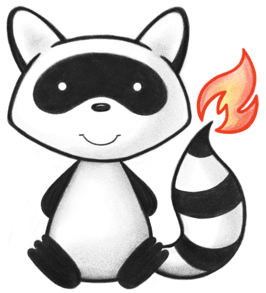
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A specific set of Roles/Locations/specialties/services that a practitioner 048 * may perform at an organization for a period of time. 049 */ 050@ResourceDef(name = "PractitionerRole", profile = "http://hl7.org/fhir/StructureDefinition/PractitionerRole") 051public class PractitionerRole extends DomainResource { 052 053 public enum DaysOfWeek { 054 /** 055 * Monday. 056 */ 057 MON, 058 /** 059 * Tuesday. 060 */ 061 TUE, 062 /** 063 * Wednesday. 064 */ 065 WED, 066 /** 067 * Thursday. 068 */ 069 THU, 070 /** 071 * Friday. 072 */ 073 FRI, 074 /** 075 * Saturday. 076 */ 077 SAT, 078 /** 079 * Sunday. 080 */ 081 SUN, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 087 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("mon".equals(codeString)) 091 return MON; 092 if ("tue".equals(codeString)) 093 return TUE; 094 if ("wed".equals(codeString)) 095 return WED; 096 if ("thu".equals(codeString)) 097 return THU; 098 if ("fri".equals(codeString)) 099 return FRI; 100 if ("sat".equals(codeString)) 101 return SAT; 102 if ("sun".equals(codeString)) 103 return SUN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 108 } 109 110 public String toCode() { 111 switch (this) { 112 case MON: 113 return "mon"; 114 case TUE: 115 return "tue"; 116 case WED: 117 return "wed"; 118 case THU: 119 return "thu"; 120 case FRI: 121 return "fri"; 122 case SAT: 123 return "sat"; 124 case SUN: 125 return "sun"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getSystem() { 134 switch (this) { 135 case MON: 136 return "http://hl7.org/fhir/days-of-week"; 137 case TUE: 138 return "http://hl7.org/fhir/days-of-week"; 139 case WED: 140 return "http://hl7.org/fhir/days-of-week"; 141 case THU: 142 return "http://hl7.org/fhir/days-of-week"; 143 case FRI: 144 return "http://hl7.org/fhir/days-of-week"; 145 case SAT: 146 return "http://hl7.org/fhir/days-of-week"; 147 case SUN: 148 return "http://hl7.org/fhir/days-of-week"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDefinition() { 157 switch (this) { 158 case MON: 159 return "Monday."; 160 case TUE: 161 return "Tuesday."; 162 case WED: 163 return "Wednesday."; 164 case THU: 165 return "Thursday."; 166 case FRI: 167 return "Friday."; 168 case SAT: 169 return "Saturday."; 170 case SUN: 171 return "Sunday."; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDisplay() { 180 switch (this) { 181 case MON: 182 return "Monday"; 183 case TUE: 184 return "Tuesday"; 185 case WED: 186 return "Wednesday"; 187 case THU: 188 return "Thursday"; 189 case FRI: 190 return "Friday"; 191 case SAT: 192 return "Saturday"; 193 case SUN: 194 return "Sunday"; 195 case NULL: 196 return null; 197 default: 198 return "?"; 199 } 200 } 201 } 202 203 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 204 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 205 if (codeString == null || "".equals(codeString)) 206 if (codeString == null || "".equals(codeString)) 207 return null; 208 if ("mon".equals(codeString)) 209 return DaysOfWeek.MON; 210 if ("tue".equals(codeString)) 211 return DaysOfWeek.TUE; 212 if ("wed".equals(codeString)) 213 return DaysOfWeek.WED; 214 if ("thu".equals(codeString)) 215 return DaysOfWeek.THU; 216 if ("fri".equals(codeString)) 217 return DaysOfWeek.FRI; 218 if ("sat".equals(codeString)) 219 return DaysOfWeek.SAT; 220 if ("sun".equals(codeString)) 221 return DaysOfWeek.SUN; 222 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 223 } 224 225 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 226 if (code == null) 227 return null; 228 if (code.isEmpty()) 229 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 230 String codeString = code.asStringValue(); 231 if (codeString == null || "".equals(codeString)) 232 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 233 if ("mon".equals(codeString)) 234 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON, code); 235 if ("tue".equals(codeString)) 236 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE, code); 237 if ("wed".equals(codeString)) 238 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED, code); 239 if ("thu".equals(codeString)) 240 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU, code); 241 if ("fri".equals(codeString)) 242 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI, code); 243 if ("sat".equals(codeString)) 244 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT, code); 245 if ("sun".equals(codeString)) 246 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN, code); 247 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 248 } 249 250 public String toCode(DaysOfWeek code) { 251 if (code == DaysOfWeek.MON) 252 return "mon"; 253 if (code == DaysOfWeek.TUE) 254 return "tue"; 255 if (code == DaysOfWeek.WED) 256 return "wed"; 257 if (code == DaysOfWeek.THU) 258 return "thu"; 259 if (code == DaysOfWeek.FRI) 260 return "fri"; 261 if (code == DaysOfWeek.SAT) 262 return "sat"; 263 if (code == DaysOfWeek.SUN) 264 return "sun"; 265 return "?"; 266 } 267 268 public String toSystem(DaysOfWeek code) { 269 return code.getSystem(); 270 } 271 } 272 273 @Block() 274 public static class PractitionerRoleAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 275 /** 276 * Indicates which days of the week are available between the start and end 277 * Times. 278 */ 279 @Child(name = "daysOfWeek", type = { 280 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 281 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 283 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 284 285 /** 286 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 287 */ 288 @Child(name = "allDay", type = { 289 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 290 @Description(shortDefinition = "Always available? e.g. 24 hour service", formalDefinition = "Is this always available? (hence times are irrelevant) e.g. 24 hour service.") 291 protected BooleanType allDay; 292 293 /** 294 * The opening time of day. Note: If the AllDay flag is set, then this time is 295 * ignored. 296 */ 297 @Child(name = "availableStartTime", type = { 298 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 299 @Description(shortDefinition = "Opening time of day (ignored if allDay = true)", formalDefinition = "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.") 300 protected TimeType availableStartTime; 301 302 /** 303 * The closing time of day. Note: If the AllDay flag is set, then this time is 304 * ignored. 305 */ 306 @Child(name = "availableEndTime", type = { 307 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 308 @Description(shortDefinition = "Closing time of day (ignored if allDay = true)", formalDefinition = "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.") 309 protected TimeType availableEndTime; 310 311 private static final long serialVersionUID = -2139510127L; 312 313 /** 314 * Constructor 315 */ 316 public PractitionerRoleAvailableTimeComponent() { 317 super(); 318 } 319 320 /** 321 * @return {@link #daysOfWeek} (Indicates which days of the week are available 322 * between the start and end Times.) 323 */ 324 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 325 if (this.daysOfWeek == null) 326 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 327 return this.daysOfWeek; 328 } 329 330 /** 331 * @return Returns a reference to <code>this</code> for easy method chaining 332 */ 333 public PractitionerRoleAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 334 this.daysOfWeek = theDaysOfWeek; 335 return this; 336 } 337 338 public boolean hasDaysOfWeek() { 339 if (this.daysOfWeek == null) 340 return false; 341 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 342 if (!item.isEmpty()) 343 return true; 344 return false; 345 } 346 347 /** 348 * @return {@link #daysOfWeek} (Indicates which days of the week are available 349 * between the start and end Times.) 350 */ 351 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 352 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 353 if (this.daysOfWeek == null) 354 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 355 this.daysOfWeek.add(t); 356 return t; 357 } 358 359 /** 360 * @param value {@link #daysOfWeek} (Indicates which days of the week are 361 * available between the start and end Times.) 362 */ 363 public PractitionerRoleAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { // 1 364 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 365 t.setValue(value); 366 if (this.daysOfWeek == null) 367 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 368 this.daysOfWeek.add(t); 369 return this; 370 } 371 372 /** 373 * @param value {@link #daysOfWeek} (Indicates which days of the week are 374 * available between the start and end Times.) 375 */ 376 public boolean hasDaysOfWeek(DaysOfWeek value) { 377 if (this.daysOfWeek == null) 378 return false; 379 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 380 if (v.getValue().equals(value)) // code 381 return true; 382 return false; 383 } 384 385 /** 386 * @return {@link #allDay} (Is this always available? (hence times are 387 * irrelevant) e.g. 24 hour service.). This is the underlying object 388 * with id, value and extensions. The accessor "getAllDay" gives direct 389 * access to the value 390 */ 391 public BooleanType getAllDayElement() { 392 if (this.allDay == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.allDay"); 395 else if (Configuration.doAutoCreate()) 396 this.allDay = new BooleanType(); // bb 397 return this.allDay; 398 } 399 400 public boolean hasAllDayElement() { 401 return this.allDay != null && !this.allDay.isEmpty(); 402 } 403 404 public boolean hasAllDay() { 405 return this.allDay != null && !this.allDay.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #allDay} (Is this always available? (hence times are 410 * irrelevant) e.g. 24 hour service.). This is the underlying 411 * object with id, value and extensions. The accessor "getAllDay" 412 * gives direct access to the value 413 */ 414 public PractitionerRoleAvailableTimeComponent setAllDayElement(BooleanType value) { 415 this.allDay = value; 416 return this; 417 } 418 419 /** 420 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour 421 * service. 422 */ 423 public boolean getAllDay() { 424 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 425 } 426 427 /** 428 * @param value Is this always available? (hence times are irrelevant) e.g. 24 429 * hour service. 430 */ 431 public PractitionerRoleAvailableTimeComponent setAllDay(boolean value) { 432 if (this.allDay == null) 433 this.allDay = new BooleanType(); 434 this.allDay.setValue(value); 435 return this; 436 } 437 438 /** 439 * @return {@link #availableStartTime} (The opening time of day. Note: If the 440 * AllDay flag is set, then this time is ignored.). This is the 441 * underlying object with id, value and extensions. The accessor 442 * "getAvailableStartTime" gives direct access to the value 443 */ 444 public TimeType getAvailableStartTimeElement() { 445 if (this.availableStartTime == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableStartTime"); 448 else if (Configuration.doAutoCreate()) 449 this.availableStartTime = new TimeType(); // bb 450 return this.availableStartTime; 451 } 452 453 public boolean hasAvailableStartTimeElement() { 454 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 455 } 456 457 public boolean hasAvailableStartTime() { 458 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 459 } 460 461 /** 462 * @param value {@link #availableStartTime} (The opening time of day. Note: If 463 * the AllDay flag is set, then this time is ignored.). This is the 464 * underlying object with id, value and extensions. The accessor 465 * "getAvailableStartTime" gives direct access to the value 466 */ 467 public PractitionerRoleAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 468 this.availableStartTime = value; 469 return this; 470 } 471 472 /** 473 * @return The opening time of day. Note: If the AllDay flag is set, then this 474 * time is ignored. 