deprecated 1994 public Endpoint addEndpointTarget() { 1995 Endpoint r = new Endpoint(); 1996 if (this.endpointTarget == null) 1997 this.endpointTarget = new ArrayList<Endpoint>(); 1998 this.endpointTarget.add(r); 1999 return r; 2000 } 2001 2002 protected void listChildren(List<Property> children) { 2003 super.listChildren(children); 2004 children.add(new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 2005 0, java.lang.Integer.MAX_VALUE, identifier)); 2006 children.add( 2007 new Property("active", "boolean", "Whether this practitioner role record is in active use.", 0, 1, active)); 2008 children.add(new Property("period", "Period", 2009 "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 2010 0, 1, period)); 2011 children.add(new Property("practitioner", "Reference(Practitioner)", 2012 "Practitioner that is able to provide the defined services for the organization.", 0, 1, practitioner)); 2013 children.add(new Property("organization", "Reference(Organization)", 2014 "The organization where the Practitioner performs the roles associated.", 0, 1, organization)); 2015 children.add(new Property("code", "CodeableConcept", 2016 "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, 2017 code)); 2018 children.add(new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, 2019 java.lang.Integer.MAX_VALUE, specialty)); 2020 children.add(new Property("location", "Reference(Location)", 2021 "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location)); 2022 children.add(new Property("healthcareService", "Reference(HealthcareService)", 2023 "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, 2024 java.lang.Integer.MAX_VALUE, healthcareService)); 2025 children.add(new Property("telecom", "ContactPoint", 2026 "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2027 children.add(new Property("availableTime", "", 2028 "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.", 2029 0, java.lang.Integer.MAX_VALUE, availableTime)); 2030 children.add(new Property("notAvailable", "", 2031 "The practitioner is not available or performing this role during this period of time due to the provided reason.", 2032 0, java.lang.Integer.MAX_VALUE, notAvailable)); 2033 children.add(new Property("availabilityExceptions", "string", 2034 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2035 0, 1, availabilityExceptions)); 2036 children.add(new Property("endpoint", "Reference(Endpoint)", 2037 "Technical endpoints providing access to services operated for the practitioner with this role.", 0, 2038 java.lang.Integer.MAX_VALUE, endpoint)); 2039 } 2040 2041 @Override 2042 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2043 switch (_hash) { 2044 case -1618432855: 2045 /* identifier */ return new Property("identifier", "Identifier", 2046 "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier); 2047 case -1422950650: 2048 /* active */ return new Property("active", "boolean", "Whether this practitioner role record is in active use.", 2049 0, 1, active); 2050 case -991726143: 2051 /* period */ return new Property("period", "Period", 2052 "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 2053 0, 1, period); 2054 case 574573338: 2055 /* practitioner */ return new Property("practitioner", "Reference(Practitioner)", 2056 "Practitioner that is able to provide the defined services for the organization.", 0, 1, practitioner); 2057 case 1178922291: 2058 /* organization */ return new Property("organization", "Reference(Organization)", 2059 "The organization where the Practitioner performs the roles associated.", 0, 1, organization); 2060 case 3059181: 2061 /* code */ return new Property("code", "CodeableConcept", 2062 "Roles which this practitioner is authorized to perform for the organization.", 0, 2063 java.lang.Integer.MAX_VALUE, code); 2064 case -1694759682: 2065 /* specialty */ return new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, 2066 java.lang.Integer.MAX_VALUE, specialty); 2067 case 1901043637: 2068 /* location */ return new Property("location", "Reference(Location)", 2069 "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location); 