475 */ 476 public String getAvailableStartTime() { 477 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 478 } 479 480 /** 481 * @param value The opening time of day. Note: If the AllDay flag is set, then 482 * this time is ignored. 483 */ 484 public PractitionerRoleAvailableTimeComponent setAvailableStartTime(String value) { 485 if (value == null) 486 this.availableStartTime = null; 487 else { 488 if (this.availableStartTime == null) 489 this.availableStartTime = new TimeType(); 490 this.availableStartTime.setValue(value); 491 } 492 return this; 493 } 494 495 /** 496 * @return {@link #availableEndTime} (The closing time of day. Note: If the 497 * AllDay flag is set, then this time is ignored.). This is the 498 * underlying object with id, value and extensions. The accessor 499 * "getAvailableEndTime" gives direct access to the value 500 */ 501 public TimeType getAvailableEndTimeElement() { 502 if (this.availableEndTime == null) 503 if (Configuration.errorOnAutoCreate()) 504 throw new Error("Attempt to auto-create PractitionerRoleAvailableTimeComponent.availableEndTime"); 505 else if (Configuration.doAutoCreate()) 506 this.availableEndTime = new TimeType(); // bb 507 return this.availableEndTime; 508 } 509 510 public boolean hasAvailableEndTimeElement() { 511 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 512 } 513 514 public boolean hasAvailableEndTime() { 515 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 516 } 517 518 /** 519 * @param value {@link #availableEndTime} (The closing time of day. Note: If the 520 * AllDay flag is set, then this time is ignored.). This is the 521 * underlying object with id, value and extensions. The accessor 522 * "getAvailableEndTime" gives direct access to the value 523 */ 524 public PractitionerRoleAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 525 this.availableEndTime = value; 526 return this; 527 } 528 529 /** 530 * @return The closing time of day. Note: If the AllDay flag is set, then this 531 * time is ignored. 532 */ 533 public String getAvailableEndTime() { 534 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 535 } 536 537 /** 538 * @param value The closing time of day. Note: If the AllDay flag is set, then 539 * this time is ignored. 540 */ 541 public PractitionerRoleAvailableTimeComponent setAvailableEndTime(String value) { 542 if (value == null) 543 this.availableEndTime = null; 544 else { 545 if (this.availableEndTime == null) 546 this.availableEndTime = new TimeType(); 547 this.availableEndTime.setValue(value); 548 } 549 return this; 550 } 551 552 protected void listChildren(List<Property> children) { 553 super.listChildren(children); 554 children.add(new Property("daysOfWeek", "code", 555 "Indicates which days of the week are available between the start and end Times.", 0, 556 java.lang.Integer.MAX_VALUE, daysOfWeek)); 557 children.add(new Property("allDay", "boolean", 558 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 559 children.add(new Property("availableStartTime", "time", 560 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 561 availableStartTime)); 562 children.add(new Property("availableEndTime", "time", 563 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 564 availableEndTime)); 565 } 566 567 @Override 568 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 569 switch (_hash) { 570 case 68050338: 571 /* daysOfWeek */ return new Property("daysOfWeek", "code", 572 "Indicates which days of the week are available between the start and end Times.", 0, 573 java.lang.Integer.MAX_VALUE, daysOfWeek); 574 case -1414913477: 575 /* allDay */ return new Property("allDay", "boolean", 576 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 577 case -1039453818: 578 /* availableStartTime */ return new Property("availableStartTime", "time", 579 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 580 availableStartTime); 581 case 101151551: 582 /* availableEndTime */ return new Property("availableEndTime", "time", 583 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 584 availableEndTime); 585 default: 586 return super.getNamedProperty(_hash, _name, _checkValid); 587 } 588 589 } 590 591 @Override 592 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 593 switch (hash) { 594 case 68050338: 595 /* daysOfWeek */ return this.daysOfWeek == null ? new Base[0] 596 : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 597 case -1414913477: 598 /* allDay */ return this.allDay == null ? new Base[0] : new Base[] { this.allDay }; // BooleanType 599 case -1039453818: 600 /* availableStartTime */ return this.availableStartTime == null ? new Base[0] 601 : new Base[] { this.availableStartTime }; // TimeType 602 case 101151551: 603 /* availableEndTime */ return this.availableEndTime == null ? new Base[0] 604 : new Base[] { this.availableEndTime }; // TimeType 605 default: 606 return super.getProperty(hash, name, checkValid); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 613 switch (hash) { 614 case 68050338: // daysOfWeek 615 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 616 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 617 return value; 618 case -1414913477: // allDay 619 this.allDay = castToBoolean(value); // BooleanType 620 return value; 621 case -1039453818: // availableStartTime 622 this.availableStartTime = castToTime(value); // TimeType 623 return value; 624 case 101151551: // availableEndTime 625 this.availableEndTime = castToTime(value); // TimeType 626 return value; 627 default: 628 return super.setProperty(hash, name, value); 629 } 630 631 } 632 633 @Override 634 public Base setProperty(String name, Base value) throws FHIRException { 635 if (name.equals("daysOfWeek")) { 636 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 637 this.getDaysOfWeek().add((Enumeration) value); 638 } else if (name.equals("allDay")) { 639 this.allDay = castToBoolean(value); // BooleanType 640 } else if (name.equals("availableStartTime")) { 641 this.availableStartTime = castToTime(value); // TimeType 642 } else if (name.equals("availableEndTime")) { 643 this.availableEndTime = castToTime(value); // TimeType 644 } else 645 return super.setProperty(name, value); 646 return value; 647 } 648 649 @Override 650 public Base makeProperty(int hash, String name) throws FHIRException { 651 switch (hash) { 652 case 68050338: 653 return addDaysOfWeekElement(); 654 case -1414913477: 655 return getAllDayElement(); 656 case -1039453818: 657 return getAvailableStartTimeElement(); 658 case 101151551: 659 return getAvailableEndTimeElement(); 660 default: 661 return super.makeProperty(hash, name); 662 } 663 664 } 665 666 @Override 667 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 668 switch (hash) { 669 case 68050338: 670 /* daysOfWeek */ return new String[] { "code" }; 671 case -1414913477: 672 /* allDay */ return new String[] { "boolean" }; 673 case -1039453818: 674 /* availableStartTime */ return new String[] { "time" }; 675 case 101151551: 676 /* availableEndTime */ return new String[] { "time" }; 677 default: 678 return super.getTypesForProperty(hash, name); 679 } 680 681 } 682 683 @Override 684 public Base addChild(String name) throws FHIRException { 685 if (name.equals("daysOfWeek")) { 686 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.daysOfWeek"); 687 } else if (name.equals("allDay")) { 688 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.allDay"); 689 } else if (name.equals("availableStartTime")) { 690 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableStartTime"); 691 } else if (name.equals("availableEndTime")) { 692 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availableEndTime"); 693 } else 694 return super.addChild(name); 695 } 696 697 public PractitionerRoleAvailableTimeComponent copy() { 698 PractitionerRoleAvailableTimeComponent dst = new PractitionerRoleAvailableTimeComponent(); 699 copyValues(dst); 700 return dst; 701 } 702 703 public void copyValues(PractitionerRoleAvailableTimeComponent dst) { 704 super.copyValues(dst); 705 if (daysOfWeek != null) { 706 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 707 for (Enumeration<DaysOfWeek> i : daysOfWeek) 708 dst.daysOfWeek.add(i.copy()); 709 } 710 ; 711 dst.allDay = allDay == null ? null : allDay.copy(); 712 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 713 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 714 } 715 716 @Override 717 public boolean equalsDeep(Base other_) { 718 if (!super.equalsDeep(other_)) 719 return false; 720 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 721 return false; 722 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 723 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 724 && compareDeep(availableStartTime, o.availableStartTime, true) 725 && compareDeep(availableEndTime, o.availableEndTime, true); 726 } 727 728 @Override 729 public boolean equalsShallow(Base other_) { 730 if (!super.equalsShallow(other_)) 731 return false; 732 if (!(other_ instanceof PractitionerRoleAvailableTimeComponent)) 733 return false; 734 PractitionerRoleAvailableTimeComponent o = (PractitionerRoleAvailableTimeComponent) other_; 735 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 736 && compareValues(availableStartTime, o.availableStartTime, true) 737 && compareValues(availableEndTime, o.availableEndTime, true); 738 } 739 740 public boolean isEmpty() { 741 return super.isEmpty() 742 && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime, availableEndTime); 743 } 744 745 public String fhirType() { 746 return "PractitionerRole.availableTime"; 747 748 } 749 750 } 751 752 @Block() 753 public static class PractitionerRoleNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 754 /** 755 * The reason that can be presented to the user as to why this time is not 756 * available. 757 */ 758 @Child(name = "description", type = { 759 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 760 @Description(shortDefinition = "Reason presented to the user explaining why time not available", formalDefinition = "The reason that can be presented to the user as to why this time is not available.") 761 protected StringType description; 762 763 /** 764 * Service is not available (seasonally or for a public holiday) from this date. 765 */ 766 @Child(name = "during", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 767 @Description(shortDefinition = "Service not available from this date", formalDefinition = "Service is not available (seasonally or for a public holiday) from this date.") 768 protected Period during; 769 770 private static final long serialVersionUID = 310849929L; 771 772 /** 773 * Constructor 774 */ 775 public PractitionerRoleNotAvailableComponent() { 776 super(); 777 } 778 779 /** 780 * Constructor 781 */ 782 public PractitionerRoleNotAvailableComponent(StringType description) { 783 super(); 784 this.description = description; 785 } 786 787 /** 788 * @return {@link #description} (The reason that can be presented to the user as 789 * to why this time is not available.). This is the underlying object 790 * with id, value and extensions. The accessor "getDescription" gives 791 * direct access to the value 792 */ 793 public StringType getDescriptionElement() { 794 if (this.description == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.description"); 797 else if (Configuration.doAutoCreate()) 798 this.description = new StringType(); // bb 799 return this.description; 800 } 801 802 public boolean hasDescriptionElement() { 803 return this.description != null && !this.description.isEmpty(); 804 } 805 806 public boolean hasDescription() { 807 return this.description != null && !this.description.isEmpty(); 808 } 809 810 /** 811 * @param value {@link #description} (The reason that can be presented to the 812 * user as to why this time is not available.). This is the 813 * underlying object with id, value and extensions. The accessor 814 * "getDescription" gives direct access to the value 815 */ 816 public PractitionerRoleNotAvailableComponent setDescriptionElement(StringType value) { 817 this.description = value; 818 return this; 819 } 820 821 /** 822 * @return The reason that can be presented to the user as to why this time is 823 * not available. 