2070 case 1289661064: 2071 /* healthcareService */ return new Property("healthcareService", "Reference(HealthcareService)", 2072 "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, 2073 java.lang.Integer.MAX_VALUE, healthcareService); 2074 case -1429363305: 2075 /* telecom */ return new Property("telecom", "ContactPoint", 2076 "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom); 2077 case 1873069366: 2078 /* availableTime */ return new Property("availableTime", "", 2079 "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.", 2080 0, java.lang.Integer.MAX_VALUE, availableTime); 2081 case -629572298: 2082 /* notAvailable */ return new Property("notAvailable", "", 2083 "The practitioner is not available or performing this role during this period of time due to the provided reason.", 2084 0, java.lang.Integer.MAX_VALUE, notAvailable); 2085 case -1149143617: 2086 /* availabilityExceptions */ return new Property("availabilityExceptions", "string", 2087 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2088 0, 1, availabilityExceptions); 2089 case 1741102485: 2090 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 2091 "Technical endpoints providing access to services operated for the practitioner with this role.", 0, 2092 java.lang.Integer.MAX_VALUE, endpoint); 2093 default: 2094 return super.getNamedProperty(_hash, _name, _checkValid); 2095 } 2096 2097 } 2098 2099 @Override 2100 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2101 switch (hash) { 2102 case -1618432855: 2103 /* identifier */ return this.identifier == null ? new Base[0] 2104 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2105 case -1422950650: 2106 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 2107 case -991726143: 2108 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2109 case 574573338: 2110 /* practitioner */ return this.practitioner == null ? new Base[0] : new Base[] { this.practitioner }; // Reference 2111 case 1178922291: 2112 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 2113 case 3059181: 2114 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 2115 case -1694759682: 2116 /* specialty */ return this.specialty == null ? new Base[0] 2117 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 2118 case 1901043637: 2119 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 2120 case 1289661064: 2121 /* healthcareService */ return this.healthcareService == null ? new Base[0] 2122 : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 2123 case -1429363305: 2124 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2125 case 1873069366: 2126 /* availableTime */ return this.availableTime == null ? new Base[0] 2127 : this.availableTime.toArray(new Base[this.availableTime.size()]); // PractitionerRoleAvailableTimeComponent 2128 case -629572298: 2129 /* notAvailable */ return this.notAvailable == null ? new Base[0] 2130 : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // PractitionerRoleNotAvailableComponent 2131 case -1149143617: 2132 /* availabilityExceptions */ return this.availabilityExceptions == null ? new Base[0] 2133 : new Base[] { this.availabilityExceptions }; // StringType 2134 case 1741102485: 2135 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2136 default: 2137 return super.getProperty(hash, name, checkValid); 2138 } 2139 2140 } 2141 2142 @Override 2143 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2144 switch (hash) { 2145 case -1618432855: // identifier 2146 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2147 return value; 2148 case -1422950650: // active 2149 this.active = castToBoolean(value); // BooleanType 2150 return value; 2151 case -991726143: // period 2152 this.period = castToPeriod(value); // Period 2153 return value; 2154 case 574573338: // practitioner 2155 this.practitioner = castToReference(value); // Reference 2156 return value; 2157 case 1178922291: // organization 2158 this.organization = castToReference(value); // Reference 2159 return value; 2160 case 3059181: // code 2161 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 2162 return value; 2163 case -1694759682: // specialty 2164 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 2165 return value; 2166 case 1901043637: // location 2167 this.getLocation().add(castToReference(value)); // Reference 2168 return value; 2169 case 1289661064: // healthcareService 2170 this.getHealthcareService().add(castToReference(value)); // Reference 2171 return value; 2172 case -1429363305: // telecom 2173 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2174 return value; 2175 case 1873069366: // availableTime 2176 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); // PractitionerRoleAvailableTimeComponent 2177 return value; 2178 case -629572298: // notAvailable 2179 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); // PractitionerRoleNotAvailableComponent 2180 return value; 2181 case -1149143617: // availabilityExceptions 2182 this.availabilityExceptions = castToString(value); // StringType 2183 return value; 2184 case 1741102485: // endpoint 2185 this.getEndpoint().add(castToReference(value)); // Reference 2186 return value; 2187 default: 2188 return super.setProperty(hash, name, value); 2189 } 2190 2191 } 2192 2193 @Override 2194 public Base setProperty(String name, Base value) throws FHIRException { 2195 if (name.equals("identifier")) { 2196 this.getIdentifier().add(castToIdentifier(value)); 2197 } else if (name.equals("active")) { 2198 this.active = castToBoolean(value); // BooleanType 2199 } else if (name.equals("period")) { 2200 this.period = castToPeriod(value); // Period 2201 } else if (name.equals("practitioner")) { 2202 this.practitioner = castToReference(value); // Reference 2203 } else if (name.equals("organization")) { 2204 this.organization = castToReference(value); // Reference 2205 } else if (name.equals("code")) { 2206 this.getCode().add(castToCodeableConcept(value)); 2207 } else if (name.equals("specialty")) { 2208 this.getSpecialty().add(castToCodeableConcept(value)); 2209 } else if (name.equals("location")) { 2210 this.getLocation().add(castToReference(value)); 2211 } else if (name.equals("healthcareService")) { 2212 this.getHealthcareService().add(castToReference(value)); 2213 } else if (name.equals("telecom")) { 2214 this.getTelecom().add(castToContactPoint(value)); 2215 } else if (name.equals("availableTime")) { 2216 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); 2217 } else if (name.equals("notAvailable")) { 2218 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); 2219 } else if (name.equals("availabilityExceptions")) { 2220 this.availabilityExceptions = castToString(value); // StringType 2221 } else if (name.equals("endpoint")) { 2222 this.getEndpoint().add(castToReference(value)); 2223 } else 2224 return super.setProperty(name, value); 2225 return value; 2226 } 2227 2228 @Override 2229 public void removeChild(String name, Base value) throws FHIRException { 2230 if (name.equals("identifier")) { 2231 this.getIdentifier().remove(castToIdentifier(value)); 2232 } else if (name.equals("active")) { 2233 this.active = null; 2234 } else if (name.equals("period")) { 2235 this.period = null; 2236 } else if (name.equals("practitioner")) { 2237 this.practitioner = null; 2238 } else if (name.equals("organization")) { 2239 this.organization = null; 2240 } else if (name.equals("code")) { 2241 this.getCode().remove(castToCodeableConcept(value)); 2242 } else if (name.equals("specialty")) { 2243 this.getSpecialty().remove(castToCodeableConcept(value)); 2244 } else if (name.equals("location")) { 2245 this.getLocation().remove(castToReference(value)); 2246 } else if (name.equals("healthcareService")) { 2247 this.getHealthcareService().remove(castToReference(value)); 2248 } else if (name.equals("telecom")) { 2249 this.getTelecom().remove(castToContactPoint(value)); 2250 } else if (name.equals("availableTime")) { 2251 this.getAvailableTime().remove((PractitionerRoleAvailableTimeComponent) value); 2252 } else if (name.equals("notAvailable")) { 2253 this.getNotAvailable().remove((PractitionerRoleNotAvailableComponent) value); 2254 } else if (name.equals("availabilityExceptions")) { 2255 