824 */ 825 public String getDescription() { 826 return this.description == null ? null : this.description.getValue(); 827 } 828 829 /** 830 * @param value The reason that can be presented to the user as to why this time 831 * is not available. 832 */ 833 public PractitionerRoleNotAvailableComponent setDescription(String value) { 834 if (this.description == null) 835 this.description = new StringType(); 836 this.description.setValue(value); 837 return this; 838 } 839 840 /** 841 * @return {@link #during} (Service is not available (seasonally or for a public 842 * holiday) from this date.) 843 */ 844 public Period getDuring() { 845 if (this.during == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create PractitionerRoleNotAvailableComponent.during"); 848 else if (Configuration.doAutoCreate()) 849 this.during = new Period(); // cc 850 return this.during; 851 } 852 853 public boolean hasDuring() { 854 return this.during != null && !this.during.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #during} (Service is not available (seasonally or for a 859 * public holiday) from this date.) 860 */ 861 public PractitionerRoleNotAvailableComponent setDuring(Period value) { 862 this.during = value; 863 return this; 864 } 865 866 protected void listChildren(List<Property> children) { 867 super.listChildren(children); 868 children.add(new Property("description", "string", 869 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 870 children.add(new Property("during", "Period", 871 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 872 } 873 874 @Override 875 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 876 switch (_hash) { 877 case -1724546052: 878 /* description */ return new Property("description", "string", 879 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 880 case -1320499647: 881 /* during */ return new Property("during", "Period", 882 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 883 default: 884 return super.getNamedProperty(_hash, _name, _checkValid); 885 } 886 887 } 888 889 @Override 890 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 891 switch (hash) { 892 case -1724546052: 893 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 894 case -1320499647: 895 /* during */ return this.during == null ? new Base[0] : new Base[] { this.during }; // Period 896 default: 897 return super.getProperty(hash, name, checkValid); 898 } 899 900 } 901 902 @Override 903 public Base setProperty(int hash, String name, Base value) throws FHIRException { 904 switch (hash) { 905 case -1724546052: // description 906 this.description = castToString(value); // StringType 907 return value; 908 case -1320499647: // during 909 this.during = castToPeriod(value); // Period 910 return value; 911 default: 912 return super.setProperty(hash, name, value); 913 } 914 915 } 916 917 @Override 918 public Base setProperty(String name, Base value) throws FHIRException { 919 if (name.equals("description")) { 920 this.description = castToString(value); // StringType 921 } else if (name.equals("during")) { 922 this.during = castToPeriod(value); // Period 923 } else 924 return super.setProperty(name, value); 925 return value; 926 } 927 928 @Override 929 public Base makeProperty(int hash, String name) throws FHIRException { 930 switch (hash) { 931 case -1724546052: 932 return getDescriptionElement(); 933 case -1320499647: 934 return getDuring(); 935 default: 936 return super.makeProperty(hash, name); 937 } 938 939 } 940 941 @Override 942 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 943 switch (hash) { 944 case -1724546052: 945 /* description */ return new String[] { "string" }; 946 case -1320499647: 947 /* during */ return new String[] { "Period" }; 948 default: 949 return super.getTypesForProperty(hash, name); 950 } 951 952 } 953 954 @Override 955 public Base addChild(String name) throws FHIRException { 956 if (name.equals("description")) { 957 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.description"); 958 } else if (name.equals("during")) { 959 this.during = new Period(); 960 return this.during; 961 } else 962 return super.addChild(name); 963 } 964 965 public PractitionerRoleNotAvailableComponent copy() { 966 PractitionerRoleNotAvailableComponent dst = new PractitionerRoleNotAvailableComponent(); 967 copyValues(dst); 968 return dst; 969 } 970 971 public void copyValues(PractitionerRoleNotAvailableComponent dst) { 972 super.copyValues(dst); 973 dst.description = description == null ? null : description.copy(); 974 dst.during = during == null ? null : during.copy(); 975 } 976 977 @Override 978 public boolean equalsDeep(Base other_) { 979 if (!super.equalsDeep(other_)) 980 return false; 981 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 982 return false; 983 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 984 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 985 } 986 987 @Override 988 public boolean equalsShallow(Base other_) { 989 if (!super.equalsShallow(other_)) 990 return false; 991 if (!(other_ instanceof PractitionerRoleNotAvailableComponent)) 992 return false; 993 PractitionerRoleNotAvailableComponent o = (PractitionerRoleNotAvailableComponent) other_; 994 return compareValues(description, o.description, true); 995 } 996 997 public boolean isEmpty() { 998 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 999 } 1000 1001 public String fhirType() { 1002 return "PractitionerRole.notAvailable"; 1003 1004 } 1005 1006 } 1007 1008 /** 1009 * Business Identifiers that are specific to a role/location. 1010 */ 1011 @Child(name = "identifier", type = { 1012 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1013 @Description(shortDefinition = "Business Identifiers that are specific to a role/location", formalDefinition = "Business Identifiers that are specific to a role/location.") 1014 protected List<Identifier> identifier; 1015 1016 /** 1017 * Whether this practitioner role record is in active use. 1018 */ 1019 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1020 @Description(shortDefinition = "Whether this practitioner role record is in active use", formalDefinition = "Whether this practitioner role record is in active use.") 1021 protected BooleanType active; 1022 1023 /** 1024 * The period during which the person is authorized to act as a practitioner in 1025 * these role(s) for the organization. 1026 */ 1027 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1028 @Description(shortDefinition = "The period during which the practitioner is authorized to perform in these role(s)", formalDefinition = "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.") 1029 protected Period period; 1030 1031 /** 1032 * Practitioner that is able to provide the defined services for the 1033 * organization. 1034 */ 1035 @Child(name = "practitioner", type = { 1036 Practitioner.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1037 @Description(shortDefinition = "Practitioner that is able to provide the defined services for the organization", formalDefinition = "Practitioner that is able to provide the defined services for the organization.") 1038 protected Reference practitioner; 1039 1040 /** 1041 * The actual object that is the target of the reference (Practitioner that is 1042 * able to provide the defined services for the organization.) 1043 */ 1044 protected Practitioner practitionerTarget; 1045 1046 /** 1047 * The organization where the Practitioner performs the roles associated. 1048 */ 1049 @Child(name = "organization", type = { 1050 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1051 @Description(shortDefinition = "Organization where the roles are available", formalDefinition = "The organization where the Practitioner performs the roles associated.") 1052 protected Reference organization; 1053 1054 /** 1055 * The actual object that is the target of the reference (The organization where 1056 * the Practitioner performs the roles associated.) 1057 */ 1058 protected Organization organizationTarget; 1059 1060 /** 1061 * Roles which this practitioner is authorized to perform for the organization. 1062 */ 1063 @Child(name = "code", type = { 1064 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1065 @Description(shortDefinition = "Roles which this practitioner may perform", formalDefinition = "Roles which this practitioner is authorized to perform for the organization.") 1066 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/practitioner-role") 1067 protected List<CodeableConcept> code; 1068 1069 /** 1070 * Specific specialty of the practitioner. 1071 */ 1072 @Child(name = "specialty", type = { 1073 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1074 @Description(shortDefinition = "Specific specialty of the practitioner", formalDefinition = "Specific specialty of the practitioner.") 1075 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 1076 protected List<CodeableConcept> specialty; 1077 1078 /** 1079 * The location(s) at which this practitioner provides care. 1080 */ 1081 @Child(name = "location", type = { 1082 Location.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1083 @Description(shortDefinition = "The location(s) at which this practitioner provides care", formalDefinition = "The location(s) at which this practitioner provides care.") 1084 protected List<Reference> location; 1085 /** 1086 * The actual objects that are the target of the reference (The location(s) at 1087 * which this practitioner provides care.) 1088 */ 1089 protected List<Location> locationTarget; 1090 1091 /** 1092 * The list of healthcare services that this worker provides for this role's 1093 * Organization/Location(s). 1094 */ 1095 @Child(name = "healthcareService", type = { 1096 HealthcareService.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1097 @Description(shortDefinition = "The list of healthcare services that this worker provides for this role's Organization/Location(s)", formalDefinition = "The list of healthcare services that this worker provides for this role's Organization/Location(s).") 1098 protected List<Reference> healthcareService; 1099 /** 1100 * The actual objects that are the target of the reference (The list of 1101 * healthcare services that this worker provides for this role's 1102 * Organization/Location(s).) 1103 */ 1104 protected List<HealthcareService> healthcareServiceTarget; 1105 1106 /** 1107 * Contact details that are specific to the role/location/service. 1108 */ 1109 @Child(name = "telecom", type = { 1110 ContactPoint.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1111 @Description(shortDefinition = "Contact details that are specific to the role/location/service", formalDefinition = "Contact details that are specific to the role/location/service.") 1112 protected List<ContactPoint> telecom; 1113 1114 /** 1115 * A collection of times the practitioner is available or performing this role 1116 * at the location and/or healthcareservice. 1117 */ 1118 @Child(name = "availableTime", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1119 @Description(shortDefinition = "Times the Service Site is available", formalDefinition = "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.") 1120 protected List<PractitionerRoleAvailableTimeComponent> availableTime; 1121 1122 /** 1123 * The practitioner is not available or performing this role during this period 1124 * of time due to the provided reason. 1125 */ 1126 @Child(name = "notAvailable", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1127 @Description(shortDefinition = "Not available during this time due to provided reason", formalDefinition = "The practitioner is not available or performing this role during this period of time due to the provided reason.") 1128 protected List<PractitionerRoleNotAvailableComponent> notAvailable; 1129 1130 /** 1131 * A description of site availability exceptions, e.g. public holiday 1132 * availability. Succinctly describing all possible exceptions to normal site 1133 * availability as details in the available Times and not available Times. 1134 */ 1135 @Child(name = "availabilityExceptions", type = { 1136 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1137 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.") 1138 protected StringType availabilityExceptions; 1139 1140 /** 1141 * Technical endpoints providing access to services operated for the 1142 * practitioner with this role. 1143 */ 1144 @Child(name = "endpoint", type = { 1145 Endpoint.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1146 @Description(shortDefinition = "Technical endpoints providing access to services operated for the practitioner with this role", formalDefinition = "Technical endpoints providing access to services operated for the practitioner with this role.") 1147 protected List<Reference> endpoint; 1148 /** 1149 * The actual objects that are the target of the reference (Technical endpoints 1150 * providing access to services operated for the practitioner with this role.) 1151 */ 1152 protected List<Endpoint> endpointTarget; 1153 1154 private static final long serialVersionUID = 423338051L; 1155 1156 /** 1157 * Constructor 1158 */ 1159 public PractitionerRole() { 1160 super(); 1161 } 1162 1163 /** 1164 * @return {@link #identifier} (Business Identifiers that are specific to a 1165 * role/location.) 1166 */ 1167 public List<Identifier> getIdentifier() { 1168 if (this.identifier == null) 1169 this.identifier = new ArrayList<Identifier>(); 1170 return this.identifier; 1171 } 1172 1173 /** 1174 * @return Returns a reference to <code>this</code> for easy method chaining 1175 */ 1176 public PractitionerRole setIdentifier(List<Identifier> theIdentifier) { 1177 this.identifier = theIdentifier; 1178 return this; 1179 } 1180 1181 public boolean hasIdentifier() { 1182 if (this.identifier == null) 1183 return false; 1184 for (Identifier item : this.identifier) 1185 if (!item.isEmpty()) 1186 return true; 1187 return false; 1188 } 1189 1190 public Identifier addIdentifier() { // 3 1191 Identifier t = new Identifier(); 1192 if (this.identifier == null) 1193 this.identifier = new ArrayList<Identifier>(); 1194 this.identifier.add(t); 1195 return t; 1196 } 1197 1198 public PractitionerRole addIdentifier(Identifier t) { // 3 1199 if (t == null) 1200 return this; 1201 if (this.identifier == null) 1202 this.identifier = new ArrayList<Identifier>(); 1203 this.identifier.add(t); 1204 return this; 1205 } 1206 1207 /** 1208 * @return The first repetition of repeating field {@link #identifier}, creating 1209 * it if it does not already exist 1210 */ 1211 public Identifier getIdentifierFirstRep() { 1212 if (getIdentifier().isEmpty()) { 1213 addIdentifier(); 1214 } 1215 return getIdentifier().get(0); 1216 } 1217 1218 /** 1219 * @return {@link #active} (Whether this practitioner role record is in active 1220 * use.). This is the underlying object with id, value and extensions. 1221 * The accessor "getActive" gives direct access to the value 1222 */ 1223 public BooleanType getActiveElement() { 1224 if (this.active == null) 1225 if (Configuration.errorOnAutoCreate()) 1226 throw new Error("Attempt to auto-create PractitionerRole.active"); 1227 else if (Configuration.doAutoCreate()) 1228 this.active = new BooleanType(); // bb 1229 return this.active; 1230 } 1231 1232 public boolean hasActiveElement() { 1233 return this.active != null && !this.active.isEmpty(); 1234 } 1235 1236 public boolean hasActive() { 1237 return this.active != null && !this.active.isEmpty(); 1238 } 1239 1240 /** 1241 * @param value {@link #active} (Whether this practitioner role record is in 1242 * active use.). This is the underlying object with id, value and 1243 * extensions. The accessor "getActive" gives direct access to the 1244 * value 1245 */ 1246 public PractitionerRole setActiveElement(BooleanType value) { 1247 this.active = value; 1248 return this; 1249 } 1250 1251 /** 1252 * @return Whether this practitioner role record is in active use. 1253 */ 1254 public boolean getActive() { 1255 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1256 } 1257 1258 /** 1259 * @param value Whether this practitioner role record is in active use. 1260 */ 1261 public PractitionerRole setActive(boolean value) { 1262 if (this.active == null) 1263 this.active = new BooleanType(); 1264 this.active.setValue(value); 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #period} (The period during which the person is authorized to 1270 * act as a practitioner in these role(s) for the organization.) 1271 */ 1272 public Period getPeriod() { 1273 if (this.period == null) 1274 if (Configuration.errorOnAutoCreate()) 1275 throw new Error("Attempt to auto-create PractitionerRole.period"); 1276 else if (Configuration.doAutoCreate()) 1277 this.period = new Period(); // cc 1278 return this.period; 1279 } 1280 1281 public boolean hasPeriod() { 1282 return this.period != null && !this.period.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #period} (The period during which the person is 1287 * authorized to act as a practitioner in these role(s) for the 1288 * organization.) 1289 */ 1290 public PractitionerRole setPeriod(Period value) { 1291 this.period = value; 1292 return this; 1293 } 1294 1295 /** 1296 * @return {@link #practitioner} (Practitioner that is able to provide the 1297 * defined services for the organization.) 1298 */ 1299 public Reference getPractitioner() { 1300 if (this.practitioner == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1303 else if (Configuration.doAutoCreate()) 1304 this.practitioner = new Reference(); // cc 1305 return this.practitioner; 1306 } 1307 1308 public boolean hasPractitioner() { 1309 return this.practitioner != null && !this.practitioner.isEmpty(); 1310 } 1311 1312 /** 1313 * @param value {@link #practitioner} (Practitioner that is able to provide the 1314 * defined services for the organization.) 1315 */ 1316 public PractitionerRole setPractitioner(Reference value) { 1317 this.practitioner = value; 1318 return this; 1319 } 1320 1321 /** 1322 * @return {@link #practitioner} The actual object that is the target of the 1323 * reference. The reference library doesn't populate this, but you can 1324 * use it to hold the resource if you resolve it. (Practitioner that is 1325 * able to provide the defined services for the organization.) 1326 */ 1327 public Practitioner getPractitionerTarget() { 1328 if (this.practitionerTarget == null) 1329 if (Configuration.errorOnAutoCreate()) 1330 throw new Error("Attempt to auto-create PractitionerRole.practitioner"); 1331 else if (Configuration.doAutoCreate()) 1332 this.practitionerTarget = new Practitioner(); // aa 1333 return this.practitionerTarget; 1334 } 1335 1336 /** 1337 * @param value {@link #practitioner} The actual object that is the target of 1338 * the reference. The reference library doesn't use these, but you 1339 * can use it to hold the resource if you resolve it. (Practitioner 1340 * that is able to provide the defined services for the 1341 * organization.) 1342 */ 1343 public PractitionerRole setPractitionerTarget(Practitioner value) { 1344 this.practitionerTarget = value; 1345 return this; 1346 } 1347 1348 /** 1349 * @return {@link #organization} (The organization where the Practitioner 1350 * performs the roles associated.) 1351 */ 1352 public Reference getOrganization() { 1353 if (this.organization == null) 1354 if (Configuration.errorOnAutoCreate()) 1355 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1356 else if (Configuration.doAutoCreate()) 1357 this.organization = new Reference(); // cc 1358 return this.organization; 1359 } 1360 1361 public boolean hasOrganization() { 1362 return this.organization != null && !this.organization.isEmpty(); 1363 } 1364 1365 /** 1366 * @param value {@link #organization} (The organization where the Practitioner 1367 * performs the roles associated.) 1368 */ 1369 public PractitionerRole setOrganization(Reference value) { 1370 this.organization = value; 1371 return this; 1372 } 1373 1374 /** 1375 * @return {@link #organization} The actual object that is the target of the 1376 * reference. The reference library doesn't populate this, but you can 1377 * use it to hold the resource if you resolve it. (The organization 1378 * where the Practitioner performs the roles associated.) 1379 */ 1380 public Organization getOrganizationTarget() { 1381 if (this.organizationTarget == null) 1382 if (Configuration.errorOnAutoCreate()) 1383 throw new Error("Attempt to auto-create PractitionerRole.organization"); 1384 else if (Configuration.doAutoCreate()) 1385 this.organizationTarget = new Organization(); // aa 1386 return this.organizationTarget; 1387 } 1388 1389 /** 1390 * @param value {@link #organization} The actual object that is the target of 1391 * the reference. The reference library doesn't use these, but you 1392 * can use it to hold the resource if you resolve it. (The 1393 * organization where the Practitioner performs the roles 1394 * associated.) 1395 */ 1396 public PractitionerRole setOrganizationTarget(Organization value) { 1397 this.organizationTarget = value; 1398 return this; 1399 } 1400 1401 /** 1402 * @return {@link #code} (Roles which this practitioner is authorized to perform 1403 * for the organization.) 1404 */ 1405 public List<CodeableConcept> getCode() { 1406 if (this.code == null) 1407 this.code = new ArrayList<CodeableConcept>(); 1408 return this.code; 1409 } 1410 1411 /** 1412 * @return Returns a reference to <code>this</code> for easy method chaining 1413 */ 1414 public PractitionerRole setCode(List<CodeableConcept> theCode) { 1415 this.code = theCode; 1416 return this; 1417 } 1418 1419 public boolean hasCode() { 1420 if (this.code == null) 1421 return false; 1422 for (CodeableConcept item : this.code) 1423 if (!item.isEmpty()) 1424 return true; 1425 return false; 1426 } 1427 1428 public CodeableConcept addCode() { // 3 1429 CodeableConcept t = new CodeableConcept(); 1430 if (this.code == null) 1431 this.code = new ArrayList<CodeableConcept>(); 1432 this.code.add(t); 1433 return t; 1434 } 1435 1436 public PractitionerRole addCode(CodeableConcept t) { // 3 1437 if (t == null) 1438 return this; 1439 if (this.code == null) 1440 this.code = new ArrayList<CodeableConcept>(); 1441 this.code.add(t); 1442 return this; 1443 } 1444 1445 /** 1446 * @return The first repetition of repeating field {@link #code}, creating it if 1447 * it does not already exist 1448 */ 1449 public CodeableConcept getCodeFirstRep() { 1450 if (getCode().isEmpty()) { 1451 addCode(); 1452 } 1453 return getCode().get(0); 1454 } 1455 1456 /** 1457 * @return {@link #specialty} (Specific specialty of the practitioner.) 