this.availabilityExceptions = null; 2256 } else if (name.equals("endpoint")) { 2257 this.getEndpoint().remove(castToReference(value)); 2258 } else 2259 super.removeChild(name, value); 2260 2261 } 2262 2263 @Override 2264 public Base makeProperty(int hash, String name) throws FHIRException { 2265 switch (hash) { 2266 case -1618432855: 2267 return addIdentifier(); 2268 case -1422950650: 2269 return getActiveElement(); 2270 case -991726143: 2271 return getPeriod(); 2272 case 574573338: 2273 return getPractitioner(); 2274 case 1178922291: 2275 return getOrganization(); 2276 case 3059181: 2277 return addCode(); 2278 case -1694759682: 2279 return addSpecialty(); 2280 case 1901043637: 2281 return addLocation(); 2282 case 1289661064: 2283 return addHealthcareService(); 2284 case -1429363305: 2285 return addTelecom(); 2286 case 1873069366: 2287 return addAvailableTime(); 2288 case -629572298: 2289 return addNotAvailable(); 2290 case -1149143617: 2291 return getAvailabilityExceptionsElement(); 2292 case 1741102485: 2293 return addEndpoint(); 2294 default: 2295 return super.makeProperty(hash, name); 2296 } 2297 2298 } 2299 2300 @Override 2301 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2302 switch (hash) { 2303 case -1618432855: 2304 /* identifier */ return new String[] { "Identifier" }; 2305 case -1422950650: 2306 /* active */ return new String[] { "boolean" }; 2307 case -991726143: 2308 /* period */ return new String[] { "Period" }; 2309 case 574573338: 2310 /* practitioner */ return new String[] { "Reference" }; 2311 case 1178922291: 2312 /* organization */ return new String[] { "Reference" }; 2313 case 3059181: 2314 /* code */ return new String[] { "CodeableConcept" }; 2315 case -1694759682: 2316 /* specialty */ return new String[] { "CodeableConcept" }; 2317 case 1901043637: 2318 /* location */ return new String[] { "Reference" }; 2319 case 1289661064: 2320 /* healthcareService */ return new String[] { "Reference" }; 2321 case -1429363305: 2322 /* telecom */ return new String[] { "ContactPoint" }; 2323 case 1873069366: 2324 /* availableTime */ return new String[] {}; 2325 case -629572298: 2326 /* notAvailable */ return new String[] {}; 2327 case -1149143617: 2328 /* availabilityExceptions */ return new String[] { "string" }; 2329 case 1741102485: 2330 /* endpoint */ return new String[] { "Reference" }; 2331 default: 2332 return super.getTypesForProperty(hash, name); 2333 } 2334 2335 } 2336 2337 @Override 2338 public Base addChild(String name) throws FHIRException { 2339 if (name.equals("identifier")) { 2340 return addIdentifier(); 2341 } else if (name.equals("active")) { 2342 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.active"); 2343 } else if (name.equals("period")) { 2344 this.period = new Period(); 2345 return this.period; 2346 } else if (name.equals("practitioner")) { 2347 this.practitioner = new Reference(); 2348 return this.practitioner; 2349 } else if (name.equals("organization")) { 2350 this.organization = new Reference(); 2351 return this.organization; 2352 } else if (name.equals("code")) { 2353 return addCode(); 2354 } else if (name.equals("specialty")) { 2355 return addSpecialty(); 2356 } else if (name.equals("location")) { 2357 return addLocation(); 2358 } else if (name.equals("healthcareService")) { 2359 return addHealthcareService(); 2360 } else if (name.equals("telecom")) { 2361 return addTelecom(); 2362 } else if (name.equals("availableTime")) { 2363 return addAvailableTime(); 2364 } else if (name.equals("notAvailable")) { 2365 return addNotAvailable(); 2366 } else if (name.equals("availabilityExceptions")) { 2367 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availabilityExceptions"); 2368 } else if (name.equals("endpoint")) { 2369 return addEndpoint(); 2370 } else 2371 return super.addChild(name); 2372 } 2373 2374 public String fhirType() { 2375 return "PractitionerRole"; 2376 2377 } 2378 2379 public PractitionerRole copy() { 2380 PractitionerRole dst = new PractitionerRole(); 2381 copyValues(dst); 2382 return dst; 2383 } 2384 2385 public void copyValues(PractitionerRole dst) { 2386 super.copyValues(dst); 2387 if (identifier != null) { 2388 dst.identifier = new ArrayList<Identifier>(); 2389 for (Identifier i : identifier) 2390 dst.identifier.add(i.copy()); 2391 } 2392 ; 2393 dst.active = active == null ? null : active.copy(); 2394 dst.period = period == null ? null : period.copy(); 2395 dst.practitioner = practitioner == null ? null : practitioner.copy(); 2396 dst.organization = organization == null ? null : organization.copy(); 2397 if (code != null) { 2398 dst.code = new ArrayList<CodeableConcept>(); 2399 for (CodeableConcept i : code) 2400 dst.code.add(i.copy()); 2401 } 2402 ; 2403 if (specialty != null) { 2404 dst.specialty = new ArrayList<CodeableConcept>(); 2405 for (CodeableConcept i : specialty) 2406 dst.specialty.add(i.copy()); 2407 } 2408 ; 2409 if (location != null) { 2410 dst.location = new ArrayList<Reference>(); 2411 for (Reference i : location) 2412 dst.location.add(i.copy()); 2413 } 2414 ; 2415 if (healthcareService != null) { 2416 dst.healthcareService = new ArrayList<Reference>(); 2417 for (Reference i : healthcareService) 2418 dst.healthcareService.add(i.copy()); 2419 } 2420 ; 2421 if (telecom != null) { 2422 dst.telecom = new ArrayList<ContactPoint>(); 2423 for (ContactPoint i : telecom) 2424 dst.telecom.add(i.copy()); 2425 } 2426 ; 2427 if (availableTime != null) { 2428 dst.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 2429 for (PractitionerRoleAvailableTimeComponent i : availableTime) 2430 dst.availableTime.add(i.copy()); 2431 } 2432 ; 2433 if (notAvailable != null) { 2434 dst.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 2435 for (PractitionerRoleNotAvailableComponent i : notAvailable) 2436 dst.notAvailable.add(i.copy()); 2437 } 2438 ; 2439 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 2440 if (endpoint != null) { 2441 dst.endpoint = new ArrayList<Reference>(); 2442 for (Reference i : endpoint) 2443 dst.endpoint.add(i.copy()); 2444 } 2445 ; 2446 } 2447 2448 protected PractitionerRole typedCopy() { 2449 return copy(); 2450 } 2451 2452 @Override 2453 public boolean equalsDeep(Base other_) { 2454 if (!super.equalsDeep(other_)) 2455 return false; 2456 if (!(other_ instanceof PractitionerRole)) 2457 return false; 2458 PractitionerRole o = (PractitionerRole) other_; 2459 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2460 && compareDeep(period, o.period, true) && compareDeep(practitioner, o.practitioner, true) 2461 && compareDeep(organization, o.organization, true) && compareDeep(code, o.code, true) 2462 && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) 2463 && compareDeep(healthcareService, o.healthcareService, true) && compareDeep(telecom, o.telecom, true) 2464 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 2465 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) 2466 && compareDeep(endpoint, o.endpoint, true); 2467 } 2468 2469 @Override 2470 public boolean equalsShallow(Base other_) { 2471 if (!super.equalsShallow(other_)) 2472 return false; 2473 if (!(other_ instanceof PractitionerRole)) 2474 return false; 2475 PractitionerRole o = (PractitionerRole) other_; 2476 return compareValues(active, o.active, true) 2477 && compareValues(availabilityExceptions, o.availabilityExceptions, true); 2478 } 2479 2480 public boolean isEmpty() { 2481 return super.isEmpty() 2482 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period, practitioner, organization, code, specialty, 2483 location, healthcareService, telecom, availableTime, notAvailable, availabilityExceptions, endpoint); 2484 } 2485 2486 @Override 2487 public ResourceType getResourceType() { 2488 return ResourceType.PractitionerRole; 2489 } 2490 2491 /** 2492 * Search parameter: <b>date</b> 2493 * <p> 2494 * Description: <b>The period during which the practitioner is authorized to 2495 * perform in these role(s)</b><br> 2496 * Type: <b>date</b><br> 2497 * Path: <b>PractitionerRole.period</b><br> 2498 * </p> 2499 */ 2500 @SearchParamDefinition(name = "date", path = "PractitionerRole.period", description = "The period during which the practitioner is authorized to perform in these role(s)", type = "date") 