1458 */ 1459 public List<CodeableConcept> getSpecialty() { 1460 if (this.specialty == null) 1461 this.specialty = new ArrayList<CodeableConcept>(); 1462 return this.specialty; 1463 } 1464 1465 /** 1466 * @return Returns a reference to <code>this</code> for easy method chaining 1467 */ 1468 public PractitionerRole setSpecialty(List<CodeableConcept> theSpecialty) { 1469 this.specialty = theSpecialty; 1470 return this; 1471 } 1472 1473 public boolean hasSpecialty() { 1474 if (this.specialty == null) 1475 return false; 1476 for (CodeableConcept item : this.specialty) 1477 if (!item.isEmpty()) 1478 return true; 1479 return false; 1480 } 1481 1482 public CodeableConcept addSpecialty() { // 3 1483 CodeableConcept t = new CodeableConcept(); 1484 if (this.specialty == null) 1485 this.specialty = new ArrayList<CodeableConcept>(); 1486 this.specialty.add(t); 1487 return t; 1488 } 1489 1490 public PractitionerRole addSpecialty(CodeableConcept t) { // 3 1491 if (t == null) 1492 return this; 1493 if (this.specialty == null) 1494 this.specialty = new ArrayList<CodeableConcept>(); 1495 this.specialty.add(t); 1496 return this; 1497 } 1498 1499 /** 1500 * @return The first repetition of repeating field {@link #specialty}, creating 1501 * it if it does not already exist 1502 */ 1503 public CodeableConcept getSpecialtyFirstRep() { 1504 if (getSpecialty().isEmpty()) { 1505 addSpecialty(); 1506 } 1507 return getSpecialty().get(0); 1508 } 1509 1510 /** 1511 * @return {@link #location} (The location(s) at which this practitioner 1512 * provides care.) 1513 */ 1514 public List<Reference> getLocation() { 1515 if (this.location == null) 1516 this.location = new ArrayList<Reference>(); 1517 return this.location; 1518 } 1519 1520 /** 1521 * @return Returns a reference to <code>this</code> for easy method chaining 1522 */ 1523 public PractitionerRole setLocation(List<Reference> theLocation) { 1524 this.location = theLocation; 1525 return this; 1526 } 1527 1528 public boolean hasLocation() { 1529 if (this.location == null) 1530 return false; 1531 for (Reference item : this.location) 1532 if (!item.isEmpty()) 1533 return true; 1534 return false; 1535 } 1536 1537 public Reference addLocation() { // 3 1538 Reference t = new Reference(); 1539 if (this.location == null) 1540 this.location = new ArrayList<Reference>(); 1541 this.location.add(t); 1542 return t; 1543 } 1544 1545 public PractitionerRole addLocation(Reference t) { // 3 1546 if (t == null) 1547 return this; 1548 if (this.location == null) 1549 this.location = new ArrayList<Reference>(); 1550 this.location.add(t); 1551 return this; 1552 } 1553 1554 /** 1555 * @return The first repetition of repeating field {@link #location}, creating 1556 * it if it does not already exist 1557 */ 1558 public Reference getLocationFirstRep() { 1559 if (getLocation().isEmpty()) { 1560 addLocation(); 1561 } 1562 return getLocation().get(0); 1563 } 1564 1565 /** 1566 * @deprecated Use Reference#setResource(IBaseResource) instead 1567 */ 1568 @Deprecated 1569 public List<Location> getLocationTarget() { 1570 if (this.locationTarget == null) 1571 this.locationTarget = new ArrayList<Location>(); 1572 return this.locationTarget; 1573 } 1574 1575 /** 1576 * @deprecated Use Reference#setResource(IBaseResource) instead 1577 */ 1578 @Deprecated 1579 public Location addLocationTarget() { 1580 Location r = new Location(); 1581 if (this.locationTarget == null) 1582 this.locationTarget = new ArrayList<Location>(); 1583 this.locationTarget.add(r); 1584 return r; 1585 } 1586 1587 /** 1588 * @return {@link #healthcareService} (The list of healthcare services that this 1589 * worker provides for this role's Organization/Location(s).) 1590 */ 1591 public List<Reference> getHealthcareService() { 1592 if (this.healthcareService == null) 1593 this.healthcareService = new ArrayList<Reference>(); 1594 return this.healthcareService; 1595 } 1596 1597 /** 1598 * @return Returns a reference to <code>this</code> for easy method chaining 1599 */ 1600 public PractitionerRole setHealthcareService(List<Reference> theHealthcareService) { 1601 this.healthcareService = theHealthcareService; 1602 return this; 1603 } 1604 1605 public boolean hasHealthcareService() { 1606 if (this.healthcareService == null) 1607 return false; 1608 for (Reference item : this.healthcareService) 1609 if (!item.isEmpty()) 1610 return true; 1611 return false; 1612 } 1613 1614 public Reference addHealthcareService() { // 3 1615 Reference t = new Reference(); 1616 if (this.healthcareService == null) 1617 this.healthcareService = new ArrayList<Reference>(); 1618 this.healthcareService.add(t); 1619 return t; 1620 } 1621 1622 public PractitionerRole addHealthcareService(Reference t) { // 3 1623 if (t == null) 1624 return this; 1625 if (this.healthcareService == null) 1626 this.healthcareService = new ArrayList<Reference>(); 1627 this.healthcareService.add(t); 1628 return this; 1629 } 1630 1631 /** 1632 * @return The first repetition of repeating field {@link #healthcareService}, 1633 * creating it if it does not already exist 1634 */ 1635 public Reference getHealthcareServiceFirstRep() { 1636 if (getHealthcareService().isEmpty()) { 1637 addHealthcareService(); 1638 } 1639 return getHealthcareService().get(0); 1640 } 1641 1642 /** 1643 * @deprecated Use Reference#setResource(IBaseResource) instead 1644 */ 1645 @Deprecated 1646 public List<HealthcareService> getHealthcareServiceTarget() { 1647 if (this.healthcareServiceTarget == null) 1648 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 1649 return this.healthcareServiceTarget; 1650 } 1651 1652 /** 1653 * @deprecated Use Reference#setResource(IBaseResource) instead 1654 */ 1655 @Deprecated 1656 public HealthcareService addHealthcareServiceTarget() { 1657 HealthcareService r = new HealthcareService(); 1658 if (this.healthcareServiceTarget == null) 1659 this.healthcareServiceTarget = new ArrayList<HealthcareService>(); 1660 this.healthcareServiceTarget.add(r); 1661 return r; 1662 } 1663 1664 /** 1665 * @return {@link #telecom} (Contact details that are specific to the 1666 * role/location/service.) 1667 */ 1668 public List<ContactPoint> getTelecom() { 1669 if (this.telecom == null) 1670 this.telecom = new ArrayList<ContactPoint>(); 1671 return this.telecom; 1672 } 1673 1674 /** 1675 * @return Returns a reference to <code>this</code> for easy method chaining 1676 */ 1677 public PractitionerRole setTelecom(List<ContactPoint> theTelecom) { 1678 this.telecom = theTelecom; 1679 return this; 1680 } 1681 1682 public boolean hasTelecom() { 1683 if (this.telecom == null) 1684 return false; 1685 for (ContactPoint item : this.telecom) 1686 if (!item.isEmpty()) 1687 return true; 1688 return false; 1689 } 1690 1691 public ContactPoint addTelecom() { // 3 1692 ContactPoint t = new ContactPoint(); 1693 if (this.telecom == null) 1694 this.telecom = new ArrayList<ContactPoint>(); 1695 this.telecom.add(t); 1696 return t; 1697 } 1698 1699 public PractitionerRole addTelecom(ContactPoint t) { // 3 1700 if (t == null) 1701 return this; 1702 if (this.telecom == null) 1703 this.telecom = new ArrayList<ContactPoint>(); 1704 this.telecom.add(t); 1705 return this; 1706 } 1707 1708 /** 1709 * @return The first repetition of repeating field {@link #telecom}, creating it 1710 * if it does not already exist 1711 */ 1712 public ContactPoint getTelecomFirstRep() { 1713 if (getTelecom().isEmpty()) { 1714 addTelecom(); 1715 } 1716 return getTelecom().get(0); 1717 } 1718 1719 /** 1720 * @return {@link #availableTime} (A collection of times the practitioner is 1721 * available or performing this role at the location and/or 1722 * healthcareservice.) 1723 */ 1724 public List<PractitionerRoleAvailableTimeComponent> getAvailableTime() { 1725 if (this.availableTime == null) 1726 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1727 return this.availableTime; 1728 } 1729 1730 /** 1731 * @return Returns a reference to <code>this</code> for easy method chaining 1732 */ 1733 public PractitionerRole setAvailableTime(List<PractitionerRoleAvailableTimeComponent> theAvailableTime) { 1734 this.availableTime = theAvailableTime; 1735 return this; 1736 } 1737 1738 public boolean hasAvailableTime() { 1739 if (this.availableTime == null) 1740 return false; 1741 for (PractitionerRoleAvailableTimeComponent item : this.availableTime) 1742 if (!item.isEmpty()) 1743 return true; 1744 return false; 1745 } 1746 1747 public PractitionerRoleAvailableTimeComponent addAvailableTime() { // 3 1748 PractitionerRoleAvailableTimeComponent t = new PractitionerRoleAvailableTimeComponent(); 1749 if (this.availableTime == null) 1750 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1751 this.availableTime.add(t); 1752 return t; 1753 } 1754 1755 public PractitionerRole addAvailableTime(PractitionerRoleAvailableTimeComponent t) { // 3 1756 if (t == null) 1757 return this; 1758 if (this.availableTime == null) 1759 this.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 1760 this.availableTime.add(t); 1761 return this; 1762 } 1763 1764 /** 1765 * @return The first repetition of repeating field {@link #availableTime}, 1766 * creating it if it does not already exist 1767 */ 1768 public PractitionerRoleAvailableTimeComponent getAvailableTimeFirstRep() { 1769 if (getAvailableTime().isEmpty()) { 1770 addAvailableTime(); 1771 } 1772 return getAvailableTime().get(0); 1773 } 1774 1775 /** 1776 * @return {@link #notAvailable} (The practitioner is not available or 1777 * performing this role during this period of time due to the provided 1778 * reason.) 1779 */ 1780 public List<PractitionerRoleNotAvailableComponent> getNotAvailable() { 1781 if (this.notAvailable == null) 1782 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1783 return this.notAvailable; 1784 } 1785 1786 /** 1787 * @return Returns a reference to <code>this</code> for easy method chaining 1788 */ 1789 public PractitionerRole setNotAvailable(List<PractitionerRoleNotAvailableComponent> theNotAvailable) { 1790 this.notAvailable = theNotAvailable; 1791 return this; 1792 } 1793 1794 public boolean hasNotAvailable() { 1795 if (this.notAvailable == null) 1796 return false; 1797 for (PractitionerRoleNotAvailableComponent item : this.notAvailable) 1798 if (!item.isEmpty()) 1799 return true; 1800 return false; 1801 } 1802 1803 public PractitionerRoleNotAvailableComponent addNotAvailable() { // 3 1804 PractitionerRoleNotAvailableComponent t = new PractitionerRoleNotAvailableComponent(); 1805 if (this.notAvailable == null) 1806 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1807 this.notAvailable.add(t); 1808 return t; 1809 } 1810 1811 public PractitionerRole addNotAvailable(PractitionerRoleNotAvailableComponent t) { // 3 1812 if (t == null) 1813 return this; 1814 if (this.notAvailable == null) 1815 this.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 1816 this.notAvailable.add(t); 1817 return this; 1818 } 1819 1820 /** 1821 * @return The first repetition of repeating field {@link #notAvailable}, 1822 * creating it if it does not already exist 1823 */ 1824 public PractitionerRoleNotAvailableComponent getNotAvailableFirstRep() { 1825 if (getNotAvailable().isEmpty()) { 1826 addNotAvailable(); 1827 } 1828 return getNotAvailable().get(0); 1829 } 1830 1831 /** 1832 * @return {@link #availabilityExceptions} (A description of site availability 1833 * exceptions, e.g. public holiday availability. Succinctly describing 1834 * all possible exceptions to normal site availability as details in the 1835 * available Times and not available Times.). This is the underlying 1836 * object with id, value and extensions. The accessor 1837 * "getAvailabilityExceptions" gives direct access to the value 1838 */ 1839 public StringType getAvailabilityExceptionsElement() { 1840 if (this.availabilityExceptions == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create PractitionerRole.availabilityExceptions"); 1843 else if (Configuration.doAutoCreate()) 1844 this.availabilityExceptions = new StringType(); // bb 1845 return this.availabilityExceptions; 1846 } 1847 1848 public boolean hasAvailabilityExceptionsElement() { 1849 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1850 } 1851 1852 public boolean hasAvailabilityExceptions() { 1853 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 1854 } 1855 1856 /** 1857 * @param value {@link #availabilityExceptions} (A description of site 1858 * availability exceptions, e.g. public holiday availability. 1859 * Succinctly describing all possible exceptions to normal site 1860 * availability as details in the available Times and not available 1861 * Times.). This is the underlying object with id, value and 1862 * extensions. The accessor "getAvailabilityExceptions" gives 1863 * direct access to the value 1864 */ 1865 public PractitionerRole setAvailabilityExceptionsElement(StringType value) { 1866 this.availabilityExceptions = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return A description of site availability exceptions, e.g. public holiday 1872 * availability. Succinctly describing all possible exceptions to normal 1873 * site availability as details in the available Times and not available 1874 * Times. 1875 */ 1876 public String getAvailabilityExceptions() { 1877 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 1878 } 1879 1880 /** 1881 * @param value A description of site availability exceptions, e.g. public 1882 * holiday availability. Succinctly describing all possible 1883 * exceptions to normal site availability as details in the 1884 * available Times and not available Times. 1885 */ 1886 public PractitionerRole setAvailabilityExceptions(String value) { 1887 if (Utilities.noString(value)) 1888 this.availabilityExceptions = null; 1889 else { 1890 if (this.availabilityExceptions == null) 1891 this.availabilityExceptions = new StringType(); 1892 this.availabilityExceptions.setValue(value); 1893 } 1894 return this; 1895 } 1896 1897 /** 1898 * @return {@link #endpoint} (Technical endpoints providing access to services 1899 * operated for the practitioner with this role.) 1900 */ 1901 public List<Reference> getEndpoint() { 1902 if (this.endpoint == null) 1903 this.endpoint = new ArrayList<Reference>(); 1904 return this.endpoint; 1905 } 1906 1907 /** 1908 * @return Returns a reference to <code>this</code> for easy method chaining 1909 */ 1910 public PractitionerRole setEndpoint(List<Reference> theEndpoint) { 1911 this.endpoint = theEndpoint; 1912 return this; 1913 } 1914 1915 public boolean hasEndpoint() { 1916 if (this.endpoint == null) 1917 return false; 1918 for (Reference item : this.endpoint) 1919 if (!item.isEmpty()) 1920 return true; 1921 return false; 1922 } 1923 1924 public Reference addEndpoint() { // 3 1925 Reference t = new Reference(); 1926 if (this.endpoint == null) 1927 this.endpoint = new ArrayList<Reference>(); 1928 this.endpoint.add(t); 1929 return t; 1930 } 1931 1932 public PractitionerRole addEndpoint(Reference t) { // 3 1933 if (t == null) 1934 return this; 1935 if (this.endpoint == null) 1936 this.endpoint = new ArrayList<Reference>(); 1937 this.endpoint.add(t); 1938 return this; 1939 } 1940 1941 /** 1942 * @return The first repetition of repeating field {@link #endpoint}, creating 1943 * it if it does not already exist 1944 */ 1945 public Reference getEndpointFirstRep() { 1946 if (getEndpoint().isEmpty()) { 1947 addEndpoint(); 1948 } 1949 return getEndpoint().get(0); 1950 } 1951 1952 /** 1953 * @deprecated Use Reference#setResource(IBaseResource) instead 1954 */ 1955 @Deprecated 1956 public List<Endpoint> getEndpointTarget() { 1957 if (this.endpointTarget == null) 1958 this.endpointTarget = new ArrayList<Endpoint>(); 1959 return this.endpointTarget; 1960 } 1961 1962 /** 1963 * @deprecated Use Reference#setResource(IBaseResource) instead 1964 */ 1965 @Deprecated 1966 public Endpoint addEndpointTarget() { 1967 Endpoint r = new Endpoint(); 1968 if (this.endpointTarget == null) 1969 this.endpointTarget = new ArrayList<Endpoint>(); 1970 this.endpointTarget.add(r); 1971 return r; 1972 } 1973 1974 protected void listChildren(List<Property> children) { 1975 super.listChildren(children); 1976 children.add(new Property("identifier", "Identifier", "Business Identifiers that are specific to a role/location.", 1977 0, java.lang.Integer.MAX_VALUE, identifier)); 1978 children.add( 1979 new Property("active", "boolean", "Whether this practitioner role record is in active use.", 0, 1, active)); 1980 children.add(new Property("period", "Period", 1981 "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 1982 0, 1, period)); 1983 children.add(new Property("practitioner", "Reference(Practitioner)", 1984 "Practitioner that is able to provide the defined services for the organization.", 0, 1, practitioner)); 1985 children.add(new Property("organization", "Reference(Organization)", 1986 "The organization where the Practitioner performs the roles associated.", 0, 1, organization)); 1987 children.add(new Property("code", "CodeableConcept", 1988 "Roles which this practitioner is authorized to perform for the organization.", 0, java.lang.Integer.MAX_VALUE, 1989 code)); 1990 children.add(new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, 1991 java.lang.Integer.MAX_VALUE, specialty)); 1992 children.add(new Property("location", "Reference(Location)", 1993 "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location)); 1994 children.add(new Property("healthcareService", "Reference(HealthcareService)", 1995 "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, 1996 java.lang.Integer.MAX_VALUE, healthcareService)); 1997 children.add(new Property("telecom", "ContactPoint", 1998 "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1999 children.add(new Property("availableTime", "", 2000 "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.", 2001 0, java.lang.Integer.MAX_VALUE, availableTime)); 2002 children.add(new Property("notAvailable", "", 2003 "The practitioner is not available or performing this role during this period of time due to the provided reason.", 2004 0, java.lang.Integer.MAX_VALUE, notAvailable)); 2005 children.add(new Property("availabilityExceptions", "string", 2006 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2007 0, 1, availabilityExceptions)); 2008 children.add(new Property("endpoint", "Reference(Endpoint)", 2009 "Technical endpoints providing access to services operated for the practitioner with this role.", 0, 2010 java.lang.Integer.MAX_VALUE, endpoint)); 2011 } 2012 2013 @Override 2014 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2015 switch (_hash) { 2016 case -1618432855: 2017 /* identifier */ return new Property("identifier", "Identifier", 2018 "Business Identifiers that are specific to a role/location.", 0, java.lang.Integer.MAX_VALUE, identifier); 2019 case -1422950650: 2020 /* active */ return new Property("active", "boolean", "Whether this practitioner role record is in active use.", 2021 0, 1, active); 2022 case -991726143: 2023 /* period */ return new Property("period", "Period", 2024 "The period during which the person is authorized to act as a practitioner in these role(s) for the organization.", 2025 0, 1, period); 2026 case 574573338: 2027 /* practitioner */ return new Property("practitioner", "Reference(Practitioner)", 2028 "Practitioner that is able to provide the defined services for the organization.", 0, 1, practitioner); 2029 case 1178922291: 2030 /* organization */ return new Property("organization", "Reference(Organization)", 2031 "The organization where the Practitioner performs the roles associated.", 0, 1, organization); 2032 case 3059181: 2033 /* code */ return new Property("code", "CodeableConcept", 2034 "Roles which this practitioner is authorized to perform for the organization.", 0, 2035 java.lang.Integer.MAX_VALUE, code); 2036 case -1694759682: 2037 /* specialty */ return new Property("specialty", "CodeableConcept", "Specific specialty of the practitioner.", 0, 2038 java.lang.Integer.MAX_VALUE, specialty); 2039 case 1901043637: 2040 /* location */ return new Property("location", "Reference(Location)", 2041 "The location(s) at which this practitioner provides care.", 0, java.lang.Integer.MAX_VALUE, location); 2042 case 1289661064: 2043 /* healthcareService */ return new Property("healthcareService", "Reference(HealthcareService)", 2044 "The list of healthcare services that this worker provides for this role's Organization/Location(s).", 0, 2045 java.lang.Integer.MAX_VALUE, healthcareService); 2046 case -1429363305: 2047 /* telecom */ return new Property("telecom", "ContactPoint", 2048 "Contact details that are specific to the role/location/service.", 0, java.lang.Integer.MAX_VALUE, telecom); 2049 case 1873069366: 2050 /* availableTime */ return new Property("availableTime", "", 2051 "A collection of times the practitioner is available or performing this role at the location and/or healthcareservice.", 2052 0, java.lang.Integer.MAX_VALUE, availableTime); 2053 case -629572298: 2054 /* notAvailable */ return new Property("notAvailable", "", 2055 "The practitioner is not available or performing this role during this period of time due to the provided reason.", 2056 0, java.lang.Integer.MAX_VALUE, notAvailable); 2057 case -1149143617: 2058 /* availabilityExceptions */ return new Property("availabilityExceptions", "string", 2059 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2060 0, 1, availabilityExceptions); 2061 case 1741102485: 2062 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 2063 "Technical endpoints providing access to services operated for the practitioner with this role.", 0, 2064 java.lang.Integer.MAX_VALUE, endpoint); 2065 default: 2066 return super.getNamedProperty(_hash, _name, _checkValid); 2067 } 2068 2069 } 2070 2071 @Override 2072 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2073 switch (hash) { 2074 case -1618432855: 2075 /* identifier */ return this.identifier == null ? new Base[0] 2076 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2077 case -1422950650: 2078 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 2079 case -991726143: 2080 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2081 case 574573338: 2082 /* practitioner */ return this.practitioner == null ? new Base[0] : new Base[] { this.practitioner }; // Reference 2083 case 1178922291: 2084 /* organization */ return this.organization == null ? new Base[0] : new Base[] { this.organization }; // Reference 2085 case 3059181: 2086 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 2087 case -1694759682: 2088 /* specialty */ return this.specialty == null ? new Base[0] 2089 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 2090 case 1901043637: 2091 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 2092 case 1289661064: 2093 /* healthcareService */ return this.healthcareService == null ? new Base[0] 2094 : this.healthcareService.toArray(new Base[this.healthcareService.size()]); // Reference 2095 case -1429363305: 2096 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2097 case 1873069366: 2098 /* availableTime */ return this.availableTime == null ? new Base[0] 2099 : this.availableTime.toArray(new Base[this.availableTime.size()]); // PractitionerRoleAvailableTimeComponent 2100 case -629572298: 2101 /* notAvailable */ return this.notAvailable == null ? new Base[0] 2102 : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // PractitionerRoleNotAvailableComponent 2103 case -1149143617: 2104 /* availabilityExceptions */ return this.availabilityExceptions == null ? new Base[0] 2105 : new Base[] { this.availabilityExceptions }; // StringType 2106 case 1741102485: 2107 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2108 default: 2109 return super.getProperty(hash, name, checkValid); 2110 } 2111 2112 } 2113 2114 @Override 2115 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2116 switch (hash) { 2117 case -1618432855: // identifier 2118 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2119 return value; 2120 case -1422950650: // active 2121 this.active = castToBoolean(value); // BooleanType 2122 return value; 2123 case -991726143: // period 2124 this.period = castToPeriod(value); // Period 2125 return value; 2126 case 574573338: // practitioner 2127 