2501 public static final String SP_DATE = "date"; 2502 /** 2503 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2504 * <p> 2505 * Description: <b>The period during which the practitioner is authorized to 2506 * perform in these role(s)</b><br> 2507 * Type: <b>date</b><br> 2508 * Path: <b>PractitionerRole.period</b><br> 2509 * </p> 2510 */ 2511 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2512 SP_DATE); 2513 2514 /** 2515 * Search parameter: <b>identifier</b> 2516 * <p> 2517 * Description: <b>A practitioner's Identifier</b><br> 2518 * Type: <b>token</b><br> 2519 * Path: <b>PractitionerRole.identifier</b><br> 2520 * </p> 2521 */ 2522 @SearchParamDefinition(name = "identifier", path = "PractitionerRole.identifier", description = "A practitioner's Identifier", type = "token") 2523 public static final String SP_IDENTIFIER = "identifier"; 2524 /** 2525 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2526 * <p> 2527 * Description: <b>A practitioner's Identifier</b><br> 2528 * Type: <b>token</b><br> 2529 * Path: <b>PractitionerRole.identifier</b><br> 2530 * </p> 2531 */ 2532 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2533 SP_IDENTIFIER); 2534 2535 /** 2536 * Search parameter: <b>specialty</b> 2537 * <p> 2538 * Description: <b>The practitioner has this specialty at an 2539 * organization</b><br> 2540 * Type: <b>token</b><br> 2541 * Path: <b>PractitionerRole.specialty</b><br> 2542 * </p> 2543 */ 2544 @SearchParamDefinition(name = "specialty", path = "PractitionerRole.specialty", description = "The practitioner has this specialty at an organization", type = "token") 2545 public static final String SP_SPECIALTY = "specialty"; 2546 /** 2547 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 2548 * <p> 2549 * Description: <b>The practitioner has this specialty at an 2550 * organization</b><br> 2551 * Type: <b>token</b><br> 2552 * Path: <b>PractitionerRole.specialty</b><br> 2553 * </p> 2554 */ 2555 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2556 SP_SPECIALTY); 2557 2558 /** 2559 * Search parameter: <b>role</b> 2560 * <p> 2561 * Description: <b>The practitioner can perform this role at for the 2562 * organization</b><br> 2563 * Type: <b>token</b><br> 2564 * Path: <b>PractitionerRole.code</b><br> 2565 * </p> 2566 */ 2567 @SearchParamDefinition(name = "role", path = "PractitionerRole.code", description = "The practitioner can perform this role at for the organization", type = "token") 2568 public static final String SP_ROLE = "role"; 2569 /** 2570 * <b>Fluent Client</b> search parameter constant for <b>role</b> 2571 * <p> 2572 * Description: <b>The practitioner can perform this role at for the 2573 * organization</b><br> 2574 * Type: <b>token</b><br> 2575 * Path: <b>PractitionerRole.code</b><br> 2576 * </p> 2577 */ 2578 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2579 SP_ROLE); 2580 2581 /** 2582 * Search parameter: <b>practitioner</b> 2583 * <p> 2584 * Description: <b>Practitioner that is able to provide the defined services for 2585 * the organization</b><br> 2586 * Type: <b>reference</b><br> 2587 * Path: <b>PractitionerRole.practitioner</b><br> 2588 * </p> 2589 */ 2590 @SearchParamDefinition(name = "practitioner", path = "PractitionerRole.practitioner", description = "Practitioner that is able to provide the defined services for the organization", type = "reference", providesMembershipIn = { 2591 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 2592 public static final String SP_PRACTITIONER = "practitioner"; 2593 /** 2594 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 2595 * <p> 2596 * Description: <b>Practitioner that is able to provide the defined services for 2597 * the organization</b><br> 2598 * Type: <b>reference</b><br> 2599 * Path: <b>PractitionerRole.practitioner</b><br> 2600 * </p> 2601 */ 2602 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2603 SP_PRACTITIONER); 2604 2605 /** 2606 * Constant for fluent queries to be used to add include statements. Specifies 2607 * the path value of "<b>PractitionerRole:practitioner</b>". 