this.practitioner = castToReference(value); // Reference 2128 return value; 2129 case 1178922291: // organization 2130 this.organization = castToReference(value); // Reference 2131 return value; 2132 case 3059181: // code 2133 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 2134 return value; 2135 case -1694759682: // specialty 2136 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 2137 return value; 2138 case 1901043637: // location 2139 this.getLocation().add(castToReference(value)); // Reference 2140 return value; 2141 case 1289661064: // healthcareService 2142 this.getHealthcareService().add(castToReference(value)); // Reference 2143 return value; 2144 case -1429363305: // telecom 2145 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2146 return value; 2147 case 1873069366: // availableTime 2148 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); // PractitionerRoleAvailableTimeComponent 2149 return value; 2150 case -629572298: // notAvailable 2151 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); // PractitionerRoleNotAvailableComponent 2152 return value; 2153 case -1149143617: // availabilityExceptions 2154 this.availabilityExceptions = castToString(value); // StringType 2155 return value; 2156 case 1741102485: // endpoint 2157 this.getEndpoint().add(castToReference(value)); // Reference 2158 return value; 2159 default: 2160 return super.setProperty(hash, name, value); 2161 } 2162 2163 } 2164 2165 @Override 2166 public Base setProperty(String name, Base value) throws FHIRException { 2167 if (name.equals("identifier")) { 2168 this.getIdentifier().add(castToIdentifier(value)); 2169 } else if (name.equals("active")) { 2170 this.active = castToBoolean(value); // BooleanType 2171 } else if (name.equals("period")) { 2172 this.period = castToPeriod(value); // Period 2173 } else if (name.equals("practitioner")) { 2174 this.practitioner = castToReference(value); // Reference 2175 } else if (name.equals("organization")) { 2176 this.organization = castToReference(value); // Reference 2177 } else if (name.equals("code")) { 2178 this.getCode().add(castToCodeableConcept(value)); 2179 } else if (name.equals("specialty")) { 2180 this.getSpecialty().add(castToCodeableConcept(value)); 2181 } else if (name.equals("location")) { 2182 this.getLocation().add(castToReference(value)); 2183 } else if (name.equals("healthcareService")) { 2184 this.getHealthcareService().add(castToReference(value)); 2185 } else if (name.equals("telecom")) { 2186 this.getTelecom().add(castToContactPoint(value)); 2187 } else if (name.equals("availableTime")) { 2188 this.getAvailableTime().add((PractitionerRoleAvailableTimeComponent) value); 2189 } else if (name.equals("notAvailable")) { 2190 this.getNotAvailable().add((PractitionerRoleNotAvailableComponent) value); 2191 } else if (name.equals("availabilityExceptions")) { 2192 this.availabilityExceptions = castToString(value); // StringType 2193 } else if (name.equals("endpoint")) { 2194 this.getEndpoint().add(castToReference(value)); 2195 } else 2196 return super.setProperty(name, value); 2197 return value; 2198 } 2199 2200 @Override 2201 public Base makeProperty(int hash, String name) throws FHIRException { 2202 switch (hash) { 2203 case -1618432855: 2204 return addIdentifier(); 2205 case -1422950650: 2206 return getActiveElement(); 2207 case -991726143: 2208 return getPeriod(); 2209 case 574573338: 2210 return getPractitioner(); 2211 case 1178922291: 2212 return getOrganization(); 2213 case 3059181: 2214 return addCode(); 2215 case -1694759682: 2216 return addSpecialty(); 2217 case 1901043637: 2218 return addLocation(); 2219 case 1289661064: 2220 return addHealthcareService(); 2221 case -1429363305: 2222 return addTelecom(); 2223 case 1873069366: 2224 return addAvailableTime(); 2225 case -629572298: 2226 return addNotAvailable(); 2227 case -1149143617: 2228 return getAvailabilityExceptionsElement(); 2229 case 1741102485: 2230 return addEndpoint(); 2231 default: 2232 return super.makeProperty(hash, name); 2233 } 2234 2235 } 2236 2237 @Override 2238 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2239 switch (hash) { 2240 case -1618432855: 2241 /* identifier */ return new String[] { "Identifier" }; 2242 case -1422950650: 2243 /* active */ return new String[] { "boolean" }; 2244 case -991726143: 2245 /* period */ return new String[] { "Period" }; 2246 case 574573338: 2247 /* practitioner */ return new String[] { "Reference" }; 2248 case 1178922291: 2249 /* organization */ return new String[] { "Reference" }; 2250 case 3059181: 2251 /* code */ return new String[] { "CodeableConcept" }; 2252 case -1694759682: 2253 /* specialty */ return new String[] { "CodeableConcept" }; 2254 case 1901043637: 2255 /* location */ return new String[] { "Reference" }; 2256 case 1289661064: 2257 /* healthcareService */ return new String[] { "Reference" }; 2258 case -1429363305: 2259 /* telecom */ return new String[] { "ContactPoint" }; 2260 case 1873069366: 2261 /* availableTime */ return new String[] {}; 2262 case -629572298: 2263 /* notAvailable */ return new String[] {}; 2264 case -1149143617: 2265 /* availabilityExceptions */ return new String[] { "string" }; 2266 case 1741102485: 2267 /* endpoint */ return new String[] { "Reference" }; 2268 default: 2269 return super.getTypesForProperty(hash, name); 2270 } 2271 2272 } 2273 2274 @Override 2275 public Base addChild(String name) throws FHIRException { 2276 if (name.equals("identifier")) { 2277 return addIdentifier(); 2278 } else if (name.equals("active")) { 2279 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.active"); 2280 } else if (name.equals("period")) { 2281 this.period = new Period(); 2282 return this.period; 2283 } else if (name.equals("practitioner")) { 2284 this.practitioner = new Reference(); 2285 return this.practitioner; 2286 } else if (name.equals("organization")) { 2287 this.organization = new Reference(); 2288 return this.organization; 2289 } else if (name.equals("code")) { 2290 return addCode(); 2291 } else if (name.equals("specialty")) { 2292 return addSpecialty(); 2293 } else if (name.equals("location")) { 2294 return addLocation(); 2295 } else if (name.equals("healthcareService")) { 2296 return addHealthcareService(); 2297 } else if (name.equals("telecom")) { 2298 return addTelecom(); 2299 } else if (name.equals("availableTime")) { 2300 return addAvailableTime(); 2301 } else if (name.equals("notAvailable")) { 2302 return addNotAvailable(); 2303 } else if (name.equals("availabilityExceptions")) { 2304 throw new FHIRException("Cannot call addChild on a singleton property PractitionerRole.availabilityExceptions"); 2305 } else if (name.equals("endpoint")) { 2306 return addEndpoint(); 2307 } else 2308 return super.addChild(name); 2309 } 2310 2311 public String fhirType() { 2312 return "PractitionerRole"; 2313 2314 } 2315 2316 public PractitionerRole copy() { 2317 PractitionerRole dst = new PractitionerRole(); 2318 copyValues(dst); 2319 return dst; 2320 } 2321 2322 public void copyValues(PractitionerRole dst) { 2323 super.copyValues(dst); 2324 if (identifier != null) { 2325 dst.identifier = new ArrayList<Identifier>(); 2326 for (Identifier i : identifier) 2327 dst.identifier.add(i.copy()); 2328 } 2329 ; 2330 dst.active = active == null ? null : active.copy(); 2331 dst.period = period == null ? null : period.copy(); 2332 dst.practitioner = practitioner == null ? null : practitioner.copy(); 2333 dst.organization = organization == null ? null : organization.copy(); 2334 if (code != null) { 2335 dst.code = new ArrayList<CodeableConcept>(); 2336 for (CodeableConcept i : code) 2337 dst.code.add(i.copy()); 2338 } 2339 ; 2340 if (specialty != null) { 2341 dst.specialty = new ArrayList<CodeableConcept>(); 2342 for (CodeableConcept i : specialty) 2343 dst.specialty.add(i.copy()); 2344 } 2345 ; 2346 if (location != null) { 2347 dst.location = new ArrayList<Reference>(); 2348 for (Reference i : location) 2349 dst.location.add(i.copy()); 2350 } 2351 ; 2352 if (healthcareService != null) { 2353 dst.healthcareService = new ArrayList<Reference>(); 2354 for (Reference i : healthcareService) 2355 dst.healthcareService.add(i.copy()); 2356 } 2357 ; 2358 if (telecom != null) { 2359 dst.telecom = new ArrayList<ContactPoint>(); 2360 for (ContactPoint i : telecom) 2361 dst.telecom.add(i.copy()); 2362 } 2363 ; 2364 if (availableTime != null) { 2365 dst.availableTime = new ArrayList<PractitionerRoleAvailableTimeComponent>(); 2366 for (PractitionerRoleAvailableTimeComponent i : availableTime) 2367 dst.availableTime.add(i.copy()); 2368 } 2369 ; 2370 if (notAvailable != null) { 2371 dst.notAvailable = new ArrayList<PractitionerRoleNotAvailableComponent>(); 2372 for (PractitionerRoleNotAvailableComponent i : notAvailable) 2373 dst.notAvailable.add(i.copy()); 2374 } 2375 ; 2376 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 2377 if (endpoint != null) { 2378 dst.endpoint = new ArrayList<Reference>(); 2379 for (Reference i : endpoint) 2380 dst.endpoint.add(i.copy()); 2381 } 2382 ; 2383 } 2384 2385 protected PractitionerRole typedCopy() { 2386 return copy(); 2387 } 2388 2389 @Override 2390 public boolean equalsDeep(Base other_) { 2391 if (!super.equalsDeep(other_)) 2392 return false; 2393 if (!(other_ instanceof PractitionerRole)) 2394 return false; 2395 PractitionerRole o = (PractitionerRole) other_; 2396 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2397 && compareDeep(period, o.period, true) && compareDeep(practitioner, o.practitioner, true) 2398 && compareDeep(organization, o.organization, true) && compareDeep(code, o.code, true) 2399 && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) 2400 && compareDeep(healthcareService, o.healthcareService, true) && compareDeep(telecom, o.telecom, true) 2401 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 2402 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) 2403 && compareDeep(endpoint, o.endpoint, true); 2404 } 2405 2406 @Override 2407 public boolean equalsShallow(Base other_) { 2408 if (!super.equalsShallow(other_)) 2409 return false; 2410 if (!(other_ instanceof PractitionerRole)) 2411 return false; 2412 PractitionerRole o = (PractitionerRole) other_; 2413 return compareValues(active, o.active, true) 2414 && compareValues(availabilityExceptions, o.availabilityExceptions, true); 2415 } 2416 2417 public boolean isEmpty() { 2418 return super.isEmpty() 2419 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, period, practitioner, organization, code, specialty, 2420 location, healthcareService, telecom, availableTime, notAvailable, availabilityExceptions, endpoint); 2421 } 2422 2423 @Override 2424 public ResourceType getResourceType() { 2425 return ResourceType.PractitionerRole; 2426 } 2427 2428 /** 2429 * Search parameter: <b>date</b> 2430 * <p> 2431 * Description: <b>The period during which the practitioner is authorized to 2432 * perform in these role(s)</b><br> 2433 * Type: <b>date</b><br> 2434 * Path: <b>PractitionerRole.period</b><br> 2435 * </p> 2436 */ 2437 @SearchParamDefinition(name = "date", path = "PractitionerRole.period", description = "The period during which the practitioner is authorized to perform in these role(s)", type = "date") 2438 public static final String SP_DATE = "date"; 2439 /** 2440 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2441 * <p> 2442 * Description: <b>The period during which the practitioner is authorized to 2443 * perform in these role(s)</b><br> 2444 * Type: <b>date</b><br> 2445 * Path: <b>PractitionerRole.period</b><br> 2446 * </p> 2447 */ 2448 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2449 SP_DATE); 2450 2451 /** 2452 * Search parameter: <b>identifier</b> 2453 * <p> 2454 * Description: <b>A practitioner's Identifier</b><br> 2455 * Type: <b>token</b><br> 2456 * Path: <b>PractitionerRole.identifier</b><br> 2457 * </p> 2458 */ 2459 @SearchParamDefinition(name = "identifier", path = "PractitionerRole.identifier", description = "A practitioner's Identifier", type = "token") 2460 public static final String SP_IDENTIFIER = "identifier"; 2461 /** 2462 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2463 * <p> 2464 * Description: <b>A practitioner's Identifier</b><br> 2465 * Type: <b>token</b><br> 2466 * Path: <b>PractitionerRole.identifier</b><br> 2467 * </p> 2468 */ 2469 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2470 SP_IDENTIFIER); 2471 2472 /** 2473 * Search parameter: <b>specialty</b> 2474 * <p> 2475 * Description: <b>The practitioner has this specialty at an 2476 * organization</b><br> 2477 * Type: <b>token</b><br> 2478 * Path: <b>PractitionerRole.specialty</b><br> 2479 * </p> 2480 */ 2481 @SearchParamDefinition(name = "specialty", path = "PractitionerRole.specialty", description = "The practitioner has this specialty at an organization", type = "token") 2482 public static final String SP_SPECIALTY = "specialty"; 2483 /** 2484 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 2485 * <p> 2486 * Description: <b>The practitioner has this specialty at an 2487 * organization</b><br> 2488 * Type: <b>token</b><br> 2489 * Path: <b>PractitionerRole.specialty</b><br> 2490 * </p> 2491 */ 2492 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2493 SP_SPECIALTY); 2494 2495 /** 2496 * Search parameter: <b>role</b> 2497 * <p> 2498 * Description: <b>The practitioner can perform this role at for the 2499 * organization</b><br> 2500 * Type: <b>token</b><br> 2501 * Path: <b>PractitionerRole.code</b><br> 2502 * </p> 2503 */ 2504 @SearchParamDefinition(name = "role", path = "PractitionerRole.code", description = "The practitioner can perform this role at for the organization", type = "token") 2505 public static final String SP_ROLE = "role"; 2506 /** 2507 * <b>Fluent Client</b> search parameter constant for <b>role</b> 2508 * <p> 2509 * Description: <b>The practitioner can perform this role at for the 2510 * organization</b><br> 2511 * Type: <b>token</b><br> 2512 * Path: <b>PractitionerRole.code</b><br> 2513 * </p> 2514 */ 2515 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2516 SP_ROLE); 2517 2518 /** 2519 * Search parameter: <b>practitioner</b> 2520 * <p> 2521 * Description: <b>Practitioner that is able to provide the defined services for 2522 * the organization</b><br> 2523 * Type: <b>reference</b><br> 2524 * Path: <b>PractitionerRole.practitioner</b><br> 2525 * </p> 2526 */ 2527 @SearchParamDefinition(name = "practitioner", path = "PractitionerRole.practitioner", description = "Practitioner that is able to provide the defined services for the organization", type = "reference", providesMembershipIn = { 2528 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 2529 public static final String SP_PRACTITIONER = "practitioner"; 2530 /** 2531 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 2532 * <p> 2533 * Description: <b>Practitioner that is able to provide the defined services for 2534 * the organization</b><br> 2535 * Type: <b>reference</b><br> 2536 * Path: <b>PractitionerRole.practitioner</b><br> 2537 * </p> 2538 */ 2539 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2540 SP_PRACTITIONER); 2541 2542 /** 2543 * Constant for fluent queries to be used to add include statements. Specifies 2544 * the path value of "<b>PractitionerRole:practitioner</b>". 2545 */ 2546 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 2547 "PractitionerRole:practitioner").toLocked(); 2548 2549 /** 2550 * Search parameter: <b>active</b> 2551 * <p> 2552 * Description: <b>Whether this practitioner role record is in active 2553 * use</b><br> 2554 * Type: <b>token</b><br> 2555 * Path: <b>PractitionerRole.active</b><br> 2556 * </p> 2557 */ 2558 @SearchParamDefinition(name = "active", path = "PractitionerRole.active", description = "Whether this practitioner role record is in active use", type = "token") 2559 public static final String SP_ACTIVE = "active"; 2560 /** 2561 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2562 * <p> 2563 * Description: <b>Whether this practitioner role record is in active 2564 * use</b><br> 2565 * Type: <b>token</b><br> 2566 * Path: <b>PractitionerRole.active</b><br> 2567 * </p> 2568 */ 2569 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2570 SP_ACTIVE); 2571 2572 /** 2573 * Search parameter: <b>endpoint</b> 2574 * <p> 2575 * Description: <b>Technical endpoints providing access to services operated for 2576 * the practitioner with this role</b><br> 2577 * Type: <b>reference</b><br> 2578 * Path: <b>PractitionerRole.endpoint</b><br> 2579 * </p> 2580 */ 2581 @SearchParamDefinition(name = "endpoint", path = "PractitionerRole.endpoint", description = "Technical endpoints providing access to services operated for the practitioner with this role", type = "reference", target = { 2582 Endpoint.class }) 2583 public static final String SP_ENDPOINT = "endpoint"; 2584 /** 2585 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2586 * <p> 2587 * Description: <b>Technical endpoints providing access to services operated for 2588 * the practitioner with this role</b><br> 2589 * Type: <b>reference</b><br> 2590 * Path: <b>PractitionerRole.endpoint</b><br> 2591 * </p> 2592 */ 2593 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2594 SP_ENDPOINT); 2595 2596 /** 2597 * Constant for fluent queries to be used to add include statements. Specifies 2598 * the path value of "<b>PractitionerRole:endpoint</b>". 2599 */ 2600 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 2601 "PractitionerRole:endpoint").toLocked(); 2602 2603 /** 2604 * Search parameter: <b>phone</b> 2605 * <p> 2606 * Description: <b>A value in a phone contact</b><br> 2607 * Type: <b>token</b><br> 2608 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2609 * </p> 2610 */ 2611 @SearchParamDefinition(name = "phone", path = "PractitionerRole.telecom.where(system='phone')", description = "A value in a phone contact", type = "token") 2612 public static final String SP_PHONE = "phone"; 2613 /** 2614 * <b>Fluent Client</b> search parameter constant for <b>phone</b> 2615 * <p> 2616 * Description: <b>A value in a phone contact</b><br> 2617 * Type: <b>token</b><br> 2618 * Path: <b>PractitionerRole.telecom(system=phone)</b><br> 2619 * </p> 2620 */ 2621 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PHONE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2622 SP_PHONE); 2623 2624 /** 2625 * Search parameter: <b>service</b> 2626 * <p> 2627 * Description: <b>The list of healthcare services that this worker provides for 2628 * this role's Organization/Location(s)</b><br> 2629 * Type: <b>reference</b><br> 2630 * Path: <b>PractitionerRole.healthcareService</b><br> 2631 * </p> 2632 */ 2633 @SearchParamDefinition(name = "service", path = "PractitionerRole.healthcareService", description = "The list of healthcare services that this worker provides for this role's Organization/Location(s)", type = "reference", target = { 2634 HealthcareService.class }) 2635 public static final String SP_SERVICE = "service"; 2636 /** 2637 * <b>Fluent Client</b> search parameter constant for <b>service</b> 2638 * <p> 2639 * Description: <b>The list of healthcare services that this worker provides for 2640 * this role's Organization/Location(s)</b><br> 2641 * Type: <b>reference</b><br> 2642 * Path: <b>PractitionerRole.healthcareService</b><br> 2643 * </p> 2644 */ 2645 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2646 SP_SERVICE); 2647 2648 /** 2649 * Constant for fluent queries to be used to add include statements. Specifies 2650 * the path value of "<b>PractitionerRole:service</b>". 2651 */ 2652 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE = new ca.uhn.fhir.model.api.Include( 2653 "PractitionerRole:service").toLocked(); 2654 2655 /** 2656 * Search parameter: <b>organization</b> 2657 * <p> 2658 * Description: <b>The identity of the organization the practitioner represents 2659 * / acts on behalf of</b><br> 2660 * Type: <b>reference</b><br> 2661 * Path: <b>PractitionerRole.organization</b><br> 2662 * </p> 2663 */ 2664 @SearchParamDefinition(name = "organization", path = "PractitionerRole.organization", description = "The identity of the organization the practitioner represents / acts on behalf of", type = "reference", target = { 2665 Organization.class }) 2666 public static final String SP_ORGANIZATION = "organization"; 2667 /** 2668 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2669 * <p> 2670 * Description: <b>The identity of the organization the practitioner represents 2671 * / acts on behalf of</b><br> 2672 * Type: <b>reference</b><br> 2673 * Path: <b>PractitionerRole.organization</b><br> 2674 * </p> 2675 */ 2676 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2677 SP_ORGANIZATION); 2678 2679 /** 2680 * Constant for fluent queries to be used to add include statements. Specifies 2681 * the path value of "<b>PractitionerRole:organization</b>". 2682 */ 2683 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 2684 "PractitionerRole:organization").toLocked(); 2685 2686 /** 2687 * Search parameter: <b>telecom</b> 2688 * <p> 2689 * Description: <b>The value in any kind of contact</b><br> 2690 * Type: <b>token</b><br> 2691 * Path: <b>PractitionerRole.telecom</b><br> 2692 * </p> 2693 */ 2694 @SearchParamDefinition(name = "telecom", path = "PractitionerRole.telecom", description = "The value in any kind of contact", type = "token") 2695 public static final String SP_TELECOM = "telecom"; 2696 /** 2697 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2698 * <p> 2699 * Description: <b>The value in any kind of contact</b><br> 2700 * Type: <b>token</b><br> 2701 * Path: <b>PractitionerRole.telecom</b><br> 2702 * </p> 2703 */ 2704 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2705 SP_TELECOM); 2706 2707 /** 2708 * Search parameter: <b>location</b> 2709 * <p> 2710 * Description: <b>One of the locations at which this practitioner provides 2711 * care</b><br> 2712 * Type: <b>reference</b><br> 2713 * Path: <b>PractitionerRole.location</b><br> 2714 * </p> 2715 */ 2716 @SearchParamDefinition(name = "location", path = "PractitionerRole.location", description = "One of the locations at which this practitioner provides care", type = "reference", target = { 2717 Location.class }) 2718 public static final String SP_LOCATION = "location"; 2719 /** 2720 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2721 * <p> 2722 * Description: <b>One of the locations at which this practitioner provides 2723 * care</b><br> 2724 * Type: <b>reference</b><br> 2725 * Path: <b>PractitionerRole.location</b><br> 2726 * </p> 2727 */ 2728 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2729 SP_LOCATION); 2730 2731 /** 2732 * Constant for fluent queries to be used to add include statements. Specifies 2733 * the path value of "<b>PractitionerRole:location</b>". 2734 */ 2735 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 2736 "PractitionerRole:location").toLocked(); 2737 2738 /** 2739 * Search parameter: <b>email</b> 2740 * <p> 2741 * Description: <b>A value in an email contact</b><br> 2742 * Type: <b>token</b><br> 2743 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2744 * </p> 2745 */ 2746 @SearchParamDefinition(name = "email", path = "PractitionerRole.telecom.where(system='email')", description = "A value in an email contact", type = "token") 2747 public static final String SP_EMAIL = "email"; 2748 /** 2749 * <b>Fluent Client</b> search parameter constant for <b>email</b> 2750 * <p> 2751 * Description: <b>A value in an email contact</b><br> 2752 * Type: <b>token</b><br> 2753 * Path: <b>PractitionerRole.telecom(system=email)</b><br> 2754 * </p> 2755 */ 2756 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMAIL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2757 SP_EMAIL); 2758 2759}