2608 */ 2609 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 2610 "PractitionerRole:practitioner").toLocked(); 2611 2612 /** 2613 * Search parameter: <b>active</b> 2614 * <p> 2615 * Description: <b>Whether this practitioner role record is in active 2616 * use</b><br> 2617 * Type: <b>token</b><br> 2618 * Path: <b>PractitionerRole.active</b><br> 2619 * </p> 2620 */ 2621 @SearchParamDefinition(name = "active", path = "PractitionerRole.active", description = "Whether this practitioner role record is in active use", type = "token") 2622 public static final String SP_ACTIVE = "active"; 2623 /** 2624 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2625 * <p> 2626 * Description: <b>Whether this practitioner role record is in active 2627 * use</b><br> 2628 * Type: <b>token</b><br> 2629 * Path: <b>PractitionerRole.active</b><br> 2630 * </p> 2631 */ 2632 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2633 SP_ACTIVE); 2634 2635 /** 2636 * Search parameter: <b>endpoint</b> 2637 * <p> 2638 * Description: <b>Technical endpoints providing access to services operated for 2639 * the practitioner with this role</b><br> 2640 * Type: <b>reference</b><br> 2641 * Path: <b>PractitionerRole.endpoint</b><br> 2642 * </p> 2643 */ 2644 @SearchParamDefinition(name = "endpoint", path = "PractitionerRole.endpoint", description = "Technical endpoints providing access to services operated for the practitioner with this role", type = "reference", target = { 2645 Endpoint.class }) 2646 public static final String SP_ENDPOINT = "endpoint"; 2647 /** 2648 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2649 * <p> 2650 * Description: <b>Technical endpoints providing access to services operated for 2651 * the practitioner with this role</b><br> 2652 * Type: <b>reference</b><br> 2653 * Path: <b>PractitionerRole.endpoint</b><br> 2654 * </p> 2655 */ 2656 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2657 SP_ENDPOINT); 2658 2659 /** 2660 * Constant for fluent queries to be used to add include statements. Specifies 2661 * the path value of "<b>PractitionerRole:endpoint</b>". 2662 */ 2663 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 2664 "PractitionerRole:endpoint").toLocked(); 2665 2666 /** 2667 * Search parameter: <b>phone</b> 2668 * <p> 2669 * Description: <b>A value in a phone contact</b><br> 2670 * Type: <b>token</b><br> 2671 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2672 * </p> 2673 */ 2674 @SearchParamDefinition(name = "phone", path = "PractitionerRole.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 2675 public static final String SP_PHONE = "phone"; 2676 /** 2677 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2678 * <p> 2679 * Description: <b>A value in a phone contact</b><br> 2680 * Type: <b>token</b><br> 2681 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2682 * </p> 2683 */ 2684 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2685 SP_PHONE); 2686 2687 /** 2688 * Search parameter: <b>service</b> 2689 * <p> 2690 * Description: <b>The list of healthcare services that this worker provides for 2691 * this role's Organization/Location(s)</b><br> 2692 * Type: <b>reference</b><br> 2693 * Path: <b>PractitionerRole.healthcareService</b><br> 2694 * </p> 2695 */ 2696 @SearchParamDefinition(name = "service", path = "PractitionerRole.healthcareService", description = "The list of healthcare services that this worker provides for this role's Organization/Location(s)", type = "reference", target = { 2697 HealthcareService.class }) 2698 public static final String SP_SERVICE = "service"; 2699 /** 2700 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2701 * <p> 2702 * Description: <b>The list of healthcare services that this worker provides for 2703 * this role's Organization/Location(s)</b><br> 2704 * Type: <b>reference</b><br> 2705 * Path: <b>PractitionerRole.healthcareService</b><br> 2706 * </p> 2707 */ 2708 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2709 SP_SERVICE); 2710 2711 /** 2712 * Constant for fluent queries to be used to add include statements. Specifies 2713 * the path value of "<b>PractitionerRole:service</b>". 2714 */ 2715 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include( 2716 "PractitionerRole:service").toLocked(); 2717 2718 /** 2719 * Search parameter: <b>organization</b> 2720 * <p> 2721 * Description: <b>The identity of the organization the practitioner represents 2722 * / acts on behalf of</b><br> 2723 * Type: <b>reference</b><br> 2724 * Path: <b>PractitionerRole.organization</b><br> 2725 * </p> 2726 */ 2727 @SearchParamDefinition(name = "organization", path = "PractitionerRole.organization", description = "The identity of the organization the practitioner represents / acts on behalf of", type = "reference", target = { 2728 Organization.class }) 2729 public static final String SP_ORGANIZATION = "organization"; 2730 /** 2731 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2732 * <p> 2733 * Description: <b>The identity of the organization the practitioner represents 2734 * / acts on behalf of</b><br> 2735 * Type: <b>reference</b><br> 2736 * Path: <b>PractitionerRole.organization</b><br> 2737 * </p> 2738 */ 2739 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2740 SP_ORGANIZATION); 2741 2742 /** 2743 * Constant for fluent queries to be used to add include statements. Specifies 2744 * the path value of "<b>PractitionerRole:organization</b>". 2745 */ 2746 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 2747 "PractitionerRole:organization").toLocked(); 2748 2749 /** 2750 * Search parameter: <b>telecom</b> 2751 * <p> 2752 * Description: <b>The value in any kind of contact</b><br> 2753 * Type: <b>token</b><br> 2754 * Path: <b>PractitionerRole.telecom</b><br> 2755 * </p> 2756 */ 2757 @SearchParamDefinition(name = "telecom", path = "PractitionerRole.telecom", description = "The value in any kind of contact", type = "token") 2758 public static final String SP_TELECOM = "telecom"; 2759 /** 2760 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2761 * <p> 2762 * Description: <b>The value in any kind of contact</b><br> 2763 * Type: <b>token</b><br> 2764 * Path: <b>PractitionerRole.telecom</b><br> 2765 * </p> 2766 */ 2767 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2768 SP_TELECOM); 2769 2770 /** 2771 * Search parameter: <b>location</b> 2772 * <p> 2773 * Description: <b>One of the locations at which this practitioner provides 2774 * care</b><br> 2775 * Type: <b>reference</b><br> 2776 * Path: <b>PractitionerRole.location</b><br> 2777 * </p> 2778 */ 2779 @SearchParamDefinition(name = "location", path = "PractitionerRole.location", description = "One of the locations at which this practitioner provides care", type = "reference", target = { 2780 Location.class }) 2781 public static final String SP_LOCATION = "location"; 2782 /** 2783 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2784 * <p> 2785 * Description: <b>One of the locations at which this practitioner provides 2786 * care</b><br> 2787 * Type: <b>reference</b><br> 2788 * Path: <b>PractitionerRole.location</b><br> 2789 * </p> 2790 */ 2791 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2792 SP_LOCATION); 2793 2794 /** 2795 * Constant for fluent queries to be used to add include statements. Specifies 2796 * the path value of "<b>PractitionerRole:location</b>". 2797 */ 2798 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 2799 "PractitionerRole:location").toLocked(); 2800 2801 /** 2802 * Search parameter: <b>email</b> 2803 * <p> 2804 * Description: <b>A value in an email contact</b><br> 2805 * Type: <b>token</b><br> 2806 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2807 * </p> 2808 */ 2809 @SearchParamDefinition(name = "email", path = "PractitionerRole.telecom.where(system='email')", description = "A value in an email contact", type = "token") 2810 public static final String SP_EMAIL = "email"; 2811 /** 2812 * <b>Fluent Client</b> search parameter constant for <b>email</b> 2813 * <p> 2814 * Description: <b>A value in an email contact</b><br> 2815 * Type: <b>token</b><br> 2816 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2817 * </p> 2818 */ 2819 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2820 SP_EMAIL); 2821 2822}